java实现图论中的经典算法
java算法总结

java算法总结一、排序1、冒泡排序:t冒泡排序是一种简单的排序算法,它重复地走访过要排序的数列,一次比较两个元素,如果他们的顺序错误就把他们交换过来。
走访数列的工作是重复地进行直到没有再需要交换,也就是说该数列已经排序完成。
这个算法的名字由来是因为越小的元素会经由交换慢慢“浮”到数列的顶端。
2、选择排序:t选择排序是一种简单直观的排序算法,无论什么数据进去都是O(n)的时间复杂度。
所以用到它的时候,数据规模越小越好。
唯一的好处可能就是不占用额外的内存空间了吧。
3、插入排序:t插入排序(Insertion-Sort)的算法描述是一种简单直观的排序算法。
它的工作原理是通过构建有序序列,对于未排序数据,在已排序序列中从后向前扫描,找到相应位置并插入。
4、希尔排序:t希尔排序,也称递减增量排序算法,是插入排序的一种更高效的改进版本。
希尔排序是非稳定排序算法。
该方法的基本思想是:先将整个待排序的记录序列分割成为若干子序列分别进行直接插入排序,待整个序列中的记录“基本有序”时,再对全体记录进行依次直接插入排序。
二、查找1、线性查找:t线性查找又称顺序查找,是一种最简单的查找算法。
从数据结构线形表的一端开始,顺序扫描,依次将扫描到的结点关键字与给定值k相比较,若相等则查找成功;若扫描结束仍没有找到关键字等于k的结点,则表示表中不存在关键字等于k的结点,查找失败。
2、二分查找:t二分查找又称折半查找,要求待查找的序列有序。
每次取中间位置的值与待查关键字比较,如果中间位置的值更大,则在前半部分循环这个查找的过程,如果中间位置的值更小,则在后半部分循环这个查找的过程。
3、二叉查找树:t二叉查找树(Binary Search Tree,简称BST),又被称为二叉搜索树、有序二叉树。
它是一棵空树或者是具有下列性质的二叉树:若任意节点的左子树不空,则左子树上所有结点的值均小于它的根结点的值;若任意节点的右子树不空,则右子树上所有结点的值均大于它的根结点的值;任意节点的左、右子树也分别为二叉查找树;没有键值相等的节点三、字符串处理1、KMP算法:tKMP算法是由Donald E.Knuth、Vaughn R. Pratt和James H.Morris三人于1977年提出的一种改进的字符串匹配算法,它利用匹配失败后的信息,尽量减少模式串与主串的匹配次数以达到快速匹配的目的。
java常用算法和数据结构

java常用算法和数据结构Java是一种面向对象的编程语言,它具有丰富的算法库和数据结构库,为开发人员提供了许多常用的算法和数据结构。
下面将介绍一些Java常用的算法和数据结构。
1.排序算法-冒泡排序(Bubble Sort):比较相邻的两个元素,如果顺序错误则交换位置,重复该过程直到整个序列有序。
-插入排序(Insertion Sort):将数组分为已排序和未排序两部分,每次从未排序部分取出一个元素,插入到已排序部分合适的位置。
-选择排序(Selection Sort):每次从未排序部分选择最小(或最大)的元素,放到已排序部分的末尾。
-快速排序(Quick Sort):选择一个基准元素,将数组分为两部分,小于基准的放左边,大于基准的放右边,递归地对左右两部分进行快速排序。
-归并排序(Merge Sort):将数组分为两部分,分别对每个子数组进行排序,然后合并两个有序子数组。
2.搜索算法-二分查找(Binary Search):对有序数组进行查找,每次将查找范围缩小一半。
-广度优先搜索(BFS):以树或图的形式搜索,从根节点开始,逐层扩展搜索范围,直到找到目标节点。
-深度优先搜索(DFS):以树或图的形式搜索,从根节点开始,逐个访问节点的所有邻居节点,直到找到目标节点或搜索完所有节点。
3.数据结构-数组(Array):一组按顺序存储的相同类型元素的集合,通过索引访问元素,可以快速访问元素,但插入和删除元素较慢。
-链表(Linked List):一组通过指针连接的节点存储的元素的集合,支持灵活的插入和删除操作,但访问元素较慢。
-栈(Stack):一种特殊的线性数据结构,遵循先进后出(LIFO)原则,只能在栈顶进行插入和删除操作。
-队列(Queue):一种特殊的线性数据结构,遵循先进先出(FIFO)原则,在队尾插入元素,队头删除元素。
-堆(Heap):一种特殊的树形数据结构,可以快速找到最小(或最大)元素,常用于实现优先队列。
图论中的常用经典算法

图论中的常用经典算法第一节最小生成树算法一、生成树的概念若图是连通的无向图或强连通的有向图,则从其中任一个顶点出发调用一次bfs或dfs后便可以系统地访问图中所有顶点;若图是有根的有向图,则从根出发通过调用一次dfs或bfs亦可系统地访问所有顶点。
在这种情况下,图中所有顶点加上遍历过程中经过的边所构成的子图称为原图的生成树。
对于不连通的无向图和不是强连通的有向图,若有根或者从根外的任意顶点出发,调用一次bfs或dfs后不能系统地访问所有顶点,而只能得到以出发点为根的连通分支(或强连通分支)的生成树。
要访问其它顶点则还需要从没有访问过的顶点中找一个顶点作为起始点,再次调用bfs 或dfs,这样得到的是生成森林。
由此可以看出,一个图的生成树是不唯一的,不同的搜索方法可以得到不同的生成树,即使是同一种搜索方法,出发点不同亦可导致不同的生成树。
如下图:但不管如何,我们都可以证明:具有n个顶点的带权连通图,其对应的生成树有n-1条边。
二、求图的最小生成树算法严格来说,如果图G=(V,E)是一个连通的无向图,则把它的全部顶点V和一部分边E’构成一个子图G’,即G’=(V, E’),且边集E’能将图中所有顶点连通又不形成回路,则称子图G’是图G的一棵生成树。
对于加权连通图,生成树的权即为生成树中所有边上的权值总和,权值最小的生成树称为图的最小生成树。
求图的最小生成树具有很高的实际应用价值,比如下面的这个例题。
例1、城市公交网[问题描述]有一张城市地图,图中的顶点为城市,无向边代表两个城市间的连通关系,边上的权为在这两个城市之间修建高速公路的造价,研究后发现,这个地图有一个特点,即任一对城市都是连通的。
现在的问题是,要修建若干高速公路把所有城市联系起来,问如何设计可使得工程的总造价最少。
[输入]n(城市数,1<=n<=100)e(边数)以下e行,每行3个数i,j,w ij,表示在城市i,j之间修建高速公路的造价。
算法学习中的经典算法实现与应用案例

算法学习中的经典算法实现与应用案例在计算机科学领域中,算法是解决问题的一种方法或步骤的描述。
它是一种确定性的、有限的、有效的计算过程,可以将输入转换为输出。
算法学习是计算机科学的基础,它涉及到各种经典算法的实现和应用。
一、排序算法排序算法是算法学习中最基础也是最常用的一类算法。
它们的目标是将一组元素按照特定的顺序进行排列。
其中,冒泡排序是最简单的一种排序算法,它的基本思想是通过相邻元素的比较和交换来实现排序。
另一个经典的排序算法是快速排序,它基于分治法的思想,通过选择一个基准元素将数组划分为两个子数组,然后递归地对子数组进行排序。
这些排序算法在实际应用中有着广泛的应用。
例如,在搜索引擎中,对搜索结果进行排序可以提高用户的搜索体验。
在电商平台中,对商品进行排序可以帮助用户更快地找到自己想要的产品。
此外,在数据分析和机器学习领域,排序算法也扮演着重要的角色。
二、图算法图算法是解决图论问题的一类算法。
图是由节点和边组成的数据结构,它可以用来表示各种关系和网络。
