java文件读写操作
java四种方式读取文件

java四种⽅式读取⽂件读取⽂件的四种⽅式:按字节读取、按字符读取、按⾏读取、随机读取⼀、按字节读取//1.按字节读写⽂件,⽤FileInputStream、FileOutputStreamString path = "D:\\iotest.txt";File file = new File(path);InputStream in;//每次只读⼀个字节try {System.out.println("以字节为单位,每次读取⼀个字节");in = new FileInputStream(file);int c;while((c=in.read())!=-1){if(c!=13&&c!=10){ // \n回车的Ascall码是10 ,\r换⾏是Ascall码是13,不现实挥着换⾏System.out.println((char)c);}}} catch (FileNotFoundException e) {e.printStackTrace();}//每次读多个字节try {System.out.println("以字节为单位,每次读取多个字节");in = new FileInputStream(file);byte[] bytes = new byte[10]; //每次读是个字节,放到数组⾥int c;while((c=in.read(bytes))!=-1){System.out.println(Arrays.toString(bytes));}} catch (Exception e) {// TODO: handle exception}⼆、按字符读取//2.按字符读取⽂件,⽤InputStreamReader,OutputStreamReaderReader reader = null;try {//每次读取⼀个字符System.out.println("以字符为单位读取⽂件,每次读取⼀个字符");in = new FileInputStream(file);reader = new InputStreamReader(in);int c;while((c=reader.read())!=-1){if(c!=13&&c!=10){ // \n回车的Ascall码是10 ,\r换⾏是Ascall码是13,不现实挥着换⾏System.out.println((char)c);}}} catch (Exception e) {// TODO: handle exception}try {//每次读取多个字符System.out.println("以字符为单位读取⽂件,每次读取⼀个字符");in = new FileInputStream(file);reader = new InputStreamReader(in);int c;char[] chars = new char[5];while((c=reader.read(chars))!=-1){System.out.println(Arrays.toString(chars));}} catch (Exception e) {// TODO: handle exception}三、按⾏读取//3.按⾏读取⽂件try {System.out.println("按⾏读取⽂件内容");in = new FileInputStream(file);Reader reader2 = new InputStreamReader(in);BufferedReader bufferedReader = new BufferedReader(reader2);String line;while((line=bufferedReader.readLine())!=null){System.out.println(line);}bufferedReader.close();} catch (Exception e) {// TODO: handle exception}四、随机读取//4.随机读取try {System.out.println("随机读取⽂件");//以只读的⽅式打开⽂件RandomAccessFile randomAccessFile = new RandomAccessFile(file, "r");//获取⽂件长度,单位为字节long len = randomAccessFile.length();//⽂件起始位置int beginIndex = (len>4)?4:0;//将⽂件光标定位到⽂件起始位置randomAccessFile.seek(beginIndex);byte[] bytes = new byte[5];int c;while((c=randomAccessFile.read(bytes))!=-1){System.out.println(Arrays.toString(bytes));}} catch (Exception e) {// TODO: handle exception}。
如何在Java中进行数据的持久化和读取操作
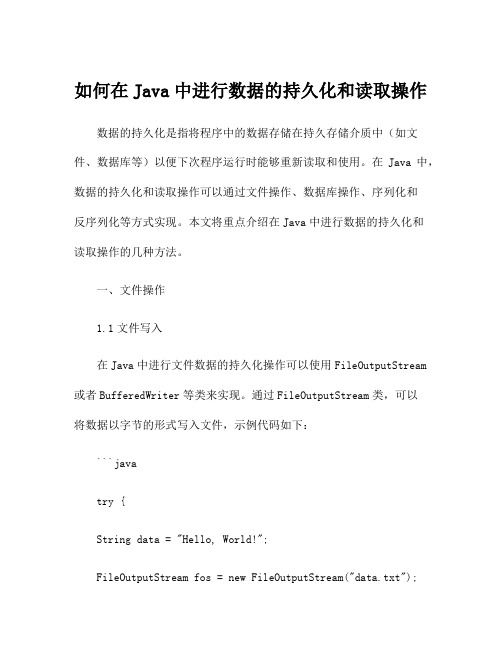
如何在Java中进行数据的持久化和读取操作数据的持久化是指将程序中的数据存储在持久存储介质中(如文件、数据库等)以便下次程序运行时能够重新读取和使用。
在Java中,数据的持久化和读取操作可以通过文件操作、数据库操作、序列化和反序列化等方式实现。
本文将重点介绍在Java中进行数据的持久化和读取操作的几种方法。
一、文件操作1.1文件写入在Java中进行文件数据的持久化操作可以使用FileOutputStream 或者BufferedWriter等类来实现。
通过FileOutputStream类,可以将数据以字节的形式写入文件,示例代码如下:```javatry {String data = "Hello, World!";FileOutputStream fos = new FileOutputStream("data.txt");fos.write(data.getBytes());fos.close();} catch (IOException e) {e.printStackTrace();}```上述代码中,首先定义了一个字符串数据并赋值给data变量,然后通过FileOutputStream类打开文件输出流,并将字符串数据以字节形式写入文件中,最后关闭文件输出流。
1.2文件读取使用FileInputStream或者BufferedReader类可以实现对文件数据的读取操作。
示例代码如下:```javatry {FileInputStream fis = new FileInputStream("data.txt");int content;while ((content = fis.read()) != -1) {System.out.print((char) content);}fis.close();} catch (IOException e) {e.printStackTrace();}```上述代码中,首先使用FileInputStream类打开文件输入流,并定义一个整型变量content用于存储读取的字节数据。
Java一次性读取或者写入文本文件所有内容

Java⼀次性读取或者写⼊⽂本⽂件所有内容⼀次性读取⽂本⽂件所有内容我们做⽂本处理的时候的最常⽤的就是读写⽂件了,尤其是读取⽂件,不论是什么⽂件,我都倾向于⼀次性将⽂本的原始内容直接读取到内存中再做处理,当然,这需要你有⼀台⼤内存的机器,内存不够者……可以⼀次读取少部分内容,分多次读取。
读取⽂件效率最快的⽅法就是⼀次全读进来,很多⼈⽤readline()之类的⽅法,可能需要反复访问⽂件,⽽且每次readline()都会调⽤编码转换,降低了速度,所以,在已知编码的情况下,按字节流⽅式先将⽂件都读⼊内存,再⼀次性编码转换是最快的⽅式,典型的代码如下:public String readToString(String fileName) {String encoding = "UTF-8";File file = new File(fileName);Long filelength = file.length();byte[] filecontent = new byte[filelength.intValue()];try {FileInputStream in = new FileInputStream(file);in.read(filecontent);in.close();} catch (FileNotFoundException e) {e.printStackTrace();} catch (IOException e) {e.printStackTrace();}try {return new String(filecontent, encoding);} catch (UnsupportedEncodingException e) {System.err.println("The OS does not support " + encoding);e.printStackTrace();return null;}}⼀次性写⼊⽂本⽂件所有内容/*** ⼀次性写⼊⽂本⽂件所有内容** @param fileName* @param content*/public static void saveStringTOFile(String fileName, String content){FileWriter writer=null;try {writer = new FileWriter(new File(fileName));writer.write(content);} catch (IOException e) {// TODO ⾃动⽣成的 catch 块e.printStackTrace();} finally {try {writer.close();} catch (IOException e) {// TODO ⾃动⽣成的 catch 块e.printStackTrace();}}System.out.println("写⼊成功!!!");}。
java 顺序读写文件的原理

java 顺序读写文件的原理Java顺序读写文件的原理顺序读写文件是一种常见的文件操作方式,特别是用于处理大型文件或者需要按照固定顺序访问文件内容的情况。
Java提供了多种API和技术来实现顺序读写文件,下面将介绍其原理。
1. 读取文件(顺序读取):顺序读取文件主要通过FileInputStream类来实现。
以下是其原理:- 使用FileInputStream类的构造函数创建一个文件输入流对象,并指定要读取的文件路径。
- 创建一个字节数组或者字符数组作为缓冲区,用来存放从文件中读取的数据。
- 使用read方法从文件输入流中读取数据,并将数据存入缓冲区。
read方法会返回读取的字节数或者字符数,如果已经读取到文件末尾,则返回-1。
- 重复执行上述步骤,直到读取完整个文件内容。
2. 写入文件(顺序写入):顺序写入文件主要通过FileOutputStream类来实现。
以下是其原理:- 使用FileOutputStream类的构造函数创建一个文件输出流对象,并指定要写入的文件路径。
- 创建一个字节数组或者字符数组作为缓冲区,用来存放待写入的数据。
