calloc(), malloc(), realloc(), free(),alloca()区别
c语言知识点大纲

c语言知识点大纲以下是C语言的知识点大纲,适用于初学者和中级水平的学习者:基础概念1.数据类型:整型、浮点型、字符型等2.变量和常量:声明、定义、初始化3.运算符:算术、关系、逻辑、赋值等4.控制流:条件语句(if-else)、循环语句(for、while、do-while)5.函数:声明、定义、调用、参数传递、递归6.数组:声明、初始化、访问、多维数组、数组与指针关系7.指针:地址、指针变量、指针运算、指针和数组、指针和函数8.字符串:字符数组、字符串处理函数、字符串常用操作9.结构体:定义、访问结构成员、结构体数组、结构体指针高级概念10.内存管理:动态内存分配((malloc、calloc、realloc、free)、内存泄漏和内存错误11.文件操作:文件读写、文件指针、文件操作函数12.预处理器:宏定义、条件编译、头文件13.位运算:位操作、位掩码、位运算符14.函数指针:指向函数的指针、回调函数高级主题15.数据结构:链表、栈、队列、树、图等16.算法:排序算法((冒泡排序、快速排序等)、搜索算法((线性搜索、二分搜索等)17.指针与内存:内存布局、内存对齐、指针算术18.多线程编程:线程、同步与互斥、线程安全性19.网络编程:Socket编程、TCP/IP、HTTP协议20.C标准库函数:常用函数库((stdio.h、stdlib.h、string.h(等)实践和应用21.项目开发:使用C语言构建小型项目或工具22.调试和优化:学习调试技巧、代码优化技巧以上列举的知识点可以帮助你建立起对C语言的基础认识并逐渐深入。
实践是掌握编程语言的关键,因此建议在学习过程中不断地练习并尝试编写各种类型的程序,以加深对C语言的理解和掌握。
c语言释放内存的函数
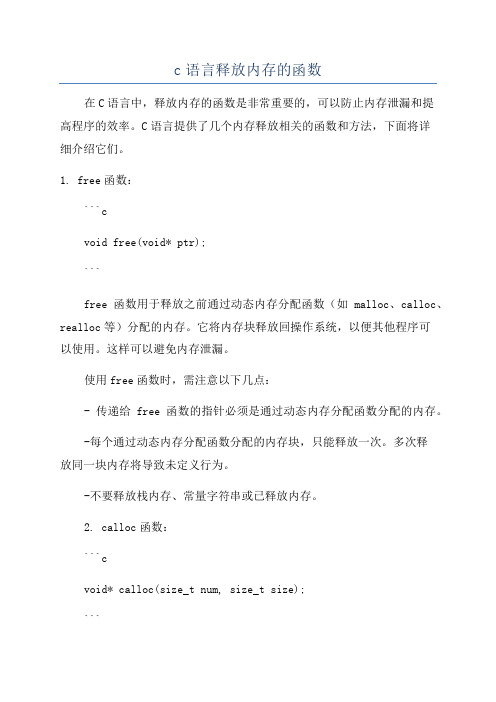
c语言释放内存的函数在C语言中,释放内存的函数是非常重要的,可以防止内存泄漏和提高程序的效率。
C语言提供了几个内存释放相关的函数和方法,下面将详细介绍它们。
1. free函数:```cvoid free(void* ptr);```free函数用于释放之前通过动态内存分配函数(如malloc、calloc、realloc等)分配的内存。
它将内存块释放回操作系统,以便其他程序可以使用。
这样可以避免内存泄漏。
使用free函数时,需注意以下几点:- 传递给free函数的指针必须是通过动态内存分配函数分配的内存。
-每个通过动态内存分配函数分配的内存块,只能释放一次。
多次释放同一块内存将导致未定义行为。
-不要释放栈内存、常量字符串或已释放内存。
2. calloc函数:```cvoid* calloc(size_t num, size_t size);```calloc函数用于分配一个指定类型和大小的连续内存块,并将其初始化为0。
它接受两个参数,num表示要分配的元素数量,size表示每个元素的大小。
如果分配成功,将返回指向分配内存的指针;否则返回NULL。
calloc函数使用示例:```cint* p = (int*)calloc(5, sizeof(int));if (p != NULL)//内存分配成功,可以使用指针p指向的内存} else//内存分配失败```3. malloc函数:```cvoid* malloc(size_t size);```malloc函数用于分配指定大小的连续内存块,但不会进行初始化。
它接受一个参数size,表示要分配的字节数。
如果分配成功,将返回指向分配内存的指针;否则返回NULL。
malloc函数使用示例:```cint* p = (int*)malloc(5 * sizeof(int));if (p != NULL)//内存分配成功,可以使用指针p指向的内存} else//内存分配失败```4. realloc函数:```cvoid* realloc(void* ptr, size_t size);```realloc函数用于重新分配之前通过动态内存分配函数分配的内存块的大小。
C语言常用数学函数

C语言常用数学函数1.数学函数:如sqrt、sin、cos、tan、exp、log等,可用于处理数学运算。
比如:x表示需要计算平方根的数值。
sqrt函数会返回x的正平方根,如果x是负数,则返回NaN(Not a Number)。
exp用于计算一个数的自然指数值(以e为底的指数)。
log函数返回x的自然对数,即ln(x)。
Doubl esqrt(doublex);2.字符串函数:如strcpy、strncpy、strcat、strcmp、strlen等,用于处理字符串。
strcpy用于将一个字符串复制到另一个字符串中。
dest表示目标字符串的起始地址,src表示源字符串的起始地址。
当使用strcpy函数进行字符串复制时,必须确保目标字符串空间足够大,否则可能会导致内存访问越界等问题。
char*strcpy(char*dest,constchar*src);strncpy用于将一个字符串的部分内容复制到另一个字符串中。
,n表示需要复制的字符个数。
如果源字符串长度小于n,则目标字符串将被填充一些空字符直到长度为n。
char*strncpy(char*dest,constchar*src,size_tn);strcat用于将一个字符串连接到另一个字符串的末尾。
strcmp用于比较两个字符串的大小关系。
strlen用于计算一个字符串的长度(即包含多少个字符)。
注意的是,strlen函数不会计算空字符'\0'的长度。
3.文件操作函数:如fopen、fclose、fread、fwrite、fgets 等,可用于文件的读取和写入。
4.内存函数:如malloc、calloc、realloc、free等,用于动态内存分配和释放。
malloc用于动态分配内存空间。
free用于释放动态分配的内存空间。
5.格式化函数:如printf、scanf、sprintf、sscanf等,用于输入输出和格式化字符串。
C++辅导:内存分配和释放
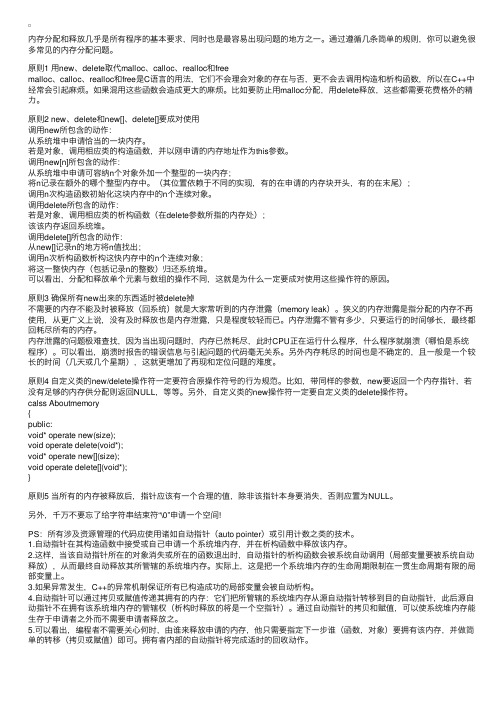
内存分配和释放⼏乎是所有程序的基本要求,同时也是最容易出现问题的地⽅之⼀。
通过遵循⼏条简单的规则,你可以避免很多常见的内存分配问题。
原则1 ⽤new、delete取代malloc、calloc、realloc和freemalloc、calloc、realloc和free是C语⾔的⽤法,它们不会理会对象的存在与否,更不会去调⽤构造和析构函数,所以在C++中经常会引起⿇烦。
如果混⽤这些函数会造成更⼤的⿇烦。
⽐如要防⽌⽤malloc分配,⽤delete释放,这些都需要花费格外的精⼒。
原则2 new、delete和new[]、delete[]要成对使⽤调⽤new所包含的动作:从系统堆中申请恰当的⼀块内存。
若是对象,调⽤相应类的构造函数,并以刚申请的内存地址作为this参数。
调⽤new[n]所包含的动作:从系统堆中申请可容纳n个对象外加⼀个整型的⼀块内存;将n记录在额外的哪个整型内存中。
(其位置依赖于不同的实现,有的在申请的内存块开头,有的在末尾);调⽤n次构造函数初始化这块内存中的n个连续对象。
调⽤delete所包含的动作:若是对象,调⽤相应类的析构函数(在delete参数所指的内存处);该该内存返回系统堆。
调⽤delete[]所包含的动作:从new[]记录n的地⽅将n值找出;调⽤n次析构函数析构这快内存中的n个连续对象;将这⼀整快内存(包括记录n的整数)归还系统堆。
可以看出,分配和释放单个元素与数组的操作不同,这就是为什么⼀定要成对使⽤这些操作符的原因。
原则3 确保所有new出来的东西适时被delete掉不需要的内存不能及时被释放(回系统)就是⼤家常听到的内存泄露(memory leak)。
狭义的内存泄露是指分配的内存不再使⽤,从更⼴义上说,没有及时释放也是内存泄露,只是程度较轻⽽已。
内存泄露不管有多少,只要运⾏的时间够长,最终都回耗尽所有的内存。
内存泄露的问题极难查找,因为当出现问题时,内存已然耗尽,此时CPU正在运⾏什么程序,什么程序就崩溃(哪怕是系统程序)。
c语言_头文件_stdlib
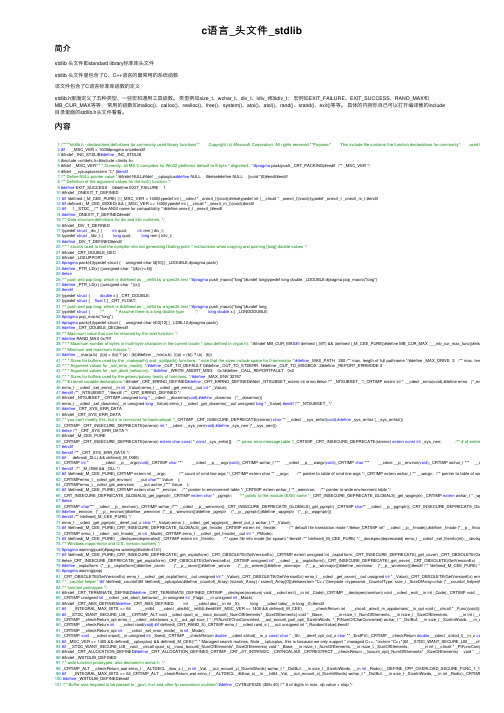
c语⾔_头⽂件_stdlib简介stdlib 头⽂件即standard library标准库头⽂件stdlib 头⽂件⾥包含了C、C++语⾔的最常⽤的系统函数该⽂件包含了C语⾔标准库函数的定义stdlib.h⾥⾯定义了五种类型、⼀些宏和通⽤⼯具函数。
类型例如size_t、wchar_t、div_t、ldiv_t和lldiv_t;宏例如EXIT_FAILURE、EXIT_SUCCESS、RAND_MAX和MB_CUR_MAX等等;常⽤的函数如malloc()、calloc()、realloc()、free()、system()、atoi()、atol()、rand()、srand()、exit()等等。
具体的内容你⾃⼰可以打开编译器的include⽬录⾥⾯的stdlib.h头⽂件看看。
内容1/*****stdlib.h - declarations/definitions for commonly used library functions** Copyright (c) Microsoft Corporation. All rights reserved.**Purpose:* This include file contains the function declarations for commonly* used library functio 2#if _MSC_VER > 1000#pragma once#endif3 #ifndef _INC_STDLIB#define _INC_STDLIB4 #include <crtdefs.h>#include <limits.h>5 #ifdef _MSC_VER/** * Currently, all MS C compilers for Win32 platforms default to 8 byte * alignment. */#pragma pack(push,_CRT_PACKING)#endif /** _MSC_VER */6 #ifdef __cplusplusextern "C" {#endif7/** Define NULL pointer value */#ifndef NULL#ifdef __cplusplus#define NULL 0#else#define NULL ((void *)0)#endif#endif8/** Definition of the argument values for the exit() function */9#define EXIT_SUCCESS 0#define EXIT_FAILURE 110 #ifndef _ONEXIT_T_DEFINED11#if !defined (_M_CEE_PURE) || (_MSC_VER < 1400)typedef int (__cdecl * _onexit_t)(void);#elsetypedef int (__clrcall * _onexit_t)(void);typedef _onexit_t _onexit_m_t;#endif12#if defined (_M_CEE_MIXED) && (_MSC_VER >= 1400)typedef int (__clrcall * _onexit_m_t)(void);#endif13#if !