公司员工管理系统
公司员工绩效管理系统

自我评价
员工需对自己在工作中的 表现进行自我评价,提供 自评报告。
360度评价
鼓励同事、上级、下级等 多方参与评价,从多角度 了解员工绩效。
绩效数据收集与整理
数据来源
确保数据来源的可靠性和 准确性,如工作日志、项 目成果等。
数据整理
对收集到的数据进行分类 、整理,以便后续的绩效 分析和评估。
数据存储
06
未来展望
技术发展趋势
人工智能与大数据
01
利用AI和大数据技术,实现更精准的员工绩效评估,提供个性
化发展建议。
云计算
02
通过云计算技术,实现绩效数据的实时共享和存储,提高管理
效率。
移动应用
03
开发移动端应用,方便员工随时随地查看个人绩效数据,参与
绩效管理。
管理理念创新
以人为本
重视员工个人发展,强调员工参与和自我管理,激发员工潜能。
案例启示与借鉴
启示一
成功的绩效管理系统需要与企业文化 和战略目标相契合,才能真正发挥效 果。
启示二
在推行绩效管理系统的过程中,应注 重与员工的沟通和培训,以提高员工 的参与度和接受度。
启示三
绩效管理系统的实施需要分阶段进行 ,逐步完善,避免一步到位的做法。
启示四
在制定绩效指标时,应注重平衡短期 和长期目标,以及定量和定性指标。
评估方法
360度评价法、目标管理法、关 键绩效指标法、平衡计分卡等。
评估结果分析
员工绩效分析
对员工的绩效进行全面分析,了解员工在工作中的表现和优缺点 。
部门绩效分析
对部门整体绩效进行分析,了解部门在工作中的表现和优缺点。
绩效差异分析
分析员工绩效差异的原因,为制定优化方案提供依据。
人员管理系统

人员管理系统在当今的企业和组织中,人员管理系统扮演着至关重要的角色。
它就像是一个高效运转的中枢神经系统,协调着人员的各项活动,保障着组织的正常运作和持续发展。
一个完善的人员管理系统首先要具备全面而准确的人员信息采集和存储功能。
从员工的基本个人信息,如姓名、性别、年龄、联系方式,到工作经历、教育背景、技能特长等,都应被详细记录。
这些信息不仅是对员工个体的清晰画像,更是为后续的人员调配、培训发展等决策提供了坚实的数据支持。
在招聘环节,人员管理系统能够发挥重要作用。
它可以帮助企业发布招聘信息,管理招聘流程,筛选简历,安排面试。
通过系统的智能化匹配功能,能够快速找出与岗位要求最为契合的候选人,大大提高招聘效率和准确性。
而且,招聘过程中的所有数据和文档都能在系统中有序保存,方便后续的查询和分析。
人员管理系统在员工的培训与发展方面也功不可没。
它可以根据员工的岗位需求和个人能力,为其量身定制培训计划。
无论是线上课程学习,还是线下实践操作,系统都能进行有效的跟踪和评估。
员工在培训中的表现、学习成果以及能力提升情况都能一目了然,这有助于企业及时调整培训策略,确保培训的效果达到最佳。
绩效考核是人员管理中的关键一环,而人员管理系统能够为这一环节提供有力支持。
它可以设定多样化的考核指标和评估标准,让考核更加科学、公正。
员工的工作成果、工作态度、团队协作等方面都能被全面考量。
考核结果不仅能够及时反馈给员工,让他们清楚自己的工作表现,还能为薪酬调整、晋升决策等提供重要依据。
薪酬管理也是人员管理系统的重要组成部分。
它可以根据员工的岗位级别、绩效表现等因素,自动计算薪酬,确保薪酬发放的准确性和及时性。
同时,系统还能对薪酬数据进行分析,帮助企业了解人力成本的构成和变化趋势,为制定合理的薪酬策略提供参考。
人员管理系统还能助力员工的职业规划。
通过对员工能力和兴趣的分析,为他们提供个性化的职业发展建议和路径。
这有助于提高员工的工作满意度和忠诚度,降低员工流失率。
员工信息管理系统
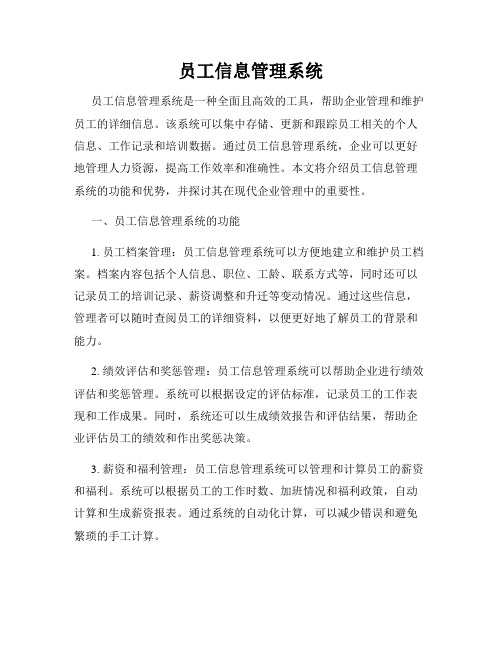
员工信息管理系统员工信息管理系统是一种全面且高效的工具,帮助企业管理和维护员工的详细信息。
该系统可以集中存储、更新和跟踪员工相关的个人信息、工作记录和培训数据。
通过员工信息管理系统,企业可以更好地管理人力资源,提高工作效率和准确性。
本文将介绍员工信息管理系统的功能和优势,并探讨其在现代企业管理中的重要性。
一、员工信息管理系统的功能1. 员工档案管理:员工信息管理系统可以方便地建立和维护员工档案。
档案内容包括个人信息、职位、工龄、联系方式等,同时还可以记录员工的培训记录、薪资调整和升迁等变动情况。
通过这些信息,管理者可以随时查阅员工的详细资料,以便更好地了解员工的背景和能力。
2. 绩效评估和奖惩管理:员工信息管理系统可以帮助企业进行绩效评估和奖惩管理。
系统可以根据设定的评估标准,记录员工的工作表现和工作成果。
同时,系统还可以生成绩效报告和评估结果,帮助企业评估员工的绩效和作出奖惩决策。
3. 薪资和福利管理:员工信息管理系统可以管理和计算员工的薪资和福利。
系统可以根据员工的工作时数、加班情况和福利政策,自动计算和生成薪资报表。
通过系统的自动化计算,可以减少错误和避免繁琐的手工计算。
4. 培训管理:员工信息管理系统可以记录和跟踪员工的培训记录。
系统可以帮助企业制定培训计划、安排培训课程,并记录员工的培训成绩和证书等情况。
通过培训管理,企业可以提升员工的技能水平,提高组织的综合竞争力。
5. 出勤管理:员工信息管理系统可以记录和管理员工的考勤和请假情况。
系统可以方便地统计员工的出勤时间,掌握员工的工作态度和纪律。
同时,系统还可以自动生成考勤报表和请假申请表,提高考勤管理的效率和准确性。
二、员工信息管理系统的优势1. 提高工作效率:员工信息管理系统可以实现信息的集中管理和自动化处理,减少手工操作和重复工作。
员工的信息和数据可以方便地被检索和更新,节省了大量的时间和人力。