- 使用write方法将缓冲区中的数据写入文件输出流。
write方法会将数据写入文件并返回写入的字节数或者字符数。
- 重复执行上述步骤,直到写入完所有数据。
- 使用close方法关闭文件输出流,确保所有数据都被写入文件。
需要注意的是,顺序读写文件时要合理设置缓冲区的大小。
缓冲区太小会导致频繁的IO操作,影响性能;缓冲区太大则会占用过多内存。
因此,根据实际情况调整缓冲区大小以达到最佳性能。
另外,为了保证顺序读写文件的稳定性和可靠性,建议在读写文件时使用try-catch-finally或者try-with-resources语句块,确保资源能够被正确释放。
总结:顺序读写文件是通过Java的FileInputStream和FileOutputStream类来实现的。
java读取文件和写入文件的方式(简单实例)
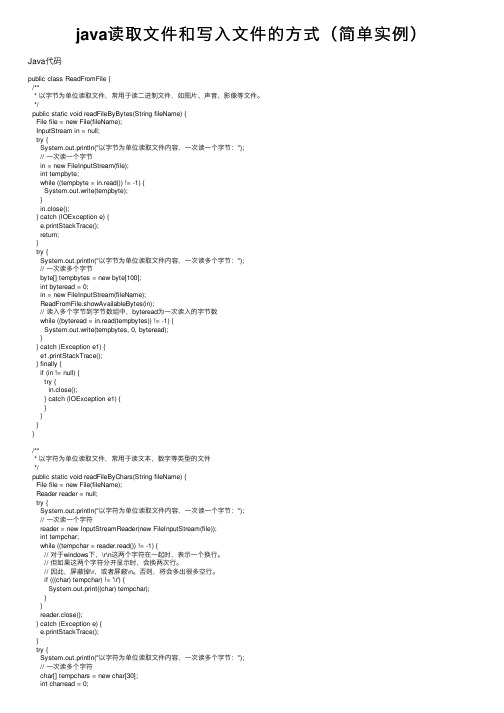
java读取⽂件和写⼊⽂件的⽅式(简单实例)Java代码public class ReadFromFile {/*** 以字节为单位读取⽂件,常⽤于读⼆进制⽂件,如图⽚、声⾳、影像等⽂件。
*/public static void readFileByBytes(String fileName) {File file = new File(fileName);InputStream in = null;try {System.out.println("以字节为单位读取⽂件内容,⼀次读⼀个字节:");// ⼀次读⼀个字节in = new FileInputStream(file);int tempbyte;while ((tempbyte = in.read()) != -1) {System.out.write(tempbyte);}in.close();} catch (IOException e) {e.printStackTrace();return;}try {System.out.println("以字节为单位读取⽂件内容,⼀次读多个字节:");// ⼀次读多个字节byte[] tempbytes = new byte[100];int byteread = 0;in = new FileInputStream(fileName);ReadFromFile.showAvailableBytes(in);// 读⼊多个字节到字节数组中,byteread为⼀次读⼊的字节数while ((byteread = in.read(tempbytes)) != -1) {System.out.write(tempbytes, 0, byteread);}} catch (Exception e1) {e1.printStackTrace();} finally {if (in != null) {try {in.close();} catch (IOException e1) {}}}}/*** 以字符为单位读取⽂件,常⽤于读⽂本,数字等类型的⽂件*/public static void readFileByChars(String fileName) {File file = new File(fileName);Reader reader = null;try {System.out.println("以字符为单位读取⽂件内容,⼀次读⼀个字节:");// ⼀次读⼀个字符reader = new InputStreamReader(new FileInputStream(file));int tempchar;while ((tempchar = reader.read()) != -1) {// 对于windows下,\r\n这两个字符在⼀起时,表⽰⼀个换⾏。
用Java对CSV文件进行读写操作

⽤Java对CSV⽂件进⾏读写操作需要jar包:javacsv-2.0.jar读操作// 读取csv⽂件的内容public static ArrayList<String> readCsv(String filepath) {File csv = new File(filepath); // CSV⽂件路径csv.setReadable(true);//设置可读csv.setWritable(true);//设置可写BufferedReader br = null;try {br = new BufferedReader(new FileReader(csv));} catch (FileNotFoundException e) {e.printStackTrace();}String line = "";String everyLine = "";ArrayList<String> allString = new ArrayList<>();try {while ((line = br.readLine()) != null) // 读取到的内容给line变量{everyLine = line;System.out.println(everyLine);allString.add(everyLine);}System.out.println("csv表格中所有⾏数:" + allString.size());} catch (IOException e) {e.printStackTrace();}return allString;}写操作public void writeCSV(String path) {String csvFilePath = path;try {// 创建CSV写对象例如:CsvWriter(⽂件路径,分隔符,编码格式);CsvWriter csvWriter = new CsvWriter(csvFilePath, ',', Charset.forName("GBK"));// 写内容String[] headers = {"FileName","FileSize","FileMD5"};csvWriter.writeRecord(headers);for(int i=0;i<writearraylist.size();i++){String[] writeLine=writearraylist.get(i).split(",");System.out.println(writeLine);csvWriter.writeRecord(writeLine);}csvWriter.close();System.out.println("--------CSV⽂件已经写⼊--------");} catch (IOException e) {e.printStackTrace();}}。
java代码实现读写txt文件(txt文件转换成java文件)
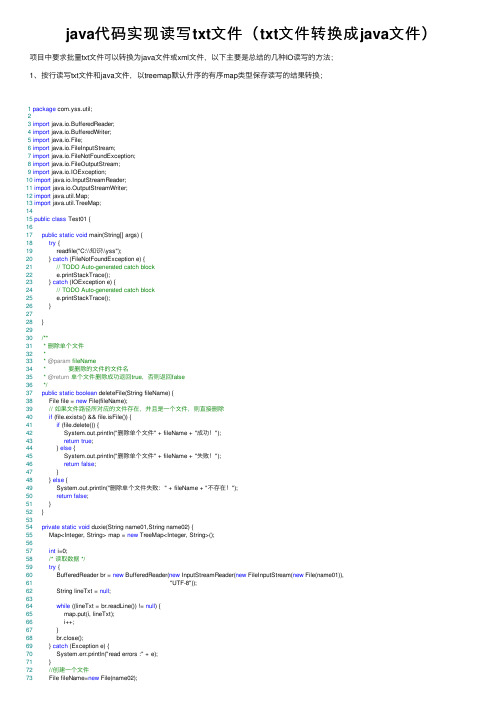
29
30 /**
31 * 删除单个文件
32 *
33 * @param fileName
34 *
要删除的文件的文件名
35 * @return 单个文件删除成功返回true,否则返回false
36 */
37 public static boolean deleteFile(String fileName) {
64
fileOutputStream.close();
65
flag=true;
66
} catch (Exception e) {
80
}
81
//删除掉原来的文件
82
deleteFile(name01);
83
84
/* 输出数据 */
85
try {
86
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(new File(name02)),
35
String result = "";
36
try {
37
InputStreamReader reader = new InputStreamReader(new FileInputStream(file),"gbk");
38
BufferedReader br = new BufferedReader(reader);
59
boolean flag=false;
60
FileOutputStream fileOutputStream=null;
java读写CSV文件的两种方法

java读写CSV⽂件的两种⽅法---------------------------------------------------------⼩路原创,转载请注明出处!------------------------------------------起初,我⾃⼰连什么叫CSV⽂件都不知道,这个问题是来⾃⼀个⽹友的问题,他要我帮他做⼀个对csv⽂件数据的操作的题⽬。
要求:如果原来数据是“江苏省南京市南京街……”换成“江苏省南京市 CSV⽂件简介:Comma Separated Values,简称CSV,即逗号分隔值,是⼀种纯⽂本格式,⽤来存储数据。
在CSV中,数据的字段由逗号分开。
CSV⽂件是⼀个计算机数据⽂件⽤于执⾏审判和真正的组织⼯具,逗号分隔的清单下⾯是最开始写的⽐较累赘的代码:package test;import java.io.BufferedReader;import java.io.BufferedWriter;import java.io.File;import java.io.FileNotFoundException;import java.io.FileReader;import java.io.FileWriter;import java.io.IOException;public class OperateCSVfile {public static void main(String[] args){String [] str = {"省","市","区","街","路","⾥","幢","村","室","园","苑","巷","号"};File inFile = new File("C://in.csv"); // 读取的CSV⽂件File outFile = new File("C://out.csv");//写出的CSV⽂件String inString = "";String tmpString = "";try {BufferedReader reader = new BufferedReader(new FileReader(inFile));BufferedWriter writer = new BufferedWriter(new FileWriter(outFile));while((inString = reader.readLine())!