同济大学 C# 数据库 SQL语句
行路难,停车难——同济大学ABC广场调研分析

幽 5停车数量分析图
行路难 、 停 车 难 的 问题 在 我 国高 校 中较 为 突 出 , 同济 大 学 A B C广 场 也 存 在 上 述 问题 。作 为 动 态 交 通 空间 ,其功能在于疏导人流 和车流;作为静态交通 空 间 , 它 承 载 着 学 院 机 动 车 、 非 机 动 车 的 停 放 ; 作 为学院的活动空 间, 它 又 需 要 考 虑 人 的 使 用 和 体验 。
行路 难 ,停车难 济大 学 A B C广场调研 分析
Di f f i c u l t s o f Wa l k i n g a n d P ar k i n g
-- ---- --- --— —
R e s e a r c h a n d A n a l y s i s t h e S t a t u s o f A B C S q u a r e i n T o n g j i U n i v e r s i t y
C s q ua r e
图 1 行 人 交 通 流 最 分 析
I Ke ) a v o r d s 1 s t a t i c t r a f i f c , d y n a mi c t r a f i c . s p a c e a n a l y s i s , s p a —
c e d e s i g n a n d s p a c e d i s t r i b u t i o n , t h i s p a p e r a n a l y z e s t h e p r o b l — e m o f p a r k i n g a n d wa l k i n g Af t e r r e v i e wi n g nd a s u mm a ri z i n g s t a t u s , t h e a r t i c l e p u t s f o r wa r d s o me f e a s i b l e p r o p o s a l s f o r AB—
同济大学简介
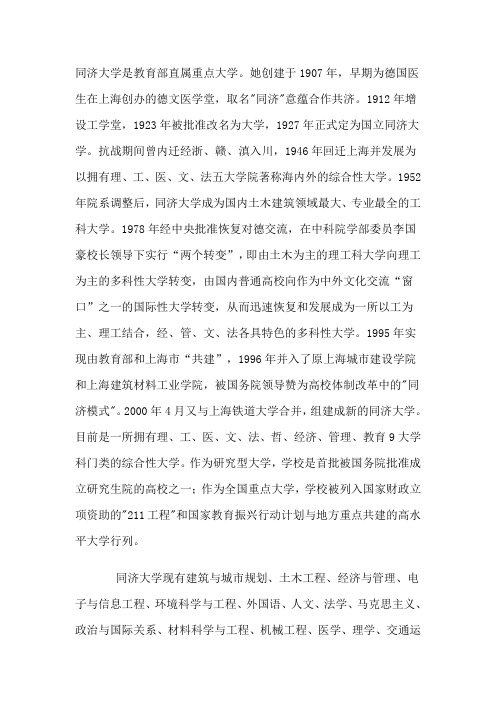
同济大学是教育部直属重点大学。
她创建于1907年,早期为德国医生在上海创办的德文医学堂,取名"同济"意蕴合作共济。
1912年增设工学堂,1923年被批准改名为大学,1927年正式定为国立同济大学。
抗战期间曾内迁经浙、赣、滇入川,1946年回迁上海并发展为以拥有理、工、医、文、法五大学院著称海内外的综合性大学。
1952年院系调整后,同济大学成为国内土木建筑领域最大、专业最全的工科大学。
1978年经中央批准恢复对德交流,在中科院学部委员李国豪校长领导下实行“两个转变”,即由土木为主的理工科大学向理工为主的多科性大学转变,由国内普通高校向作为中外文化交流“窗口”之一的国际性大学转变,从而迅速恢复和发展成为一所以工为主、理工结合,经、管、文、法各具特色的多科性大学。
1995年实现由教育部和上海市“共建”,1996年并入了原上海城市建设学院和上海建筑材料工业学院,被国务院领导赞为高校体制改革中的"同济模式"。
2000年4月又与上海铁道大学合并,组建成新的同济大学。
目前是一所拥有理、工、医、文、法、哲、经济、管理、教育9大学科门类的综合性大学。
作为研究型大学,学校是首批被国务院批准成立研究生院的高校之一;作为全国重点大学,学校被列入国家财政立项资助的"211工程"和国家教育振兴行动计划与地方重点共建的高水平大学行列。
同济大学现有建筑与城市规划、土木工程、经济与管理、电子与信息工程、环境科学与工程、外国语、人文、法学、马克思主义、政治与国际关系、材料科学与工程、机械工程、医学、理学、交通运输、汽车、海洋与地球科学、生命科学与技术、航空航天与力学、传播与艺术、设计创意、女子学院及软件学院等学院,还建有继续教育、高等技术、职业技术教育、国际文化交流、网络教育、汽车营销及电影学院等,学校还设有经中德政府批准合作培养硕士研究生的中德学院、与法国巴黎高科大学集团合作举办的中法工程和管理学院、中德工程学院、中意学院等。
中国一级学科工科分专业排行榜电气类专业排名

控制理论与控制工程(158)
排名
学校名称
等级
排名
学校名称
等级
排名
学校名称
等级
1
浙江大学
A+
12
南京理工大学
A
23
天津大学
A
2
清华大学
A+
13
哈尔滨工程大学
A
24
华北电力大学
A
3
东北大学
A+
14
大连理工大学
A
25
中国科学技术大学
A
4
上海交通大学
A+
15
燕山大学
A
26
北京交通大学
A
6
华南理工大学
A
12
西南交通大学
A
