二叉树可视化Java语言实现(完整版,打开即可运行)
用Java实现的二叉树算法

//用Java实现的二叉树算法2008年01月12日星期六23:08//************************************************************** ****************************************////*****本程序包括简单的二叉树类的实现和前序,中序,后序,层次遍历二叉树算法,*******////******以及确定二叉树的高度,制定对象在树中的所处层次以及将树中的左右***********////******孩子节点对换位置,返回叶子节点个数删除叶子节点,并输出所删除的叶子节点**// //*******************************CopyRight Byphoenix*******************************************////************************************Jan12,2008*************************************************////******************************************************************* *********************************//public class BinTree {public final static int MAX = 40;private Object data; // 数据元数private BinTree left, right; // 指向左,右孩子结点的链BinTree[] elements = new BinTree[MAX];// 层次遍历时保存各个节点int front;// 层次遍历时队首int rear;// 层次遍历时队尾public BinTree() {}public BinTree(Object data) { // 构造有值结点this.data = data;left = right = null;}public BinTree(Object data, BinTree left, BinTree right) { // 构造有值结点this.data = data;this.left = left;this.right = right;}public String toString() {return data.toString();}// 前序遍历二叉树public static void preOrder(BinTree parent) {if (parent == null)return;System.out.print(parent.data + " ");preOrder(parent.left);preOrder(parent.right);}// 中序遍历二叉树public void inOrder(BinTree parent) {if (parent == null)return;inOrder(parent.left);System.out.print(parent.data + " ");inOrder(parent.right);}// 后序遍历二叉树public void postOrder(BinTree parent) {if (parent == null)return;postOrder(parent.left);postOrder(parent.right);System.out.print(parent.data + " ");}// 层次遍历二叉树public void LayerOrder(BinTree parent) {elements[0] = parent;front = 0;rear = 1;while (front < rear) {try {if (elements[front].data != null) {System.out.print(elements[front].data + " ");if (elements[front].left != null)elements[rear++] = elements[front].left;if (elements[front].right != null)elements[rear++] = elements[front].right;front++;}} catch (Exception e) {break;}}}// 返回树的叶节点个数public int leaves() {if (this == null)return 0;if (left == null && right == null)return 1;return (left == null ? 0 : left.leaves())+ (right == null ? 0 : right.leaves());}// 结果返回树的高度public int height() {int heightOfTree;if (this == null)return -1;int leftHeight = (left == null ? 0 : left.height());int rightHeight = (right == null ? 0 : right.height());heightOfTree = leftHeight < rightHeight ? rightHeight : leftHeight;return 1 + heightOfTree;}// 如果对象不在树中,结果返回-1;否则结果返回该对象在树中所处的层次,规定根节点为第一层public int level(Object object) {int levelInTree;if (this == null)return -1;if (object == data)return 1;// 规定根节点为第一层int leftLevel = (left == null ? -1 : left.level(object));int rightLevel = (right == null ? -1 : right.level(object));if (leftLevel < 0 && rightLevel < 0)return -1;levelInTree = leftLevel < rightLevel ? rightLevel : leftLevel;return 1 + levelInTree;}// 将树中的每个节点的孩子对换位置public void reflect() {if (this == null)return;if (left != null)left.reflect();if (right != null)right.reflect();BinTree temp = left;left = right;right = temp;}// 将树中的所有节点移走,并输出移走的节点public void defoliate() {String innerNode = "";if (this == null)return;// 若本节点是叶节点,则将其移走if (left == null && right == null) { System.out.print(this + " ");data = null;return;}// 移走左子树若其存在if (left != null) {left.defoliate();left = null;}// 移走本节点,放在中间表示中跟移走...innerNode += this + " ";data = null;// 移走右子树若其存在if (right != null) {right.defoliate();right = null;}}/***@param args*/public static void main(String[] args) { // TODO Auto-generated method stubBinTree e = new BinTree("E");BinTree g = new BinTree("G");BinTree h = new BinTree("H");BinTree i = new BinTree("I");BinTree d = new BinTree("D", null, g);BinTree f = new BinTree("F", h, i);BinTree b = new BinTree("B", d, e);BinTree c = new BinTree("C", f, null);BinTree tree = new BinTree("A", b, c);System.out.println("前序遍历二叉树结果: ");tree.preOrder(tree);System.out.println();System.out.println("中序遍历二叉树结果: ");tree.inOrder(tree);System.out.println();System.out.println("后序遍历二叉树结果: ");tree.postOrder(tree);System.out.println();System.out.println("层次遍历二叉树结果: ");yerOrder(tree);System.out.println();System.out.println("F所在的层次: " + tree.level("F"));System.out.println("这棵二叉树的高度: " + tree.height());System.out.println("--------------------------------------");tree.reflect();System.out.println("交换每个节点的孩子节点后......");System.out.println("前序遍历二叉树结果: ");tree.preOrder(tree);System.out.println();System.out.println("中序遍历二叉树结果: ");tree.inOrder(tree);System.out.println();System.out.println("后序遍历二叉树结果: ");tree.postOrder(tree);System.out.println();System.out.println("层次遍历二叉树结果: ");yerOrder(tree);System.out.println();System.out.println("F所在的层次: " + tree.level("F"));System.out.println("这棵二叉树的高度: " + tree.height());}}。
java代码实现二叉树

import java.util.ArrayList;// 树的一个节点class TreeNode {Object _value = null; // 他的值TreeNode _parent = null; // 他的父节点,根节点没有PARENTArrayList _childList = new ArrayList(); // 他的孩子节点public TreeNode( Object value, TreeNode parent ){this._parent = parent;this._value = value;}public TreeNode getParent(){return _parent;}public String toString() {return _value.toString();}}public class Tree {// 给出宽度优先遍历的值数组,构建出一棵多叉树// null 值表示一个层次的结束// "|" 表示一个层次中一个父亲节点的孩子输入结束// 如:给定下面的值数组:// { "root", null, "left", "right", null }// 则构建出一个根节点,带有两个孩子("left","right")的树public Tree( Object[] values ){// 创建根_root = new TreeNode( values[0], null );// 创建下面的子节点TreeNode currentParent = _root; // 用于待创建节点的父亲//TreeNode nextParent = null;int currentChildIndex = 0; // 表示currentParent 是他的父亲的第几个儿子//TreeNode lastNode = null; // 最后一个创建出来的TreeNode,用于找到他的父亲for ( int i = 2; i < values.length; i++ ){// 如果null ,表示下一个节点的父亲是当前节点的父亲的第一个孩子节点if ( values[i] == null ){currentParent = (TreeNode)currentParent._childList.get(0);currentChildIndex = 0;continue;}// 表示一个父节点的所有孩子输入完毕if ( values[i].equals("|") ){if ( currentChildIndex+1 < currentParent._childList.size() ){currentChildIndex++;currentParent =(TreeNode)currentParent._parent._childList.get(currentChildIndex);}continue;}TreeNode child = createChildNode( currentParent, values[i] );}}TreeNode _root = null;public TreeNode getRoot(){return _root;}/**// 按宽度优先遍历,打印出parent子树所有的节点private void printSteps( TreeNode parent, int currentDepth ){for ( int i = 0; i < parent._childList.size(); i++ ){TreeNode child = (TreeNode)parent._childList.get(i);System.out.println(currentDepth+":"+child);}if ( parent._childList.size() != 0 ) System.out.println(""+null);// 为了避免叶子节点也会打印null//打印parent 同层的节点的孩子if ( parent._parent != null ){ // 不是rootint i = 1;while ( i < parent._parent._childList.size() ){// parent 的父亲还有孩子TreeNode current =(TreeNode)parent._parent._childList.get(i);printSteps( current, currentDepth );i++;}}// 递归调用,打印所有节点for ( int i = 0; i < parent._childList.size(); i++ ){TreeNode child = (TreeNode)parent._childList.get(i);printSteps( child, currentDepth+1 );}}// 按宽度优先遍历,打印出parent子树所有的节点public void printSteps(){System.out.println(""+_root);System.out.println(""+null);printSteps(_root, 1 );}**/// 将给定的值做为parent 的孩子,构建节点private TreeNode createChildNode( TreeNode parent, Object value ){ TreeNode child = new TreeNode( value , parent );parent._childList.add( child );return child;}public static void main(String[] args) {Tree tree = new Tree( new Object[]{ "root", null,"left", "right", null,"l1","l2","l3", "|", "r1","r2",null } );//tree.printSteps();System.out.println(""+( (TreeNode)tree.getRoot()._childList.get(0) )._childList.get(0) );System.out.println(""+( (TreeNode)tree.getRoot()._childList.get(0) )._childList.get(1) );System.out.println(""+( (TreeNode)tree.getRoot()._childList.get(0) )._childList.get(2) );System.out.println(""+( (TreeNode)tree.getRoot()._childList.get(1) )._childList.get(0) );System.out.println(""+( (TreeNode)tree.getRoot()._childList.get(1) )._childList.get(1) );}}java:二叉树添加和查询方法package arrays.myArray;public class BinaryTree {private Node root;// 添加数据public void add(int data) {// 递归调用if (null == root)root = new Node(data, null, null);elseaddTree(root, data);}private void addTree(Node rootNode, int data) {// 添加到左边if (rootNode.data > data) {if (rootNode.left == null)rootNode.left = new Node(data, null, null);elseaddTree(rootNode.left, data);} else {// 添加到右边if (rootNode.right == null)rootNode.right = new Node(data, null, null);elseaddTree(rootNode.right, data);}}// 查询数据public void show() {showTree(root);}private void showTree(Node node) {if (node.left != null) {showTree(node.left);}System.out.println(node.data);if (node.right != null) {showTree(node.right);}}}class Node {int data;Node left;Node right;public Node(int data, Node left, Node right) { this.data = data;this.left = left;this.right = right;}}。
java实现数据结构二叉树