= null){char [] c = inString.toCharArray();String [] value = new String[c.length];String result = "";for(int i = 0;i < c.length;i++){value[i] = String.valueOf(c[i]);for(int j = 0;j < str.length;j++){if(value[i].equals(str[j])){String tmp = value[i];value[i] = "," + tmp + ",";}}result += value[i];}writer.write(inString);writer.newLine();}reader.close();writer.close();} catch (FileNotFoundException ex) {System.out.println("没找到⽂件!");} catch (IOException ex) {System.out.println("读写⽂件出错!");}}}利⽤String类的replace()⽅法之后的代码简化为;package test;import java.io.BufferedReader;import java.io.BufferedWriter;import java.io.File;import java.io.FileNotFoundException;import java.io.FileReader;import java.io.FileWriter;import java.io.IOException;public class OperateCSVfile {public static void main(String[] args){String [] str = {"省","市","区","街","路","⾥","幢","村","室","园","苑","巷","号"};File inFile = new File("C://in.csv"); // 读取的CSV⽂件File outFile = new File("C://out.csv");//写出的CSV⽂件String inString = "";String tmpString = "";try {BufferedReader reader = new BufferedReader(new FileReader(inFile));BufferedWriter writer = new BufferedWriter(new FileWriter(outFile));while((inString = reader.readLine())!= null){for(int i = 0;i<str.length;i++){tmpString = inString.replace(str[i], "," + str[i] + ",");inString = tmpString;}writer.write(inString);writer.newLine();}reader.close();writer.close();} catch (FileNotFoundException ex) {System.out.println("没找到⽂件!");} catch (IOException ex) {System.out.println("读写⽂件出错!");}}}效果图;之后我⼜在⽹上查了⼀下资料,发现java有专门操作CSV⽂件的类和⽅法。
java从文件中读取数据并存入对象中的方法
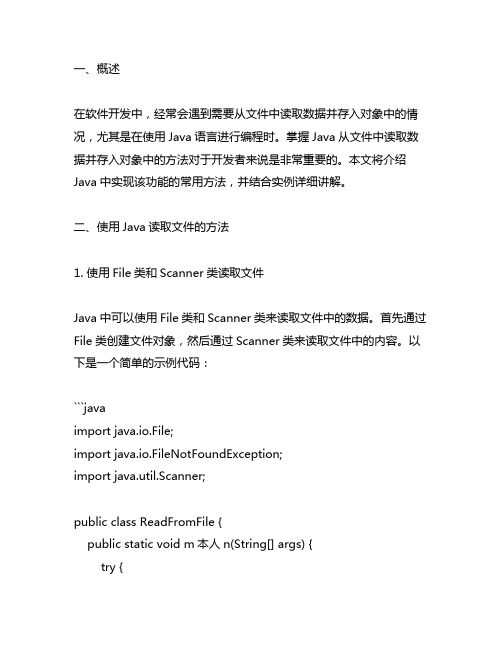
一、概述在软件开发中,经常会遇到需要从文件中读取数据并存入对象中的情况,尤其是在使用Java语言进行编程时。
掌握Java从文件中读取数据并存入对象中的方法对于开发者来说是非常重要的。
本文将介绍Java中实现该功能的常用方法,并结合实例详细讲解。
二、使用Java读取文件的方法1. 使用File类和Scanner类读取文件Java中可以使用File类和Scanner类来读取文件中的数据。
首先通过File类创建文件对象,然后通过Scanner类来读取文件中的内容。
以下是一个简单的示例代码:```javaimport java.io.File;import java.io.FileNotFoundException;import java.util.Scanner;public class ReadFromFile {public static void m本人n(String[] args) {try {File file = new File("data.txt");Scanner scanner = new Scanner(file);while (scanner.hasNextLine()) {String data = scanner.nextLine();System.out.println(data);}scanner.close();} catch (FileNotFoundException e) {System.out.println("File not found");e.printStackTrace();}}}```2. 使用BufferedReader类读取文件除了Scanner类,还可以使用BufferedReader类来读取文件中的数据。
与Scanner类不同,BufferedReader类提供了更高效的读取方式。
以下是一个使用BufferedReader类读取文件的示例代码:```javaimport java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;public class ReadFromFile {public static void m本人n(String[] args) {try {BufferedReader reader = new BufferedReader(new FileReader("data.txt"));String line = reader.readLine();while (line != null) {System.out.println(line);line = reader.readLine();}reader.close();} catch (IOException e) {System.out.println("IO Exception");e.printStackTrace();}}}```以上是使用Java读取文件的基本方法,开发者可以根据实际需求选择合适的方式来读取文件中的数据。
Java的RandomAccessFile对文件内容进行读写

Java的RandomAccessFile对⽂件内容进⾏读写RandomAccessFile是Java提供的对⽂件内容的访问,她既可以读⽂件,也可以写⽂件,并且RandomAccessFile⽀持随机访问⽂件,也就是说他可以指定位置进⾏访问。
我们知道Java的⽂件模型,⽂件硬盘上的⽂件是byte byte byte的字节进⾏存储的,是数据的集合。
下⾯就是⽤这个类的步骤。
(1)打开指定的⽂件,有两种模式“rw”(读写) “r”(只读),创建对象,并且指定file和模式,例如:RandomAccessFile ac=new RandomAccessFile(file,”rw”);因为它⽀持随机访问⽂件,所以他引⼊了指针,可以通过指针来写⼊写出在指定的位置。
⽂件指针,打开⽂件时指针在开头pointer=0 (2)RandomAccessFile的往⽂件中写的⽅法(还有其他的写⽅法)Ac.write(int)----->只能写⼀个字节(后⼋位),同时⽂件指针也会移动,指向下⼀个位置。
(3)RandomAccessFile读的⽅法(还有其他的读⽅法)int b=ac.read()--->读⼀个字节(4)⽂件读写完毕后必须要把他关闭,调⽤close()的⽅法。
下⾯就是例⼦://创建相对路径的⽂件,就是在这个项⽬中创建⼀个⽂件夹File file=new File("random");if(!file.exists()) {file.mkdir();}File fileName=new File(file,"javaio.txt");if(!fileName.exists()) {fileName.createNewFile();//创建⽂件}//创建⼀个RandomAccessFile的对象,并指定模式rw,能读能写,//注意:必须是⽂件,不能是路径RandomAccessFile raf=new RandomAccessFile(fileName,"rw");//获取此时的⽂件指针的位置,起始位置为0System.out.println(raf.getFilePointer());//向⽂件中写⼊字符A,字符类型有两个字节,但她写⼊的是后⼋位的字节//字符A正好可以⽤后⼋位的字节表⽰出来raf.write('A');//字符的位置会⾃动向后移动,⽂件指针会向后⾃动移动System.out.println("输⼊⼀个字符之后,⽂件指针的位置"+raf.getFilePointer());raf.write('B');//每次write只能写⼊⼀个字节,如果要把i写进去,就需要写四次int i=0x7fffffff;raf.write(i>>>24 & 0xff);//写⼊⾼⼋位的raf.write(i>>>16 & 0xff);raf.write(i>>>8 & 0xff);raf.write(i);//写⼊低⼋位System.out.println("写⼊整数的时候⽂件指针的位置是"+raf.getFilePointer());/*** writeInt()的内置⽅法* public final void writeInt(int v) throws IOException {write((v >>> 24) & 0xFF);write((v >>> 16) & 0xFF);write((v >>> 8) & 0xFF);write((v >>> 0) & 0xFF);//written += 4;}*///也可以直接写⼊int整数raf.writeInt(i);//写⼊⼀个汉字,汉字为两个字节String str="欢迎学习java";byte[] b=str.getBytes("gbk");raf.write(b);System.out.println("此时的长度为"+raf.length());//读⽂件必须将⽂件指针放在开头位置raf.seek(0);byte[] buf=new byte[(int)raf.length()];raf.read(buf);//将内容写⼊buf字节数组中String str1=new String(buf,"gbk");System.out.println("⽂件中的内容为"+str1); raf.close();。
java读写word文档 完美解决方案
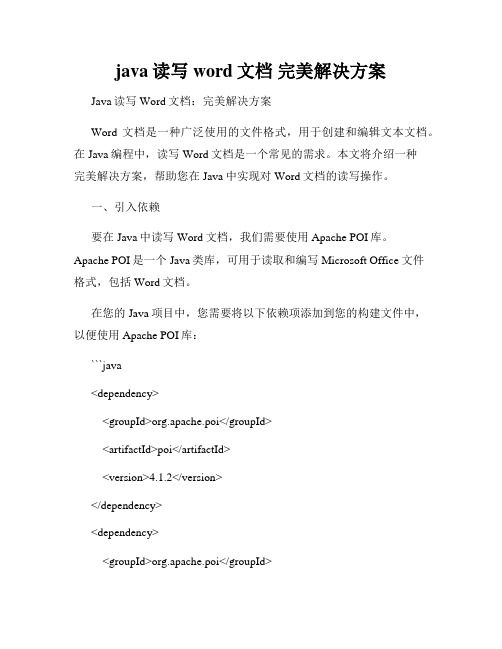
java读写word文档完美解决方案Java读写Word文档:完美解决方案Word文档是一种广泛使用的文件格式,用于创建和编辑文本文档。
在Java编程中,读写Word文档是一个常见的需求。
本文将介绍一种完美解决方案,帮助您在Java中实现对Word文档的读写操作。
一、引入依赖要在Java中读写Word文档,我们需要使用Apache POI库。
Apache POI是一个Java类库,可用于读取和编写Microsoft Office文件格式,包括Word文档。
在您的Java项目中,您需要将以下依赖项添加到您的构建文件中,以便使用Apache POI库:```java<dependency><groupId>org.apache.poi</groupId><artifactId>poi</artifactId><version>4.1.2</version></dependency><dependency><groupId>org.apache.poi</groupId><artifactId>poi-ooxml</artifactId><version>4.1.2</version></dependency>```二、读取Word文档要读取一个Word文档,您可以使用Apache POI提供的XWPFDocument类。
