填充算法C++实现
魔方阵算法及C语言实现

魔方阵算法及C语言实现1 魔方阵概念魔方阵是指由1,2,3……n2填充的,每一行、每一列、对角线之和均相等的方阵,阶数n = 3,4,5…。
魔方阵也称为幻方阵。
例如三阶魔方阵为:魔方阵有什么的规律呢?魔方阵分为奇幻方和偶幻方。
而偶幻方又分为是4的倍数(如4,8,12……)和不是4的倍数(如6,10,14……)两种。
下面分别进行介绍。
2 奇魔方的算法2.1 奇魔方的规律与算法奇魔方(阶数n = 2 * m + 1,m =1,2,3……)规律如下:1.数字1位于方阵中的第一行中间一列;2.数字a(1 < a ≤ n2)所在行数比a-1行数少1,若a-1的行数为1,则a的行数为n;3.数字a(1 < a ≤ n2)所在列数比a-1列数大1,若a-1的列数为n,则a的列数为1;4.如果a-1是n的倍数,则a(1 < a ≤ n2)的行数比a-1行数大1,列数与a-1相同。
2.2 奇魔方算法的C语言实现1 #include <stdio.h> 2// Author: / 3// N为魔方阶数 4#define N 115 6int main()7{8int a[N][N]; 9int i;10 int col,row;1112 col = (N-1)/2;13 row = 0;1415a[row][col] = 1;1617for(i = 2; i <= N*N; i++)18 {19if((i-1)%N == 0 )20 {21 row++;22 }23else24 {25// if row = 0, then row = N-1, or row = row - 126 row--;27 row = (row+N)%N;2829// if col = N, then col = 0, or col = col + 130 col ++;31 col %= N;32 }33 a[row][col] = i;34 }35for(row = 0;row<N;row++)36 {37for(col = 0;col < N; col ++)38{39 printf("%6d",a[row][col]);40 }41printf("\n");42 }43return0;44 }3 偶魔方的算法偶魔方的情况比较特殊,分为阶数n = 4 * m(m =1,2,3……)的情况和阶数n = 4 * m + 2(m = 1,2,3……)情况两种。
填充三角形 c 算法 -回复
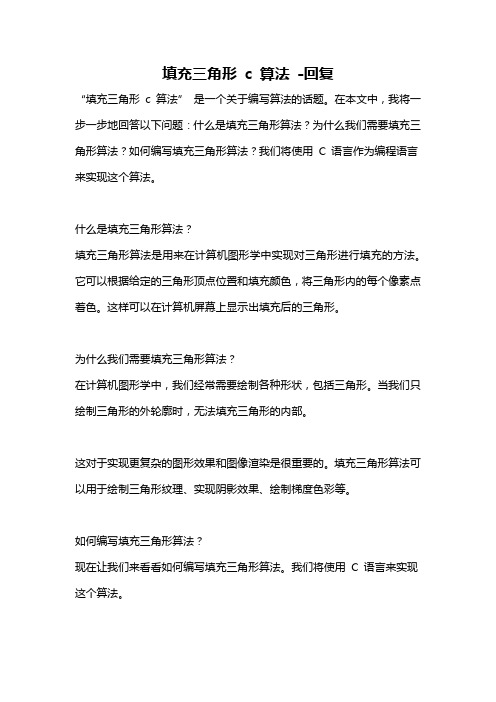
填充三角形c 算法-回复“填充三角形c 算法”是一个关于编写算法的话题。
在本文中,我将一步一步地回答以下问题:什么是填充三角形算法?为什么我们需要填充三角形算法?如何编写填充三角形算法?我们将使用C 语言作为编程语言来实现这个算法。
什么是填充三角形算法?填充三角形算法是用来在计算机图形学中实现对三角形进行填充的方法。
它可以根据给定的三角形顶点位置和填充颜色,将三角形内的每个像素点着色。
这样可以在计算机屏幕上显示出填充后的三角形。
为什么我们需要填充三角形算法?在计算机图形学中,我们经常需要绘制各种形状,包括三角形。
当我们只绘制三角形的外轮廓时,无法填充三角形的内部。
这对于实现更复杂的图形效果和图像渲染是很重要的。
填充三角形算法可以用于绘制三角形纹理、实现阴影效果、绘制梯度色彩等。
如何编写填充三角形算法?现在让我们来看看如何编写填充三角形算法。
我们将使用C 语言来实现这个算法。
步骤1:确定画布大小和三角形顶点坐标首先,我们需要确定画布的大小,并且使用三个点来定义三角形。
我们将使用屏幕上的坐标系,左上角为原点,x 轴向右增长,y 轴向下增长。
我们可以定义如下的数据结构来表示点的坐标:ctypedef struct {int x;int y;} Point;步骤2:绘制三角形外轮廓接下来,我们编写一个函数来绘制三角形的外轮廓。
我们可以使用直线绘制算法(如Bresenham 算法)来连接三个顶点,绘制三角形的外轮廓。
cvoid drawTriangle(Point p1, Point p2, Point p3) {drawLine(p1, p2);drawLine(p2, p3);drawLine(p3, p1);}步骤3:计算三角形的边界框在填充三角形之前,我们需要计算三角形的边界框。
边界框是一个矩形,它包围了完全包含三角形的最小区域。
我们可以定义如下的数据结构来表示边界框:ctypedef struct {int xMin;int xMax;int yMin;int yMax;} BoundingBox;为了计算边界框,我们可以找到三个顶点的最小和最大x、y 坐标。
c语言多边形区域填充算法

c语言多边形区域填充算法C语言多边形区域填充算法一、介绍多边形区域填充算法是计算机图形学中的一项重要技术,用于将给定的多边形区域进行填充,使其呈现出丰富的颜色或纹理,增强图形的效果和表现力。
本文将介绍一种常用的C语言多边形区域填充算法——扫描线填充算法。
二、扫描线填充算法原理扫描线填充算法是一种基于扫描线的填充方法,其基本思想是将多边形区域按照水平扫描线的顺序,从上到下逐行扫描,通过判断扫描线与多边形边界的交点个数来确定是否进入多边形区域。
具体步骤如下:1. 首先,确定多边形的边界,将其存储为一个边表。
边表中的每个边都包含起点和终点的坐标。
2. 创建一个活性边表(AET),用于存储当前扫描线与多边形边界的交点。
初始时,AET为空。
3. 从上到下逐行扫描多边形区域,对每一条扫描线,从边表中找出与该扫描线相交的边,并将其加入AET中。
4. 对于AET中的每一对交点,按照从左到右的顺序两两配对,形成水平线段,将其填充为指定的颜色或纹理。
5. 在扫描线的下一行,更新AET中的交点的坐标,然后重复步骤4,直到扫描到多边形区域的底部。
三、代码实现下面是一个简单的C语言实现扫描线填充算法的示例代码:```#include <stdio.h>#include <stdlib.h>#include <stdbool.h>typedef struct {int x;int y;} Point;typedef struct {int yMax;float x;float dx;int next;} Edge;void fillPolygon(int n, Point* points, int color) {// 获取多边形的边界int yMin = points[0].y;int yMax = points[0].y;for (int i = 1; i < n; i++) {if (points[i].y < yMin) {yMin = points[i].y;}if (points[i].y > yMax) {yMax = points[i].y;}}// 创建边表Edge* edges = (Edge*)malloc(sizeof(Edge) * n);int k = n - 1;for (int i = 0; i < n; i++) {if (points[i].y < points[k].y) {edges[i].yMax = points[k].y;edges[i].x = points[i].x;edges[i].dx = (float)(points[k].x - points[i].x) / (points[k].y - points[i].y);edges[i].next = k;} else {edges[i].yMax = points[i].y;edges[i].x = points[k].x;edges[i].dx = (float)(points[i].x - points[k].x) / (points[i].y - points[k].y);edges[i].next = i;}k = i;}// 扫描线填充for (int y = yMin; y < yMax; y++) {int xMin = INT_MAX;int xMax = INT_MIN;for (int i = 0; i < n; i++) {if (y >= edges[i].yMax) {continue;}edges[i].x += edges[i].dx;if (edges[i].x < xMin) {xMin = edges[i].x;}if (edges[i].x > xMax) {xMax = edges[i].x;}int j = edges[i].next;while (j != i) {edges[j].x += edges[j].dx; if (edges[j].x < xMin) {xMin = edges[j].x;}if (edges[j].x > xMax) {xMax = edges[j].x;}j = edges[j].next;}}for (int x = xMin; x < xMax; x++) { drawPixel(x, y, color);}}free(edges);}int main() {// 定义多边形的顶点坐标Point points[] = {{100, 100},{200, 200},{300, 150},{250, 100}};// 填充多边形区域为红色fillPolygon(4, points, RED);return 0;}```四、总结通过扫描线填充算法,我们可以实现对多边形区域的填充,从而提升图形的表现效果。
