Linux进程通信:命名管道FIFO小结
linux 实验五 命名管道实验
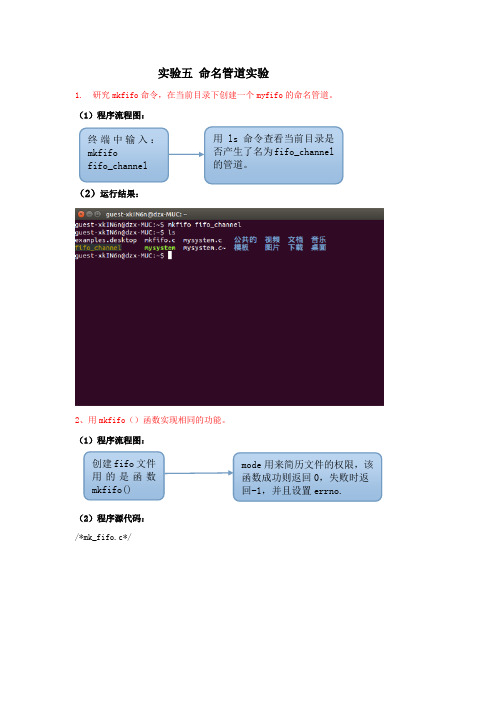
实验五 命名管道实验
1. 研究mkfifo 命令,在当前目录下创建一个myfifo 的命名管道。
(1)程序流程图:
(2)运行结果:
2、用mkfifo ()函数实现相同的功能。
(1)程序流程图:
(2)程序源代码:
/*mk_fifo.c*/ 终端中输入:
mkfifo
fifo_channel 用ls 命令查看当前目录是否产生了名为fifo_channel 的管道。
创建fifo 文件用的是函数mkfifo() mode 用来简历文件的权限,该函数成功则返回0,失败时返回-1,并且设置errno.
(3)运行结果:
3、编写一个服务器程序server.c ,实现从管道myfifo 中读取内容,并在终端中显示出来。
打开一个命令行终端,运行server ,然后打开另一个命令行终端,使用“cp 文件1 myfifo ”命令把文件1的内容输出到myfifo ,测试server.c 的功能。
(1)程序流程图:
(2)程序源代码: 创建管道,打开
管道,读出数据 利用命令cp ,把a 的内容复制到管道中
(3)运行结果:
4、编写客户机程序,实现把指定文件输出到myfifo的功能,从而实现和服务器程序的通信。
测试该程序的功能。
(1)程序流程图:基本同3,就不再画了。
(2)程序源代码:
/*server2.c*/
(3)实验结果:。
c语言有名管道fifo管道的用法

c语言有名管道fifo管道的用法【C语言有名管道(FIFO管道)的用法】中括号为主题的文章【引言】C语言是一种广泛应用于系统软件开发的编程语言,拥有丰富的库函数和系统调用接口。
在C语言中,有名管道(也称为FIFO管道)是一种特殊的文件类型,用于实现不同进程之间的通信。
本文将一步一步详细介绍C语言有名管道(FIFO 管道)的用法,以帮助读者更好地理解和使用该功能。
【目录】一、什么是有名管道二、有名管道的创建三、有名管道的打开和关闭四、有名管道的读写操作五、有名管道的进程间通信六、有名管道的应用示例七、有名管道的优缺点八、总结【一、什么是有名管道】有名管道是一种特殊的文件类型,在文件系统中以文件的形式存在。
它是C语言用于进程间通信的一种方式,可以实现不同进程之间的数据传输。
有名管道是一种半双工的通信机制,允许一个进程写入数据到管道,同时另一个进程从管道中读取数据。
有名管道适用于在不相关的进程之间进行通信,特别是父子进程之间。
【二、有名管道的创建】要创建一个有名管道,首先需要使用C语言库函数mkfifo来创建一个文件节点(相当于管道的入口)。
mkfifo函数的原型如下:int mkfifo(const char *pathname, mode_t mode);其中,pathname代表要创建的有名管道的文件路径,mode用于设置管道的权限。
例如,以下代码会创建一个名为myfifo的有名管道:#include <sys/types.h>#include <sys/stat.h>int main() {mkfifo("myfifo", 0666);return 0;}运行该程序后,系统中将会被创建一个名为myfifo的有名管道。
【三、有名管道的打开和关闭】打开一个有名管道以进行读取和写入操作,可以使用C语言库函数open。
open 函数的原型如下:int open(const char *pathname, int flags);其中,pathname代表要打开的有名管道的文件路径,flags用于指定打开管道的方式(只读、只写、读写)。
命名管道FIFO和mkfifo函数

命名管道FIFO和mkfifo函数进程间通信必须通过内核提供的通道,⽽且必须有⼀种办法在进程中标识内核提供的某个通道,前⾯讲过的是⽤打开的⽂件描述符来标识的。
如果要互相通信的⼏个进程没有从公共祖先那⾥继承⽂件描述符,它们怎么通信呢?内核提供⼀条通道不成问题,问题是如何标识这条通道才能使各进程都可以访问它?⽂件系统中的路径名是全局的,各进程都可以访问,因此可以⽤⽂件系统中的路径名来标识⼀个IPC通道。
FIFO和UNIX Domain Socket这两种IPC机制都是利⽤⽂件系统中的特殊⽂件来标识的。
FIFO⽂件在磁盘上没有数据块,仅⽤来标识内核中的⼀条通道,如 prw-rw-r-- 1 simba simba 0 May 21 10:13 p2,⽂件类型标识为p表⽰FIFO,⽂件⼤⼩为0。
各进程可以打开这个⽂件进⾏read/write,实际上是在读写内核通道(根本原因在于这个file结构体所指向的read、write函数和常规⽂件不⼀样),这样就实现了进程间通信。
UNIX Domain Socket和FIFO的原理类似,也需要⼀个特殊的socket⽂件来标识内核中的通道,例如/run⽬录下有很多系统服务的socket⽂件:srw-rw-rw- 1 root root 0 May 21 09:59 acpid.socket....................⽂件类型s表⽰socket,这些⽂件在磁盘上也没有数据块。
⼀、命名管道(FIFO)匿名管道应⽤的⼀个限制就是只能在具有共同祖先(具有亲缘关系)的进程间通信。
如果我们想在不相关的进程之间交换数据,可以使⽤FIFO⽂件来做这项⼯作,它经常被称为命名管道。
命名管道可以从命令⾏上创建,命令⾏⽅法是使⽤下⾯这个命令:$ mkfifo filename命名管道也可以从程序⾥创建,相关函数有:int mkfifo(const char *filename,mode_t mode);⼆、命名管道和匿名管道匿名管道由pipe函数创建并打开。
无名管道和有名管道区别

2011-06-08 21:45linux 管道 FIFO——写的很全,转来的pipe是Linux中最经典的进程间通信手段,在终端里通常用来组合命令,例如“ls -l|wc -l”。