以下是一个简单的示例代码,演示如何使用XWPFDocument读取Word文档:```javaimport ermodel.XWPFDocument;import org.apache.poi.xwpf.extractor.XWPFWordExtractor;import java.io.FileInputStream;import java.io.IOException;public class ReadWordDocument {public static void main(String[] args) {try {FileInputStream fis = newFileInputStream("path/to/your/word/document.docx");XWPFDocument document = new XWPFDocument(fis);XWPFWordExtractor extractor = new XWPFWordExtractor(document);String content = extractor.getText();System.out.println(content);fis.close();} catch (IOException e) {e.printStackTrace();}}}```在上面的代码中,您需要将"path/to/your/word/document.docx"替换为您实际的Word文档路径。
在MacOS上使用Java实现文件读写操作

在MacOS上使用Java实现文件读写操作在MacOS系统上,使用Java语言进行文件读写操作是一种常见的需求。
Java提供了丰富的API和库,使得文件处理变得简单而高效。
本文将介绍如何在MacOS上使用Java实现文件读写操作,包括文件的创建、写入、读取和删除等操作。
1. 文件的创建在Java中,可以使用File类来表示文件或目录,并通过该类的方法来创建文件。
下面是一个简单的示例代码,演示了如何在MacOS上创建一个新的文件:示例代码star:编程语言:javaimport java.io.File;import java.io.IOException;public class CreateFileExample {public static void main(String[] args) {File file = new File("example.txt");try {if (file.createNewFile()) {System.out.println("File created: " + file.getName());} else {System.out.println("File already exists.");}} catch (IOException e) {System.out.println("An error occurred.");e.printStackTrace();}}}示例代码end运行以上代码后,将在当前目录下创建一个名为example.txt的文件。
2. 文件的写入要向文件中写入数据,可以使用FileWriter类。
下面是一个简单的示例代码,演示了如何在MacOS上向文件中写入内容:示例代码star:编程语言:javaimport java.io.FileWriter;import java.io.IOException;public class WriteToFileExample {public static void main(String[] args) {try {FileWriter writer = newFileWriter("example.txt");writer.write("Hello, World!");writer.close();System.out.println("Successfully wrote to the file.");} catch (IOException e) {System.out.println("An error occurred.");e.printStackTrace();}}}示例代码end运行以上代码后,example.txt文件中将会写入”Hello, World!“这行内容。
用java逐行读取和写入大文件的最快方法

在软件开发过程中,经常会遇到需要处理大文件的情况,而对于Java语言来说,如何高效地逐行读取和写入大文件是一个常见的问题。
本文将介绍使用Java语言逐行读取和写入大文件的最快方法,帮助开发人员更加高效地处理大文件。
一、使用BufferedReader和BufferedWriter进行逐行读取和写入BufferedReader和BufferedWriter是Java标准库中提供的用于缓冲输入和输出的类,它们可以显著提高文件读写的效率。
下面是使用BufferedReader和BufferedWriter进行逐行读取和写入的代码示例:```javapublic class FileUtil {public static void copyFile(String sourceFileName, String targetFileName) {try (BufferedReader br = new BufferedReader(new FileReader(sourceFileName));BufferedWriter bw = new BufferedWriter(new FileWriter(targetFileName))) {String line;while ((line = br.readLine()) != null) {bw.write(line);bw.newLine();}} catch (IOException e) {e.printStackTrace();}}}```在上面的代码中,我们使用了BufferedReader逐行读取文件,并使用BufferedWriter逐行写入文件,通过缓冲输入和输出来提高读写效率。
这种方法适用于处理中等大小的文件,但对于大文件来说,还有更加高效的方法可供选择。
二、使用RandomAccessFile进行逐行读取和写入RandomAccessFile是Java标准库中提供的用于随机访问文件的类,它可以在文件中进行任意位置的读写操作。
java读取资源文件的方法

java读取资源文件的方法Java是一种广泛应用于开发各种应用程序的编程语言。
在Java中,读取资源文件是一项常见的任务,它允许我们从外部文件中获取数据或配置信息。
本文将介绍几种常用的Java读取资源文件的方法。
一、使用ClassLoader读取资源文件Java中的ClassLoader是用于加载类的关键组件之一。
它不仅可以加载类,还可以加载其他类型的资源文件。
通过ClassLoader,我们可以很方便地读取资源文件。
我们需要使用ClassLoader的getResourceAsStream()方法获取资源文件的输入流。
这个方法可以接受一个相对路径作为参数,并返回一个InputStream对象。
然后,我们可以使用这个输入流来读取资源文件的内容。
下面是一个使用ClassLoader读取资源文件的示例代码:```javaClassLoader classLoader = getClass().getClassLoader(); InputStream inputStream = classLoader.getResourceAsStream("config.properties");```在上面的代码中,我们使用了getClass().getClassLoader()方法获取当前类的ClassLoader。
然后,我们调用getResourceAsStream()方法获取资源文件config.properties的输入流。
二、使用InputStream读取资源文件除了使用ClassLoader,我们还可以使用InputStream来读取资源文件。
这种方法适用于读取位于文件系统中的资源文件。
我们需要创建一个File对象,用于表示资源文件的路径。
然后,我们可以使用FileInputStream来打开这个文件,并获取其输入流。
最后,我们可以使用这个输入流来读取资源文件的内容。
下面是一个使用InputStream读取资源文件的示例代码:```javaFile file = new File("path/to/config.properties"); InputStream inputStream = new FileInputStream(file);```在上面的代码中,我们创建了一个File对象,表示资源文件config.properties的路径。
hdfs java读写

hdfs java读写HDFS(Hadoop Distributed File System)是Hadoop生态系统中的核心组件之一,它提供了一种在集群中存储和处理大规模数据集的分布式文件系统。
在Java中读写HDFS需要使用Hadoop API。
下面是一个简单的示例,展示如何在Java中读取和写入HDFS文件。
读取HDFS文件:```javaimport org.apache.hadoop.conf.Configuration;import org.apache.hadoop.fs.FileSystem;import org.apache.hadoop.fs.Path;import java.io.BufferedReader;import java.io.InputStreamReader;public class HDFSReader {public static void main(String[] args) throws Exception {Configuration conf = new Configuration();conf.set("fs.defaultFS", "hdfs://localhost:9000"); // HDFS NameNode地址FileSystem fs = FileSystem.get(conf);Path filePath = new Path("/path/to/file"); // HDFS文件路径BufferedReader reader = new BufferedReader(new InputStreamReader(fs.open(filePath)));String line;while ((line = reader.readLine()) != null) {System.out.println(line);}reader.close();fs.close();}}```写入HDFS文件:```javaimport org.apache.hadoop.conf.Configuration;import org.apache.hadoop.fs.FileSystem;import org.apache.hadoop.fs.Path;import java.io.BufferedWriter;import java.io.OutputStreamWriter;public class HDFSWriter {public static void main(String[] args) throws Exception {Configuration conf = new Configuration();conf.set("fs.defaultFS", "hdfs://localhost:9000"); // HDFS NameNode地址FileSystem fs = FileSystem.get(conf);Path filePath = new Path("/path/to/file"); // HDFS文件路径BufferedWriter writer = new BufferedWriter(new OutputStreamWriter(fs.create(filePath)));writer.write("Hello, HDFS!");writer.close();fs.close();}}```在上面的示例中,我们使用了Hadoop API中的FileSystem类来读取和写入HDFS文件。
