c语言大作业物流配送系统程序
C物流配送管理系统Aldy小组个人任务实施方案
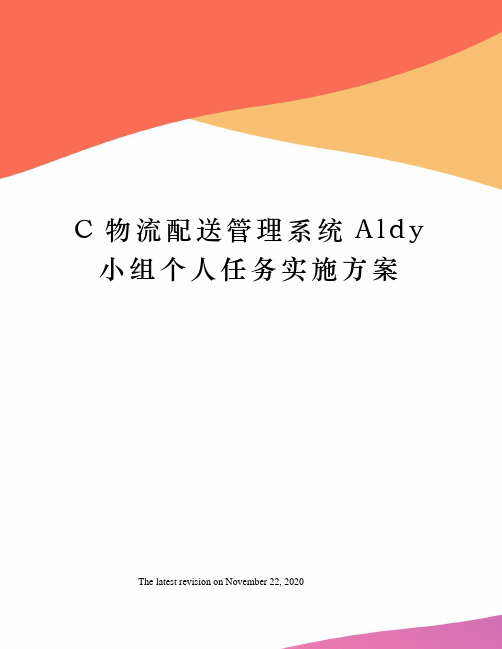
C物流配送管理系统A l d y小组个人任务实施方案 The latest revision on November 22, 2020《课程设计名称》存档资料之三《物流配送管理系统》个人任务实施方案Aldy软件09416班长春大学软件学院二一一年一月目录2组长:杨树良物流配送管理系统个人任务实施方案1 相关的理论知识(1)E-R图由实体、实体的属性和实体之间的联系三个要素组成,关系模型逻辑结构是一组关系模式的集合将E-R图转换为关系模型就是将实体、实体的属性和实体之间的联系转化为关系模式。
(2)将E-R图转换成关系模式:一个实体型转换为一个关系模式。
实体的属性就是关系的属性,实体的码就是关系的码。
(3)关系模型的数据结构:一个关系模型的数据结构,也是逻辑结构,是一张二维表,它由行和列组成。
每一行是一个元组,每一列称为一个字段。
通常在关系模型中将表称为关系。
(4)关系模式的存储结构:实体及实体间的联系都用表来表示,这是关系模型的逻辑结构。
在数据库的物理组织中,表以文件形式存储,每一个表通常对应一种文件结构,因此关系模型的存储结构是文件。
(5)根据关系模式,划分出系统的功能模块,以C#中的类实现。
类是C#语言的核心,C#的一切类型都是类。
类是一个数据结构,类定义数据类型的数据(字段)和行为(方法和其他函数成员)。
(6)应用数据库访问,即使用连接和操作数据库2 参考文献[1]《SQL Server 2005 奥秘》.赵松涛.北京:电子工业出版社,2007。
[2]《SQL Server 2000 培训教程》.余晨,李文炬.北京:清华大学出版社,2001。
[3]《SQL Server 2005 数据库开发应用教程》.孙余党,张军,钟德源.北京:电子工业出版社,2008。
[4]《C#.NET程序设计教程》.江红,于青松.北京:清华大学出版社,2010。
[5]《C#基础与案例开发详解》.王国胜,刘攀,尼春雨.北京:清华大学出版社,2009。
C语言在物流管理中的应用解决方案

C语言在物流管理中的应用解决方案物流管理是现代企业中至关重要的环节之一,能够有效地提高运输效率、降低成本,并确保顺畅的货物流转。
而C语言作为一种高效、灵活的编程语言,可以在物流管理中发挥重要作用。
本文将探讨C语言在物流管理中的应用解决方案。
一、货物跟踪与管理系统货物跟踪与管理是物流管理中的核心任务之一。
通过使用C语言,我们可以开发一个系统,实时追踪货物的位置和状态。
该系统可以通过传感器、GPS等设备获取货物信息,并使用C语言编写的算法实时解析和处理数据。
通过这个系统,物流管理人员可以随时获得关于货物位置、目的地预计达到时间等信息,从而提高货物追踪和管理的效率。
二、货物配送优化货物配送优化是物流管理中的另一个重要方面。
C语言可以用来编写算法,对货物配送路线进行优化,降低运输成本,提高配送效率。
利用C语言编写的算法可以分析货物的数量、目的地等因素,并根据实际情况建立数学模型,通过优化算法实现最佳配送路线的选择。
这样可以减少行驶距离、节约燃料消耗,并且确保货物按时送达。
三、库存管理系统库存管理是物流管理中的重要环节,也是部分企业管理的痛点之一。
利用C语言,我们可以开发一个库存管理系统,帮助企业实时跟踪和管理库存情况。
该系统可以通过C语言编写的程序自动记录进货和出货的时间、数量等信息,实时更新库存数据。
基于这些数据,可以编写算法来进行库存预警和补货提醒。
这样可以大大提高库存管理的效率,并避免因库存过多或过少而导致的问题。
四、运输路线规划在物流管理中,选择最佳的运输路线是一个关键问题。
利用C语言编程,可以实现运输路线的规划和优化。
通过C语言的算法,可以计算出不同路线的时间、成本等指标,并进行对比。
根据这些指标,可以选择最佳的运输路线,减少运输时间和成本。
此外,C语言还可以结合与其他领域的知识,如地理信息系统等,实现更加精确和智能的运输路线规划。
总结:本文探讨了C语言在物流管理中的应用解决方案。
通过C语言编程,我们可以开发货物跟踪与管理系统,货物配送优化系统,库存管理系统和运输路线规划系统。
快递管理系统c语言课程设计

快递管理系统c语言课程设计一、课程目标知识目标:1. 让学生掌握C语言中数据类型、变量、运算符、控制结构等基本知识,并能运用到快递管理系统的设计与实现中。
2. 使学生了解文件操作在C语言中的应用,能够实现快递信息的存储和读取。
3. 帮助学生理解结构体在C语言中的作用,能够使用结构体存储和管理快递信息。
技能目标:1. 培养学生运用C语言进行程序设计的能力,能够独立完成快递管理系统的模块编写。
2. 提高学生分析问题、解决问题的能力,能够针对快递管理系统中的具体需求进行有效设计和实现。
3. 培养学生团队协作能力,通过分组合作完成课程设计,提高沟通与协作水平。
情感态度价值观目标:1. 培养学生对待编程的兴趣和热情,激发他们主动探索新知识的精神。
2. 培养学生认真负责的态度,对待课程设计任务能够严谨、细心、精益求精。
3. 引导学生关注快递行业的发展,了解快递管理系统在实际应用中的重要性,提高社会责任感。
课程性质:本课程设计旨在让学生将所学的C语言知识运用到实际项目中,提高编程实践能力和问题解决能力。
学生特点:学生已具备一定的C语言基础,但缺乏实际项目经验,需在课程设计中巩固和提高。
教学要求:教师需根据学生特点和课程性质,分解课程目标为具体的学习成果,注重引导学生动手实践,提高编程能力。
同时,关注学生的情感态度价值观培养,使他们在完成课程设计的过程中得到全面发展。
二、教学内容1. 快递管理系统需求分析:介绍快递管理系统的功能需求,分析系统所需处理的数据和业务流程。
2. C语言基础知识回顾:回顾数据类型、变量、运算符、控制结构等基本知识,为后续编程打下基础。
3. 文件操作:讲解C语言中文件操作的相关知识,包括文件的打开、关闭、读写等操作,为存储和读取快递信息提供技术支持。
4. 结构体与链表:介绍结构体的定义和使用,以及如何利用链表实现快递信息的动态存储和管理。
5. 函数设计与实现:分析快递管理系统中的功能模块,引导学生设计相应的函数,实现模块功能。
物流信息管理系统(C语言源程序)

FILE*fp;
goodsnode*p;
if((fp =fopen("goods、txt",”r"))==NULL)
ﻩ{
ﻩprintf("无任何货品信息,请新建!\n\n");
ﻩ}
else
{
ﻩfseek(fp,0,SEEK_END);
ﻩint n,i;
ﻩﻩﻩn= ftell(fp)/sizeof(structgoods);
ﻩﻩintn,i;
ﻩn = ftell(fp)/sizeof(structs〈n;i++)
ﻩﻩ{
ﻩﻩﻩp =(stuffnode*)malloc(sizeof(stuffnode));
ﻩﻩﻩfread(&p->s,sizeof(p->s),1,fp);ﻩﻩﻩ//从文件FP中读取数据到P中
ﻩrewind(fp);
ﻩﻩﻩfor(i=0;i<n;i++)
ﻩ{
ﻩﻩﻩﻩp=(goodsnode *)malloc(sizeof(goodsnode));
ﻩﻩﻩfread(&p->g,sizeof(p—〉g),1,fp);
ﻩp->next= NULL;
ﻩﻩif(goodshead==NULL)
ﻩﻩ{
};
struct goods//货品数据结构体
{
char number[20];
