java简易电子时钟代码
JAVA计时器(秒表)程序代码

Java计时器(秒表)功能:能实现计时,暂停,清零,记录功能。
如下图:程序运行状态:源代码:import javax.swing.*;import java.awt.*;import .*;public class Test {public static void main(String[] args){new window("计时器");}}class window extends JFrame{int ON=0,i=0,j=0,k=0,count=0,num=1,R=0;JButton button1,button2,button3,button4,button5; JTextField file1,file2,file3;JTextArea file;FlowLayout flow;String a,b,c;window(String name){file1=new JTextField(2);file2=new JTextField(2);file3=new JTextField(2);file1.setEditable(false);file2.setEditable(false);file3.setEditable(false);file=new JTextArea(10,8);file.setEditable(false);button1=new JButton("开始");button2=new JButton("暂停");button3=new JButton("清零");button4=new JButton("记录");button5=new JButton("清空记录");flow=new FlowLayout();flow.setAlignment(FlowLayout.LEFT); flow.setHgap(20);flow.setVgap(10);setTitle(name);setSize(210,400);setLayout(flow);add(file1);add(new JLabel(":"));add(file2);add(new JLabel(":"));add(file3);add(button1);add(button2);add(button3);add(button4);add(button5);add(file);setVisible(true); setDefaultCloseOperation(EXIT_ON_CLOSE); file1.setText("0");file2.setText("0");file3.setText("0");validate();button1.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { ON=1; //开始,暂停控制开关}}); //开始按钮button2.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e){ ON=0;}}); //暂停按钮button3.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e){ R=1; //清零控制开关}}); //清零按钮button4.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e){count=1; //记录控制开关a=String.valueOf(i);b=String.valueOf(j);c=String.valueOf(k);}}); //记录按钮button5.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e){file.setText("");num=1;}}); //清除记录按钮while(true){validate();if(ON==1) //开始或暂停判断{file1.setText(String.valueOf(i));file2.setText(String.valueOf(j));file3.setText(String.valueOf(k));if(k==99){k=-1;j++;}if(j==60){k=0;j=0;i++;}if(i==24){i=0;j=0;k=0;}try{Thread.sleep(10);}catch(Exception e){}k++;}if(count==1)//记录判断{file.append(String.valueOf(num));file.append(". ");file.append(a);file.append(":");file.append(b);file.append(":");file.append(c);file.append("\n");num++;count=0;}if(R==1)//清零判断{i=j=k=0;file1.setText(String.valueOf(i));file2.setText(String.valueOf(j));file3.setText(String.valueOf(k));R=0;}}}}。
java实验一:写一个桌面时钟
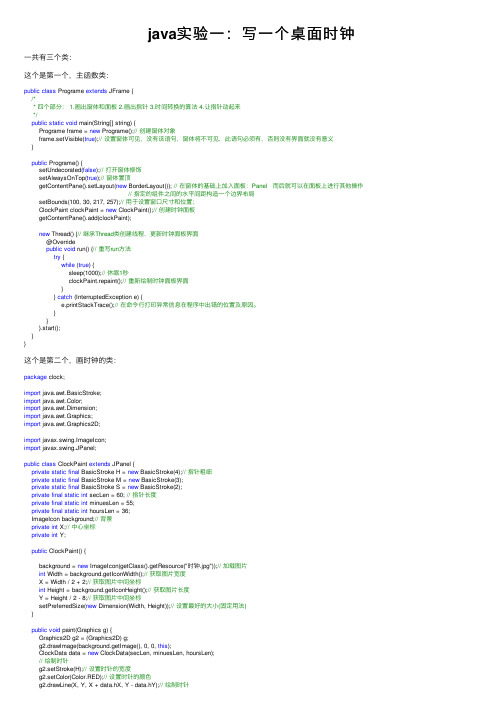
java实验⼀:写⼀个桌⾯时钟⼀共有三个类:这个是第⼀个,主函数类:public class Programe extends JFrame {/** 四个部分: 1.画出窗体和⾯板 2.画出指针 3.时间转换的算法 4.让指针动起来*/public static void main(String[] string) {Programe frame = new Programe();// 创建窗体对象frame.setVisible(true);// 设置窗体可见,没有该语句,窗体将不可见,此语句必须有,否则没有界⾯就没有意义}public Programe() {setUndecorated(false);// 打开窗体修饰setAlwaysOnTop(true);// 窗体置顶getContentPane().setLayout(new BorderLayout()); // 在窗体的基础上加⼊⾯板:Panel ⽽后就可以在⾯板上进⾏其他操作 // 指定的组件之间的⽔平间距构造⼀个边界布局setBounds(100, 30, 217, 257);// ⽤于设置窗⼝尺⼨和位置;ClockPaint clockPaint = new ClockPaint();// 创建时钟⾯板getContentPane().add(clockPaint);new Thread() {// 继承Thread类创建线程,更新时钟⾯板界⾯@Overridepublic void run() {// 重写run⽅法try {while (true) {sleep(1000);// 休眠1秒clockPaint.repaint();// 重新绘制时钟⾯板界⾯}} catch (InterruptedException e) {e.printStackTrace();// 在命令⾏打印异常信息在程序中出错的位置及原因。
}}}.start();}}这个是第⼆个,画时钟的类:package clock;import java.awt.BasicStroke;import java.awt.Color;import java.awt.Dimension;import java.awt.Graphics;import java.awt.Graphics2D;import javax.swing.ImageIcon;import javax.swing.JPanel;public class ClockPaint extends JPanel {private static final BasicStroke H = new BasicStroke(4);// 指针粗细private static final BasicStroke M = new BasicStroke(3);private static final BasicStroke S = new BasicStroke(2);private final static int secLen = 60; // 指针长度private final static int minuesLen = 55;private final static int hoursLen = 36;ImageIcon background;// 背景private int X;// 中⼼坐标private int Y;public ClockPaint() {background = new ImageIcon(getClass().getResource("时钟.jpg"));// 加载图⽚int Width = background.getIconWidth();// 获取图⽚宽度X = Width / 2 + 2;// 获取图⽚中间坐标int Height = background.getIconHeight();// 获取图⽚长度Y = Height / 2 - 8;// 获取图⽚中间坐标setPreferredSize(new Dimension(Width, Height));// 设置最好的⼤⼩(固定⽤法)}public void paint(Graphics g) {Graphics2D g2 = (Graphics2D) g;g2.drawImage(background.getImage(), 0, 0, this);ClockData data = new ClockData(secLen, minuesLen, hoursLen);// 绘制时针g2.setStroke(H);// 设置时针的宽度g2.setColor(Color.RED);// 设置时针的颜⾊g2.drawLine(X, Y, X + data.hX, Y - data.hY);// 绘制时针// 绘制分针g2.setStroke(M);// 设置分针的宽度g2.setColor(Color.orange);// 设置时针的颜⾊g2.drawLine(X, Y, X + data.mX, Y - data.mY);// 绘制分针// 绘制秒针g2.setStroke(S);// 设置秒针的宽度g2.setColor(Color.GREEN);// 设置时针的颜⾊g2.drawLine(X, Y, X + data.sX, Y - data.sY);// 绘制秒针// 绘制中⼼圆g2.setColor(Color.BLUE);g2.fillOval(X - 5, Y - 5, 10, 10);}}这个是第三个,获取时钟的数据:package clock;import static java.util.Calendar.HOUR;import static java.util.Calendar.MINUTE;import static java.util.Calendar.SECOND;import java.util.Calendar;public class ClockData {public int sX, sY, mX, mY, hX, hY;public ClockData(int secLen, int minuesLen, int hoursLen) {Calendar calendar = Calendar.getInstance();// 获取⽇历对象int sec = calendar.get(SECOND);// 获取秒值int minutes = calendar.get(MINUTE);// 获取分值int hours = calendar.get(HOUR);// 获取时值// 计算⾓度int hAngle = hours * 360 / 12 + (minutes / 2) ;// 时针⾓度(每分钟时针偏移⾓度)int sAngle = sec * 6; // 秒针⾓度int mAngle = minutes * 6 + (sec / 10);// 分针⾓度// 计算秒针、分针、时针指向的坐标sX = (int) (secLen * Math.sin(Math.toRadians(sAngle)));// 秒针指向点的X坐标(将⾓度转换为弧度) sY = (int) (secLen * Math.cos(Math.toRadians(sAngle))); // 秒针指向点的Y坐标mX = (int) (minuesLen * Math.sin(Math.toRadians(mAngle))); // 分针指向点的X坐标mY = (int) (minuesLen * Math.cos(Math.toRadians(mAngle))); // 分针指向点的Y坐标hX = (int) (hoursLen * Math.sin(Math.toRadians(hAngle))); // 时针指向点的X坐标hY = (int) (hoursLen * Math.cos(Math.toRadians(hAngle))); // 时针指向点的Y坐标}}以上参考了其他⼤佬的代码,等我找到原地址再补上:D做了部分修改,加了部分注释,java⼩⽩还请客官您多多包含呀!。
java简易电子时钟代码
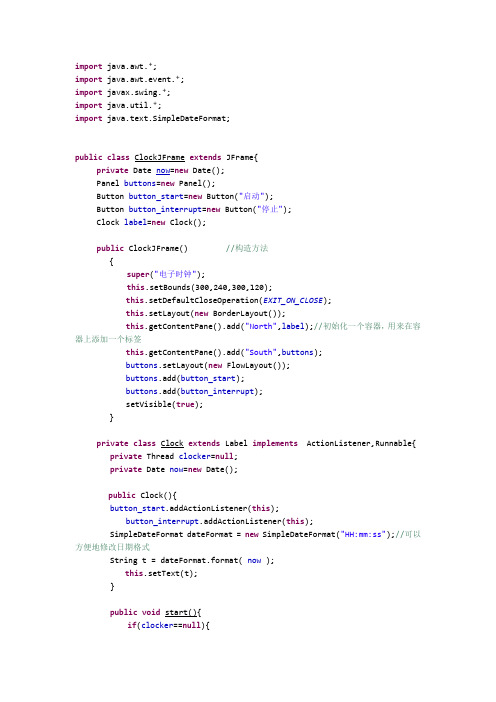
