C Primer Plus(第5版)习题答案
《C Primer Plus》(Fifth Edition)编程练习参考答案 第五章
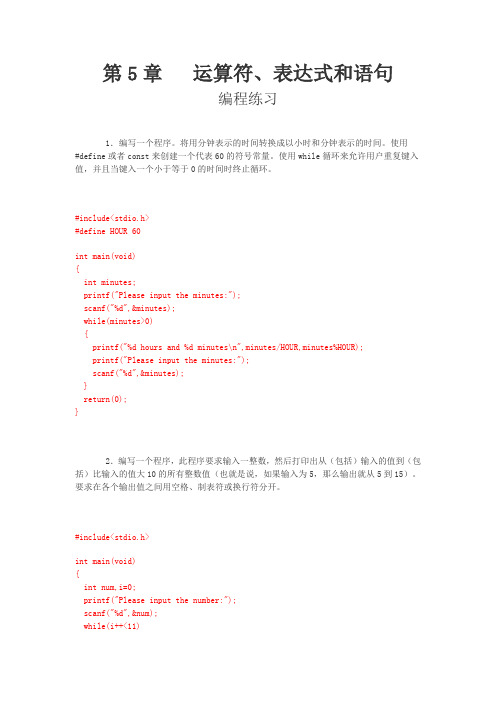
第5章运算符、表达式和语句编程练习1.编写一个程序。
将用分钟表示的时间转换成以小时和分钟表示的时间。
使用#define或者const来创建一个代表60的符号常量。
使用while循环来允许用户重复键入值,并且当键入一个小于等于0的时间时终止循环。
#include<stdio.h>#define HOUR 60int main(void){int minutes;printf("Please input the minutes:");scanf("%d",&minutes);while(minutes>0){printf("%d hours and %d minutes\n",minutes/HOUR,minutes%HOUR);printf("Please input the minutes:");scanf("%d",&minutes);}return(0);}2.编写一个程序,此程序要求输入一整数,然后打印出从(包括)输入的值到(包括)比输入的值大10的所有整数值(也就是说,如果输入为5,那么输出就从5到15)。
要求在各个输出值之间用空格、制表符或换行符分开。
#include<stdio.h>int main(void){int num,i=0;printf("Please input the number:");scanf("%d",&num);while(i++<11)printf("%d ",num++);}return(0);}3.编写一个程序,该程序要求用户输入天数,然后将该值转换为周数和天数。
例如,此程序将把18天转换成2周4天。
用下面的格式显示结果:使用一个while循环让用户重复输入天数;当用户输入一个非正数(如0或-20)时,程序将终止循环。
C++Primer_Plus_第五版习题参考答案

Solutions for Programming Exercises in C++ Primer Plus, 5th Edition Chapter 2// pe2-2.cpp#include <iostream>int main(void){using namespace std;cout << "Enter a distance in furlongs: ";double furlongs;cin >> furlongs;double feet;feet = 220 * furlongs;cout << furlongs << " furlongs = "<< feet << " feet\n";return 0;}// pe2-3.cpp#include <iostream>using namespace std;void mice();void run();int main(){mice();mice();run();run();return 0;}void mice(){cout << "Three blind mice\n";}void run(){cout << "See how they run\n";}// pe2-4.cpp#include <iostream>double C_to_F(double);int main(){using namespace std;cout << "Enter a temperature in Celsius: ";double C;cin >> C;double F;F = C_to_F(C);cout << C << " degrees Celsius = "<< F << " degrees Fahrenheit\n";return 0;}double C_to_F(double temp){return 1.8 * temp + 32.0;}Chapter 3// pe3-1.cpp#include <iostream>const int Inch_Per_Foot = 12;int main(void){using namespace std;// Note: some environments don't support the backspace charactercout << "Please enter your height in inches: ___/b/b/b ";int ht_inch;cin >> ht_inch;int ht_feet = ht_inch / Inch_Per_Foot;int rm_inch = ht_inch % Inch_Per_Foot;cout << "Your height is " << ht_feet << " feet, ";cout << rm_inch << " inch(es).\n";return 0;}// pe3-3.cpp#include <iostream>const double MINS_PER_DEG = 60.0;const double SECS_PER_MIN = 60.0;int main(){using namespace std;int degrees;int minutes;int seconds;double latitude;cout << "Enter a latitude in degrees, minutes, and seconds:\n";cout << "First, enter the degrees: ";cin >> degrees;cout << "Next, enter the minutes of arc: ";cin >> minutes;cout << "Finally, enter the seconds of arc: ";cin >> seconds;latitude = degrees + (minutes + seconds / SECS_PER_MIN)/MINS_PER_DEG;cout << degrees << " degrees, " << minutes << " minutes, "<< seconds << " seconds = " << latitude << " degrees\n";return 0;}// pe3-5.cpp#include <iostream>int main(void){using namespace std;cout << "How many miles have you driven your car? ";float miles;cin >> miles;cout << "How many gallons of gasoline did the car use? ";float gallons;cin >> gallons;cout << "Your car got " << miles / gallons;cout << " miles per gallon.\n";return 0;}// pe3-6.cpp#include <iostream>const double KM100_TO_MILES = 62.14;const double LITERS_PER_GALLON = 3.875;int main ( void ){using namespace std;double euro_rating;double us_rating;cout << "Enter fuel consumption in liters per 100 km: ";cin >> euro_rating;// divide by LITER_PER_GALLON to get gallons per 100-km// divide by KM100_TO_MILES to get gallons per mile// invert result to get miles per gallonus_rating = (LITERS_PER_GALLON * KM100_TO_MILES) / euro_rating;cout << euro_rating << " liters per 100 km is ";cout << us_rating << " miles per gallon.\n";return 0;}Chapter 4// pe4-2.cpp -- storing strings in string objects#include <iostream>#include <string>int main(){using namespace std;string name;string dessert;cout << "Enter your name:\n";getline(cin, name); // reads through newlinecout << "Enter your favorite dessert:\n";getline(cin, dessert);cout << "I have some delicious " << dessert;cout << " for you, " << name << ".\n";return 0;}// pe4-3.cpp -- storing strings in char arrays#include <iostream>#include <cstring>const int SIZE = 20;int main(){using namespace std;char firstName[SIZE];char lastName[SIZE];char fullName[2*SIZE + 1];cout << "Enter your first name: ";cin >> firstName;cout << "Enter your last name: ";cin >> lastName;strncpy(fullName,lastName,SIZE);strcat(fullName, ", ");strncat(fullName, firstName, SIZE);fullName[SIZE - 1] = '\0';cout << "Here's the information in a single string: "<< fullName << endl;return 0;}// pe4-5.cpp// a candybar structurestruct CandyBar {char brand[40];double weight;int calories;};#include <iostream>int main(){using namespace std; //introduces namespace stdCandyBar snack = { "Mocha Munch", 2.3, 350 };cout << "Brand name: " << snack.brand << endl;cout << "Weight: " << snack.weight << endl;cout << "Calories: " << snack.calories << endl;return 0;}// p#include <iostream>const int Slen = 70;struct pizza {char name[Slen];float diameter;float weight;};int main(void){using namespace std;pizza pie;cout << "What is the name of the pizza company? ";cin.getline(, Slen);cout << "What is the diameter of the pizza in inches? ";cin >> pie.diameter;cout << "How much does the pizza weigh in ounces? ";cin >> pie.weight;cout << "Company: " << << "\n";cout << "Diameter: " << pie.diameter << " inches\n";cout << "Weight: " << pie.weight << " ounces\n";return 0;}Chapter 5// pe5-2.cpp#include <iostream>int main(void){using namespace std;double sum = 0.0;double in;cout << "Enter a number (0 to terminate) : ";cin >> in;while (in != 0) {sum += in;cout << "Running total = " << sum << "\n";cout << "Enter next number (0 to terminate) : ";cin >> in;}cout << "Bye!\n";return 0;}// pe5-4.cpp// book sales#include <iostream>const int MONTHS = 12;const char * months[MONTHS] = {"January", "February", "March", "April","May", "June", "July", "August", "September","October", "November", "December"};int main(){using namespace std; //introduces namespace stdint sales[MONTHS];int month;cout << "Enter the monthly sales for \"C++ for Fools\":\n";for (month = 0; month < MONTHS; month++){cout << "Sales for " << months[month] << ": ";cin >> sales[month];}double total = 0.0;for (month = 0; month < MONTHS; month++)total += sales[month];cout << "Total sales: " << total << endl;return 0;}// pe5-6.cpp#include <iostream>struct car { char name[20]; int year;};int main(void){using namespace std;int n;cout << "How many cars do you wish to catalog?: ";cin >> n;while(cin.get() != '\n') // get rid of rest of line;car * pc = new car [n];int i;for (i = 0; i < n; i++){cout << "Car #" << (i + 1) << ":\n";cout << "Please enter the make: ";cin.getline(pc[i].name,20);cout << "Please enter the year made: ";cin >> pc[i].year;while(cin.get() != '\n') // get rid of rest of line;}cout << "Here is your collection:\n";for (i = 0; i < n; i++)cout << pc[i].year << " " << pc[i].name << "\n";delete [] pc;return 0;}// pe5-7.cpp -- count words using C-style string#include <iostream>#include <cstring> // prototype for strcmp()const int STR_LIM = 50;int main(){using namespace std;char word[STR_LIM];int count = 0;cout << "Enter words (to stop, type the word done):\n";while (cin >> word && strcmp("done", word))++count;cout << "You entered a total of " << count << " words.\n";return 0;}// pe5-9.cpp//nested loops#include <iostream>int main(){using namespace std; //introduces namespace stdint rows;int row;int col;int periods;cout << "Enter number of rows: ";cin >> rows;for (row = 1; row <= rows; row++){periods = rows - row;for (col = 1; col <= periods; col++)cout << '.';// col already has correct value for next loopfor ( ; col <= rows; col++)cout << '*';cout << endl;}return 0;}Chapter 6// pe6-1.cpp#include <iostream>#include <cctype>int main( ){using namespace std; //introduces namespace std char ch;cin.get(ch);while(ch != '@'){if (!isdigit(ch)){if (isupper(ch))ch = tolower(ch);else if (islower(ch))ch = toupper(ch);cout << ch;}cin.get(ch);}return 0;}// pe6-3.cpp#include <iostream>int main(void){using namespace std;cout << "Please enter one of the following choices:\n";cout << "c) carnivore p) pianist\n"<< "t) tree g) game\n";char ch;cin >> ch;while (ch != 'c' && ch != 'p' && ch != 't' && ch != 'g'){cout << "Please enter a c, p, t, or g: ";cin >> ch;}switch (ch){case 'c' : cout << "A cat is a carnivore.\n";break;case 'p' : cout << "Radu Lupu is a pianist.\n";break;case 't' : cout << "A maple is a tree.\n";break;case 'g' : cout << "Golf is a game.\n";break;default : cout << "The program shouldn't get here!\n";}return 0;}// pe6-5.cpp// Neutronia taxation#include <iostream>const double LEV1 = 5000;const double LEV2 = 15000;const double LEV3 = 35000;const double RATE1 = 0.10;const double RATE2 = 0.15;const double RATE3 = 0.20;int main( ){using namespace std;double income;double tax;cout << "Enter your annual income in tvarps: ";cin >> income;if (income <= LEV1)tax = 0;else if (income <= LEV2)tax = (income - LEV1) * RATE1;else if (income <= LEV3)tax = RATE1 * (LEV2 - LEV1) + RATE2 * (income - LEV2);elsetax = RATE1 * (LEV2 - LEV1) + RATE2 * (LEV3 - LEV2) + RATE3 * (income - LEV3);cout << "You owe Neutronia " << tax << " tvarps in taxes.\n";return 0;}// pe6-7.cpp#include <iostream>#include <string>int main(){using namespace std;string word;char ch;int vowel = 0;int consonant = 0;int other = 0;cout << "Enter words (q to quit):\n";cin >> word;while ( word != "q"){ch = tolower(word[0]);if (isalpha(ch)){if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o'|| ch == 'u')vowel++;elseconsonant++;}elseother++;cin >> word;}cout << vowel <<" words beginning with vowels\n";cout << consonant << " words beginning with consonants\n";cout << other << " others\n";return 0;}// pe6-8.cpp -- counting characters#include <iostream>#include <fstream> // file I/O suppport#include <cstdlib> // support for exit()const int SIZE = 60;int main(){using namespace std;char filename[SIZE];char ch;ifstream inFile; // object for handling file inputcout << "Enter name of data file: ";cin.getline(filename, SIZE);inFile.open(filename); // associate inFile with a fileif (!inFile.is_open()) // failed to open file{cout << "Could not open the file " << filename << endl;cout << "Program terminating.\n";exit(EXIT_FAILURE);}int count = 0; // number of items readinFile >> ch; // get first valuewhile (inFile.good()) // while input good and not at EOF {count++; // one more item readinFile >> ch; // get next value}cout << count << " characters in " << filename << endl;inFile.close(); // finished with the filereturn 0;}Chapter 7//pe7-1.cpp -- harmonic mean#include <iostream>double h_mean(double x, double y);int main(void){using namespace std;double x,y;cout << "Enter two numbers (a 0 terminates): ";while (cin >> x >> y && x * y != 0)cout << "harmonic mean of " << x << " and "<< y << " = " << h_mean(x,y) << "\n";/* or do the reading and testing in two parts:while (cin >> x && x != 0){cin >> y;if (y == 0)break;...*/cout << "Bye\n";return 0;}double h_mean(double x, double y){return 2.0 * x * y / (x + y);}// pe7-3.cpp#include <iostream>struct box {char maker[40];float height;float width;float length;float volume;};void showbox(box b);void setbox(box * pb);int main(void){box carton = {"Bingo Boxer", 2, 3, 5}; // no volume provided setbox(&carton);showbox(carton);return 0;}void showbox(box b){using namespace std;cout << "Box maker: " << b.maker<< "\nheight: " << b.height<< "\nlwidth: " << b.width<< "\nlength: " << b.length<< "\nvolume: " << b.volume << "\n";}void setbox(box * pb){pb->volume = pb->height * pb->width * pb->length;}// pe7-4.cpp -- probability of winning#include <iostream>long double probability(unsigned numbers, unsigned picks);int main(){using namespace std;double total, choices;double mtotal;double probability1, probability2;cout << "Enter total number of game card choices and\n""number of picks allowed for the field:\n";while ((cin >> total >> choices) && choices <= total){cout << "Enter total number of game card choices ""for the mega number:\n";if (!(cin >> mtotal))break;cout << "The chances of getting all " << choices << " picks is one in "<< (probability1 = probability(total, choices) ) << ".\n";cout << "The chances of getting the megaspot is one in "<< (probability2 = probability(mtotal, 1) ) << ".\n";cout << "You have one chance in ";cout << probability1 * probability2; // compute the probabilitycout << " of winning.\n";cout << "Next set of numbers (q to quit): ";}cout << "bye\n";return 0;}// the following function calculates the probability of picking picks// numbers correctly from numbers choiceslong double probability(unsigned numbers, unsigned picks){long double result = 1.0; // here come some local variableslong double n;unsigned p;for (n = numbers, p = picks; p > 0; n--, p--)result = result * n / p ;return result;// pe7-6.cpp#include <iostream>int Fill_array(double ar[], int size);void Show_array(const double ar[], int size);void Reverse_array(double ar[], int size);const int LIMIT = 10;int main( ){using namespace std;double values[LIMIT];int entries = Fill_array(values, LIMIT);cout << "Array values:\n";Show_array(values, entries);cout << "Array reversed:\n";Reverse_array(values, entries);Show_array(values, entries);cout << "All but end values reversed:\n";Reverse_array(values + 1, entries - 2);Show_array(values, entries);return 0;}int Fill_array(double ar[], int size){using namespace std;int n;cout << "Enter up to " << size << " values (q to quit):\n";for (n = 0; n < size; n++){cin >> ar[n];if (!cin)break;}return n;}void Show_array(const double ar[], int size){using namespace std;int n;for (n = 0; n < size; n++){cout << ar[n];if (n % 8 == 7)cout << endl;elsecout << ' ';}if (n % 8 != 0)cout << endl;}void Reverse_array(double ar[], int size){int i, j;double temp;for (i = 0, j = size - 1; i < j; i++, j--){temp = ar[i];ar[i] = ar[j];ar[j] = temp;}}//pe7-9.cpp#include <iostream>double calculate(double x, double y, double (*pf)(double, double)); double add(double x, double y);double sub(double x, double y);double mean(double x, double y);int main(void){using namespace std;double (*pf[3])(double,double) = {add, sub, mean};char * op[3] = {"sum", "difference", "mean"};double a, b;cout << "Enter pairs of numbers (q to quit): ";int i;while (cin >> a >> b){// using function namescout << calculate(a, b, add) << " = sum\n";cout << calculate(a, b, mean) << " = mean\n";// using pointersfor (i = 0; i < 3; i++)cout << calculate(a, b, pf[i]) << " = "<< op[i] << "\n";}cout << "Done!\n";return 0;}double calculate(double x, double y, double (*pf)(double, double)) {return (*pf)(x, y);}double add(double x, double y){return x + y;}double sub(double x, double y){return x - y;}double mean(double x, double y){return (x + y) / 2.0;}Chapter 8// pe8-1.cpp#include <iostream>void silly(const char * s, int n = 0);int main(void){using namespace std;char * p1 = "Why me?\n";silly(p1);for (int i = 0; i < 3; i++){cout << i << " = i\n";silly(p1, i);}cout << "Done\n";return 0;}void silly(const char * s, int n){using namespace std;static int uses = 0;int lim = ++uses;if (n == 0)lim = 1;for (int i = 0; i < lim; i++)cout << s;}// pe8-4.cpp#include <iostream>#include <cstring> // for strlen(), strcpy()using namespace std;struct stringy {char * str; // points to a stringint ct; // length of string (not counting '\0')};void show(const char *str, int cnt = 1);void show(const stringy & bny, int cnt = 1);void set(stringy & bny, const char * str);int main(void){stringy beany;char testing[] = "Reality isn't what it used to be.";set(beany, testing); // first argument is a reference, // allocates space to hold copy of testing,// sets str member of beany to point to the// new block, copies testing to new block,// and sets ct member of beanyshow(beany); // prints member string once show(beany, 2); // prints member string twicetesting[0] = 'D';testing[1] = 'u';show(testing); // prints testing string onceshow(testing, 3); // prints testing string thriceshow("Done!");return 0;}void show(const char *str, int cnt){while(cnt-- > 0){cout << str << endl;}}void show(const stringy & bny, int cnt){while(cnt-- > 0){cout << bny.str << endl;}}void set(stringy & bny, const char * str){bny.ct = strlen(str);bny.str = new char[bny.ct+1];strcpy(bny.str, str);}// pe8-5.cpp#include <iostream>template <class T>T max5(T ar[]){int n;T max = ar[0];for (n = 1; n < 5; n++)if (ar[n] > max)max = ar[n];return max;}const int LIMIT = 5;int main( ){using namespace std;double ard[LIMIT] = { -3.4, 8.1, -76.4, 34.4, 2.4};int ari[LIMIT] = {2, 3, 8, 1, 9};double md;int mi;md = max5(ard);mi = max5(ari);cout << "md = " << md << endl;cout << "mi = " << mi << endl;return 0;}Chapter 9PE 9-1// pe9-golf.h - for pe9-1.cppconst int Len = 40;struct golf{char fullname[Len];int handicap;};// non-interactive version// function sets golf structure to provided name, handicap // using values passed as arguments to the functionvoid setgolf(golf & g, const char * name, int hc);// interactive version// function solicits name and handicap from user// and sets the members of g to the values entered// returns 1 if name is entered, 0 if name is empty stringint setgolf(golf & g);// function resets handicap to new valuevoid handicap(golf & g, int hc);// function displays contents of golf structurevoid showgolf(const golf & g);// pe9-golf.cpp - for pe9-1.cpp#include <iostream>#include "pe9-golf.h"#include <cstring>// function solicits name and handicap from user// returns 1 if name is entered, 0 if name is empty stringint setgolf(golf & g){std::cout << "Please enter golfer's full name: ";std::cin.getline(g.fullname, Len);if (g.fullname[0] == '\0')return 0; // premature termination std::cout << "Please enter handicap for " << g.fullname << ": ";while (!(std::cin >> g.handicap)){std::cin.clear();std::cout << "Please enter an integer: ";}while (std::cin.get() != '\n')continue;return 1;}// function sets golf structure to provided name, handicapvoid setgolf(golf & g, const char * name, int hc){std::strcpy(g.fullname, name);g.handicap = hc;}// function resets handicap to new valuevoid handicap(golf & g, int hc){g.handicap = hc;}// function displays contents of golf structurevoid showgolf(const golf & g){std::cout << "Golfer: " << g.fullname << "\n";std::cout << "Handicap: " << g.handicap << "\n\n";}// pe9-1.cpp#include <iostream>#include "pe9-golf.h"// link with pe9-golf.cppconst int Mems = 5;int main(void){using namespace std;golf team[Mems];cout << "Enter up to " << Mems << " golf team members:\n";int i;for (i = 0; i < Mems; i++)if (setgolf(team[i]) == 0)break;for (int j = 0; j < i; j++)showgolf(team[j]);setgolf(team[0], "Fred Norman", 5);showgolf(team[0]);handicap(team[0], 3);showgolf(team[0]);return 0;}PE 9-3//pe9-3.cpp -- using placement new#include <iostream>#include <new>#include <cstring>struct chaff{char dross[20];int slag;};// char buffer[500]; // option 1int main(){using std::cout;using std::endl;chaff *p1;int i;char * buffer = new char [500]; // option 2p1 = new (buffer) chaff[2]; // place structures in buffer std::strcpy(p1[0].dross, "Horse Feathers");p1[0].slag = 13;std::strcpy(p1[1].dross, "Piffle");p1[1].slag = -39;for (i = 0; i < 2; i++)cout << p1[i].dross << ": " << p1[i].slag << endl;delete [] buffer; // option 2return 0;}Chapter 10PE 10-1// pe10-1.cpp#include <iostream>#include <cstring>// class declarationclass BankAccount{private:char name[40];char acctnum[25];double balance;public:BankAccount(char * client = "no one", char * num = "0",double bal = 0.0); void show(void) const;void deposit(double cash); void withdraw(double cash); };// method definitionsBankAccount::BankAccount(char * client, char * num, double bal) {std::strncpy(name, client, 39);name[39] = '\0';std::strncpy(acctnum, num, 24);acctnum[24] = '\0';balance = bal;}void BankAccount::show(void) const{using std::cout;using std:: endl;cout << "Client: " << name << endl;cout << "Account Number: " << acctnum << endl;cout << "Balance: " << balance << endl;}void BankAccount::deposit(double cash){if (cash >= 0)balance += cash;elsestd::cout << "Illegal transaction attempted";}void BankAccount::withdraw(double cash){if (cash < 0)std::cout << "Illegal transaction attempted";else if (cash <= balance)balance -=cash;elsestd::cout << "Request denied due to insufficient funds.\n"; }// sample useint main(){BankAccount bird;BankAccount frog("Kermit", "croak322", 123.00);bird.show();frog.show();bird = BankAccount("Chipper", "peep8282", 214.00);bird.show();frog.deposit(20);frog.show();frog.withdraw(4000);frog.show();frog.withdraw(50);frog.show();}PE10-4// pe10-4.h#ifndef SALES__#define SALES__namespace SALES{const int QUARTERS = 4;class Sales{private:double sales[QUARTERS];double average;double max;double min;public:// default constructorSales();// copies the lesser of 4 or n items from the array ar// to the sales member and computes and stores the// average, maximum, and minimum values of the entered items;// remaining elements of sales, if any, set to 0Sales(const double ar[], int n);// gathers sales for 4 quarters interactively, stores them// in the sales member of object and computes and stores the // average, maximum, and minumum valuesvoid setSales();// display all information in objectvoid showSales();};}#endif// pe10-4a.cpp#include <iostream>#include "pe10-4.h"int main(){using SALES::Sales;double vals[3] = {2000, 3000, 5000};Sales forFiji(vals, 3);forFiji.showSales();Sales red;red.showSales();red.setSales();red.showSales();return 0;}// pe10-4b.cpp#include <iostream>#include "pe10-4.h"namespace SALES{using std::cin;using std::cout;using std::endl;。
