数据结构与算法 Data Structures and Algorithms
coderwhy typescript数据结构与算法

coderwhy typescript数据结构与算法Title: TypeScript Data Structures and Algorithms: An In-depth ExplorationIntroduction:TypeScript, a superset of JavaScript, offers a strong type system and supports advanced features such as static typing, interfaces, and generics. With these features, TypeScript can be a powerful tool for implementing data structures and algorithms. In this article, we will dive deep into TypeScript's capabilities for building robust and efficient data structures and algorithms. We will cover various key data structures like arrays, linked lists, stacks, queues, trees, graphs, and hash tables, along with their associated algorithms.Table of Contents:1. Overview of Data Structures and Algorithms2. Arrays and Their Operations3. Linked Lists and Their Operations4. Stacks and Their Operations5. Queues and Their Operations6. Trees and Their Operations7. Graphs and Their Operations8. Hash Tables and Their Operations9. Sorting Algorithms10. Searching Algorithms11. Complexity Analysis12. Conclusion1. Overview of Data Structures and Algorithms:Start by explaining the concept of data structures and algorithms and their importance in programming. Discuss the role of data structures in organizing data efficiently and algorithms in solving various computational problems. Highlight the importance of understanding the time and space complexities of data structures and algorithms.2. Arrays and Their Operations:Explain what arrays are and how they work. Discuss common operations such as accessing elements, inserting and deleting elements, and searching for elements. Provide TypeScript code examples illustrating these operations.3. Linked Lists and Their Operations:Introduce linked lists and compare them to arrays. Explain the concept of nodes and pointers. Discuss common operations such as insertion, deletion, searching, and traversal in linked lists. Present TypeScript code examples to demonstrate these operations.4. Stacks and Their Operations:Define stacks as an abstract data type. Explain the concept of LIFO (Last-In, First-Out) ordering. Discuss common operations such as push, pop, top, and isEmpty. Provide TypeScript code examples for stack operations.5. Queues and Their Operations:Introduce queues as another abstract data type. Explain the concept of FIFO (First-In, First-Out) ordering. Discuss common operations such as enqueue, dequeue, front, and rear. Present TypeScript code examples for queue operations.6. Trees and Their Operations:Discuss the fundamental concepts of trees, nodes, and edges. Introduce different types of trees such as binary trees, binary search trees, and AVL trees. Explain common operations likeinsertion, deletion, traversal, and searching in trees. Provide TypeScript code examples to illustrate these operations.7. Graphs and Their Operations:Explain the concept of graphs and their representation using adjacency lists or matrices. Discuss different types of graphs, including directed and undirected graphs. Present common operations such as traversal, searching, and shortest path algorithms. Provide TypeScript code examples for graph operations.8. Hash Tables and Their Operations:Introduce hash tables as a way to store data using key-value pairs. Explain the concept of a hash function and collision resolution techniques. Discuss common operations such as insert, retrieve, delete, and resizing. Provide TypeScript code examples for hash table operations.9. Sorting Algorithms:Discuss various sorting algorithms such as bubble sort, selection sort, insertion sort, merge sort, quick sort, and heap sort. Compare their time and space complexities, and explain when to use eachalgorithm. Provide TypeScript code examples for sorting algorithms.10. Searching Algorithms:Explain common searching algorithms like linear search, binary search, and interpolation search. Discuss their time complexities and scenarios where each algorithm is applicable. Provide TypeScript code examples for searching algorithms.11. Complexity Analysis:Discuss time and space complexity analysis and their importance in evaluating the efficiency of algorithms. Explain Big O notation and its significance in representing algorithmic complexities. Provide examples and discuss best and worst-case scenarios.12. Conclusion:Summarize the key points discussed in the article, emphasizing the importance of understanding data structures and algorithms for efficient programming. Encourage readers to explore TypeScript's capabilities further and apply their knowledge in real-world applications.By following this step-by-step approach, the article will provide readers with a comprehensive understanding of TypeScript's data structures and algorithms. Each section will build upon the previous one, guiding readers through the fundamental concepts, operations, and code examples. In the end, readers will have a solid foundation in TypeScript's capabilities for implementing efficient and scalable data structures and algorithms.。
算法和数据结构有什么区别?

