DES算法源代码
DES加密算法代码
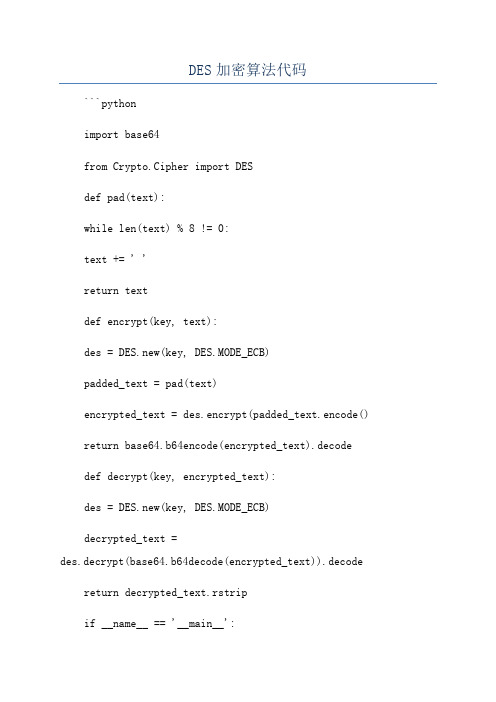
DES加密算法代码```pythonimport base64from Crypto.Cipher import DESdef pad(text):while len(text) % 8 != 0:text += ' 'return textdef encrypt(key, text):des = DES.new(key, DES.MODE_ECB)padded_text = pad(text)encrypted_text = des.encrypt(padded_text.encode() return base64.b64encode(encrypted_text).decodedef decrypt(key, encrypted_text):des = DES.new(key, DES.MODE_ECB)decrypted_text =des.decrypt(base64.b64decode(encrypted_text)).decode return decrypted_text.rstripif __name__ == '__main__':key = b'secretkey' # 8字节密钥plaintext = 'Hello, World!'encrypted_text = encrypt(key, plaintext)print('Encrypted text:', encrypted_text)decrypted_text = decrypt(key, encrypted_text)print('Decrypted text:', decrypted_text)```在上述代码中,我们首先导入了`base64`和`Crypto.Cipher`模块,用于进行编码和DES加密操作。
然后定义了两个辅助函数:`pad`用于填充文本,保证其长度能够被8整除;`encrypt`用于进行DES加密操作;`decrypt`用于进行DES解密操作。
DES子密钥生成算法DES加密算法源代码

DES子密钥生成算法DES加密算法源代码下面是一个Java实现的DES密钥生成算法和DES加密算法的源代码示例:```import javax.crypto.Cipher;import javax.crypto.KeyGenerator;import javax.crypto.SecretKey;import javax.crypto.spec.SecretKeySpec;import java.security.NoSuchAlgorithmException;import java.security.SecureRandom;import java.util.Base64;public class DESExamplepublic static void main(String[] args)try//生成DES密钥SecretKey secretKey = generateDESKey(;System.out.println("生成的DES密钥:" +Base64.getEncoder(.encodeToString(secretKey.getEncoded());//加密String plainText = "Hello, World!";byte[] encryptedText = encrypt(plainText, secretKey);System.out.println("加密后的数据:" +Base64.getEncoder(.encodeToString(encryptedText));//解密byte[] decryptedText = decrypt(encryptedText, secretKey);System.out.println("解密后的数据:" + newString(decryptedText));} catch (Exception e)e.printStackTrace(;}}//生成DES密钥public static SecretKey generateDESKey( throws NoSuchAlgorithmExceptionKeyGenerator keyGenerator = KeyGenerator.getInstance("DES");SecureRandom secureRandom = new SecureRandom(;keyGenerator.init(secureRandom);return keyGenerator.generateKey(;}//加密数据public static byte[] encrypt(String plainText, SecretKey secretKey) throws ExceptionCipher cipher = Cipher.getInstance("DES");cipher.init(Cipher.ENCRYPT_MODE, secretKey);return cipher.doFinal(plainText.getBytes();}//解密数据public static byte[] decrypt(byte[] cipherText, SecretKey secretKey) throws ExceptionCipher cipher = Cipher.getInstance("DES");cipher.init(Cipher.DECRYPT_MODE, secretKey);return cipher.doFinal(cipherText);}```该代码中使用了Java的标准库javax.crypto来实现DES密钥生成和DES加密解密操作。
DES算法的C语言实现

DES算法的C语⾔实现利⽤C语⾔实现DES算法,分组密码原理过程很简单,但是在写的过程中检查了好久才发现错误原因,主要有两点:1.在加密过程16轮迭代过程中,最后⼀轮迭代运算后的结果并没有进⾏交换,即C=IP-1(R16,L16),这样做的⽬的是为了加密解密使⽤同⼀个算法2.在S盒的过程中,移位后应该加括号,否则+的优先级⾼于<<,会出错,下⾯是算法源码:1 #include "des.h"2 #include <stdio.h>3 #include <stdlib.h>45const unsigned char IP_table[64] = {658, 50, 42, 34, 26, 18, 10, 2,760, 52, 44, 36, 28, 20, 12, 4,862, 54, 46, 38, 30, 22, 14, 6,964, 56, 48, 40, 32, 24, 16, 8,1057, 49, 41, 33, 25, 17, 9, 1,1159, 51, 43, 35, 27, 19, 11, 3,1261, 53, 45, 37, 29, 21, 13, 5,1363, 55, 47, 39, 31, 23, 15, 714 };1516const unsigned char IPR_table[64] = {1740, 8, 48, 16, 56, 24, 64, 32,1839, 7, 47, 15, 55, 23, 63, 31,1938, 6, 46, 14, 54, 22, 62, 30,2037, 5, 45, 13, 53, 21, 61, 29,2136, 4, 44, 12, 52, 20, 60, 28,2235, 3, 43, 11, 51, 19, 59, 27,2334, 2, 42, 10, 50, 18, 58, 26,2433, 1, 41, 9, 49, 17, 57, 2525 };2627const unsigned char E_table[48] = {2832, 1, 2, 3, 4, 5,294, 5, 6, 7, 8, 9,308, 9, 10, 11, 12, 13,3112, 13, 14, 15, 16, 17,3216, 17, 18, 19, 20, 21,3320, 21, 22, 23, 24, 25,3424, 25, 26, 27, 28, 29,3528, 29, 30, 31, 32, 136 };3738const unsigned char P_table[32] = {3916, 7, 20, 21, 29, 12, 28, 17,401, 15, 23, 26, 5, 18, 31, 10,412, 8, 24, 14, 32, 27, 3, 9,4219, 13, 30, 6, 22, 11, 4, 2543 };4445const unsigned char PC1_table[56] = {4657, 49, 41, 33, 25, 17, 9,471, 58, 50, 42, 34, 26, 18,4810, 2, 59, 51, 43, 35, 27,4919, 11, 3, 60, 52, 44, 36,5063, 55, 47, 39, 31, 23, 15,517, 62, 54, 46, 38, 30, 22,5214, 6, 61, 53, 45, 37, 29,5321, 13, 5, 28, 20, 12, 454 };5556const unsigned char LOOP_table[16] = {571, 1, 2, 2, 2, 2, 2, 2, 1, 2, 2, 2, 2, 2, 2, 158 };5960const unsigned char PC2_table[48] = {6114, 17, 11, 24, 1, 5,623, 28, 15, 6, 21, 10,6323, 19, 12, 4, 26, 8,6416, 7, 27, 20, 13, 2,6541, 52, 31, 37, 47, 55,6630, 40, 51, 45, 33, 48,6744, 49, 39, 56, 34, 53,6846, 42, 50, 36, 29, 3269 };7071const unsigned char sbox[8][4][16] = {72// S17314, 4, 13, 1, 2, 15, 11, 8, 3, 10, 6, 12, 5, 9, 0, 7,740, 15, 7, 4, 14, 2, 13, 1, 10, 6, 12, 11, 9, 5, 3, 8,754, 1, 14, 8, 13, 6, 2, 11, 15, 12, 9, 7, 3, 10, 5, 0,7615, 12, 8, 2, 4, 9, 1, 7, 5, 11, 3, 14, 10, 0, 6, 13,77//S27815, 1, 8, 14, 6, 11, 3, 4, 9, 7, 2, 13, 12, 0, 5, 10,793, 13, 4, 7, 15, 2, 8, 14, 12, 0, 1, 10, 6, 9, 11, 5,800, 14, 7, 11, 10, 4, 13, 1, 5, 8, 12, 6, 9, 3, 2, 15,8113, 8, 10, 1, 3, 15, 4, 2, 11, 6, 7, 12, 0, 5, 14, 9,82//S38310, 0, 9, 14, 6, 3, 15, 5, 1, 13, 12, 7, 11, 4, 2, 8,8413, 7, 0, 9, 3, 4, 6, 10, 2, 8, 5, 14, 12, 11, 15, 1,8513, 6, 4, 9, 8, 15, 3, 0, 11, 1, 2, 12, 5, 10, 14, 7,861, 10, 13, 0, 6, 9, 8, 7, 4, 15, 14, 3, 11, 5, 2, 12,87//S4887, 13, 14, 3, 0, 6, 9, 10, 1, 2, 8, 5, 11, 12, 4, 15,8913, 8, 11, 5, 6, 15, 0, 3, 4, 7, 2, 12, 1, 10, 14, 9,9010, 6, 9, 0, 12, 11, 7, 13, 15, 1, 3, 14, 5, 2, 8, 4,913, 15, 0, 6, 10, 1, 13, 8, 9, 4, 5, 11, 12, 7, 2, 14,92//S5932, 12, 4, 1, 7, 10, 11, 6, 8, 5, 3, 15, 13, 0, 14, 9,9414, 11, 2, 12, 4, 7, 13, 1, 5, 0, 15, 10, 3, 9, 8, 6,954, 2, 1, 11, 10, 13, 7, 8, 15, 9, 12, 5, 6, 3, 0, 14,9611, 8, 12, 7, 1, 14, 2, 13, 6, 15, 0, 9, 10, 4, 5, 3,97//S69812, 1, 10, 15, 9, 2, 6, 8, 0, 13, 3, 4, 14, 7, 5, 11,9910, 15, 4, 2, 7, 12, 9, 5, 6, 1, 13, 14, 0, 11, 3, 8,1009, 14, 15, 5, 2, 8, 12, 3, 7, 0, 4, 10, 1, 13, 11, 6,1014, 3, 2, 12, 9, 5, 15, 10, 11, 14, 1, 7, 6, 0, 8, 13,102//S71034, 11, 2, 14, 15, 0, 8, 13, 3, 12, 9, 7, 5, 10, 6, 1,10413, 0, 11, 7, 4, 9, 1, 10, 14, 3, 5, 12, 2, 15, 8, 6,1051, 4, 11, 13, 12, 3, 7, 14, 10, 15, 6, 8, 0, 5, 9, 2,1066, 11, 13, 8, 1, 4, 10, 7, 9, 5, 0, 15, 14, 2, 3, 12,107//S810813, 2, 8, 4, 6, 15, 11, 1, 10, 9, 3, 14, 5, 0, 12, 7,1091, 15, 13, 8, 10, 3, 7, 4, 12, 5, 6, 11, 0, 14, 9, 2,1107, 11, 4, 1, 9, 12, 14, 2, 0, 6, 10, 13, 15, 3, 5, 8,1112, 1, 14, 7, 4, 10, 8, 13, 15, 12, 9, 0, 3, 5, 6, 11112 };113114void ByteToBit(unsigned char* out, const unsigned char* in, const int bits){115for(int i=0;i<bits;i++)116out[i]=(in[i/8]>>(7-i%8))&1;117 }118119void BitToByte(unsigned char* out, const unsigned char* in, const int bits){120 memset(out, 0, (bits + 7) / 8);121for(int i=0;i<bits;i++)122out[i/8]|=in[i]<<(7-i%8);123 }124125void Transform(unsigned char* out,const unsigned char* in, const unsigned char* table, const int len){ 126 unsigned char tmp[64] = {0};127for (int i = 0; i < len; i++)128 tmp[i] = in[table[i] - 1];129 memcpy(out, tmp, len);130 }131132void RotateL(unsigned char* in, const int len, int loop){133static unsigned char tmp[64];134 memcpy(tmp, in, len);135 memcpy(in, in + loop, len - loop);136 memcpy(in + len - loop, tmp, loop);137 }138139static unsigned char subKey[16][48] = { 0 };140void setKey(const unsigned char* in){141char key[64] = { 0 };142 ByteToBit(key, in, 64);143char temp[56]={0};144 Transform(temp, key, PC1_table, 56);145for(int i=0;i<16;i++){146 RotateL(temp, 28, LOOP_table[i]);147 RotateL(temp + 28, 28, LOOP_table[i]);148 Transform(subKey[i], temp, PC2_table, 48);149 }150 }151152void xor(unsigned char* in1,const unsigned char* in2,int len){153for(int i=0;i<len;i++)154 in1[i]^=in2[i];155 }156157void sbox_exchange(unsigned char* out,const unsigned char* in){158char row, column;159for (int i = 0; i < 8; i++){160char num = 0;161 row = (in[6 * i]<<1)+ in[6 * i + 5];162 column = (in[6 * i + 1] << 3) + (in[6 * i + 2] << 2) + (in[6 * i + 3] << 1) + in[6 * i + 4]; 163 num = sbox[i][row][column];164for (int j = 0; j < 4; j++)165 {166out[4 * i + j] = (num >> (3 - j)) & 1;167 }168 }169 }170171void F_func(unsigned char* out,const unsigned char* in,unsigned char* subKey){172 unsigned char temp[48]={0};173 unsigned char res[32]={0};174 Transform(temp, in, E_table, 48);175 xor(temp,subKey,48);176 sbox_exchange(res,temp);177 Transform(out, res, P_table, 32);178 }179180void encryptDES(unsigned char* out,const unsigned char* in, const unsigned char* key){ 181 unsigned char input[64] = { 0 };182 unsigned char output[64] = { 0 };183 unsigned char tmp[64] = { 0 };184 ByteToBit(input, in, 64);185 Transform(tmp, input, IP_table, 64);186char* Li = &tmp[0], *Ri = &tmp[32];187 setKey(key);188for(int i=0;i<16;i++){189char temp[32]={0};190 memcpy(temp,Ri,32);191 F_func(Ri, Ri,subKey[i]);192 xor(Ri, Li, 32);193 memcpy(Li,temp,32);194 }195 RotateL(tmp, 64, 32);//the input is LR,output is RL196 Transform(output, tmp, IPR_table, 64);197 BitToByte(out, output,64);198 }199200void decryptDES(unsigned char* out,const unsigned char* in, const unsigned char* key){ 201 unsigned char input[64] = { 0 };202 unsigned char output[64] = { 0 };203 unsigned char tmp[64] = { 0 };204 ByteToBit(input, in, 64);205 Transform(tmp, input, IP_table, 64);206char* Li = &tmp[0], *Ri = &tmp[32];207 setKey(key);208 RotateL(tmp, 64, 32);209for (int i = 0; i < 16; i++){210char temp[32] = { 0 };211 memcpy(temp, Li, 32);212 F_func(Li, Li,subKey[15 - i]);213 xor(Li, Ri, 32);214 memcpy(Ri, temp, 32);215 }216 Transform(output, tmp, IPR_table, 64);217 BitToByte(out, output, 64);218 }。
DES加解密算法C语言源代码
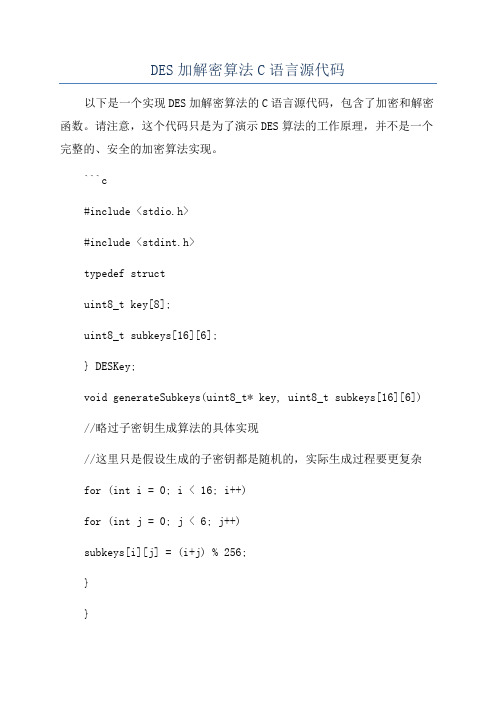
DES加解密算法C语言源代码以下是一个实现DES加解密算法的C语言源代码,包含了加密和解密函数。
请注意,这个代码只是为了演示DES算法的工作原理,并不是一个完整的、安全的加密算法实现。
```c#include <stdio.h>#include <stdint.h>typedef structuint8_t key[8];uint8_t subkeys[16][6];} DESKey;void generateSubkeys(uint8_t* key, uint8_t subkeys[16][6]) //略过子密钥生成算法的具体实现//这里只是假设生成的子密钥都是随机的,实际生成过程要更复杂for (int i = 0; i < 16; i++)for (int j = 0; j < 6; j++)subkeys[i][j] = (i+j) % 256;}}void DES(uint8_t* input, uint8_t key[8], uint8_t* output, int encrypt)//略过DES加密算法的具体实现DESKey desKey;for (int i = 0; i < 8; i++)desKey.key[i] = key[i];}generateSubkeys(key, desKey.subkeys);//这里只是假设输入输出是8字节长,实际上可以支持任意长度//执行加解密操作if (encrypt)printf("Encrypting: ");} elseprintf("Decrypting: ");}for (int i = 0; i < 8; i++)output[i] = encrypt ? input[i] ^ desKey.subkeys[0][i%6] : input[i] ^ desKey.subkeys[15][i%6];printf("%02X ", output[i]);}printf("\n");int maiuint8_t input[8] = {0x12, 0x34, 0x56, 0x78, 0x9A, 0xBC, 0xDE, 0xF0};uint8_t key[8] = {0xA1, 0xB2, 0xC3, 0xD4, 0xE5, 0xF6, 0x07,0x08};uint8_t output[8];DES(input, key, output, 1);DES(output, key, output, 0);return 0;```在这个代码中,`generateSubkeys` 函数用于生成 16 个子密钥,之后分别在加密和解密函数 `DES` 中使用。
DES算法用C++实现的源代码

DES算法用C++实现的源代码用C++实现的源代码#include "memory.h"#include "stdio.h"enum {encrypt,decrypt};//ENCRYPT:加密,DECRYPT:解密void des_run(char out[8],char in[8],bool type=encrypt);//设置密钥void des_setkey(const char key[8]);static void f_func(bool in[32],const bool ki[48]);//f函数static void s_func(bool out[32],const bool in[48]);//s盒代替//变换static void transform(bool *out, bool *in, const char *table, int len);static void xor(bool *ina, const bool *inb, int len);//异或static void rotatel(bool *in, int len, int loop);//循环左移//字节组转换成位组static void bytetobit(bool *out,const char *in, int bits);//位组转换成字节组static void bittobyte(char *out, const bool *in, int bits);//置换IP表conststatic charip_table[64]={58,50,42,34,26,18,10,2,60,52,44,36,28,20,12,4,62,54,46,38,30,22,1 4,6,64,56,48,40,32,24,16,8,57,49,41,33,25,17,9,1,59,51,43,35,27,19,11,3,61,53,4 5,37,29,21,13,5,63,55,47,39,31,23,15,7};//逆置换IP-1表const static charipr_table[64]={40,8,48,16,56,24,64,32,39,7,47,15,55,23,63,31,38,6,46,14,54,22,6 2,30,37,5,45,13,53,21,61,29,36,4,44,12,52,20,60,28,35,3,43,11, 51,19,59,27,34, 2,42,10,50,18,58,26,33,1,41,9,49,17,57,25};//E 位选择表static const char e_table[48]={32,1, 2, 3, 4, 5,4, 5, 6, 7, 8, 9,8, 9,10,11,12,13,12,13,14,15,16,17,16,17,18,19,20,21,20,21,22,23,24,25,24,25,26,27,2 8,29,28,29,30,31,32,1};//P换位表const static charp_table[32]={16,7,20,21,29,12,28,17,1,15,23,26,5,18,31,10,2,8,24,14,32,27,3,9,1 9,13,30,6,22,11,4,25};//pc1选位表const static char pc1_table[56]={57,49,41,33,25,17,9,1 ,58,50,42,34,26,18,10, 2,59,51,43,35,27,19,11,3 ,60,52,44,36,63,55,47, 39,31,23,15,7,62,54,46,3 8,30,22,14,6,61,53,45,37 ,29,21,13,5,28,20,12,4 };//pc2选位表const static char pc2_table[48]={14,17,11, 24,1,5,3,28,15,6,21, 10,23,19,12,4,26,8,16 ,7,27,20,13,2,41,52,3 1,37,47,55,30,40,51,45,33 ,48,44,49,39,56,34,53,46 ,42,50,36,29,32};//左移位数表const static char loop_table[16]={1,1,2,2,2,2,2,2,1,2,2,2,2,2,2,1};//S盒const static char s_box[8][4][16]={//s114, 4, 13, 1, 2, 15, 11, 8, 3, 10, 6, 12, 5, 9, 0, 7,0, 15, 7, 4, 14, 2, 13, 1, 10, 6, 12, 11, 9, 5, 3, 8,4, 1, 14, 8, 13, 6, 2, 11, 15, 12, 9, 7, 3, 10, 5, 0,15, 12, 8, 2, 4, 9, 1, 7, 5, 11, 3, 14, 10, 0, 6, 13,//s215, 1, 8, 14, 6, 11, 3, 4, 9, 7, 2, 13, 12, 0, 5, 10, 3, 13, 4, 7, 15, 2, 8, 14, 12, 0, 1, 10, 6, 9, 11, 5,0, 14, 7, 11, 10, 4, 13, 1, 5, 8, 12, 6, 9, 3, 2, 15,13, 8, 10, 1, 3, 15, 4, 2, 11, 6, 7, 12, 0, 5, 14, 9,//s310, 0, 9, 14, 6, 3, 15, 5, 1, 13, 12, 7, 11, 4, 2, 8,13, 7, 0, 9, 3, 4, 6, 10, 2, 8, 5, 14, 12, 11, 15, 1,13, 6, 4, 9, 8, 15, 3, 0, 11, 1, 2, 12, 5, 10, 14, 7,1, 10, 13, 0, 6, 9, 8, 7, 4, 15, 14, 3, 11, 5, 2, 12,//s47, 13, 14, 3, 0, 6, 9, 10, 1, 2, 8, 5, 11, 12, 4, 15,13, 8, 11, 5, 6, 15, 0, 3, 4, 7, 2, 12, 1, 10, 14, 9,10, 6, 9, 0, 12, 11, 7, 13, 15, 1, 3, 14, 5, 2, 8, 4,3, 15, 0, 6, 10, 1, 13, 8, 9, 4, 5, 11, 12, 7, 2, 14,//s52, 12, 4, 1, 7, 10, 11, 6, 8, 5, 3, 15, 13, 0, 14, 9,14, 11, 2, 12, 4, 7, 13, 1, 5, 0, 15, 10, 3, 9, 8, 6,4, 2, 1, 11, 10, 13, 7, 8, 15, 9, 12, 5, 6, 3, 0, 14,11, 8, 12, 7, 1, 14, 2, 13, 6, 15, 0, 9, 10, 4, 5, 3,//s612, 1, 10, 15, 9, 2, 6, 8, 0, 13, 3, 4, 14, 7, 5, 11,10, 15, 4, 2, 7, 12, 9, 5, 6, 1, 13, 14, 0, 11, 3, 8,9, 14, 15, 5, 2, 8, 12, 3, 7, 0, 4, 10, 1, 13, 11, 6,4, 3, 2, 12, 9, 5, 15, 10, 11, 14, 1, 7, 6, 0, 8, 13,//s74, 11, 2, 14, 15, 0, 8, 13, 3, 12, 9, 7, 5, 10, 6, 1,13, 0, 11, 7, 4, 9, 1, 