java实现客户端与服务端之间的通信
gPRC简介以及Java中使用gPRC实现客户端与服务端通信(附代码下载)
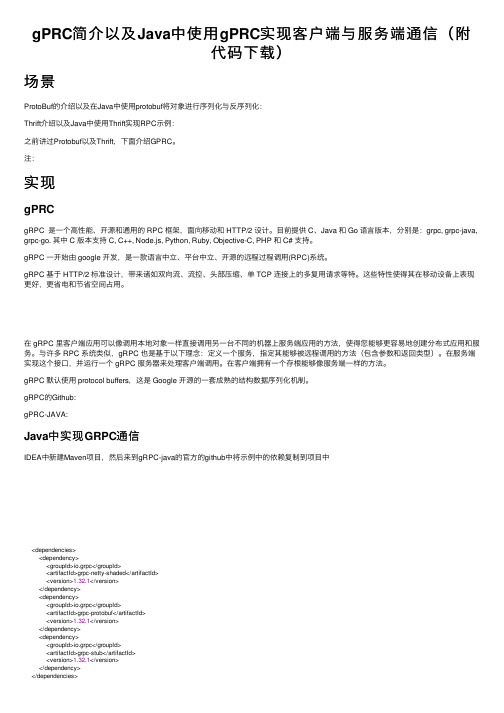
gPRC简介以及Java中使⽤gPRC实现客户端与服务端通信(附代码下载)场景ProtoBuf的介绍以及在Java中使⽤protobuf将对象进⾏序列化与反序列化:Thrift介绍以及Java中使⽤Thrift实现RPC⽰例:之前讲过Protobuf以及Thrift,下⾯介绍GPRC。
注:实现gPRCgRPC 是⼀个⾼性能、开源和通⽤的 RPC 框架,⾯向移动和 HTTP/2 设计。
⽬前提供 C、Java 和 Go 语⾔版本,分别是:grpc, grpc-java, grpc-go. 其中 C 版本⽀持 C, C++, Node.js, Python, Ruby, Objective-C, PHP 和 C# ⽀持。
gRPC ⼀开始由 google 开发,是⼀款语⾔中⽴、平台中⽴、开源的远程过程调⽤(RPC)系统。
gRPC 基于 HTTP/2 标准设计,带来诸如双向流、流控、头部压缩、单 TCP 连接上的多复⽤请求等特。
这些特性使得其在移动设备上表现更好,更省电和节省空间占⽤。
在 gRPC ⾥客户端应⽤可以像调⽤本地对象⼀样直接调⽤另⼀台不同的机器上服务端应⽤的⽅法,使得您能够更容易地创建分布式应⽤和服务。
与许多 RPC 系统类似,gRPC 也是基于以下理念:定义⼀个服务,指定其能够被远程调⽤的⽅法(包含参数和返回类型)。
在服务端实现这个接⼝,并运⾏⼀个 gRPC 服务器来处理客户端调⽤。
在客户端拥有⼀个存根能够像服务端⼀样的⽅法。
gRPC 默认使⽤ protocol buffers,这是 Google 开源的⼀套成熟的结构数据序列化机制。
gRPC的Github:gPRC-JAVA:Java中实现GRPC通信IDEA中新建Maven项⽬,然后来到gRPC-java的官⽅的github中将⽰例中的依赖复制到项⽬中<dependencies><dependency><groupId>io.grpc</groupId><artifactId>grpc-netty-shaded</artifactId><version>1.32.1</version></dependency><dependency><groupId>io.grpc</groupId><artifactId>grpc-protobuf</artifactId><version>1.32.1</version></dependency><dependency><groupId>io.grpc</groupId><artifactId>grpc-stub</artifactId><version>1.32.1</version></dependency></dependencies>因为gRPC是基于protobuf的,同样要将.proto⽂件编译成代码。
java即时通讯原理

java即时通讯原理Java即时通讯(Instant Messaging)是一种通过网络实现即时信息传送的技术。
它利用网络通信协议和 Java 编程语言,通过客户端和服务器端之间的交互,实现用户之间的实时消息传递。
Java即时通讯的原理可以概括为以下几个步骤:1. 客户端与服务器的连接:客户端使用Java编程语言创建并启动一个与服务器建立连接的套接字(Socket)。
该套接字是一个网络通信端点,用于实现客户端与服务器之间的双向通信。
2. 客户端发送消息:客户端通过套接字将消息发送给服务器。
可以使用Java提供的Socket类中的输出流(OutputStream)将消息数据写入到套接字中。
3. 服务器接收消息:服务器端通过一个监听套接字(ServerSocket)监听客户端的连接请求。
当有新的客户端连接时,服务器创建一个新的线程来处理该客户端的请求。
服务器端可以使用Java中的Socket类中的输入流(InputStream)从套接字中读取客户端发送的消息数据。
4. 服务器转发消息:服务器端接收到客户端的消息后,可以将消息转发给其他客户端。
服务器通过维护一个客户端列表,保存所有已连接的客户端信息。
当服务器接收到消息后,遍历客户端列表,通过各客户端的套接字,将消息发送给每个客户端。
5. 客户端接收消息:客户端通过套接字的输入流从服务器接收到其他客户端发送的消息数据。
客户端可以通过Java提供的线程机制在一个独立的线程中实时接收并处理服务器发送的消息。
6. 客户端显示消息:客户端接收到消息后,可以将消息展示给用户。
这可以通过Java的GUI编程实现,将消息显示在用户界面的聊天框中。
通过以上步骤,Java即时通讯实现了用户之间的实时消息传递。
整个过程涉及到客户端与服务器的连接建立、消息的发送和接收、服务器的消息转发以及客户端的消息显示等环节。
java socketioserver类的方法
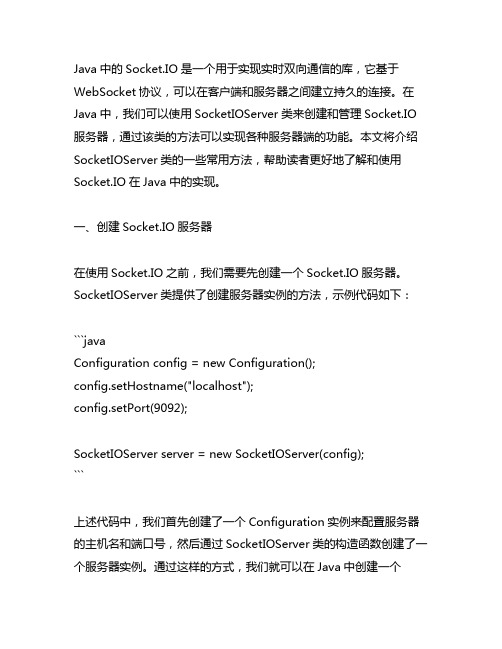
Java中的Socket.IO是一个用于实现实时双向通信的库,它基于WebSocket协议,可以在客户端和服务器之间建立持久的连接。
在Java中,我们可以使用SocketIOServer类来创建和管理Socket.IO 服务器,通过该类的方法可以实现各种服务器端的功能。
本文将介绍SocketIOServer类的一些常用方法,帮助读者更好地了解和使用Socket.IO在Java中的实现。
一、创建Socket.IO服务器在使用Socket.IO之前,我们需要先创建一个Socket.IO服务器。
SocketIOServer类提供了创建服务器实例的方法,示例代码如下:```javaConfiguration config = new Configuration();config.setHostname("localhost");config.setPort(9092);SocketIOServer server = new SocketIOServer(config);```上述代码中,我们首先创建了一个Configuration实例来配置服务器的主机名和端口号,然后通过SocketIOServer类的构造函数创建了一个服务器实例。
通过这样的方式,我们就可以在Java中创建一个Socket.IO服务器,为后续的通信提供支持。
二、服务器端事件的处理在Socket.IO服务器上,我们通常需要处理一些事件,例如连接事件、断开事件、自定义事件等。
