实验三:多态性答案—专业版
C++多态性实验报告含代码和结果截图

C++多态性实验报告含代码和结果截图实验报告课程:面向对象技术学号:姓名:班级:教师:计算机科学与技术系实验六多态性一、实验目的及要求1.掌握运算符重载的方法;2.掌握使用虚函数实现动态多态性。
二、实验环境硬件:计算机软件:Microsoft Visual C++三、实验内容声明一个车(vehicle)基类,有Run、Stop等成员函数,由此派生出自行车(bicycle)类、汽车(motorcar)类,从bicycle和motorcar派生出摩托车(motorcycle)类,它们都有Run、Stop等成员函数。
观察虚函数的作用。
四、实验结果(附截图)五、总结通过本次实验,我对虚函数、多态性有了进一步了解,对多态性也有了更深的认识,实验中还是有很多的问题不是很清楚,平时要认真学习好理论知识,这样才能在做实验时更好的理解代码,才能更快的改正自己调试时遇到的错误。
六、附录(源程序清单)#includeusing namespace std;int sign=0;class vehicle{vehicle(float m,float w){if(m<240&&m>0)MaxSpeed=m;else{cout<<"汽车超速!"<<endl;< p="">sign=1;return;}if(w<500&&w>0)Weight=w;else{cout<<"汽车超重!"<<endl;< p="">sign=1;return;}cout<<"构造了一个vehicle对象"<<endl;< p="">}virtual void Run() { cout<<"vehicle Run 函数被调用"<<endl;}< p="">virtual void Stop(){ cout<<"vehicle Stop 函数被调用"<<endl<<="">float MaxSpeed;float Weight;}class bicycle:virtual public vehicle{public:bicycle(float h,float m,float w):vehicle(m,w){if(h<1.5&&h>0)Height=h;elsecout<<"自行车超高!"<<endl;< p="">sign=1;return;}cout<<"构造了一个bicycle对象"<<endl;< p="">}void Run() { cout<<"bicycle Run 函数被调用"<<endl;}< p=""> void Stop(){ cout<<"bicycle Stop 函数被调用"<<endl<<endl;}< p="">private:float Height;}class motorcar:virtual public vehicle{public:motorcar(float s,float m,float w):vehicle(m,w){if(s<2&&s>0)SeatNum=s;else{cout<<"摩托车超载!"<<endl;< p="">sign=1;return;}cout<<"构造了一个motorcar对象"<<endl;< p="">}void Run() { cout<<"motorcar Run 函数被调用"<<endl;}< p="">void Stop(){ cout<<"motorcar Stop 函数被调用"<<endl<<endl;}< p="">private:float SeatNum;}class motorcycle:public bicycle,public motorcar{public:motorcycle(float h,float s,float m,float w):bicycle(h,m,w),motorcar(s,m,w),vehi cle(m,w){if(sign==0){cout<<"构造了一个motorcycle对象"<<endl;< p="">}}void Run() { cout<<"motorcycle Run 函数被调用"<<endl;}< p="">void Stop(){ cout<<"motorcycle Stop 函数被调用"<<endl<<endl;}< p="">};void main (){float m,w,h,s;int p;do{sign=0;cout<<"请输入参数:"<<endl<<endl;< p="">cout<<"汽车最高时速(km/h)";cin>>m;cout<<"汽车重量(t)";cin>>w;cout<<"自行车高度(m)";cin>>h;cout<<"摩托车座位(个)";cin>>s;motorcycle car(h,s,m,w);if(sign==0){car.Run();car.Stop();}else{cout<<"1—重新输入2——结束程序";cin>>p;if(p==2)return;elsecout<<endl<<endl;< p=""> }}while(sign==1);}</endl<<endl;<></endl<<endl;<></endl<<endl;}<></endl;}<></endl;<></endl<<endl;}<></endl;}<></endl;<></endl;<></endl<<endl;}<></endl;}<></endl;<></endl;<></endl<</endl;}<></endl;<></endl;<></endl;<>。
C++实验 多态性

C++程序设计实验名称:多态性专业班级:计科1402学号:2014006935学生姓名:陈志棚指导教师:王丽娟2016年5月8日一、实验目的:1、掌握C++中运算符重载的机制和运算符重载的方式;2、理解类型转换的必要性,掌握类型转换的使用方法;3、理解多态性,掌握虚函数的设计方法;4、学习使用visual studio 调试虚函数;二、案例:某小型公司,主要有三类人员:管理人员、计时人员和计件人员。
现在,需要存储这些人的姓名、编号、时薪、工时、每件工件薪金、工件数,计算月薪并显示全部信息。
三:程序及其运行结果:#include <iostream>#include <cstring>using namespace std;class Employee{ //雇员类private:int number;//编号string name;//姓名public:Employee(int nu,string na);int getnumber();string getname();virtual double earnings()=0;//计算工资virtual void display();//显示员工信息};class Manager:public Employee{ //管理员类private:double monthlySalary;//月薪public:Manager(int nu,string na,double ms=0.0);void setMonthlySalary(double sms);//置月薪virtual double earnings();//计算月薪virtual void display();//显示所有信息};class HourlyWorker:public Employee{private:double wage;//时薪int hours;//工时public:HourlyWorker(int nu,string na,double w=0,int h=0);void setWage(double