10, 14, 3, 5, 12, 2, 15, 8, 6,1, 4, 11, 13, 12, 3, 7, 14, 10, 15, 6, 8, 0, 5, 9, 2,6, 11, 13, 8, 1, 4, 10, 7, 9, 5, 0, 15, 14, 2, 3, 12,//s813, 2, 8, 4, 6, 15, 11, 1, 10, 9, 3, 14, 5, 0, 12, 7,1, 15, 13, 8, 10, 3, 7, 4, 12, 5, 6, 11, 0, 14, 9, 2,7, 11, 4, 1, 9, 12, 14, 2, 0, 6, 10, 13, 15, 3, 5, 8,2, 1, 14, 7, 4, 10, 8, 13, 15, 12, 9, 0, 3, 5, 6, 11 };static bool subkey[16][48];//16圈子密钥void des_run(char out[8],char in[8], bool type){static bool m[64],tmp[32],*li=&m[0], *ri=&m[32];bytetobit(m,in,64);transform(m,m,ip_table,64);if(type==encrypt){for(int i=0;i<16;i++){memcpy(tmp,ri,32);f_func(ri,subkey[i]);xor(ri,li,32);memcpy(li,tmp,32);}}else{for(int i=15;i>=0;i--){memcpy(tmp,li,32);f_func(li,subkey[i]);xor(li,ri,32);memcpy(ri,tmp,32);}}transform(m,m,ipr_table,64);bittobyte(out,m,64);}void des_setkey(const char key[8]){static bool k[64], *kl=&k[0], *kr=&k[28];bytetobit(k,key,64);transform(k,k,pc1_table,56);for(int i=0;i<16;i++){rotatel(kl,28,loop_table[i]);rotatel(kr,28,loop_table[i]);transform(subkey[i],k,pc2_table,48);}}void f_func(bool in[32],const bool ki[48]){static bool mr[48];transform(mr,in,e_table,48);xor(mr,ki,48);s_func(in,mr);transform(in,in,p_table,32);}void s_func(bool out[32],const bool in[48]){for(char i=0,j,k;i<8;i++,in+=6,out+=4){j=(in[0]<<1)+in[5];k=(in[1]<<3)+(in[2]<<2)+(in[3]<<1)+in[4];bytetobit(out,&s_box[i][j][k],4);}}void transform(bool *out,bool *in,const char *table,int len){static bool tmp[256];for(int i=0;i<len;i++)tmp[i]=in[table[i]-1];memcpy(out,tmp,len);}void xor(bool *ina,const bool *inb,int len){for(int i=0;i<len;i++)ina[i]^=inb[i];}void rotatel(bool *in,int len,int loop){static bool tmp[256];memcpy(tmp,in,loop);memcpy(in,in+loop,len-loop);memcpy(in+len-loop,tmp,loop);}void bytetobit(bool *out,const char *in,int bits){for(int i=0;i<bits;i++)out[i]=(in[i/8]>>(i%8)) &1;}void bittobyte(char *out,const bool *in,int bits){memset(out,0,(bits+7)/8);for(int i=0;i<bits;i++)out[i/8]|=in[i]<<(i%8);}void main(){char key[8]={'p','r','o','g','r','a','m'},str[8];puts("*****************DES***********************");printf("\n");printf("\n");puts("please input your words");gets(str);printf("\n");puts("****************************************");des_setkey(key);des_run(str,str,encrypt);puts("after encrypting:");puts(str);printf("\n");puts("****************************************");puts("after decrypting:");des_run(str,str,decrypt);puts(str);printf("\n");puts("****************************************");printf("\n");}。
DES算法实现
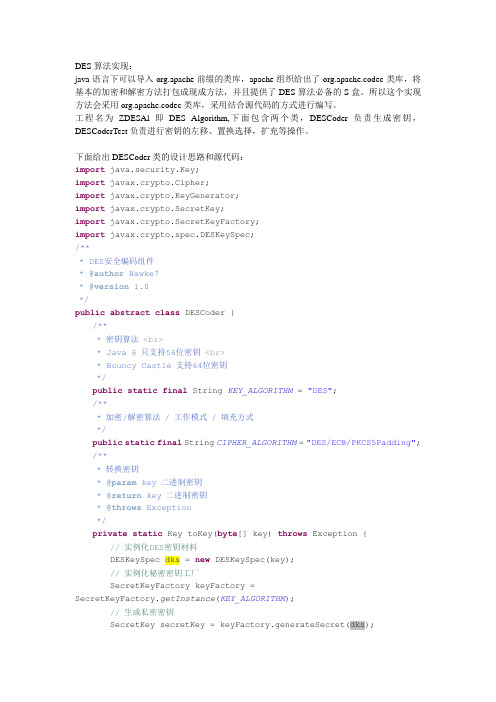
DES算法实现:java语言下可以导入org.apache前缀的类库,apache组织给出了org.apache.codec类库,将基本的加密和解密方法打包成现成方法,并且提供了DES算法必备的S盒。
所以这个实现方法会采用org.apache.codec类库,采用结合源代码的方式进行编写。
工程名为ZDESAl即DES Algorithm,下面包含两个类,DESCoder负责生成密钥,DESCoderTest负责进行密钥的左移、置换选择,扩充等操作。
下面给出DESCoder类的设计思路和源代码:import java.security.Key;import javax.crypto.Cipher;import javax.crypto.KeyGenerator;import javax.crypto.SecretKey;import javax.crypto.SecretKeyFactory;import javax.crypto.spec.DESKeySpec;/***DES安全编码组件*@author Hawke7*@version 1.0*/public abstract class DESCoder {/***密钥算法<br>*Java6只支持56位密钥<br>*Bouncy Castle支持64位密钥*/public static final String KEY_ALGORITHM = "DES";/***加密/解密算法/工作模式/填充方式*/public static final String CIPHER_ALGORITHM= "DES/ECB/PKCS5Padding";/***转换密钥*@param key二进制密钥*@return key二进制密钥*@throws Exception*/private static Key toKey(byte[] key) throws Exception {// 实例化DES密钥材料DESKeySpec dks = new DESKeySpec(key);// 实例化秘密密钥工厂SecretKeyFactory keyFactory =SecretKeyFactory.getInstance(KEY_ALGORITHM);// 生成私密密钥SecretKey secretKey = keyFactory.generateSecret(dks);return secretKey;}/***解密*@param data待解密数据*@param key密钥*@return byte[]解密数据*@throws Exception*/public static byte[] decrypt(byte[] data, byte[] key) throws Exception {// 还原密钥Key k = toKey(key);// 实例化Cipher cipher = Cipher.getInstance(CIPHER_ALGORITHM);// 初始化,设置为解密模式cipher.init(Cipher.DECRYPT_MODE, k);// 执行操作return cipher.doFinal(data);}/***加密*@param data待加密数据*@param key密钥*@return byte[]加密数据*@throws Exception*/public static byte[] encrypt(byte[] data, byte[] key) throws Exception {// 还原密钥Key k = toKey(key);// 实例化Cipher cipher = Cipher.getInstance(CIPHER_ALGORITHM);// 初始化,设置为解密模式cipher.init(Cipher.ENCRYPT_MODE, k);// 执行操作return cipher.doFinal(data);}/***生成密钥<br>*Java6只支持56位密钥<br>*Bouncy Castle支持64位密钥<br>*@return byte[]二进制密钥*@throws Exception*/public static byte[] initKey() throws Exception {/** 实例化密钥生成器* 若要使用64位密钥注意替换* 将下述代码中的* KeyGenerator.getInstance(CIPHER_ALGORITHM);* 替换为* KeyGenerator.getInstance(CIPHER_ALGORITHM, "BC");*/KeyGenerator kg = KeyGenerator.getInstance(KEY_ALGORITHM);/** 初始化密钥生成器* 若要使用64位密钥注意替换* 将下面代码kg.init(56)* 替换为kg.init(64)*/kg.init(56);// 生成私密密钥SecretKey secretKey = kg.generateKey();// 获得密钥的二进制编码形式return secretKey.getEncoded();}}首先DESCoder要定义成抽象类,因为其中全部是static静态方法toKey,toKey初始化密钥材料,并且实例化密钥工厂,因为DES算法要进行16轮迭代,所以工厂每一次都要给出不同的密钥,从而增强雪崩效应。
des加密算法c语言源代码

#include<iostream.h>int IP[64] = {58,50,42,34,26,18,10,2,60,52,44,36,28,20,12,4,62,54,46,38,30,22,14,6,64,56,48,40,32,24,16,8,57,49,41,33,25,17,9,1,59,51,43,35,27,19,11,3,61,53,45,37,29,21,13,5,63,55,47,39,31,23,15,7};int IP_1[64] = {40,8,48,16,56,24,64,32,39,7,47,15,55,23,63,31,38,6,46,14,54,22,62,30,37,5,45,13,53,21,61,29,36,4,44,12,52,20,60,28,35,3,43,11,51,19,59,27,34,2,42,10,50,18,58,26,33,1,41,9,49,17,57,25};int E[48] = {32,1, 2, 3, 4, 5,4, 5, 6, 7, 8, 9,8, 9, 10,11,12,13,12,13,14,15,16,17,16,17,18,19,20,21,20,21,22,23,24,25,24,25,26,27,28,29,28,29,30,31,32,1};int P[32]={16 ,7 , 20 , 21 ,29,12 ,28 , 17 ,1, 15 ,23 , 26 ,5, 18 ,31 , 10 ,2, 8 , 24 , 14 ,32,27, 3 , 9 ,19,13, 30 , 6 ,22,11 ,4 , 25 };void Char_to_Int(unsigned char in[],int out[],int len){for(int i=0;i<len;i++)out[i]=int(in[i]);}void Int_to_Char(int in[],unsigned char out[],int len){for(int i=0;i<len;i++)out[i]=char(in[i]);}void B_to_H(int in[],unsigned char out[]){for(int i=0;i<16;i++){int temp=in[i*4]*8+in[i*4+1]*4+in[i*4+2]*2+in[i*4+3];switch(temp){case 10 :out[i]='A';break;case 11 :out[i]='B';break;case 12 :out[i]='C';break;case 13 :out[i]='D';break;case 14 :out[i]='E';break;case 15 :out[i]='F';break;default :out[i]=unsigned char(temp+48);break;}}}void H_to_B(unsigned char in[],int out[]){for(int i=0;i<16;i++)switch(in[i]){case 'A':out[i*4]=1;out[i*4+1]=0;out[i*4+2]=1;out[i*4+3]=0;break;case 'B':out[i*4]=1;out[i*4+1]=0;out[i*4+2]=1;out[i*4+3]=1;break;case 'C':out[i*4]=1;out[i*4+1]=1;out[i*4+2]=0;out[i*4+3]=0;break;case 'D':out[i*4]=1;out[i*4+1]=1;out[i*4+2]=0;out[i*4+3]=1;break;case 'E':out[i*4]=1;out[i*4+1]=1;out[i*4+2]=1;out[i*4+3]=0;break;case 'F':out[i*4]=1;out[i*4+1]=1;out[i*4+2]=1;out[i*4+3]=1;break;case '0':out[i*4]=0;out[i*4+1]=0;out[i*4+2]=0;out[i*4+3]=0;break;case '1':out[i*4]=0;out[i*4+1]=0;out[i*4+2]=0;out[i*4+3]=1;break;case '2':out[i*4]=0;out[i*4+1]=0;out[i*4+2]=1;out[i*4+3]=0;break;case '3':out[i*4]=0;out[i*4+1]=0;out[i*4+2]=1;out[i*4+3]=1;break;case '4':out[i*4]=0;out[i*4+1]=1;out[i*4+2]=0;out[i*4+3]=0;break;case '5':out[i*4]=0;out[i*4+1]=1;out[i*4+2]=0;out[i*4+3]=1;break;case '6':out[i*4]=0;out[i*4+1]=1;out[i*4+2]=1;out[i*4+3]=0;break;case '7':out[i*4]=0;out[i*4+1]=1;out[i*4+2]=1;out[i*4+3]=1;break;case '8':out[i*4]=1;out[i*4+1]=0;out[i*4+2]=0;out[i*4+3]=0;break;case '9':out[i*4]=1;out[i*4+1]=0;out[i*4+2]=0;out[i*4+3]=1;break;}}void O_to_B(int in[],int out[],int len){int i,j;for(i=0;i<len;i++)for(j=i*len+7;j>=i*len;j--){out[j]=in[i]%2; in[i]=in[i]/2;}}void B_to_O(int in[],int out[],int len){int j;for(int i=0;i<len;i++){j=8*i;out[i]=in[j]*128+in[j+1]*64+in[j+2]*32+in[j+3]*16+in[j+4]*8+in[j+5]*4+in[j+6]*2+in[j+7]*1;}}void Convert(int in[],int out[],int table[],int len){for(int i=0;i<len;i++)out[i]=in[table[i]-1];}void Divide(int in[],int out1[],int out2[],int len){for(int i=0;i<len;i++)if(i<len/2)out1[i]=in[i];elseout2[i-len/2]=in[i];}//正确void Combine(int in1[],int in2[],int out[],int len){for(int i=0;i<len;i++)if(i<len/2)out[i]=in1[i];elseout[i]=in2[i-len/2];}//正确void RLC1(int in[],int out[],int len){int temp=in[0];for(int i=0;i<len-1;i++)out[i]=in[i+1];out[len-1]=temp;}//正确void RLC2(int in[],int out[],int len){int temp1=in[0],temp2=in[1];for(int i=0;i<len-2;i++)out[i]=in[i+2];out[len-2]=temp1;out[len-1]=temp2;}//正确void COPY(int in[],int out[],int len){for(int i=0;i<len;i++)out[i]=in[i];}//ZHENGQUEvoid XOR(int in1[],int in2[],int out[],int len){for(int i=0;i<len;i++)out[i]=(in1[i]+in2[i])%2;}void GetKey(unsigned char in[],int out[16][48]){int i;int Msg_Int[8],Msg_Bin[64],Msg_Bin_PC1[56],Key_Bin[56],C[17][28],D[17][28];char Msg_H[16];int PC1[56]={57,49,41,33,25,17,9,1,58,50,42,34,26,18,10,2,59,51,43,35,27,19,11,3,60,52,44,36,63,55,47,39,31,23,15,7,62,54,46,38,30,22,14,6,61,53,45,37,29,21,13,5,28,20,12,4},PC2[48]={14,17,11,24,1,5,3,28,15,6,21,10,23,19,12,4,26,8,16,7,27,20,13,2,41,52,31,37,47,55,30,40,51,45,33,48,44,49,39,56,34,53,46,42,50,36,29,32};Char_to_Int(in,Msg_Int,8);//正确O_to_B(Msg_Int,Msg_Bin,8);//正确cout<<endl;Convert(Msg_Bin,Msg_Bin_PC1,PC1,56); Divide(Msg_Bin_PC1,C[0],D[0],56);for(i=0;i<16;i++){if(i==0){RLC1(C[i],C[i+1],28);RLC1(D[i],D[i+1],28);}else if(i==1){RLC1(C[i],C[i+1],28);RLC1(D[i],D[i+1],28);}else if(i==8){RLC1(C[i],C[i+1],28);RLC1(D[i],D[i+1],28);}else if(i==15){RLC1(C[i],C[i+1],28);RLC1(D[i],D[i+1],28);}else{RLC2(C[i],C[i+1],28);RLC2(D[i],D[i+1],28);}Combine(C[i+1],D[i+1],Key_Bin,56);Convert(Key_Bin,out[i],PC2,48);}} //生成子密钥正确void S_box(int in[],int out[]){int i;int SBox[8][64] ={{14,4,13,1,2,15,11,8,3,10,6,12,5,9,0,7,0,15,7,4,14,2,13,1,10,6,12,11,9,5,3,8,4,1,14,8,13,6,2,11,15,12,9,7,3,10,5,0,15,12,8,2,4,9,1,7,5,11,3,14,10,0,6,13},{15,1,8,14,6,11,3,4,9,7,2,13,12,0,5,10,3,13,4,7,15,2,8,14,12,0,1,10,6,9,11,5,0,14,7,11,10,4,13,1,5,8,12,6,9,3,2,15,13,8,10,1,3,15,4,2,11,6,7,12,0,5,14,9},{10,0,9,14,6,3,15,5,1,13,12,7,11,4,2,8,13,7,0,9,3,4,6,10,2,8,5,14,12,11,15,1,13,6,4,9,8,15,3,0,11,1,2,12,5,10,14,7,1,10,13,0,6,9,8,7,4,15,14,3,11,5,2,12},{7,13,14,3,0,6,9,10,1,2,8,5,11,12,4,15,13,8,11,5,6,15,0,3,4,7,2,12,1,10,14,9,10,6,9,0,12,11,7,13,15,1,3,14,5,2,8,4,3,15,0,6,10,1,13,8,9,4,5,11,12,7,2,14},{2,12,4,1,7,10,11,6,8,5,3,15,13,0,14,9,14,11,2,12,4,7,13,1,5,0,15,10,3,9,8,6,4,2,1,11,10,13,7,8,15,9,12,5,6,3,0,14,11,8,12,7,1,14,2,13,6,15,0,9,10,4,5,3,},{12,1,10,15,9,2,6,8,0,13,3,4,14,7,5,11,10,15,4,2,7,12,9,5,6,1,13,14,0,11,3,8,9,14,15,5,2,8,12,3,7,0,4,10,1,13,11,6,4,3,2,12,9,5,15,10,11,14,1,7,6,0,8,13},{4,11,2,14,15,0,8,13,3,12,9,7,5,10,6,1,13,0,11,7,4,9,1,10,14,3,5,12,2,15,8,6,1,4,11,13,12,3,7,14,10,15,6,8,0,5,9,2,6,11,13,8,1,4,10,7,9,5,0,15,14,2,3,12},{13,2,8,4,6,15,11,1,10,9,3,14,5,0,12,7,1,15,13,8,10,3,7,4,12,5,6,11,0,14,9,2,7,11,4,1,9,12,14,2,0,6,10,13,15,3,5,8,2,1,14,7,4,10,8,13,15,12,9,0,3,5,6,11}};int s1,s3,s2[8];for(i=0;i<8;i++){s1=in[i*6]*2+in[i*6+5]*1;s3=in[i*6+1]*8+in[i*6+2]*4+in[i*6+3]*2+in[i*6+4]*1;s2[i]=SBox[i][s1*16+s3];}int j;for(i=0;i<8;i++)for(j=i*4+3;j>=i*4;j--){out[j]=s2[i]%2; s2[i]=s2[i]/2;}}void Encode(unsigned char in[],unsigned char Final_H[],int K[16][48]){int i;intORINT[8],ORBYTE[64],FinalBYTE[64],LR[64],R48[48],S_in[48],S_out[32],F_RL[32],FinalINT[8],L[17] [32],R[17][32];char ORH[16];Char_to_Int(in,ORINT,8);cout<<""<<endl;O_to_B(ORINT,ORBYTE,8);Convert(ORBYTE,LR,IP,64);Divide(LR,L[0],R[0],64);for(i=1;i<17;i++){Convert(R[i-1],R48,E,48);XOR(R48,K[i-1],S_in,48);S_box(S_in,S_out);Convert(S_out,F_RL,P,32);XOR(F_RL,L[i-1],R[i],32);COPY(R[i-1],L[i],32);}Combine(R[16],L[16],LR,64);Convert(LR,FinalBYTE,IP_1,64);cout<<"二进制的密文:"<<endl;for(i=0;i<64;i++){cout<<FinalBYTE[i];}cout<<endl<<endl;B_to_H(FinalBYTE,Final_H);}void DeCode(unsigned char in[],unsigned char Final_H[],int K[16][48]){int i;intORINT[8],ORBYTE[64],LR[64],R48[48],S_in[48],S_out[32],F_RL[32],FinalBYTE[64],FinalINT[8],L[17] [32],R[17][32];char ORH[16];Char_to_Int(in,ORINT,8);cout<<""<<endl;O_to_B(ORINT,ORBYTE,8);Convert(ORBYTE,LR,IP,64);Divide(LR,L[0],R[0],64);for(i=1;i<17;i++){COPY(R[i-1],L[i],32);Convert(R[i-1],R48,E,48);XOR(R48,K[16-i],S_in,48);S_box(S_in,S_out);Convert(S_out,F_RL,P,32);XOR(L[i-1],F_RL,R[i],32);}Combine(R[16],L[16],LR,64);Convert(LR,FinalBYTE,IP_1,64);B_to_H(FinalBYTE,Final_H);}void main(){int i;unsigned char Msg[8],UnCodeMsg[16],CodeMsg[16],Key[8],Msg_H[16],CodeChar[8];int SKey[16][48];int OrByte[64],OrInt[8],FinalByte[64],FinalInt[8];cout<<"请输入明文:"<<endl;for(i=0;i<8;i++)cin>>Msg[i];cout<<"请输入初始密钥:"<<endl;for(i=0;i<8;i++)cin>>Key[i];GetKey(Key,SKey);Encode(Msg,CodeMsg,SKey);cout<<"十六进制的密文:"<<endl;for(i=0;i<16;i++)cout<<CodeMsg[i];cout<<endl;cout<<"字符型密文:"<<endl;H_to_B(CodeMsg,FinalByte);B_to_O(FinalByte,FinalInt,8);Int_to_Char(FinalInt,CodeChar,8);for(i=0;i<8;i++)cout<<CodeChar[i];cout<<endl;cout<<"请输入十六进制的密文:"<<endl;for(i=0;i<16;i++)cin>>CodeMsg[i];B_to_O(FinalByte,FinalInt,8);Int_to_Char(FinalInt,CodeChar,8);cout<<"请输入解密密钥:"<<endl;for(i=0;i<8;i++)cin>>Key[i];GetKey(Key,SKey);DeCode(CodeChar,Msg_H,SKey);//for(i=0;i<16;i++)// cout<<Msg_H[i];cout<<endl;H_to_B(Msg_H,OrByte);B_to_O(OrByte,OrInt,8);Int_to_Char(OrInt,Msg,8);cout<<"明文是:"<<endl;for(i=0;i<8;i++)cout<<Msg[i];cout<<endl;}。
des密码算法程序c语言

des密码算法程序c语言一、概述DES(数据加密标准)是一种常用的对称加密算法,它采用64位的密钥,对数据进行加密和解密。
本程序使用C语言实现DES算法,包括密钥生成、数据加密和解密等操作。
二、算法实现1.密钥生成:使用初始置换算法IP(56位)将明文转化为56位的分组,再将该分组经过一系列的逻辑函数F进行6轮处理,最终生成一个56位的密文。
其中密钥包括56位数据位和8位奇偶校验位。
2.数据加密:将需要加密的数据转化为56位的分组,再经过DES 算法处理,得到密文。
3.数据解密:将密文经过DES算法处理,还原成原始明文。
