三层架构实例
python三层架构实例
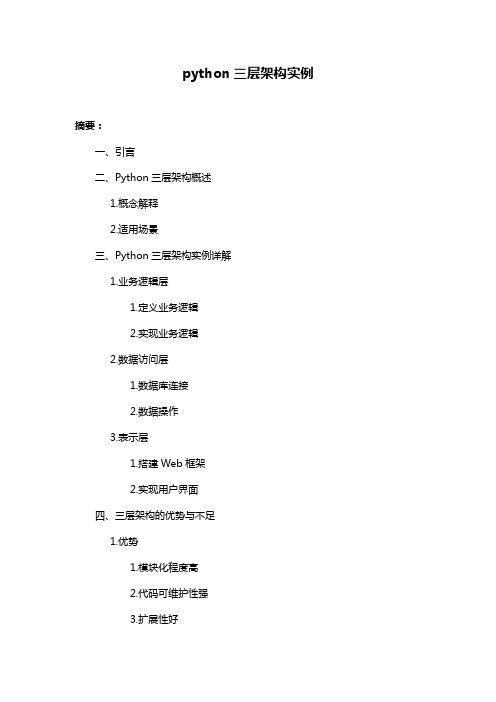
python三层架构实例摘要:一、引言二、Python三层架构概述1.概念解释2.适用场景三、Python三层架构实例详解1.业务逻辑层1.定义业务逻辑2.实现业务逻辑2.数据访问层1.数据库连接2.数据操作3.表示层1.搭建Web框架2.实现用户界面四、三层架构的优势与不足1.优势1.模块化程度高2.代码可维护性强3.扩展性好2.不足1.开发效率较低2.过度设计可能导致冗余代码五、总结与展望正文:一、引言在软件开发过程中,架构设计是至关重要的。
Python作为一种广泛应用于各个领域的编程语言,其三层架构模型在很多项目中都得到了很好的实践。
本文将为您详细介绍Python三层架构实例,帮助您更好地理解和应用这一架构模型。
二、Python三层架构概述1.概念解释Python三层架构,即分为业务逻辑层(Business Logic Layer,简称BLL)、数据访问层(Data Access Layer,简称DAL)和表示层(Presentation Layer,简称PL)。
这种架构模型将复杂的系统划分为三个相对独立的层次,各层之间通过接口进行通信,降低了模块间的耦合度,提高了代码的可维护性和扩展性。
2.适用场景Python三层架构适用于大多数面向对象的软件系统,尤其是业务逻辑复杂、数据访问频繁的项目。
这种架构有利于将业务逻辑与数据访问分离,提高了代码的可读性和可维护性。
三、Python三层架构实例详解1.业务逻辑层业务逻辑层主要负责处理应用程序的核心功能。
在这一层,我们需要定义业务规则、数据处理和业务流程。
以下是一个简单的业务逻辑层示例:```pythonclass UserService:def __init__(self):er_dao = UserDao()def register(self, username, password):user = er_dao.find_by_username(username)if not user:user = User(username, password)er_dao.add(user)return Truereturn Falsedef login(self, username, password):user = er_dao.find_by_username(username)if user and user.password == password:return Truereturn False```2.数据访问层数据访问层主要负责与数据库进行交互,实现数据的存取。
java三层架构实例

java三层架构实例摘要:一、Java 三层架构概述1.Java 三层架构的定义2.Java 三层架构的优势二、Java 三层架构实例1.实例简介2.实例分层说明a.界面层b.业务逻辑层c.数据持久层3.实例具体实现a.界面层的实现b.业务逻辑层的实现c.数据持久层的实现三、Java 三层架构的优缺点分析1.优点a.高内聚、低耦合b.提高代码可重用性和可移植性2.缺点a.降低系统性能b.可能导致级联修改正文:一、Java 三层架构概述Java 三层架构,即MVC(Model-View-Controller)架构,是一种常见的软件设计模式。
它将整个系统分为三个部分:界面层、业务逻辑层和数据持久层。
这种分层设计有助于实现高内聚、低耦合的目标,提高代码的可重用性和可移植性。
二、Java 三层架构实例1.实例简介本文将以一个简单的Java 三层架构实例为基础,介绍如何实现一个完整的Web 应用。
该实例包含一个用户注册功能,涉及Ajax、Json、Servlet、HTML、CSS 和JDBC 等技术。
2.实例分层说明(1)界面层(View):负责展示用户界面,接收用户输入,与用户进行交互。
(2)业务逻辑层(Controller):处理用户请求,调用相关业务逻辑,将数据传递给界面层或数据持久层。
(3)数据持久层(DAO):负责与数据库进行交互,存储和检索数据。
3.实例具体实现(1)界面层的实现首先,我们创建一个HTML 页面(regist.html),设置用户输入表单,包括用户名、密码、邮箱等。
接着,编写JavaScript 代码对用户输入进行非空检验、邮箱格式检验等。
最后,为表单添加点击事件,将用户输入的数据通过Ajax 技术传递给业务逻辑层。
(2)业务逻辑层的实现在业务逻辑层,我们编写一个CustomerServlet.java 文件,接收来自界面层的请求参数,并对数据进行封装。
然后,调用相关业务逻辑,如查询数据库,判断用户名和邮箱是否唯一等。
三层架构及实例演示

三层架构及实例演示简述通常意义上的三层架构就是将整个业务应用划分为:表现层(UI)、业务逻辑层(BLL)、数据访问(DAL)。
实例 登录界面这是一个经过改版的登录,视频里的用c#来实现的。
使用的工具:sql server 2010 、vs 2010建立数据库:Login 表:Scores、UsersScore表Users表LoginModel(实体层)创建实体层LoginModel,建立Userinfo类,存储从UI返回的数据。
这里我们称为业务实体层,因为他也是为业务逻辑服务的。
[vb] view plaincopyprint?Public Class UserInfoPrivate _ID As StringPrivate _Username As StringPrivate _Password As StringPrivate _Email As StringPublic Property ID As IntegerGetReturn _IDEnd GetSet(ByVal value As Integer)_ID = valueEnd SetEnd PropertyPublic Property Username As StringGetReturn _UsernameEnd GetSet(ByVal value As String)_Username = valueEnd SetEnd PropertyPublic Property Password As StringGetReturn _PasswordEnd GetSet(ByVal value As String)_Password = valueEnd SetEnd PropertyPublic Property Email As StringGetReturn _EmailEnd GetSet(ByVal value As String)_Email = valueEnd SetEnd PropertyEnd ClassLoginBLL(业务逻辑层)UI层调用业务逻辑层来执行登录的操作。
python三层架构实例

python三层架构实例以下是一个简单的Python三层架构实例:1. 数据层(Data Layer):负责与数据存储层进行交互,进行数据的存储和检索。
这可以是数据库、文件系统等。
```pythonclass UserRepository:def save(self, user):# 存储用户信息到数据库def get_user_by_id(self, user_id):# 通过用户ID从数据库中获取用户信息```2. 业务逻辑层(Business Logic Layer):负责处理业务逻辑,对数据进行处理和组织,提供给上层UI层使用。
```pythonclass UserService:def __init__(self):er_repository = UserRepository()def create_user(self, name, age):user = User(name, age)er_repository.save(user)def get_user(self, user_id):return er_repository.get_user_by_id(user_id)```3. 用户界面层(User Interface Layer):负责与用户进行交互,包括接收用户输入和展示输出结果。
```pythonclass UserInterface:def __init__(self):er_service = UserService()def create_user(self):name = input("请输入用户名:")age = int(input("请输入年龄:"))er_service.create_user(name, age)def get_user(self):user_id = int(input("请输入用户ID:"))user = er_service.get_user(user_id)print("用户信息:", , user.age)```在这个实例中,数据层负责用户信息的存储和检索,业务逻辑层负责处理业务逻辑,用户界面层负责与用户进行交互。
java三层架构实例 -回复
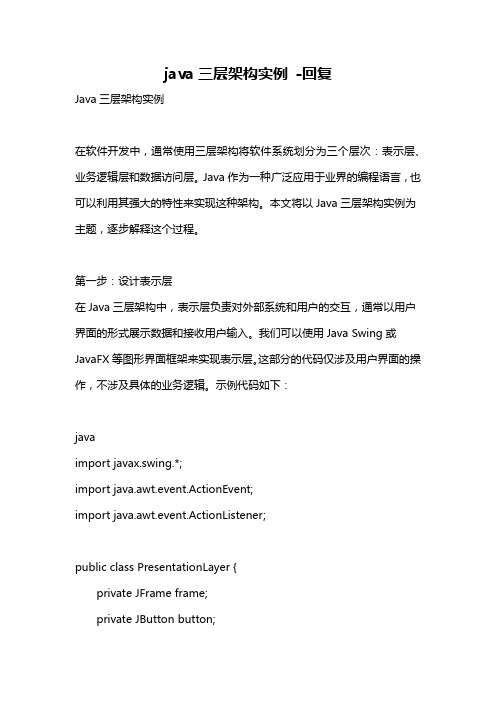
java三层架构实例-回复Java三层架构实例在软件开发中,通常使用三层架构将软件系统划分为三个层次:表示层、业务逻辑层和数据访问层。
Java作为一种广泛应用于业界的编程语言,也可以利用其强大的特性来实现这种架构。
本文将以Java三层架构实例为主题,逐步解释这个过程。
第一步:设计表示层在Java三层架构中,表示层负责对外部系统和用户的交互,通常以用户界面的形式展示数据和接收用户输入。
我们可以使用Java Swing或JavaFX等图形界面框架来实现表示层。
这部分的代码仅涉及用户界面的操作,不涉及具体的业务逻辑。
示例代码如下:javaimport javax.swing.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;public class PresentationLayer {private JFrame frame;private JButton button;private JLabel label;public PresentationLayer() {frame = new JFrame("Java三层架构示例");button = new JButton("查询");label = new JLabel();button.addActionListener(new ActionListener() {Overridepublic void actionPerformed(ActionEvent e) {String result = BusinessLayer.queryData();label.setText(result);}});frame.add(button);frame.add(label);frame.setLayout(new FlowLayout());frame.setSize(300, 200);frame.setLocationRelativeTo(null);frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);frame.setVisible(true);}}第二步:设计业务逻辑层在Java三层架构中,业务逻辑层负责处理表示层传递过来的数据,进行相应的数据处理和业务逻辑操作。