C+Primer+Plus+5+answer

Chapter 2PE 2-1/* Programming Exercise 2-1 */#include <stdio.h>int main(void){printf("Anton Bruckner\n");printf("Anton\nBruckner\n");printf("Anton ");printf("Bruckner\n");return 0;}PE 2-3/* Programming Exercise 2-3 */#include <stdio.h>int main(void){int ageyears; /* age in years */int agedays; /* age in days *//* large ages may require the long type */ageyears = 44;agedays = 365 * ageyears;printf("An age of %d years is %d days.\n", ageyears, agedays); return 0;}PE 2-4/* Programming Exercise 2-4 */#include <stdio.h>void jolly(void);void deny(void);int main(void){jolly();jolly();jolly();deny();return 0;}void jolly(void){printf("For he's a jolly good fellow!\n");}void deny(void){printf("Which nobody can deny!\n");}PE 2-5/* Programming Exercise 2-5 */#include <stdio.h>int main(void){int toes;toes = 10;printf("toes = %d\n", toes);printf("Twice toes = %d\n", 2 * toes);printf("toes squared = %d\n", toes * toes);return 0;}/* or create two more variables, set them to 2 * toes and toes * toes */PE 2-7/* Programming Exercise 2-7 */#include <stdio.h>void one_three(void);void two(void);int main(void){printf("starting now:\n");one_three();printf("done!\n");return 0;}void one_three(void){printf("one\n");two();printf("three\n");}void two(void){printf("two\n");}Chapter 3PE 3-2/* Programming Exercise 3-2 */#include <stdio.h>int main(void){int ascii;printf("Enter an ASCII code: ");scanf("%d", &ascii);printf("%d is the ASCII code for %c.\n", ascii, ascii);return 0;}PE 3-4/* Programming Exercise 3-4 */#include <stdio.h>int main(void){float num;printf("Enter a floating-point value: ");scanf("%f", &num);printf("fixed-point notation: %f\n", num);printf("exponential notation: %e\n", num);return 0;}PE 3-6/* Programming Exercise 3-6 */#include <stdio.h>int main(void){float mass_mol = 3.0e-23; /* mass of water molecule in grams */ float mass_qt = 950; /* mass of quart of water in grams */ float quarts;float molecules;printf("Enter the number of quarts of water: ");scanf("%f", &quarts);molecules = quarts * mass_qt / mass_mol;printf("%f quarts of water contain %e molecules.\n", quarts,molecules);return 0;}Chapter 4PE 4-1/* Programming Exercise 4-1 */#include <stdio.h>int main(void){char fname[40];char lname[40];printf("Enter your first name: ");scanf("%s", fname);printf("Enter your last name: ");scanf("%s", lname);printf("%s, %s\n", lname, fname);return 0;}PE 4-4/* Programming Exercise 4-4 */#include <stdio.h>int main(void){float height;char name[40];printf("Enter your height in inches: ");scanf("%f", &height);printf("Enter your name: ");scanf("%s", name);printf("%s, you are %.3f feet tall\n", name, height / 12.0); return 0;}PE 4-6/* Programming Exercise 4-6 */#include <stdio.h>#include <float.h>int main(void){float ot_f = 1.0 / 3.0;double ot_d = 1.0 / 3.0;printf(" float values: ");printf("%.4f %.12f %.16f\n", ot_f, ot_f, ot_f);printf("double values: ");printf("%.4f %.12f %.16f\n", ot_d, ot_d, ot_d);printf("FLT_DIG: %d\n", FLT_DIG);printf("DBL_DIG: %d\n", DBL_DIG);return 0;}Chapter 5PE 5-1/* Programming Exercise 5-1 */#include <stdio.h>int main(void){const int minperhour = 60;int minutes, hours, mins;printf("Enter the number of minutes to convert: ");scanf("%d", &minutes);while (minutes > 0 ){hours = minutes / minperhour;mins = minutes % minperhour;printf("%d minutes = %d hours, %d minutes\n", minutes, hours, mins); printf("Enter next minutes value (0 to quit): ");scanf("%d", &minutes);}printf("Bye\n");return 0;}PE 5-3/* Programming Exercise 5-3 */#include <stdio.h>int main(void){const int daysperweek = 7;int days, weeks, day_rem;printf("Enter the number of days: ");scanf("%d", &days);weeks = days / daysperweek;day_rem = days % daysperweek;printf("%d days are %d weeks and %d days.\n", days, weeks, day_rem);return 0;}PE 5-5/* Programming Exercise 5-5 */#include <stdio.h>int main(void) /* finds sum of first n integers */ {int count, sum;int n;printf("Enter the upper limit: ");scanf("%d", &n);count = 0;sum = 0;while (count++ < n)sum = sum + count;printf("sum = %d\n", sum);return 0;}PE 5-7/* Programming Exercise 5-7 */#include <stdio.h>void showCube(double x);int main(void) /* finds cube of entered number */{double val;printf("Enter a floating-point value: ");scanf("%lf", &val);showCube(val);return 0;}void showCube(double x){printf("The cube of %e is %e.\n", x, x*x*x );}Chapter 6PE 6-1/* pe6-1.c *//* this implementation assumes the character codes *//* are sequential, as they are in ASCII. */#include <stdio.h>#define SIZE 26int main( void ){char lcase[SIZE];int i;for (i = 0; i < SIZE; i++)lcase[i] = 'a' + i;for (i = 0; i < SIZE; i++)printf("%c", lcase[i]);printf("\n");return 0;}PE 6-3/* pe6-3.c *//* this implementation assumes the character codes *//* are sequential, as they are in ASCII. */#include <stdio.h>int main( void ){char let = 'F';char start;char end;for (end = let; end >= 'A'; end--){for (start = let; start >= end; start--)printf("%c", start);printf("\n");}return 0;}PE 6-5/* pe6-5.c */#include <stdio.h>int main( void ){int lower, upper, index;int square, cube;printf("Enter starting integer: ");scanf("%d", &lower);printf("Enter ending integer: ");scanf("%d", &upper);printf("%5s %10s %15s\n", "num", "square", "cube"); for (index = lower; index <= upper; index++){square = index * index;cube = index * square;printf("%5d %10d %15d\n", index, square, cube); }return 0;}PE 6-7/* pe6-7.c */#include <stdio.h>int main( void ){double n, m;double res;printf("Enter a pair of numbers: ");while (scanf("%lf %lf", &n, &m) == 2){res = (n - m) / (n * m);printf("(%.3g - %.3g)/(%.3g*%.3g) = %.5g\n", n, m, n, m, res); printf("Enter next pair (non-numeric to quit): ");}return 0;}PE 6-10/* pe6-10.c */#include <stdio.h>#define SIZE 8int main( void ){int vals[SIZE];int i;printf("Please enter %d integers.\n", SIZE);for (i = 0; i < SIZE; i++)scanf("%d", &vals[i]);printf("Here, in reverse order, are the values you entered:\n");for (i = SIZE - 1; i > 0; i--)printf("%d ", vals[i]);printf("\n");return 0;}PE 6-12/* pe6-12.c *//* This version starts with the 0 power */#include <stdio.h>#define SIZE 8int main( void ){int twopows[SIZE];int i;int value = 1; /* 2 to the 0 */for (i = 0; i < SIZE; i++){twopows[i] = value;value *= 2;}i = 0;do{printf("%d ", twopows[i]);i++;} while (i < SIZE);printf("\n");return 0;}PE 6-13/* pe-13.c *//* Programming Exercise 6-13 */#include <stdio.h>#define SIZE 8int main(void){double arr[SIZE];double arr_cumul[SIZE];int i;printf("Enter %d numbers:\n", SIZE);for (i = 0; i < SIZE; i++){printf("value #%d: ", i + 1);scanf("%lf", &arr[i]);/* or scanf("%lf", arr + i); */}arr_cumul[0] = arr[0]; /* set first element */ for (i = 1; i < SIZE; i++)arr_cumul[i] = arr_cumul[i-1] + arr[i];for (i = 0; i < SIZE; i++)printf("%8g ", arr[i]);printf("\n");for (i = 0; i < SIZE; i++)printf("%8g ", arr_cumul[i]);printf("\n");return 0;}PE 6-15/* pe6-15.c */#include <stdio.h>#define RATE_SIMP 0.10#define RATE_COMP 0.05#define INIT_AMT 100.0int main( void ){double daphne = INIT_AMT;double deidre = INIT_AMT;int years = 0;while (deidre <= daphne){daphne += RATE_SIMP * INIT_AMT;deidre += RATE_COMP * deidre;++years;}printf("Investment values after %d years:\n", years);printf("Daphne: $%.2f\n", daphne);printf("Deidre: $%.2f\n", deidre);return 0;}Chapter 7PE 7-1/* Programming Exercise 7-1 */#include <stdio.h>int main(void){char ch;int sp_ct = 0;int nl_ct = 0;int other = 0;while ((ch = getchar()) != '#'){if (ch == ' ')sp_ct++;else if (ch == '\n')nl_ct++;elseother++;}printf("spaces: %d, newlines: %d, others: %d\n", sp_ct, nl_ct, other); return 0;}PE 7-3/* Programming Exercise 7-3 */#include <stdio.h>int main(void){int n;double sumeven = 0.0;int ct_even = 0;double sumodd = 0.0;int ct_odd = 0;while (scanf("%d", &n) == 1 && n != 0){if (n % 2 == 1){sumodd += n;++ct_odd;}else{sumeven += n;++ct_even;}}printf("Number of evens: %d", ct_even);if (ct_even > 0)printf(" average: %g", sumeven / ct_even); putchar('\n');printf("Number of odds: %d", ct_odd);if (ct_odd > 0)printf(" average: %g", sumodd / ct_odd);putchar('\n');printf("\ndone\n");return 0;}PE 7-5/* Programming Exercise 7-5 */#include <stdio.h>int main(void){char ch;int ct1 = 0;int ct2 = 0;while ((ch = getchar()) != '#'){switch(ch){case '.' : putchar('!');++ct1;break;case '!' : putchar('!');putchar('!');++ct2;break;default : putchar(ch);}}printf("%d replacements of . with !\n", ct1);printf("%d replacements of ! with !!\n", ct2);return 0;}PE 7-7/* Programming Exercise 7-7 */#include <stdio.h>#define BASEPAY 10 /* $10 per hour */#define BASEHRS 40 /* hours at basepay */#define OVERTIME 1.5 /* 1.5 time */#define AMT1 300 /* 1st rate tier */#define AMT2 150 /* 2st rate tier */#define RATE1 0.15 /* rate for 1st tier */#define RATE2 0.20 /* rate for 2nd tier */#define RATE3 0.25 /* rate for 3rd tier */int main(void){double hours;double gross;double net;double taxes;printf("Enter the number of hours worked this week: ");scanf("%lf", &hours);if (hours <= BASEHRS)gross = hours * BASEPAY;elsegross = BASEHRS * BASEPAY + (hours - BASEHRS) * BASEPAY * OVERTIME; if (gross <= AMT1)taxes = gross * RATE1;else if (gross <= AMT1 + AMT2)taxes = AMT1 * RATE1 + (gross - AMT1) * RATE2;elsetaxes = AMT1 * RATE1 + AMT2 * RATE2 + (gross - AMT1 - AMT2) * RATE3; net = gross - taxes;printf("gross: $%.2f; taxes: $%.2f; net: $%.2f\n", gross, taxes, net);return 0;}PE 7-9/* Programmming Exercise 7-9 */#include <stdio.h>#define NO 0#define YES 1int main(void){long num; /* value to be checked */long div; /* potential divisors */long lim; /* limit to values */int prime;printf("Please enter limit to values to be checked; ");printf("Enter q to quit.\n");while (scanf("%ld", &lim) == 1 && lim > 0){for (num = 2; num <= lim; num++){for (div = 2, prime = YES; (div * div) <= num; div++)if (num % div == 0)prime = NO; /* number is not prime */if (prime == YES)printf("%ld is prime.\n", num);}printf("Please enter another limit; ");printf("Enter q to quit.\n");}return 0;}PE 7-11/* pe7-11.c *//* Programming Exercise 7-11 */#include <stdio.h>#include <ctype.h>int main(void){const double price_artichokes = 1.25;const double price_beets = 0.65;const double price_carrots = 0.89;const double DISCOUNT_RATE = 0.05;char ch;double lb_artichokes;double lb_beets;double lb_carrots;double lb_total;double cost_artichokes;double cost_beets;double cost_carrots;double cost_total;double final_total;double discount;double shipping;printf("Enter a to buy artichokes, b for beets, ");printf("c for carrots, q to quit: ");while ((ch = getchar()) != 'q' && ch != 'Q'){if (ch == '\n')continue;while (getchar() != '\n')continue;ch = tolower(ch);switch (ch){case 'a' : printf("Enter pounds of artichokes: "); scanf("%lf", &lb_artichokes);break;case 'b' : printf("Enter pounds of beets: ");scanf("%lf", &lb_beets);break;case 'c' : printf("Enter pounds of carrots: ");scanf("%lf", &lb_carrots);break;default : printf("%c is not a valid choice.\n");}printf("Enter a to buy artichokes, b for beets, ");printf("c for carrots, q to quit: ");}cost_artichokes = price_artichokes * lb_artichokes;cost_beets = price_beets * lb_beets;cost_carrots = price_carrots * lb_carrots;cost_total = cost_artichokes + cost_beets + cost_carrots;lb_total = lb_artichokes + lb_beets + lb_carrots;if (lb_total <= 0)shipping = 0.0;else if (lb_total < 5.0)shipping = 3.50;else if (lb_total < 20)shipping = 10.0;elseshipping = 8.0 + 0.1 * lb_total;if (cost_total > 100.0)discount = DISCOUNT_RATE * cost_total;elsediscount = 0.0;final_total = cost_total + shipping - discount;printf("Your order:\n");printf("%.2f lbs of artichokes at $%.2f per pound:$ %.2f\n",lb_artichokes, price_artichokes, cost_artichokes); printf("%.2f lbs of beets at $%.2f per pound: $%.2f\n",lb_beets, price_beets, cost_beets);printf("%.2f lbs of carrots at $%.2f per pound: $%.2f\n",lb_carrots, price_carrots, cost_carrots);printf("Total cost of vegetables: $%.2f\n", cost_total);if (cost_total > 100)printf("Volume discount: $%.2f\n", discount);printf("Shipping: $%.2f\n", shipping);printf("Total charges: $%.2f\n", final_total);return 0;}Chapter 8PE 8-1/* Programming Exercise 8-1 */#include <stdio.h>int main(void){int ch;int ct = 0;while ((ch = getchar()) != EOF)ct++;printf("%d characters read\n", ct);return 0;}PE 8-3/* Programming Exercise 8-3 *//* Using ctype.h eliminates need to assume ASCII coding */#include <stdio.h>#include <ctype.h>int main(void){int ch;int uct = 0;int lct = 0;while ((ch = getchar()) != EOF)if (isupper(ch))uct++;else if (islower(ch))lct++;printf("%d uppercase characters read\n", uct);printf("%d lowercase characters read\n", lct);return 0;}/*or you could useif (ch >= 'A' && ch <= 'Z')uct++;else if (ch >= 'a' && ch <= 'z')lct++;*/PE 8-5/* Programming Exercise 8-5 *//* binaryguess.c -- an improved number-guesser */#include <stdio.h>#include <ctype.h>int main(void){int high = 100;int low = 1;int guess = (high + low) / 2;char response;printf("Pick an integer from 1 to 100. I will try to guess ");printf("it.\nRespond with a y if my guess is right, with");printf("\na h if it is high, and with an l if it is low.\n");printf("Uh...is your number %d?\n", guess);while ((response = getchar()) != 'y') /* get response */{if (response == '\n')continue;if (response != 'h' && response != 'l'){printf("I don't understand that response. Please enter h for\n"); printf("high, l for low, or y for correct.\n");continue;}if (response == 'h')high = guess - 1;else if (response == 'l')low = guess + 1;guess = (high + low) / 2;printf("Well, then, is it %d?\n", guess);}printf("I knew I could do it!\n");return 0;}PE 8-7/* Programming Exercise 8-7 */#include <stdio.h>#include <ctype.h>#define BASEPAY1 8.75 /* $8.75 per hour */#define BASEPAY2 9.33 /* $9.33 per hour */#define BASEPAY3 10.00 /* $10.00 per hour */#define BASEPAY4 11.20 /* $11.20 per hour */#define BASEHRS 40 /* hours at basepay */#define OVERTIME 1.5 /* 1.5 time */#define AMT1 300 /* 1st rate tier */#define AMT2 150 /* 2st rate tier */#define RATE1 0.15 /* rate for 1st tier */#define RATE2 0.20 /* rate for 2nd tier */#define RATE3 0.25 /* rate for 3rd tier */int getfirst(void);void menu(void);int main(void){double hours;double gross;double net;double taxes;double pay;char response;menu();while ((response = getfirst()) != 'q'){if (response == '\n') /* skip over newlines */continue;response = tolower(response); /* accept A as a, etc. */switch (response){case 'a' : pay = BASEPAY1; break;case 'b' : pay = BASEPAY2; break;case 'c' : pay = BASEPAY3; break;case 'd' : pay = BASEPAY4; break;default : printf("Please enter a, b, c, d, or q.\n");menu();continue; /* go to beginning of loop */}printf("Enter the number of hours worked this week: ");scanf("%lf", &hours);if (hours <= BASEHRS)gross = hours * pay;elsegross = BASEHRS * pay + (hours - BASEHRS) * pay * OVERTIME; if (gross <= AMT1)taxes = gross * RATE1;else if (gross <= AMT1 + AMT2)taxes = AMT1 * RATE1 + (gross - AMT1) * RATE2;elsetaxes = AMT1 * RATE1 + AMT2 * RATE2 + (gross - AMT1 - AMT2) * RATE3;net = gross - taxes;printf("gross: $%.2f; taxes: $%.2f; net: $%.2f\n", gross, taxes, net);menu();}printf("Done.\n");return 0;}void menu(void){printf("********************************************************""*********\n");printf("Enter the number corresponding to the desired pay rate"" or action:\n");printf("a) $%4.2f/hr b) $%4.2f/hr\n", BASEPAY1,BASEPAY2);printf("c) $%5.2f/hr d) $%5.2f/hr\n", BASEPAY3,BASEPAY4);printf("q) quit\n");printf("********************************************************""*********\n");}int getfirst(void){int ch;ch = getchar();while (isspace(ch))ch = getchar();while (getchar() != '\n')continue;return ch;}Chapter 9PE 9-1/* Programming Exercise 9-1 */#include <stdio.h>double min(double a, double b);int main(void){double x, y;printf("Enter two numbers (q to quit): ");while (scanf("%lf %lf", &x, &y) == 2){printf("The smaller number is %f.\n", min(x,y));printf("Next two values (q to quit): ");}printf("Bye!\n");return 0;}double min(double a, double b){return a < b ? a : b;}/* alternative implementationdouble min(double a, double b){if (a < b)return a;elsereturn b;}*/PE 9-3/* Programming Exercise 9-3 */#include <stdio.h>void chLineRow(char ch, int c, int r);int main(void){char ch;int col, row;printf("Enter a character (# to quit): ");while ( (ch = getchar()) != '#'){if (ch == '\n')continue;printf("Enter number of columns and number of rows: "); if (scanf("%d %d", &col, &row) != 2)break;chLineRow(ch, col, row);printf("\nEnter next character (# to quit): ");}printf("Bye!