opencv实现分水岭,金字塔,均值漂移算法进行分割
基于OpenCV的视频道路车辆检测与跟踪

基于OpenCV的视频道路车辆检测与跟踪近年来,智能驾驶技术飞速进步,视频道路车辆检测与跟踪技术成为了分外重要的探究方向之一。
OpenCV是一个广泛应用于计算机视觉领域的开源库,可以提供各种强大的图像处理和分析功能。
本文将介绍技术,并展示其在智能交通系统中的应用。
1. 引言在智能交通系统中,视频监控系统可以实时得到道路上的交通信息,并援助提高交通安全性和效率。
其中,车辆检测与跟踪是视频监控系统中一个重要的环节。
本文将使用OpenCV实现车辆检测与跟踪算法,并探讨其在实际应用中的效果和问题。
2. 车辆检测车辆检测是智能交通系统中关键的一环。
起首,需要将视频图像进行预处理,包括去噪、图像增强和尺寸归一化等。
接下来,可以使用机器进修算法或深度进修算法训练一个目标检测模型,来检测图像中的车辆位置。
其中,传统的机器进修算法如Haar特征分类器、HOG+SVM等已经被证明有效。
此外,深度进修算法如YOLO、Faster R-CNN等也能够在车辆检测任务中取得不俗效果。
3. 车辆跟踪车辆跟踪是在车辆检测的基础上,通过追踪连续的视频帧来实现对车辆的跟踪。
在OpenCV中,有多种跟踪算法可供选择,如均值漂移、卡尔曼滤波、基于流的光流跟踪等。
这些算法可以依据车辆的运动特点和场景要求,选择最适合的算法进行车辆跟踪。
4. 算法实现与优化基于OpenCV,可以通过编程实现车辆检测与跟踪算法。
在实现过程中,需要注意优化算法的效率和准确性。
起首,可以通过图像金字塔技术来提高算法的检测和跟踪速度。
其次,可以利用GPU加速和多线程技术来提高算法的处理速度。
此外,还可以借助OpenCL等并行计算框架来加速算法的执行。
5. 试验与结果分析为了验证基于OpenCV的车辆检测与跟踪技术的有效性,进行了一系列试验。
试验数据包括不同场景下的道路视频,通过与手动标注的真值进行比较,评估了算法的检测准确度和跟踪精度。
试验结果表明,基于OpenCV的车辆检测与跟踪技术在不同场景下都具备一定的检测和跟踪能力。
分水岭 算法
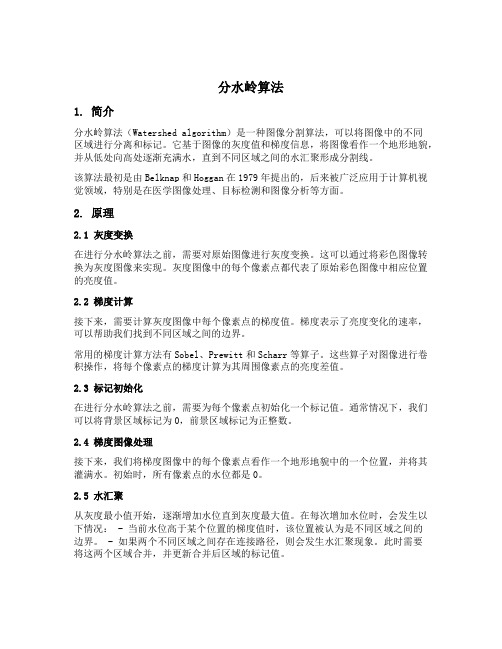
分水岭算法1. 简介分水岭算法(Watershed algorithm)是一种图像分割算法,可以将图像中的不同区域进行分离和标记。
它基于图像的灰度值和梯度信息,将图像看作一个地形地貌,并从低处向高处逐渐充满水,直到不同区域之间的水汇聚形成分割线。
该算法最初是由Belknap和Hoggan在1979年提出的,后来被广泛应用于计算机视觉领域,特别是在医学图像处理、目标检测和图像分析等方面。
2. 原理2.1 灰度变换在进行分水岭算法之前,需要对原始图像进行灰度变换。
这可以通过将彩色图像转换为灰度图像来实现。
灰度图像中的每个像素点都代表了原始彩色图像中相应位置的亮度值。
2.2 梯度计算接下来,需要计算灰度图像中每个像素点的梯度值。
梯度表示了亮度变化的速率,可以帮助我们找到不同区域之间的边界。
常用的梯度计算方法有Sobel、Prewitt和Scharr等算子。
这些算子对图像进行卷积操作,将每个像素点的梯度计算为其周围像素点的亮度差值。
2.3 标记初始化在进行分水岭算法之前,需要为每个像素点初始化一个标记值。
通常情况下,我们可以将背景区域标记为0,前景区域标记为正整数。
2.4 梯度图像处理接下来,我们将梯度图像中的每个像素点看作一个地形地貌中的一个位置,并将其灌满水。
初始时,所有像素点的水位都是0。
2.5 水汇聚从灰度最小值开始,逐渐增加水位直到灰度最大值。
在每次增加水位时,会发生以下情况: - 当前水位高于某个位置的梯度值时,该位置被认为是不同区域之间的边界。
- 如果两个不同区域之间存在连接路径,则会发生水汇聚现象。
此时需要将这两个区域合并,并更新合并后区域的标记值。
2.6 分割结果当水位达到最大值时,分割过程结束。
此时所有不同区域之间都有了明确的边界,并且每个区域都有了唯一的标记值。
3. 算法优缺点3.1 优点•分水岭算法是一种无监督学习方法,不需要依赖任何先验知识或训练数据。
•可以对图像中的任意区域进行分割,不受形状、大小和数量的限制。
分水岭算法步骤
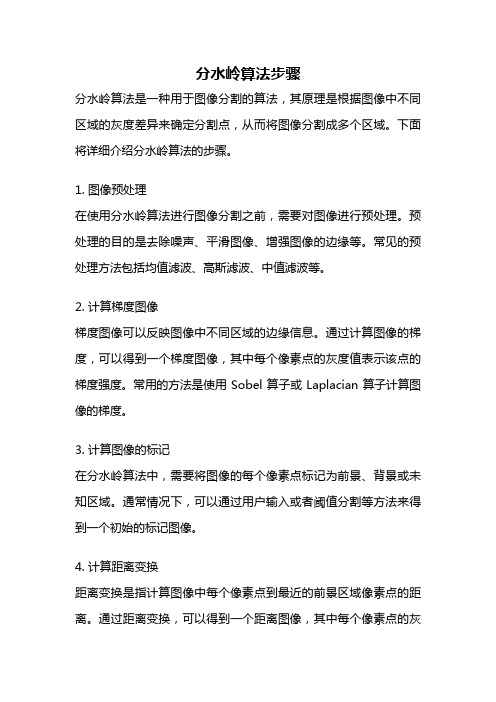
分水岭算法步骤分水岭算法是一种用于图像分割的算法,其原理是根据图像中不同区域的灰度差异来确定分割点,从而将图像分割成多个区域。
下面将详细介绍分水岭算法的步骤。
1. 图像预处理在使用分水岭算法进行图像分割之前,需要对图像进行预处理。
预处理的目的是去除噪声、平滑图像、增强图像的边缘等。
常见的预处理方法包括均值滤波、高斯滤波、中值滤波等。
2. 计算梯度图像梯度图像可以反映图像中不同区域的边缘信息。
通过计算图像的梯度,可以得到一个梯度图像,其中每个像素点的灰度值表示该点的梯度强度。
常用的方法是使用Sobel算子或Laplacian算子计算图像的梯度。
3. 计算图像的标记在分水岭算法中,需要将图像的每个像素点标记为前景、背景或未知区域。
通常情况下,可以通过用户输入或者阈值分割等方法来得到一个初始的标记图像。
4. 计算距离变换距离变换是指计算图像中每个像素点到最近的前景区域像素点的距离。
通过距离变换,可以得到一个距离图像,其中每个像素点的灰度值表示该点到最近前景像素点的距离。
5. 寻找种子点种子点是指位于图像中的一些特殊点,用于标记不同的区域。
通常情况下,种子点位于图像的前景和背景之间的边界处。
可以通过阈值分割等方法来寻找种子点。
6. 计算分水岭变换分水岭变换是一种基于图像的梯度和距离变换来确定图像分割的方法。