import java.util.*;public class main {public static void main(String[] args){//确保输入的是整数Scanner read=new Scanner(System.in);int depth=0;boolean b=false;do{b=false;try{System.out.print("请输入该二叉树的深度(大于等于0,小于等于20):");depth=Integer.parseInt(read.next());}catch(Exception e){b=true;System.out.println("请输入数值!");}}while(b||depth<0||depth>20);//前序建立int i=0;int Sn=0;if(depth==0)Sn=1;else Sn=(int)((1-Math.pow(2, depth+1))/(1-2));String[] data=new String[Sn];System.out.println("请按前序的顺序输入该满二叉树的数据:");int count=Sn;while (i<Sn){System.out.print("尚余"+count+"个:");data[i]=read.next();i++;count--;}tree newtree=new tree(data,depth,Sn);search(newtree,depth);}public static void search(tree tr,int depth){System.out.println("层序遍历的数据顺序是:");LinkedList stack=new LinkedList();stack.add(tr);while(!stack.isEmpty()){tree temp=(tree)stack.getFirst();if(temp.getLeft()!=null){stack.add(temp.getLeft());stack.add(temp.getRight());}System.out.print(temp.getData()+" ");try{stack.removeFirst();}catch(Exception e){}}}}public class tree {private tree root;private tree left;private tree right;private tree head;private String data;private int depth=0,count=1;public tree(String a){data=a;this.left=null;this.right=null;}public tree (String a[],int dep,int sn){this.data=a[0];root=this;if(dep==0)return;do{if(root.left!=null&&root.right!=null){root=root.head;depth--;}else{//未到倒数第二的深度时左右子树的建立if(depth!=dep-1){tree temp=new tree(a[count]);//建立左子树if(root.left==null){root.left=temp;temp.head=root;root=root.left;}//建立右子树else {root.right=temp;temp.head=root;root=root.right;}depth++;count++;}//到达倒数第二的深度时左右子树的建立else {root.left=new tree(a[count]);count++;root.right=new tree(a[count]);count++;}}}while(count<sn);}public String getData() {return data;}public int getDepth() {return depth;}public tree getHead() {return head;}public tree getLeft() {return left;}public tree getRight() {return right;}}。
用Java实现一个二叉树

⽤Java实现⼀个⼆叉树介绍使⽤Java实现⼀个int值类型的排序⼆叉树⼆叉树⼆叉树是⼀个递归的数据结构,每个节点最多有两个⼦节点。
通常⼆叉树是⼆分查找树,每个节点它的值⼤于或者等于在它左⼦树节点上的值,⼩于或者等于在它右⼦树节点上的值,如下图为了实现⼆叉树,我们使⽤⼀个Node类来表⽰节点,节点存储int类型的值,还有对⼦节点的引⽤。
package com.java.node.BinaryTree;public class Node {int data;Node left;Node right;public Node(int data) {this.data = data;this.left = null;this.right = null;}}然后添加树的root节点package com.java.node.BinaryTree;public class BinaryTree {Node root;}通常的操作添加节点⾸先我们必须找到新节点的位置,是为了保持树排序。
我们必须从root节点开始,必须遵循下⾯的规则:如果新节点⼩于当前的值,我们将会进⼊左⼦树如果新节点⼤于当前的节点。
我们将会进⼊右⼦树当当前的节点是null时,我们已经到达叶⼦节点,我们可以添加新节点到这个位置。
我们将会创建递归⽅法做添加节点操作private Node addNode(Node current, int value) {if (current == null) {return new Node(value);}if (value < current.data) {current.left = addNode(current.left, value);} else if (value > current.data) {current.right = addNode(current.right, value);} else {return current;}return current;}public void addNode(int value) {root = addNode(root, value);}可以使⽤⽅法创建⼀个⼆叉树了public BinaryTree createBinaryTree() {BinaryTree bt = new BinaryTree();bt.addNode(6);bt.addNode(4);bt.addNode(8);bt.addNode(10);return bt;}查找⼀个节点private boolean containNode(Node current, int value) {if (current == null) {return false;}if (value == current.data) {return true;}return value < current.data ? containNode(current.left, value) : containNode(current.right, value); }public boolean containNode(int value) {return containNode(root, value);}删除⼀个节点private Node deleteNode(Node current, int value) {if (current == null) {return null;}if (value == current.data) {if (current.left == null && current.right == null) {return null;}if (current.left == null) {return current.right;}if (current.right == null) {return current.left;}int smallestValue = findSmallestValue(current.right);current.data = smallestValue;current.right = deleteNode(current.right, smallestValue);return current;}if (value < current.data) {current.left = deleteNode(current.left, value);return current;}current.right = deleteNode(current.right, value);return current;}private int findSmallestValue(Node root) {return root.left == null ? root.data : findSmallestValue(root.right);}遍历树我们将以不同的⽅式遍历树,以depth-first,breadth-first⽅式遍历树。
用java实现二叉树的创建和遍历

用java实现二叉树的创建和遍历输入方式:用先序方式输入创建二叉树(代码:FOBiTree.java)输入:输入一个字符串(例ABC##DE#G##F###,#表示空格),这个字符串是二叉树的先序遍历方式的。
public char data; //存储数据部分public FOBiT ree lNode; //定义左子树public FOBiT ree rNode; //定义右子树创建二叉树public FOBiT ree CreateBiT ree(FOBiT ree T) {char ch = str.charAt(j);j++;if (ch == ' ') {T = null;//如果ch等于空字符,则表示该枝上为空} else {N++;T.lNode = new FOBiT ree();//初始化左子树T.rNode = new FOBiT ree();//初始化右子树T.data = ch; //输入数据T.lNode = CreateBiT ree(T.lNode);//创建左子树T.rNode = CreateBiT ree(T.rNode);//创建右子树}return T;}//二叉树的创建先序遍历函数public void fsOrder(FOBiT ree T) {if (T != null) {System.out.print(T.data + " ");//输出数据fsOrder(T.lNode);//先序遍历左子树fsOrder(T.rNode);//先序遍历右子树}}//先序遍历中序遍历函数public void InOrder(FOBiT ree T) {if (T != null) {InOrder(T.lNode);//中序遍历左子树System.out.print(T.data + " ");//输出数据InOrder(T.rNode);//中序遍历右子树}}//中序遍历后序遍历函数public void edOrder(FOBiT ree T) {if (T != null) {edOrder(T.lNode);//后序遍历左子树edOrder(T.rNode);//后序遍历右子树System.out.print(T.data + " ");//输出数据}}//后序遍历层次遍历函数public void gradation(FOBiT ree T) {FOBiT ree[] st = new FOBiT ree[100];st[0]=new FOBiT ree();st[0] = T;k = 1;m = 0;while (k < N) {if (st[m].lNode != null) {st[k]=new FOBiT ree();//初始化st[k++] = st[m].lNode;//存储st[m]的左子树(非空的)}if (st[m].rNode != null) {st[k]=new FOBiT ree();//初始化st[k++] = st[m].rNode;//存储st[m]的右子树(非空的)}m++;}for (int i = 0; i < N; i++) {System.out.print(st[i].data + " ");}System.out.println("");}//层次遍历输入:ABC##DE#G##F### (#表示空格)输出:先序遍历A B C D E G F中序遍历C B E GD F A后序遍历C G E FD B A层次遍历A B C D E F G输入方式:广义表表示法表示的二叉树(代码:WideBiTree.java)输入:输入一个字符串(例A(B,C(,E))#,#表示结束)定义二叉树:public char data; //定义数据public WideBiT ree lchild, rchild; //定义左子树和右子树public WideBiT ree T; //根结点创建二叉树:public void CreateBiT ree(String str) {WideBiT ree[] S t = new WideBiT ree[100];WideBiT ree p = new WideBiT ree();p = null;int top = -1, k = 0, j = 0, t = 0;char ch;ch = str.charAt(j);j++;while (ch != '#') {switch (ch) {case '(':S t[++top] = p;//跳过'(',存储下一个字符k = 1;//这是左子树break;//如果字符是'('的情况case ')':top--;//减去这个树break;//如果字符是')'的情况case ',':k = 2;//后面的是右子树break;//如果字符是','的情况default: //其它情况p = new WideBiT ree();//创建结点N++;p.data = ch;//将字符存入结点p.lchild = p.rchild = null;//将子树赋为空if (t == 0) {T = p;t = 1;} else {switch (k) {case 1:St[top].lchild = p;//k等于1表示左子树break;case 2:St[top].rchild = p;break;//k等于2表示右子树}}}ch = str.charAt(j);//获取字符j++;}}前序、中序、后序和前面的程序一样输入:输入广义表A(B,C(,E))#输出:广义表表示法表示的二叉树——先序遍历ABCE广义表表示法表示的二叉树——中序遍历BACE广义表表示法表示的二叉树——后序遍历BECA。
JAVA实现二叉树(简易版--实现了二叉树的各种遍历)