算法(Algorithm)和数据结构(Data Structure)是计算机科学中两个关键概念,常常在设计和实现计算机程序时同时考虑。
它们的主要区别如下:
1. 定义和目的:算法是一种解决问题的方法或步骤的有序集合,描述了在给定输入的情况下如何产生所需输出。
它强调解决问题的方法和步骤。
数据结构是组织和存储数据的方式,提供了存储、访问和操作数据的方法。
它关注于数据的组织和操作。
2. 侧重点:算法的重点在于如何解决问题,并考虑算法的效率、正确性和优化。
它涉及到选择合适的控制结构、操作符、变量等,以及算法的复杂度分析。
数据结构的重点在于如何有效地组织和管理数据,以便提高程序的效率和性能。
3. 相互关系:算法和数据结构之间存在密切的关系。
算法依赖于数据结构来组织和存储数据,而数据结构则为算法提供了合适的数据操作环境。
在设计算法时,需要选择合适的数据结构以支持算法的执行。
4. 应用范围:算法是通用的方法论,用于解决各种问题,如排序、搜索、图算法等。
数据结构是一种具体的实现,用于
组织和操作数据。
它包括数组、链表、栈、队列、树、图等常用的数据结构。
总而言之,算法和数据结构是计算机科学中两个重要的概念,它们相互依赖、相互关联。
算法是问题解决的方法和步骤,而数据结构是组织和管理数据的方式。
在实际编程中,算法和数据结构常常一起考虑,以提高程序的效率和性能。
《数据结构与算法分析》课程教学大纲

本科生课程大纲课程属性:公共基础/通识教育/学科基础/专业知识/工作技能,课程性质:必修、选修一、课程介绍1.课程描述:数据结构与算法分析是学习利用计算机语言编写质量更好的程序以及软件的一门课程,是提高计算机编程水平的必由之路,为日后学习相关课程打下一个坚实的基础。
本课程针对低年级地球信息科学与技术专业和勘查技术与工程专业本科生学生开设,课程主要内容包括:数据结构及其算法,文件读写,查找和排序算法等。
通过课程学习,要求学生能够掌握计算机存储(包括内存和外存)数据的基本方法和常用模式以及其算法,提高编写程序、调试程序的能力,课程结束后能够完成较复杂程序的设计和编制。
2.设计思路:本课程引导低年级地球信息科学与技术专业和勘查技术与工程专业学生掌握利用计算机语言编写实用可靠的程序的基础理论和实际操作方法,提升自身的科研和工作技能。
课程内容的选取基于学生掌握了一定的计算机语言知识。
课程内容分为四个模块:数据结构介绍;常用的数据结构及其算法;文件读写;查找和排序算法。
这三个方面相互关联,互为补充,覆盖了计算机数据存储、管理和处理等的主要模式和方法。
3. 课程与其他课程的关系:- 1 -本课程需要本科生在完成低年级阶段的计算机语言的基础上开设。
先修课程:《C 程序设计》。
二、课程目标本课程目标是为低年级地球信息科学与技术专业和勘查技术与工程专业学生提供一个深入学习计算机编程的平台,引导并培养学生使用计算机语言来描述、管理和处理数据的能力,提高计算机编程水平。
到课程结束时,学生应能:(1)熟练掌握常用的计算机数据在内存中存储的方法及其常用算法;(2)掌握文件的读写操作,合理的利用文件存储数据;(3)掌握查找和排序常用的算法;(4)掌握如何编制可靠的程序以及程序调试的技巧。
三、学习要求要完成所有的课程任务,学生必须:(1)按时上课,上课认真听讲,积极参与课堂讨论。
(2)按时完成上机练习,对地质数据进行分析和处理,提交正式的上机报告。
数据结构经典书籍
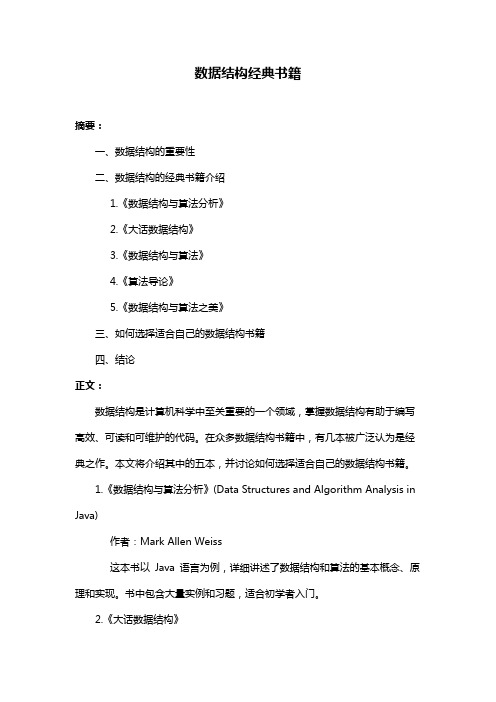
数据结构经典书籍摘要:一、数据结构的重要性二、数据结构的经典书籍介绍1.《数据结构与算法分析》2.《大话数据结构》3.《数据结构与算法》4.《算法导论》5.《数据结构与算法之美》三、如何选择适合自己的数据结构书籍四、结论正文:数据结构是计算机科学中至关重要的一个领域,掌握数据结构有助于编写高效、可读和可维护的代码。
在众多数据结构书籍中,有几本被广泛认为是经典之作。
本文将介绍其中的五本,并讨论如何选择适合自己的数据结构书籍。
1.《数据结构与算法分析》(Data Structures and Algorithm Analysis in Java)作者:Mark Allen Weiss这本书以Java 语言为例,详细讲述了数据结构和算法的基本概念、原理和实现。
书中包含大量实例和习题,适合初学者入门。
2.《大话数据结构》作者:程云本书采用轻松幽默的语言和丰富的图解,讲解了数据结构的基本原理和常用算法。
内容通俗易懂,适合编程初学者。
