福建师范大学C语言第一次作业及答案
C语言程序设计-第一次作业的答案

printf("y=%d\n",y);
}
输出结果为:
y=21.
Press any key to continue
n
n--
y
10
1
10
3
9
9
5
8
8
7
7
7
9
6
6
11
5
5
13
4
4
15
3
3
17
2
2
19
1
1
21
0
0
-1
y=21
5.#include<stdio.h>
1 1 2 3 5
8 13 21 34 55
Press any key to continue
i
f
f1
f2
i%5
1
1
1 1(不换行)
3
2
1
2
2
2(不换行)
4
3
2
3
4
3(不换行)
5
5
3
5
0
5(换行)
6
8
5
8
1
8(不பைடு நூலகம்行)
7
13
8
13
2
13(不换行)
8
21
13
21
3
21(不换行)
9
34
21
34
i
s
i%2
i%3
s+=i
1
大一C语言选择题及答案

第1章C语言程序设计概述一.选择题1.以下叙述中正确的是(A)A.C语言程序中注释部分可以出现在程序中任意合适的地方B.花括号“{”和“}”只能作为函数体的定界符C.构成C语言程序的基本单位是函数,所有函数名都可以由用户命名D.分号是C语言语句之间的分隔符,不是语句的一部分2.以下叙述中错误的是(A)A.用户所定义的标识符允许使用关键字B.用户所定义的标识符应尽量做到“见名知意”C.用户所定义的标识符必须以字母或下划线开头D.用户所定义的标识符中,大,小写字母代表不同标识3.下列关于C语言用户标识符的叙述中正确的是(B)A.用户标识符中可以出现下划线和中划线(减号)B.用户标识符中不可以出现中划线,但可以出现下划线C.用户标识符中可以出现下划线,但不可以放在用户标识符的开头D.用户标识符中可以出现下划线和数字,它们都可以放在用户标识符的开头4.以下关于C语言标识符的描述中,正确的是(D)A.标识符可以由汉子组成B.标识符只能以字母开头C.关键字可以作为用户标识符D.Area与area是不同的标识符5.以下正确叙述是(C)A.在C语言中,main函数必须位于文件的开头B.C语言每行只能写一条语句C.C语言本身没有输入,输出语句D.对一个C语言进行编译预处理时,可检查宏定义的语法错误6.下面说法正确的是(C)A.C程序由符号构成B.C程序由标识符构成C.C程序由函数构成D.C程序由C语句构成7.以下叙述不正确的是(D)A.一个C源程序可以由一个或多个函数组成B.一个C源程序必须包含一个main函数C.C程序的基本组成单位是函数D.在C程序中,注释说明只能位于一条语句后面8.C语言规定:在一个源程序中,main函数的位置可以任意9.以下不能定义为用户标识符的是(D)A.scanfB.V oidC._3comD.int10.以下不合法的用户标识符是(C)A。
j2_KEY B.Double C.4d D._8_11.下列四组选项中,均不是C语言关键字的是(A)A.define IF typeB.getc char printfC.include scanf caseD.while go pow12.以下不能定义为用户标识符的是(D)A.MainB._0C._intD.sizeof13.以下不合法的用户标识符是(C)A。
C语言 (福师大考的)

一、编程的第二道题1. 完成函数fun(int a【】,int n)实现将一维数组a个元素在数组中循环右移n个位置例数组a个元素为 6 7 8 9 0参数n=2时循环右移2个位置后数组个元素为:9 0 6 7 8#include <stdio.h>#include <conio.h>#define COL 5void fun(int a[],int n){/** int i,b[COL];for(i=0;i<COL;i++){if (i-n<0)b[i]=a[COL-n+i];elseb[i]=a[i-n];}for (i=0;i<COL;i++)a[i]=b[i];/**/}void main(){ int arra[COL] = {6,7,8,9,0};int i,j,n;printf("The array a is:\n");for(i=0; i<COL; i++)printf("%5d", arra[i]);printf("\n");printf("Sites of moving(n>0): ");scanf("%d",&n);fun(arra,n);printf("Now The array a is:\n");for(i=0; i<COL; i++)printf("%5d", arra[i]);printf("\n");getch();}2.把数组里基数的并且尾巴是3的放在一个数组,然后把偶数的与后一个求平均值放在另一个数组#include <stdio.h>#include <conio.h>#define N 10void fun(int a[]){/**/int b[N],j,m=0,n=0;float c[N];for (j=0;j<N;j++){if ((a[j]%2!=0)&&(a[j]%10==3))b[m++]=a[j];if (a[j]%2==0)c[n++]=(a[j]+a[j+1])/2.0;}for (j=0;j<m;j++)printf("%3d",b[j]);printf("\n");for (j=0;j<n;j++)printf("%.2f",c[j]);/**/}void main(){int a[N],i;printf("The array a is:\n");for(i=0;i<N;i++)scanf("%d",&a[i]);fun(a);getch();}3.以x为首项,d为公差的等差数列n项,吧n项赋值给数组a【】再求n项和。
C语言上机作业试题5套含答案

第一次上机作业(2021):要求:独立完成,上机调试通事后上交作业提交邮件主题命名:班级学号姓名(第1次作业),例如:电1301班2王鹏(第1次作业)以附件形式将每次作业的所有程序源程序紧缩打包后提交,紧缩文件命名格式同上。
程序必需采用缩进格式1.大写字母转换成小写字母从键盘输入一个大写英文字母,输出相应的小写字母。
例:输入G输出g2.求平方根输入一个实数x,计算并输出其平方根(保留1 位小数)。
例:输入17输出The square root of is3.温度转换设计一个程序将华氏温度转换成摄氏温度c = 5/9(f-32)a)输入华氏温度(实型)b)输出的摄氏温度结果保留两位小数例:Please input Fahrenheit temperature:The corresponding Celsius temperature is4. 计算旅途时刻输入二个整数time1 和time2,表示火车的起身时刻和抵达时刻,计算并输出旅途时刻。
(输入的有效的时刻范围是0000 到2359,不需要考虑起身时刻晚于抵达时刻的情形。
)例:输入712 1411 (起身时刻是7:12,抵达时刻是14:11)输出The train journey time is 6 hours 59 minutes.5. 数字加密输入一个四位数,将其加密后输出。
方式是将该数每一名上的数字加9,然后除以10 取余,做为该位上的新数字,最后将第1 位和第3 位上的数字互换,第2 位和第4 位上的数字互换,组成加密后的新数。
例:输入1257输出The encrypted number is 4601试探题:你可否编程找出谁做的好事?有四位同窗中的一名做了好事,不留名,夸奖信来了以后,校长问这四位是谁做的好事。
⏹A说:不是我。
⏹B说:是C。
⏹C说:是D。
⏹D说:他乱说。
已知三个人说的是实话,一个人说的是谎话。
此刻要依照这些信息,找出做了好事的人。
福师《C++语言程序设计》在线作业1答案