__STDC__/** Non-ANSI name for compatibility */#define onexit_t _onexit_t#endif14#define _ONEXIT_T_DEFINED#endif15/** Data structure definitions for div and ldiv runtimes. */16 #ifndef _DIV_T_DEFINED17 typedef struct _div_t { int quot; int rem;} div_t;18 typedef struct _ldiv_t { long quot; long rem;} ldiv_t;19#define _DIV_T_DEFINED#endif20/** * structs used to fool the compiler into not generating floating point * instructions when copying and pushing [long] double values */21 #ifndef _CRT_DOUBLE_DEC22 #ifndef _LDSUPPORT23#pragma pack(4)typedef struct { unsigned char ld[10];} _LDOUBLE;#pragma pack()24#define _PTR_LD(x) ((unsigned char *)(&(x)->ld))25#else26/** push and pop long, which is #defined as __int64 by a spec2k test */#pragma push_macro("long")#undef longtypedef long double _LDOUBLE;#pragma pop_macro("long")27#define _PTR_LD(x) ((unsigned char *)(x))28#endif29 typedef struct { double x;} _CRT_DOUBLE;30 typedef struct { float f;} _CRT_FLOAT;31/** push and pop long, which is #defined as __int64 by a spec2k test */#pragma push_macro("long")#undef long32 typedef struct { /** * Assume there is a long double type */long double x;} _LONGDOUBLE;33#pragma pop_macro("long")34#pragma pack(4)typedef struct { unsigned char ld12[12];} _LDBL12;#pragma pack()35#define _CRT_DOUBLE_DEC#endif36/** Maximum value that can be returned by the rand function. */37#define RAND_MAX 0x7fff38/** * Maximum number of bytes in multi-byte character in the current locale * (also defined in ctype.h). */#ifndef MB_CUR_MAX#if defined (_MT) && (defined (_M_CEE_PURE))#define MB_CUR_MAX ___mb_cur_max_func()#else#define MB_ 39/** Minimum and maximum macros */40#define __max(a,b) (((a) > (b)) ? (a) : (b))#define __min(a,b) (((a) < (b)) ? (a) : (b))41/** * Sizes for buffers used by the _makepath() and _splitpath() functions. * note that the sizes include space for 0-terminator */#define _MAX_PATH 260 /** max. length of full pathname */#define _MAX_DRIVE 3 /** max. length of drive c 42/** * Argument values for _set_error_mode(). */#define _OUT_TO_DEFAULT 0#define _OUT_TO_STDERR 1#define _OUT_TO_MSGBOX 2#define _REPORT_ERRMODE 343/** * Argument values for _set_abort_behavior(). */#define _WRITE_ABORT_MSG 0x1#define _CALL_REPORTFAULT 0x244/** * Sizes for buffers used by the getenv/putenv family of functions. */#define _MAX_ENV 3276745/** External variable declarations */#ifndef _CRT_ERRNO_DEFINED#define _CRT_ERRNO_DEFINED#ifdef _NTSUBSET_extern int errno;#else /** _NTSUBSET_ */_CRTIMP extern int * __cdecl _errno(void);#define errno (*_errno())46 errno_t __cdecl _set_errno(__in int _Value);errno_t __cdecl _get_errno(__out int * _Value);47#endif /** _NTSUBSET_ */#endif /** _CRT_ERRNO_DEFINED */48 #ifndef _NTSUBSET__CRTIMP unsigned long * __cdecl __doserrno(void);#define _doserrno (*__doserrno())49 errno_t __cdecl _set_doserrno(__in unsigned long _Value);errno_t __cdecl _get_doserrno(__out unsigned long * _Value);#endif /** _NTSUBSET_ */50#define _CRT_SYS_ERR_DATA51 #ifndef _CRT_SYS_ERR_DATA52/** you can't modify this, but it is non-const for backcompat */_CRTIMP _CRT_INSECURE_DEPRECATE(strerror) char ** __cdecl __sys_errlist(void);#define _sys_errlist (__sys_errlist())53 _CRTIMP _CRT_INSECURE_DEPRECATE(strerror) int * __cdecl __sys_nerr(void);#define _sys_nerr (*__sys_nerr())54#else /** _CRT_SYS_ERR_DATA */55 #ifndef _M_CEE_PURE56 _CRTIMP _CRT_INSECURE_DEPRECATE(strerror) extern char const * const _sys_errlist[]; /** perror error message table */_CRTIMP _CRT_INSECURE_DEPRECATE(strerror) extern const int _sys_nerr; /** # of entries in sys_errlis 57#endif58#endif /** _CRT_SYS_ERR_DATA */59#if defined(_DLL) && defined(_M_IX86)60 _CRTIMP int * __cdecl __p___argc(void);_CRTIMP char *** __cdecl __p___argv(void);_CRTIMP wchar_t *** __cdecl __p___wargv(void);_CRTIMP char *** __cdecl __p__environ(void);_CRTIMP wchar_t *** __cdecl __p__w 61#endif /** _M_IX86 && _DLL */62#if !defined(_M_CEE_PURE)_CRTIMP extern int __argc; /** count of cmd line args */_CRTIMP extern char ** __argv; /** pointer to table of cmd line args */_CRTIMP extern wchar_t ** __wargv; /** pointer to table of wide cmd line ar63 _CRTIMPerrno_t__cdecl_get_environ( __out char*** Value );64 _CRTIMPerrno_t__cdecl_get_wenviron( __out wchar_t*** Value );65#if !defined(_M_CEE_PURE)_CRTIMP extern char ** _environ; /** pointer to environment table */_CRTIMP extern wchar_t ** _wenviron; /** pointer to wide environment table */66 _CRT_INSECURE_DEPRECATE_GLOBALS(_get_pgmptr) _CRTIMP extern char * _pgmptr; /** points to the module (EXE) name */_CRT_INSECURE_DEPRECATE_GLOBALS(_get_wpgmptr) _CRTIMP extern wchar_t * _wpgmptr;67#else68 _CRTIMP char*** __cdecl __p__environ();_CRTIMP wchar_t*** __cdecl __p__wenviron();_CRT_INSECURE_DEPRECATE_GLOBALS(_get_pgmptr) _CRTIMP char** __cdecl __p__pgmptr();_CRT_INSECURE_DEPRECATE_GLOBALS(_get_ 69#define _environ (*__p__environ())#define _wenviron (*__p__wenviron())#define _pgmptr (*__p__pgmptr())#define _wpgmptr (*__p__wpgmptr())70#endif /** !defined(_M_CEE_PURE) */71 errno_t __cdecl _get_pgmptr(__deref_out_z char ** _Value);errno_t __cdecl _get_wpgmptr(__deref_out_z wchar_t ** _Value);72#if !defined(_M_CEE_PURE)_CRT_INSECURE_DEPRECATE_GLOBALS(_get_fmode) _CRTIMP extern int _fmode; /** default file translation mode */#else_CRTIMP int* __cdecl __p__fmode();#define _fmode (*__p__fmode())#endif /** 73 _CRTIMP errno_t __cdecl _set_fmode(__in int _Mode);_CRTIMP errno_t __cdecl _get_fmode(__out int * _PMode);74#if !defined(_M_CEE_PURE) __declspec(deprecated) _CRTIMP extern int _fileinfo; /** open file info mode (for spawn) */#endif /** !defined(_M_CEE_PURE) */__declspec(deprecated) errno_t __cdecl _set_fileinfo(int);__declspec(deprecate 75/** Windows major/minor and O.S. version numbers */76#pragma warning(push)#pragma warning(disable:4141)77#if !defined(_M_CEE_PURE)_CRT_INSECURE_DEPRECATE(_get_osplatform) _CRT_OBSOLETE(GetVersionEx) _CRTIMP extern unsigned int _osplatform;_CRT_INSECURE_DEPRECATE(_get_osver) _CRT_OBSOLETE(GetVersionEx) 78 #else_CRT_INSECURE_DEPRECATE(_get_osplatform) _CRT_OBSOLETE(GetVersionEx) _CRTIMP unsigned int* __cdecl __p__osplatform();_CRT_INSECURE_DEPRECATE(_get_osver) _CRT_OBSOLETE(GetVersionEx) _CRTIMP uns 79#define _osplatform (*__p__osplatform())#define _osver (*__p__osver())#define _winver (*__p__winver())#define _winmajor (*__p__winmajor())#define _winminor (*__p__winminor())#endif /** !defined(_M_CEE_PURE) */80#pragma warning(pop)81 _CRT_OBSOLETE(GetVersionEx) errno_t __cdecl _get_osplatform(__out unsigned int * _Value);_CRT_OBSOLETE(GetVersionEx) errno_t __cdecl _get_osver(__out unsigned int * _Value);_CRT_OBSOLETE(GetVersionEx) errno_t __cdecl _ 82/** _countof helper */#if !defined(_countof)#if !defined(__cplusplus)#define _countof(_Array) (sizeof(_Array) / sizeof(_Array[0]))#elseextern "C++"{template <typename _CountofType, size_t _SizeOfArray>char (*__countof_helper(UNALIGNED 83/** function prototypes */84 #ifndef _CRT_TERMINATE_DEFINED#define _CRT_TERMINATE_DEFINED_CRTIMP __declspec(noreturn) void __cdecl exit(__in int _Code);_CRTIMP __declspec(noreturn) void __cdecl _exit(__in int _Code);_CRTIMP void __cdecl abort(v85 _CRTIMP unsigned int __cdecl _set_abort_behavior(__in unsigned int _Flags, __in unsigned int _Mask);86 #ifndef _CRT_ABS_DEFINED#define _CRT_ABS_DEFINED int __cdecl abs(__in int _X); long __cdecl labs(__in long _X);#endif87#if _INTEGRAL_MAX_BITS >= 64 __int64 __cdecl _abs64(__int64);#endif#if _MSC_VER >= 1400 && defined(_M_CEE) __checkReturn int __clrcall _atexit_m_appdomain(__in_opt void (__clrcall * _Func)(void));#if defined(_M 88#if __STDC_WANT_SECURE_LIB___CRTIMP_ALT void __cdecl qsort_s(__inout_bcount(_NumOfElements* _SizeOfElements) void * _Base, __in rsize_t _NumOfElements, __in rsize_t _SizeOfElements, __in int (__cdecl * _PtFu89 _CRTIMP __checkReturn_opt errno_t __cdecl _mbstowcs_s_l(__out_opt size_t * _PtNumOfCharConverted, __out_ecount_part_opt(_SizeInWords, *_PtNumOfCharConverted) wchar_t * _DstBuf, __in size_t _SizeInWords, __in_ecount_z(_Ma90 _CRTIMP __checkReturn int __cdecl rand(void);#if defined(_CRT_RAND_S)_CRTIMP errno_t __cdecl rand_s ( __out unsigned int *_RandomValue);#endif91 _CRTIMP __checkReturn_opt int __cdecl _set_error_mode(__in int _Mode);92 _CRTIMP void __cdecl srand(__in unsigned int _Seed);_CRTIMP __checkReturn double __cdecl strtod(__in_z const char * _Str, __deref_opt_out_z char ** _EndPtr);_CRTIMP __checkReturn double __cdecl _strtod_l(__in_z const93#if _MSC_VER >= 1400 && defined(__cplusplus) && defined(_M_CEE)/** * Managed search routines. Note __cplusplus, this is because we only support * managed C++. */extern "C++"{#if __STDC_WANT_SECURE_LIB____checkReturn vo 94#if __STDC_WANT_SECURE_LIB__void __clrcall qsort_s(__inout_bcount(_NumOfElements*_SizeOfElements) void * _Base, __in rsize_t _NumOfElements, __in rsize_t _SizeOfElements, __in int (__clrcall * _PtFuncCompare)(void *, c95 #ifndef _CRT_ALLOCATION_DEFINED#define _CRT_ALLOCATION_DEFINED_CRTIMP _CRT_JIT_INTRINSIC _CRTNOALIAS _CRTRESTRICT __checkReturn __bcount_opt(_NumOfElements* _SizeOfElements) void * __cdecl calloc(_96 #ifndef _WSTDLIB_DEFINED97/** wide function prototypes, also declared in wchar.h */98 _CRTIMP_ALT __checkReturn_wat errno_t __ALTDECL _itow_s (__in int _Val, __out_ecount_z(_SizeInWords) wchar_t * _DstBuf, __in size_t _SizeInWords, __in int _Radix);__DEFINE_CPP_OVERLOAD_SECURE_FUNC_1_1(errno_t, _itow 99#if _INTEGRAL_MAX_BITS >= 64_CRTIMP_ALT __checkReturn_wat errno_t __ALTDECL _i64tow_s(__in __int64 _Val, __out_ecount_z(_SizeInWords) wchar_t * _DstBuf, __in size_t _SizeInWords, __in int _Radix);_CRTIMP _CRT_INSE 100#define _WSTDLIB_DEFINED#endif101/** Buffer size required to be passed to _gcvt, fcvt and other fp conversion routines*/#define _CVTBUFSIZE (309+40) /** # of digits in max. dp value + slop */102#if (defined(_DEBUG) || defined(_SYSCRT_DEBUG)) && defined(_CRTDBG_MAP_ALLOC)#pragma push_macro("_fullpath")#undef _fullpath#endif103 _CRTIMP __checkReturn char * __cdecl _fullpath(__out_ecount_z_opt(_SizeInBytes) char * _FullPath, __in_z const char * _Path, __in size_t _SizeInBytes);104#if (defined(_DEBUG) || defined(_SYSCRT_DEBUG)) && defined(_CRTDBG_MAP_ALLOC)#pragma pop_macro("_fullpath")#endif105 _CRTIMP_ALT __checkReturn_wat errno_t __cdecl _ecvt_s(__out_ecount_z(_Size) char * _DstBuf, __in size_t _Size, __in double _Val, __in int _NumOfDights, __out int * _PtDec, __out int * _PtSign);__DEFINE_CPP_OVERLOAD_SECUR 106 _CRTIMP __checkReturn int __cdecl _atodbl(__out _CRT_DOUBLE * _Result, __in_z char * _Str);_CRTIMP __checkReturn int __cdecl _atoldbl(__out _LDOUBLE * _Result, __in_z char * _Str);_CRTIMP __checkReturn int __cdecl _atoflt(__ 107#if _MSC_VER >= 1400 && defined(_M_CEE) _onexit_m_t __clrcall _onexit_m_appdomain(_onexit_m_t _Function); #if defined(_M_CEE_MIXED) _onexit_m_t __clrcall _onexit_m(_onexit_m_t _Function); #else inline _onexit_m_t __ 108 _CRT_INSECURE_DEPRECATE(_splitpath_s) _CRTIMP void __cdecl _splitpath(__in_z const char * _FullPath, __out_z_opt char * _Drive, __out_z_opt char * _Dir, __out_z_opt char * _Filename, __out_z_opt char * _Ext);_CRTIMP_ALT _ 109 _CRTIMP void __cdecl _swab(__inout_ecount_full(_SizeInBytes) char * _Buf1, __inout_ecount_full(_SizeInBytes) char * _Buf2, int _SizeInBytes);110 #ifndef _WSTDLIBP_DEFINED111/** wide function prototypes, also declared in wchar.h */112#if (defined(_DEBUG) || defined(_SYSCRT_DEBUG)) && defined(_CRTDBG_MAP_ALLOC)#pragma push_macro("_wfullpath")#undef _wfullpath#endif113 _CRTIMP __checkReturn wchar_t * __cdecl _wfullpath(__out_ecount_z_opt(_SizeInWords) wchar_t * _FullPath, __in_z const wchar_t * _Path, __in size_t _SizeInWords);114#if (defined(_DEBUG) || defined(_SYSCRT_DEBUG)) && defined(_CRTDBG_MAP_ALLOC)#pragma pop_macro("_wfullpath")#endif115 _CRTIMP_ALT __checkReturn_wat errno_t __ALTDECL _wmakepath_s(__out_ecount_z(_SizeInWords) wchar_t * _PathResult, __in_opt size_t _SizeInWords, __in_z_opt const wchar_t * _Drive, __in_z_opt const wchar_t * _Dir, __in_z_opt 116#define _WSTDLIBP_DEFINED#endif117/** The Win32 API SetErrorMode, Beep and Sleep should be used instead. */_CRT_OBSOLETE(SetErrorMode) _CRTIMP void __cdecl _seterrormode(__in int _Mode);_CRT_OBSOLETE(Beep) _CRTIMP void __cdecl _beep(__in unsigned _F 118#if !