Symmetric Multiprocessing on Programmable Chips Made Easy
python 多进程 process 的方法

python多进程process的方法Python多进程编程的方法简介多进程编程是一种利用多个进程同时执行不同任务,提高程序运行效率和并发性的编程方法。
在Python中,可以使用multiprocessing模块中的Process类来创建和管理多个进程,实现多进程编程。
本文将介绍Process类的基本用法,以及如何使用Process类实现一些常见的多进程编程场景,如进程间的通信和同步,进程池的使用,以及进程的异常处理。
一、Process类的基本用法Process类是multiprocessing模块中的一个类,它表示一个进程对象,可以用来创建和启动一个新的进程,以及控制进程的状态和属性。
Process类的构造方法如下:pythonmultiprocessing.Process(group=None, target=None,name=None, args=(), kwargs={}, daemon=None)其中,group参数用于指定进程所属的进程组,一般为None;target参数用于指定进程要执行的函数;name参数用于指定进程的名称;args参数用于指定传递给target函数的位置参数;kwargs 参数用于指定传递给target函数的关键字参数;daemon参数用于指定进程是否为守护进程,即是否随主进程的结束而结束。
创建一个Process对象后,可以调用其start方法来启动进程,调用其join方法来等待进程结束,调用其terminate方法来强制终止进程,调用其is_alive方法来判断进程是否存活,以及调用其name、pid、exitcode等属性来获取进程的相关信息。
下面是一个简单的例子,演示了如何使用Process类创建和启动两个进程,分别打印出自己的名称和进程号:pythonimport multiprocessingimport time# 定义一个函数,打印出进程的名称和进程号def print_info():print(f"进程名称:{multiprocessing.current_process().name}")print(f"进程号:{multiprocessing.current_process().pid}")# 创建两个Process对象,分别指定target为print_info函数,name为P1和P2p1 = multiprocessing.Process(target=print_info,name="P1")p2 = multiprocessing.Process(target=print_info,name="P2")# 启动两个进程p1.start()p2.start()# 等待两个进程结束p1.join()p2.join()运行结果如下:进程名称:P1进程号:1234进程名称:P2进程号:5678二、进程间的通信和同步在多进程编程中,经常需要实现进程间的通信和同步,即让不同的进程能够相互发送和接收数据,以及协调好各自的执行顺序和速度。
python multiprocessing 使用方法

python multiprocessing 使用方法Python是一门强大的编程语言,提供了许多方便的功能和库,其中包括对多进程编程的支持。
Python的`multiprocessing`模块为我们提供了通过创建多个进程来并行执行代码的能力。
在本文中,我们将一步一步地了解如何使用`multiprocessing`模块来实现多进程编程。
目录:1. 什么是多进程编程?2. 为什么要使用多进程编程?3. Python中的multiprocessing模块4. 使用multiprocessing模块创建进程5. 进程间通信6. 进程池7. 常见的多进程编程用例8. 总结1. 什么是多进程编程?多进程编程是一种软件设计技术,通过同时创建多个进程来执行任务。
每个进程都是独立运行的,拥有自己的内存空间和系统资源。
这就意味着多个进程可以并行执行任务,从而加快整体程序的执行速度。
2. 为什么要使用多进程编程?使用多进程编程可以带来许多好处,包括:- 提高程序的执行速度:通过将任务拆分成多个进程来并行执行,可以加速程序的运行,尤其是在有大量计算密集型任务的情况下。
- 充分利用多核处理器:现代计算机通常都配备有多个核心的处理器,使用多进程编程可以使得程序能够同时利用多个核心,从而提高性能。
- 更好地响应用户输入:在某些情况下,用户需要与程序进行交互,但程序又需要执行耗时的计算任务。
通过将计算任务放在独立的进程中执行,可以使程序能够同时响应用户输入和执行计算任务。
3. Python中的multiprocessing模块Python的`multiprocessing`模块提供了一种方便的方式来实现多进程编程。
该模块提供了创建、启动和管理进程的工具,还提供了进程间通信的机制。
要使用`multiprocessing`模块,首先需要导入该模块:pythonimport multiprocessing4. 使用multiprocessing模块创建进程使用`multiprocessing`模块创建进程非常简单。
Python并行计算库multiprocessing的使用方法
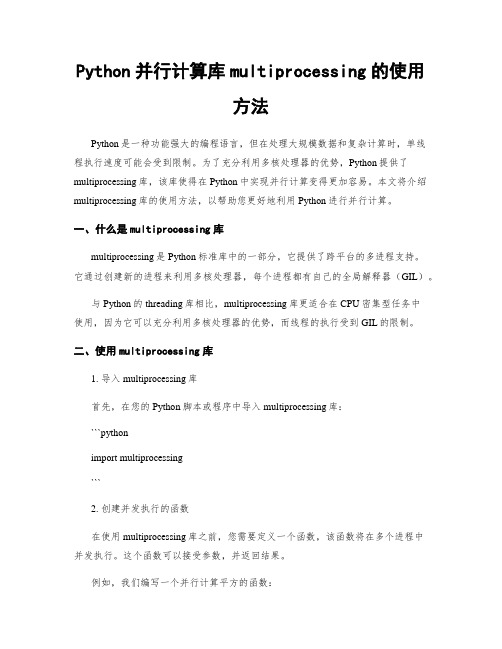
Python并行计算库multiprocessing的使用方法Python是一种功能强大的编程语言,但在处理大规模数据和复杂计算时,单线程执行速度可能会受到限制。
为了充分利用多核处理器的优势,Python提供了multiprocessing库,该库使得在Python中实现并行计算变得更加容易。
本文将介绍multiprocessing库的使用方法,以帮助您更好地利用Python进行并行计算。
一、什么是multiprocessing库multiprocessing是Python标准库中的一部分,它提供了跨平台的多进程支持。
它通过创建新的进程来利用多核处理器,每个进程都有自己的全局解释器(GIL)。
与Python的threading库相比,multiprocessing库更适合在CPU密集型任务中使用,因为它可以充分利用多核处理器的优势,而线程的执行受到GIL的限制。
二、使用multiprocessing库1. 导入multiprocessing库首先,在您的Python脚本或程序中导入multiprocessing库:```pythonimport multiprocessing```2. 创建并发执行的函数在使用multiprocessing库之前,您需要定义一个函数,该函数将在多个进程中并发执行。
这个函数可以接受参数,并返回结果。
例如,我们编写一个并行计算平方的函数:```pythondef square(x):return x ** 2```3. 创建进程池接下来,您可以使用multiprocessing.Pool来创建一个进程池。
进程池是一组准备好的工作者进程,它们可以并发地执行任务。
```pythonpool = multiprocessing.Pool()```您可以使用可选的参数来指定进程池的大小,例如:```pythonpool = multiprocessing.Pool(processes=4)```这将创建一个包含4个工作者进程的进程池。
pythonmultiprocessing用法