三层架构简易实例详解
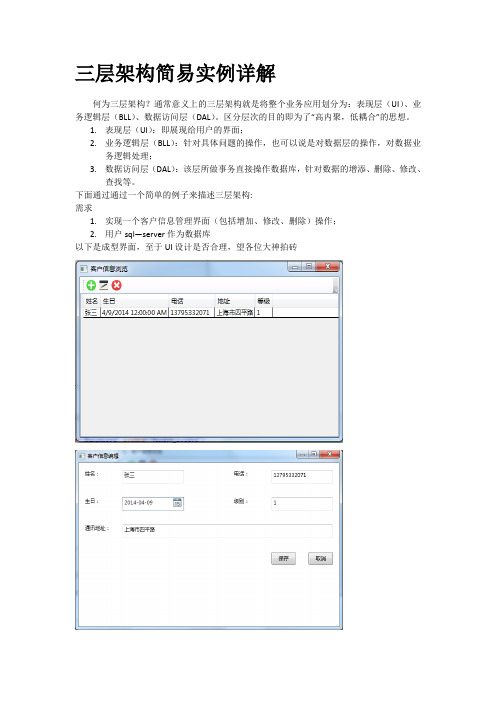
三层架构简易实例详解何为三层架构?通常意义上的三层架构就是将整个业务应用划分为:表现层(UI)、业务逻辑层(BLL)、数据访问层(DAL)。
区分层次的目的即为了“高内聚,低耦合”的思想。
1.表现层(UI):即展现给用户的界面;2.业务逻辑层(BLL):针对具体问题的操作,也可以说是对数据层的操作,对数据业务逻辑处理;3.数据访问层(DAL):该层所做事务直接操作数据库,针对数据的增添、删除、修改、查找等。
下面通过通过一个简单的例子来描述三层架构:需求1.实现一个客户信息管理界面(包括增加、修改、删除)操作;2.用户sql—server作为数据库以下是成型界面,至于UI设计是否合理,望各位大神拍砖UI层设计设计器代码:<Grid><DockPanel><ToolBar DockPanel.Dock="Top" Height="30"><Button Name="BtnAdd" Click="BtnAdd_Click" ToolTip="新增"><Image Source="Image\add.ico"></Image></Button><Button Name="BtnEdit" Click="BtnEdit_Click" ToolTip="编辑"><Image Source="Image\edit.ico"></Image></Button><Button Name="BtnDel" Click="BtnDel_Click" ToolTip="删除"><Image Source="Image\delete.ico"></Image></Button></ToolBar><DataGrid Name="DataGrid1" DockPanel.Dock="Top" IsReadOnly="True" AutoGenerateColumns="False"><DataGrid.Columns><DataGridTextColumn Header="姓名" Binding="{Binding Name}"></DataGridTextColumn><DataGridTextColumn Header="生日" Binding="{Binding BirthDay}"></DataGridTextColumn><DataGridTextColumn Header="电话" Binding="{Binding TelNum}"></DataGridTextColumn><DataGridTextColumn Header="地址" Binding="{Binding Address}"></DataGridTextColumn><DataGridTextColumn Header="等级" Binding="{BindingCustlevel}"></DataGridTextColumn></DataGrid.Columns></DataGrid></DockPanel></Grid>主要是通过设计器后台代码,DataGrid控件绑定数据,代码如下:/// <summary>/// 初始化加载数据/// </summary>private void LoadData(){DataGrid1.ItemsSource = CustomDAL.GetAll();}数据业务逻辑代码如下:/// <summary>/// 新增/// </summary>/// <param name="sender"></param>/// <param name="e"></param>private void BtnAdd_Click(object sender, RoutedEventArgs e){CustomEditUI editUI = new CustomEditUI();editUI.isInsert = true;if (editUI.ShowDialog() == true){LoadData();}}/// <summary>/// 编辑/// </summary>/// <param name="sender"></param>/// <param name="e"></param>private void BtnEdit_Click(object sender, RoutedEventArgs e){Custom customer = (Custom)DataGrid1.SelectedItem;if (customer == null){MessageBox.Show("请选择要编辑的行!");return;}CustomEditUI editUI = new CustomEditUI();//添加标识便于区分是编辑保存还是新增保存editUI.isInsert = false;editUI.editId = customer.Id;if (editUI.ShowDialog() == true){LoadData();}}/// <summary>/// 删除/// </summary>/// <param name="sender"></param>/// <param name="e"></param>private void BtnDel_Click(object sender, RoutedEventArgs e){if (MessageBox.Show("确认删除此条数据?", "提醒", MessageBoxButton.YesNo) == MessageBoxResult.Yes){Custom custom = DataGrid1.SelectedItem as Custom;if (custom != null){if ((MessageBox.Show("确认要删除此调数据", "提醒") == MessageBoxResult.OK)){CustomDAL.Delete(custom.Id);LoadData();}}else{MessageBox.Show("请选择要删除的数据", "提醒");return;}}}数据访问层(DAL),代码如下:public class CustomDAL{/// <summary>/// 查询数据总条数/// </summary>/// <returns></returns>public static int GetCount(){return (int)SqlHelper.ExecuteScalar("select * from T_Custom");}/// <summary>///根据ID查询数据/// </summary>/// <param name="id"></param>/// <returns></returns>public static Custom GetByid(long id){DataTable table = SqlHelper.ExecuteDataTable("select * from T_custom where id=@id", new SqlParameter("@id", id));return ToCustom(table.Rows[0]); ;}/// <summary>/// 根据id删除数据/// </summary>/// <param name="id"></param>public static void Delete(long id){SqlHelper.ExecuteNonQuery("delete from T_custom where id=@id", new SqlParameter("@id", id));}/// <summary>/// 插入空值处理/// </summary>/// <param name="value"></param>/// <returns></returns>public static object FromDBNull(object value){if (value == null){return DBNull.Value;}else{return value;}}/// <summary>/// 查询空值处理/// </summary>/// <param name="value"></param>/// <returns></returns>public static object ToDBNull(object value){if (DBNull.Value == null){return null;}else{return value;}}/// <summary>/// 新增数据/// </summary>/// <param name="custom"></param>public static void InSert(Custom custom){SqlHelper.ExecuteNonQuery(@"insert into T_Custom(Name,Birthday,Address,Telnum,Custlevel)values(@Name,@Birthday,@Address,@Teln um,@Custlevel)",new SqlParameter("@Name", ),new SqlParameter("@Birthday", FromDBNull(custom.BirthDay)),new SqlParameter("@Address", custom.Address),new SqlParameter("@Telnum", custom.TelNum),new SqlParameter("@Custlevel", custom.Custlevel));}/// <summary>/// 更新数据/// </summary>/// <param name="custom"></param>public static void UpDate(Custom