\n");return 0;}void chLineRow(char ch, int c, int r){int col, row;for (row = 0; row < r ; row++){for (col = 0; col < c; col++)putchar(ch);putchar('\n');}return;}PE 9-5/* Programming Exercise 9-5 */#include <stdio.h>void larger_of(double *p1, double *p2);int main(void){double x, y;printf("Enter two numbers (q to quit): ");while (scanf("%lf %lf", &x, &y) == 2){larger_of(&x, &y);printf("The modified values are %f and %f.\n", x, y);printf("Next two values (q to quit): ");}printf("Bye!\n");return 0;}void larger_of(double *p1, double *p2){double temp = *p1 > *p2 ? *p1 : *p2;*p1= *p2 = temp;}PE 9-7/* Programming Exercise 9-7 */#include <stdio.h>double power(double a, int b); /* ANSI prototype */int main(void){double x, xpow;int n;printf("Enter a number and the integer power");printf(" to which\nthe number will be raised. Enter q");printf(" to quit.\n");while (scanf("%lf%d", &x, &n) == 2){xpow = power(x,n); /* function call */printf("%.3g to the power %d is %.5g\n", x, n, xpow);printf("Enter next pair of numbers or q to quit.\n");}printf("Hope you enjoyed this power trip -- bye!\n");return 0;}double power(double a, int b) /* function definition */{double pow = 1;int i;if (b == 0){if (a == 0)printf("0 to the 0 undefined; using 1 as the value\n"); pow = 1.0;}else if (a == 0)pow = 0.0;else if (b > 0)for(i = 1; i <= b; i++)pow *= a;else /* b < 0 */pow = 1.0 / power(a, - b);return pow; /* return the value of pow */}PE 9-9/* Programming Exercise 9-9 */#include <stdio.h>void to_base_n(int x, int base);int main(void){int number;int b;printf("Enter an integer (q to quit):\n");while (scanf("%d", &number) == 1){printf("Enter number base (2-10): ");scanf("%d", &b);printf("Base %d equivalent: ", b);to_base_n(number, b);putchar('\n');printf("Enter an integer (q to quit):\n");}return 0;}void to_base_n(int x, int base) /* recursive function */{int r;r = x % base;if (x >= 2)to_base_n(x / base, base);putchar('0' + r);return;}Chapter 10PE 10-1/* Programming Exercise 10-1 */#include <stdio.h>#define MONTHS 12 /* number of months in a year */#define YRS 5 /* number of years of data */int main(void){/* initializing rainfall data for 1990 - 1994 */const float rain[YRS][MONTHS] = {{10.2, 8.1, 6.8, 4.2, 2.1, 1.8, 0.2, 0.3, 1.1, 2.3, 6.1, 7.4},{9.2, 9.8, 4.4, 3.3, 2.2, 0.8, 0.4, 0.0, 0.6, 1.7, 4.3, 5.2},{6.6, 5.5, 3.8, 2.8, 1.6, 0.2, 0.0, 0.0, 0.0, 1.3, 2.6, 4.2},{4.3, 4.3, 4.3, 3.0, 2.0, 1.0, 0.2, 0.2, 0.4, 2.4, 3.5, 6.6},{8.5, 8.2, 1.2, 1.6, 2.4, 0.0, 5.2, 0.9, 0.3, 0.9, 1.4, 7.2}};int year, month;float subtot, total;printf(" YEAR RAINFALL (inches)\n");for (year = 0, total = 0; year < YRS; year++){ /* for each year, sum rainfall for each month */for (month = 0, subtot = 0; month < MONTHS; month++)subtot += *(*(rain + year) + month);printf("%5d %15.1f\n", 1990 + year, subtot);total += subtot; /* total for all years */}printf("\nThe yearly average is %.1f inches.\n\n", total/YRS);printf("MONTHLY AVERAGES:\n\n");printf(" Jan Feb Mar Apr May Jun Jul Aug Sep Oct ");printf(" Nov Dec\n");for (month = 0; month < MONTHS; month++){ /* for each month, sum rainfall over years */for (year = 0, subtot =0; year < YRS; year++)subtot += *(*(rain + year) + month);printf("%4.1f ", subtot/YRS);}printf("\n");return 0;}PE 10-3/* Programming Exercise 10-3 */#include <stdio.h>#define LEN 10int max_arr(const int ar[], int n);void show_arr(const int ar[], int n);int main(void){int orig[LEN] = {1,2,3,4,12,6,7,8,9,10};int max;show_arr(orig, LEN);max = max_arr(orig, LEN);printf("%d = largest value\n", max);return 0;}int max_arr(const int ar[], int n){int i;int max = ar[0];/* don't use 0 as initial max value -- fails if all array values are neg */for (i = 1; i < n; i++)if (max < ar[i])max = ar[i];return max;}void show_arr(const int ar[], int n){int i;for (i = 0; i < n; i++)printf("%d ", ar[i]);putchar('\n');}PE 10-5/* Programming Exercise 10-5 */#include <stdio.h>#define LEN 10float max_diff(const float ar[], int n);void show_arr(const float ar[], int n);int main(void){float orig[LEN] = {1.1,2,3,4,12,6,7,8,9,10}; float max;show_arr(orig, LEN);max = max_diff(orig, LEN);printf("%g = maximum difference\n", max);return 0;}float max_diff(const float ar[], int n){int i;float max = ar[0];float min = ar[0];for (i = 1; i < n; i++){if (max < ar[i])max = ar[i];else if (min > ar[i])min = ar[i];}return max - min;}void show_arr(const float ar[], int n){int i;for (i = 0; i < n; i++)printf("%g ", ar[i]);putchar('\n');}PE 10-7/* Programming Exercise 10-7 */#include <stdio.h>#define LEN1 7#define LEN2 3void copy_arr(int ar1[], const int ar2[], int n); void show_arr(const int ar[], int n);int main(void){int orig[LEN1] = {1,2,3,4,5,6,7};int copy[LEN2];show_arr(orig, LEN1);copy_arr(copy, orig + 2, LEN2);show_arr(copy, LEN2);return 0;}void copy_arr(int ar1[], const int ar2[], int n) {int i;for (i = 0; i < n; i++)ar1[i] = ar2[i];}void show_arr(const int ar[], int n){int i;for (i = 0; i < n; i++)printf("%d ", ar[i]);putchar('\n');}PE 10-10/* Programming Exercise 10-10 */#include <stdio.h>#define ROWS 3#define COLS 5void times2(int ar[][COLS], int r);void showarr2(int ar[][COLS], int r);int main(void){int stuff[ROWS][COLS] = { {1,2,3,4,5},{6,7,8,9,10},{11,12,13,14,15} };showarr2(stuff, ROWS);putchar('\n');times2(stuff, ROWS);showarr2(stuff, ROWS);return 0;}。
标准c程序设计第5版答案
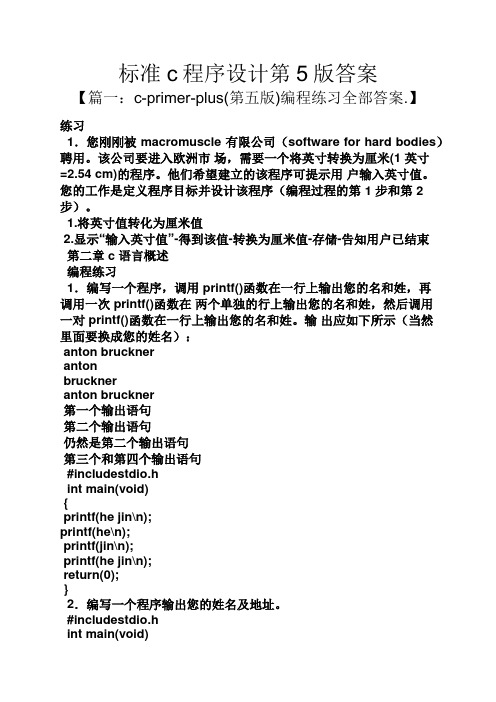
标准c程序设计第5版答案【篇一:c-primer-plus(第五版)编程练习全部答案.】练习1.您刚刚被 macromuscle 有限公司(software for hard bodies)聘用。
该公司要进入欧洲市场,需要一个将英寸转换为厘米(1 英寸=2.54 cm)的程序。
他们希望建立的该程序可提示用户输入英寸值。
您的工作是定义程序目标并设计该程序(编程过程的第 1 步和第 2 步)。
1.将英寸值转化为厘米值2.显示“输入英寸值”-得到该值-转换为厘米值-存储-告知用户已结束第二章 c 语言概述编程练习1.编写一个程序,调用 printf()函数在一行上输出您的名和姓,再调用一次 printf()函数在两个单独的行上输出您的名和姓,然后调用一对 printf()函数在一行上输出您的名和姓。
输出应如下所示(当然里面要换成您的姓名):anton brucknerantonbruckneranton bruckner第一个输出语句第二个输出语句仍然是第二个输出语句第三个和第四个输出语句#includestdio.hint main(void){printf(he jin\n);printf(he\n);printf(jin\n);printf(he jin\n);return(0);}2.编写一个程序输出您的姓名及地址。
#includestdio.hint main(void){printf(name:he jin\n);printf(address:cauc\n);return(0);}3.编写一个程序,把您的年龄转换成天数并显示二者的值。
不用考虑平年( fractional year) 和闰年(leapyear)的问题。
#includestdio.hint main(void){int age=22;printf(age:%d\n,age);printf(day:%d\n,age*356);return(0);}4.编写一个能够产生下面输出的程序:for hes a jolly good fellow!for hes a jolly good fellow!for hes a jolly good fellow!which nobody can deny!程序中除了 main()函数之外,要使用两个用户定义的函数:一个用于把上面的夸奖消息输出一次:另一个用于把最后一行输出一次。
C++Primer_Plus第五版习题答案

Solutions for Programming Exercises in C++ Primer Plus, 5th Edition Chapter 2// pe2-2.cpp#include <iostream>int main(void){using namespace std;cout << "Enter a distance in furlongs: ";double furlongs;cin >> furlongs;double feet;feet = 220 * furlongs;cout << furlongs << " furlongs = "<< feet << " feet\n";return 0;}// pe2-3.cpp#include <iostream>using namespace std;void mice();void run();int main(){mice();mice();run();run();return 0;}void mice(){cout << "Three blind mice\n";}void run(){cout << "See how they run\n";}// pe2-4.cpp#include <iostream>double C_to_F(double);int main(){using namespace std;cout << "Enter a temperature in Celsius: ";double C;cin >> C;double F;F = C_to_F(C);cout << C << " degrees Celsius = "<< F << " degrees Fahrenheit\n";return 0;}double C_to_F(double temp){return 1.8 * temp + 32.0;}Chapter 3// pe3-1.cpp#include <iostream>const int Inch_Per_Foot = 12;int main(void){using namespace std;// Note: some environments don't support the backspace charactercout << "Please enter your height in inches: ___/b/b/b ";int ht_inch;cin >> ht_inch;int ht_feet = ht_inch / Inch_Per_Foot;int rm_inch = ht_inch % Inch_Per_Foot;cout << "Your height is " << ht_feet << " feet, ";cout << rm_inch << " inch(es).\n";return 0;}// pe3-3.cpp#include <iostream>const double MINS_PER_DEG = 60.0;const double SECS_PER_MIN = 60.0;int main(){using namespace std;int degrees;int minutes;int seconds;double latitude;cout << "Enter a latitude in degrees, minutes, and seconds:\n";cout << "First, enter the degrees: ";cin >> degrees;cout << "Next, enter the minutes of arc: ";cin >> minutes;cout << "Finally, enter the seconds of arc: ";cin >> seconds;latitude = degrees + (minutes + seconds / SECS_PER_MIN)/MINS_PER_DEG;cout << degrees << " degrees, " << minutes << " minutes, "<< seconds << " seconds = " << latitude << " degrees\n";return 0;}// pe3-5.cpp#include <iostream>int main(void){using namespace std;cout << "How many miles have you driven your car? ";float miles;cin >> miles;cout << "How many gallons of gasoline did the car use? ";float gallons;cin >> gallons;cout << "Your car got " << miles / gallons;cout << " miles per gallon.\n";return 0;}// pe3-6.cpp#include <iostream>const double KM100_TO_MILES = 62.14;const double LITERS_PER_GALLON = 3.875;int main ( void ){using namespace std;double euro_rating;double us_rating;cout << "Enter fuel consumption in liters per 100 km: ";cin >> euro_rating;// divide by LITER_PER_GALLON to get gallons per 100-km// divide by KM100_TO_MILES to get gallons per mile// invert result to get miles per gallonus_rating = (LITERS_PER_GALLON * KM100_TO_MILES) / euro_rating;cout << euro_rating << " liters per 100 km is ";cout << us_rating << " miles per gallon.\n";return 0;}Chapter 4// pe4-2.cpp -- storing strings in string objects#include <iostream>#include <string>int main(){using namespace std;string name;string dessert;cout << "Enter your name:\n";getline(cin, name); // reads through newlinecout << "Enter your favorite dessert:\n";getline(cin, dessert);cout << "I have some delicious " << dessert;cout << " for you, " << name << ".\n";return 0;}// pe4-3.cpp -- storing strings in char arrays#include <iostream>#include <cstring>const int SIZE = 20;int main(){using namespace std;char firstName[SIZE];char lastName[SIZE];char fullName[2*SIZE + 1];cout << "Enter your first name: ";cin >> firstName;cout << "Enter your last name: ";cin >> lastName;strncpy(fullName,lastName,SIZE);strcat(fullName, ", ");strncat(fullName, firstName, SIZE);fullName[SIZE - 1] = '\0';cout << "Here's the information in a single string: "<< fullName << endl;return 0;}// pe4-5.cpp// a candybar structurestruct CandyBar {char brand[40];double weight;int calories;};#include <iostream>int main(){using namespace std; //introduces namespace stdCandyBar snack = { "Mocha Munch", 2.3, 350 };cout << "Brand name: " << snack.brand << endl;cout << "Weight: " << snack.weight << endl;cout << "Calories: " << snack.calories << endl;return 0;}// p#include <iostream>const int Slen = 70;struct pizza {char name[Slen];float diameter;float weight;};int main(void){using namespace std;pizza pie;cout << "What is the name of the pizza company? ";cin.getline(, Slen);cout << "What is the diameter of the pizza in inches? ";cin >> pie.diameter;cout << "How much does the pizza weigh in ounces? ";cin >> pie.weight;cout << "Company: " << << "\n";cout << "Diameter: " << pie.diameter << " inches\n";cout << "Weight: " << pie.weight << " ounces\n";return 0;}Chapter 5// pe5-2.cpp#include <iostream>int main(void){using namespace std;double sum = 0.0;double in;cout << "Enter a number (0 to terminate) : ";cin >> in;while (in != 0) {sum += in;cout << "Running total = " << sum << "\n";cout << "Enter next number (0 to terminate) : ";cin >> in;}cout << "Bye!\n";return 0;}// pe5-4.cpp// book sales#include <iostream>const int MONTHS = 12;const char * months[MONTHS] = {"January", "February", "March", "April","May", "June", "July", "August", "September","October", "November", "December"};int main(){using namespace std; //introduces namespace stdint sales[MONTHS];int month;cout << "Enter the monthly sales for \"C++ for Fools\":\n";for (month = 0; month < MONTHS; month++){cout << "Sales for " << months[month] << ": ";cin >> sales[month];}double total = 0.0;for (month = 0; month < MONTHS; month++)total += sales[month];cout << "Total sales: " << total << endl;return 0;}// pe5-6.cpp#include <iostream>struct car { char name[20]; int year;};int main(void){using namespace std;int n;cout << "How many cars do you wish to catalog?: ";cin >> n;while(cin.get() != '\n') // get rid of rest of line;car * pc = new car [n];int i;for (i = 0; i < n; i++){cout << "Car #" << (i + 1) << ":\n";cout << "Please enter the make: ";cin.getline(pc[i].name,20);cout << "Please enter the year made: ";cin >> pc[i].year;while(cin.get() != '\n') // get rid of rest of line;}cout << "Here is your collection:\n";for (i = 0; i < n; i++)cout << pc[i].year << " " << pc[i].name << "\n";delete [] pc;return 0;}// pe5-7.cpp -- count words using C-style string#include <iostream>#include <cstring> // prototype for strcmp()const int STR_LIM = 50;int main(){using namespace std;char word[STR_LIM];int count = 0;cout << "Enter words (to stop, type the word done):\n";while (cin >> word && strcmp("done", word))++count;cout << "You entered a total of " << count << " words.\n";return 0;}// pe5-9.cpp//nested loops#include <iostream>int main(){using namespace std; //introduces namespace stdint rows;int row;int col;int periods;cout << "Enter number of rows: ";cin >> rows;for (row = 1; row <= rows; row++){periods = rows - row;for (col = 1; col <= periods; col++)cout << '.';// col already has correct value for next loopfor ( ; col <= rows; col++)cout << '*';cout << endl;}return 0;}Chapter 6// pe6-1.cpp#include <iostream>#include <cctype>int main( ){using namespace std; //introduces namespace std char ch;cin.get(ch);while(ch != '@'){if (!isdigit(ch)){if (isupper(ch))ch = tolower(ch);else if (islower(ch))ch = toupper(ch);cout << ch;}cin.get(ch);}return 0;}// pe6-3.cpp#include <iostream>int main(void){using namespace std;cout << "Please enter one of the following choices:\n";cout << "c) carnivore p) pianist\n"<< "t) tree g) game\n";char ch;cin >> ch;while (ch != 'c' && ch != 'p' && ch != 't' && ch != 'g'){cout << "Please enter a c, p, t, or g: ";cin >> ch;}switch (ch){case 'c' : cout << "A cat is a carnivore.\n";break;case 'p' : cout << "Radu Lupu is a pianist.\n";break;case 't' : cout << "A maple is a tree.\n";break;case 'g' : cout << "Golf is a game.\n";break;default : cout << "The program shouldn't get here!\n";}return 0;}// pe6-5.cpp// Neutronia taxation#include <iostream>const double LEV1 = 5000;const double LEV2 = 15000;const double LEV3 = 35000;const double RATE1 = 0.10;const double RATE2 = 0.15;const double RATE3 = 0.20;int main( ){using namespace std;double income;double tax;cout << "Enter your annual income in tvarps: ";cin >> income;if (income <= LEV1)tax = 0;else if (income <= LEV2)tax = (income - LEV1) * RATE1;else if (income <= LEV3)tax = RATE1 * (LEV2 - LEV1) + RATE2 * (income - LEV2);elsetax = RATE1 * (LEV2 - LEV1) + RATE2 * (LEV3 - LEV2) + RATE3 * (income - LEV3);cout << "You owe Neutronia " << tax << " tvarps in taxes.\n";return 0;}// pe6-7.cpp#include <iostream>#include <string>int main(){using namespace std;string word;char ch;int vowel = 0;int consonant = 0;int other = 0;cout << "Enter words (q to quit):\n";cin >> word;while ( word != "q"){ch = tolower(word[0]);if (isalpha(ch)){if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o'|| ch == 'u')vowel++;elseconsonant++;}elseother++;cin >> word;}cout << vowel <<" words beginning with vowels\n";cout << consonant << " words beginning with consonants\n";cout << other << " others\n";return 0;}// pe6-8.cpp -- counting characters#include <iostream>#include <fstream> // file I/O suppport#include <cstdlib> // support for exit()const int SIZE = 60;int main(){using namespace std;char filename[SIZE];char ch;ifstream inFile; // object for handling file inputcout << "Enter name of data file: ";cin.getline(filename, SIZE);inFile.open(filename); // associate inFile with a fileif (!inFile.is_open()) // failed to open file{cout << "Could not open the file " << filename << endl;cout << "Program terminating.\n";exit(EXIT_FAILURE);}int count = 0; // number of items readinFile >> ch; // get first valuewhile (inFile.good()) // while input good and not at EOF {count++; // one more item readinFile >> ch; // get next value}cout << count << " characters in " << filename << endl;inFile.close(); // finished with the filereturn 0;}Chapter 7//pe7-1.cpp -- harmonic mean#include <iostream>double h_mean(double x, double y);int main(void){using namespace std;double x,y;cout << "Enter two numbers (a 0 terminates): ";while (cin >> x >> y && x * y != 0)cout << "harmonic mean of " << x << " and "<< y << " = " << h_mean(x,y) << "\n";/* or do the reading and testing in two parts:while (cin >> x && x != 0){cin >> y;if (y == 0)break;...*/cout << "Bye\n";return 0;}double h_mean(double x, double y){return 2.0 * x * y / (x + y);}// pe7-3.cpp#include <iostream>struct box {char maker[40];float height;float width;float length;float volume;};void showbox(box b);void setbox(box * pb);int main(void){box carton = {"Bingo Boxer", 2, 3, 5}; // no volume provided setbox(&carton);showbox(carton);return 0;}void showbox(box b){using namespace std;cout << "Box maker: " << b.maker<< "\nheight: " << b.height<< "\nlwidth: " << b.width<< "\nlength: " << b.length<< "\nvolume: " << b.volume << "\n";}void setbox(box * pb){pb->volume = pb->height * pb->width * pb->length;}// pe7-4.cpp -- probability of winning#include <iostream>long double probability(unsigned numbers, unsigned picks);int main(){using namespace std;double total, choices;double mtotal;double probability1, probability2;cout << "Enter total number of game card choices and\n""number of picks allowed for the field:\n";while ((cin >> total >> choices) && choices <= total){cout << "Enter total number of game card choices ""for the mega number:\n";if (!(cin >> mtotal))break;cout << "The chances of getting all " << choices << " picks is one in "<< (probability1 = probability(total, choices) ) << ".\n";cout << "The chances of getting the megaspot is one in "<< (probability2 = probability(mtotal, 1) ) << ".\n";cout << "You have one chance in ";cout << probability1 * probability2; // compute the probabilitycout << " of winning.\n";cout << "Next set of numbers (q to quit): ";}cout << "bye\n";return 0;}// the following function calculates the probability of picking picks// numbers correctly from numbers choiceslong double probability(unsigned numbers, unsigned picks){long double result = 1.0; // here come some local variableslong double n;unsigned p;for (n = numbers, p = picks; p > 0; n--, p--)result = result * n / p ;return result;// pe7-6.cpp#include <iostream>int Fill_array(double ar[], int size);void Show_array(const double ar[], int size);void Reverse_array(double ar[], int size);const int LIMIT = 10;int main( ){using namespace std;double values[LIMIT];int entries = Fill_array(values, LIMIT);cout << "Array values:\n";Show_array(values, entries);cout << "Array reversed:\n";Reverse_array(values, entries);Show_array(values, entries);cout << "All but end values reversed:\n";Reverse_array(values + 1, entries - 2);Show_array(values, entries);return 0;}int Fill_array(double ar[], int size){using namespace std;int n;cout << "Enter up to " << size << " values (q to quit):\n";for (n = 0; n < size; n++){cin >> ar[n];if (!cin)break;}return n;}void Show_array(const double ar[], int size){using namespace std;int n;for (n = 0; n < size; n++){cout << ar[n];if (n % 8 == 7)cout << endl;elsecout << ' ';}if (n % 8 != 0)cout << endl;}void Reverse_array(double ar[], int size){int i, j;double temp;for (i = 0, j = size - 1; i < j; i++, j--){temp = ar[i];ar[i] = ar[j];ar[j] = temp;}}//pe7-9.cpp#include <iostream>double calculate(double x, double y, double (*pf)(double, double)); double add(double x, double y);double sub(double x, double y);double mean(double x, double y);int main(void){using namespace std;double (*pf[3])(double,double) = {add, sub, mean};char * op[3] = {"sum", "difference", "mean"};double a, b;cout << "Enter pairs of numbers (q to quit): ";int i;while (cin >> a >> b){// using function namescout << calculate(a, b, add) << " = sum\n";cout << calculate(a, b, mean) << " = mean\n";// using pointersfor (i = 0; i < 3; i++)cout << calculate(a, b, pf[i]) << " = "<< op[i] << "\n";}cout << "Done!