JAVA实现⼆叉树(简易版--实现了⼆叉树的各种遍历)1,个⼈感觉⼆叉树的实现主要还是如何构造⼀颗⼆叉树。
构造⼆叉树函数的设计⽅法多种多样,本例采⽤ addNode ⽅法实现。
以下程序通过定义内部类来表⽰⼆叉树的结点,然后再实现了⼆叉树这种数据结构的⼀些基本操作。
2,说说以下程序的⼀些不⾜:a,56⾏中的判断树是否为空时,依据根结点的数据域是否为空来判断。
⽽使⽤不带参数的构造函数构造⼆叉树时,根结点的不空的,此时说明树已经有了根结点,但是根结点的数据却是空的,此时的树⾼度为1,但是不能访问树根结点,因为树根结点的数据域没有值。
3,重点讲解下⼆叉树遍历的⼏个⽅法:先序遍历:将先序遍历过程中遇到的结点添加到ArrayList<TreeNode>中。
根据先序遍历的递归的性质,调⽤addAll(Container c)⽅法完成遍历主要过程。
层序遍历:层序遍历需要使⽤队列,⽅法level_Traverse 中定义了ArrayDeque<TreeNode> 类型的队列。
1package tree;23import java.util.ArrayDeque;4import java.util.ArrayList;5import java.util.List;6import java.util.Queue;78public class BinaryTree<E> {9//为什么要⽤静态内部类?静态内部类中不能访问外部类的⾮静态成员10public static class TreeNode{11// E data;12 Object data;13 TreeNode left;14 TreeNode right;15public TreeNode(){1617 }18public TreeNode(Object data){19this.data = data;20 }21//构造⼀个新节点,该节点以left节点为其左孩⼦,right节点为其右孩⼦22public TreeNode(Object data, TreeNode left, TreeNode right){23this.data = data;24this.left = left;25this.right = right;26 }27 }2829private TreeNode root;//实现⼆叉树的类的数据域,即根结点来表⽰⼆叉树3031public BinaryTree(){32this.root = new TreeNode();33 }34//以指定的根元素创建⼀颗⼆叉树35public BinaryTree(E data){36this.root = new TreeNode(data);37 }3839//为指定的结点添加⼦结点,为什么要有addNode⽅法?因为给定⼀系列的结点,通过调⽤该⽅法来构造成⼀颗树40public TreeNode addNode(TreeNode parent, E data, boolean isLeft){41if(parent == null)42throw new RuntimeException("⽗节点为空,⽆法添加⼦结点");43if(isLeft && parent.left != null)44throw new RuntimeException("节点已经左⼦节点,添加失败");45if(!isLeft && parent.right != null)46throw new RuntimeException("节点已经有右⼦节点,添加失败");47 TreeNode newNode = new TreeNode(data);48if(isLeft)49 parent.left = newNode;50else51 parent.right = newNode;52return newNode;53 }5455public boolean empty(){56return root.data == null;//根据根元素判断⼆叉树是否为空57 }5859public TreeNode root(){60if(empty())61throw new RuntimeException("树空,⽆法访问根结点");62return root;63 }6465public E parent(TreeNode node){66return null;//采⽤⼆叉树链表存储时,访问⽗结点需要遍历整棵⼆叉树,因为这⾥不实现67 }6869//访问指定节点的左结点,返回的是其左孩⼦的数据域70public E leftChild(TreeNode parent){71if(parent == null)72throw new RuntimeException("空结点不能访问其左孩⼦");73return parent.left == null ? null : (E)parent.left.data;74 }75public E rightChild(TreeNode parent){76if(parent == null)77throw new RuntimeException("空结点不能访问其右孩⼦");78return parent.right == null ? null : (E)parent.right.data;79 }8081public int deep(){82return deep(root);83 }84private int deep(TreeNode node){85if(node == null)86return 0;87else if(node.left == null && node.right == null)88return 1;89else{90int leftDeep = deep(node.left);91int rightDeep = deep(node.right);92int max = leftDeep > rightDeep ? leftDeep : rightDeep;93return max + 1;94 }95 }9697/*⼆叉树的先序遍历,实现思想如下:树是⼀种⾮线性结构,树中各个结点的组织⽅式有多种⽅式 98 * 先序,即是⼀种组织⽅式。
二叉树的简单实现(Java)

⼆叉树的简单实现(Java)实际开发中,Java已经为开发者提供了TreeMap、TreeSet这种红⿊树集合。
本⽂的⽬的主要是通过实现简单的⼆叉树,熟练掌握泛型、⽐较器、递归、⼆叉树等知识。
以下是代码实现:(1)⼆叉树 BinaryTree 类import parable;import java.util.ArrayList;/*** 类名:BinaryTree* 功能:实现⼆叉树,具有排序功能*/public class BinaryTree<T extends Comparable<T>> {/* 对象属性 */private Node<T> rootNode; // 根节点private int size; // 节点个数/* 对象⽅法 */// 添加数据 value 对象,该对象必须实现 Comparable 接⼝public boolean add(T value) {// 1.定义⼀个节点Node<T> node = new Node<>();// 2.将数据 value 封装到节点 node 中node.setValue(value);// 3.判断根节点是否为空if(this.rootNode == null) {this.rootNode = node; // 如果根节点为空,直接赋值this.size++;return true;}// 4.将节点 node 与⼆叉数上的节点⽐较Node<T> nodeOnTree = rootNode; // 定义⼀个临时节点,先指向根节点while(true) {// ⽐较节点if(pareTo(node) > 0) {// 如果⼤于 0,则看左节点if(nodeOnTree.getLeftNode() == null) {nodeOnTree.setLeftNode(node);this.size++;return true;} else {nodeOnTree = nodeOnTree.getLeftNode();}} else if(pareTo(node) == 0) {// 如果等于 0return false; // 两个节点相同,添加失败} else {// 如果⼩于 0,则看右节点if(nodeOnTree.getRightNode() == null) {nodeOnTree.setRightNode(node);this.size++;return true;} else {nodeOnTree = nodeOnTree.getRightNode();}}}}// 左序遍历所有节点,并转化为 ArrayListpublic ArrayList<T> getLeftArrayList() {// 1.定义⼀个有节点个数 size ⼤⼩的ArrayListArrayList<T> list = new ArrayList<>(this.size);// 2.调⽤根节点的 traverseLeft ⽅法rootNode.traverseLeft(list);// 3.返回该 ArrayListreturn list;}// getter 和 setterpublic int getSize() {return this.size;}/* 内部类 *//*** 类名:Node* 功能:⼆叉树的节点*/private class Node<T extends Comparable<T>> {/* 对象属性 */private Node<T> leftNode; // 左⼦节点private Node<T> rightNode; // 右⼦节点private T value;/* 对象⽅法 */// 节点⽐较,value 对象必须实现 Comparable 接⼝public int compareTo(Node<T> anotherNode) {return this.getValue().compareTo(anotherNode.getValue()); // 调⽤ value 的 compareTo ⽅法 }// 左序遍历,存⼊节点数组public void traverseLeft(ArrayList<T> list) {// 查看左节点if(this.leftNode != null) {this.leftNode.traverseLeft(list);}// 将 value 添加到 list 中list.add(this.getValue());// 查看右节点if(this.rightNode != null) {this.rightNode.traverseLeft(list);}}// getter和setter⽅法public Node<T> getLeftNode() {return this.leftNode;}public void setLeftNode(Node<T> leftNode) {this.leftNode = leftNode;}public Node<T> getRightNode() {return this.rightNode;}public void setRightNode(Node<T> rightNode) {this.rightNode = rightNode;}public T getValue() {return this.value;}public void setValue(T value) {this.value = value;}}}(2)数据对象 Value 类import parable;/*** 类名:Value* 功能:数据对象,实现了 Comparable<T> 接⼝,可添加到 BinaryTree 中*/public class Value implements Comparable<Value> {/* 对象属性 */private String name;private int num;/* 构造⽅法 */public Value(String name, int num) { = name;this.num = num;}/* 对象⽅法 */// 重写了 Comparable<T> 接⼝的 compareTo(T anotherObject) ⽅法,设置⽐较规则@Overridepublic int compareTo(Value anotherValue) {return (this.getNum() - anotherValue.getNum());}// getter 和 setter ⽅法public String getName() {return ;}public void setName(String name) { = name;}public int getNum() {return this.num;}public void setName(int num) {this.num = num;}}(3)测试import java.util.List;import java.util.ArrayList;public class Test {/* 程序⼊⼝ */public static void main(String[] args) {// 定义数据对象 Value 数组Value[] values = new Value[5];values[0] = new Value("value0", 2);values[1] = new Value("value1", 4);values[2] = new Value("value2", 1);values[3] = new Value("value3", 0);values[4] = new Value("value4", 5);// 添加到⼆叉树中BinaryTree<Value> bt = new BinaryTree<>();for(int n = 0; n < values.length; n++) {bt.add(values[n]);}// 得到左序遍历的 ArrayListArrayList<Value> valueList = bt.getLeftArrayList();// 输出遍历结果for(Value v : valueList) {System.out.println("[" + v.getName() + ":" + v.getNum() + "]"); }System.out.println(bt.getSize());}}。
java实现二叉树的创建及5种遍历方法(总结)