SocketIOServer类提供了一系列方法来注册和处理这些事件,示例代码如下:```javaserver.addConnectListener(client -> {System.out.println("客户端连接:" + client.getSessionId()); });server.addDisconnectListener(client -> {System.out.println("客户端断开连接:" + client.getSessionId()); });server.addEventListener("chat message", String.class, (client, data, ackRequest) -> {System.out.println("收到消息:" + data);});```上述代码中,我们使用addConnectListener方法和addDisconnectListener方法分别注册了客户端连接和断开连接的事件处理函数,使用addEventListener方法注册了一个名为"chat message"的自定义事件的处理函数。
java 不同系统之间传输数据的方法

java 不同系统之间传输数据的方法Java是一种强大且广泛应用的编程语言,用于开发各种类型的应用程序。
在实际开发中,经常需要在不同的系统之间传输数据。
本文将介绍一些常用的方法来实现Java不同系统之间的数据传输。
1. 使用Socket通信Socket通信是一种常用的网络通信方式,可以实现不同系统之间的数据传输。
通过Socket,我们可以在客户端和服务器之间建立一条双向通道进行数据交换。
在Java中,可以使用Java的原生Socket库来实现Socket通信。
客户端和服务器端通过准确的IP地址和端口号来建立连接。
客户端可以使用Socket类来与服务器进行通信,而服务器则使用ServerSocket类监听并接受客户端连接。
2. 使用HTTP协议HTTP协议是一种应用层协议,常用于Web应用程序中。
通过HTTP协议,不同系统之间可以通过发送和接收HTTP请求和响应来进行数据传输。
在Java中,可以使用Java的HttpURLConnection类或者第三方库,如Apache 的HttpClient来实现HTTP通信。
通过发送HTTP请求,可以将数据以请求参数或JSON/XML等格式发送到目标系统,并接收目标系统的HTTP响应。
3. 使用WebServiceWebService是一种通过网络进行通信的软件系统。
它可以使不同系统之间的应用程序通过Web服务接口进行数据传输和交互。
在Java中,可以使用Java的JAX-WS和JAX-RPC等API来开发和使用WebService。
通过定义WebService接口和实现相应的服务端和客户端,可以在不同系统之间轻松地传输数据。
4. 使用消息队列消息队列是一种常用的异步通信方式,允许不同系统之间以消息的形式传递数据。
消息队列将数据发送方发送的消息存储在队列中,接收方从队列中接收并处理消息。
在Java中,可以使用ActiveMQ、RabbitMQ等消息中间件来实现消息队列。
java 数据对接方法

java 数据对接方法Java 数据对接方法1. 数据对接介绍数据对接是指不同系统之间进行数据传输和共享的过程。
在Java 中,我们可以使用多种方法实现数据对接,包括但不限于以下方法:•Java Socket:基于TCP/IP协议的套接字通信方式,可以实现实时数据传输和双向通信。
•Java URL:提供了一种简单的访问网页和资源的方法,可以处理HTTP请求和响应。
•Java HttpURLConnection:是Java中处理HTTP网络请求的基础类,提供了丰富的方法用于发送和接收HTTP请求和响应。
•Java Sockets与Java Server Sockets:分别用于实现客户端和服务器端的套接字通信,在局域网中可用于数据传输和通信。
•Java RMI(Remote Method Invocation):是一种支持在远程服务器上调用方法的Java API,可以实现分布式应用程序之间的数据传输。
•Java JMS(Java Message Service):Java消息服务,是一种用于在分布式系统中发送、接收消息的API,常用于异步通信。
2. Java SocketJava Socket是Java程序进行网络通信的基础类,它提供了一种简单而底层的方式来进行数据对接。
使用Java Socket可以实现客户端和服务器之间的双向通信,具体步骤如下:1.创建一个Socket对象,指定服务器的IP地址和端口号。
2.调用Socket对象的getOutputStream()方法获取输出流,用于向服务器发送数据。
3.调用Socket对象的getInputStream()方法获取输入流,用于从服务器接收数据。
4.使用输入流和输出流进行数据的读写操作。
5.使用完毕后,调用Socket对象的close()方法关闭连接。
3. Java URLJava URL类是Java提供的用于处理URL(Uniform Resource Locator)的类,可以用于访问网页和其他资源。
socket建立tcp连接的java代码

socket建立tcp连接的java代码Socket是Java中常用的网络编程类,可以用于建立TCP连接,完成客户端和服务器间的通信。
下面是Socket建立TCP连接的Java代码:1. 建立Socket对象TCP协议在建立连接时,需要同时指定服务器的IP地址和端口号。
因此,在客户端程序中,需要先创建一个Socket对象来指定需要连接的服务器IP地址和端口号。
Socket socket=new Socke t(“192.168.1.1”, 8888);2. 获取输入输出流建立连接之后,客户端可以向服务器发送数据,还可以接收服务器返回的数据。
为了完成这些操作,需要获取输入输出流对象。
InputStream input=socket.getInputStream();OutputStream output=socket.getOutputStream();3. 发送数据客户端想要向服务器发送数据,可以通过输出流对象write()方法实现。
byte[] data=”Hello Server”.getBytes();output.write(data);4. 接收数据客户端从服务器接收数据,可以通过输入流对象read()方法实现。
byte[] buffer=new byte[1024];int len=input.read(buffer);5. 断开连接客户端和服务器通信结束之后,需要关闭连接。
input.close();output.close();socket.close();综上所述,以上代码实现了Socket建立TCP连接的过程,使得客户端和服务器能够互相通信,完成所需的业务操作。
java grpc实现原理

java grpc实现原理Java gRPC(Google Remote Procedure Call)是一种高性能的远程过程调用框架,它基于谷歌开源的Protocol Buffers(简称ProtoBuf)序列化协议,并使用HTTP/2作为底层通信协议。
本文将介绍Java gRPC的实现原理及其工作流程。
一、gRPC的基本概念1.1 Protocol Buffers(ProtoBuf)Protocol Buffers是一种轻量级的数据序列化协议,可以将结构化数据序列化为二进制格式,以便于存储和传输。