import java.awt.*;import java.awt.event.*;import javax.swing.*;import java.util.*;import java.text.SimpleDateFormat;public class ClockJFrame extends JFrame{private Date now=new Date();Panel buttons=new Panel();Button button_start=new Button("启动");Button button_interrupt=new Button("停止");Clock label=new Clock();public ClockJFrame() //构造方法{super("电子时钟");this.setBounds(300,240,300,120);this.setDefaultCloseOperation(EXIT_ON_CLOSE);this.setLayout(new BorderLayout());this.getContentPane().add("North",label);//初始化一个容器,用来在容器上添加一个标签this.getContentPane().add("South",buttons);buttons.setLayout(new FlowLayout());buttons.add(button_start);buttons.add(button_interrupt);setVisible(true);}private class Clock extends Label implements ActionListener,Runnable{ private Thread clocker=null;private Date now=new Date();public Clock(){button_start.addActionListener(this);button_interrupt.addActionListener(this);SimpleDateFormat dateFormat = new SimpleDateFormat("HH:mm:ss");//可以方便地修改日期格式String t = dateFormat.format( now );this.setText(t);}public void start(){if(clocker==null){clocker=new Thread(this);clocker.start();}}public void stop(){clocker=null;}public void run(){Thread currentThread=Thread.currentThread();while(clocker==currentThread){now=new Date();SimpleDateFormat dateFormat = newSimpleDateFormat("HH:mm:ss");//可以方便地修改日期格式String t = dateFormat.format( now );this.setText(t);try{clocker.sleep(1000);}catch(InterruptedException ie){JOptionPane.showMessageDialog(this,"Thread error:+ie");}}}public void actionPerformed(ActionEvent e){if (e.getSource()==button_start) {clocker = new Thread(this); //重新创建一个线程对象clocker.start();button_start.setEnabled(false);button_interrupt.setEnabled(true);}if (e.getSource()==button_interrupt) //单击中断按钮时{clocker.stop(); //设置当前线程对象停止标记button_start.setEnabled(true);button_interrupt.setEnabled(false);}}}//内部类结束public static void main(String[] args) {ClockJFrame time=new ClockJFrame();}}运行结果:。
JAVA数字时钟源程序代码

import java.awt.*;import java.util.*;import javax.swing.*;//数字时钟public class ClockDemo extends JFrame implements Runnable{Thread clock;public ClockDemo(){super("数字时钟"); //调用父类构造函数setFont(new Font("Times New Roman",Font.BOLD,60)); //设置时钟的显示字体start(); //开始进程setSize(280,100); //设置窗口尺寸setVisible(true); //窗口可视this.setLocation(440,330);setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //关闭窗口时退出程序}public void start(){ //开始进程if (clock==null){ //如果进程为空值clock=new Thread(this); //实例化进程clock.start(); //开始进程}}public void run(){ //运行进程while (clock!=null){repaint(); //调用paint方法重绘界面try{Thread.sleep(1000); //线程暂停一秒(1000毫秒)}catch (InterruptedException ex){ex.printStackTrace(); //输出出错信息}}}public void stop(){ //停止进程clock=null;}public void paint(Graphics g){ //重载组件的paint方法Graphics2D g2=(Graphics2D)g; //得到Graphics2D对象Calendar now=new GregorianCalendar(); //实例化日历对象String timeInfo=""; //输出信息int hour=now.get(Calendar.HOUR_OF_DAY); //得到小时数int minute=now.get(Calendar.MINUTE); //得到分数int second=now.get(Calendar.SECOND); //得到秒数if (hour<=9)timeInfo+="0"+hour+":"; //格式化输出elsetimeInfo+=hour+":";if (minute<=9)timeInfo+="0"+minute+":";elsetimeInfo+=minute+":";if (second<=9)timeInfo+="0"+second;elsetimeInfo+=second;g.setColor(Color.orange); //设置当前颜色为白色Dimension dim=getSize(); //得到窗口尺寸g.fillRect(0,0,dim.width,dim.height); //填充背景色为白色g.setColor(Color.black); //设置当前颜色为橙色g.drawString(timeInfo,20,80); //显示时间字符串}public static void main(String[] args){new ClockDemo();}}。
JAVA时钟代码

import javax.swing.*; //时钟import java.awt.event.ActionListener;import java.awt.event.ActionEvent;import java.awt.*;import java.util.Calendar;import java.util.GregorianCalendar;class Clock extends JFrame implements ActionListener{ int x,y,x0,y0,r,h,olds_x,olds_y,oldm_x,oldm_y,oldh_x,oldh_y,ss,mm,hh,old_m,old_h,ang;final double RAD=Math.PI/180; //度数转换成弧度的比例//构造函数创建了一个窗体public Clock(){ super("时钟"); //设置标题setSize(250,250); //设置窗口尺寸setBackground(Color.WHITE); //设置背景颜色setLocation(300,150); //设置窗口位置坐标setResizable(false); //使窗口可以最小化和关闭,但是不能任意改变大小setVisible(true); //设置组建可见int delay = 100; //设置延时//创建一个监听事件ActionListener drawClock = new ActionListener(){ public void actionPerformed(ActionEvent evt) { repaint(); } };new Timer(delay,drawClock).start(); //创建时间计数器,每秒触发一次}public void actionPerformed(ActionEvent e){//实现ActionListener接口必须实现的方法}//绘制图形public void paint(Graphics g){Graphics2D g2D = (Graphics2D)g;Insets insets = getInsets();int L = insets.left/2,T = insets.top/2;h = getSize().height;g.setColor(Color.black);//画圆g2D.setStroke(new BasicStroke(4.0f));g.drawOval(L+40,T+40,h-80,h-80);r=h/2-40;x0=40+r-5+L;y0=40+r-5-T;ang=60;//绘制时钟上的12个数字for(int i=1;i<=12;i++){x=(int)((r-9)*Math.cos(RAD*ang)+x0);y=(int)((r-9)*Math.sin(RAD*ang)+y0);g.drawString(""+i,x,h-y);ang-=30;}//获得当前系统时间Calendar now= new GregorianCalendar();int nowh= now.get(Calendar.HOUR_OF_DAY); int nowm= now.get(Calendar.MINUTE);int nows= now.get(Calendar.SECOND);String st;if(nowh<10) st="0"+nowh;else st=""+nowh;if(nowm<10) st+=":0"+nowm;else st+=":"+nowm; if(nows<10) st+=":0"+nows;else st+=":"+nows;//在窗体上显示时间g.setColor(Color.white);//g.fillRect(L,T,50,28);//g.setColor(Color.black);//g.drawString(st,L+2,T+26);////计算时间与度数的关系ss=90-nows*6;mm=90-nowm*6;hh=90-nowh*30-nowm/2;x0=r+40+L;y0=r+40+T;g2D.setStroke(new BasicStroke(1.0f));//秒针粗细//擦除秒针if(olds_x>0){g.setColor(getBackground());g.drawLine(x0,y0,olds_x,h-olds_y);}Else{old_m = mm;old_h = hh;}//绘制秒针x=(int)(r*0.9*Math.cos(RAD*ss))+x0;//长度y=(int)(r*0.9*Math.sin(RAD*ss))+y0-2*T;g.setColor(Color.black);//指针颜色g.drawLine(x0,y0,x,h-y);//轨迹olds_x=x;olds_y=y;g2D.setStroke(new BasicStroke(2.2f));//分针粗细//擦除分针if(old_m!=mm){g.setColor(getBackground());g.drawLine(x0,y0,oldm_x,h-oldm_y);}//绘制分针x=(int)(r*0.7*Math.cos(RAD*mm))+x0;//长度y=(int)(r*0.7*Math.sin(RAD*mm))+y0-2*T;g.setColor(Color.red);//颜色g.drawLine(x0,y0,x,h-y);oldm_x=x;oldm_y=y;old_m=mm;g2D.setStroke(new BasicStroke(3.4f));//时针粗细//擦除时针if(old_h!=hh){g.setColor(getBackground());g.drawLine(x0,y0,oldh_x,h-oldh_y);}//绘制时针x=(int)(r*0.5*Math.cos(RAD*hh))+x0;//长度y=(int)(r*0.5*Math.sin(RAD*hh))+y0-2*T;g.setColor(Color.red);//颜色g.drawLine(x0,y0,x,h-y);oldh_x=x;oldh_y=y;old_h=hh;}public static void main(String[] args){ Clock c = new Clock(); } }3Dimport java.applet.Applet;import java.awt.BorderLayout;import com.sun.j3d.utils.applet.MainFrame;import com.sun.j3d.utils.geometry.*;import com.sun.j3d.utils.universe.*;import javax.media.j3d.*;import javax.vecmath.*;import com.sun.j3d.utils.behaviors.mouse.MouseRotate;import com.sun.j3d.utils.behaviors.mouse.MouseZoom;import com.sun.j3d.utils.behaviors.mouse.MouseTranslate;public class AWTFrameJ3D{ private static final long serialVersionUID = 1L;Canvas3D cv = null;public static void main(String s[]){ AWTFrameJ3D hd = new AWTFrameJ3D();hd.constractJava3D();}/*构造方法创建Frame和Canvas3D画布对象,并将Canvas3D嵌入到Frame中*/public AWTFrameJ3D(){GraphicsConfigTemplate3D template = new GraphicsConfigTemplate3D();GraphicsEnvironment env = GraphicsEnvironment.getLocalGraphicsEnvironment();GraphicsDevice device = env.getDefaultScreenDevice();GraphicsConfiguration config = device.getBestConfiguration(template);// 新建Canvas3D对象,Canvas3D对象是一个用于显示虚拟世界场景的绘制结果的画布cv = new Canvas3D(config);// 新建Frame对象Frame dframe = new Frame(config);dframe.setTitle("模型读取中");dframe.setLayout(new BorderLayout());// 将Canvas3D对象嵌入到Frame对象中dframe.add(cv, BorderLayout.CENTER);dframe.setSize(500, 400);// 添加窗口监听器实现关闭窗口(Frame),关闭窗口时退出程序dframe.addWindowListener(new WindowAdapter(){ public void windowClosing(WindowEvent ev){ System.out.print("退出程序!");System.exit(0);} }};// 