ﻩcharname[20];
intquantity;
charcost[20];
char volume[20];
charweight[20];
};
typedefstruct a
{
ﻩstruct stuffs;
c语言课程设计快递管理

c语言课程设计快递管理一、教学目标本章节的教学目标是使学生掌握C语言编程的基本技能,能够运用C语言实现简单的快递管理系统。
具体分为以下三个部分:1.知识目标:使学生掌握C语言的基本语法、数据类型、运算符、控制结构等基本知识。
2.技能目标:使学生能够使用C语言进行程序设计,具备基本的编程能力。
能够运用C语言实现简单的快递管理系统。
3.情感态度价值观目标:培养学生对计算机科学的兴趣和热情,提高学生解决问题的能力,培养学生的创新精神和团队合作意识。
二、教学内容本章节的教学内容主要包括以下几个部分:1.C语言的基本语法和数据类型。
2.C语言的运算符和控制结构。
3.C语言的函数和数组。
4.快递管理系统的需求分析和设计。
5.快递管理系统的实现。
三、教学方法为了达到本章节的教学目标,我们将采用以下几种教学方法:1.讲授法:用于讲解C语言的基本语法、数据类型、运算符、控制结构等基本知识。
2.案例分析法:通过分析实际的快递管理案例,使学生更好地理解快递管理系统的需求分析和设计。
3.实验法:让学生通过动手实践,运用C语言实现简单的快递管理系统。
四、教学资源为了支持本章节的教学内容和教学方法的实施,我们将准备以下教学资源:1.教材:《C语言程序设计》。
2.多媒体资料:包括C语言的语法和数据类型的PPT讲解,快递管理系统的案例分析等。
3.实验设备:计算机、网络等。
五、教学评估为了全面、公正地评估学生在本次课程中的学习成果,我们将采取以下评估方式:1.平时表现:通过学生在课堂上的参与度、提问回答、小组讨论等表现,评估其对C语言编程的理解和应用能力。
2.作业:布置相关的编程作业,评估学生对C语言编程的掌握程度,以及解决问题的能力。
3.考试:进行期中和期末考试,以闭卷的形式,评估学生对C语言编程知识的掌握程度和应用能力。
六、教学安排本章节的教学安排如下:1.教学进度:按照教材的章节顺序,逐步讲解C语言的基本语法、数据类型、运算符、控制结构等基本知识,然后进行快递管理系统的需求分析和设计,最后实现快递管理系统。
基于C的物流管理系统设计与实施
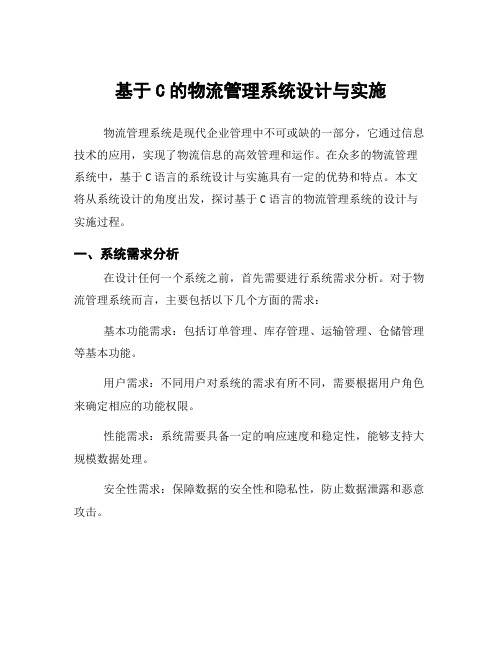
基于C的物流管理系统设计与实施物流管理系统是现代企业管理中不可或缺的一部分,它通过信息技术的应用,实现了物流信息的高效管理和运作。
在众多的物流管理系统中,基于C语言的系统设计与实施具有一定的优势和特点。
本文将从系统设计的角度出发,探讨基于C语言的物流管理系统的设计与实施过程。
一、系统需求分析在设计任何一个系统之前,首先需要进行系统需求分析。
对于物流管理系统而言,主要包括以下几个方面的需求:基本功能需求:包括订单管理、库存管理、运输管理、仓储管理等基本功能。
用户需求:不同用户对系统的需求有所不同,需要根据用户角色来确定相应的功能权限。
性能需求:系统需要具备一定的响应速度和稳定性,能够支持大规模数据处理。
安全性需求:保障数据的安全性和隐私性,防止数据泄露和恶意攻击。
二、系统架构设计基于C语言的物流管理系统通常采用客户端/服务器(Client/Server)架构,其中客户端负责与用户交互,服务器端负责处理业务逻辑和数据存储。
整体架构如下:客户端:提供用户界面,接收用户输入,并将请求发送给服务器端进行处理。
服务器端:负责处理客户端请求,执行相应的业务逻辑,并与数据库交互进行数据处理。
三、数据库设计数据库设计是物流管理系统中至关重要的一环,它直接影响到系统的性能和稳定性。
在设计数据库时,需要考虑以下几个方面:数据表设计:根据系统需求设计相应的数据表,包括订单表、库存表、运输表等。
索引设计:合理设置索引可以提高数据库查询效率,加快数据检索速度。
关系建立:不同数据表之间可能存在关联关系,需要建立相应的外键约束来保证数据完整性。
四、功能模块设计基于C语言的物流管理系统通常包括以下几个功能模块:订单管理模块:实现订单的创建、修改、查询等功能。
库存管理模块:对库存进行实时监控,确保货物供应充足。
运输管理模块:安排货物运输路线、运输工具等信息。
仓储管理模块:对仓库内货物进行管理和调度。
五、系统实施与测试在完成系统设计后,需要进行系统实施和测试。
物流信息管理系统c语言大作业

物流信息管理系统1. 简介物流信息管理系统是一个基于C语言开发的大型软件工程项目,旨在帮助企业高效地管理物流运输过程中的各种信息,提升物流管理的效率和准确性。
该系统可以实现货物的跟踪、配送、库存管理等功能,并提供了数据分析和报表生成等辅助功能。
2. 功能需求该物流信息管理系统具备以下主要功能:2.1 货物跟踪系统能够根据货物的运输单号或其他标识符,查询并显示货物的当前位置和状态。
用户可以通过输入货物相关信息,实时追踪货物的运输情况,从而了解货物的到达时间和目的地等重要信息。
2.2 配送管理系统可以记录和管理货物的配送过程。
用户可以输入配送员信息、收件人信息以及货物详细描述等相关内容,系统会自动分配合适的配送员,并生成相应的配送路线和时间表。
系统还能够实时更新配送进度,并提供签收确认功能。
2.3 库存管理系统能够对仓库中的货物进行管理。
用户可以查看当前仓库中所有货物的数量、状态以及存放位置等信息。
系统还具备自动库存报警功能,当货物库存低于设定的阈值时,系统会自动发送提醒通知,以便及时采购或调拨货物。
2.4 数据分析与报表生成系统具备数据分析和报表生成功能,可以根据用户需求生成各类统计报表。
用户可以选择特定时间段、地区、货物类型等条件,系统会根据这些条件进行数据筛选和分析,并生成相应的报表,帮助用户了解物流运输过程中的各项指标和趋势。
3. 技术实现3.1 界面设计系统采用图形用户界面(GUI)进行设计,以提供友好的操作界面。
界面布局清晰明了,便于用户快速上手操作。
界面风格简洁大方,符合现代软件应用的审美要求。
3.2 数据存储与管理系统使用数据库来存储和管理各种物流信息。
数据库采用关系型数据库管理系统(如MySQL)或非关系型数据库(如MongoDB),以满足不同规模企业的需求。
通过数据库,系统能够高效地存储、查询和更新各类物流数据。
3.3 数据通信与追踪为实现货物跟踪功能,系统需要与物流公司或第三方接口进行数据通信。
c语言大作业物流配送系统程序
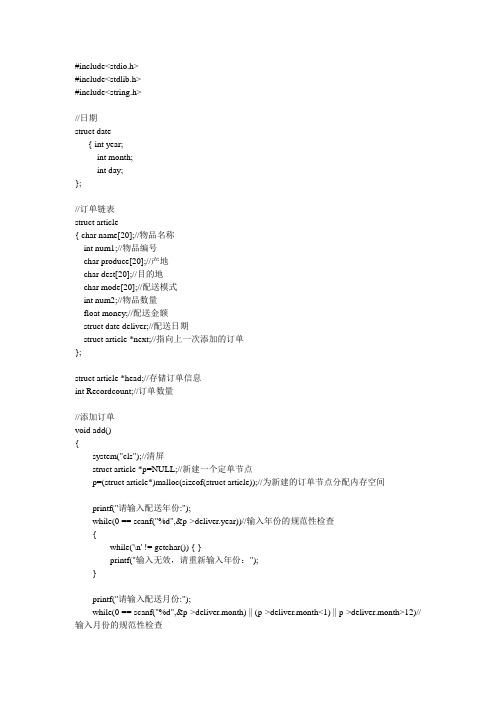
void myPrint(struct article *p)
{
printf("%s\t",p->name);
printf("%d\t",p->num1);
printf("%s\t",p->produce);
printf("%s\t",p->dest);
printf("%s\t",p->mode);
printf("请输入配送年份:");
while(0 == scanf("%d",&p->deliver.year))//输入年份的规范性检查
{
while('\n' != getchar()) { }
printf("输入无效,请重新输入年份:");
}
printf("请输入配送月份:");
while(0 == scanf("%d",&p->deliver.month) || (p->deliver.month<1) || p->deliver.month>12)//输入月份的规范性检查