ProtoBuf定义了一种语言无关、平台无关的数据格式,使得不同平台和语言之间可以轻松地进行数据交换。
1.2 Remote Procedure Call(RPC)远程过程调用是一种计算机通信协议,它允许程序在不同的计算机上通过网络进行通信和调用,就像调用本地方法一样。
RPC隐藏了底层网络通信的细节,使得分布式系统开发变得更加简单和方便。
二、gRPC的实现原理2.1 代码生成在使用gRPC之前,需要先定义服务和消息类型的ProtoBuf文件,并使用gRPC提供的编译器将其编译成相应的Java代码。
编译器会根据ProtoBuf文件生成服务接口、消息类和服务桩(Stub)的代码。
2.2 通信协议gRPC使用HTTP/2作为底层通信协议,HTTP/2相较于HTTP/1.1具有更低的延迟和更高的并发性能。
HTTP/2使用二进制帧来传输数据,可以同时发送多个请求和响应,充分利用了网络的带宽和连接。
2.3 序列化与反序列化在通信过程中,消息需要在客户端和服务端之间进行序列化和反序列化。
gRPC使用ProtoBuf作为默认的序列化协议,将消息对象序列化为二进制数据进行传输,接收方再将二进制数据反序列化为消息对象。
2.4 连接管理gRPC使用Channel来管理客户端与服务端之间的连接。
在客户端,通过Channel可以创建一个与服务端的连接,并维护该连接的状态。
java实现两台电脑间TCP协议文件传输

java实现两台电脑间TCP协议⽂件传输记录下之前所做的客户端向服务端发送⽂件的⼩项⽬,总结下学习到的⼀些⽅法与思路。
注:本⽂参考⾃《⿊马程序员》视频。
⾸先明确需求,在同⼀局域⽹下的机器⼈A想给喜欢了很久的机器⼈B发送情书,但是机器⼈B事先并不知道⼩A的⼼思,那么作为⽉⽼(红娘)该如何帮助他们呢?然后建⽴模型并拆分需求。
这⾥两台主机使⽤⽹线直连,在物理层上确保建⽴了连接,接下来便是利⽤相应的协议将信息从电脑A传给电脑B。
在这⼀步上,可以将此过程抽象为⽹络+I/O(Input、Output)的过程。
如果能在⼀台电脑上实现⽂件之间的传输,再加上相互的⽹络协议,羞涩的A不就可以将情书发送给B了吗?因此要先解决在⼀台电脑上传输信息的问题。
为了在⽹络上传输,使⽤必要的协议是必要的,TCP/IP协议簇就是为了解决计算机间通信⽽⽣,⽽这⾥主要⽤到UDP和TCP两种协议。
当⼩A可以向⼩B发送情书后,⼜出现了众多的追求者,那么⼩B如何去处理这么多的并发任务呢?这时便要⽤到多线程的技术。
因此接下来将分别介绍此过程中所⽤到了I/O流(最基础)、⽹络编程(最重要)、多线程知识(较重要)和其中⼀些⼩技巧。
⼀、I/O流I/O流⽤来处理设备之间的数据传输,Java对数据的传输通过流的⽅式。
流按操作数据分为两种:字节流与字符流。
如果数据是⽂本类型,那么需要使⽤字符流;如果是其他类型,那么使⽤字节流。
简单来说,字符流=字节流+编码表。
流按流向分为:输⼊流(将硬盘中的数据读⼊内存),输出流(将内存中的数据写⼊硬盘)。
简单来说,想要将某⽂件传到⽬的地,需要将此⽂件关联输⼊流,然后将输⼊流中的信息写⼊到输出流中。
将⽬的关联输出流,就可以将信息传输到⽬的地了。
Java提供了⼤量的流对象可供使⽤,其中有两⼤基类,字节流的两个顶层⽗InputStream与OutputStream;字符流的两个顶层⽗类Reader 与Writer。
这些体系的⼦类都以⽗类名作为后缀,⽽⼦类名的前缀就是该对象的功能。
java socket 实现原理

java socket 实现原理
Java的Socket是一种用于网络通信的编程接口。
它基于
TCP/IP协议,通过在不同计算机之间建立连接,实现了进程
之间的通信。
在Java中,Socket通信包括客户端和服务器端两个角色。
客
户端通过创建一个Socket对象来发起连接,服务器端通过创
建一个ServerSocket对象来监听连接请求。
具体实现原理如下:
1. 客户端创建一个Socket对象,指定服务器的IP地址和端口号。
Socket对象会封装了TCP/IP协议的相关信息,如IP地址
和端口号等。
2. 客户端通过Socket对象的connect()方法发起连接请求,向
服务器发送一个特定格式的数据包。
3. 服务器端创建一个ServerSocket对象,绑定到指定的IP地
址和端口号上。
4. 服务器端通过ServerSocket对象的accept()方法监听来自客
户端的连接请求。
当有连接请求到达时,accept()方法会返回
一个新的Socket对象,用于和客户端进行通信。
5. 客户端和服务器端通过各自的Socket对象进行数据的收发。
客户端通过输出流向服务器发送数据,服务器通过输入流接收
客户端发送的数据;服务器通过输出流向客户端发送数据,客户端通过输入流接收服务器发送的数据。
6. 当通信完成后,可以通过关闭Socket对象来结束连接。
通过以上步骤,客户端和服务器端能够通过Socket对象实现双向的数据传输。
Socket提供了简单、灵活和高效的网络通信方式,广泛应用于各种应用场景中。
Java实现客户端与服务器端的时间同步

Java实现客户端与服务器端的时间同步在客户端获取的当前时间和在服务端获取的当前时间往往会存在差异。
有时我们需要知道在客户端创建数据时,相对于服务器的时间是多少。
这是我们需要知道客户端和服务端获取当前时间的时间差,从⽽可以算出相对于服务器的时间。
主要的过程分为:“在客户端启动时,请求服务器端,发送当前客户端时间T1”、“服务器端收到请求,返回T2和T3,T2表⽰获取到客户端请求的时间,T3表⽰响应客户端请求的时间”、“客户端收到服务器的响应后,记录当前时间T4”、“算出客户端和服务端的时间差TimeDifference”。
1、在客户端启动时,请求服务器端,发送当前客户端时间T1。
在客户端定义个long类型的变量,存储时间差,初始值为0:/*** 同步Ntp时,服务器与POS之间的时差*/public static long TimeDifference;/*** 同步APP的时间和服务器的时间*/private void syncTime() {long timeStamp = new Date(System.currentTimeMillis() + NtpHttpBO.TimeDifference).getTime();if (!ntpHttpBO.syncTime(timeStamp)) {log.error("POS机启动时,同步服务器时间失败!");Toast.makeText(this, "POS机启动时,同步服务器时间失败!", Toast.LENGTH_SHORT).show();}}请求服务器时,将初始客户端的时间戳作为t1参数,传给服务器端:HTTP_Ntp_SyncTime = "ntp/syncEx.bx?t1=":public boolean syncTime(long