它的作用很直观,就是使得前一个进程的输出作为后一个进程的输入,在概念上很符合“管道”的意思。
用管道实现“ls -l | wc -l”《情景分析》上有这个例子的代码,我觉得很适合用来了解管道。
这里假设终端对应的进程为PA,wc、ls是PA先后创建的两个子进程child_B与child_C。
代码简化后抄录如下:int main(){int pipefds[2], child_B, child_C;pipe(pipefds);if (!(child_B=fork()){ //先创建“读”的一端,它要关闭“写”的的一端close(pipefds[1]);close(0);dup2(pipefds[0], 0); //在执行系统调用execve后,child_B会释放0,1,2之外由父进程打开的文件,close(pipefds[0]); //所以要把pipefds[0]复制到标准输入对应的文件句柄0 execl("/usr/bin/wc", "-l", NULL);} //这里之后,A和B可以通过管道进行通信close(pipefds[0]);if (!(child_C=fork()){ //再创建“写”的一端,它要关闭“读”的的一端close(1);dup2(pipefds[1],1); //道理同前面close(pipefds[1]);execl("/bin/ls", "-1", NULL);} //这里之后,B和C可以通过管道进行通信close(pipefds[1]);wait4(child_B, NULL, 0, NULL);return 0;}FIFOFIFO就是命名管道,或有名管道。
linux进程管理实验心得
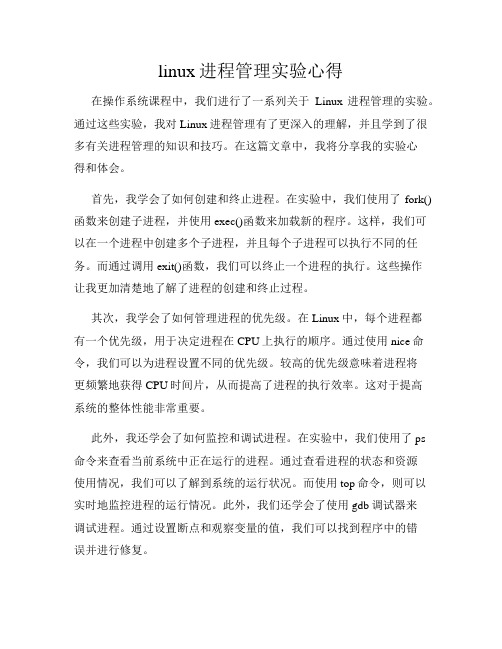
linux进程管理实验心得在操作系统课程中,我们进行了一系列关于Linux进程管理的实验。
通过这些实验,我对Linux进程管理有了更深入的理解,并且学到了很多有关进程管理的知识和技巧。
在这篇文章中,我将分享我的实验心得和体会。
首先,我学会了如何创建和终止进程。
在实验中,我们使用了fork()函数来创建子进程,并使用exec()函数来加载新的程序。
这样,我们可以在一个进程中创建多个子进程,并且每个子进程可以执行不同的任务。
而通过调用exit()函数,我们可以终止一个进程的执行。
这些操作让我更加清楚地了解了进程的创建和终止过程。
其次,我学会了如何管理进程的优先级。
在Linux中,每个进程都有一个优先级,用于决定进程在CPU上执行的顺序。
通过使用nice命令,我们可以为进程设置不同的优先级。
较高的优先级意味着进程将更频繁地获得CPU时间片,从而提高了进程的执行效率。
这对于提高系统的整体性能非常重要。
此外,我还学会了如何监控和调试进程。
在实验中,我们使用了ps命令来查看当前系统中正在运行的进程。
通过查看进程的状态和资源使用情况,我们可以了解到系统的运行状况。
而使用top命令,则可以实时地监控进程的运行情况。
此外,我们还学会了使用gdb调试器来调试进程。
通过设置断点和观察变量的值,我们可以找到程序中的错误并进行修复。
最后,我认识到进程管理是操作系统中非常重要的一部分。
一个好的进程管理系统可以提高系统的性能和稳定性。
通过合理地管理进程的创建、终止和调度,可以使系统更加高效地利用资源,并且能够更好地响应用户的需求。
因此,学习和掌握进程管理技术对于成为一名优秀的系统管理员或开发人员来说是非常重要的。
通过这些实验,我不仅学到了很多关于Linux进程管理的知识,还提高了自己的实践能力和问题解决能力。
在实验过程中,我遇到了各种各样的问题,但通过查阅资料、与同学讨论和不断尝试,我最终成功地解决了这些问题。
这让我更加自信地面对未来的挑战。
你不知道的Linux有名管道(FIFO)的阻塞和非阻塞读写
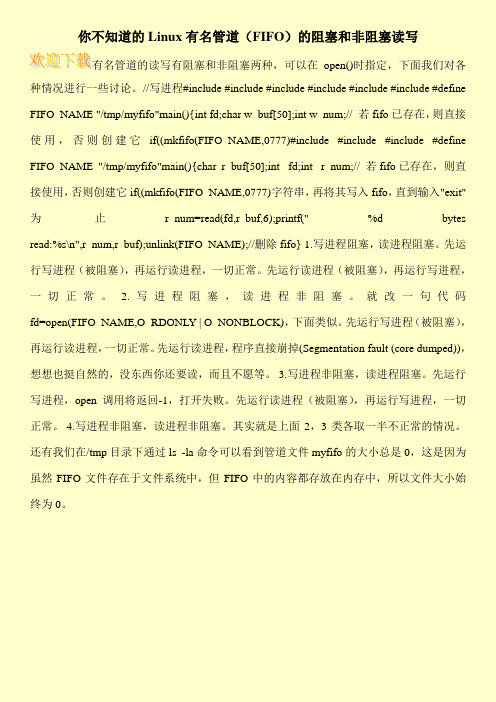
你不知道的Linux有名管道(FIFO)的阻塞和非阻塞读写有名管道的读写有阻塞和非阻塞两种,可以在open()时指定,下面我们对各种情况进行一些讨论。
//写进程#include #include #include #include #include #include #define FIFO_NAME "/tmp/myfifo"main(){int fd;char w_buf[50];int w_num;// 若fifo已存在,则直接使用,否则创建它if((mkfifo(FIFO_NAME,0777)#include #include #include #define FIFO_NAME "/tmp/myfifo"main(){char r_buf[50];int fd;int r_num;// 若fifo已存在,则直接使用,否则创建它if((mkfifo(FIFO_NAME,0777)字符串,再将其写入fifo,直到输入"exit"为止r_num=read(fd,r_buf,6);printf(" %d bytes read:%s\n",r_num,r_buf);unlink(FIFO_NAME);//删除fifo} 1.写进程阻塞,读进程阻塞。
先运行写进程(被阻塞),再运行读进程,一切正常。
先运行读进程(被阻塞),再运行写进程,一切正常。
2.写进程阻塞,读进程非阻塞。
就改一句代码fd=open(FIFO_NAME,O_RDONLY | O_NONBLOCK),下面类似。
先运行写进程(被阻塞),再运行读进程,一切正常。
先运行读进程,程序直接崩掉(Segmentation fault (core dumped)),想想也挺自然的,没东西你还要读,而且不愿等。
3.写进程非阻塞,读进程阻塞。
先运行写进程,open调用将返回-1,打开失败。
简述linux中进程间各种通信方式特点

简述linux中进程间各种通信方式特点Linux中进程间通信方式有多种,包括管道,命名管道,消息队列,信号量,共享内存和套接字。