java实现⼆叉树的创建及5种遍历⽅法(总结)⽤java实现的数组创建⼆叉树以及递归先序遍历,递归中序遍历,递归后序遍历,⾮递归前序遍历,⾮递归中序遍历,⾮递归后序遍历,深度优先遍历,⼴度优先遍历8种遍历⽅式:package myTest;import java.util.ArrayList;import java.util.LinkedList;import java.util.List;import java.util.Stack;public class myClass {public static void main(String[] args) {// TODO Auto-generated method stubmyClass tree = new myClass();int[] datas = new int[]{1,2,3,4,5,6,7,8,9};List<Node> nodelist = new LinkedList<>();tree.creatBinaryTree(datas, nodelist);Node root = nodelist.get(0);System.out.println("递归先序遍历:");tree.preOrderTraversal(root);System.out.println();System.out.println("⾮递归先序遍历:");tree.preOrderTraversalbyLoop(root);System.out.println();System.out.println("递归中序遍历:");tree.inOrderTraversal(root);System.out.println();System.out.println("⾮递归中序遍历:");tree.inOrderTraversalbyLoop(root);System.out.println();System.out.println("递归后序遍历:");tree.postOrderTraversal(root);System.out.println();System.out.println("⾮递归后序遍历:");tree.postOrderTraversalbyLoop(root);System.out.println();System.out.println("⼴度优先遍历:");tree.bfs(root);System.out.println();System.out.println("深度优先遍历:");List<List<Integer>> rst = new ArrayList<>();List<Integer> list = new ArrayList<>();tree.dfs(root,rst,list);System.out.println(rst);}/**** @param datas 实现⼆叉树各节点值的数组* @param nodelist ⼆叉树list*/private void creatBinaryTree(int[] datas,List<Node> nodelist){//将数组变成node节点for(int nodeindex=0;nodeindex<datas.length;nodeindex++){Node node = new Node(datas[nodeindex]);nodelist.add(node);}//给所有⽗节点设定⼦节点for(int index=0;index<nodelist.size()/2-1;index++){//编号为n的节点他的左⼦节点编号为2*n 右⼦节点编号为2*n+1 但是因为list从0开始编号,所以还要+1//这⾥⽗节点有1(2,3),2(4,5),3(6,7),4(8,9)但是最后⼀个⽗节点有可能没有右⼦节点需要单独处理nodelist.get(index).setLeft(nodelist.get(index*2+1));nodelist.get(index).setRight(nodelist.get(index*2+2));}//单独处理最后⼀个⽗节点因为它有可能没有右⼦节点int index = nodelist.size()/2-1;nodelist.get(index).setLeft(nodelist.get(index*2+1)); //先设置左⼦节点if(nodelist.size() % 2 == 1){ //如果有奇数个节点,最后⼀个⽗节点才有右⼦节点nodelist.get(index).setRight(nodelist.get(index*2+2));}}/*** 遍历当前节点的值* @param nodelist* @param node*/public void checkCurrentNode(Node node){System.out.print(node.getVar()+" ");}/*** 先序遍历⼆叉树* @param root ⼆叉树根节点*/public void preOrderTraversal(Node node){if (node == null) //很重要,必须加上当遇到叶⼦节点⽤来停⽌向下遍历return;checkCurrentNode(node);preOrderTraversal(node.getLeft());preOrderTraversal(node.getRight());}/*** 中序遍历⼆叉树* @param root 根节点*/public void inOrderTraversal(Node node){if (node == null) //很重要,必须加上return;inOrderTraversal(node.getLeft());checkCurrentNode(node);inOrderTraversal(node.getRight());}/*** 后序遍历⼆叉树* @param root 根节点*/public void postOrderTraversal(Node node){if (node == null) //很重要,必须加上return;postOrderTraversal(node.getLeft());postOrderTraversal(node.getRight());checkCurrentNode(node);}/*** ⾮递归前序遍历* @param node*/public void preOrderTraversalbyLoop(Node node){Stack<Node> stack = new Stack();Node p = node;while(p!=null || !stack.isEmpty()){while(p!=null){ //当p不为空时,就读取p的值,并不断更新p为其左⼦节点,即不断读取左⼦节点 checkCurrentNode(p);stack.push(p); //将p⼊栈p = p.getLeft();}if(!stack.isEmpty()){p = stack.pop();p = p.getRight();}}}/*** ⾮递归中序遍历* @param node*/public void inOrderTraversalbyLoop(Node node){Stack<Node> stack = new Stack();Node p = node;while(p!=null || !stack.isEmpty()){while(p!=null){stack.push(p);p = p.getLeft();}if(!stack.isEmpty()){p = stack.pop();checkCurrentNode(p);p = p.getRight();}}}/*** ⾮递归后序遍历* @param node*/public void postOrderTraversalbyLoop(Node node){Stack<Node> stack = new Stack<>();Node p = node,prev = node;while(p!=null || !stack.isEmpty()){while(p!=null){stack.push(p);p = p.getLeft();}if(!stack.isEmpty()){Node temp = stack.peek().getRight();if(temp == null||temp == prev){p = stack.pop();checkCurrentNode(p);prev = p;p = null;}else{p = temp;}}}}/*** ⼴度优先遍历(从上到下遍历⼆叉树)* @param root*/public void bfs(Node root){if(root == null) return;LinkedList<Node> queue = new LinkedList<Node>();queue.offer(root); //⾸先将根节点存⼊队列//当队列⾥有值时,每次取出队⾸的node打印,打印之后判断node是否有⼦节点,若有,则将⼦节点加⼊队列 while(queue.size() > 0){Node node = queue.peek();queue.poll(); //取出队⾸元素并打印System.out.print(node.var+" ");if(node.left != null){ //如果有左⼦节点,则将其存⼊队列queue.offer(node.left);}if(node.right != null){ //如果有右⼦节点,则将其存⼊队列queue.offer(node.right);}}}/*** 深度优先遍历* @param node* @param rst* @param list*/public void dfs(Node node,List<List<Integer>> rst,List<Integer> list){if(node == null) return;if(node.left == null && node.right == null){list.add(node.var);/* 这⾥将list存⼊rst中时,不能直接将list存⼊,⽽是通过新建⼀个list来实现,* 因为如果直接⽤list的话,后⾯remove的时候也会将其最后⼀个存的节点删掉*/rst.add(new ArrayList<>(list));list.remove(list.size()-1);}list.add(node.var);dfs(node.left,rst,list);dfs(node.right,rst,list);list.remove(list.size()-1);}/*** 节点类* var 节点值* left 节点左⼦节点* right 右⼦节点*/class Node{int var;Node left;Node right;public Node(int var){this.var = var;this.left = null;this.right = null;}public void setLeft(Node left) {this.left = left;}public void setRight(Node right) {this.right = right;}public int getVar() {return var;}public void setVar(int var) {this.var = var;}public Node getLeft() {return left;}public Node getRight() {return right;}}}运⾏结果:递归先序遍历:1 2 4 8 9 5 3 6 7⾮递归先序遍历:1 2 4 8 9 5 3 6 7递归中序遍历:8 4 9 2 5 1 6 3 7⾮递归中序遍历:8 4 9 2 5 1 6 3 7递归后序遍历:8 9 4 5 2 6 7 3 1⾮递归后序遍历:8 9 4 5 2 6 7 3 1⼴度优先遍历:1 2 3 4 5 6 7 8 9深度优先遍历:[[1, 2, 4, 8], [1, 2, 4, 9], [1, 2, 5], [1, 3, 6], [1, 3, 7]]以上这篇java实现⼆叉树的创建及5种遍历⽅法(总结)就是⼩编分享给⼤家的全部内容了,希望能给⼤家⼀个参考,也希望⼤家多多⽀持。
使用Java实现二叉树的添加,删除,获取以及遍历

使⽤Java实现⼆叉树的添加,删除,获取以及遍历 ⼀段来⾃百度百科的对⼆叉树的解释: 在计算机科学中,⼆叉树是每个结点最多有两个⼦树的树结构。
通常⼦树被称作“左⼦树”(left subtree)和“右⼦树”(right subtree)。
⼆叉树常被⽤于实现⼆叉查找树和⼆叉堆。
⼀棵深度为k,且有2^k-1个节点的⼆叉树,称为满⼆叉树。
这种树的特点是每⼀层上的节点数都是最⼤节点数。
⽽在⼀棵⼆叉树中,除最后⼀层外,若其余层都是满的,并且最后⼀层或者是满的,或者是在右边缺少连续若⼲节点,则此⼆叉树为完全⼆叉树。
具有n个节点的完全⼆叉树的深度为floor(log2n)+1。
深度为k的完全⼆叉树,⾄少有2k-1个叶⼦节点,⾄多有2k-1个节点。
⼆叉树的结构: ⼆叉树节点的声明:static final class Entry<T extends Comparable<T>>{//保存的数据private T item;//左⼦树private Entry<T> left;//右⼦树private Entry<T> right;//⽗节点private Entry<T> parent;Entry(T item,Entry<T> parent){this.item = item;this.parent = parent;}} 类属性://根节点private Entry<T> root;//数据量private int size = 0; ⼆叉树添加数据:/*** 添加元素* @param item 待添加元素* @return 已添加元素*/public T put(T item){//每次添加数据的时候都是从根节点向下遍历Entry<T> t = root;if (t == null){//当前的叉树树的为空,将新节点设置为根节点,⽗节点为nullroot = new Entry<>(item,null); size++; return root.item;}//⾃然排序结果,如果传⼊的数据⼩于当前节点返回-1,⼤于当前节点返回1,否则返回0int ret = 0;//记录⽗节点Entry<T> p = t;while (t != null){//与当前节点⽐较ret = pareTo(t.item);p = t;//插⼊节点⽐当前节点⼩,把当前节点设置为左⼦节点,然后与左⼦⽐较,以此类推找到合适的位置if (ret < 0)t = t.left;//⼤于当前节点else if (ret > 0)t = t.right;else {//相等就把旧值覆盖掉t.item = item;return t.item;}}//创建新节点Entry<T> e = new Entry<>(item,p);//根据⽐较结果将新节点放⼊合适的位置if (ret < 0)p.left = e;elsep.right = e; size++;return e.item;} 在put的过程中,使⽤Comparable<T>中的compareTo来⽐较两个元素的⼤⼩的,所以在⼆叉树中存储的元素必须要继承Comparable<T> 类,覆写compareTo⽅法。
二叉树的JAVA实现-二叉树的增删改查CRUD