custom){SqlHelper.ExecuteNonQuery(@"update T_Custom set Name=@Name,Birthday=@Birthday,Address=@Address,Telnum=@Telnum,Custlevel=@Custlevel where id=@id",new SqlParameter("@Name", ),new SqlParameter("@Birthday", FromDBNull(custom.BirthDay)),new SqlParameter("@Address", custom.Address),new SqlParameter("@TelNum", custom.TelNum),new SqlParameter("@Custlevel", custom.Custlevel),new SqlParameter("@Id", custom.Id));}/// <summary>/// 查询所有数据/// </summary>/// <returns></returns>public static List<Custom> GetAll(){DataTable table = SqlHelper.ExecuteDataTable("select * from T_custom");List<Custom> lst = new List<Custom>();for (int i = 0; i < table.Rows.Count; i++){lst.Add(ToCustom(table.Rows[i]));}return lst;}/// <summary>/// 影射关系赋值/// </summary>/// <param name="row"></param>/// <returns></returns>public static Custom ToCustom(DataRow row){Custom custom = new Custom(); = row["Name"].ToString();custom.Address = row["Address"].ToString();custom.BirthDay = (DateTime?)ToDBNull(row["BirthDay"]);custom.TelNum = row["TelNum"].ToString();custom.Custlevel = (int)row["CUstlevel"];custom.Id = (long)row["id"];return custom;}}public class SqlHelper{private static string connstr = ConfigurationManager.ConnectionStrings["conn"].ConnectionString;/// <summary>/// 查询数据/// </summary>/// <param name="sql"></param>/// <param name="parameters"></param>/// <returns></returns>public static Object ExecuteScalar(string sql, params SqlParameter[] parameters){using (SqlConnection cnn = new SqlConnection(connstr)){cnn.Open();using (SqlCommand cmd = cnn.CreateCommand()){mandText = sql;cmd.Parameters.AddRange(parameters);return cmd.ExecuteScalar();}}}/// <summary>/// 增、删、改操作/// </summary>/// <param name="sql"></param>/// <param name="parameters"></param>public static void ExecuteNonQuery(string sql, params SqlParameter[] parameters){using (SqlConnection cnn = new SqlConnection(connstr)){cnn.Open();using (SqlCommand cmd = cnn.CreateCommand()){mandText = sql;cmd.Parameters.AddRange(parameters);cmd.ExecuteNonQuery();}}}/// <summary>/// 单个查询结果返回/// </summary>/// <param name="sql"></param>/// <param name="parameters"></param>/// <returns></returns>public static DataTable ExecuteDataTable(string sql, params SqlParameter[] parameters){using (SqlConnection cnn = new SqlConnection(connstr)){cnn.Open();using (SqlCommand cmd = cnn.CreateCommand()){mandText = sql;cmd.Parameters.AddRange(parameters);SqlDataAdapter apter = new SqlDataAdapter(cmd);DataSet dataset = new DataSet();apter.Fill(dataset);return dataset.Tables[0];}}}}业务模型层public class Custom{public long Id { set; get; }public string Name { set; get; }public DateTime? BirthDay { set; get; }public string Address { set; get; }public string TelNum { set; get; }public int Custlevel { set; get; }}业务模型层public class Custom{public long Id { set; get; }public string Name { set; get; }public DateTime? BirthDay { set; get; }public string Address { set; get; }public string TelNum { set; get; }public int Custlevel { set; get; } }上述用思想,图形表示如下:。
三层架构简易实例详解

三层架构简易实例详解三层架构是一种软件设计模式,它将软件系统分为三个层次:表现层、业务逻辑层和数据访问层。
每个层次都有特定的职责,通过分层的方式提高了系统的可维护性、可扩展性和可复用性。
以下是一个简单的示例来解释三层架构的概念:1. 表现层(Presentation Layer):这是用户与系统交互的界面。
它负责接收用户的输入、展示数据和呈现界面效果。
可以使用 Web 页面、桌面应用程序或移动应用程序等来实现。
2. 业务逻辑层(Business Logic Layer):该层处理系统的核心业务逻辑。
它接收来自表现层的请求,执行相应的业务规则和计算,并与数据访问层进行交互以获取和保存数据。
3. 数据访问层(Data Access Layer):这一层负责与数据库或其他数据源进行交互。
它封装了数据的读取、写入、修改和查询操作,提供了一个统一的数据访问接口。
以下是一个简单的示例,以在线书店为例:1. 表现层:用户通过网站或移动应用程序浏览图书列表、查看图书详细信息、添加到购物车和进行结算。
2. 业务逻辑层:处理用户的请求,例如检查购物车中的图书数量、计算价格、应用折扣等。
它还负责与数据访问层交互以获取图书信息和保存用户的订单。
3. 数据访问层:与数据库进行交互,执行图书的查询、插入、更新和删除操作。
通过将系统划分为三层,每层专注于特定的职责,可以提高代码的可维护性和可复用性。
当需求发生变化或需要进行系统扩展时,只需修改相应层次的代码,而不会影响其他层次。
这种分层的架构也有助于团队协作和开发效率。
请注意,这只是一个简单的示例,实际的三层架构应用可能会更加复杂,并涉及更多的模块和技术。
具体的实现方式会根据项目的需求和规模而有所不同。
三层架构程序设计实例

三层架构C/S程序设计实例(C#描述)1.三层之间的关系:三层是指:界面显示层(UI),业务逻辑层(Business),数据操作层(Data Access)文字描述:Clients对UI进行操作,UI调用Business进行相应的运算和处理,Business通过Data Access对Data Base进行操作。
优点:l 增加了代码的重用。
Data Access可在多个项目中公用;Business可在同一项目的不同地方使用(如某个软件B/S和C/S部分可以共用一系列的Business组件)。
l 使得软件的分层更加明晰,便于开发和维护。
美工人员可以很方便地设计UI设计,并在其中调用Business给出的接口,而程序开发人员则可以专注的进行代码的编写和功能的实现。