2. 数据准确性和安全性:员工信息管理系统可以确保信息的准确性和安全性。
(完整word版)员工管理系统
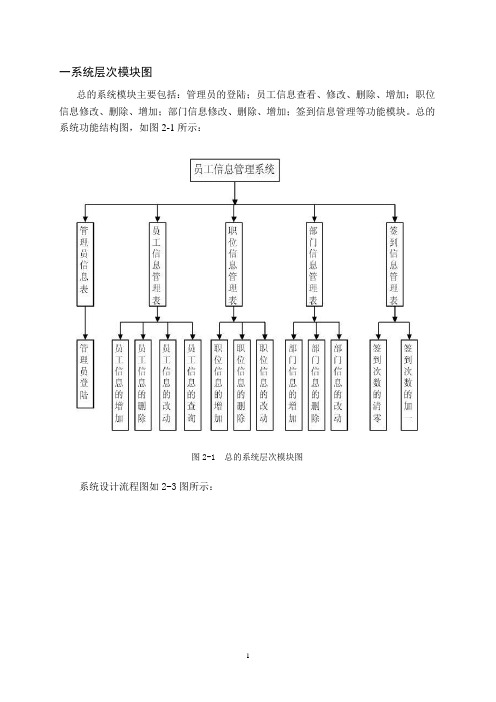
一系统层次模块图总的系统模块主要包括:管理员的登陆;员工信息查看、修改、删除、增加;职位信息修改、删除、增加;部门信息修改、删除、增加;签到信息管理等功能模块。
总的系统功能结构图,如图2-1所示:图2-1 总的系统层次模块图系统设计流程图如2-3图所示:图2-3 系统设计流程图一个员工对应一个职位,一个员工对应一个部门,一个员工对应一个签到信息。
员工与相关信息表的E-R图如图3-5所示:1. admin(管理员信息表)管理员信息表用来存储管理员的基本信息。
其中包括管理员用户名和管理员登录密码等字段,表admin的结构如表3-1所示:字段名数据类型长度是否主键描述Id int 4 是唯一标识ldy_str_uname varchar30管理员用户名ldy_str_pwd varchar 20 登陆密码2. users (员工信息表)员工信息表(users)此表主要用于存储员工的相关信息,包括员工编号,员工名,员工地址,员工性别,员工备注,员工年龄以及与各表连接的相关字段等。
表users的结构如表3-2所示。
3. dep(部门信息表)此表主要用于存储部门的信息,包括部门编号,部门名称,部门备注等。
表dep的结构如表3-3所示。
4. job(职位信息表)职位信息表主要用于保存各类职位信息,包括职位编号,职位名称,职位工资,职位备注等字段。
表job的结构如表3-4所示。
5. qiandao(签到信息表)签到信息表主要用于保存员工签到信息,包括签到编号,员工编号,签到次数等字段。
表qiandao的结构如表3-5所示。
企业员工信息管理系统
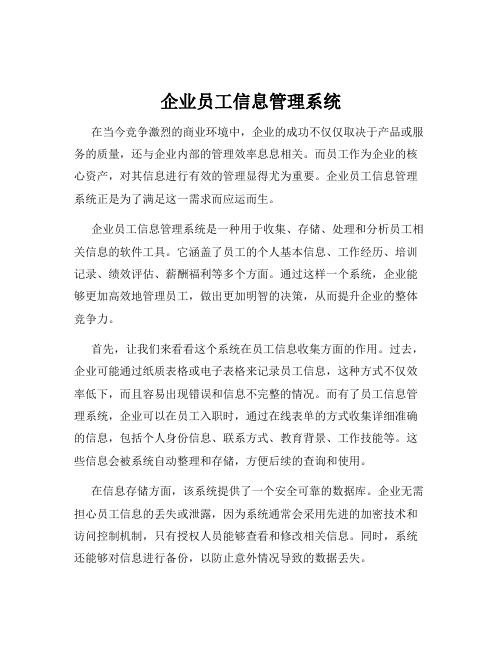
企业员工信息管理系统在当今竞争激烈的商业环境中,企业的成功不仅仅取决于产品或服务的质量,还与企业内部的管理效率息息相关。
而员工作为企业的核心资产,对其信息进行有效的管理显得尤为重要。
企业员工信息管理系统正是为了满足这一需求而应运而生。
企业员工信息管理系统是一种用于收集、存储、处理和分析员工相关信息的软件工具。
它涵盖了员工的个人基本信息、工作经历、培训记录、绩效评估、薪酬福利等多个方面。
通过这样一个系统,企业能够更加高效地管理员工,做出更加明智的决策,从而提升企业的整体竞争力。
首先,让我们来看看这个系统在员工信息收集方面的作用。
过去,企业可能通过纸质表格或电子表格来记录员工信息,这种方式不仅效率低下,而且容易出现错误和信息不完整的情况。
而有了员工信息管理系统,企业可以在员工入职时,通过在线表单的方式收集详细准确的信息,包括个人身份信息、联系方式、教育背景、工作技能等。
这些信息会被系统自动整理和存储,方便后续的查询和使用。
在信息存储方面,该系统提供了一个安全可靠的数据库。
企业无需担心员工信息的丢失或泄露,因为系统通常会采用先进的加密技术和访问控制机制,只有授权人员能够查看和修改相关信息。
同时,系统还能够对信息进行备份,以防止意外情况导致的数据丢失。
处理和分析员工信息是该系统的另一个重要功能。
例如,通过对员工绩效评估数据的分析,企业可以了解到员工的工作表现趋势,找出优秀员工和需要改进的员工,为制定培训计划和晋升决策提供依据。
此外,系统还可以对员工的薪酬福利数据进行处理,帮助企业进行成本核算和预算规划。
对于员工的培训管理,企业员工信息管理系统也发挥着重要作用。
系统可以记录员工参加过的培训课程、培训时间、培训效果等信息。
企业可以根据这些数据,评估培训的效果,调整培训计划,以提高员工的技能水平和工作能力。
在人力资源规划方面,系统能够提供有价值的信息支持。
企业可以通过分析员工的年龄结构、学历分布、专业技能等数据,预测未来的人力资源需求,提前做好招聘和人才储备工作。
企业员工管理系统有哪些

企业员工管理系统有哪些企业员工管理系统是指一套用于管理企业员工信息、日常工作、绩效考核及培训等的软件系统。
它通过集成现代信息技术,实现对企业员工的全面管理,为企业提供高效、便捷的人力资源管理工具。
以下将介绍企业员工管理系统的主要功能和优势。
一、员工信息管理员工信息管理是企业员工管理系统的核心功能之一。
通过该系统,企业能够高效地管理员工的个人信息、合同信息、职位和岗位信息等。
同时,还可以实时记录员工的工作经历、教育背景和培训记录,方便企业对员工进行全面的档案管理。
二、考勤管理考勤管理是企业员工管理系统的重要组成部分。
系统可以帮助企业自动化管理员工的考勤记录,包括打卡考勤、请假请销假等。
通过系统的数据分析功能,企业能够及时了解员工的考勤情况,减少无效劳动时间,提高出勤率和工作效率。
三、工作任务分配与跟踪企业员工管理系统提供了工作任务分配和跟踪功能,可以帮助领导者合理安排员工的工作任务,并实时追踪任务进度。
领导者可以通过系统给员工指派任务,并设置截止日期和优先级,以确保任务按时完成,并及时发现和解决问题。
四、绩效考核与奖惩员工绩效考核是企业管理的重要环节之一,也是提高企业员工工作积极性和效率的关键。
企业员工管理系统提供了绩效考核的功能,可以帮助企业制定科学合理的考核标准,并根据员工的绩效评分进行奖惩。
同时,系统还能根据绩效数据进行绩效分析,为企业管理层提供决策依据。
五、培训管理企业员工管理系统可以支持企业的培训管理工作。
企业可以通过系统发布培训计划和培训资源,实现对员工的培训需求和培训进度的管理。
系统还能够记录员工的培训记录,为企业管理层提供员工培训情况的数据分析和评估。