图算法的应用非常广泛,例如最短路径算法可以用来计算两个节点之间的最短路径,广度优先搜索算法可以用来遍历图中的所有节点,深度优先搜索算法可以用来查找图中的环路等等。
在社交网络中,图算法可以用来发现社区结构和关键节点。
在交通规划中,图算法可以用来寻找最佳路径和优化交通流量。
此外,图算法还被广泛应用于网络安全、电信网络优化、推荐系统等领域。
三、动态规划算法动态规划算法是一种通过将问题分解为子问题并存储子问题的解来解决复杂问题的方法。
它通常用于求解具有重叠子问题和最优子结构性质的问题。
动态规划算法的核心思想是通过利用已解决的子问题的解来构建更大的问题的解。
动态规划算法在实际应用中有着广泛的应用。
例如,在旅行商问题中,动态规划算法可以用来求解最短路径问题。
在背包问题中,动态规划算法可以用来求解最大价值问题。
此外,动态规划算法还被广泛应用于自然语言处理、图像处理、机器人路径规划等领域。
Dijkstra算法描述

Dijkstra算法描述目录一、算法概述1二、算法原理及计算12.1算法原理12.2计算过程22.3改良的算法〔Dijkstra-like〕分析5三、源码分析6四、接口调用7一、算法概述Dijkstra〔迪杰斯特拉〕算法是典型的单源最短路径计算算法,用于解决源点到所有结点最短路径计算的问题,它采用了分治和贪心〔动态规划的特殊形式〕的思想搜索全局最优解。
本系统采用了主流、开源的JAVA图论库——Jgrapht来解决源点到终点间所有可能路径输出的问题,它的核心计算引擎采用了一种Dijkstra-like算法,由经典的Dijkstra〔迪杰斯特拉〕算法演化和改良而来。
二、算法原理及计算2.1算法原理Dijkstra算法思想为:设(,)= 是带权有向图,V代表图中顶点集合,E代G V E表图中含权重的边集合。
将全部顶点集合V分成两组,第一组为已求出最短路径的顶点集合,用S表示〔初始时S中只有一个源点,以后每求得一条最短路径,就将该路径的终点参加到集合S中〕;第二组为其余待确定最短路径的顶点集合,用U表示。
按最短路径长度的递增次序依次把U集合的顶点逐个参加到S集合中,约束条件是保持从源点v到S中各顶点的最短路径长度不大于从源点v到U 中任何顶点的最短路径长度。
算法的终止条件是集合U为空集,即集合U的顶点全部参加到集合S中。
2.2计算过程以图1为例讨论Dijkstra算法的计算过程,即计算某源点到网络上其余各结点的最短路径,设源点为①,逐步搜索,每次找出一个结点到源点①的最短路径,直至完成所有结点的计算。
图1 带权有向图记()D v为源点①到某终点v的距离,是源点①到终点v某条路径的所有链路长度之和。
记(,)l w v 是源点w到终点v的距离。
Dijkstra算法归纳如下:S=,U是其余未确〔1〕初始化,令S是已求出最短路径的顶点集合,{}U=,可写出:定最短路径的顶点集合,{}(1,)()l v D v ⎧=⎨∞⎩(1-1) 公式1-1中,(1,)l v 是源点①与终点v 的直连路径长度,而∞代表源点①与终点v 不相连,初始化结果如表1所示;〔2〕遍历集合U 中的所有结点v 并计算[]min (),()(,)D v D w l w v + 。
dijkstra算法 java最短路径

dijkstra算法java最短路径Dijkstra算法是一种用于寻找图中两个节点之间最短路径的算法。
它采用的是贪心策略,将图中的节点分为两个集合:已访问节点集S和未访问节点集T。
算法从源节点开始,每次从T中选择到源节点距离最短的节点加入S集合,并更新S集合中各节点到源节点的最短路径。
直到T集合中的节点全部加入S集合,算法结束。
Dijkstra算法的Java实现如下:●public class Dijkstra{●public static void main(String[]args){●创建图●Graph graph=new Graph();●graph.addVertex("A");●graph.addVertex("B");●graph.addVertex("C");●graph.addEdge("A","B",10);●graph.addEdge("A","C",20);●graph.addEdge("B","C",30);●计算最短路径●dijkstra(graph,"A");}●private static void dijkstra(Graph graph,String startVertex){●初始化●Set<String>visited=new HashSet<>();●Map<String,Integer>distances=new HashMap<>();●for(String vertex:graph.getVertices()){●distances.put(vertex,Integer.MAX_VALUE);}●distances.put(startVertex,0);●遍历所有节点●for(String vertex:graph.getVertices()){●找到未访问节点中距离源节点最小的节点●String nearestVertex=findNearestVertex(distances,visited);●将该节点加入已访问节点集合●visited.add(nearestVertex);●更新该节点到其他节点的最短路径●for(String neighbor:graph.getAdjacentVertices(nearestVertex)){●intnewDistance=distances.get(nearestVertex)+graph.getEdgeWeight(nearestVertex,neighbor ●if(newDistance<distances.get(neighbor)){●distances.put(neighbor,newDistance);}}}●输出结果●System.out.println("从"+startVertex+"到其他节点的最短路径:");●for(String vertex:graph.getVertices()){●System.out.println(vertex+"的最短路径是:"+distances.get(vertex));}}●private static String findNearestVertex(Map<String,Integer>distances,Set<String>visited){●int minDistance=Integer.MAX_VALUE;●String nearestVertex=null;●for(String vertex:distances.keySet()){●if(!visited.contains(vertex)&&distances.get(vertex)<minDistance){●minDistance=distances.get(vertex);●nearestVertex=vertex;}}●return nearestVertex;}}该算法的工作原理如下:1.初始化距离表,将所有节点的距离初始化为无穷大。
Floyd算法求解最短路径问题(完整程序代码)
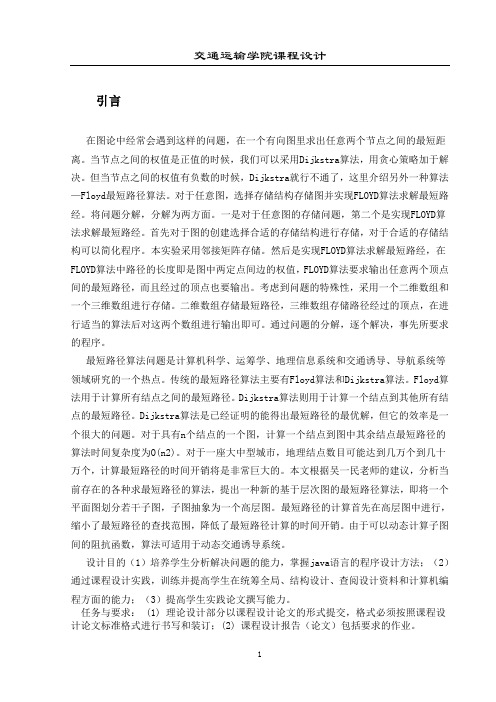
引言在图论中经常会遇到这样的问题,在一个有向图里求出任意两个节点之间的最短距离。