图形填充之种子填充算法

图形填充之种⼦填充算法编译器:VS2013算法:在图形内选择⼀个点为种⼦,然后对这个种⼦四⽅位坐标未着⾊的⼊栈,出栈便着⾊,如此重复,等到栈内为空,则着⾊完成代码:1 #include "stdafx.h"2 #include<stdio.h>3 #include"graphics.h"4 #include<stdlib.h>5 #include<stack>67using namespace std;89//定义结构体存储像素坐标10struct Point11 {12int x;13int y;14 };1516//函数声明17void Boundaryfilling(Point a[], int n);18void judgecolor(int x, int y, stack<int> &sx, stack<int> &sy);1920int main()21 {22int gdriver = DETECT, gmode, n, i;2324 printf("please input number of point:\n");25 scanf_s("%d", &n);2627 Point *p=(Point *)malloc(n*sizeof(Point)); //动态分配内存2829 printf("please input point :\n");30for (i = 0; i < n; i++)31 scanf_s("%d%d", &p[i].x, &p[i].y);3233 initgraph(&gdriver, &gmode, "");3435 setcolor(BLUE);36 setbkcolor(BLACK);3738//画出多边形39for (i = 0; i < n-1; i++)40 line(p[i].x, p[i].y, p[i + 1].x, p[i + 1].y);4142 Boundaryfilling(p, n);4344 system("pause");45 closegraph();4647return0;48 }4950void Boundaryfilling(Point a[], int n)51 {52 stack<int> sx,sy;53int x=0 , y=0 ,x0,y0,i;5455for (i = 0; i < n; i++)56 {57 x += a[i].x;58 y += a[i].y;59 }6061 x = x / (n-1);62 y = y / (n-1);6364 sx.push(x);//x坐标⼊栈65 sy.push(y);//y坐标⼊栈6667while (!sx.empty()) //判断栈是否为空68 {69 x0 = sx.top();70 y0 = sy.top();7172 putpixel(sx.top(), sy.top(), YELLOW); //栈顶元素着⾊73 sx.pop();//栈顶元素出栈74 sy.pop();//栈顶元素出栈7576 judgecolor(x0 - 1, y0, sx, sy);//左边点77 judgecolor(x0 + 1, y0, sx, sy);//右边点78 judgecolor(x0, y0 - 1, sx, sy);//下边点79 judgecolor(x0, y0 + 1, sx, sy);//上边点80 }81 }8283//判断该像素是否没有着⾊84void judgecolor(int x, int y,stack<int> &sx,stack<int> &sy) 85 {86if (getpixel(x, y) == BLACK)87 {88 sx.push(x);89 sy.push(y);90 }91 }结果:。
区域填充算法的实现
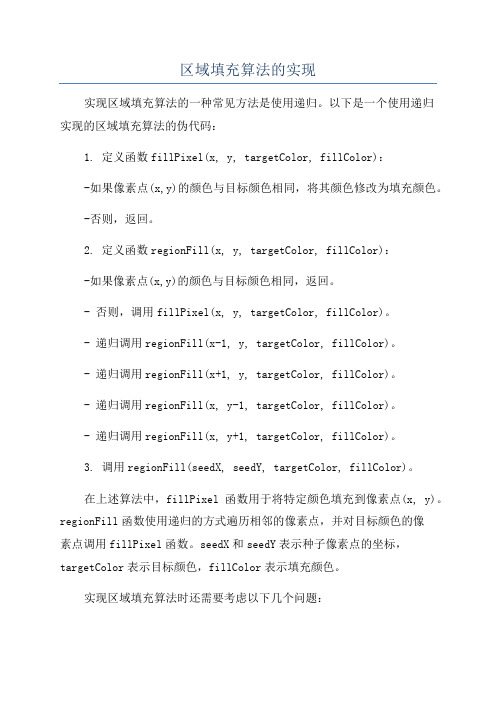
区域填充算法的实现实现区域填充算法的一种常见方法是使用递归。
以下是一个使用递归实现的区域填充算法的伪代码:1. 定义函数fillPixel(x, y, targetColor, fillColor):-如果像素点(x,y)的颜色与目标颜色相同,将其颜色修改为填充颜色。
-否则,返回。
2. 定义函数regionFill(x, y, targetColor, fillColor):-如果像素点(x,y)的颜色与目标颜色相同,返回。
- 否则,调用fillPixel(x, y, targetColor, fillColor)。
- 递归调用regionFill(x-1, y, targetColor, fillColor)。
- 递归调用regionFill(x+1, y, targetColor, fillColor)。
- 递归调用regionFill(x, y-1, targetColor, fillColor)。
- 递归调用regionFill(x, y+1, targetColor, fillColor)。
3. 调用regionFill(seedX, seedY, targetColor, fillColor)。
在上述算法中,fillPixel函数用于将特定颜色填充到像素点(x, y)。
regionFill函数使用递归的方式遍历相邻的像素点,并对目标颜色的像素点调用fillPixel函数。
seedX和seedY表示种子像素点的坐标,targetColor表示目标颜色,fillColor表示填充颜色。
实现区域填充算法时还需要考虑以下几个问题:1.像素点的表示:图像可以由二维数组表示,其中每个元素表示一个像素点的颜色。
2.填充颜色选择:填充颜色可以由RGB值表示,或者在预定义的颜色集合中选择。
3.边界处理:对于位于图像边界上的种子像素点,需要特殊处理以防止数组越界错误。
4.递归终止条件:填充算法使用递归,需要定义递归终止的条件,以防止无限递归。
c语言将二叉树填充为完全二叉树的方法

c语言将二叉树填充为完全二叉树的方法要将二叉树填充为完全二叉树,需要按照层次遍历方式进行操作。
下面是使用C语言来实现这个过程的方法:#include <stdio.h>#include <stdlib.h>#define MAX_SIZE 100// 二叉树节点结构体typedef struct Node {int data; // 当前节点的数据struct Node* left; // 左子节点指针struct Node* right; // 右子节点指针} Node;// 辅助队列结构体typedef struct Queue {Node* data[MAX_SIZE];int front, rear;} Queue;// 创建新节点Node* createNode(int data) {Node* newNode = (Node*)malloc(sizeof(Node)); newNode->data = data;newNode->left = NULL;newNode->right = NULL;return newNode;}// 插入节点Node* insertNode(Node* root, int data) {if (root == NULL) {return createNode(data);}Queue queue;queue.front = -1;queue.rear = -1;queue.data[++queue.rear] = root;while (queue.front != queue.rear) {Node* temp = queue.data[++queue.front];if (temp->left) {queue.data[++queue.rear] = temp->left; } else {temp->left = createNode(data);return root;}if (temp->right) {queue.data[++queue.rear] = temp->right; } else {temp->right = createNode(data);return root;}}return root;}// 中序遍历打印二叉树void inorderTraversal(Node* root) {if (root == NULL) {return;}inorderTraversal(root->left);printf("%d ", root->data);inorderTraversal(root->right);}int main() {Node* root = createNode(1);// 假设以下数据为二叉树的节点数据int data[] = {2, 3, 4, 5, 6, 7};int dataSize = sizeof(data) / sizeof(data[0]);for (int i = 0; i < dataSize; i++) {root = insertNode(root, data[i]);}printf("填充完全二叉树后的中序遍历结果:");inorderTraversal(root);return 0;}通过以上代码,我们可以将给定的二叉树填充为完全二叉树,并通过中序遍历方式打印出填充完后的结果。
计算机图形学四连通区域种子填充算法实验
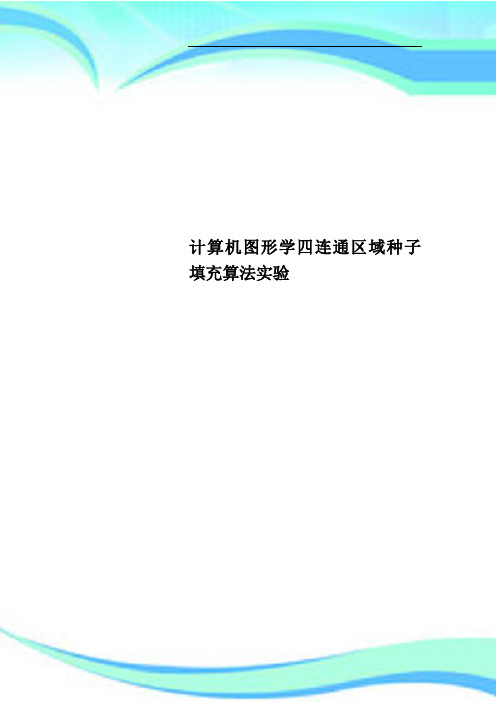
计算机图形学四连通区域种子填充算法实验————————————————————————————————作者: ————————————————————————————————日期:ﻩ《计算机图形学实验》报告任课教师:钱文华2016年春季学期实验:四连通区域种子填充算法实验时间:2016年12月8日实验地点:信息学院2204实验目的:掌握种子填充算法的原理,并会用种子填充算法和opengl并结合使用c++语言编写程序绘制多边形。
实验原理:种子填充算法又称为边界填充算法。
其基本思想是:从多边形区域的一个内点开始,由内向外用给定的颜色画点直到边界为止。
如果边界是以一种颜色指定的,则种子填充算法可逐个像素地处理直到遇到边界颜色为止。
内点的检测条件:if(interiorColor!=bo rderColor&&interiorColor!=fillColor)。
种子填充算法常用四连通域和八连通域技术进行填充操作。
从区域内任意一点出发,通过上、下、左、右四个方向到达区域内的任意像素。
用这种方法填充的区域就称为四连通域;这种填充方法称为四向连通算法。
从区域内任意一点出发,通过上、下、左、右、左上、左下、右上和右下八个方向到达区域内的任意像素。
用这种方法填充的区域就称为八连通域;这种填充方法称为八向连通算法。
一般来说,八向连通算法可以填充四向连通区域,而四向连通算法有时不能填充八向连通区域。
四向连通填充算法:a)种子像素压入栈中;b)如果栈为空,则转e);否则转c);c) 弹出一个像素,并将该像素置成填充色;并判断该像素相邻的四连通像素是否为边界色或已经置成多边形的填充色,若不是,则将该像素压入栈;d)转b);e)结束。
四连通填充算法利用到了递归的思想。
本实验只包括四连通填充算法程序代码:#include<glut.h>#include<stdlib.h>#include<math.h>#include<windows.h>voidinit(void){ glClearColor(1.0,1.0,1.0,0.0);glMatrixMode(GL_PROJECTION);gluOrtho2D(0.0,300.0,0.0,300.0);}void setPixel(intx,inty,longfillColor){ glColor3f(fillColor<<16,fillColor<<8,fillColor);glBegin(GL_POINTS);glVertex2i(x,y);glEnd();}voidboundaryFill4(int x,inty,long fillColor,long borderColor){ unsignedchar params[3];long interiorColor;glReadPixels(x,y,1,1,GL_RGB,GL_UNSIGNED_BYTE,par ams);interiorColor=RGB(params[0],params[1],params[2]);if(interiorColor!=borderColor&&interiorColor!=fillColor){ setPixel(x,y,fillColor);boundaryFill4(x+1,y,fillColor,borderColor);boundaryFill4(x-1,y,fillColor,borderColor); boundaryFill4(x,y+1,fillColor,borderColor);boundaryFill4(x,y-1,fillColor,borderColor);} }voidlineSegment(void) {long borderColor=RGB(255,0,0);longfillColor=RGB(0,0,255);glClear(GL_COLOR_BUFFER_BIT); glColor3f(255,0,0); glBegin(GL_LINE_LOOP);glVertex2i(0,40);glVertex2i(20,0);glVertex2i(60,0);glVertex2i(80,40);glVertex2i(60,80);glVertex2i(20,80);glEnd();boundaryFill4(60,60,fillColor,borderColor);glFlush();}voidmain(int argc,char**argv){glutInit(&ar gc,argv);glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB); glutInitWindowPosition(150,100);glutInitWindowSize(300,300);glutCreateWindow("种子填充");init();glutDisplayFunc(lineSegment);glutMainLoop();}上实验课时机房的实验结果:后来的实验结果:glVertex2i(0,40);glVertex2i(20,0);glVertex2i(60,0);glVertex2i(80,40);glVertex2i(60,80);glVertex2i(20,80);glEnd();boundaryFill4(60,60,fillColor,borderColor);以上这段程序改成如下glVertex2i(90,40);glVertex2i(120, 100);glVertex2i(90,160);glVertex2i(60, 160);glVertex2i(60, 40);glEnd();boundaryFill4(70,60,fillColor,borderColor); 改变参数后:再把glVertex2i(90,40);glVertex2i(120, 100);glVertex2i(90,160);glVertex2i(60, 160);glVertex2i(60, 40);glEnd();boundaryFill4(70,60,fillColor,borderColor);改成glVertex2i(100, 100);glVertex2i(200, 100);glVertex2i(150,150);//glVertex2i(60, 160);//glVertex2i(60, 40);glEnd();boundaryFill4(150,120,fillColor,borderColor);后的结果如下图:实验总结:通过多组数据的测试,知道了上面算法的正确,普适性。