⼆叉树的JAVA实现-⼆叉树的增删改查CRUD package org.lyk.interfaces;import java.util.List;public interface IBiTree<T extends Comparable<T>>{public void addWithOrder(T data);public void addFirst(T[] data,T endFlag);public Object[] toArrayFirst();public Object[] toArrayMiddle();public Object[] toArrayLast();public int size();public boolean isEmpty();public boolean contains(T data);public void delete(T data);}package org.lyk.entities;import java.util.HashMap;import java.util.Map;import org.lyk.interfaces.IBiTree;public class BiTree<T extends Comparable<T>> implements IBiTree<T>{private class Node<N extends Comparable<N>>{private N data;private Node<N> left;private Node<N> right;private Node(N data){if (null == data)return;this.data = data;}public void addWithOrder(N data){// if(pareTo(data) < 0)if (pareTo(this.data) < 0){if (null == this.left){this.left = new Node<N>(data);BiTree.this.count++;} elsethis.left.addWithOrder(data);} else{if (null == this.right){this.right = new Node<N>(data);BiTree.this.count++;} elsethis.right.addWithOrder(data);}}public void toArrayFirst(Object[] retVal){retVal[BiTree.this.foot++] = this.data;if (null != this.left)this.left.toArrayFirst(retVal);if (null != this.right)this.right.toArrayFirst(retVal);}public void toArrayMiddle(Object[] retVal){if (null != this.left)this.left.toArrayMiddle(retVal);retVal[BiTree.this.foot++] = this.data;if (null != this.right)public void toArrayLast(Object[] retVal){if (null != this.left)this.left.toArrayLast(retVal);if (null != this.right)this.right.toArrayLast(retVal);retVal[BiTree.this.foot++] = this.data;}}private int count = 0;private Node<T> root = null;private int foot = 0;@Overridepublic void addWithOrder(T data){if (null == data)return;if (null == this.root){this.root = new Node<T>(data);this.count++;} else{this.root.addWithOrder(data);}}@Overridepublic void addFirst(T[] data, T endFlag){if (null == data){return;}this.foot = 0;this.root = this.addFirstInternal(data, endFlag, this.root);}private Node<T> addFirstInternal(T[] data, T endFlag, Node<T> node) {if (data[this.foot].equals(endFlag)){this.foot++;return null;} else{node.data = data[this.foot];this.foot++;node.left = this.addFirstInternal(data, endFlag, node.left);node.right = this.addFirstInternal(data, endFlag, node.right);return node;}}@Overridepublic Object[] toArrayFirst(){if (this.isEmpty())return null;Object[] retVal = new Object[this.count];this.foot = 0;this.root.toArrayFirst(retVal);return retVal;}@Overridepublic Object[] toArrayMiddle(){if (this.isEmpty())return null;Object[] retVal = new Object[this.count];this.foot = 0;this.root.toArrayMiddle(retVal);return retVal;}@Overrideif (this.isEmpty())return null;Object[] retVal = new Object[this.count];this.foot = 0;this.root.toArrayLast(retVal);return retVal;}@Overridepublic int size(){return this.count;}@Overridepublic boolean isEmpty(){if (0 == this.count && null == this.root)return true;elsereturn false;}@Overridepublic void delete(T data){if (this.isEmpty())return;Map<String, Object> nodeDel = this.find(data);if (null == nodeDel)return;Node<T> node = (Node<T>) nodeDel.get("node"); Node<T> parent = (Node<T>) nodeDel.get("parent"); String direction = (String) nodeDel.get("direction");if (null == node.left && null == node.right){if (null == direction){this.root = null;} else{if ("left".equals(direction)){parent.left = null;} else if ("right".equals(direction)){parent.right = null;}}this.count--;return;}if (null != node.left && null == node.right){// 该节点只有左⼦树if (null == direction){Node<T> temp = this.root;this.root = this.root.left;temp.left = null;} else{if ("left".equals(direction)){parent.left = node.left;node.left = null;} else if ("right".equals(direction)){parent.right = node.left;node.left = null;}}this.count--;return;}if (null == node.left && null != node.right){// 该节点只有右⼦树Node<T> temp = this.root;this.root = this.root.right;temp.right = null;} else{if ("left".equals(direction)){parent.left = node.right;node.right = null;} else if ("right".equals(direction)){parent.right = node.right;node.right = null;}}this.count--;return;}Node<T> subsessor = this.getSubsessor(node);if (null != subsessor){//System.out.println("subsessor:" + subsessor.data);subsessor.left = node.left;if (null == direction){this.root = subsessor;} else if ("left".equals(direction)){parent.left = subsessor;} else if ("right".equals(direction)){parent.right = subsessor;}this.count--;}}private Node<T> getSubsessor(Node<T> nodeDel){Node<T> parent = nodeDel;Node<T> subsessor = nodeDel.right;subsessor = this.getSubsessorInternal(parent, subsessor, nodeDel);return subsessor;}private Node<T> getSubsessorInternal(Node<T> parent, Node<T> subsessor, Node<T> nodeDel) {if (null != subsessor.left){parent = subsessor;subsessor = subsessor.left;return getSubsessorInternal(parent, subsessor, nodeDel);} else{if (subsessor != nodeDel.right){parent.left = subsessor.right;subsessor.right = nodeDel.right;}return subsessor;}}private Map<String, Object> find(T data){return this.findInternal(this.root, null, null, data);}private Map<String, Object> findInternal(Node<T> node, Node<T> parent, String direction, T data) {Map<String, Object> retVal = null;if (node.data.equals(data)){retVal = new HashMap<>();retVal.put("node", node);retVal.put("parent", parent);retVal.put("direction", direction);return retVal;} else{// parent = node;// node = node.left;retVal = this.findInternal(node.left, node, "left", data);if(null != retVal)return retVal;}if (null != node.right){// parent = node;// node = node.right;retVal = this.findInternal(node.right, node, "right", data);if(null != retVal)return retVal;}}return retVal;}@Overridepublic boolean contains(T data){// TODO Auto-generated method stubreturn false;}}。
java实现二叉树的基本操作
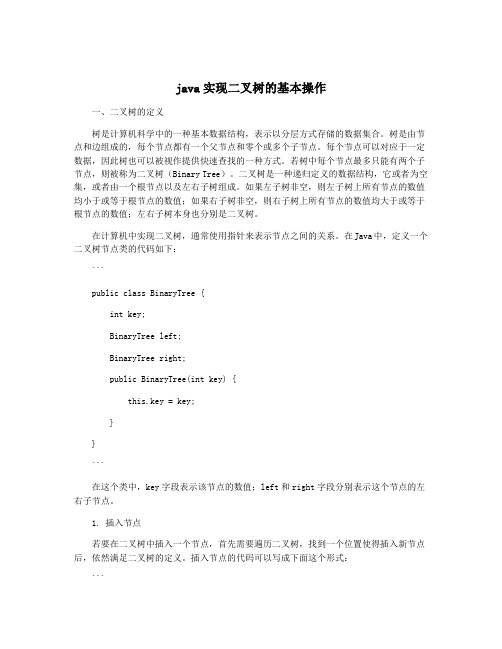
java实现二叉树的基本操作一、二叉树的定义树是计算机科学中的一种基本数据结构,表示以分层方式存储的数据集合。
树是由节点和边组成的,每个节点都有一个父节点和零个或多个子节点。
每个节点可以对应于一定数据,因此树也可以被视作提供快速查找的一种方式。
若树中每个节点最多只能有两个子节点,则被称为二叉树(Binary Tree)。
二叉树是一种递归定义的数据结构,它或者为空集,或者由一个根节点以及左右子树组成。
如果左子树非空,则左子树上所有节点的数值均小于或等于根节点的数值;如果右子树非空,则右子树上所有节点的数值均大于或等于根节点的数值;左右子树本身也分别是二叉树。
在计算机中实现二叉树,通常使用指针来表示节点之间的关系。
在Java中,定义一个二叉树节点类的代码如下:```public class BinaryTree {int key;BinaryTree left;BinaryTree right;public BinaryTree(int key) {this.key = key;}}```在这个类中,key字段表示该节点的数值;left和right字段分别表示这个节点的左右子节点。
1. 插入节点若要在二叉树中插入一个节点,首先需要遍历二叉树,找到一个位置使得插入新节点后,依然满足二叉树的定义。
插入节点的代码可以写成下面这个形式:```public void insert(int key) {BinaryTree node = new BinaryTree(key); if (root == null) {root = node;return;}BinaryTree temp = root;while (true) {if (key < temp.key) {if (temp.left == null) {temp.left = node;break;}temp = temp.left;} else {if (temp.right == null) {temp.right = node;break;}temp = temp.right;}}}```上面的代码首先创建了一个新的二叉树节点,然后判断二叉树根是否为空,若为空,则将这个节点作为根节点。
java实现的二叉树