\n";return 0;}double calculate(double x, double y, double (*pf)(double, double)) {return (*pf)(x, y);}double add(double x, double y){return x + y;}double sub(double x, double y){return x - y;}double mean(double x, double y){return (x + y) / 2.0;}Chapter 8// pe8-1.cpp#include <iostream>void silly(const char * s, int n = 0);int main(void){using namespace std;char * p1 = "Why me?\n";silly(p1);for (int i = 0; i < 3; i++){cout << i << " = i\n";silly(p1, i);}cout << "Done\n";return 0;}void silly(const char * s, int n){using namespace std;static int uses = 0;int lim = ++uses;if (n == 0)lim = 1;for (int i = 0; i < lim; i++)cout << s;}// pe8-4.cpp#include <iostream>#include <cstring> // for strlen(), strcpy()using namespace std;struct stringy {char * str; // points to a stringint ct; // length of string (not counting '\0')};void show(const char *str, int cnt = 1);void show(const stringy & bny, int cnt = 1);void set(stringy & bny, const char * str);int main(void){stringy beany;char testing[] = "Reality isn't what it used to be.";set(beany, testing); // first argument is a reference, // allocates space to hold copy of testing,// sets str member of beany to point to the// new block, copies testing to new block,// and sets ct member of beanyshow(beany); // prints member string once show(beany, 2); // prints member string twicetesting[0] = 'D';testing[1] = 'u';show(testing); // prints testing string onceshow(testing, 3); // prints testing string thriceshow("Done!");return 0;}void show(const char *str, int cnt){while(cnt-- > 0){cout << str << endl;}}void show(const stringy & bny, int cnt){while(cnt-- > 0){cout << bny.str << endl;}}void set(stringy & bny, const char * str){bny.ct = strlen(str);bny.str = new char[bny.ct+1];strcpy(bny.str, str);}// pe8-5.cpp#include <iostream>template <class T>T max5(T ar[]){int n;T max = ar[0];for (n = 1; n < 5; n++)if (ar[n] > max)max = ar[n];return max;}const int LIMIT = 5;int main( ){using namespace std;double ard[LIMIT] = { -3.4, 8.1, -76.4, 34.4, 2.4};int ari[LIMIT] = {2, 3, 8, 1, 9};double md;int mi;md = max5(ard);mi = max5(ari);cout << "md = " << md << endl;cout << "mi = " << mi << endl;return 0;}Chapter 9PE 9-1// pe9-golf.h - for pe9-1.cppconst int Len = 40;struct golf{char fullname[Len];int handicap;};// non-interactive version// function sets golf structure to provided name, handicap // using values passed as arguments to the functionvoid setgolf(golf & g, const char * name, int hc);// interactive version// function solicits name and handicap from user// and sets the members of g to the values entered// returns 1 if name is entered, 0 if name is empty stringint setgolf(golf & g);// function resets handicap to new valuevoid handicap(golf & g, int hc);// function displays contents of golf structurevoid showgolf(const golf & g);// pe9-golf.cpp - for pe9-1.cpp#include <iostream>#include "pe9-golf.h"#include <cstring>// function solicits name and handicap from user// returns 1 if name is entered, 0 if name is empty stringint setgolf(golf & g){std::cout << "Please enter golfer's full name: ";std::cin.getline(g.fullname, Len);if (g.fullname[0] == '\0')return 0; // premature termination std::cout << "Please enter handicap for " << g.fullname << ": ";while (!(std::cin >> g.handicap)){std::cin.clear();std::cout << "Please enter an integer: ";}while (std::cin.get() != '\n')continue;return 1;}// function sets golf structure to provided name, handicapvoid setgolf(golf & g, const char * name, int hc){std::strcpy(g.fullname, name);g.handicap = hc;}// function resets handicap to new valuevoid handicap(golf & g, int hc){g.handicap = hc;}// function displays contents of golf structurevoid showgolf(const golf & g){std::cout << "Golfer: " << g.fullname << "\n";std::cout << "Handicap: " << g.handicap << "\n\n";}// pe9-1.cpp#include <iostream>#include "pe9-golf.h"// link with pe9-golf.cppconst int Mems = 5;int main(void){using namespace std;golf team[Mems];cout << "Enter up to " << Mems << " golf team members:\n";int i;for (i = 0; i < Mems; i++)if (setgolf(team[i]) == 0)break;for (int j = 0; j < i; j++)showgolf(team[j]);setgolf(team[0], "Fred Norman", 5);showgolf(team[0]);handicap(team[0], 3);showgolf(team[0]);return 0;}PE 9-3//pe9-3.cpp -- using placement new#include <iostream>#include <new>#include <cstring>struct chaff{char dross[20];int slag;};// char buffer[500]; // option 1int main(){using std::cout;using std::endl;chaff *p1;int i;char * buffer = new char [500]; // option 2p1 = new (buffer) chaff[2]; // place structures in buffer std::strcpy(p1[0].dross, "Horse Feathers");p1[0].slag = 13;std::strcpy(p1[1].dross, "Piffle");p1[1].slag = -39;for (i = 0; i < 2; i++)cout << p1[i].dross << ": " << p1[i].slag << endl;delete [] buffer; // option 2return 0;}Chapter 10PE 10-1// pe10-1.cpp#include <iostream>#include <cstring>// class declarationclass BankAccount{private:char name[40];char acctnum[25];double balance;public:BankAccount(char * client = "no one", char * num = "0",double bal = 0.0); void show(void) const;void deposit(double cash); void withdraw(double cash); };// method definitionsBankAccount::BankAccount(char * client, char * num, double bal) {std::strncpy(name, client, 39);name[39] = '\0';std::strncpy(acctnum, num, 24);acctnum[24] = '\0';balance = bal;}void BankAccount::show(void) const{using std::cout;using std:: endl;cout << "Client: " << name << endl;cout << "Account Number: " << acctnum << endl;cout << "Balance: " << balance << endl;}void BankAccount::deposit(double cash){if (cash >= 0)balance += cash;elsestd::cout << "Illegal transaction attempted";}void BankAccount::withdraw(double cash){if (cash < 0)std::cout << "Illegal transaction attempted";else if (cash <= balance)balance -=cash;elsestd::cout << "Request denied due to insufficient funds.\n"; }// sample useint main(){BankAccount bird;BankAccount frog("Kermit", "croak322", 123.00);bird.show();frog.show();bird = BankAccount("Chipper", "peep8282", 214.00);bird.show();frog.deposit(20);frog.show();frog.withdraw(4000);frog.show();frog.withdraw(50);frog.show();}PE10-4// pe10-4.h#ifndef SALES__#define SALES__namespace SALES{const int QUARTERS = 4;class Sales{private:double sales[QUARTERS];double average;double max;double min;public:// default constructorSales();// copies the lesser of 4 or n items from the array ar// to the sales member and computes and stores the// average, maximum, and minimum values of the entered items;// remaining elements of sales, if any, set to 0Sales(const double ar[], int n);// gathers sales for 4 quarters interactively, stores them// in the sales member of object and computes and stores the // average, maximum, and minumum valuesvoid setSales();// display all information in objectvoid showSales();};}#endif// pe10-4a.cpp#include <iostream>#include "pe10-4.h"int main(){using SALES::Sales;double vals[3] = {2000, 3000, 5000};Sales forFiji(vals, 3);forFiji.showSales();Sales red;red.showSales();red.setSales();red.showSales();return 0;}// pe10-4b.cpp#include <iostream>#include "pe10-4.h"namespace SALES{using std::cin;using std::cout;using std::endl;。
C++_Primer_Plus(第五版)习题解答
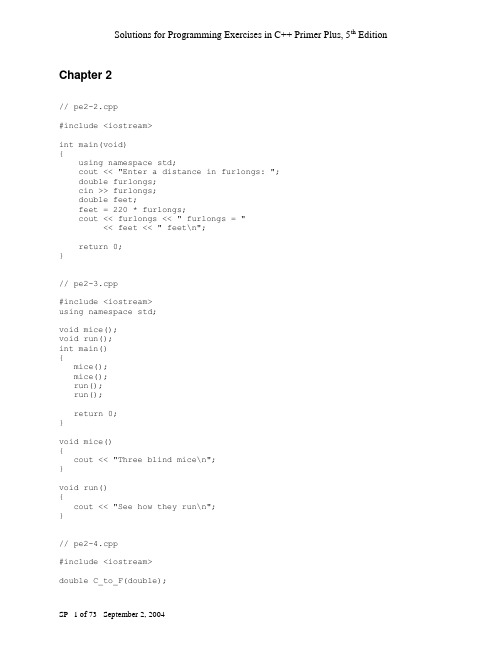
Chapter 2// pe2-2.cpp#include <iostream>int main(void){using namespace std;cout << "Enter a distance in furlongs: ";double furlongs;cin >> furlongs;double feet;feet = 220 * furlongs;cout << furlongs << " furlongs = "<< feet << " feet\n";return 0;}// pe2-3.cpp#include <iostream>using namespace std;void mice();void run();int main(){mice();mice();run();run();return 0;}void mice(){cout << "Three blind mice\n";}void run(){cout << "See how they run\n";}// pe2-4.cpp#include <iostream>double C_to_F(double);int main(){using namespace std;cout << "Enter a temperature in Celsius: "; double C;cin >> C;double F;F = C_to_F(C);cout << C << " degrees Celsius = "<< F << " degrees Fahrenheit\n";return 0;}double C_to_F(double temp){return 1.8 * temp + 32.0;}Chapter 3// pe3-1.cpp#include <iostream>const int Inch_Per_Foot = 12;int main(void){using namespace std;// Note: some environments don't support the backspace charactercout << "Please enter your height in inches: ___/b/b/b ";int ht_inch;cin >> ht_inch;int ht_feet = ht_inch / Inch_Per_Foot;int rm_inch = ht_inch % Inch_Per_Foot;cout << "Your height is " << ht_feet << " feet, ";cout << rm_inch << " inch(es).\n";return 0;}// pe3-3.cpp#include <iostream>const double MINS_PER_DEG = 60.0;const double SECS_PER_MIN = 60.0;int main(){using namespace std;int degrees;int minutes;int seconds;double latitude;cout << "Enter a latitude in degrees, minutes, and seconds:\n";cout << "First, enter the degrees: ";cin >> degrees;cout << "Next, enter the minutes of arc: ";cin >> minutes;cout << "Finally, enter the seconds of arc: ";cin >> seconds;latitude = degrees + (minutes + seconds / SECS_PER_MIN)/MINS_PER_DEG; cout << degrees << " degrees, " << minutes << " minutes, "<< seconds << " seconds = " << latitude << " degrees\n";return 0;}// pe3-5.cpp#include <iostream>int main(void){using namespace std;cout << "How many miles have you driven your car? ";float miles;cin >> miles;cout << "How many gallons of gasoline did the car use? ";float gallons;cin >> gallons;cout << "Your car got " << miles / gallons;cout << " miles per gallon.\n";return 0;}// pe3-6.cpp#include <iostream>const double KM100_TO_MILES = 62.14;const double LITERS_PER_GALLON = 3.875;int main ( void ){using namespace std;double euro_rating;double us_rating;cout << "Enter fuel consumption in liters per 100 km: ";cin >> euro_rating;// divide by LITER_PER_GALLON to get gallons per 100-km// divide by KM100_TO_MILES to get gallons per mile// invert result to get miles per gallonus_rating = (LITERS_PER_GALLON * KM100_TO_MILES) / euro_rating; cout << euro_rating << " liters per 100 km is ";cout << us_rating << " miles per gallon.\n";return 0;}Chapter 4// pe4-2.cpp -- storing strings in string objects#include <iostream>#include <string>int main(){using namespace std;string name;string dessert;cout << "Enter your name:\n";getline(cin, name); // reads through newlinecout << "Enter your favorite dessert:\n";getline(cin, dessert);cout << "I have some delicious " << dessert;cout << " for you, " << name << ".\n";return 0;}// pe4-3.cpp -- storing strings in char arrays#include <iostream>#include <cstring>const int SIZE = 20;int main(){using namespace std;char firstName[SIZE];char lastName[SIZE];char fullName[2*SIZE + 1];cout << "Enter your first name: ";cin >> firstName;cout << "Enter your last name: ";cin >> lastName;strncpy(fullName,lastName,SIZE);strcat(fullName, ", ");strncat(fullName, firstName, SIZE);fullName[SIZE - 1] = '\0';cout << "Here's the information in a single string: " << fullName << endl;return 0;}// pe4-5.cpp// a candybar structurestruct CandyBar {char brand[40];double weight;int calories;};#include <iostream>int main(){using namespace std; //introduces namespace stdCandyBar snack = { "Mocha Munch", 2.3, 350 };cout << "Brand name: " << snack.brand << endl;cout << "Weight: " << snack.weight << endl;cout << "Calories: " << snack.calories << endl;return 0;}// p#include <iostream>const int Slen = 70;struct pizza {char name[Slen];float diameter;float weight;};int main(void)using namespace std;pizza pie;cout << "What is the name of the pizza company? ";cin.getline(, Slen);cout << "What is the diameter of the pizza in inches? ";cin >> pie.diameter;cout << "How much does the pizza weigh in ounces? ";cin >> pie.weight;cout << "Company: " << << "\n";cout << "Diameter: " << pie.diameter << " inches\n";cout << "Weight: " << pie.weight << " ounces\n";return 0;}Chapter 5// pe5-2.cpp#include <iostream>int main(void){using namespace std;double sum = 0.0;double in;cout << "Enter a number (0 to terminate) : ";cin >> in;while (in != 0) {sum += in;cout << "Running total = " << sum << "\n";cout << "Enter next number (0 to terminate) : ";cin >> in;}cout << "Bye!\n";return 0;}// pe5-4.cpp// book sales#include <iostream>const int MONTHS = 12;const char * months[MONTHS] = {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"};int main(){using namespace std; //introduces namespace stdint sales[MONTHS];int month;cout << "Enter the monthly sales for \"C++ for Fools\":\n";for (month = 0; month < MONTHS; month++){cout << "Sales for " << months[month] << ": ";cin >> sales[month];}double total = 0.0;for (month = 0; month < MONTHS; month++)total += sales[month];cout << "Total sales: " << total << endl;return 0;}// pe5-6.cpp#include <iostream>struct car { char name[20]; int year;};int main(void){using namespace std;int n;cout << "How many cars do you wish to catalog?: ";cin >> n;while(cin.get() != '\n') // get rid of rest of line;car * pc = new car [n];int i;for (i = 0; i < n; i++){cout << "Car #" << (i + 1) << ":\n";cout << "Please enter the make: ";cin.getline(pc[i].name,20);cout << "Please enter the year made: ";cin >> pc[i].year;while(cin.get() != '\n') // get rid of rest of line ;}cout << "Here is your collection:\n";for (i = 0; i < n; i++)cout << pc[i].year << " " << pc[i].name << "\n";delete [] pc;return 0;}// pe5-7.cpp -- count words using C-style string#include <iostream>#include <cstring> // prototype for strcmp()const int STR_LIM = 50;int main(){using namespace std;char word[STR_LIM];int count = 0;cout << "Enter words (to stop, type the word done):\n";while (cin >> word && strcmp("done", word))++count;cout << "You entered a total of " << count << " words.\n"; return 0;}// pe5-9.cpp//nested loops#include <iostream>int main(){using namespace std; //introduces namespace stdint rows;int row;int col;int periods;cout << "Enter number of rows: ";cin >> rows;for (row = 1; row <= rows; row++){periods = rows - row;for (col = 1; col <= periods; col++)cout << '.';// col already has correct value for next loopfor ( ; col <= rows; col++)cout << '*';cout << endl;}return 0;}Chapter 6// pe6-1.cpp#include <iostream>#include <cctype>int main( ){using namespace std; //introduces namespace stdchar ch;cin.get(ch);while(ch != '@'){if (!isdigit(ch)){if (isupper(ch))ch = tolower(ch);else if (islower(ch))ch = toupper(ch);cout << ch;}cin.get(ch);}return 0;}// pe6-3.cpp#include <iostream>int main(void){using namespace std;cout << "Please enter one of the following choices:\n";cout << "c) carnivore p) pianist\n"<< "t) tree g) game\n";char ch;cin >> ch;while (ch != 'c' && ch != 'p' && ch != 't' && ch != 'g'){cout << "Please enter a c, p, t, or g: ";cin >> ch;}switch (ch){case 'c' : cout << "A cat is a carnivore.\n";break;case 'p' : cout << "Radu Lupu is a pianist.\n";break;case 't' : cout << "A maple is a tree.\n";break;case 'g' : cout << "Golf is a game.\n";break;default : cout << "The program shouldn't get here!\n"; }return 0;}// pe6-5.cpp// Neutronia taxation#include <iostream>const double LEV1 = 5000;const double LEV2 = 15000;const double LEV3 = 35000;const double RATE1 = 0.10;const double RATE2 = 0.15;const double RATE3 = 0.20;int main( ){using namespace std;double income;double tax;cout << "Enter your annual income in tvarps: ";cin >> income;if (income <= LEV1)tax = 0;else if (income <= LEV2)tax = (income - LEV1) * RATE1;else if (income <= LEV3)tax = RATE1 * (LEV2 - LEV1) + RATE2 * (income - LEV2);elsetax = RATE1 * (LEV2 - LEV1) + RATE2 * (LEV3 - LEV2)+ RATE3 * (income - LEV3);cout << "You owe Neutronia " << tax << " tvarps in taxes.\n"; return 0;}// pe6-7.cpp#include <iostream>#include <string>int main(){using namespace std;string word;char ch;int vowel = 0;int consonant = 0;int other = 0;cout << "Enter words (q to quit):\n";cin >> word;while ( word != "q"){ch = tolower(word[0]);if (isalpha(ch)){if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u')vowel++;elseconsonant++;}elseother++;cin >> word;}cout << vowel <<" words beginning with vowels\n";cout << consonant << " words beginning with consonants\n";cout << other << " others\n";return 0;}// pe6-8.cpp -- counting characters#include <iostream>#include <fstream> // file I/O suppport#include <cstdlib> // support for exit()const int SIZE = 60;int main(){using namespace std;char filename[SIZE];char ch;ifstream inFile; // object for handling file inputcout << "Enter name of data file: ";cin.getline(filename, SIZE);inFile.open(filename); // associate inFile with a fileif (!inFile.is_open()) // failed to open file{cout << "Could not open the file " << filename << endl;cout << "Program terminating.\n";exit(EXIT_FAILURE);}int count = 0; // number of items readinFile >> ch; // get first valuewhile (inFile.good()) // while input good and not at EOF {count++; // one more item readinFile >> ch; // get next value}cout << count << " characters in " << filename << endl;inFile.close(); // finished with the filereturn 0;}Chapter 7//pe7-1.cpp -- harmonic mean#include <iostream>double h_mean(double x, double y);int main(void){using namespace std;double x,y;cout << "Enter two numbers (a 0 terminates): ";while (cin >> x >> y && x * y != 0)cout << "harmonic mean of " << x << " and "<< y << " = " << h_mean(x,y) << "\n";/* or do the reading and testing in two parts:while (cin >> x && x != 0){cin >> y;if (y == 0)break;...*/cout << "Bye\n";return 0;}double h_mean(double x, double y){return 2.0 * x * y / (x + y);}// pe7-3.cpp#include <iostream>struct box {char maker[40];float height;float width;float length;float volume;};void showbox(box b);void setbox(box * pb);int main(void){box carton = {"Bingo Boxer", 2, 3, 5}; // no volume providedsetbox(&carton);showbox(carton);return 0;}void showbox(box b){using namespace std;cout << "Box maker: " << b.maker<< "\nheight: " << b.height<< "\nlwidth: " << b.width<< "\nlength: " << b.length<< "\nvolume: " << b.volume << "\n";}void setbox(box * pb){pb->volume = pb->height * pb->width * pb->length;}// pe7-4.cpp -- probability of winning#include <iostream>long double probability(unsigned numbers, unsigned picks);int main(){using namespace std;double total, choices;double mtotal;double probability1, probability2;cout << "Enter total number of game card choices and\n""number of picks allowed for the field:\n";while ((cin >> total >> choices) && choices <= total){cout << "Enter total number of game card choices ""for the mega number:\n";if (!(cin >> mtotal))break;cout << "The chances of getting all " << choices << " picks is one in "<< (probability1 = probability(total, choices) ) << ".