三、程序代码```c#include<stdio.h>#include<string.h>#include<stdlib.h>#include<time.h>//DES算法参数定义#defineITERATIONS6//加密轮数#defineKEY_LENGTH8//密钥长度,单位为字节#defineBLOCK_SIZE8//数据分组长度,单位为字节#definePADDINGPKCS7Padding//填充方式#defineMAX_INPUT_LENGTH(BLOCK_SIZE*2)//数据输入的最大长度//初始置换函数voidinit_permutation(unsignedcharinput[BLOCK_SIZE]){inti;for(i=0;i<BLOCK_SIZE;i++){input[i]=i;}}//逻辑函数F的定义voidlogic_function(unsignedcharinput[BLOCK_SIZE],unsigned charoutput[BLOCK_SIZE]){inti;for(i=0;i<BLOCK_SIZE;i++){output[i]=input[(i+1)%BLOCK_SIZE]^input[i]^(i+1)/BLOCK_SI ZE;}}//DES算法主函数voiddes_encrypt(unsignedchar*input,unsignedchar*output){ unsignedcharkey[KEY_LENGTH];//密钥数组unsignedchariv[BLOCK_SIZE];//初始置换的输入数组unsignedcharciphertext[MAX_INPUT_LENGTH];//密文数组unsignedcharpadding[BLOCK_SIZE];//填充数组unsignedintlength=strlen((char*)input);//数据长度(以字节为单位)unsignedintpadding_length=(length+BLOCK_SIZE-1)%BLOCK_SIZE;//需要填充的字节数unsignedintround=0;//加密轮数计数器unsignedintj=0;//数据指针,用于循环读取数据和填充数据intkey_offset=((1<<(32-KEY_LENGTH))-1)<<(32-(ITERATIONS*BLOCK_SIZE));//密钥索引值,用于生成密钥数组和填充数组的初始值unsignedintk=0;//DES算法中每个轮次的密钥索引值,用于生成每个轮次的密钥数组和填充数组的值unsignedintkplus1=(k+1)%((1<<(32-BLOCK_SIZE))-1);//DES算法中每个轮次的密钥索引值加一后的值,用于下一个轮次的密钥生成charseed[32];//使用MD5作为初始种子值生成随机数序列chartmp[MAX_INPUT_LENGTH];//临时变量数组,用于数据交换和中间计算结果存储等操作time_tt;//时间戳变量,用于生成随机数序列的种子值srand((unsignedint)time(&t));//设置随机数种子值,确保每次运行生成的随机数序列不同init_permutation(iv);//初始置换操作,将输入数据转化为56位分组(需要重复填充时)或一个随机的分组(不需要重复填充时)memcpy(key,key_offset,sizeof(key));//将初始化的密钥数组复制到相应的位置上,以便于接下来的轮次生成不同的密钥值memcpy(padding,seed,sizeof(seed));//将种子值复制到填充数组中,以便于接下来的轮次生成不同的随机数序列值for(round=0;round<ITERATIONS;round++){//进行加密轮次操作,每轮包括。
DES算法代码及实验报告

DES算法代码及实验报告DES算法(Data Encryption Standard,数据加密标准)是一种对称密钥加密算法,是密码学中最为经典的算法之一、DES算法的核心是Feistel结构,通过将明文分成多个块,然后对每个块进行一系列的置换和替换操作,最后得到密文。
本文将给出DES算法的代码实现,并进行实验报告。
一、DES算法的代码实现:以下是使用Python语言实现的DES算法代码:```pythondef str_to_bitlist(text):bits = []for char in text:binval = binvalue(char, 8)bits.extend([int(x) for x in list(binval)])return bitsdef bitlist_to_str(bits):chars = []for b in range(len(bits) // 8):byte = bits[b * 8:(b + 1) * 8]chars.append(chr(int(''.join([str(bit) for bit in byte]), 2)))return ''.join(chars)def binvalue(val, bitsize):binary = bin(val)[2:] if isinstance(val, int) elsebin(ord(val))[2:]if len(binary) > bitsize:raise Exception("Binary value larger than the expected size.")while len(binary) < bitsize:binary = "0" + binaryreturn binarydef permute(sbox, text):return [text[pos - 1] for pos in sbox]def generate_round_keys(key):key = str_to_bitlist(key)key = permute(self.permuted_choice_1, key)left, right = key[:28], key[28:]round_keys = []for i in range(16):left, right = shift(left, self.shift_table[i]), shift(right, self.shift_table[i])round_key = left + rightround_key = permute(self.permuted_choice_2, round_key)round_keys.append(round_key)return round_keysdef shift(bits, shift_val):return bits[shift_val:] + bits[:shift_val]def xor(bits1, bits2):return [int(bit1) ^ int(bit2) for bit1, bit2 in zip(bits1, bits2)]def encrypt(text, key):text_bits = str_to_bitlist(text)round_keys = generate_round_keys(key)text_bits = permute(self.initial_permutation, text_bits)left, right = text_bits[:32], text_bits[32:]for i in range(16):expansion = permute(self.expansion_table, right)xor_val = xor(round_keys[i], expansion)substitution = substitute(xor_val)permut = permute(self.permutation_table, substitution)temp = rightright = xor(left, permut)left = tempreturn bitlist_to_str(permute(self.final_permutation, right + left))```二、DES算法的实验报告:1.实验目的通过实现DES算法,加深对DES算法原理的理解,验证算法的正确性和加密效果。
DES算法Java实现源代码
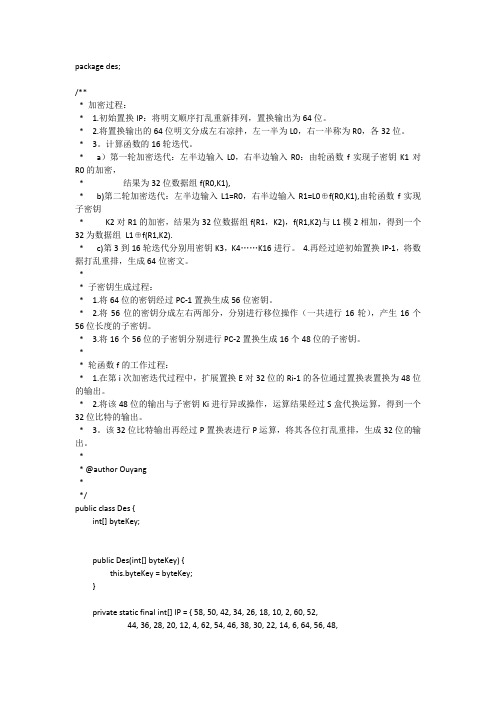
*b)第二轮加密迭代:左半边输入L1=R0,右半边输入R1=L0⊕f(R0,K1),由轮函数f实现子密钥
*K2对R1的加密,结果为32位数据组f(R1,K2),f(R1,K2)与L1模2相加,得到一个32为数据组L1⊕f(R1,K2).
*c)第3到16轮迭代分别用密钥K3,K4……K16进行。4.再经过逆初始置换IP-1,将数据打乱重排,生成64位密文。
{ 9, 14, 15, 5, 2, 8, 12, 3, 7, 0, 4, 10, 1, 13, 11, 6 },
{ 4, 3, 2, 12, 9, 5, 15, 10, 11, 14, 1, 7, 6, 0, 8, 13 } },
{ // S_Box[7]
{ 4, 11, 2, 14, 15, 0, 8, 13, 3, 12, 9, 7, 5, 10, 6, 1 },
package des;
/**
*加密过程:
* 1.初始置换IP:将明文顺序打乱重新排列,置换输出为64位。
* 2.将置换输出的64位明文分成左右凉拌,左一半为L0,右一半称为R0,各32位。
*3。计算函数的16轮迭代。
*a)第一轮加密迭代:左半边输入L0,右半边输入R0:由轮函数f实现子密钥K1对R0的加密,
{ 13, 0, 11, 7, 4, 9, 1, 10, 14, 3, 5, 12, 2, 15, 8, 6 },
{ 1, 4, 11, 13, 12, 3, 7, 14, 10, 15, 6, 8, 0, 5, 9, 2 },
{ 6, 11, 13, 8, 1, 4, 10, 7, 9, 5, 0, 15, 14, 2, 3, 12 } },
10, 11, 12, 13, 12, 13, 14, 15, 16, 17, 16, 17, 18, 19, 20, 21, 20,
des加密算法python代码

序号1:什么是DES加密算法?DES(Data Encryption Standard)是一种对称密钥加密算法,由IBM研制并于1977年被美国国家标准局(NBS)确定为联邦数据处理标准(FIPS)的一部分。
DES算法使用56位的密钥对64位的数据进行加密,经过多轮的置换、替换和移位运算后,得到密文。
DES算法已经被AES算法所代替,但在一些旧系统中仍然在使用。
序号2:DES加密算法的Python实现下面是一个简单的使用Python实现DES加密算法的例子:```pythonfrom Crypto.Cipher import DESfrom Crypto.Random import get_random_bytesdef des_encrypt(key, data):cipher = DES.new(key, DES.MODE_ECB)padded_data = data + b'\0' * (8 - len(data) % 8)encrypted_data = cipher.encrypt(padded_data)return encrypted_datadef des_decrypt(key, encrypted_data):cipher = DES.new(key, DES.MODE_ECB)decrypted_data = cipher.decrypt(encrypted_data).rstrip(b'\0') return decrypted_datakey = get_random_bytes(8)data = b'This is a test'encrypted_data = des_encrypt(key, data)decrypted_data = des_decrypt(key, encrypted_data)print('Original data:', data)print('Encrypted data:', encrypted_data)print('Decrypted data:', decrypted_data)```序号3:代码解读3.1 导入必要的模块```pythonfrom Crypto.Cipher import DESfrom Crypto.Random import get_random_bytes```在这行代码中,我们使用了`Crypto.Cipher`模块中的DES类和`Crypto.Random`模块的`get_random_bytes`函数。
DES算法代码及实验报告
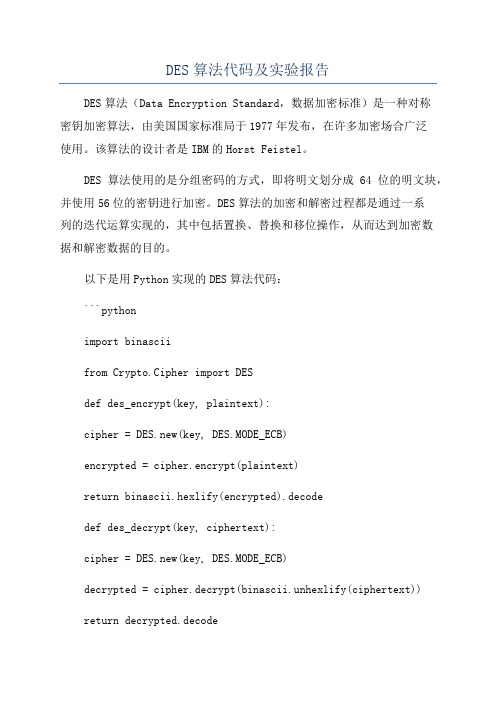
DES算法代码及实验报告DES算法(Data Encryption Standard,数据加密标准)是一种对称密钥加密算法,由美国国家标准局于1977年发布,在许多加密场合广泛使用。
该算法的设计者是IBM的Horst Feistel。
DES算法使用的是分组密码的方式,即将明文划分成64位的明文块,并使用56位的密钥进行加密。
DES算法的加密和解密过程都是通过一系列的迭代运算实现的,其中包括置换、替换和移位操作,从而达到加密数据和解密数据的目的。
以下是用Python实现的DES算法代码:```pythonimport binasciifrom Crypto.Cipher import DESdef des_encrypt(key, plaintext):cipher = DES.new(key, DES.MODE_ECB)encrypted = cipher.encrypt(plaintext)return binascii.hexlify(encrypted).decodedef des_decrypt(key, ciphertext):cipher = DES.new(key, DES.MODE_ECB)decrypted = cipher.decrypt(binascii.unhexlify(ciphertext))return decrypted.decode#示例代码plaintext = b'this is a test'ciphertext = des_encrypt(key, plaintext)print(f'Ciphertext: {ciphertext}')decryptedtext = des_decrypt(key, ciphertext)print(f'Decryptedtext: {decryptedtext}')```上述代码使用了`pycryptodome`库提供的DES加密算法实现。
DES算法源代码

DES算法源代码DES(Data Encryption Standard)是一种对称加密算法,使用相同的密钥对数据进行加密和解密。
它在1977年被美国联邦信息处理标准(FIPS)采纳为联邦标准,至今仍然在很多场景中广泛使用。
以下是一个使用Python实现DES算法的源代码:```pythonfrom Crypto.Cipher import DESfrom Crypto.Random import get_random_bytes# 生成一个由字节(byte)组成的密钥def generate_key(:return get_random_bytes(8)#使用给定的密钥加密数据def encrypt(key, data):cipher = DES.new(key, DES.MODE_ECB)#如果数据的长度不是8的倍数,需要进行填充if len(data) % 8 != 0:data += b'\x00' * (8 - len(data) % 8)ciphertext = cipher.encrypt(data)return ciphertext#使用给定的密钥解密数据def decrypt(key, ciphertext):cipher = DES.new(key, DES.MODE_ECB)data = cipher.decrypt(ciphertext)#去除填充数据data = data.rstrip(b'\x00')return data#测试代码key = generate_keyplaintext = b'This is a test message.'ciphertext = encrypt(key, plaintext)decrypted_text = decrypt(key, ciphertext)print('Key:', key)print('Plaintext:', plaintext)print('Ciphertext:', ciphertext)print('Decrypted Text:', decrypted_text)```这段代码使用Python的`Crypto`库中的`DES`模块来实现DES加密和解密。
C语言实现DES加密解密算法
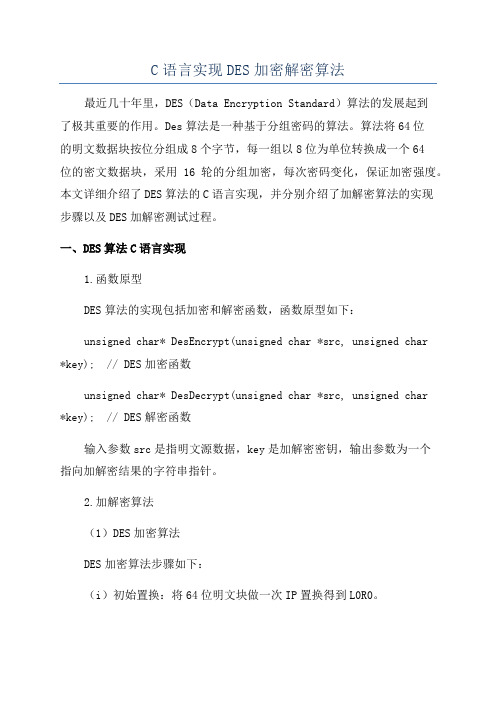
C语言实现DES加密解密算法
最近几十年里,DES(Data Encryption Standard)算法的发展起到
了极其重要的作用。
Des算法是一种基于分组密码的算法。
算法将64位
的明文数据块按位分组成8个字节,每一组以8位为单位转换成一个64
位的密文数据块,采用16轮的分组加密,每次密码变化,保证加密强度。