当节点之间的权值是正值的时候,我们可以采用Dijkstra算法,用贪心策略加于解决。
但当节点之间的权值有负数的时候,Dijkstra就行不通了,这里介绍另外一种算法—Floyd最短路径算法。
对于任意图,选择存储结构存储图并实现FLOYD算法求解最短路经。
将问题分解,分解为两方面。
一是对于任意图的存储问题,第二个是实现FLOYD算法求解最短路经。
首先对于图的创建选择合适的存储结构进行存储,对于合适的存储结构可以简化程序。
本实验采用邻接矩阵存储。
然后是实现FLOYD算法求解最短路经,在FLOYD算法中路径的长度即是图中两定点间边的权值,FLOYD算法要求输出任意两个顶点间的最短路径,而且经过的顶点也要输出。
考虑到问题的特殊性,采用一个二维数组和一个三维数组进行存储。
二维数组存储最短路径,三维数组存储路径经过的顶点,在进行适当的算法后对这两个数组进行输出即可。
通过问题的分解,逐个解决,事先所要求的程序。
最短路径算法问题是计算机科学、运筹学、地理信息系统和交通诱导、导航系统等领域研究的一个热点。
传统的最短路径算法主要有Floyd算法和Dijkstra算法。
Floyd算法用于计算所有结点之间的最短路径。
Dijkstra算法则用于计算一个结点到其他所有结点的最短路径。
Dijkstra算法是已经证明的能得出最短路径的最优解,但它的效率是一个很大的问题。
对于具有n个结点的一个图,计算一个结点到图中其余结点最短路径的算法时间复杂度为O(n2)。
对于一座大中型城市,地理结点数目可能达到几万个到几十万个,计算最短路径的时间开销将是非常巨大的。
本文根据吴一民老师的建议,分析当前存在的各种求最短路径的算法,提出一种新的基于层次图的最短路径算法,即将一个平面图划分若干子图,子图抽象为一个高层图。
最短路径的计算首先在高层图中进行,缩小了最短路径的查找范围,降低了最短路径计算的时间开销。
java 二维数组的最短路径算法
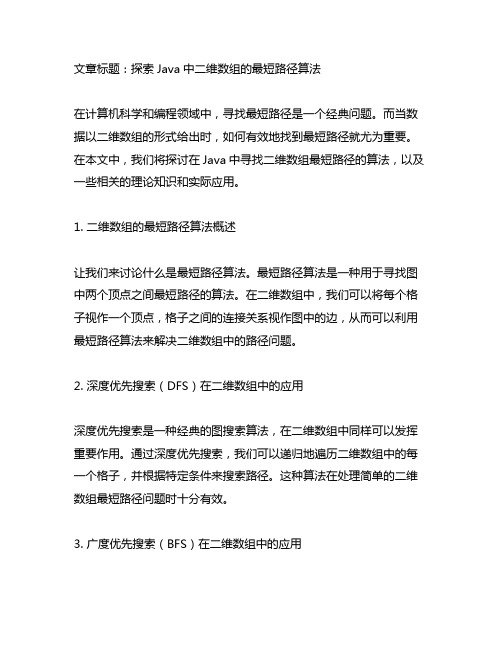
文章标题:探索Java中二维数组的最短路径算法在计算机科学和编程领域中,寻找最短路径是一个经典问题。
而当数据以二维数组的形式给出时,如何有效地找到最短路径就尤为重要。
在本文中,我们将探讨在Java中寻找二维数组最短路径的算法,以及一些相关的理论知识和实际应用。
1. 二维数组的最短路径算法概述让我们来讨论什么是最短路径算法。
最短路径算法是一种用于寻找图中两个顶点之间最短路径的算法。
在二维数组中,我们可以将每个格子视作一个顶点,格子之间的连接关系视作图中的边,从而可以利用最短路径算法来解决二维数组中的路径问题。
2. 深度优先搜索(DFS)在二维数组中的应用深度优先搜索是一种经典的图搜索算法,在二维数组中同样可以发挥重要作用。
通过深度优先搜索,我们可以递归地遍历二维数组中的每一个格子,并根据特定条件来搜索路径。
这种算法在处理简单的二维数组最短路径问题时十分有效。
3. 广度优先搜索(BFS)在二维数组中的应用与深度优先搜索类似,广度优先搜索也是一种用于图搜索的经典算法。
在二维数组中,广度优先搜索可以非常高效地找到最短路径,特别是在求解迷宫、寻找连通性等问题时具有很强的应用能力。
4. Dijkstra算法在二维数组中的应用Dijkstra算法是一种经典的最短路径算法,通过计算起始点到所有其他点的最短路径来找到最优解。
在二维数组中,我们可以利用Dijkstra算法来解决复杂的最短路径问题,例如地图路径规划、网络数据传输等。
5. Floyd-Warshall算法在二维数组中的应用Floyd-Warshall算法是一种动态规划算法,用于求解图中所有顶点对之间的最短路径。
在二维数组中,Floyd-Warshall算法可以高效地计算出任意两个格子之间的最短路径,对于解决复杂的二维数组路径问题十分重要。
总结回顾在本文中,我们讨论了在Java中寻找二维数组最短路径的算法。
通过深度优先搜索、广度优先搜索、Dijkstra算法和Floyd-Warshall算法,我们可以高效地解决各种二维数组路径问题,为实际应用提供了重要的理论支持。
java算法大全

java算法大全
Java算法大全可以包含许多不同的算法,包括排序算法、搜索算法、图算法等等。
下面是一些常见和常用的Java算法示例:
1. 排序算法:
- 冒泡排序
- 插入排序
- 选择排序
- 快速排序
- 归并排序
- 堆排序
2. 搜索算法:
- 二分查找
- 广度优先搜索(BFS)
- 深度优先搜索(DFS)
3. 图算法:
- 最短路径算法(如Dijkstra算法、Floyd-Warshall算法)
- 最小生成树算法(如Prim算法、Kruskal算法)
- 拓扑排序算法
4. 动态规划算法:
- 背包问题
- 最长上升子序列(LIS)问题
- 最长公共子序列(LCS)问题
5. 字符串算法:
- 字符串匹配(如暴力匹配、KMP算法、Boyer-Moore
算法)
- 字符串排序(如基数排序)
6. 数值算法:
- 求解线性方程组
- 求解方程的根
- 求解数值积分
以上只是一些常见的算法示例,Java算法的范围非常广泛,涉及到各种不同的问题和应用领域。
如果你有特定的算法
需求,可以提供更具体的问题描述,我可以为你提供更详
细的解答。
java邻接矩阵计算连通分量

java邻接矩阵计算连通分量以Java邻接矩阵计算连通分量为主题,我们将介绍如何使用Java 编程语言来实现连通分量的计算,并且通过邻接矩阵来表示图的结构。
在此之前,我们先来了解一下什么是连通分量。
连通分量是图论中的一个概念,用来描述图中的若干个顶点组成的子集,其中任意两个顶点之间都存在路径。
换句话说,连通分量是指图中可以互相到达的顶点的集合。
在计算连通分量之前,我们需要先了解一下邻接矩阵的概念。
邻接矩阵是一种常用的图的表示方法,它是一个二维矩阵,其中矩阵的行和列分别表示图中的顶点,矩阵的元素表示顶点之间的边的关系。
如果两个顶点之间存在边,则对应的邻接矩阵元素为1;如果两个顶点之间不存在边,则对应的邻接矩阵元素为0。
接下来我们将介绍如何使用Java编程语言来实现邻接矩阵的计算连通分量。
我们需要定义一个Graph类来表示图的结构。
这个类需要包含一个邻接矩阵作为成员变量,并且提供一些方法来操作邻接矩阵。
```javapublic class Graph {private int[][] adjacencyMatrix;public Graph(int numVertices) {adjacencyMatrix = new int[numVertices][numVertices]; }public void addEdge(int source, int destination) {adjacencyMatrix[source][destination] = 1;adjacencyMatrix[destination][source] = 1;}public void removeEdge(int source, int destination) {adjacencyMatrix[source][destination] = 0;adjacencyMatrix[destination][source] = 0;}public boolean isConnected(int source, int destination) {return adjacencyMatrix[source][destination] == 1;}// 其他操作方法...}```在Graph类中,我们使用一个二维数组adjacencyMatrix来表示邻接矩阵。