\n";cout << "The chances of getting the megaspot is one in "<< (probability2 = probability(mtotal, 1) ) << ".\n";cout << "You have one chance in ";cout << probability1 * probability2; // compute the probability cout << " of winning.\n";cout << "Next set of numbers (q to quit): ";}cout << "bye\n";return 0;}// the following function calculates the probability of picking picks// numbers correctly from numbers choiceslong double probability(unsigned numbers, unsigned picks){long double result = 1.0; // here come some local variablesunsigned p;for (n = numbers, p = picks; p > 0; n--, p--)result = result * n / p ;return result;}// pe7-6.cpp#include <iostream>int Fill_array(double ar[], int size);void Show_array(const double ar[], int size);void Reverse_array(double ar[], int size);const int LIMIT = 10;int main( ){using namespace std;double values[LIMIT];int entries = Fill_array(values, LIMIT);cout << "Array values:\n";Show_array(values, entries);cout << "Array reversed:\n";Reverse_array(values, entries);Show_array(values, entries);cout << "All but end values reversed:\n";Reverse_array(values + 1, entries - 2);Show_array(values, entries);return 0;}int Fill_array(double ar[], int size){using namespace std;int n;cout << "Enter up to " << size << " values (q to quit):\n"; for (n = 0; n < size; n++){cin >> ar[n];if (!cin)break;}return n;}void Show_array(const double ar[], int size){using namespace std;int n;for (n = 0; n < size; n++){cout << ar[n];if (n % 8 == 7)cout << endl;elsecout << ' ';}if (n % 8 != 0)cout << endl;}void Reverse_array(double ar[], int size){int i, j;for (i = 0, j = size - 1; i < j; i++, j--){temp = ar[i];ar[i] = ar[j];ar[j] = temp;}}//pe7-9.cpp#include <iostream>double calculate(double x, double y, double (*pf)(double, double)); double add(double x, double y);double sub(double x, double y);double mean(double x, double y);int main(void){using namespace std;double (*pf[3])(double,double) = {add, sub, mean};char * op[3] = {"sum", "difference", "mean"};double a, b;cout << "Enter pairs of numbers (q to quit): ";int i;while (cin >> a >> b){// using function namescout << calculate(a, b, add) << " = sum\n";cout << calculate(a, b, mean) << " = mean\n";// using pointersfor (i = 0; i < 3; i++)cout << calculate(a, b, pf[i]) << " = "<< op[i] << "\n";}cout << "Done!\n";return 0;}double calculate(double x, double y, double (*pf)(double, double)) {return (*pf)(x, y);}double add(double x, double y){return x + y;}double sub(double x, double y){return x - y;}double mean(double x, double y){return (x + y) / 2.0;}Chapter 8// pe8-1.cpp#include <iostream>void silly(const char * s, int n = 0);int main(void){using namespace std;char * p1 = "Why me?\n";silly(p1);for (int i = 0; i < 3; i++){cout << i << " = i\n";silly(p1, i);}cout << "Done\n";return 0;}void silly(const char * s, int n){using namespace std;static int uses = 0;int lim = ++uses;if (n == 0)lim = 1;for (int i = 0; i < lim; i++)cout << s;}// pe8-4.cpp#include <iostream>#include <cstring> // for strlen(), strcpy()using namespace std;struct stringy {char * str; // points to a stringint ct; // length of string (not counting '\0')};void show(const char *str, int cnt = 1);void show(const stringy & bny, int cnt = 1);void set(stringy & bny, const char * str);int main(void){stringy beany;char testing[] = "Reality isn't what it used to be.";set(beany, testing); // first argument is a reference, // allocates space to hold copy of testing,// sets str member of beany to point to the// new block, copies testing to new block,// and sets ct member of beanyshow(beany); // prints member string onceshow(beany, 2); // prints member string twicetesting[0] = 'D';testing[1] = 'u';show(testing); // prints testing string onceshow(testing, 3); // prints testing string thrice show("Done!");return 0;}void show(const char *str, int cnt){while(cnt-- > 0){cout << str << endl;}}void show(const stringy & bny, int cnt){while(cnt-- > 0){cout << bny.str << endl;}}void set(stringy & bny, const char * str){bny.ct = strlen(str);bny.str = new char[bny.ct+1];strcpy(bny.str, str);}// pe8-5.cpp#include <iostream>template <class T>T max5(T ar[]){int n;T max = ar[0];for (n = 1; n < 5; n++)if (ar[n] > max)max = ar[n];return max;}const int LIMIT = 5;int main( ){using namespace std;double ard[LIMIT] = { -3.4, 8.1, -76.4, 34.4, 2.4}; int ari[LIMIT] = {2, 3, 8, 1, 9};double md;int mi;md = max5(ard);mi = max5(ari);cout << "md = " << md << endl;cout << "mi = " << mi << endl;return 0;}Chapter 9PE 9-1// pe9-golf.h - for pe9-1.cppconst int Len = 40;struct golf{char fullname[Len];int handicap;};// non-interactive version// function sets golf structure to provided name, handicap// using values passed as arguments to the functionvoid setgolf(golf & g, const char * name, int hc);// interactive version// function solicits name and handicap from user// and sets the members of g to the values entered// returns 1 if name is entered, 0 if name is empty stringint setgolf(golf & g);// function resets handicap to new valuevoid handicap(golf & g, int hc);// function displays contents of golf structurevoid showgolf(const golf & g);// pe9-golf.cpp - for pe9-1.cpp#include <iostream>#include "pe9-golf.h"#include <cstring>// function solicits name and handicap from user// returns 1 if name is entered, 0 if name is empty stringint setgolf(golf & g){std::cout << "Please enter golfer's full name: ";std::cin.getline(g.fullname, Len);if (g.fullname[0] == '\0')return 0; // premature terminationstd::cout << "Please enter handicap for " << g.fullname << ": "; while (!(std::cin >> g.handicap)){std::cin.clear();std::cout << "Please enter an integer: ";}while (std::cin.get() != '\n')continue;return 1;}// function sets golf structure to provided name, handicapvoid setgolf(golf & g, const char * name, int hc){std::strcpy(g.fullname, name);g.handicap = hc;}// function resets handicap to new valuevoid handicap(golf & g, int hc){g.handicap = hc;}// function displays contents of golf structurevoid showgolf(const golf & g){std::cout << "Golfer: " << g.fullname << "\n";std::cout << "Handicap: " << g.handicap << "\n\n";}// pe9-1.cpp#include <iostream>#include "pe9-golf.h"// link with pe9-golf.cppconst int Mems = 5;int main(void){using namespace std;golf team[Mems];cout << "Enter up to " << Mems << " golf team members:\n";int i;for (i = 0; i < Mems; i++)if (setgolf(team[i]) == 0)break;for (int j = 0; j < i; j++)showgolf(team[j]);setgolf(team[0], "Fred Norman", 5);showgolf(team[0]);handicap(team[0], 3);showgolf(team[0]);return 0;}PE 9-3//pe9-3.cpp -- using placement new#include <iostream>#include <new>#include <cstring>struct chaff{char dross[20];int slag;};// char buffer[500]; // option 1int main(){using std::cout;using std::endl;chaff *p1;int i;char * buffer = new char [500]; // option 2p1 = new (buffer) chaff[2]; // place structures in buffer std::strcpy(p1[0].dross, "Horse Feathers");p1[0].slag = 13;std::strcpy(p1[1].dross, "Piffle");p1[1].slag = -39;for (i = 0; i < 2; i++)cout << p1[i].dross << ": " << p1[i].slag << endl;delete [] buffer; // option 2return 0;}Chapter 10PE 10-1// pe10-1.cpp#include <iostream>#include <cstring>// class declarationclass BankAccount{private:char name[40];char acctnum[25];double balance;public:BankAccount(char * client = "no one", char * num = "0",double bal = 0.0); void show(void) const; void deposit(double cash); void withdraw(double cash); };// method definitionsBankAccount::BankAccount(char * client, char * num, double bal) {std::strncpy(name, client, 39);name[39] = '\0';std::strncpy(acctnum, num, 24);acctnum[24] = '\0';balance = bal;}void BankAccount::show(void) const{using std::cout;using std:: endl;cout << "Client: " << name << endl;cout << "Account Number: " << acctnum << endl;cout << "Balance: " << balance << endl;}void BankAccount::deposit(double cash){if (cash >= 0)balance += cash;elsestd::cout << "Illegal transaction attempted";}void BankAccount::withdraw(double cash){if (cash < 0)std::cout << "Illegal transaction attempted";else if (cash <= balance)balance -=cash;elsestd::cout << "Request denied due to insufficient funds.\n"; }// sample useint main(){BankAccount bird;BankAccount frog("Kermit", "croak322", 123.00);bird.show();frog.show();bird = BankAccount("Chipper", "peep8282", 214.00);bird.show();frog.deposit(20);frog.show();frog.withdraw(4000);frog.show();frog.withdraw(50);frog.show();}PE10-4// pe10-4.h#ifndef SALES__#define SALES__namespace SALES{const int QUARTERS = 4;class Sales{private:double sales[QUARTERS];double average;double max;double min;public:// default constructorSales();// copies the lesser of 4 or n items from the array ar// to the sales member and computes and stores the// average, maximum, and minimum values of the entered items;// remaining elements of sales, if any, set to 0Sales(const double ar[], int n);// gathers sales for 4 quarters interactively, stores them// in the sales member of object and computes and stores the// average, maximum, and minumum valuesvoid setSales();// display all information in objectvoid showSales();};}#endif。
C++_Primer_Plus(第五版)习题解答
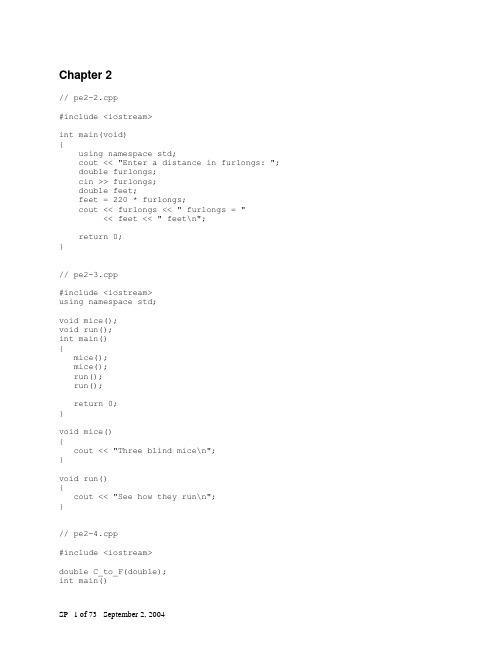
Chapter 2// pe2-2.cpp#include <iostream>int main(void){using namespace std;cout << "Enter a distance in furlongs: ";double furlongs;cin >> furlongs;double feet;feet = 220 * furlongs;cout << furlongs << " furlongs = "<< feet << " feet\n";return 0;}// pe2-3.cpp#include <iostream>using namespace std;void mice();void run();int main(){mice();mice();run();run();return 0;}void mice(){cout << "Three blind mice\n";}void run(){cout << "See how they run\n";}// pe2-4.cpp#include <iostream>double C_to_F(double);int main(){using namespace std;cout << "Enter a temperature in Celsius: "; double C;cin >> C;double F;F = C_to_F(C);cout << C << " degrees Celsius = "<< F << " degrees Fahrenheit\n";return 0;}double C_to_F(double temp){return 1.8 * temp + 32.0;}Chapter 3// pe3-1.cpp#include <iostream>const int Inch_Per_Foot = 12;int main(void){using namespace std;// Note: some environments don't support the backspace charactercout << "Please enter your height in inches: ___/b/b/b ";int ht_inch;cin >> ht_inch;int ht_feet = ht_inch / Inch_Per_Foot;int rm_inch = ht_inch % Inch_Per_Foot;cout << "Your height is " << ht_feet << " feet, ";cout << rm_inch << " inch(es).\n";return 0;}// pe3-3.cpp#include <iostream>const double MINS_PER_DEG = 60.0;const double SECS_PER_MIN = 60.0;int main(){using namespace std;int degrees;int minutes;int seconds;double latitude;cout << "Enter a latitude in degrees, minutes, and seconds:\n";cout << "First, enter the degrees: ";cin >> degrees;cout << "Next, enter the minutes of arc: ";cin >> minutes;cout << "Finally, enter the seconds of arc: ";cin >> seconds;latitude = degrees + (minutes + seconds / SECS_PER_MIN)/MINS_PER_DEG; cout << degrees << " degrees, " << minutes << " minutes, "<< seconds << " seconds = " << latitude << " degrees\n";return 0;}// pe3-5.cpp#include <iostream>int main(void){using namespace std;cout << "How many miles have you driven your car? ";float miles;cin >> miles;cout << "How many gallons of gasoline did the car use? ";float gallons;cin >> gallons;cout << "Your car got " << miles / gallons;cout << " miles per gallon.\n";return 0;}// pe3-6.cpp#include <iostream>const double KM100_TO_MILES = 62.14;const double LITERS_PER_GALLON = 3.875;int main ( void ){using namespace std;double euro_rating;double us_rating;cout << "Enter fuel consumption in liters per 100 km: ";cin >> euro_rating;// divide by LITER_PER_GALLON to get gallons per 100-km// divide by KM100_TO_MILES to get gallons per mile// invert result to get miles per gallonus_rating = (LITERS_PER_GALLON * KM100_TO_MILES) / euro_rating; cout << euro_rating << " liters per 100 km is ";cout << us_rating << " miles per gallon.\n";return 0;}Chapter 4// pe4-2.cpp -- storing strings in string objects#include <iostream>#include <string>int main(){using namespace std;string name;string dessert;cout << "Enter your name:\n";getline(cin, name); // reads through newlinecout << "Enter your favorite dessert:\n";getline(cin, dessert);cout << "I have some delicious " << dessert;cout << " for you, " << name << ".\n";return 0;}// pe4-3.cpp -- storing strings in char arrays#include <iostream>#include <cstring>const int SIZE = 20;int main(){using namespace std;char firstName[SIZE];char lastName[SIZE];char fullName[2*SIZE + 1];cout << "Enter your first name: ";cin >> firstName;cout << "Enter your last name: ";cin >> lastName;strncpy(fullName,lastName,SIZE);strcat(fullName, ", ");strncat(fullName, firstName, SIZE);fullName[SIZE - 1] = '\0';cout << "Here's the information in a single string: " << fullName << endl;return 0;}// pe4-5.cpp// a candybar structurestruct CandyBar {char brand[40];double weight;int calories;};#include <iostream>int main(){using namespace std; //introduces namespace stdCandyBar snack = { "Mocha Munch", 2.3, 350 };cout << "Brand name: " << snack.brand << endl;cout << "Weight: " << snack.weight << endl;cout << "Calories: " << snack.calories << endl;return 0;}// p#include <iostream>const int Slen = 70;struct pizza {char name[Slen];float diameter;float weight;};int main(void){using namespace std;pizza pie;cout << "What is the name of the pizza company? ";cin.getline(, Slen);cout << "What is the diameter of the pizza in inches? ";cin >> pie.diameter;cout << "How much does the pizza weigh in ounces? ";cin >> pie.weight;cout << "Company: " << << "\n";cout << "Diameter: " << pie.diameter << " inches\n";cout << "Weight: " << pie.weight << " ounces\n";return 0;}Chapter 5// pe5-2.cpp#include <iostream>int main(void){using namespace std;double sum = 0.0;double in;cout << "Enter a number (0 to terminate) : ";cin >> in;while (in != 0) {sum += in;cout << "Running total = " << sum << "\n";cout << "Enter next number (0 to terminate) : ";cin >> in;}cout << "Bye!\n";return 0;}// pe5-4.cpp// book sales#include <iostream>const int MONTHS = 12;const char * months[MONTHS] = {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"};int main(){using namespace std; //introduces namespace stdint sales[MONTHS];int month;cout << "Enter the monthly sales for \"C++ for Fools\":\n";for (month = 0; month < MONTHS; month++){cout << "Sales for " << months[month] << ": ";cin >> sales[month];}double total = 0.0;for (month = 0; month < MONTHS; month++)total += sales[month];cout << "Total sales: " << total << endl;return 0;}// pe5-6.cpp#include <iostream>struct car { char name[20]; int year;};int main(void){using namespace std;int n;cout << "How many cars do you wish to catalog?: ";cin >> n;while(cin.get() != '\n') // get rid of rest of line;car * pc = new car [n];int i;for (i = 0; i < n; i++){cout << "Car #" << (i + 1) << ":\n";cout << "Please enter the make: ";cin.getline(pc[i].name,20);cout << "Please enter the year made: ";cin >> pc[i].year;while(cin.get() != '\n') // get rid of rest of line ;}cout << "Here is your collection:\n";for (i = 0; i < n; i++)cout << pc[i].year << " " << pc[i].name << "\n";delete [] pc;return 0;}// pe5-7.cpp -- count words using C-style string#include <iostream>#include <cstring> // prototype for strcmp()const int STR_LIM = 50;int main(){using namespace std;char word[STR_LIM];int count = 0;cout << "Enter words (to stop, type the word done):\n";while (cin >> word && strcmp("done", word))++count;cout << "You entered a total of " << count << " words.\n"; return 0;}// pe5-9.cpp//nested loops#include <iostream>int main(){using namespace std; //introduces namespace stdint rows;int row;int col;int periods;cout << "Enter number of rows: ";cin >> rows;for (row = 1; row <= rows; row++){periods = rows - row;for (col = 1; col <= periods; col++)cout << '.';// col already has correct value for next loopfor ( ; col <= rows; col++)cout << '*';cout << endl;}return 0;}Chapter 6// pe6-1.cpp#include <iostream>#include <cctype>int main( ){using namespace std; //introduces namespace stdchar ch;cin.get(ch);while(ch != '@'){if (!isdigit(ch)){if (isupper(ch))ch = tolower(ch);else if (islower(ch))ch = toupper(ch);cout << ch;}cin.get(ch);}return 0;}// pe6-3.cpp#include <iostream>int main(void){using namespace std;cout << "Please enter one of the following choices:\n";cout << "c) carnivore p) pianist\n"<< "t) tree g) game\n";char ch;cin >> ch;while (ch != 'c' && ch != 'p' && ch != 't' && ch != 'g'){cout << "Please enter a c, p, t, or g: ";cin >> ch;}switch (ch){case 'c' : cout << "A cat is a carnivore.\n";break;case 'p' : cout << "Radu Lupu is a pianist.\n";break;case 't' : cout << "A maple is a tree.\n";break;case 'g' : cout << "Golf is a game.\n";break;default : cout << "The program shouldn't get here!\n"; }return 0;}// pe6-5.cpp// Neutronia taxation#include <iostream>const double LEV1 = 5000;const double LEV2 = 15000;const double LEV3 = 35000;const double RATE1 = 0.10;const double RATE2 = 0.15;const double RATE3 = 0.20;int main( ){using namespace std;double income;double tax;cout << "Enter your annual income in tvarps: ";cin >> income;if (income <= LEV1)tax = 0;else if (income <= LEV2)tax = (income - LEV1) * RATE1;else if (income <= LEV3)tax = RATE1 * (LEV2 - LEV1) + RATE2 * (income - LEV2);elsetax = RATE1 * (LEV2 - LEV1) + RATE2 * (LEV3 - LEV2)+ RATE3 * (income - LEV3);cout << "You owe Neutronia " << tax << " tvarps in taxes.\n";return 0;}// pe6-7.cpp#include <iostream>#include <string>int main(){using namespace std;string word;char ch;int vowel = 0;int consonant = 0;int other = 0;cout << "Enter words (q to quit):\n";cin >> word;while ( word != "q"){ch = tolower(word[0]);if (isalpha(ch)){if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u')vowel++;elseconsonant++;}elseother++;cin >> word;}cout << vowel <<" words beginning with vowels\n";cout << consonant << " words beginning with consonants\n";cout << other << " others\n";return 0;}// pe6-8.cpp -- counting characters#include <iostream>#include <fstream> // file I/O suppport#include <cstdlib> // support for exit()const int SIZE = 60;int main(){using namespace std;char filename[SIZE];char ch;ifstream inFile; // object for handling file inputcout << "Enter name of data file: ";cin.getline(filename, SIZE);inFile.open(filename); // associate inFile with a fileif (!inFile.is_open()) // failed to open file{cout << "Could not open the file " << filename << endl;cout << "Program terminating.\n";exit(EXIT_FAILURE);}int count = 0; // number of items readinFile >> ch; // get first valuewhile (inFile.good()) // while input good and not at EOF {count++; // one more item readinFile >> ch; // get next value}cout << count << " characters in " << filename << endl;inFile.close(); // finished with the filereturn 0;}Chapter 7//pe7-1.cpp -- harmonic mean#include <iostream>double h_mean(double x, double y);int main(void){using namespace std;double x,y;cout << "Enter two numbers (a 0 terminates): ";while (cin >> x >> y && x * y != 0)cout << "harmonic mean of " << x << " and "<< y << " = " << h_mean(x,y) << "\n";/* or do the reading and testing in two parts:while (cin >> x && x != 0){cin >> y;if (y == 0)break;...