在分水岭变换中,首先将种子点填充到距离图像中,然后通过计算梯度和距离变换来确定分割线的位置,从而将图像分割为多个区域。
7. 后处理在得到分割后的图像之后,可能会存在一些图像分割不准确或者存在过度分割的问题。
因此,需要进行一些后处理的操作,如去除小的区域、合并相邻的区域等,以得到最终的分割结果。
总结起来,分水岭算法是一种基于图像的梯度和距离变换来进行图像分割的算法。
通过对图像进行预处理、计算梯度图像、计算标记、计算距离变换、寻找种子点、计算分水岭变换和后处理等步骤,可以得到一个准确的图像分割结果。
分水岭算法在图像分割领域具有广泛的应用,并且在处理复杂图像时能够取得较好的效果。
Matlab的标记分水岭分割算法

Matlab的标记分⽔岭分割算法1 综述Separating touching objects in an image is one of the more difficult image processing operations. The watershed transform is often applied to this problem. The watershed transform finds "catchment basins"(集⽔盆) and "watershed ridge lines"(⼭脊线) in an image by treating it as a surface where light pixels are high and dark pixels are low.如果图像中的⽬标物体是连接在⼀起的,则分割起来会更困难,分⽔岭分割算法经常⽤于处理这类问题,通常会取得⽐较好的效果。
分⽔岭分割算法把图像看成⼀幅“地形图”,其中亮度⽐较强的区域像素值较⼤,⽽⽐较暗的区域像素值较⼩,通过寻找“汇⽔盆地”和“分⽔岭界限”,对图像进⾏分割。
Segmentation using the watershed transform works better if you can identify, or "mark," foreground objects and background locations. Marker-controlled watershed segmentation follows this basic procedure:直接应⽤分⽔岭分割算法的效果往往并不好,如果在图像中对前景对象和背景对象进⾏标注区别,再应⽤分⽔岭算法会取得较好的分割效果。
基于标记控制的分⽔岭分割⽅法有以下基本步骤:1. Compute a segmentation function. This is an image whose dark regions are the objects you are trying to segment.1.计算分割函数。
opencv 项目案例

opencv 项目案例OpenCV是一个开源的计算机视觉库,它提供了丰富的函数和算法,用于处理和分析图像和视频数据。
下面是一些基于OpenCV的项目案例以及相关参考内容,希望对您有所帮助。
1. 人脸识别人脸识别是计算机视觉领域的一项重要任务,可以应用于安防监控、人机交互等领域。
参考内容可以包括:- 人脸检测:使用OpenCV的人脸检测器(如Haar级联分类器)对输入图像进行人脸检测。
- 特征提取:使用OpenCV的特征提取算法(如局部二值模式直方图)从人脸图像中提取特征向量。
- 训练分类器:使用OpenCV的机器学习算法(如支持向量机)来训练一个人脸分类器。
- 人脸识别:使用训练好的分类器对新的人脸图像进行识别。
2. 手势识别手势识别可以应用于人机交互、虚拟现实等领域。
参考内容可以包括:- 手势检测:使用OpenCV的背景减除算法和运动跟踪算法对输入视频中的手部进行检测和跟踪。
- 手势识别:根据手势的形状、轮廓、手指数量等特征,使用OpenCV的图像处理和机器学习算法对手势进行识别。
- 手势控制:根据识别出的手势,实现对计算机或设备的控制(如控制鼠标、游戏操作等)。
3. 目标检测与跟踪目标检测与跟踪可以应用于安防监控、自动驾驶等领域。
参考内容可以包括:- 目标检测:使用OpenCV的目标检测器(如级联分类器、深度学习模型)对输入图像或视频中的目标进行检测。
- 目标跟踪:根据检测到的目标,使用OpenCV的运动跟踪算法(如卡尔曼滤波、均值漂移)对目标进行跟踪。
- 多目标跟踪:对于多个目标,使用OpenCV的多目标跟踪算法(如多种滤波方法的组合)进行跟踪与管理。
4. 图像处理与增强图像处理与增强可以应用于图像编辑、美颜相机等领域。
参考内容可以包括:- 图像滤波:使用OpenCV的滤波算法(如均值滤波、高斯滤波)对图像进行平滑处理或边缘增强。
- 图像增强:使用OpenCV的直方图均衡化、自适应直方图均衡化等算法对图像进行增强。
分水岭 算法
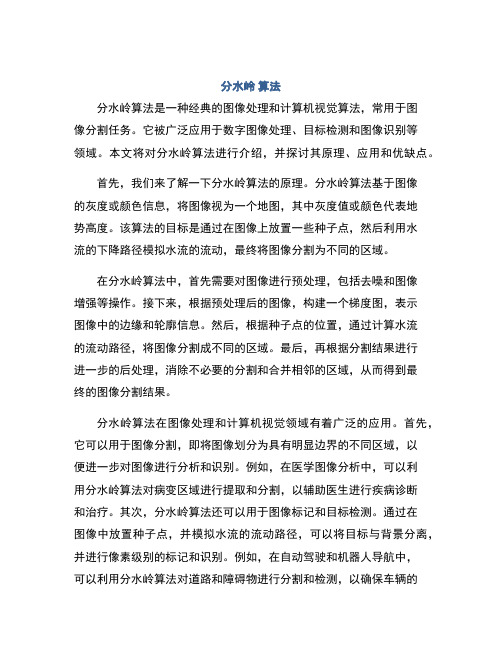
分水岭算法分水岭算法是一种经典的图像处理和计算机视觉算法,常用于图像分割任务。
它被广泛应用于数字图像处理、目标检测和图像识别等领域。
本文将对分水岭算法进行介绍,并探讨其原理、应用和优缺点。
首先,我们来了解一下分水岭算法的原理。
分水岭算法基于图像的灰度或颜色信息,将图像视为一个地图,其中灰度值或颜色代表地势高度。
该算法的目标是通过在图像上放置一些种子点,然后利用水流的下降路径模拟水流的流动,最终将图像分割为不同的区域。
在分水岭算法中,首先需要对图像进行预处理,包括去噪和图像增强等操作。
接下来,根据预处理后的图像,构建一个梯度图,表示图像中的边缘和轮廓信息。