福师《C++语言程序设计》在线作业1答案福师《C++语言程序设计》在线作业1一、单选题(共30 道试题,共60 分。
)V 1. 函数重载体现了面向对象的(b)A. 封装性B. 多态性C. 继承性D. 抽象性满分:2 分2. 已知下列语句中的x和y都是int型变量,其中不正确的语句是(d)A. y+=++x;B. ++x+=y;C. y=x++;D. x++=y;满分:2 分3. 循环体至少被执行一次的语句为(c )A. for循环B. while循环C. do循环D. 任一种循环满分:2 分4. 下列对引用的陈述中,不正确的是(c )A. 每一个引用都是其所引用对象的别名,因此必须初始化B. 形式上针对引用的操作实际上作用于它所引用的对象C. 一旦定义了引用,一切针对其所引用对象的操作只能通过该引用间接进行D. 不需要单独为引用分配存储空间满分:2 分5. 关于异常和C++提供的异常处理机制。
不正确的说法是(d)A. 若程序员预见到程序的异常,则一定可以通过修改程序代码来避免异常出现B. 使用dyname cast操作符可能会发生异常C. 异常可以用catch捕获处理D. 异常可以是对象,也可以是普通函数满分:2 分6. 已知:print( )函数是一个类的常成员函数,且无返回值。
在下列表示中,正确的是(a)A. void print( ) const;B. const void print( );C. void print( );D. void print(const);满分:2 分7. 在C++语言中,为了使得类中的某些数据成员及某些成员函数的返回值能取任意类型,应使用(b )A. 类B. 类模板C. 抽象类D. 虚基类满分:2 分8. 下列各个成员函数中,纯虚函数是(d)A. void fun(int)=0B. virtual void fun(int)C. virtual void fun(int){}D. virtual void fun(int)=0满分:2 分9. 下列函数中,可以为虚函数的是(d )A. 自定义的构造函数B. 拷贝构造函数C. 静态成员函数D. 析构函数满分:2 分10. 若x,y,z均被定义为整数,则下列表达式终能正确表达代数式1/(x*y*z)的是(b)A. 1/x*y*zB. 1.0/(x*y*z)C. 1/(x*y*z)D. 1/x/y/(float)z满分:2 分11. 下面不能正确表示a*b/(c*d)的表达式是(a )A. (a*b)/c*dB. a*b/(c*d)C. a/c/d*bD. a*b/c/d满分:2 分12. 如果有char* ssl=“0123456789”,则,sizeof(ssl)的值是(a)A. 4B. 10C. 11D. 40满分:2 分13. 如果变量x,y已经正确定义,下列语句哪一项不能正确将x,y 的值进行交换(d)A. x=x+y,y=x-y,x=x-yB. t=x,x=y,y=tC. t=y,y=x,x=tD. x=t,t=y,y=x满分:2 分14. 下列哪项不是面向对象程序设计的主要特征(d)A. 封装B. 继承C. 多态D. 结构满分:2 分15. 在下列运算符中,优先级最低的是(a )A. ||B. !=C. <D. +满分:2 分16. 在一个源文件中定义的全局变量的作用域为(c )A. 本程序的全部范围B. 本函数的全部范围C. 从定义该变量的位置开始到本文件结束D. 以上说法都不正确满分:2 分17. 下列关于函数模板和模板函数的描述,错误的是(d)A. 函数模板是一组函数的模板B. 模板函数是一个实在的函数C. 函数模板是定义重载函数的一种工具D. 模板函数在编译时不生成可执行代码满分:2 分18. 下面程序的结果是什么?Int i=3,j=4;i?i++:j++;printf (“%d %d\n”,i,j);(b )A. 3 3B. 4 4C. 3 4D. 4 3满分:2 分19. 已知字符'b'的ASCII码为98,语句printf("%d,%c",'b','b'+1 );的输出为(d)A. 98,bB. 语句不合法C. 98,99D. 98,c满分:2 分20. 派生类公有继承基类时,基类中的所有公有成员成为派生类的______。
2022秋计本福师《C语言程序设计》在线作业1答案1

2022秋计本福师《C语言程序设计》在线作业1答案1福师《C++语言程序设计》在线作业一试卷总分:100测试时间:--一、单选题(共20道试题,共40分。
)V1.下面有关重载函数的说法中正确的是()A.重载函数必须具有不同的返回值类型B.重载函数形参个数必须不同C.重载函数必须有不同的形参列表D.重载函数名可以不同满分:2分2.下列描述正确的是()。
A.表示m>n为true或mn&&mB.witch语句结构中必须有default语句C.if语句结构中必须有ele语句D.如果至少有一个操作数为true,则包含“‖”运算符的表达式为true满分:2分3.C++语言对C语言做了很多改进,C++语言相对于C语言的最根本的变化是()。
A.增加了一些新的运算符B.允许函数重载,并允许设置缺省参数C.规定函数说明符必须用原型D.引进了类和对象的概念满分:2分4.下列函数中,可以是虚函数的是______。
()A.自定义的构造函数B.拷贝构造函数C.静态成员函数D.析构函数满分:2分5.设voidf1(int某m,long&n);inta;longb;则以下调用合法的是()。
A.f1(a,b);B.f1(&a,b);C.f1(a,&b);D.f1(&a,&b);满分:2分6.如果有char某l=“0123456789”,则,izeof(l)的值是()A.4B.10C.11D.40满分:2分7.下列描述中哪个是正确的()。
A.私有派生的子类无法访问父类的成员B.类A的私有派生子类的派生类C无法初始化其祖先类A对象的属性,因为类A的成员对类C是不可访问的C.私有派生类不能作为基类派生子类D.私有派生类的所有子孙类将无法继续继承该类的成员满分:2分8.下面对静态数据成员的描述中,正确的是_________。
A.静态数据成员可以在类体内进行初始化B.静态数据成员不可以被类的对象调用C.静态数据成员不能受private控制符的作用D.静态数据成员可以直接用类名调用满分:2分9.类模板规定了如何创建一个______。
福建师范大学C语言程序设计考试题