每种通信方式都有自己的特点和适用场景。
一、管道1. 特点:- 管道是最简单的进程间通信方式之一,只能用于具有父子关系的进程间通信。
- 管道是一个单向通道,数据只能在一个方向上流动。
- 管道的容量有限,在写度满之前,读进程阻塞;在读度空之前,写进程阻塞。
2. 使用场景:- 父子进程之间需要进行简单的数据传输。
二、命名管道1. 特点:- 命名管道是一种特殊类型的文件,可以实现不相关进程的通信。
- 命名管道是半双工的,只能在一个方向上传输数据。
- 命名管道是顺序读写的,进程可以按照顺序读取其中的数据。
2. 使用场景:- 不相关的进程需要进行数据传输。
- 需要按照顺序进行传输的场景。
三、消息队列1. 特点:- 消息队列是一组消息的链表,具有特定的格式和标识符。
- 消息队列独立于发送和接收进程的生命周期,可以实现不相关进程间的通信。
- 消息队列可以根据优先级进行消息的传输。
2. 使用场景:- 需要实现进程间相对复杂的数据传输。
- 数据传输具有优先级。
四、信号量1. 特点:- 信号量是一个计数器,用于实现多个进程之间的互斥和同步。
- 信号量有一个整数值,只能通过定义的操作进行访问。
- 信号量可以用于控制临界区的访问次数。
2. 使用场景:- 多个进程需要共享公共资源。
- 需要进行互斥和同步操作。
五、共享内存1. 特点:- 共享内存是一块可以被多个进程共同访问的内存区域。
- 共享内存是最快的进程间通信方式,因为数据不需要在进程之间拷贝。
- 共享内存需要通过同步机制(如信号量)进行互斥访问。
2. 使用场景:- 需要高效地进行大量数据传输。
- 数据读写频繁,需要最小化数据拷贝的开销。
六、套接字1. 特点:- 套接字是一种网络编程中常用的进程间通信方式。
- 套接字支持不同主机上的进程进行通信。
Linux命令高级技巧通过mknod命令创建特殊文件和设备节点

Linux命令高级技巧通过mknod命令创建特殊文件和设备节点Linux命令高级技巧:通过mknod命令创建特殊文件和设备节点Linux作为一种开源操作系统,广泛用于服务器、嵌入式系统等领域,因其稳定性和灵活性备受青睐。
使用Linux操作系统,掌握一些高级命令技巧可以更好地发挥其功能。
本文将介绍如何使用mknod命令创建特殊文件和设备节点,以提升Linux系统的应用。
一、mknod命令概述mknod命令是Linux系统中用于创建特殊文件和设备节点的命令。
特殊文件和设备节点是Linux系统中用于访问硬件设备或其他特殊操作的接口,如磁盘、串口等。
通过mknod命令,用户可以手动创建这些接口,以满足特定需求。
二、创建块设备节点在Linux系统中,块设备是一种按固定大小的数据块进行读写的设备,常见的块设备有硬盘、USB闪存等。
通过mknod命令可以创建块设备节点,步骤如下:1. 以root身份登录Linux系统。
2. 打开终端,并执行以下命令:```sudo mknod /dev/mydevice b major-number minor-number```其中,`/dev/mydevice`为所需创建的块设备节点路径;`major-number`为主设备号;`minor-number`为次设备号。
3. 通过以下命令设置所创建的块设备节点的权限:```sudo chmod permissions /dev/mydevice```其中,`permissions`为所需设置的权限。
三、创建字符设备节点在Linux系统中,字符设备是一种以字符流的形式进行读写的设备,常见的字符设备有串口设备、打印机等。
通过mknod命令可以创建字符设备节点,步骤如下:1. 以root身份登录Linux系统。
2. 打开终端,并执行以下命令:```sudo mknod /dev/mydevice c major-number minor-number```其中,`/dev/mydevice`为所需创建的字符设备节点路径;`major-number`为主设备号;`minor-number`为次设备号。
【linux】下的mkfifo命令和【C语言】中的mkfifo函数
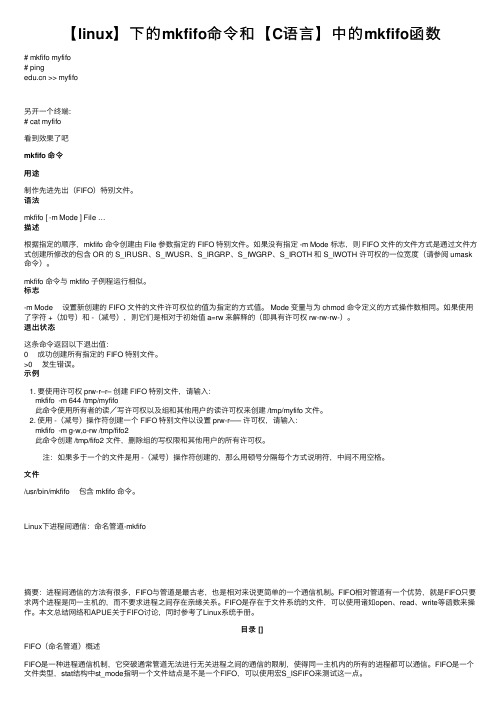
【linux】下的mkfifo命令和【C语⾔】中的mkfifo函数# mkfifo myfifo# ping >> myfifo另开⼀个终端:# cat myfifo看到效果了吧mkfifo 命令⽤途制作先进先出(FIFO)特别⽂件。
语法mkfifo [ -m Mode ] File …描述根据指定的顺序,mkfifo 命令创建由 File 参数指定的 FIFO 特别⽂件。
如果没有指定 -m Mode 标志,则 FIFO ⽂件的⽂件⽅式是通过⽂件⽅式创建所修改的包含 OR 的 S_IRUSR、S_IWUSR、S_IRGRP、S_IWGRP、S_IROTH 和 S_IWOTH 许可权的⼀位宽度(请参阅 umask 命令)。
mkfifo 命令与 mkfifo ⼦例程运⾏相似。
标志-m Mode 设置新创建的 FIFO ⽂件的⽂件许可权位的值为指定的⽅式值。
Mode 变量与为 chmod 命令定义的⽅式操作数相同。
如果使⽤了字符 +(加号)和 -(减号),则它们是相对于初始值 a=rw 来解释的(即具有许可权 rw-rw-rw-)。
退出状态这条命令返回以下退出值:0 成功创建所有指定的 FIFO 特别⽂件。
>0 发⽣错误。
⽰例1. 要使⽤许可权 prw-r–r– 创建 FIFO 特别⽂件,请输⼊:mkfifo -m 644 /tmp/myfifo此命令使⽤所有者的读/写许可权以及组和其他⽤户的读许可权来创建 /tmp/myfifo ⽂件。
2. 使⽤ -(减号)操作符创建⼀个 FIFO 特别⽂件以设置 prw-r—– 许可权,请输⼊:mkfifo -m g-w,o-rw /tmp/fifo2此命令创建 /tmp/fifo2 ⽂件,删除组的写权限和其他⽤户的所有许可权。