然后,根据种子点的位置,通过计算水流的流动路径,将图像分割成不同的区域。
最后,再根据分割结果进行进一步的后处理,消除不必要的分割和合并相邻的区域,从而得到最终的图像分割结果。
分水岭算法在图像处理和计算机视觉领域有着广泛的应用。
首先,它可以用于图像分割,即将图像划分为具有明显边界的不同区域,以便进一步对图像进行分析和识别。
例如,在医学图像分析中,可以利用分水岭算法对病变区域进行提取和分割,以辅助医生进行疾病诊断和治疗。
其次,分水岭算法还可以用于图像标记和目标检测。
通过在图像中放置种子点,并模拟水流的流动路径,可以将目标与背景分离,并进行像素级别的标记和识别。
例如,在自动驾驶和机器人导航中,可以利用分水岭算法对道路和障碍物进行分割和检测,以确保车辆的安全行驶。
此外,分水岭算法还可以用于图像修复和图像融合等应用,提高图像质量和视觉效果。
然而,分水岭算法也存在一些局限性和挑战。
首先,由于算法本身是基于像素的,对图像中的噪声和弱边缘比较敏感,容易产生过分割和欠分割的问题。
因此,对于复杂的图像或具有相似纹理的区域,分水岭算法可能无法准确地将其分割开来。
其次,分水岭算法在计算复杂度和时间消耗方面比较高,对于大规模图像和实时应用来说,可能会造成较高的计算负担。
因此,对于实时应用和大规模图像处理,需要进一步对算法进行改进和优化。
分水岭算法
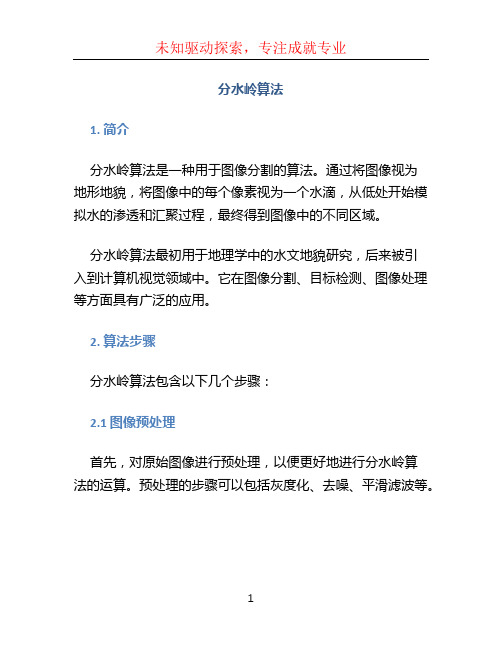
分水岭算法1. 简介分水岭算法是一种用于图像分割的算法。
通过将图像视为地形地貌,将图像中的每个像素视为一个水滴,从低处开始模拟水的渗透和汇聚过程,最终得到图像中的不同区域。
分水岭算法最初用于地理学中的水文地貌研究,后来被引入到计算机视觉领域中。
它在图像分割、目标检测、图像处理等方面具有广泛的应用。
2. 算法步骤分水岭算法包含以下几个步骤:2.1 图像预处理首先,对原始图像进行预处理,以便更好地进行分水岭算法的运算。
预处理的步骤可以包括灰度化、去噪、平滑滤波等。
2.2 计算图像的梯度梯度表示图像中每个像素的边缘强度。
通过计算图像的梯度,可以找到图像中的边缘和纹理信息。
常用的计算梯度的方法包括Sobel、Prewitt等算子。
2.3 寻找图像中的标记点标记点是分水岭算法中的关键概念,表示图像中的起始点或分水岭点。
标记点的选取对于最终分割结果有很大的影响。
通常情况下,可以通过阈值分割、连通区域分析等方法寻找图像中的标记点。
2.4 计算距离变换图距离变换图是一个将图像中每个像素替换为其与最近标记点之间距离的图像。
通过计算距离变换图,可以评估每个像素到最近标记点的距离。
2.5 计算分水岭线分水岭线是指图像中的边缘或过渡区域,它将不同的区域分隔开来。
通过计算距离变换图,可以找到图像中的分水岭线。
2.6 执行分水岭漫水算法最后,执行分水岭漫水算法,将图像中的每个像素与标记点进行比较,并根据像素值和距离变换图进行分割。
分水岭漫水算法会将图像中的不同区域分割成若干个连通区域。
3. 算法优缺点3.1 优点•分水岭算法可以对图像进行多种类型的分割,包括分割不完全的区域和不规则形状的目标。
•分水岭算法不需要预先知道目标的数量。
•分水岭算法可以自动识别图像中的背景和前景。
3.2 缺点•分水岭算法对于噪声和纹理较强的图像分割效果不理想。
•分水岭算法对于图像中的非连通区域分割效果差。
•分水岭算法具有较高的计算复杂度,对于大规模图像处理较为困难。
基于OpenCV的视频道路车辆检测与跟踪

基于OpenCV的视频道路车辆检测与跟踪摘要:随着城市交通的不断发展,道路车辆的数量不断增加,为了保证交通的安全性和顺畅性,对道路上的车辆进行检测和跟踪显得尤为重要。
本文基于OpenCV库,研究了一种基于图像处理技术的视频道路车辆检测与跟踪方法,通过对视频帧进行预处理、车辆区域的提取和目标跟踪的算法实现,可以有效地检测和跟踪道路上的车辆,具有较高的准确性和稳定性。
1. 引言随着城市的发展和人民生活水平的提高,道路交通日益拥挤,车辆数量不断增加,给交通管理工作带来了巨大的挑战。
为了解决交通拥堵和事故隐患问题,对道路上的车辆进行有效的检测和跟踪至关重要。
基于计算机视觉技术的图像处理方法成为道路车辆检测与跟踪的重要手段。
2. 方法2.1 视频帧预处理在进行车辆检测与跟踪之前,首先需要对视频帧进行预处理。
主要包括图像的灰度化、平滑处理、图像二值化等步骤。
通过灰度化处理,可以将彩色图像转换为灰度图像,便于后续的车辆检测。
平滑处理通过滤波器对图像进行处理,减少图像中的噪声。
图像二值化将灰度图像转换为二值图像,将车辆与背景分离出来,便于车辆区域的提取。
2.2 车辆区域的提取在预处理之后,需要对图像中的车辆区域进行提取。
通过阈值分割方法,可以将图像中的车辆区域与背景分离开来。
同时,为了减少车辆区域中的噪声,可以使用形态学操作进行进一步的处理。
利用腐蚀和膨胀操作,可以使车辆区域更加完整和连续。
2.3 目标跟踪在提取了车辆区域之后,需要对车辆进行跟踪。
本文采用的方法是基于均值漂移算法的目标跟踪方法。
通过计算车辆区域的直方图信息,结合均值漂移算法的迭代法则,可以实现对车辆的跟踪。
在迭代过程中,通过调整窗口的大小和位置,可以对车辆进行准确地跟踪。
3. 实验与结果为了验证本文方法的有效性,我们在OpenCV的环境下进行了一系列的实验。
实验采用了多段道路视频,包含了不同道路条件和车辆数量的场景。
实验结果表明,本文方法可以准确地检测和跟踪道路上的车辆,且具有较高的稳定性。
分水岭分割算法matlab
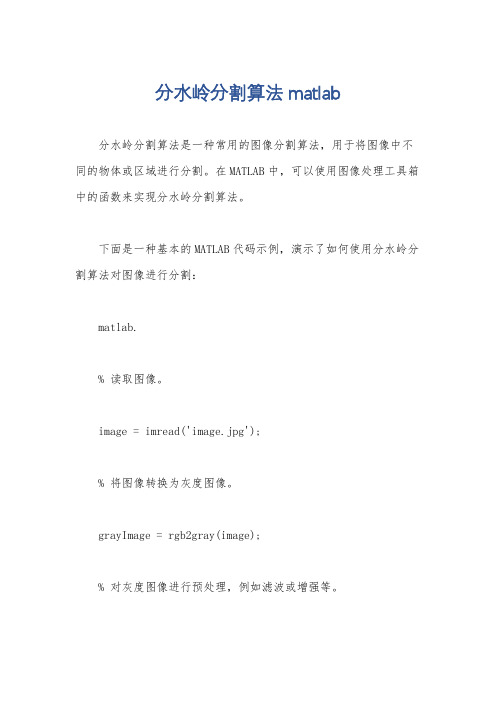