本文详细介绍了DES算法的C语言实现,并分别介绍了加解密算法的实现
步骤以及DES加解密测试过程。
一、DES算法C语言实现
1.函数原型
DES算法的实现包括加密和解密函数,函数原型如下:
unsigned char* DesEncrypt(unsigned char *src, unsigned char
*key); // DES加密函数
unsigned char* DesDecrypt(unsigned char *src, unsigned char
*key); // DES解密函数
输入参数src是指明文源数据,key是加解密密钥,输出参数为一个
指向加解密结果的字符串指针。
2.加解密算法
(1)DES加密算法
DES加密算法步骤如下:
(i)初始置换:将64位明文块做一次IP置换得到L0R0。
(ii)迭代轮换:对L0R0经过16次迭代轮换后,最终结果为
L16R16
(iii)逆置换:L16R16进行逆置换得到64位密文。
(2)DES解密算法
DES解密算法步骤和DES加密算法步骤是一样的,只是将置换步骤改为逆置换,将轮换步骤改为逆轮换即可。
三、DES加解密测试
1.程序测试
在C语言编写完DES加解密算法之后。
DES加密算法与解密(带流程图)

一、DES加密及解密算法程序源代码:#include <iostream>using namespace std;const static char IP_Table[] = { //IP_Table置换 58, 50, 42, 34, 26, 18, 10, 2,60, 52, 44, 36, 28, 20, 12, 4,62, 54, 46, 38, 30, 22, 14, 6,64, 56, 48, 40, 32, 24, 16, 8,57, 49, 41, 33, 25, 17, 9, 1,59, 51, 43, 35, 27, 19, 11, 3,61, 53, 45, 37, 29, 21, 13, 5,63, 55, 47, 39, 31, 23, 15, 7};const static char Final_Table[] = { //最终置换 40, 8, 48, 16, 56, 24, 64, 32,39, 7, 47, 15, 55, 23, 63, 31,38, 6, 46, 14, 54, 22, 62, 30,37, 5, 45, 13, 53, 21, 61, 29,36, 4, 44, 12, 52, 20, 60, 28,35, 3, 43, 11, 51, 19, 59, 27,34, 2, 42, 10, 50, 18, 58, 26,33, 1, 41, 9, 49, 17, 57, 25};const static char S_Box[8][64] = { //s_box/* S1 */{14, 4, 13, 1, 2, 15, 11, 8, 3, 10, 6, 12, 5, 9, 0, 7, 0, 15, 7, 4, 14, 2, 13, 1, 10, 6, 12, 11, 9, 5, 3, 8,4, 1, 14, 8, 13, 6, 2, 11, 15, 12, 9, 7, 3, 10, 5, 0, 15, 12, 8, 2, 4, 9, 1, 7, 5, 11, 3, 14, 10, 0, 6, 13},/* S2 */{15, 1, 8, 14, 6, 11, 3, 4, 9, 7, 2, 13, 12, 0, 5, 10, 3, 13, 4, 7, 15, 2, 8, 14, 12, 0, 1, 10, 6, 9, 11, 5,0, 14, 7, 11, 10, 4, 13, 1, 5, 8, 12, 6, 9, 3, 2, 15, 13, 8, 10, 1, 3, 15, 4, 2, 11, 6, 7, 12, 0, 5, 14, 9},/* S3 */{10, 0, 9, 14, 6, 3, 15, 5, 1, 13, 12, 7, 11, 4, 2, 8, 13, 7, 0, 9, 3, 4, 6, 10, 2, 8, 5, 14, 12, 11, 15, 1, 13, 6, 4, 9, 8, 15, 3, 0, 11, 1, 2, 12, 5, 10, 14, 7, 1, 10, 13, 0, 6, 9, 8, 7, 4, 15, 14, 3, 11, 5, 2, 12},/* S4 */{7, 13, 14, 3, 0, 6, 9, 10, 1, 2, 8, 5, 11, 12, 4, 15, 13, 8, 11, 5, 6, 15, 0, 3, 4, 7, 2, 12, 1, 10, 14, 9, 10, 6, 9, 0, 12, 11, 7, 13, 15, 1, 3, 14, 5, 2, 8, 4, 3, 15, 0, 6, 10, 1, 13, 8, 9, 4, 5, 11, 12, 7, 2, 14},/* S5 */{2, 12, 4, 1, 7, 10, 11, 6, 8, 5, 3, 15, 13, 0, 14, 9, 14, 11, 2, 12, 4, 7, 13, 1, 5, 0, 15, 10, 3, 9, 8, 6, 4, 2, 1, 11, 10, 13, 7, 8, 15, 9, 12, 5, 6, 3, 0, 14, 11, 8, 12, 7, 1, 14, 2, 13, 6, 15, 0, 9, 10, 4, 5, 3},/* S6 */{12, 1, 10, 15, 9, 2, 6, 8, 0, 13, 3, 4, 14, 7, 5, 11, 10, 15, 4, 2, 7, 12, 9, 5, 6, 1, 13, 14, 0, 11, 3, 8, 9, 14, 15, 5, 2, 8, 12, 3, 7, 0, 4, 10, 1, 13, 11, 6,4, 3, 2, 12, 9, 5, 15, 10, 11, 14, 1, 7, 6, 0, 8, 13},/* S7 */{4, 11, 2, 14, 15, 0, 8, 13, 3, 12, 9, 7, 5, 10, 6, 1, 13, 0, 11, 7, 4, 9, 1, 10, 14, 3, 5, 12, 2, 15, 8, 6, 1, 4, 11, 13, 12, 3, 7, 14, 10, 15, 6, 8, 0, 5, 9, 2,6, 11, 13, 8, 1, 4, 10, 7, 9, 5, 0, 15, 14, 2, 3, 12},/* S8 */{13, 2, 8, 4, 6, 15, 11, 1, 10, 9, 3, 14, 5, 0, 12, 7, 1, 15, 13, 8, 10, 3, 7, 4, 12, 5, 6, 11, 0, 14, 9, 2,7, 11, 4, 1, 9, 12, 14, 2, 0, 6, 10, 13, 15, 3, 5, 8,2, 1, 14, 7, 4, 10, 8, 13, 15, 12, 9, 0, 3, 5, 6, 11} };const static char Rar_Table[] = { //压缩置换 14, 17, 11, 24, 1, 5,3, 28, 15, 6, 21, 10,23, 19, 12, 4, 26, 8,16, 7, 27, 20, 13, 2,41, 52, 31, 37, 47, 55,30, 40, 51, 45, 33, 48,44, 49, 39, 56, 34, 53,46, 42, 50, 36, 29, 32};const static char Exp_Table[] = { //扩展置换 32, 1, 2, 3, 4, 5,4, 5, 6, 7, 8, 9,8, 9, 10, 11, 12, 13,12, 13, 14, 15, 16, 17,16, 17, 18, 19, 20, 21,20, 21, 22, 23, 24, 25,24, 25, 26, 27, 28, 29,28, 29, 30, 31, 32, 1};const static char P_Table[]={ //P置换16, 7, 20, 21,29, 12, 28, 17,1, 15, 23, 26,5, 18, 31, 10,2, 8, 24, 14,32, 27, 3, 9,19, 13, 30, 6,22, 11, 4, 25};const static char KeyRar_Table[]={57, 49, 41, 33, 25, 17, 9,1, 58, 50, 42, 34, 26, 18,10, 2, 59, 51, 43, 35, 27,19, 11, 3, 60, 52, 44, 36,63, 55, 47, 39, 31, 23, 15,7, 62, 54, 46, 38, 30, 22,14, 6, 61, 53, 45, 37, 29,21, 13, 5, 28, 20, 12, 4};//设置全局变量,16轮密钥bool key[16][48]={{0}};void ByteToBit(bool *Out,char *In,int bits) //字节到位转换函数{int i;for(i=0;i<bits;i++)Out[i]=(In[i/8]>>(i%8))&1;}void BitToByte(char *Out,bool *In,int bits) //位到字节转换函数{int i;for(i=0;i<bits/8;i++)Out[i]=0;for(i=0;i<bits;i++)Out[i/8]|=In[i]<<(i%8);}void Xor(bool *InA,const bool *InB,int length) //按位异或{for(int i=0;i<length;i++)InA[i]^=InB[i];}void keyfc(char *In) //密钥生成函数{int i,j=0,mov,k,m;bool* key0 = new bool[56];bool* keyin = new bool[64];bool temp;ByteToBit(keyin,In,64); //字节到位的转换for(i=0;i<56;i++) //密钥压缩为56位key0[i]=keyin[KeyRar_Table[i]-1];for(i=0;i<16;i++) //16轮密钥产生{if(i==0||i==1||i==8||i==15)mov=1;elsemov=2;for(k=0;k<mov;k++) //分左右两块循环左移{for(m=0;m<8;m++){temp=key0[m*7];for(j=m*7;j<m*7+7;j++)key0[j]=key0[j+1];key0[m*7+6]=temp;}temp=key0[0];for(m=0;m<27;m++)key0[m]=key0[m+1];key0[27]=temp;temp=key0[28];for(m=28;m<55;m++)key0[m]=key0[m+1];key0[55]=temp;}for(j=0;j<48;j++) //压缩置换并储存key[i][j]=key0[Rar_Table[j]-1];}delete[] key0;delete[] keyin;}void DES(char Out[8],char In[8],bool Type)//加密核心程序,Type=0时加密,反之解密{bool* MW = new bool[64];bool* tmp = new bool[32];bool* PMW = new bool[64];bool* kzmw = new bool[48];bool* keytem = new bool[48];bool* ss = new bool[32];int hang,lie,i;ByteToBit(PMW,In,64);for(int j=0;j<64;j++){MW[j]=PMW[IP_Table[j]-1]; //初始置换}bool *Li=&MW[0],*Ri=&MW[32];for(i=0;i<48;i++) //右明文扩展置换kzmw[i]=Ri[Exp_Table[i]-1];if(Type==0) //DES加密过程{for(int lun=0;lun<16;lun++){for(i=0;i<32;i++)ss[i]=Ri[i];for(i=0;i<48;i++) //右明文扩展置换kzmw[i]=Ri[Exp_Table[i]-1];for(i=0;i<48;i++)keytem[i]=key[lun][i];Xor(kzmw,keytem,48);/*S盒置换*/for(i=0;i<8;i++){hang=kzmw[i*6]*2+kzmw[i*6+5];lie =kzmw[i*6+1]*8+kzmw[i*6+2]*4+kzmw[i*6+3]*2+kzmw[i*6+4];tmp[i*4+3]=S_Box[i][(hang+1)*16+lie]%2;tmp[i*4+2]=(S_Box[i][(hang+1)*16+lie]/2)%2;tmp[i*4+1]=(S_Box[i][(hang+1)*16+lie]/4)%2;tmp[i*4]=(S_Box[i][(hang+1)*16+lie]/8)%2;}for(i=0;i<32;i++) //P置换Ri[i]=tmp[P_Table[i]-1];Xor(Ri,Li,32); //异或for(i=0;i<32;i++) //交换左右明文{Li[i]=ss[i];}}for(i=0;i<32;i++){tmp[i]=Li[i];Li[i]=Ri[i];Ri[i]=tmp[i];}for(i=0;i<64;i++)PMW[i]=MW[Final_Table[i]-1];BitToByte(Out,PMW,64); //位到字节的转换}else //DES解密过程{for(int lun=15;lun>=0;lun--){for(i=0;i<32;i++)ss[i]=Ri[i];for(i=0;i<48;i++) //右明文扩展置换kzmw[i]=Ri[Exp_Table[i]-1];for(i=0;i<48;i++)keytem[i]=key[lun][i];Xor(kzmw,keytem,48);/*S盒置换*/for(i=0;i<8;i++){hang=kzmw[i*6]*2+kzmw[i*6+5];lie =kzmw[i*6+1]*8+kzmw[i*6+2]*4+kzmw[i*6+3]*2+kzmw[i*6+4];tmp[i*4+3]=S_Box[i][(hang+1)*16+lie]%2;tmp[i*4+2]=(S_Box[i][(hang+1)*16+lie]/2)%2;tmp[i*4+1]=(S_Box[i][(hang+1)*16+lie]/4)%2;tmp[i*4]=(S_Box[i][(hang+1)*16+lie]/8)%2;}for(i=0;i<32;i++) //P置换Ri[i]=tmp[P_Table[i]-1];Xor(Ri,Li,32); //异或for(i=0;i<32;i++) //交换左右明文{Li[i]=ss[i];}}for(i=0;i<32;i++){tmp[i]=Li[i];Li[i]=Ri[i];Ri[i]=tmp[i];}for(i=0;i<64;i++)PMW[i]=MW[Final_Table[i]-1];BitToByte(Out,PMW,64); //位到字节的转换}delete[] MW;delete[] tmp;delete[] PMW;delete[] kzmw;delete[] keytem;delete[] ss;}bool RunDes(char *Out, char *In, int datalength, char *Key, bool Type) //加密运行函数,判断输入以及对输入文本8字节分割{if( !( Out && In && Key && (datalength=(datalength+7)&0xfffffff8) ) ) return false;keyfc(Key);for(int i=0,j=datalength%8; i<j; ++i,Out+=8,In+=8)DES(Out, In, Type);return true;}int main(){char* Ki = new char[8];char Enter[]="This is the test of DES!";char* Print = new char[200];int len = sizeof(Enter);int i_mf;cout << "请输入密钥(8位):" <<"\n";for(i_mf=0;i_mf<8;i_mf++)cin >> Ki[i_mf];cout << "\n";RunDes(Print,Enter,len,Ki,0);//加密cout << "----加密前----" << "\n";for(i_mf=0;i_mf<len;i_mf++)cout << Enter[i_mf];cout << "\n\n";cout << "----加密后----" << "\n";for(i_mf=0;i_mf<len;i_mf++)cout<<Print[i_mf];cout << "\n\n";//此处进行不同密钥输入测试cout << "请输入密钥(8位):" <<"\n";for(i_mf=0;i_mf<8;i_mf++)cin >> Ki[i_mf];cout << "\n";RunDes(Enter,Print,len,Ki,1);//解密cout << "----解密后----" << "\n";for(i_mf=0;i_mf<len;i_mf++)cout << Enter[i_mf];cout << endl;delete[] Ki;delete[] Print;return 0;}二、程序编译、运行结果图:三、程序总体框架图:四、程序实现流程图:。