pythonmultiprocessing用法Python multiprocessing用法介绍Python的multiprocessing模块是Python标准库中用于并行处理的模块。
它提供了一个Process类,通过创建多个进程来执行任务,从而实现并行处理。
本文将介绍一些常用的multiprocessing用法,让你更好地利用这个强大的模块。
1. 导入multiprocessing模块在使用multiprocessing模块之前,首先需要导入该模块。
import multiprocessing2. 创建进程可以使用Process类来创建一个新的进程。
创建进程时,需要指定要执行的函数或方法。
def func():# 进程要执行的任务if __name__ == '__main__':p = (target=func)()()3. 传递参数可以使用args参数来传递参数给进程的任务函数。
def func(name, age):# 进程要执行的任务if __name__ == '__main__':p = (target=func, args=('Alice', 25))()()4. 多个进程可以同时创建多个进程来并行执行任务。
def func(name):# 进程要执行的任务if __name__ == '__main__':processes = []names = ['Alice', 'Bob', 'Charlie']for name in names:p = (target=func, args=(name,))(p)()for p in processes:()5. 进程之间的通信可以使用multiprocessing模块提供的Queue来实现多个进程之间的数据通信。
import multiprocessingdef producer(q):# 生产数据('data')def consumer(q):# 消费数据data = ()if __name__ == '__main__':q = ()p1 = (target=producer, args=(q,))p2 = (target=consumer, args=(q,))()()()()6. 进程池可以使用multiprocessing模块提供的Pool来创建一个进程池,从而复用进程并限制并发数量。
multiprocessing 类参数

multiprocessing 类参数如下:1.processes: 指定进程池中的进程数量。
如果未指定,则默认为CPU 核心数。
2.initializer: 一个可调用的函数,在每个子进程开始之前被调用。
通常用于设置共享内存或其他初始化操作。
3.initargs: 传递给initializer的参数元组。
4.maxtasksperchild: 子进程在开始下一个任务之前可以执行的最大任务数。
这有助于避免资源过度使用。
5.context: 指定使用的multiprocessing 上下文。
默认为multiprocessing,但也可以是mp。
6.authkey: 用于进程间安全的字符串。
它可以用于安全地交换信息(例如在远程进程中使用map函数时)。
7.**return_紧密结合的输出**:如果设置为True,则Pool.map()和Pool.map_async()` 将返回一个紧密结合的列表,而不是一个松散结合的列表。
8.error_callback: 当任务引发异常时调用的函数。
下面是一个简单的例子,演示如何使用multiprocessing.Pool:在这个例子中,我们定义了一个简单的函数square(),它接受一个数字并返回其平方。
然后,我们使用Pool类创建一个进程池,并使用map()方法将列表[1, 2, 3, 4, 5]中的每个元素传递给square()函数。
由于我们指定了processes=4,因此任务将在4个不同的进程中并行执行。
最后,我们打印出结果列表,该列表包含每个输入元素的平方值。
python mutilprocessing用法

python mutilprocessing用法Python multiprocessing是Python提供的一个支持多进程并发编程的模块,可以利用多核CPU来加速程序的执行。
以下是Python multiprocessing的基本用法:1. 导入multiprocessing模块```pythonimport multiprocessing```2. 创建进程```pythonp = multiprocessing.Process(target=func, args=(arg1, arg2))```其中,func是要执行的函数,arg1和arg2是函数的参数,可以是任何类型的数据。
3. 启动进程```pythonp.start()```4. 等待进程结束```pythonp.join()```5. 获取进程返回值```pythonresult = p.returnvalue```其中,result是func函数的返回值。
6. 进程池如果有多个任务需要执行并发处理,可以使用进程池,避免频繁创建和销毁进程。
```pythonpool = multiprocessing.Pool(processes=numProcess)result = pool.map(func, listArgs)```其中,numProcess是进程池中进程的数量,listArgs是一个列表,包含了func函数需要处理的所有参数。
map函数会将listArgs中的每个参数分发给进程池中的进程,并获取每个进程的返回值,最后返回一个包含了所有返回值的列表result。
除了map函数外,还有apply、imap和imap_unordered等函数,可以根据需要选择使用。
7. 进程通信进程之间需要通信时,可以使用管道、队列等机制。
```pythonparent_conn, child_conn = multiprocessing.Pipe()parent_conn.send('hello')msg = child_conn.recv()```上述代码中,通过Pipe函数创建了一个双向管道,parent_conn是父进程的管道,child_conn是子进程的管道。
《操作系统概念》作业解答

Chapter 4
4.2 Describe the differences among short-term, medium-term, and long-term scheduling.
Short-term,CPU调度 Long-term,job调度 Medium-term,分时系统中的中间调度级
e. Network
在通用OS上添加
联网、通信功能 远程过程调用 文件共享
f. Distributed
具有联网、通信功能 提供远程过程调用 提供多处理机的统一调度调度 统一的存储管理 分布式文件系统
1-cont.
1.9 Describe the differences between symmetric and asymmetric multiprocessing. What are three advantages and one disadvantage of multiprocessor systems?
1-cont.
1.5 In a multiprogramming and time-sharing environment, several users share the system simultaneously. This situation can result in various security problems.