w);//置时薪void setHours(int h);//置工时virtual double earnings();//计算月薪virtual void display();//显示所有信息};class PieceWorker:public Employee{//计件人员类private:double wagePerPiece;//每件工件薪金int quantity;//工件数public:PieceWorker(int nu,string na,double wp=0.0,int q=0);void setWagePerPiece(double wp);//置每件工件薪金void setQuantity(int q);//置工件数virtual double earnings();//计算月薪virtual void display();//显示所有信息};int main(){Manager m1(101,"Chen Xiao",10000);Manager m2(102,"Chen YanXi");m2.setMonthlySalary(12000);HourlyWorker hw1(201,"Zhou Rong",10,100);HourlyWorker hw2(202,"Yang Zi");hw2.setWage(8);hw2.setHours(120);PieceWorker pw1(301,"Huo JianHua",1.5,1000);PieceWorker pw2(302,"Zhao LiYin");pw2.setWagePerPiece(1.8);pw2.setQuantity(800);Employee *p;p=&m1;p->display();p=&m2;p->display();p=&hw1;p->display();p=&hw2;p->display();p=&pw1;p->display();p=&pw2;p->display();return 0;}//****************************************雇员类中函数定义Employee::Employee(int nu,string na){number=nu;name=na;}int Employee::getnumber(){return number;}string Employee::getname(){return name;}void Employee::display(){cout<<"编号:"<<number<<" 姓名:"<<name<<endl;}//****************************************管理员类中函数定义Manager::Manager(int nu,string na,double ms):Employee(nu,na){monthlySalary=ms;}void Manager::setMonthlySalary(double sms){monthlySalary=sms;}double Manager::earnings(){return monthlySalary;}void Manager::display(){cout<<"编号:"<<Employee::getnumber()<<" 姓名:"<<Employee::getname()<<" 月薪:"<<monthlySalary<<endl;}//*****************************************************计时人员类中函数定义HourlyWorker::HourlyWorker(int nu,string na,double w,int h):Employee(nu,na){wage=w;hours=h;}void HourlyWorker::setWage(double w){wage=w;}void HourlyWorker::setHours(int h){hours=h;}double HourlyWorker::earnings(){return wage*hours;}void HourlyWorker::display(){cout<<"编号:"<<Employee::getnumber()<<" 姓名:"<<Employee::getname()<<" 时薪:"<<wage<<" 工时:"<<hours<<" 月薪:"<<HourlyWorker::earnings()<<endl; }//*****************************************************计件人员类中函数定义PieceWorker::PieceWorker(int nu,string na,double wp,int q):Employee(nu,na){wagePerPiece=wp;quantity=q;}void PieceWorker::setWagePerPiece(double wp){wagePerPiece=wp;}void PieceWorker::setQuantity(int q){quantity=q;}double PieceWorker::earnings(){return wagePerPiece*quantity;}void PieceWorker::display(){cout<<"编号:"<<Employee::getnumber()<<" 姓名:"<<Employee::getname()<<" 每件工件薪金:"<<wagePerPiece<<" 工件数:"<<quantity<<" 月薪:"<<PieceWorker::earnings()<<endl;}运行结果:四:分析与总结:1、通过该程序让我更加了解C++的多态性,熟悉虚函数的定义和使用;2、进一步熟悉函数的继承以及继承的特点!。
多态性实验报告

多态性实验报告一、实验目的本次实验的主要目的是深入研究和理解多态性这一重要的概念,并通过实际操作和观察来验证多态性在不同情境下的表现和作用。
二、实验原理多态性是面向对象编程中的一个关键特性,它允许不同的对象对相同的消息或操作做出不同的响应。
这种特性基于类的继承和方法的重写机制。
当一个父类的引用指向子类对象时,通过该引用调用方法时,实际执行的是子类中重写的方法,从而实现了多态性。
三、实验材料与环境1、编程语言:选择了 Java 作为实验的编程语言。
2、开发工具:使用了 IntelliJ IDEA 作为集成开发环境。
3、实验设备:一台配置良好的计算机,操作系统为 Windows 10。
四、实验步骤1、创建父类`Shape````javaclass Shape {public void draw(){Systemoutprintln("Drawing a shape");}}```2、创建子类`Circle` 和`Square` 继承自`Shape````javaclass Circle extends Shape {@Overridepublic void draw(){Systemoutprintln("Drawing a circle");}}class Square extends Shape {@Overridepublic void draw(){Systemoutprintln("Drawing a square");}}```3、在主函数中进行测试```javapublic class PolymorphismTest {public static void main(String args) {Shape shape1 = new Circle();Shape shape2 = new Square();shape1draw();shape2draw();}}```五、实验结果运行上述代码,输出结果为:```Drawing a circleDrawing a square```这表明,通过父类的引用调用`draw` 方法时,实际执行的是子类中重写的方法,实现了多态性。