B+等(26个):武汉大学、上海海事大学、河北工业大学、大连交通大学、武汉理工大学、江苏大学、燕山大学、东南大学、湖南大学、南京理工大学、沈阳工业大学、上海大学、东北大学、辽宁工程技术大学、河海大学、江南大学、西华大学、大连海事大学、北京航空航天大学、兰州交通大学、西安电子科技大学、湖北工业大学、同济大学、中南大学、电子科技大学、东华大学
排名
学校名称
等级
排名
学校名称
等级
排名
学校名称
等级
1
上海交通大学
A+
4
东南大学
A
7
清华大学
A
2
西安电子科技大学
A+
5
电子科技大学
A
8
西安交通大学
A
3
北京邮电大学
A
6
高等数学c教材答案同济大学

高等数学c教材答案同济大学高等数学C教材答案 - 同济大学导言高等数学C是同济大学在数学系开设的一门课程,旨在帮助学生深入理解高等数学的概念、原理和应用。
本文将提供同济大学高等数学C教材的答案,以供学生参考和学习。
第一章导数与微分1.1 函数、极限与连续题目1:计算极限$\lim\limits_{x\to 2}(x^2+3x-4)$。
解答:将$x$代入函数中,得到$\lim\limits_{x\to 2}(2^2+3\cdot2-4)$,计算得$\lim\limits_{x\to 2}(4+6-4)=6$。
题目2:判断函数$f(x)=\begin{cases} x^2-1, & \text{如果 }x<0\\ 2, & \text{如果 }x=0\\ \sqrt{x}, & \text{如果 }x>0 \end{cases}$在$x=0$处是否连续。
解答:由定义,函数在$x=0$处连续,当且仅当$\lim\limits_{x\to 0^-}f(x)=f(0)=\lim\limits_{x\to 0^+}f(x)$。
代入函数并计算可得$-1=2=0$,显然不成立,因此函数在$x=0$处不连续。
1.2 导数与微分题目1:计算函数$f(x)=3x^2+5x-2$在$x=1$处的导数。
解答:根据导数的定义,函数$f(x)$在$x=1$处的导数为$f'(1)=\lim\limits_{h\to 0}\frac{f(1+h)-f(1)}{h}$。
代入函数并计算可得$f'(1)=\lim\limits_{h\to 0}\frac{3(1+h)^2+5(1+h)-2-(3-5-2)}{h}$,进一步计算可得$f'(1)=\lim\limits_{h\to 0}\frac{3h+3}{h}=3$。
题目2:判断函数$f(x)=\begin{cases} x^2, & \text{如果 }x\neq 0\\ 0,& \text{如果 }x=0 \end{cases}$在$x=0$处是否可导。
同济大学拟录博士公示

曹培会 曹祥 曹雨奇 曹湛 曾辰 曾繁兴 曾劲涛 曾鹏 曾诗扬 常生龙 常西银 晁岳栋 陈常艳 陈晨 陈法安 陈海波 陈涵子 陈鸿 陈佳琛 陈洁 陈进晓 陈菊香 陈娟 陈军宇 陈俊驰 陈开明 陈林松 陈明 陈明宽 陈南南
录取院 系所码
录取院系所名称
090 外国语学院
030 机械与能源工程学院
020 土木工程学院
陈宁 陈鹏 陈鹏 陈清勇 陈嵘 陈世培 陈帅 陈思敏 陈天懿 陈伟 陈晓东 陈晓兰 陈笑建 陈鑫 陈星 陈旭 陈尧东 陈义波 陈异凡 陈应军 陈渊文 陈蕴谷 陈志炜 陈志霞 陈钟宇 陈洲泉 陈竹书 谌衡 成国庆 成嘉琪
录取院 系所码
录取院系所名称
060 材料科学与工程学院
260 马克思主义学院
020 土木工程学院
082300 交通运输工程
100210 外科学
120100 管理科学与工程
082300 交通运输工程
085271 电子与信息
080500 材料科学与工程
080100 力学
120200 工商管理
081400 土木工程
070700 海洋科学
083000 环境科学与工程
081100 控制科学与工程
070700 海洋科学
附:2014年博士生拟录取名单(按姓名拼音排序)
考生编号
姓名
102474100009101 102474100002978 102474100009001 102474100002258 102474100001722 102474100000858 102474100009182 102474100002970 102474100009089 102474100009122 102474100000990 102474100002850 102474100002449 102474200000042 102474100001241 102474100002441
(完整版)同济大学建筑系馆C楼简析

基地分析
。
Add Your Title
—LOGO—
基地分析
ADD Your Title
Add Your Title
WPS演示助您快速创建极具感染 力的演示文稿,打造令人震撼的
影院效果。
Add Your Title