使用Toolkit更改Java应用程序标题栏默认图标Toolkit tk = Toolkit.getDefaultToolkit();Image image = tk.createImage("images/earth.jpg"); /* image.gif是你的图标 */ dframe.setIconImage(image);// 使用Toolkit把默认的鼠标图标改成指定的图标:// Toolkit tk=Toolkit.getDefaultToolkit();Image img = tk.getImage("images/earth.jpg"); /* mouse.gif是你的图标 */Cursor cu = tk.createCustomCursor(img, new Point(10, 10), "stick");dframe.setCursor(cu);// 现将Frame窗口可视化之后,再绘制3D场景内容dframe.setVisible(true);}/**构建3D虚拟世界场景*/public void constractJava3D(){ // 创建场景图分支BranchGroup bg = createSceneGraph();pile();// 将观察分支关联到一个Canvas3D对象,以显示视图的绘制结果SimpleUniverse su = new SimpleUniverse(cv);su.getViewingPlatform().setNominalViewingTransform();// 把场景图关联到SimpleUniverse对象之后,整个场景就开始绘制了su.addBranchGraph(bg);// 现绘制3D场景内容,再将Frame窗口可视化// dframe.setVisible(true);}/**创建3D场景内容*/private BranchGroup createSceneGraph(){ // 创建BranchGroup对象作为根节点BranchGroup root = new BranchGroup();// objectAppearance ap = new Appearance();ap.setMaterial(new Material());Font3D font = new Font3D(new Font("SansSerif", Font.PLAIN, 1),new FontExtrusion());Text3D text = new Text3D(font, "Hello 3D");Shape3D shape = new Shape3D(text, ap);// transformationTransform3D tr = new Transform3D();tr.setScale(0.5);tr.setTranslation(new Vector3f(-0.95f, -0.2f, 0f));TransformGroup tg = new TransformGroup(tr);root.addChild(tg);tg.addChild(shape);// lightPointLight light = new PointLight(new Color3f(Color.white), new Point3f(1f, 1f, 1f), new Point3f(1f, 0.1f, 0f));BoundingSphere bounds = new BoundingSphere();light.setInfluencingBounds(bounds);root.addChild(light);return root;}}。
Java模拟时钟制作案例
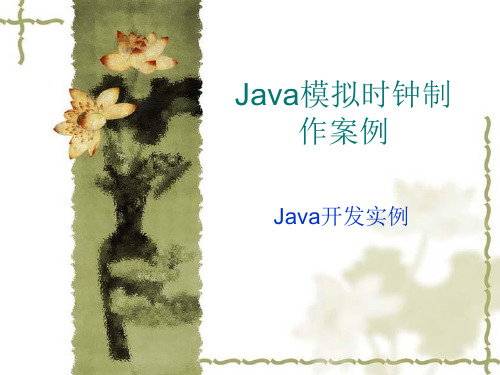
美化指针
调用AffineTransform
public AffineTransform sc =new AffineTransform(); sc.setToRotation(Math.PI/30f*(s1-15),125,125); g2.fill(tick_s.createTransformedShape(sc));
程序代码
主类
程序代码
程序代码
运行效果
运行效果
面板刻度
绘制刻度
l1=new Line2D.Double[60]; for(int i=0 ;i<l1.length;i++) { double b[]=new double[4]; int j; if(i%5==0){ if(i%15==0){ j=50; }else { j=60; }
运行效果程序代码程序代码程序代码程序代码程序代码代码优化与改进以上的代码已经能基本完成时钟的功能
Java模拟时钟制 作案例
Java开发实例
需求分析
模拟时钟,把它放在程序中可以给人一种清 新的感觉。比起数字时钟来说,有一定的真 实感怀旧情结。看到秒针一下一下的转动, 时时提醒,时间在不断流逝,要我们珍惜时 间。 子在川上曰:逝者如斯夫。
坐标的转换,使用方法
调用方法:
p0=xy(125,125,75,s1*6); s.x1=p0.getX(); s.y1=p0.getY(); p0=xy(125,125,65,m1*6); m.x1=p0.getX(); m.y1=p0.getY(); p0=xy(125,125,55,(h1*30+m1/2f)); h.x1=p0.getX(); h.y1=p0.getX();
数字时钟代码

数字时钟代码1. 介绍数字时钟是一种常见的显示时间的装置,它通过数字显示屏显示当前的小时和分钟。
本文档将介绍如何编写一个简单的数字时钟代码。
2. 代码实现以下是一个基本的数字时钟代码实现示例:import timewhile True:current_time = time.localtime()hour = str(current_time.tm_hour).zfill(2)minute = str(current_time.tm_min).zfill(2)second = str(current_time.tm_sec).zfill(2)clock_display = f"{hour}:{minute}:{second}"print(clock_display, end="\r")time.sleep(1)代码说明:- `time.localtime()` 函数返回当前时间的结构化对象,包括小时、分钟和秒等信息。
- `str(current_time.tm_hour).zfill(2)` 将小时转换为字符串,并使用 `zfill()` 方法填充到两位数。
- `str(current_time.tm_min).zfill(2)` 和`str(current_time.tm_sec).zfill(2)` 同理处理分钟和秒。
- 使用 f-string 格式化字符串 `clock_display`,将小时、分钟和秒显示为 `` 的形式。
- `print(clock_display, end="\r")` 使用 `\r` 实现覆盖打印,使得时钟在同一行连续显示。
- `time.sleep(1)` 让程序每隔一秒更新一次时间。
请注意,上述代码需要在支持 Python 的环境中运行。
3. 结束语通过以上的代码实现,我们可以编写一个简单的数字时钟。
JAVA可视化闹钟源码
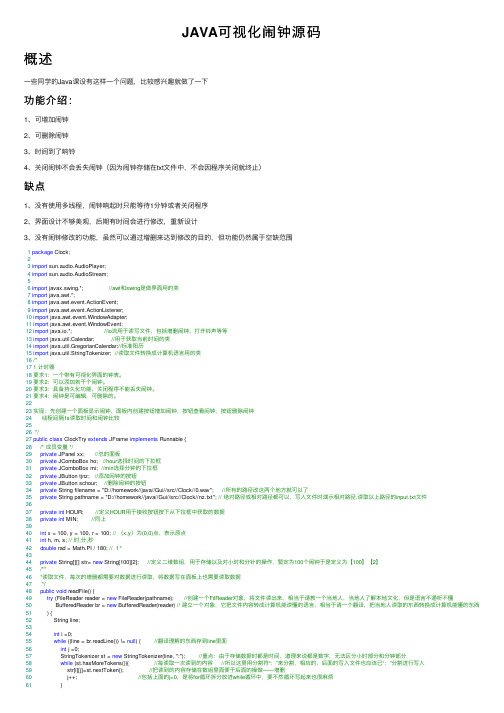
JAVA可视化闹钟源码概述⼀些同学的Java课设有这样⼀个问题,⽐较感兴趣就做了⼀下功能介绍:1、可增加闹钟2、可删除闹钟3、时间到了响铃4、关闭闹钟不会丢失闹钟(因为闹钟存储在txt⽂件中,不会因程序关闭就终⽌)缺点1、没有使⽤多线程,闹钟响起时只能等待1分钟或者关闭程序2、界⾯设计不够美观,后期有时间会进⾏修改,重新设计3、没有闹钟修改的功能,虽然可以通过增删来达到修改的⽬的,但功能仍然属于空缺范围1package Clock;23import sun.audio.AudioPlayer;4import sun.audio.AudioStream;56import javax.swing.*; //awt和swing是做界⾯⽤的类7import java.awt.*;8import java.awt.event.ActionEvent;9import java.awt.event.ActionListener;10import java.awt.event.WindowAdapter;11import java.awt.event.WindowEvent;12import java.io.*; //io流⽤于读写⽂件,包括增删闹钟、打开铃声等等13import java.util.Calendar; //⽤于获取当前时间的类14import java.util.GregorianCalendar;//标准阳历15import java.util.StringTokenizer; //读取⽂件转换成计算机语⾔⽤的类16/*171 计时器18要求1:⼀个带有可视化界⾯的钟表。
19要求2:可以添加若⼲个闹钟。
20要求3:具备持久化功能,关闭程序不能丢失闹钟。
21要求4:闹钟是可编辑,可删除的。
2223实现:先创建⼀个⾯板显⽰闹钟,⾯板内创建按钮增加闹钟,按钮查看闹钟,按钮删除闹钟24线程间隔1s读取时间和闹钟⽐较2526*/27public class ClockTry extends JFrame implements Runnable {28/* 成员变量 */29private JPanel xx; //总的⾯板30private JComboBox ho; //hour选择时间的下拉框31private JComboBox mi; //min选择分钟的下拉框32private JButton tjnz; //添加闹钟的按钮33private JButton schour; //删除闹钟的按钮34private String filename = "D://homework//java//Gui//src//Clock//0.wav"; //所有的路径改这两个地⽅就可以了35private String pathname = "D://homework//java//Gui//src//Clock//nz.txt"; // 绝对路径或相对路径都可以,写⼊⽂件时演⽰相对路径,读取以上路径的input.txt⽂件3637private int HOUR; //定义HOUR⽤于接收按钮按下从下拉框中获取的数据38private int MIN; //同上3940int x = 100, y = 100, r = 100; // (x,y)为(0,0)点,表⽰原点41int h, m, s; // 时,分,秒42double rad = Math.PI / 180; // 1°4344private String[][] str= new String[100][2]; //定义⼆维数组,⽤于存储以及对⼩时和分针的操作,暂定为100个闹钟于是定义为【100】【2】45/**46 *读取⽂件,每次的增删都需要对数据进⾏读取,将数据写在⾯板上也需要读取数据47*/48public void readFile() {49try (FileReader reader = new FileReader(pathname); //创建⼀个FilReader对象,将⽂件读出来,相当于请教⼀个当地⼈,当地⼈了解本地⽂化,但是语⾔不通听不懂50 BufferedReader br = new BufferedReader(reader) // 建⽴⼀个对象,它把⽂件内容转成计算机能读懂的语⾔,相当于请⼀个翻译,把当地⼈读取的东西转换成计算机能懂的东西51 ) {52 String line;5354int i =0;55while ((line = br.readLine()) != null) { //翻译理解的东西存到line⾥⾯56int j =0;57 StringTokenizer st = new StringTokenizer(line, ":"); //重点:由于存储数据时都是时间,道理来说都是数字,⽆法区分⼩时部分和分钟部分58while (st.hasMoreTokens()){ //每读取⼀次读到的内容 //所以这⾥⽤分割符“:”来分割,相应的,后⾯的写⼊⽂件也应该已“:”分割进⾏写⼊59 str[i][j]=st.nextToken(); //把读到的内容存储在数组⾥⾯便于后⾯的操做——增删60 j++; //包括上⾯的j=0,是将for循环拆分放进while循环中,要不然循环写起来也很⿇烦61 }62//System.out.print(str[i][0]+":"+str[i][1]); 写的时候⽤来在控制台打印查看效果63//System.out.println();64 i++;65 j = 0;66 }67 } catch (IOException e) {68 e.printStackTrace(); //try……catch抛出异常69 }70 }717273/**74 * 写⼊TXT⽂件75*/76public void writeFile() {77 HOUR = Integer.valueOf(ho.getSelectedIndex()); //获取下拉框中的值,存储到HOUR中78 MIN = Integer.valueOf(mi.getSelectedIndex());79 String x = HOUR + ":" + MIN;80try (FileWriter writer = new FileWriter(pathname,true); //同上⾯的读取,本地⼈写⼊,注意:后⾯的append:true是表⽰不是重新写,⽽是在后⾯追加81 BufferedWriter out = new BufferedWriter(writer) //翻译⼀下再写⼊82 ) {8384 out.write(HOUR + ":" + MIN + "\r\n"); //这⾥写⼊的时候把:写进去了!85 out.flush(); // 把缓存区内容压⼊⽂件,计算机的存储过程,存在缓存区再写⼊⽂件86 JOptionPane.showMessageDialog(null,"闹钟添加成功!","添加闹钟提醒",RMATION_MESSAGE); //提⽰框:添加闹钟成功87 } catch (IOException e) {88 e.printStackTrace();8990 }9192 }939495/**96 * 删除闹钟,实际上是先将要删除的数据找到移除数组,再将数组重新写⼊,所以要先读取⽂件,再重新写⼊97*/98public void shanchuFile() {99 HOUR = Integer.valueOf(ho.getSelectedIndex());100 MIN = Integer.valueOf(mi.getSelectedIndex());101try (FileWriter writer = new FileWriter(pathname); //没有append:true,表⽰重新写!102 BufferedWriter out = new BufferedWriter(writer)103 ) {104 readFile();105for (int i = 0; i < 100; i++) {106if (Integer.valueOf(str[i][0])==HOUR && Integer.valueOf(str[i][1])==MIN){107continue;108 }109else{110 out.write(str[i][0]+":"+str[i][1]+"\r\n"); // \r\n即为换⾏111 }112 }113114//out.write("1"+"1"+"\r\n"); // \r\n即为换⾏115 out.flush(); // 把缓存区内容压⼊⽂件116 } catch (IOException e) {117 e.printStackTrace();118 }catch (NumberFormatException e){119 System.out.println("this isn't exist!");120 JOptionPane.showMessageDialog(null,"该闹钟已删除!","删除闹钟提醒",RMATION_MESSAGE); //弹窗提⽰121 }122 }123124/* 初始化函数 */125public void init() {126127 Calendar now = new GregorianCalendar(); //获取当前时间128/*129 * GregorianCalendar(标准阳历)130 * 是Calendar(⽇历)【国际环境下都能运⾏的程序】131 * 的⼦类132*/133 s = now.get(Calendar.SECOND) * 6; // 秒针转换成⾓度:1秒,秒针动⼀次,转动6°134 m = now.get(Calendar.MINUTE) * 6; // 分针转换为⾓度:1分,分针动⼀次,转动6°135 h = now.get(Calendar.HOUR) * 30 + now.get(Calendar.MINUTE) / 12 * 6; // 先把分化为⼩时,再乘以6°,因为分针转12°,时针才会转1°,⼀⼩时中间有5格,数学问题136/*137 * Calendar.HOUR 显⽰范围:1-12(⽆论AM还是PM) Calendar.HOUR_OF_DAY 显⽰范围:1-24(包括PM138*/139140 Thread t = new Thread(this); //添加线程,线程⽬标是整个程序,this141 t.start(); //线程就绪142 }143144public void paint(Graphics g) { //awt中的⽅法,因为要时时显⽰闹钟,所以不得不使⽤绘画的⽅式,不断重绘145super.paint(g);146/*147 * paint(g)函数会重绘图像,要加上super.paint(g),表⽰在原来图像的基础上,再画图。
基于JavaScript的电子时钟效果实现

基于JavaScript的电子时钟效果实现在JavaScript中实现电子时钟效果可以借助HTML和CSS来完成。
主要的实现思路是使用JavaScript来获取当前的时间,并将其动态地更新到HTML元素中。
下面是一个基本的电子时钟效果实现的示例代码:```html<!DOCTYPE html><html><head><meta charset="UTF-8"><title>电子时钟</title><style>#clock {font-size: 48px;text-align: center;margin-top: 200px;}</style></head><body><div id="clock"></div><script>function updateClock() {var now = new Date();var hours = now.getHours();var minutes = now.getMinutes();var seconds = now.getSeconds();// 格式化时间hours = hours < 10 ? "0" + hours : hours;minutes = minutes < 10 ? "0" + minutes : minutes;seconds = seconds < 10 ? "0" + seconds : seconds;// 更新HTML元素var clockDiv = document.getElementById("clock");clockDiv.textContent = hours + ":" + minutes + ":" + seconds; }// 每秒更新一次时间setInterval(updateClock, 1000);</script></body></html>```在这个例子中,通过`new Date()`获取当前的时间,并使用`getHours()`、`getMinutes()`和`getSeconds()`方法来获取小时、分钟和秒数。
时钟java

win.validate();
win.addWindowListener(new WindowAdapter()
{ public void windowClosing(WindowEvent e)
{ System.exit(0);
}
});
}
}
pointMX[i+1]=pointMX[i]*Math.cos(angle)-Math.sin(angle)*pointMY[i];
pointMY[i+1]=pointMY[i]*Math.cos(angle)+pointMX[i]*Math.sin(angle);
for(int i=0;i<59;i++)
{ pointSX[i+1]=pointSX[i]*Math.cos(angle)-Math.sin(angle)*pointSY[i];
pointSY[i+1]=pointSY[i]*Math.cos(angle)+pointSX[i]*Math.sin(angle);
g.fillOval(m-2,n-2,4,4);
}
}
g.fillOval(115,115,10,10);
Graphics2D g_2d=(Graphics2D)g;
g_2d.setColor(Color.red);
g_2d.draw(secondLine);
bs=new BasicStroke(6f,BasicStroke.CAP_ROUND,BasicStroke.JOIN_MITER);
g_2d.setStroke(bs);
java简易流动字幕代码(用电子时钟控制)

import java.awt.*;import java.awt.event.*;import java.text.SimpleDateFormat;import java.util.Date;import javax.swing.*;public class Ticker_Tape extends JFrame {private Date now=new Date();private Panel buttons=new Panel();private Button button_start=new Button("启动");private Button button_interrupt=new Button("停止");private Clock time=new Clock();private Label word=new Label("Welcom");char space[]=new char[75];public Ticker_Tape(){super("滚动字");this.setBounds(300,240,300,200);this.setDefaultCloseOperation(EXIT_ON_CLOSE);this.setLayout(new GridLayout(3,1));this.add(word);this.add(time);this.add(buttons);buttons.setLayout(new FlowLayout());buttons.add(button_start);buttons.add(button_interrupt);java.util.Arrays.fill(space, ' '); //将字符数组space填充满空格word.setText(new String(space)+word.getText()); //text前加空格字符串 setVisible(true);}private class Clock extends Label implements ActionListener, Runnable{ private Thread clocker=null;private Date now=new Date();public Clock(){b utton_start.addActionListener(this);button_interrupt.addActionListener(this);S impleDateFormat dateFormat = new SimpleDateFormat("HH:mm:ss");//可以方便地修改日期格式S tring t = dateFormat.format(now );t his.setText(t);}public void start(){if(clocker==null){clocker=new Thread(this);clocker.start();}}public void stop(){clocker=null;}public void run(){Thread currentThread=Thread.currentThread();while(clocker==currentThread){now=new Date();SimpleDateFormat dateFormat = new SimpleDateFormat("HH:mm:ss");//可以方便地修改日期格式String t = dateFormat.format( now );this.setText(t);try{String str =word.getText();str = str.substring(1)+ str.substring(0,1);word.setText(str);clocker.sleep(1000);}catch(InterruptedException ie){JOptionPane.showMessageDialog(this,"Thread error:+ie");}}}public void actionPerformed(ActionEvent e){if (e.getSource()==button_start) {clocker = new Thread(this); //重新创建一个线程对象clocker.start();button_start.setEnabled(false);button_interrupt.setEnabled(true);}if (e.getSource()==button_interrupt) //单击中断按钮时{clocker.stop(); //设置当前线程对象停止标记button_start.setEnabled(true);button_interrupt.setEnabled(false);}}}//内部类结束public static void main(String[] args) { Ticker_Tape time=new Ticker_Tape(); }}运行结果:。
电子时钟设计代码

#include<reg51.h>#define uint unsigned int //宏定义,将unsigned int 用uint代替,下同#define uchar unsigned charsbit S1=P1^0;sbit S2=P1^1;sbit S3=P1^2;sbit S4=P1^3;char second;char minute;char hour;uchar T_time;//计数变量int a=0;uchar tab[]={0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,0x07,0x7f,0x6f};//0~9 void delay(uint k){uint i;for(i=0;i<k;i++);}void keyscan();void Displaysecond(uchar);void Displayminute(uchar);void Displayhour(uchar);void main(){TMOD=0x01; //定时器/计数器T0的工作方式1EA=1; //总中断允许ET0=1; //计时器T0中断允许位TR0=1; //开始计数TH0=(65536-46083)/256;TL0=(65536-46083)%256;minute=0;second=0;hour=0;T_time=0;while(1){keyscan();Displaysecond(second);delay(100);Displayminute(minute);delay(100);Displayhour(hour);delay(100);}}void Displaysecond(uchar s){P2=0xbf; //"秒十位"位选端P0=tab[s/10]; //刚开始显示数字0 delay(100); //延时一段时间P0=0x00; //消隐P2=0x7f; //"秒个位"位选端P0=tab[s%10]; //显示段码delay(100); //延时一段时间P0=0x00; //消隐}void Displayminute(uchar m){P2=0xf7; //"分十位"位选端P0=tab[m/10]; //刚开始显示数字0 delay(100); //延时一段时间P0=0x00; //消隐P2=0xef; //"分个位"位选端P0=tab[m%10]; //显示段码delay(100); //延时一段时间P0=0x00; //消隐P2=0xdf; //分隔符位选端P0=0x40; //显示分隔符delay(100); //延时一段时间P0=0x00; //消隐}void Displayhour(uchar h){P2=0xfe; //"小时十位位选端" P0=tab[h/10]; //显示段码1delay(100);P0=0x00; //消隐P2=0xfd; //小时个位位选端P0=tab[(h)%10]; //显示段码2 delay(100);P0=0x00;//消隐P2=0xfb;P0=0x40;delay(100);P0=0x00;//消隐}void keyscan(){if(S1==0) //S1的功能是秒加1{delay(100);if(S1==0){if(a==0){second++;if(second>=60){minute++;if(minute>=60){minute=0;hour++;if(hour>=24){hour=0;}}second=0;}}if(a==1){minute++;if(minute>=60){hour++;if(hour>=24)hour=0;minute=0;}}if(a==2){hour++;if(hour>=24)hour=0;}while(!S1){Displaysecond(second);delay(100);Displayminute(minute);delay(100);Displayhour(hour);delay(100);}}}if(S2==0) //S2的功能是秒减1 {delay(100);if(S2==0){if(a==0){second--;if(second<=-1){minute--;if(minute<=-1){hour--;if(hour<=-1)hour=23;minute=59;}second=59;}}if(a==1){minute--;if(minute<=-1){minute=59;hour--;if(hour<=-1)hour=23;}}if(a==2){hour--;if(hour<=-1)hour=23;}while(!S2){Displaysecond(second);delay(100);Displayminute(minute);delay(100);Displayhour(hour);delay(100);}}}if(S3==0) //S3的功能是清零{delay(100);if(S3==0){a++;if(a==3)a=0;while(!S3){Displaysecond(second);delay(100);Displayminute(minute);delay(100);Displayhour(hour);delay(100);}}}if(S4==0) //S4的功能是启动或停止计数{while(!S4){Displaysecond(second);delay(100);Displayminute(minute);delay(100);Displayhour(hour);delay(100);}TR0=~TR0;}}void Time0() interrupt 1{T_time++;if(T_time==20){T_time=0;second++;}if(second==60){second=0;minute++;}if(minute==60){minute=0;hour++;}if(hour==24){hour=0;}TH0=(65536-50000)/256;TL0=(65536-50000)%256;}。