福建师范大学C语言程序设计考试题系别 _______ 班级 _______ 姓名_________ 学号________ 得分__________一、选择题(本大题共40个选项,每个选择项1.5分,共60分)1.在C语言中,合法的字符常数是【1】A)‘程序设计’ B)“程序设计”C)c D)‘\\’2.在C语言中,以下不正确的八进制或不正确的十六进制数是【2】A)090 B)0xABC)0xla D)-0163.执行int x=2,y=3;后表达式x=(y==3)的值是【3】A)0 B)1C)2 D)34.执行语句int a,b,c; a=(b=c=3, c++, b+=c);则a的值是【4】A) 3 B) 4C) 7 D) 115.在循环结构的循环体中执行break语句,其作用是【5】A) 结束本次循环,进行下次循环B) 继续执行break语句之后的循环体中各语句C) 跳出该循环体,提前结束循环D) 终止程序运行6.以下程序运行结果是【6】main(){char s[]=”\’Address\’”;printf(%d\n”, strlen(s));}A) 9 B) 10C) 11 D) 127.以下程序运行结果是【7】main(){int a[]={2, 4, 6, 8, 10}, y=1, x;for(x=0; x<3; x++) y+=a[x+1];printf(“%d\n”, y);}A) 17 B) 18C) 19 D) 208.已定义char x[8]; int i;为了给x数组赋值,以下正确的语句是【8】A) x[8]=”Turbo C”; B) x=”Turbo C”;C) x[]=”Turbo C”; D) for(i=0; i<7; i++) x[i]=getchar();9.设int a[][4]={0, 0};则以下错误的描述是【9】A) 数组a的每个元素都可得到初值0B) 二维数组a的第一维大小为1C) 数组a的行数为1D) 只有元素a[0][0]和a[0][1]可得到初值0,其余元素得不到初值010.以下程序运行结果是【10】fun3(int x){static int a=3;a+=x;return a;}main(){int k=2, m=1, n;n=fun3(k);n=fun3(m);printf(“%d\n”, n);}A) 3 B) 4C) 6 D) 911.以下四个运算符,按优先级由高到低的排列顺序是【11】A) ++ % += > B) % ++ > +=C) % += > ++ D) ++ % > +=12.以下不正确的描述是【12】A) 在函数外部定义的变量是全局变量B) 在函数内部定义的变量是局部变量C) 函数的形参是局部变量D) 局部变量不能与全局变量同名13.“文件包含”预处理语句的使用形式中,当#include后面的文件名用“”括起时,寻找被包含的文件的方式为【13】A) 直接按系统设定的标准方式搜索目录B) 先在源程序所在目录搜索,再按系统设定的标准方式搜索C) 仅仅搜索源程序所在的目录D) 仅仅搜索当前目录14.设有语句int(*p)();则p是一个【14】A) 指向一维整型数组的指针变量B) 指针函数,该函数的返回值为指向整型变量的指针C) 指向函数的指针变量,该函数的返回值为整型D) 数组指针,它的每个元素都是一个可以指向整型变量的指针变量15.若有typedef float AR[5];AR a;则以下叙述正确的是【15】A) a是一个新类型名B) AR是一个实型数组C) AR是一个实型变量D) a是一个实型数组16.C语言的简单数据类型包括【16】A)整型、实型、逻辑型B)整型、实型、字符型、逻辑型C)整型、字符型、逻辑型D)整型、实型、字符型17.在C语言中,变量所分配的内存空间大小【17】A)均为一个字节 B)由用户自己定义C)由变量的类型决定 D)是任意的18.在C语言中,合法的整型常数是【18】A)-0x123 B)3.14159 C)01001101b D)6.7e1019.下列各变量均为整型,选项中不正确的C语言赋值语句是【19】A)i+=++i; B)i=j==k;C)i=j+=i; D)i=j+1=k;20.数学关系式x≤y≤z可用C语言的表达式表示为【20】A)(x<=y)&&(y<=z) B)(x<=y)and(y<=z)C)(x<=y<=z) D)(x<=y)&(y<z)21.若定义float a;现要从键盘输入a数据,其整数位为3位,小数位为2位,则选用【21】A)scanf(“%6f”,&a); B)scanf(“%5.2f”,a);C)scanf(“%6.2f”,&a); D)scanf(“%f”,a);22.下面程序段的运行结果是【22】unsigned a=0356,b;b=~a|a<<2+1;printf(“%x\n”,b);A)ffba B)ff71 C)fff8 D)fc0223.要使以下程序的输出结果为4,则a和b应满足的条件是【23】main(){int s,t,a,b;scanf(“%d%d”,&a,&b);s=t=1;if(a>0) s += 1;if(a>b) t= s + t;elseif(a==b) t=5;else t=2*s;printf(“%d\n”,t);}A)a>0并且a<b B)a<0并且a<bC)a>0并且a>b D)a<0并且a<b24.若int i;则以下循环语句的循环执行次数是【24】for (i=2;i==0;) printf(“%d”,i--);A)无限次 B)0次C)1次 D)2次25.下面程序的输出结果为【25】main(){int i;for(i=100;i<200;i++){if(i%5==0) continue;printf(“%d\n”,i);break;}}A)100 B)101C)无限循环 D)无输出结果26.下列程序段的运行结果是【26】int i=1,a[]={1,5,10,9,13,7};while(a[i]<=10) a[i++]+=2;for (i=0;i<6;i++) printf(“%d”,a[i]);A)2 7 12 11 13 9 B)1 7 12 11 13 7C)1 7 12 11 13 9 D)1 7 12 9 13 727.以下语句把字符串“abcde”赋初值给字符数组,不正确的语句是【27】A)char s[]=“abcde”; B)char s[]={’a’, ’b’,’c’,’d’,’e’,’\0’};C)char s[]={“abcde”}; D)char s[5]= “abcde”;28.若变量c为char类型,能正确判断出c为小写字母的表达式是【28】A) 'a'<=c<= 'z' B) (c>= 'a')||(c<= 'z')C) ('a'<=c)and ('z'>=c) D) (c>= 'a')&&(c<= 'z')29.下列程序段的输出结果是【29】void fun(int *x, int *y){ printf("%d %d", *x, *y); *x=3; *y=4;}main(){ int x=1,y=2;fun(&y,&x);printf("%d %d",x, y);}A) 2 1 4 3 B) 1 2 1 2 C) 1 2 3 4 D) 2 1 1 230.以下程序的输出结果是【30】main(){ int i, k, a[10], p[3];k=5;for (i=0;i<10;i++) a[i ]=i;for (i=0;i<3;i++) p[i ]=a[i *(i+1)];for (i=0;i<3;i++) k+=p[i] *2;printf("%d\n",k);}A) 20 B) 21 C) 22 D)2331.当执行下面的程序时,如果输入ABC,则输出结果是【31】main(){ char ss[10]="1,2,3,4,5";gets(ss); strcat(ss, "6789"); printf("%s\n",ss);}A) ABC6789 B) ABC67 C) 12345ABC6 D) ABC45678932.以下不能对二维数组a进行正确的初始化的语句是【32】A) int a[2][3]={0}; B) int a[][3]={1,2,3,4,5};C) int a[2][3]={{1,2},{3,4},{5,6}}; D) int a[2][3]={{1},{3,4,5}};33.若int a[][3]={1,2,3,4,5,6,7};则a数组第一维的大小是【33】A) 2 B) 3 C) 4 D)无确定值34.下面程序段的运行结果是【34】int a[2][3]={1,2,3},i,j;for(i=0;i<2;i++)for(j=0;j<3;j++){a[i][j]=a[i*j%2][j]+a[i][(i+j)%3];printf(“%d,”,a[i][j]);}A) 2,4,6,2,0,8, B) 1,2,3,0,0,0,C) 1,2,3,2,0,6, D) 2,4,6,2,0,6,35.下面程序段的运行结果是【35】int a[][3]={1,2,3,4,5,6,7,8,9,10,11,12};printf(“%d\n”,a[2][1]);A) 2 B) 4 C) 7 D)836.定义一个结构体变量时,系统分配给它的内存大小是【36】A)各成员所需内存量的总和B)成员中占内存量最大者所需内存容量C)结构中第一个成员所需内存容量D)结构中最后一个成员所需内存容量37.若typedef char STRING[255];STRING s;,则s是【37】A)字符指针数组变量B)字符数组变量C)字符变量D)字符指针变量38.若已定义:int *p,a;,则语句p=&a;中的运算符“&”的含义是【38】A) 位与运算B) 逻辑与运算C) 取指针内容D)取变量地址39.若有定义char *p,ch;则不能正确赋值的语句组是【39】A)p=&ch;scanf(“%c”,p);B)p=(char *)malloc(1); *p=getchar();C)*p=getchar();p=&ch;D)p=&ch;*p=getchar();40.下面程序段的运行结果是【40】char s[]=“ABC”;int i;for(i=0;i<3;i++) printf(“%s”,&s[i]);A) ABC B) ABCABCABC C) AABABC D)ABCBCC1.下列叙述中正确的是A) C语言编译时不检查语法B) C语言的子程序有过程和函数两种C) C语言的函数可以嵌套定义D) C语言所有函数都是外部函数2.以下所列的C语言常量中,错误的是A) 0xFF B) 1.2e0.5 C) 2L D) '\72'3.下列选项中,合法的C语言关键字是A) V AR B) cher C) integer D) default4.设x、y、t均为int型变量,则执行语句:x=y=3;t=++x||++y;后,y的值为A) 不定值B) 4 C) 3 D) 15.下面程序段的运行结果是【5】float y=0.0,a[]={2.0,4.0,6.0,8.0,10.0},*p;int i;p=&a[1];fo r(i=0;i<3;i++) y+=*(p+i);printf(“%f\n”,y);A) 12.0000 B) 28.0000 C) 20.0000 D) 18.00006.下面程序段的运行结果是【6】char c[]={‘a’,‘b’,‘\0’,‘c’,‘\0’};printf(“%s\n”,c);A) ab c B) ‘a’‘b’C) abc D) ab7.下面程序段的运行结果是【7】char s[10],*sp=“HELLO”;strcpy(s,sp);s[0]=‘h’;s[6]=‘!’;puts(s);A) hELLO B) HELLO C) hHELLO! D) h!8.若有定义int a[3][5],i,j;(且0≤i<3,0≤j<5),则a[i][j]的地址不正确表示是【8】A) &a[i][j] B) a[i]+jC) *(a+i)+j D) *(*(a+i)+j)9.若有定义int *p[4];,则标识符p是一个【9】A) 变量的指针变量B) 指向函数的指针变量C) 指向有四个整数元素的一维数组的指针变量D)指针数组名,有四个元素,每个元素均为一个指向整型变量的指针10.若有定义char *language[]={“FORTRAN”, “BASIC”,“PASCAL”,“JAVA”, “C”};则language[2]的值是【10】A) 一个字符B) 一个地址C) 一个字符串D) 不确定11.C语言规定,函数返回值的类型由【11】所决定。
大一c语言笔试题及答案