实验报告多态性
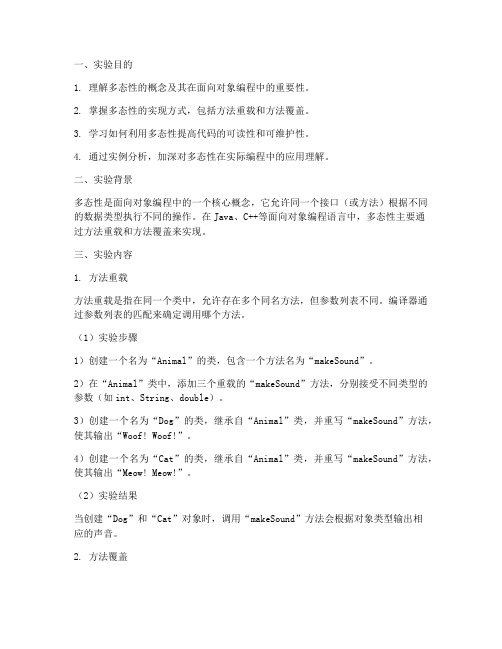
一、实验目的1. 理解多态性的概念及其在面向对象编程中的重要性。
2. 掌握多态性的实现方式,包括方法重载和方法覆盖。
3. 学习如何利用多态性提高代码的可读性和可维护性。
4. 通过实例分析,加深对多态性在实际编程中的应用理解。
二、实验背景多态性是面向对象编程中的一个核心概念,它允许同一个接口(或方法)根据不同的数据类型执行不同的操作。
在Java、C++等面向对象编程语言中,多态性主要通过方法重载和方法覆盖来实现。
三、实验内容1. 方法重载方法重载是指在同一个类中,允许存在多个同名方法,但参数列表不同。
编译器通过参数列表的匹配来确定调用哪个方法。
(1)实验步骤1)创建一个名为“Animal”的类,包含一个方法名为“makeSound”。
2)在“Animal”类中,添加三个重载的“makeSound”方法,分别接受不同类型的参数(如int、String、double)。
3)创建一个名为“Dog”的类,继承自“Animal”类,并重写“makeSound”方法,使其输出“Woof! Woof!”。
4)创建一个名为“Cat”的类,继承自“Animal”类,并重写“makeSound”方法,使其输出“Meow! Meow!”。
(2)实验结果当创建“Dog”和“Cat”对象时,调用“makeSound”方法会根据对象类型输出相应的声音。
2. 方法覆盖方法覆盖是指在子类中重写父类的方法,使子类的方法具有与父类方法相同的签名,但具有不同的实现。
(1)实验步骤1)创建一个名为“Vehicle”的类,包含一个方法名为“move”,该方法无参数。
2)创建一个名为“Car”的类,继承自“Vehicle”类,并重写“move”方法,使其输出“Car is moving”。
3)创建一个名为“Bike”的类,继承自“Vehicle”类,并重写“move”方法,使其输出“Bike is moving”。
(2)实验结果当创建“Car”和“Bike”对象时,调用“move”方法会根据对象类型输出相应的移动信息。
实验三 继承和多态
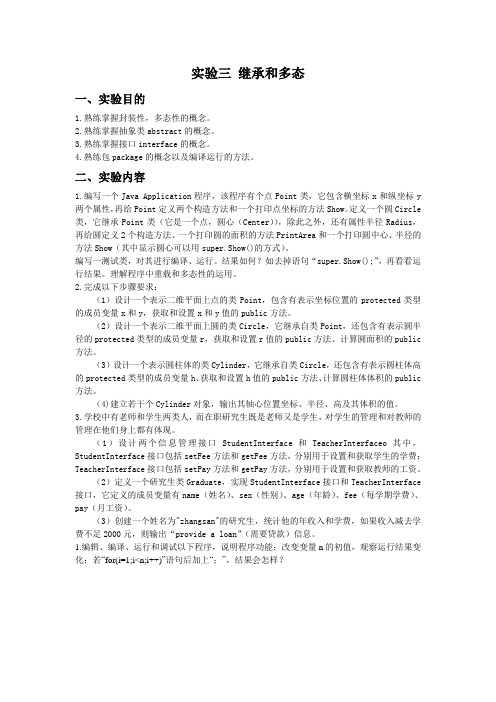
实验三继承和多态一、实验目的1.熟练掌握封装性,多态性的概念。
2.熟练掌握抽象类abstract的概念。
3.熟练掌握接口interface的概念。
4.熟练包package的概念以及编译运行的方法。
二、实验内容1.编写一个Java Application程序,该程序有个点Point类,它包含横坐标x和纵坐标y 两个属性,再给Point定义两个构造方法和一个打印点坐标的方法Show。
定义一个圆Circle 类,它继承Point类(它是一个点,圆心(Center)),除此之外,还有属性半径Radius,再给圆定义2个构造方法、一个打印圆的面积的方法PrintArea和一个打印圆中心、半径的方法Show(其中显示圆心可以用super.Show()的方式)。
编写一测试类,对其进行编译、运行。
结果如何?如去掉语句“super.Show();”,再看看运行结果。
理解程序中重载和多态性的运用。
2.完成以下步骤要求:(1)设计一个表示二维平面上点的类Point,包含有表示坐标位置的protected类型的成员变量x和y,获取和设置x和y值的public方法。
(2)设计一个表示二维平面上圆的类Circle,它继承自类Point,还包含有表示圆半径的protected类型的成员变量r,获取和设置r值的public方法、计算圆面积的public 方法。
(3)设计一个表示圆柱体的类Cylinder,它继承自类Circle,还包含有表示圆柱体高的protected类型的成员变量h、获取和设置h值的public方法、计算圆柱体体积的public 方法。
(4)建立若干个Cylinder对象,输出其轴心位置坐标、半径、高及其体积的值。
3.学校中有老师和学生两类人,而在职研究生既是老师又是学生,对学生的管理和对教师的管理在他们身上都有体现。
(1)设计两个信息管理接口StudentInterface和TeacherInterfaceo其中,StudentInterface接口包括setFee方法和getFee方法,分别用于设置和获取学生的学费;TeacherInterface接口包括setPay方法和getPay方法,分别用于设置和获取教师的工资。
湖北理工(黄石理工)C++实验 实验三多态性#精选
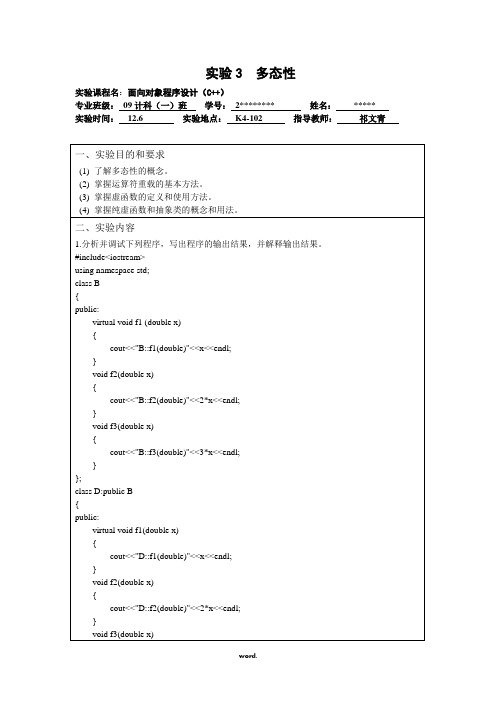
实验3 多态性实验课程名:面向对象程序设计(C++)专业班级:09计科(一)班学号:2******** 姓名:***** 实验时间:12.6 实验地点:K4-102 指导教师:祁文青{cout<<"D::f3(double)"<<3*x<<endl;}};int main(){D d;B*pb=&d;D*pd=&d;pb->f1(1.23);pb->f1(1.23);pb->f2(1.23);pb->f3(1.23);pb->f3(3.14);return 0;}程序的运行结果。
答:2.编写一个程序,其中设计一个时间类Time,用来保存时、分、秒等私有数据成员,通过重载操作符“+”实现两个时间的相加。
要求将小时范围限制在大于等于0,分钟范围限制在0~59,秒钟范围限制在0~59秒。
【提示】时间类Time{public:Time(int h=0,int m=0,int s=0);Time operator+(Time&);void disptime(string);private:int hourse;cout<<s<<hourse<<":"<<minutes<<":"<<seconds<<endl;}int main(){int hh,mm,ss;do{cout<<"输入第一个时间时分秒(例如2 30 42)"<<endl;cin>>hh>>mm>>ss;}while(hh<0||mm<0||mm>59||ss<0||ss>59);Time t(hh,mm,ss);do{cout<<"输入第二个时间时分秒(例如2 30 42)"<<endl;cin>>hh>>mm>>ss;}while(hh<0||mm<0||mm>59||ss<0||ss>59);Time T(hh,mm,ss),t_total;t_total=t+T;t_total.disptime("输出结果(时/分/秒):");return 0;}程序运行结果:3.给出下面的抽象基类container;class container{protected:double radius;public:container(double radius1);virtual double surface_area()=0;virtual double volume()=0;};要求建立3个继承container的派生类cube、sphere与cylinder,让每一个派生类都包含虚函数surface_area()和volume(),分别用来计算正方体、球体和圆柱体的表面积及体积。