六、福利管理福利管理是企业员工管理系统的一项重要功能。
通过系统,企业可以管理员工的福利待遇,如工资、社会保险、福利性住房等。
系统可以自动生成工资表和报表,方便企业核对和管理工资福利发放,提高工资福利管理的准确性和效率。
七、通知公告发布企业员工管理系统还可以用于企业内部通知公告的发布。
员工管理系统的功能有哪些

员工管理系统的功能有哪些员工管理系统的功能有哪些员工管理系统是很多企业都具备的系统软件之一,然而很多的员工都不知道员工管理系统有作用。
下面为您精心推荐了员工管理系统的作用,希望对您有所帮助。
员工管理系统的功能部门分类按照公司内部部门不同、职能不同来定义不同的工作界面,如:销售部、技术部、财务部、采购部、办公室等。
并可授权增加、修改、查询。
设定员工的权限根据员工的工作性质可设定员工的工作权限,并可对权限进行增加与修改,所以具有不同权限的员工在网络上只能浏览到权限内的相关网页,而具有特殊权限的员工方可对网页内容进行修改。
建立员工的工作日志根据公司的`工作性质,按照部门及人员的工作性质建立员工的工作日志,根据员工的权限可具有编辑、浏览、修改、重复提交、批复等功能。
实现网上员工培训公司可将对员工的培训在网上进行,首先它具有时时化,员工可自主地安排自己的工作及选择的内容,从而避免了培训的重复性,其次它具有人性化,企业员工一般分为新员工和老员工,将要培训的内容分为企业文化类、产品服务类、管理销售类、技能与技巧类等。
所以可对所要培训的内容建立数据库,这样就可以时时更新,根据不同员工的权限来划分培训的内容,支持教学课件,支持动画及Powerpoint展示,如果要求培训质量较高可进行语言配音等,也可建立培训考核与测评系统,定期对公司员工当前文化状况进行考核,使考核结果更具有公正性与准确性,这样公司的培训就可以利用网络来完成。
建立公司公告与员工留言版公司公告是公司公布最新信息,下发公司文件与通知的窗口,员工留言版是员工对公司的产品服务等情况发表自己的想法与意见的工具,通过公司公告与员工留言版,使公司与员工之间建立沟通的桥梁,可根据员工的不同权限,设立留言版的浏览与修改的权限。
,设立留言版的浏览与修改的权限。
员工管理系统的分类单机版主要功能包括机构管理、信息录入、批量处理、查询输出、工资管理、报表管理、系统维护、用户管理、人员类别管理、数据库管理等。
公司人员管理系统

公司人员管理系统一、引言近年来,随着经济和社会的发展,企业逐渐变得庞大、复杂,而公司人员管理系统的出现可以为公司人力资源管理提供便利。
公司人员管理系统即为一种以计算机技术为基础,通过人员管理软件对企业人员进行管理、监督、考核的一种信息系统。
本文就公司人员管理系统的定义、特点及优势等进行探讨,并提出建设公司人员管理系统的几点建议。
二、公司人员管理系统的特点1. 信息化与集成化:公司人员管理系统是一种基于信息技术的人力资源管理方式,可以将人员信息集成到一个系统中,方便企业进行统一的管理。
2. 动态监控:公司人员管理系统可以实时监控员工的工作情况,包括工作状态、工作效率等,有利于企业管理者对员工情况进行反馈和指导。
3. 数据统计与分析:公司人员管理系统可以对企业内部数据进行统计和分析,从而为公司决策提供重要参考依据。
三、公司人员管理系统的优势1. 提高管理效率:公司人员管理系统可以提高人力资源管理的效率,加速企业内部信息的传递,以及数据的收集与分析。
2. 精准管理:公司人员管理系统可以通过实时监控员工工作状态,提高管理者对员工的管理精准度。
3. 降低管理成本:公司人员管理系统的使用可以大大降低企业员工管理的成本,避免因人工管理而产生的管理费用。
4. 保护员工隐私:公司人员管理系统可以对员工的个人信息进行安全保护,有效避免员工的个人信息被滥用、泄露等情况。
四、公司人员管理系统的建设1. 信息分类清晰:在建设公司人员管理系统时,需要对员工信息进行分类,包括基本信息、薪资信息、考勤信息等,确保信息清晰明了。
2. 数据安全保护:在公司人员管理系统的建设中,数据安全保护是十分重要的一个方面,建议采取数据加密、访问控制等措施保护数据安全。
3. 系统人性化设计:公司人员管理系统的界面设计要人性化,使得员工和经理都能轻松上手。
同时,提供了完善的员工培训和技术支持也是必须的。
4. 实用性要求高:公司人员管理系统是为了提高工作效率和管理水平而设计的,因此其实用性要求高,系统的功能要覆盖公司实际的人力资源管理需求。
公司员工管理系统

公司员工管理系统第一部分:项目背景与需求分析一、项目背景随着市场竞争的日益激烈,企业对管理效率和员工素质的要求不断提高。
为提高公司管理水平,降低人力资源成本,实现企业可持续发展,公司决定开发一套员工管理系统。
该系统将涵盖员工基本信息管理、薪资福利管理、考勤管理、培训发展管理等多个方面,为公司提供一个便捷、高效、安全的管理工具。
二、需求分析1. 功能需求(1)员工基本信息管理:包括员工基本资料、岗位信息、联系方式等,支持信息的添加、修改、查询和删除。
(2)薪资福利管理:自动计算员工工资、奖金、扣款等,支持工资单的导出和打印。
(3)考勤管理:记录员工考勤数据,包括迟到、早退、请假等,支持考勤数据的统计和查询。
(4)培训发展管理:记录员工培训经历、晋升记录等,支持培训计划的制定和跟踪。
(5)权限管理:根据不同角色分配不同权限,确保系统数据安全。
2. 性能需求(1)响应速度:系统需在短时间内完成数据处理,确保用户体验。
(2)稳定性:系统运行稳定,数据安全可靠。
(3)可扩展性:系统具备良好的可扩展性,以便后期根据公司需求进行功能扩展。
3. 系统架构需求(1)采用B/S架构,便于用户通过浏览器访问。
(2)前后端分离,提高系统开发效率和可维护性。