java小时钟程序

import java.awt.Color; //提供构建和管理图形用户界面功能的包,颜色类import java.awt.Graphics; //图形类import java.awt.event.WindowAdapter;//AWTEvent类,窗口事件适配器import java.awt.event.WindowEvent;//窗口事件类import java.util.Calendar;//提供常用工具类,日历类import java.util.GregorianCalendar;//Calendar的一个子类,使用GregorianCalendar对象的get 方法(参数)获取时、分、秒import javax.swing.JFrame;//java.awt.Frame 的扩展版本,该版本添加了对Swing 组件架构的支持,顶层容器,x:是extension的意思,扩展import javax.swing.JPanel;//中间容器,作为容器组件添加到JFrame容器中,层次化管理图形用户界面的各个组件// 定制系统Clock类,继承父类JFramepublic class Clock extends JFrame {//无参数构造器public Clock() {ClockPaint cp = new ClockPaint(20, 20, 70);// 表盘this.add(cp);//用add方法添加cpthis.setSize(200, 200); //设置框架大小this.setResizable(false);//设置框架大小,不能改变this.setLocation(260, 120);//设置组件左上角的位置this.setTitle("小时钟"); //设置框架的标题this.setVisible(true); //设置组件可见// WindowListener接口的方法addWindowListener(new WindowAdapter() { //注册监听器WindowAdapter//窗口正在被关闭时调用的方法public void windowClosing(WindowEvent e) {System.exit(0);// 退出程序}});}//main():程序入口public static void main(String[] s) {new Clock();}}// 表盘类,继承父类JPanel,并实现Runnable接口,用于多线程class ClockPaint extends JPanel implements Runnable {int x, y, r;int h, m, s; // 小时,分钟,秒double rad = Math.PI / 180; //PI=π,为180°,Math.PI/180就为1°// 构造器,初始化public ClockPaint(int x, int y, int r) {this.x = x; //坐标x=20this.y = y; //坐标y=20this.r = r; //表盘的半径=70//new它的子类GregorianCalendar,对抽象类Calendar进行实例化Calendar now = new GregorianCalendar();s = now.get(Calendar.SECOND) * 6; // 获取当前系统秒,转换成度数m = now.get(Calendar.MINUTE) * 6; // 获取当前系统分,转化成度数h = (now.get(Calendar.HOUR_OF_DAY) - 12) * 30+ now.get(Calendar.MINUTE) / 12 * 6;// 获得当前系统小时Thread t = new Thread(this); //创建一个线程t.start(); //用start方法启动这个线程,表开始走动}//绘制图形,画表盘public void paint(Graphics g) {// 清屏super.paint(g); //调用父类JPanel的paint方法g.setColor(Color.BLACK); //设置面板颜色为黑色g.fillRect(0, 0, r * 3, r * 3); //填充一个矩形区域,0,0为起始坐标,后两个数是宽和高//画圆g.setColor(Color.WHITE); //白色g.drawOval(x, y, r * 2, r * 2); //画出表盘轮廓// 用红色在点(x+r,y+r)和(x+r+x1,y+r-y1)之间画秒针g.setColor(Color.RED);int x1 = (int) ((r - 10) * Math.sin(rad * s));int y1 = (int) ((r - 10) * Math.cos(rad * s));g.drawLine(x + r, y + r, x + r + x1, y + r - y1);// 用蓝色在点(x+r,y+r)和(x+r+x1,y+r-y1)之间画分针g.setColor(Color.BLUE);x1 = (int) ((r - r / 2.5) * Math.sin(rad * m));y1 = (int) ((r - r / 2.5) * Math.cos(rad * m));g.drawLine(x + r, y + r, x + r + x1, y + r - y1);// 用青色在点(x+r,y+r)和(x+r+x1,y+r-y1)之间画时针g.setColor(Color.CYAN);x1 = (int) ((r - r / 1.5) * Math.sin(rad * h));y1 = (int) ((r - r / 1.5) * Math.cos(rad * h));g.drawLine(x + r, y + r, x + r + x1, y + r - y1);// 用黄色画表盘上的12个数字g.setColor(Color.YELLOW);int d = 29;for (int i = 1; i <= 12; i++) {x1 = (int) ((r - 10) * Math.sin(rad * d));y1 = (int) ((r - 10) * Math.cos(rad * d));g.drawString(i + "", x + r + x1 - 4, x + r - y1 + 5);d += 30;}// 用60个小点,表示刻度d = 0;for (int i = 0; i < 60; i++) {x1 = (int) ((r - 2) * Math.sin(rad * d));y1 = (int) ((r - 2) * Math.cos(rad * d));g.drawString(".", x + r + x1 - 1, x + r - y1 + 1);d += 6;}// 显示数字时间Calendar now1 = new GregorianCalendar();g.drawString(now1.get(Calendar.HOUR_OF_DAY) + ":"+ now1.get(Calendar.MINUTE) + ":" + now1.get(Calendar.SECOND),0, 10);}//实现Runnable的run()方法public void run() {while (true) {try {//每间隔一秒运行一次Thread.sleep(1000);} catch (Exception ex) {}//秒针每次走6度s += 6;//秒针走一圈后,s归零if (s >= 360) {s = 0;m += 6;//分针每走72度,时针走6度if (m == 72 || m == 144 || m == 216 || m == 288) {h += 6;}//分针走一圈后,m归零if (m >= 360) {m = 0;h += 6;}//时针走一圈后,h归零if (h >= 360) {h = 0;}}//重新绘制表盘this.repaint();}}}。
电子时钟程序代码

#include<reg52.h>#define uchar unsigned char#define uint unsigned intuchar code weixuan[8]={0x80,0x40,0x20,0x10,0x08,0x04,0x02,0x01}; //位选,控制哪个数码管亮。
(从右到左)ucharcodeduanxuan[12]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90,0xbf, 0xff}; //0-9,'-','灭'uchar data timedata[3]={0x00,0x00,0x00}; //时间缓冲区,分别为:秒、时、分。
uchar data datetime[8]={0xc0,0xc0,0xbf,0xc0,0xc0,0xbf,0xc0,0xc0}; //时间缓冲区,初始化显示00-00-00。
uchar tt1,tt2,tt,tt0,num=0;uchar flag,flag1,flag2,flash;sbit key1=P1^3;sbit key2=P1^5;sbit key3=P1^7;//**************延时函数*********************************void delay(uint del){uchar i, j;for(i=0; i<del; i++)for(j=0; j<=148; j++);}//********************调用显示************************void display(){datetime[0]=timedata[0]%10; datetime[1]=timedata[0]/10; //秒datetime[3]=timedata[1]%10; datetime[4]=timedata[1]/10; //分 datetime[6]=timedata[2]%10; datetime[7]=timedata[2]/10; //时 if(!flag){P2=0X80; //秒显示P0=duanxuan[datetime[0]];delay(2);P2=0X40;P0=duanxuan[datetime[1]];delay(2);}else{P2=0X80; //秒显示P0=duanxuan[datetime[0]]|flash;delay(2);P2=0X40;P0=duanxuan[datetime[1]]|flash;delay(2);}P2=0X20; //显示'-'P0=duanxuan[10];delay(2);if(!flag1){P2=0X10; //分显示P0=duanxuan[datetime[3]];delay(2);P2=0X08;P0=duanxuan[datetime[4]];delay(2);}else{P2=0X10; //分显示P0=duanxuan[datetime[3]]|flash;delay(2);P2=0X08;P0=duanxuan[datetime[4]]|flash;delay(2);}P2=0X04; //显示'-' P0=duanxuan[10];delay(2);if(!flag2){P2=0X02; //小时P0=duanxuan[datetime[6]];delay(2);P2=0X01;P0=duanxuan[datetime[7]];delay(2);}else{P2=0X02; //小时P0=duanxuan[datetime[6]]|flash;delay(2);P2=0X01;P0=duanxuan[datetime[7]]|flash;delay(2);}}/******************按键调节时间*****************************/ void keyscan(){if(key1==0){delay(10);if(key1==0){num++;while(!key1);while(1){if(num==1){flag=1;flag1=0;flag2=0;if(key2==0){delay(10);if(key2==0){timedata[0]--;if(timedata[0]==-1)timedata[0]=60;while(!key2);}}if(key3==0){delay(10);if(key3==0){timedata[0]++;if(timedata[0]==60)timedata[0]=0;while(!key3);}}}if(key1==0){delay(10);if(key1==0)num++;while(!key1);}if(num==2){flag=0;flag1=1;flag2=0;if(key2==0){delay(10);if(key2==0){timedata[1]--;if(timedata[1]==-1)timedata[1]=60;while(!key2);}}if(key3==0){delay(10);if(key3==0){timedata[1]++;if(timedata[1]==60)timedata[1]=0;while(!key3);}}if(num==3){flag=0;flag1=0;flag2=1;if(key2==0){delay(10);if(key2==0){timedata[2]--;if(timedata[2]==-1)timedata[2]=24;while(!key2);}}if(key3==0){delay(10);if(key3==0){timedata[2]++;if(timedata[2]==25)timedata[2]=0;while(!key3);}}if(num==4){num=0;flag=0;flag1=0;flag2=0;break;}}}}}//主函数void main(){TMOD=0x01;ET0=1;TR0=1;TH0=0x40;TL0=0x00;EA=1;while(1){keyscan();}}//***************定时器函数*************************** void timer1() interrupt 1{TH0=0x40; //50ms自加一次。