Symmetric multiprocessing中所有处理器同等对待,I/O可以 在任意CPU上处理。
Asymmetric multiprocessing具有一个主CPU和多个从CPU,主 CPU将任务分派到从CPU,I/O通常只能由主CPU处理。
multiprocessing process 的返回值-概述说明以及解释

multiprocessing process 的返回值-概述说明以及解释1.引言1.1 概述概述部分的内容可以从多进程编程的背景与概念入手,介绍多进程编程的基本概念和其在现代计算机领域中的重要性。
以下是一种可能的方式:概述:多进程编程是一种并行处理技术,它通过同时启动多个进程来提高程序的执行效率和性能。
它在现代计算机领域中被广泛应用于诸如数据处理、图像处理、科学计算和机器学习等领域。
相对于单进程编程,多进程编程能够充分利用计算机的多核处理能力,实现任务的并行执行,从而提高程序的响应速度和处理能力。
多进程编程的基本概念是将一个程序拆分为多个并行执行的进程,每个进程独立执行指定的任务,进程之间通过进程间通信(IPC)来实现数据的共享与交互。
常用的多进程编程模块包括Python中的multiprocessing模块,它提供了丰富的函数和类来简化多进程编程的实现。
在多进程编程中,multiprocessing process是一个重要的概念。
它表示一个独立的进程,可以通过创建multiprocessing process对象来实现多进程编程。
然而,与单进程编程不同的是,multiprocessing process 并不能直接返回结果给主进程,而是需要通过其他方式来获取其执行结果。
本文将重点讨论multiprocessing process的返回值问题。
我们将介绍multiprocessing模块的使用方法,深入探讨multiprocessing process的返回值的重要性,并分析如何处理这些返回值以实现程序的正确执行。
通过对多进程编程与multiprocessing process的返回值进行全面的分析与研究,我们可以更好地应用多进程编程技术来提高程序的性能和效率。
接下来的章节将详细介绍多进程编程的基本概念、multiprocessing 模块的使用方法,以及深入探讨multiprocessing process的返回值问题。
multiprocessing循环调用matlab函数-概述说明以及解释

multiprocessing循环调用matlab函数-概述说明以及解释1.引言1.1 概述概述在数据处理和科学计算中,使用多进程处理可以大大提高程序的运行效率。
而在实际的工程应用中,经常会遇到需要循环调用Matlab函数处理大量数据的情况。
本文将介绍如何利用Python的Multiprocessing模块来实现循环调用Matlab函数的并行处理,从而提高程序的运行效率和加快数据处理速度。
同时,本文还将探讨在实际应用场景中如何灵活运用这一技术,以及可能遇到的挑战和解决方案。
通过深入探讨Multiprocessing和Matlab函数的结合运用,读者将能够更好地理解并掌握这一高效的并行计算方法。
1.2 文章结构文章结构是指整篇文章的框架和组织方式。
在本篇文章中,我们将会按照以下结构来呈现我们的内容。
第一部分是引言部分,在这里我们将对multiprocessing循环调用matlab函数这一主题做一个简单的概述,说明文章结构和目的。
第二部分是正文部分,我们将首先介绍multiprocessing的简介,说明其概念和作用。
然后详细讨论如何在python中循环调用Matlab函数来提高程序运行效率。
最后,我们会给出实际应用场景,通过案例展示如何在实际项目中应用这种方法。
第三部分是结论部分,我们将对整篇文章进行总结,简要回顾并强调本文的重点。
同时,我们也会展望未来的研究方向和可能的发展趋势。
最后,我们将以一段结束语来结束整篇文章,为读者留下深刻的印象。
通过以上结构,本文将系统地介绍multiprocessing循环调用matlab 函数的相关知识,为读者提供清晰的思路和深入的理解。
1.3 目的在本文中,我们的目的是探讨如何利用multiprocessing模块来实现对Matlab函数的循环调用,以提高程序运行效率和节省时间。
通过分析multiprocessing的基本概念和原理,结合Matlab函数的特点,我们将展示如何利用多进程并行计算的方式来加快对大数据集的处理速度,提高计算效率。
python multiprocessing的多进程用法

python multiprocessing的多进程用法Python multiprocessing是Python标准库中的一个模块,它提供了一种简单而有效的方式来处理多进程编程。
多进程编程是一种并行计算的方法,通过同时执行多个子任务来提高程序的性能。
在本文中,我们将一步一步地学习如何使用Python multiprocessing模块进行多进程编程。
我们将探讨一些概念、函数和方法,以及一些常见的应用场景。
1. 导入multiprocessing模块在使用multiprocessing模块之前,首先需要导入它。
导入的语句如下:import multiprocessing2. 创建进程在创建进程之前,我们需要明确要执行的任务。
通常情况下,我们将任务定义为一个函数。
下面是一个简单的示例:def worker():print("Worker process")然后,我们使用multiprocessing模块的`Process`类来创建一个进程,示例代码如下:p = multiprocessing.Process(target=worker)这里,我们将worker函数作为目标函数传递给Process类的构造函数。
注意,`target`参数必须指定为一个可调用的对象。
3. 启动进程创建进程后,我们需要使用`start()`方法来启动它。
示例代码如下:p.start()4. 等待进程结束一旦进程开始运行,我们可以使用`join()`方法来等待进程的结束。
示例代码如下:p.join()5. 进程间的通信在多进程编程中,进程之间通常需要进行一些数据的交换和共享。
Python 的multiprocessing模块提供了一些机制来实现进程间的通信,例如队列、管道等。
在本文中,我们将介绍一个常用的进程间通信机制:队列。
可以使用`multiprocessing.Queue`类来创建一个队列,示例代码如下:q = multiprocessing.Queue()然后,可以使用队列的`put()`和`get()`方法来向队列中添加和获取数据。
multiprocessing 结束子程序 -回复

multiprocessing 结束子程序-回复multiprocessing是Python中的一个标准库,可以用来实现多进程编程。
在编写多进程程序时,我们经常需要在主进程中启动一个子进程,然后在必要的时候结束子进程。
本文将详细介绍如何使用multiprocessing库来结束子进程的方法。
在使用multiprocessing库之前,首先需要导入相关的模块:pythonimport multiprocessing接下来,我们可以使用multiprocessing库中的Process类来创建一个子进程。
通过创建一个Process对象,我们可以指定需要在子进程中执行的函数和参数。
下面是一个简单的例子:pythonimport timeimport multiprocessingdef print_func(name):for i in range(5):print("Hello,", name)time.sleep(1)if __name__ == '__main__':p = multiprocessing.Process(target=print_func, args=('Alice',)) p.start()在上面的例子中,我们创建了一个名为print_func的函数来输出"Hello, name",然后通过创建一个Process对象并调用start()方法来启动子进程。
子进程将会在独立的进程中执行print_func函数。
在主进程中,p.start()会立即返回,而子进程会在后台运行。
为了结束子进程,multiprocessing库提供了terminate()方法。
我们可以在需要结束子进程的时候调用该方法。
下面是一个修改后的例子,演示了如何结束子进程:pythonimport timeimport multiprocessingdef print_func(name):for i in range(5):print("Hello,", name)time.sleep(1)if __name__ == '__main__':p = multiprocessing.Process(target=print_func, args=('Alice',)) p.start()# 在子进程执行一段时间后,结束子进程time.sleep(3)p.terminate()p.join()在上面的例子中,我们使用了time模块来模拟子进程运行的时间。
multiprocessing queue 方法
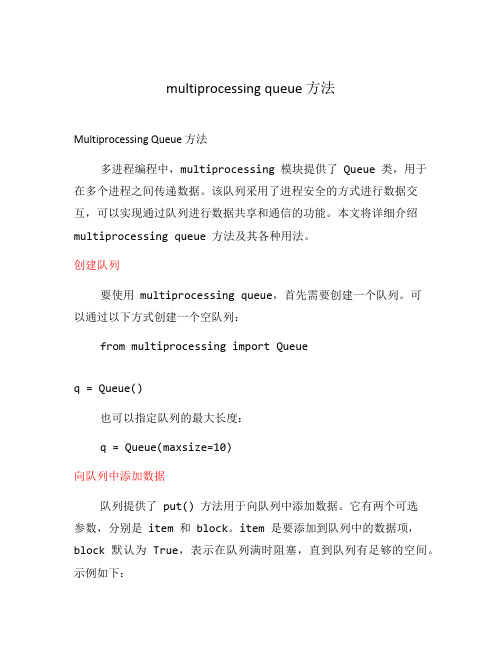
multiprocessing queue 方法Multiprocessing Queue 方法多进程编程中,multiprocessing模块提供了Queue类,用于在多个进程之间传递数据。
该队列采用了进程安全的方式进行数据交互,可以实现通过队列进行数据共享和通信的功能。
本文将详细介绍multiprocessing queue方法及其各种用法。
创建队列要使用multiprocessing queue,首先需要创建一个队列。
可以通过以下方式创建一个空队列:from multiprocessing import Queueq = Queue()也可以指定队列的最大长度:q = Queue(maxsize=10)向队列中添加数据队列提供了put()方法用于向队列中添加数据。
它有两个可选参数,分别是item和block。
item是要添加到队列中的数据项,block默认为True,表示在队列满时阻塞,直到队列有足够的空间。
示例如下:(item, block=True)如果要添加多个数据项,可以使用put()方法的循环调用。
示例如下:for item in items:(item)从队列中获取数据队列提供了get()方法用于从队列中获取数据。
它有一个可选参数block,表示在队列为空时是否阻塞等待,默认为True。
调用示例如下:item = (block=True)如果要连续获取多个数据项,可以使用get()方法的循环调用。
示例如下:while not ():item = ()队列判空队列提供了empty()方法用于判断队列是否为空。
示例如下:if ():print("队列为空")else:print("队列不为空")获取队列大小队列提供了qsize()方法用于获取队列中数据项的数量。
示例如下:size = ()print("队列大小为:", size)清空队列队列提供了()方法用于清空队列中的所有数据。
torch.multiprocessing推理

torch.multiprocessing推理TensorFlow是深度学习中最常用的框架之一,而其中的torch.multiprocessing模块则提供了一种并发处理的方法。
本文将介绍torch.multiprocessing模块的推理功能,并通过一步一步的回答来帮助读者深入了解这个功能。
一、什么是torch.multiprocessing?torch.multiprocessing是PyTorch框架中的一个模块,它提供了一种并发处理的方法,可以有效地利用多个CPU核心或多个机器的计算资源。
torch.multiprocessing允许用户通过在不同的进程中执行任务来同时进行多个任务,从而提高了模型训练和推理的效率。
二、为什么需要torch.multiprocessing?深度学习模型在训练和推理过程中通常需要大量的计算资源。
在单台机器上进行训练和推理往往会面临计算资源不足的问题,而使用分布式计算可以充分利用多台机器资源来加速模型的训练和推理。
torch.multiprocessing提供了一种方便的接口,使用户可以很容易地实现分布式计算。
三、如何使用torch.multiprocessing进行推理?下面我们将一步一步介绍如何使用torch.multiprocessing进行推理。
1.导入必要的模块和类首先,我们需要导入必要的模块和类。