(3)采用分布式数据库,确保数据存储安全可靠。
三、项目目标1. 提高管理效率:通过系统化管理,降低人力成本,提高工作效率。
2. 优化员工体验:为员工提供一个便捷、高效的工作环境,提升员工满意度。
3. 促进企业可持续发展:通过系统对员工培训、晋升等管理,提升企业核心竞争力。
4. 确保数据安全:采用先进的技术手段,确保系统数据安全可靠。
四、项目范围1. 项目范围:涵盖公司全体员工。
2. 项目实施地点:公司内部网络环境。
3. 项目周期:预计项目周期为6个月,包括需求分析、系统设计、开发、测试和部署等阶段。
本部分详细阐述了公司员工管理系统的项目背景、需求分析、项目目标和项目范围,为后续系统设计和开发提供了基础。
简单的员工管理系统

简单的员工管理系统员工管理是一项重要的任务,对于任何一家公司而言,有效的员工管理可以提高工作效率,加强团队合作,最终实现组织的目标。
为了更好地实现员工管理的目的,许多公司开始采用员工管理系统。
本文将介绍一个简单的员工管理系统的功能和实施方法。
一、系统概述简单的员工管理系统是一种用于管理公司员工信息、考勤记录、培训计划和绩效评估的软件系统。
该系统的目标是提供一个集中存储和管理员工数据的平台,从而方便公司管理层对员工的管理和决策。
二、系统功能1. 员工信息管理:系统可以记录和管理所有员工的基本信息,包括姓名、性别、出生日期、联系方式、职位、薪资等。
同时,系统还可以为每个员工分配一个唯一的员工编号,方便唯一标识和查询。
2. 考勤记录:系统可以记录员工的考勤情况,包括迟到、早退、请假、加班等。
通过考勤记录,管理层可以了解到员工的工作态度和出勤情况,及时发现问题并进行相应的处理。
3. 培训管理:系统可以制定并记录员工的培训计划。
管理层可以根据员工的需求和公司的发展需求,安排相应的培训课程,并记录员工的培训情况和成绩。
4. 绩效评估:系统可以对员工进行绩效评估,并为每个员工生成相应的评估报告。
通过绩效评估,可以对员工的工作表现进行全面评估,为员工的晋升、加薪或奖惩提供依据。
三、系统实施方法1. 软件选择:根据公司的需求和预算,选择适合的员工管理软件。
可以考虑购买商业化的员工管理系统,也可以自行开发或定制。
2. 数据录入:将公司现有的员工信息录入系统,包括基本信息、工作经历、职位等。
同时,可以将以往的考勤记录、培训记录和绩效评估结果导入系统中,以便于管理和查询。
3. 培训计划制定:根据员工的职位和发展需求,制定相应的培训计划。
计划包括培训课程内容、培训时间和培训地点等。
同时,可以设定培训计划的执行进度和完成情况的跟踪。
4. 绩效评估:系统可以根据公司设定的绩效评估指标,自动生成绩效评估表。
评估结果可以由管理层进行评审,并对员工的绩效进行相应的奖励或处罚。
员工信息管理系统
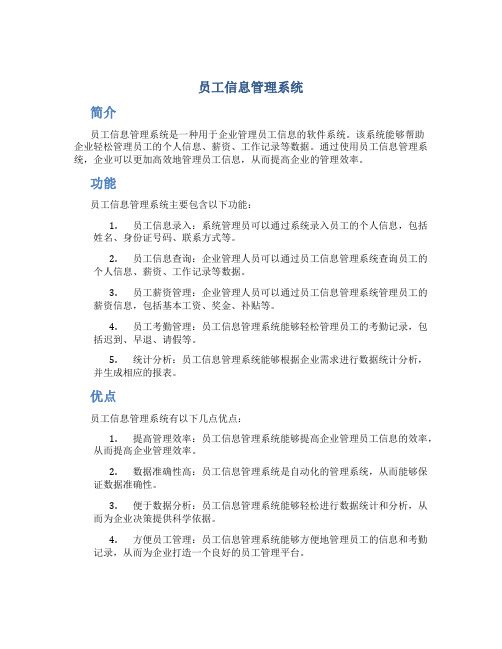
员工信息管理系统简介员工信息管理系统是一种用于企业管理员工信息的软件系统。
该系统能够帮助企业轻松管理员工的个人信息、薪资、工作记录等数据。
通过使用员工信息管理系统,企业可以更加高效地管理员工信息,从而提高企业的管理效率。
功能员工信息管理系统主要包含以下功能:1.员工信息录入:系统管理员可以通过系统录入员工的个人信息,包括姓名、身份证号码、联系方式等。
2.员工信息查询:企业管理人员可以通过员工信息管理系统查询员工的个人信息、薪资、工作记录等数据。
3.员工薪资管理:企业管理人员可以通过员工信息管理系统管理员工的薪资信息,包括基本工资、奖金、补贴等。
4.员工考勤管理:员工信息管理系统能够轻松管理员工的考勤记录,包括迟到、早退、请假等。
5.统计分析:员工信息管理系统能够根据企业需求进行数据统计分析,并生成相应的报表。
优点员工信息管理系统有以下几点优点:1.提高管理效率:员工信息管理系统能够提高企业管理员工信息的效率,从而提高企业管理效率。
2.数据准确性高:员工信息管理系统是自动化的管理系统,从而能够保证数据准确性。
3.便于数据分析:员工信息管理系统能够轻松进行数据统计和分析,从而为企业决策提供科学依据。
4.方便员工管理:员工信息管理系统能够方便地管理员工的信息和考勤记录,从而为企业打造一个良好的员工管理平台。
使用注意事项使用员工信息管理系统需要注意以下几点:1.系统管理员需要妥善保存系统数据,避免数据丢失或泄露。
2.企业管理人员需要密切关注员工信息管理系统的使用情况,并及时对系统进行维护和升级。
3.每个员工需要保护自己的个人信息,避免泄露。
员工信息管理系统能够帮助企业更加高效地管理员工信息,提高企业管理效率。
虽然使用员工信息管理系统需要注意一些问题,但是这些问题可以通过企业和员工的共同努力来解决。
建议企业尽早使用员工信息管理系统,提高管理效率,促进企业发展。
员工管理系统的四种模式
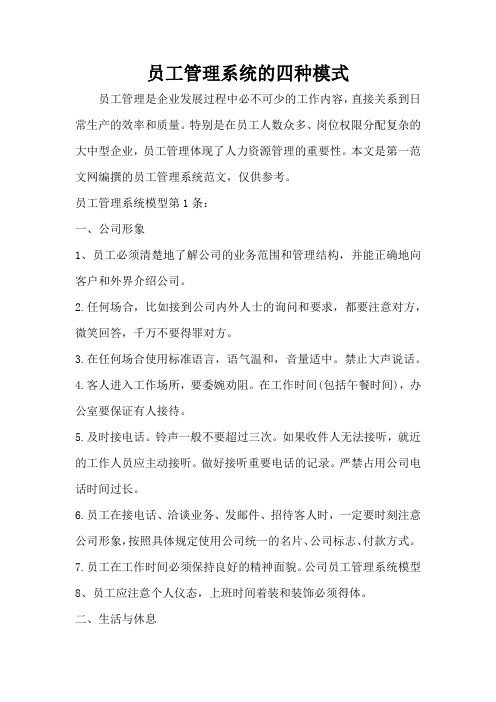
员工管理系统的四种模式员工管理是企业发展过程中必不可少的工作内容,直接关系到日常生产的效率和质量。
特别是在员工人数众多、岗位权限分配复杂的大中型企业,员工管理体现了人力资源管理的重要性。
本文是第一范文网编撰的员工管理系统范文,仅供参考。
员工管理系统模型第1条:一、公司形象1、员工必须清楚地了解公司的业务范围和管理结构,并能正确地向客户和外界介绍公司。
2.任何场合,比如接到公司内外人士的询问和要求,都要注意对方,微笑回答,千万不要得罪对方。
3.在任何场合使用标准语言,语气温和,音量适中。
禁止大声说话。
4.客人进入工作场所,要委婉劝阻。
在工作时间(包括午餐时间),办公室要保证有人接待。
5.及时接电话。