while(0 == scanf("%d",&choice) || (choice!=1 && choice!=2))//输入选择的规范性检查
{
while('\n' != getchar()) { }
printf("输入无效,请重新选择:");
}
if(choice==1)//按物品名称查找订单
C语言实现简单物流方案

C语言实现简单物流方案(计算两地间采取的最短路径)已事先构建好物流表。
发货地,收货地中1,2,3,4分表代表四个不同的省份,数组里的值代表两个省份间的权值(价格,距离,时间,综合)运行结果如下:#include<stdio.h>#define maxsize 1000 //表示两点间不可达,距离为无穷远#define n 4 //结点的数目#define a 5typedef struct smessage{char name[20];double points;int begin;int end;}message;int price[n][n]={{maxsize,15,10,maxsize},{15,maxsize,12,14},{10,12,maxsize,7},{maxsize,14,7,maxsize}},distance[n][n]={{maxsize,24,13,maxsize},{24,maxsize,14,30},{13,14,maxsize,8},{maxsize,30,8,maxsize}},time[n][n]={{maxsize,10,4,maxsize},{10,maxsize,7,11},{4,7,maxsize,3},{maxsize,11,3,maxsize}},all[n][n]={{maxsize,16,9,maxsize},{16,maxsize,11,18},{9,11,maxsize,6},{maxsize,18,6,maxsize}};void dijkstra(int C[][n],int v,int p){int D[n];int P[n],S[n];int i,j,k,v1,pre;int min,max=maxsize,inf=1200;v1=v-1;for(i=0;i<n;i++){D[i]=C[v1][i]; //置初始距离值if(D[i]!=max)P[i]=v;elseP[i]=0;}for(i=0;i<n;i++)S[i]=0; //红点集S开始为空S[v1]=1;D[v1]=0; //开始点v送Sfor(i=0;i<n-1;i++) //扩充红点集{min=inf;//令inf>max,保证距离值为max的蓝点能扩充到S中for(j=0;j<n;j++)//在当前蓝点中选距离值最小的点k+1{if((!S[j])&&(D[j]<min)){min=D[j];k=j;}}S[k]=1; //将k+1加入红点集for(j=0;j<n;j++){if((!S[j])&&(D[j]>D[k]+C[k][j]))//调整各蓝点的距离值{D[j]=D[k]+C[k][j]; //修改蓝点j+1的距离P[j]=k+1; //k+1是j+1的前趋}}} //所有顶点均已扩充到S中printf("%d到%d的最优方案为",v,p);printf("%d\n",D[p-1]); //打印结果pre=P[p-1];printf("路径:%d",p);while(pre!=0) //继续找前趋顶点{printf("<——%d",pre);pre=P[pre-1];}printf("\n");} //dijkstravoid sort(struct smessage *p){message b;for(int i=0;i<a;i++){for(int j=i+1;j<a;j++){if(p[i].points<p[j].points){b=p[i];p[i]=p[j];p[j]=b;}}}}int main(){while(1){message mes[a]={0};printf("——————欢迎来到物流系统————————\n");printf("请依次输入货品的名称,发货地,收货地,会员积分,按-1退出\n"); for(int i=0;i<5;i++){scanf("%s %d %d %d",&mes[i].name,&mes[i].begin,&mes[i].end,&mes[i].points); }sort(mes);printf("根据会员积分,按以下顺序进行发货\n");for(int i=0;i<5;i++){printf("%s %d %d %d\n",mes[i].name,mes[i].begin,mes[i].end,mes[i].points); }printf("请选择相应的物流方案\n") ;printf("1.价格最小\n2.距离最短\n3.时间最短\n4.综合最优\n按-1退出\n"); while(1){int z;scanf("%d",&z);switch(z){case 1:{for(int i=0;i<a;i++)dijkstra(price,mes[i].begin,mes[i].end);break;}case 2:{for(int i=0;i<a;i++)dijkstra(distance,mes[i].begin,mes[i].end);break;}case 3:{for(int i=0;i<a;i++)dijkstra(time,mes[i].begin,mes[i].end);break;}case 4:{for(int i=0;i<a;i++)dijkstra(all,mes[i].begin,mes[i].end);break;}default:break;}}}return 0;}。
C语言程序设计-物流管理系统
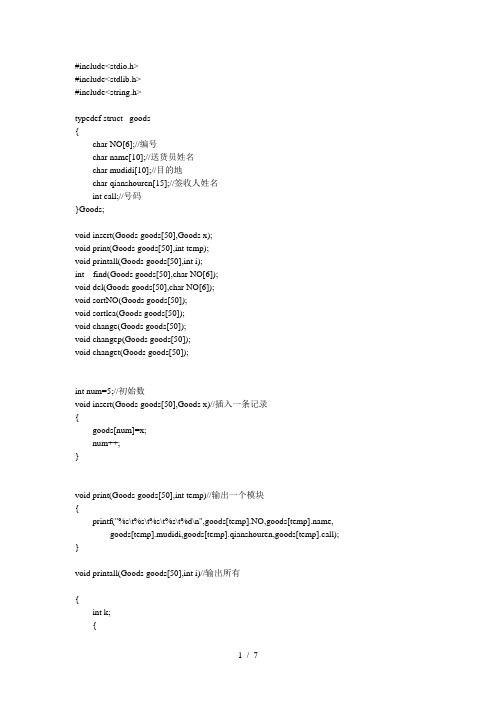
#include<stdio.h>#include<stdlib.h>#include<string.h>typedef struct _goods{char NO[6];//编号char name[10];//送货员姓名char mudidi[10];//目的地char qianshouren[15];//签收人姓名int call;//号码}Goods;void insert(Goods goods[50],Goods x);void print(Goods goods[50],int temp);void printall(Goods goods[50],int i);int find(Goods goods[50],char NO[6]);void del(Goods goods[50],char NO[6]);void sortNO(Goods goods[50]);void sortlea(Goods goods[50]);void change(Goods goods[50]);void changep(Goods goods[50]);void changet(Goods goods[50]);int num=5;//初始数void insert(Goods goods[50],Goods x)//插入一条记录{goods[num]=x;num++;}void print(Goods goods[50],int temp)//输出一个模块{printf("%s\t%s\t%s\t%s\t%d\n",goods[temp].NO,goods[temp].name, goods[temp].mudidi,goods[temp].qianshouren,goods[temp].call); }void printall(Goods goods[50],int i)//输出所有{int k;{printf("\t编号\t名称\t目的地\t签收人\t号码\n");for(k=0;k<num;k++){printf("\t%s\t%s\t%s\t%s\t%d\n ",goods[k].NO,goods[k].name,goods[k].mudidi,goods[k].qianshouren,goods[k].call);}}}int find(Goods goods[50],char NO[6])//查找{int i;for(i=0;i<num;i++){if(strcmp(goods[i].NO,NO)==0)return i;}return -1;}void del(Goods goods[50],char NO[6])//删除{int i;int temp=find(goods,NO);if(temp==-1)printf("不存在!