实验三单核苷酸多态性的检测

单核苷酸多态性的检测原理
总结词
单核苷酸多态性的检测原理基于分子生物学技术,如DNA测序、PCR扩增和电泳分离 等技术。
详细描述
目前检测单核苷酸多态性的方法有多种,主要包括直接测序法、单链构象多态性分析、 限制性片段长度多态性分析、变性梯度凝胶电泳和基于PCR的引物延伸技术等。这些方 法均可用于检测基因组中单核苷酸的变异,为遗传学研究和医学应用提供有力支持。
关系。
04
实验结果与数据分析
实验结果展示
实验结果表格
提供了各个样本的单核苷酸多态性位点检测结果,包括基因型、 等位基因频率等数据。
实验结果图
通过条形图、饼图等形式展示了不同样本间的单核苷酸多态性分 布和比较结果。
数据解读
对实验结果表格和图进行了详细的解读,包括各个位点的基因型 分布、等位基因频率等信息。
点样与电泳
将PCR产物点样至电泳介 质上,进行电泳分离。
染色与观察
对分离后的DNA片段进行 染色,以便观察和记录结 果。
结果分析
条带识别
01
根据电泳结果,识别并记录不同样本间的差异条带。
数据分析
02
对数据进行统计分析,比较不同样本间的单核苷酸多态性分布
和频率。
结果解释
03
根据数据分析结果,解释单核苷酸多态性与相关表型或疾病的
掌握实验操作技能
通过实验操作,掌握SNP检测 的实验操作技能,包括DNA提 取、PCR扩增、电泳检测和基 因测序等。
02
实验原理
单核苷酸多态性的定义与特性
总结词
单核苷酸多态性是指基因组中单个核苷酸的变异,包括碱基的替换、插入或缺 失。
详细描述
单核苷酸多态性是基因组中常见的变异形式,通常表现为单个碱基的差异,例 如A、T、C、G之间的替换、插入或缺失。这些变异在人群中具有一定的频率, 并呈现出一定的遗传特征。
实验三多态性实验报告
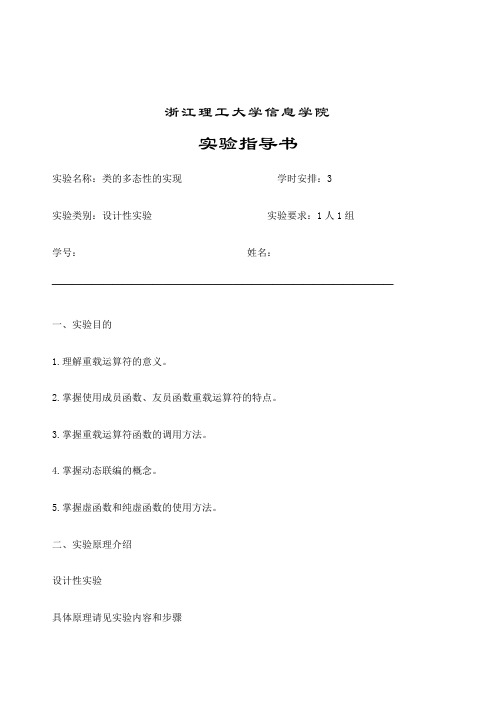
浙江理工大学信息学院实验指导书实验名称:类的多态性的实现学时安排:3实验类别:设计性实验实验要求:1人1组学号:姓名: ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄一、实验目的1.理解重载运算符的意义。
2.掌握使用成员函数、友员函数重载运算符的特点。
3.掌握重载运算符函数的调用方法。
4.掌握动态联编的概念。
5.掌握虚函数和纯虚函数的使用方法。
二、实验原理介绍设计性实验具体原理请见实验内容和步骤实现对抽象类的继承,通过operator函数调用的形式,实现运算符的重载三、实验设备介绍软件需求: windows或linux下的c++编译器硬件需求: 对于硬件方面的要求,建议配置是Pentium III 450以上的CPU处理器,64MB以上的内存,200MB的自由硬盘空间、CD-ROM驱动器、能支持24位真彩色的显示卡、彩色显示器、打印机。
四、实验内容某公司的员工有经理Manager、技术人员Technicist和营销人员SalsePerson,他们的薪金计算方法如下:经理按月计酬,方法是:基本工资+奖金;技术人员按月计酬,方法是:基本工资;营销人员按月计酬,方法是:基本工资+销售利润*5%。
每类人员都有职工编号、姓名、性别、入职时间、职位、基本工资等数据;各类人员使用统一接口get_pay()计算各类人员的月薪,重载<<运算符实现员工信息的输出。
其次,设计一个统计并输出该公司员工当月薪金情况的报表类Report,该类提供insert接口向Report类的容器中添加员工信息,并提供print接口用于展示以职位为单位的每个员工的职工编号、姓名、性别、入职时间以及当月该员工的薪酬,并统计出该职位员工薪酬的最高值和最低值。
为了提供更方便的查找功能,请为Report类重载[]运算符,下标值为职位,能根据职位信息查找出所有符合该职位的员工。
在主函数中对实现的类进行测试,首先,创建各类人员对象,通过Report类的insert接口向报表中添加这些人员信息,然后通过Report类的print接口输出当月员工薪酬情况报表。
多态性例题

多态性1 知识要点1.多态性:多态是指同样的消息被不同类型的对象接收后导致完全不同的行为。
2.面向对象的多态性可以分为4类:重载多态、强制多态、包含多态和参数多态。
3.多态从实现的角度来讲可以划分为两类:编译时的多态和运行时的多态。
4.运算符重载是对已有的运算符赋予多重含义,使同一个运算符作用于不同类型的数据导致不同类型的行为。
5.运算符重载的规则如下:1)C++语言中的运算符除了少数几个之外,全部可以重载,而且只能重载C++语言中已有的运算符。
2)重载之后运算符的优先级和结合性都不会改变。
3)运算符重载是针对新类型数据的实际需要,对原有运算符进行适当的改造。
一般来讲,重载的功能应当与原有功能相类似,不能改变原运算符的操作对象个数,同时至少要有一个操作对象是自定义类型。
不能重载的运算符只有5个,它们是类属关系运算符“.”、成员指针运算符“*”、作用域分辨符“::”、sizeof运算符和三目运算符“?:”。
前面两个运算符保证了C++语言中访问成员功能的含义不被改变。
作用域分辨符和sizeof运算符的操作数是类型,而不是普通的表达式,也不具备重载的特征。
6.运算符的重载形式有两种,重载为类的成员函数和重载为类的友元函数。
运算符重载为类的成员函数的一般语法形式为:函数类型 operater 运算符(形参表){ 函数体;}运算符重载为类的友元函数的一般语法形式为:friend 函数类型 operater 运算符(形参表){ 函数体;}7.虚函数说明虚函数的一般格式如下:virtual <函数返回类型说明符> <函数名>(<参数表>)在定义虚函数时要注意:(1) 虚函数是非静态的、非内联的成员函数,而不能是友元函数,但虚函数可以在另一个类中被声明为友元函数。
(2) 虚函数声明只能出现在类定义的函数原型声明中,而不能在成员函数的函数体实现的时候声明。
(3) 一个虚函数无论被公有继承多少次,它仍然保持其虚函数的特性。
实验3-多态性

A5=a1.operator*(a2)
A6=A1/A2;
A6=a1.perator/(a2)
A3=++A1;
A3=operator++(a1)
A4=A2++;
A4=a2.operator++
实 验 报 告
四、实验小结(包括问题和解决方法、心得体会、意见与建议等)
1.在题目(1)中由①②两步,请总结关于动态多态性的实现方法。
纯虚函数不能被调用,因为它只有函数名,而无具体实现代码,无法实现具体的
功能。
3.其它问题和解决方法:
在做第二个题时,把成员函数当作友元函数那样去重载运算符了,编译时报错说
参数过多,还没搞明白为什么,最后被老师指出来才发现,
4.心得体会:
看着教材上的例题照猫画虎竟然还出错了,有时针对不同的情况要有相应的处理
a6=a1/a2;
cout<<"a1=";
a1.print();
cout<<"a2=";
a2.print();
cout<<"a3=a1+a2=";
a3.print();
cout<<"a4=a1-a2=";
a4.print();
cout<<"a5=a1*a2=";
a5.print();
cout<<"a6=a1/a2=";
friend Complex operator-(const Complex &c1,const Complex &c2);
多态性 c 实验报告
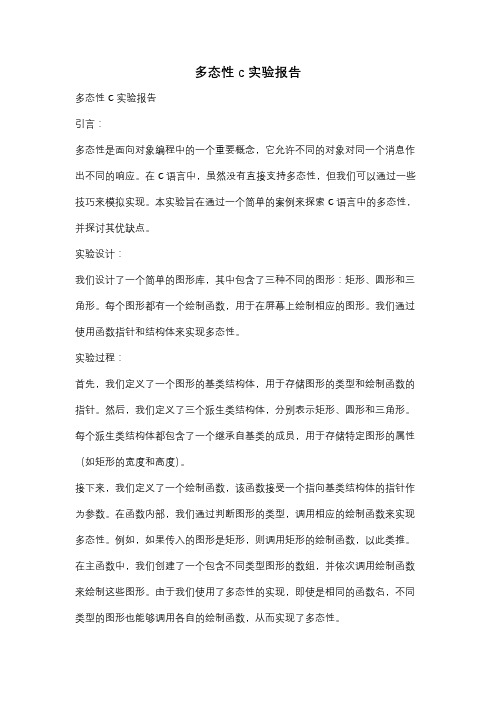
多态性 c 实验报告多态性 C 实验报告引言:多态性是面向对象编程中的一个重要概念,它允许不同的对象对同一个消息作出不同的响应。