C语言实现MD5算法

C语言实现MD5算法MD5(Message-Digest Algorithm 5)是一种常用的哈希函数算法,广泛用于验证数据完整性、密码存储和数字证书等领域。
下面是使用C语言实现MD5算法的代码。
这段代码包含了MD5算法的各个步骤,包括初始化MD5结构体、填充数据、更新状态、计算摘要等。
```c#include <stdio.h>#include <stdint.h>#include <string.h>//定义MD5常量#define B 0xEFCDAB89#define C 0x98BADCFE//循环左移宏定义#define LEFT_ROTATE(x, n) (((x) << (n)) , ((x) >> (32-(n)))) //填充消息void padMessage(uint8_t *message, uint32_t length)//计算需要填充的字节数uint32_t padLength = (length % sizeof(uint32_t) == 56) ? 64 : 56;padLength = padLength - (length % sizeof(uint32_t));//填充1位1message[length++] = 0x80;//填充0位for (uint32_t i = 0; i < padLength; i++) message[length++] = 0x00;}//在消息末尾添加原始消息的长度(以位表示)for (uint32_t i = 0; i < sizeof(uint32_t); i++) message[length++] = (length << 3) >> (i * 8); }//初始化MD5结构体void initMD5(uint32_t *state)state[0] = A;state[1] = B;state[2] = C;state[3] = D;//更新状态void updateState(uint32_t *state, uint32_t *M)uint32_t A = state[0], B = state[1], C = state[2], D = state[3];//定义MD5循环运算函数#define MD5_FUNCTION(a, b, c, d, k, s, i) \a=b+LEFT_ROTATE((a+F(b,c,d)+M[k]+T[i]),s)//迭代压缩消息MD5_FUNCTION(A,B,C,D,0,7,1);MD5_FUNCTION(D,A,B,C,1,12,2);MD5_FUNCTION(C,D,A,B,2,17,3);MD5_FUNCTION(B,C,D,A,3,22,4);MD5_FUNCTION(A,B,C,D,4,7,5);MD5_FUNCTION(D,A,B,C,5,12,6);MD5_FUNCTION(C,D,A,B,6,17,7);MD5_FUNCTION(B,C,D,A,7,22,8);MD5_FUNCTION(A,B,C,D,8,7,9);MD5_FUNCTION(D,A,B,C,9,12,10);MD5_FUNCTION(C,D,A,B,10,17,11);MD5_FUNCTION(B,C,D,A,11,22,12);MD5_FUNCTION(A,B,C,D,12,7,13);MD5_FUNCTION(C,D,A,B,14,17,15); MD5_FUNCTION(B,C,D,A,15,22,16); MD5_FUNCTION(A,B,C,D,1,5,17); MD5_FUNCTION(D,A,B,C,6,9,18); MD5_FUNCTION(C,D,A,B,11,14,19); MD5_FUNCTION(B,C,D,A,0,20,20); MD5_FUNCTION(A,B,C,D,5,5,21); MD5_FUNCTION(D,A,B,C,10,9,22); MD5_FUNCTION(C,D,A,B,15,14,23); MD5_FUNCTION(B,C,D,A,4,20,24); MD5_FUNCTION(A,B,C,D,9,5,25); MD5_FUNCTION(D,A,B,C,14,9,26); MD5_FUNCTION(C,D,A,B,3,14,27); MD5_FUNCTION(B,C,D,A,8,20,28); MD5_FUNCTION(A,B,C,D,13,5,29); MD5_FUNCTION(D,A,B,C,2,9,30); MD5_FUNCTION(C,D,A,B,7,14,31); MD5_FUNCTION(B,C,D,A,12,20,32);MD5_FUNCTION(D,A,B,C,8,11,34); MD5_FUNCTION(C,D,A,B,11,16,35); MD5_FUNCTION(B,C,D,A,14,23,36); MD5_FUNCTION(A,B,C,D,1,4,37); MD5_FUNCTION(D,A,B,C,4,11,38); MD5_FUNCTION(C,D,A,B,7,16,39); MD5_FUNCTION(B,C,D,A,10,23,40); MD5_FUNCTION(A,B,C,D,13,4,41); MD5_FUNCTION(D,A,B,C,0,11,42); MD5_FUNCTION(C,D,A,B,3,16,43); MD5_FUNCTION(B,C,D,A,6,23,44); MD5_FUNCTION(A,B,C,D,9,4,45); MD5_FUNCTION(D,A,B,C,12,11,46); MD5_FUNCTION(C,D,A,B,15,16,47); MD5_FUNCTION(B,C,D,A,2,23,48); MD5_FUNCTION(A,B,C,D,0,6,49); MD5_FUNCTION(D,A,B,C,7,10,50); MD5_FUNCTION(C,D,A,B,14,15,51);MD5_FUNCTION(A,B,C,D,12,6,53); MD5_FUNCTION(D,A,B,C,3,10,54); MD5_FUNCTION(C,D,A,B,10,15,55); MD5_FUNCTION(B,C,D,A,1,21,56); MD5_FUNCTION(A,B,C,D,8,6,57); MD5_FUNCTION(D,A,B,C,15,10,58); MD5_FUNCTION(C,D,A,B,6,15,59); MD5_FUNCTION(B,C,D,A,13,21,60); MD5_FUNCTION(A,B,C,D,4,6,61); MD5_FUNCTION(D,A,B,C,11,10,62); MD5_FUNCTION(C,D,A,B,2,15,63); MD5_FUNCTION(B,C,D,A,9,21,64); #undef MD5_FUNCTION//更新状态state[0] += A;state[1] += B;state[2] += C;state[3] += D;//计算MD5摘要void md5(uint8_t *message, uint32_t length, uint32_t *digest) //初始化MD5结构体uint32_t state[4];initMD5(state);//填充消息padMessage(message, length);//计算消息分组数量uint32_t numOfBlocks = length / 64;//处理每个分组for (uint32_t i = 0; i < numOfBlocks; i++)uint32_t M[16];memcpy(M, message + (i * 64), 64);//更新状态updateState(state, M);}//获取MD5摘要memcpy(digest, state, 16);int mai//测试用例uint8_t message[] = "Hello, MD5!";uint32_t length = sizeof(message) - 1;//计算MD5摘要uint32_t digest[4];md5(message, length, digest);//输出摘要printf("MD5 Digest: ");for (int i = 0; i < 4; i++)printf("%02x", ((uint8_t*)digest)[i]);}printf("\n");return 0;```以上是使用C语言实现MD5算法的代码。