3.《数据结构与算法》(Data Structures and Algorithms)作者:Alfred V.Aho, John E.Hopcroft, and Jeffrey D.Ullman这本书是数据结构和算法的经典教材,详细介绍了各种数据结构及其操作,以及排序、查找等基本算法。
内容较为深入,适合已经掌握基本编程技能的读者。
4.《算法导论》(Introduction to Algorithms)作者:Thomas H.Cormen, Charles E.Leiserson, Ronald L.Rivest, and Clifford Stein本书全面讲述了算法设计与分析的基本概念,涵盖了许多经典算法和数据结构。
书中包含大量实例和习题,适合对算法有一定了解的读者深入学习。
5.《数据结构与算法之美》(The Art of Computer Programming, Volume 1: Fundamental Algorithms)作者:Donald E.Knuth本书是计算机编程艺术的卷一,讲述了计算机科学的基本算法。
数据结构单词译本

Greedy approach
贪心算法
singly-linked list
单链表
node
结点
Traversal
遍历
Memory allocation
内存分配
doubly-linked list
双向链表
circular linked list
循环链表
polynomial
多项式
stack
栈
top
栈顶
Last-In-First-Out (LIFO)
后进先出
PUSH
进栈
POP
出栈
queue
队列
First-In-First-Out(FIFO)
先进先出
EnQueue(x)
在队尾中插入元素
Dequeue()
从队列中删除队头元素
The queue in the array is treated as if they are arranged in a ring
普里姆算法求最小代价生成树
void Kruskal();
克鲁斯卡尔算法求最小代价生成树
void Dijkstra(int v,T *d,int *p);
迪杰斯特拉算法求单源最短路经
Adjacency Matrix
邻接矩阵
Adjacency List
邻接表
adjacent
相邻的
unvisited
没有访问的
生成子图
Spanning Tree
生成树
void DFS();Depth First Search
深度优先搜索图
void BFS();Breadth First Search
数据结构与算法分析C++版英文版第二版课程设计

Course Design of Data Structures and AlgorithmAnalysis C++ Version (2nd Edition) ObjectiveThe primary objective of this course design is to reinforce the understanding of data structures and algorithms through implementationin C++ programming language. The course also ms to familiarize students with the usage of various C++ libraries while designing and implementing algorithms. By the end of this course design, students should be able to: •Understand the characteristics and properties of basic data structures such as arrays, stacks, queues, trees, graphs, andsearching/sorting algorithms.•Analyze the efficiency and complexity of algorithms using big O notation•Design and implement data structures and algorithms using C++ programming language, including OOP concepts such asinheritance and polymorphism.