__STDC__119/** Non-ANSI names for compatibility */120 #ifndef __cplusplus#define max(a,b) (((a) > (b)) ? (a) : (b))#define min(a,b) (((a) < (b)) ? (a) : (b))#endif121#define sys_errlist _sys_errlist#define sys_nerr _sys_nerr#define environ _environ122#pragma warning(push)#pragma warning(disable: 4141) /** Using deprecated twice */ _CRT_NONSTDC_DEPRECATE(_ecvt) _CRT_INSECURE_DEPRECATE(_ecvt_s) _CRTIMP __checkReturn char * __cdecl ecvt(__in double _Val, __in in 123#endif /** __STDC__ */124 #ifdef __cplusplus}125#endif126 #ifdef _MSC_VER#pragma pack(pop)#endif /** _MSC_VER */127#endif /** _INC_STDLIB *//** 88bf0570-3001-4e78-a5f2-be5765546192 */View Code包含的函数输⼊样式:C语⾔模式:#include <stdlib.h>C++样式:#include <cstdlib>1函数名称:calloc函数原型: void * calloc(unsigned n,unsigned size);函数功能: 分配n个数据项的内存连续空间,每个数据项的⼤⼩为size函数返回: 分配内存单元的起始地址,如果不成功,返回02函数名称:free函数原型: void free(void* p);函数功能: 释放p所指的内存区函数返回:参数说明: p-被释放的指针3函数名称:malloc函数原型: void * malloc(unsigned size);函数功能: 分配size字节的存储区函数返回: 所分配的内存区地址,如果内存不够,返回04函数名称: realloc函数原型: void * realloc(void * p,unsigned size);函数功能: 将p所指出的已分配内存区的⼤⼩改为size,size可以⽐原来分配的空间⼤或⼩函数返回: 返回指向该内存区的指针.NULL-分配失败5函数名称: rand函数原型: int rand(void);函数功能: 产⽣0到32767间的随机整数(0到0x7fff之间)函数返回: 随机整数6函数名称: abort函数原型: void abort(void)函数功能: 异常终⽌⼀个进程.7函数名称: exit函数原型: void exit(int state)函数功能: 程序中⽌执⾏,返回调⽤过程函数返回:参数说明: state:0-正常中⽌,⾮0-⾮正常中⽌8函数名称: getenv函数原型: char* getenv(const char *name)函数功能: 返回⼀个指向环境变量的指针函数返回:环境变量的定义参数说明: name-环境字符串9函数名称: putenv函数原型: int putenv(const char *name)函数功能: 将字符串name增加到DOS环境变量中函数返回: 0:操作成功,-1:操作失败参数说明: name-环境字符串10函数名称: labs函数原型: long labs(long num)函数功能: 求长整型参数的绝对值函数返回:绝对值11函数名称: atof函数原型: double atof(char *str)函数功能: 将字符串转换成⼀个双精度数值函数返回: 转换后的数值参数说明: str-待转换浮点型数的字符串12函数名称: atoi函数原型: int atoi(char *str)函数功能: 将字符串转换成⼀个整数值函数返回: 转换后的数值参数说明: str-待转换为整型数的字符串13函数名称: atol函数原型: long atol(char *str)函数功能: 将字符串转换成⼀个长整数函数返回: 转换后的数值参数说明: str-待转换为长整型的字符串14函数名称:ecvt函数原型: char *ecvt(double value,int ndigit,int *dec,int *sign)函数功能: 将浮点数转换为字符串函数返回: 转换后的字符串指针参数说明: value-待转换底浮点数,ndigit-转换后的字符串长度15函数名称:fcvt函数原型: char *fcvt(double value,int ndigit,int *dec,int *sign)函数功能: 将浮点数变成⼀个字符串函数返回: 转换后字符串指针参数说明: value-待转换底浮点数,ndigit-转换后底字符串长度。
c中内存分配与释放(malloc,realloc,calloc,free)函数内容的整理.wps

c中内存分配与释放(malloc,realloc,calloc,free)函数内容的整理malloc:原型:extern void *malloc(unsigned int num_bytes); 头文件:在TC2.0中可以用malloc.h 或alloc.h (注意:alloc.h 与malloc.h 的内容是完全一致的),而在V isual C++6.0中可以用malloc.h或者stdlib.h。
功能:分配长度为num_bytes字节的内存块返回值:如果分配成功则返回指向被分配内存的指针(此存储区中的初始值不确定),否则返回空指针NULL。
当内存不再使用时,应使用free()函数将内存块释放。
函数返回的指针一定要适当对齐,使其可以用于任何数据对象。
说明:关于该函数的原型,在旧的版本中malloc 返回的是char型指针,新的ANSIC标准规定,该函数返回为void型指针,因此必要时要进行类型转换。
名称解释:malloc的全称是memory allocation,中文叫动态内存分配。
函数声明void *malloc(size_t size); 说明:malloc 向系统申请分配指定size个字节的内存空间。
返回类型是void* 类型。
void* 表示未确定类型的指针。
C,C++规定,void* 类型可以强制转换为任何其它类型的指针。
备注:void* 表示未确定类型的指针,更明确的说是指申请内存空间时还不知道用户是用这段空间来存储什么类型的数据(比如是char还是int或者...)从函数声明上可以看出。
malloc 和new 至少有两个不同: new 返回指定类型的指针,并且可以自动计算所需要大小。
比如:int *p; p = new int; //返回类型为int* 类型(整数型指针),分配大小为sizeof(int); 或:int* parr; parr = new int [100]; //返回类型为int* 类型(整数型指针),分配大小为sizeof(int) * 100; 而malloc 则必须要由我们计算字节数,并且在返回后强行转换为实际类型的指针。
2024版C程序设计教程教与学教学大纲
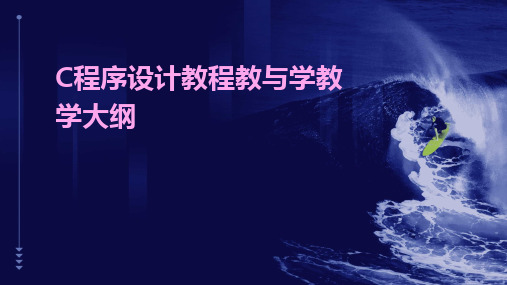
结构体与联合体模块
结构体的定义与使用
01
了解结构体的概念和定义方式,掌握结构体成员的访问和结构
体变量的操作。
联合体的定义与使用
02
了解联合体的概念和定义方式,掌握联合体成员的访问和联合
体变量的操作。
枚举类型与typedef
03
学习枚举类型的定义和使用,了解typedef的作用和使用方法。
课时安排及进度计划
05
考核评价与反馈机制
平时成绩评定标准
课堂表现
包括学生出勤率、课堂参与度、 回答问题准确性等。
作业完成情况
根据作业难度、完成质量、提交 时间等因素进行评定。
阶段性测试
针对重要知识点进行阶段性测试, 检验学生掌握情况。
期末考试形式及内容
考试形式
闭卷考试,采用笔试形式进行。
考试内容
涵盖本课程所有重要知识点,包括基本概念、语法规则、算法设 计等。
学生意见收集渠道
课堂调研
通过课堂调研了解学生对本课程的意见和建议。
课后反馈
鼓励学生课后向教师反馈学习情况和问题,以便 教师及时改进教学。
线上交流平台
利用线上交流平台收集学生意见和建议,加强与 学生的沟通和互动。
06
教师团队建设与培训
师资队伍现状介绍
1 2 3
现有教师数量、结构和特点 包括教师的年龄、学历、职称、专业背景等方面 的介绍,以及教师队伍的整体优势和不足。
掌握C语言对于理解 计算机底层原理、培 养编程思维具有重要 意义。
教学目标与要求
掌握C语言的基本语法、 数据类型、运算符和表达 式。
学会使用数组、函数、指 针等高级特性编写程序。
理解程序控制结构,如顺 序、选择、循环结构。
c语言分配内存并且赋值的函数

C语言分配内存并赋值的函数1. 概述在C语言中,我们经常需要动态地分配内存来存储数据。
为了方便地进行内存分配和赋值操作,C语言提供了一些特定的函数。
这些函数可以帮助我们在程序运行时动态地分配内存,并将指定的值赋给所分配的内存空间。
本文将详细介绍C语言中的几个常用的分配内存并赋值的函数,包括malloc、calloc和realloc。
我们将分别介绍它们的定义、用途和工作方式,并给出一些示例代码来说明它们的使用方法。
2. malloc函数2.1 定义malloc函数是C语言中用于动态分配内存的函数。
它的原型定义在stdlib.h头文件中,其定义如下:void* malloc(size_t size);2.2 用途malloc函数用于在程序运行时动态地分配指定大小的内存空间。
这个函数返回一个指向分配内存的指针,如果分配失败则返回NULL。
2.3 工作方式malloc函数的工作方式如下:1.接收一个size_t类型的参数size,表示需要分配的内存空间的大小。
2.在堆(heap)中分配一块大小为size的连续内存空间。
3.返回指向分配内存的指针,如果分配失败则返回NULL。
2.4 示例代码下面是一个使用malloc函数分配内存并赋值的示例代码:#include <stdio.h>#include <stdlib.h>int main() {int* ptr;int size = 5;ptr = (int*)malloc(size * sizeof(int));if (ptr == NULL) {printf("内存分配失败\n");return 1;}for (int i = 0; i < size; i++) {ptr[i] = i + 1;}for (int i = 0; i < size; i++) {printf("%d ", ptr[i]);}free(ptr);return 0;}上述代码中,我们使用malloc函数分配了一块大小为 5 * sizeof(int)的内存空间,并将其地址赋给指针ptr。
c语言中给指针的指针申请内存空间

《深入探讨C语言中给指针的指针申请内存空间》1.前言在C语言中,指针的使用是非常重要的一部分。
而指针的指针,也就是双重指针,更是在一些特定情况下非常实用和必要。
本文将针对C语言中给指针的指针申请内存空间这一复杂而重要的话题展开深入探讨。
2.简述指针和指针的指针在C语言中,指针可以用来存储变量的位置区域,通过指针可以直接访问和修改变量的值。
而指针的指针则是指向指针的指针变量,用来存储指针变量的位置区域。
这种多重间接性的概念在一些场景下可以极大地提高程序的灵活性和效率。
3.为指针的指针申请内存空间在C语言中,为指针的指针申请内存空间需要使用二级指针,也就是指向指针的指针。
通过使用一级指针来动态分配内存空间,从而为二级指针分配内存空间。
4.动态内存分配 C语言提供了标准库函数来进行动态内存分配,如malloc()、calloc()、realloc()等。
在为指针的指针申请内存空间时,可以通过这些函数来实现。
5.实际操作和示例接下来,我们将通过具体的代码示例来演示如何为指针的指针申请内存空间。
假设我们需要定义一个二维数组,而数组的行数是动态的,那么我们就需要使用指针的指针来实现。