一维数组快速填充算法 -回复

一维数组快速填充算法-回复一维数组快速填充算法是一种用于将一维数组按照特定规则填充数据的算法。
它可以非常高效地将一维数组填充为指定的值,加快数据处理的速度。
在本文中,我将一步一步回答关于一维数组快速填充算法的问题,并详细介绍其原理和实现方法。
首先,我们需要清楚地了解什么是一维数组。
一维数组是一种最简单的数据结构,它由相同类型的元素组成,并按照一定的顺序排列。
一维数组通常用于存储大量的数据,例如存储学生的考试成绩、员工的工号等。
在计算机科学领域,我们经常需要对一维数组进行操作,而一维数组快速填充算法就是其中的一种。
接下来,我将介绍一维数组快速填充算法的原理。
该算法的主要思想是通过循环遍历数组的每个元素,将指定的值填充到数组中。
这种填充方式有多种实现方法,例如可以使用普通的循环语句、递归函数或者库函数。
这些方法各有优劣,主要取决于具体的应用场景和性能需求。
然后,让我们来看一下一维数组快速填充算法的具体实现方法。
以下是一种基于循环的填充算法:1. 首先,我们需要定义一个一维数组。
可以使用编程语言提供的数组数据类型,或者使用指针和动态内存分配来创建数组。
例如,在C语言中可以使用int类型的数组来存储整数数据。
2. 接下来,我们需要定义一个变量来存储填充的值。
这个值可以是任何与数组元素类型兼容的数据,例如整数、字符或者自定义的数据类型。
3. 然后,我们使用一个循环语句遍历数组的每个元素,并将填充的值赋给数组元素。
循环的次数通常与数组的长度相同,可以使用数组的长度属性或者计算得到。
4. 最后,我们输出或使用填充后的数组。
可以将数组的值打印到控制台上,或者将其传递给其他函数进行进一步处理。
该算法的时间复杂度为O(n),其中n是数组的长度。
这是因为我们需要遍历数组的每个元素,将填充的值复制到数组中。
如果使用递归函数来实现填充算法,时间复杂度可能更高,取决于递归的深度和执行效率。
虽然一维数组快速填充算法非常简单,但在实际应用中却具有重要的意义。
C语言图形的填充函数
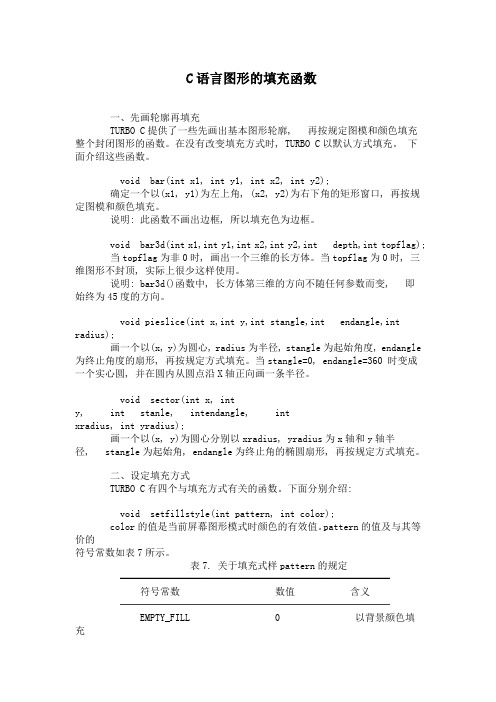
C语言图形的填充函数一、先画轮廓再填充TURBO C提供了一些先画出基本图形轮廓, 再按规定图模和颜色填充整个封闭图形的函数。
在没有改变填充方式时, TURBO C以默认方式填充。
下面介绍这些函数。
void bar(int x1, int y1, int x2, int y2);确定一个以(x1, y1)为左上角, (x2, y2)为右下角的矩形窗口, 再按规定图模和颜色填充。
说明: 此函数不画出边框, 所以填充色为边框。
void bar3d(int x1,int y1,int x2,int y2,int depth,int topflag);当topflag为非0时, 画出一个三维的长方体。
当topflag为0时, 三维图形不封顶, 实际上很少这样使用。
说明: bar3d()函数中, 长方体第三维的方向不随任何参数而变, 即始终为45度的方向。
void pieslice(int x,int y,int stangle,int endangle,int radius);画一个以(x, y)为圆心, radius为半径, stangle为起始角度, endangle 为终止角度的扇形, 再按规定方式填充。
当stangle=0, endangle=360 时变成一个实心圆, 并在圆内从圆点沿X轴正向画一条半径。
void sector(int x, int y, int stanle, intendangle, int xradius, int yradius);画一个以(x, y)为圆心分别以xradius, yradius为x轴和y轴半径, stangle为起始角, endangle为终止角的椭圆扇形, 再按规定方式填充。
二、设定填充方式TURBO C有四个与填充方式有关的函数。
下面分别介绍:void setfillstyle(int pattern, int color);color的值是当前屏幕图形模式时颜色的有效值。
实验三区域四连通填充算法

实验三区域四连通填充算法
1. 课程名称:计算机图形学 2. 实验目的和要求: 目的:理解、掌握区域填充算法原理。 要求:读懂示范代码并对其改进。 3. 实验题目:区域四连通填充算法 4. 实验过程: (1) 复习区域填充算法原理; (2) 根据算法原理,读懂示范代码; (3) 尝试在示范代码的基础上,实现扫描线填充算法。 5. 实验结果 6. 实验分析 试比较扫描线填充算法与简单
C语言图形的填充函数
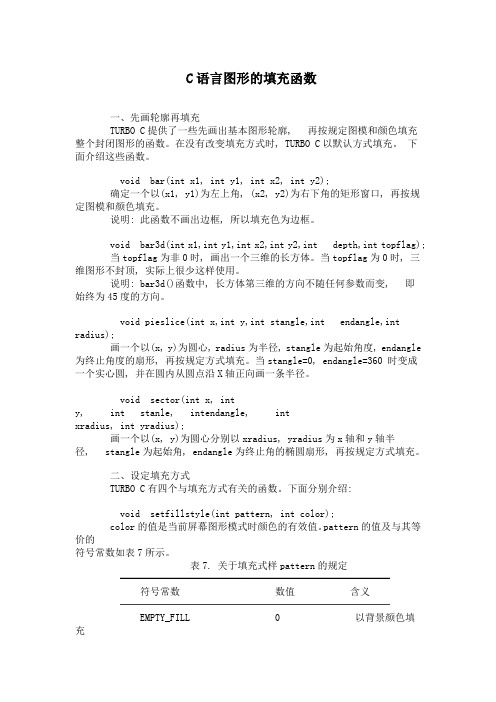
C语言图形的填充函数一、先画轮廓再填充TURBO C提供了一些先画出基本图形轮廓, 再按规定图模和颜色填充整个封闭图形的函数。
在没有改变填充方式时, TURBO C以默认方式填充。
下面介绍这些函数。
void bar(int x1, int y1, int x2, int y2);确定一个以(x1, y1)为左上角, (x2, y2)为右下角的矩形窗口, 再按规定图模和颜色填充。
说明: 此函数不画出边框, 所以填充色为边框。
void bar3d(int x1,int y1,int x2,int y2,int depth,int topflag);当topflag为非0时, 画出一个三维的长方体。
当topflag为0时, 三维图形不封顶, 实际上很少这样使用。
说明: bar3d()函数中, 长方体第三维的方向不随任何参数而变, 即始终为45度的方向。
void pieslice(int x,int y,int stangle,int endangle,int radius);画一个以(x, y)为圆心, radius为半径, stangle为起始角度, endangle 为终止角度的扇形, 再按规定方式填充。
当stangle=0, endangle=360 时变成一个实心圆, 并在圆内从圆点沿X轴正向画一条半径。
void sector(int x, int y, int stanle, intendangle, int xradius, int yradius);画一个以(x, y)为圆心分别以xradius, yradius为x轴和y轴半径, stangle为起始角, endangle为终止角的椭圆扇形, 再按规定方式填充。
二、设定填充方式TURBO C有四个与填充方式有关的函数。
下面分别介绍:void setfillstyle(int pattern, int color);color的值是当前屏幕图形模式时颜色的有效值。
区域填充算法运行代码
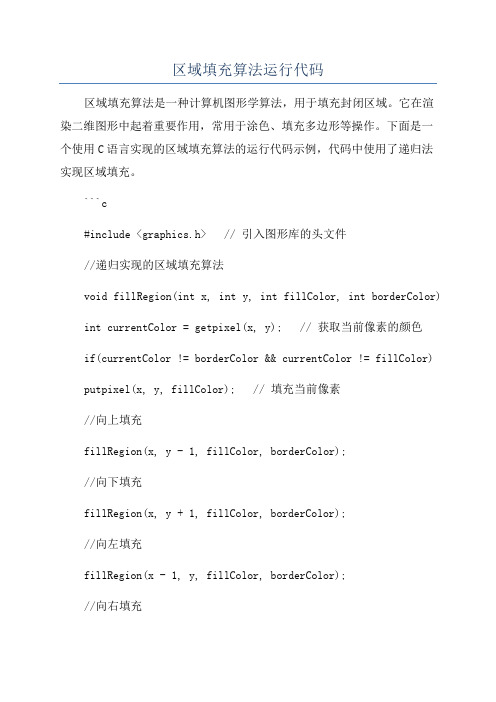
区域填充算法运行代码区域填充算法是一种计算机图形学算法,用于填充封闭区域。
它在渲染二维图形中起着重要作用,常用于涂色、填充多边形等操作。
下面是一个使用C语言实现的区域填充算法的运行代码示例,代码中使用了递归法实现区域填充。
```c#include <graphics.h> // 引入图形库的头文件//递归实现的区域填充算法void fillRegion(int x, int y, int fillColor, int borderColor) int currentColor = getpixel(x, y); // 获取当前像素的颜色if(currentColor != borderColor && currentColor != fillColor) putpixel(x, y, fillColor); // 填充当前像素//向上填充fillRegion(x, y - 1, fillColor, borderColor);//向下填充fillRegion(x, y + 1, fillColor, borderColor);//向左填充fillRegion(x - 1, y, fillColor, borderColor);//向右填充fillRegion(x + 1, y, fillColor, borderColor);}int mainint gd = DETECT, gm; // 图形模式和图形驱动程序检测initgraph(&gd, &gm, ""); // 初始化图形界面//绘制一个闭合多边形作为填充区域int poly[8] = {100, 100, 200, 100, 200, 200, 100, 200};drawpoly(4, poly);//设置填充颜色和边界颜色int fillColor = YELLOW;int borderColor = BLACK;//调用区域填充函数fillRegion(150, 150, fillColor, borderColor);getch(;closegraph(;return 0;```在上面的代码中,我们首先引入了图形库的头文件`graphics.h`,然后使用`initgraph`函数初始化了图形界面。