JavaFX实现简易时钟效果(一)

JavaFX实现简易时钟效果(⼀)本⽂实例为⼤家分享了JavaFX实现简易时钟效果的具体代码,供⼤家参考,具体内容如下效果图⽤当前时间创建时钟,绘制表盘。
钟表是静⽌的。
让指针动起来,请参照:主函数⽂件 ShowClock:package primier;import javafx.application.Application;import javafx.geometry.Insets;import javafx.geometry.Pos;import javafx.scene.Scene;import javafx.stage.Stage;import javafx.scene.paint.Color;import yout.*;import javafx.scene.control.*;import javafx.scene.image.Image;import javafx.scene.image.ImageView;import javafx.scene.shape.Line;public class ShowClock extends Application {@Override //Override the start method in the Application classpublic void start(Stage primaryStage) {// 创建时钟⾯板ClockPane clock = new ClockPane();// 当前时间整理为字符串String timeString = clock.getHour() + ":" + clock.getMinute()+ ":" + clock.getSecond();Label lbCurrentTime = new Label(timeString);BorderPane pane = new BorderPane();pane.setCenter(clock);pane.setBottom(lbCurrentTime);// 将时钟字符串设为靠上居中BorderPane.setAlignment(lbCurrentTime, Pos.TOP_CENTER);Scene scene = new Scene(pane, 250,250);primaryStage.setTitle("Display Clock");primaryStage.setScene(scene);primaryStage.show();}public static void main (String[] args) {unch(args);}}ClockPane 类package primier;import java.util.Calendar;import java.util.GregorianCalendar;import yout.Pane;import javafx.scene.paint.Color;import javafx.scene.shape.Circle;import javafx.scene.shape.Line;import javafx.scene.text.Text;public class ClockPane extends Pane {private int hour;private int minute;private int second;// 时钟⾯板的宽度和⾼度private double w = 250, h = 250;/** ⽤当前时间创建时钟 */public ClockPane() {setCurrentTime();}/** Return hour */public int getHour() { return hour; }/** Return minute */public int getMinute() { return minute; }/** Return second */public int getSecond() { return second; }/** Set the current time for the clock */public void setCurrentTime() {// ⽤当前时间创建Calendar类Calendar calendar = new GregorianCalendar();this.hour = calendar.get(Calendar.HOUR_OF_DAY);this.minute = calendar.get(Calendar.MINUTE);this.second = calendar.get(Calendar.SECOND);paintClock();}/** 绘制时钟 */protected void paintClock() {double clockRadius = Math.min(w,h)*0.4; // 时钟半径// 时钟中⼼x, y坐标double centerX = w/2;double centerY = h/2;// 绘制钟表Circle circle = new Circle(centerX, centerY, clockRadius);circle.setFill(Color.WHITE); // 填充颜⾊circle.setStroke(Color.BLACK); // 笔画颜⾊Text t1 = new Text(centerX-5, centerY-clockRadius+12,"12");Text t2 = new Text(centerX-clockRadius+3, centerY +5, "9");Text t3 = new Text(centerX+clockRadius-10, centerY+3, "3");Text t4 = new Text(centerX-3, centerY+clockRadius-3,"6");// 秒针double sLength = clockRadius * 0.8;double secondX = centerX + sLength * Math.sin(second * (2 * Math.PI / 60)); double secondY = centerY - sLength * Math.cos(second * (2 * Math.PI / 60)); Line sLine = new Line(centerX, centerY, secondX, secondY);sLine.setStroke(Color.GRAY);// 分针double mLength = clockRadius * 0.65;double minuteX = centerX + mLength * Math.sin(minute * (2 * Math.PI / 60)); double minuteY = centerY - mLength * Math.cos(minute * (2 * Math.PI / 60)); Line mLine = new Line(centerX, centerY, minuteX, minuteY);mLine.setStroke(Color.BLUE);// 时针double hLength = clockRadius * 0.5;double hourX = centerX + hLength *Math.sin((hour % 12 + minute / 60.0) * (2 * Math.PI / 12));double hourY = centerY - hLength *Math.cos((hour % 12 + minute / 60.0) * (2 * Math.PI / 12));Line hLine = new Line(centerX, centerY, hourX, hourY);sLine.setStroke(Color.GREEN);// 将之前的结点清空,绘制新创建的结点getChildren().clear();getChildren().addAll(circle, t1, t2, t3, t4, sLine, mLine, hLine);}}以上就是本⽂的全部内容,希望对⼤家的学习有所帮助,也希望⼤家多多⽀持。
Java数字时钟(简单的桌面应用)
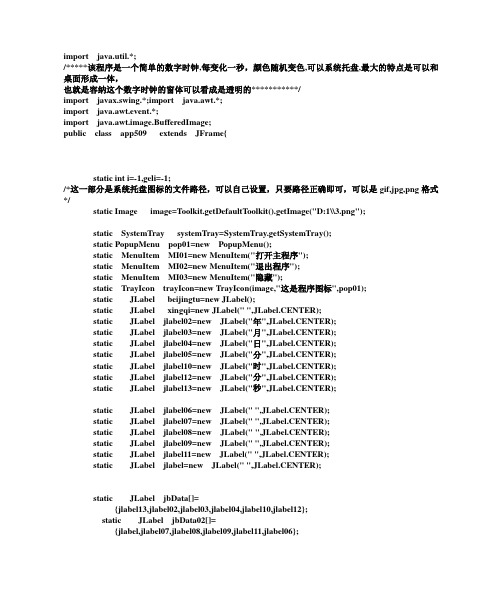
import java.util.*;/*****该程序是一个简单的数字时钟,每变化一秒,颜色随机变色,可以系统托盘,最大的特点是可以和桌面形成一体,也就是容纳这个数字时钟的窗体可以看成是透明的***********/import javax.swing.*;import java.awt.*;import java.awt.event.*;import java.awt.image.BufferedImage;public class app509 extends JFrame{static int i=-1,geli=-1;/*这一部分是系统托盘图标的文件路径,可以自己设置,只要路径正确即可,可以是gif,jpg,png格式*/static Image image=Toolkit.getDefaultToolkit().getImage("D:1\\3.png");static SystemTray systemTray=SystemTray.getSystemTray();static PopupMenu pop01=new PopupMenu();static MenuItem MI01=new MenuItem("打开主程序");static MenuItem MI02=new MenuItem("退出程序");static MenuItem MI03=new MenuItem("隐藏");static TrayIcon trayIcon=new TrayIcon(image,"这是程序图标",pop01);static JLabel beijingtu=new JLabel();static JLabel xingqi=new JLabel(" ",JLabel.CENTER);static JLabel jlabel02=new JLabel("年",JLabel.CENTER);static JLabel jlabel03=new JLabel("月",JLabel.CENTER);static JLabel jlabel04=new JLabel("日",JLabel.CENTER);static JLabel jlabel05=new JLabel("分",JLabel.CENTER);static JLabel jlabel10=new JLabel("时",JLabel.CENTER);static JLabel jlabel12=new JLabel("分",JLabel.CENTER);static JLabel jlabel13=new JLabel("秒",JLabel.CENTER);static JLabel jlabel06=new JLabel(" ",JLabel.CENTER);static JLabel jlabel07=new JLabel(" ",JLabel.CENTER);static JLabel jlabel08=new JLabel(" ",JLabel.CENTER);static JLabel jlabel09=new JLabel(" ",JLabel.CENTER);static JLabel jlabel11=new JLabel(" ",JLabel.CENTER);static JLabel jlabel=new JLabel(" ",JLabel.CENTER);static JLabel jbData[]={jlabel13,jlabel02,jlabel03,jlabel04,jlabel10,jlabel12};static JLabel jbData02[]={jlabel,jlabel07,jlabel08,jlabel09,jlabel11,jlabel06};static int mill=0;static int minute=0;static int hour=0;static int day=0;static int month=0;static int year=0;static int week;static int zuobiaoX,zuobiaoY;static JFrame JF01=new JFrame();static JDialog JF=new JDialog(JF01," ");static Robot robot;static BufferedImage image1;static Rectangle rec;static class mouseListener extends MouseAdapter{public void mouseClicked(MouseEvent a){if(a.getSource()==trayIcon){if(a.getClickCount()==2){i++;if(i%2==1){geli++;if(geli%2==1){image1=robot.createScreenCapture(rec);beijingtu.setIcon(new ImageIcon(image1));JF.setBounds(0,0,120,560);JF.setVisible(true);}}else{JF.setBounds(0,0,400,1);}}}}public void mouseEntered(MouseEvent a){if(a.getSource()==JF){image1=robot.createScreenCapture(rec);beijingtu.setIcon(new ImageIcon(image1));JF.setBounds(0,0,120,560);JF.setVisible(true);}}public void mouseExited(MouseEvent a){if(a.getSource()==JF){JF.setBounds(0,0,400,1);}}}public static void main(String args[]) throws Exception{trayIcon.addMouseListener(new mouseListener());rec=new Rectangle(0,0,(int)Toolkit.getDefaultToolkit().getScreenSize().getWidth(),(int)Toolkit.getDefaultToolkit().getScreenSize().getHeight());try{robot=new Robot(); }catch(Exception b){}image1=robot.createScreenCapture(rec);beijingtu.setIcon(new ImageIcon(image1));MI01.