计算机图形学实验C++代码
计算机图形学实习全部代码 View调用

// GraphicsView.cpp : CGraphicsView 类的实现//#include "stdafx.h"// SHARED_HANDLERS 可以在实现预览、缩略图和搜索筛选器句柄的// ATL 项目中进行定义,并允许与该项目共享文档代码。
#ifndef SHARED_HANDLERS#include "Graphics.h"#endif#include "GraphicsDoc.h"#include "GraphicsView.h"#include <cmath>#ifdef _DEBUG#define new DEBUG_NEW#endif// CGraphicsViewIMPLEMENT_DYNCREATE(CGraphicsView, CView)BEGIN_MESSAGE_MAP(CGraphicsView, CView)// 标准打印命令ON_COMMAND(ID_FILE_PRINT, &CView::OnFilePrint)ON_COMMAND(ID_FILE_PRINT_DIRECT, &CView::OnFilePrint)ON_COMMAND(ID_FILE_PRINT_PREVIEW, &CGraphicsView::OnFilePrintPreview) ON_WM_CONTEXTMENU()ON_WM_RBUTTONUP()ON_COMMAND(ID_32771, &CGraphicsView::OnDDA)ON_COMMAND(ID_32772, &CGraphicsView::OnMidline)ON_COMMAND(ID_32773, &CGraphicsView::On32773)ON_COMMAND(ID_32774, &CGraphicsView::OncircleMid)ON_COMMAND(ID_32775, &CGraphicsView::ONddacircle)ON_COMMAND(ID_32776, &CGraphicsView::OnBresenhamcircle)ON_COMMAND(ID_32777, &CGraphicsView::OnMidEllipse)ON_COMMAND(ID_32778, &CGraphicsView::Onlugrange)ON_COMMAND(ID_32779, &CGraphicsView::OnScanLineseed)ON_COMMAND(ID_32780, &CGraphicsView::Ontranslation)ON_COMMAND(ID_32781, &CGraphicsView::Onrotation)ON_COMMAND(ID_32782, &CGraphicsView::Onscaling)ON_COMMAND(ID_32783, &CGraphicsView::OnBezier)ON_COMMAND(ID_32784, &CGraphicsView::OnSeedfilledALg) END_MESSAGE_MAP()// CGraphicsView 构造/析构CGraphicsView::CGraphicsView(){// TODO: 在此处添加构造代码}CGraphicsView::~CGraphicsView(){}BOOL CGraphicsView::PreCreateWindow(CREATESTRUCT& cs){// TODO: 在此处通过修改// CREATESTRUCT cs 来修改窗口类或样式return CView::PreCreateWindow(cs);}// CGraphicsView 绘制//Bezier曲线的混合函数////////////double b03(double t)//{return(pow(1-t,3)); }//double b13(double t)//{return(3*t*pow(1-t,2));}////double b23(double t)//{return(3*(1-t)*t*t);}////double b33(double t)//{return(t*t*t);}#include "SeqStack.h"void CGraphicsView::OnDraw(CDC*pDC){CGraphicsDoc* pDoc = GetDocument();ASSERT_VALID(pDoc);if (!pDoc)return;//pDC->MoveTo(100,100);//pDC->LineTo(300,300);// pDC->MoveTo(300,300);//pDC->LineTo(200,300);// pDC->MoveTo(200,300);//pDC->LineTo(100,100);//// TODO: 在此处为本机数据添加绘制代码// SeqStack<POINT> sta;// POINT s[100000];//int i=0;// s[i].x=150;//s[i].y=160;//int m,n;//sta.Push(s[i]);//起始点入栈//// while(!sta.IsEmpty())// {// sta.Pop(s[i]);// m=s[i].x;// n=s[i].y;// if(pDC->GetPixel(m,n)!=RGB(0,0,0) )// {// pDC->SetPixel(m,n,RGB(0,0,0));// i++;// }// if(pDC->GetPixel(m+1,n)!=RGB(0,0,0) ) // {// s[i].x=m+1;// s[i].y=n;// sta.Push(s[i]);// i++;// }// if(pDC->GetPixel(m-1,n)!=RGB(0,0,0))// {// s[i].x=m-1;// s[i].y=n;// sta.Push(s[i]);// i++;// }// if(pDC->GetPixel(m,n+1)!=RGB(0,0,0) )// {// s[i].x=m;// s[i].y=n+1;// sta.Push(s[i]);// i++;// }// if(pDC->GetPixel(m,n-1)!=RGB(0,0,0))// {// s[i].x=m;// s[i].y=n-1;// sta.Push(s[i]);// }//}}// CGraphicsView 打印void CGraphicsView::OnFilePrintPreview(){#ifndef SHARED_HANDLERSAFXPrintPreview(this);#endif}BOOL CGraphicsView::OnPreparePrinting(CPrintInfo* pInfo){// 默认准备return DoPreparePrinting(pInfo);}void CGraphicsView::OnBeginPrinting(CDC* /*pDC*/, CPrintInfo* /*pInfo*/) {// TODO: 添加额外的打印前进行的初始化过程}void CGraphicsView::OnEndPrinting(CDC* /*pDC*/, CPrintInfo* /*pInfo*/) {// TODO: 添加打印后进行的清理过程}void CGraphicsView::OnRButtonUp(UINT /* nFlags */, CPoint point){ClientToScreen(&point);OnContextMenu(this, point);}void CGraphicsView::OnContextMenu(CWnd* /* pWnd */, CPoint point){#ifndef SHARED_HANDLERStheApp.GetContextMenuManager()->ShowPopupMenu(IDR_POPUP_EDIT, point.x, point.y, this, TRUE);#endif}// CGraphicsView 诊断#ifdef _DEBUGvoid CGraphicsView::AssertValid() const{CView::AssertValid();}void CGraphicsView::Dump(CDumpContext& dc) const{CView::Dump(dc);}CGraphicsDoc* CGraphicsView::GetDocument() const // 非调试版本是内联的{ASSERT(m_pDocument->IsKindOf(RUNTIME_CLASS(CGraphicsDoc)));return (CGraphicsDoc*)m_pDocument;}#endif //_DEBUG// CGraphicsView 消息处理程序void CGraphicsView::OnDDA(){CDC* pDC = GetDC();Geo obj(pDC);pDC-> SetMapMode(MM_LOMETRIC); //设置坐标系pDC-> SetViewportOrg(100,100);obj.DDA(100,100,200,200,pDC);ReleaseDC(pDC);// TODO: 在此添加命令处理程序代码}void CGraphicsView::OnMidline(){CDC* pDC = GetDC();Geo obj(pDC);pDC-> SetMapMode(MM_LOMETRIC); //设置坐标系pDC-> SetViewportOrg(0,100);obj.mpline(100,100,200,200,pDC);ReleaseDC(pDC);// TODO: 在此添加命令处理程序代码}void CGraphicsView::On32773(){CDC* pDC = GetDC();Geo obj(pDC);pDC-> SetMapMode(MM_LOMETRIC);//该函数设置指定设备环境的映射方式,映射方式定义了将逻辑单位转换为设备单位的度量单位,并定义了设备的X、Y轴的方向pDC-> SetViewportOrg(500,200);//pDC->SetWindowOrg(100,100);obj.Bresenham(100,100,200,200,pDC);ReleaseDC(pDC);// TODO: 在此添加命令处理程序代码}void CGraphicsView::OncircleMid(){CDC* pDC = GetDC();Geo obj(pDC);pDC-> SetMapMode(MM_LOMETRIC);pDC-> SetViewportOrg(500,200);obj.circleMidpoint(10,100,300,pDC);ReleaseDC(pDC);// TODO: 在此添加命令处理程序代码}////////////////dda画圆算法//////////////////////// void CGraphicsView::ONddacircle(){// TODO: 在此添加命令处理程序代码CDC* pDC = GetDC();Geo obj(pDC);pDC-> SetMapMode(MM_LOMETRIC);pDC-> SetViewportOrg(500,200);obj.ddacircle(10,100,500,pDC);ReleaseDC(pDC);}void CGraphicsView::OnBresenhamcircle(){// TODO: 在此添加命令处理程序代码CDC* pDC = GetDC();Geo obj(pDC);pDC-> SetMapMode(MM_LOMETRIC);pDC-> SetViewportOrg(500,200);obj.Bresenham_Circle(100,pDC);ReleaseDC(pDC);}void CGraphicsView::OnMidEllipse(){// TODO: 在此添加命令处理程序代码CDC* pDC = GetDC();Geo obj(pDC);pDC-> SetMapMode(MM_LOMETRIC);pDC-> SetViewportOrg(500,200);obj.ellipseMidpoint( 20,100,100, 200,pDC);ReleaseDC(pDC);}void CGraphicsView::Onlugrange(){// TODO: 在此添加命令处理程序代码CDC* pDC = GetDC();Geo obj(pDC);pDC-> SetMapMode(MM_LOMETRIC);pDC-> SetViewportOrg(500,200);int xa[4]={100,50,280,400};int ya[4]={100,150,280,400};int za[4]={100,150,280,400};int