WPS演示助您快速创建极具感染 力的演示文稿,打造令人震撼的
影院效果。
Add Your Title
SU模型
ADD Your Title
Add Your Title
WPS演示助您快速创建极具感染 力的演示文稿,打造令人震撼的
影院效果。
Add Your Title
WPS演示助您快速创建极具感染 力的演示文稿,打造令人震撼的
影院效果。
Add Your Title
WPS演示助您快速创建极具感染 力的演示文稿,打造令人震撼的
水混凝土仿佛随着音符跳 动起来。
Add Your Title
WPS演示助您快速创建极具感染 力的演示文稿,打造令人震撼的
影院效果。
—LOGO—
Add Your Title
WPS演示助您快速创建极具感染 力的演示文稿,打造令人震撼的 影院效果。
居中贯穿东西的连廊系统是C 楼的核心,其中包含一部贯 穿所有工作面的的直跑楼梯, 以及一系列的上下贯通的光 井。
多远化、差异化
Thank You
2020/2/29
汇报人:余江 组员: 周振冬 张慧慧 朱坤 龙 刘振生 李艳博
—LOGO—
。
WPS演示助您快速创建极具感染
力的演示文稿,打造令人震撼的
影院效果。
—LOGO—
ADD Your Title
(完整版)同济大学建筑系馆C楼简析

Add Your Title
WPS演示助您快速创建极具感染 力的演示文稿,打造令人震撼的
影院效果。
Add Your Title
WPS演示助您快速创建极具感染 力的演示文稿,打造令人震撼的
影院效果。
Add Your Title
WPS演示助您快速创建极具感染 力的演示文稿,打造令人震撼的
影院效果。
—LOGO—
—LOGO—
形体表现弱于材质表 现
夺人眼球的新颖材料以及建筑材料的多元化也是C 楼的特色之一,为了达到空间从不透明到透明的不 同变化,建筑内部使用了大量的钢材与玻璃。支撑
结构部分主要是清水素混凝土面。
—LOGO—
对中国建筑系馆未来的展望
参
建筑系馆的大量化和群体 化
数字化教示文稿,打造令人震撼的
影院效果。
—LOGO—
ADD Your Title
Add Your Title
WPS演示助您快速创建极具感染 力的演示文稿,打造令人震撼的
影院效果。
Add Your Title
WPS演示助您快速创建极具感染 力的演示文稿,打造令人震撼的
影院效果。
Add Your Title
同济大学建筑与城市规划学 院C楼 调研报告
工程简介 位置:同济大学本部东北角 建筑面积:9672.2平方米 占地面积:1008.5平方米 用地面积:4140平方米 建筑层数:地上7层 地下一层 机房一层 结构形式:钢筋混凝土框架|钢结构 工程造价:4000万元
—LOGO—
基地分析
。
Add Your Title
影院效果。
—LOGO—
ADD Your Title
SU``
Add Your Title
高等数学c教材同济
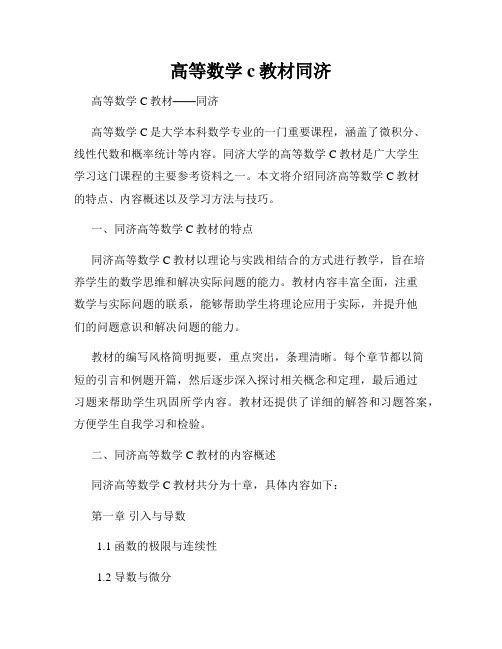
高等数学c教材同济高等数学C教材——同济高等数学C是大学本科数学专业的一门重要课程,涵盖了微积分、线性代数和概率统计等内容。
同济大学的高等数学C教材是广大学生学习这门课程的主要参考资料之一。
本文将介绍同济高等数学C教材的特点、内容概述以及学习方法与技巧。
一、同济高等数学C教材的特点同济高等数学C教材以理论与实践相结合的方式进行教学,旨在培养学生的数学思维和解决实际问题的能力。
教材内容丰富全面,注重数学与实际问题的联系,能够帮助学生将理论应用于实际,并提升他们的问题意识和解决问题的能力。
教材的编写风格简明扼要,重点突出,条理清晰。
每个章节都以简短的引言和例题开篇,然后逐步深入探讨相关概念和定理,最后通过习题来帮助学生巩固所学内容。
教材还提供了详细的解答和习题答案,方便学生自我学习和检验。
二、同济高等数学C教材的内容概述同济高等数学C教材共分为十章,具体内容如下:第一章引入与导数1.1 函数的极限与连续性1.2 导数与微分1.3 高阶导数与函数的图像1.4 泰勒公式第二章微分学应用2.1 函数的极值与最值2.2 函数的均值定理2.3 函数的凹凸性与拐点2.4 曲线的单调性与曲率第三章定积分与反常积分3.1 定积分概念与性质3.2 罗尔中值定理与柯西中值定理 3.3 反常积分第四章定积分应用4.1 物理应用题4.2 几何应用题4.3 统计应用题第五章微分方程5.1 微分方程与一阶微分方程5.2 可分离变量与线性微分方程 5.3 齐次与一致变量微分方程5.4 二阶线性微分方程第六章多元函数微分学6.1 多元函数的极限与连续性6.2 方向导数与梯度6.3 多元函数的偏导数6.4 隐函数与参数方程第七章重积分7.1 二重积分概念与性质7.2 二重积分的计算方法7.3 三重积分概念与性质7.4 三重积分的计算方法第八章空间解析几何与曲面积分 8.1 点、直线、面的方程8.2 空间曲线与曲线积分8.3 曲面与曲面积分第九章矢量场与线积分9.1 矢量场的概念与性质9.2 线积分9.3 延伸的格林公式与斯托克斯公式第十章微分方程应用10.1 包络与微分方程10.2 微分方程的模型与应用10.3 系数问题与振动问题10.4 相空间与稳定性三、学习方法与技巧学习高等数学C教材时,有几个重要的方法和技巧可以帮助我们更好地掌握知识:1. 系统学习:按照教材的章节顺序进行学习,逐步扎实地掌握每个知识点,不要跳跃学习或断章取义。