•Solve real-world problems using data structures and algorithms.SynopsisThe course design focuses on the following topics:1.Introduction to Data Structures and Algorithm Analysis2.Arrays and Vectors3.Linked Lists4.Stacks and Queues5.Trees6.Graphs7.Searching8.Sorting9.Hashing10.Binary Heaps and Priority Queues11.Heapsort12.Balanced Search Trees13.Advanced TopicsThe course design emphasizes practical implementation, therefore, each topic is accompanied by coding exercises using C++ programming language. Additionally, students are required to implement one major project in a team of two or three, which involves the usage of data structures and algorithms studied in class to solve a real-world problem.GradingThe final grade for this course design will be based on thefollowing criteria:•30%: Programming assignments•30%: Final project•20%: Mid-term exam•20%: Final examPrerequisitesIt is expected that students have a good understanding of programming concepts and basic data structures such as arrays, linked lists, and stacks/queues. Additionally, knowledge of object-oriented programming (OOP) concepts such as inheritance, polymorphism, and encapsulation is required.Course MaterialsThe primary course material will be the textbook。
数据结构与算法 (2)
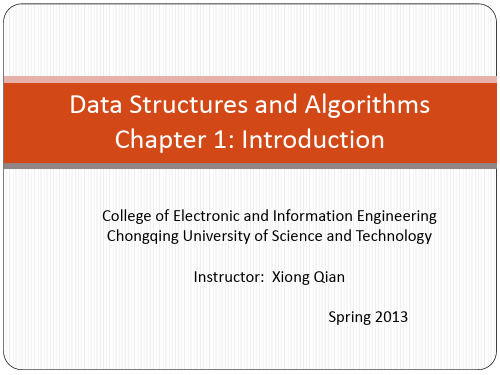
First started programming : have no ADT
–Writing the same code over and over
Data Sagtrauincture can be defined as:Exa•mAtpolemfiocr DADatTa: tahreecsoidneglteo arenadd the 1ea.Ak♠stcheWehcayAtebotoon•adohcmkAfAdwsoaafoanwtebrntwndinoadoatc-ihwha,ndmaiblsttatfiyetbauewcaihirspcstncahtheipaeotcdotaordeintimooaotniscforadnpcanofpnatoeotentaosdtprhidfriostmasaaetaheiatmystcahetlibopatokeidisrtlpcaenymedtatpogyeswedieepnesrdntaonanedhcadtttthaaapiiitesttsoneotayafai.ngaardndntresoseaeddt,,dthaaaaet.ar
bank toopdeertaetrimoninse tellers.
1-3 Model for an Abstract Data Type
In this section we provide a conceptual model for an Abstract Data Type (ADT).