\n");else{for(i=temp+1;i<num;i++){goods[i-1]=goods[i];}num--;}}void sortlea(Goods goods[50])//号码排序函数{int i,j;Goods temp;for(i=num-1;i>0;i--){for(j=0;j<i;j++){if(goods[j].call>goods[j+1].call){temp=goods[j];goods[j]=goods[j+1];goods[j+1]=temp;}elsecontinue;}}printall(goods,1);}void sortNO(Goods goods[50])//订单排序{int i,j;Goods temp;for(i=num-1;i>0;i--){for(j=0;j<i;j++){if(strcmp(goods[j].NO,goods[j+1].NO)>0){temp=goods[j];goods[j]=goods[j+1];goods[j+1]=temp;}elsecontinue;}}printall(goods,1);}void change(Goods goods[50])//修改函数(号码){int i,j;printf("请输入要修改的编号");scanf("%d",&i);printf("将号码改为");scanf("%d",&j);goods[i-00001].call=j;}void changep(Goods goods[50])//修改函数(目的地){int i;char j[10];printf("请输入要修改的编号");scanf("%d",&i);printf("将目的地改为");scanf("%s",j);strcpy(goods[i-00001].mudidi,j);}void changet(Goods goods[50])//修改函数(送货员){int i;char j[10];printf("请输入要修改的编号");scanf("%d",&i);printf("将送货员改为");scanf("%s",j);strcpy(goods[i-00001].mudidi,j);}void main()//主函数开始{Goods goods[50]={{"00001","张三","南京","赵先生",150422},{"00002","李四","常州","钱先生",150423},{"00003","小周","上海","孙先生",150424},{"00004","小王","武汉","李先生",150425},{"00005","小刘","杭州","周先生",150426} };//初始化五个数据Goods x;int i=1,j=1;char NO[6];int temp;while(i){printf("物流查询系统\n");printf("\t1:输入并插入订单\n");printf("\t2:按订单号查找\n");printf("\t3:删除一个订单\n");printf("\t4:排序(仅号码)\n");printf("\t5:输出订单的信息(顺序)\n");printf("\t6:修改数据\n");printf("\t0:退出\n");printf("输入项目序号:\n");scanf("%d",&i);switch (i){case 1:printf("请输入要插入订单的编号、送货员、目的地、签收人、号码,用空格隔开:\n");scanf("%s %s %s%s%d",x.NO,,&x.mudidi,&x.qianshouren,&x.call);insert(goods,x);printf("插入后的数据:\n");printall(goods,0);printf("\n");break;case 2:printf("请输入要查找订单的编号:");scanf("\n");gets(NO);temp=find(goods,NO);if(temp==-1)printf("ERROR!订单不存在!");else{printf("编号\t名称\t目的地\t签收人\t号码\n");print(goods,temp);}printf("\n");break;case 3:printf("请输入要删除订单的编号:");scanf("\n");gets(NO);del(goods,NO);printf("删除后的结果:\n");printall(goods,0);printf("\n");break;case 4:while(j){printf("排序选项:\n");printf("\t1:按号码排序\n");printf("\t0:返回主菜单\n");printf("输入项目序号:\n");scanf("%d",&j);switch(j){case 1:sortlea(goods);//按号码break;case 0:break;//返回主菜单default:printf("项目不存在!\n");break;}}j=1;printf("\n");break;case 5:sortNO(goods);printf("\n");break;case 6:while(j){printf("\t1:改号码\n");printf("\t2:改目的地\n");printf("\t3:改送货员\n");printf("\t0:返回主菜单\n");printf("输入项目序号:\n");scanf("%d",&j);switch(j){case 1:change(goods);printall(goods,0);break;case 2:changep(goods);printall(goods,0);break;case 3:changet(goods);printall(goods,0);break;case 0:break;default:printf("ERROR,项目不存在!");}}case 0:break;default:printf("项目不存在!\n");break;}}}。
C语言物流管理系统跟踪和优化物流过程

C语言物流管理系统跟踪和优化物流过程物流管理在现代企业运作中扮演着重要的角色。
随着科技的不断发展,物流管理系统的使用变得越来越普遍。
本文将介绍如何利用C语言编写物流管理系统,以跟踪和优化物流过程。
1. 系统概述物流管理系统的目标是实现物流流程的顺畅进行,包括订单管理、货物跟踪、仓储管理、运输管理等。