中国大学mooc《CC++程序设计(同济大学)》满分章节测试答案

titleC/C++程序设计(同济大学)中国大学mooc答案100分最新版content第1讲 C-C++程序设计入门第1讲单元测验1、以下__是C/C++合法的标识符。
A:char2B:@xC:intD:7Bw答案: char22、下面的程序,对于输入:2 9 15,输出的结果是__。
#include “iostream”using namespace std;int main(){int a;int b;cout<<“input a,b:”<<endl;cin>>a>>b;cout<<“a+b=”<<a+b<<endl;system(“pause”);return 0;=”” }=”” =”” a:输入的数据超过要求的数据,程序运行出现错误=”” b:11=”” c:26=”” d:24=”” 答案:=””<span=””>11</a+b<<endl;system(“pause”);return></endl;cin>3、在VS C++中,要在原有程序中修改程序代码应打开扩展名为__的文件。
A:objB:slnC:exeD:cpp答案: sln4、有关C语言和C++语言以下正确的说法是__。
A:C语言和C++语言都是结构化程序设计语言B:C语言和C++语言都是面向对象的程序设计语言C:C语言是结构化语言,C++语言是面向对象的语言D:C++语言是结构化语言,C语言是面向对象的语言答案: C语言是结构化语言,C++语言是面向对象的语言5、程序设计语言的发展通常被划分为三个阶段,其中不包括__。
A:高级语言B:汇编语言C:机器语言D:数据库语言答案: 数据库语言6、通过cin语句为多个变量输入数据时,不能用______分隔多个数据。
A:空格B:回车C:制表符D:逗号答案: 逗号7、 C和C++语言中不区分大小写字母。
画法几何_同济大学中国大学mooc课后章节答案期末考试题库2023年
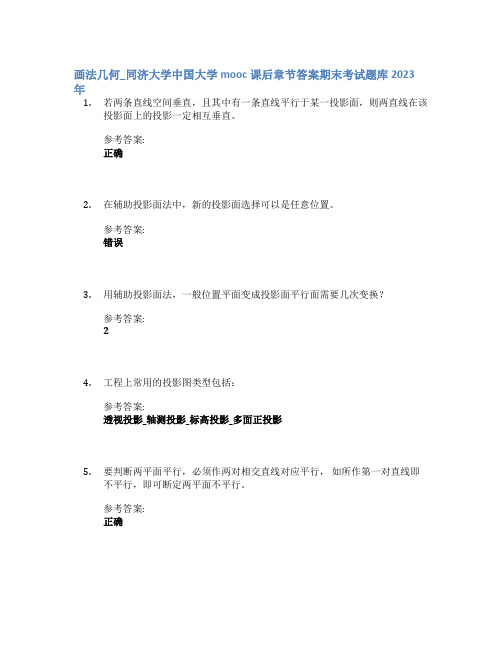
画法几何_同济大学中国大学mooc课后章节答案期末考试题库2023年1.若两条直线空间垂直,且其中有一条直线平行于某一投影面,则两直线在该投影面上的投影一定相互垂直。
参考答案:正确2.在辅助投影面法中,新的投影面选择可以是任意位置。
参考答案:错误3.用辅助投影面法,一般位置平面变成投影面平行面需要几次变换?参考答案:24.工程上常用的投影图类型包括:参考答案:透视投影_轴测投影_标高投影_多面正投影5.要判断两平面平行,必须作两对相交直线对应平行,如所作第一对直线即不平行,即可断定两平面不平行。
参考答案:正确6.平面将三棱柱截断后的截断面形状,可能为:参考答案:五边形_三角形_四边形7.的平面,其W面投影反映其实形。
参考答案:平行W面8.两平面立体相交,在全贯的情况下,应该将被贯的立体作为被动体,找出主动体的穿过被动体的棱线相贯点,即可用同面相连的方法求出交线。
参考答案:错误9.两平面相交,平面边界转折点的可见性一定相反。
参考答案:错误10.平面截切棱柱,截切方式若是全部穿透棱线,则截平面多边形的边数与棱线数相一致。
参考答案:正确11.用辅助投影面法,一般位置直线经过一次变换就可以变成投影面垂直线。
参考答案:错误12.任意位置的截平面与球面交得的截交线形状为:参考答案:圆或圆弧13.直线与平面相交,直线于交点两侧的可见性一定相反。
参考答案:正确14.两特殊位置平面相交,其交线可能是()参考答案:垂直线、平行线或一般位置线15.过一已知点可以作多少个平面垂直于一已知直线?参考答案:116.直线与平面及平面与平面相交共有___ __种情况。
参考答案:617.投影变换的目的是什么?参考答案:有利于图示和图解18.一直线垂直于平面中的任意一条H面平行线,则直线就垂直于该平面。
参考答案:错误19.点的Y坐标,即点到的距离。
参考答案:V面20.投影面上的特殊点,有两个坐标值为0。
参考答案:错误21.圆、椭圆、抛物线、双曲线都可以从正圆锥面上截得,因此它们统称为圆锥曲线。
同济-杭州市市民中心 C楼 C-000(C楼目录)

同济大学 高等数学C 教材