k=4,l_section=40;obj.Lugrange( xa, ya,za, k, l_section,pDC);ReleaseDC(pDC);}void CGraphicsView::OnScanLineseed(){// TODO: 在此添加命令处理程序代码CDC*pDC=GetDC();Geo obj(pDC);/*pDC-> SetMapMode(MM_LOMETRIC);pDC-> SetViewportOrg(500,400);*///obj.ScanLineSeedFill(150,160,pDC);//obj.ScanLineSeedFill(400,600-200,pDC);obj.ScanLineSeedFill(101,200,pDC);ReleaseDC(pDC);}void CGraphicsView::Ontranslation(){// TODO: 在此添加命令处理程序代码CDC* pDC = GetDC();Geo obj(pDC);obj.Algorithm1(100,100,200,200,RGB(255,0,0),pDC);obj.Algorithm2(100,100,200,200,RGB(255,0,0),pDC);ReleaseDC(pDC);}void CGraphicsView::Onrotation(){// TODO: 在此添加命令处理程序代码CDC* pDC = GetDC();Geo obj(pDC);obj.Algorithm3(100,100,200,200,RGB(255,0,0),pDC);ReleaseDC(pDC);}void CGraphicsView::Onscaling(){// TODO: 在此添加命令处理程序代码CDC* pDC = GetDC();Geo obj(pDC);obj.Algorithm4(100,100,200,200,RGB(255,0,0),pDC);ReleaseDC(pDC);}void CGraphicsView::OnBezier(){// TODO: 在此添加命令处理程序代码CDC *pDC=GetDC();pDC-> SetMapMode(MM_LOMETRIC);pDC-> SetViewportOrg(150,600);Geo obj(pDC);obj.Bezier(pDC);ReleaseDC(pDC);}void CGraphicsView::OnSeedfilledALg(){// TODO: 在此添加命令处理程序代码CDC *pDC=GetDC();//pDC-> SetMapMode(MM_LOMETRIC);//pDC-> SetViewportOrg(150,600);Geo obj(pDC);obj.SeedFiled(pDC);ReleaseDC(pDC);}。
计算机图形学利用C语言图形函数绘图
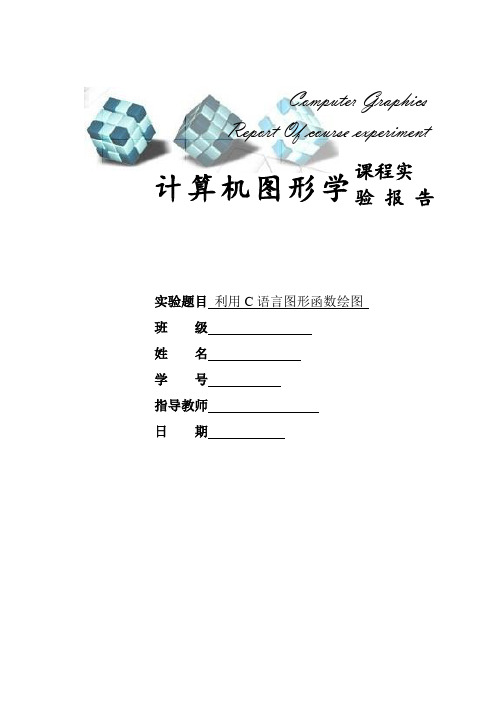
计算机图形学课程实验 报 告实验题目 利用C 语言图形函数绘图 班 级 姓 名 学 号 指导教师 日 期Computer GraphicsReport Of course experiment-图形学课程实验报告-- 1 -实验说明试验目的: 掌握TurboC 语言图形函数的使用和学会绘制一般图形。
试验地点: 教九楼401 数学系机房实验要求(Direction ):1. 每个学生单独完成;2.开发语言为TurboC 或C++,也可使用其它语言;3.请在自己的实验报告上写明姓名、学号、班级;4.每次交的实验报告内容包括:题目、试验目的和意义、程序制作步骤、主程序、运行结果图以及参考文件;5. 自己保留一份可执行程序,考试前统一检查和上交。
实验内容实验题一1.1实验题目用如下图1所示,图中最大正n 边形的外接圆半径为R ,旋转该正n 边形,每次旋转θ角度,旋转后的的n 边形顶点落在前一个正六边形的边上,共旋转N 次,请上机编程绘制N+1个外接圆半径逐渐缩小且旋转的正n 边形。
要求:(1) n 、R 、N 、θ要求可以人为自由控制输入;(2)N+1个正六边形的中心(即外接圆的圆心)在显示屏幕中心。
1.2实验目的和意义1. 了解如何利用C 语言和图形函数进行绘图;2. 熟悉并掌握C 语言的图形模式控制函数,图形屏幕操作函数,以及基本图形函数;CB AR1R210图1利用C 语言图形函数绘图实验1如左图(一)所示:n=6 θ=100、N =1、R=R1、旋转一次。
依次类推,共旋转N 次。
3.通过对Turbo C进行图形程序设计的基本方法的学习,能绘制出简单的图形;4. 通过绘制N+1个正n边形,了解图形系统初始化、图形系统关闭和图形模式的控制并熟练运用图形坐标的设置,包括定点、读取光标、读取x和y轴的最大值以及图形颜色的设置。
1.3程序制作步骤(包括算法思想、算法流程图等)1.自动搜索显示器类型和显示模式,初始化图形系统,通过printf、scanf语句控制半径r、边数n、多边形的个数k、边的每次旋转角度d,的自由输入;2.给定一内接圆半径r,由圆内接多边形的算法公式:x[i]=r*cos((i+1) *2.0*pi/n)+320.0y[i]=240.0-r*sin(2.0*pi/n *(i+1))确定出多边形N的各个顶点坐标,然后利用划线函数line(),连接相邻两点,即形成一个正多边形。
计算机图形学 使用VC开发绘图程序的基本方法

七、思考题(尽量做,计入成绩) :
1、为什么要用 CDC 定义一个对象来作图? Windows 使用与设备无关的图形设备环境进行显示 。MFC 基础类库定义了设备环境对象类----CDC 类。 因为使用的是 mfc,如果是 sdk 的话就得用 dc,这个 cdc 想当是一个给设备画画的笔。 2、如果要绘制多条直线,应该如何编程?
4、 VC 中编程环境中,工程(project)是什么意义?为什么要用 project 的方式管理代码?
Project 文件的扩展名是 dsp,这个文件中存放的是一个特定的工程,也就是特定的应用程序的有关信息, 每个工程都对应有一个 dsp 类型的文件。 clw 为扩展名的文件是用来存放应用程序中用到的类和资源的信 以 息的,这些信息是 VC 中的 ClassWizard 工具管理和使用类的信息来源。 因为用 project 的方式管理代码比较方便,可以看成是在一个文件夹下进行的。
3、 在 MyView.cpp 文件的 OnDraw 函数中加入如下代码。 pDC->MoveTo(100,100); pDC->LineTo(200,200); CPen *pOldPen; CPen dashPen; dashPen.CreatePen(PS_DASH,1,RGB(255,0,0)); pOldPen = pDC->SelectObject(&dashPen); pDC->LineTo(300,100); pDC->SelectObject(pOldPen); pDC->LineTo(400,200);
实验(No. 1
)题目:使用 VC 开发绘图程序的基本方法
实验目的及要求:
一、实验目的:
编写一个具有一定功能的 MFC 类库应用程序 (引入简单的视图/文档概念) 掌握 GDI 画图的基本方法, , 并调用系统函数画直线。
计算机图形学实验(全)

实验1 直线的绘制实验目的1、通过实验,进一步理解和掌握DDA和Bresenham算法;2、掌握以上算法生成直线段的基本过程;3、通过编程,会在TC环境下完成用DDA或中点算法实现直线段的绘制。
实验环境计算机、Turbo C或其他C语言程序设计环境实验学时2学时,必做实验。
实验内容用DDA算法或Besenham算法实现斜率k在0和1之间的直线段的绘制。
实验步骤1、算法、原理清晰,有详细的设计步骤;2、依据算法、步骤或程序流程图,用C语言编写源程序;3、编辑源程序并进行调试;4、进行运行测试,并结合情况进行调整;5、对运行结果进行保存与分析;6、把源程序以文件的形式提交;7、按格式书写实验报告。
实验代码:DDA:# include <graphics.h># include <math.h>void DDALine(int x0,int y0,int x1,int y1,int color){int dx,dy,epsl,k;float x,y,xIncre,yIncre;dx=x1-x0;dy=y1-y0;x=x0;y=y0;if(abs(dx)>abs(dy))epsl=abs(dx);elseepsl=abs(dy);xIncre=(float)dx/(float)epsl;yIncre=(float)dy/(float)epsl;for(k=0;k<=epsl;k++){putpixel((int)(x+0.5),(int)(y+0.5),4);x+=xIncre;y+=yIncre;}}main(){int gdriver ,gmode ;gdriver = DETECT;initgraph(&gdriver , &gmode ,"C:\\TC20\\BGI");DDALine(0,0,35,26,4);getch ( );closegraph ( );}Bresenham:#include<graphics.h>#include<math.h>void BresenhamLine(int x0,int y0,int x1,int y1,int color) {int x,y,dx,dy,e;dx=x1-x0;dy=y1-y0;e=-dx;x=x0;y=y0;while(x<=x1){putpixel(x,y,color);x++;e=e+2*dy;if(e>0){y++;e=e-2*dx;}}}main(){int gdriver ,gmode ;gdriver = DETECT;initgraph(&gdriver , &gmode ,"c:\\TC20\\BGI");BresenhamLine(0, 0 , 120, 200,5 );getch ( );closegraph ( );}实验2 圆和椭圆的绘制实验目的1、通过实验,进一步理解和掌握中点算法;2、掌握以上算法生成椭圆或圆的基本过程;3、通过编程,会在TC环境下完成用中点算法实现椭圆或圆的绘制。
图形学三视图、正轴测投影c#代码

实验5三维图形生成三视图、正轴测投影实验目的:掌握第5章所讲述的三维图形的变换和生成。
实验内容:E5 绘制所示图形的Form1.csusing System;using System.Collections.Generic;using ponentModel;using System.Data;using System.Drawing;using System.Text;using System.Windows.Forms;namespace E5{//!!说明:主视图有两点连线不对,应该是坐标连线的问题,但连线太多所以没找到是哪两点连错。
public partial class Form1 : Form{int x0 = 150;int z0 = -150;double c = Math.Cos(Math.PI / 2);double s = Math.Sin(Math.PI / 2);double c1 = Math.Cos(Math.PI / 4);double s1 = Math.Sin(Math.PI / 4);Matrix[] p3d = new Matrix[20];//三维顶点Matrix[] p3dv = new Matrix[20];//主视图顶点Matrix[] p3dw = new Matrix[20];//侧视图顶点Matrix[] p3dh = new Matrix[20];//俯视图顶点Matrix[] p3dc = new Matrix[20];//正轴侧顶点/* double[,] p = new double[17,4]{{0,0,42,1},{55,0,42,1},{55,0,10,1},{70,0,10,1},{70,0,0,1},{70,40,0,1},{0,40,0,1},{0,40,27,1},{0,30,27,1},{0,30,42,1},{10,30,42,1},{10,10,42,1},{55,10,42,1},{55,10,10,1},{30,10,10,1},{30,40,10,1},{20,40,27,1},{20,30,27,1},{70,40,10,1}}; */void Getp3d(ref Matrix[] p3d)//三维顶点赋值{for(int i=0;i<19;i++){p3d[i]=new Matrix(1,4);//三维顶点}p3d[0].Data = new double[1, 4] { {0,0,42,1} };p3d[1].Data = new double[1, 4] { { 55, 0, 42, 1 } }; p3d[2].Data = new double[1, 4] { { 55, 0, 10, 