在C语言中,虽然没有直接支持多态性,但我们可以通过一些技巧来模拟实现。
本实验旨在通过一个简单的案例来探索C语言中的多态性,并探讨其优缺点。
实验设计:我们设计了一个简单的图形库,其中包含了三种不同的图形:矩形、圆形和三角形。
每个图形都有一个绘制函数,用于在屏幕上绘制相应的图形。
我们通过使用函数指针和结构体来实现多态性。
实验过程:首先,我们定义了一个图形的基类结构体,用于存储图形的类型和绘制函数的指针。
然后,我们定义了三个派生类结构体,分别表示矩形、圆形和三角形。
每个派生类结构体都包含了一个继承自基类的成员,用于存储特定图形的属性(如矩形的宽度和高度)。
接下来,我们定义了一个绘制函数,该函数接受一个指向基类结构体的指针作为参数。
在函数内部,我们通过判断图形的类型,调用相应的绘制函数来实现多态性。
例如,如果传入的图形是矩形,则调用矩形的绘制函数,以此类推。
在主函数中,我们创建了一个包含不同类型图形的数组,并依次调用绘制函数来绘制这些图形。
由于我们使用了多态性的实现,即使是相同的函数名,不同类型的图形也能够调用各自的绘制函数,从而实现了多态性。
实验结果:经过编译和运行,我们成功地绘制出了矩形、圆形和三角形。
每个图形都按照其特定的形状和属性在屏幕上绘制出来。
这证明了我们通过函数指针和结构体模拟实现的多态性是有效的。
讨论:尽管C语言本身没有直接支持多态性的特性,但我们可以通过一些技巧来模拟实现。
使用函数指针和结构体是一种常见的方法,它允许不同类型的对象调用相同的函数名,从而实现了多态性。
然而,这种模拟实现也存在一些局限性。
首先,由于C语言的静态类型特性,我们需要在编译时确定对象的类型,无法在运行时动态确定。
这意味着我们需要手动管理对象的类型,并在调用函数时进行判断,增加了一定的复杂性。
实验三 多态性
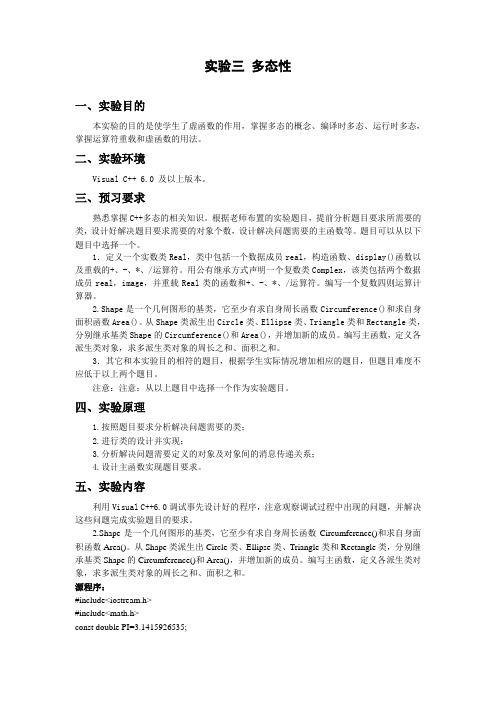
实验三多态性一、实验目的本实验的目的是使学生了虚函数的作用,掌握多态的概念、编译时多态、运行时多态,掌握运算符重载和虚函数的用法。
二、实验环境Visual C++ 6.0 及以上版本。
三、预习要求熟悉掌握C++多态的相关知识。
根据老师布置的实验题目,提前分析题目要求所需要的类,设计好解决题目要求需要的对象个数,设计解决问题需要的主函数等。
题目可以从以下题目中选择一个。
1.定义一个实数类Real,类中包括一个数据成员real,构造函数、display()函数以及重载的+、-、*、/运算符。
用公有继承方式声明一个复数类Complex,该类包括两个数据成员real,image,并重载Real类的函数和+、-、*、/运算符。
编写一个复数四则运算计算器。
2.Shape是一个几何图形的基类,它至少有求自身周长函数Circumference()和求自身面积函数Area()。
从Shape类派生出Circle类、Ellipse类、Triangle类和Rectangle类,分别继承基类Shape的Circumference()和Area(),并增加新的成员。
编写主函数,定义各派生类对象,求多派生类对象的周长之和、面积之和。
3.其它和本实验目的相符的题目,根据学生实际情况增加相应的题目,但题目难度不应低于以上两个题目。
注意:注意:从以上题目中选择一个作为实验题目。
四、实验原理1.按照题目要求分析解决问题需要的类;2.进行类的设计并实现;3.分析解决问题需要定义的对象及对象间的消息传递关系;4.设计主函数实现题目要求。
五、实验内容利用Visual C++6.0调试事先设计好的程序,注意观察调试过程中出现的问题,并解决这些问题完成实验题目的要求。
2.Shape是一个几何图形的基类,它至少有求自身周长函数Circumference()和求自身面积函数Area()。
从Shape类派生出Circle类、Ellipse类、Triangle类和Rectangle类,分别继承基类Shape的Circumference()和Area(),并增加新的成员。
多态性_C++程序设计习题解析与实践教程_[共12页]
![多态性_C++程序设计习题解析与实践教程_[共12页]](https://img.taocdn.com/s3/m/3158f958cc22bcd127ff0c8c.png)
第6章多态性一、填空题1.重载运算符仍然保持其原来的操作数个数、优先级和不变。
2.在C++重载运算符中,如用成员函数重载双目运算符,参数表中需要参数;如用友元函数重载双目运算符,参数表中需要参数。
3.重载运算符函数的函数名由关键字开头。
4.关联分为两种:静态关联和动态关联。
如果在程序就能确定调用哪个函数,称为静态关联;如果只有在程序才能确定调用哪个函数,称为动态关联。
5.通过函数重载和运算符重载实现的多态性属于多态性;通过虚函数实现的多态性属于多态性。
6.如果一个类中至少有一个纯虚函数,那么这个类称为。
7.由格式virtual 返回值类型函数名(参数表)=0定义的虚函数称为函数。
8.如果将基类的析构函数声明为虚函数,由该基类派生的所有派生类的析构函数也自动成为,即使派生类的析构函数与基类的析构函数名字不相同。
9.C++中虚构造函数,但虚析构函数。
10.若对复数数据类型Complex的对象a、b实现“+”操作运算,则定义“+”运算符重载为友元函数原型是。
二、判断题1.所有运算符都可以进行重载。
2.构造函数和析构函数都可以声明为虚函数。
3.虚函数既可以在函数说明时定义,也可以在函数实现时定义。
4.动态关联调用虚函数操作是通过指向对象的指针或对象引用的。
5.抽象类只能作为其他类的基类,不能建立抽象类的对象。
6.程序编译时就能确定的关联是一种动态关联。
7.一个基类中含有纯虚函数,则它的派生类一定不再是抽象类。
8.虚函数可以定义成类的静态成员函数。
9.如果派生类的成员函数的原型与基类中被定义为虚函数的成员函数原型相同,那么,这个函数自动继承基类中虚函数的特性。
10.如果声明了某个成员函数为虚函数,则在该类中不能出现和这个成员函数同名,并且返回值、参数个数、参数类型都相同的非虚函数。
6566 三、选择题1.下列有关运算符重载的描述中,正确的是()。
A.运算符重载可以改变操作数的个数B.运算符重载可以改变操作数的优先级C.运算符重载可以改变运算符的结合性D.运算符重载可以使运算符对用户定义新类型的对象实现特殊的操作。
多态性实验报告

一、实验目的通过本次实验,加深对多态性的理解,掌握多态性的基本概念、实现方式以及在Java语言中的应用,提高面向对象编程的能力。
二、实验环境1. 操作系统:Windows 102. 开发工具:Eclipse3. 编程语言:Java三、实验内容1. 多态性基本概念多态性是指同一个操作作用于不同的对象,可以有不同的解释,产生不同的执行结果。
在Java中,多态性主要表现在两个方面:方法重载和方法重写。
2. 方法重载方法重载是指在一个类中,允许存在多个名称相同、参数列表不同的方法。
编译器通过参数列表来区分这些方法,实现多态性。
(1)实验步骤1) 创建一个名为“Shape”的类,包含一个方法“draw()”,该方法不接受任何参数。
2) 在“Shape”类中添加三个方法:draw(String color),draw(String color, int size),draw(int x, int y)。
3) 创建一个名为“Circle”的类,继承自“Shape”类,并添加一个名为“draw”的方法,该方法接受一个int类型的参数radius。
4) 创建一个名为“Rectangle”的类,继承自“Shape”类,并添加一个名为“draw”的方法,该方法接受两个int类型的参数width和height。