timeStamp) {("正在执⾏NtpHttpBO的syncTime,timeStamp=" + timeStamp);Request req = new Request.Builder().url(Configuration.HTTP_IP + Ntp.HTTP_Ntp_SyncTime + timeStamp).addHeader(BaseHttpBO.COOKIE, GlobalController.getInstance().getSessionID()).build();HttpRequestUnit hru = new NtpSync();hru.setRequest(req);hru.setTimeout(TIME_OUT);hru.setbPostEventToUI(true);httpEvent.setEventProcessed(false);httpEvent.setStatus(BaseEvent.EnumEventStatus.EES_Http_ToDo);hru.setEvent(httpEvent);NtpHttpBO.bForNtpOnly = true;HttpRequestManager.getCache(HttpRequestManager.EnumDomainType.EDT_Communication).pushHttpRequest(hru);("正在请求服务器同步Ntp...");return true;}2、服务器端收到请求,返回T2和T3,T2表⽰获取到客户端请求的时间,T3表⽰响应客户端请求的时间。
java accept方法

Java accept方法什么是accept方法在Java中,accept方法是一个用于网络编程的方法。
它是.ServerSocket类中的一个重要方法。
ServerSocket类是用于实现服务器端套接字的类,它提供了一系列的方法来创建和管理服务器套接字。
accept方法的作用是接受客户端的连接请求,并返回一个新的Socket对象,用于与客户端进行通信。
它在服务器端的代码中调用,用于监听指定端口上的连接请求,并在有连接请求到来时进行响应。
使用accept方法的基本步骤在使用accept方法之前,我们需要先创建一个ServerSocket对象,并通过调用其bind方法来绑定到指定的端口上。
然后,我们可以通过调用accept方法来接受客户端的连接请求。
接下来,我们将详细介绍使用accept方法的基本步骤:1.创建ServerSocket对象:通过调用new ServerSocket(port)来创建一个ServerSocket对象,其中port是服务器要监听的端口号。
2.绑定到指定端口:通过调用ServerSocket对象的bind方法,将服务器绑定到指定的端口上。
例如,serverSocket.bind(new InetSocketAddress(port))用于绑定到指定端口上。
3.接受连接请求:通过调用ServerSocket对象的accept方法,等待客户端的连接请求。
当有客户端连接请求到来时,该方法会返回一个新的Socket对象,可以通过该对象与客户端进行通信。
4.处理连接:使用返回的Socket对象与客户端进行通信,发送和接收数据。
可以使用InputStream和OutputStream来进行数据的读写操作。
5.关闭连接:在通信完成后,需要调用Socket对象的close方法来关闭连接。
accept方法的返回值accept方法的返回值是一个Socket对象,可以通过该对象与客户端进行通信。
用JAVA分别实现WebSocket客户端与服务端

⽤JAVA分别实现WebSocket客户端与服务端最近公司在搞⼀个项⽬刚好需要⽤到WebSocket技术来实现实时数据的传输,因为之前也没接触过,所以捣⿎了好些天,最近恰巧有空就写写。
有误的地⽅还请⼤⽜们能及时指正。
项⽬背景:基于spring+spring MVC+mybatis框架的maven项⽬服务端:1、添加Jar包依赖:<dependency> <groupId>javax.websocket</groupId> <artifactId>javax.websocket-api</artifactId> <version>1.1</version> <scope>provided</scope></dependency>2、创建⼀个WebSocket服务端类MyWebSocketServer,并在类前添加@ServerEndpoint(value = "/websocket")注解,@ServerEndpoint(value = "/websocket")注释端点表⽰将 WebSocket 服务端运⾏在 ws://[Server 端 IP 或域名]:[Server 端⼝]/项⽬名/websocket 的访问端点3、实现onOpen、onClose、onMessage、onError等⽅法实例代码:MyWebSocketServer 类import java.io.IOException;import javax.websocket.EncodeException;import javax.websocket.OnClose;import javax.websocket.OnError;import javax.websocket.OnMessage;import javax.websocket.OnOpen;import javax.websocket.Session;import javax.websocket.server.PathParam;import javax.websocket.server.ServerEndpoint;import ng.StringUtils;import org.apache.log4j.Logger;import net.sf.json.JSONObject;@ServerEndpoint(value = "/websocket")public class MyWebSocketServer { private Logger logger = Logger.getLogger(MyWebSocketServer.class); private Session session; /** * 连接建⽴后触发的⽅法 */ @OnOpen public void onOpen(Session session){ this.session = session; ("onOpen"+session.getId()); WebSocketMapUtil.put(session.getId(),this); } /** * 连接关闭后触发的⽅法 */ @OnClose public void onClose(){ //从map中删除 WebSocketMapUtil.remove(session.getId()); ("====== onClose:"+session.getId()+" ======"); } /** * 