注:如果多于⼀个的⽂件是⽤ -(减号)操作符创建的,那么⽤顿号分隔每个⽅式说明符,中间不⽤空格。
⽂件/usr/bin/mkfifo 包含 mkfifo 命令。
linux管道fifo监听用法
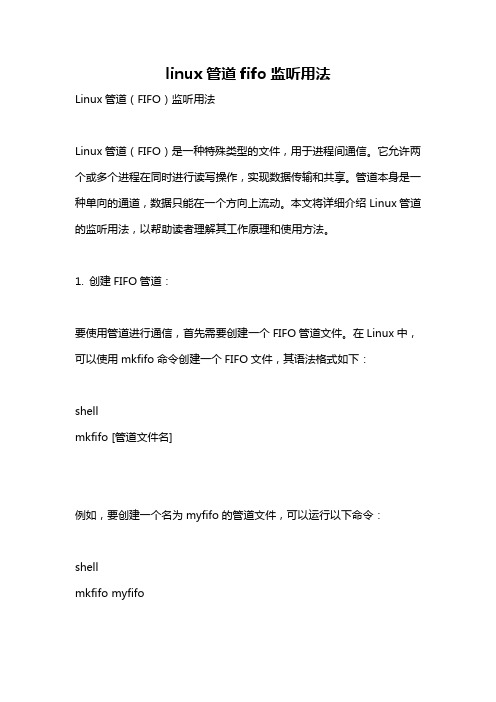
linux管道fifo监听用法Linux管道(FIFO)监听用法Linux管道(FIFO)是一种特殊类型的文件,用于进程间通信。
它允许两个或多个进程在同时进行读写操作,实现数据传输和共享。
管道本身是一种单向的通道,数据只能在一个方向上流动。
本文将详细介绍Linux管道的监听用法,以帮助读者理解其工作原理和使用方法。
1. 创建FIFO管道:要使用管道进行通信,首先需要创建一个FIFO管道文件。
在Linux中,可以使用mkfifo命令创建一个FIFO文件,其语法格式如下:shellmkfifo [管道文件名]例如,要创建一个名为myfifo的管道文件,可以运行以下命令:shellmkfifo myfifo2. 打开FIFO管道:创建了FIFO管道文件后,进程需要打开该管道以进行数据的读写操作。
在Linux中,可以使用open系统调用在C语言程序中打开一个管道文件。
以下是open函数的原型:c#include <fcntl.h>int open(const char *pathname, int flags);其中,`pathname`是要打开的管道文件名,`flags`是打开文件的标志。
常见的标志有O_RDONLY(只读模式打开管道)、O_WRONLY(只写模式打开管道)和O_RDWR(读写模式打开管道)。
例如,在C语言程序中,要以只读模式打开myfifo管道文件,可以使用以下代码:cint fd = open("myfifo", O_RDONLY);3. 监听FIFO管道:打开管道后,进程就可以监听管道,等待其他进程向其写入数据。
在Linux 中,可以使用read函数从管道中读取数据。
以下是read函数的原型:c#include <unistd.h>ssize_t read(int fd, void *buf, size_t count);其中,`fd`是打开的管道文件的文件描述符,`buf`是接收数据的缓冲区,`count`是要读取的字节数。
linux管道通信(C语言)
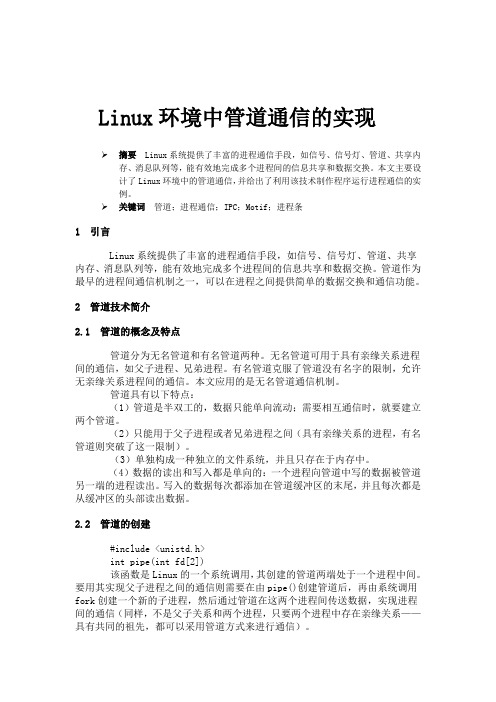
char buf_r[200];
memset(buf_r,0,sizeof(buf_r));
if(pipe(pipe_fd)<0;
return -1;
}
result=fork();
if(result<0){
printf("创建子进程失败");
Linux系统提供了丰富的进程通信手段,如信号、信号灯、管道、共享内存、消息队列等,能有效地完成多个进程间的信息共享和数据交换。管道作为最早的进程间通信机制之一,可以在进程之间提供简单的数据交换和通信功能。
2 管道技术简介
2.1 管道的概念及特点
管道分为无名管道和有名管道两种。无名管道可用于具有亲缘关系进程间的通信,如父子进程、兄弟进程。有名管道克服了管道没有名字的限制,允许无亲缘关系进程间的通信。本文应用的是无名管道通信机制。
exit(0);
}
else{
close(pipe_fd[0]);
if(write(pipe_fd[1],"**Hello world !**",17)!=-1)
printf("父进程向管道写入**Hello world !**\n");
if(write(pipe_fd[1]," **Welcome !**",15)!=-1)
命令gcc首先调用cpp进行预处理,在预处理过程中,对源代码文件中的文件包含(include)、预编译语句(如宏定义define等)进行分析。
接着调用cc1进行编译,这个阶段根据输入文件生成以.o为后缀的目标文件。汇编过程是针对汇编语言的步骤,调用as进行工作,一般来讲,.S为后缀的汇编语言源代码文件和汇编、.s为后缀的汇编语言文件经过预编译和汇编之后都生成以.o为后缀的目标文件。
linux中的文件类型
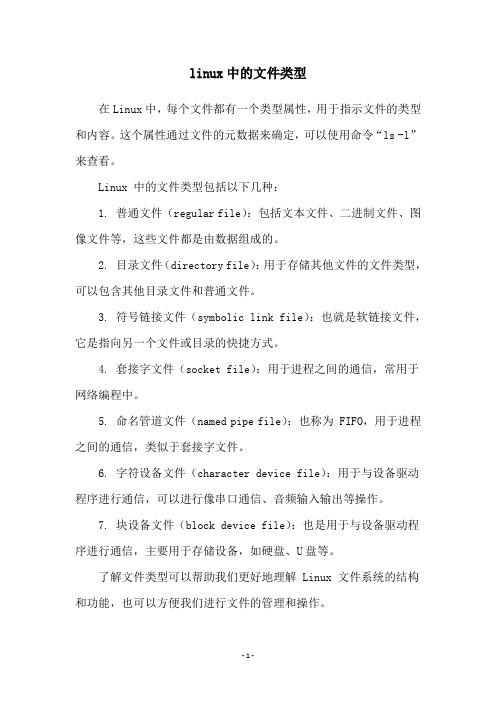
linux中的文件类型
在Linux中,每个文件都有一个类型属性,用于指示文件的类型和内容。
这个属性通过文件的元数据来确定,可以使用命令“ls -l”来查看。
Linux 中的文件类型包括以下几种:
1. 普通文件(regular file):包括文本文件、二进制文件、图像文件等,这些文件都是由数据组成的。
2. 目录文件(directory file):用于存储其他文件的文件类型,可以包含其他目录文件和普通文件。
3. 符号链接文件(symbolic link file):也就是软链接文件,它是指向另一个文件或目录的快捷方式。
4. 套接字文件(socket file):用于进程之间的通信,常用于网络编程中。
5. 命名管道文件(named pipe file):也称为 FIFO,用于进程之间的通信,类似于套接字文件。
6. 字符设备文件(character device file):用于与设备驱动程序进行通信,可以进行像串口通信、音频输入输出等操作。
7. 块设备文件(block device file):也是用于与设备驱动程序进行通信,主要用于存储设备,如硬盘、U盘等。
了解文件类型可以帮助我们更好地理解 Linux 文件系统的结构和功能,也可以方便我们进行文件的管理和操作。
- 1 -。
命名管道FIFO及其读写规则

命名管道FIFO及其读写规则 ⼀、匿名管道的⼀个限制就是只能在具有共同祖先的进程间通信命名管道(FIFO):如果我们想在不相关的进程之间切换数据,可以使⽤FIFO⽂件来做这项⼯作注意:命名管道是⼀种特殊类型⽂件。