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent a){Image1=robot.createScreenCapture(rec);beijingtu.setIcon(new ImageIcon(image1));JF.setBounds(0,0,120,560);JF.setVisible(true);}});MI03.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent a){JF.setBounds(0,0,400,1);}});MI02.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent a){System.exit(0);}});try{pop01.add(MI01);pop01.add(MI03);pop01.add(MI02);systemTray.add(trayIcon);trayIcon.setImageAutoSize(true);trayIcon.addMouseListener(new mouseListener());}catch(Exception a){} JF.setResizable(false) ;JF.addMouseListener(new mouseListener());JF.setUndecorated(true);beijingtu.setBounds(0,0,(int)Toolkit.getDefaultToolkit().getScreenSize().getWidth(),(int)Toolkit.getDefaultToolkit().getScreenSize().getHeight());JF.setLayout(null);JF.setBounds(0,0,120,560);JF.setVisible(true);jlabel02.setBounds(91,94,24,25);jlabel06.setBounds(15,94,64,28);jlabel03.setBounds(91,175,24,25);jlabel07.setBounds(2,125,86,75);jlabel04.setBounds(91,261,24,25);jlabel08.setBounds(2,210,86,75);jlabel10.setBounds(91,346,24,25);jlabel09.setBounds(2,296,86,75);jlabel11.setBounds(2,382,86,75);jlabel12.setBounds(91,433,24,25);jlabel13.setBounds(91,520,24,25);jlabel.setBounds(2,468,86,75);xingqi.setBounds(2,30,118,62);JF.add(xingqi);xingqi.setHorizontalTextPosition(JLabel.CENTER);xingqi.setFont(new Font("微软雅黑",Font.BOLD,20));for(int i=0;i<jbData.length;i++){JF.add(jbData[i]);JF.add(jbData02[i]);}for(int i=0;i<jbData.length;i++){jbData[i].setFont(new Font("微软雅黑",Font.BOLD,15));jbData02[i].setFont(new Font("微软雅黑",Font.BOLD,30));}jlabel06.setFont(new Font("微软雅黑",Font.BOLD,15));for(int i=0;i<jbData.length;i++){jbData[i].setForeground(Color.blue);jbData02[i].setForeground(Color.red);}for(int i=0;i<jbData.length;i++){jbData[i].setHorizontalTextPosition(JLabel.CENTER);jbData02[i].setHorizontalTextPosition(JLabel.CENTER);}jlabel02.setHorizontalTextPosition(JLabel.RIGHT);JF.add(beijingtu);xiancheng xiancheng01=new xiancheng();xiancheng01.start();}}class xiancheng extends Thread{static GregorianCalendar date=new GregorianCalendar();app509 app=new app509();public void run(){for(int i=0;i<60;){try{sleep(1000);}catch(Exception a){}app.year=(date=new GregorianCalendar()).get(date.YEAR);app.jlabel06.setText(Integer.toString(app.year));app.month=((date=new GregorianCalendar()).get(date.MONTH)+1);app.jlabel07.setText(Integer.toString(app.month));app.day=(date=new GregorianCalendar()).get(date.DAY_OF_MONTH);app.jlabel08.setText(Integer.toString(app.day));app.week=(date=new GregorianCalendar()).get(date.DAY_OF_WEEK);app.hour=(date=new GregorianCalendar()).get(date.HOUR_OF_DAY);app.jlabel09.setText(Integer.toString(app.hour));app.minute=(date=new GregorianCalendar()).get(date.MINUTE);app.jlabel11.setText(Integer.toString(app.minute));l=(date=new GregorianCalendar()).get(date.SECOND);app.jlabel.setText(Integer.toString(l));if(app.jlabel.getText()!=" "){app.xingqi.setForeground(new Color((int)(255*Math.random()),(int)(255*Math.random()),(int)(255*Math.random())));for(int j=0;j<app.jbData.length;j++){app.jbData[j].setForeground(new Color((int)(255*Math.random()),(int)(255*Math.random()),(int)(255*Math.random())));app.jbData02[j].setForeground(new Color((int)(255*Math.random()),(int)(255*Math.random()),(int)(255*Math.random())));}} switch(app.week){case 1 : app.xingqi.setText("星期日");break;case 2 : app.xingqi.setText("星期一");break;case 3 : app.xingqi.setText("星期二");break;case 4 : app.xingqi.setText("星期三");break;case 5 : app.xingqi.setText("星期四");break;case 6 : app.xingqi.setText("星期五");break;case 7 : app.xingqi.setText("星期六");break;}System.gc();}}}/****复制以上代码进行编译即可*****/程序效果图:。
电子时钟程序代码

(一)计时模块1. 秒计数是由一个六十进制的计数器构成,生成元器件如下Clk:驱动秒计时器的时钟信号Clr:校准时间时清零的输入端En:使能端Sec0[3..0]sec1[3..0]:秒的高位显示,低位显示Co:进位输出端,作为分的clk输入代码如下:library ieee;use ieee.std_logic_1164.all;use ieee.std_logic_unsigned.all;entity second isport (clk,clr,en:in std_logic;sec0,sec1:out std_logic_vector(3 downto 0);co:outstd_logic);end second;architecture sec of second isSIGNAL cnt1,cnt0:std_logic_vector(3 downto0);beginprocess(clk)beginif(clr='0')thencnt0<="0000";cnt1<="0000";elsif(clk'eventand clk='1')thenif(en='1')thenifcnt1="0101" and cnt0="1000" thenco<='1';cnt0<="1001";elsifcnt0<"1001" thencnt0<=(cnt0+1);elsecnt0<="0000";ifcnt1<"0101"thencnt1<=cnt1+1;elsecnt1<="0000";co<='0';endif;endif;endif;endif;sec1<=cnt1;sec0<=cnt0;endprocess;end sec;2.分计数是由六十进制的计数器构成,生成元器件如下Clk:设置分输入和秒进位的或输入En:使能输入Min1[3..0] min0[3..0]:分的高位显示,低位显示Co:向时的进位输出代码如下:library ieee;use ieee.std_logic_1164.all;use ieee.std_logic_unsigned.all;entity minute isport (clk,en:in std_logic;min1,min0:out std_logic_vector(3 downto 0);co:outstd_logic);end minute;architecture min of minute isSIGNAL cnt1,cnt0:std_logic_vector(3 downto0);beginprocess(clk)beginif(clk'eventand clk='1')thenifen='1' thenifcnt1="0101" and cnt0="1001" thenco<='1';cnt0<="0000";cnt1<="0000";elsifcnt0<"1001" thencnt0<=(cnt0+1);elsecnt0<="0000";cnt1<=cnt1+1;co<='0';endif;endif;endif;min1<=cnt1;min0<=cnt0;endprocess;end min;3.时计数是由二十四进制的计数器构成,生成元器件如下Clk:设置时间输入和分进位输入的或en:使能端h1[3..0] h0[3..0]:时的高位显示和低位显示代码如下:library ieee;use ieee.std_logic_1164.all;use ieee.std_logic_unsigned.all;entity hour isport(clk,en:in std_logic;h1,h0:out std_logic_vector(3 downto 0));end hour;architecture beha of hour issignal cnt1,cnt0:std_logic_vector(3 downto0);beginprocess(clk)beginif(clk'event and clk='1') thenif en='1' thenif cnt1="0010" andcnt0="0011" thencnt1<="0000";cnt0<="0000";elsif cnt0<"1001" thencnt0<=cnt0+1;elsecnt0<="0000";cnt1<=cnt1+1;end if;end if;end if;h1<=cnt1;h0<=cnt0;end process;end beha;。
Java实现的简单数字时钟功能示例
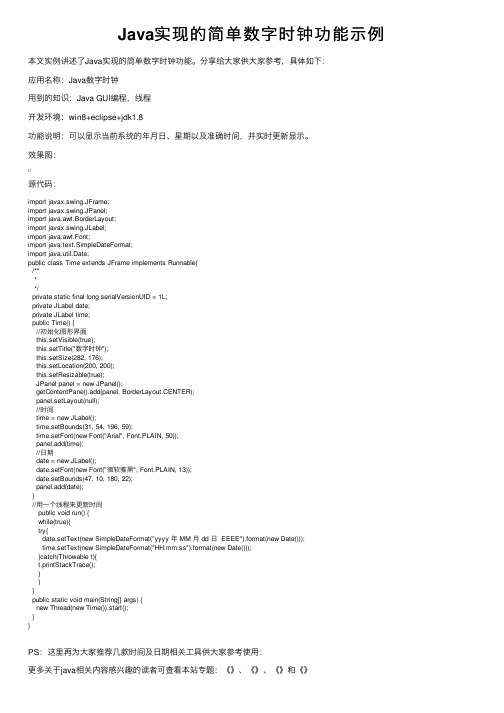
Java实现的简单数字时钟功能⽰例本⽂实例讲述了Java实现的简单数字时钟功能。
分享给⼤家供⼤家参考,具体如下:应⽤名称:Java数字时钟⽤到的知识:Java GUI编程,线程开发环境:win8+eclipse+jdk1.8功能说明:可以显⽰当前系统的年⽉⽇、星期以及准确时间,并实时更新显⽰。