通过物流管理系统,企业能够实时监控物流信息,实现货物追踪和管理,提高物流效率,降低成本。
2. 数据库设计物流管理系统需要一个强大且高效的数据库来存储和管理各种物流相关的数据。
数据库设计应考虑到以下几个方面:- 客户信息:包括客户名称、联系方式、地址等。
- 订单信息:包括订单编号、订单日期、货物描述、数量等。
- 仓储信息:包括仓库编号、仓库地址、仓库容量等。
- 运输信息:包括运输方式、运输费用、运输路线等。
3. 功能模块物流管理系统可以分为以下几个功能模块,每个功能模块都对应着一个或多个具体的任务:- 订单管理:包括订单的生成、修改和删除等操作。
- 货物跟踪:提供实时的货物跟踪功能,可以根据订单编号或货物信息查询货物的当前位置和状态。
- 仓储管理:负责管理仓库的入库和出库操作,根据货物与仓库的匹配度进行自动调度。
- 运输管理:负责管理物流运输的各项工作,包括运输车辆的调度、路线规划和费用计算等。
4. 系统优化为了提高物流管理系统的效率和性能,可以采取以下优化措施:- 数据库索引的优化:通过合理设置索引,可以加快数据库的查询速度。
- 并发控制和事务管理:有效的并发控制和事务管理可以提高系统的并发性和数据一致性。
- 算法优化:运用合适的算法来实现系统中的各项功能,例如使用最短路径算法进行运输路线规划。
总结:物流管理系统在企业的物流流程中扮演着重要的角色,它能够帮助企业追踪和优化物流过程,提高运输效率和降低成本。
通过C语言的编程,我们可以开发出一个功能强大且高效的物流管理系统,为企业的运营提供有力支持。
以上只是对物流管理系统的一个简要介绍,实际开发中还需要更加详细的设计和实现,希望这篇文章能够对你理解物流管理系统的开发有所帮助。
基于C的智能物流系统的设计与实现

基于C的智能物流系统的设计与实现智能物流系统是利用先进的技术手段,对物流过程进行智能化管理和优化的系统。
在当今信息化社会,智能物流系统已经成为提高物流效率、降低成本、提升服务质量的重要工具。
本文将介绍基于C语言的智能物流系统的设计与实现,包括系统架构设计、功能模块划分、算法实现等内容。
一、系统架构设计智能物流系统的架构设计是系统开发的基础,合理的架构设计可以提高系统的可扩展性和稳定性。
基于C语言的智能物流系统通常包括以下几个核心模块:数据管理模块:负责管理物流系统中的各类数据,包括货物信息、仓储信息、运输信息等。
路径规划模块:根据货物的起始地点和目的地点,通过算法计算最优路径,实现货物的快速运输。
调度模块:根据路径规划模块计算出的最优路径,对运输车辆进行合理调度,确保货物按时送达目的地。
监控模块:实时监控货物运输过程中的各项指标,如车辆位置、货物状态等,及时发现并解决问题。
二、功能模块划分在基于C语言的智能物流系统中,各功能模块之间需要良好的协作,以实现整个系统的高效运行。
下面将对各功能模块进行详细介绍:1. 数据管理模块数据管理模块是整个系统的核心,主要包括以下功能:数据存储:使用文件或数据库存储各类数据,确保数据安全可靠。
数据更新:及时更新数据,保持数据与实际情况一致。
数据查询:提供查询接口,方便其他模块获取所需数据。
2. 路径规划模块路径规划模块是智能物流系统中最关键的功能之一,其主要功能包括:路径计算:根据货物起始地点和目的地点,通过最优化算法计算最短路径。
路况考虑:考虑实时路况信息,避开拥堵路段,提高运输效率。
路径优化:对多个货物进行批量规划,实现多点配送。
3. 调度模块调度模块是保证货物按时送达目的地的关键环节,其主要功能包括:车辆调度:根据路径规划结果和车辆状态,合理调度运输车辆。
任务分配:将货物分配给不同车辆,并安排合适的送货顺序。
异常处理:处理运输途中出现的异常情况,如交通事故、车辆故障等。
C语言下的电商物流管理系统设计与开发
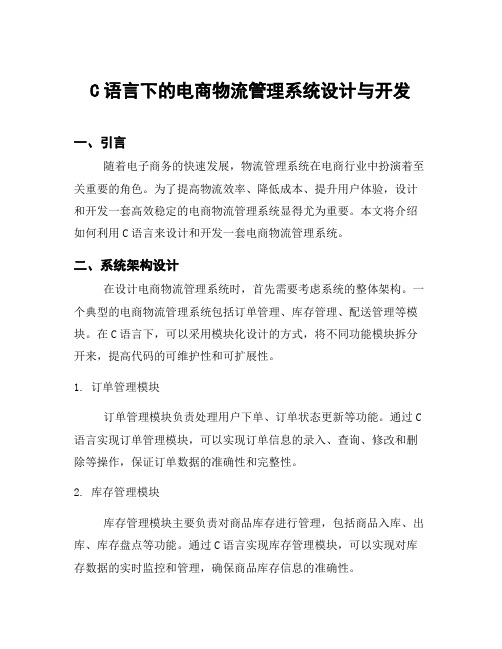
C语言下的电商物流管理系统设计与开发一、引言随着电子商务的快速发展,物流管理系统在电商行业中扮演着至关重要的角色。
为了提高物流效率、降低成本、提升用户体验,设计和开发一套高效稳定的电商物流管理系统显得尤为重要。
本文将介绍如何利用C语言来设计和开发一套电商物流管理系统。
二、系统架构设计在设计电商物流管理系统时,首先需要考虑系统的整体架构。
一个典型的电商物流管理系统包括订单管理、库存管理、配送管理等模块。
在C语言下,可以采用模块化设计的方式,将不同功能模块拆分开来,提高代码的可维护性和可扩展性。
1. 订单管理模块订单管理模块负责处理用户下单、订单状态更新等功能。
通过C 语言实现订单管理模块,可以实现订单信息的录入、查询、修改和删除等操作,保证订单数据的准确性和完整性。
2. 库存管理模块库存管理模块主要负责对商品库存进行管理,包括商品入库、出库、库存盘点等功能。
通过C语言实现库存管理模块,可以实现对库存数据的实时监控和管理,确保商品库存信息的准确性。
3. 配送管理模块配送管理模块负责处理订单配送、配送路线规划等功能。
通过C语言实现配送管理模块,可以实现对配送信息的实时跟踪和调度,提高配送效率和准时率。
三、数据库设计在电商物流管理系统中,数据库设计是至关重要的一环。
合理的数据库设计可以提高系统的数据存储效率和查询速度。
在C语言下,可以使用SQLite等轻量级数据库来存储系统数据。
1. 订单信息表订单信息表包括订单号、商品信息、下单时间、订单状态等字段,用于存储用户下单信息。
2. 库存信息表库存信息表包括商品编号、商品名称、库存数量、入库时间等字段,用于存储商品库存信息。
3. 配送信息表配送信息表包括配送单号、配送员信息、配送路线、配送状态等字段,用于存储配送相关信息。
四、功能实现在设计完系统架构和数据库结构后,接下来是功能实现阶段。
通过C语言编写各个功能模块的代码,并进行集成测试,确保系统能够正常运行并满足需求。
c语言课程设计发快递

c语言课程设计发快递一、教学目标本课程旨在通过C语言编程的教学,使学生掌握C语言的基本语法、数据结构、算法和编程思想,培养学生具备基本的程序设计能力。
通过课程的学习,学生应能够熟练使用C语言编写简单的程序,理解程序的基本结构和工作原理,并能够运用C语言解决实际问题。
同时,通过编程实践,培养学生的逻辑思维能力、创新意识和团队协作能力,激发学生对计算机科学的兴趣和热情。
二、教学内容本课程的教学内容主要包括C语言的基本语法、数据结构、算法和编程思想。
具体包括以下几个方面的内容:1.C语言的基本语法:包括变量、数据类型、运算符、表达式、语句等基本概念和用法。
2.数据结构:包括数组、字符串、指针、结构体等数据类型的使用和操作。
3.算法:包括排序算法、查找算法、递归算法等基本算法的设计和实现。
4.编程思想:包括面向过程的编程思想、面向对象的编程思想等。
三、教学方法为了提高学生的学习兴趣和主动性,本课程将采用多种教学方法相结合的方式进行教学。
具体包括以下几种教学方法:1.讲授法:通过讲解C语言的基本语法、数据结构、算法和编程思想,使学生掌握相关知识。
2.案例分析法:通过分析实际编程案例,使学生理解编程思想和方法。
3.实验法:通过上机编程实验,使学生巩固所学知识,培养学生的实际编程能力。
4.讨论法:通过分组讨论和课堂讨论,激发学生的思考,培养学生的团队协作能力和创新意识。
四、教学资源为了支持教学内容和教学方法的实施,丰富学生的学习体验,我们将选择和准备以下教学资源:1.教材:选用权威、实用的C语言编程教材,为学生提供系统、科学的学习材料。
2.参考书:提供相关的C语言编程参考书籍,供学生自主学习和拓展知识。