利⽤命令:$ mkfifo filename或者相关函数:int mkfifo(const char*filename,mode_t mode); ⼆、区别与联系匿名管道由pipe函数创建或打开命名管道由mkfifo函数创建,打开⽤open.FIFO与PIPE的区别在它们创建与打开的⽅式不同,⼀旦这些⼯作完后,它们语义相同。
#include<unistd.h>#include<sys/types.h>#include<sys/stat.h>#include<fcntl.h>#include<stdlib.h>#include<stdio.h>#include<errno.h>#include<string.h>#include<signal.h>#define ERR_EXIT(m)\do\{\perror(m);\exit(EXIT_FAILURE);\}while(0) //宏要求⼀条语句int main(int argc,char*argv[]){umask(0);//掩码先要清零。
mode & (~umask)mkfifo("p2",0644);//当前路径下创建⼀个p2return0;} 有名管道读写规则:命名管道打开规则:如果当前打开操作是为读⽽打开FIFOO_NONBLOCK disable:阻塞直到有相应的进程为写⽽打开FIFOO_NONBLOCK enable:⾮阻塞模式⽴刻返回成功。
并不是-1。
不要等到有⼀个进程写⽽打开。
如果当前打开操作是为写⽽打开FIFOO_NONBLOCK disable:阻塞直到有相应的进程为读⽽打开FIFOO_NONBLOCK enable:⽴刻返回失败,错误码ENXIO 下⾯两个进程,⼀个是读打开,⼀个是为写⽽打开,阻塞⾮阻塞的区别见程序:#include<unistd.h>#include<sys/types.h>#include<sys/stat.h>#include<fcntl.h>#include<stdlib.h>#include<stdio.h>#include<errno.h>#include<string.h>#include<signal.h>#define ERR_EXIT(m)\do\{\perror(m);\exit(EXIT_FAILURE);\}while(0) //宏要求⼀条语句int main(int argc,char*argv[]){int fd;fd=open("p1",O_RDONLY);//打开p1,默认阻塞模式.⽤mkfifo p1已经创建好了p1管道⽂件。
Linux_mkfifo命名管道操作

Linux_mkfifo命名管道操作1. 管道的缺点管道只能在具有“亲戚”关系的进程之间通信。
即仅当管道由某个进程创建之后,在该进程的所有⼦孙进程之间,可通过该管道来通信。
其他情况下的⽆此“亲戚”关系的进程不能使⽤管道通信。
解决办法:使⽤命名管道2. 什么是命名管道?命名管道是⼀种特殊的⽂件,命名管道以普通⽂件的形式(在⽂件系统中有⼀个确定的路径和⽂件名)存在。
任意进程只要使⽤该⽂件就能通信。
注意:⽆名管道(即管道),是通过⽂件描述符的形式使⽤。
命名管道,是通过该命令管道⽂件的“⽂件名”来使⽤。
3. 命名管道的创建1) 使⽤mkfifo⽤法: man 3 mkfifo原型: int mkfifo(const char *pathname, mode_t mode);参数: mode,类似于open的第三个参数,表⽰该管道的访问权限。
pathname, 表⽰该命名管道的绝对路径。
返回值:成功,返回0失败,返回-1实例: main1.c查看已创建的管道(1) ls -l /tmp/myfifo第⼀个字符为p,表⽰该⽂件是⼀个管道(2) ls -F /tmp/myfifo最后⼀个字符为|, 表⽰该⽂件是⼀个管道2) 使⽤mknod⽤法: man 2 mknod原型:int mknod(const char *pathname, mode_t mode, dev_t dev);参数:mode, ⼀定要含有 | S_IFIFO注:该API能⽤来创建各种特殊⽂件。
dev, 取0实例:main2.c返回值:成功,返回0失败,返回-14. 命令管道的使⽤1) 通过shell命令使⽤假设已有命名管道“/tmp/fifo”,(1) 从命名管道读数据,如果没有数据,则将被阻塞# cat /tmp/myfifo(2) 在另⼀个终端上,向该命名管道发送数据# echo hello > /tmp/myfifo(3) 第(1)中的终端,将读取到数据。
linux进程间通信实验心得
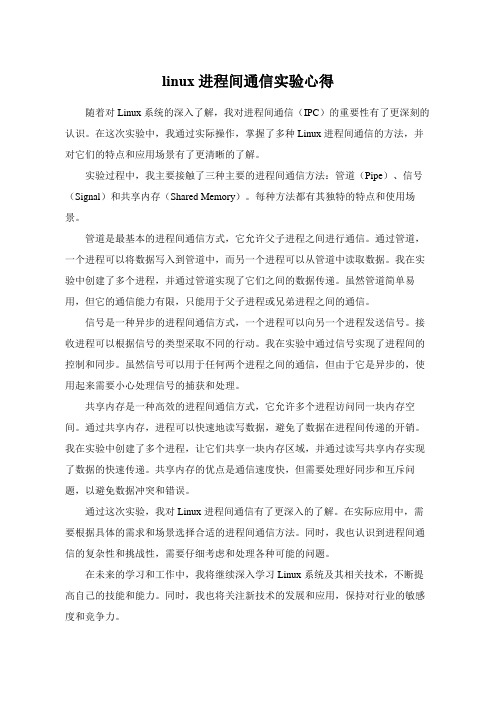
linux进程间通信实验心得随着对Linux系统的深入了解,我对进程间通信(IPC)的重要性有了更深刻的认识。
在这次实验中,我通过实际操作,掌握了多种Linux进程间通信的方法,并对它们的特点和应用场景有了更清晰的了解。
实验过程中,我主要接触了三种主要的进程间通信方法:管道(Pipe)、信号(Signal)和共享内存(Shared Memory)。
每种方法都有其独特的特点和使用场景。
管道是最基本的进程间通信方式,它允许父子进程之间进行通信。
通过管道,一个进程可以将数据写入到管道中,而另一个进程可以从管道中读取数据。
我在实验中创建了多个进程,并通过管道实现了它们之间的数据传递。
虽然管道简单易用,但它的通信能力有限,只能用于父子进程或兄弟进程之间的通信。
信号是一种异步的进程间通信方式,一个进程可以向另一个进程发送信号。
接收进程可以根据信号的类型采取不同的行动。
我在实验中通过信号实现了进程间的控制和同步。
虽然信号可以用于任何两个进程之间的通信,但由于它是异步的,使用起来需要小心处理信号的捕获和处理。
共享内存是一种高效的进程间通信方式,它允许多个进程访问同一块内存空间。
通过共享内存,进程可以快速地读写数据,避免了数据在进程间传递的开销。
我在实验中创建了多个进程,让它们共享一块内存区域,并通过读写共享内存实现了数据的快速传递。
共享内存的优点是通信速度快,但需要处理好同步和互斥问题,以避免数据冲突和错误。