java实现读写服务器文件
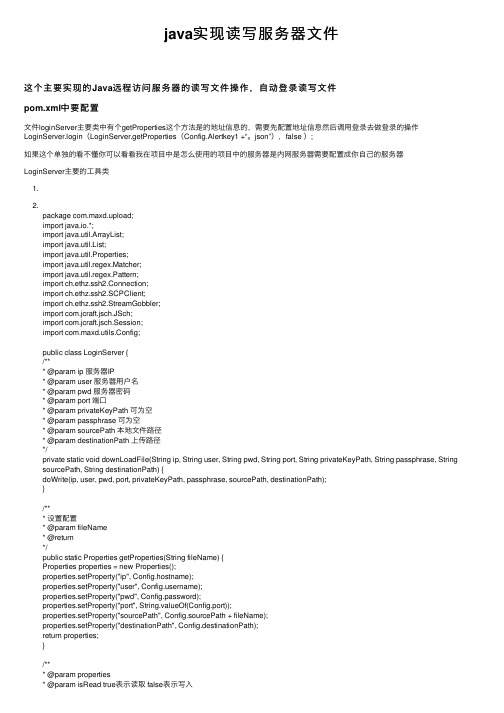
java实现读写服务器⽂件这个主要实现的Java远程访问服务器的读写⽂件操作,⾃动登录读写⽂件pom.xml中要配置⽂件loginServer主要类中有个getProperties这个⽅法是的地址信息的,需要先配置地址信息然后调⽤登录去做登录的操作LoginServer.login(LoginServer.getProperties(Config.Alertkey1 +“。
json”),false );如果这个单独的看不懂你可以看看我在项⽬中是怎么使⽤的项⽬中的服务器是内⽹服务器需要配置成你⾃⼰的服务器LoginServer主要的⼯具类1.2.package com.maxd.upload;import java.io.*;import java.util.ArrayList;import java.util.List;import java.util.Properties;import java.util.regex.Matcher;import java.util.regex.Pattern;import ch.ethz.ssh2.Connection;import ch.ethz.ssh2.SCPClient;import ch.ethz.ssh2.StreamGobbler;import com.jcraft.jsch.JSch;import com.jcraft.jsch.Session;import com.maxd.utils.Config;public class LoginServer {/*** @param ip 服务器IP* @param user 服务器⽤户名* @param pwd 服务器密码* @param port 端⼝* @param privateKeyPath 可为空* @param passphrase 可为空* @param sourcePath 本地⽂件路径* @param destinationPath 上传路径*/private static void downLoadFile(String ip, String user, String pwd, String port, String privateKeyPath, String passphrase, String sourcePath, String destinationPath) {doWrite(ip, user, pwd, port, privateKeyPath, passphrase, sourcePath, destinationPath);}/*** 设置配置* @param fileName* @return*/public static Properties getProperties(String fileName) {Properties properties = new Properties();properties.setProperty("ip", Config.hostname);properties.setProperty("user", ername);properties.setProperty("pwd", Config.password);properties.setProperty("port", String.valueOf(Config.port));properties.setProperty("sourcePath", Config.sourcePath + fileName);properties.setProperty("destinationPath", Config.destinationPath);return properties;}/*** @param properties* @param isRead true表⽰读取 false表⽰写⼊*/public static void login(Properties properties, boolean isRead) {String ip = properties.getProperty("ip");String user = properties.getProperty("user");String pwd = properties.getProperty("pwd");String port = properties.getProperty("port");String privateKeyPath = properties.getProperty("privateKeyPath");String passphrase = properties.getProperty("passphrase");String sourcePath = properties.getProperty("sourcePath");String destinationPath = properties.getProperty("destinationPath");if (!isRead) {//写⼊本地⽂件到远程服务器doWrite(ip, user, pwd, port, privateKeyPath, passphrase, sourcePath, destinationPath);} else {//读取远程⽂件到本地readConnect();}}/*** @throws IOException* @description 读⽂件*/public static String readTxtFile(File fileName) throws IOException {String result = null;FileReader fileReader = null;BufferedReader bufferedReader = null;fileReader = new FileReader(fileName);InputStreamReader isr = new InputStreamReader(new FileInputStream(fileName), "UTF-8");BufferedReader bufferedReader1 = new BufferedReader(isr);String read = null;int count = 0;while ((read = bufferedReader1.readLine()) != null) {result = read + "\r\n";count++;}if (bufferedReader != null) {bufferedReader.close();}if (fileReader != null) {fileReader.close();}return result;}/*** @throws UnsupportedEncodingException* @throws IOException* @description 写⽂件*/public static boolean writeTxtFile(String content, File fileName) throws UnsupportedEncodingException, IOException { FileOutputStream o = null;o = new FileOutputStream(fileName);o.write(content.getBytes("UTF-8"));o.close();return true;}private static void doWrite(String ip, String user, String pwd, String port, String privateKeyPath, String passphrase, String sourcePath, String destinationPath) {if (ip != null && !ip.equals("") && user != null && !user.equals("") && port != null && !port.equals("") && sourcePath != null &&!sourcePath.equals("") && destinationPath != null && !destinationPath.equals("")) {if (privateKeyPath != null && !privateKeyPath.equals("")) {sshSftp2(ip, user, Integer.parseInt(port), privateKeyPath,passphrase, sourcePath, destinationPath);} else if (pwd != null && !pwd.equals("")) {sshSftp(ip, user, pwd, Integer.parseInt(port), sourcePath,destinationPath);} else {Console console = System.console();System.out.print("Enter password:");char[] readPassword = console.readPassword();sshSftp(ip, user, new String(readPassword),Integer.parseInt(port), sourcePath, destinationPath);}} else {System.out.println("请先设置配置⽂件");}}/*** 密码⽅式登录** @param ip* @param user* @param psw* @param port* @param sPath* @param dPath*/private static void sshSftp(String ip, String user, String psw, int port, String