在使用torch.multiprocessing之前,我们需要导入torch模块以及torch.multiprocessing模块。
pythonimport torchimport torch.multiprocessing as mp2.定义推理函数接下来,我们需要定义一个推理函数。
推理函数输入模型和输入数据,输出模型对输入数据的预测。
这个函数的实现与单机环境下的推理函数类似。
pythondef inference(model, input_data):将模型设为评估模式model.eval()输入数据预处理input_data = torch.tensor(input_data)模型推理with torch.no_grad():output = model(input_data)return output3.定义推理进程在使用torch.multiprocessing进行推理时,我们需要定义一个推理进程。
multiprocessing.value使用方法 -回复
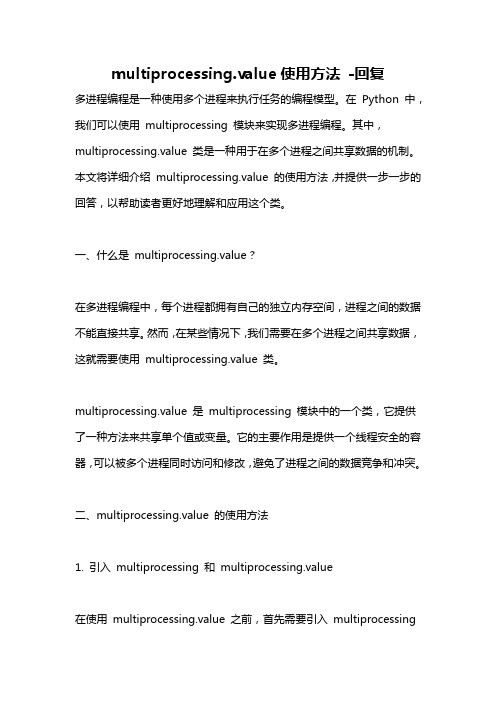
multiprocessing.value使用方法-回复多进程编程是一种使用多个进程来执行任务的编程模型。
在Python 中,我们可以使用multiprocessing 模块来实现多进程编程。
其中,multiprocessing.value 类是一种用于在多个进程之间共享数据的机制。
本文将详细介绍multiprocessing.value 的使用方法,并提供一步一步的回答,以帮助读者更好地理解和应用这个类。
一、什么是multiprocessing.value?在多进程编程中,每个进程都拥有自己的独立内存空间,进程之间的数据不能直接共享。
然而,在某些情况下,我们需要在多个进程之间共享数据,这就需要使用multiprocessing.value 类。
multiprocessing.value 是multiprocessing 模块中的一个类,它提供了一种方法来共享单个值或变量。
它的主要作用是提供一个线程安全的容器,可以被多个进程同时访问和修改,避免了进程之间的数据竞争和冲突。
二、multiprocessing.value 的使用方法1. 引入multiprocessing 和multiprocessing.value在使用multiprocessing.value 之前,首先需要引入multiprocessing模块和multiprocessing.value 类。
可以通过以下代码来实现:pythonimport multiprocessing# 注意:我们也可以单独引入特定的类# from multiprocessing import Process, Value2. 创建multiprocessing.value 对象创建一个multiprocessing.value 对象需要指定数据类型和初始值。
可以通过Value 函数来创建一个multiprocessing.value 对象。
Value 函数的参数包括数据类型和初始值,示例如下:pythonvalue = multiprocessing.Value('i', 0)# 'i' 表示数据类型为整型,0 是初始值3. 修改multiprocessing.value 的值要修改multiprocessing.value 对象的值,可以使用它的value 属性。
multiprocessing函数
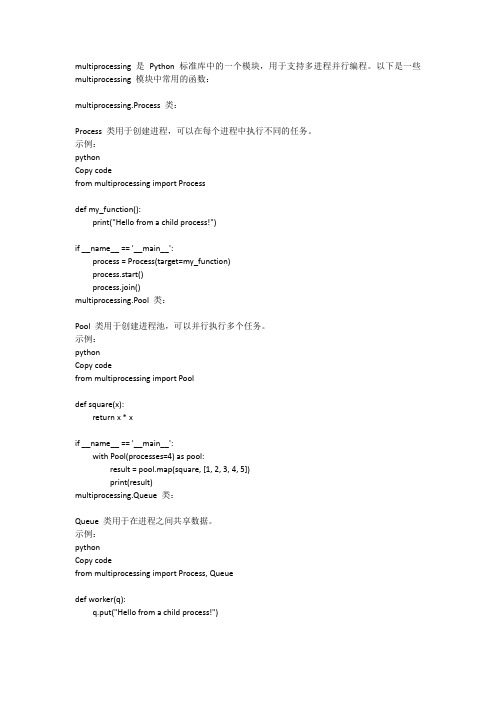
multiprocessing 是Python 标准库中的一个模块,用于支持多进程并行编程。
以下是一些multiprocessing 模块中常用的函数:multiprocessing.Process 类:Process 类用于创建进程,可以在每个进程中执行不同的任务。
示例:pythonCopy codefrom multiprocessing import Processdef my_function():print("Hello from a child process!")if __name__ == '__main__':process = Process(target=my_function)process.start()process.join()multiprocessing.Pool 类:Pool 类用于创建进程池,可以并行执行多个任务。
示例:pythonCopy codefrom multiprocessing import Pooldef square(x):return x * xif __name__ == '__main__':with Pool(processes=4) as pool:result = pool.map(square, [1, 2, 3, 4, 5])print(result)multiprocessing.Queue 类:Queue 类用于在进程之间共享数据。
示例:pythonCopy codefrom multiprocessing import Process, Queuedef worker(q):q.put("Hello from a child process!")if __name__ == '__main__':q = Queue()process = Process(target=worker, args=(q,))process.start()process.join()message = q.get()print(message)multiprocessing.Lock 类:Lock 类用于在多进程之间同步共享资源的访问。
mutilprocess详解

mutilprocess详解多进程详解多进程是指在一个程序中运行多个进程,每个进程独立执行,并有自己的内存空间。
它可以充分利用多核处理器的优势,提高程序的执行效率和并发能力。
下面我们将详细讨论多进程的相关概念和特点。
1. 进程和线程的区别:进程是操作系统分配资源的基本单位,每个进程都拥有自己的独立内存空间和系统资源。
线程是在进程内部执行的任务单元,多个线程共享同一进程的资源。
进程之间相互独立,而线程之间可以共享数据。
2. 创建和管理多进程:在Python中,可以使用`multiprocessing`模块来创建和管理多进程。
通过创建`Process`类的实例,可以启动一个新的进程。
使用`start()`方法来启动进程,使用`join()`方法来等待进程执行完毕。
同时,`multiprocessing`模块还提供了进程间通信的功能,如`Queue`、`Pipe`等。
3. 多进程的优势:多进程能够充分利用多核处理器的并行计算能力,提高程序的执行效率。
通过将任务分配给不同的进程,可以实现并行处理,加快程序运行速度。
同时,多进程还可以增加程序的健壮性,一个进程出现问题不会影响其他进程的正常运行。
4. 多进程的应用场景:多进程广泛应用于需要并行处理大量数据或耗时任务的场景。
例如,在图像处理领域,可以将图像分成多块,为每个进程分配一块图像进行处理;在网络爬虫中,可以为每个URL创建一个进程来同时抓取多个网页。
总结:多进程是提高程序并发能力和执行效率的一种重要手段。
通过合理利用多进程,可以实现任务的并行处理,加快程序的运行速度。
在Python中,使用`multiprocessing`模块可以方便地创建和管理多进程,同时还提供了进程间通信的功能。
多进程的应用场景广泛,特别适用于需要并行处理大量数据或耗时任务的场景。
multiprocessing.process的用法

multiprocessing.process的⽤法⽬录multiprocessing模块仔细说来,multiprocess不是⼀个模块⽽是python中⼀个操作、管理进程的包。
之所以叫multi是取⾃multiple的多功能的意思,在这个包中⼏乎包含了和进程有关的所有⼦模块。
由于提供的⼦模块⾮常多,为了⽅便⼤家归类记忆,我将这部分⼤致分为四个部分:创建进程部分,进程同步部分,进程池部分,进程之间数据共享。
multiprocessing.Process模块process模块是⼀个创建进程的模块,借助这个模块,就可以完成进程的创建。
process模块介绍Process([group [, target [, name [, args [, kwargs]]]]]),由该类实例化得到的对象,表⽰⼀个⼦进程中的任务(尚未启动)强调:1. 需要使⽤关键字的⽅式来指定参数2. args指定的为传给target函数的位置参数,是⼀个元组形式,必须有逗号参数介绍:group参数未使⽤,值始终为Nonetarget表⽰调⽤对象,即⼦进程要执⾏的任务args表⽰调⽤对象的位置参数元组,args=(1,2,'egon',)kwargs表⽰调⽤对象的字典,kwargs={'name':'egon','age':18}name为⼦进程的名称⽅法介绍p.start():启动进程,并调⽤该⼦进程中的p.run()p.run():进程启动时运⾏的⽅法,正是它去调⽤target指定的函数,我们⾃定义类的类中⼀定要实现该⽅法p.terminate():强制终⽌进程p,不会进⾏任何清理操作,如果p创建了⼦进程,该⼦进程就成了僵⼫进程,使⽤该⽅法需要特别⼩⼼这种情况。
如果p还保存了⼀个锁那么也将不会被释放,进⽽导致死锁p.is_alive():如果p仍然运⾏,返回Truep.join([timeout]):主线程等待p终⽌(强调:是主线程处于等的状态,⽽p是处于运⾏的状态)。
Python基础之如何使用multiprocessing模块

Python基础之如何使⽤multiprocessing模块⼀、multiprocessing模块multiprocessing包是Python中的多进程管理包。
与threading.Thread类似,它可以使⽤multiprocessing.Proces 对象来创建⼀个进程。
该进程可以运⾏在Python程序内部编写的函数。
该Process对象与Thread对象的⽤法相同,也start(),run()的⽅法。
此外multiprocessing包中也有Lock/Event/Semaphore/Condition类(这些对象可以像多线程那样,通过参数传递给各个进程),⽤以同步进程,其⽤法与threading包中的同名类⼀致。
所以,multiprocessing的很⼤⼀部份与threading使⽤同⼀套API,只不过换到了多进程的情境。
接下来我们通过⼀个案例学习:import timeimport multiprocessingdef download () :print("开始下载⽂件...")time.sleep(1)print("完成下载⽂件...")def upload() :print("开始上传⽂件...")time.sleep(1)print("完成上传⽂件...")#download()# upload()#多进程与多线程的使⽤⽅式是差不多的download_process = multiprocessing.Process(target=download)upload_process = multiprocessing. Process(target=upload)if _name_ == '_main_':#多进程必须要在 if _name_ == "_main_”⾥⾯download_process.start()upload_process.start ()#默认情况下,主进程代码运⾏完毕之后会等待⼦进程结束print('--主进程运⾏完了---')上述代码是⼀个⾮常简单的程序,⼀旦运⾏这个程序,按照代码的执⾏顺序,download 函数执⾏完毕后才能执⾏upload 函数﹒如果可以让download和upload同时运⾏,显然执⾏这个程序的效率会⼤⼤提升。
RedHatLinux:ELSMP与EL的区别

Red Hat Linux:ELSMP与EL的区别Red Hat Linux:ELSMP与EL的区别Red Hat Linux开机的时候,GRUB的启动菜单会有两个选项,分别是:Red Hat Enterprise Linux ES (版本号.ELsmp)Red Hat Enterprise Linux ES-up (版本号.EL)会有疑问,这两个分别是代表什么含义呢?其实这个就是系统开机时由GRUB引导启动-单处理器与对称多处理器启动核心文件的区别。