接收到客户端消息时触发的⽅法 */ @OnMessage public void onMessage(String params,Session session) throws Exception{ //获取服务端到客户端的通道 MyWebSocketServer myWebSocket = WebSocketMapUtil.get(session.getId()); ("收到来⾃"+session.getId()+"的消息"+params); String result = "收到来⾃"+session.getId()+"的消息"+params; //返回消息给Web Socket客户端(浏览器) myWebSocket.sendMessage(1,”成功!”,result); } /** * 发⽣错误时触发的⽅法 */ @OnError public void onError(Session session,Throwable error){ (session.getId()+"连接发⽣错误"+error.getMessage()); error.printStackTrace(); } public void sendMessage(int status,String message,Object datas) throws IOException{ JSONObject result = new JSONObject(); result.put("status", status); result.put("message", message); result.put("datas", datas);this.session.getBasicRemote().sendText(result.toString());}}WebSocketMapUtil ⼯具类import java.util.Collection;import java.util.concurrent.ConcurrentHashMap;import java.util.concurrent.ConcurrentMap;public class WebSocketMapUtil { public static ConcurrentMap<String, MyWebSocketServer> webSocketMap = new ConcurrentHashMap<>(); public static void put(String key, MyWebSocketServer myWebSocketServer){ webSocketMap.put(key, myWebSocketServer);}public static MyWebSocketServer get(String key){return webSocketMap.get(key);}public static void remove(String key){webSocketMap.remove(key);}public static Collection<MyWebSocketServer> getValues(){return webSocketMap.values();}}客户端:1、添加Jar包依赖:<dependency> <groupId>org.java-websocket</groupId> <artifactId>Java-WebSocket</artifactId> <version>1.3.8</version></dependency>2、创建Web Socket客户端类MyWebSocketClient,并继承WebSocketClient3、实现构造器,重写onOpen、onClose、onMessage、onError等⽅法实例代码:MyWebSocketClient 类import .URI;import org.activiti.engine.impl.util.json.JSONObject;import org.apache.log4j.Logger;import org.java_websocket.client.WebSocketClient;import org.java_websocket.handshake.ServerHandshake;public class MyWebSocketClient extends WebSocketClient{ Logger logger = Logger.getLogger(MyWebSocketClient.class); public MyWebSocketClient(URI serverUri) { super(serverUri); } @Override public void onOpen(ServerHandshake arg0) { // TODO Auto-generated method stub ("------ MyWebSocket onOpen ------"); } @Override public void onClose(int arg0, String arg1, boolean arg2) { // TODO Auto-generated method stub ("------ MyWebSocket onClose ------"); } @Override public void onError(Exception arg0) { // TODO Auto-generated method stub ("------ MyWebSocket onError ------"); } @Override public void onMessage(String arg0) { // TODO Auto-generated method stub ("-------- 接收到服务端数据: " + arg0 + "--------"); }}MyTest 测试类public class MyTest{ public static void main(String[] arg0){ // 此处的WebSocket服务端URI,上⾯服务端第2点有详细说明 MyWebSocketClient myClient = new MyWebSocketClient("此处为websocket服务端URI"); // 往websocket服务端发送数据 myClient.send("此为要发送的数据内容"); }}。
JavaWebSocket技术简介

JavaWebSocket技术简介WebSocket是一种协议,它允许客户端和服务器进行双向通信。
在传统的HTTP协议中,浏览器向服务器发出请求,服务器响应请求并发送数据。
但是,当需要不断地更新数据时,这种方式就显得有些不太可行了。
WebSocket则具有实时、低延迟、高效等特点,逐渐成为了Web应用程序中热门的技术。