sPath, String dPath) {System.out.println("password login");Session session = null;JSch jsch = new JSch();try {if (port <= 0) {// 连接服务器,采⽤默认端⼝session = jsch.getSession(user, ip);} else {// 采⽤指定的端⼝连接服务器session = jsch.getSession(user, ip, port);}// 如果服务器连接不上,则抛出异常if (session == null) {throw new Exception("session is null");}// 设置登陆主机的密码session.setPassword(psw);// 设置密码// 设置第⼀次登陆的时候提⽰,可选值:(ask | yes | no) session.setConfig("StrictHostKeyChecking", "no");// 设置登陆超时时间session.connect(300000);UpLoadFile.upLoadFile(session, sPath, dPath);//DownLoadFile.downLoadFile(session, sPath, dPath);} catch (Exception e) {e.printStackTrace();}System.out.println("success");}/*** 密匙⽅式登录** @param ip* @param user* @param port* @param privateKey* @param passphrase* @param sPath* @param dPath*/private static void sshSftp2(String ip, String user, int port,String privateKey, String passphrase, String sPath, String dPath) { System.out.println("privateKey login");Session session = null;JSch jsch = new JSch();try {// 设置密钥和密码// ⽀持密钥的⽅式登陆,只需在jsch.getSession之前设置⼀下密钥的相关信息就可以了if (privateKey != null && !"".equals(privateKey)) {if (passphrase != null && "".equals(passphrase)) {// 设置带⼝令的密钥jsch.addIdentity(privateKey, passphrase);} else {// 设置不带⼝令的密钥jsch.addIdentity(privateKey);}}if (port <= 0) {// 连接服务器,采⽤默认端⼝session = jsch.getSession(user, ip);} else {// 采⽤指定的端⼝连接服务器session = jsch.getSession(user, ip, port);}// 如果服务器连接不上,则抛出异常if (session == null) {throw new Exception("session is null");}// 设置第⼀次登陆的时候提⽰,可选值:(ask | yes | no)session.setConfig("StrictHostKeyChecking", "no");// 设置登陆超时时间session.connect(300000);UpLoadFile.upLoadFile(session, sPath, dPath);System.out.println("success");} catch (Exception e) {e.printStackTrace();}}/*** 读取远程⽂件到本地*/private static void readConnect() {Connection conn = new Connection(Config.hostname, Config.port);ch.ethz.ssh2.Session ssh = null;try {//连接到主机conn.connect();//使⽤⽤户名和密码校验boolean isconn = conn.authenticateWithPassword(ername, Config.password); if (!isconn) {System.out.println("⽤户名称或者是密码不正确");} else {System.out.println("已经连接OK");File folder = new File(Config.writePath);if (!folder.exists()) {folder.mkdir();}SCPClient clt = conn.createSCPClient();ssh = conn.openSession();ssh.execCommand("find /app/s3-configuration/ -name '*.json'");InputStream is = new StreamGobbler(ssh.getStdout());BufferedReader brs = new BufferedReader(new InputStreamReader(is));while (true) {String line = brs.readLine();if (line == null) {break;}clt.get(line, Config.writePath);List<File> lf = new ArrayList<File>();lf = getFileList(new File(Config.writePath), "json");for (File f : lf) {/*System.out.println(f.getPath());*/String path = f.getPath();File file = new File(path);try {FileReader fr = new FileReader(file);BufferedReader br = new BufferedReader(fr);String s = null;Pattern p = pile(".*?error.*?");while ((s = br.readLine()) != null) {Matcher m = p.matcher(s);if (m.find()) {/*System.out.println(m.matches());*/System.out.println(line);System.out.println("find error!");}/*else{System.out.println("no error");} */}br.close();} catch (FileNotFoundException e) {System.err.println("file not found");} catch (IOException e) {e.printStackTrace();}}System.out.println("⽂件输出成功,请在" + Config.writePath + "中查看");}}} catch (IOException e) {System.out.println(e.getMessage());e.printStackTrace();} finally {//连接的Session和Connection对象都需要关闭if (ssh != null) {ssh.close();}if (conn != null) {conn.close();}}}private static List<File> getFileList(File fileDir, String fileType) {List<File> lfile = new ArrayList<File>();File[] fs = fileDir.listFiles();for (File f : fs) {if (f.isFile()) {if (fileType.equals(f.getName().substring(f.getName().lastIndexOf(".") + 1, f.getName().length()))) lfile.add(f);} else {List<File> ftemps = getFileList(f, fileType);lfile.addAll(ftemps);}}return lfile;}}package com.maxd.upload;import java.io.File;import java.io.FileInputStream;import java.io.IOException;import java.io.InputStream;import java.io.OutputStream;import java.util.Scanner;import com.jcraft.jsch.Channel;import com.jcraft.jsch.ChannelSftp;import com.jcraft.jsch.Session;import com.jcraft.jsch.SftpException;public class UpLoadFile {public static void upLoadFile(Session session, String sPath, String dPath) { Channel channel = null;try {channel = (Channel) session.openChannel("sftp");channel.connect(10000000);ChannelSftp sftp = (ChannelSftp) channel;try {//上传sftp.cd(dPath);Scanner scanner = new Scanner(System.in);/* System.out.println(dPath + ":此⽬录已存在,⽂件可能会被覆盖!