3.多媒体资料:制作精美的PPT、教学视频等多媒体资料,提高学生的学习兴趣。
4.实验设备:提供计算机实验室,让学生进行上机编程实验,培养实际编程能力。
五、教学评估本课程的教学评估将采取多元化、全过程的评价方式,以全面、客观、公正地评估学生的学习成果。
C语言智能物流管理系统构建

C语言智能物流管理系统构建智能物流管理系统是一个基于C语言的软件系统,旨在帮助企业高效地管理其物流运营。
通过整合订单管理、库存管理、运输管理等模块,系统能够实现货物的快速、安全和准确的运输。
首先,订单管理模块是系统的核心部分之一。
用户可以通过该模块查看订单信息、创建新订单、取消订单等。
订单信息包括客户信息、货物信息、运输方式、送货地址等,用户可以根据需要进行查阅和修改。
系统还能够自动生成订单编号和跟踪号码,方便用户追踪订单状态。
其次,库存管理模块是系统的另一重要组成部分。
用户可以在该模块中添加货物信息、查看库存数量、进行库存盘点等操作。
系统还提供了自动发货和自动补货的功能,能够根据库存情况自动生成出货和进货订单,帮助企业及时补充货物,提高库存周转率。
另外,运输管理模块是系统的关键组成部分之一。
用户可以在该模块中查看运输路线、调度车辆、跟踪货物位置等。
系统能够实时监测货物的位置和状态,确保货物能够按时到达目的地。
同时,系统还可以优化运输路线,降低物流成本,提高运输效率。
除了以上几个主要模块外,系统还包括报表生成、权限管理、用户管理等功能,为用户提供全面的物流管理解决方案。
用户可以根据需要生成各种报表,例如销售分析报表、库存盘点报表等,帮助企业了解业务运营情况。
同时,系统还支持多级权限管理,管理员可以根据用户需求设置不同的权限,保护企业数据安全。
总的来说,C语言智能物流管理系统具有简单易用、功能全面、效率高的特点,能够帮助企业提升物流运营效率,降低物流成本,增强竞争力。
希望通过不断优化和升级,系统能够更好地满足企业的物流管理需求,促进企业的持续发展。
C语言在智能物流配送系统中的应用技术研究
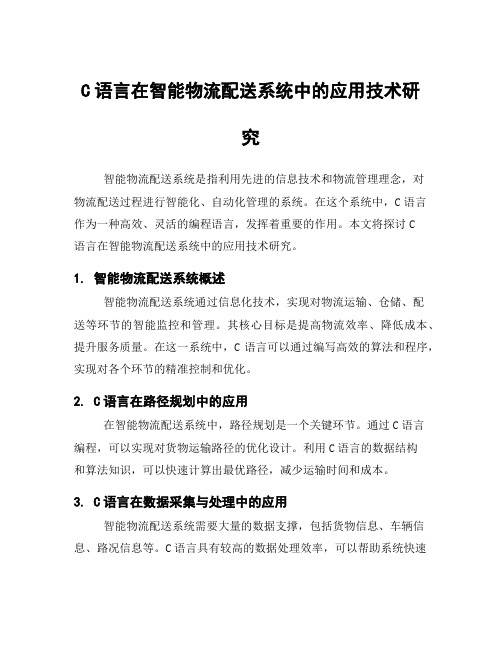
C语言在智能物流配送系统中的应用技术研究智能物流配送系统是指利用先进的信息技术和物流管理理念,对物流配送过程进行智能化、自动化管理的系统。
在这个系统中,C语言作为一种高效、灵活的编程语言,发挥着重要的作用。
本文将探讨C语言在智能物流配送系统中的应用技术研究。
1. 智能物流配送系统概述智能物流配送系统通过信息化技术,实现对物流运输、仓储、配送等环节的智能监控和管理。
其核心目标是提高物流效率、降低成本、提升服务质量。
在这一系统中,C语言可以通过编写高效的算法和程序,实现对各个环节的精准控制和优化。
2. C语言在路径规划中的应用在智能物流配送系统中,路径规划是一个关键环节。
通过C语言编程,可以实现对货物运输路径的优化设计。
利用C语言的数据结构和算法知识,可以快速计算出最优路径,减少运输时间和成本。
3. C语言在数据采集与处理中的应用智能物流配送系统需要大量的数据支撑,包括货物信息、车辆信息、路况信息等。
C语言具有较高的数据处理效率,可以帮助系统快速准确地采集和处理各类数据。
同时,C语言也可以实现数据的实时更新和同步,保证系统运行的及时性和准确性。
4. C语言在任务调度中的应用任务调度是智能物流配送系统中的重要组成部分。
通过C语言编程,可以实现对各项任务的合理调度和分配。
利用C语言多线程编程技术,可以实现多任务并行处理,提高系统整体运行效率。
5. C语言在异常处理中的应用在实际运行过程中,智能物流配送系统可能会遇到各种异常情况,如交通堵塞、设备故障等。
C语言可以通过异常处理机制,及时捕获和处理异常情况,保证系统稳定可靠地运行。
6. 结语综上所述,C语言作为一种高效、灵活的编程语言,在智能物流配送系统中发挥着重要作用。
通过对路径规划、数据采集与处理、任务调度、异常处理等方面的应用研究,可以进一步提升智能物流配送系统的管理水平和运行效率。
相信随着技术的不断发展和完善,C语言在智能物流领域的应用将会更加广泛和深入。
c语言大作业物流配送系统-文档
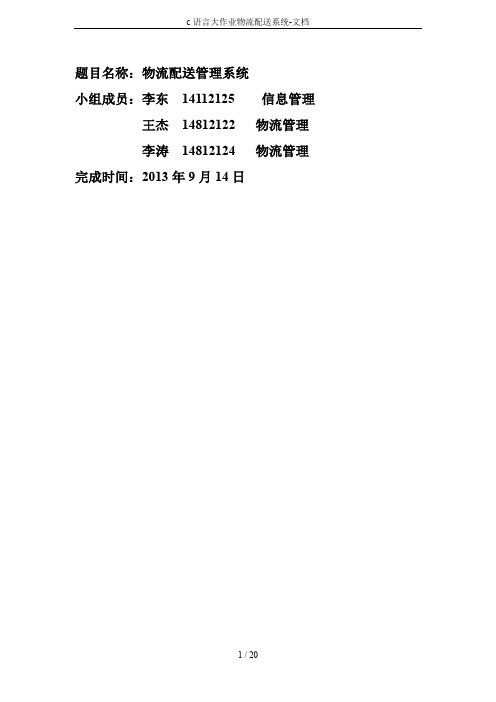
题目名称:物流配送管理系统小组成员:李东14112125 信息管理王杰14812122 物流管理李涛14812124 物流管理完成时间:2013年9月14日目录一、案例描述 (3)二、界面设计 (4)三、模块化设计方案 (5)四、数据结构描述 (7)五、算法设计 (8)六、程序运行结果 (12)七、总结 (18)一、案例描述1.总体描述:物流配送管理系统主要针对物流公司的货物进行添加、删除、更改、查询等操作,实现物流配送管理最基本的功能。
2模块描述:1)菜单描述:本系统提供简单易操作的界面。
有提示,帮助用户根据需要对物流管理系统进行操作。
2)添加模块:即添加新增的物流配送基本信息。
3)显示模块:即在屏幕上显示每个物流配送基本信息。
4)存储模块:即将每个物流配送基本信息保存在一个文件中。
5)查询模块:按物品名称或物品编号查询每个物流配送基本信息。
6)更改模块:可更改每个物流配送基本信息。
7)删除模块:可删除每个物流配送基本信息8)退出模块:退出物流配送管理管理系统并保存文件。
二、界面设计界面有相应的选择,根据自己的需要对该管理系统进行添加,查找,删除,显示功能操作。
本软件界面友好,不难操作,用户可根据自己的需要按提示进行。
三、模块化设计方案1.系统主要函数及功能1)DataInit函数:功能为数据初始化,将数据从硬盘传到内存。
2)add函数:功能为添加货物的基本信息3)search函数:功能为查找货物,输入关键字,从文件中搜索相应的记录并显示出来。
4)change函数:功能为更改货物的基本信息5)delete_rec函数:功能为删除货物的基本信息,若某货物已经配送出去或者不需要则可以删除。
5)show函数:功能为显示货物的基本信息,将添加的显示在屏幕上。
、6)save函数:功能为存储货物的基本信息7)quit函数:功能为保存信息退出系统。
2.主函数调用各功能函数流程:四、数据结构描述struct date{ int year;int month;int day;};struct article{ char name[20];int num1;char produce[20];char dest[20];char mode[20];int num2;float money;struct date deliver;struct article *next;};该数据结构为链表,是一种动态进行存储分配的结构。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