通过这次实验,我对Linux进程间通信有了更深入的了解。
在实际应用中,需要根据具体的需求和场景选择合适的进程间通信方法。
同时,我也认识到进程间通信的复杂性和挑战性,需要仔细考虑和处理各种可能的问题。
在未来的学习和工作中,我将继续深入学习Linux系统及其相关技术,不断提高自己的技能和能力。
同时,我也将关注新技术的发展和应用,保持对行业的敏感度和竞争力。
linux管道用法

linux管道用法摘要:1.Linux 管道概述2.Linux 管道的基本用法3.Linux 管道的高级用法4.Linux 管道的实战案例正文:【Linux 管道概述】Linux 管道是Linux 系统中一种重要的进程间通信工具,它可以让一个进程的输出直接传递给另一个进程作为输入。
管道在Linux 系统中的应用非常广泛,例如:将一个命令的输出传递给另一个命令、实现日志的实时监控等。
管道的使用可以提高系统的运行效率,简化操作流程。
【Linux 管道的基本用法】1.创建管道:使用`pipe()`函数可以创建一个管道,`pipe()`函数的原型为`int pipe(int size);`,其中`size`表示管道的缓冲区大小,默认为0,表示使用系统默认的大小。
2.使用管道:在创建管道后,可以通过`dup2()`函数将管道与文件描述符进行关联,`dup2()`函数的原型为`int dup2(int oldfd, int newfd);`,其中`oldfd`表示要复制的文件描述符,`newfd`表示目标文件描述符。
将管道与文件描述符关联后,进程的输入/输出将通过管道进行。
3.关闭管道:在管道不再需要时,应使用`close()`函数关闭管道,以释放资源。
【Linux 管道的高级用法】1.命名管道:可以使用`mkfifo()`函数创建一个命名管道,`mkfifo()`函数的原型为`int mkfifo(const char *name, int size);`,其中`name`表示管道的名称,`size`表示管道的缓冲区大小,默认为0,表示使用系统默认的大小。
2.查询管道状态:使用`fifo()`函数可以查询命名管道的状态,`fifo()`函数的原型为`int fifo(const char *name);`,参数`name`表示管道的名称。
3.打开管道:使用`open()`函数可以打开命名管道,`open()`函数的原型为`int open(const char *name, int flags);`,其中`name`表示管道的名称,`flags`表示打开管道的标志,例如:O_RDONLY 表示只读模式,O_WRONLY 表示只写模式。
linux管道用法

linux管道用法摘要:1.Linux 管道简介2.Linux 管道的类型3.Linux 管道的用法4.管道的优缺点正文:【Linux 管道简介】Linux 管道是Linux 系统中一种重要的进程间通信(IPC) 机制。
管道是一种单向通信的IPC 方式,允许一个进程的输出连接到另一个进程的输入,从而实现进程间的数据传输。
在Linux 系统中,管道广泛应用于将一个进程的输出传递到另一个进程的输入,例如将命令的输出传递到文件,或将一个程序的输出传递到另一个程序的输入等。
【Linux 管道的类型】Linux 管道主要有两种类型:无名管道(匿名管道)和命名管道(有名管道)。
1.无名管道(匿名管道):无名管道是一种临时的、自动创建的管道。
它没有文件名,是系统根据需要自动创建的。
无名管道主要用于具有亲缘关系的父子进程之间的通信,如在fork() 函数创建子进程时,系统会自动为子进程创建一个管道,供子进程与父进程通信。
2.命名管道(有名管道):命名管道是一种永久的、有文件名的管道。
它类似于文件,可以在文件系统中创建、删除和重命名。
命名管道可以实现无亲缘关系的进程之间的通信,如两个相互独立的进程之间的通信。
【Linux 管道的用法】管道的用法主要包括以下几个方面:1.创建管道:使用mkfifo 命令可以创建一个管道。
例如:```mkfifo mypipe```2.打开管道:使用open() 函数打开管道。
管道打开后,进程可以读取或写入管道。
例如:```int fd = open("mypipe", O_RDONLY);```3.读取管道:使用read() 函数从管道中读取数据。
例如:```int data;read(fd, &data, sizeof(data));```4.写入管道:使用write() 函数向管道中写入数据。
例如:```int result;write(fd, &result, sizeof(result));```5.关闭管道:使用close() 函数关闭管道。
使用管道完成进程间通信(匿名管道pipe、命名管道fifo)

使⽤管道完成进程间通信(匿名管道pipe、命名管道fifo)每⼀个进程来说这个进程看到属于它的⼀块内存资源,这块资源是它所独占的,所以进程之间的通信就会⽐较⿇烦,原理就是需要让不同的进程间能够看到⼀份公共的资源。
所以交换数据必须通过内核,在内核中开辟⼀块缓冲区,进程1把数据从⽤户空间 拷到内核缓冲区,进程2再从内核缓冲区把数据读⾛,内核提供的这种机制称为进程间通信。
⼀般我们采⽤的进程间通信⽅式有:管道(pipe)和有名管道(FIFO)信号(signal)消息队列共享内存信号量套接字(socket)我们先来从最简单的通信⽅式来说起。
匿名管道也简称管道,管道的创建管道是⼀种最基本的进程间通信机制。
管道由pipe函数来创建:SYNOPSIS#include <unistd.h>int pipe(int pipefd[2]);调⽤pipe函数,会在内核中开辟出⼀块缓冲区⽤来进⾏进程间通信,这块缓冲区称为管道,它有⼀个读端和⼀个写端。