效果图:源代码:import javax.swing.JFrame;import javax.swing.JPanel;import java.awt.BorderLayout;import javax.swing.JLabel;import java.awt.Font;import java.text.SimpleDateFormat;import java.util.Date;public class Time extends JFrame implements Runnable{/****/private static final long serialVersionUID = 1L;private JLabel date;private JLabel time;public Time() {//初始化图形界⾯this.setVisible(true);this.setTitle("数字时钟");this.setSize(282, 176);this.setLocation(200, 200);this.setResizable(true);JPanel panel = new JPanel();getContentPane().add(panel, BorderLayout.CENTER);panel.setLayout(null);//时间time = new JLabel();time.setBounds(31, 54, 196, 59);time.setFont(new Font("Arial", Font.PLAIN, 50));panel.add(time);//⽇期date = new JLabel();date.setFont(new Font("微软雅⿊", Font.PLAIN, 13));date.setBounds(47, 10, 180, 22);panel.add(date);}//⽤⼀个线程来更新时间public void run() {while(true){try{date.setText(new SimpleDateFormat("yyyy 年 MM ⽉ dd ⽇ EEEE").format(new Date()));time.setText(new SimpleDateFormat("HH:mm:ss").format(new Date()));}catch(Throwable t){t.printStackTrace();}}}public static void main(String[] args) {new Thread(new Time()).start();}}PS:这⾥再为⼤家推荐⼏款时间及⽇期相关⼯具供⼤家参考使⽤:更多关于java相关内容感兴趣的读者可查看本站专题:《》、《》、《》和《》希望本⽂所述对⼤家java程序设计有所帮助。
java 时钟

这是时钟类import javax.swing.*;import java.awt.*;import java.util.*;public class StillClock extends JPanel{private int hour; //小时private int minute; //分钟private int second; //秒钟public StillClock(){setCurrentTime();}public StillClock(int hour, int minute, int second){this.hour = hour;this.minute = minute;this.second = second;}public int getHour(){return hour;}public void setHour(int hour){this.hour = hour;repaint();}public int getMinute(){return minute;}public void setMinute(int minute){this.minute = minute;repaint();}public int getSecond(){return second;}public void setSecond(int second){this.second = second;repaint();}protected void paintComponent(Graphics g){super.paintComponent(g);int clockRadius = (int)(Math.min(getWidth(), getHeight()) * 0.8 * 0.5);int xCenter = getWidth() / 2;int yCenter = getHeight() / 2;/*g.setColor(Color.black);g.drawString("12", xCenter - 5, yCenter - clockRadius + 16);g.drawString("6", xCenter - 3, yCenter + clockRadius - 3);g.drawString("3", xCenter + clockRadius - 10, yCenter - 3);g.drawString("9", xCenter - clockRadius + 3, yCenter + 5);*/ //写数字int hLength = (int)(clockRadius * 0.5);int xHour = (int)(xCenter + hLength * Math.sin((hour % 12 + minute / 60.0) * (2 * Math.PI / 12)));int yHour = (int)(yCenter - hLength * Math.cos((hour % 12 + minute / 60.0) * (2 * Math.PI / 12)));g.setColor(Color.green);g.drawLine(xCenter, yCenter, xHour, yHour);//g.drawLine(xCenter - 1, yCenter - 1, xHour - 1, yHour - 1);//g.drawLine(xCenter + 1, yCenter + 1, xHour - 1, yHour - 1);//g.drawLine(xCenter - 2, yCenter - 2, xHour - 2, yHour - 2);//g.drawLine(xCenter + 2, yCenter + 2, xHour - 2, yHour - 2);int mLength = (int)(clockRadius * 0.658);int xMinute = (int)(xCenter + mLength * Math.sin(minute * (2 * Math.PI / 60)));int yMinute = (int)(yCenter - mLength * Math.cos(minute * (2 * Math.PI / 60)));g.setColor(Color.blue);g.drawLine(xCenter, yCenter, xMinute, yMinute);int sLength = (int)(clockRadius * 0.8);int xSecond = (int)(xCenter + sLength * Math.sin(second * (2 * Math.PI / 60)));int ySecond = (int)(yCenter - sLength * Math.cos(second * (2 * Math.PI / 60)));g.setColor(Color.red);g.drawLine(xCenter, yCenter, xSecond, ySecond);int s = 0;//int x = (int)(xCenter + d * Math.sin(s * (2 * Math.PI / 60)));//int y = (int)(yCenter - d * Math.cos(s * (2 * Math.PI / 60)));//int x1 = (int)(xCenter + clockRadius * Math.sin(s * (2 * Math.PI / 60)));//int y1 = (int)(yCenter - clockRadius * Math.cos(s * (2 * Math.PI / 60)));for (s = 0; s < 60; s++){int i = 3;int d = (int)(clockRadius * 0.95);;if(s % 5 == 0){i = 6;g.setColor(Color.GRAY);}else{i = 4;g.setColor(Color.BLACK);}//g.drawLine((int)(xCenter + d * Math.sin(s * (2 * Math.PI / 60))), (int)(yCenter - d * Math.cos(s * (2 * Math.PI / 60))), (int)(xCenter + clockRadius * Math.sin(s * (2 * Math.PI / 60))),(int)(yCenter - clockRadius * Math.cos(s * (2 * Math.PI / 60))));g.fillOval((int)(xCenter + d * Math.sin(s * (2 * Math.PI / 60))), (int)(yCenter - d * Math.cos(s * (2 * Math.PI / 60))), i, i);}g.setColor(Color.PINK);g.fillOval(xCenter - 3, yCenter - 3, 6, 6);}void setCurrentTime() {Calendar calendar = new GregorianCalendar();this.hour = calendar.get(Calendar.HOUR_OF_DAY);this.minute = calendar.get(Calendar.MINUTE);this.second = calendar.get(Calendar.SECOND);}public Dimension getPreferredSize(){return new Dimension(200, 200);}}ClockAinmation 类继承StillClock 类import javax.swing.*;import java.awt.event.*;public class ClockAinmation extends StillClock {public ClockAinmation(){Timer timer = new Timer(1000, new TimerListener());timer.start();}private class TimerListener implements ActionListener {public void actionPerformed(ActionEvent e) {setCurrentTime();repaint();}}public static void main(String[] args) {JFrame frame = new JFrame("时钟");ClockAnimation clock = new ClockAnimation();frame.add(clock);frame.setLocationRelativeTo(null);frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);frame.setSize(300,300);frame.setResizable(false);frame.setVisible(true);}}。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import java.util.*;
import java.text.SimpleDateFormat;
public class ClockJFrame extends JFrame{
private Date now=new Date();
Panel buttons=new Panel();
Button button_start=new Button("启动");
Button button_interrupt=new Button("停止");
Clock label=new Clock();
public ClockJFrame() //构造方法
{
super("电子时钟");
this.setBounds(300,240,300,120);
this.setDefaultCloseOperation(EXIT_ON_CLOSE);
this.setLayout(new BorderLayout());
this.getContentPane().add("North",label);//初始化一个容器,用来在容器上添加一个标签
this.getContentPane().add("South",buttons);
buttons.setLayout(new FlowLayout());
buttons.add(button_start);
buttons.add(button_interrupt);
setVisible(true);
}
private class Clock extends Label implements ActionListener,Runnable{ private Thread clocker=null;
private Date now=new Date();
public Clock(){
button_start.addActionListener(this);
button_interrupt.addActionListener(this);
SimpleDateFormat dateFormat = new SimpleDateFormat("HH:mm:ss");//可以方便地修改日期格式
String t = dateFormat.format( now );
this.setText(t);
}
public void start(){
if(clocker==null){
clocker=new Thread(this);
clocker.start();
}
}
public void stop(){
clocker=null;
}
public void run(){
Thread currentThread=Thread.currentThread();
while(clocker==currentThread){
now=new Date();
SimpleDateFormat dateFormat = new
SimpleDateFormat("HH:mm:ss");//可以方便地修改日期格式
String t = dateFormat.format( now );
this.setText(t);
try{
clocker.sleep(1000);
}catch(InterruptedException ie){
JOptionPane.showMessageDialog(this,"Thread error:+ie");
}
}
}
public void actionPerformed(ActionEvent e){
if (e.getSource()==button_start) {
clocker = new Thread(this); //重新创建一个线程对象
clocker.start();
button_start.setEnabled(false);
button_interrupt.setEnabled(true);
}
if (e.getSource()==button_interrupt) //单击中断按钮时
{
clocker.stop(); //设置当前线程对象停止标记
button_start.setEnabled(true);
button_interrupt.setEnabled(false);
}
}
}//内部类结束
public static void main(String[] args) {
ClockJFrame time=new ClockJFrame();
}
}
运行结果:。