Java 实现的二叉树Name: jiadongdong (10软件3)QQ:942011334南京信息工程大学tree12345//自定义的二叉树节点public class BinaryNode<T> {T data;BinaryNode left, right;public T getData() {return data;}public void setData(T data) {this.data = data;}public BinaryNode getLeft() {return left;}public void setLeft(BinaryNode left) {this.left = left;}public BinaryNode getRight() {return right;}public void setRight(BinaryNode right) {this.right = right;}public BinaryNode(){}public BinaryNode(T data){this.data = data;this.left = null;this.right = null;}public BinaryNode(T data,BinaryNode<T> left, BinaryNode<T> right ){this.data = data;this.left = left;this.right = right;}}//自定义的二叉树public class BinaryTree<T> {BinaryNode<T> root;BinaryTree<T> leftTree , rightTree;public BinaryTree<T> getLeftTree() {return leftTree;}public void setLeftTree(BinaryTree<T> leftTree) { this.leftTree = leftTree;}public BinaryTree<T> getRightTree() {return rightTree;}public void setRightTree(BinaryTree<T> rightTree) { this.rightTree = rightTree;}public BinaryNode<T> getRoot() {return root;}public void setRoot(BinaryNode<T> root) {this.root = root;}public BinaryTree(){}public BinaryTree(T data){this(data,null, null);}public BinaryTree(T data ,BinaryTree<T> leftTree , BinaryTree<T> rightTree){root = new BinaryNode<T>();root.setData(data);if(leftTree!=null)root.setLeft(leftTree.getRoot());if(rightTree!=null)root.setRight(rightTree.getRoot());}//中序遍历public void Inorder(BinaryTree<T> tree ){if(tree!=null){Inorder(tree.getLeftTree());System.out.print(tree.getRoot().getData());Inorder(tree.getRightTree());}}//先序遍历public void preOrder(BinaryTree<T> tree ){if(tree!=null){System.out.print(tree.getRoot().getData());preOrder(tree.getLeftTree());preOrder(tree.getRightTree());}}//后序遍历public void afterOrder(BinaryTree<T> tree ){if(tree!=null){afterOrder(tree.getLeftTree());afterOrder(tree.getRightTree());System.out.print(tree.getRoot().getData());}}//树的深度public int getDeepth(BinaryTree<T> tree){int deep =0;if(tree!=null)deep = 1+ Math.max(getDeepth(tree.getLeftTree()), getDeepth(tree.getRightTree()));return deep;}//统计叶子节点数public int getLeafNodes(BinaryTree<T> tree){int count = 0;if(tree!= null){if(tree.getLeftTree()==null&&tree.getRightTree()==null){return 1;}count = getLeafNodes(tree.getLeftTree())+getLeafNodes(tree.getRightTree());}return count;}}//测试二叉树public class TestTree {/***@param args*/public static void main(String[] args) {//初始化二叉树BinaryTree<Integer> treeroot = new BinaryTree<Integer>(0);BinaryTree<Integer> leftroot = new BinaryTree<Integer>(1);BinaryTree<Integer> leftroot1 = new BinaryTree<Integer>(3);BinaryTree<Integer> leftroot2 = new BinaryTree<Integer>(4);leftroot.setLeftTree(leftroot1);leftroot.setRightTree(leftroot2);BinaryTree<Integer> rightroot = new BinaryTree<Integer>(2); BinaryTree<Integer> rightroot1 = new BinaryTree<Integer>(5);rightroot.setLeftTree(rightroot1);treeroot.setLeftTree(leftroot);treeroot.setRightTree(rightroot);System.out.print("中序遍历结果为: ");treeroot.Inorder(treeroot);System.out.println();System.out.print("先序遍历结果为: ");treeroot.preOrder(treeroot);System.out.println();System.out.print("后序遍历结果为: ");treeroot.afterOrder(treeroot);System.out.println();System.out.print("此树的深度为: ");System.out.print(treeroot.getDeepth(treeroot));System.out.println();System.out.print("此树叶子节点的个数为:");System.out.println(treeroot.getLeafNodes(treeroot));}}运行结果:中序遍历结果为: 314052 先序遍历结果为: 013425 后序遍历结果为: 341520 此树的深度为: 3此树叶子节点的个数为:3。
数据结构和算法的Java实现二叉树

实现代码:略(具体 实现代码需要根据具 体需求和编程语言进 行编写)
应用场景举例
数据压缩
加密通信
哈夫曼编码是一种广泛使用的数据压缩算法, 它可以用于文件压缩、图像压缩等领域。通 过哈夫曼编码,可以将数据中的冗余信息去 除,从而达到压缩数据的效果。
哈夫曼编码也可以用于加密通信中。在通信 过程中,发送方和接收方可以事先约定好一 套哈夫曼编码规则,然后对传输的数据进行 编码和解码。由于哈夫曼编码具有前缀编码 的特性,因此可以保证数据传输的准确性和 安全性。
AVL树插入操作过程演示
查找插入位置
插入新节点
从根节点开始,按照二叉搜索树的规则查 找插入位置。
在找到的位置插入新节点。
更新节点高度
调整平衡
从插入位置开始,沿着父节点路径向上更 新节点高度。
在更新节点高度的过程中,检查每个节点 的平衡因子,若发现不平衡,则进行相应 的旋转操作以恢复平衡。
AVL树删除操作过程演示
完全二叉树
对于深度为D的二叉树,如果其前D-1层是一个满二叉树,且最后一层(第D层) 的节点都连续集中在左边,则称该二叉树为完全二叉树。
二叉树性质总结
在二叉树的第i层上至多有2^(i-1) 个节点(i≥1)。
深度为k的二叉树至多有2^k - 1 个节点(k≥1)。
对任何一棵二叉树T,如果其终 端节点数为n0,度为2的节点数 为n2,则n0 = n2 + 1。
红黑树删除操作过程演示
删除节点
首先,按照二叉查找树的规则删除指定节点。如果删 除的是黑色节点,需要进行颜色调整和旋转操作以保 持红黑树的性质。
调整颜色与旋转
具体调整过程包括:如果被删除节点的兄弟节点是红色 ,则将其兄弟节点变为黑色,将父节点变为红色,并进 行左旋操作;如果被删除节点的兄弟节点是黑色且其两 个子节点也是黑色,则将其兄弟节点变为红色,并向上 递归调整;如果被删除节点的兄弟节点是黑色且其左子 节点是红色而右子节点是黑色或空节点,则交换兄弟节 点与其左子节点的颜色,并进行右旋操作;最后将被删 除节点的父节点变为黑色,兄弟节点变为红色。
Java实现二叉树,Java实现队列

Java实现二叉树,Java实现队列实验 Java 实现二叉树一、实验目的利用JAVA的代码实现二叉树的结构二、实验代码定义一个结点类:package com.xiao.tree; /** ** @author WJQ 树结点类 */public class TreeNode { /*存数据的*/private Object value; /*左孩子结点*/private TreeNode leftChild; /*右孩子结点*/private TreeNode rightChild; /*以下是setter和getter方法*/ public Object getValue() { return value; }public void setValue(Object value) { this.value = value; }public TreeNode getLeftChild() {return leftChild;}public void setLeftChild(TreeNode leftChild) { this.leftChild = leftChild; }public TreeNode getRightChild() { return rightChild; }public void setRightChild(TreeNode rightChild) { this.rightChild = rightChild; } }定义一个树类:package com.xiao.tree; /** ** @author WJQ* 树的结构,树中只有结点 */public class Tree { /*结点属性*/private TreeNode node;public TreeNode getNode() { return node; }public void setNode(TreeNode node) { this.node = node; } }//定义一个队列类:package com.xiao.tree; /*** @author WJQ* 该类是在向树中加入结点时需要使用的*/import java.util.LinkedList;public class Queue {private LinkedList list; }/*一初始化就new一个list*/public Queue(){list = new LinkedList(); }/*结点入队列*/public void enQueue(TreeNode node){ this.list.add(node); } /*队首元素出队列*/public TreeNode outQueue(){return this.list.removeFirst(); }/*队列是否为空*/public boolean isEmpty(){return this.list.isEmpty(); }public LinkedList getList() { return list; }public void setList(LinkedList list) { this.list = list; }//定义一个二叉树类: package com.xiao.tree; /*** @author WJQ 二叉树,增加结点,前序遍历,中序遍历,后序遍历 */public class BinaryTree {private Tree tree; private Queue queue;/* 构造函数,初始化的时候就生成一棵树 */ public BinaryTree() { tree = new Tree(); }/* 向树中插入结点 */public void insertNode(TreeNode node) {/* 如果树是空树,则生成一颗树,之后把当前结点插入到树中,作为根节点 ,根节点处于第0层 */if (tree.getNode() == null) { tree.setNode(node); return; } else {/* 根节点入队列 */queue = new Queue();queue.enQueue(tree.getNode()); /** 队列不空,取出队首结点,如果队首结点的左右孩子有一个为空的或者都为空,则将新结点插入到相应的左右位置,跳出循环,如果左右孩子都不为空* ,则左右孩子入队列,继续while循环*/while (!queue.isEmpty()) {TreeNode temp = queue.outQueue(); if (temp.getLeftChild() == null){ temp.setLeftChild(node); return;} else if (temp.getRightChild() == null) { temp.setRightChild(node); return; } else {/* 左右孩子不空,左右孩子入队列 */}}}queue.enQueue(temp.getLeftChild());queue.enQueue(temp.getRightChild()); } }/* 中序遍历 */public void midOrder(TreeNode node) { if (node != null) {this.midOrder(node.getLeftChild()); System.out.println(node.getValue()); this.midOrder(node.getRightChild()); } }/* 先序遍历 */public void frontOrder(TreeNode node) { if (node != null) {System.out.println(node.getValue());this.frontOrder(node.getLeftChild());this.frontOrder(node.getRightChild()); } }/* 后序遍历 */public void lastOrder(TreeNode node) { if (node != null) {stOrder(node.getLeftChild());stOrder(node.getRightChild());System.out.println(node.getValue()); } }public Tree getTree() { return tree; }最好来一个客户端测试一下:package com.xiao.tree;感谢您的阅读,祝您生活愉快。
Java程序设计中的二叉查找树与图的实现案例