2.Data Access的具体实现:DataAgent类型中变量和方法的说明:private string m_strConnectionString; //连接字符串private OleDbConnection m_objConnection; //数据库连接public DataAgent(string strConnection) //构造方法,传入的参数为连接字符串private void OpenDataBase() //打开数据库连接private void #region CloseDataBase() //关闭数据库连接public DataView GetDataView(string strSqlStat) //根据传入的连接字符串返回DataView具体实现代码如下:public class DataAgent{private string m_strConnectionString;private OleDbConnection m_objConnection;#region DataAgend///<summary>/// Initial Function///</summary>///<param name="strConnection"></param>public DataAgent(string strConnection){this.m_strConnectionString = strConnection;}#endregion#region OpenDataBase///<summary>/// Open Database///</summary>private void OpenDataBase(){try{this.m_objConnection = new OleDbConnection();this.m_objConnection.ConnectionString = this.m_strConnectionString;if (this.m_objConnection.State != ConnectionState.Open){this.m_objConnection.Open();}}catch (Exception e){throw e;}}#endregion#region CloseDataBase///<summary>/// Close Database///</summary>private void CloseDataBase(){if (this.m_objConnection != null){if (this.m_objConnection.State == ConnectionState.Open){this.m_objConnection.Close();}}}#endregion#region GetDataView///<summary>/// Execute the sql and return the default table view///</summary>///<param name="strSelectString">Select String</param>///<returns>DataView of the DataTable</returns>public DataView GetDataView(string strSqlStat){try{this.OpenDataBase();OleDbDataAdapter objDataAdapter= new OleDbDataAdapter(strSqlStat.Trim(), this.m_objConnection);DataSet objDataSet = new DataSet();objDataAdapter.Fill(objDataSet);return objDataSet.Tables[0].DefaultView;}catch (Exception e){throw e;}finally{this.CloseDataBase();}}#endregion}3.Business的具体实现:建立名为Base的类,此类作为其他事务类的基类,其中定义了一个DataAgent的实例。
三层架构实例

三层架构实例4、三层架构下的包图:注:Entity中放的都是实体类,即由数据库中的表抽象出来的类。
实体类主要作为数据的载体,在各个层之间被传递。
我们现在要做的就是对⽤例图中所⽰的这个功能进⾏抽象,即分别在UI,BLL,DAL三层抽象类。
数据访问层DAL:数据库中的⼀张表对应DAL层的⼀个类,所以这⾥要有⼀个dal_DealStudentInfo类,它必然有⼀个⽅法AddRecord,⽤于向数据库中添加数据。
这个⽅法的参数便是studentInfo这张表映射出的⼀个实体类的⼀个实例,⽅法中包含了⼀些SQL语句。
IsExist是验证数据有效性,这⾥主要是检验⽤户是否已经存在。
图⽰:关键代码如下:Public Class dal_DealStudentInfoPublic Function AddRecord(ByVal RecInfo As Student) As Boolean'……'连接数据库'……'……Try'SQL语句向数据库中写⼊数据'……Return TrueCatchReturn FalseFinally'关闭数据库End TryEnd FunctionPublic Function IsExist() As Boolean'查询数据库,如果存在该卡,则返回True,不存在则返回falseIf '存在Return TrueElseReturn FalseEnd IfEnd FunctionEnd Class业务逻辑层BLL:这⾥会有⼀个类bll_ControlStudent与DAL层中的dal_DealStudentInfo类对应,调⽤dal_DealStudentInfo的AddRecord⽅法,这个⽅法传递的参数就是UI层传递过来的studentInfo实体类的⼀个实例。
如果需要验证输⼊合法性,也放在bll_ControlStudent中,并与dal_DealStudentInfo中的⽅法相对应,可以单独⽤⼀个⽅法,也可以集成到AddStudent中,我这⾥因为只是进⾏了简单的判断,就把它放到了AddStudent。
MVC三层架构学习总结实例

MVC三层架构学习总结实例⼀个简单的转账Servlet Demo使⽤MVC三层架构实现前端<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8"><title>Title</title><style>#mainApp{font-size: 20px;font-family: "Microsoft YaHei UI",serif;text-align: center;margin-top: 200px;font-weight: bold;}</style></head><body><div id="mainApp"><form action="servlet/transfer" method="post"><p><label for="transOut">请填写转出⽤户名:</label><input type="text" id="transOut" name="transOut" required /></p> <p><label for="transIn">请填写转⼊⽤户名:</label><input type="text" id="transIn" name="transIn" required /></p><p><label for="money">请填写转账数⽬:</label><input type="text" id="money" name="money" required /></p><p><input type="submit"><input type="reset"></p></form></div></body></html>后端数据库⼯具类保证数据库调⽤的统⼀public class C3P0Utils {/*** 获取连接池* @return 返回c3p0默认连接池*/public static DataSource getDataSource(){return new ComboPooledDataSource();}/*** 获取连接* @return 返回⼀个基于c3p0连接池的连接*/public static Connection getConnection(){try {return getDataSource().getConnection();} catch (SQLException e){throw new RuntimeException("⽆法获取连接,请检查数据库配置⽂件");}}/*** 实现资源的释放* 细节在于⾸先是对于顺序的先开后关* 对于每个对象都要有try...catch保证哪怕报错了其他的对象也可以关闭* @param connection 数据库连接* @param ps 预编译sql对象* @param resultSet 数据库结果集*/public static void release(Connection connection, PreparedStatement ps, ResultSet resultSet){try {if (resultSet != null){resultSet.close();}} catch (SQLException throwables) {throwables.printStackTrace();}try {if (ps != null){ps.close();}} catch (SQLException throwables) {throwables.printStackTrace();}try {if (connection != null){connection.close();}} catch (SQLException throwables) {throwables.printStackTrace();}}}model⽤于封装数据库对象public class User {private String name;private BigDecimal money;public User() {}public User(String name, BigDecimal money) { = name;this.money = money;}public String getName() {return name;}public void setName(String name) { = name;}public BigDecimal getMoney() {return money;}public void setMoney(BigDecimal money) {this.money = money;}}Dao层⽤于操作数据库/*** 此接⼝规定对User数据库的操作* @author Rainful* @create 2021/07/29*/public interface UserDao {/*** 通过name查询⽤户* @param connection 数据库连接* @param ps 预编译sql对象* @param sql sql语句* @param name ⽤户名* @param money 钱数* @throws SQLException 抛出⼀个查询错误让业务代码回滚* @return 返回⼀个可以查找到的⽤户*/int moneyTransfer(Connection connection, PreparedStatement ps,String sql, String name, double money) throws