1 } }; p3d[3].Data = new double[1, 4] { { 70, 0, 10, 1 } }; p3d[4].Data = new double[1, 4] { { 70, 0, 0, 1 } };p3d[5].Data = new double[1, 4] { { 70, 40, 0, 1 } }; p3d[6].Data = new double[1, 4] { { 0, 40, 0, 1 } };p3d[7].Data = new double[1, 4] { { 0, 40, 27, 1 } }; p3d[8].Data = new double[1, 4] { { 0, 30, 27, 1 } }; p3d[9].Data = new double[1, 4] { { 0, 30, 42, 1 } }; p3d[10].Data = new double[1, 4] { { 10, 30, 42, 1 } }; p3d[11].Data = new double[1, 4] { { 10, 10, 42, 1 } }; p3d[12].Data = new double[1, 4] { { 55, 10, 42, 1 } }; p3d[13].Data = new double[1, 4] { { 55, 10, 10, 1 } }; p3d[14].Data = new double[1, 4] { { 30, 10, 10, 1 } }; p3d[15].Data = new double[1, 4] { { 30, 40, 10, 1 } }; p3d[16].Data = new double[1, 4] { { 20, 40, 27, 1 } }; p3d[17].Data = new double[1, 4] { { 20, 30, 27, 1 } }; p3d[18].Data = new double[1, 4] { { 70, 40, 10, 1 } };}public Form1(){InitializeComponent();}private void Form1_Paint(object sender, PaintEventArgs e){Getp3d(ref p3d);//主视图矩阵Matrix Tv = new Matrix(4);Tv.Data = new double[4,4]{{1 , 0 , 0 , 0},{0 , 0 , 0 , 0},{0 , 0 , 1 , 0},{0 , 0 , 0 , 1}};//侧视图Matrix Tw1 = new Matrix(4); Tw1.Data = new double[4, 4] {{0 , 0 , 0 , 0},{0 , 1 , 0 , 0},{0 , 0 , 1 , 0},{0 , 0 , 0 , 1}};Matrix Tw2 = new Matrix(4); Tw2.Data = new double[4, 4] {{c , s , 0 , 0},{-s , c , 0 , 0},{0 , 0 , 1 , 0},{0 , 0 , 0 , 1}};Matrix Tw3 = new Matrix(4); Tw3.Data = new double[4, 4] {{1 , 0 , 0 , 0},{0 , 1 , 0 , 0},{0 , 0 , 1 , 0},{x0 , 0 , 0 , 1}};//俯视图//俯视图的投影变换Matrix Th1 = new Matrix(4); Th1.Data = new double[4, 4] {{1 , 0 , 0 , 0},{0 , 1 , 0 , 0},{0 , 0 , 0 , 0},{0 , 0 , 0 , 1}};//使H面绕X轴负向旋转90°Matrix Th2 = new Matrix(4);Th2.Data = new double[4, 4]{{1 , 0 , 0 , 0},{0 , c , -s , 0},{0 , s , c , 0},{0 , 0 , 0 , 1}};//使H面沿Z轴负向平移一段距离z0Matrix Th3 = new Matrix(4);Th3.Data = new double[4, 4]{{1 , 0 , 0 , 0},{0 , 1 , 0 , 0},{0 , 0 , 1 , 0},{0 , 0 , -z0,1}};//正轴侧// 先绕Z轴正向旋转α角//再绕X轴反向旋转β角//将三维形体向XOZ平面(V面)作正投影得到正轴测投影的投影变换矩阵Matrix Tc1 = new Matrix(4);Tc1.Data = new double[4, 4]{{c1 , s1 , 0 , 0},{-s1 , c1 , 0 , 0},{0 , 0 , 0 , 0},{0 , 0 , 0 , 1}};Matrix Tc2 = new Matrix(4);Tc2.Data = new double[4, 4]{{1 , 0 , 0 , 0},{0 , c1 , -s1 , 0},{0 , s1 , c1 , 0},{0 , 0 , 0 , 1}};Matrix Tc3 = new Matrix(4);Tc3.Data = new double[4, 4]{{1 , 0 , 0 , 0},{0 , 0 , 0 , 0},{0 , 0 , 1 , 0},{150 , 0 , 150 , 1}};//矩阵变换for(int i=0;i<19;i++){p3dv[i] = new Matrix(1, 4);//p3dv[i] = p3d[i] * Tv;p3dw[i] = new Matrix(1, 4);//p3dw[i] = p3d[i] * Tw1 * Tw2 * Tw3;p3dh[i] = new Matrix(1, 4);//p3dh[i] = p3d[i] * Th1 * Th2 * Th3;p3dc[i] = new Matrix(1, 4);//p3dc[i] = p3d[i] * Tc1 * Tc2 * Tc3;}Graphics g=e.Graphics;Pen mypen = new Pen(Color.Yellow, 1.0f);//主视图Point[] pp3d=new Point[19];for (int i = 0; i < 18; i++){//矩阵变换为二维坐标pp3d[i].X=Convert.ToInt16( p3dv[i].Data[0,0]); pp3d[i].Y =Convert.ToInt16( p3dv[i].Data[0,2]); }g.DrawLines(mypen, pp3d);// g.DrawLine(mypen, pp3d[0], pp3d[9]);// g.DrawLine(mypen, pp3d[1], pp3d[12]);// g.DrawLine(mypen, pp3d[2], pp3d[13]);// g.DrawLine(mypen, pp3d[3], pp3d[18]);g.DrawLine(mypen, pp3d[7], pp3d[16]);// g.DrawLine(mypen, pp3d[8], pp3d[17]);g.DrawLine(mypen, pp3d[10], pp3d[17]);// g.DrawLine(mypen, pp3d[11], pp3d[14]);// g.DrawLine(mypen, pp3d[15], pp3d[18]);//侧视图Point[] pp3d1 = new Point[18];for (int i = 0; i < 18; i++){pp3d1[i].X = Convert.ToInt16(p3dw[i].Data[0, 0]); pp3d1[i].Y = Convert.ToInt16(p3dw[i].Data[0, 2]); }g.DrawLines(mypen, pp3d1);// pp3d1[19] = pp3d1[0];g.DrawLine(mypen, pp3d1[0], pp3d1[9]);g.DrawLine(mypen, pp3d1[1], pp3d1[12]);g.DrawLine(mypen, pp3d1[2], pp3d1[13]);// g.DrawLine(mypen, pp3d1[3], pp3d1[18]);g.DrawLine(mypen, pp3d1[7], pp3d1[16]);g.DrawLine(mypen, pp3d1[8], pp3d1[17]);g.DrawLine(mypen, pp3d1[10], pp3d1[17]);g.DrawLine(mypen, pp3d1[11], pp3d1[14]);// g.DrawLine(mypen, pp3d1[15], pp3d1[18]);//俯视图Point[] pp3d2 = new Point[18];for (int i = 0; i < 18; i++){pp3d2[i].X = Convert.ToInt16(p3dh[i].Data[0, 0]); pp3d2[i].Y = Convert.ToInt16(p3dh[i].Data[0, 2]); }g.DrawLines(mypen, pp3d2);g.DrawLine(mypen, pp3d2[0], pp3d2[9]);g.DrawLine(mypen, pp3d2[1], pp3d2[12]);g.DrawLine(mypen, pp3d2[2], pp3d2[13]);// g.DrawLine(mypen, pp3d2[3], pp3d2[18]);g.DrawLine(mypen, pp3d2[7], pp3d2[16]);g.DrawLine(mypen, pp3d2[8], pp3d2[17]);g.DrawLine(mypen, pp3d2[10], pp3d2[17]);g.DrawLine(mypen, pp3d2[11], pp3d2[14]);// g.DrawLine(mypen, pp3d2[15], pp3d2[18]);//正轴侧Point[] pp3d3 = new Point[18];for (int i = 0; i < 18; i++){pp3d3[i].X = Convert.ToInt16(p3dc[i].Data[0, 0]); pp3d3[i].Y = Convert.ToInt16(p3dc[i].Data[0, 2]); }//pp3d3[19] = pp3d3[0];g.DrawLines(mypen, pp3d3);g.DrawLine(mypen, pp3d3[0], pp3d3[9]);g.DrawLine(mypen, pp3d3[1], pp3d3[12]);g.DrawLine(mypen, pp3d3[2], pp3d3[13]);// g.DrawLine(mypen, pp3d[3], pp3d[18]);g.DrawLine(mypen, pp3d3[7], pp3d3[16]);g.DrawLine(mypen, pp3d3[8], pp3d3[17]);g.DrawLine(mypen, pp3d3[10], pp3d3[17]);g.DrawLine(mypen, pp3d3[11], pp3d3[14]);// g.DrawLine(mypen, pp3d[15], pp3d[18]);}}}Matrix.csusing System;using System.Collections.Generic;using System.Text;namespace E5{class Matrix{public double[,] Data;public Matrix(){ }public Matrix(int size){this.Data = new double[size, size];}public Matrix(int rows, int cols){this.Data = new double[rows, cols];}public Matrix(double[,] data){Data = data;}public static Matrix operator *(Matrix M1, Matrix M2){int r1 = M1.Data.GetLength(0); int r2 = M2.Data.GetLength(0);int c1 = M1.Data.GetLength(1); int c2 = M2.Data.GetLength(1);// try// {if (c1 != r2){throw new System.Exception("Matrix dimensions donot agree ");}// }double[,] res = new double[r1, c2];for (int i = 0; i < r1; i++){for (int j = 0; j < c2; j++){for (int k = 0; k < r2; k++){res[i, j] = res[i, j] + (M1.Data[i, k] * M2.Data[k, j]); }}}return new Matrix(res);}}}。