趣学算法——约瑟夫环问题(java版)

趣学算法——约瑟夫环问题(java版)1 什么是约瑟夫环问题?约瑟夫,是⼀个古犹太⼈,曾经在⼀次罗马叛乱中担任将军,后来战败,他和朋友及另外39个⼈躲在⼀⼝井⾥,但还是被发现了。
罗马⼈表⽰只要投降就不死,约瑟夫想投降,可是其他⼈坚决不同意。
怎么办呢,他想到⼀个主意:让41个⼈围成⼀个圆圈,从第⼀个⼈开始报数,数到3的那个⼈被旁边的⼈杀死。
这样就可以避免⾃杀了,因为犹太⼈的信仰是禁⽌⾃杀的。
结果⼀群⼈杀来杀去最后只剩下两个了,就是约瑟夫和他朋友,于是两⼈愉快地去投降了。
约瑟夫和朋友站在什么位置才保住了性命呢,这就是我们今天要讲的约瑟夫环问题。
2 问题的重要性这是个BAT常⽤⾯试题,⽽且本质上是⼀个游戏,可以⼴泛应⽤于⽣活中,⼯作⽣活好帮⼿就是它了。
3 约瑟夫环抽象问题这个问题实际在讲:N个⼈围成⼀圈,第⼀个⼈从1开始报数,报M的被杀掉,下⼀个⼈接着从1开始报,循环反复,直到剩下最后⼀个,那最后胜利者的初始位置在哪⾥?模拟流程:假如有5个⼈报数,报到3被杀,情况如下A B C1 D E (初始位置,C第⼀个被杀)D E A2 B (C死后的第⼆次排位,A第⼆个被杀)B D E3 (A死后的第三次排位,E第三个被杀)B4 D (E死后的第四次排位,B第四个被杀)D (D留在了最后,初始位置是4)解决⽅法:1 循环遍历法public static int josephus(int n, int m) {//n个⼈, 0 1 2..n-1int[] people = new int[n];//⼈的索引int index = -1;//报数记录, 1 2 3..mint count = 0;//剩余⼈数初始值为nint remain = n;//为了找到最后⼀个幸存者的位置,假设所有⼈都会被杀while (remain > 0) {index++; //找到报数的⼈if (index == n) { //所有⼈遍历⼀圈后从头遍历index = 0;}if (people[index] == -1) { //如果当前的⼈被杀跳过continue;}count++; //报数if (count == m) {people[index] = -1; //报数到m后杀⼈count = 0; //报数重置remain--; //剩余⼈数递减}}return index;}将41传⼊⽅法后,可得结果为30, 因为是从0开始计数,所以等价于现实世界的第31位。
图论中的最短路径问题及其算法实现

图论中的最短路径问题及其算法实现引言:图论是离散数学的一个重要分支,研究的是表示物体间关系的图及其性质、结构和相关算法。
其中,最短路径问题是图论中的一类经典问题,它在实际应用中有着广泛的应用价值。
本文将探讨最短路径问题的定义、性质以及常见的算法实现,旨在帮助读者深入了解这一重要的图论问题。
一、最短路径问题的定义和特性在图论中,最短路径问题是指在有向图或无向图中找到连接两个顶点之间路径长度最短的路径。
根据具体的问题,最短路径可以有不同的定义,如边的权重、顶点的权重等。
下面介绍最常见的两种最短路径问题:单源最短路径和全源最短路径。
1. 单源最短路径问题单源最短路径问题是指在给定图中,从一个源顶点出发,找到到达其余所有顶点的最短路径。
其中,最短路径可以使用不同的度量标准来定义,如路径长度、路径权重等。
研究单源最短路径问题的常见算法有迪杰斯特拉算法和贝尔曼-福特算法。
2. 全源最短路径问题全源最短路径问题是指在给定图中,找到任意两个顶点之间的最短路径。
全源最短路径问题可以通过多次应用单源最短路径算法来解决。
在常见的全源最短路径算法中,弗洛伊德算法和约翰逊算法是两种常用的解法。
二、常见最短路径算法的实现1. 迪杰斯特拉算法迪杰斯特拉算法是用于解决单源最短路径问题的一种贪心算法。
其主要思想是通过不断更新从源顶点到其他顶点的距离,直到找到最短路径。
具体实现步骤如下:- 初始化距离数组dist,将源顶点到其他顶点的距离初始化为无穷大(或一个很大的数),源顶点的距离初始化为0。
- 在未访问顶点集合中选择距离最短的顶点,将其标记为已访问。
- 更新源顶点到其他顶点的距离,如果经过当前顶点的路径比之前记录的距离要短,则更新距离数组dist。
- 重复上述步骤,直到所有顶点都被标记为已访问。
2. 贝尔曼-福特算法贝尔曼-福特算法是一种用于解决单源最短路径问题的动态规划算法。
与迪杰斯特拉算法不同的是,贝尔曼-福特算法可以处理带有负权边的图。
最短路径dijkstra算法 java

文章主题:深入探讨最短路径Dijkstra算法在Java中的应用在计算机科学领域中,图论和算法一直是研究的热点之一。
而最短路径问题,则是图论中的一个重要问题,具有广泛的应用价值。
为了解决最短路径问题,Dijkstra算法应运而生,它是一种十分高效的算法,在实际项目中经常会用到。
本文将深入探讨最短路径Dijkstra算法在Java中的应用,分别从算法原理、Java代码实现、应用实例等方面展开讨论。
1. 算法原理最短路径Dijkstra算法是由荷兰计算机科学家艾兹格·戴克斯特拉于1956年提出的,用于解决带权重的有向图中的最短路径问题。
该算法使用了广度优先搜索解决问题的思想,并且在搜索的过程中动态地维护每个顶点到起点的最短距离。
在算法执行过程中,会逐步确定起点到各个顶点的最短路径,直到确定所有顶点的最短路径为止。
通过松弛操作来逐步缩小起点到各个顶点的距离,最终得到最短路径。
2. Java代码实现为了更好地理解Dijkstra算法在Java中的实现,我们首先需要定义图的数据结构,并实现松弛操作和最短路径搜索的过程。
在Java中,可以使用邻接矩阵或邻接表来表示图的结构,然后通过优先队列来维护顶点之间的最短距离。
在代码实现中,我们可以通过循环遍历各个顶点,并根据最短路径的规则来更新各个顶点的距离,直到得到最终的最短路径。
以下是一个简单的最短路径Dijkstra算法的Java代码示例:```java// Java实现最短路径Dijkstra算法public class DijkstraAlgorithm {public void dijkstra(int[][] graph, int start) {int n = graph.length;int[] dist = new int[n];boolean[] visited = new boolean[n];Arrays.fill(dist, Integer.MAX_VALUE);dist[start] = 0;for (int i = 0; i < n - 1; i++) {int u = minDistance(dist, visited);visited[u] = true;for (int v = 0; v < n; v++) {if (!visited[v] && graph[u][v] != 0 && dist[u] != Integer.MAX_VALUE && dist[u] + graph[u][v] < dist[v]) {dist[v] = dist[u] + graph[u][v];}}}printSolution(dist);}private int minDistance(int[] dist, boolean[] visited) { int min = Integer.MAX_VALUE, minIndex = -1;for (int i = 0; i < dist.length; i++) {if (!visited[i] && dist[i] <= min) {min = dist[i];minIndex = i;}}return minIndex;}private void printSolution(int[] dist) {System.out.println("顶点最短路径");for (int i = 0; i < dist.length; i++) {System.out.println(i + " " + dist[i]);}}}```3. 应用实例最短路径Dijkstra算法在实际项目中有着广泛的应用。