#include<stdio.h>#include<stdlib.h>#include<string.h>//日期struct date{ int year;int month;int day;};//订单链表struct article{ char name[20];//物品名称int num1;//物品编号char produce[20];//产地char dest[20];//目的地char mode[20];//配送模式int num2;//物品数量float money;//配送金额struct date deliver;//配送日期struct article *next;//指向上一次添加的订单};struct article *head;//存储订单信息int Recordcount;//订单数量//添加订单void add(){system("cls");//清屏struct article *p=NULL;//新建一个定单节点p=(struct article*)malloc(sizeof(struct article));//为新建的订单节点分配内存空间printf("请输入配送年份:");while(0 == scanf("%d",&p->deliver.year))//输入年份的规范性检查{while('\n' != getchar()) { }printf("输入无效,请重新输入年份:");}printf("请输入配送月份:");while(0 == scanf("%d",&p->deliver.month) || (p->deliver.month<1) || p->deliver.month>12)//输入月份的规范性检查{while('\n' != getchar()) { }printf("输入无效,请重新输入月份:");}printf("请输入配送日期:");scanf("%d",&p->deliver.day);printf("请输入物品名称:");scanf("%s",p->name);printf("请输入物品编号:");scanf("%d",&p->num1);printf("请输入物品出厂地:");scanf("%s",p->produce);printf("请输入物品配送地:");scanf("%s",p->dest);printf("请输入配送方式:");scanf("%s",p->mode);printf("请输入配送个数:");scanf("%d",&p->num2);printf("请输入配送金额:");scanf("%f",&p->money);p->next=head;//将新建的订单加入订单链表head=p;//链表头指向新添加的定单节点Recordcount++;//订单数量加一system("PAUSE");}//显示指定订单的详细信息void myPrint(struct article *p){printf("%s\t",p->name);printf("%d\t",p->num1);printf("%s\t",p->produce);printf("%s\t",p->dest);printf("%s\t",p->mode);printf("%d\t",p->num2);printf("%.2f\t",p->money);printf("%d-%d-%d\n",p->deliver.year,p->deliver.month,p->deliver.day); }//显示所有的订单信息void show(){system("cls");//遍历订单链表struct article *p=NULL;printf("********************************************************************* *******\n");printf("名称\t编号\t产地\t目的地\t模式\t数量\t金额\t日期\n");for(p=head;p!=NULL;p=p->next){myPrint(p);}printf("********************************************************************* *******\n");}//查找订单void search(){system("cls");char namesea[30];//名称int type;//编号int choice;struct article *p=NULL;printf("1按姓名查找\n2按编号查找\n ");printf("请输入你的选择:");//scanf("%d",&choice);while(0 == scanf("%d",&choice) || (choice!=1 && choice!=2))//输入选择的规范性检查{while('\n' != getchar()) { }printf("输入无效,请重新选择:");}if(choice==1)//按物品名称查找订单{printf("请输入物品名称:");scanf("%s",namesea);for(p=head;p!=NULL;p=p->next)if(strcmp(p->name,namesea)==0)//找到订单,输出其详细信息{printf("********************************************************************* *******\n");printf("名称\t编号\t产地\t目的地\t模式\t数量\t金额\t日期\n");myPrint(p);printf("********************************************************************* *******\n");}}else//按物品编号查找订单{printf("请输入物品编号\n ");scanf("%d",&type);for(p=head;p!=NULL;p=p->next)if(p->num1==type)//找到订单,输出其详细信息{printf("********************************************************************* *******\n");printf("名称\t编号\t产地\t目的地\t模式\t数量\t金额\t日期\n");myPrint(p);printf("********************************************************************* *******\n");}}system("PAUSE");}//根据物品名称修改对应订单信息void change(){char name[30];system("cls");struct article *p=NULL;printf("请输入要更改物品的名称:\n");scanf("%s",name);//遍历订单链表,找到对应的订单for(p=head;p!=NULL;p=p->next)if(strcmp(p->name,name)==0)//找到对应的订单,重新输入其信息{printf("请输入配送年份:");while(0 == scanf("%d",&p->deliver.year))//输入年份的规范性检查{while('\n' != getchar()) { }printf("输入无效,请重新输入年份:");}printf("请输入配送月份:");while(0 == scanf("%d",&p->deliver.month) || (p->deliver.month<1) || p->deliver.month>12)//输入月份的规范性检查{while('\n' != getchar()) { }printf("输入无效,请重新输入月份:");}printf("请输入配送日期:");scanf("%d",&p->deliver.day);printf("请输入物品名称:");scanf("%s",p->name);printf("请输入物品编号:");scanf("%d",&p->num1);printf("请输入物品出厂地:");scanf("%s",p->produce);printf("请输入物品配送地:");scanf("%s",p->dest);printf("请输入配送方式:");scanf("%s",p->mode);printf("请输入配送个数:");scanf("%d",&p->num2);printf("请输入配送金额:");scanf("%f",&p->money);}system("pause");}//根据物品名称和配送金额删除对应订单信息void delete_rec(){system("cls");char name[20];float money;int choice;struct article *p=NULL,*q=NULL;p=head;q=head;printf("请输入要删除的物品名称:");scanf("%s",name);printf("请输入金额:");scanf("%f",&money);for(;q!