#include <stdio.h>#include <stdlib.h>int main() {int i, j;int **arr; // 定义指针的指针int rows = 3, cols = 4; // 假设行数为3,列数为4// 申请内存空间arr = (int **)malloc(rows * sizeof(int *));for (i = 0; i < rows; i++) {arr[i] = (int *)malloc(cols * sizeof(int)); }// 对二维数组赋值并输出for (i = 0; i < rows; i++) {for (j = 0; j < cols; j++) {arr[i][j] = i * cols + j;printf("%d ", arr[i][j]);}printf("\n");}// 释放内存空间for (i = 0; i < rows; i++) {free(arr[i]);}free(arr);return 0;}在这个示例中,我们首先定义了一个指向指针的指针arr,然后使用malloc()函数为一级指针和二级指针分别申请了内存空间。
c语言释放内存的方式

c语言释放内存的方式以C语言释放内存的方式在C语言中,动态分配内存是一项非常重要的功能。
当我们在程序中使用malloc或calloc函数来动态分配内存时,必须要记得在使用完之后将其释放掉,以避免内存泄漏的问题。
本文将介绍C语言中释放内存的几种方式。
1. 使用free函数释放内存在C语言中,使用malloc或calloc函数动态分配内存后,我们可以使用free函数来释放已分配的内存。
free函数的原型如下:```cvoid free(void *ptr);```其中,ptr是指向要释放的内存的指针。
当我们使用完已分配的内存后,可以通过调用free函数来释放它,以便将内存归还给操作系统。
2. 释放动态分配的数组在C语言中,我们可以使用数组来存储一组数据。
当我们使用malloc或calloc函数动态分配数组内存时,释放内存的方式与释放普通内存的方式相同。
例如,下面的代码演示了如何释放动态分配的整型数组内存:```cint *arr = (int*)malloc(5 * sizeof(int));// 使用arr数组free(arr);```需要注意的是,释放数组内存时应该使用与分配内存时相对应的函数。
即,如果是使用malloc函数分配的内存,则应使用free函数进行释放;如果是使用calloc函数分配的内存,则应使用free函数进行释放。
3. 使用realloc函数调整内存大小在某些情况下,我们可能需要调整已分配内存的大小。
C语言提供了realloc函数来实现这一功能。
realloc函数的原型如下:```cvoid *realloc(void *ptr, size_t size);```其中,ptr是指向要调整大小的内存的指针,size是新的内存大小。
realloc函数会尝试重新分配ptr指向的内存,并将其大小调整为size。
需要注意的是,realloc函数可能会将原有的内容复制到新的内存空间中,因此在调用realloc函数后,原有的指针可能会失效。
#stdib.h的用法

stdlib.hstdlib 头文件里包含了C、C++语言的一些函数该文件包含了的C语言标准库函数的定义stdlib.h里面定义了五种类型、一些宏和通用工具函数。
类型例如size_t、wch ar_t、div_t、ldiv_t和lldiv_t;宏例如EXIT_FAILURE、EXIT_SUCCESS、RAND_ MAX和MB_CUR_MAX等等;常用的函数如malloc()、calloc()、realloc()、free()、system()、atoi()、atol()、rand()、srand()、exit()等等。
具体的内容你自己可以打开编译器的include目录里面的stdlib.h头文件看看。
stdlib.h内容如下:/****stdlib.h - declarations/definitions for commonly used library functions** Copyright (c) 1985-1997, Microsoft Corporation. All rights reserved.**Purpose:* This include file contains the function declarations for commonly* used library functions which either don't fit somewhere else, or,* cannot be declared in the normal place for other reasons.* [ANSI]** [Public]*****/#if _MSC_VER > 1000#pragma once#endif#ifndef _INC_STDLIB#define _INC_STDLIB#if !defined(_WIN32) && !defined(_MAC)#error ERROR: Only Mac or Win32 targets supported!#endif#ifdef _MSC_VER/** Currently, all MS C compilers for Win32 platforms default to 8 byte* alignment.*/#pragma pack(push,8)#endif /* _MSC_VER */#ifdef __cplusplusextern "C" {#endif/* Define _CRTIMP */#ifndef _CRTIMP#ifdef _DLL#define _CRTIMP __declspec(dllimport)#else /* ndef _DLL */#define _CRTIMP#endif /* _DLL */#endif /* _CRTIMP *//* Define __cdecl for non-Microsoft compilers */#if ( !defined(_MSC_VER) && !defined(__cdecl) )#define __cdecl#endif/* Define _CRTAPI1 (for compatibility with the NT SDK) */ #ifndef _CRTAPI1#if _MSC_VER >= 800 && _M_IX86 >= 300#define _CRTAPI1 __cdecl#else#define _CRTAPI1#endif#endif#ifndef _SIZE_T_DEFINEDtypedef unsigned int size_t;#define _SIZE_T_DEFINED#endif#ifndef _WCHAR_T_DEFINEDtypedef unsigned short wchar_t;#define _WCHAR_T_DEFINED#endif/* Define NULL pointer value */#ifndef NULL#ifdef __cplusplus#define NULL 0#else#define NULL ((void *)0)#endif#endif/* Definition of the argument values for the exit() function */#define EXIT_SUCCESS 0#define EXIT_FAILURE 1#ifndef _ONEXIT_T_DEFINEDtypedef int (__cdecl * _onexit_t)(void);#if !__STDC__/* Non-ANSI name for compatibility */#define onexit_t _onexit_t#endif#define _ONEXIT_T_DEFINED#endif/* Data structure definitions for div and ldiv runtimes. */#ifndef _DIV_T_DEFINEDtypedef struct _div_t {int quot;int rem;} div_t;typedef struct _ldiv_t {long quot;long rem;} ldiv_t;#define _DIV_T_DEFINED#endif/* Maximum value that can be returned by the rand function. */#define RAND_MAX 0x7fff/** Maximum number of bytes in multi-byte character in the current locale * (also defined in ctype.h).*/#ifndef MB_CUR_MAX#define MB_CUR_MAX __mb_cur_max_CRTIMP extern int __mb_cur_max;#endif /* MB_CUR_MAX *//* Minimum and maximum macros */#define __max(a,b) (((a) > (b)) ? (a) : (b))#define __min(a,b) (((a) < (b)) ? (a) : (b))/** Sizes for buffers used by the _makepath() and _splitpath() functions. * note that the sizes include space for 0-terminator*/#ifndef _MAC#define _MAX_PATH 260 /* max. length of full pathname */#define _MAX_DRIVE 3 /* max. length of drive component */#define _MAX_DIR 256 /* max. length of path component */#define _MAX_FNAME 256 /* max. length of file name component */ #define _MAX_EXT 256 /* max. length of extension component */#else /* def _MAC */#define _MAX_PATH 256 /* max. length of full pathname */#define _MAX_DIR 32 /* max. length of path component */#define _MAX_FNAME 64 /* max. length of file name component */#endif /* _MAC *//** Argument values for _set_error_mode().*/#define _OUT_TO_DEFAULT 0#define _OUT_TO_STDERR 1#define _OUT_TO_MSGBOX 2#define _REPORT_ERRMODE 3/* External variable declarations */#if (defined(_MT) || defined(_DLL)) && !defined(_MAC)_CRTIMP int * __cdecl _errno(void);_CRTIMP unsigned long * __cdecl __doserrno(void);#define errno (*_errno())#define _doserrno (*__doserrno())#else /* ndef _MT && ndef _DLL */_CRTIMP extern int errno; /* XENIX style error number */_CRTIMP extern unsigned long _doserrno; /* OS system error value */ #endif /* _MT || _DLL */#ifdef _MAC_CRTIMP extern int _macerrno; /* OS system error value */#endif_CRTIMP extern char * _sys_errlist[]; /* perror error message table */ _CRTIMP extern int _sys_nerr; /* # of entries in sys_errlist table */#if defined(_DLL) && defined(_M_IX86)#define __argc (*__p___argc()) /* count of cmd line args */#define __argv (*__p___argv()) /* pointer to table of cmd line args */ #define __wargv (*__p___wargv()) /* pointer to table of wide cmd line ar gs */#define _environ (*__p__environ()) /* pointer to environment table */#ifdef _POSIX_extern char ** environ; /* pointer to environment table */#else#ifndef _MAC#define _wenviron (*__p__wenviron()) /* pointer to wide environment tabl e */#endif /* ndef _MAC */#endif /* _POSIX_ */#define _pgmptr (*__p__pgmptr()) /* points to the module (EXE) name */ #ifndef _MAC#define _wpgmptr (*__p__wpgmptr()) /* points to the module (EXE) wide name */#endif /* ndef _MAC */_CRTIMP int * __cdecl __p___argc(void);_CRTIMP char *** __cdecl __p___argv(void);_CRTIMP wchar_t *** __cdecl __p___wargv(void);_CRTIMP char *** __cdecl __p__environ(void);_CRTIMP wchar_t *** __cdecl __p__wenviron(void);_CRTIMP char ** __cdecl __p__pgmptr(void);_CRTIMP wchar_t ** __cdecl __p__wpgmptr(void);#else_CRTIMP extern int __argc; /* count of cmd line args */_CRTIMP extern char ** __argv; /* pointer to table of cmd line args */ #ifndef _MAC_CRTIMP extern wchar_t ** __wargv; /* pointer to table of wide cmd lin e args */#endif /* ndef _MAC */#ifdef _POSIX_extern char ** environ; /* pointer to environment table */#else_CRTIMP extern char ** _environ; /* pointer to environment table */#ifndef _MAC_CRTIMP extern wchar_t ** _wenviron; /* pointer to wide environment ta ble */#endif /* ndef _MAC */#endif /* _POSIX_ */_CRTIMP extern char * _pgmptr; /* points to the module (EXE) name */ #ifndef _MAC_CRTIMP extern wchar_t * _wpgmptr; /* points to the module (EXE) wid e name */#endif /* ndef _MAC */#endif_CRTIMP extern int _fmode; /* default file translation mode */_CRTIMP extern int _fileinfo; /* open file info mode (for spawn) *//* Windows major/minor and O.S. version numbers */_CRTIMP extern unsigned int _osver;_CRTIMP extern unsigned int _winver;_CRTIMP extern unsigned int _winmajor;_CRTIMP extern unsigned int _winminor;/* function prototypes */#if _MSC_VER >= 1200_CRTIMP __declspec(noreturn) void __cdecl abort(void);_CRTIMP __declspec(noreturn) void __cdecl exit(int);#else_CRTIMP void __cdecl abort(void);_CRTIMP void __cdecl exit(int);#endif#if defined(_M_MRX000)_CRTIMP int __cdecl abs(int);#elseint __cdecl abs(int);#endifint __cdecl atexit(void (__cdecl *)(void));_CRTIMP double __cdecl atof(const char *);_CRTIMP int __cdecl atoi(const char *);_CRTIMP long __cdecl atol(const char *);#ifdef _M_M68K_CRTIMP long double __cdecl _atold(const char *);#endif_CRTIMP void * __cdecl bsearch(const void *, const void *, size_t, size _t,int (__cdecl *)(const void *, const void *));_CRTIMP void * __cdecl calloc(size_t, size_t);_CRTIMP div_t __cdecl div(int, int);_CRTIMP void __cdecl free(void *);_CRTIMP char * __cdecl getenv(const char *);_CRTIMP char * __cdecl _itoa(int, char *, int);#if _INTEGRAL_MAX_BITS >= 64_CRTIMP char * __cdecl _i64toa(__int64, char *, int);_CRTIMP char * __cdecl _ui64toa(unsigned __int64, char *, int);_CRTIMP __int64 __cdecl _atoi64(const char *);#endif#if defined(_M_MRX000)_CRTIMP long __cdecl labs(long);#elselong __cdecl labs(long);#endif_CRTIMP ldiv_t __cdecl ldiv(long, long);_CRTIMP char * __cdecl _ltoa(long, char *, int);_CRTIMP void * __cdecl malloc(size_t);_CRTIMP int __cdecl mblen(const char *, size_t);_CRTIMP size_t __cdecl _mbstrlen(const char *s);_CRTIMP int __cdecl mbtowc(wchar_t *, const char *, size_t);_CRTIMP size_t __cdecl mbstowcs(wchar_t *, const char *, size_t); _CRTIMP void __cdecl qsort(void *, size_t, size_t, int (__cdecl *) (const void *, const void *));_CRTIMP int __cdecl rand(void);_CRTIMP void * __cdecl realloc(void *, size_t);_CRTIMP int __cdecl _set_error_mode(int);_CRTIMP void __cdecl srand(unsigned int);_CRTIMP double __cdecl strtod(const char *, char **);_CRTIMP long __cdecl strtol(const char *, char **, int);#ifdef _M_M68K_CRTIMP long double __cdecl _strtold(const char *, char **);#endif_CRTIMP unsigned long __cdecl strtoul(const char *, char **, int); #ifndef _MAC_CRTIMP int __cdecl system(const char *);#endif_CRTIMP char * __cdecl _ultoa(unsigned long, char *, int);_CRTIMP int __cdecl wctomb(char *, wchar_t);_CRTIMP size_t __cdecl wcstombs(char *, const wchar_t *, size_t);#ifndef _MAC#ifndef _WSTDLIB_DEFINED/* wide function prototypes, also declared in wchar.h */_CRTIMP wchar_t * __cdecl _itow (int, wchar_t *, int);_CRTIMP wchar_t * __cdecl _ltow (long, wchar_t *, int);_CRTIMP wchar_t * __cdecl _ultow (unsigned long, wchar_t *, int);_CRTIMP double __cdecl wcstod(const wchar_t *, wchar_t **);_CRTIMP long __cdecl wcstol(const wchar_t *, wchar_t **, int);_CRTIMP unsigned long __cdecl wcstoul(const wchar_t *, wchar_t **, in t);_CRTIMP wchar_t * __cdecl _wgetenv(const wchar_t *);_CRTIMP int __cdecl _wsystem(const wchar_t *);_CRTIMP int __cdecl _wtoi(const wchar_t *);_CRTIMP long __cdecl _wtol(const wchar_t *);#if _INTEGRAL_MAX_BITS >= 64_CRTIMP wchar_t * __cdecl _i64tow(__int64, wchar_t *, int);_CRTIMP wchar_t * __cdecl _ui64tow(unsigned __int64, wchar_t *, int);_CRTIMP __int64 __cdecl _wtoi64(const wchar_t *);#endif#define _WSTDLIB_DEFINED#endif#endif /* ndef _MAC */#ifndef _POSIX__CRTIMP char * __cdecl _ecvt(double, int, int *, int *);#if _MSC_VER >= 1200_CRTIMP __declspec(noreturn) void __cdecl _exit(int);#else_CRTIMP void __cdecl _exit(int);#endif_CRTIMP char * __cdecl _fcvt(double, int, int *, int *);_CRTIMP char * __cdecl _fullpath(char *, const char *, size_t);_CRTIMP char * __cdecl _gcvt(double, int, char *);unsigned long __cdecl _lrotl(unsigned long, int);unsigned long __cdecl _lrotr(unsigned long, int);#ifndef _MAC_CRTIMP void __cdecl _makepath(char *, const char *, const char *, co nst char *,const char *);#endif_onexit_t __cdecl _onexit(_onexit_t);_CRTIMP void __cdecl perror(const char *);_CRTIMP int __cdecl _putenv(const char *);unsigned int __cdecl _rotl(unsigned int, int);unsigned int __cdecl _rotr(unsigned int, int);_CRTIMP void __cdecl _searchenv(const