Java程序设计中的二叉查找树与图的实现案例一、二叉查找树的实现案例在Java程序设计中,二叉查找树是一种非常常用的数据结构,它可以高效地进行插入、删除和查找操作。
下面我们将通过一个实例来介绍二叉查找树的实现和应用。
1.1 实现二叉查找树的节点类首先,我们需要定义一个二叉查找树的节点类,包含数据域和左右子节点域。
```javaclass Node {int data;Node leftChild;Node rightChild;public Node(int data) {this.data = data;this.leftChild = null;this.rightChild = null;}}```1.2 实现二叉查找树类接下来,我们定义一个二叉查找树类,包含插入节点、删除节点和查找节点的方法。
```javaclass BinarySearchTree {private Node root;public BinarySearchTree() {root = null;}// 插入节点public void insert(int data) {root = insertNode(root, data);}private Node insertNode(Node root, int data) {if (root == null) {root = new Node(data);return root;}if (data < root.data) {root.leftChild = insertNode(root.leftChild, data); } else if (data > root.data) {root.rightChild = insertNode(root.rightChild, data); }return root;}// 删除节点public void delete(int data) {root = deleteNode(root, data);}private Node deleteNode(Node root, int data) {// 空树if (root == null) {return root;}if (data < root.data) {root.leftChild = deleteNode(root.leftChild, data);} else if (data > root.data) {root.rightChild = deleteNode(root.rightChild, data);} else {// 找到待删除节点// 情况1:无子节点或只有一个子节点if (root.leftChild == null) {return root.rightChild;} else if (root.rightChild == null) {return root.leftChild;}// 情况2:有两个子节点root.data = getMinValue(root.rightChild);root.rightChild = deleteNode(root.rightChild, root.data); }return root;}// 获取最小值private int getMinValue(Node root) {if (root.leftChild == null) {return root.data;}return getMinValue(root.leftChild);}// 查找节点public boolean search(int data) {return searchNode(root, data);}private boolean searchNode(Node root, int data) { if (root == null) {return false;}if (data == root.data) {return true;} else if (data < root.data) {return searchNode(root.leftChild, data);} else {return searchNode(root.rightChild, data);}}}```1.3 二叉查找树的应用案例现在,我们使用二叉查找树来实现一个基于学生信息的系统。
数据结构之二叉树java实现

数据结构之⼆叉树java实现⼆叉树是⼀种⾮线性数据结构,属于树结构,最⼤的特点就是度为2,也就是每个节点只有⼀个左⼦树和⼀个右⼦树。
⼆叉树的操作主要为创建,先序遍历,中序遍历,后序遍历。
还有层次遍历。
遍历有两种⽅式,⼀是采⽤递归的⽅式,⼆是采⽤转换为栈进⾏遍历,对⼆叉树的遍历本质上市将⾮线性结构转换为线性序列。
1package tree;23import java.util.ArrayList;4import java.util.List;5import java.util.Queue;67//通过先序⽅式将数组依次添加到⼆叉树8public class CreateTreeByArray<E> {9public static class Node<E>{10 Node<E> left = null; //左⼦树11 Node<E> right = null; //右⼦树12 E data = null; //数据域1314//初始化节点15public Node(E data){16this.data = data;17this.left = null;18this.right = null;19 }2021public Node(){2223 }24 }25private Node<E> root = null; //根节点26private List<Node<E>> list = null; //节点列表,⽤于将数组元素转化为节点2728public Node<E> getRoot(){29return this.root;30 }3132//将将数组转化为⼀颗⼆叉树,转换规则:依次为节点列表中节点的左右孩⼦赋值。
左孩⼦为i*2+1,右孩⼦为i*2+233 @SuppressWarnings("unchecked")34public void createTreeByArray(Object[] array){35this.list = new ArrayList<Node<E>>();3637//将数组添加到节点列表38for (int i = 0; i < array.length; i++) {39 list.add(new Node<E>((E) array[i]));40 }4142 System.out.println("头节点->" + list.get(0).data);43this.root = new Node<E>(list.get(0).data); //根节点4445//为⼆叉树指针赋值46for(int j = 0; j < (list.size() / 2); j ++){47try {48//为左⼦树赋值 j*2+149 list.get(j).left = list.get(j * 2 + 1);50 System.out.println("节点" + list.get(j).data + "左⼦树 ->" + list.get(j * 2 + 1).data);51//为右⼦树赋值 j*2+252 list.get(j).right = list.get(j * 2 + 2);53 System.out.println("节点" + list.get(j).data + "右⼦树 ->" + list.get(j * 2 + 2).data);54 } catch (Exception e) {5556 }57 }5859 }6061//先序遍历⼆叉树62public void Indorder(Node<E> root){63if(root == null){64return;65 }66 System.out.println(root.data);67 Indorder(root.left); //递归输出左⼦树68 Indorder(root.right); //递归遍历右⼦树69 }7071//中序遍历⼆叉树72public void inOrderTraverse(Node<E> root){73if(root == null){74return;75 }76 inOrderTraverse(root.left); //遍历左⼦树77 System.out.println(root.data);78 inOrderTraverse(root.right); //遍历右⼦树79 }8081//后序遍历82public void postOrderTraverse(Node<E> root){83if(root == null){84return;85 }86 postOrderTraverse(root.left); //遍历左⼦数节点87 postOrderTraverse(root.right); //遍历右⼦树节点88 System.out.println(root.data); //从下往上遍历89 }909192public static void main(String[] args) {93 CreateTreeByArray<Integer> createTreeByArray = new CreateTreeByArray<Integer>();94 Object[] arrays = {new Integer(1),new Integer(2),new Integer(3),new Integer(4),5,6,7,8,9,10};95 createTreeByArray.createTreeByArray(arrays);96 System.out.println("===============================");97 createTreeByArray.postOrderTraverse(createTreeByArray.list.get(0));9899 }100101 }。
二叉树可视化Java语言实现(完整版-打开即可运行)

一、public class BinaryNode<E> {private E element;private BinaryNode<E> left;private BinaryNode<E> right;public E getElement() {return element;public void setElement(E element) {this.element = element;public BinaryNode<E> getLeft() {return left;public void setLeft(BinaryNode<E> left) {this.left = left;public BinaryNode<E> getRight() {return right;public void setRight(BinaryNode<E> right) {this.right = right;// 递归方法构造tree,单个输出:public static void structPrint(BinaryNode<Integer> e) { if (e.element > 12)return;else {// System.out.print("(" + e.element + ")");BinaryNode<Integer> left = new BinaryNode<Integer>();left.setElement(e.element * 2 + 1);BinaryNode<Integer> right = new BinaryNode<Integer>();right.setElement(e.element * 2 + 2);// System.out.print(" ");// System.out.print("(" + e.element + ")");// System.out.print("["+left.getElement()+" "+right.getElement()+"]");if (left.getElement() > 12)return;else {e.setLeft(left);e.setRight(right);structPrint(left);structPrint(right);// 递归前序遍历public static void inorder(BinaryNode<Integer> root) { if (root != null) {// System.out.print(root.getElement());//调整位置得到中、后序。
二叉树(Java实现)

⼆叉树(Java实现)⼀、常见⽤语1、逻辑结构:描述数据之间逻辑上的相关关系。
分为线性结构(如,字符串),和⾮线性结构(如,树,图)。
2、物理结构:描述数据的存储结构,分为顺序结构(如,数组)和链式结构。
3、结点的度:⼀个节点⼦树(即分⽀)个数。
4、叶结点:也称为终端节点,指度为0的节点。
5、树的深度(⾼度):树中结点层次的最⼤值。
6、有序树:⼦树有先后次序之分(我的理解就是左右节点次序不可以颠倒)。
7、同构:将节点适当重命名,即可得两颗完全相同的树8、孩⼦节点:⼀个节点的直接后继节点。
9、双亲节点:⼀个节点的直接前驱节点。
⼆、⼆叉树中的概念1、⼆叉树:满⾜以下两个条件的树称为⼆叉树①节点的度不可以超过2②节点的孩⼦节点次序不可颠倒2、满⼆叉树:每层得节点数都是满的,即2i-13、完全⼆叉树:节点1~n分别对应于满⼆叉树的节点1~n4、完全⼆叉树的性质:(1)若节点序号为i(i>1),则其双亲节点序号为i/2。
(这⾥是整除)(2)若节点序号为i(i>=1),则其左⼦节点序号为2i。
(3)若节点序号为i (i>=1),则其右⼦节点序号为2i+1。
三、⼆叉树的操作1、⼆叉树节点的存储结构public class TreeNode {String data;TreeNode LChild;TreeNode RChild;TreeNode(String data) {this.data = data;}public String toString() {return data;}}使⽤前序遍历创建⼆叉树是⽐较合适,按照逻辑,总要先创建根节点在创建左右⼦树,总不能还没有创建根节点就把root.LChild传递出去吧。
private static String[] tree = {"A","B",".",".","C","D",".",".","."};private static int i = 0;//先序创建⼆叉树是⽐较合适的TreeNode inOrderCreateBTree() {TreeNode bt = null;String s = tree[i++];if(s == ".") {return bt;}else {bt = new TreeNode(s);bt.LChild = inOrderCreateBTree();bt.RChild = inOrderCreateBTree();return bt;}}可以⽤⾮递归的⽅式建树,但是难度还是挺⼤的。
转二叉树之Java实现二叉树基本操作