(计算机图形学)多边形区域扫描线填充或种子填充

实验2:多边形区域扫描线填充或种子填充实验类型:验证、设计所需时间:3学时主要实验内容及要求:实现多边形区域扫描线填充的有序边表算法,并将实现的算法应用于任意多边形的填充,要求多边形的顶点由键盘输入或鼠标拾取,填充要准确,不能多填也不能少填。
要求掌握边形区域扫描线填充的有序边表算法的基本原理和算法设计,画出算法实现的程序流程图,使用C或者VC++实现算法,并演示。
参考试验步骤:1)分析多边形区域扫描线填充算法的原理,确定算法流程①初始化:构造边表,AET表置空②将第一个不空的ET表中的边插入AET表③由AET表取出交点进行配对(奇偶)获得填充区间,依次对这些填充区间着色④y=y i+1时,根据x=x i+1/k修改AET表所有结点中交点的x坐标。
同时如果相应的ET表不空,则将其中的结点插入AET表,形成新的AET表⑤AET表不空,则转(3),否则结束。
2)编程实现①首先确定多边形顶点和ET/AET表中结点的结构②编写链表相关操作(如链表结点插入、删除和排序等)③根据1)中的算法结合上述已有的链表操作函数实现多边形区域扫描线填充的主体功能④编写主函数,测试该算法源代码:#include<gl/glut.h>#include<iostream>using namespace std;typedef struct dePt{int x;int y;}dePt;void fill(GLint x1,GLint y1,GLint z1){glBegin(GL_POINTS);glVertex3f(x1,y1,0.0f);glEnd();}typedef struct Edge{int yUpper;float xIntersect, dxPerScan;struct Edge *next;}Edge;void insertEdge(Edge *list, Edge *edge){Edge *p,*q=list;p=q->next;while(p!=NULL){if(edge->xIntersect<p->xIntersect)p=NULL;else{q=p;p=p->next;}}edge->next=q->next;q->next=edge;}int yNext(int k, int cnt, dePt*pts){int j;if((k+1)>(cnt-1))j=0;elsej=k+1;while(pts[k].y==pts[j].y)if((j+1)>(cnt-1))j=0;else j++;return (pts[j].y);}void makeEdgeRec(dePt lower, dePt upper,int yComp,Edge *edge,Edge *edges[]) {edge->dxPerScan=(float)(upper.x-lower.x)/(upper.y-lower.y);edge->xIntersect=lower.x;if(upper.y<yComp)edge->yUpper=upper.y-1;elseedge->yUpper=upper.y;insertEdge(edges[lower.y],edge);}void buildEdgeList(int cnt,dePt *pts,Edge *edges[]){Edge *edge;dePt v1,v2;int i,yPrev=pts[cnt-2].y;v1.x=pts[cnt-1].x;v1.y=pts[cnt-1].y;for(i=0;i<cnt;i++){v2=pts[i];if(v1.y!=v2.y){edge=(Edge *)malloc(sizeof(Edge));if(v1.y<v2.y)makeEdgeRec(v1,v2,yNext(i,cnt,pts),edge,edges);elsemakeEdgeRec(v2,v1,yPrev,edge,edges);}yPrev=v1.y;v1=v2;}}void buildActiveList(int scan,Edge *active,Edge *edges[]) {Edge *p,*q;p=edges[scan]->next;while(p){q=p->next;insertEdge(active,p);p=q;}}void fillScan(int scan,Edge *active){Edge *p1,*p2;int i;p1=active->next;while(p1){p2=p1->next;for(i=p1->xIntersect;i<p2->xIntersect;i++)fill((int)i,scan,3);p1=p2->next;}}void deleteAfter(Edge *q){Edge *p=q->next;q->next=p->next;free(p);}void updateActiveList(int scan,Edge *active) {Edge *q=active, *p=active->next;while(p)if(scan>=p->yUpper){p=p->next;deleteAfter(q);}else{p->xIntersect=p->xIntersect+p->dxPerScan; q=p;p=p->next;}}void resortActiveList(Edge *active){Edge *q,*p=active->next;active->next=NULL;while(p){q=p->next;insertEdge(active,p);p=q;}}void scanFill(int cnt,dePt *pts){Edge *edges[1024],*active;int i,scan;for(i=0;i<1024;i++){edges[i]=(Edge *)malloc(sizeof(Edge)); edges[i]->next=NULL;}buildEdgeList(cnt,pts,edges);active=(Edge *)malloc(sizeof(Edge)); active->next=NULL;for(scan=0;scan<1024;scan++)buildActiveList(scan,active,edges);if(active->next){fillScan(scan,active);updateActiveList(scan,active);resortActiveList(active);}}}void ChangeSize(GLsizei w,GLsizei h){GLfloat nRange=400.0f;if(h==0) h=1;glViewport(0,0,w,h);glMatrixMode(GL_PROJECTION);glLoadIdentity();if(w<=h)glOrtho(-nRange,nRange,-nRange*h/w,nRange*h/w,-nRange,nRange);elseglOrtho(-nRange*h/w,nRange*h/w,-nRange,nRange,-nRange,nRange); glMatrixMode(GL_MODELVIEW);glLoadIdentity();}void Display(void){glClear(GL_COLOR_BUFFER_BIT);glLineWidth(5.0);int n,x,y,i;cout<<"请输入多边形顶点数:"<<endl;cin>>n;dePt *t=new dePt[n];for(i=0;i<n;i++){cout<<"请输入第"<<i+1<<"个顶点坐标"<<endl;cin>>x>>y;t[i].x=x;t[i].y=y;glVertex2i(t[i].x,t[i].y);} glEnd();glFlush();scanFill(n,t);glFlush();}void SetupRC()glClearColor(1.0f,1.0f,1.0f,1.0f); glColor3f(1.0f,0.0f,0.0f);}实验结果:。