char *, const char *, char *);#ifndef _MAC_CRTIMP void __cdecl _splitpath(const char *, char *, char *, char *, ch ar *);#endif_CRTIMP void __cdecl _swab(char *, char *, int);#ifndef _MAC#ifndef _WSTDLIBP_DEFINED/* wide function prototypes, also declared in wchar.h */_CRTIMP wchar_t * __cdecl _wfullpath(wchar_t *, const wchar_t *, size_ t);_CRTIMP void __cdecl _wmakepath(wchar_t *, const wchar_t *, const w char_t *, const wchar_t *,const wchar_t *);_CRTIMP void __cdecl _wperror(const wchar_t *);_CRTIMP int __cdecl _wputenv(const wchar_t *);_CRTIMP void __cdecl _wsearchenv(const wchar_t *, const wchar_t *, w char_t *);_CRTIMP void __cdecl _wsplitpath(const wchar_t *, wchar_t *, wchar_t *, wchar_t *, wchar_t *);#define _WSTDLIBP_DEFINED#endif#endif /* ndef _MAC *//* --------- The following functions are OBSOLETE --------- *//* The Win32 API SetErrorMode, Beep and Sleep should be used instea d. */#ifndef _MAC_CRTIMP void __cdecl _seterrormode(int);_CRTIMP void __cdecl _beep(unsigned, unsigned);_CRTIMP void __cdecl _sleep(unsigned long);#endif /* ndef _MAC *//* --------- The preceding functions are OBSOLETE --------- */#endif /* _POSIX_ */#if !__STDC__/* --------- The declarations below should not be in stdlib.h --------- */ /* --------- and will be removed in a future release. Include --------- */ /* --------- ctype.h to obtain these declarations. --------- */#ifndef tolower /* tolower has been undefined - use function */_CRTIMP int __cdecl tolower(int);#endif /* tolower */#ifndef toupper /* toupper has been undefined - use function */_CRTIMP int __cdecl toupper(int);#endif /* toupper *//* --------- The declarations above will be removed. --------- */#endif#if !__STDC__#ifndef _POSIX_/* Non-ANSI names for compatibility */#ifndef __cplusplus#define max(a,b) (((a) > (b)) ? (a) : (b))#define min(a,b) (((a) < (b)) ? (a) : (b))#endif#define sys_errlist _sys_errlist#define sys_nerr _sys_nerr#define environ _environ_CRTIMP char * __cdecl ecvt(double, int, int *, int *);_CRTIMP char * __cdecl fcvt(double, int, int *, int *);_CRTIMP char * __cdecl gcvt(double, int, char *);_CRTIMP char * __cdecl itoa(int, char *, int);_CRTIMP char * __cdecl ltoa(long, char *, int);onexit_t __cdecl onexit(onexit_t);_CRTIMP int __cdecl putenv(const char *);_CRTIMP void __cdecl swab(char *, char *, int);_CRTIMP char * __cdecl ultoa(unsigned long, char *, int);#endif /* _POSIX_ */#endif /* __STDC__ */#ifdef __cplusplus}#endif#ifdef _MSC_VER#pragma pack(pop)#endif /* _MSC_VER */ #endif /* _INC_STDLIB */。
华为机试题目

华为机试题【2011】1、(stdlib.h里面定义了五种类型、一些宏和通用工具函数。
类型例如size_t、wchar_t、div_t、ldiv_t和lldiv_t;宏例如EXIT_FAILURE、EXIT_SUCCESS、RAND_MAX和MB_CUR_MAX等等;常用的函数如malloc()、calloc()、realloc()、free()、system()、atoi()、atol()、rand()、srand()、exit())#include<stdio.h>#include<stdlib.h>#include<assert.h>#include<string.h>#define LENGTH 13int verifyMsisdn(char *inMsisdn){char *pchar=NULL;assert(inMsisdn!=NULL);if(LENGTH==strlen(inMsisdn)){if(('8'==*inMsisdn)&&(*(inMsisdn+1)=='6')){while(*inMsisdn!='\0'){if((*inMsisdn>='0')&&(*inMsisdn<='9'))inMsisdn++;elsereturn 2 ;}}elsereturn 3;}elsereturn 1;return 0;}int main(){char *pchar=NULL;unsigned char ichar=0;int result;switch(ichar){case 0:pchar="8612345363789";break; case 1:pchar="861111111111111";break; case 2:pchar="86s1234536366"; break; default:break;}result =verifyMsisdn(pchar);printf("result is %d\n",result);}华赛面试:1.char m[]={"I", "LOVE", "CHINA"} char* p=m;printf("%s", *p++);printf("%c", **p);int main(){double x=1;double y;y=x+3/2;printf("%f\n",y);return 0;} //////结果为2.0000003.4.找错unsigned int f(){unsigned char a=123;unsigned char res;while(a-->=0){res+=a;}return res;}//res没有初始化5.struct node{int data;node* pre;node* next;}结构体数组转双向循环链表1. 数组比较(20 分)•问题描述:比较两个数组,要求从数组最后一个元素开始逐个元素向前比较,如果2 个数组长度不等,则只比较较短长度数组个数元素。
C语言程序设计(李刚第3版)教案

作。
01
02
队列的定义与特性
队列是一种先进先出(FIFO )的数据结构,只允许在一 端(队尾)进行插入操作, 在另一端(队头)进行删除
操作。
03
04
栈的应用场景
函数调用、表达式求值、括 号匹配等。
队列的应用场景
缓冲区处理、打印任务队列 、CPU任务调度等。
04
关闭文件
使用fclose()函数关
03
闭文件,释放相关
资源。
写入文件
使用fprintf()或 fputs()等函数向文
件中写入数据。
随机文件读写操作示例
打开文件
同样使用fopen()函数打开文件, 但需要指定读写模式为"rb"或 "wb"等。
读取文件
使用fread()函数从文件中读取指 定长度的数据。
教学目标与要求
知识目标
掌握C语言的基本语法、数据类型、运算符、控制结构、函数、数组、指针等核心知识。
能力目标
能够运用C语言进行程序设计,解决实际问题,具备良好的编程习惯和风格。
素养目标
培养学生的计算思维、创新精神和团队协作精神,提高学生的自主学习能力和终身学习能力。
教材结构与内容
教材结构
《C语言程序设计(李刚第3版)》共分为XX章,包括引言、基 本数据类型与表达式、控制结构、函数、数组、指针、结构 体与共用体、文件操作等内容。
变量与常量
阐述变量和常量的概念,以及它们 在程序中的作用和使用方法。
运算符与表达式
讲解C语言中的各种运算符(如算术 运算符、关系运算符、逻辑运算符 等)以及表达式的构成和求值规则 。
C语言的简答题包含解答共70道题

C语言的简答题包含解答共70道题1. 什么是C语言?- C语言是一种通用的、高级的编程语言,由Dennis Ritchie于1972年开发。
它被广泛用于系统编程和应用程序开发。
2. C语言的特点是什么?- C语言具有高效性、可移植性和灵活性等特点。
3. 什么是C语言的注释符号?- C语言使用`//`表示单行注释,使用`/* */`表示多行注释。
4. 如何在C语言中声明一个整数变量?-使用如下语句:`int myVariable;`5. C语言的变量命名规则是什么?-变量名可以包含字母、数字和下划线,但必须以字母或下划线开头。
6. 如何给变量赋值?-使用赋值操作符`=`,例如:`myVariable = 10;`7. 如何在C语言中打印文本?-使用`printf()`函数,例如:`printf("Hello, World!\n");`8. 如何读取用户输入?-使用`scanf()`函数,例如:`scanf("%d", &myVariable);`9. 什么是数据类型?-数据类型定义了变量可以存储的数据种类和范围,如整数、浮点数、字符等。
10. 什么是`sizeof`运算符?- `sizeof`运算符用于获取数据类型或变量的字节数。
11. 什么是类型转换?-类型转换是将一个数据类型的值转换为另一个数据类型的过程。
12. 如何定义常量?-使用`#define`指令或`const`关键字来定义常量,例如:`#define PI 3.14159`或`const int MAX_VALUE = 100;`13. 什么是运算符?-运算符是用于执行各种操作的符号,如加法、减法、乘法等。
14. C语言中的算术运算符有哪些?-加法`+`、减法`-`、乘法`*`、除法`/`、取模`%`等。
15. 如何进行条件判断?-使用`if`语句,例如:`if (x > 10) { /* 代码块*/ }`16. 什么是循环?-循环是重复执行一组语句的控制结构。
2024年qnx培训教程

QNX培训教程1.引言QNX是一款高性能、可扩展的实时操作系统,广泛应用于汽车、医疗、工业控制等领域。
本教程旨在帮助读者了解QNX的基本概念、开发环境和编程技术,从而为QNX应用程序开发奠定基础。
2.QNX基础知识2.1实时操作系统可预测性:QNX提供了确定性调度策略,确保任务按照预定的时间执行。
高可靠性:QNX采用微内核架构,将内核与用户态应用程序隔离开来,降低了系统崩溃的风险。
高性能:QNX内核经过优化,能够在多核处理器上高效运行。
可扩展性:QNX支持多种硬件平台和操作系统,便于跨平台开发。
2.2微内核架构模块化:便于维护和更新,提高系统稳定性。
可扩展性:可根据需求添加或删除模块,实现定制化开发。
高效性:微内核只包含基本功能,减少了系统资源占用。
2.3QNX网络协议栈高效性:采用零拷贝技术,减少数据传输过程中的CPU开销。