计算机图形学实验报告代码
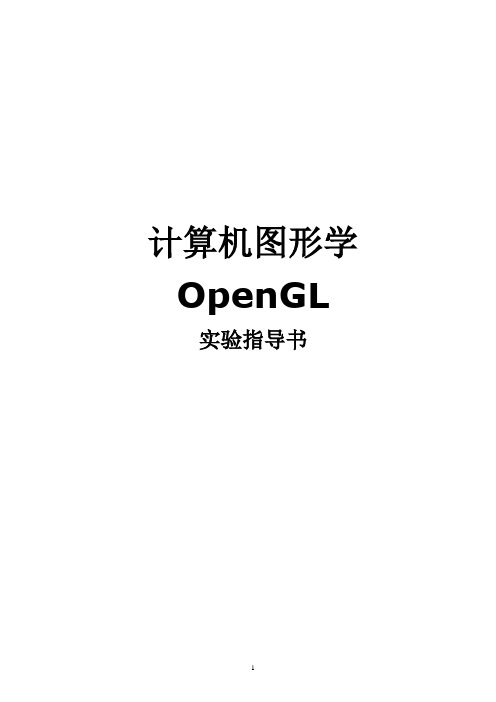
计算机图形学OpenGL实验指导书目录实验一:OpenGL基础知识 (1)实验二OpenGL中的建模与变换 (2)实验三OpenGL中的光照 (6)实验四OpenGL中的拾取 (9)实验五OpenGL中的纹理映射 (12)实验一:OpenGL基础知识一、实验目的1、建立Windows系统下的OpenGL实验框架。
2、学习理解OpenGL工作流程。
二、实验环境⒈硬件:每个学生需配备计算机一台。
⒉软件:Visual C++;三、实验内容1、建立非控制台的Windows程序框架。
2、建立OpenGL框架。
3、建立OpenGL框架的类文件。
4、完善Windows框架。
5、理解程序间的相互关系。
四、实验要求1、学习教材第2章的内容。
2、理解教材中相关实例的代码,按要求添加到适当的地方,调试并通过运行。
3、运行结果应该与教材中的相关实例相符。
4、编译第2章的框架代码,修改背景色、窗口标题。
五、程序设计提示(略)六、报告要求1.认真书写实验报告,字迹清晰,格式规范。
报告中应写清姓名、学号、实验日期、实验题目、实验目的、实验原理。
2.报告中应书写主要源程序,且源程序中要有注释。
3.报告中应包含运行结果及结果分析。
如调试通过并得到预期的效果,请注明…通过‟并粘贴运行效果图;如未调试通过或结果不正确,试分析原因。
4.报告最后包含实验总结和体会。
实验二OpenGL中的建模与变换一、实验目的1.学习配置OpenGL环境。
2.掌握在OpenGL中指定几何模型的方法。
3. 掌握OpenGL中的透视投影和正投影的设置方法,学会诊断投影问题导致的显示异常。
二、实验环境⒈硬件:每个学生需配备计算机一台。
⒉软件:Visual C++;三、实验内容1.建立OpenGL编程环境(注:Windows自带gl和glu,还需要安装glut库文件。
)(a)查看Windows自带的OpenGL文件。
在文件夹c:\windows\system32下查看是否存在文件opengl32.dll和glu32.dll;在Visual Studio的安装目录Vc7\PlatformSDK\Include\gl下查看是否存在gl.h和glu.h;在Vc7\PlatformSDK\Lib下是否存在opengl32.lib和glu32.lib。
计算机图形学上机代码

glRectf(0.0f, 0.0f, 0.5f, 0.5f); //在右上方绘制一个无镂空效果的正方形
glFlush();
}
E.g.7着色模型
#include <GL/glut.h>
#include <math.h>
const GLdouble Pi = 3.1415926536;
*/
static GLubyte Mask[128];
FILE *fp;
fp = fopen("D:/Mask/Mask.bmp", "rb"); //注意此处的格式fp = fopen("D:/vclx/mask.bmp", "rb");
if( !fp )
exit(0);
if( fseek(fp, -(int)sizeof(Mask), SEEK_END) )
glVertex2f(1.0f, 0.0f); //以上两个点可以画x轴
glVertex2f(0.0f, -1.0f);
glVertex2f(0.0f, 1.0f); //以上两个点可以画y轴
glEnd();
glBegin(GL_LINE_STRIP);
for(x=-1.0f/factor; x<1.0f/factor; x+=0.01f)
0x18, 0xCC, 0x33, 0x18,
0x10, 0xC4, 0x23, 0x08,
0x10, 0x63, 0xC6, 0x08,
0x10, 0x30, 0x0C, 0x08,
0x10, 0x18, 0x18, 0x08,
计算机图形学(C语言)教案
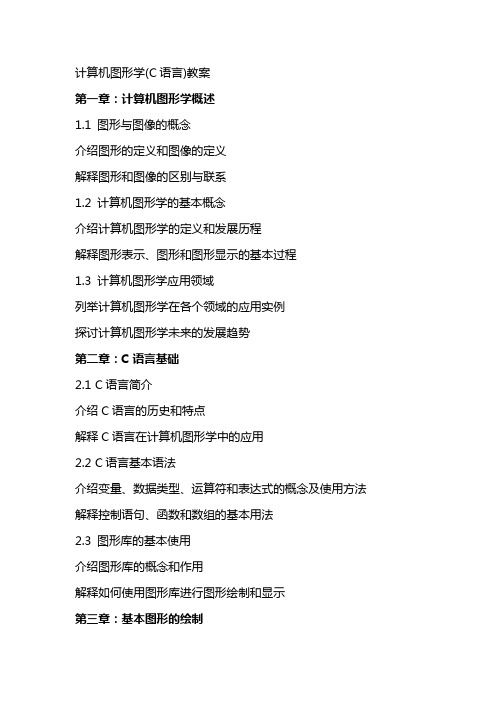
计算机图形学(C语言)教案第一章:计算机图形学概述1.1 图形与图像的概念介绍图形的定义和图像的定义解释图形和图像的区别与联系1.2 计算机图形学的基本概念介绍计算机图形学的定义和发展历程解释图形表示、图形和图形显示的基本过程1.3 计算机图形学应用领域列举计算机图形学在各个领域的应用实例探讨计算机图形学未来的发展趋势第二章:C语言基础2.1 C语言简介介绍C语言的历史和特点解释C语言在计算机图形学中的应用2.2 C语言基本语法介绍变量、数据类型、运算符和表达式的概念及使用方法解释控制语句、函数和数组的基本用法2.3 图形库的基本使用介绍图形库的概念和作用解释如何使用图形库进行图形绘制和显示第三章:基本图形的绘制3.1 点的绘制介绍如何使用C语言绘制点解释点的坐标系统和绘点函数的用法3.2 线的绘制介绍如何使用C语言绘制直线和曲线解释线的参数方程和绘线函数的用法3.3 填充区域的绘制介绍如何使用C语言绘制填充区域解释填充算法和绘填充区域函数的用法第四章:图形变换4.1 坐标变换介绍坐标变换的概念和作用解释平移、旋转和缩放等基本变换的实现方法4.2 投影变换介绍投影变换的概念和种类解释正交投影和透视投影的实现方法4.3 视图变换介绍视图变换的概念和作用解释平视、俯视和仰视等视图变换的实现方法第五章:动画与交互5.1 动画的基本概念介绍动画的定义和分类解释动画的方法和播放原理5.2 C语言实现动画介绍如何使用C语言实现简单的动画效果解释动画的播放控制和效果实现方法5.3 用户交互的基本概念介绍用户交互的定义和作用解释图形用户界面(GUI)的基本组件和交互方式5.4 C语言实现用户交互介绍如何使用C语言实现用户交互功能解释用户输入处理和输出显示的方法和技巧第六章:图形界面设计6.1 图形界面设计基础介绍图形界面设计的目的和重要性解释图形界面设计的基本原则和方法6.2 常用图形界面组件介绍按钮、菜单、对话框等常用图形界面组件的使用方法解释如何使用C语言绘制和处理这些组件6.3 图形界面事件处理介绍图形界面事件的概念和类型解释如何使用C语言处理用户交互事件第七章:图像处理基础7.1 图像处理概述介绍图像处理的概念和任务解释图像处理在计算机图形学中的应用7.2 图像文件格式介绍常见图像文件格式如BMP、JPG、PNG等的特点和用法解释如何使用C语言读取和写入这些图像文件7.3 图像基本处理技术介绍图像处理的基本技术如图像滤波、图像增强、图像分割等解释这些技术在计算机图形学中的应用和实现方法第八章:曲面建模8.1 参数曲面的基本概念介绍参数曲面的定义和表示方法解释参数曲面的性质和绘制方法8.2 常见曲面类型的绘制介绍柱面、球面、环面等常见曲面的定义和绘制方法解释如何使用C语言实现这些曲面的绘制8.3 曲面变换介绍曲面变换的概念和作用解释如何使用C语言实现曲面的变换第九章:光照与渲染9.1 光照模型介绍光照模型的概念和重要性解释朗伯光照模型和Phong 光照模型的原理和实现方法9.2 材质属性介绍材质属性的概念和作用解释如何设置和调整材质的反射率、透射率和光泽度等属性9.3 渲染技术介绍渲染的概念和任务解释如何使用C语言实现简单的渲染技术如纹理映射、阴影等第十章:案例分析与实践10.1 案例分析分析具体计算机图形学应用案例解释案例中的关键技术和实现方法10.2 实践项目提供实践项目让学生动手实现计算机图形学的基本功能指导学生如何设计程序结构、编写代码并调试程序10.3 课程设计介绍课程设计的要求和评价标准第十一章:OpenGL基础11.1 OpenGL简介介绍OpenGL的概念和作用解释OpenGL的特点和应用领域11.2 OpenGL基本设置介绍如何设置OpenGL环境解释如何使用OpenGL进行窗口创建、初始化等基本操作11.3 OpenGL绘制基本图形介绍如何使用OpenGL绘制点、线、三角形等基本图形解释OpenGL中的坐标系统、视图变换等概念第十二章:OpenGL高级图形绘制12.1 颜色与纹理映射介绍颜色和纹理映射的概念解释如何使用OpenGL进行颜色和纹理映射的设置和绘制12.2 光照与材质介绍光照和材质的概念解释如何使用OpenGL实现光照和材质的效果12.3 动画与交互介绍动画和交互的概念解释如何使用OpenGL实现动画和交互的效果第十三章:OpenGL图形编程实例13.1 实例一:绘制一个三角形解释如何使用OpenGL绘制一个简单的三角形指导如何编写代码和调试程序13.2 实例二:3D旋转立方体解释如何使用OpenGL绘制一个旋转的立方体指导如何编写代码和调试程序13.3 实例三:动态光照变化解释如何使用OpenGL实现动态光照变化的效果指导如何编写代码和调试程序第十四章:计算机图形学项目实践14.1 项目设计思路介绍项目的设计思路和目标解释项目中的关键技术和实现方法14.2 项目实现提供项目实现的过程和步骤指导学生如何编写代码、调试程序和优化性能14.3 项目总结与评价总结项目的完成情况和成果评价项目的创新性、实用性和稳定性等指标第十五章:计算机图形学前沿技术15.1 虚拟现实技术介绍虚拟现实技术的概念和应用领域解释虚拟现实技术在计算机图形学中的重要性15.2 增强现实技术介绍增强现实技术的概念和应用领域解释增强现实技术在计算机图形学中的重要性15.3 计算机图形学未来发展探讨计算机图形学未来的发展趋势和研究方向激发学生对计算机图形学的研究兴趣和创新思维重点和难点解析本文主要介绍了计算机图形学(C语言)的教案,内容涵盖了图形与图像的概念、C语言基础、基本图形的绘制、图形变换、动画与交互、图形界面设计、图像处理基础、曲面建模、光照与渲染、案例分析与实践、OpenGL基础、OpenGL高级图形绘制、OpenGL图形编程实例、计算机图形学项目实践以及计算机图形学前沿技术等十五个章节。
计算机图形学c语言代码(笑脸、时钟、还有一个实在是不好意思说)
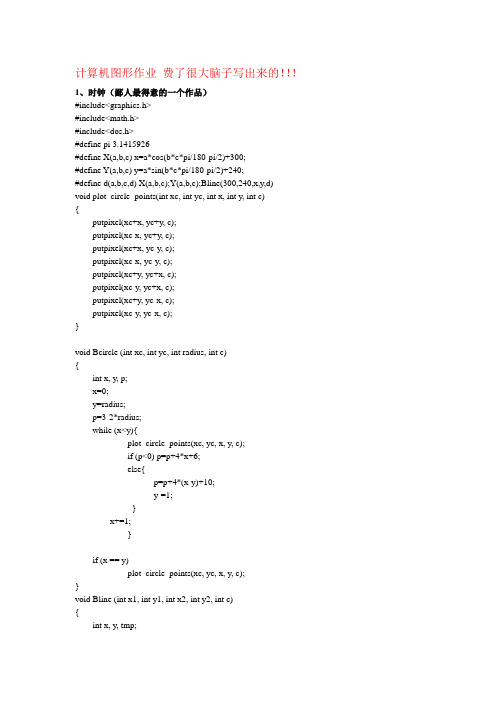
计算机图形作业费了很大脑子写出来的!!!1、时钟(鄙人最得意的一个作品)#include<graphics.h>#include<math.h>#include<dos.h>#define pi 3.1415926#define X(a,b,c) x=a*cos(b*c*pi/180-pi/2)+300;#define Y(a,b,c) y=a*sin(b*c*pi/180-pi/2)+240;#define d(a,b,c,d) X(a,b,c);Y(a,b,c);Bline(300,240,x,y,d)void plot_circle_points(int xc, int yc, int x, int y, int c){putpixel(xc+x, yc+y, c);putpixel(xc-x, yc+y, c);putpixel(xc+x, yc-y, c);putpixel(xc-x, yc-y, c);putpixel(xc+y, yc+x, c);putpixel(xc-y, yc+x, c);putpixel(xc+y, yc-x, c);putpixel(xc-y, yc-x, c);}void Bcircle (int xc, int yc, int radius, int c){int x, y, p;x=0;y=radius;p=3-2*radius;while (x<y){plot_circle_points(xc, yc, x, y, c);if (p<0) p=p+4*x+6;else{p=p+4*(x-y)+10;y-=1;}x+=1;}if (x == y)plot_circle_points(xc, yc, x, y, c);}void Bline (int x1, int y1, int x2, int y2, int c){int x, y, tmp;int dx, dy, d;float k;if( 0 == (x1 - x2)){x = x1;if(y1 > y2){ tmp = y1;y1 = y2; y2 =tmp;}for(y = y1; y < y2; y ++){putpixel(x, y, abs(getpixel(x,y)-c));}return;}k = (float)(y2 -y1)/(float)(x2 - x1);if(k > 1.0){tmp = x1; x1 = y1; y1 = tmp;tmp = x2; x2 = y2; y2 = tmp;}else if(k > 0){}else if(k < -1.0){x1 = -x1;x2 = -x2;tmp = x1; x1 = y1; y1 = tmp;tmp = x2; x2 = y2; y2 = tmp;}else if( k < 0){y1 = - y1;y2 = - y2;}else{y = y1;if(x1 > x2){ tmp = x1; x1 = x2; x2 = tmp;}for(x = x1; x <= x2; x ++){putpixel(x, y, abs(getpixel(x,y)-c));}return;}if(x1 > x2){tmp = x1; x1 = x2; x2 = tmp;tmp = y1; y1 = y2; y2 = tmp;}dx = x2 - x1;dy = y2 - y1;d = 2 * dy - dx;x = x1; y = y1;for(; x < x2; x ++){if(k > 1){putpixel(y, x, abs(getpixel(y, x) - c));}else if(k > 0){putpixel(x, y, abs(getpixel(x,y)-c));}else if( k < -1){putpixel(-y, x, abs(getpixel(-y,x)-c));}else if(k < 0){putpixel(x, -y, abs(getpixel(x,-y)-c));}else {}if(d >= 0){y++;d+=2*dy-2*dx;}else{d+=2*dy;}}}void init(){int i,l,x1,x2,y1,y2;setbkcolor(1);Bcircle(300,240,200,15);Bcircle(300,240,205,15);Bcircle(300,240,5,15);for(i=0;i<60;i++){if(i%5==0) l=15;else l=5;x1=200*cos(i*6*pi/180)+300;y1=200*sin(i*6*pi/180)+240;x2=(200-l)*cos(i*6*pi/180)+300;y2=(200-l)*sin(i*6*pi/180)+240;Bline(x1,y1,x2,y2,15);}}void main(){int x,y;int gd,gm;unsigned char h,m,s;struct time t[1];detectgraph(&gd, &gm);initgraph(&gd,&gm," ");init();gettime(t);h=t[0].ti_hour;m=t[0].ti_min;s=t[0].ti_sec;d(150,h,30,7);d(170,m,6,14);d(190,s,6,4);while(!kbhit()){while(t[0].ti_sec==s)gettime(t);sound(400);delay(70);sound(200);delay(30);nosound();d(190,s,6,4);s=t[0].ti_sec;d(190,s,6,4);if (t[0].ti_min!=m){d(170,m,6,14);m=t[0].ti_min;d(170,m,6,14);}if (t[0].ti_hour!=h){d(150,h,30,7);h=t[0].ti_hour;d(150,h,30,7);sound(1000);delay(240);nosound();delay(140);sound(2000);delay(240);nosound();}}}2、笑脸(初学者作品很漂亮的!)#include "Conio.h"#include "graphics.h"#define closegr closegraphvoid initgr(void){int graphdriver=DETECT, graphmode=0;initgraph(&graphdriver, &graphmode, " "); }int main(){int i,j;initgr();setbkcolor(0);cleardevice();setcolor(2);circle(320,240,200);setcolor(14);line(320,280,320,200);ellipse(230,210,40,140,70,60);ellipse(410,210,40,140,70,60);ellipse(320,220,220,320,120,140);outtextxy(500,450,"xiao xiao!");getch();closegraph();return 0;}3、电扇(只能叫这个了本来想画车轮,没想到。
计算机图形学编码裁剪算法c语言程序
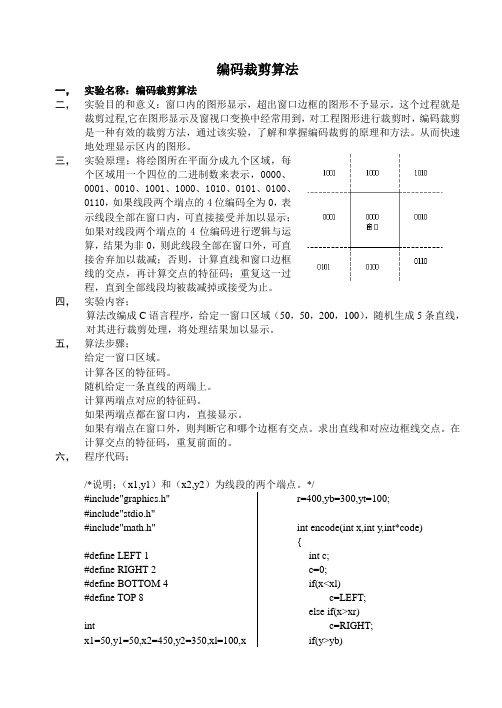
一,
二, 实验目的和意义:窗口内的图形显示,超出窗口边框的图形不予显示。这个过程就是裁剪过程,它在图形显示及窗视口变换中经常用到,对工程图形进行裁剪时,编码裁剪是一种有效的裁剪方法,通过该实验,了解和掌握编码裁剪的原理和方法。从而快速地处理显示区内的图形。
三,实验原理;将绘图所在平面分成九个区域,每个区域用一个四位的二进制数来表示,0000、0001、0010、1001、1000、1010、0101、0100、0110,如果线段两个端点的4位编码全为0,表示线段全部在窗口内,可直接接受并加以显示;如果对线段两个端点的4位编码进行逻辑与运算,结果为非0,则此线段全部在窗口外,可直接舍弃加以裁减;否则,计算直线和窗口边框线的交点,再计算交点的特征码;重复这一过程,直到全部线段均被裁减掉或接受为止。
{
x=x1;
y=y1+(long)(y2-y1)*(xl-x1)/(x2-x1);
}
else if((RIGHT&code)!=0)
/*线段与右边界相交*/
{
x=xr;
y=y1+(long)(y2-y1)*(xr-x1/x2-x1);
}
else if((BOTTOM&code)!=0)
/*线段与上边界相交*/
{
y=yb;
x=x1+(long)(x2-x1)*(yb-y1)/(y2-y1);
}
else if((TOP&code)!=0)
/*线段与下边界相交*/
{
y=yt;
x=x1+(long)(x2-x1)*(yt-y1)/(y2-y1);
}
if(code==code1)
{x1=x;
图形学实验一Visual C++图形程序设计
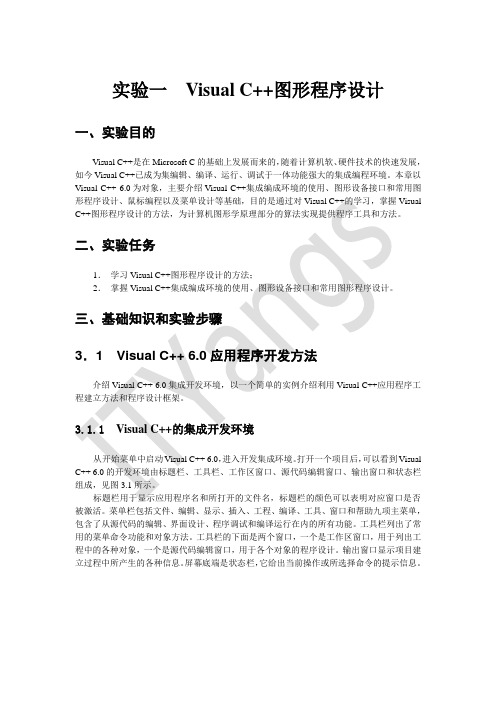
实验一Visual C++图形程序设计一、实验目的Visual C++是在Microsoft C的基础上发展而来的,随着计算机软、硬件技术的快速发展,如今Visual C++已成为集编辑、编译、运行、调试于一体功能强大的集成编程环境。
本章以Visual C++ 6.0为对象,主要介绍Visual C++集成编成环境的使用、图形设备接口和常用图形程序设计、鼠标编程以及菜单设计等基础,目的是通过对Visual C++的学习,掌握Visual C++图形程序设计的方法,为计算机图形学原理部分的算法实现提供程序工具和方法。
二、实验任务1.学习Visual C++图形程序设计的方法;2.掌握Visual C++集成编成环境的使用、图形设备接口和常用图形程序设计。
三、基础知识和实验步骤3.1 Visual C++ 6.0应用程序开发方法介绍Visual C++ 6.0集成开发环境,以一个简单的实例介绍利用Visual C++应用程序工程建立方法和程序设计框架。
3.1.1 Visual C++的集成开发环境从开始菜单中启动Visual C++ 6.0,进入开发集成环境。
打开一个项目后,可以看到Visual C++ 6.0的开发环境由标题栏、工具栏、工作区窗口、源代码编辑窗口、输出窗口和状态栏组成,见图3.1所示。
标题栏用于显示应用程序名和所打开的文件名,标题栏的颜色可以表明对应窗口是否被激活。
菜单栏包括文件、编辑、显示、插入、工程、编译、工具、窗口和帮助九项主菜单,包含了从源代码的编辑、界面设计、程序调试和编译运行在内的所有功能。
工具栏列出了常用的菜单命令功能和对象方法。
工具栏的下面是两个窗口,一个是工作区窗口,用于列出工程中的各种对象,一个是源代码编辑窗口,用于各个对象的程序设计。
输出窗口显示项目建立过程中所产生的各种信息。
屏幕底端是状态栏,它给出当前操作或所选择命令的提示信息。
标题栏菜单栏工作区源代码编辑窗口状态栏图3.1 Visual C++ 6.0集成开发环境3.1.2 应用程序工程的建立方法Visual C++提供了一种称为App Wizard的工具,利用该工具,用户可以方便地按照自己的需要创建符合需要的应用程序框架。
计算机图形学(C语言)教案

计算机图形学(C语言)教案第一章:C语言基础1.1 教学目标让学生掌握C语言的基本语法和结构。
让学生了解C语言在计算机图形学中的应用。
1.2 教学内容C语言的基本语法和数据类型。
控制结构和函数。
C语言在计算机图形学中的应用。
1.3 教学方法讲解和示例相结合。
让学生通过编写简单的C语言程序来加深对语法和结构的理解。
1.4 教学评估课后作业:编写简单的C语言程序。
课堂讨论:学生之间的交流和问题解答。
第二章:图形设备2.1 教学目标让学生了解图形设备的基本概念和原理。
让学生掌握如何在C语言中与图形设备进行交互。
2.2 教学内容图形设备的概念和分类。
图形设备的驱动程序。
在C语言中使用图形设备的方法。
2.3 教学方法讲解和实验相结合。
通过示例让学生了解图形设备的使用方法。
2.4 教学评估课后作业:编写与图形设备交互的C语言程序。
第三章:基本图形操作3.1 教学目标让学生掌握基本的图形操作方法。
让学生了解图形坐标系统和变换。
3.2 教学内容基本图形操作:点、直线、圆等。
图形坐标系统:笛卡尔坐标系、极坐标系等。
图形变换:平移、旋转、缩放等。
3.3 教学方法讲解和示例相结合。
通过编程实验让学生掌握基本图形操作。
3.4 教学评估课后作业:编写实现基本图形操作的C语言程序。
第四章:颜色和文本4.1 教学目标让学生了解颜色和文本在计算机图形学中的基本概念。
让学生掌握如何在C语言中设置颜色和显示文本。
4.2 教学内容颜色的概念和表示方法。
设置颜色的方法。
文本的显示和字体设置。
4.3 教学方法讲解和示例相结合。
通过编程实验让学生掌握颜色和文本的设置方法。
4.4 教学评估课后作业:编写实现颜色和文本设置的C语言程序。
第五章:图形绘制综合实例5.1 教学目标让学生综合运用所学的图形操作方法来绘制复杂的图形。
让学生了解图形绘制的基本技巧和优化方法。
5.2 教学内容综合实例:绘制复杂的图形。
图形绘制技巧和优化方法。
5.3 教学方法讲解和实验相结合。
c图形学课程设计源码
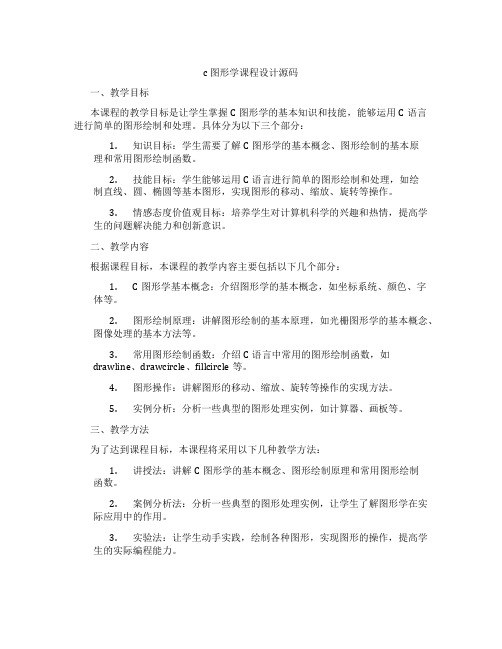
c 图形学课程设计源码一、教学目标本课程的教学目标是让学生掌握C图形学的基本知识和技能,能够运用C语言进行简单的图形绘制和处理。
具体分为以下三个部分:1.知识目标:学生需要了解C图形学的基本概念、图形绘制的基本原理和常用图形绘制函数。
2.技能目标:学生能够运用C语言进行简单的图形绘制和处理,如绘制直线、圆、椭圆等基本图形,实现图形的移动、缩放、旋转等操作。
3.情感态度价值观目标:培养学生对计算机科学的兴趣和热情,提高学生的问题解决能力和创新意识。
二、教学内容根据课程目标,本课程的教学内容主要包括以下几个部分:1.C图形学基本概念:介绍图形学的基本概念,如坐标系统、颜色、字体等。
2.图形绘制原理:讲解图形绘制的基本原理,如光栅图形学的基本概念、图像处理的基本方法等。
3.常用图形绘制函数:介绍C语言中常用的图形绘制函数,如drawline、drawcircle、fillcircle等。
4.图形操作:讲解图形的移动、缩放、旋转等操作的实现方法。
5.实例分析:分析一些典型的图形处理实例,如计算器、画板等。
三、教学方法为了达到课程目标,本课程将采用以下几种教学方法:1.讲授法:讲解C图形学的基本概念、图形绘制原理和常用图形绘制函数。
2.案例分析法:分析一些典型的图形处理实例,让学生了解图形学在实际应用中的作用。
3.实验法:让学生动手实践,绘制各种图形,实现图形的操作,提高学生的实际编程能力。
4.讨论法:学生进行小组讨论,分享学习心得和解决问题的方法,培养学生的团队合作意识。
四、教学资源为了支持教学内容和教学方法的实施,本课程将准备以下教学资源:1.教材:选用权威、实用的C图形学教材,为学生提供系统的学习资料。
2.参考书:提供一些相关的参考书籍,拓展学生的知识面。
3.多媒体资料:制作精美的PPT课件,直观地展示图形学的基本概念和实例分析。
4.实验设备:准备计算机实验室,让学生进行上机实践。
五、教学评估本课程的评估方式包括平时表现、作业和考试三个部分,旨在全面、客观地评估学生的学习成果。
计算机图形学实验C++代码