5) 在主类中创建“Circle”和“Rectangle”对象,并调用它们的draw()方法。
(2)实验结果当执行程序时,根据传入的参数不同,调用不同的draw()方法。
3. 方法重写方法重写是指子类在继承父类的基础上,对父类的方法进行修改,实现多态性。
(1)实验步骤1) 创建一个名为“Animal”的类,包含一个名为“makeSound”的方法。
2) 创建一个名为“Dog”的类,继承自“Animal”类,并重写“makeSound”方法,使其输出“汪汪”。
3) 创建一个名为“Cat”的类,继承自“Animal”类,并重写“makeSound”方法,使其输出“喵喵”。
多态性实验报告

多态性实验报告多态性实验报告引言:多态性是面向对象编程中的一个重要概念,它允许对象在不同的上下文中表现出不同的行为。
本实验旨在通过一系列的实验来探究多态性的概念和应用,以及它对程序设计的影响。
实验一:多态性的概念在本实验的第一部分,我们首先对多态性的概念进行了深入的研究。
多态性是指同一个方法在不同的对象上表现出不同的行为。
例如,在一个动物类中,不同的子类(如狗、猫、鸟)都可以实现一个叫声的方法,但是每个子类的叫声是不同的。
这种灵活性使得我们可以编写更加通用和可扩展的代码。
实验二:多态性的应用在第二个实验中,我们通过一个图形绘制的例子来展示多态性的应用。
我们创建了一个抽象的图形类,并派生出不同的子类,如圆形、矩形和三角形。
每个子类都实现了一个绘制方法,但是绘制的方式和结果是不同的。
通过将这些不同的子类对象存储在一个通用的图形数组中,我们可以轻松地遍历并绘制每个图形,而无需关心具体的子类类型。
实验三:多态性的优势和局限性在第三个实验中,我们深入研究了多态性的优势和局限性。
多态性使得代码更加灵活和可扩展,可以减少代码的重复性。
然而,过度使用多态性可能会导致代码的复杂性增加,降低程序的性能。
因此,在设计和实现中需要权衡多态性的使用。
实验四:多态性在实际项目中的应用在最后一个实验中,我们通过一个实际的项目来展示多态性的应用。
我们选择了一个图书管理系统作为例子,其中包括了不同类型的图书,如小说、教材和杂志。
通过使用多态性,我们可以统一管理这些不同类型的图书,并实现一套通用的借阅和归还功能。
这样,无论新增了多少种类型的图书,我们都可以轻松地扩展和修改代码。
结论:通过本次实验,我们深入了解了多态性的概念和应用,并通过一系列的实验来验证和探究多态性在程序设计中的作用。
多态性的使用可以使代码更加灵活和可扩展,但也需要在设计和实现中进行合理的权衡。
在实际项目中,多态性可以帮助我们更好地管理和处理不同类型的对象,提高代码的可维护性和可扩展性。
c++实验多态性实验报告

c++实验多态性实验报告C++实验多态性实验报告一、实验目的本次实验的主要目的是深入理解和掌握 C++中的多态性概念及其实现方式。
通过实际编程和运行代码,体会多态性在面向对象编程中的重要作用,提高编程能力和对 C++语言特性的运用水平。
二、实验环境本次实验使用的编程环境为 Visual Studio 2019,操作系统为Windows 10。
三、实验内容(一)虚函数1、定义一个基类`Shape`,其中包含一个虚函数`area()`用于计算图形的面积。
2、派生类`Circle` 和`Rectangle` 分别重写`area()`函数来计算圆形和矩形的面积。
(二)纯虚函数1、定义一个抽象基类`Vehicle`,其中包含一个纯虚函数`move()`。
2、派生类`Car` 和`Bicycle` 分别实现`move()`函数来描述汽车和自行车的移动方式。
(三)动态多态性1、创建一个基类指针数组,分别指向不同的派生类对象。
2、通过基类指针调用虚函数,观察多态性的效果。
四、实验步骤(一)虚函数实现1、定义基类`Shape` 如下:```cppclass Shape {public:virtual double area()= 0;};```2、派生类`Circle` 的定义及`area()`函数的实现:```cppclass Circle : public Shape {private:double radius;public:Circle(double r) : radius(r) {}double area(){return 314 radius radius;}};```3、派生类`Rectangle` 的定义及`area()`函数的实现:```cppclass Rectangle : public Shape {private:double length, width;public:Rectangle(double l, double w) : length(l), width(w) {}double area(){return length width;}```(二)纯虚函数实现1、定义抽象基类`Vehicle` 如下:```cppclass Vehicle {public:virtual void move()= 0;};```2、派生类`Car` 的定义及`move()`函数的实现:```cppclass Car : public Vehicle {public:void move(){std::cout <<"Car is moving on the road"<< std::endl;}};3、派生类`Bicycle` 的定义及`move()`函数的实现:```cppclass Bicycle : public Vehicle {public:void move(){std::cout <<"Bicycle is moving on the path"<< std::endl;}};```(三)动态多态性实现1、创建基类指针数组并指向不同的派生类对象:```cppShape shapes2;shapes0 = new Circle(50);shapes1 = new Rectangle(40, 60);```2、通过基类指针调用虚函数:```cppfor (int i = 0; i < 2; i++){std::cout <<"Area: "<< shapesi>area()<< std::endl;}```五、实验结果(一)虚函数实验结果运行程序后,能够正确计算出圆形和矩形的面积,并输出到控制台。
C++多态编程三参考答案
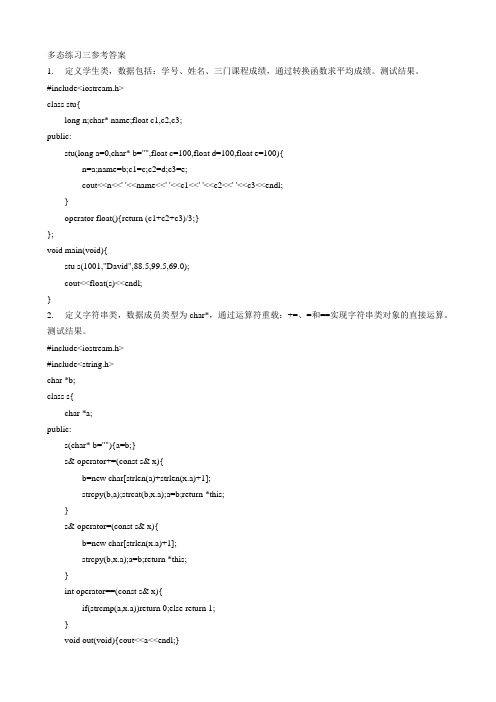
多态练习三参考答案1.定义学生类,数据包括:学号、姓名、三门课程成绩,通过转换函数求平均成绩。
测试结果。
#include<iostream.h>class stu{long n;char* name;float c1,c2,c3;public:stu(long a=0,char* b="",float c=100,float d=100,float e=100){n=a;name=b;c1=c;c2=d;c3=e;cout<<n<<' '<<name<<' '<<c1<<' '<<c2<<' '<<c3<<endl;}operator float(){return (c1+c2+c3)/3;}};void main(void){stu s(1001,"David",88.5,99.5,69.0);cout<<float(s)<<endl;}2.定义字符串类,数据成员类型为char*,通过运算符重载:+=、=和==实现字符串类对象的直接运算。
测试结果。