同济大学高等数学C 教材高等数学C教材是同济大学为理工科相关专业学生编写的一本重要教材。
它涵盖了许多数学的重要概念、定理和方法,帮助学生建立起扎实的数学基础,为他们未来的学习和研究打下坚实的基础。
第一章导数与微分高等数学C教材的第一章主要讲述了导数与微分的概念与性质。
在这一章中,学生将学习如何计算函数的导数,以及导数在几何和物理问题中的应用。
通过学习导数的性质,学生将掌握函数的极值、凹凸性以及函数图像的性质等重要概念。
第二章不定积分第二章主要介绍了不定积分的基本概念和计算方法。
学生将学习如何求出函数的不定积分,并了解积分的线性性质和曲线下面积的计算方法。
此外,该章还会讨论反常积分以及更高级的积分方法,如分部积分和换元积分等。
第三章定积分与其应用第三章主要讲述了定积分的概念与性质。
学生将学习如何计算函数在给定区间上的定积分,并了解定积分的几何和物理应用。
在该章中,学生将遇到求曲线长度、曲线面积和旋转体体积等问题,并学会通过定积分解决这些实际问题。
第四章微分方程第四章介绍了微分方程的基本理论和解法。
学生将学习如何求解一阶和二阶常微分方程,并了解微分方程在自然科学和工程科学中的广泛应用。
此外,该章还涵盖了一些重要的高阶微分方程及其特殊解法。
第五章无穷级数第五章着重讲述了无穷级数的定义和性质。
学生将学习如何判断级数的敛散性,以及如何计算常见级数的和。
此外,该章还讨论了幂级数的性质以及如何利用幂级数求解常微分方程的解。
第六章空间解析几何与向量代数第六章主要介绍了三维空间解析几何和向量代数的基本概念和方法。
学生将学习如何计算向量的模、方向和数量积,并了解向量在平面和空间几何问题中的应用。
此外,该章还会介绍向量的叉乘、混合积以及直线和平面的方程和性质等内容。
第七章多元函数微分学第七章讲述了多元函数的导数和微分。
学生将学习如何计算多元函数的偏导数以及全微分,并了解多元函数的极值和条件极值的判定方法。
此外,该章还讨论了多元函数的隐函数和参数方程,以及二重积分的计算方法。
同济大学2019-2020学年第一学期C 语言程序设计试题及答案(三)

同济大学2019-2020学年第一学期C 语言程序设计试题及答案(三)一、单项选择题(20 分,每题 2 分)1.执行下列程序段后,正确的结果是(B)int k, a[3][3] = {1,2,3,4,5,6,7,8,9};for(k=0; k<3; k++)printf(“%2d”, a[k][k]);A) 1 2 3 B) 1 5 9 C) 1 4 7 D) 3 6 92.若a 是int 类型变量,则计算表达式a=25/3%3 的值是:(B)A)3 B)2 C)1 D)03.下面正确的字符常量是:(C)A)“c”B)‘\\’’ C)‘W’ D)‘’4. C 语言中,运算对象必须是整型的运算符是:(B)A)% B)/ C)* D)+5.数字字符0 的ASCII 值为48,若有以下程序main(){char a='1', b='2';printf("%c,",b++);printf("%d\n",b-a);}程序运行后的输出结果是。
(B)A)3,2 B)50,2 C)2,2 D)2,506.以下语句或语句组中,能正确进行字符串赋值的是。
(D)A)char *sp;*sp="right!"; B)char s[10];s="right!";C)char s[10];*s="right!"; D)char *sp="right!";7.for(i=0;i<10;i++)if(i〈=5〉break;则循环结束后i 的值为(B)A)0 B)1 C)5 D)108.执行下列程序段后,正确的结果是(C)char x[8] = {8,7,6,5,0,0}, *s;s = x+1;printf(“%d\n”, s[2]);A) n B) 0 C) 5 D) 69.C 语言规定,简单变量作为实参时,他和对应形参之间的数据传递方式是:AA)单向值传递B) 地址传递C) 相互传递D) 由用户指定方式10.设有数组定义:char str[]=”China”;则下面的输出为(C)printf(“%d”,strlen(str));A)4 B)5 C)6 D)7二、填空题(30 分,每空 2 分)1.下列程序段的输出结果是3 。
A楼·2楼·C楼同济校园新建筑评述

A楼·B楼·C楼——同济校园新建筑评述BuildingA,BandC:NewBuildingsinTongjiCampus支文军宋丹峰ZHIWen-jun,SONGDan-feng摘要同济大学校园在几十年发展过程中出现了各种形式的个性建筑。
从20世纪50年代的文远楼到80年代的建筑城规学院明成楼再到2l世纪的建筑城规学院新楼,代表了三个历史时期的建筑特色.并共同坚持着同一种精神.那就是勇于创新、紧跟潮流的时代精神。