是否继续y/n?"); String next = scanner.next();if (!next.toLowerCase().equals("y")) {return;}*/} catch (SftpException e) {sftp.mkdir(dPath);sftp.cd(dPath);}File file = new File(sPath);copyFile(sftp, file, sftp.pwd());} catch (Exception e) {e.printStackTrace();} finally {session.disconnect();channel.disconnect();}}public static void copyFile(ChannelSftp sftp, File file, String pwd) {if (file.isDirectory()) {File[] list = file.listFiles();try {try {String fileName = file.getName();sftp.cd(pwd);System.out.println("正在创建⽬录:" + sftp.pwd() + "/" + fileName);sftp.mkdir(fileName);System.out.println("⽬录创建成功:" + sftp.pwd() + "/" + fileName);} catch (Exception e) {// TODO: handle exception}pwd = pwd + "/" + file.getName();try {sftp.cd(file.getName());} catch (SftpException e) {// TODO: handle exceptione.printStackTrace();}} catch (Exception e) {// TODO Auto-generated catch blocke.printStackTrace();}for (int i = 0; i < list.length; i++) {copyFile(sftp, list[i], pwd);}} else {try {sftp.cd(pwd);} catch (SftpException e1) {// TODO Auto-generated catch blocke1.printStackTrace();}System.out.println("正在复制⽂件:" + file.getAbsolutePath());InputStream instream = null;OutputStream outstream = null;try {outstream = sftp.put(file.getName());instream = new FileInputStream(file);byte b[] = new byte[1024];int n;try {while ((n = instream.read(b)) != -1) {outstream.write(b, 0, n);}} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();}} catch (SftpException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();} finally {try {outstream.flush();outstream.close();instream.close();} catch (Exception e2) {// TODO: handle exceptione2.printStackTrace();}}}}}⽂件地址。
java filehelper.readfile 使用方法 -回复

java filehelper.readfile 使用方法-回复如何使用Java的FileHelper类中的readFile方法。
Java的FileHelper类是一个文件操作工具类,提供了一些方便的方法来处理文件的读写操作。
其中,readFile方法用于读取文件内容。
本文将详细介绍如何使用FileHelper类中的readFile方法,让读取文件变得更加简单便捷。
步骤一:导入FileHelper类首先,在Java文件中导入FileHelper类。
可以通过以下代码实现:import com.example.FileHelper;这里假设FileHelper类位于com.example包下。
步骤二:创建FileHelper对象接下来,需要创建一个FileHelper对象。
可以通过以下代码实现:FileHelper fileHelper = new FileHelper();步骤三:指定文件路径在调用readFile方法之前,需要指定要读取的文件路径。
可以通过以下代码实现:String filePath = "path/to/your/file.txt"; 将路径替换为实际的文件路径请确保路径中的斜杠方向正确,Windows系统使用反斜杠(\),而大部分其他系统使用正斜杠(/)。
步骤四:调用readFile方法现在,可以调用readFile方法来读取文件内容。
可以通过以下代码实现:String fileContent = fileHelper.readFile(filePath);readFile方法将会返回一个字符串,其中包含了指定文件的全部内容。
可以将其赋值给一个字符串变量,以便后续的处理。
步骤五:处理读取到的文件内容一旦成功读取文件内容,接下来可以对其进行处理。
比如,可以将读取到的内容打印到控制台:System.out.println(fileContent);或者,可以将内容写入到另一个文件中:String outputFilePath = "path/to/your/output/file.txt"; 将路径替换为实际的输出文件路径fileHelper.writeFile(outputFilePath, fileContent);在这里,假设FileHelper类中还提供了一个writeFile方法,用于将内容写入到指定文件中。
lesson11-05 Java文件读写 -- zip文件读写

Java 核心技术第十一章Java文件读写第五节Zip文件读写1Java zip 包•压缩包:zip, rar, gz, ……•Java zip 包支持Zip和Gzip包的压缩和解压•zip文件操作类: java.util.zip包中–java.io.InputStream, java.io.OutputStream的子类–ZipInputStream, ZipOutputSream 压缩文件输入/输出流–ZipEntry 压缩项压缩•单个/多个压缩–打开输出zip文件–添加一个ZipEntry–打开一个输入文件,读数据,向ZipEntry写数据,关闭输入文件–重复以上两个步骤,写入多个文件到zip文件中–关闭zip文件–查看SingleFileZip.java 和MultipleFileZip.java解压•单个/多个解压–打开输入的zip文件–获取下一个ZipEntry–新建一个目标文件,从ZipEntry读取数据,向目标文件写入数据,关闭目标文件–重复以上两个步骤,从zip包中读取数据到多个目标文件–关闭zip文件–查看SingleFileUnzip.java 和MultipleFileUnzip.java总结•Java支持Zip和Gzip文件解压缩•重点在Entry和输入输出流向, 无需关注压缩算法代码(1) SingleFileZip.java代码(2) MultipleFileZip.java代码(3) MultipleFileZip.java代码(4) SingleFileUnzip.java代码(5) MultipleFileUnzip.java代码(6) MultipleFileUnzip.java代码(7) MultipleFileUnzip.java谢谢!13。
parquet java 读写
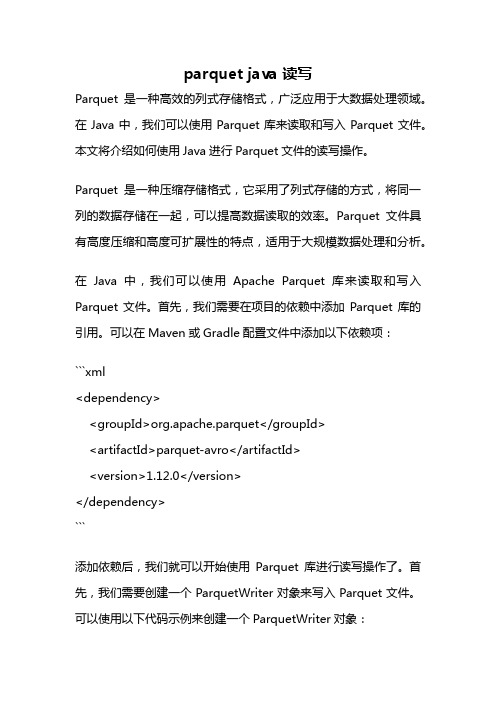
parquet java 读写Parquet是一种高效的列式存储格式,广泛应用于大数据处理领域。
在Java中,我们可以使用Parquet库来读取和写入Parquet文件。
本文将介绍如何使用Java进行Parquet文件的读写操作。
Parquet是一种压缩存储格式,它采用了列式存储的方式,将同一列的数据存储在一起,可以提高数据读取的效率。
Parquet文件具有高度压缩和高度可扩展性的特点,适用于大规模数据处理和分析。
在Java中,我们可以使用Apache Parquet库来读取和写入Parquet文件。
首先,我们需要在项目的依赖中添加Parquet库的引用。
可以在Maven或Gradle配置文件中添加以下依赖项:```xml<dependency><groupId>org.apache.parquet</groupId><artifactId>parquet-avro</artifactId><version>1.12.0</version></dependency>```添加依赖后,我们就可以开始使用Parquet库进行读写操作了。
首先,我们需要创建一个ParquetWriter对象来写入Parquet文件。
可以使用以下代码示例来创建一个ParquetWriter对象:```javaConfiguration conf = new Configuration();ParquetWriter<GenericRecord> writer = AvroParquetWriter.<GenericRecord>builder(newPath("output.parquet")).withSchema(schema).withConf(conf).build();```上述代码中,我们首先创建了一个Configuration对象,并将其传递给ParquetWriter的withConf方法。
filereader和filewriter用法

filereader和filewriter用法使用FileReader和FileWriter进行文件读写是Java中常用的操作之一。
FileReader用于读取文本文件的内容,而FileWriter用于向文本文件中写入内容。
下面将逐步介绍这两个类的使用方法。
一、FileReader的使用方法1. 导入FileReader类:首先,需要在Java程序中导入java.io.FileReader类,才能使用它的方法。
可以通过以下代码导入该类:javaimport java.io.FileReader;2. 创建FileReader对象:使用FileReader类的构造方法可以创建FileReader对象。
可以在构造方法中传递文件路径作为参数,示例如下:javaFileReader reader = new FileReader("文件路径");3. 读取文件内容:通过FileReader对象,可以使用read()方法逐字符读取文件内容,直到达到文件末尾。
读取的字符可以存储在一个字符数组或字符流中。
示例如下:javaint data;while((data = reader.read()) != -1) {char ch = (char) data;处理读取到的字符}4. 关闭FileReader对象:在文件读取完成后,需要关闭FileReader对象以释放系统资源。
关闭对象的方法是调用close()方法。
示例如下:javareader.close();二、FileWriter的使用方法1. 导入FileWriter类:同样,需要首先导入java.io.FileWriter类才能使用它的方法。
可以使用以下代码导入该类:javaimport java.io.FileWriter;2. 创建FileWriter对象:使用FileWriter类的构造方法可以创建FileWriter对象。
可以在构造方法中传递文件路径作为参数,示例如下:javaFileWriter writer = new FileWriter("文件路径");3. 写入文件内容:通过FileWriter对象,可以使用write()方法将字符、字符数组或字符串写入文件。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