一、bresenham算法画直线#include<glut.h>#include<math.h>#include<stdio.h>void draw_pixel(int ix,int iy){glBegin(GL_POINTS);glVertex2i(ix,iy);glEnd();}void Bresenham(int x1,int y1,int xEnd,int yEnd) {int dx=abs(xEnd-x1),dy=abs(yEnd-y1);int p=2*dy-dx;int twoDy=2*dy,twoDyMinusDx=2*dy-2*dx;int x,y;if (x1>xEnd){x=xEnd;y=yEnd;xEnd=x1;}else{x=x1;y=y1;}draw_pixel(x,y);while(x<xEnd){x++;if(p<0)p+=twoDy;else{y++;p+=twoDyMinusDx;draw_pixel(x,y);}}}void display(){glClear(GL_COLOR_BUFFER_BIT);Bresenham(0,0,400,400);glFlush();}void myinit(){glClearColor(0.8,1.0,1.0,1.0);glColor3f(0.0,0.0,1.0);glPointSize(1.0);glMatrixMode(GL_PROJECTION);glLoadIdentity();gluOrtho2D(0.0,500.0,0.0,500.0);}void main(int argc,char **argv ){glutInit(&argc,argv);glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB);glutInitWindowSize(500,500);glutInitWindowPosition(200.0,200.0);glutCreateWindow("CG_test_Bresenham_Line example");glutDisplayFunc(display);myinit();glutMainLoop();}二、中点法绘制椭圆#include<glut.h>#include<math.h>#include<stdio.h>inline int round(const float a){return int (a+0.5);}void setPixel(GLint xCoord,GLint yCoord){glBegin(GL_POINTS);glVertex2i(xCoord,yCoord);glEnd();}void ellipseMidpoint(int xCenter,int yCenter,int Rx,int Ry) {int Rx2=Rx*Rx;int Ry2=Ry*Ry;int twoRx2=2*Rx2;int twoRy2=2*Ry2;int p;int x=0;int y=Ry;int px=0;int py=twoRx2*y;void ellipsePlotPoints(int,int,int,int);ellipsePlotPoints(xCenter,yCenter,x,y);p=round(Ry2-(Rx2*Ry)+(0.25*Rx2));while(px<py){x++;px+=twoRy2;if(p<0)p+=Ry2+px;else{y--;py-=twoRx2;p+=Ry2+px-py;}ellipsePlotPoints(xCenter,yCenter,x,y);}p=round(Ry2*(x+0.5)*(x+0.5)+Rx2*(y-1)*(y-1)-Rx2*Ry2);while(y>0){y--;py-=twoRx2;if(p>0)p+=Rx2-py;else{x++;px+=twoRy2;p+=Rx2-py+px;}ellipsePlotPoints(xCenter,yCenter,x,y);}}void ellipsePlotPoints(int xCenter,int yCenter,int x,int y) {setPixel(xCenter+x,yCenter+y);setPixel(xCenter-x,yCenter+y);setPixel(xCenter+x,yCenter-y);setPixel(xCenter-x,yCenter-y);}void display(){glClear(GL_COLOR_BUFFER_BIT);ellipseMidpoint(200,200,50,30);glFlush();}void myinit(){glClearColor(0.8,1.0,1.0,1.0);glColor3f(0.0,0.0,1.0);glPointSize(1.0);glMatrixMode(GL_PROJECTION);glLoadIdentity();gluOrtho2D(0.0,300.0,0.0,300.0);}void main(int argc,char **argv ){glutInit(&argc,argv);glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB);glutInitWindowSize(300,300);glutInitWindowPosition(200.0,200.0);glutCreateWindow("circleMId example");glutDisplayFunc(display);myinit();glutMainLoop();}三、抛物线#include<glut.h>#include<math.h>#include<stdio.h>inline int round(const float a){return int (a+0.5);}void setPixel(GLint xCoord,GLint yCoord){glBegin(GL_POINTS);glVertex2i(xCoord,yCoord);glEnd();}void ellipseMidpoint(int xCenter,int yCenter,int a,int b){int p;int x=xCenter;int y=yCenter;int px=0,py=0;void ellipsePlotPoints(int,int,int,int);ellipsePlotPoints(xCenter,yCenter,px,py);p=yCenter+a*(x+1-xCenter)*(x+1-xCenter)+b*(x+1-xCenter)-y-0.5;do{if(p<0){x=x+1;y=y;p=yCenter+a*(x+1-xCenter)*(x+1-xCenter)+b*(x+1-xCenter)-y-0.5;}else{x=x+1;y=y-1;p=yCenter+a*(x+1-xCenter)*(x+1-xCenter)+b*(x+1-xCenter)-y-0.5;}px=x-xCenter;py=y-yCenter;ellipsePlotPoints(xCenter,yCenter,px,py);}while(px<py);for(;;){if(p<0){x=x-1;y=y+1;p=yCenter+a*(x+0.5-xCenter)*(x+0.5-xCenter)+b*(x+0.5-xCenter)-y-1;}else{x=x;y=y+1;p=yCenter+a*(x+0.5-xCenter)*(x+0.5-xCenter)+b*(x+0.5-xCenter)-y-1;}px=x-xCenter;py=y-yCenter;ellipsePlotPoints(xCenter,yCenter,px,py);};}void ellipsePlotPoints(int xCenter,int yCenter,int x,int y){setPixel(xCenter+x,yCenter+y);setPixel(xCenter-x,yCenter+y);}void display(){glClear(GL_COLOR_BUFFER_BIT);ellipseMidpoint(150,150,1,0);glFlush();}void myinit(){glClearColor(0.8,1.0,1.0,1.0);glColor3f(0.0,0.0,1.0);glPointSize(1.0);glMatrixMode(GL_PROJECTION);glLoadIdentity();gluOrtho2D(0.0,300.0,0.0,300.0);}void main(int argc,char **argv ){glutInit(&argc,argv);glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB);glutInitWindowSize(500,500);glutInitWindowPosition(200.0,200.0);glutCreateWindow("circleMId example");glutDisplayFunc(display);myinit();glutMainLoop();}四、基本图元输出#include<glut.h>#include<math.h>#include<stdio.h>void Polygon(int*p1,int*p2,int*p3,int*p4,int*p5,int*p6) {glBegin(GL_POLYGON);glVertex2iv(p1);glVertex2iv(p2);glVertex2iv(p3);glVertex2iv(p4);glVertex2iv(p5);glVertex2iv(p6);glEnd();}void Triangles(int*p1,int*p2,int*p3,int*p4,int*p5,int*p6) {glBegin(GL_TRIANGLES);glVertex2iv(p1);glVertex2iv(p2);glVertex2iv(p6);glVertex2iv(p3);glVertex2iv(p4);glVertex2iv(p5);glEnd();}void Trianglefan(int*p1,int*p2,int*p3,int*p4,int*p5,int*p6) {glBegin(GL_TRIANGLE_FAN);glVertex2iv(p1);glVertex2iv(p2);glVertex2iv(p3);glVertex2iv(p4);glVertex2iv(p5);glVertex2iv(p6);glEnd();}void Trianglestrip(int*p1,int*p2,int*p3,int*p4,int*p5,int*p6) {glBegin(GL_TRIANGLE_STRIP);glVertex2iv(p1);glVertex2iv(p2);glVertex2iv(p6);glVertex2iv(p3);glVertex2iv(p5);glVertex2iv(p4);glEnd();}void glRect_s(GLint a,GLint b,GLint c,GLint d){glRecti(a,b,c,d);}void display(){int p1[]={60,170};int p2[]={100,100};int p3[]={180,100};int p4[]={220,170};int p5[]={180,240};int p6[]={100,240};int p7[]={60,100};glClear(GL_COLOR_BUFFER_BIT);//Triangles(p1,p2,p3,p4,p5,p6);//Polygon(p1,p2,p3,p4,p5,p6);//glRect_s(160,30,10,100);Trianglestrip(p1,p2,p3,p4,p5,p6);//Trianglefan(p1,p2,p3,p4,p5,p6);glFlush();}void myinit(){glClearColor(0.8,1.0,1.0,1.0);glColor3f(0.0,0.0,1.0);glPointSize(1.0);glMatrixMode(GL_PROJECTION);glLoadIdentity();gluOrtho2D(0.0,300.0,0.0,300.0);}void main(int argc,char **argv ){glutInit(&argc,argv);glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB);glutInitWindowSize(500,500);glutInitWindowPosition(300.0,300.0);glutCreateWindow("circleMId example");glutDisplayFunc(display);myinit();glutMainLoop();}五、区域填充#include"glut.h"#include"windows.h"const int POINTNUM=7; //多边形点数.