分水岭分割算法matlab分水岭分割算法是一种常用的图像分割算法,用于将图像中不同的物体或区域进行分割。
在MATLAB中,可以使用图像处理工具箱中的函数来实现分水岭分割算法。
下面是一种基本的MATLAB代码示例,演示了如何使用分水岭分割算法对图像进行分割:matlab.% 读取图像。
image = imread('image.jpg');% 将图像转换为灰度图像。
grayImage = rgb2gray(image);% 对灰度图像进行预处理,例如滤波或增强等。
% 计算图像的梯度。
gradientImage = imgradient(grayImage);% 使用分水岭算法进行图像分割。
segmented = watershed(gradientImage);% 将分割结果可视化。
figure;imshow(label2rgb(segmented));% 可以选择性地将不同的分割区域标记出来。
hold on;boundaries = imdilate(segmented == 0, ones(1)); imshow(boundaries, 'Color', 'red');% 添加标题和标签。
title('分水岭分割结果');上述代码中,首先读取了一张图像,并将其转换为灰度图像。
然后对灰度图像进行预处理,例如滤波或增强等操作。
接下来,计算图像的梯度,这将有助于找到图像中的边缘和区域边界。
最后,使用`watershed`函数进行图像分割,并将分割结果可视化。
需要注意的是,分水岭分割算法对图像的预处理和参数选择非常重要,可以根据具体的应用场景进行调整和优化。
此外,MATLAB 还提供了其他图像分割算法和工具函数,可以根据实际需求选择合适的方法进行图像分割。
希望以上内容能够对你理解分水岭分割算法在MATLAB中的应用有所帮助。
如有更多问题,请随时提问。
分水岭分割算法
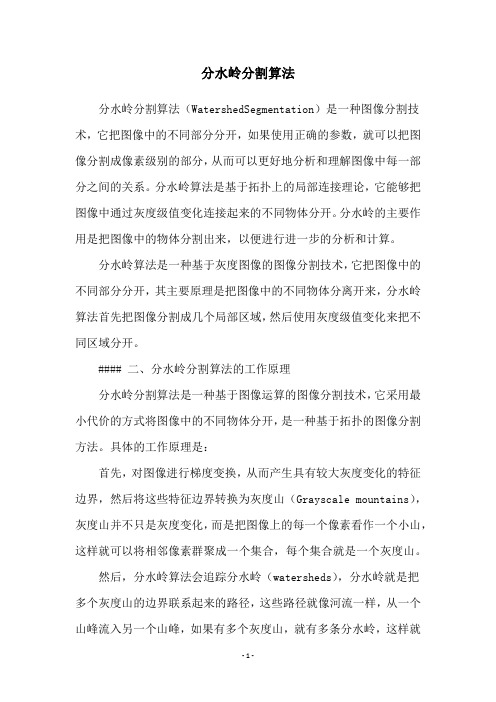
分水岭分割算法分水岭分割算法(WatershedSegmentation)是一种图像分割技术,它把图像中的不同部分分开,如果使用正确的参数,就可以把图像分割成像素级别的部分,从而可以更好地分析和理解图像中每一部分之间的关系。
分水岭算法是基于拓扑上的局部连接理论,它能够把图像中通过灰度级值变化连接起来的不同物体分开。
分水岭的主要作用是把图像中的物体分割出来,以便进行进一步的分析和计算。
分水岭算法是一种基于灰度图像的图像分割技术,它把图像中的不同部分分开,其主要原理是把图像中的不同物体分离开来,分水岭算法首先把图像分割成几个局部区域,然后使用灰度级值变化来把不同区域分开。
#### 二、分水岭分割算法的工作原理分水岭分割算法是一种基于图像运算的图像分割技术,它采用最小代价的方式将图像中的不同物体分开,是一种基于拓扑的图像分割方法。
具体的工作原理是:首先,对图像进行梯度变换,从而产生具有较大灰度变化的特征边界,然后将这些特征边界转换为灰度山(Grayscale mountains),灰度山并不只是灰度变化,而是把图像上的每一个像素看作一个小山,这样就可以将相邻像素群聚成一个集合,每个集合就是一个灰度山。
然后,分水岭算法会追踪分水岭(watersheds),分水岭就是把多个灰度山的边界联系起来的路径,这些路径就像河流一样,从一个山峰流入另一个山峰,如果有多个灰度山,就有多条分水岭,这样就可以把图像中的不同物体分隔开来。
最后,通过对每条分水岭的分析,就可以把图像中的不同物体分开,达到图像分割的目的。
#### 三、分水岭分割算法的优点分水岭分割算法是一种图像分割技术,它可以把图像分割成几个局部区域,然后使用灰度级值变化来把不同区域分开,从而把图像中的不同物体分离开来。
分水岭分割算法有以下优点:(1)计算简单:分水岭分割算法的计算是基于拓扑的局部连接理论,而不是复杂的理论,因此它的运算速度快,计算量少,是一种高效的图像分割技术。
分水岭分割算法及其基本步骤

分水岭分割算法及其基本步骤
宝子,今天咱来唠唠分水岭分割算法哈。
分水岭分割算法呢,就像是在一幅图像的“地形”上找分界线。
想象一下图像的灰度值就像地形的高度,灰度高的地方像山峰,灰度低的地方像山谷。
这个算法的目标呀,就是找到那些把不同“区域”分开的“分水岭”。
比如说一幅有多个物体的图像,它能把每个物体所在的区域分开来。
那它的基本步骤大概是这样滴。
先得把图像看成是一个拓扑地貌。
这就好比把图像变成了一个有山有谷的小世界。
然后呢,要确定一些“种子点”,这些种子点就像是每个区域的起始点。
比如说,你想把图像里的一个圆形物体和周围分开,就在圆形物体内部选个点当种子点。
接着呀,从这些种子点开始,像水从源头往外流一样,根据图像的灰度信息往外扩展。
灰度变化平缓的地方就容易被包含进来,而灰度变化突然的地方,就像是遇到了悬崖或者堤坝,就成了可能的分界线。
在这个过程中呢,算法会不断判断哪些区域该合并,哪些该分开。
就像你在整理东西,把同类的放在一起,不同类的分开。
最后呢,就形成了分割后的各个区域啦。
这个算法可有趣了,就像是在图像的小世界里当一个规划师,给每个物体或者区域划分地盘呢。
不过它也有小缺点哦,有时候可能会对噪声比较敏感,就像你在一个有点乱的地方划分区域,那些小干扰就可能让划分不那么准确啦。
但总体来说,在图像分割领域,分水岭分割算法还是很厉害的一个小能手哦。
。
基于分水岭分割算法

基于分水岭分割算法
分水岭分割算法是一种常用的图像分割算法,也称为基于区域的分割算法。
它基于图像中不同区域的灰度差异,通过将图像看作是一幅地形图,将图像中的每个像素视为地形上的一个点,通过计算该点的梯度来确定其高度。