图论的基本算法及性质
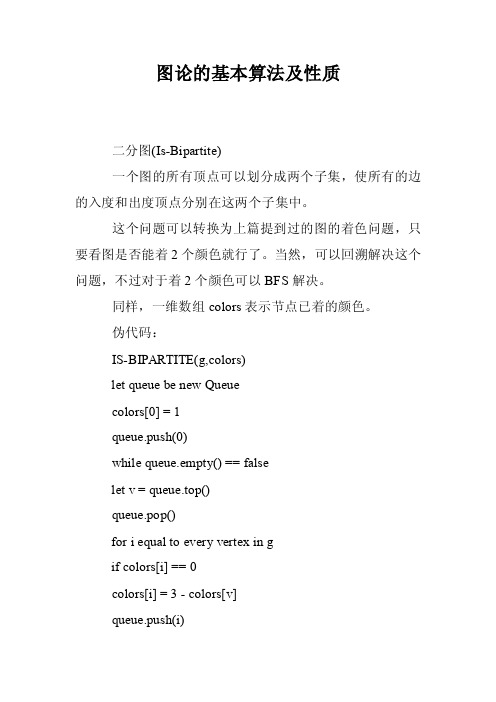
图论的基本算法及性质二分图(Is-Bipartite)一个图的所有顶点可以划分成两个子集,使所有的边的入度和出度顶点分别在这两个子集中。
这个问题可以转换为上篇提到过的图的着色问题,只要看图是否能着2个颜色就行了。
当然,可以回溯解决这个问题,不过对于着2个颜色可以BFS解决。
同样,一维数组colors表示节点已着的颜色。
伪代码:IS-BIPARTITE(g,colors)let queue be new Queuecolors[0] = 1queue.push(0)while queue.empty() == falselet v = queue.top()queue.pop()for i equal to every vertex in gif colors[i] == 0colors[i] = 3 - colors[v]queue.push(i)else if colors[i] == colors[v]return falseendendreturn true时间复杂度:Θ(V+E),V表示顶点的个数,E表示边的个数DFS改良(DFS-Improve)上篇文章提到过,搜索解空间是树形的,也就是在说BFS和DFS。
那么在对图进行BFS和DFS有什么区别呢,这个问题要从解空间角度去理解。
对图进行BFS的解空间是一颗树,可叫广度优先树。
而DFS是多棵树构成的森林,可叫深度优先森林。
这里要对DFS进行小小的改良,它的性质会对解多个问题会很有帮助。
原版DFS搜索的时候,会先遍历本顶点,再递归遍历临接的顶点。
DFS改良希望能先递归遍历临接的顶点,再遍历本顶点,并且按遍历顺序逆序存储起来。
伪代码:DFS-IMPROVE(v,visited,stack)visited[v] = truefor i equal to every vertex adjacent to vif visited[i] == falseDFS-IMPROVE(i,visited,stack)endstack.push(v)这个改良版DFS有个很有用的性质就是,对于两个顶点A、B,存在A到B的路径,而不存在B到A的路径,则从记录的顺序中取出的时候,一定会先取出顶点A,再取出顶点B。
dijkstra最短路径经典例题 java
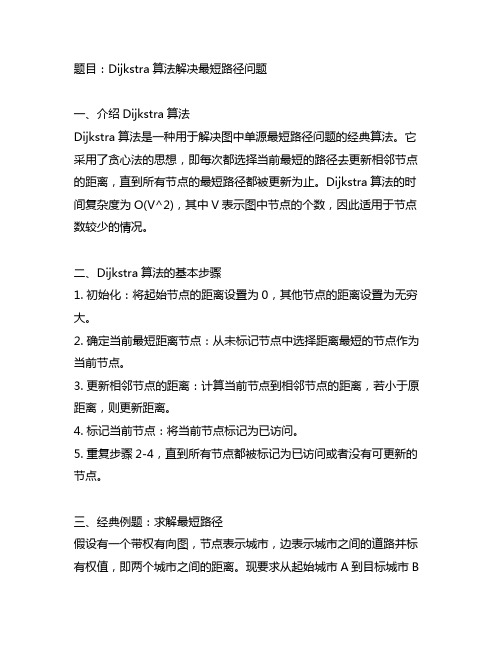
题目:Dijkstra算法解决最短路径问题一、介绍Dijkstra算法Dijkstra算法是一种用于解决图中单源最短路径问题的经典算法。
它采用了贪心法的思想,即每次都选择当前最短的路径去更新相邻节点的距离,直到所有节点的最短路径都被更新为止。
Dijkstra算法的时间复杂度为O(V^2),其中V表示图中节点的个数,因此适用于节点数较少的情况。
二、Dijkstra算法的基本步骤1. 初始化:将起始节点的距离设置为0,其他节点的距离设置为无穷大。
2. 确定当前最短距离节点:从未标记节点中选择距离最短的节点作为当前节点。
3. 更新相邻节点的距离:计算当前节点到相邻节点的距离,若小于原距离,则更新距离。
4. 标记当前节点:将当前节点标记为已访问。
5. 重复步骤2-4,直到所有节点都被标记为已访问或者没有可更新的节点。
三、经典例题:求解最短路径假设有一个带权有向图,节点表示城市,边表示城市之间的道路并标有权值,即两个城市之间的距离。
现要求从起始城市A到目标城市B的最短路径。
四、Java代码实现Dijkstra算法```javaimport java.util.Arrays;public class DijkstraAlgorithm {private static final int INF = Integer.MAX_VALUE; // 无穷大表示两节点不直接相连public int[] dijkstra(int[][] graph, int start) {int n = graph.length;int[] distance = new int[n]; // 存储起始节点到各节点的最短距离boolean[] visited = new boolean[n]; // 记录节点是否已被访问// 初始化distance数组Arrays.fill(distance, INF);distance[start] = 0;// 循环更新最短距离for (int i = 0; i < n - 1; i++) {int minIndex = findMinIndex(distance, visited); // 找到未被访问且距禃最短的节点visited[minIndex] = true;for (int j = 0; j < n; j++) {if (!visited[j] graph[minIndex][j] != INFdistance[minIndex] + graph[minIndex][j] < distance[j]) {distance[j] = distance[minIndex] +graph[minIndex][j];}}}return distance;}private int findMinIndex(int[] distance, boolean[] visited) { int minDist = INF, minIndex = -1;for (int i = 0; i < distance.length; i++) {if (!visited[i] distance[i] < minDist) {minDist = distance[i];minIndex = i;}}return minIndex;}public static void m本人n(String[] args) {int[][] graph = {{0, 6, 3, INF, INF},{INF, 0, INF, 1, INF},{INF, 2, 0, 1, 1},{INF, INF, INF, 0, 3},{INF, INF, INF, INF, 0}};DijkstraAlgorithm dijkstra = new DijkstraAlgorithm();int[] distance = dijkstra.dijkstra(graph, 0);for (int i = 0; i < distance.length; i++) {System.out.println("节点0到节点" + i + "的最短距禿:" + (distance[i] == INF ? "不可达" : distance[i]));}}}```五、代码解析1. 首先定义了一个常量INF表示无穷大,在实际应用中可以根据具体情况设置为合适的数值。