*/cout << "Bye\n";return 0;}double h_mean(double x, double y){return 2.0 * x * y / (x + y);}// pe7-3.cpp#include <iostream>struct box {char maker[40];float height;float width;float length;float volume;};void showbox(box b);void setbox(box * pb);int main(void){box carton = {"Bingo Boxer", 2, 3, 5}; // no volume providedsetbox(&carton);showbox(carton);return 0;}void showbox(box b){using namespace std;cout << "Box maker: " << b.maker<< "\nheight: " << b.height<< "\nlwidth: " << b.width<< "\nlength: " << b.length<< "\nvolume: " << b.volume << "\n";}void setbox(box * pb){pb->volume = pb->height * pb->width * pb->length;}// pe7-4.cpp -- probability of winning#include <iostream>long double probability(unsigned numbers, unsigned picks);int main(){using namespace std;double total, choices;double mtotal;double probability1, probability2;cout << "Enter total number of game card choices and\n""number of picks allowed for the field:\n";while ((cin >> total >> choices) && choices <= total){cout << "Enter total number of game card choices ""for the mega number:\n";if (!(cin >> mtotal))break;cout << "The chances of getting all " << choices << " picks is one in "<< (probability1 = probability(total, choices) ) << ".\n";cout << "The chances of getting the megaspot is one in "<< (probability2 = probability(mtotal, 1) ) << ".\n";cout << "You have one chance in ";cout << probability1 * probability2; // compute the probability cout << " of winning.\n";cout << "Next set of numbers (q to quit): ";}cout << "bye\n";return 0;}// the following function calculates the probability of picking picks// numbers correctly from numbers choiceslong double probability(unsigned numbers, unsigned picks){long double result = 1.0; // here come some local variableslong double n;unsigned p;for (n = numbers, p = picks; p > 0; n--, p--)result = result * n / p ;return result;}// pe7-6.cpp#include <iostream>int Fill_array(double ar[], int size);void Show_array(const double ar[], int size);void Reverse_array(double ar[], int size);const int LIMIT = 10;int main( ){using namespace std;double values[LIMIT];int entries = Fill_array(values, LIMIT);cout << "Array values:\n";Show_array(values, entries);cout << "Array reversed:\n";Reverse_array(values, entries);Show_array(values, entries);cout << "All but end values reversed:\n";Reverse_array(values + 1, entries - 2);Show_array(values, entries);return 0;}int Fill_array(double ar[], int size){using namespace std;int n;cout << "Enter up to " << size << " values (q to quit):\n"; for (n = 0; n < size; n++){cin >> ar[n];if (!cin)break;}return n;}void Show_array(const double ar[], int size){using namespace std;int n;for (n = 0; n < size; n++){cout << ar[n];if (n % 8 == 7)cout << endl;elsecout << ' ';}if (n % 8 != 0)cout << endl;}void Reverse_array(double ar[], int size){int i, j;double temp;for (i = 0, j = size - 1; i < j; i++, j--){temp = ar[i];ar[i] = ar[j];ar[j] = temp;}}//pe7-9.cpp#include <iostream>double calculate(double x, double y, double (*pf)(double, double)); double add(double x, double y);double sub(double x, double y);double mean(double x, double y);int main(void){using namespace std;double (*pf[3])(double,double) = {add, sub, mean};char * op[3] = {"sum", "difference", "mean"};double a, b;cout << "Enter pairs of numbers (q to quit): ";int i;while (cin >> a >> b){// using function namescout << calculate(a, b, add) << " = sum\n";cout << calculate(a, b, mean) << " = mean\n";// using pointersfor (i = 0; i < 3; i++)cout << calculate(a, b, pf[i]) << " = "<< op[i] << "\n";}cout << "Done!\n";return 0;}double calculate(double x, double y, double (*pf)(double, double)) {return (*pf)(x, y);}double add(double x, double y){return x + y;}double sub(double x, double y){return x - y;}double mean(double x, double y){return (x + y) / 2.0;}Chapter 8// pe8-1.cpp#include <iostream>void silly(const char * s, int n = 0);int main(void){using namespace std;char * p1 = "Why me?\n";silly(p1);for (int i = 0; i < 3; i++){cout << i << " = i\n";silly(p1, i);}cout << "Done\n";return 0;}void silly(const char * s, int n){using namespace std;static int uses = 0;int lim = ++uses;if (n == 0)lim = 1;for (int i = 0; i < lim; i++)cout << s;}// pe8-4.cpp#include <iostream>#include <cstring> // for strlen(), strcpy()using namespace std;struct stringy {char * str; // points to a stringint ct; // length of string (not counting '\0')};void show(const char *str, int cnt = 1);void show(const stringy & bny, int cnt = 1);void set(stringy & bny, const char * str);int main(void){stringy beany;char testing[] = "Reality isn't what it used to be.";set(beany, testing); // first argument is a reference, // allocates space to hold copy of testing,// sets str member of beany to point to the// new block, copies testing to new block,// and sets ct member of beanyshow(beany); // prints member string onceshow(beany, 2); // prints member string twicetesting[0] = 'D';testing[1] = 'u';show(testing); // prints testing string onceshow(testing, 3); // prints testing string thrice show("Done!");return 0;}void show(const char *str, int cnt){while(cnt-- > 0){cout << str << endl;}}void show(const stringy & bny, int cnt){while(cnt-- > 0){cout << bny.str << endl;}}void set(stringy & bny, const char * str){bny.ct = strlen(str);bny.str = new char[bny.ct+1];strcpy(bny.str, str);}// pe8-5.cpp#include <iostream>template <class T>T max5(T ar[]){int n;T max = ar[0];for (n = 1; n < 5; n++)if (ar[n] > max)max = ar[n];return max;}const int LIMIT = 5;int main( ){using namespace std;double ard[LIMIT] = { -3.4, 8.1, -76.4, 34.4, 2.4}; int ari[LIMIT] = {2, 3, 8, 1, 9};double md;int mi;md = max5(ard);mi = max5(ari);cout << "md = " << md << endl;cout << "mi = " << mi << endl;return 0;}Chapter 9PE 9-1// pe9-golf.h - for pe9-1.cppconst int Len = 40;struct golf{char fullname[Len];int handicap;};// non-interactive version// function sets golf structure to provided name, handicap// using values passed as arguments to the functionvoid setgolf(golf & g, const char * name, int hc);// interactive version// function solicits name and handicap from user// and sets the members of g to the values entered// returns 1 if name is entered, 0 if name is empty stringint setgolf(golf & g);// function resets handicap to new valuevoid handicap(golf & g, int hc);// function displays contents of golf structurevoid showgolf(const golf & g);// pe9-golf.cpp - for pe9-1.cpp#include <iostream>#include "pe9-golf.h"#include <cstring>// function solicits name and handicap from user// returns 1 if name is entered, 0 if name is empty stringint setgolf(golf & g){std::cout << "Please enter golfer's full name: ";std::cin.getline(g.fullname, Len);if (g.fullname[0] == '\0')return 0; // premature terminationstd::cout << "Please enter handicap for " << g.fullname << ": "; while (!(std::cin >> g.handicap)){std::cin.clear();std::cout << "Please enter an integer: ";}while (std::cin.get() != '\n')continue;return 1;}// function sets golf structure to provided name, handicapvoid setgolf(golf & g, const char * name, int hc){std::strcpy(g.fullname, name);g.handicap = hc;}// function resets handicap to new valuevoid handicap(golf & g, int hc){g.handicap = hc;}// function displays contents of golf structurevoid showgolf(const golf & g){std::cout << "Golfer: " << g.fullname << "\n";std::cout << "Handicap: " << g.handicap << "\n\n";}// pe9-1.cpp#include <iostream>#include "pe9-golf.h"// link with pe9-golf.cppconst int Mems = 5;int main(void){using namespace std;golf team[Mems];cout << "Enter up to " << Mems << " golf team members:\n";int i;for (i = 0; i < Mems; i++)if (setgolf(team[i]) == 0)break;for (int j = 0; j < i; j++)showgolf(team[j]);setgolf(team[0], "Fred Norman", 5);showgolf(team[0]);handicap(team[0], 3);showgolf(team[0]);return 0;}PE 9-3//pe9-3.cpp -- using placement new#include <iostream>#include <new>#include <cstring>struct chaff{char dross[20];int slag;};// char buffer[500]; // option 1int main(){using std::cout;using std::endl;chaff *p1;int i;char * buffer = new char [500]; // option 2p1 = new (buffer) chaff[2]; // place structures in buffer std::strcpy(p1[0].dross, "Horse Feathers");p1[0].slag = 13;std::strcpy(p1[1].dross, "Piffle");p1[1].slag = -39;for (i = 0; i < 2; i++)cout << p1[i].dross << ": " << p1[i].slag << endl;delete [] buffer; // option 2return 0;}Chapter 10PE 10-1// pe10-1.cpp#include <iostream>#include <cstring>// class declarationclass BankAccount{private:char name[40];char acctnum[25];double balance;public:BankAccount(char * client = "no one", char * num = "0",double bal = 0.0); void show(void) const; void deposit(double cash); void withdraw(double cash); };// method definitionsBankAccount::BankAccount(char * client, char * num, double bal) {std::strncpy(name, client, 39);name[39] = '\0';std::strncpy(acctnum, num, 24);acctnum[24] = '\0';balance = bal;}void BankAccount::show(void) const{using std::cout;using std:: endl;cout << "Client: " << name << endl;cout << "Account Number: " << acctnum << endl;cout << "Balance: " << balance << endl;}void BankAccount::deposit(double cash){if (cash >= 0)balance += cash;elsestd::cout << "Illegal transaction attempted";}void BankAccount::withdraw(double cash){if (cash < 0)std::cout << "Illegal transaction attempted";else if (cash <= balance)balance -=cash;elsestd::cout << "Request denied due to insufficient funds.\n"; }// sample useint main(){BankAccount bird;BankAccount frog("Kermit", "croak322", 123.00);bird.show();frog.show();bird = BankAccount("Chipper", "peep8282", 214.00);bird.show();frog.deposit(20);frog.show();frog.withdraw(4000);frog.show();frog.withdraw(50);frog.show();}PE10-4// pe10-4.h#ifndef SALES__#define SALES__namespace SALES{const int QUARTERS = 4;class Sales{private:double sales[QUARTERS];double average;double max;double min;public:// default constructorSales();// copies the lesser of 4 or n items from the array ar// to the sales member and computes and stores the// average, maximum, and minimum values of the entered items;// remaining elements of sales, if any, set to 0Sales(const double ar[], int n);// gathers sales for 4 quarters interactively, stores them// in the sales member of object and computes and stores the// average, maximum, and minumum valuesvoid setSales();// display all information in objectvoid showSales();};}#endif。
C++_Primer_Plus中文第五版编程练习答案-非扫描

// pe2-2.cpp#include <iostream>int main(void){using namespace std;cout << "Enter a distance in furlongs: ";double furlongs;cin >> furlongs;double feet;feet = 220 * furlongs;cout << furlongs << " furlongs = "<< feet << " feet\n";return 0;}// pe2-3.cpp#include <iostream>using namespace std;void mice();void run();int main(){mice();mice();run();run();return 0;}void mice(){cout << "Three blind mice\n";}void run(){cout << "See how they run\n";}// pe2-4.cpp#include <iostream>double C_to_F(double);int main(){using namespace std;cout << "Enter a temperature in Celsius: "; double C;cin >> C;double F;F = C_to_F(C);cout << C << " degrees Celsius = "<< F << " degrees Fahrenheit\n";return 0;}double C_to_F(double temp){return 1.8 * temp + 32.0;}// pe3-1.cpp#include <iostream>const int Inch_Per_Foot = 12;int main(void){using namespace std;// Note: some environments don't support the backspace charactercout << "Please enter your height in inches: ___/b/b/b ";int ht_inch;cin >> ht_inch;int ht_feet = ht_inch / Inch_Per_Foot;int rm_inch = ht_inch % Inch_Per_Foot;cout << "Your height is " << ht_feet << " feet, ";cout << rm_inch << " inch(es).\n";return 0;}// pe3-3.cpp#include <iostream>const double MINS_PER_DEG = 60.0;const double SECS_PER_MIN = 60.0;int main(){using namespace std;int degrees;int minutes;int seconds;double latitude;cout << "Enter a latitude in degrees, minutes, and seconds:\n";cout << "First, enter the degrees: ";cin >> degrees;cout << "Next, enter the minutes of arc: ";cin >> minutes;cout << "Finally, enter the seconds of arc: ";cin >> seconds;latitude = degrees + (minutes + seconds / SECS_PER_MIN)/MINS_PER_DEG; cout << degrees << " degrees, " << minutes << " minutes, "<< seconds << " seconds = " << latitude << " degrees\n";return 0;}// pe3-5.cpp#include <iostream>int main(void){using namespace std;cout << "How many miles have you driven your car? ";float miles;cin >> miles;cout << "How many gallons of gasoline did the car use? ";float gallons;cin >> gallons;cout << "Your car got " << miles / gallons;cout << " miles per gallon.\n";return 0;}// pe3-6.cpp#include <iostream>const double KM100_TO_MILES = 62.14;const double LITERS_PER_GALLON = 3.875;int main ( void ){using namespace std;double euro_rating;double us_rating;cout << "Enter fuel consumption in liters per 100 km: ";cin >> euro_rating;// divide by LITER_PER_GALLON to get gallons per 100-km// divide by KM100_TO_MILES to get gallons per mile// invert result to get miles per gallonus_rating = (LITERS_PER_GALLON * KM100_TO_MILES) / euro_rating; cout << euro_rating << " liters per 100 km is ";cout << us_rating << " miles per gallon.\n";return 0;}// pe4-2.cpp -- storing strings in string objects#include <iostream>#include <string>int main(){using namespace std;string name;string dessert;cout << "Enter your name:\n";getline(cin, name); // reads through newlinecout << "Enter your favorite dessert:\n";getline(cin, dessert);cout << "I have some delicious " << dessert;cout << " for you, " << name << ".\n";return 0;}// pe4-3.cpp -- storing strings in char arrays#include <iostream>#include <cstring>const int SIZE = 20;int main(){using namespace std;char firstName[SIZE];char lastName[SIZE];char fullName[2*SIZE + 1];cout << "Enter your first name: ";cin >> firstName;cout << "Enter your last name: ";cin >> lastName;strncpy(fullName,lastName,SIZE);strcat(fullName, ", ");strncat(fullName, firstName, SIZE);fullName[SIZE - 1] = '\0';cout << "Here's the information in a single string: " << fullName << endl;return 0;}// pe4-5.cpp// a candybar structurestruct CandyBar {char brand[40];double weight;int calories;};#include <iostream>int main(){using namespace std; //introduces namespace stdCandyBar snack = { "Mocha Munch", 2.3, 350 };cout << "Brand name: " << snack.brand << endl;cout << "Weight: " << snack.weight << endl;cout << "Calories: " << snack.calories << endl;return 0;}// p#include <iostream>const int Slen = 70;struct pizza {char name[Slen];float diameter;float weight;};int main(void){using namespace std;pizza pie;cout << "What is the name of the pizza company? ";cin.getline(, Slen);cout << "What is the diameter of the pizza in inches? ";cin >> pie.diameter;cout << "How much does the pizza weigh in ounces? ";cin >> pie.weight;cout << "Company: " << << "\n";cout << "Diameter: " << pie.diameter << " inches\n";cout << "Weight: " << pie.weight << " ounces\n";return 0;}// pe5-2.cpp#include <iostream>int main(void){using namespace std;double sum = 0.0;double in;cout << "Enter a number (0 to terminate) : ";cin >> in;while (in != 0) {sum += in;cout << "Running total = " << sum << "\n";cout << "Enter next number (0 to terminate) : ";cin >> in;}cout << "Bye!\n";return 0;}// pe5-4.cpp// book sales#include <iostream>const int MONTHS = 12;const char * months[MONTHS] = {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"};int main(){using namespace std; //introduces namespace stdint sales[MONTHS];int month;cout << "Enter the monthly sales for \"C++ for Fools\":\n";for (month = 0; month < MONTHS; month++){cout << "Sales for " << months[month] << ": ";cin >> sales[month];}double total = 0.0;for (month = 0; month < MONTHS; month++)total += sales[month];cout << "Total sales: " << total << endl;return 0;}// pe5-6.cpp#include <iostream>struct car { char name[20]; int year;};int main(void){using namespace std;int n;cout << "How many cars do you wish to catalog?: ";cin >> n;while(cin.get() != '\n') // get rid of rest of line;car * pc = new car [n];int i;for (i = 0; i < n; i++){cout << "Car #" << (i + 1) << ":\n";cout << "Please enter the make: ";cin.getline(pc[i].name,20);cout << "Please enter the year made: ";cin >> pc[i].year;while(cin.get() != '\n') // get rid of rest of line ;}cout << "Here is your collection:\n";for (i = 0; i < n; i++)cout << pc[i].year << " " << pc[i].name << "\n";delete [] pc;return 0;}// pe5-7.cpp -- count words using C-style string#include <iostream>#include <cstring> // prototype for strcmp()const int STR_LIM = 50;int main(){using namespace std;char word[STR_LIM];int count = 0;cout << "Enter words (to stop, type the word done):\n";while (cin >> word && strcmp("done", word))++count;cout << "You entered a total of " << count << " words.\n"; return 0;}// pe5-9.cpp//nested loops#include <iostream>int main(){using namespace std; //introduces namespace stdint rows;int row;int col;int periods;cout << "Enter number of rows: ";cin >> rows;for (row = 1; row <= rows; row++){periods = rows - row;for (col = 1; col <= periods; col++)cout << '.';// col already has correct value for next loopfor ( ; col <= rows; col++)cout << '*';cout << endl;}return 0;}// pe6-1.cpp#include <iostream>#include <cctype>int main( ){using namespace std; //introduces namespace stdchar ch;cin.get(ch);while(ch != '@'){if (!isdigit(ch)){if (isupper(ch))ch = tolower(ch);else if (islower(ch))ch = toupper(ch);cout << ch;}cin.get(ch);}return 0;}// pe6-3.cpp#include <iostream>int main(void){using namespace std;cout << "Please enter one of the following choices:\n";cout << "c) carnivore p) pianist\n"<< "t) tree g) game\n";char ch;cin >> ch;while (ch != 'c' && ch != 'p' && ch != 't' && ch != 'g') {cout << "Please enter a c, p, t, or g: ";cin >> ch;}switch (ch){case 'c' : cout << "A cat is a carnivore.\n";break;case 'p' : cout << "Radu Lupu is a pianist.\n";break;case 't' : cout << "A maple is a tree.\n";break;case 'g' : cout << "Golf is a game.\n";break;default : cout << "The program shouldn't get here!\n"; }return 0;}// pe6-5.cpp// Neutronia taxation#include <iostream>const double LEV1 = 5000;const double LEV2 = 15000;const double LEV3 = 35000;const double RATE1 = 0.10;const double RATE2 = 0.15;const double RATE3 = 0.20;int main( ){using namespace std;double income;double tax;cout << "Enter your annual income in tvarps: ";cin >> income;if (income <= LEV1)tax = 0;else if (income <= LEV2)tax = (income - LEV1) * RATE1;else if (income <= LEV3)tax = RATE1 * (LEV2 - LEV1) + RATE2 * (income - LEV2);elsetax = RATE1 * (LEV2 - LEV1) + RATE2 * (LEV3 - LEV2)+ RATE3 * (income - LEV3);cout << "You owe Neutronia " << tax << " tvarps in taxes.\n";return 0;}// pe6-7.cpp#include <iostream>#include <string>int main(){using namespace std;string word;char ch;int vowel = 0;int consonant = 0;int other = 0;cout << "Enter words (q to quit):\n";cin >> word;while ( word != "q"){ch = tolower(word[0]);if (isalpha(ch)){if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o'|| ch == 'u')vowel++;elseconsonant++;}elseother++;cin >> word;}cout << vowel <<" words beginning with vowels\n";cout << consonant << " words beginning with consonants\n";cout << other << " others\n";return 0;}// pe6-8.cpp -- counting characters#include <iostream>#include <fstream> // file I/O suppport#include <cstdlib> // support for exit()const int SIZE = 60;int main(){using namespace std;char filename[SIZE];char ch;ifstream inFile; // object for handling file inputcout << "Enter name of data file: ";cin.getline(filename, SIZE);inFile.open(filename); // associate inFile with a fileif (!inFile.is_open()) // failed to open file{cout << "Could not open the file " << filename << endl;cout << "Program terminating.