c语言 aes256 ecb 算法
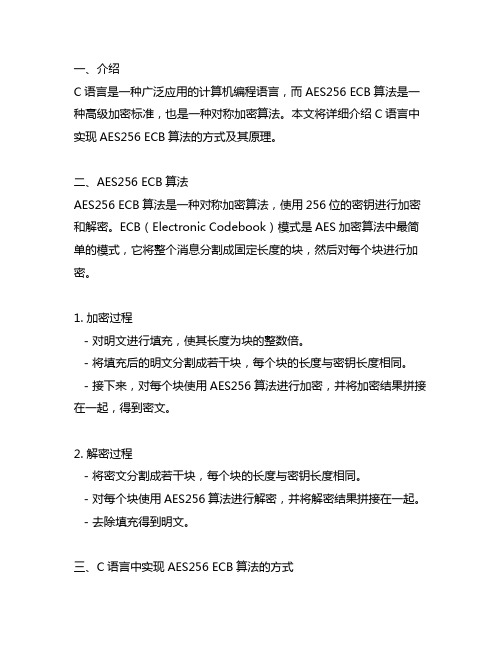
一、介绍C语言是一种广泛应用的计算机编程语言,而AES256 ECB算法是一种高级加密标准,也是一种对称加密算法。
本文将详细介绍C语言中实现AES256 ECB算法的方式及其原理。
二、AES256 ECB算法AES256 ECB算法是一种对称加密算法,使用256位的密钥进行加密和解密。
ECB(Electronic Codebook)模式是AES加密算法中最简单的模式,它将整个消息分割成固定长度的块,然后对每个块进行加密。
1. 加密过程- 对明文进行填充,使其长度为块的整数倍。
- 将填充后的明文分割成若干块,每个块的长度与密钥长度相同。
- 接下来,对每个块使用AES256算法进行加密,并将加密结果拼接在一起,得到密文。
2. 解密过程- 将密文分割成若干块,每个块的长度与密钥长度相同。
- 对每个块使用AES256算法进行解密,并将解密结果拼接在一起。
- 去除填充得到明文。
三、C语言中实现AES256 ECB算法的方式在C语言中实现AES256 ECB算法可以借助开源的加密库,例如openssl库。
以下是使用openssl库实现AES256 ECB算法的示例代码:```#include <openssl/aes.h>void aes_encrypt_ecb(const unsigned char *pl本人ntext, const unsigned char *key, unsigned char *ciphertext) {AES_KEY aes_key;AES_set_encrypt_key(key, 256, aes_key);AES_encrypt(pl本人ntext, ciphertext, aes_key);}void aes_decrypt_ecb(const unsigned char *ciphertext, const unsigned char *key, unsigned char *pl本人ntext) {AES_KEY aes_key;AES_set_decrypt_key(key, 256, aes_key);AES_decrypt(ciphertext, pl本人ntext, aes_key);}```以上代码中,aes_encrypt_ecb函数用于对明文进行加密,aes_decrypt_ecb函数用于对密文进行解密。
C++编程递归-[填充方阵-快速排序-僧侣搬盘]
![C++编程递归-[填充方阵-快速排序-僧侣搬盘]](https://img.taocdn.com/s3/m/e5524979a26925c52cc5bff7.png)
与结点要涂黑,相关 联的 B 与 C 之间要用 弧线连起来。
C
B
A 为与结点,A 的最终取值为 C 结点的值, 但为了求得 C 的值,得先求出 B 结点的值, C 是B 的函数。
7
与结点可能有多个相关联的点,这时可描述为下图
A
B
C
D
A 结点的值最终为 D 的值,但为了求 D 需先求 B 和 C。从图上看, 先求左边的点才能求最右边的点 的值,我们约定最右边 D 点的值就是 A 结点的值。
2
任务
有人可能会会觉得这个问题很简单,而实际上,如果
真的动手进行数学推算就会发现:即便一秒钟移动一只盘 子,按照上述规则,要将64只盘子从一个柱子移至另一个 柱子上,需要大约5800亿年! 这个僧侣移盘问题,通常被称为汉诺(Hanoi)塔问题。
解决这个问题最经典的算法就是递归。
3
9.1 递归及其实现
递归算法在可计算性理论中占有重要地位, 它是算法设计的有力工具,对于拓展编程思路非 常有用。 递归算法丌涉及高深数学知识,丌过初学者 要建立起递归概念并丌十分了帮助我们思考和表述递归算法的思路,使算法更 直观和清晰,我们定义两个结点:或结点和与结点。 1、或结点
A 条件 Z B 条件!Z C
1 2 3 4 5 6
27
从左至右,填B1 begin=0 ; number=7; size=6 h=5 ; v=begin; for( i=0 ; i<size-1 ; i++ ) { v++ ; p[h][v]=number; number++; h=5 7 8 9 10 11
}
v: 1 2
3 4 5
D2
填充三角形 c 算法 -回复
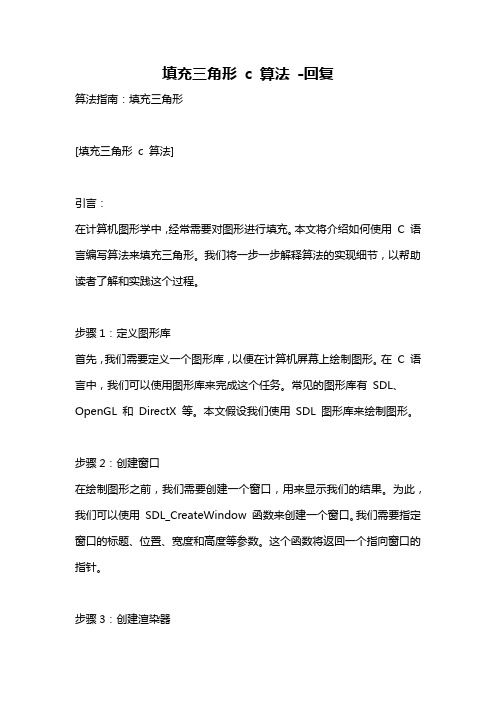
填充三角形c 算法-回复算法指南:填充三角形[填充三角形c 算法]引言:在计算机图形学中,经常需要对图形进行填充。
本文将介绍如何使用C 语言编写算法来填充三角形。
我们将一步一步解释算法的实现细节,以帮助读者了解和实践这个过程。
步骤1:定义图形库首先,我们需要定义一个图形库,以便在计算机屏幕上绘制图形。
在C 语言中,我们可以使用图形库来完成这个任务。
常见的图形库有SDL、OpenGL 和DirectX 等。
本文假设我们使用SDL 图形库来绘制图形。
步骤2:创建窗口在绘制图形之前,我们需要创建一个窗口,用来显示我们的结果。
为此,我们可以使用SDL_CreateWindow 函数来创建一个窗口。
我们需要指定窗口的标题、位置、宽度和高度等参数。
这个函数将返回一个指向窗口的指针。
步骤3:创建渲染器接下来,我们需要创建一个渲染器来处理绘制图形的任务。
我们可以使用SDL_CreateRenderer 函数来创建一个渲染器。
我们需要将窗口指针作为参数传递给这个函数。
这个函数将返回一个指向渲染器的指针。
步骤4:设置渲染器颜色在填充三角形之前,我们需要设置渲染器的颜色。
我们可以使用SDL_SetRenderDrawColor 函数来设置渲染器的颜色。
我们需要指定渲染器、颜色的红、绿、蓝和透明度等参数。
这个函数将以指定的颜色绘制图形。
步骤5:绘制三角形接下来,我们需要绘制一个三角形。
在填充三角形之前,我们需要知道三角形的顶点坐标。
我们可以使用SDL_RenderDrawLine 函数来绘制一个线段。
我们需要指定渲染器、线段的起点和终点坐标等参数。
我们可以重复调用这个函数来绘制三角形的三个边。
步骤6:填充三角形一旦我们绘制了三角形的边,接下来我们可以填充这个三角形。
为了实现这个目标,我们可以使用扫描线填充算法。
该算法通过计算三角形的每一行,并在每一行上绘制线段来填充三角形。
在此过程中,我们需要知道三角形的顶点坐标,请确保所有的顶点按顺时针或逆时针顺序排列。
VC++多边形的填充

if(ccd.DoModal()==IDOK)//调用调色板选取前景色
{
GetColor=ccd.GetColor();
}
RedrawWindow();//刷新屏幕
CreatBucket();//初始化桶
Et();//建立边表
PolygonFill();//多边形填充
{
T1=HeadE;
if(T1==NULL)//边表为空,将边表置为TempEdge
{
T1=NewEdge;
HeadE=T1;
}
else
{
while(T1->next!=NULL)//边表不为空,将TempEdge连在该边之后
{
T1=T1->next;
}
T1->next=NewEdge;
}
}
void CTestView::EdgeOrder()//对边表进行排序函数
ASSERT_VALID(pDoc);
pDC->Polygon(Point,7);//绘制多边形
//输出多边形的顶点编号
pDC->TextOut(550,410,"P0");
pDC->TextOut(350,600,"P1");
pDC->TextOut(230,340,"P2");
pDC->TextOut(350,30,"P3");
class CTestView : public CView
{
。。。。。。。。。
public:
void PolygonFill();//上闭下开填充多边形
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