#include<iostream.h>#include<string.h>char *b;class s{char *a;public:s(char* b=""){a=b;}s& operator+=(const s& x){b=new char[strlen(a)+strlen(x.a)+1];strcpy(b,a);strcat(b,x.a);a=b;return *this;}s& operator=(const s& x){b=new char[strlen(x.a)+1];strcpy(b,x.a);a=b;return *this;}int operator==(const s& x){if(strcmp(a,x.a))return 0;else return 1;}void out(void){cout<<a<<endl;}~s(){if(a)delete[]a;}};void main(void){s s1("Good morning!"),s2(" Southeast University.");s1+=s2;s1.out();s1=s2;s1.out();if(s1==s2)cout<<"Equall."<<endl;else cout<<"Not equall."<<endl; }。
python中有关多态的试题

python中有关多态的试题含解答共20道1. 什么是多态性?-解答:多态性是指同一种操作作用于不同的对象上时,可以产生不同的行为。
2. Python中多态性的实现方式是什么?-解答:在Python中,多态性通过对象的特殊方法(例如`__len__`、`__add__`等)和动态类型特性实现。
3. 举例说明Python中的鸭子类型。
-解答:在Python中,不关心对象的具体类型,只关心对象是否具有特定的方法或属性。
例如,`len()`函数可以用于任何实现了`__len__`方法的对象。
4. 什么是函数的多态性?-解答:函数的多态性指同一函数名可以接受不同类型或数量的参数,并根据实际参数的类型或数量执行不同的操作。
5. 什么是运算符的多态性?-解答:运算符的多态性指同一运算符可以用于不同类型的操作数,并根据操作数的类型执行不同的操作。
6. 如何在Python中实现多态性?-解答:通过使用继承和重写方法,或者通过使用鸭子类型,可以实现多态性。
7. 什么是方法重写?它与多态性有什么关系?-解答:方法重写是子类覆盖父类中相同名称的方法。
多态性的一种表现就是通过方法重写实现,不同的子类可以提供不同的实现。
8. Python中的抽象基类是什么?它与多态性有什么关系?-解答:抽象基类是指包含抽象方法的类,它定义了一组子类必须实现的方法。
通过使用抽象基类,可以确保子类实现了必要的方法,从而实现多态性。
9. 什么是函数重载?Python支持函数重载吗?-解答:函数重载是指可以定义多个同名函数,但它们的参数类型或数量不同。
Python 不直接支持函数重载,因为它是一门动态类型语言,但可以通过默认参数值和可变参数来模拟一些重载的效果。
10. 如何使用`isinstance()`函数实现多态性?-解答:`isinstance()`函数用于检查对象是否属于指定的类型,通过使用该函数,可以在运行时根据对象的类型采取不同的操作,从而实现多态性。
2024年高考生物遗传多态性答案解析

2024年高考生物遗传多态性答案解析在2024年的高考生物科目中,遗传多态性是一个重要的知识点。
本文将对2024年高考生物遗传多态性的答案进行解析,帮助考生更好地理解和掌握这一知识点。
遗传多态性是指在一个物种中的个体间存在基因型和表型差异的现象。
这些差异可以来自于不同等位基因的存在,也可以来自于同一等位基因的不同表达方式。
遗传多态性对于物种的适应性和进化有重要的影响,因此在生物学中备受关注。
2024年高考生物的选择题部分涉及到了遗传多态性的相关知识。
下面是几道典型的考题及其解析,供考生参考:1. “在人类中,血型的遗传是遵循经典的孟德尔遗传规律的。
”这一说法正确吗?请解释原因。
解析:这一说法是不正确的。
血型的遗传并不完全符合孟德尔遗传规律,而是存在多基因遗传与等位基因共存的情况。
人类的血型主要有A、B、O和Rh因子四个血型,它们由3个基因所决定。
A、B和O三个血型的基因之间是互为共显性的关系,不同血型的基因之间可以共存。
2. 假设在一种虫子中,某个基因位点上有3个等位基因A、B和C,分别对应着黄色、褐色和黑色的体色。
交配实验显示,这3个等位基因是隐性关系。
某一杂合子虫子的体色是黑色。
请推测该杂合子虫子的基因型。
解析:根据题意可知,该杂合子虫子的体色是黑色,黑色的体色是由等位基因C决定的。
因此,该杂合子虫子的基因型为Cc。
3. 外部环境对遗传多态性的表现是否有影响?请阐述你的观点。
解析:外部环境可以对遗传多态性的表现产生一定的影响。
某些基因在特定环境下会表现出不同的表型。
例如,在季节变化明显的地区,同一物种的个体可能会表现出不同的大小和颜色,这是由外部环境对基因的影响所引起的。
总结起来,2024年高考生物遗传多态性的答案解析涉及了遗传规律、基因型推断以及外部环境对遗传多态性的影响等多个方面。
希望通过本文的解析,考生能够对这一知识点有更深入的理解和掌握,提升在高考中的得分。
加油!。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
1.定义一个复数类,通过重载运算符实现复数的四则运算、求负运算和赋值运算。
其中,要求加法“+”和减法“-”用友元函数实现重载,其他运算符用成员函数实现重载。
//main.cpp:#include "complex.h"int main(int argc , char *argv[]){COMPLEX c1,c2;cout<<"请输入第一个复数:\n";cin>>c1;cout<<"请输入第二个复数:\n";cin>>c2;cout<<"第一个复数是:"<<c1<<endl;cout<<"第二个复数是:"<<c2<<endl;cout<<"c1+c2的结果为:"<<c1+c2<<endl;cout<<"c1-c2的结果为:"<<c1-c2<<endl;cout<<"c1*c2的结果为:"<<c1*c2<<endl;cout<<"c1/c2的结果为:"<<c1/c2<<endl;cout<<"-c2的结果为:"<<-c2<<endl;return 0;}// complex.h#include<iostream>using namespace std;class COMPLEX{protected:double real;double image;public:COMPLEX(double r=0, double i=0);COMPLEX(const COMPLEX& other);friend COMPLEX operator+(const COMPLEX& left,const COMPLEX& right);friend COMPLEX operator-(const COMPLEX& left,const COMPLEX& right);COMPLEX operator*(const COMPLEX& other);COMPLEX operator/(const COMPLEX& other);COMPLEX operator-();COMPLEX operator=(const COMPLEX& other);friend ostream& operator <<(ostream& stream, COMPLEX& obj);friend istream& operator >>(istream& stream, COMPLEX& obj); };COMPLEX::COMPLEX(double r, double i){real=r;image=i;}COMPLEX::COMPLEX(const COMPLEX& other){real=other.real;image=other.image;}COMPLEX COMPLEX::operator*(const COMPLEX& other){COMPLEX temp;temp.real=real*other.real-image*other.image;temp.image=real*other.image+other.real*image;return temp;}COMPLEX COMPLEX::operator/(const COMPLEX& other){COMPLEX temp;double v=other.real*other.real+other.image*other.image;if (v!