Red Hat Enterprise Linux ES (版本号.ELsmp) <---- multiple processor (symmetric multiprocessing )Red Hat Enterprise Linux ES-up (版本号.EL) <---- uniprocessor下面就把SUSE与Red Hat启动菜单内可选择的选项,一一列举出来SUSE版本号-default: SUSE Linux kernel for uniprocessor machines <---- 默认选项,支持单处理器机器版本号-smp: SUSE Linux kernel that supports symmetric multiprocessing (multiple processor machines) and up to 4 GB of RAM <---- 支持4GB内存的对称多处理器机器版本号-bigsmp: SUSE Linux kernel that supports symmetric multiprocessing and up to 64 GB of RAM <---- 支持64GB内存的对称多处理器机器Red Hat Linux版本号.EL: Red Hat Linux kernel for uniprocessor machines <---- 支持单处理器机器版本号.ELhugemem: Red Hat Linux kernel that supports up to 64 GB of RAM <---- 支持64GB内存的对称多处理器机器版本号.ELsmp: Red Hat Linux kernel that supports symmetric multiprocessing (multiple processor machines) <---- 对称多处理器机器1 / 1。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
Symmetric Multiprocessing on Programmable Chips Made Easy∗Austin Hung William Bishop Andrew Kennings ahung@uwaterloo.ca wdbishop@uwaterloo.ca akennings@uwaterloo.caDepartment of Electrical and Computer EngineeringUniversity of WaterlooWaterloo,Ontario,Canada,N2L3G1.AbstractVendor-provided softcore processors often support ad-vanced features such as caching that work well in unipro-cessor or uncoupled multiprocessor architectures.How-ever,it is a challenge to implement Symmetric Multipro-cessor on a Programmable Chip(SMPoPC)systems usingsuch processors.This paper presents an implementation ofa tightly-coupled,cache-coherent symmetric multiprocess-ing architecture using a vendor-provided softcore processor.Experimental results show that this implementation can beachieved without invasive changes to the vendor-providedsoftcore processor and without degradation of the perfor-mance of the memory system.1.IntroductionAdvances in Field-Programmable Gate Array(FPGA)technologies have led to programmable devices with greaterdensity,speed and functionality.It is possible to implementa highly complex System-on-Programmable-Chip(SoPC)using on-chip FPGA resources(e.g.,DSP blocks,PLLs,RAM blocks,etc.)and vendor-provided intellectual prop-erty(IP)cores.Furthermore,it is possible to build MPoPCsystems,where the number of softcore processors(e.g.,Al-tera Nios[3]or Xilinx MicroBlaze[14])that can be used ina MPoPC system is only limited by device resources.Pre-vious research[13,8,5]investigated application-specificMPoPC systems that did not require shared memory re-sources.This paper focuses on general-purpose MPoPCsystems that utilize cache-coherent,symmetric multipro-cessing.design for a cache-coherent SMPoPC system is described in Section4.Section5describes the experimental results obtained using several cache-coherent SMPoPC systems. Conclusions and future work are presented in Section6. 2.Symmetric MultiprocessingSMP systems are a subset of multiple instruction,mul-tiple data stream parallel computer architectures[6].Each processing element independently executes instructions on its own stream of data.SMP systems can function equally well as a single-user machine focusing on a single task with high efficiency,or as a multiprogrammed machine running multiple tasks[7].SMP systems share memory resources using a shared bus as illustrated in Figure1.Figure1.Typical SMP system architectureThe symmetry aspect is three-fold:(i)all processors are functionally identical and the hierarchy isflat with no master-slave or interprocessor communication relation-ships;(ii)all processors share the same address space;(iii) all processors share access to the same I/O subsystems with interrupts available to all processors.This symmetry helps to eliminate and reduce potential bottlenecks in critical sub-systems and leads to software standardization in which sys-tem developers can produce systems with different numbers of processors that can execute the same binaries[11].2.1.Processor IdentificationSMP systems require a means to uniquely identify pro-cessors to permit selection of a bootstrap processor(BSP) for global initialization upon startup and to allow software (e.g.,an operating system)to assign processes and threads to processors.Unique identification of processors is one is-sue addressed by our system.2.2.Cache CoherencyProcessor performance can be improved via one or more levels of cache located between the processor and main memory.A processor accessing cached data does not re-quire any memory bus transactions,thereby reducing bus contention.Caches are designed to follow either a write-through or write-back policy.A write-through policy spec-ifies that a value to be stored is written into the cache and the next level of the memory hierarchy.A write-back pol-icy only writes to the next level of the memory hierarchy when the written cache line is replaced.The presence of multiple,independent processor caches in a SMPoPC system leads to a cache coherency problem. Put simply,the view of the shared memory by different pro-cessors may be different.Consider a system utilizing two processors(P1and P2)with local write-through caches(C1 and C2).When processor P1reads memory location X,the value is stored in cache C1.If processor P2subsequently writes a different value to memory location X,cache C1 will contain a stale value in memory location X.If proces-sor P1subsequently reads location X,it will retrieve old data from the local cache.