转⼆叉树之Java实现⼆叉树基本操作参考⾃《Java数据结构与算法》定义⼀个节点类,使节点与⼆叉树操作分离1. class Node {2. int value;3. Node leftChild;4. Node rightChild;5.6. Node(int value) {7. this.value = value;8. }9.10. public void display() {11. System.out.print(this.value + "\t");12. }13.14. @Override15. public String toString() {16. // TODO Auto-generated method stub17. return String.valueOf(value);18. }19. }需要实现的⼆叉树操作1. class BinaryTree {2. private Node root = null;3.4. BinaryTree(int value) {5. root = new Node(value);6. root.leftChild = null;7. root.rightChild = null;8. }9. public Node findKey(int value) {} //查找10. public String insert(int value) {} //插⼊11. public void inOrderTraverse() {} //中序遍历递归操作12. public void inOrderByStack() {} //中序遍历⾮递归操作13. public void preOrderTraverse() {} //前序遍历14. public void preOrderByStack() {} //前序遍历⾮递归操作15. public void postOrderTraverse() {} //后序遍历16. public void postOrderByStack() {} //后序遍历⾮递归操作17. public int getMinValue() {} //得到最⼩(⼤)值18. public boolean delete(int value) {} //删除19. }查找数据:1. public Node findKey(int value) {2. Node current = root;3. while (true) {4. if (value == current.value) {5. return current;6. } else if (value < current.value) {7. current = current.leftChild;8. } else if (value > current.value) {9. current = current.rightChild;10. }11.12. if (current == null) {13. return null;14. }15. }16. }插⼊数据:与查找数据类似,不同点在于当节点为空时,不是返回⽽是插⼊1. public String insert(int value) {2. String error = null;3.4. Node node = new Node(value);5. if (root == null) {6. root = node;7. root.leftChild = null;8. root.rightChild = null;9. } else {10. Node current = root;11. Node parent = null;12. while (true) {13. if (value < current.value) {14. parent = current;15. current = current.leftChild;16. if (current == null) {17. parent.leftChild = node;18. break;19. }20. } else if (value > current.value) {21. parent = current;22. current = current.rightChild;23. if (current == null) {24. parent.rightChild = node;25. break;26. }27. } else {28. error = "having same value in binary tree";29. }30. } // end of while31. }32. return error;33. }遍历数据:1)中序遍历:最常⽤的⼀种遍历⽅法1. /**2. * //中序遍历(递归):3. * 1、调⽤⾃⾝来遍历节点的左⼦树4. * 2、访问这个节点5. * 3、调⽤⾃⾝来遍历节点的右⼦树6. */7. public void inOrderTraverse() {8. System.out.print("中序遍历:");9. inOrderTraverse(root);10. System.out.println();11. }12.13. private void inOrderTraverse(Node node) {14. if (node == null)15. return ;16.17. inOrderTraverse(node.leftChild);18. node.display();19. inOrderTraverse(node.rightChild);20. }1. /**2. * 中序⾮递归遍历:3. * 1)对于任意节点current,若该节点不为空则将该节点压栈,并将左⼦树节点置为current,重复此操作,直到current为空。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
一、public class BinaryNode<E> {private E element;private BinaryNode<E> left;private BinaryNode<E> right;public E getElement() {return element;}public void setElement(E element) {this.element = element;}public BinaryNode<E> getLeft() {return left;}public void setLeft(BinaryNode<E> left) {this.left = left;}public BinaryNode<E> getRight() {return right;}public void setRight(BinaryNode<E> right) {this.right = right;}// 递归方法构造tree,单个输出:public static void structPrint(BinaryNode<Integer> e) {if (e.element > 12)return;else {// System.out.print("(" + e.element + ")");BinaryNode<Integer> left = new BinaryNode<Integer>();left.setElement(e.element * 2 + 1);BinaryNode<Integer> right = new BinaryNode<Integer>();right.setElement(e.element * 2 + 2);// System.out.print(" ");// System.out.print("(" + e.element + ")");// System.out.print("["+left.getElement()+" "+right.getElement()+"]");if (left.getElement() > 12)return;else {e.setLeft(left);e.setRight(right);structPrint(left);structPrint(right);}}}// 递归前序遍历public static void inorder(BinaryNode<Integer> root) {if (root != null) {// System.out.print(root.getElement());//调整位置得到中、后序。
inorder(root.getLeft());// System.out.print(root.getElement());inorder(root.getRight());System.out.print("(" + root.element + ")");}}// 查找叶子(前序)public static BinaryNode<Integer> search(BinaryNode<Integer> root, int e) { if (root != null) {if (root.getElement() == e) {// System.out.print("*" + root.element + "*");return root;} else {search(root.getLeft(), e);search(root.getRight(), e);}}return root;}public static void test(BinaryNode<Integer> root, int e) {BinaryNode<Integer> parent = new BinaryNode<Integer>();int i1 = (e - 2) / 2;parent = BinaryNode.search(root, i1);System.out.println("*~!!" + BinaryNode.search(root, i1).getElement() + "!!*");}// 删除节点(前序):public static void delete(BinaryNode<Integer> root, int e) { // if(e==0) BinaryNode.setnull(root);if (e == 0) {root.setLeft(null);root.setRight(null);root.setElement(null);} else {if (root != null) {if (root.getElement() * 2 + 1 == e) {root.setLeft(null);} else {if (root.getElement() * 2 + 2 == e) {root.setRight(null);}// System.out.print("*" + root.element + "*");}delete(root.getLeft(), e);delete(root.getRight(), e);}}}// 使用删除中的setnull;public static void setnull(BinaryNode<Integer> root) {// root.setElement(null);// root.setLeft(null);// root.setRight(null);// System.out.println(root.getElement());root = null;}}二、package Lessons13_9;public class Show1_12 {public static void main(String[] args){BinaryNode<Integer> root=new BinaryNode<Integer>();root.setElement(0);System.out.println("前序遍历显示结果:");BinaryNode.structPrint(root);System.out.println();System.out.println("----------------------分割线1---------------------");System.out.println("后序遍历显示结果:");BinaryNode.inorder(root);System.out.println();System.out.println("----------------------分割线2---------------------");System.out.println("查询结果显示:");BinaryNode.search(root,4);System.out.println();System.out.println("total nodes number1: "+Show1_12.nodes(root)); System.out.println("----------------------分割线3---------------------");System.out.println("删除结果显示:");BinaryNode.delete(root,0);//BinaryNode.inorder(root);System.out.println();System.out.println("----------------------分割线4---------------------");//BinaryNode.setnull(root);//root = null;//root = null;//BinaryNode.structPrint(root);//BinaryNode.test(root, 7);//BinaryNode.inorder(root);//System.out.println("total nodes number2.1: "+Show1_12.nodes(root));//BinaryNode.setnull(root);//BinaryNode.inorder(root);//root = null;//System.out.println();//System.out.println("total nodes number2: "+Show1_12.nodes(root));}//total nodes number:static int nodes(BinaryNode<Integer> tree) {if (tree.getElement()==0)return 0;else {int left = nodes(tree.getLeft());int right = nodes(tree.getRight());return left + right + 1;//注意:必须+1!!!}}}三、——————————————————————————————————————————package Lessons13_9;import java.awt.*;import java.awt.event.*;import javax.swing.*;public class TreeControl extends JPanel {private BinaryNode<Integer> tree;//private JTextField jtfKey = new JTextField(5);private TreeView View = new TreeView();//private JButton jbtInsert = new JButton("Insert");//private JButton jbtDelete = new JButton("Delete");public TreeControl(BinaryNode<Integer> tree){this.tree = tree;setUI();}private void setUI(){this.setLayout(new BorderLayout());add(View,BorderLayout.CENTER);JPanel panel = new JPanel();//.add(new JLabel("Enter a key: "));//panel.add(jtfKey);//panel.add(jbtInsert);//panel.add(jbtDelete);//add(panel,BorderLayout.SOUTH);/*jbtInsert.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){int key = Integer.parseInt(jtfKey.getText());if(BinaryNode.search(tree, key) != null){JOPtionPanel=showMessageDialog(null,key+"is already in the tree" );}else{tree.insert(key);view.repaint();}}});*/}//jbtDelete;class TreeView extends JPanel{private int radius = 20;private int vGap = 50;protected void paintComponent(Graphics g){super.paintComponent(g);if(tree!=null){//displayTree(g,tree,getWidth()/2,30,getWidth()/4);displayTree(g,tree,getWidth()/2,30,getWidth()/4);}}private void displayTree(Graphics g,BinaryNode root,int x, int y, int hGap){//这里x,y 是结点中心!g.drawOval(x-radius,y-radius,2*radius,2*radius);g.drawString(root.getElement()+"", x-6, y+4);if(root.getLeft()!=null){connectLeftChild(g,x,y,x-hGap,y+vGap);displayTree(g,root.getLeft(),x-hGap,y+vGap,hGap/2);}if(root.getRight()!=null){connectRightChild(g,x,y,x+hGap,y+vGap);displayTree(g,root.getRight(),x+hGap,y+vGap,hGap/2);}}private void connectLeftChild(Graphics g,int x1,int y1,int x2,int y2){double d = Math.sqrt(vGap*vGap+(x2-x1)*(x2-x1));int a1=(int)(radius*vGap/d);int a2=(int)(radius*(x1-x2)/d);int x11=(int)(x1 - a2);int y11=(int)(y1 + a1);int x21=(int)(x2 + a2);int y21=(int)(y2 - a1);g.drawLine(x11,y11,x21,y21);//g.drawLine(x1,y1,x2,y2);}private void connectRightChild(Graphics g,int x1,int y1,int x2,int y2){double d = Math.sqrt(vGap*vGap+(x2-x1)*(x2-x1));int a1=(int)(radius*vGap/d);int a2=(int)(radius*(x2-x1)/d);int x11=(int)(x1 + a2);int y11=(int)(y1 + a1);int x21=(int)(x2 - a2);int y21=(int)(y2 - a1);g.drawLine(x11,y11,x21,y21);//g.drawLine(x1,y1,x2,y2);}}}四——————————————————————————————————————————————————package Lessons13_9;import javax.swing.*;public class Display_Tree extends JApplet{public Display_Tree(){BinaryNode<Integer> root=new BinaryNode<Integer>();root.setElement(0);BinaryNode.structPrint(root);add(new TreeControl(root));}}。