\n";exit(EXIT_FAILURE);}int count = 0; // number of items readinFile >> ch; // get first valuewhile (inFile.good()) // while input good and not at EOF {count++; // one more item readinFile >> ch; // get next value}cout << count << " characters in " << filename << endl;inFile.close(); // finished with the file return 0;}//pe7-1.cpp -- harmonic mean#include <iostream>double h_mean(double x, double y);int main(void){using namespace std;double x,y;cout << "Enter two numbers (a 0 terminates): ";while (cin >> x >> y && x * y != 0)cout << "harmonic mean of " << x << " and "<< y << " = " << h_mean(x,y) << "\n";/* or do the reading and testing in two parts:while (cin >> x && x != 0){cin >> y;if (y == 0)break;...*/cout << "Bye\n";return 0;}double h_mean(double x, double y){return 2.0 * x * y / (x + y);}// pe7-3.cpp#include <iostream>struct box {char maker[40];float height;float width;float length;float volume;};void showbox(box b);void setbox(box * pb);int main(void){box carton = {"Bingo Boxer", 2, 3, 5}; // no volume providedsetbox(&carton);showbox(carton);return 0;}void showbox(box b){using namespace std;cout << "Box maker: " << b.maker<< "\nheight: " << b.height<< "\nlwidth: " << b.width<< "\nlength: " << b.length<< "\nvolume: " << b.volume << "\n";}void setbox(box * pb){pb->volume = pb->height * pb->width * pb->length;}// pe7-4.cpp --probability of winning#include <iostream>long double probability(unsigned numbers, unsigned picks);int main(){using namespace std;double total, choices;double mtotal;double probability1, probability2;cout << "Enter total number of game card choices and\n""number of picks allowed for the field:\n";while ((cin >> total >> choices) && choices <= total){cout << "Enter total number of game card choices ""for the mega number:\n";if (!(cin >> mtotal))break;cout << "The chances of getting all " << choices << " picks is one in "<< (probability1 = probability(total, choices) ) << ".\n";cout << "The chances of getting the megaspot is one in "<< (probability2 = probability(mtotal, 1) ) << ".\n";cout << "You have one chance in ";cout << probability1 * probability2; // compute the probabilitycout << " of winning.\n";cout << "Next set of numbers (q to quit): ";}cout << "bye\n";return 0;}// the following function calculates the probability of picking picks// numbers correctly from numbers choiceslong double probability(unsigned numbers, unsigned picks){long double result = 1.0; // here come some local variableslong double n;unsigned p;for (n = numbers, p = picks; p > 0; n--, p--)result = result * n / p ;return result;}// pe7-6.cpp#include <iostream>int Fill_array(double ar[], int size);void Show_array(const double ar[], int size);void Reverse_array(double ar[], int size);const int LIMIT = 10;int main( ){using namespace std;double values[LIMIT];int entries = Fill_array(values, LIMIT);cout << "Array values:\n";Show_array(values, entries);cout << "Array reversed:\n";Reverse_array(values, entries);Show_array(values, entries);cout << "All but end values reversed:\n";Reverse_array(values + 1, entries - 2);Show_array(values, entries);return 0;}int Fill_array(double ar[], int size){using namespace std;int n;cout << "Enter up to " << size << " values (q to quit):\n"; for (n = 0; n < size; n++){cin >> ar[n];if (!cin)break;}return n;}void Show_array(const double ar[], int size){using namespace std;int n;for (n = 0; n < size; n++){cout << ar[n];if (n % 8 == 7)cout << endl;elsecout << ' ';}if (n % 8 != 0)cout << endl;}void Reverse_array(double ar[], int size){int i, j;double temp;for (i = 0, j = size - 1; i < j; i++, j--){temp = ar[i];ar[i] = ar[j];ar[j] = temp;}}//pe7-9.cpp#include <iostream>double calculate(double x, double y, double (*pf)(double, double)); double add(double x, double y);double sub(double x, double y);double mean(double x, double y);int main(void){using namespace std;double (*pf[3])(double,double) = {add, sub, mean};char * op[3] = {"sum", "difference", "mean"};double a, b;cout << "Enter pairs of numbers (q to quit): ";int i;while (cin >> a >> b){// using function namescout << calculate(a, b, add) << " = sum\n";cout << calculate(a, b, mean) << " = mean\n";// using pointersfor (i = 0; i < 3; i++)cout << calculate(a, b, pf[i]) << " = "<< op[i] << "\n";}cout << "Done!\n";return 0;}double calculate(double x, double y, double (*pf)(double, double)) {return (*pf)(x, y);}double add(double x, double y){return x + y;}double sub(double x, double y){return x - y;}double mean(double x, double y){return (x + y) / 2.0;}// pe8-1.cpp#include <iostream>void silly(const char * s, int n = 0);int main(void){using namespace std;char * p1 = "Why me?\n";silly(p1);for (int i = 0; i < 3; i++){cout << i << " = i\n";silly(p1, i);}cout << "Done\n";return 0;}void silly(const char * s, int n){using namespace std;static int uses = 0;int lim = ++uses;if (n == 0)lim = 1;for (int i = 0; i < lim; i++)cout << s;}// pe8-4.cpp#include <iostream>#include <cstring> // for strlen(), strcpy()using namespace std;struct stringy {char * str; // points to a stringint ct; // length of string (not counting '\0')};void show(const char *str, int cnt = 1);void show(const stringy & bny, int cnt = 1);void set(stringy & bny, const char * str);int main(void){stringy beany;char testing[] = "Reality isn't what it used to be.";set(beany, testing); // first argument is a reference, // allocates space to hold copy of testing,// sets str member of beany to point to the// new block, copies testing to new block,// and sets ct member of beanyshow(beany); // prints member string onceshow(beany, 2); // prints member string twicetesting[0] = 'D';testing[1] = 'u';show(testing); // prints testing string onceshow(testing, 3); // prints testing string thrice show("Done!");return 0;}void show(const char *str, int cnt){while(cnt-- > 0){cout << str << endl;}}void show(const stringy & bny, int cnt){while(cnt-- > 0){cout << bny.str << endl;}}void set(stringy & bny, const char * str){bny.ct = strlen(str);bny.str = new char[bny.ct+1];strcpy(bny.str, str);}// pe8-5.cpp#include <iostream>template <class T>T max5(T ar[]){int n;T max = ar[0];for (n = 1; n < 5; n++)if (ar[n] > max)max = ar[n];return max;}const int LIMIT = 5;int main( ){using namespace std;double ard[LIMIT] = { -3.4, 8.1, -76.4, 34.4, 2.4}; int ari[LIMIT] = {2, 3, 8, 1, 9};double md;int mi;md = max5(ard);mi = max5(ari);cout << "md = " << md << endl;cout << "mi = " << mi << endl;return 0;}// pe9-golf.h - for pe9-1.cppconst int Len = 40;struct golf{char fullname[Len];int handicap;};// non-interactive version// function sets golf structure to provided name, handicap// using values passed as arguments to the functionvoid setgolf(golf & g, const char * name, int hc);// interactive version// function solicits name and handicap from user// and sets the members of g to the values entered// returns 1 if name is entered, 0 if name is empty stringint setgolf(golf & g);// function resets handicap to new valuevoid handicap(golf & g, int hc);// function displays contents of golf structurevoid showgolf(const golf & g);// pe9-golf.cpp - for pe9-1.cpp#include <iostream>#include "pe9-golf.h"#include <cstring>// function solicits name and handicap from user// returns 1 if name is entered, 0 if name is empty stringint setgolf(golf & g){std::cout << "Please enter golfer's full name: ";std::cin.getline(g.fullname, Len);if (g.fullname[0] == '\0')return 0; // premature termination std::cout << "Please enter handicap for " << g.fullname << ": "; while (!(std::cin >> g.handicap)){std::cin.clear();std::cout << "Please enter an integer: ";}while (std::cin.get() != '\n')continue;return 1;}// function sets golf structure to provided name, handicapvoid setgolf(golf & g, const char * name, int hc){std::strcpy(g.fullname, name);g.handicap = hc;}// function resets handicap to new valuevoid handicap(golf & g, int hc){g.handicap = hc;}// function displays contents of golf structurevoid showgolf(const golf & g){std::cout << "Golfer: " << g.fullname << "\n";std::cout << "Handicap: " << g.handicap << "\n\n";}// pe9-1.cpp#include <iostream>#include "pe9-golf.h"// link with pe9-golf.cppconst int Mems = 5;int main(void){using namespace std;golf team[Mems];cout << "Enter up to " << Mems << " golf team members:\n";int i;for (i = 0; i < Mems; i++)if (setgolf(team[i]) == 0)break;for (int j = 0; j < i; j++)showgolf(team[j]);setgolf(team[0], "Fred Norman", 5);showgolf(team[0]);handicap(team[0], 3);showgolf(team[0]);return 0;}//pe9-3.cpp -- using placement new#include <iostream>#include <new>#include <cstring>struct chaff{char dross[20];int slag;};// char buffer[500]; // option 1int main(){using std::cout;using std::endl;chaff *p1;int i;char * buffer = new char [500]; // option 2p1 = new (buffer) chaff[2]; // place structures in buffer std::strcpy(p1[0].dross, "Horse Feathers");p1[0].slag = 13;std::strcpy(p1[1].dross, "Piffle");p1[1].slag = -39;for (i = 0; i < 2; i++)cout << p1[i].dross << ": " << p1[i].slag << endl;delete [] buffer; // option 2return 0;}// pe10-1.cpp#include <iostream>#include <cstring>// class declarationclass BankAccount{private:char name[40];char acctnum[25];double balance;public:BankAccount(char * client = "no one", char * num = "0",double bal = 0.0); void show(void) const; void deposit(double cash); void withdraw(double cash); };// method definitionsBankAccount::BankAccount(char * client, char * num, double bal) {std::strncpy(name, client, 39);name[39] = '\0';std::strncpy(acctnum, num, 24);acctnum[24] = '\0';balance = bal;}void BankAccount::show(void) const{using std::cout;using std:: endl;cout << "Client: " << name << endl;cout << "Account Number: " << acctnum << endl;cout << "Balance: " << balance << endl;}void BankAccount::deposit(double cash){if (cash >= 0)balance += cash;elsestd::cout << "Illegal transaction attempted";}void BankAccount::withdraw(double cash){if (cash < 0)std::cout << "Illegal transaction attempted";else if (cash <= balance)balance -=cash;elsestd::cout << "Request denied due to insufficient funds.\n"; }// sample useint main(){BankAccount bird;BankAccount frog("Kermit", "croak322", 123.00);bird.show();frog.show();bird = BankAccount("Chipper", "peep8282", 214.00);bird.show();frog.deposit(20);frog.show();frog.withdraw(4000);frog.show();frog.withdraw(50);frog.show();}// pe10-4.h#ifndef SALES__#define SALES__namespace SALES{const int QUARTERS = 4;class Sales{private:double sales[QUARTERS];double average;double max;double min;public:// default constructorSales();// copies the lesser of 4 or n items from the array ar// to the sales member and computes and stores the// average, maximum, and minimum values of the entered items;// remaining elements of sales, if any, set to 0Sales(const double ar[], int n);// gathers sales for 4 quarters interactively, stores them// in the sales member of object and computes and stores the// average, maximum, and minumum valuesvoid setSales();// display all information in objectvoid showSales();};}#endif。
C primer plus(第五版)课后编程练习答案解析
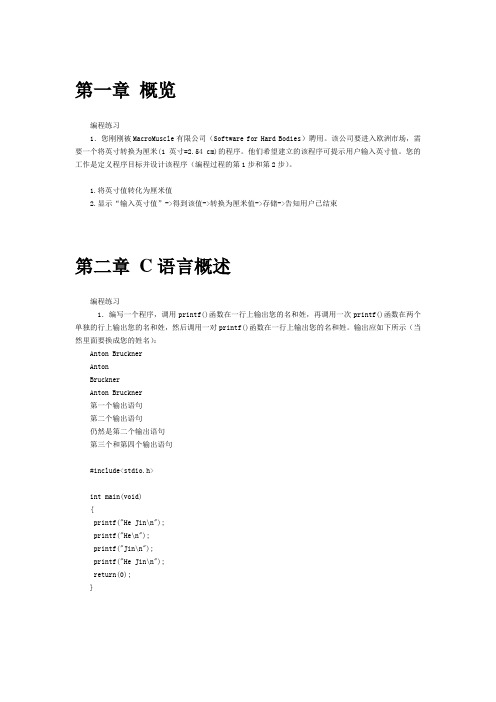
第一章概览编程练习1.您刚刚被MacroMuscle有限公司(Software for Hard Bodies)聘用。
该公司要进入欧洲市场,需要一个将英寸转换为厘米(1英寸=2.54 cm)的程序。
他们希望建立的该程序可提示用户输入英寸值。
您的工作是定义程序目标并设计该程序(编程过程的第1步和第2步)。
1.将英寸值转化为厘米值2.显示“输入英寸值”->得到该值->转换为厘米值->存储->告知用户已结束第二章C语言概述编程练习1.编写一个程序,调用printf()函数在一行上输出您的名和姓,再调用一次printf()函数在两个单独的行上输出您的名和姓,然后调用一对printf()函数在一行上输出您的名和姓。
输出应如下所示(当然里面要换成您的姓名):Anton BrucknerAntonBrucknerAnton Bruckner第一个输出语句第二个输出语句仍然是第二个输出语句第三个和第四个输出语句#include<stdio.h>int main(void){printf("He Jin\n");printf("He\n");printf("Jin\n");printf("He Jin\n");return(0);}2.编写一个程序输出您的姓名及地址。
#include<stdio.h>int main(void){printf("Name:He Jin\n");printf("Address:CAUC\n");return(0);}3.编写一个程序,把您的年龄转换成天数并显示二者的值。
不用考虑平年( fractional year)和闰年(leapyear)的问题。
#include<stdio.h>int main(void){int age=22;printf("Age:%d\n",age);printf("Day:%d\n",age*356);return(0);}4.编写一个能够产生下面输出的程序:For he's a jolly good fellow!For he's a jolly good fellow!For he's a jolly good fellow!Which nobody can deny!程序中除了main()函数之外,要使用两个用户定义的函数:一个用于把上面的夸奖消息输出一次:另一个用于把最后一行输出一次。
C+Primer+Plus中文版(第五版)+的课后编程练习答案

PE 4-6
/* Programming Exercise 4-6 */ #include <stdio.h> #include <float.h>
int main(void) {
float ot_f = 1.0 / 3.0; double ot_d = 1.0 / 3.0;
printf(" float values: "); printf("%.4f %.12f %.16f\n", ot_f, ot_f, ot_f); printf("double values: "); printf("%.4f %.12f %.16f\n", ot_d, ot_d, ot_d); printf("FLT_DIG: %d\n", FLT_DIG); printf("DBL_DIG: %d\n", DBL_DIG); return 0; }
molecules);
return 0; }
Chapter 4
PE 4-1
/* Programming Exercise 4-1 */ #include <stdio.h>
int main(void) {
char fname[40]; char lname[40];
printf("Enter your first name: "); scanf("%s", fname); printf("Enter your last name: "); scanf("%s", lname); printf("%s, %s\n", lname, fname);
Cprimerplus(第五版)课后编程练习答案(完整)
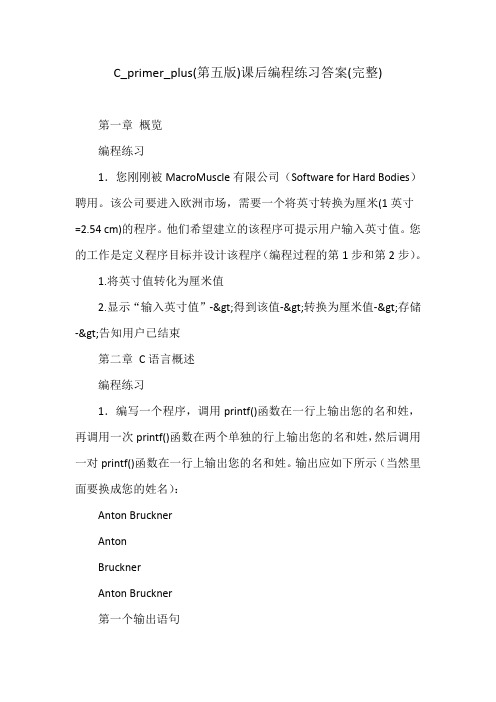
C_primer_plus(第五版)课后编程练习答案(完整)第一章概览编程练习1.您刚刚被MacroMuscle有限公司(Software for Hard Bodies)聘用。
该公司要进入欧洲市场,需要一个将英寸转换为厘米(1英寸=2.54 cm)的程序。
他们希望建立的该程序可提示用户输入英寸值。
您的工作是定义程序目标并设计该程序(编程过程的第1步和第2步)。
1.将英寸值转化为厘米值2.显示“输入英寸值”->得到该值->转换为厘米值->存储->告知用户已结束第二章C语言概述编程练习1.编写一个程序,调用printf()函数在一行上输出您的名和姓,再调用一次printf()函数在两个单独的行上输出您的名和姓,然后调用一对printf()函数在一行上输出您的名和姓。
输出应如下所示(当然里面要换成您的姓名):Anton BrucknerAntonBrucknerAnton Bruckner第一个输出语句第二个输出语句仍然是第二个输出语句第三个和第四个输出语句#include<stdio.h>int main(void){printf("He Jin\n");printf("He\n");printf("Jin\n");printf("He Jin\n");return(0);}2.编写一个程序输出您的姓名及地址。
#include<stdio.h>int main(void){printf("Name:He Jin\n");printf("Address:CAUC\n");return(0);}3.编写一个程序,把您的年龄转换成天数并显示二者的值。
不用考虑平年( fractional year)和闰年(leapyear)的问题。
C++_Primer_Plus(第五版)编程习题解答

Chapter 2// pe2-2.cpp#include <iostream>int main(void){using namespace std;cout << "Enter a distance in furlongs: ";double furlongs;cin >> furlongs;double feet;feet = 220 * furlongs;cout << furlongs << " furlongs = "<< feet << " feet\n";return 0;}// pe2-3.cpp#include <iostream>using namespace std;void mice();void run();int main(){mice();mice();run();run();return 0;}void mice(){cout << "Three blind mice\n";}void run(){cout << "See how they run\n";}// pe2-4.cpp#include <iostream>double C_to_F(double);int main(){using namespace std;cout << "Enter a temperature in Celsius: "; double C;cin >> C;double F;F = C_to_F(C);cout << C << " degrees Celsius = "<< F << " degrees Fahrenheit\n";return 0;}double C_to_F(double temp){return 1.8 * temp + 32.0;}Chapter 3// pe3-1.cpp#include <iostream>const int Inch_Per_Foot = 12;int main(void){using namespace std;// Note: some environments don't support the backspace charactercout << "Please enter your height in inches: ___/b/b/b ";int ht_inch;cin >> ht_inch;int ht_feet = ht_inch / Inch_Per_Foot;int rm_inch = ht_inch % Inch_Per_Foot;cout << "Your height is " << ht_feet << " feet, ";cout << rm_inch << " inch(es).\n";return 0;}// pe3-3.cpp#include <iostream>const double MINS_PER_DEG = 60.0;const double SECS_PER_MIN = 60.0;int main(){using namespace std;int degrees;int minutes;int seconds;double latitude;cout << "Enter a latitude in degrees, minutes, and seconds:\n";cout << "First, enter the degrees: ";cin >> degrees;cout << "Next, enter the minutes of arc: ";cin >> minutes;cout << "Finally, enter the seconds of arc: ";cin >> seconds;latitude = degrees + (minutes + seconds / SECS_PER_MIN)/MINS_PER_DEG; cout << degrees << " degrees, " << minutes << " minutes, "<< seconds << " seconds = " << latitude << " degrees\n";return 0;}// pe3-5.cpp#include <iostream>int main(void){using namespace std;cout << "How many miles have you driven your car? ";float miles;cin >> miles;cout << "How many gallons of gasoline did the car use? ";float gallons;cin >> gallons;cout << "Your car got " << miles / gallons;cout << " miles per gallon.\n";return 0;}// pe3-6.cpp#include <iostream>const double KM100_TO_MILES = 62.14;const double LITERS_PER_GALLON = 3.875;int main ( void ){using namespace std;double euro_rating;double us_rating;cout << "Enter fuel consumption in liters per 100 km: ";cin >> euro_rating;// divide by LITER_PER_GALLON to get gallons per 100-km// divide by KM100_TO_MILES to get gallons per mile// invert result to get miles per gallonus_rating = (LITERS_PER_GALLON * KM100_TO_MILES) / euro_rating; cout << euro_rating << " liters per 100 km is ";cout << us_rating << " miles per gallon.\n";return 0;}Chapter 4// pe4-2.cpp -- storing strings in string objects#include <iostream>#include <string>int main(){using namespace std;string name;string dessert;cout << "Enter your name:\n";getline(cin, name); // reads through newlinecout << "Enter your favorite dessert:\n";getline(cin, dessert);cout << "I have some delicious " << dessert;cout << " for you, " << name << ".\n";return 0;}// pe4-3.cpp -- storing strings in char arrays#include <iostream>#include <cstring>const int SIZE = 20;int main(){using namespace std;char firstName[SIZE];char lastName[SIZE];char fullName[2*SIZE + 1];cout << "Enter your first name: ";cin >> firstName;cout << "Enter your last name: ";cin >> lastName;strncpy(fullName,lastName,SIZE);strcat(fullName, ", ");strncat(fullName, firstName, SIZE);fullName[SIZE - 1] = '\0';cout << "Here's the information in a single string: " << fullName << endl;return 0;}// pe4-5.cpp// a candybar structurestruct CandyBar {char brand[40];double weight;int calories;};#include <iostream>int main(){using namespace std; //introduces namespace stdCandyBar snack = { "Mocha Munch", 2.3, 350 };cout << "Brand name: " << snack.brand << endl;cout << "Weight: " << snack.weight << endl;cout << "Calories: " << snack.calories << endl;return 0;}// p#include <iostream>const int Slen = 70;struct pizza {char name[Slen];float diameter;float weight;};int main(void){using namespace std;pizza pie;cout << "What is the name of the pizza company? ";cin.getline(, Slen);cout << "What is the diameter of the pizza in inches? ";cin >> pie.diameter;cout << "How much does the pizza weigh in ounces? ";cin >> pie.weight;cout << "Company: " << << "\n";cout << "Diameter: " << pie.diameter << " inches\n";cout << "Weight: " << pie.weight << " ounces\n";return 0;}Chapter 5// pe5-2.cpp#include <iostream>int main(void){using namespace std;double sum = 0.0;double in;cout << "Enter a number (0 to terminate) : ";cin >> in;while (in != 0) {sum += in;cout << "Running total = " << sum << "\n";cout << "Enter next number (0 to terminate) : ";cin >> in;}cout << "Bye!