大一c语言笔试题及答案一、选择题(每题2分,共20分)1. 在C语言中,以下哪个关键字用于定义一个结构体?A. structB. unionC. enumD. typedef答案:A2. 下列关于C语言函数的描述,正确的是:A. 函数必须有返回值B. 函数可以没有返回值C. 函数必须有参数D. 函数不能有参数答案:B3. 在C语言中,以下哪个运算符用于比较两个值是否不相等?A. ==B. !=C. <=D. >=答案:B4. 下列关于数组的描述,错误的是:A. 数组的元素必须是同一数据类型B. 数组可以是多维的C. 数组的大小在编译时确定D. 数组的大小在运行时确定答案:D5. 在C语言中,以下哪个关键字用于定义一个指针?A. intB. charC. floatD. *答案:D6. 下列关于C语言中变量作用域的描述,正确的是:A. 局部变量只能在函数内部使用B. 全局变量只能在函数外部使用C. 局部变量可以在函数外部使用D. 全局变量可以在函数内部使用答案:A7. 在C语言中,以下哪个关键字用于定义一个枚举?A. enumB. structC. unionD. typedef答案:A8. 下列关于C语言中指针的描述,错误的是:A. 指针可以存储地址B. 指针可以存储值C. 指针可以作为函数的参数D. 指针不能作为函数的返回值答案:D9. 在C语言中,以下哪个关键字用于定义一个联合体?A. structB. unionC. enumD. typedef答案:B10. 下列关于C语言中循环的描述,正确的是:A. for循环不能嵌套B. while循环不能嵌套C. do-while循环不能嵌套D. 以上都是错误的答案:D二、填空题(每题3分,共15分)1. 在C语言中,定义一个整型变量的关键字是________。
答案:int2. 如果要定义一个指向整型变量的指针,应该使用________。
C语言第一次作业+答案

1.C语言程序的执行,总是起始于(C )。
A.程序中的第一条可执行语句B.程序中的第一个函数C.main函数D.包含文件中的第一个函数2.以下叙述不正确的是( D )。
A.一个C源程序必须包含一个main函数B.一个C源程序可由一个或多个函数组成C.C程序的基本组成单位是函数D.在C程序中,注释说明只能位于一条语句的后面3.一个C语言程序是由( B )。
A.一个主程序和若干个子程序组成B.若干函数组成C.若干过程组成D.若干子程序组成4.C语言程序能够在不同的操作系统下运行,这说明C语言具有很好的( B )。
A.适应性B.移植性C.兼容性D.操作性5.C语言规定,在一个源程序中,main函数的位置( C )。
A.必须在最开始B.必须在系统调用的库函数的后面C.可以任意D.必须在最后6.下列说法中正确的是( C ).A.C语言程序书写时,不区分大小写字母B.C语言程序书写时,一行只能写一个语句C.C语言程序书写时,一个语句可分成几行书写D.C语言程序书写时每行必须有行号7.下面对C语言特点,不正确描述的是(C).A.C语言兼有高级语言和低级语言的双重特点,执行效率高B.C语言既可以用来编写应用程序,又可以用来编写系统软件C.C语言的可移植性较差D.C语言是一种结构化模块化程序设计语言8.C语言程序的注释是( A )。
A.由“/*"开头,“*/"结尾B.由“/*”开头,“/*”结尾C.由“//"开头D.由“*/”或“//"开头9.C语言程序的语句都是以( B )结尾.A.“。
”B.“;”C.“," D.都不是10.标准C语言程序的文件名的后缀为(A )。
A..c B..cpp C.。
obj D..exe11.C语言程序经过编译以后生成的文件名的后缀为( B )。
A.。
c B..obj C.。
exe D..cpp12.C语言程序经过链接以后生成的文件名的后缀为( C ).A.。
c语言大一上学期试题及答案