铃声一般不要超过三次。
如果收件人无法接听,就近的工作人员应主动接听。
做好接听重要电话的记录。
严禁占用公司电话时间过长。
6.员工在接电话、洽谈业务、发邮件、招待客人时,一定要时刻注意公司形象,按照具体规定使用公司统一的名片、公司标志、付款方式。
7.员工在工作时间必须保持良好的精神面貌。
公司员工管理系统模型8、员工应注意个人仪态,上班时间着装和装饰必须得体。
二、生活与休息1.员工应严格按照公司统一的工作时间表上班。
2.作息时间表1)、夏季计划表(4月——9)工作时间是早上9点。
午休12: 00——13: 00下班后18: 002)冬季作息时间表(10月——3)工作时间是早上9点。
午休12: 00——12: 30下班后17: 303.员工上下班要签到,上下班必须亲自签到,不得为他人签到。
4.员工的考勤记录将是公司绩效考核的重要组成部分。
5.如果员工因公需要在工作时间外出,应在离开公司前向主管经理请示。
6.员工突发疾病,当天必须向主管经理请假,事后提交相关证明。
7.事假要提前向主管经理申请,填写《请假申请表》。
批准后才能休息。
8.员工在国家法定节假日享有正常休息的权利。
公司不提倡员工加班,鼓励员工在日常工作时间做好自己的工作。
员工管理信息系统

员工管理信息系统在当今竞争激烈的商业环境中,企业的成功离不开高效的员工管理。
而员工管理信息系统作为一种现代化的管理工具,正逐渐成为企业提升管理效率、优化人力资源配置的重要手段。
员工管理信息系统是什么呢?简单来说,它是一个利用信息技术对员工的各类信息进行收集、存储、分析和处理的系统。
通过这个系统,企业能够更加便捷、准确地掌握员工的基本情况、工作表现、培训经历、薪酬福利等重要信息,从而为企业的决策提供有力支持。
员工管理信息系统的重要性不言而喻。
首先,它大大提高了管理效率。
以往,人力资源部门可能需要花费大量的时间和精力来处理员工的各种信息,如整理档案、计算薪酬、统计考勤等。
有了这个系统,这些工作可以实现自动化处理,大大节省了时间和人力成本。
其次,系统能够提供更准确和及时的信息。
人工处理信息难免会出现错误,而系统可以减少这类失误,保证数据的准确性。
同时,系统能够实时更新数据,让管理者随时掌握最新的员工动态。
再者,它有助于优化人力资源配置。
通过对员工信息的分析,企业可以了解员工的技能、特长和潜力,从而将员工安排在最适合的岗位上,充分发挥他们的优势,提高整体工作效率。
一个完善的员工管理信息系统通常包括以下几个主要模块。
员工基本信息模块是基础。
这里记录着员工的个人资料,如姓名、性别、年龄、联系方式、学历、工作经历等。
这些信息是企业了解员工的第一步,也是进行后续管理的重要依据。
工作表现评估模块则用于记录员工的工作绩效。
包括工作任务的完成情况、工作质量、工作效率、团队合作能力等方面的评估。
通过这个模块,企业可以清楚地看到每个员工的工作表现,为奖惩、晋升等决策提供参考。
培训与发展模块也是不可或缺的一部分。
它记录了员工参加的培训课程、培训效果以及未来的发展计划。
这有助于企业根据员工的需求和企业的发展战略,为员工提供有针对性的培训,提升员工的能力和素质。
薪酬福利管理模块负责管理员工的薪酬和福利信息。
包括工资、奖金、社保、公积金、福利发放等。
人员管理系统,员工管理系统(一)2024

人员管理系统,员工管理系统(一)引言概述:人员管理系统,又称员工管理系统,是一种用于管理组织内员工信息、工作分配、考核绩效等的软件系统。
该系统能够提高人力资源管理的效率和质量,帮助组织实现对人力资源的全面管理和优化。
本文将详细介绍人员管理系统的五个主要模块:员工信息管理、工作分配与监控、考核绩效管理、培训发展和福利管理,以及系统安全与权限控制。
一、员工信息管理:1. 基本信息录入:系统能够方便地录入员工的基本信息,如姓名、性别、出生日期等;2. 个人档案管理:系统可以存储和管理每个员工的个人档案,包括联系方式、学历、工作经历等;3. 职位调动与晋升:系统能够记录员工的职位变动情况,包括调动的原因和晋升的依据;4. 工作合同管理:系统可以管理员工的工作合同信息,包括合同起止日期、合同类型和签订地点等;5. 离职管理:系统可以记录员工的离职信息,包括离职原因和离职时间。
二、工作分配与监控:1. 工作任务分配:系统能够将工作任务分配给合适的员工,实现任务的合理分配;2. 进度监控与报告:系统可以实时监控员工的工作进度,并生成相应的工作报告;3. 协同办公:系统支持员工之间的协同办公,提高工作效率和沟通效果;4. 考勤管理:系统可以记录员工的考勤情况,包括请假、加班和迟到早退等;5. 绩效评估:系统可以对员工的工作绩效进行评估,提供一定的绩效考核依据。
三、考核绩效管理:1. 考核指标设定:系统支持设定多种考核指标,如工作质量、工作态度和工作效率等;2. 绩效评估方法:系统可以根据设定的考核指标,采用定量或定性方法对员工进行绩效评估;3. 绩效报告生成:系统可以生成员工的绩效报告,提供对绩效情况的简明概括;4. 绩效奖励与惩罚:系统能够根据员工的绩效表现,给予适当的奖励或者惩罚;5. 绩效数据分析:系统可以对员工的绩效数据进行分析,为公司提供决策支持和人力资源优化建议。
四、培训发展与福利管理:1. 培训需求分析:系统可以根据员工的发展需求和公司的培训计划,进行培训需求分析;2. 培训计划制定:系统支持制定公司的培训计划,包括培训内容、时间和地点等;3. 培训资源管理:系统可以管理培训资源,包括讲师、培训材料和场地等;4. 员工福利管理:系统能够管理员工的福利信息,如社保、医疗保险和节假日福利等;5. 员工发展规划:系统可以记录和管理员工的职业发展规划,为员工提供职业成长的支持和指导。