pipe函数接受⼀个参数,是包含两个整数的数组,如果调⽤成功,会通过pipefd[2]传出给⽤户程序两个⽂件描述符,需要注意pipefd [0]指向管道的读端, pipefd [1]指向管道的写端,那么此时这个管道对于⽤户程序就是⼀个⽂件,可以通过read(pipefd [0]);或者write(pipefd [1])进⾏操作。
pipe函数调⽤成功返回0,否则返回-1..那么再来看看通过管道进⾏通信的步骤:1.⽗进程创建管道,得到两个⽂件描述符指向管道的两端2. 利⽤fork函数创建⼦进程,则⼦进程也得到两个⽂件描述符指向同⼀管道3. ⽗进程关闭读端(pipe[0]),⼦进程关闭写端(pipe[1]),则此时⽗进程可以往管道中进⾏写操作,⼦进程可以从管道中读,从⽽实现了通过管道的进程间通信。
#include<stdio.h>#include<unistd.h>#include<string.h>int main(){int _pipe[2];int ret = pipe(_pipe);if(ret < 0){perror("pipe\n");}pid_t id = fork();if(id < 0){perror("fork\n");}else if(id == 0){close(_pipe[0]);int i = 0;char* msg = NULL;while(i < 100){msg = "I am child";write(_pipe[1], msg, strlen(msg));sleep(1);++i;}}else{close(_pipe[1]);int i = 0;char msg[100];while(i < 100){memset(msg, '\0', sizeof(msg));read(_pipe[0], msg, sizeof(msg));printf("%s\n", msg);++i;}}return0;}pipe的特点:1. 只能单向通信2. 只能⾎缘关系的进程进⾏通信(⽗⼦进程、兄弟进程)3. 依赖于⽂件系统4. ⽣命周期随进程(在内存中,进程结束被释放)5. ⾯向字节流的服务6. 管道内部提供了同步机制(锁、等待队列、信号)说明:因为管道通信是单向的,在上⾯的例⼦中我们是通过⼦进程写⽗进程来读,如果想要同时⽗进程写⽽⼦进程来读,就需要再打开另外的管道;管道的读写端通过打开的⽂件描述符来传递,因此要通信的两个进程必须从它们的公共祖先那⾥继承管道的件描述符。
linux创建管道命令
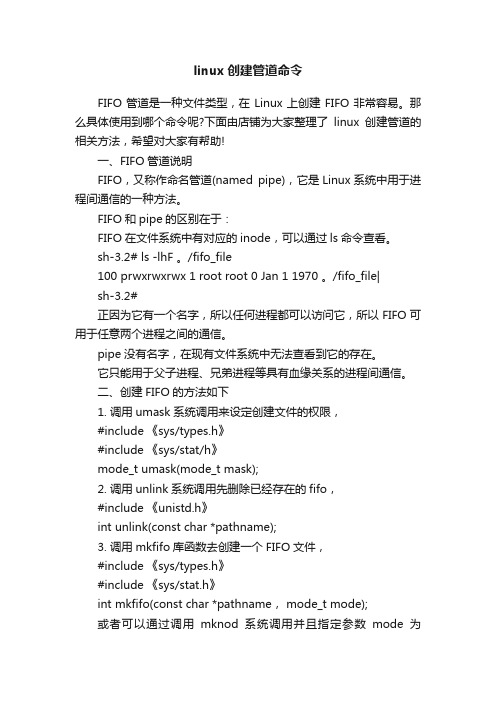
linux创建管道命令FIFO管道是一种文件类型,在Linux上创建FIFO非常容易。
那么具体使用到哪个命令呢?下面由店铺为大家整理了linux创建管道的相关方法,希望对大家有帮助!一、FIFO管道说明FIFO,又称作命名管道(named pipe),它是Linux系统中用于进程间通信的一种方法。
FIFO和pipe的区别在于:FIFO在文件系统中有对应的inode,可以通过ls命令查看。
sh-3.2# ls -lhF 。
/fifo_file100 prwxrwxrwx 1 root root 0 Jan 1 1970 。
/fifo_file|sh-3.2#正因为它有一个名字,所以任何进程都可以访问它,所以FIFO可用于任意两个进程之间的通信。
pipe没有名字,在现有文件系统中无法查看到它的存在。
它只能用于父子进程、兄弟进程等具有血缘关系的进程间通信。
二、创建FIFO的方法如下1. 调用umask系统调用来设定创建文件的权限,#include 《sys/types.h》#include 《sys/stat/h》mode_t umask(mode_t mask);2. 调用unlink系统调用先删除已经存在的fifo,#include 《unistd.h》int unlink(const char *pathname);3. 调用mkfifo库函数去创建一个FIFO文件,#include 《sys/types.h》#include 《sys/stat.h》int mkfifo(const char *pathname, mode_t mode);或者可以通过调用mknod系统调用并且指定参数mode为S_IFIFO也可以创建一个FIFO文件,#include 《sys/types.h》#include 《sys/stat.h》#include 《fcntl.h》#include 《unistd.h》int mknod(const char *pathname,mode_t mode,dev_t dev);三、注意事项1. 使用FIFO进行通信,每次传输的数据要限定在PIPE_BUF之内;2. 对于FIFO的访问就像访问正规文件(regular file)一样,可以使用open/read/write/close等系统调用进行访问。
linuxfifo命令使用
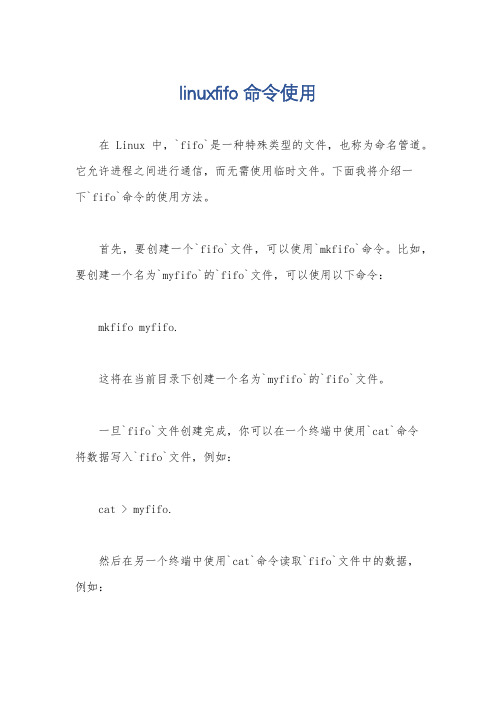
linuxfifo命令使用
在Linux中,`fifo`是一种特殊类型的文件,也称为命名管道。
它允许进程之间进行通信,而无需使用临时文件。
下面我将介绍一
下`fifo`命令的使用方法。
首先,要创建一个`fifo`文件,可以使用`mkfifo`命令。
比如,要创建一个名为`myfifo`的`fifo`文件,可以使用以下命令:
mkfifo myfifo.
这将在当前目录下创建一个名为`myfifo`的`fifo`文件。
一旦`fifo`文件创建完成,你可以在一个终端中使用`cat`命令
将数据写入`fifo`文件,例如:
cat > myfifo.
然后在另一个终端中使用`cat`命令读取`fifo`文件中的数据,
例如:
cat < myfifo.
这样,你就可以在两个进程之间实现数据的传输。
除了`cat`命令外,你还可以使用其他命令来操作`fifo`文件,比如`echo`、`printf`等。
需要注意的是,`fifo`文件在使用完毕后,需要使用`rm`命令手动删除,以免占用系统资源,例如:
rm myfifo.