c语言大一上学期试题及答案一、选择题(每题2分,共20分)1. C语言中,以下哪个关键字用于定义一个结构体?A. structB. unionC. enumD. typedef答案:A2. 在C语言中,以下哪个函数用于将字符串从大写转换为小写?A. toupper()B. tolower()C. strcat()D. strcmp()答案:B3. 下列关于C语言数组的描述,错误的是?A. 数组的下标从0开始B. 数组的大小在声明时确定C. 数组可以存储不同类型的数据D. 数组可以包含另一个数组答案:C4. C语言中,以下哪个运算符用于执行逻辑与操作?A. &&B. ||C. !D. ^答案:A5. 在C语言中,用于定义一个指针的关键字是?A. intB. charC. floatD. *答案:D6. 下列哪个函数用于计算字符串的长度?A. strlen()B. strcpy()C. strcat()D. strcmp()答案:A7. 在C语言中,以下哪个关键字用于定义一个函数?A. intB. voidC. returnD. struct答案:B8. C语言中,以下哪个关键字用于定义一个枚举类型?A. structB. unionC. enumD. typedef答案:C9. 在C语言中,以下哪个运算符用于执行按位取反操作?A. ~B. ^C. &D. |答案:A10. 下列哪个函数用于将整数转换为字符串?A. atoi()B. itoa()C. sprintf()D. printf()答案:B二、填空题(每题2分,共20分)1. 在C语言中,一个变量的声明语句可以包含关键字________。
答案:int2. 函数________用于将浮点数转换为字符串。
答案:sprintf3. 在C语言中,________运算符用于执行按位与操作。
答案:&4. 用于定义一个函数的返回类型是________。
福师《C++语言程序设计》在线作业1答案
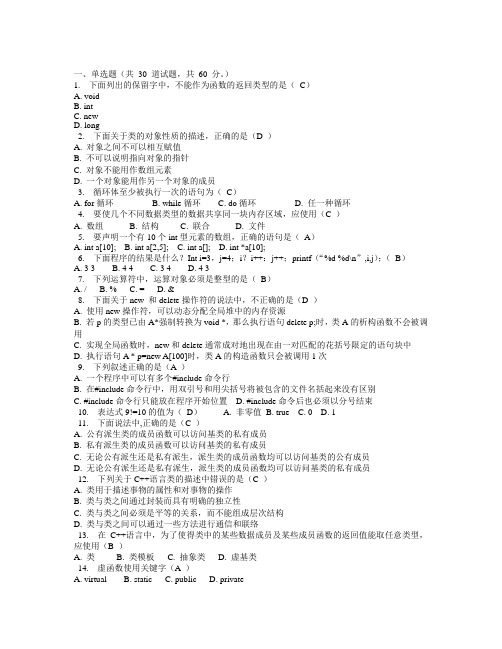
一、单选题(共30 道试题,共60 分。
)1. 下面列出的保留字中,不能作为函数的返回类型的是(C)A. voidB. intC. newD. long2. 下面关于类的对象性质的描述,正确的是(D )A. 对象之间不可以相互赋值B. 不可以说明指向对象的指针C. 对象不能用作数组元素D. 一个对象能用作另一个对象的成员3. 循环体至少被执行一次的语句为(C)A. for循环B. while循环C. do循环D. 任一种循环4. 要使几个不同数据类型的数据共享同一块内存区域,应使用(C )A. 数组B. 结构C. 联合D. 文件5. 要声明一个有10个int型元素的数组,正确的语句是(A)A. int a[10];B. int a[2,5];C. int a[];D. int *a[10];6. 下面程序的结果是什么?Int i=3,j=4;i?i++:j++;printf(“%d %d\n”,i,j);(B)A. 3 3B. 4 4C. 3 4D. 4 37. 下列运算符中,运算对象必须是整型的是(B)A. /B. %C. =D. &8. 下面关于new 和delete操作符的说法中,不正确的是(D )A. 使用new操作符,可以动态分配全局堆中的内存资源B. 若p的类型已由A*强制转换为void *,那么执行语句delete p;时,类A的析构函数不会被调用C. 实现全局函数时,new和delete通常成对地出现在由一对匹配的花括号限定的语句块中D. 执行语句A * p=new A[100]时,类A的构造函数只会被调用1次9. 下列叙述正确的是(A )A. 一个程序中可以有多个#include命令行B. 在#include命令行中,用双引号和用尖括号将被包含的文件名括起来没有区别C. #include命令行只能放在程序开始位置D. #include命令后也必须以分号结束10. 表达式9!=10的值为(D) A. 非零值B. true C. 0 D. 111. 下面说法中,正确的是(C )A. 公有派生类的成员函数可以访问基类的私有成员B. 私有派生类的成员函数可以访问基类的私有成员C. 无论公有派生还是私有派生,派生类的成员函数均可以访问基类的公有成员D. 无论公有派生还是私有派生,派生类的成员函数均可以访问基类的私有成员12. 下列关于C++语言类的描述中错误的是(C )A. 类用于描述事物的属性和对事物的操作B. 类与类之间通过封装而具有明确的独立性C. 类与类之间必须是平等的关系,而不能组成层次结构D. 类与类之间可以通过一些方法进行通信和联络13. 在C++语言中,为了使得类中的某些数据成员及某些成员函数的返回值能取任意类型,应使用(B )A. 类B. 类模板C. 抽象类D. 虚基类14. 虚函数使用关键字(A )A. virtualB. staticC. publicD. private15. 下列字符中不能构成标识符的是(D )A. 数字字符B. 大写字母C. 下划线字符D. 连接符16. 已知函数test定义为:void test() { …………} 则函数定义中void的含义是(A)A. 执行函数test后,函数没有返回值B. 执行函数test后,函数不再返回C. 执行函数test后,函数返回任意类型值D. 以上三个答案都是错误的17. 下列运算符中,(C)运算符在C++中不能重载A. &&B. []C. ::D. new18. 若x,y,z均被定义为整数,则下列表达式终能正确表达代数式1/(x*y*z)的是(B )A. 1/x*y*zB. 1.0/(x*y*z)C. 1/(x*y*z)D. 1/x/y/(float)z19. 已知:print( )函数是一个类的常成员函数,且无返回值。
大一c语言考试题及答案