员工信息管理系统

员工信息管理系统简介员工信息管理系统是一种用于组织和存储公司员工信息的系统。
通过该系统,公司可以轻松地管理员工相关信息,包括基本信息、工作经历、薪酬数据等。
本文将介绍员工信息管理系统的设计、功能以及优势。
设计员工信息管理系统的设计包括数据库设计、界面设计以及功能模块设计。
数据库设计是系统的核心,需要合理地设计员工信息表、部门表、项目表等,以便快速查询和管理数据。
界面设计应简洁明了,方便用户操作。
功能模块设计需要考虑系统的全面性,包括员工信息录入、查询、修改、删除等功能。
功能员工信息管理系统的主要功能包括: 1. 员工信息录入:管理员可以通过系统录入员工的基本信息,如姓名、工号、部门等。
2. 员工信息查询:可以根据不同条件查询员工的信息,如姓名、部门、工号等。
3. 员工信息修改:管理员可以修改员工的信息,保证数据的准确性。
4. 员工信息删除:允许管理员删除不必要的员工信息,保持数据的清洁。
5. 权限管理:不同级别的用户拥有不同的权限,保证信息安全和管理的合理性。
优势员工信息管理系统的优势主要体现在以下几个方面: 1. 高效性:通过系统化管理员工信息,可以提高信息的查找和管理效率。
2. 准确性:系统可以减少人为错误,保障员工信息的准确性。
3. 安全性:权限管理功能可以确保员工信息不被未授权人员查看或修改。
4. 便捷性:员工信息管理系统可以随时随地查看员工信息,方便管理人员及时做出决策。
结语员工信息管理系统是一种重要的企业管理工具,可以有效地管理和维护员工信息,提高公司的管理效率和信息的安全性。
希望本文对员工信息管理系统有所启发,帮助读者更好地了解和使用这一工具。
员工管理系统功能模块

员工管理系统功能模块一、简介员工管理系统是一种用于管理和组织公司内部员工信息的软件系统。
它提供一系列功能模块,用于跟踪、管理和分析员工的工作情况、培训需求以及其他相关信息。
本文将深入探讨员工管理系统的功能模块,包括员工信息管理、考勤管理、培训管理、绩效管理以及薪资管理。
二、员工信息管理模块员工信息管理模块是员工管理系统的核心功能模块之一。
它用于记录和管理员工的基本信息,包括姓名、性别、出生日期、联系方式等。
在该模块中,管理员可以进行员工信息的增加、删除、修改和查询等操作。
1. 员工信息录入在该功能模块中,管理员可以通过表单或者导入Excel文件的方式将新员工的信息录入系统中。
录入信息的同时,系统还可以自动生成员工工号和登录账号等唯一标识符。
2. 员工信息查询管理员可以通过不同的条件对员工信息进行查询,例如按照部门、职位、入职时间等进行筛选。
查询结果将以列表的形式展示,包括关键信息如姓名、工号和所在部门等。
3. 员工信息修改和删除当员工信息发生变动时,管理员可以对员工的信息进行修改。
同时,如果员工离职或者其他原因不再需要在系统中存在,管理员也可以将其信息从系统中删除。
三、考勤管理模块考勤管理模块用于记录员工的工作情况,包括上下班打卡时间、请假、加班等信息。
该模块可以帮助企业监控员工的工作态度和工作效率,及时发现并解决问题。
1. 打卡管理系统可以提供打卡功能,员工通过刷卡、指纹识别或者人脸识别等方式进行打卡,将打卡时间自动记录在系统中。
管理员可以查看员工的打卡记录,及时发现迟到、早退等情况。
2. 请假管理员工可以通过系统提交请假申请,在该模块中管理员可以审批请假申请,并将请假信息记录在系统中。
员工可以随时查询自己的请假历史记录,避免冲突和纠纷。
3. 加班管理员工加班时可以通过系统进行加班申请,管理员可以根据需要进行审批。
加班记录将被记录在系统中,方便薪资计算和统计分析。
四、培训管理模块培训管理模块用于记录和管理员工的培训情况,包括参加的培训课程、培训成绩等。
企业员工信息管理系统
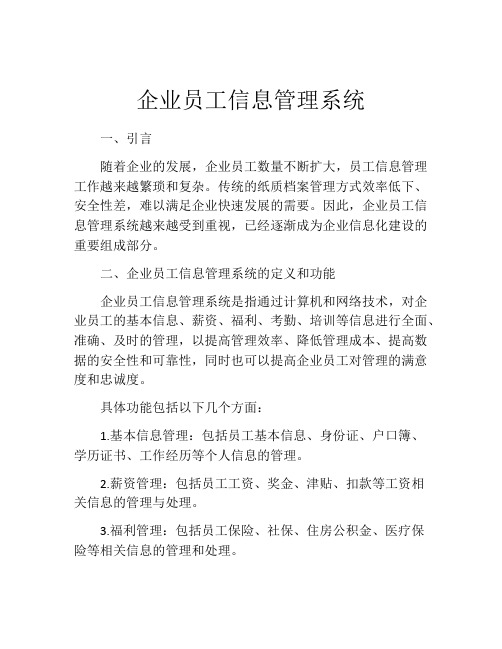
企业员工信息管理系统一、引言随着企业的发展,企业员工数量不断扩大,员工信息管理工作越来越繁琐和复杂。
传统的纸质档案管理方式效率低下、安全性差,难以满足企业快速发展的需要。
因此,企业员工信息管理系统越来越受到重视,已经逐渐成为企业信息化建设的重要组成部分。
二、企业员工信息管理系统的定义和功能企业员工信息管理系统是指通过计算机和网络技术,对企业员工的基本信息、薪资、福利、考勤、培训等信息进行全面、准确、及时的管理,以提高管理效率、降低管理成本、提高数据的安全性和可靠性,同时也可以提高企业员工对管理的满意度和忠诚度。
具体功能包括以下几个方面:1.基本信息管理:包括员工基本信息、身份证、户口簿、学历证书、工作经历等个人信息的管理。
2.薪资管理:包括员工工资、奖金、津贴、扣款等工资相关信息的管理与处理。
3.福利管理:包括员工保险、社保、住房公积金、医疗保险等相关信息的管理和处理。
4.考勤管理:包括员工考勤、请假、出差、加班等考勤信息的管理。
5.培训管理:包括员工培训计划、培训课程、培训成绩、培训证书等关于员工培训的管理。
三、企业员工信息管理系统的优点1. 提高管理效率传统的人工维护员工信息,难以胜任大规模员工信息管理。
而企业员工信息管理系统通过计算机和网络等高效的技术手段,能够提高数据的管理效率,降低管理成本,提高管理的效率和准确性。
2. 减少工作量员工信息管理系统能够在很大程度上自动完成工作,不仅可以避免冗杂的人工操作,还可以把人工操作简化至最低程度,从而有效减少工作量,提高工作效率。
3. 提高数据的安全性和可靠性员工信息管理系统采用密码保护、安全备份等技术手段,保证企业员工信息的安全性和可靠性,避免信息的泄露和损坏。
可以有效地保护企业员工信息的真实性和准确性,避免错误和偏差。