[7]Two classes of protocols are often used to enforce cache coherency:snooping and directory.Snooping protocols in-volve having processor caches monitor(snoop)the mem-ory bus for writes by other processors.If the local cache contains the address being written,the cache either invali-dates the cache line or updates its contents.Directory pro-tocols use a central directory to track the status of blocks of shared memory.When a processor writes to a shared mem-ory block,it secures exclusive-write access to the block. Messages are passed to ensure that no stale memory blocks exist in the local processor caches.Neither of these two methods are appropriate for the SMPoPC system described in this paper as shown in Section3and Section4.3.The Nios Processor and Avalon BusThe SMPoPC system described in this paper is based on one or more Nios processors connected to an Avalon Bus. The Nios processor and the Avalon Bus are vendor-provided IP cores designed for rapid SoPC development.3.1.Nios Embedded Softcore ProcessorThe Nios embedded softcore processor is designed specifically for SoPC design.It features a5-stage pipeline architecture and comes in both16-and32-bit vari-ants.The memory system uses a modified Harvard mem-ory architecture with separate bus masters for data and instruction memory.Memory-mapped I/O is used to ac-cess memory and peripherals attached to an Avalon bus. The global address space is configurable at system gener-ation time.The processor can implement up to four cus-tom user instructions.Custom logic can be placed within the arithmetic logic unit to implement single or multiple cy-cle instructions.Further details are found in[3].The following Nios features are particularly relevant for this paper:(i)takes advantage of on-chip memory for caching,(ii)provides control registers for invalidating par-ticular cache lines,(iii)supports of up to64vectored excep-tions including interrupts generated by external hardware, and(iv)interfaces to an Avalon Bus.It is also important to note that the Nios was never intended for use in a cached SMP architecture.3.2.Avalon BusThe Avalon Bus is not a“traditional”bus,but rather a “switch fabric”used to interconnect processors and other devices in a Nios embedded processor system[2].The bus provides point-to-point connections and supports multiple, simultaneous bus masters[1](i.e.,there is a dedicated con-nection from each potential bus master to each slave device that it can master).Although each processor and device ap-pears to connect to a shared bus,there are no shared lines in the system.This structure is illustrated in Figure2.Figure2.Structure of an Avalon Bus moduleThe Avalon Bus effectively prevents the use of bus snooping protocols and directory protocols to implement cache coherency.A non-trivial amount of invasive hardware re-development would be necessary after system generation to monitor every set of primary bus connections,denoted by ovals in Figure2.4.Design and ImplementationWe now describe the design and implementation of a cache coherency module(CCM).The goal of the CCM is to enforce cache coherency with a minimum of alterations to existing vendor-provided IP.This requires a careful exami-nation of the Nios and the Avalon Bus to understand which features facilitate and hinder cache coherency.It is also ad-vantageous to make the process of instantiating a cache co-herent SMPoPC as seamless and transparent as possible,with little if any deviation from the existing system gen-eration procedure.4.1.Processor IdentificationThe Nios processor has a CPU ID control register that stores the version number of the Nios processor.This regis-ter is read-only so it is not well-suited for processor identifi-cation.Several solutions exist:(i)changing the value of the CPU ID control register,(ii)adding a control register to the Nios,(iii)implementing a small ROM for each processor to store a unique processor ID(PID),and(iv)implementing a custom instruction in each Nios to return a unique value. The only non-invasive solution is(iii).In addition to be-ing simple and non-invasive,this approach takes advantage of the Avalon Bus architecture to make each ROM accessi-ble to its corresponding processor.This exclusivity also al-lows the ROMs to be assigned the same address to conserve address space.4.2.ArchitectureIn the context of a SMP Nios system,traditional snoop-ing or directory protocols are not suitable for ensuring cache coherency.A directory protocol would either require a ded-icated bus or consume additional bandwidth on an already congested system bus.A directory protocol would require invasive changes to each Nios processor so that its cache could send,receive and understand directory protocol mes-sages.Such a protocol would also incur a large hardware cost in the form of the central directory.A snooping protocol would require snooping hardware to be added to monitor the caches.The Nios processor im-plements a pair of instruction and data caches with a write-through policy[4].Traditionally,cache coherency is en-forced by creating a hardware module for each cache that monitors the processor’s memory bus.This,unfortunately, is not feasible due to the point-to-point nature of the Avalon Bus.Thus,a traditional snooping protocol cannot be used.Our solution involves adding a slave peripheral to the system module to inform processors of memory writes.Im-plementing cache coherency through a slave peripheral al-lows system developers to instantiate the peripheral using the