大一c语言考试题及答案一、选择题(每题2分,共20分)1. C语言中,以下哪个关键字用于定义一个函数?A. classB. structC. functionD. void答案:D2. 以下哪个选项是C语言中的合法整型常量?A. 0x12B. 0x12.5C. 0x12LD. 0x12.5L答案:A3. 在C语言中,以下哪个选项是正确的字符串字面量?A. "Hello, World"B. 'Hello, World'C. "Hello", "World"D. "Hello" 'World'答案:A4. 下面哪个是C语言中的合法变量名?A. 2variableB. variable2C. variable-nameD. variable$name答案:B5. 在C语言中,以下哪个选项是正确的数组声明?A. int array[5];B. int [5] array;C. int array[];D. int array[5][];答案:A6. C语言中,以下哪个运算符用于计算两个整数的差?A. +B. -C. *D. /答案:B7. C语言中,以下哪个选项是正确的条件语句?A. if (condition) { statement; }B. if condition { statement; }C. if (condition) statement;D. if condition statement;答案:A8. 在C语言中,以下哪个关键字用于定义一个结构体?A. structB. unionC. enumD. typedef答案:A9. C语言中,以下哪个选项是正确的指针声明?A. int *ptr;B. int ptr*;C. *int ptr;D. ptr int*;答案:A10. 在C语言中,以下哪个选项是正确的循环语句?A. for (int i = 0; i < 10; i++) { statement; }B. for (int i = 0; i < 10; i++) statement;C. for i = 0; i < 10; i++ statement;D. for (i = 0; i < 10; i++) { statement; }答案:A二、填空题(每题2分,共20分)1. C语言中,用于定义一个整型变量的关键字是________。
大一计算机c语言考试题及答案
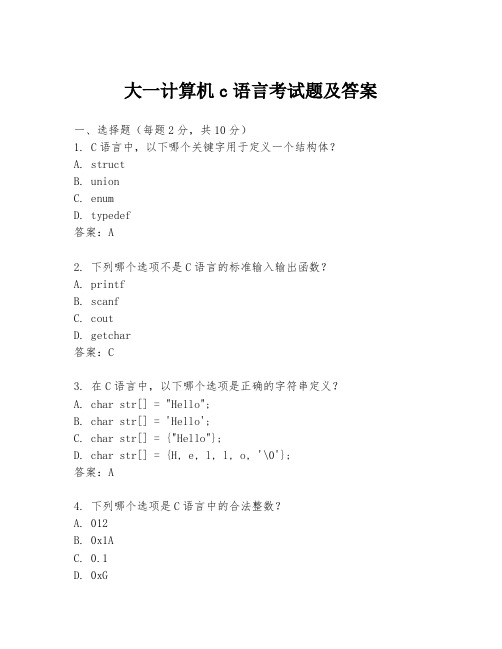
大一计算机c语言考试题及答案一、选择题(每题2分,共10分)1. C语言中,以下哪个关键字用于定义一个结构体?A. structB. unionC. enumD. typedef答案:A2. 下列哪个选项不是C语言的标准输入输出函数?A. printfB. scanfC. coutD. getchar答案:C3. 在C语言中,以下哪个选项是正确的字符串定义?A. char str[] = "Hello";B. char str[] = 'Hello';C. char str[] = {"Hello"};D. char str[] = {H, e, l, l, o, '\0'};答案:A4. 下列哪个选项是C语言中的合法整数?A. 012B. 0x1AC. 0.1D. 0xG答案:B5. 在C语言中,以下哪个选项是正确的数组定义?A. int a[5] = {0, 1, 2, 3, 4};B. int a[] = {0, 1, 2, 3, 4};C. int a[5] = {0, 1, 2};D. int a[] = {0};答案:A二、填空题(每题3分,共15分)1. C语言中,用于定义一个函数的关键字是______。
答案:void2. 在C语言中,表示逻辑与的运算符是______。
答案:&&3. C语言中,用于定义一个字符常量的是______。
答案:''4. C语言中,用于定义一个整型变量的关键字是______。
答案:int5. 在C语言中,用于定义一个指针变量的关键字是______。
答案:*三、简答题(每题5分,共20分)1. 简述C语言中变量的作用域。
答案:变量的作用域是指变量可以被访问的代码区域。
局部变量的作用域是定义它的函数或代码块,全局变量的作用域是整个程序。
2. 解释C语言中的指针是什么?答案:指针是C语言中的一种数据类型,它存储的是变量的内存地址,而不是变量的值。
2022年福建师范大学公共课《C语言》科目期末试卷A(有答案)
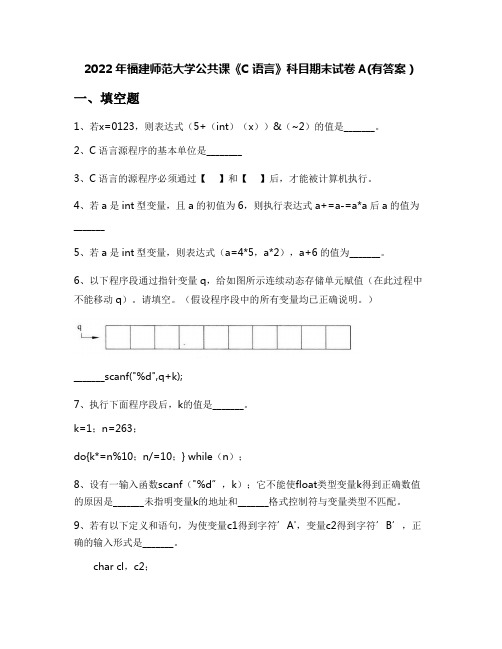
2022年福建师范大学公共课《C语言》科目期末试卷A(有答案)一、填空题1、若x=0123,则表达式(5+(int)(x))&(~2)的值是_______。
2、C语言源程序的基本单位是________3、C语言的源程序必须通过【】和【】后,才能被计算机执行。
4、若a是int型变量,且a的初值为6,则执行表达式a+=a-=a*a后a的值为_______5、若a是int型变量,则表达式(a=4*5,a*2),a+6的值为_______。
6、以下程序段通过指针变量q,给如图所示连续动态存储单元赋值(在此过程中不能移动q)。
请填空。
(假设程序段中的所有变量均已正确说明。
)_______scanf("%d",q+k);7、执行下面程序段后,k的值是_______。
k=1;n=263;do{k*=n%10;n/=10;} while(n);8、设有一输入函数scanf("%d”,k);它不能使float类型变量k得到正确数值的原因是_______未指明变量k的地址和_______格式控制符与变量类型不匹配。
9、若有以下定义和语句,为使变量c1得到字符’A',变量c2得到字符’B’,正确的输入形式是_______。
char cl,c2;scanf("%4c%4c",&cl,8&c2);10、若有定义floata[3][5];则a数组所含数组元素个数是_______,a数组所占的字节数是_______。
二、选择题11、下面4个选项中,是正确的数值常量或字符常量的选项是( )12、设有说明语句:char w;intx;float y;double z;则表达式w*x+z-y值的数据类型为()。
A.float.B.charC.intD.double13、以下C程序的运行结果是。
(注:口表示空格)#include <stdio.h>int main(){long y=-43456;printf("y=%-8ld\n",y);printf("y=%-08ld\n",y);printf("y=%08ld\n",y);printf("y=%+8ld\n",y);return 0;}A.y=囗囗-43456 y=-囗囗43456 y=-0043456 y=-43456B.y=-43456 y=-43456 y=-0043456 y=+囗-43456C.y=-43456 y=-43456 y=-0043456 y=囗囗-43456D.y=囗囗-43456 y=-0043456 y=00043456 y=+4345614、根据下面的程序及数据的输入方式和输出形式,程序中输入语句的正确形式应该为。
大一第一学期c语言考试题及答案