4. 提高员工满意度和忠诚度员工信息管理系统能够减轻管理人员的工作量,使得管理人员有更多的精力处理公司事务和员工管理,从而能够更好地解决员工的问题和需求。
员工管理系统
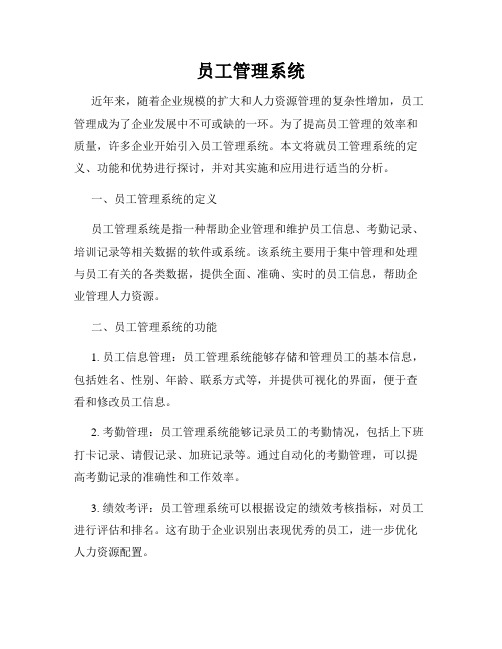
员工管理系统近年来,随着企业规模的扩大和人力资源管理的复杂性增加,员工管理成为了企业发展中不可或缺的一环。
为了提高员工管理的效率和质量,许多企业开始引入员工管理系统。
本文将就员工管理系统的定义、功能和优势进行探讨,并对其实施和应用进行适当的分析。
一、员工管理系统的定义员工管理系统是指一种帮助企业管理和维护员工信息、考勤记录、培训记录等相关数据的软件或系统。
该系统主要用于集中管理和处理与员工有关的各类数据,提供全面、准确、实时的员工信息,帮助企业管理人力资源。
二、员工管理系统的功能1. 员工信息管理:员工管理系统能够存储和管理员工的基本信息,包括姓名、性别、年龄、联系方式等,并提供可视化的界面,便于查看和修改员工信息。
2. 考勤管理:员工管理系统能够记录员工的考勤情况,包括上下班打卡记录、请假记录、加班记录等。
通过自动化的考勤管理,可以提高考勤记录的准确性和工作效率。
3. 绩效考评:员工管理系统可以根据设定的绩效考核指标,对员工进行评估和排名。
这有助于企业识别出表现优秀的员工,进一步优化人力资源配置。
4. 培训管理:员工管理系统可以记录员工的培训记录和培训成绩。
通过这一功能,管理者可以及时了解员工的培训需求,有针对性地进行培训计划。
5. 薪资管理:员工管理系统可以管理和计算员工的薪资,并提供差旅费、补贴等其他相关费用的管理功能。
这有助于提高薪资发放的准确性和透明度。
三、员工管理系统的优势1. 提高工作效率:员工管理系统通过自动化的数据处理和报表生成,节省了人力资源管理的时间和精力,提高了工作效率。
管理者可以更加专注于员工发展和团队建设。
2. 降低人力成本:传统的员工管理需要大量的人力资源投入,而员工管理系统可以大幅度降低人力成本,减少了人工处理数据和文件的工作量。
3. 提供决策依据:员工管理系统能够提供全面、准确的员工数据和报表,为管理者提供决策依据。
管理者可以根据员工的绩效情况和培训需求,合理分配人力资源,提高企业整体的竞争力。
员工管理系统
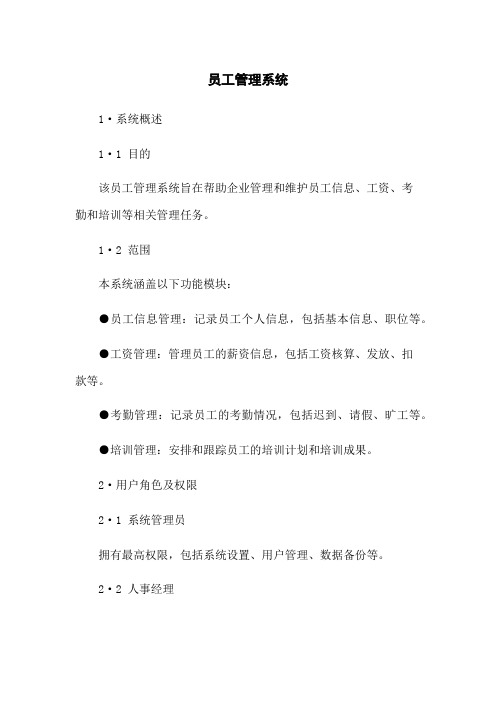
员工管理系统1·系统概述1·1 目的该员工管理系统旨在帮助企业管理和维护员工信息、工资、考勤和培训等相关管理任务。
1·2 范围本系统涵盖以下功能模块:●员工信息管理:记录员工个人信息,包括基本信息、职位等。
●工资管理:管理员工的薪资信息,包括工资核算、发放、扣款等。
●考勤管理:记录员工的考勤情况,包括迟到、请假、旷工等。
●培训管理:安排和跟踪员工的培训计划和培训成果。
2·用户角色及权限2·1 系统管理员拥有最高权限,包括系统设置、用户管理、数据备份等。
2·2 人事经理可对员工信息进行增删改查、工资管理、考勤管理、培训管理等操作。
2·3 普通员工仅具有查看自己的信息、申请请假等操作。
3·数据库设计为实现以上功能,系统将使用以下数据库表格:●员工表格:存储员工的个人信息。
●工资表格:存储员工的薪资信息。
●考勤表格:记录员工的考勤情况。
●培训表格:记录员工的培训计划和成果。
4·系统界面设计4·1 登录界面用户通过该界面输入用户名和密码进行登录。
4·2 员工信息管理界面该界面用于显示和管理员工的个人信息,包括查看、添加、修改和删除员工信息的功能。
4·3 工资管理界面该界面用于管理员工的薪资信息,包括工资核算、发放、扣款等操作。
4·4 考勤管理界面该界面用于记录员工的考勤情况,包括迟到、请假、旷工等。
4·5 培训管理界面该界面用于安排和跟踪员工的培训计划和培训成果。
5·系统部署及运行5·1 硬件要求●服务器:至少4核CPU,16GB内存,500GB硬盘空间。
●客户端:支持最新版本的浏览器。
5·2 软件要求●操作系统:Windows Server 2016/Linux。
●数据库:MySQL 8·0或以上。
●Web服务器:Apache Tomcat 9·0或以上。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
公司员工管理系统
/////////////////////////////////////////////////////////
//公司员工管理系统--CEMS
#include<fstream.h> //文件操作头文件
#include<stdlib.h> //包含system()等函数的头文件#include<string> //字符串处理头文件
#include<iomanip.h> //输入输出流重载需要的头文件#include<stdio.h>
//////////////////////////////////////////////////////////
// 工资明细结构体
typedef struct WAGE
{
float Base_Wage; // 基本工资
float Merit_Wage; // 绩效工资
float Sum_Wage; // 总工资
}WAGE;
// 包括职工姓名、职工号的工资记录
typedef struct Emplo_Wage
{
char id[10]; // 职工编号
char name[10]; // 职工姓名
WAGE data; // 工资
}Emplo_Wage;
typedef struct Node
{
char E_id[10]; //职工号