t2rienyel.eApvwl–l♠adb3aiasqmoAene2raittsuehlbit7tevoeeo1ewa––s6{s•idauniut6rxdnFAt+Aens8mT123rneitetaboesuoasra,eegsn~s...th:r-mtinncefmhnanp,tti3DEDtaon*stcatoatyaeioipci,2ihtotpntgfeectc/ncisihotls,o…o7nnmeceuasccohiisienlfdmnn:6mtailliil(pneeaaeoscos}sgasg7ppruikrdhegrra:ioecfcattf}ssraaelaurhr,enadiaomintuiCcshmdudmttaalitsetrsaiipdagtleauooaatdtcfyabfrl:opncieetoieoiirannnnbtsaptvootatlioadmarrhaefuilgntte-ieotonnmoozeambinstnfrette.pntfhaisffheyhuetediwobdesto{tenrapdeo)eyoim-lenoneouasdgnbeapptfdtinfohricbamistotte.riedtainhahapaotteneotr.ipauthtkeaoetiagfrkathTltserndesretoaedlaeiahtsmniroapnyna.te,taenitepeatosanriasneusannod.t.ctfatsiohoatinoasnssa
动画演示数据结构与算法

动画演示数据结构与算法## English Response.### Introduction.Data structures and algorithms are fundamental concepts in computer science. They provide the building blocks for organizing and manipulating data efficiently. Visualizing these concepts through animations can greatly enhance understanding and retention. This article presents a comprehensive guide to animated demonstrations of data structures and algorithms.### Types of Data Structures.Arrays: A linear collection of elements that can be accessed randomly.Linked Lists: A linear collection of elements that are connected by pointers.Stacks: A last-in, first-out (LIFO) data structure that follows the principle of a stack of plates.Queues: A first-in, first-out (FIFO) data structurethat follows the principle of a queue of people waiting in line.Trees: A hierarchical data structure that organizes elements in a parent-child relationship.Hash Tables: A data structure that maps keys to values, enabling efficient retrieval based on key lookup.Graphs: A data structure that represents a network of nodes connected by edges, often used for representing relationships.### Types of Algorithms.Sorting Algorithms: Algorithms that arrange elements in a specific order, such as ascending or descending.Searching Algorithms: Algorithms that find an element within a data structure.Tree Traversal Algorithms: Algorithms that visit nodes in a tree in a specific order.Graph Traversal Algorithms: Algorithms that visit nodes in a graph in a specific order.Hashing Algorithms: Algorithms that map keys to values in a hash table.Dynamic Programming Algorithms: Algorithms that solve complex problems by breaking them down into smaller subproblems and storing the solutions.Greedy Algorithms: Algorithms that make locally optimal choices at each step, aiming for a globally optimal solution.