Java作为一种流行的编程语言,提供了许多工具和框架来支持WebSocket,JavaWebSocket是其中之一。
JavaWebSocket是一个基于Java的WebSocket API,它使得Java应用程序能够轻松地处理WebSocket通信。
在本文中,我们将深入了解JavaWebSocket技术,并学习如何在Java应用程序中使用它。
JavaWebSocket的优点JavaWebSocket拥有几个特征和优点,使得它成为许多Java应用程序的首选:1.简单易用:JavaWebSocket API简单易用,旨在简化WebSocket通信的处理和管理,因此它对开发人员而言非常直观。
2.兼容性强:JavaWebSocket API不依赖于任何特定的Web容器或HTTP服务器,因此它在所有Java平台上都可以使用,包括Java SE、Java EE和Android等。
3.高效性:JavaWebSocket利用了非阻塞I/O模型,在数据传输效率上优于传统的Servlet API。
4.稳定性:JavaWebSocket被广泛使用,而且由于其良好的设计和文档,获得了稳定性和可靠性方面的好评。
JavaWebSocket的基本架构JavaWebSocket的基本架构包括三个主要组成部分:1. WebSocketServer:WebSocketServer是WebSocket服务器的主要组成部分,它相当于HTTP服务器。
WebSocketServer的主要任务是监听客户端的连接请求,并向客户端提供WebSocket连接。
socket,java,通信协议
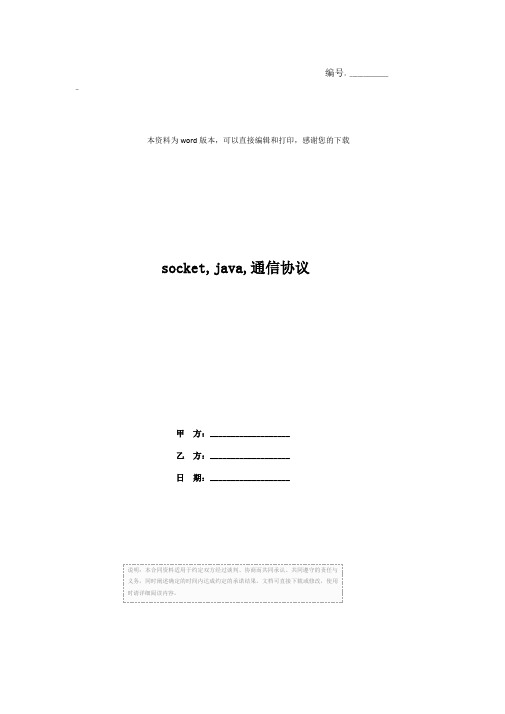
编号:______________ _本资料为word版本,可以直接编辑和打印,感谢您的下载socket,java,通信协议甲方:___________________乙方:___________________日期:___________________socket,java, 通信协议篇一:socket通信java代码客户端代码:importjava.io.bufferedReader; importjava.io.ioexception;importjava.io.inputstream;importjava.io.inputstreamReader; importjava.io.outputstream;.socket;.unknownhostexception; publicclassloginclient{ publicstaticvoidmain(string[]args){//todoauto-generatedmethodstubtry{socketsocket=newsocket("localhost”,8800); inputstreamis=socket.getinputstream(); outputstreamos=socket.getoutputstream(); stringinfo=" 用户名:tom;用户密码:123456”; os.write(info.getbytes());socket.shutdownoutput();stringreply=null;bufferedReaderbr=newbufferedReader(newinputstreamRe ader(is));while(!((reply=br.readline())==null)){system.out.println(" 我是客户端,服务器响应为:"+reply);}br.close();os.close();is.close();socket.close();}catch(unknownhostexceptione){//todoauto-generatedcatchblocke.printstacktrace();}catch(ioexceptione){//todoauto-generatedcatchblocke.printstacktrace();}}}服务器端代码:importjava.io.bufferedReader;importjava.io.ioexception;importjava.io.inputstream;importjava.io.inputstreamReader;importjava.io.outputstream;.serversocket;.socket;publicclassloginserver( /** *@paramargs */publicstaticvoidmain(string[]args){ //todoauto-generatedmethodstubtry{ serversocketserversocket=newserversocket(8800 );while(true){ socketsocket=serversocket.accept();inputstreamis=socket.getinputstream();outputstreamos=socket.getoutputstream(); bufferedReaderbr=newbufferedReader(newinputstreamRe ader(is));stringinfo=null;while(!((info=br.readline())==null))(system.out.println(" 我是服务器,用户信息为: +info);}stringreply=" 欢迎你,登陆成功!";os.write(reply.getbytes());br.close();os.close();is.close();socket.close();serversocket.close();}}catch(ioexceptione)(//todoauto-generatedcatchblocke.printstacktrace();}}}篇二:java+socket实现双机通信河南理工大学计算机科学与技术学院课程设计报告20xx — 20xx学年第二学期课程名称计算机网络设计题目利用javasocket实现双机通信学生姓名学号专业班级指导教师20xx年6月20日目录利用javasock实现双机通信一、设计任务1. 利用winsock来实现双机通信,理解tcp状态机图。
java长连接和短连接实例

java长连接和短连接实例
长连接和短连接是网络通信中常用的两种连接方式,它们在
Java中的实现可以通过Socket编程来完成。
首先,让我们来看看长连接的实现。
在Java中,可以使用Socket和ServerSocket来实现长连接。
ServerSocket负责监听客
户端的连接请求,而Socket则负责实际的通信。
当客户端与服务器
建立连接后,可以通过Socket进行数据的传输。
在服务器端,可以
使用多线程来处理多个客户端的长连接请求,这样可以实现并发处理。