java矩阵最短路径算法

java矩阵最短路径算法Java矩阵最短路径算法矩阵最短路径算法是一种常见的图算法,它用于寻找两个顶点之间的最短路径。
在某些应用场景中,我们需要找到一个矩阵中两个给定顶点之间的最短路径,这时就可以使用矩阵最短路径算法。
在Java中,我们可以使用邻接矩阵来表示一个图,其中矩阵中的元素表示两个顶点之间的边的权重。
邻接矩阵是一个二维数组,其中矩阵的行和列分别表示图的顶点,而矩阵中的元素表示两个顶点之间的边的权重。
如果两个顶点之间没有边,则对应的矩阵元素为无穷大。
矩阵最短路径算法的基本思想是动态规划。
我们定义一个二维数组dist,其中dist[i][j]表示顶点i到顶点j的最短路径的长度。
初始时,我们将dist[i][j]初始化为邻接矩阵中对应的元素值。
接下来,我们使用动态规划的思想来更新dist数组。
假设我们已经求得了顶点0到顶点k的最短路径长度dist[0][k],以及顶点k到顶点j的最短路径长度dist[k][j]。
那么,顶点0到顶点j的最短路径长度就可以通过dist[0][k] + dist[k][j]来更新。
如果dist[0][k] + dist[k][j]的值小于dist[0][j],我们就更新dist[0][j]的值。
为了找到顶点0到顶点j的最短路径,我们需要遍历矩阵中的所有顶点k,然后更新dist[0][j]的值。
具体的算法如下:```javapublic static void shortestPath(int[][] matrix, int start, int end) {int numVertices = matrix.length;int[][] dist = new int[numVertices][numVertices];// 初始化dist数组for (int i = 0; i < numVertices; i++) {for (int j = 0; j < numVertices; j++) {dist[i][j] = matrix[i][j];}}// 动态规划更新dist数组for (int k = 0; k < numVertices; k++) {for (int i = 0; i < numVertices; i++) {for (int j = 0; j < numVertices; j++) {if (dist[i][k] + dist[k][j] < dist[i][j]) { dist[i][j] = dist[i][k] + dist[k][j];}}}}System.out.println("从顶点" + start + "到顶点" + end + "的最短路径长度为:" + dist[start][end]);}```在上述代码中,我们首先获取矩阵的大小,并创建一个和矩阵同样大小的dist数组。
java贪心算法几个经典例子

java贪心算法几个经典例子
1. 零钱兑换问题
给定面额为1、5、10、25的硬币,以及一个需要兑换的金额,问最少需要多少硬币才能兑换成功。
解法:每次选择面额最大的硬币兑换,直到兑换完毕为止。
2. 分糖果问题
有m个糖果,要分给n个孩子,每个孩子至少分到一个糖果,且每个孩子分到的糖果数应尽量相近,求最小的糖果差。
解法:将m个糖果按照大小排序,依次将糖果分给n个孩子,每次将糖果分给最少的孩子。
3. 区间覆盖问题
给定多个区间,问最少需要选多少个区间才能覆盖全集。
解法:每次选择与当前未被覆盖的部分交集最大的区间添加到答案中,直到所有部分被覆盖完毕为止。
4. 任务调度问题
有n个任务需要完成,每个任务需要占用不同的时间,同时每个任务都有一个
最后期限,问如何调度任务才能最大程度地避免超时。
解法:将所有任务按照最后期限排序,依次将任务安排到最后期限之前的最近空闲时间点,尽量将任务时间安排得紧凑。
与图论相关的算法

广度优先搜索
procedure bfs(i:integer); var p:arcptr;
closed,open:integer; q:array[1..maxn] of integer; begin 访问并处理顶点i; map[i].visited:=true; 顶点i进入队列q; closed:=0; open:=1; repeat
编号都不超过k的路径。 递推公式:
t(k)[i,j]= t(k-1)[i,j] or (t(k-1)[i,k] and t(k-1)[k,j])
Johnson算法
Johnson算法常用于求顶点个数较多的稀 疏图的每对点间最短路问题。
感兴趣的同学请参阅《国际信息学奥林 匹克竞赛指导——实用算法的分析与程 序设计》
inc(closed); v:=q[closed]; p:=map[v].firstarc; while p<>nil do begin
if map[p^.v].visited=false then begin 访问并处理顶点q^.v; map[q^.v].visited=true; inc(open); q[open]:=q^.v; end;
q:=q^.nextarc; end; until closed=open; end;
计算连通分支数
count:=0; for i:=1 to n do
map[i].visited:=false; for i:=1 to n do
if map[i].visited=false do begin inc(count); dfs(i); end;
java实现leaderrank算法

Leaderrank算法是一种用于社交网络中影响力分析的算法,它可以帮助我们找到在网络中具有领导地位的个体。
而在实际应用中,我们可以通过Java语言来实现Leaderrank算法。
本文将详细介绍Leaderrank算法的原理和Java实现方法。
一、Leaderrank算法原理1.1 算法简介Leaderrank算法是一种用于计算网路中节点影响力的算法,基于图论中的PageRank算法进行了优化。
它通过分析节点之间的连接关系和节点的行为特征,来评估节点在网络中的影响力大小,从而找出网络中的领导者。
1.2 算法步骤Leaderrank算法的计算分为以下几个步骤:(1)构建网络图:将网络中的节点和节点之间的连接关系表示为一个图。
(2)初始化节点权重:给每个节点初始化一个权重值,表示节点的初始影响力。
(3)计算转移矩阵:根据节点之间的连接关系,构建一个转移矩阵,表示节点之间的信息传递概率。
(4)迭代计算节点影响力:通过多次迭代计算,更新节点的权重值,直到达到收敛状态。
(5)得出结果:根据节点的最终权重值,确定网络中的领导者节点。
1.3 算法特点Leaderrank算法具有以下特点:(1)考虑节点行为特征:不仅考虑节点之间的连接关系,还考虑节点本身的行为特征,如发帖、转发、评论等。
(2)精准度高:通过多次迭代计算,可以得到较为准确的节点影响力值。
(3)适用范围广:适用于各类社交网络、信息传播网络等场景。
二、Java实现Leaderrank算法2.1 算法框架设计在Java中实现Leaderrank算法,我们可以按照以下框架设计程序:(1)构建图模型:使用Java中的图论库,如JUNG库,构建网络图模型。