char E_name[10]; //姓名
char E_sex[3]; //性别
char E_dep[20]; //部门
char E_job[20]; //职务
float E_wage; //工资
struct Node *prior; //前驱指针
struct Node *next; //后继指针
}Node,*DLink;
////////////////////////////////////////////////////////// // 工资大于1000的员工,超过部门需要按税率交税float Tax_Rate1=0.05f; // 3000 以下
float Tax_Rate2=0.1f; // 3000-8000
float Tax_Rate3=0.15f; // 8000 以上
void SetWage(DLink p);
//////////////////////////////////////////////////////////
// 职工类
class employee
{
private:
Node data; // 结构体类型的数据成员
public:
friend ostream & operator<<(ostream & stream,const DLink p); //友元重载输出
流运算符
friend istream & operator>>(istream & stream,DLink p); //友元重载输入
流运算符
employee(); // 构造函数
DLink CreateLink(); // 创立链表
DLink InsertNode(DLink Head); // 插入一个结点
};
///////////////////////////////////////////////////////////
// 全局常量,一个结点的大小
const int NUM=sizeof(Node);
///////////////////////////////////////////////////////////
// 重载输出流运算符
ostream & operator<<(ostream & stream,const DLink p)
{
stream<<setiosflags(ios::left)<<setw(10)<<p-
>E_id<<setiosflags(ios::left)<<setw
(10)<<p->E_name<<setiosflags(ios::left)<<setw(7)<<p->E_sex
<<setiosflags(ios::left)<<setw(15)<<p-
>E_dep<<setiosflags(ios::left)<<setw
(15)<<p->E_job<<setiosflags(ios::left)<<setw(8)<<p->E_wage<<endl; return stream;
}
///////////////////////////////////////////////////////////
// 重载输入流运算符
istream & operator>>(istream & stream,DLink p)
{
cout<<"姓名:"; //输入姓名
stream>>p->E_name;
cout<<"性别:"; //输入性别
stream>>p->E_sex;
cout<<"部门:"; //输入所在部门
stream>>p->E_dep;
cout<<"职务:"; //输入职务
stream>>p->E_job;
SetWage(p); //输入工资
cout<<endl;
return stream;
}
////////////////////////////////////////////////////////// //构造函数
employee::employee()
{ }
////////////////////////////////////////////////////////// //创立链表(申请一个头结点)
DLink employee::CreateLink()
{
DLink Head;
Head=new Node; //申请一个空间
Head->prior=NULL;
Head->next=NULL;
return Head;
}
////////////////////////////////////////////////////////// //插入结点
DLink employee::InsertNode(DLink Head)
{
DLink p;
ofstream file("EmployeeInfo.txt",ios::app); // 打开文件if(!file)
{ cout<<"Cannot open the file!\n"; return 0; }
p=new Node;
cout<<"请输入员工信息<以'00'结束>:\n";
cout<<"职工号:";
cin>>p->E_id;
while(strcmp(p->E_id,"00")) //循环输入,以"00"结束输入{
cin>>p;
p->prior=Head;
p->next=Head->next;
if(Head->next!=NULL) //如果不是空链
Head->next->prior=p;
Head->next=p;
file.write((char *)p,NUM);
p=new Node;
cout<<"请输入员工信息<以'00'结束>:\n";
cout<<"ID:";
cin>>p->E_id;。