然后,根据图像的高度差异将图像分割成多个不同的区域。
分水岭分割算法的主要思想是首先将图像中的每个像素看作是一个水滴,并将水滴放在图像中的极小值点上。
然后根据像素之间的连通性以及梯度信息,逐步合并水滴,最终得到图像的分割结果。
算法步骤如下:
1. 预处理:对图像进行去噪处理,例如使用高斯滤波器。
2. 计算梯度:计算图像中每个像素的梯度值,一般使用Sobel
算子或Laplacian算子。
3. 标记种子点:根据梯度信息找到图像中的极小值点,并将这些点作为种子点。
4. 标记像素:通过种子点进行扩张,将每个像素标记为其所属的种子点。
5. 构建水流线:将图像中未标记的像素分配到最近的种子点,形成水流线。
6. 汇合水流线:当水流线汇合时,形成边界,将水流线连接到其最近的汇合点。
7. 分割图像:根据水流线和汇合点,将图像分割成多个不同的区域。
分水岭分割算法能够有效地处理图像中的多个前景物体以及复杂背景,但在一些情况下可能会出现过分割或欠分割的问题,需要根据具体应用场景进行调优和改进。
opencv 粘连区域分割算法

opencv 粘连区域分割算法OpenCV是一个强大的计算机视觉库,提供了许多图像处理和计算机视觉算法。
对于粘连区域分割,你可以使用OpenCV中的一些算法,如区域生长、分水岭算法等。
这里,我将介绍一种基于区域生长和分水岭算法的粘连区域分割方法。
1. 区域生长:区域生长是一种基于像素相似性的图像分割方法,通过定义一个种子像素和一个阈值,与种子像素相似度较高的像素会被合并到一起。
对于粘连区域,我们可以通过设定一个较小的阈值,使得相邻的像素被合并到一起。
```pythonimport cv2import numpy as np# 加载图像img = cv2.imread('image.jpg', 0)# 区域生长kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (5, 5)) dilated = cv2.dilate(img, kernel)seeds = np.where(dilated == 255)area = len(seeds[0])print('Number of seeds: ', area)# 将区域生长得到的区域作为分水岭算法的种子点seeds = np.vstack((seeds, np.where(dilated == 0)[0][0,:] +np.arange(area))).Tseeds = np.hstack((seeds, np.where(dilated ==0)[1][:,0].reshape(-1,1)))seeds = np.vstack((seeds, np.where(dilated ==0)[1][:,:,np.newaxis]))seeds = np.unique(seeds, axis=0)# 分水岭算法分割markers = cv2.watershed(img, seeds)img[markers == -1] = [255, 0, 0] # 将未标记的区域填充为白色```这段代码首先使用区域生长算法找到所有相邻的像素,并将这些像素作为分水岭算法的种子点。
分水岭算法解析
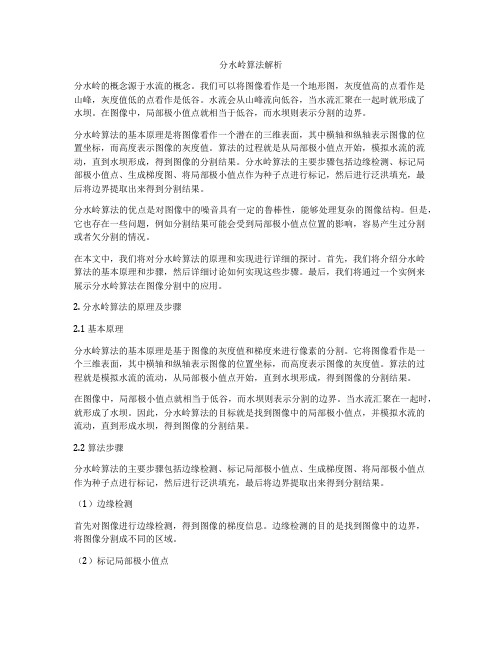
分水岭算法解析分水岭的概念源于水流的概念。
我们可以将图像看作是一个地形图,灰度值高的点看作是山峰,灰度值低的点看作是低谷。
水流会从山峰流向低谷,当水流汇聚在一起时就形成了水坝。
在图像中,局部极小值点就相当于低谷,而水坝则表示分割的边界。
分水岭算法的基本原理是将图像看作一个潜在的三维表面,其中横轴和纵轴表示图像的位置坐标,而高度表示图像的灰度值。
算法的过程就是从局部极小值点开始,模拟水流的流动,直到水坝形成,得到图像的分割结果。
分水岭算法的主要步骤包括边缘检测、标记局部极小值点、生成梯度图、将局部极小值点作为种子点进行标记,然后进行泛洪填充,最后将边界提取出来得到分割结果。
分水岭算法的优点是对图像中的噪音具有一定的鲁棒性,能够处理复杂的图像结构。
但是,它也存在一些问题,例如分割结果可能会受到局部极小值点位置的影响,容易产生过分割或者欠分割的情况。
在本文中,我们将对分水岭算法的原理和实现进行详细的探讨。
首先,我们将介绍分水岭算法的基本原理和步骤,然后详细讨论如何实现这些步骤。
最后,我们将通过一个实例来展示分水岭算法在图像分割中的应用。
2. 分水岭算法的原理及步骤2.1 基本原理分水岭算法的基本原理是基于图像的灰度值和梯度来进行像素的分割。
它将图像看作是一个三维表面,其中横轴和纵轴表示图像的位置坐标,而高度表示图像的灰度值。
算法的过程就是模拟水流的流动,从局部极小值点开始,直到水坝形成,得到图像的分割结果。
在图像中,局部极小值点就相当于低谷,而水坝则表示分割的边界。
当水流汇聚在一起时,就形成了水坝。
因此,分水岭算法的目标就是找到图像中的局部极小值点,并模拟水流的流动,直到形成水坝,得到图像的分割结果。
2.2 算法步骤分水岭算法的主要步骤包括边缘检测、标记局部极小值点、生成梯度图、将局部极小值点作为种子点进行标记,然后进行泛洪填充,最后将边界提取出来得到分割结果。
(1)边缘检测首先对图像进行边缘检测,得到图像的梯度信息。
【OpenCV学习笔记】之图像金字塔(ImagePyramid)

【OpenCV学习笔记】之图像金字塔(ImagePyramid)一、尺度调整顾名思义,即对源图像的尺寸进行放大或者缩小变换。
在opencv 里面可以用resize函数,将源图像精准地转化为指定尺寸的目标图像。