关键词同济大学校园建筑特色个性创新时代精神务实作风ABSTRACTTherehasbeenbuildingswithvariousindividualcharacteristicsappearedinTongjicampusinthepastdecades.BuildingA,BuildingBandBuildingCbuildingsfOrtheCollegeofArchitectureandUrbanPlanningcompletedrespectivelyin950s,I980sand2004,representingdisversecharacteristicsofthreehistoricalperiods.butpersistedinthesamespiritwhichistobringforthnewideasandtokeepabreastwiththetrendsofthetimesKEYWORDSTongiUniversity,CampusBuilding,Characteristic.Individual,Innovation,SpiritoftheTimes,PragmaticAttitude中图分类号:TU一86(25I):TU244.3(251)文献标识码:A文章编号:l005—684X(2004)06—0044—08坐落于同济大学四平路校园I东北角的建筑城规学院新楼(2004年建成)的出现,无疑为平静的校园掀起~阵小小的波澜。
上海同济大学专业排名「最新」

上海同济大学专业排名「最新」2017上海同济大学专业排名「最新」同济大学现有13个学院,拥有理、工、文、法、医、管、经、教育等八大学科门类,10名两院院士,长江学者数位居全国第7位,科研经费第8位。
其中建筑、土木、交通、环境、海洋、汽车等专业为国内领先。
:第一档:(1)建筑系:同济大学最具盛名的专业,实力很强,培养了很多有名的校友(不仅有著名的建筑师,也有歌唱家、天文学家、计算机专家、艺术家、导演、摄影家、作家、企业家),分配形势好,发展势头也很猛。
与清华、东南(最近东南建筑系被南大挖了墙角,实力大损)并为三大名校。
建筑系的考分很高,尤其是在外地,也是同济少数收到过高考状元的系。
50多年来基本上是同济最难考的专业,应具有考清华一般专业的分数才能进。
学本专业要够酷够眩,及足够的钱。
考分等级“高”。
(2)规划系:中国的男拨万,实力不容质疑,分配形势也不错,硕士生年收入基本在10万以上老师年收入在百万以上的也比比皆是,不信只要看看建筑成规学院门口停满的私家车。
考分评级“上”。
(3)土木系:同济大学最具实力的王牌系,包含了同济原工民建、桥梁、地下、岩土、力学等众多优势专业,其整体实力应在清华之上,尤其在桥梁、地下建筑、岩土、钢结构结构工程、抗震防灾等方面已具有国际影响。
该系是同济学风最好的,刻苦勤奋,成材率高,培养了很多英才。
就业前景不错,考分评级“中上”。
土木是学院,不是系(4)海洋系:同济的后起之秀,教师实力非常强大,在海洋地质、地球物理、海洋古生物、地震探测研究等是国内最好的,并具有世界影响力,也是学校、上海市重点发展的对象,近年为同济挣得很多荣誉。
专业未来前景很好,但要耐住目前的寂寞。
考分等级“下”。
海洋学院,属于基础学科(5)汽车系:也是同济的后起之秀,目前总体实力在清华、吉大之后,能居第3位,但发展势头很好,有赶超清华的势头。
学风很好,有双外语优势,及和德国的紧密联系,及上海汽车工业发展的依托,学校也十分重视。
同济大学C楼

总体布局
C楼西侧紧邻城规学院老楼,并作为其扩建部分,供研究 生教学使用。南侧为能源楼,东侧为苗圃,北侧紧靠校园围墙, 因无道路进入,一直是校园中“隐匿”的角落,学院的扩张让 他进入了我们的视线。总建筑面积仅10000平面,建筑师张斌 对他特殊性与潜力进行开发,重新建立他与学校整体环境的关 系。
整体环境
• 室外庭院与 室内中庭的 整块透明玻璃
东立面
西立面
庭院
• C楼内部空间设计的一个亮点就是地下一层,三层的通高内庭和七层的室外 观景平台,不仅将内部空间分割化,还为教师与学生提供很好的交流休息的 场所。
直跑楼梯
• 进入C楼内部,从二层开始的直跑楼梯贯穿整个工作楼面,使空间连 续而又彼此分割,而一系列上下通光的光井,充足得有些肆意的天光 和连续弥漫的空间使他成为所有师生的交往场所。
总建筑面积仅10000平面建筑师张斌对他特殊性与潜力进行开发重新建立他与学校整体环境的关整体环境北侧的围墙新楼的核心是居中贯穿的连廊系统其中包含了一部贯穿所有工作楼面的直跑楼梯以及一系列上下贯穿的光景充足的天光和连续的空间使它成为所有师生的交往场所一个充满不连续情节的随机空间它容纳了所有的可能
同济大学C楼
印花清玻
二、为了在仅有1万平面的土地创造出功能合理,体型优美的建筑,