(2)初始化节点权重:为每个节点初始化一个权重值。
(3)计算转移矩阵:根据节点之间的连接关系,计算得到转移矩阵。
(4)迭代计算节点权重:通过多次迭代计算,更新节点的权重值,直到收敛。
(5)输出结果:输出节点的最终权重值,确定网络中的领导者节点。
java 力扣经典算法思路总结

java 力扣经典算法思路总结1.暴力枚举暴力枚举是一种朴素的算法思路,适用于解决一些简单的问题。
在 Java 中,可以使用循环和条件语句来实现暴力枚举。
例如,在力扣题目中,可以使用嵌套循环来求解两个数组的交集、最长公共子序列等问题。
2.递归递归是一种常用的算法思路,适用于解决一些分治的问题。
在 Java 中,可以通过定义递归函数来实现递归算法。
例如,在力扣题目中,可以使用递归来求解二叉树的高度、反转链表等问题。
3.动态规划动态规划是一种经典的算法思路,适用于解决一些最优化的问题。
在 Java 中,可以通过定义数组和循环来实现动态规划算法。
例如,在力扣题目中,可以使用动态规划来求解最长公共子序列、背包问题等问题。
4.深度优先搜索深度优先搜索是一种常用的搜索算法,适用于解决一些图论问题。
在 Java 中,可以通过定义递归函数和栈来实现深度优先搜索算法。
例如,在力扣题目中,可以使用深度优先搜索来求解图的连通性、染色问题等问题。
5.广度优先搜索广度优先搜索是一种常用的搜索算法,适用于解决一些图论问题。
在 Java 中,可以通过定义队列来实现广度优先搜索算法。
例如,在力扣题目中,可以使用广度优先搜索来求解图的连通性、最短路径等问题。
6.双指针双指针是一种常用的算法思路,适用于解决一些区间查询和区间更新的问题。
在 Java 中,可以通过定义两个指针来实现双指针算法。
例如,在力扣题目中,可以使用双指针来求解滑动窗口的最大值、链表中重复的元素等问题。
7.排序与查找排序与查找是计算机科学中最基本的算法思路之一。
在 Java 中,可以使用Arrays 类的 sort 方法实现排序,使用 ArrayList 类的 indexOf 方法实现查找。
例如,在力扣题目中,可以使用排序来解决三数之和问题、使用查找来解决两数之和问题。
总之,以上这些算法思路是 Java 在力扣题目中的经典应用,掌握这些算法思路可以有效地解决各种不同类型的问题。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
1.最短路的笛杰斯特拉算法/**** @author Administrator*///这个算法用来解决无向图中任意两点的最短路径,同时输出路径(起点到所有点的) public class MinPath {public static String dijkstra(int[][] W1, int start, int end) {System.out.println("起点:" + start + "终点:" + end);boolean[] isLabel = new boolean[W1[0].length];// 是否标号int[] indexs = new int[W1[0].length];// 所有标号的点的下标集合,以标号的先后顺序进行存储,实际上是一个以数组表示的栈int i_count = -1;// 栈的顶点int[] distance = W1[start].clone();// v0到各点的最短距离的初始值int index = start;// 从初始点开始int presentShortest = 0;// 当前临时最短距离indexs[++i_count] = index;// 把已经标号的下标存入下标集中isLabel[index] = true;while (i_count < W1[0].length) {// 第一步:得到与原点最近的某个点int min = Integer.MAX_V ALUE;for (int i = 0; i < distance.length; i++) {if (!isLabel[i] && distance[i] != -1 && i != index) {// 如果到这个点有边,并且没有被标号if (distance[i] < min) {min = distance[i];index = i;// 把下标改为当前下标}}}i_count = i_count + 1;if (i_count == W1[0].length) {break;}isLabel[index] = true;// 对点进行标号indexs[i_count] = index;// 把已经标号的下标存入下标集中if (W1[indexs[i_count - 1]][index] == -1|| presentShortest + W1[indexs[i_count - 1]][index] > distance[index]) { // 如果两个点没有直接相连,或者两个点的路径大于最短路径presentShortest = distance[index];} else {presentShortest += W1[indexs[i_count - 1]][index];}// 第二步:加入vi后,重新计算distance中的距离for (int i = 0; i < distance.length; i++) {// 如果vi到那个点有边,则v0到后面点的距离加if (distance[i] == -1 && W1[index][i] != -1) {// 如果以前不可达,则现在可达了distance[i] = presentShortest + W1[index][i];} else if (W1[index][i] != -1 && presentShortest + W1[index][i] < distance[i]) {// 如果以前可达,但现在的路径比以前更短,则更换成更短的路径distance[i] = presentShortest + W1[index][i];}}}getRoute(W1, indexs, end);return "最短距离是:" + (distance[end] - distance[start]);}public static void main(String[] args) {// 建立一个权值矩阵int[][] W1 = { // 测试数据1{0, 1, 4, -1, -1, -1},{1, 0, 2, 7, 5, -1},{4, 2, 0, -1, 1, -1},{-1, 7, -1, 0, 3, 2},{-1, 5, 1, 3, 0, 6},{-1, -1, -1, 2, 6, 0}};// System.out.println("f" + W1[0][4]);int[][] W = { // 测试数据2{0, 1, 3, 4},{1, 0, 2, -1},{3, 2, 0, 5},{4, -1, 5, 0}};System.out.println(dijkstra(W1, 5, 0)); // (int[][] W1, int start, int end) }// indexs:1,0,2,4,3,5 放顶点的顺序// end:最后要的顶点名称:5// routeLength:长度:8/*** seven 输出路径(起点到所有点的)*/public static