int ymax;
float xБайду номын сангаасdeltax;
struct _edge *next;
}Edge;
/*---------------------------------------------------------*/
/*一个绘制多边形的类
*/
//drawpolygon(CDC *pDC,POINT *polyGP,int m_polygonNum,int m_pColor);为外部接口
20条边以下的多边形,算法为边填充算法。
边填充算法程序实现的基本思路如下:
①构造初始边表(EOT)——对各斜边按
ymax值从上到下排序,存放于一个高度表(y桶表)中,高
度表的内容为指向各斜边的指针。
②构造活性边表(
AET)——从上到下计算出每条扫描线与多边形各斜边的交点,这些交点用活性边
};
#endif
// !defined(AFX_POLYGON_H__EA547C5F_92DD_4847_A7BF_D702BFB41CDA__INCLUDED_)
#include "stdafx.h"
#include "MyPriciple.h"
#include "Polygon.h"
//参数分别为:
//POINT *polyGP,存放多边形顶点的
POINT型数组
//int m_polygonNum,多边形的顶点个数
//int m_pColor, 画笔颜色
/*---------------------------------------------------------*/
#ifdef _DEBUG
#undef THIS_FILE
static char THIS_FILE[]=__FILE__;
#define new DEBUG_NEW
#endif
//////////////////////////////////////////////////////////////////////
{
m_Triangle.drawtriangle(pDC,polyGP,m_pColor);
inLength=m_PolygonNum;
m_PolygonNum=0;
}
break;
case 5:
polyGP[m_PolygonNum++]=point;
pDC->SetPixel(point.x,point.y,RGB(255,0,0));
为空,则返回
true
不为空,则返回
false
CTriangle类:
该类与
CPolygon类类似,但期间调用了
CPolygon类的结构体,故需包含其头文件。
步骤三:
创建相应的菜单,填充三角形和填充多边形
IDM_MTRIANGLE
IDM_MSCAN
附加一选择填充颜色的菜单
{
}
/*---------------------------------------------------------*/
/*此函数完成多边形边的分类表
ET的建立
/*参数
POINT *polyGP为存放多边形顶点的数组
/*在此过程中得到多边形的最低点
pymin及最高点
//相应抬起鼠标消息
void CMyPricipleView::OnLButtonUp(UINT nFlags, CPoint point)
{
// TODO: Add your message handler code here and/or call default
CDC* pDC=GetDC();
int m_pColor,画笔颜色
该函数需要调用两个函数:建立边表和绘制填充多边形。
建立边表函数如下:
void CPolygon::CreateET(POINT *polyGP)
此函数完成多边形边的分类表
ET的建立
参数
POINT *polyGP为存放多边形顶点的数组
在此过程中得到多边形的最低点
#define AFX_POLYGON_H__EA547C5F_92DD_4847_A7BF_D702BFB41CDA__INCLUDED_
#if _MSC_VER > 1000
#pragma once
#endif // _MSC_VER > 1000
//定义边的结构体
typedef struct _edge
{
// TODO: Add your command handler code here
m_dSort=4;
}
void CMyPricipleView::OnMscan()
{
// TODO: Add your command handler code here
m_dSort=5;
class CPolygon
{
public:
void drawpolygon(CDC *pDC,POINT *polyGP,int polygonNum,int m_pColor);
CPolygon();
virtual ~CPolygon();
private:
bool isemptyET();
步骤二:
创建两个新类:CPolygon、CTriangle,分别用于绘制三角形和多边形
实现的基本功能分别如下:
CPolygon类:
①定义边的结构体
typedef struct _edge
{
int ymax;
float x,deltax;
struct _edge *next;
{
// TODO: Add your message handler code here and/or call default
CDC *pDC=GetDC();
if(m_dSort==5)
{
if(m_PolygonNum>=3)
m_polygon.drawpolygon(pDC,polyGP,m_PolygonNum,m_pColor);
pymax
/*---------------------------------------------------------*/
}Edge;
②实现绘图的外部接口:
函数为:
drawpolygon(CDC *pDC,POINT *polyGP,int m_polygonNum,int m_pColor);为外部接口
参数分别为:
POINT *polyGP,存放多边形顶点的
POINT型数组
int m_polygonNum,多边形的顶点个数
按照
p->x的增序插入
绘制填充多边形函数如下:
void CPolygon::dPolygon(CDC * pDC,int color)
在绘制多边形过程中用到判断边表是否为空,其相应的函数如下:
bool CPolygon::isemptyET()
此函数判断边的分类表是否为空
// Construction/Destruction
//////////////////////////////////////////////////////////////////////
CPolygon::CPolygon()
{
}
CPolygon::~CPolygon()
CDC *pDC=GetDC();
OnDraw(pDC);
pDC->TextOut(50,50,"单击左键确定多边形顶点,右击开始绘图");
}
其参数传递给相应的鼠标单击和右击事件,鼠标单击和右击事件通过相应参数实现相应操作。
步骤四:
添加鼠标左键、右键单击消息映射,左键为抬起,右键为按下,并完成相应代码如下:
IDM_MFILL,该菜单调用颜色对话框其程序代码如下:
//填充颜色的选择
void CMyPricipleView::OnMfill()
{
// TODO: Add your command handler code here
m_dSort=6;
m_oColor=m_pColor;
if(m_PolygonNum>20)
MessageBox("您的输入超过了本程序允许的最大顶点个数
20!\t\n绘图中
止!!",NULL,MB_OK);
break;
}
CView::OnLButtonUp(nFlags, point);
}
void CMyPricipleView::OnRButtonDown(UINT nFlags, CPoint point)
int pymin;
int pymax;
int Num;
Edge **ET;
Edge *AEL;
void dPolygon(CDC *pDC,int color);
void insertEdge(Edge* & head,Edge* &p);
void CreateET(POINT *polyGP);
表来保存,活性边表有与初始边表具有相同的节点结构。