=0.0){temp.real=(real*other.real+image*other.image)/v;temp.image=(image*other.real-real*other.image)/v;return temp;}cout<<"除法无效\n";return temp;}COMPLEX COMPLEX::operator-(){COMPLEX temp;temp.real=-real;temp.image=-image;return temp;}COMPLEX COMPLEX::operator=(const COMPLEX& other){real=other.real;image=other.image;return *this;}/////////////////////////////////////////////////////// 友元函数不是类的成员函数!!COMPLEX operator+(const COMPLEX& left, const COMPLEX& right) {COMPLEX temp;temp.real=left.real+right.real;temp.image=left.image+right.image;return temp;}COMPLEX operator-(const COMPLEX& left, const COMPLEX& right) {COMPLEX temp;temp.real=left.real-right.real;temp.image=left.image-right.image;return temp;}ostream& operator <<(ostream& stream, COMPLEX& obj){stream<<obj.real;if(obj.image>0) stream<<"+"<<obj.image<<"i";else if(obj.image<0) stream<<obj.image<<"i";return stream;}istream& operator >>(istream& stream, COMPLEX& obj){cout<<"输入实部:";stream>> obj.real;cout<<"输入虚部:";stream>> obj.image;return stream;}2.设计一个多态数组,该数组用于存放四种几何图形(三角形、矩形、正方形和圆),要求利用虚函数实现的多态性来求多态数组中几何图形的面积之和。
几何图形的类型可以通过构造函数或通过成员函数来设置。
//main.cpp#include "figure.h"int main(int argc, char* argv[]){FIGURE* pf[4];int i;double sum;TRIANGLE t(1,4,3,4);RECTANGLE r(3,4,5,6);SQUARE s(4,5,9);CIRCLE c(5,6,2);// 动态重载的要素:继承、指针pf[0]=&t;pf[1]=&r;pf[2]=&s;pf[3]=&c;// 求面积之和sum=0.0;for(i=0;i<4;i++){sum+=pf[i]->getArea();}cout<<"面积之和为: "<<sum<<endl;return 0;}//igure.h#include <iostream>using namespace std;const double PI=3.1415926;class FIGURE{public:FIGURE(double x, double y);virtual double getArea()=0;private:double x_pos;double y_pos;};class TRIANGLE:public FIGURE{public:TRIANGLE(double x, double y, double len, double hei);virtual double getArea();private:double length;double height;};class RECTANGLE:public FIGURE{public:RECTANGLE(double x, double y, double len, double wid);virtual double getArea();private:double length;double width;};class SQUARE:public FIGURE{public:SQUARE(double x, double y, double len);virtual double getArea();private:double length;};class CIRCLE:public FIGURE{public:CIRCLE(double x, double y, double r);virtual double getArea();private:double radius;};//////////////////////////////////////////////////////////////////////////////////// FIGURE::FIGURE(double x, double y)x_pos=x;y_pos=y;}TRIANGLE::TRIANGLE(double x, double y, double len, double hei):FIGURE(x,y) {length=len;height=hei;}double TRIANGLE::getArea(){return 0.5*length*height;}RECTANGLE::RECTANGLE(double x, double y, double len, double wid):FIGURE(x,y) {length=len;width=wid;}double RECTANGLE::getArea(){return length*width;}SQUARE::SQUARE(double x, double y, double len):FIGURE(x,y){length=len;}double SQUARE::getArea(){return length*length;}CIRCLE::CIRCLE(double x, double y, double r):FIGURE(x,y){radius=r;}double CIRCLE::getArea(){return PI*radius*radius;}。