要缩小图像,一般推荐使用CV_INETR_AREA(区域插值)来插值;若要放大图像,推荐使用CV_INTER_LINEAR(线性插值)。
这个函数可以用来做简单的图像尺度变换。
而下面要说的图像金字塔的用处很大,在特征检测中都是基础理论和技术;Opencv里面的API介绍:1.void resize(src,dst,size,int interpolation)2.//src:源图像;dst:目标图像,3.//size目标图像大小,可以是指定的尺寸或者放大缩小的比例4.//指定插值方式,一般有四种插值方式可供选择,默认为线性插值法二、图像金字塔(Image Pyramid)图像金字塔是图像中多尺度表达的一种,最主要用于图像的分割,是一种以多分辨率来解释图像的有效但概念简单的结构。
图像金字塔最初用于机器视觉和图像压缩,一幅图像的金字塔是一系列以金字塔形状排列的分辨率逐步降低,且来源于同一张原始图的图像集合。
其通过梯次向下采样获得,直到达到某个终止条件才停止采样。
金字塔的底部是待处理图像的高分辨率表示,而顶部是低分辨率的近似。
我们将一层一层的图像比喻成金字塔,层级越高,则图像越小,分辨率越低。
常见两类图像金字塔•高斯金字塔 ( Gaussian pyramid): 用来向下/降采样,主要的图像金字塔•拉普拉斯金字塔(Laplacian pyramid): 用来从金字塔低层图像重建上层未采样图像,在数字图像处理中也即是预测残差,可以对图像进行最大程度的还原,配合高斯金字塔一起使用。
•两者的简要区别:高斯金字塔用来向下降采样图像,注意降采样其实是由金字塔底部向上采样,分辨率降低,它和我们理解的金字塔概念相反(注意);而拉普拉斯金字塔则用来从金字塔底层图像中向上采样重建一个图像。
opencv光照不均匀去除算法

opencv光照不均匀去除算法
随着计算机视觉技术的发展,图像处理成为了研究热点之一。
在一些应用中,由于光照条件的不同,图像的光照不均匀问题十分常见。
而光照不均匀问题会影响到图像的质量和后续处理的准确性,因此去除光照不均匀是很有必要的。
本文介绍了一种基于opencv的光照不均匀去除算法。
该算法的基本思路是通过图像金字塔采样得到不同尺度的图像,然后对每个尺度的图像进行均值迭代和高斯加权迭代,最后将不同尺度的图像加权融合得到最终的去除光照不均匀后的图像。
具体来说,首先对原始图像进行高斯模糊,得到一个比原始图像稍微模糊的图像,然后对这个图像进行缩放得到不同尺度的图像。
对每个尺度的图像进行均值迭代和高斯加权迭代,直到达到一定的精度,即可得到去除光照不均匀后的图像。
最后将不同尺度的图像按照权重加权融合,得到最终的图像。
该算法具有较好的去除光照不均匀效果,且操作简单易行,可以应用于实际图像处理中。
- 1 -。
分水岭分割法
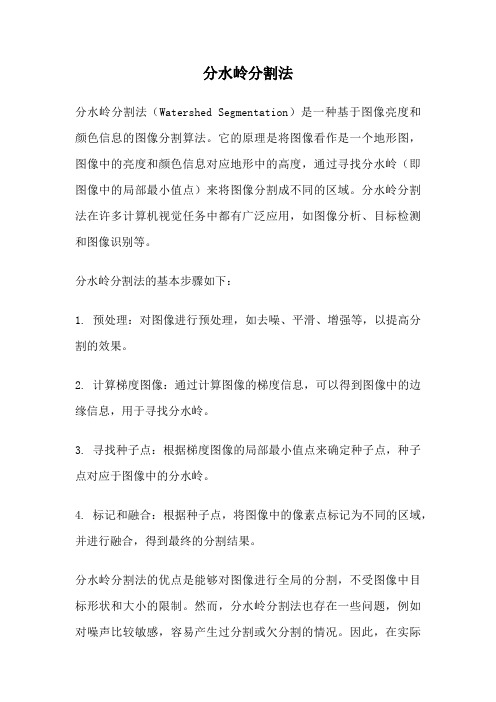
分水岭分割法分水岭分割法(Watershed Segmentation)是一种基于图像亮度和颜色信息的图像分割算法。
它的原理是将图像看作是一个地形图,图像中的亮度和颜色信息对应地形中的高度,通过寻找分水岭(即图像中的局部最小值点)来将图像分割成不同的区域。
分水岭分割法在许多计算机视觉任务中都有广泛应用,如图像分析、目标检测和图像识别等。
分水岭分割法的基本步骤如下:1. 预处理:对图像进行预处理,如去噪、平滑、增强等,以提高分割的效果。
2. 计算梯度图像:通过计算图像的梯度信息,可以得到图像中的边缘信息,用于寻找分水岭。
3. 寻找种子点:根据梯度图像的局部最小值点来确定种子点,种子点对应于图像中的分水岭。
4. 标记和融合:根据种子点,将图像中的像素点标记为不同的区域,并进行融合,得到最终的分割结果。
分水岭分割法的优点是能够对图像进行全局的分割,不受图像中目标形状和大小的限制。
然而,分水岭分割法也存在一些问题,例如对噪声比较敏感,容易产生过分割或欠分割的情况。
因此,在实际应用中,需要根据具体的任务和图像特点选择合适的参数和方法来改进分割效果。
分水岭分割法的应用非常广泛。
在医学图像处理中,可以用于分割病变区域、提取器官结构等。
在遥感图像处理中,可以用于分割地物、提取道路网络等。
在计算机视觉中,可以用于目标检测、图像识别、图像分析等。
此外,分水岭分割法还可以与其他图像处理方法相结合,如边缘检测、阈值分割等,以提高分割效果。
总结起来,分水岭分割法是一种基于图像亮度和颜色信息的图像分割算法,通过寻找分水岭将图像分割成不同的区域。
它在许多计算机视觉任务中都有广泛应用,具有全局分割的优点。
然而,分水岭分割法也存在一些问题,需要根据具体的任务和图像特点来选择合适的方法和参数。
分水岭分割法的应用非常广泛,可以用于医学图像处理、遥感图像处理和计算机视觉等领域。
通过合理地使用分水岭分割法,可以提高图像分割的效果,为后续的图像分析和处理任务提供有力支持。
C++函数pyrUp和pyrDown来实现图像金字塔功能

C++函数pyrUp和pyrDown来实现图像⾦字塔功能⽬标本⽂档尝试解答如下问题:如何使⽤OpenCV函数 pyrUp 和 pyrDown 对图像进⾏向上和向下采样。
原理Note 以下内容来⾃于Bradski和Kaehler的⼤作: Learning OpenCV 。
当我们需要将图像转换到另⼀个尺⼨的时候,有两种可能:放⼤图像或者缩⼩图像。
尽管OpenCV ⼏何变换部分提供了⼀个真正意义上的图像缩放函数(resize, 在以后的教程中会学到),不过在本篇我们⾸先学习⼀下使⽤图像⾦字塔来做图像缩放, 图像⾦字塔是视觉运⽤中⼴泛采⽤的⼀项技术。
图像⾦字塔⼀个图像⾦字塔是⼀系列图像的集合 - 所有图像来源于同⼀张原始图像 - 通过梯次向下采样获得,直到达到某个终⽌条件才停⽌采样。