### Animation Tools and Resources.Visualgo: An online platform that provides interactive visualizations of data structures and algorithms.Khan Academy: A non-profit educational organizationthat offers animated videos explaining data structures and algorithms.Coursera: An online learning platform that offers courses on data structures and algorithms, many of which include animated demonstrations.edX: Another online learning platform that offers courses on data structures and algorithms with animated content.YouTube: A vast repository of videos, including many animated demonstrations of data structures and algorithms.### Benefits of Animation.Enhanced Understanding: Visualizing data structures andalgorithms in motion allows students to grasp their behavior more intuitively.Increased Retention: Animated demonstrations can create a lasting impression, making it easier for students to remember and apply the concepts.Improved Problem-Solving Skills: By witnessing thestep-by-step execution of algorithms, students can develop stronger problem-solving abilities.Engaging Learning Experience: Animations add an element of interactivity and engagement, making the learning process more enjoyable.Foundation for Real-World Applications: Understanding data structures and algorithms is essential for building efficient and effective software applications.### Conclusion.Animated demonstrations of data structures andalgorithms are a powerful tool for enhancing understanding, retention, and problem-solving skills. By utilizing the resources available online, educators and students can leverage these visualizations to make learning more effective and engaging.## 中文回答:### 介绍。
DS and Algorithm(数据结构)
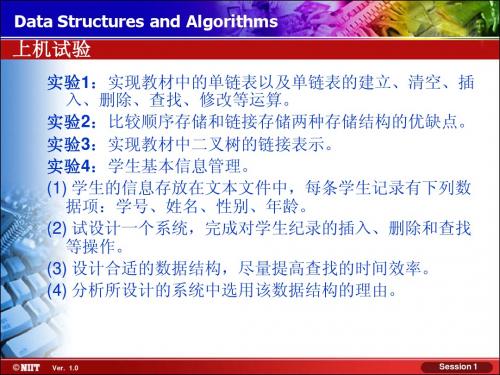
Data Structures and Algorithms
Role of Data Structures (Contd.) Data structure is defined as a way of organizing the various data elements in memory with respect to each other Data can be organized in many different ways. Therefore, you can create as many data structures as you want. Some data structures that have proved useful over the years are: – Arrays – Linked Lists – Stacks – Queues – Trees – Graphs
Ver. 1.0
Session 1
Data Structures and Algorithms
数据的存储表示
1.顺序 顺序 2.链接 链接 3.索引 索引 4.散列 散列
顺序和链接是两种最基本的存储表示方法。 顺序和链接是两种最基本的存储表示方法。
Ver. 1.0
Session 1
Data Structures and Algorithms
Ver. 1.0
Session 1
Data Structures and Algorithms
Objectives
In this session, you will learn to:
– Explain the role of data structures and algorithms in problem solving through computers – Identify techniques to design algorithms and measure their efficiency
计算机系统基础 英语
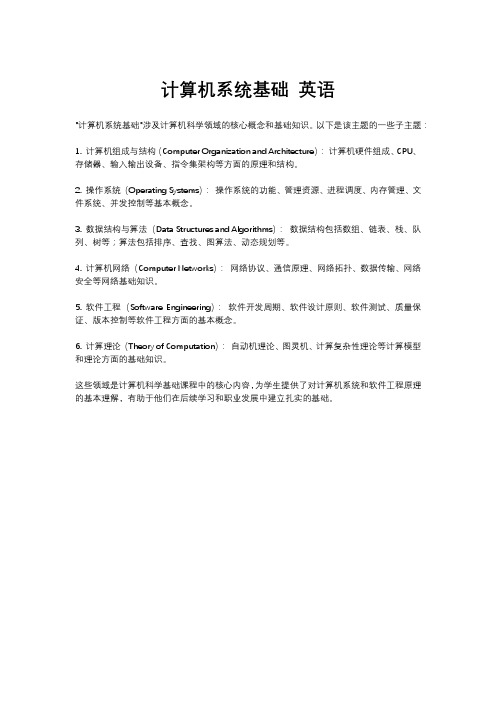
计算机系统基础英语
"计算机系统基础"涉及计算机科学领域的核心概念和基础知识。
以下是该主题的一些子主题:
1. 计算机组成与结构(Computer Organization and Architecture):计算机硬件组成、CPU、存储器、输入输出设备、指令集架构等方面的原理和结构。
2. 操作系统(Operating Systems):操作系统的功能、管理资源、进程调度、内存管理、文件系统、并发控制等基本概念。
3. 数据结构与算法(Data Structures and Algorithms):数据结构包括数组、链表、栈、队列、树等;算法包括排序、查找、图算法、动态规划等。
4. 计算机网络(Computer Networks):网络协议、通信原理、网络拓扑、数据传输、网络安全等网络基础知识。
5. 软件工程(Software Engineering):软件开发周期、软件设计原则、软件测试、质量保证、版本控制等软件工程方面的基本概念。
6. 计算理论(Theory of Computation):自动机理论、图灵机、计算复杂性理论等计算模型和理论方面的基础知识。