SQLException;}public class UserDaoImpl implements UserDao {@Overridepublic int moneyTransfer(Connection connection, PreparedStatement ps,String sql, String name, double money) throws SQLException { ps = connection.prepareStatement(sql);ps.setDouble(1, money);ps.setString(2, name);return ps.executeUpdate();}}业务层⽤于调⽤Dao层验证从控制层传来的参数等/*** 此接⼝⽤于规范数据库查询* @author Rainful* @create 2021/07/29*/public interface UserServlet {/*** 业务层调⽤dao层完成数据库更新及控制事务* @param name1 转出账户⽤户名* @param name2 转⼊账户⽤户名* @param money 修改* @return 修改结果*/boolean moneyTransfer(String name1, String name2, double money);}public class UserServletImpl implements UserServlet {private final UserDao userDao;public UserServletImpl() {erDao = new UserDaoImpl();}@Overridepublic boolean moneyTransfer(String name1, String name2, double money) {Connection connection = null;PreparedStatement ps = null;try {connection = C3P0Utils.getConnection();// 开启事务connection.setAutoCommit(false);// 转出账户int transOutRow = transfer(connection, ps, name1, money, -1);// 转⼊账户int transInRow = transfer(connection, ps, name2, money, 1);// 提交事务mit();return transOutRow > 0 && transInRow > 0;} catch (Exception e) {// 发⽣异常进⾏回滚处理try {connection.rollback();} catch (SQLException throwables) {throwables.printStackTrace();}e.printStackTrace();return false;} finally {// 关闭数据库连接try {connection.setAutoCommit(true);} catch (SQLException throwables) {throwables.printStackTrace();}C3P0Utils.release(connection, ps, null);}}/*** 返回转账sql的影响⾏数** @param connection 数据库连接* @param ps 预编译sql对象* @param name 账户更改姓名* @param money 更改的钱数* @param value 为了保证⽅法共⽤性⽽设置的修改参数* 转⼊为 1, 转出为 -1* @return 返回影响的⾏数* @throws SQLException 抛出sql异常回滚*/private int transfer(Connection connection, PreparedStatement ps, String name, double money, int value) throws SQLException { // 转出账户的话因为钱是减少的String sql = "update account set money = money + ? where name = ?";return userDao.moneyTransfer(connection, ps, sql, name, money * value);}}控制层⽤于接收前端数据传输给业务层做逻辑判断@WebServlet("/servlet/transfer")public class TransferMoney extends HttpServlet {@Overrideprotected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {String transOut = req.getParameter("transOut");String transIn = req.getParameter("transIn");String money = req.getParameter("money");// 三者都在前端进⾏了⾮空判断// 后续如果再加上判断就好了,因为要防⽌前端被⼈恶意修改传输数据过来long moneyNum;System.out.println(money);//System.out.println(Long.parseLong(money));try {moneyNum = Long.parseLong(money);} catch (Exception e){resp.getWriter().print("⾦额不符合规范");return;}// 调⽤业务层进⾏处理UserServlet userServlet = new UserServletImpl();boolean flag = userServlet.moneyTransfer(transOut, transIn, moneyNum);if (flag){resp.getWriter().print("转账成功");} else {resp.getWriter().print("转账失败");}}@Overrideprotected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {doGet(req, resp);}}其他编码转换过滤@WebFilter("/servlet/*")public class CodeChange implements Filter {@Overridepublic void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException {request.setCharacterEncoding("utf-8");response.setCharacterEncoding("utf-8");response.setContentType("text/html;charset=utf-8");chain.doFilter(request, response);}}权限管理过滤防⽌恶意直接访问servlet@WebFilter("/servlet/*")public class FilterServlet implements Filter {@Overridepublic void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException {String money = request.getParameter("money");if (money == null){((HttpServletResponse)response).sendRedirect("../index.html");}chain.doFilter(request, response);}@Overridepublic void init(FilterConfig filterConfig) throws ServletException {}@Overridepublic void destroy() {}}总结bug反思整体来说⼀次性写完,但是在数据库调⽤的时候发现数据库没有修改,经过单元测试排除⽅法最后发现调⽤sql的时候参数传递问题整个servlet优点完成了全部功能,实现了sql调⽤的时候connection复⽤,对⼀次业务进⾏connection的统⼀关闭sql调⽤的时候进⾏参数传递到dao层可以⼀个⽅法完成增加和减少实现了MVC三层架构,并且使⽤接⼝实现多态,并且规范了实现类的⾏为实现了编码转换及权限过滤优化⽅向后续增加业务的时候,可以抽取sql代码完成sqlUtils类的封装规范Dao层的sql调⽤增加常⽤变量的时候可以进⾏⼀个静态变量⼯具类的封装等。
java三层架构实例

java三层架构实例摘要:1.三层架构概述2.Java三层架构实例详解3.三层架构的优缺点4.总结正文:一、三层架构概述三层架构(3-Tier Architecture)是一种常见的软件架构模式,它将系统分为三个层次:显示层、业务逻辑层和数据层。
这种架构模式使得系统具有高内聚、低耦合的特点,提高了程序的可重用性和可移植性。
在Java开发中,显示层通常使用HTML、CSS和JavaScript等技术,业务逻辑层使用JavaBean、Servlet等技术,数据层则使用JDBC等数据库操作技术。
二、Java三层架构实例详解1.显示层(View):编写注册界面,用户可以通过界面输入用户名、密码和邮箱等信息。
2.业务逻辑层(Controller):编写CustomerServlet.java,接收请求参数并封装,保证用户名的唯一性,调用Service层将用户信息添加到数据库。
3.数据层(Model):编写User实体类,表示用户的信息。
4.数据库(Database):使用JDBC技术,实现用户信息的增删查改。
三、三层架构的优缺点优点:1.高内聚、低耦合:每个层次职责明确,相互独立,便于维护和扩展。
2.可重用性和可移植性:各层次可以根据需求独立更换,提高系统的灵活性。
缺点:1.系统性能稍低:由于分层处理,可能导致请求处理的效率降低。
2.级联修改:当某个层次发生变化时,可能需要修改其他层次,增加了维护难度。
四、总结Java三层架构是一种理想的软件开发模式,它使得系统具有更好的可维护性、可扩展性和灵活性。
通过具体的实例,我们可以更深入地理解三层架构的实现方式和注意事项。
C#三层架构 简单实例分析.