大一第一学期c语言考试题及答案一、选择题(每题2分,共20分)1. 下列哪个选项是C语言中合法的变量名?A. 2variableB. intC. _myVarD. my-variable答案:C2. C语言中,哪个关键字用于定义一个函数?A. functionB. defC. defineD. void答案:D3. 在C语言中,以下哪个数据类型用于存储字符?A. intB. charC. floatD. double答案:B4. 下列哪个选项不是C语言中的控制语句?A. ifB. whileC. forD. switch答案:D5. C语言中,哪个函数用于计算并返回字符串的长度?A. strlenB. lengthC. sizeD. len答案:A6. 在C语言中,下列哪个运算符用于比较两个值是否不相等?A. ==B. !=C. =D. <=答案:B7. C语言中,下列哪个关键字用于定义一个结构体?A. structB. unionC. enumD. typedef答案:A8. 在C语言中,如何定义一个指向整型的指针?A. int *p;B. int *p = 0;C. int p = 0;D. int *p = 1;答案:A9. C语言中,下列哪个选项是正确的数组定义?A. int arr[5] = {1, 2, 3};B. int arr[] = {1, 2, 3};C. int arr[3] = {1, 2, 3, 4, 5};D. int arr[5] = {1, 2};答案:B10. 下列哪个选项是C语言中正确的注释方式?A. // 这是注释B. /* 这是注释 */C. //* 这是注释D. /* 这是注释答案:A二、填空题(每题2分,共20分)1. 在C语言中,使用________关键字定义一个函数。
答案:void2. C语言中,________关键字用于声明一个变量。
答案:int3. 在C语言中,________运算符用于将一个值赋给变量。
C语言第一次作业及答案
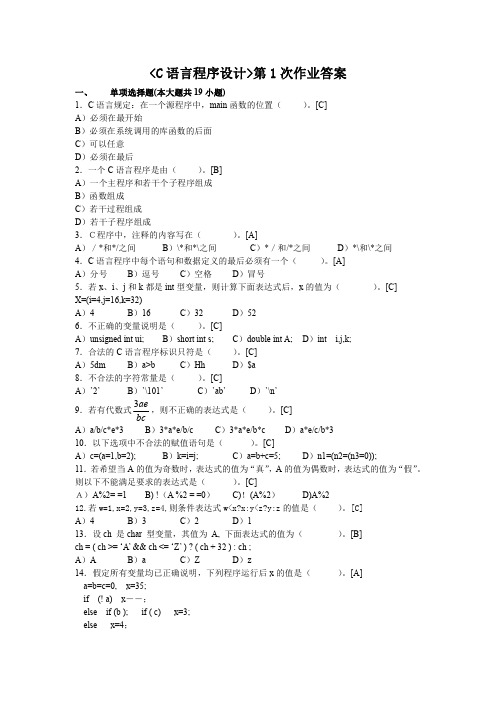
10.下列的 for 循环执行 次。[4] for ( x=0, y=0; ( y!=99) && x<4; x++ ); 11.若 x 是 int 型变量,下面程序段的输出结果是 [**3##4 **5] for ( x=3; x<6 ; x++ ) printf ( ( x%2 ) ? ( “ ** %d”) : (“## %d\n”), x); 12.补足程序,实现如下功能:从键盘上输入若干个学生的成绩,统计并输出最高成绩和最 低成绩,当输入负数时结束输入。 [ (1)score >=0 && score <=100 (2)score<min 或 score <=min] void main() { float score, max=0, min=100; printf(“ Input the score”); 。
3ae ,则不正确的表达式是( bc
Байду номын сангаас
) 。[C]
A)a/b/c*e*3 B)3*a*e/b/c C)3*a*e/b*c D)a*e/c/b*3 10.以下选项中不合法的赋值语句是( ) 。[C] A)c=(a=1,b=2); B)k=i=j; C)a=b+c=5; D)n1=(n2=(n3=0)); 11.若希望当 A 的值为奇数时,表达式的值为“真” ,A 的值为偶数时,表达式的值为“假” 。 则以下不能满足要求的表达式是( ) 。[C] A)A%2= =1 B) !(A %2 = =0) C)!(A%2) D)A%2 12.若 w=1,x=2,y=3,z=4,则条件表达式 w<x?x:y<z?y:z 的值是( ) 。[C] A)4 B)3 C)2 D)1 13.设 ch 是 char 型变量,其值为 A, 下面表达式的值为( ) 。[B] ch = ( ch >= ‘A’ && ch <= ‘Z’ ) ? ( ch + 32 ) : ch ; A)A B)a C)Z D)z 14.假定所有变量均已正确说明,下列程序运行后 x 的值是( ) 。[A] a=b=c=0, x=35; if (! a) x――; else if (b ); if ( c) x=3; else x=4;
大一c语言考试题及答案详解

大一c语言考试题及答案详解一、选择题(每题2分,共20分)1. 下列关于C语言的描述中,不正确的是()。
A. C语言是一种高级语言B. C语言可以直接被计算机执行C. C语言具有结构化的特点D. C语言支持指针答案:B2. C语言中,用于定义一个整型变量的关键字是()。
A. intB. floatC. charD. double答案:A3. 在C语言中,以下哪个选项不是运算符()。
A. %B. &&C. :D. <=答案:C4. 下面哪个函数是C语言标准库函数()。
A. main()B. printf()C. scanf()D. strcpy()5. C语言中,用于定义一个字符型数组的关键字是()。
A. intB. charC. floatD. double答案:B6. 在C语言中,以下哪个选项是正确的字符串定义方式()。
A. char str[] = "Hello";B. char str = "Hello";C. char str[] = {"Hello"};D. char str[] = 'Hello';答案:A7. 下列关于C语言函数的描述中,不正确的是()。
A. 函数可以没有返回值B. 函数可以有多个返回值C. 函数可以嵌套定义D. 函数可以递归调用答案:C8. 在C语言中,以下哪个选项是正确的文件包含指令()。
A. #include <stdio.h>B. #include "stdio.h"C. #include <stdio.h>D. #include "stdio.h"答案:A9. C语言中,用于定义一个浮点型变量的关键字是()。
B. floatC. charD. double答案:B10. 在C语言中,以下哪个选项是正确的条件语句()。
大学c语言作业题,大一c语言习题练习与答案.docx

⼤学c语⾔作业题,⼤⼀c语⾔习题练习与答案.docx ⼤⼀c语⾔习题练习与答案````程序设计与C语⾔1.1 选择题考点:标识符的命名规则只能由字母、数字、下划线构成数字不能作为标识符的开头关键字不能作为标识符选项A中的“-” ,选项B中“[”与“]”不满⾜(1);选项D中的int为关键字,不满⾜(3)1.下列正确的标识符是(C )。
A.-a1B.a[i]C.a2_iD.int t2.下列C语⾔⽤户标识符中合法的是( B )。
A)3ax B)x C)case D)-e2 E)union选项A中的标识符以数字开头不满⾜(2);选项C,E均为为关键字,不满⾜(3);选项D中的“-”不满⾜(1);3.下列四组选项中,正确的C语⾔标识符是( C )。
A) %x B) a+b C) a123 D) 123选项A中的“%” ,选项B中“+”不满⾜(1);选项D中的标识符以数字开头不满⾜(2)4.下列四组字符串中都可以⽤作C语⾔程序中的标识符的是( A )。
A、print _3d db8 aBcB、I\am one_half start$it 3paiC、str_1 Cpp pow whileD、Pxq My->book line# His.age选项B中的“\”,”$” ,选项D中“>”,”#”,”.”,”-”不满⾜(1);选项C中的while为关键字,不满⾜(3)知识点:简单C语⾔程序的构成和格式5.在⼀个C程序中___B___。
A、main函数必须出现在所有函数之前B、main函数可以在任何地⽅出现C、main函数必须出现在所有函数之后D、main函数必须出现在固定位置6.以下说法中正确的是__D____。
A、#define和printf都是C语句B、#define是C语句,⽽printf不是C、printf是C语句,但#define不是D、#define和printf都不是C语句7.⼀个C程序的执⾏是从( A )。
大一第一学期c语言考试题及答案