=NULL;q=q->next){if((head->money==money)&&(strcmp(head->name,name)==0))//要删除的订单为订单列表中的第一个订单{printf("********************************************************************* *******\n");printf("名称\t编号\t产地\t目的地\t模式\t数量\t金额\t日期\n");myPrint(q);printf("********************************************************************* *******\n");printf("确认删除?\n 1是\n 2否\n");//scanf("%d",&choice);while(0 == scanf("%d",&choice) || (choice!=1 && choice!=2))//输入选择的规范性检查{while('\n' != getchar()) { }printf("输入无效,请重新选择:");}if(choice==1)//删除{head=q->next;Recordcount--;}else break;//取消删除}else//要删除的订单不是订单列表中的第一个订单{if((q->money==money)&&(strcmp(q->name,name)==0))//找到对应的订单进行删除{printf("********************************************************************* *******\n");printf("名称\t编号\t产地\t目的地\t模式\t数量\t金额\t日期\n");myPrint(q);printf("********************************************************************* *******\n");printf("确认删除?\n 1是\n 2否\n");//scanf("%d",&choice);while(0 == scanf("%d",&choice) || (choice!=1 && choice!=2))//输入选择的规范性检查{while('\n' != getchar()) { }printf("输入无效,请重新选择:");}if(choice==1)//删除{p->next=q->next;Recordcount--;}else break;//取消删除}}}}//存储订单链表到文件void logistic(){char ch;charfile_head[]="******************************************************************** ********\n名称\t编号\t产地\t目的地\t模式\t数量\t金额\t日期\n";charfile_tail[]="********************************************************************* *******\n";FILE *fp;if((fp=fopen(".\\record.txt"/*filename*/,"w"))==NULL){printf("无法打开文件\n");exit(0);}fputs(file_head,fp);//写入文件头struct article *p=NULL;for(p=head;p!=NULL;p=p->next)//遍历订单链表,将各个订单信息逐次写入文件{fputs(p->name,fp);fprintf(fp,"\t%d\t",p->num1);fputs(p->produce,fp);fprintf(fp,"\t","");fputs(p->dest,fp);fprintf(fp,"\t","");fputs(p->mode,fp);fprintf(fp,"\t%d\t",p->num2);fprintf(fp,"%.2f\t",p->money);fprintf(fp,"%d-%d-%d\n",p->deliver.year,p->deliver.month,p->deliver.day);}fputs(file_tail,fp);rewind(fp);fclose(fp);printf("所有订单信息已经存入ecord.txt文件中!\n");}//打印主菜单void menu(){system("cls");printf("************************************\n");printf("欢迎使用物流配送管理系统\n");printf("************************************\n");printf("1:添加记录\n");printf("2:显示记录\n");printf("3:存储记录\n");printf("4:查询记录\n");printf("5:更改记录\n");printf("6:删除记录\n");printf("7:退出\n");}void main(){ int n,b=1,s;struct article *head=NULL;void add();void show();void logistic();void search();void change();void delete_rec();void menu();while(b==1){menu();printf("请输入您的选择:\n");while(0 == scanf("%d",&n)){while('\n' != getchar()) { }printf("输入无效,请重新输入!\n");}switch(n)//系统功能调用{case 1: add();break;case 2: show();break;case 3: logistic();break;case 4: search();break;case 5: change();break;case 6: delete_rec();break;case 7: exit(0);break;default :printf("error\n");}printf("是否返回主菜单? 0是1否\n");while(0 == scanf("%d",&s) || (s!=0 && s!=1)){while('\n' != getchar()) { }printf("输入无效!\n");}b=b+s;}}。