基于3层架构的课程管理系统本模块工作任务任务3-1:三层架构划分任务3-2:数据访问层的实现任务3-3:业务逻辑层的实现 任务3-4:表示层的实现本模块学习目标1、掌握三层架构的划分原理2、掌握各层的设计思路,和层之间的调用关系3、利用三层架构实现对课程管理模块的重构4、巩固OOP 的基本概念和 OOP 的编程思路---------------------------------------------------------------------------------------------------------------------------------任务3-1:三层架构划分效果与描述图3.1 包含多个项目的3层架构解决方案本任务要求学生能够将原来的只有1个项目的课程管理模块,重构为标准的具有5个项目的3层架构的模块,并进行恰当的初始化,仍能实现课程记录的添加、浏览功能。
在此过程中理解3层架构的划分原理,各层的任务,层之间的调用关系。
本任务的业务流程: 将原项目改为UI 层新建BLL/ DAL/COMMON/MODL 项目并初始化初始化后仍能实现课程记录的浏览和添加业务逻辑层数据访问层界面层图3.2 单层转化为3层架构的业务流程相关知识与技能3-1-1 三层架构的划分原理三层架构的划分如下图:图3.3 三层架构原理图1、各层的任务数据访问层:使用中的数据操作类,为数据库中的每个表,设计1个数据访问类。
类中实现:记录的插入、删除、单条记录的查询、记录集的查询、单条记录的有无判断等基本的数据操作方法。
对于一般的管理信息软件,此层的设计是类似的,包含的方法也基本相同。
此层的任务是:封装每个数据表的基本记录操作,为实现业务逻辑提供数据库访问基础。
业务逻辑层:为用户的每个功能模块,设计1个业务逻辑类,此时,需要利用相关的数据访问层类中,记录操作方法的特定集合,来实现每个逻辑功能。
界面层:根据用户的具体需求,为每个功能模块,部署输入控件、操作控件和输出控件,并调用业务逻辑层中类的方法实现功能。
三层架构简单示例

1、表现层(UI):通俗讲就是展现给用户的界面,即用户在使用一个系统的时候他的所见所得。
2、业务逻辑层(BLL):针对具体问题的操作,也可以说是对数据层的操作,对数据业务逻辑处理。
3、数据访问层(DAL):该层所做事务直接操作数据库,针对数据的增添、删除、修改、更新、查找等。
下面就介绍一下范例的步骤:1.打开VS2008后,文件-->新建-->项目-->其他项目类型-->Visual Studio 解决方案-->空白解决方案,起名为:MvcTest。
2.建立如图的项目,并在WebApplication1-->App_Data建一个数据文件DabaBase.mdf,里面建表:qzzm_user 表内:字段Name,类型:nvarchar(50) 非空。
3.在WEB中引用BLL,Model层新建Post.aspxPost.aspx 代码如下:<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Post.aspx.cs" Inherits="Post" %><!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "/TR/xhtml1/DTD/xhtml1-transitional.dtd"><html xmlns="/1999/xhtml"><head runat="server"><title>无标题页</title></head><body><form id="form1" runat="server"><div><asp:TextBox ID="tb_name" runat="server"></asp:T extBox><asp:Button ID="btn_post" runat="server" OnClick="btn_post_Click" Text="提交" /></div></form></body></html>Post.aspx.cs 先搁下等写好类库再写。
三层架构实例

这里以查询数据库中student表的所有信息为例:1、模型层,Student.cs文件:using System;using System.Collections.Generic;using System.Linq;using System.Text;namespace SchoolModels{public class Student{public Student(){ }public Student(int id, string name, string pwd, int age, string sex) {this.StudentId = id;this.StudentName = name;this.StudentPwd = pwd;}private int studentId;public int StudentId{get { return studentId; }set { studentId = value; }}private string studentName;public string StudentName{get { return studentName; }set { studentName = value; }}private string studentPwd;public string StudentPwd{get { return studentPwd; }set { studentPwd = value; }}}}2、数据访问层,StudentService.cs文件:using System.Text;using System.Data;using System.Data.SqlClient;using SchoolModels;namespace SchoolDal{public class StudentService{public static IList<Student> GetAllStudents(){List<Student> stus = new List<Student>();string sql = "select * from student";SqlCommand cmd = new SqlCommand(sql, DBHelper.con);DBHelper.con.Open();SqlDataReader reader = cmd.ExecuteReader();while (reader.Read()){Student student = new Student();student.StudentId = (int)reader["StudentId"];student.StudentName = reader["StudentName"].ToString();student.StudentPwd = reader["StudentPwd"].ToString();stus.Add(student);}reader.Close();DBHelper.con.Close();return stus;}}其中,里面有一个DBHelper.cs文件:using System;using System.Collections.Generic;using System.Linq;using System.Text;using System.Configuration;using SchoolModels;using System.Data;using System.Data.SqlClient;namespace SchoolDal{public class DBHelper{public static string conStr = ConfigurationManager.ConnectionStrings["conDb"].ToString();public static SqlConnection con = new SqlConnection(conStr); }}3、业务逻辑层,StudentManager.cs文件:using System;using System.Collections.Generic;using System.Linq;using System.Text;using SchoolDal;using SchoolModels;namespace SchoolBll{public class StudentManager{public static IList<Student> GetAllStudents(){return StudentService.GetAllStudents();}}}最后,假设在Form1里面把所有学生的信息显示到DataGridView中,Form1.cs 文件:using System;using System.Collections.Generic;using ponentModel;using System.Data;using System.Drawing;using System.Linq;using System.Text;using System.Windows.Forms;using SchoolBll;using SchoolModels;namespace School{public partial class Form1 : Form{public Form1(){InitializeComponent();}private void Form1_Load(object sender, EventArgs e){IList<Student> stus = StudentManager.GetAllStudents();this.dgvStudent.DataSource = stus;}}以上就是通过三层架构做的一个查询数据库某一张表所有信息的小例子,你可以根据具体的需求进行修改。