//定义结构体用于活性边表AET和新边表NETtypedef struct XET{float x;float dx,ymax;XET* next;}AET,NET;//定义点结构体pointstruct point{float x;float y;}polypoint[POINTNUM]={250,50,350,150,50,40,250,20,200,30,100,100,10,300};//多边形顶点void PolyScan(){//计算最高点的y坐标(扫描到此结束)int MaxY=0;int i;for(i=0;i<POINTNUM;i++)if(polypoint[i].y>MaxY)MaxY=polypoint[i].y;//初始化AET表AET *pAET=new AET;pAET->next=NULL;//初始化NET表NET *pNET[1024];for(i=0;i<=MaxY;i++){pNET[i]=new NET;pNET[i]->next=NULL;}glClear(GL_COLOR_BUFFER_BIT); //赋值的窗口显示.glColor3f(0.9,1.0,0.0); //设置直线的颜色红色glBegin(GL_POINTS);//扫描并建立NET表,注:构建一个图形for(i=0;i<=MaxY;i++){for(int j=0;j<POINTNUM;j++)if(polypoint[j].y==i){ //一个点跟前面的一个点形成一条线段,跟后面的点也形成线段if(polypoint[(j-1+POINTNUM)%POINTNUM].y>polypoint[j].y){NET *p=new NET;p->x=polypoint[j].x;p->ymax=polypoint[(j-1+POINTNUM)%POINTNUM].y;p->dx=(polypoint[(j-1+POINTNUM)%POINTNUM].x-polypoint[j].x)/(polypoint[(j- 1+POINTNUM)%POINTNUM].y-polypoint[j].y);p->next=pNET[i]->next;pNET[i]->next=p;}if(polypoint[(j+1+POINTNUM)%POINTNUM].y>polypoint[j].y){NET *p=new NET;p->x=polypoint[j].x;p->ymax=polypoint[(j+1+POINTNUM)%POINTNUM].y;p->dx=(polypoint[(j+1+POINTNUM)%POINTNUM].x-polypoint[j].x)/(polypoint[(j+1+POINTNUM)%POINTNUM].y-polypoint[j].y);p->next=pNET[i]->next;pNET[i]->next=p;}}}for(i=0;i<=MaxY;i++){//计算新的交点x,更新AETNET *p=pAET->next;while(p){p->x=p->x + p->dx;p=p->next;}AET *tq=pAET;p=pAET->next;tq->next=NULL;while(p){while(tq->next && p->x >= tq->next->x)tq=tq->next;NET *s=p->next;p->next=tq->next;tq->next=p;p=s;tq=pAET;}//(改进算法)先从AET表中删除ymax==i的结点* AET *q=pAET;p=q->next;while(p){if(p->ymax==i){q->next=p->next;delete p;p=q->next;}else{q=q->next;p=q->next;}}//将NET中的新点加入AET,并用插入法按X值递增排序p=pNET[i]->next;q=pAET;while(p){while(q->next && p->x >= q->next->x)q=q->next;NET *s=p->next;p->next=q->next;q->next=p;p=s;q=pAET;}//配对填充颜色p=pAET->next;while(p && p->next){for(float j=p->x;j<=p->next->x;j++)glVertex2i(static_cast<int>(j),i);p=p->next->next;//考虑端点情况}}glEnd();glFlush();}void init(void){glClearColor(1.0,1.0,1.0,0.0);//窗口的背景颜色设置为白色glMatrixMode(GL_PROJECTION);gluOrtho2D(0.0,600.0,0.0,450.0);}void lineSegment(void){glClear(GL_COLOR_BUFFER_BIT); //赋值的窗口显示.glColor3f(0.0,1.0,0.0); //设置直线的颜色红色glBegin(GL_LINES);glVertex2i(180,15); //Specify line-segment geometry.glVertex2i(10,145);glEnd();glFlush(); //Process all OpenGL routines as quickly as possible.}void main(int argc,char* argv){glutInit(&argc,&argv); //I初始化GLUT.glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB); //设置显示模式:单个缓存和使用RGB模型glutInitWindowPosition(50,100); //设置窗口的顶部和左边位置glutInitWindowSize(400,300); //设置窗口的高度和宽度glutCreateWindow("扫描线填充算法"); //创建显示窗口init(); //调用初始化过程glutDisplayFunc(PolyScan); //图形的定义传递glutMainLoop(); //显示所有的图形并等待}11。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
一、bresenham算法画直线#include<glut.h>#include<math.h>#include<stdio.h>void draw_pixel(int ix,int iy){glBegin(GL_POINTS);glVertex2i(ix,iy);glEnd();}void Bresenham(int x1,int y1,int xEnd,int yEnd) {int dx=abs(xEnd-x1),dy=abs(yEnd-y1);int p=2*dy-dx;int twoDy=2*dy,twoDyMinusDx=2*dy-2*dx;int x,y;if (x1>xEnd){x=xEnd;y=yEnd;xEnd=x1;}else{x=x1;y=y1;}draw_pixel(x,y);while(x<xEnd){x++;if(p<0)p+=twoDy;else{y++;p+=twoDyMinusDx;draw_pixel(x,y);}}}void display(){glClear(GL_COLOR_BUFFER_BIT);Bresenham(0,0,400,400);glFlush();}void myinit(){glClearColor(0.8,1.0,1.0,1.0);glColor3f(0.0,0.0,1.0);glPointSize(1.0);glMatrixMode(GL_PROJECTION);glLoadIdentity();gluOrtho2D(0.0,500.0,0.0,500.0);}void main(int argc,char **argv ){glutInit(&argc,argv);glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB);glutInitWindowSize(500,500);glutInitWindowPosition(200.0,200.0);glutCreateWindow("CG_test_Bresenham_Line example");glutDisplayFunc(display);myinit();glutMainLoop();}二、中点法绘制椭圆#include<glut.h>#include<math.h>#include<stdio.h>inline int round(const float a){return int (a+0.5);}void setPixel(GLint xCoord,GLint yCoord){glBegin(GL_POINTS);glVertex2i(xCoord,yCoord);glEnd();}void ellipseMidpoint(int xCenter,int yCenter,int Rx,int Ry) {int Rx2=Rx*Rx;int Ry2=Ry*Ry;int twoRx2=2*Rx2;int twoRy2=2*Ry2;int p;int x=0;int y=Ry;int px=0;int py=twoRx2*y;void ellipsePlotPoints(int,int,int,int);ellipsePlotPoints(xCenter,yCenter,x,y);p=round(Ry2-(Rx2*Ry)+(0.25*Rx2));while(px<py){x++;px+=twoRy2;if(p<0)p+=Ry2+px;else{y--;py-=twoRx2;p+=Ry2+px-py;}ellipsePlotPoints(xCenter,yCenter,x,y);}p=round(Ry2*(x+0.5)*(x+0.5)+Rx2*(y-1)*(y-1)-Rx2*Ry2);while(y>0){y--;py-=twoRx2;if(p>0)p+=Rx2-py;else{x++;px+=twoRy2;p+=Rx2-py+px;}ellipsePlotPoints(xCenter,yCenter,x,y);}}void ellipsePlotPoints(int xCenter,int yCenter,int x,int y) {setPixel(xCenter+x,yCenter+y);setPixel(xCenter-x,yCenter+y);setPixel(xCenter+x,yCenter-y);setPixel(xCenter-x,yCenter-y);}void display(){glClear(GL_COLOR_BUFFER_BIT);ellipseMidpoint(200,200,50,30);glFlush();}void myinit(){glClearColor(0.8,1.0,1.0,1.0);glColor3f(0.0,0.0,1.0);glPointSize(1.0);glMatrixMode(GL_PROJECTION);glLoadIdentity();gluOrtho2D(0.0,300.0,0.0,300.0);}void main(int argc,char **argv ){glutInit(&argc,argv);glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB);glutInitWindowSize(300,300);glutInitWindowPosition(200.0,200.0);glutCreateWindow("circleMId example");glutDisplayFunc(display);myinit();glutMainLoop();}三、抛物线#include<glut.h>#include<math.h>#include<stdio.h>inline int round(const float a){return int (a+0.5);}void setPixel(GLint xCoord,GLint yCoord){glBegin(GL_POINTS);glVertex2i(xCoord,yCoord);glEnd();}void ellipseMidpoint(int xCenter,int yCenter,int a,int b){int p;int x=xCenter;int y=yCenter;int px=0,py=0;void