\n";return 0;}// pe5-4.cpp// book sales#include <iostream>const int MONTHS = 12;const char * months[MONTHS] = {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"};int main(){using namespace std; //introduces namespace stdint sales[MONTHS];int month;cout << "Enter the monthly sales for \"C++ for Fools\":\n";for (month = 0; month < MONTHS; month++){cout << "Sales for " << months[month] << ": ";cin >> sales[month];}double total = 0.0;for (month = 0; month < MONTHS; month++)total += sales[month];cout << "Total sales: " << total << endl;return 0;}// pe5-6.cpp#include <iostream>struct car { char name[20]; int year;};int main(void){using namespace std;int n;cout << "How many cars do you wish to catalog?: ";cin >> n;while(cin.get() != '\n') // get rid of rest of line;car * pc = new car [n];int i;for (i = 0; i < n; i++){cout << "Car #" << (i + 1) << ":\n";cout << "Please enter the make: ";cin.getline(pc[i].name,20);cout << "Please enter the year made: ";cin >> pc[i].year;while(cin.get() != '\n') // get rid of rest of line ;}cout << "Here is your collection:\n";for (i = 0; i < n; i++)cout << pc[i].year << " " << pc[i].name << "\n";delete [] pc;return 0;}// pe5-7.cpp -- count words using C-style string#include <iostream>#include <cstring> // prototype for strcmp()const int STR_LIM = 50;int main(){using namespace std;char word[STR_LIM];int count = 0;cout << "Enter words (to stop, type the word done):\n";while (cin >> word && strcmp("done", word))++count;cout << "You entered a total of " << count << " words.\n"; return 0;}// pe5-9.cpp//nested loops#include <iostream>int main(){using namespace std; //introduces namespace stdint rows;int row;int col;int periods;cout << "Enter number of rows: ";cin >> rows;for (row = 1; row <= rows; row++){periods = rows - row;for (col = 1; col <= periods; col++)cout << '.';// col already has correct value for next loopfor ( ; col <= rows; col++)cout << '*';cout << endl;}return 0;}Chapter 6// pe6-1.cpp#include <iostream>#include <cctype>int main( ){using namespace std; //introduces namespace stdchar ch;cin.get(ch);while(ch != '@'){if (!isdigit(ch)){if (isupper(ch))ch = tolower(ch);else if (islower(ch))ch = toupper(ch);cout << ch;}cin.get(ch);}return 0;}// pe6-3.cpp#include <iostream>int main(void){using namespace std;cout << "Please enter one of the following choices:\n";cout << "c) carnivore p) pianist\n"<< "t) tree g) game\n";char ch;cin >> ch;while (ch != 'c' && ch != 'p' && ch != 't' && ch != 'g'){cout << "Please enter a c, p, t, or g: ";cin >> ch;}switch (ch){case 'c' : cout << "A cat is a carnivore.\n";break;case 'p' : cout << "Radu Lupu is a pianist.\n";break;case 't' : cout << "A maple is a tree.\n";break;case 'g' : cout << "Golf is a game.\n";break;default : cout << "The program shouldn't get here!\n"; }return 0;}// pe6-5.cpp// Neutronia taxation#include <iostream>const double LEV1 = 5000;const double LEV2 = 15000;const double LEV3 = 35000;const double RATE1 = 0.10;const double RATE2 = 0.15;const double RATE3 = 0.20;int main( ){using namespace std;double income;double tax;cout << "Enter your annual income in tvarps: ";cin >> income;if (income <= LEV1)tax = 0;else if (income <= LEV2)tax = (income - LEV1) * RATE1;else if (income <= LEV3)tax = RATE1 * (LEV2 - LEV1) + RATE2 * (income - LEV2);elsetax = RATE1 * (LEV2 - LEV1) + RATE2 * (LEV3 - LEV2)+ RATE3 * (income - LEV3);cout << "You owe Neutronia " << tax << " tvarps in taxes.\n";return 0;}// pe6-7.cpp#include <iostream>#include <string>int main(){using namespace std;string word;char ch;int vowel = 0;int consonant = 0;int other = 0;cout << "Enter words (q to quit):\n";cin >> word;while ( word != "q"){ch = tolower(word[0]);if (isalpha(ch)){if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u')vowel++;elseconsonant++;}elseother++;cin >> word;}cout << vowel <<" words beginning with vowels\n";cout << consonant << " words beginning with consonants\n";cout << other << " others\n";return 0;}// pe6-8.cpp -- counting characters#include <iostream>#include <fstream> // file I/O suppport#include <cstdlib> // support for exit()const int SIZE = 60;int main(){using namespace std;char filename[SIZE];char ch;ifstream inFile; // object for handling file inputcout << "Enter name of data file: ";cin.getline(filename, SIZE);inFile.open(filename); // associate inFile with a fileif (!inFile.is_open()) // failed to open file{cout << "Could not open the file " << filename << endl;cout << "Program terminating.\n";exit(EXIT_FAILURE);}int count = 0; // number of items readinFile >> ch; // get first valuewhile (inFile.good()) // while input good and not at EOF {count++; // one more item readinFile >> ch; // get next value}cout << count << " characters in " << filename << endl;inFile.close(); // finished with the filereturn 0;}Chapter 7//pe7-1.cpp -- harmonic mean#include <iostream>double h_mean(double x, double y);int main(void){using namespace std;double x,y;cout << "Enter two numbers (a 0 terminates): ";while (cin >> x >> y && x * y != 0)cout << "harmonic mean of " << x << " and "<< y << " = " << h_mean(x,y) << "\n";/* or do the reading and testing in two parts:while (cin >> x && x != 0){cin >> y;if (y == 0)break;...*/cout << "Bye\n";return 0;}double h_mean(double x, double y){return 2.0 * x * y / (x + y);}// pe7-3.cpp#include <iostream>struct box {char maker[40];float height;float width;float length;float volume;};void showbox(box b);void setbox(box * pb);int main(void){box carton = {"Bingo Boxer", 2, 3, 5}; // no volume providedsetbox(&carton);showbox(carton);return 0;}void showbox(box b){using namespace std;cout << "Box maker: " << b.maker<< "\nheight: " << b.height<< "\nlwidth: " << b.width<< "\nlength: " << b.length<< "\nvolume: " << b.volume << "\n";}void setbox(box * pb){pb->volume = pb->height * pb->width * pb->length;}// pe7-4.cpp -- probability of winning#include <iostream>long double probability(unsigned numbers, unsigned picks);int main(){using namespace std;double total, choices;double mtotal;double probability1, probability2;cout << "Enter total number of game card choices and\n""number of picks allowed for the field:\n";while ((cin >> total >> choices) && choices <= total){cout << "Enter total number of game card choices ""for the mega number:\n";if (!(cin >> mtotal))break;cout << "The chances of getting all " << choices << " picks is one in "<< (probability1 = probability(total, choices) ) << ".\n";cout << "The chances of getting the megaspot is one in "<< (probability2 = probability(mtotal, 1) ) << ".\n";cout << "You have one chance in ";cout << probability1 * probability2; // compute the probability cout << " of winning.\n";cout << "Next set of numbers (q to quit): ";}cout << "bye\n";return 0;}// the following function calculates the probability of picking picks// numbers correctly from numbers choiceslong double probability(unsigned numbers, unsigned picks){long double result = 1.0; // here come some local variableslong double n;unsigned p;for (n = numbers, p = picks; p > 0; n--, p--)result = result * n / p ;return result;}// pe7-6.cpp#include <iostream>int Fill_array(double ar[], int size);void Show_array(const double ar[], int size);void Reverse_array(double ar[], int size);const int LIMIT = 10;int main( ){using namespace std;double values[LIMIT];int entries = Fill_array(values, LIMIT);cout << "Array values:\n";Show_array(values, entries);cout << "Array reversed:\n";Reverse_array(values, entries);Show_array(values, entries);cout << "All but end values reversed:\n";Reverse_array(values + 1, entries - 2);Show_array(values, entries);return 0;}int Fill_array(double ar[], int size){using namespace std;int n;cout << "Enter up to " << size << " values (q to quit):\n"; for (n = 0; n < size; n++){cin >> ar[n];if (!cin)break;}return n;}void Show_array(const double ar[], int size){using namespace std;int n;for (n = 0; n < size; n++){cout << ar[n];if (n % 8 == 7)cout << endl;elsecout << ' ';}if (n % 8 != 0)cout << endl;}void Reverse_array(double ar[], int size){int i, j;double temp;for (i = 0, j = size - 1; i < j; i++, j--){temp = ar[i];ar[i] = ar[j];ar[j] = temp;}}//pe7-9.cpp#include <iostream>double calculate(double x, double y, double (*pf)(double, double)); double add(double x, double y);double sub(double x, double y);double mean(double x, double y);int main(void){using namespace std;double (*pf[3])(double,double) = {add, sub, mean};char * op[3] = {"sum", "difference", "mean"};double a, b;cout << "Enter pairs of numbers (q to quit): ";int i;while (cin >> a >> b){// using function namescout << calculate(a, b, add) << " = sum\n";cout << calculate(a, b, mean) << " = mean\n";// using pointersfor (i = 0; i < 3; i++)cout << calculate(a, b, pf[i]) << " = "<< op[i] << "\n";}cout << "Done!\n";return 0;}double calculate(double x, double y, double (*pf)(double, double)) {return (*pf)(x, y);}double add(double x, double y){return x + y;}double sub(double x, double y){return x - y;}double mean(double x, double y){return (x + y) / 2.0;}Chapter 8// pe8-1.cpp#include <iostream>void silly(const char * s, int n = 0);int main(void){using namespace std;char * p1 = "Why me?\n";silly(p1);for (int i = 0; i < 3; i++){cout << i << " = i\n";silly(p1, i);}cout << "Done\n";return 0;}void silly(const char * s, int n){using namespace std;static int uses = 0;int lim = ++uses;if (n == 0)lim = 1;for (int i = 0; i < lim; i++)cout << s;}// pe8-4.cpp#include <iostream>#include <cstring> // for strlen(), strcpy()using namespace std;struct stringy {char * str; // points to a stringint ct; // length of string (not counting '\0')};void show(const char *str, int cnt = 1);void show(const stringy & bny, int cnt = 1);void set(stringy & bny, const char * str);int main(void){stringy beany;char testing[] = "Reality isn't what it used to be.";set(beany, testing); // first argument is a reference, // allocates space to hold copy of testing,// sets str member of beany to point to the// new block, copies testing to new block,// and sets ct member of beanyshow(beany); // prints member string onceshow(beany, 2); // prints member string twicetesting[0] = 'D';testing[1] = 'u';show(testing); // prints testing string onceshow(testing, 3); // prints testing string thrice show("Done!");return 0;}void show(const char *str, int cnt){while(cnt-- > 0){cout << str << endl;}}void show(const stringy & bny, int cnt){while(cnt-- > 0){cout << bny.str << endl;}}void set(stringy & bny, const char * str){bny.ct = strlen(str);bny.str = new char[bny.ct+1];strcpy(bny.str, str);}// pe8-5.cpp#include <iostream>template <class T>T max5(T ar[]){int n;T max = ar[0];for (n = 1; n < 5; n++)if (ar[n] > max)max = ar[n];return max;}const int LIMIT = 5;int main( ){using namespace std;double ard[LIMIT] = { -3.4, 8.1, -76.4, 34.4, 2.4}; int ari[LIMIT] = {2, 3, 8, 1, 9};double md;int mi;md = max5(ard);mi = max5(ari);cout << "md = " << md << endl;cout << "mi = " << mi << endl;return 0;}Chapter 9PE 9-1// pe9-golf.h - for pe9-1.cppconst int Len = 40;struct golf{char fullname[Len];int handicap;};// non-interactive version// function sets golf structure to provided name, handicap// using values passed as arguments to the functionvoid setgolf(golf & g, const char * name, int hc);// interactive version// function solicits name and handicap from user// and sets the members of g to the values entered// returns 1 if name is entered, 0 if name is empty stringint setgolf(golf & g);// function resets handicap to new valuevoid handicap(golf & g, int hc);// function displays contents of golf structurevoid showgolf(const golf & g);// pe9-golf.cpp - for pe9-1.cpp#include <iostream>#include "pe9-golf.h"#include <cstring>// function solicits name and handicap from user// returns 1 if name is entered, 0 if name is empty stringint setgolf(golf & g){std::cout << "Please enter golfer's full name: ";std::cin.getline(g.fullname, Len);if (g.fullname[0] == '\0')return 0; // premature terminationstd::cout << "Please enter handicap for " << g.fullname << ": "; while (!(std::cin >> g.handicap)){std::cin.clear();std::cout << "Please enter an integer: ";}while (std::cin.get() != '\n')continue;return 1;}// function sets golf structure to provided name, handicapvoid setgolf(golf & g, const char * name, int hc){std::strcpy(g.fullname, name);g.handicap = hc;}// function resets handicap to new valuevoid handicap(golf & g, int hc){g.handicap = hc;}// function displays contents of golf structurevoid showgolf(const golf & g){std::cout << "Golfer: " << g.fullname << "\n";std::cout << "Handicap: " << g.handicap << "\n\n";}// pe9-1.cpp#include <iostream>#include "pe9-golf.h"// link with pe9-golf.cppconst int Mems = 5;int main(void){using namespace std;golf team[Mems];cout << "Enter up to " << Mems << " golf team members:\n";int i;for (i = 0; i < Mems; i++)if (setgolf(team[i]) == 0)break;for (int j = 0; j < i; j++)showgolf(team[j]);setgolf(team[0], "Fred Norman", 5);showgolf(team[0]);handicap(team[0], 3);showgolf(team[0]);return 0;}PE 9-3//pe9-3.cpp -- using placement new#include <iostream>#include <new>#include <cstring>struct chaff{char dross[20];int slag;};// char buffer[500]; // option 1int main(){using std::cout;using std::endl;chaff *p1;int i;char * buffer = new char [500]; // option 2p1 = new (buffer) chaff[2]; // place structures in buffer std::strcpy(p1[0].dross, "Horse Feathers");p1[0].slag = 13;std::strcpy(p1[1].dross, "Piffle");p1[1].slag = -39;for (i = 0; i < 2; i++)cout << p1[i].dross << ": " << p1[i].slag << endl;delete [] buffer; // option 2return 0;}Chapter 10PE 10-1// pe10-1.cpp#include <iostream>#include <cstring>// class declarationclass BankAccount{private:char name[40];char acctnum[25];double balance;public:BankAccount(char * client = "no one", char * num = "0",double bal = 0.0); void show(void) const; void deposit(double cash); void withdraw(double cash); };// method definitionsBankAccount::BankAccount(char * client, char * num, double bal) {std::strncpy(name, client, 39);name[39] = '\0';std::strncpy(acctnum, num, 24);acctnum[24] = '\0';balance = bal;}void BankAccount::show(void) const{using std::cout;using std:: endl;cout << "Client: " << name << endl;cout << "Account Number: " << acctnum << endl;cout << "Balance: " << balance << endl;}void BankAccount::deposit(double cash){if (cash >= 0)balance += cash;elsestd::cout << "Illegal transaction attempted";}void BankAccount::withdraw(double cash){if (cash < 0)std::cout << "Illegal transaction attempted";else if (cash <= balance)balance -=cash;elsestd::cout << "Request denied due to insufficient funds.\n"; }// sample useint main(){BankAccount bird;BankAccount frog("Kermit", "croak322", 123.00);bird.show();frog.show();bird = BankAccount("Chipper", "peep8282", 214.00);bird.show();frog.deposit(20);frog.show();frog.withdraw(4000);frog.show();frog.withdraw(50);frog.show();}PE10-4// pe10-4.h#ifndef SALES__#define SALES__namespace SALES{const int QUARTERS = 4;class Sales{private:double sales[QUARTERS];double average;double max;double min;public:// default constructorSales();// copies the lesser of 4 or n items from the array ar// to the sales member and computes and stores the// average, maximum, and minimum values of the entered items;// remaining elements of sales, if any, set to 0Sales(const double ar[], int n);// gathers sales for 4 quarters interactively, stores them// in the sales member of object and computes and stores the// average, maximum, and minumum valuesvoid setSales();// display all information in objectvoid showSales();};}#endif。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
Chapter 5
PE 5-1
/* Programming Exercise 5-1 */ #include <stdio.h> int main(void) { const int minperhour = 60; int minutes, hours, mins; printf("Enter the number of minutes to convert: "); scanf("%d", &minutes); while (minutes > 0 ) { hours = minutes / minperhour; mins = minutes % minperhour; printf("%d minutes = %d hours, %d minutes\n", minutes, hours, mins); printf("Enter next minutes value (0 to quit): "); scanf("%d", &minutes); } printf("Bye\n"); return 0; }
August 21, 1999
Answers For Programming Exercises in C Primer Plus, 5rd Edition, by Stephen Prata
return 0; } void jolly(void) { printf("For he's a jolly good fellow!\n"); } void deny(void) { printf("Which nobody can deny!\n"); }
printf("Enter the number of quarts of water: "); scanf("%f", &quarts); molecules = quarts * mass_qt / mass_mol; printf("%f quarts of water contain %e molecules.\n", quarts, molecules); return 0; }
PE 2-5
/* Programming Exercise 2-5 #include <stdio.h> int main(void) { int toes; toes = 10; printf("toes = %d\n", toes); printf("Twice toes = %d\n", 2 * toes); printf("toes squared = %d\n", toes * toes); return 0; } /* or create two more variables, set them to 2 * toes and toes * toes */ */
PE 2-3
/* Programming Exercise 2-3 #include <stdio.h> */
int main(void) { int ageyears; /* age in years */ int agedays; /* age in days */ /* large ages may require the long type */ ageyears = 44; agedays = 365 * ageyears; printf("An age of %d years is %d days.\n", ageyears, agedays); return 0; }
PE 2-7
/* Programming Exercise 2-7 #include <stdio.h> void one_three(void); void two(void); int main(void) { printf("starting now:\n"); one_three(); printf("done!\n"); return 0; } void one_three(void) { printf("one\n"); two(); printf("three\n"); */
PE 3-2
/* Programming Exercise 3-2 #include <stdio.h> int main(void) { int ascii; printf("Enter an ASCII code: "); scanf("%d", &ascii); printf("%d is the ASCII code for %c.\n", ascii, ascii); return 0; } */
PE 2-4
/* Programming Exercise 2-4 #include <stdio.h> void jolly(void); void deny(void); int main(void) { jolly(); jolly(); jolly(); deny(); */
sp
Page 1 of 86
sp
Page 5 of 86
August 21, 1999
Answers For Programming Exercises in C Primer Plus, 5rd Edition, by Stephen Prata
PE 5-3
/* Programming Exercise 5-3 */ #include <stdio.h> int main(void) { const int daysperweek = 7; int days, weeks, day_rem; printf("Enter the number of days: "); scanf("%d", &days); weeks = days / daysperweek; day_rem = days % daysperweek; printf("%d days are %d weeks and %d days.\n", days, weeks, day_rem); return 0; }
sp
Page 2 of 86
August 21, 1999
Answers For Programming Exercises in C Primer Plus, 5rd Edition, by Stephen Prata
} void two(void) { printf("two\n"); }
Chபைடு நூலகம்pter 3
PE 5-5
/* Programming Exercise 5-5 */ #include <stdio.h> int main(void) /* finds sum of first n integers */ { int count, sum; int n; printf("Enter the upper limit: "); scanf("%d", &n); count = 0; sum = 0; while (count++ < n) sum = sum + count; printf("sum = %d\n", sum); return 0; }
Chapter 4
PE 4-1
/* Programming Exercise 4-1 #include <stdio.h> int main(void) { char fname[40]; char lname[40]; printf("Enter your first name: "); scanf("%s", fname); printf("Enter your last name: "); scanf("%s", lname); printf("%s, %s\n", lname, fname); return 0; } */
PE 4-4
/* Programming Exercise 4-4 */ #include <stdio.h> int main(void) { float height; char name[40]; printf("Enter your height in inches: "); scanf("%f", &height); printf("Enter your name: "); scanf("%s", name); printf("%s, you are %.3f feet tall\n", name, height / 12.0); return 0;
float float float float mass_mol = 3.0e-23; mass_qt = 950; quarts; molecules; /* mass of water molecule in grams */ /* mass of quart of water in grams */
Answers For Programming Exercises in C Primer Plus, 5rd Edition, by Stephen Prata
Chapter 2
PE 2-1
/* Programming Exercise 2-1 #include <stdio.h> */