standard system generation tools.This solution is easy to implement since the Avalon Bus is well-specified.This is,in reality,a hybrid snooping protocol,that uses a cen-tralized peripheral module to snoop the bus and maintain a“directory”to enforce coherency.The module can access the relevant signals on the Avalon Bus.This solution works with standard interfaces to peripherals(e.g.,on-chip RAM, memory controller,etc.)or with special interfaces(e.g.,tri-state bridge used to communicate with off-chip SRAM and flash memories).Figure3shows the CCM in relation to a typical N-way SMP Nios system.The CCM must be able to detect writes as well as read the address bus.This allows the mod-ule to notify the processors of modified memory addresses, so that the appropriate cache line can be invalidated.The cache line must be invalidated,as opposed to updated,since the Nios only possesses the ability to invalidate particular cache lines.Invalidation is performed by writing the appro-priate address to specific control registers implemented in each Nios processor.An update policy would require inva-sive changes to the system.Figure3.SMPoPC Nios system architectureThe implementation of cache clearing through processor control registers requires that software play a role in main-taining coherency.Due to the importance of maintaining coherency,the software component was written as a high-priority interrupt service routine(ISR).The CCM,a slave peripheral,raises an interrupt to enforce cache coherency.4.3.Hardware Cache Coherency ModuleThe cache coherency module is divided into three com-ponents:a write detect component(one per memory de-vice to be monitored),a central register bank component (one per system),and an Avalon slave port component(one per processor).The write detect component is responsible for snooping a single memory bus and detecting writes.For each write that is detected,the address is stored in a FIFO queue.If one or more FIFOs contain entries,then the1-bit STATUS register is set,causing the Avalon slave ports to interrupt all of the processors.The32-bit ADDRESS regis-ter stores the current address being processed.Once each processor in the system has read ADDRESS,the next ad-dress is processed.The STATUS register is cleared once all addresses have been processed allowing all processors to resume normal execution.Finally,a1-bit CONTROL regis-ter allows the CCM module to be disabled to prevent the setting of the STATUS register.The CCM can be intro-duced into a system using the vendor-provided system gen-eration tools without user intervention.Further details,in-cluding schematics and VHDL,can be found in[9].This is a second-generation CCM design that improves perfor-mance and resolves aflaw in the original design[10,9]. 4.4.Interrupt Service RoutineThe CCM ISR was written as a“system”ISR in Nios assembly language(as opposed to a“user”ISR based on vendor-provided C/C++routines).The user ISR overhead (an additional61instructions,including30memory ac-cesses)was avoided,drastically reducing ISR latency and run-time.Our ISR is24instructions long and works by dis-abling both caches,and then individually retrieving mem-ory addresses to be invalidated from the CCM and invali-dating them.It re-enables the cache and spin locks until the STATUS register is cleared(which occurs when all proces-sors in the system have invalidated all relevant addresses). Details,including assembly code,can be found in[9].We note three inefficiencies related to this configuration. Thefirst is that all processors clear the specified cache line, whether it contains the data or not.This is a limitation of the Nios.The second is that the writing processor will clear its cache,even though it contains the true value,resulting in a cache miss on the next read,increasing latency.Finally, the system memory bus becomes a bottleneck when an in-terrupt is raised as all the processors in the system attempt to fetch the ISR code.This leads to ISR latency that scales with the number of processors since all processors must ac-knowledge the interrupt before normal execution can re-sume.This can be eliminated by duplicating ISR code in on-chip ROMs,one per processor.The Nios supports sixty-four exception vectors.Thefirst sixteen(0-15)vectors are unused or used for various high-priority system-level routines,including debugging and reg-ister window interrupts.Vector16is the highest priority ex-ception vector available for the CCM to use.The choice of this vector is acceptable since the system-level routines do not execute under normal circumstances.4.5.Software RequirementsThe software requirements for maintaining cache co-herency are simple.After initialization of each of the ap-plication processors(i.e.,processors not designated as the bootstrap processor),barrier synchronization causes the ap-plication processors(APs)to wait until global initializa-tion is complete.This is similar to the Intel SMP method of holding APs in a reset state until the bootstrap processor isfinished[11].In this case,global initialization involvesinstalling the ISR and enabling the CCM.The application or operating system is responsible for ensuring that the per-processor frame and stack pointers do not accidentally over-write other processors’private memory spaces.4.6.Memory ConsistencyMemory consistency refers to the rules that a particular system follows with respect to the ordering