javaweb三层架构实例
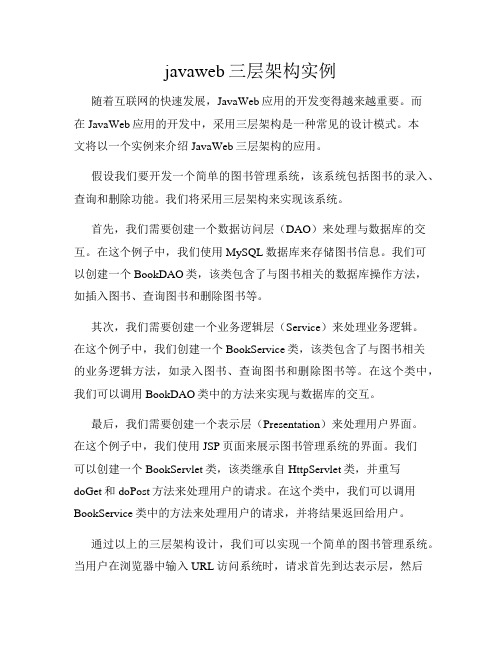
javaweb三层架构实例随着互联网的快速发展,JavaWeb应用的开发变得越来越重要。
而在JavaWeb应用的开发中,采用三层架构是一种常见的设计模式。
本文将以一个实例来介绍JavaWeb三层架构的应用。
假设我们要开发一个简单的图书管理系统,该系统包括图书的录入、查询和删除功能。
我们将采用三层架构来实现该系统。
首先,我们需要创建一个数据访问层(DAO)来处理与数据库的交互。
在这个例子中,我们使用MySQL数据库来存储图书信息。
我们可以创建一个BookDAO类,该类包含了与图书相关的数据库操作方法,如插入图书、查询图书和删除图书等。
其次,我们需要创建一个业务逻辑层(Service)来处理业务逻辑。
在这个例子中,我们创建一个BookService类,该类包含了与图书相关的业务逻辑方法,如录入图书、查询图书和删除图书等。
在这个类中,我们可以调用BookDAO类中的方法来实现与数据库的交互。
最后,我们需要创建一个表示层(Presentation)来处理用户界面。
在这个例子中,我们使用JSP页面来展示图书管理系统的界面。
我们可以创建一个BookServlet类,该类继承自HttpServlet类,并重写doGet和doPost方法来处理用户的请求。
在这个类中,我们可以调用BookService类中的方法来处理用户的请求,并将结果返回给用户。
通过以上的三层架构设计,我们可以实现一个简单的图书管理系统。
当用户在浏览器中输入URL访问系统时,请求首先到达表示层,然后表示层调用业务逻辑层的方法来处理请求,最后业务逻辑层调用数据访问层的方法来与数据库交互。
最终,数据从数据库返回到业务逻辑层,再返回到表示层,最终展示给用户。
三层架构的设计模式使得系统的各个模块之间的耦合度降低,提高了系统的可维护性和可扩展性。
例如,如果我们要增加一个新的功能,比如修改图书信息,我们只需要在数据访问层、业务逻辑层和表示层中分别添加相应的方法即可,而不需要修改其他模块的代码。
三层结构实例

关于三层结构
1、二层、三层结构思想对比
两层应用架构
三层应用架构2、三层结构的数据添加
(1)利用三层结构示意图
(2)利用三层结构技术添加用户信息示意图
(3)利用三层结构技术进行数据库开发的步骤
1) 建立SQL 语句(带参数方式或存储过程)
2) 在逻辑层中建立与数据表相对应的实体类,定义类的属性(与表
的字段一一对应);
3) 设计表现层:输入界面;数据验证。
4) 在数据层中进行参数数组的封装、利用 建立数据访问类
的数据操作方法。
5) 建立业务逻辑层中的类,设计方法调用数据层类中的有关方法,
及进行必要的业务处理编程(如将数据层返回的数据进行各种运算)。
6) 继续在表现层中进行数据封装和调用逻辑层方法,根据返回的数
据进行必要显示(查询)或提示(非查询)。
3、利用三层结构思想开发应用程序实例(以用户注册功能为例)
文件结构
1、 相关数据表:users 。
2、 存储过程设计:addUser
实体类 数据访问层
通用类 业务层 表现层
3、实体类设计:在项目Entity文件夹中建立实体类:Eusers
4、数据层设计:在项目DAL文件夹中建立DAUser类,声明方法InsertUser。
5、业务层设计:在项目BLL文件夹中建立业务类BLUser,并声明方法:AddUser。
6、表现层设计:。
java三层架构实例
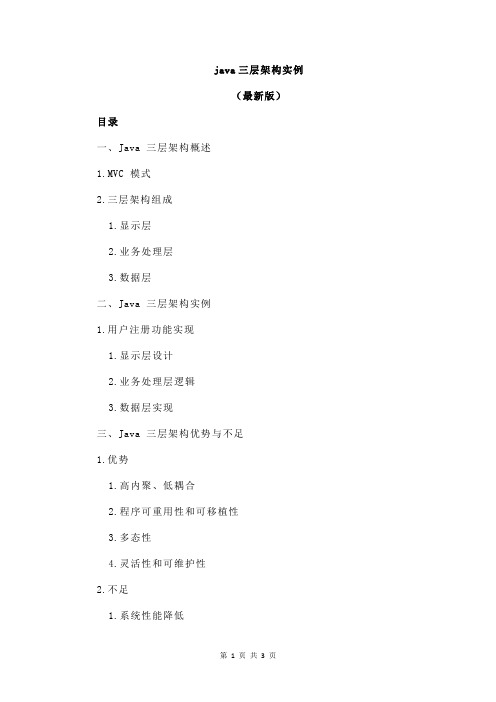
java三层架构实例(最新版)目录一、Java 三层架构概述1.MVC 模式2.三层架构组成1.显示层2.业务处理层3.数据层二、Java 三层架构实例1.用户注册功能实现1.显示层设计2.业务处理层逻辑3.数据层实现三、Java 三层架构优势与不足1.优势1.高内聚、低耦合2.程序可重用性和可移植性3.多态性4.灵活性和可维护性2.不足1.系统性能降低2.级联修改复杂四、结论正文一、Java 三层架构概述在 Java 软件开发中,三层架构是一种常见的架构模式,它包括 MVC 模式和三层架构。
MVC 模式(Model-View-Controller)是一种分层开发的模式,其中 Model 是业务模型,负责处理业务逻辑;View 是视图,负责界面展示;Controller 是控制器,负责接收浏览器发送的请求并调用Model 和 View。
三层架构是指应用程序分为三个层次:显示层、业务处理层和数据层。
显示层(Display Layer)主要负责展示界面和与用户交互;业务处理层(Business Logic Layer)负责处理具体的业务逻辑;数据层(Data Layer)负责与数据库进行交互,实现数据的增删改查等操作。
二、Java 三层架构实例下面以一个简单的用户注册功能为例,介绍如何使用 Java 三层架构进行开发。
1.显示层设计:首先需要设计一个用户注册的 HTML 页面,包括用户名、密码、邮箱等输入框,以及注册按钮。
2.业务处理层逻辑:在 Java 后端,编写一个 Servlet 类(例如:UserServlet)来处理用户注册请求。
接收客户端发送的请求参数,对其进行验证(例如:非空检验、邮箱地址格式检验等),然后将合格的请求参数封装成一个对象(例如:User 对象),并将其传递给业务处理层。
3.数据层实现:在数据层,编写一个 UserDAO 接口,定义注册用户的方法(例如:insertUser)。
三层架构简易实例详解 -回复

三层架构简易实例详解-回复什么是三层架构?三层架构是一种常见的软件架构模式,将应用程序划分为三个主要的逻辑层:表示层(UI层)、业务逻辑层(BLL层)和数据访问层(DAL层)。
这种架构模式将不同的功能和职责进行了分离,使得应用程序更易于维护、拓展和重用。
表示层(UI层):表示层是用户与系统之间的接口,负责接收用户输入并向用户展示结果。
它通常包括用户界面、控制器和视图等。
用户界面负责与用户的交互,接收用户输入;控制器负责处理用户请求,将其传递给业务逻辑层;视图负责向用户展示处理结果。
业务逻辑层(BLL层):业务逻辑层是应用程序的核心,负责处理应用程序的业务逻辑。
它包含了应用程序的主要处理逻辑、算法和规则等。
业务逻辑层负责接收来自表示层的用户请求,进行处理并将结果返回给表示层。
数据访问层(DAL层):数据访问层是与数据存储和数据库交互的层。
它主要负责将业务逻辑层的请求转化为对数据库的操作,并将数据库返回的结果返回给业务逻辑层。
数据访问层的主要目的是将业务逻辑层与具体的数据存储实现进行解耦,使得业务逻辑层的实现与数据访问细节无关。
三层架构的优势:1. 模块化和可维护性:三层架构将应用程序拆分为不同的逻辑层,使得每个层次都具备清晰的功能和职责。
这种模块化的设计使得代码更易于维护和拓展。
2. 可重用性:由于不同的层次之间的耦合度较低,有助于提高代码的可重用性。
例如,业务逻辑层可以被多个不同的表示层共享,减少了重复编写代码的工作量。
3. 性能优化:三层架构可以根据实际需求进行负载均衡和性能优化。
例如,可以将数据库部署在单独的服务器上,以提高数据访问的效率。
4. 安全性:通过将业务逻辑与数据访问逻辑分离,可以更好地保护数据安全和业务逻辑的完整性。
5. 易于团队合作开发:每个层次的功能和职责被清晰划分,有助于团队合作开发。
不同的开发人员可以并行地开发不同的层次,减少了沟通和协作的压力。
三层架构的实例:假设我们要开发一个简单的学生管理系统,其中包括学生信息的录入、查询和删除等功能。
winform 三层架构案例

winform 三层架构案例Winform三层架构是一种常见的软件架构模式,它将应用程序分为三个主要层,表示层(UI层)、业务逻辑层和数据访问层。
下面我将从这三个层面来详细介绍Winform三层架构的一个案例。
首先,我们来看表示层(UI层)。
在Winform应用程序中,表示层通常由窗体(Form)和控件(Controls)组成,用于与用户交互并展示数据。
在三层架构中,表示层主要负责接收用户输入,将用户请求传递给业务逻辑层,并将业务逻辑层返回的数据展示给用户。
例如,一个简单的Winform窗体上有一个按钮,点击按钮后触发事件,事件处理程序会调用业务逻辑层的方法来处理业务逻辑,并将结果展示在窗体上。
其次,业务逻辑层负责处理应用程序的业务逻辑。
在Winform 三层架构中,业务逻辑层通常包括各种业务逻辑的方法和逻辑处理代码,例如数据验证、计算逻辑等。
它负责接收表示层传递过来的请求,处理业务逻辑,并将结果返回给表示层。
在一个案例中,业务逻辑层可以包括各种业务逻辑的类和方法,例如处理订单、计算报表等。
最后,数据访问层负责与数据存储(如数据库、文件等)进行交互,包括数据的读取、写入、更新和删除等操作。
在Winform三层架构中,数据访问层通常包括数据访问对象(DAO)或数据访问类,用于封装对数据存储的访问操作。
例如,一个简单的数据访问层可以包括对数据库的连接、执行SQL语句等操作。
综上所述,一个典型的Winform三层架构案例可以是一个简单的订单管理系统。