一定不能让建筑对道路有过多的压迫感,因此建筑师通过下沉庭院和 将一、二层退后来减小建筑物的体量感,从三层开始才扩大建筑边线, 不仅解决了用地紧张的问题,还很自然的带来了凹凸有致的立面造型。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
SQL search testing--在选课表tblSelectCourse中查询成绩大于80分的学生选课信息:select * from tblSelectCoursewhere grade > 80--在表tblCourse中查询课程名中含有‘数学’的课程号和课程名select courseNo,courseNamefrom tblCoursewhere courseName like '%数学%'--在表tblStudent中查询生日在1989-01-01到1991-12-31之间的学生的学号,姓名select studentNo,studentNamefrom tblStudentwhere birthday between '1989-01-01' and '1991-12-31'--在表tblSelectCourse中列出旷考学生的学号和课号。
select studentNo,courseNofrom tblSelectCoursewhere grade is null-- is null和= null的区别--查询表tblStudent 列出每个同学的姓名、年龄select studentName, year(getdate())-year(birthday) as '年龄'from tblStudent--对选课表中数据,按J01课程成绩降序排列,输出学号和成绩。
select studentNo,gradefrom tblSelectCoursewhere courseNo='J01'order by grade desc--查找“数值算法”课程成绩在75分以上的学生的姓名、学号、以及具体成绩。
select s.studentName,s.studentNo,gradefrom tblCourse c,tblSelectCourse sc, tblStudent swhere s.studentNo=sc.studentNo and c.courseNo=sc.courseNo and courseName='数值算法' and grade>75--查找“数值算法”课程成绩在75分以上的学生的姓名、学号、以及具体成绩。
SELECT s.studentNo, studentName, gradeFROM tblStudent s JOIN tblSelectCourse scON s.studentNo=sc.studentNoJOIN tblCourse c ON c.courseNo=sc.courseNo AND courseName= '数值算法'AND grade>75--查找既选修了“J01”,又选修了“J04”课程的学生学号和成绩。
select a.studentNo,a.grade as'gradeJ01',b.grade as'gradeJ04'from tblSelectCourse a,tblSelectCourse bwhere a.courseNo='J01' and b.courseNo='J04' and a.studentNo=b.studentNo--找出选修了”数值算法”的学生姓名。
select studentNamefrom tblStudent s,tblSelectCourse sc,tblCourse cwhere s.studentNo=sc.studentNo and c.courseNo=sc.courseNo and c.courseName='数值算法' select studentName from tblStudentwhere studentNo in(select studentNo from tblSelectCourse sc,tblCourse cwhere sc.courseNo=c.courseNo and courseName='数值算法')--查询课程总分大于260分的学生学号、姓名和总分select s.studentNo as '学号',studentName as '姓名',sum(grade) as '总分'from tblCourse c,tblSelectCourse sc,tblStudent swhere s.studentNo=sc.studentNo and c.courseNo=sc.courseNogroup by studentName,s.studentNohaving sum(grade)>260--列出选修三门及三门以上课程的学生学号、姓名、课程数,并按学号排序select s.studentNo as '学号' ,studentName as '姓名' ,count(sc.courseNo) as '课程数'from tblCourse c,tblSelectCourse sc,tblStudent swhere c.courseNo=sc.courseNo and s.studentNo=sc.studentNogroup by s.studentNo,studentNamehaving count(sc.courseNo)>=3order by s.studentNo--向下表插入一个新同学:030101,张三,男,1982-12-20。