长连接的一个典型应用是即时通讯系统,比如聊天软件,客户
端与服务器之间需要保持长时间的连接以实时传输消息。
接下来是短连接的实现。
短连接通常指的是客户端与服务器建
立连接后,发送完请求后立即断开连接。
在Java中,同样可以使用Socket来实现短连接。
客户端发送请求后,服务器接收并处理请求
后立即关闭连接。
短连接适用于一次性的数据传输,比如HTTP请求,客户端发送请求后,服务器返回响应后即可关闭连接。
无论是长连接还是短连接,都需要注意资源的释放和管理。
在Java中,可以使用try-with-resources语句来确保在使用完
Socket后及时释放资源,避免资源泄露。
总的来说,长连接和短连接在Java中的实现都离不开Socket 编程,通过合理的资源管理和多线程处理,可以实现稳定可靠的网络通信。
在实际应用中,需要根据具体的业务需求来选择合适的连接方式。
使用eclipsepaho在java端实现MQTT消息的收发(客户端与服务端实例)

使⽤eclipsepaho在java端实现MQTT消息的收发(客户端与服务端实例)⼀、MQTT(消息队列)简介MQTT(MQ Telemetry Transport)是IBM开发的⼀种⽹络应⽤层的协议,提供轻量级的,⽀持可发布/可订阅的的消息推送模式,使设备对设备之间的短消息通信变得简单,⽐如现在应⽤⼴泛的低功耗传感器,⼿机、嵌⼊式计算机、微型控制器等移动设备。
常⽤的有eclipse paho、activeMQ、阿⾥MQTT和其他的实现,本实例使⽤paho1、使⽤场景:1、不可靠、⽹络带宽⼩的⽹络2、运⾏的设备CPU、内存⾮常有限(我个⼈主要是⽤在服务端与嵌⼊式客户端进⾏消息和⼴告的通信)2、特点:1、基于发布/订阅模型的协议2、他是⼆进制协议,⼆进制的特点就是紧凑、占⽤空间⼩。
他的协议头只有2个字节3、提供了三种消息可能性保障(Qos):0:最多⼀次、1:最少⼀次、2:只有⼀次3、关键字1、HOST:搭载MQTT的服务器地址2、TOPIC:消息主题,可以被客户端订阅,实现对应消息的收发3、clientId:客户端ID,⽤于服务器对不同客户端的识别4、subscribe/unsubscribe:客户端对消息主题的订阅和取消订阅5、Qos:消息的服务质量,当⽹络过载或拥塞时,QoS 能确保重要业务量不受延迟或丢弃6、Callback:当客户端收到消息后对消息的处理(回调)7、KeepAliveInterval:客户端与服务器之间的连接是通过发送⼼跳包来保持存活⼆、JAVA端实例⾸先导⼊所依赖jar包1、服务端代码(负责消息的发送)package com.sc.util.paho;import org.eclipse.paho.client.mqttv3.MqttClient;import org.eclipse.paho.client.mqttv3.MqttConnectOptions;import org.eclipse.paho.client.mqttv3.MqttDeliveryToken;import org.eclipse.paho.client.mqttv3.MqttException;import org.eclipse.paho.client.mqttv3.MqttMessage;import org.eclipse.paho.client.mqttv3.MqttPersistenceException;import org.eclipse.paho.client.mqttv3.MqttTopic;import org.eclipse.paho.client.mqttv3.persist.MemoryPersistence;/*** Title:Server 这是发送消息的服务端* Description: 服务器向多个客户端推送主题,即不同客户端可向服务器订阅相同主题* @author rao*/public class ServerMQTT {//tcp://MQTT安装的服务器地址:MQTT定义的端⼝号public static final String HOST = "tcp://192.168.1.102:1883";//定义⼀个主题public static final String TOPIC = "pos_message_all";//定义MQTT的ID,可以在MQTT服务配置中指定private static final String clientid = "server11";private MqttClient client;private MqttTopic topic11;private String userName = "paho"; //⾮必须private String passWord = ""; //⾮必须private MqttMessage message;/*** 构造函数* @throws MqttException*/public ServerMQTT() throws MqttException {// MemoryPersistence设置clientid的保存形式,默认为以内存保存client = new MqttClient(HOST, clientid, new MemoryPersistence());connect();}/*** ⽤来连接服务器*/private void connect() {MqttConnectOptions options = new MqttConnectOptions();options.setCleanSession(false);options.setUserName(userName);options.setPassword(passWord.toCharArray());// 设置超时时间options.setConnectionTimeout(10);// 设置会话⼼跳时间options.setKeepAliveInterval(20);try {client.setCallback(new PushCallback());client.connect(options);topic11 = client.getTopic(TOPIC);} catch (Exception e) {e.printStackTrace();}}/**** @param topic* @param message* @throws MqttPersistenceException* @throws MqttException*/public void publish(MqttTopic topic , MqttMessage message) throws MqttPersistenceException, MqttException {MqttDeliveryToken token = topic.publish(message);token.waitForCompletion();System.out.println("message