of memory ac-cesses(reads and writes).Defining a memory consistency model is critical to ensuring correct operation of parallel shared-memory programs.A model in which any read to a memory location returns the value stored by the most recent write operation to that location is called the strict consistency model.The sequen-tial consistency model relaxes the strict model,specifying that all memory accesses be serialized(they execute atomi-cally),and that operations from a single processor appear to execute in program order[12].This model is simple and be-haves as programmers expect.The Nios is a scalar pipelined processor that statically schedules instructions.Thus,a single processor Nios sys-tem supports strict memory consistency.The same is not true for an SMP Nios system.The cache allows a proces-sor to write a value,followed by a read to that new value, prior to write completion(i.e.,prior to all other processors invalidating their cache).This relaxation is called read-own-write-early,and is allowed by sequential consistency.An SMP Nios system follows strict program ordering since instructions are statically scheduled.Though the Nios does not execute the instruction following a write until the memory transaction is complete,this does not include any cache invalidations by other processors.The completion of the CCM ISR indicates the completion of a write,but processors continue to execute in-flight instructions while the CCM raises an interrupt.This can lead to the situation where a write to a data value occurs,followed by a write to a synchronizing variable S.If the data that is protected by S is cached and S is not,then a processor may read the new value of S,but operate on stale data in the cache.The program ordering problem can be solved one of two ways.Thefirst is by placing synchronizing writes three or more instructions after the data write(causing the synchro-nizing write to be located either as the last instruction prior to ISR execution,or after ISR execution,guaranteeing that the data cannot be accessed prior to being invalidated).The second solution is to force such data reads to bypass the cache by using the GCC volatile keyword or PFXIO assembly instruction.An SMP Nios system also makes writes appear atomic. Write atomicity has two requirements:(i)all processors see writes to the same location in the same order(write serial-ization),and(ii)a written value cannot be read by another processor unless all processors read the same value.Both conditions are satisfied by the ISR,since all processors are forced to execute it at the same time,and no read can oc-cur prior to invalidation.Since writes appear atomic,if program order can be en-forced,then the SMP Nios system appears to follow the se-quential consistency model(though this has not been for-mally verified).Furthermore,such a system supports the read-own-write-early relaxation.Alternatively,since the so-lution to the program order problem is not necessarily a de-sirable solution,a relaxed write-after-write(W AW)order-ing can be used instead of a sequential model.A program-mer can choose to use either of the two models.5.Experimental ResultsAll development and testing was performed using a Nios Development Kit,Stratix Professional Edition.This kit includes an EP1S40FPGA with41,250logic elements (LEs)and3,423,744bits of on-chip RAM.The develop-ment board includes a50MHz clock,1MB of SRAM, 8MB offlash and other resources.Software development was performed using the GCC toolset and hardware de-velopment was performed using Quartus II3.2SP2.Test-ing was conducted using1-,2-,4-and8-way SMP systems.5.1.Cache Coherency TestsTwo software tests were conducted to validate cache coherency.Thefirst test performs the default start-up ini-tialization which includes enabling interrupts and initializ-ing the cache.A single processor is arbitrarily designated as the bootstrap processor(BSP).The BSP waits until all other processors have loaded the value of a shared variable synch into their cache and have begun a busy-wait loop on synch.The BSP then performs global initialization by in-stalling the CCM ISR and enabling the CCM module.Fi-nally,the BSP writes to synch allowing all other proces-sors to begin normal execution.A system is cache coherent if the write to synch is propagated to all other processors and the other processors subsequently exit their busy-wait loops.The second test performs consecutive writes to differ-ent addresses immediately following a write to the synch variable(executing before the ISR).This test ensures that pipelined writes are handled correctly.A system is cache coherent if all of the written addresses are invalidated.Succinctly stated,the system with the CCM executed both tests correctly,whereas the system without the CCM failed both tests as expected.5.2.Device Usage and Performance AnalysisTable1outlines the usage statistics for the different sys-tems compiled into the EP1S40.Each Nios was configured with1kB of instruction and data cache.Additional slave peripherals(8-bit UART,CCM,and so forth)were also in-cluded.From Table1,the EP1S40can be populated with more than an8-way system.Only10%of the on-chip mem-ory bits are used,thus there are sufficient resources to in-crease the cache sizes to8kB.Table1.Device usage for SMPoPC systemsNios Logic Elements On-Chip MemoryUsage%(MHz)2,8616%100.16 292,9602%11,70828%69.66 8371,84010%。