DES加密C语言实现源代码

void *memcpy( void *dest, const void *src, unsigned char count ){// ASSERT((dest != NULL)&&(src != NULL));unsigned char *temp_dest = (unsigned char *)dest;unsigned char *temp_src = (unsigned char *)src;while(count--) // 不对是否存在重叠区域进行判断{*temp_dest++ = *temp_src++;}return dest;}unsigned char *memset(unsigned char *dst,unsigned char value,unsigned char count) {unsigned char *start = dst;while (count--)*dst++ = value;return(start);}// 初始置换表IPunsigned char IP_Table[64] = { 57,49,41,33,25,17,9,1,59,51,43,35,27,19,11,3,61,53,45,37,29,21,13,5,63,55,47,39,31,23,15,7,56,48,40,32,24,16,8,0,58,50,42,34,26,18,10,2,60,52,44,36,28,20,12,4,62,54,46,38,30,22,14,6};//逆初始置换表IP^-1unsigned char IP_1_Table[64] = {39,7,47,15,55,23,63,31,38,6,46,14,54,22,62,30,37,5,45,13,53,21,61,29,36,4,44,12,52,20,60,28,35,3,43,11,51,19,59,27,34,2,42,10,50,18,58,26,33,1,41,9,49,17,57,25,32,0,40,8,48,16,56,24}; //扩充置换表Eunsigned char E_Table[48] = {31, 0, 1, 2, 3, 4,3, 4, 5, 6, 7, 8,7, 8,9,10,11,12,11,12,13,14,15,16,15,16,17,18,19,20,19,20,21,22,23,24,23,24,25,26,27,28,27,28,29,30,31, 0};//置换函数Punsigned char P_Table[32] = {15,6,19,20,28,11,27,16,0,14,22,25,4,17,30,9,1,7,23,13,31,26,2,8,18,12,29,5,21,10,3,24};//S盒unsigned char S[8][4][16] =// S1 {{{14,4,13,1,2,15,11,8,3,10,6,12,5,9,0,7}, {0,15,7,4,14,2,13,1,10,6,12,11,9,5,3,8}, {4,1,14,8,13,6,2,11,15,12,9,7,3,10,5,0}, {15,12,8,2,4,9,1,7,5,11,3,14,10,0,6,13}}, // S2{{15,1,8,14,6,11,3,4,9,7,2,13,12,0,5,10}, {3,13,4,7,15,2,8,14,12,0,1,10,6,9,11,5}, {0,14,7,11,10,4,13,1,5,8,12,6,9,3,2,15}, {13,8,10,1,3,15,4,2,11,6,7,12,0,5,14,9}}, //S3{{10,0,9,14,6,3,15,5,1,13,12,7,11,4,2,8}, {13,7,0,9,3,4,6,10,2,8,5,14,12,11,15,1}, {13,6,4,9,8,15,3,0,11,1,2,12,5,10,14,7}, {1,10,13,0,6,9,8,7,4,15,14,3,11,5,2,12}}, // S4{{7,13,14,3,0,6,9,10,1,2,8,5,11,12,4,15}, {13,8,11,5,6,15,0,3,4,7,2,12,1,10,14,9}, {10,6,9,0,12,11,7,13,15,1,3,14,5,2,8,4}, {3,15,0,6,10,1,13,8,9,4,5,11,12,7,2,14}}, //S5{{2,12,4,1,7,10,11,6,8,5,3,15,13,0,14,9},{14,11,2,12,4,7,13,1,5,0,15,10,3,9,8,6},{4,2,1,11,10,13,7,8,15,9,12,5,6,3,0,14}, {11,8,12,7,1,14,2,13,6,15,0,9,10,4,5,3}},//S6{{12,1,10,15,9,2,6,8,0,13,3,4,14,7,5,11}, {10,15,4,2,7,12,9,5,6,1,13,14,0,11,3,8},{9,14,15,5,2,8,12,3,7,0,4,10,1,13,11,6},{4,3,2,12,9,5,15,10,11,14,1,7,6,0,8,13}},// S7{{4,11,2,14,15,0,8,13,3,12,9,7,5,10,6,1}, {13,0,11,7,4,9,1,10,14,3,5,12,2,15,8,6},{1,4,11,13,12,3,7,14,10,15,6,8,0,5,9,2},{6,11,13,8,1,4,10,7,9,5,0,15,14,2,3,12}},//S8{{13,2,8,4,6,15,11,1,10,9,3,14,5,0,12,7},{1,15,13,8,10,3,7,4,12,5,6,11,0,14,9,2},{7,11,4,1,9,12,14,2,0,6,10,13,15,3,5,8},{2,1,14,7,4,10,8,13,15,12,9,0,3,5,6,11}}};//置换选择1unsigned char PC_1[56] = {56,48,40,32,24,16,8,0,57,49,41,33,25,17,9,1,58,50,42,34,26,18,10,2,59,51,43,35,62,54,46,38,30,22,14,6,61,53,45,37,29,21,13,5,60,52,44,36,28,20,12,4,27,19,11,3};//置换选择2unsigned char PC_2[48] = {13,16,10,23,0,4,2,27,14,5,20,9,22,18,11,3,25,7,15,6,26,19,12,1,40,51,30,36,46,54,29,39,50,44,32,46,43,48,38,55,33,52,45,41,49,35,28,31};// 对左移次数的规定unsigned char MOVE_TIMES[16] = {1,1,2,2,2,2,2,2,1,2,2,2,2,2,2,1}; //字节转换成二进制unsigned char ByteToBit(unsigned char ch, unsigned char bit[8]) {unsigned char cnt;for(cnt = 0;cnt < 8; cnt++){*(bit+cnt) = (ch>>cnt)&1;}return 0;}// 二进制转换成字节unsigned char BitToByte(unsigned char bit[8],unsigned char *ch){unsigned char cnt;for(cnt = 0;cnt < 8; cnt++){*ch |= *(bit + cnt)<<cnt;}return 0;}// 将长度为8的字符串转为二进制位串unsigned char Char8ToBit64(unsigned char ch[8],unsigned char bit[64]){unsigned char cnt;for(cnt = 0; cnt < 8; cnt++){ByteToBit(*(ch+cnt),bit+(cnt<<3));}return 0;}// 将二进制位串转为长度为8的字符串unsigned char Bit64ToChar8(unsigned char bit[64],unsigned char ch[8]){unsigned char cnt;memset(ch,0,8);for(cnt = 0; cnt < 8; cnt++){BitToByte(bit+(cnt<<3),ch+cnt);}return 0;}// 密钥置换1unsigned char DES_PC1_Transform(unsigned char key[64], unsigned char tempbts[56]) {unsigned char cnt;for(cnt = 0; cnt < 56; cnt++){tempbts[cnt] = key[PC_1[cnt]];}return 0;}//密钥置换2unsigned char DES_PC2_Transform(unsigned char key[56], unsigned char tempbts[48]) {unsigned char cnt;for(cnt = 0; cnt < 48; cnt++){tempbts[cnt] = key[PC_2[cnt]];}return 0;}//循环左移unsigned char DES_ROL(unsigned char data[56], unsigned char time){unsigned char temp[56];//保存将要循环移动到右边的位memcpy(temp,data,time);memcpy(temp+time,data+28,time);//前28位移动memcpy(data,data+time,28-time);memcpy(data+28-time,temp,time);//后28位移动memcpy(data+28,data+28+time,28-time);memcpy(data+56-time,temp+time,time);return 0;}//IP置换unsigned char DES_IP_Transform(unsigned char data[64]){unsigned char cnt;unsigned char temp[64];for(cnt = 0; cnt < 64; cnt++){temp[cnt] = data[IP_Table[cnt]];}memcpy(data,temp,64);return 0;}// IP逆置换unsigned char DES_IP_1_Transform(unsigned char data[64]) {unsigned char cnt;unsigned char temp[64];for(cnt = 0; cnt < 64; cnt++){temp[cnt] = data[IP_1_Table[cnt]];}memcpy(data,temp,64);return 0;}//扩展置换unsigned char DES_E_Transform(unsigned char data[48]) {unsigned char cnt;unsigned char temp[48];for(cnt = 0; cnt < 48; cnt++){temp[cnt] = data[E_Table[cnt]];}memcpy(data,temp,48);return 0;}//P置换unsigned char DES_P_Transform(unsigned char data[32]) {unsigned char cnt;unsigned char temp[32];for(cnt = 0; cnt < 32; cnt++){temp[cnt] = data[P_Table[cnt]];}memcpy(data,temp,32);return 0;}// 异或unsigned char DES_XOR(unsigned char R[48], unsigned char L[48] ,unsigned char count) {unsigned char cnt;for(cnt = 0; cnt < count; cnt++){R[cnt] ^= L[cnt];}return 0;}// S盒置换unsigned char DES_SBOX(unsigned char data[48]){unsigned char cnt;unsigned char line,row,output;unsigned char cur1,cur2;for(cnt = 0; cnt < 8; cnt++){cur1 = cnt*6;cur2 = cnt<<2;// 计算在S盒中的行与列line = (data[cur1]<<1) + data[cur1+5];row = (data[cur1+1]<<3) + (data[cur1+2]<<2)+ (data[cur1+3]<<1) + data[cur1+4];output = S[cnt][line][row];// 化为2进制data[cur2] = (output&0X08)>>3;data[cur2+1] = (output&0X04)>>2;data[cur2+2] = (output&0X02)>>1;data[cur2+3] = output&0x01;}return 0;}//交换unsigned char DES_Swap(unsigned char left[32], unsigned char right[32]){unsigned char temp[32];memcpy(temp,left,32);memcpy(left,right,32);memcpy(right,temp,32);return 0;}//生成子密钥unsigned char DES_MakeSubKeys(unsigned char key[64],unsigned