可靠性:支持多种网络协议,保证数据传输的可靠性。
安全性:提供安全套接字层(SSL)等加密技术,确保数据传输的安全性。
3.QNX开发环境3.1工具链QNX开发环境包括一套完整的工具链,支持C、C++、汇编等编程语言。
主要工具如下:QNXMomenticsIDE:集成开发环境,提供代码编辑、调试、性能分析等功能。
QNXQCC:C/C++编译器,支持多种优化选项。
QNXQNXLINK:调试器,支持远程调试和性能分析。
QNXQMAKE:项目管理工具,用于Makefile文件。
3.2SDKQNXNeutrino库:提供实时操作系统核心功能。
QNXPhoton微型GUI库:提供图形用户界面支持。
QNXRTP库:支持实时进程通信。
QNXMultimedia库:提供音频、视频等多媒体功能。
4.QNX编程技术4.1进程与线程进程创建与销毁:fork()、exec()、exit()。
线程创建与销毁:pthread_create()、pthread_exit()。
线程同步:互斥锁(mutex)、条件变量(conditionvariable)、读写锁(read-writelock)。
c程序设计谭浩强第五版知识点总结

《C程序设计谭浩强第五版知识点总结》一、基本概念1. C程序设计概述这本书是谭浩强先生编写的C程序设计教材的第五版,内容全面,通俗易懂,适合初学者入门。
2. 程序设计基本流程本书从程序设计基础知识开始介绍,包括编程思想、程序的基本结构、编译信息过程等,为读者打下扎实的基础。
3. C语言基本数据类型本书详细介绍了C语言的基本数据类型,包括整型、浮点型、字符型等,帮助读者深入理解C语言的数据表示和操作。
二、程序设计基础1. 程序流程控制本书系统地介绍了C语言中的顺序结构、选择结构和循环结构,帮助读者掌握程序的基本控制流程。
2. 函数函数是C语言中重要的概念,本书对函数的定义、声明、调用、参数传递等方面进行了详细讲解,帮助读者理解函数的作用和使用方法。
3. 数组数组是C语言中常用的数据结构,本书介绍了数组的定义、初始化、访问等基本操作,还介绍了多维数组和数组作为函数参数的用法。
三、指针和结构体1. 指针指针是C语言中较为复杂的概念,本书对指针的定义、运算、指针与数组、指针与函数等方面进行了详细讲解,帮助读者理解指针的重要性和使用方法。
2. 结构体结构体是C语言中用于表示复杂数据结构的概念,本书介绍了结构体的定义、访问、嵌套等操作,还介绍了结构体数组和结构体作为函数参数的使用方法。
四、文件操作1. 文件输入输出文件操作是C语言中重要的知识点,本书介绍了如何打开文件、读写文件、关闭文件等基本操作,帮助读者掌握文件处理的基本技能。
2. 随机访问文件随机访问文件是C语言中较为复杂的知识点,本书介绍了如何进行文件的随机读写操作,帮助读者理解文件指针的移动和文件的定位操作。
五、综合应用1. 实例分析本书通过大量的实例分析,帮助读者将所学知识运用到实际问题中,提高解决问题的能力和编程的实际水平。
2. 项目设计本书还介绍了一些小型项目的设计思路和实现方法,帮助读者综合运用所学知识,提高程序设计能力。
总结C程序设计谭浩强第五版作为C语言教材的经典之作,系统地介绍了C语言的基本知识和程序设计的基本流程,涵盖了C语言的各个方面,适合初学者入门和进阶学习。
c语言内存分配与释放的函数

c语言内存分配与释放的函数C 语言内存分配与释放的函数非常重要,特别是在处理大型程序,以及对内存使用有严格要求的程序。
内存分配与释放是 C 语言中最常见的操作,因此,掌握内存分配与释放函数的使用方法对于程序员来说是非常必要的。
一、内存分配函数1. malloc 函数malloc 函数是 C 语言中最常用的内存分配函数之一,其基本语法格式如下:void *malloc(size_t size);其中,size_t 是无符号整型的数据类型,它表示需要分配的内存大小。
在内存分配成功后,malloc 函数将返回指向分配内存区域的指针;否则返回 NULL。
需要注意的是,分配出来的内存在函数执行结束后并不会被释放,必须由程序员调用 free 函数来释放内存。
2. calloc 函数calloc 函数可以用来分配一片连续的内存,而且会将其清零。
其函数原型如下:void *calloc(size_t nmemb, size_t size);其中,nmemb 表示需要分配的内存单元数量,size 表示单个单元的大小。
calloc 函数返回一个指向已分配内存区域的指针,其用法和 malloc 函数类似。
3. realloc 函数realloc 函数用于将原来已分配的内存区重新调整大小,其函数原型如下:void *realloc(void *ptr, size_t size);其中,ptr 是指向已分配内存区域的指针,size 表示重新分配后内存的大小。
realloc 函数返回一个指向已调整内存区域的指针。
二、内存释放函数1. free 函数free 函数用于释放一个之前已经分配的内存区域。
其语法格式如下:void free(void *ptr);其中,ptr 是指向要释放的内存区域的指针。
使用 free 函数需要注意的是,释放的只能是由 malloc、calloc 或 realloc 函数分配的内存,不能是栈或全局变量等。
C语言函数calloc
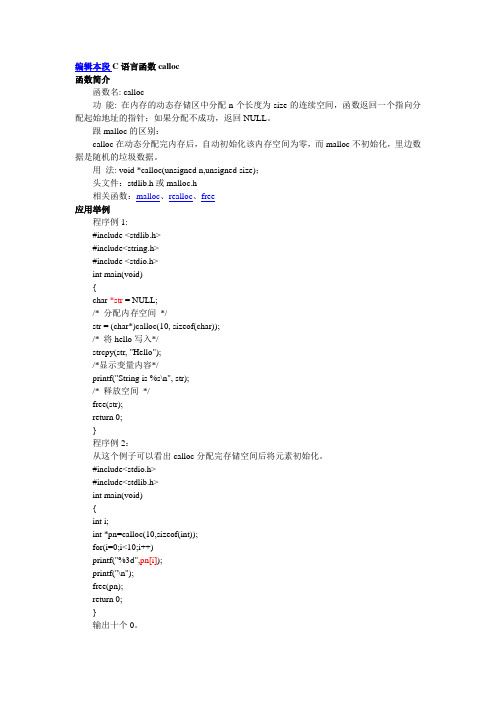
编辑本段C语言函数calloc函数简介函数名: calloc功能: 在内存的动态存储区中分配n个长度为size的连续空间,函数返回一个指向分配起始地址的指针;如果分配不成功,返回NULL。
跟malloc的区别:calloc在动态分配完内存后,自动初始化该内存空间为零,而malloc不初始化,里边数据是随机的垃圾数据。
用法: void *calloc(unsigned n,unsigned size);头文件:stdlib.h或malloc.h相关函数:malloc、realloc、free应用举例程序例1:#include <stdlib.h>#include<string.h>#include <stdio.h>int main(void){char *str = NULL;/* 分配内存空间*/str = (char*)calloc(10, sizeof(char));/* 将hello写入*/strcpy(str, "Hello");/*显示变量内容*/printf("String is %s\n", str);/* 释放空间*/free(str);return 0;}程序例2:从这个例子可以看出calloc分配完存储空间后将元素初始化。
#include<stdio.h>#include<stdlib.h>int main(void){int i;int *pn=calloc(10,sizeof(int));for(i=0;i<10;i++)printf("%3d",pn[i]);printf("\n");free(pn);return 0;}输出十个0。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
这一行,作用完全等同于:
int* p = (int *)malloc(sizeof(int) * 10);
(5)关于alloca()函数
还有一个函数也值得一提,这就是alloca()。其调用序列与malloc相同,但是它是在当前函数的栈帧上分配存储空间,而不是在堆中。其优点是:当 函数返回时,自动释放它所使用的栈帧,所以不必再为释放空间而费心。其缺点是:某些系统在函数已被调用后不能增加栈帧长度,于是也就不能支持alloca 函数。尽管如此,很多软件包还是使用alloca函数,也有很多系统支持它。
(3)calloc函数的分配的内存也需要自行释放
calloc函数的功能与malloc函数的功能相似,都是从堆分配内存,它与malloc函数的一个显著不同时是,calloc函数得到的内存空间是经过初始化的,其内容全为0。calloc函数适合为数组申请空间,可以将size设置为数组元素的空间长度,将n设置为数组的容量。
(2)使用malloc函数分配的堆空间在程序结束之前必须释放
从堆上获得的内存空间在程序结束以后,系统不会将其自动释放,需要程序员来自己管理。一个程序结束时,必须保证所有从堆上获得的内存空间已被安全释放,否则,会导致内存泄露。
我们可以使用free()函数来释放内存空间,但是,free函数只是释放指针指向的内容,而该指针仍然指向原来指向的地方,此时,指针为野指针,如果此时操作该指针会导致不可预期的错误。安全做法是:在使用free函数释放指针指向的空间之后,将指针的值置为NULL。
所以,在代码中,我们必须将realloc返回的值,重新赋值给 p :
p = (int *) realloc(p, sizeof(int) *15);
甚至,你可以传一个空指针(0)给 realloc ,则此时realloc 作用完全相当于malloc。
int* p = (int *)realloc (0,sizeof(int) * 10); //分配一个全新的内存空间,
将p所指向的对象的大小改为size个字节.
如果新分配的内存比原内存大, 那么原内存的内容保持不变, 增加的空间不进行初始化.
如果新分配的内存比原内存小, 那么新内存保持原内存的内容, 增加的空间不进行初始化.
返回指向新分配空间的指针; 若内存不够,则返回NULL, 原p指向的内存区不变.
char *p = (char *)malloc(sizeof(char));
(4)如果要使用realloc函数分配的内存,必Hale Waihona Puke 使用memset函数对其内存初始化
realloc函数的功能比malloc函数和calloc函数的功能更为丰富,可以实现内存分配和内存释放的功能。realloc 可以对给定的指针所指的空间进行扩大或者缩小,无论是扩张或是缩小,原有内存的中内容将保持不变。当然,对于缩小,则被缩小的那一部分的内容会丢失。realloc 并不保证调整后的内存空间和原来的内存空间保持同一内存地址。相反,realloc 返回的指针很可能指向一个新的地址。
四个函数之间的有区别,也有联系,我们应该学会把握这种关系,从而编出精炼而高效的程序。
在说明它们具体含义之前,先简单从字面上加以认识,前3个函数有个共同的特点,就是都带有字符”alloc”,就是”allocate”,”分配”的意思,也就是给对象分配足够的内存,” calloc()”是”分配内存给多个对象”,” malloc()”是”分配内存给一个对象”,”realloc()”是”重新分配内存”之意。”free()”就比较简单了,”释放”的意思,就是把之前所分配的内存空间给释放出来。
malloc函数和memset函数的操作语句一般如下:
int * p=NULL;
p=(int*)malloc(sizeof(int));
if(p==NULL)
printf(“Can’t get memory!\n”);
memset(p,0,siezeof(int));
calloc(), malloc(), realloc(), free(),alloca()
内存区域可以分为栈、堆、静态存储区和常量存储区,局部变量,函数形参,临时变量都是在栈上获得内存的,它们获取的方式都是由编译器自动执行的。
利用指针,我们可以像汇编语言一样处理内存地址,C 标准函数库提供了许多函数来实现对堆上内存管理,其中包括:malloc函数,free函数,calloc函数和realloc函数。使用这些函数需要包含头文件stdlib.h。
void *calloc(size_t nobj, size_t size);
分配足够的内存给nobj个大小为size的对象组成的数组, 并返回指向所分配区域的第一个字节的指针;
若内存不够,则返回NULL. 该空间的初始化大小为0字节.
char *p = (char *) calloc(100,sizeof(char));
malloc函数分配得到的内存空间是未初始化的。因此,一般在使用该内存空间时,要调用另一个函数memset来将其初始化为全0,memset函数的声明如下:void * memset (void * p,int c,int n) ;
该函数可以将指定的内存空间按字节单位置为指定的字符c,其中,p为要清零的内存空间的首地址,c为要设定的值,n为被操作的内存空间的字节长度。如果要用memset清0,变量c实参要为0。
void *malloc(size_t size);
分配足够的内存给大小为size的对象, 并返回指向所分配区域的第一个字节的指针;
若内存不够,则返回NULL. 不对分配的空间进行初始化.
char *p = (char *)malloc(sizeof(char));
void *realloc(void *p, size_t size);
p= (char *)realloc(p, 256);
void free(void *p);
释放p所指向的内存空间; 当p为NULL时, 不起作用.
p必先调用calloc, malloc或realloc.
值得注意的有以下5点:
(1)通过malloc函数得到的堆内存必须使用memset函数来初始化