在这个案例中,表示层负责展示订单信息和接收用户输入,业务逻辑层负责处理订单相关的业务逻辑,如计算订单总额、验证订单信息等,数据访问层负责与数据库进行交互,包括读取订单信息、保存订单信息等操作。
通过这个案例,我们可以清晰地看到Winform三层架构在实际应用中的具体运用和好处。
希望这个案例能够帮助你更好地理解Winform三层架构。
python三层架构实例
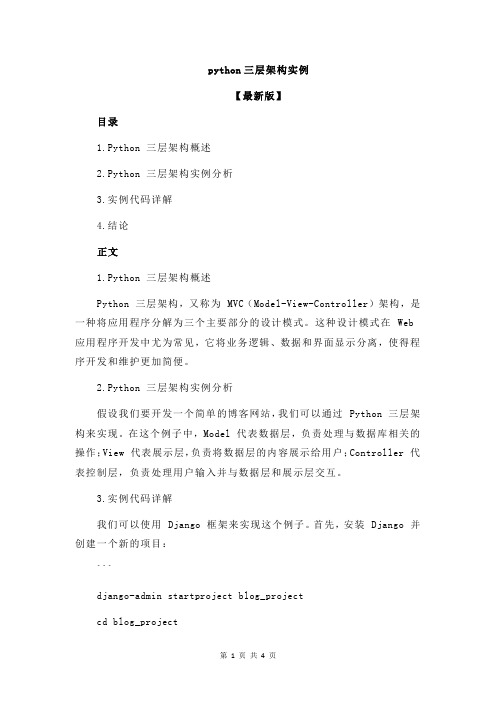
python三层架构实例【最新版】目录1.Python 三层架构概述2.Python 三层架构实例分析3.实例代码详解4.结论正文1.Python 三层架构概述Python 三层架构,又称为 MVC(Model-View-Controller)架构,是一种将应用程序分解为三个主要部分的设计模式。
这种设计模式在 Web 应用程序开发中尤为常见,它将业务逻辑、数据和界面显示分离,使得程序开发和维护更加简便。
2.Python 三层架构实例分析假设我们要开发一个简单的博客网站,我们可以通过 Python 三层架构来实现。
在这个例子中,Model 代表数据层,负责处理与数据库相关的操作;View 代表展示层,负责将数据层的内容展示给用户;Controller 代表控制层,负责处理用户输入并与数据层和展示层交互。
3.实例代码详解我们可以使用 Django 框架来实现这个例子。
首先,安装 Django 并创建一个新的项目:```django-admin startproject blog_projectcd blog_project```接下来,创建一个名为“blog”的应用:```python manage.py startapp blog```在 blog 应用中,我们首先创建一个 models.py 文件,用于编写数据层的代码。
例如,我们可以创建一个名为“Post”的模型,用于存储博客文章:```pythonfrom django.db import modelsclass Post(models.Model):title = models.CharField(max_length=200)content = models.TextField()pub_date = models.DateTimeField("date published")def __str__(self):return self.title```接下来,我们需要创建一个名为“views.py”的文件,用于编写展示层的代码。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
这里以查询数据库中student表的所有信息为例:
1、模型层,Student.cs文件:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace SchoolModels
{
public class Student
{
public Student()
{ }
public Student(int id, string name, string pwd, int age, string sex) {
this.StudentId = id;
this.StudentName = name;
this.StudentPwd = pwd;
}
private int studentId;
public int StudentId
{
get { return studentId; }
set { studentId = value; }
}
private string studentName;
public string StudentName
{
get { return studentName; }
set { studentName = value; }
}
private string studentPwd;
public string StudentPwd
{
get { return studentPwd; }
set { studentPwd = value; }
}
}
}
2、数据访问层,StudentService.cs文件:using System.Text;
using System.Data;
using System.Data.SqlClient;
using SchoolModels;
namespace SchoolDal
{
public class StudentService
{
public static IList<Student> GetAllStudents()
{
List<Student> stus = new List<Student>();
string sql = "select * from student";
SqlCommand cmd = new SqlCommand(sql, DBHelper.con);
DBHelper.con.Open();
SqlDataReader reader = cmd.ExecuteReader();
while (reader.Read())
{
Student student = new Student();
student.StudentId = (int)reader["StudentId"];
student.StudentName = reader["StudentName"].ToString();
student.StudentPwd = reader["StudentPwd"].ToString();
stus.Add(student);
}
reader.Close();
DBHelper.con.Close();
return stus;
}
}
其中,里面有一个DBHelper.cs文件:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Configuration;
using SchoolModels;
using System.Data;
using System.Data.SqlClient;
namespace SchoolDal
{
public class DBHelper
{
public static string conStr = ConfigurationManager.ConnectionStrings["conDb"].ToString();
public static SqlConnection con = new SqlConnection(conStr); }
}
3、业务逻辑层,StudentManager.cs文件:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using SchoolDal;
using SchoolModels;
namespace SchoolBll
{
public class StudentManager
{
public static IList<Student> GetAllStudents()
{
return StudentService.GetAllStudents();
}
}
}
最后,假设在Form1里面把所有学生的信息显示到DataGridView中,Form1.cs 文件:
using System;
using System.Collections.Generic;
using ponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using SchoolBll;
using SchoolModels;
namespace School
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
IList<Student> stus = StudentManager.GetAllStudents();
this.dgvStudent.DataSource = stus;
}
}
以上就是通过三层架构做的一个查询数据库某一张表所有信息的小例子,你可以根据具体的需求进行修改。
在这里还想补充一下对于三层架构的理解,因为全面的理解三层架构对于代码的编写会有很大的帮助:
1、表示层,用于与用户进行交互并显示结果。
提供用户界面,接受用户输入,
提供可视化接口,由各种可视化元素组成,包含数据控件的配置、导航系统等;
2、中间层,负责底层数据源与前端界面的沟通,它存在的主要目的是实现分层
的体系结构,将数据访问功能与逻辑程序代码从表示层抽离出来,提供系统设计的弹性。
所有的程序代码以类为单位,封装在各种类型的文件中,再经过编译成为可被引用的程序功能组件。
3、数据源层,存放底层数据库。
其中中间层又分为:业务逻辑层和数据访问层
1)业务逻辑层:本层连续数据访问层与表示层,取得数据访问层的数据内容,进行数据的维护与存取,将数据发送到表示层,或者是执行反向操作,将用户输入的数据提取,返回到数据访问层;
2)数据访问层:本层访问底层数据源,封装了访问数据所需要的程序代码,也只有这一层能够直接与数据源进行沟通。
通过分层设计,访问底层数据源的代码得以进一步与表示层分开,业务逻辑层本身只负责对数据访问层的类进行引用,不会涉及数据访问的细节,也因此我们可
以有效地对表示层隐藏数据库架构等重要的细节,同时通过数据访问层的切换,可以针对不同的数据源进行访问。
最后,如果你已经得到了一些好的建议,希望你能把对你有帮助的回复标记为答复,这样也可以帮助到其它和你遇到类似问题的朋友。
如果你还有其他的问题,欢迎在论坛里再次发帖。
谢谢你的理解和支持!。