ellipsePlotPoints(int,int,int,int);ellipsePlotPoints(xCenter,yCenter,px,py);p=yCenter+a*(x+1-xCenter)*(x+1-xCenter)+b*(x+1-xCenter)-y-0.5;do{if(p<0){x=x+1;y=y;p=yCenter+a*(x+1-xCenter)*(x+1-xCenter)+b*(x+1-xCenter)-y-0.5;}else{x=x+1;y=y-1;p=yCenter+a*(x+1-xCenter)*(x+1-xCenter)+b*(x+1-xCenter)-y-0.5;}px=x-xCenter;py=y-yCenter;ellipsePlotPoints(xCenter,yCenter,px,py);}while(px<py);for(;;){if(p<0){x=x-1;y=y+1;p=yCenter+a*(x+0.5-xCenter)*(x+0.5-xCenter)+b*(x+0.5-xCenter)-y-1;}else{x=x;y=y+1;p=yCenter+a*(x+0.5-xCenter)*(x+0.5-xCenter)+b*(x+0.5-xCenter)-y-1;}px=x-xCenter;py=y-yCenter;ellipsePlotPoints(xCenter,yCenter,px,py);};}void ellipsePlotPoints(int xCenter,int yCenter,int x,int y){setPixel(xCenter+x,yCenter+y);setPixel(xCenter-x,yCenter+y);}void display(){glClear(GL_COLOR_BUFFER_BIT);ellipseMidpoint(150,150,1,0);glFlush();}void myinit(){glClearColor(0.8,1.0,1.0,1.0);glColor3f(0.0,0.0,1.0);glPointSize(1.0);glMatrixMode(GL_PROJECTION);glLoadIdentity();gluOrtho2D(0.0,300.0,0.0,300.0);}void main(int argc,char **argv ){glutInit(&argc,argv);glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB);glutInitWindowSize(500,500);glutInitWindowPosition(200.0,200.0);glutCreateWindow("circleMId example");glutDisplayFunc(display);myinit();glutMainLoop();}四、基本图元输出#include<glut.h>#include<math.h>#include<stdio.h>void Polygon(int*p1,int*p2,int*p3,int*p4,int*p5,int*p6) {glBegin(GL_POLYGON);glVertex2iv(p1);glVertex2iv(p2);glVertex2iv(p3);glVertex2iv(p4);glVertex2iv(p5);glVertex2iv(p6);glEnd();}void Triangles(int*p1,int*p2,int*p3,int*p4,int*p5,int*p6) {glBegin(GL_TRIANGLES);glVertex2iv(p1);glVertex2iv(p2);glVertex2iv(p6);glVertex2iv(p3);glVertex2iv(p4);glVertex2iv(p5);glEnd();}void Trianglefan(int*p1,int*p2,int*p3,int*p4,int*p5,int*p6) {glBegin(GL_TRIANGLE_FAN);glVertex2iv(p1);glVertex2iv(p2);glVertex2iv(p3);glVertex2iv(p4);glVertex2iv(p5);glVertex2iv(p6);glEnd();}void Trianglestrip(int*p1,int*p2,int*p3,int*p4,int*p5,int*p6) {glBegin(GL_TRIANGLE_STRIP);glVertex2iv(p1);glVertex2iv(p2);glVertex2iv(p6);glVertex2iv(p3);glVertex2iv(p5);glVertex2iv(p4);glEnd();}void glRect_s(GLint a,GLint b,GLint c,GLint d){glRecti(a,b,c,d);}void display(){int p1[]={60,170};int p2[]={100,100};int p3[]={180,100};int p4[]={220,170};int p5[]={180,240};int p6[]={100,240};int p7[]={60,100};glClear(GL_COLOR_BUFFER_BIT);//Triangles(p1,p2,p3,p4,p5,p6);//Polygon(p1,p2,p3,p4,p5,p6);//glRect_s(160,30,10,100);Trianglestrip(p1,p2,p3,p4,p5,p6);//Trianglefan(p1,p2,p3,p4,p5,p6);glFlush();}void myinit(){glClearColor(0.8,1.0,1.0,1.0);glColor3f(0.0,0.0,1.0);glPointSize(1.0);glMatrixMode(GL_PROJECTION);glLoadIdentity();gluOrtho2D(0.0,300.0,0.0,300.0);}void main(int argc,char **argv ){glutInit(&argc,argv);glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB);glutInitWindowSize(500,500);glutInitWindowPosition(300.0,300.0);glutCreateWindow("circleMId example");glutDisplayFunc(display);myinit();glutMainLoop();}五、区域填充#include"glut.h"#include"windows.h"const int POINTNUM=7; //多边形点数.//定义结构体用于活性边表AET和新边表NETtypedef struct XET{float x;float dx,ymax;XET* next;}AET,NET;//定义点结构体pointstruct point{float x;float y;}polypoint[POINTNUM]={250,50,350,150,50,40,250,20,200,30,100,100,10,300};//多边形顶点void PolyScan(){//计算最高点的y坐标(扫描到此结束)int MaxY=0;int i;for(i=0;i<POINTNUM;i++)if(polypoint[i].y>MaxY)MaxY=polypoint[i].y;//初始化AET表AET *pAET=new AET;pAET->next=NULL;//初始化NET表NET *pNET[1024];for(i=0;i<=MaxY;i++){pNET[i]=new NET;pNET[i]->next=NULL;}glClear(GL_COLOR_BUFFER_BIT); //赋值的窗口显示.glColor3f(0.9,1.0,0.0); //设置直线的颜色红色glBegin(GL_POINTS);//扫描并建立NET表,注:构建一个图形for(i=0;i<=MaxY;i++){for(int j=0;j<POINTNUM;j++)if(polypoint[j].y==i){ //一个点跟前面的一个点形成一条线段,跟后面的点也形成线段if(polypoint[(j-1+POINTNUM)%POINTNUM].y>polypoint[j].y){NET *p=new NET;p->x=polypoint[j].x;p->ymax=polypoint[(j-1+POINTNUM)%POINTNUM].y;p->dx=(polypoint[(j-1+POINTNUM)%POINTNUM].x-polypoint[j].x)/(polypoint[(j- 1+POINTNUM)%POINTNUM].y-polypoint[j].y);p->next=pNET[i]->next;pNET[i]->next=p;}if(polypoint[(j+1+POINTNUM)%POINTNUM].y>polypoint[j].y){NET *p=new NET;p->x=polypoint[j].x;p->ymax=polypoint[(j+1+POINTNUM)%POINTNUM].y;p->dx=(polypoint[(j+1+POINTNUM)%POINTNUM].x-polypoint[j].x)/(polypoint[(j+1+POINTNUM)%POINTNUM].y-polypoint[j].y);p->next=pNET[i]->next;pNET[i]->next=p;}}}for(i=0;i<=MaxY;i++){//计算新的交点x,更新AETNET *p=pAET->next;while(p){p->x=p->x + p->dx;p=p->next;}AET *tq=pAET;p=pAET->next;tq->next=NULL;while(p){while(tq->next && p->x >= tq->next->x)tq=tq->next;NET *s=p->next;p->next=tq->next;tq->next=p;p=s;tq=pAET;}//(改进算法)先从AET表中删除ymax==i的结点* AET *q=pAET;p=q->next;while(p){if(p->ymax==i){q->next=p->next;delete p;p=q->next;}else{q=q->next;p=q->next;}}//将NET中的新点加入AET,并用插入法按X值递增排序p=pNET[i]->next;q=pAET;while(p){while(q->next && p->x >= q->next->x)q=q->next;NET *s=p->next;p->next=q->next;q->next=p;p=s;q=pAET;}//配对填充颜色p=pAET->next;while(p && p->next){for(float j=p->x;j<=p->next->x;j++)glVertex2i(static_cast<int>(j),i);p=p->next->next;//考虑端点情况}}glEnd();glFlush();}void init(void){glClearColor(1.0,1.0,1.0,0.0);//窗口的背景颜色设置为白色glMatrixMode(GL_PROJECTION);gluOrtho2D(0.0,600.0,0.0,450.0);}void lineSegment(void){glClear(GL_COLOR_BUFFER_BIT); //赋值的窗口显示.glColor3f(0.0,1.0,0.0); //设置直线的颜色红色glBegin(GL_LINES);glVertex2i(180,15); //Specify line-segment geometry.glVertex2i(10,145);glEnd();glFlush(); //Process all OpenGL routines as quickly as possible.}void main(int argc,char* argv){glutInit(&argc,&argv); //I初始化GLUT.glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB); //设置显示模式:单个缓存和使用RGB模型glutInitWindowPosition(50,100); //设置窗口的顶部和左边位置glutInitWindowSize(400,300); //设置窗口的高度和宽度glutCreateWindow("扫描线填充算法"); //创建显示窗口init(); //调用初始化过程glutDisplayFunc(PolyScan); //图形的定义传递glutMainLoop(); //显示所有的图形并等待}11。