有两种类型的图像⾦字塔常常出现在⽂献和应⽤中:⾼斯⾦字塔(Gaussian pyramid): ⽤来向下采样拉普拉斯⾦字塔(Laplacian pyramid): ⽤来从⾦字塔低层图像重建上层未采样图像在这篇⽂档中我们将使⽤⾼斯⾦字塔。
⾼斯⾦字塔想想⾦字塔为⼀层⼀层的图像,层级越⾼,图像越⼩。
每⼀层都按从下到上的次序编号,层级 (i+1) (表⽰为 G_{i+1} 尺⼨⼩于层级 i (G_{i}))。
为了获取层级为 (i+1) 的⾦字塔图像,我们采⽤如下⽅法:将 G_{i} 与⾼斯内核卷积:将所有偶数⾏和列去除。
显⽽易见,结果图像只有原图的四分之⼀。
通过对输⼊图像 G_{0} (原始图像) 不停迭代以上步骤就会得到整个⾦字塔。
以上过程描述了对图像的向下采样,如果将图像变⼤呢?:⾸先,将图像在每个⽅向扩⼤为原来的两倍,新增的⾏和列以0填充(0)使⽤先前同样的内核(乘以4)与放⼤后的图像卷积,获得 “新增像素” 的近似值。
这两个步骤(向下和向上采样) 分别通过OpenCV函数 pyrUp 和 pyrDown 实现, 我们将会在下⾯的⽰例中演⽰如何使⽤这两个函数。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
using System;using System.Collections.Generic;using ponentModel;using System.Data;using System.Drawing;using System.Linq;using System.Text;using System.Windows.Forms;using System.Diagnostics;using System.Runtime.InteropServices;using Emgu.CV;using Emgu.CV.CvEnum;using Emgu.CV.Structure;using Emgu.CV.UI;namespace ImageProcessLearn{public partial class FormImageSegment : Form{//成员变量private string sourceImageFileName = "wky_tms_2272x1704.jpg";//源图像文件名private Image<Bgr, Byte> imageSource = null; //源图像private Image<Bgr, Byte> imageSourceClone = null; //源图像的克隆private Image<Gray, Int32> imageMarkers = null; //标记图像private double xScale = 1d; //原始图像与PictureBox在x轴方向上的缩放private double yScale = 1d; //原始图像与PictureBox在y轴方向上的缩放private Point previousMouseLocation = new Point(-1, -1); //上次绘制线条时,鼠标所处的位置private const int LineWidth = 5; //绘制线条的宽度private int drawCount = 1; //用户绘制的线条数目,用于指定线条的颜色public FormImageSegment(){InitializeComponent();}//窗体加载时private void FormImageSegment_Load(object sender, EventArgs e){//设置提示toolTip.SetToolTip(rbWatershed, "可以在源图像上用鼠标绘制大致分割区域线条,该线条用于分水岭算法");toolTip.SetToolTip(txtPSLevel, "金字塔层数跟图像尺寸有关,该值只能是图像尺寸被2整除的次数,否则将得出错误结果");toolTip.SetToolTip(txtPSThreshold1, "建立连接的错误阀值");toolTip.SetToolTip(txtPSThreshold2, "分割簇的错误阀值");toolTip.SetToolTip(txtPMSFSpatialRadius, "空间窗的半径");toolTip.SetToolTip(txtPMSFColorRadius, "色彩窗的半径");toolTip.SetToolTip(btnClearMarkers, "清除绘制在源图像上,用于分水岭算法的大致分割区域线条");//加载图像LoadImage();}//当窗体关闭时,释放资源private void FormImageSegment_FormClosing(object sender, FormClosingEventArgs e){if (imageSource != null)imageSource.Dispose();if (imageSourceClone != null)imageSourceClone.Dispose();if (imageMarkers != null)imageMarkers.Dispose();}//加载源图像private void btnLoadImage_Click(object sender, EventArgs e){OpenFileDialog ofd = new OpenFileDialog();ofd.CheckFileExists = true;ofd.DefaultExt = "jpg";ofd.Filter = "图片文件|*.jpg;*.png;*.bmp|所有文件|*.