char subKeys[16][48]){unsigned char temp[56];unsigned char cnt;DES_PC1_Transform(key,temp);// PC1置换for(cnt = 0; cnt < 16; cnt++) //16轮跌代,产生16个子密钥{DES_ROL(temp,MOVE_TIMES[cnt]);// 循环左移DES_PC2_Transform(temp,subKeys[cnt]);//PC2置换,产生子密钥}return 0;}//加密单个分组unsigned char DES_EncryptBlock(unsigned char plainBlock[8], unsigned char subKeys[16][48], unsigned char cipherBlock[8]){unsigned char plainBits[64];unsigned char copyRight[48];unsigned char cnt;Char8ToBit64(plainBlock,plainBits);//初始置换(IP置换)DES_IP_Transform(plainBits);// 16轮迭代for(cnt = 0; cnt < 16; cnt++){memcpy(copyRight,plainBits+32,32);DES_E_Transform(copyRight); // 将右半部分进行扩展置换,从32位扩展到48位DES_XOR(copyRight,subKeys[cnt],48); // 将右半部分与子密钥进行异或操作DES_SBOX(copyRight); // 异或结果进入S盒,输出32位结果DES_P_Transform(copyRight); // P置换DES_XOR(plainBits,copyRight,32); //将明文左半部分与右半部分进行异或if(cnt != 15){DES_Swap(plainBits,plainBits+32);//最终完成左右部的交换}}DES_IP_1_Transform(plainBits); //逆初始置换(IP^1置换)Bit64ToChar8(plainBits,cipherBlock);return 0;}// 解密单个分组unsigned char DES_DecryptBlock(unsigned char cipherBlock[8], unsigned char subKeys[16][48],unsigned char plainBlock[8]){unsigned char cipherBits[64];unsigned char copyRight[48];short cnt;Char8ToBit64(cipherBlock,cipherBits);//初始置换(IP置换)DES_IP_Transform(cipherBits);// 16轮迭代for(cnt = 15; cnt >= 0; cnt--){memcpy(copyRight,cipherBits+32,32);//将右半部分进行扩展置换,从32位扩展到48位DES_E_Transform(copyRight);// 将右半部分与子密钥进行异或操作DES_XOR(copyRight,subKeys[cnt],48);//异或结果进入S盒,输出32位结果DES_SBOX(copyRight);// P置换DES_P_Transform(copyRight);//将明文左半部分与右半部分进行异或DES_XOR(cipherBits,copyRight,32);if(cnt != 0){// 最终完成左右部的交换DES_Swap(cipherBits,cipherBits+32);}}// 逆初始置换(IP^1置换)DES_IP_1_Transform(cipherBits);Bit64ToChar8(cipherBits,plainBlock);return 0;}//加密文件unsigned char DES_Encrypt(unsigned char *keyStr,unsigned char *plainFile,unsigned char *cipherFile){unsigned char keyBlock[8],bKey[64],subKeys[16][48];memcpy(keyBlock,keyStr,8); //设置密钥Char8ToBit64(keyBlock,bKey); //将密钥转换为二进制流DES_MakeSubKeys(bKey,subKeys); //生成子密钥DES_EncryptBlock(plainFile,subKeys,cipherFile);return 1;}//解密文件unsigned char DES_Decrypt(unsigned char *keyStr,unsigned char *cipherFile,unsigned char *plainFile){// unsigned char plainBlock[8],cipherBlock[8],unsigned char bKey[64],keyBlock[8],subKeys[16][48];memcpy(keyBlock,keyStr,8); //设置密钥Char8ToBit64(keyBlock,bKey); //将密钥转换为二进制流DES_MakeSubKeys(bKey,subKeys); //生成子密钥DES_DecryptBlock(cipherFile,subKeys,plainFile);return 1;}/****************************************************************************** ****************** 作者:win2kddk* 说明:3重DES是使用16字节密钥将8字节明文数据块进行3次DES加密和解密。
DES加密算法的C语言实现
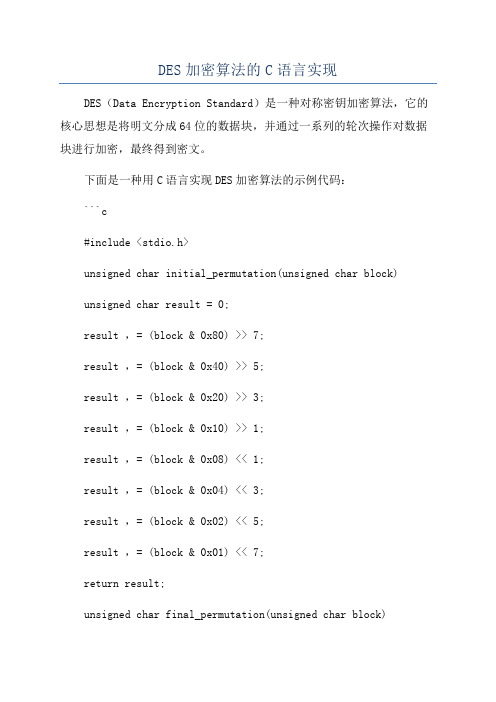
DES加密算法的C语言实现DES(Data Encryption Standard)是一种对称密钥加密算法,它的核心思想是将明文分成64位的数据块,并通过一系列的轮次操作对数据块进行加密,最终得到密文。
下面是一种用C语言实现DES加密算法的示例代码:```c#include <stdio.h>unsigned char initial_permutation(unsigned char block)unsigned char result = 0;result ,= (block & 0x80) >> 7;result ,= (block & 0x40) >> 5;result ,= (block & 0x20) >> 3;result ,= (block & 0x10) >> 1;result ,= (block & 0x08) << 1;result ,= (block & 0x04) << 3;result ,= (block & 0x02) << 5;result ,= (block & 0x01) << 7;return result;unsigned char final_permutation(unsigned char block)unsigned char result = 0;result ,= (block & 0x80) >> 7;result ,= (block & 0x40) >> 5;result ,= (block & 0x20) >> 3;result ,= (block & 0x10) >> 1;result ,= (block & 0x08) << 1;result ,= (block & 0x04) << 3;result ,= (block & 0x02) << 5;result ,= (block & 0x01) << 7;return result;void des_encrypt(unsigned char* plaintext, unsigned char* key, unsigned char* ciphertext)unsigned char block;unsigned char round_key;unsigned char i;// Initial Permutationblock = initial_permutation(*plaintext);// Round Permutationfor (i = 0; i < 16; i++)round_key = key[i];block ^= round_key;block = substitution(block);block = permutation(block);}// Final Permutation*ciphertext = final_permutation(block);int maiunsigned char plaintext = 0x55; // 明文unsigned char key[16] = {0x12, 0x34, 0x56, 0x78, 0x9A, 0xBC, 0xDE, 0xEF, 0xFE, 0xDC, 0xBA, 0x98, 0x76, 0x54, 0x32, 0x10}; // 密钥unsigned char ciphertext;des_encrypt(&plaintext, key, &ciphertext);printf("明文: 0x%02X\n", plaintext);printf("密钥: ");for (unsigned char i = 0; i < 16; i++)printf("%02X ", key[i]);}printf("\n");printf("密文: 0x%02X\n", ciphertext);return 0;```上述代码实现了DES算法的加密功能。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
57, 49, 41, 33, 25, 17, 9,
1, 58, 50, 42, 34, 26, 18,
10, 2, 59, 51, 43, 35, 27,
19, 11, 3, 60, 52, 44, 36,
63, 55, 47, 39, 31, 23, 15,
3, 13, 4, 7, 15, 2, 8, 14, 12, 0, 1, 10, 6, 9, 11, 5,
0, 14, 7, 11, 10, 4, 13, 1, 5, 8, 12, 6, 9, 3, 2, 15,
13, 8, 10, 1, 3, 15, 4, 2, 11, 6, 7, 12, 0, 5, 14, 9
4, 1, 14, 8, 13, 6, 2, 11, 15, 12, 9, 7, 3, 10, 5, 0,
15, 12, 8, 2, 4, 9, 1, 7, 5, 11, 3, 14, 10, 0, 6, 13
};
int S2[4][16]={
15, 1, 8, 14, 6, 11, 3, 4, 9, 7, 2, 13, 12, 0, 5, 10,
4, 5, 6, 7, 8, 9,
8, 9, 10, 11, 12, 13,
12, 13, 14, 15, 16, 17,
16, 17, 18, 19, 20, 21,
20, 21, 22, 23, 24, 25,
24, 25, 26, 27, 28, 29,
28, 29, 30, 31, 32, 1 };
46, 42, 50, 36, 29, 32 };
int S1[4][16]={ //S换位表
14, 4, 13, 1, 2, 15, 11, 8, 3, 10, 6, 12, 5, 9, 0, 7,
0, 15, 7, 4, 14, 2, 13, 1, 10, 6, 12, 11, 9, 5, 3, 8,
57, 49, 41, 33, 25, 17, 9, 1,
59, 51, 43, 35, 27, 19, 11, 3,
61, 53, 45, 37, 29, 21, 13, 5,
63, 55, 47, 39, 31, 23, 15, 7
};
int E[48]={ //扩展换位表
32, 1, 2, 3, 4, 5,
int RKS[8]; //存放经过查找8个S表后得到的8个十进制结果
int SP[32]; //将RKS表中的十进制数化成二进制
int RKSP[32]; //存放SP表经过P盒换位后的结果
int text_end[64]; //存放经过左右32位换位后的结果
int text_out[14][64]; //存放初始化向量和所有经过DES的分组的二进制
7, 62, 54, 46, 38, 30, 22,
14, 6, 61, 53, 45, 37, 29,
21, 13, 5, 28, 20, 12, 4 };
int move[16]={ //循环移位表
1,1,2,2,2,2,2,2,1,2,2,2,2,2,2,1};
int PC2[48]={ //PC2换位表(56—>48)
1, 10, 13, 0, 6, 9, 8, 7, 4, 15, 14, 3, 11, 5, 2, 12
};
int S3[4][16]={
10, 0, 9, 14, 6, 3, 15, 5, 1, 13, 12, 7, 11, 4, 2, 8,
13, 7, 0, 9, 3, 4, 6, 10, 2, 8, 5, 14, 12, 11, 15, 1,
13, 6, 4, 9, 8, 15, 3, 0, 11, 1, 2, 12, 5, 10, 14, 7,
char init[9]={"HTmadeit"}; //设置初始化向量为“HTmadeit”
int CBC[64];
int result[13][64];
int H[208];
char Miint M[13][8];
char choice;
int t;
int i,j;
14, 17, 11, 24, 1, 5,
3, 28, 15, 6, 21, 10,
23, 19, 12, 4, 26, 8,
16, 7, 27, 20, 13, 2,
41, 52, 31, 37, 47, 55,
30, 40, 51, 45, 33, 48,
44, 49, 39, 56, 34, 53,
int text_ip[64]; //存放第一次初始换位的结果
int L0[32],Li[32]; //将64位分成左右各32位进行迭代
int R0[32],Ri[32];
int RE0[48]; //存放右半部分经过E表扩展换位后的48位数据
int key[64]; //存放密钥的二进制形式
int keyPC1[56]; //存放密钥key经过PC1换位表后变成的56位二进制
int A[28]; //将keyPC1分成左右两部分,左部A,右部B,各28位,以便进行循环左移
int B[28];
int keyAB[56]; //将循环左移后两部分的结果合并起来
int K[16][48]; //存放16次循环左移产生的子密钥
int RK[48]; //存放RE和K异或运算后的结果
#include<stdio.h>
#include<string.h>
void main()
{ //声明变量
char MingWen[104]; //存放原始的明文
char target[8]; //将明文断成8个字符的一个分组
char InputKey[8]; //存放字符型的八位密钥
int text[64]; //存放一个分组转成二进制后的数据
int k,l,m,n;
int r[8],c[8];
int flag=1;
int IP[64]={ //初始换位表
58, 50, 42, 34, 26, 18, 10, 2,
60, 52, 44, 36, 28, 20, 12, 4,
62, 54, 46, 38, 30, 22, 14, 6,
64, 56, 48, 40, 32, 24, 16, 8,