String getRoute(int[][] WW, int[] indexs, int end) {String[] routeArray = new String[indexs.length];for (int i = 0; i < routeArray.length; i++) {routeArray[i] = "";}//自己的路线routeArray[indexs[0]] = indexs[0] + "";for (int i = 1; i < indexs.length; i++) {//看该点与前面所有点的连接线中的最短路径,然后得到该最短路径到底是连接了哪个点,进而此点的route就是找出那点的route+此点int[] thePointDis = WW[indexs[i]];int prePoint = 0;int tmp = 9999;for (int j = 0; j < thePointDis.length; j++) {boolean chooseFlag = false;//边的距离最短,而且,所连的点在前面的点当中for (int m = 0; m < i; m++) {if (j == indexs[m]) {chooseFlag = true;}}if (chooseFlag == false) {continue;}if (thePointDis[j] < tmp && thePointDis[j] > 0) {prePoint = j;tmp = thePointDis[j];}}routeArray[indexs[i]] = routeArray[prePoint] + indexs[i];}for (int i = 0; i < routeArray.length; i++) {System.out.println(routeArray[i]);}return "";}}2.最小生成树的Kruskal算法/**** @author Administrator*/public class MinTree {/*** @param args*/private int[][] List_Graph;//图的邻接矩阵private int[] Label;private int[] Weight;private int[] Index_1;private int[] Index_2;private String Result;public Class_Kruskal()//使用构造函数进行初始化数据{List_Graph = new int[][]{{0, 1, 4, 5},{1, 0, 32768, 2},{4, 32768, 0, 3},{5, 2, 3, 0}};//初始化graphic中点和点的距离,32767表示距离无穷大//另外一个测试用例是/*{0,1,2,32767,32767},{1,0,2,4,3},{2,2,0,4,4},{32767,4,4,0,2},{32767,3,4,2,0}*/Label = new int[List_Graph.length];for (int i = 0; i < Label.length; i++)//初始化标记{Label[i] = i;}int j = (int) (Label.length + 1) * Label.length / 2;//这里应该是-1吧,完全图的边数Weight = new int[j];//用于存储待排序边的权值,数组长度m=(n+1)*n*0.5,其中节点个数为nIndex_1 = new int[j];//用于存储边的两个节点Index_2 = new int[j];Result = "最小生成树的边是:" + "\n";//记录最小生成树的边}public String Get_Result()//获得变量Result{return Result;}//把边按权排序,graphic是List_Graphicpublic int[] sort() {int[] a;int index = 0;for (int i = 0; i < Label.length; i++) {for (int j = i + 1; j < Label.length; j++) {if (List_Graph[i][j] < 32767) {Weight[index] = List_Graph[i][j];Index_1[index] = i;Index_2[index] = j;index = index + 1;}}}a = new int[index - 1];a = Address_Sort(Weight, Weight.length);return a;}public int[] Address_Sort(int[] a, int n)//地址排序{int[] Res = new int[n];for (int i = 0; i < n; i++) {Res[i] = i;}int t;int k;for (int j = 0; j < n - 1; j++) {for (int i = 0; i < n - j - 1; i++) {if (a[i] >= a[i + 1]) {//冒泡法k = a[i];a[i] = a[i + 1];a[i + 1] = k;t = Res[i];Res[i] = Res[i + 1];Res[i + 1] = t;}}}return Res;}public void Min_Tree()//求最小生成树{int[] tag = new int[Weight.length];tag = sort();int i = 0;while (!Judge(Label))//Judge函数判断标记是否都是0{if (Label[Index_1[tag[i]]] != Label[Index_2[tag[i]]]) {//两个点不同Result = Result + Index_1[tag[i]] + "---" + Index_2[tag[i]] + "\n";if (Label[Index_1[tag[i]]] < Label[Index_2[tag[i]]]) {for (int k = 0; k < Label.length; k++) {if (Label[k] == Label[Index_2[tag[i]]]) {Label[k] = Label[Index_1[tag[i]]];}}} else {for (int k = 0; k < Label.length; k++) {if (Label[k] == Label[Index_1[tag[i]]]) {Label[k] = Label[Index_2[tag[i]]];}}}} else {i = i + 1;}}}public boolean Judge(int[] a)//判断标记是否都是0{for (int i = 0; i < a.length; i++) {if (a[i] != 0) {return false;}}return true;}public static void main(String[] args) //主函数{Class_Kruskal CK = new Class_Kruskal();CK.Min_Tree();System.out.println(CK.Get_Result());} }。