大一第一学期c语言考试题及答案一、选择题(每题2分,共20分)1. C语言中,以下哪个选项是合法的变量名?A. 2variableB. variable2C. variable-2D. variable$2答案:B2. 在C语言中,用于计算两个整数的和的运算符是?A. +=B. +=C. ||D. &&答案:A3. 下列哪个选项不是C语言中的控制结构?A. 顺序结构B. 选择结构C. 循环结构D. 递归结构答案:D4. C语言中,以下哪个函数用于输出字符串?A. printfB. scanfC. getcharD. putchar答案:A5. 在C语言中,以下哪个选项是正确的二维数组声明?A. int array[3][4];B. int array[][];C. int array[3][];D. int array;答案:A6. C语言中,以下哪个选项是正确的函数定义?A. int add(int a, b) { return a + b; }B. int add(int a, int b) { return a + b; }C. int add(a, b) { return a + b; }D. int add(int, int) { return a + b; }答案:B7. C语言中,以下哪个选项是正确的字符串声明?A. char str[] = "Hello";B. char str[6] = "Hello";C. char str = "Hello";D. char str[] = 'Hello';答案:A8. 在C语言中,以下哪个选项是正确的注释方式?A. // This is a commentB. /* This is a comment */C. //* This is a comment */D. /* This is a comment答案:A9. C语言中,以下哪个选项是正确的条件语句?A. if (x > 0) then { ... }B. if (x > 0) { ... }C. if x > 0 { ... }D. if (x > 0) then { ... }答案:B10. 在C语言中,以下哪个选项是正确的循环结构?A. for (int i = 0; i < 10; i++) { ... }B. for (int i = 0; i <= 10; i++) { ... }C. for (int i = 10; i > 0; i--) { ... }D. for (int i = 0; i > 10; i++) { ... }答案:A二、填空题(每题2分,共20分)1. C语言中,用于定义一个整型变量的关键字是________。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
编写程序:1.定义一个Point类来处理三维点points(x,y,z).该类有一默认的constructor,一copyconstructor, 一negate()成员函数将point的x,y和z值各乘-1, 一norm()成员函数返回该点到原点(0,0,0)的距离,一个print()成员函数显示x,y,和z的值。
#include<iostream>#include<cmath>using namespace std;class Point{private:double x,y,z;double distance;public:Point(double newX,double newY,double newZ){x=newX;y=newY;z=newZ;distance=sqrt(x*x+y*y+z*z);}Point(Point &p){x=p.x;y=p.y;z=p.z;distance=p.distance;}void negate(){x*=-1;y*=-1;z*=-1;}double norm(){return distance;}void print(){cout<<"x="<<x<<endl<<"y="<<y<<endl<<"z="<<z<<endl;}};void main(){Point dot(5,6,9);cout<<"the distance is: "<<dot.norm()<<endl;Point dot1(dot);cout<<"the distance is: "<<dot1.norm()<<endl;return;}2.定义一个Person类,它的每个对象表示一个人。
数据成员必须包含姓名、出生年份、死亡年份,一个默认的构造函数,一析构函数,读取数据的成员函数,一个print()成员函数显示所有数据。
#include<iostream>using namespace std;class Bdate{private:int year;int month;int day;public:Bdate(){}~Bdate(){cout<<"Destructor is called"<<endl;}void Bprint(){cout<<"Birthday="<<year<<"/"<<month<<"/"<<day<<endl;}void Binput(){cin>>year>>month>>day;}};//definition class Bdate;class Ddate{private:int year;int month;int day;public:Ddate(){}~Ddate(){cout<<"Destructor is called"<<endl;}void Dprint(){cout<<"Dead="<<year<<"/"<<month<<"/"<<day<<endl;}void Dinput(){cin>>year>>month>>day;}};//definition class Ddate;class Person{private:char name[10];Bdate birthday;Ddate dead;public:Person(){}~Person(){cout<<"Destructor is called"<<endl;}void print(){cout<<"name="<<name<<endl;birthday.Bprint();dead.Dprint();}void input(){cin>>name;birthday.Binput();dead.Dinput();}};void main(){Person per;per.input();per.print();return;}3。
定义一个Shape基类,由它派生出Rectanglr和Circle类,二者都有GetArea( )函数计算对象的面积。
使用Rectangle 类创建一个派生类Square。
class Shape{protected:public:double GetArea(){return 0;}};class Rectangle : public Shape{protected:double length;double width;public:Rectangle(){}Rectangle(double l, double w){length = l;width = w;}double GetArea(){return length * width;}};class Circle : public Shape{protected:double radius;public:Circle(double r){radius = r;}double GetArea(){return 3.1415926 * radius * radius;}};class Square : public Rectangle{protected:public:Square(double l) {length = width = l;}double GetArea(){return length * width;}};4. 定义一个Shape抽象类,由它派生出Rectanglr和Circle类,二者都有GetArea( )函数计算对象的面积,GetPerim( ) 函数计算对象的周长。
#include<iostream>#define PI 3.1415926;using namespace std;class Shape //抽象类的定义{public:virtual double GetArea() = 0; //纯虚函数virtual double GetPerim() = 0; //纯虚函数};class Rectangle : public Shape //矩形类,公有继承{public: Rectangle(double aa, double bb) //带参数的构造函数{a=aa;b=bb;cout<<"长"<<a<<"宽"<<b<<endl;}virtual double GetArea(){return a * b;}virtual double GetPerim(){return 2*( a + b );}private:double a;double b;};class Circle : public Shape //圆类,公有继承{public: Circle(double rr) //带参数的构造函数{r=rr;cout<<"半径"<<r<<endl;}virtual double GetArea(){return r * r * PI;}virtual double GetPerim(){return 2 * r * PI;}private:double r;};void main(){double length, width;cout << "输入长和宽: ";cin >> length >> width;Rectangle rect(length, width);cout << "面积是:"<< rect.GetArea() << endl<<"周长是:"<<rect.GetPerim()<<endl;double rr;cout << "输入半径: ";cin >> rr;Circle cir(rr);cout << "面积是:"<<cir.GetArea() << endl<<"周长是:"<<cir.GetPerim()<<endl; }。