这些领域是计算机科学基础课程中的核心内容,为学生提供了对计算机系统和软件工程原理的基本理解,有助于他们在后续学习和职业发展中建立扎实的基础。
DSA名词解释

DSA名词解释DSA是数据结构与算法的缩写,全称为Data Structures and Algorithms。
它是计算机科学中非常重要的一门学科,主要涉及如何存储和组织数据以及如何设计和分析算法。
数据结构(Data Structures)是指一组数据的组织方式,它关注的是如何在计算机中高效地存储和访问数据。
常见的数据结构包括数组、链表、栈、队列、树、图等。
不同的数据结构适用于不同的应用场景,在选择和使用数据结构时需要考虑数据的特点和操作的需求。
算法(Algorithms)是指一组解决特定问题的指令集合,它关注的是如何通过一系列的步骤来操作数据以达到预定的目标。
算法可以用来解决搜索、排序、计算等多种问题,一个好的算法能够提高程序的效率和性能。
设计和分析算法要考虑时间复杂度、空间复杂度、正确性和可读性等因素。
DSA的学习旨在让学生掌握数据结构和算法的基本概念、理论知识和实际应用技巧。
通过学习DSA可以提高算法思维和问题解决能力,提升程序的效率和质量。
DSA在软件工程、系统设计、机器学习等领域都有广泛的应用。
DSA的学习内容包括但不限于以下几个方面:1. 数据结构的基本概念和分类:了解各种数据结构的特点、优劣和适用场景,如数组是一种顺序存储结构、链表是一种链式存储结构等。
2. 常见数据结构的实现和操作:学习如何用编程语言实现各种数据结构,并掌握它们的基本操作,如插入、删除、查找等。
3. 常用算法的设计和分析:学习各种算法的设计思想和实现方法,如递归、分治、贪心、动态规划等,同时了解算法的时间复杂度和空间复杂度的分析方法。
4. 经典算法和数据结构的应用:学习经典算法和数据结构的实际应用,如搜索算法、排序算法、图算法等,同时了解它们在实际问题中的应用和优化技巧。
总之,DSA是计算机科学中一门重要的学科,它涉及到数据结构的存储和组织方式以及算法的设计和分析方法。
掌握DSA的知识可以提高程序的效率和质量,培养算法思维和问题解决能力。
dsa的ccf级别

dsa的ccf级别
DSA(Data Structures and Algorithms)是指数据结构与算法,是计算机科学中非常重要的基础知识。
而CCF(中国计算机学会)
是中国的一个学术性、公益性、全国性的学术团体。
CCF对期刊和
会议进行了分级,以评价其学术水平和影响力。
关于DSA的CCF级别,一般来说,与DSA相关的期刊和会议会被CCF评为不同的级别,具体的级别取决于该期刊或会议的学术水平、影响力和质量。
一般来说,与DSA相关的期刊和会议会被CCF评为A、B、C三
个级别。
A类期刊或会议在相关领域具有较高的学术地位和影响力,发表在这类期刊或会议上的论文往往被认为是高质量的;B类期刊
或会议在相关领域有一定的学术地位和影响力,发表在这类期刊或
会议上的论文也具有一定的学术水平;C类期刊或会议在相关领域
的学术地位和影响力相对较低。
这些级别的评定是由CCF根据一定
的评审标准和程序进行评定的。
总的来说,DSA的CCF级别取决于与之相关的期刊和会议的学
术水平和影响力,而这些级别的评定是由CCF进行的。
因此,如果
想了解具体某个期刊或会议的CCF级别,需要查询CCF的官方发布
的评定结果或相关权威信息渠道的评价。
希望这个回答能够帮到你。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
高级数据结构和算法分析Advanced Data Structures and Algorithm Analysis
主讲教师:陈越
Instructor: CHEN, YUE
E-mail: chenyue@ Courseware and homework sets can be downloaded from /dsaa/
教材(Text Book)
Data Structures and
Algorithm Analysis in C
(2nd Edition)
Mark Allen Weiss
陈越改编
Email: weiss@
参考书目(Reference)
数据结构与算法分析(C语言版)魏宝刚、陈越、王申康编著浙江大学出版社 Data Structures, Algorithms, and Applications in C++
数据结构算法与应用——C++语言描述(英文版)
Sartaj Sahni McGraw-Hill & 机械工业出版社 数据结构课程设计
何钦铭、冯雁、陈越著浙江大学出版社
课程评分方法(Grading Policies)Research Project (23 or 25)Discussions
(14)
Homework (5)Q&A (0.5 each)Total 45
Final Exam (55)
Discussions(14)
Form groups of 3 or 4
28 in-class discussion topics
Each takes 3~5 minutes
14 = 28 10 / 20
Research topics (23 or 25)
◆Done in groups
◆16 topics to choose from
◆Report (18 or 20 points)
◆In-class presentation (5~10 minutes, 5 points)
◆The speaker will be chosen randomly from all the
contributors
◆If there are many volunteers, only one group will
be chosen
◆If there is no volunteer, I will talk about it
Homework (5)
✓Done independently
✓10 problems
✓Collected before the end of the next class meeting ✓ 5 = 10 10 / 20
✓Late penalty: 2 points/week
Q&A
⏹For volunteers only
⏹0.5 point for each question asked/answered ⏹come and claim your credits after each class
session。