这样就可以删除名为`myfifo`的`fifo`文件了。
总之,`fifo`命令可以帮助你在Linux系统中实现进程间的通信,提供了一种方便的方式来进行数据传输。
希望这些信息能够帮助到你。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
Linux 下进程之间通信可以用命名管道FIFO 完成。
命名管道是一种特殊类型的文件,因为Linux 中所有事物都是文件,它在文件系统中以文件名的形式存在。
在程序中,我们可以使用两个不同的函数调用来建立管道:#include <sys/types.h>#include <sys/stat.h> int mkfifo(const char *filename, mode_t mode);int mknode(const char *filename, mode_t mode | S_IFIFO, (dev_t) 0 );下面先来创建一个管道:view plaincopy to clipboardprint? #include <stdio.h>#include <stdlib.h>#include <sys/types.h>#include <sys/stat.h>int main(){int res = mkfifo("/tmp/my_fifo", 0777);if (res == 0){printf("FIFO created\n");}exit(EXIT_SUCCESS);}#include <stdio.h>#include <stdlib.h>#include <sys/types.h>#include <sys/stat.h>int main(){int res = mkfifo("/tmp/my_fifo", 0777); if (res == 0){printf("FIFO created\n");} exit(EXIT_SUCCESS);}编译这个程序:gcc -o fifol.c fifo运行这个程序:$ ./fifo1用ls 命令查看所创建的管道$ ls -lF /tmp/my_fifoprwxr-xr-x 1 root root 0 05-08 20:10 /tmp/my_fifo|注意:Is命令的输出结果中的第一个字符为p,表示这是一个管道。
最后的|符号是由Is命令的-F 选项添加的,它也表示是这是一个管道。
虽然,我们所设置的文件创建模式为“0777”但它被用户掩码(umask)设置(022)给改变了,这与普通文件创建是一样的,所以文件的最终模式为755。
打开FIFO 一个主要的限制是,程序不能是O_RDWR 模式打开FIFO 文件进行读写操作,这样做的后果未明确定义。
这个限制是有道理的,因为我们使用FIFO 只是为了单身传递数据,所以没有必要使用O_RDWR 模式。
如果一个管道以读/写方式打开FIFO ,进程就会从这个管道读回它自己的输出。
如果确实需要在程序之间双向传递数据,最好使用一对FIFO,一个方向使用一个。
当一个Linux进程被阻塞时,它并不消耗CPU资源,这种进程的同步方式对CPU而言是非常有效率的。
有关Linux 下命名管道FIFO 的读写规则可以参见之前所写的一篇文章:Linux 命名管道FIFO 的读写规则。
、实验:使用FIFO 实现进程间通信两个独立的程序:1. 生产者程序,它在需要时创建管道,然后尽可能快地向管道中写入数据。
2. 消费者程序,它从FIFO 中读取数据并丢弃它们。
生产者程序fifo2.c :view plaincopy to clipboardprint?#include <stdio.h>#include <stdlib.h>#include <string.h>#include <fcntl.h>#include <limits.h>#include <sys/types.h>#include <sys/stat.h>#define FIFO_NAME "/tmp/Linux/my_fifo"#define BUFFER_SIZE PIPE_BUF#define TEN_MEG (1024 * 1024 * 10)int main(){int pipe_fd;int res;int open_mode = O_WRONL Y;int bytes = 0;char buffer[BUFFER_SIZE + 1];if (access(FIFO_NAME, F_OK) == -1){res = mkfifo(FIFO_NAME, 0777);if (res != 0){fprintf(stderr, "Could not create fifo %s\n", FIFO_NAME); exit(EXIT_FAILURE);}}printf("Process %d opening FIFO O_WRONL Y\n", getpid()); pipe_fd = open(FIFO_NAME, open_mode);printf("Process %d result %d\n", getpid(), pipe_fd);if (pipe_fd != -1){while (bytes < TEN_MEG){res = write(pipe_fd, buffer, BUFFER_SIZE);if (res == -1){fprintf(stderr, "Write error on pipe\n"); exit(EXIT_FAILURE);}bytes += res;}close(pipe_fd);}else{exit(EXIT_FAILURE);}printf("Process %d finish\n", getpid()); exit(EXIT_SUCCESS);}#include <stdio.h>#include <stdlib.h>#include <string.h>#include <fcntl.h>#include <limits.h>#include <sys/types.h>#include <sys/stat.h>#define FIFO_NAME "/tmp/Linux/my_fifo"#define BUFFER_SIZE PIPE_BUF#define TEN_MEG (1024 * 1024 * 10)int main(){int pipe_fd;int res;int open_mode = O_WRONL Y;int bytes = 0;char buffer[BUFFER_SIZE + 1];if (access(FIFO_NAME, F_OK) == -1){res = mkfifo(FIFO_NAME, 0777);if (res != 0){fprintf(stderr, "Could not create fifo %s\n", FIFO_NAME); exit(EXIT_FAILURE);}}printf("Process %d opening FIFO O_WRONL Y\n", getpid()); pipe_fd = open(FIFO_NAME, open_mode);printf("Process %d result %d\n", getpid(), pipe_fd);if (pipe_fd != -1){while (bytes < TEN_MEG){res = write(pipe_fd, buffer, BUFFER_SIZE);if (res == -1){fprintf(stderr, "Write error on pipe\n"); exit(EXIT_FAILURE);}bytes += res;}close(pipe_fd);}else{exit(EXIT_FAILURE);}printf("Process %d finish\n", getpid());exit(EXIT_SUCCESS);}消费者程序fifo3.c :view plaincopy to clipboardprint?#include <stdio.h>#include <stdlib.h>#include <string.h>#include <fcntl.h>#include <limits.h>#include <sys/types.h>#include <sys/stat.h>#define FIFO_NAME "/tmp/Linux/my_fifo"#define BUFFER_SIZE PIPE_BUFint main(){int pipe_fd;int res;int open_mode = O_RDONL Y; char buffer[BUFFER_SIZE + 1]; int bytes = 0;memset(buffer, '\0', sizeof(buffer));printf("Process %d opeining FIFO O_RDONL Y\n", getpid()); pipe_fd = open(FIFO_NAME, open_mode);printf("Process %d result %d\n", getpid(), pipe_fd);if (pipe_fd != -1){do{res = read(pipe_fd, buffer, BUFFER_SIZE); bytes += res;}while(res > 0);close(pipe_fd);}else{exit(EXIT_FAILURE);}printf("Process %d finished, %d bytes read\n", getpid(), bytes); exit(EXIT_SUCCESS);}#include <stdio.h>#include <stdlib.h>#include <string.h>#include <fcntl.h>#include <limits.h>#include <sys/types.h>#include <sys/stat.h>#define FIFO_NAME "/tmp/Linux/my_fifo"#define BUFFER_SIZE PIPE_BUF int main(){int pipe_fd;int res;int open_mode = O_RDONL Y; charbuffer[BUFFER_SIZE + 1];int bytes = 0;memset(buffer, '\0', sizeof(buffer));printf("Process %d opeining FIFO O_RDONL Y\n", getpid()); pipe_fd = open(FIFO_NAME, open_mode);printf("Process %d result %d\n", getpid(), pipe_fd);if (pipe_fd != -1){do{res = read(pipe_fd, buffer, BUFFER_SIZE); bytes += res;}while(res > 0);close(pipe_fd);}else{exit(EXIT_FAILURE);}printf("Process %d finished, %d bytes read\n", getpid(), bytes); exit(EXIT_SUCCESS);}编译这两个程序:gcc -o fifo2 fifo2.cgcc -o fifo3 fifo3.c运行这两个程序:[root@localhost chaper12]# ./fifo2 & a后台执行,写数据[2] 23121Process 23121 opening FIFO O_WRONL Y[root@localhost chaper12]# time ./fifo3 a读数据Process 24155 opeining FIFO O_RDONL YProcess 23121 result 3Process 24155 result 3Process 23121 finishProcess 24155 finished, 10485760 bytes read[2]- Done ./fifo2real 0m0.214suser 0m0.000ssys 0m0.179s以上两个程序均是使用阻塞模式FIFO。