*";if (ofd.ShowDialog(this) == DialogResult.OK){if (ofd.FileName != ""){sourceImageFileName = ofd.FileName;LoadImage();}}ofd.Dispose();}//清除分割线条private void btnClearMarkers_Click(object sender, EventArgs e){if (imageSourceClone != null)imageSourceClone.Dispose();imageSourceClone = imageSource.Copy();pbSource.Image = imageSourceClone.Bitmap;imageMarkers.SetZero();drawCount = 1;}//当鼠标按下并在源图像上移动时,在源图像上绘制分割线条private void pbSource_MouseMove(object sender, MouseEventArgs e){//如果按下了左键if (e.Button == MouseButtons.Left){if (previousMouseLocation.X >= 0 && previousMouseLocation.Y >= 0){Point p1 = new Point((int)(previousMouseLocation.X * xScale), (int)(previousMouseLocation.Y * yScale));Point p2 = new Point((int)(e.Location.X * xScale), (int)(e.Location.Y * yScale)); LineSegment2D ls = new LineSegment2D(p1, p2);int thickness = (int)(LineWidth * xScale);imageSourceClone.Draw(ls, new Bgr(255d, 255d, 255d), thickness);pbSource.Image = imageSourceClone.Bitmap;imageMarkers.Draw(ls, new Gray(drawCount), thickness);}previousMouseLocation = e.Location;}}//当松开鼠标左键时,将绘图的前一位置设置为(-1,-1)private void pbSource_MouseUp(object sender, MouseEventArgs e){previousMouseLocation = new Point(-1, -1);drawCount++;}//加载源图像private void LoadImage(){if (imageSource != null)imageSource.Dispose();imageSource = new Image<Bgr, byte>(sourceImageFileName);if (imageSourceClone != null)imageSourceClone.Dispose();imageSourceClone = imageSource.Copy();pbSource.Image = imageSourceClone.Bitmap;if (imageMarkers != null)imageMarkers.Dispose();imageMarkers = new Image<Gray, Int32>(imageSource.Size);imageMarkers.SetZero();xScale = 1d * imageSource.Width / pbSource.Width;yScale = 1d * imageSource.Height / pbSource.Height;drawCount = 1;}//分割图像private void btnImageSegment_Click(object sender, EventArgs e){if (rbWatershed.Checked)txtResult.Text += Watershed();else if (rbPrySegmentation.Checked)txtResult.Text += PrySegmentation();else if (rbPryMeanShiftFiltering.Checked)txtResult.Text += PryMeanShiftFiltering();}///<summary>///分水岭算法图像分割///</summary>///<returns>返回用时</returns>private string Watershed(){//分水岭算法分割Image<Gray, Int32> imageMarkers2 = imageMarkers.Copy();Stopwatch sw = new Stopwatch();sw.Start();CvInvoke.cvWatershed(imageSource.Ptr, imageMarkers2.Ptr);sw.Stop();//将分割的结果转换到256级灰度图像pbResult.Image = imageMarkers2.Bitmap;imageMarkers2.Dispose();return string.Format("分水岭图像分割,用时:{0:F05}毫秒。