tblStudent(studentNo,studentName,birthday,sex)insert into tblStudentvalues ('010044','武伟','1982-12-20','男')--将学号为' 010001 '的学生的' J01 '课程成绩+5分update tblSelectCourse set grade=grade-5where studentNo='010001' and courseNo='J01'select * from tblSelectCourse--将学号为' 010001 '的学生的'操作系统'课程成绩加5分update tblSelectCourse set grade=grade-5where studentNo='010001' and courseNo=(select courseNo from tblCourse where courseName='操作系统')select * from tblSelectCourse--从表tblStudent中删除有课程成绩不及格的学生记录。
delete from tblStudentwhere studentNo in(select studentNo from tblSelectCourse where grade <60)--查询所有女生的姓名、年龄,并分别以字段名姓名、年龄显示。
select studentName as'姓名', year(getdate())-year(birthday) as'年龄'from tblStudentwhere sex='女'--查询所有03级90后的学生信息。
select *from tblStudentwhere studentNo like'03%'--查询“数据库”成绩为优的学生的姓名、学号、性别、成绩。
select studentName,s.studentNo,sex,gradefrom tblStudent s,tblSelectCourse sc,tblCourse cwhere s.studentNo=sc.studentNo and c.courseNo=sc.courseNo and courseName='数据库' and grade>=90--查询没选课的学生姓名和学号。
提示:子查询结果参与not in 运算select studentName as'姓名',studentNo as'学号'from tblStudentwhere studentNo not in(select studentNo from tblSelectCourse)--查询各年级学生数,并显示年级、人数。
提示:按年级分组,即group by substring(studentNo,1,2) select substring(studentNo,1,2) as '年级',count(*)as'人数'from tblStudentgroup by substring(studentNo,1,2)--查询最低分大于80分的学生学号、姓名、平均分。
select s.studentNo as'学号',studentName as'姓名',avg(grade) as'平均分'from tblStudent s,tblSelectCourse sc,tblCourse cwhere s.studentNo=sc.studentNo and sc.courseNo=c.courseNogroup by s.studentNo,studentNamehaving avg(grade)>80--在学生选课表中增加“020031”课程“J04”成绩为80分这样一条记录。
*****(有问题)insert into tblSelectCoursevalues('020041','J04','80')select * from tblSelectCourse--将学生的“J04”课程的成绩提高5%(原96分以上的不变)--删除tblSelectCourse表中选修了“数值算法”的选课记录。
--删除所有01级学生的记录。