1、按字节读取文件内容2、按字符读取文件内容3、按行读取文件内容4、随机读取文件内容public class ReadFromFile {/*** 以字节为单位读取文件,常用于读二进制文件,如图片、声音、影像等文件。
*/public static void readFileByBytes(String fileName) {File file = new File(fileName);InputStream in = null;try {System.out.println("以字节为单位读取文件内容,一次读一个字节:");// 一次读一个字节in = new FileInputStream(file);int tempbyte;while ((tempbyte = in.read()) != -1) {System.out.write(tempbyte);}in.close();} catch (IOException e) {e.printStackTrace();return;}try {System.out.println("以字节为单位读取文件内容,一次读多个字节:");// 一次读多个字节byte[] tempbytes = new byte[100];int byteread = 0;in = new FileInputStream(fileName);ReadFromFile.showAvailableBytes(in);// 读入多个字节到字节数组中,byteread为一次读入的字节数while ((byteread = in.read(tempbytes)) != -1) {System.out.write(tempbytes, 0, byteread);}} catch (Exception e1) {e1.printStackTrace();} finally {if (in != null) {try {in.close();} catch (IOException e1) {}}}}/*** 以字符为单位读取文件,常用于读文本,数字等类型的文件*/public static void readFileByChars(String fileName) {File file = new File(fileName);Reader reader = null;try {System.out.println("以字符为单位读取文件内容,一次读一个字节:");// 一次读一个字符reader = new InputStreamReader(new FileInputStream(fi le));int tempchar;while ((tempchar = reader.read()) != -1) {// 对于windows下,\r\n这两个字符在一起时,表示一个换行。
// 但如果这两个字符分开显示时,会换两次行。
// 因此,屏蔽掉\r,或者屏蔽\n。
否则,将会多出很多空行。
if (((char) tempchar) != '\r') {System.out.print((char) tempchar);}}reader.close();} catch (Exception e) {e.printStackTrace();}try {System.out.println("以字符为单位读取文件内容,一次读多个字节:");// 一次读多个字符char[] tempchars = new char[30];int charread = 0;reader = new InputStreamReader(new FileInputStream(fi leName));// 读入多个字符到字符数组中,charread为一次读取字符数while ((charread = reader.read(tempchars)) != -1) {// 同样屏蔽掉\r不显示if ((charread == tempchars.length)&& (tempchars[tempchars.length - 1] != '\r')) { System.out.print(tempchars);} else {for (int i = 0; i < charread; i++) {if (tempchars[i] == '\r') {continue;} else {System.out.print(tempchars[i]);}}}}} catch (Exception e1) {e1.printStackTrace();} finally {if (reader != null) {try {reader.close();} catch (IOException e1) {}}}}/*** 以行为单位读取文件,常用于读面向行的格式化文件*/public static void readFileByLines(String fileName) {File file = new File(fileName);BufferedReader reader = null;try {System.out.println("以行为单位读取文件内容,一次读一整行:");reader = new BufferedReader(new FileReader(file));String tempString = null;int line = 1;// 一次读入一行,直到读入null为文件结束while ((tempString = reader.readLine()) != null) {// 显示行号System.out.println("line " + line + ": " + tempString);line++;}reader.close();} catch (IOException e) {e.printStackTrace();} finally {if (reader != null) {try {reader.close();} catch (IOException e1) {}}}}/*** 随机读取文件内容*/public static void readFileByRandomAccess(String fileName) { RandomAccessFile randomFile = null;try {System.out.println("随机读取一段文件内容:");// 打开一个随机访问文件流,按只读方式randomFile = new RandomAccessFile(fileName, "r");// 文件长度,字节数long fileLength = randomFile.length();// 读文件的起始位置int beginIndex = (fileLength > 4) ? 4 : 0;// 将读文件的开始位置移到beginIndex位置。
randomFile.seek(beginIndex);byte[] bytes = new byte[10];int byteread = 0;// 一次读10个字节,如果文件内容不足10个字节,则读剩下的字节。
// 将一次读取的字节数赋给bytereadwhile ((byteread = randomFile.read(bytes)) != -1) {System.out.write(bytes, 0, byteread);}} catch (IOException e) {e.printStackTrace();} finally {if (randomFile != null) {try {randomFile.close();} catch (IOException e1) {}}}}/*** 显示输入流中还剩的字节数*/private static void showAvailableBytes(InputStream in) { try {System.out.println("当前字节输入流中的字节数为:" + in.available());} catch (IOException e) {e.printStackTrace();}}public static void main(String[] args) {String fileName = "C:/temp/newTemp.txt";ReadFromFile.readFileByBytes(fileName);ReadFromFile.readFileByChars(fileName);ReadFromFile.readFileByLines(fileName);ReadFromFile.readFileByRandomAccess(fileName);}}5、将内容追加到文件尾部public class AppendToFile {/*** A方法追加文件:使用RandomAccessFile*/public static void appendMethodA(String fileName, String co ntent) {try {// 打开一个随机访问文件流,按读写方式RandomAccessFile randomFile = new RandomAccessFile (fileName, "rw");// 文件长度,字节数long fileLength = randomFile.length();//将写文件指针移到文件尾。
randomFile.seek(fileLength);randomFile.writeBytes(content);randomFile.close();} catch (IOException e) {e.printStackTrace();}}/*** B方法追加文件:使用FileWriter*/public static void appendMethodB(String fileName, String co ntent) {try {//打开一个写文件器,构造函数中的第二个参数true表示以追加形式写文件FileWriter writer = new FileWriter(fileName, true);writer.write(content);writer.close();} catch (IOException e) {e.printStackTrace();}}public static void main(String[] args) {String fileName = "C:/temp/newTemp.txt";String content = "new append!";//按方法A追加文件AppendToFile.appendMethodA(fileName, content);AppendToFile.appendMethodA(fileName, "append end. \n ");//显示文件内容ReadFromFile.readFileByLines(fileName);//按方法B追加文件AppendToFile.appendMethodB(fileName, content);AppendToFile.appendMethodB(fileName, "append end. \n" );//显示文件内容ReadFromFile.readFileByLines(fileName);}}。