is published completely! "+ token.isComplete());}/*** 启动⼊⼝* @param args* @throws MqttException*/public static void main(String[] args) throws MqttException {ServerMQTT server = new ServerMQTT();server.message = new MqttMessage();server.message.setQos(1); //保证消息能到达⼀次server.message.setRetained(true);server.message.setPayload("这是推送消息的内容".getBytes());server.publish(server.topic11 , server.message);System.out.println(server.message.isRetained() + "------ratained状态");}}2、对消息进⾏处理的回调函数代码(必须)package com.sc.util.paho;import org.eclipse.paho.client.mqttv3.IMqttDeliveryToken;import org.eclipse.paho.client.mqttv3.MqttCallback;import org.eclipse.paho.client.mqttv3.MqttMessage;/*** 发布消息的回调类** 必须实现MqttCallback的接⼝并实现对应的相关接⼝⽅法CallBack 类将实现 MqttCallBack。
java axis webservice 传参方法
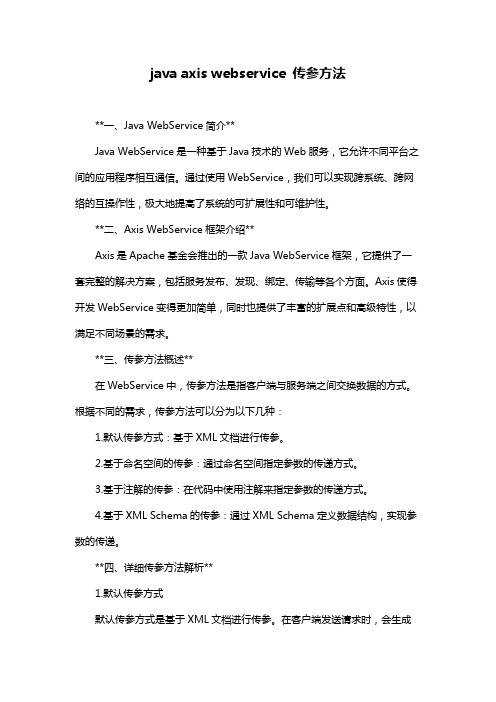
java axis webservice 传参方法**一、Java WebService简介**Java WebService是一种基于Java技术的Web服务,它允许不同平台之间的应用程序相互通信。
通过使用WebService,我们可以实现跨系统、跨网络的互操作性,极大地提高了系统的可扩展性和可维护性。
**二、Axis WebService框架介绍**Axis是Apache基金会推出的一款Java WebService框架,它提供了一套完整的解决方案,包括服务发布、发现、绑定、传输等各个方面。
Axis使得开发WebService变得更加简单,同时也提供了丰富的扩展点和高级特性,以满足不同场景的需求。
**三、传参方法概述**在WebService中,传参方法是指客户端与服务端之间交换数据的方式。
根据不同的需求,传参方法可以分为以下几种:1.默认传参方式:基于XML文档进行传参。
2.基于命名空间的传参:通过命名空间指定参数的传递方式。
3.基于注解的传参:在代码中使用注解来指定参数的传递方式。
4.基于XML Schema的传参:通过XML Schema定义数据结构,实现参数的传递。
**四、详细传参方法解析**1.默认传参方式默认传参方式是基于XML文档进行传参。
在客户端发送请求时,会生成一个XML文档,其中包含请求参数。
服务端接收请求后,解析XML文档,获取请求参数并进行处理。
2.基于命名空间的传参基于命名空间的传参是通过命名空间指定参数的传递方式。
在服务端,我们可以定义一个命名空间,并将其绑定到特定的参数上。
客户端在发送请求时,需要指定命名空间,以便服务端正确解析参数。
3.基于注解的传参基于注解的传参是在代码中使用注解来指定参数的传递方式。
服务端可以自定义注解,用于描述参数的格式、类型等信息。
客户端在发送请求时,需要遵循注解的规范,按照指定的方式传递参数。
4.基于XML Schema的传参基于XML Schema的传参是通过XML Schema定义数据结构,实现参数的传递。
java中client的用法 -回复

java中client的用法-回复Java中的Client的用法在Java中,Client是指客户端程序,用于与服务器进行通信和交互。
通过Client,我们可以发送请求并接收响应,实现与服务器的数据交换。
在本篇文章中,我们将一步步回答关于Java中Client的用法。
1. 建立一个Socket连接Java中的Client使用Socket与服务器建立连接。
首先,我们需要创建一个Socket对象,并指定服务器的IP地址和端口号。
这样,Client就可以与服务器进行通信。
String serverIP = "127.0.0.1"; 服务器IP地址int serverPort = 8080; 服务器端口号Socket socket = new Socket(serverIP, serverPort);在上面的代码中,我们使用了localhost作为服务器的IP地址,8080作为端口号。
你可以根据实际情况修改这些值。
2. 发送请求一旦Socket连接成功建立,Client就可以向服务器发送请求。
通常,我们使用OutputStream将请求数据发送给服务器。
OutputStream outputStream = socket.getOutputStream();String request = "Hello, server!"; 请求数据outputStream.write(request.getBytes());outputStream.flush();在上面的代码中,我们首先获得了OutputStream对象,然后将请求数据转换为字节数组,并使用write()方法发送给服务器。
最后,我们调用flush()方法确保数据被立即发送。
3. 接收响应接下来,Client需要从服务器接收响应数据。
我们可以使用InputStream 来接收服务器所发送的数据。
InputStream inputStream = socket.getInputStream();byte[] buffer = new byte[1024];int length = inputStream.read(buffer);String response = new String(buffer, 0, length);System.out.println("Server response: " + response);在上面的代码中,我们创建了一个用于存储响应数据的缓冲区,并使用read()方法读取从服务器传输过来的数据。
hubconnection java
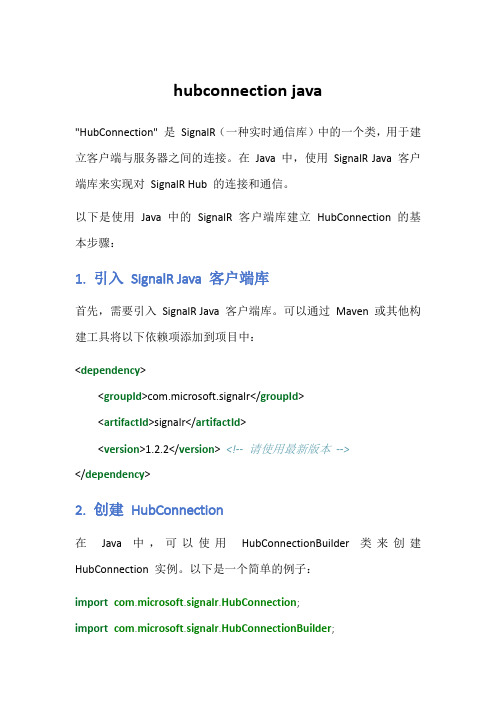
hubconnection java "HubConnection" 是SignalR(一种实时通信库)中的一个类,用于建立客户端与服务器之间的连接。
在Java 中,使用SignalR Java 客户端库来实现对SignalR Hub 的连接和通信。
以下是使用Java 中的SignalR 客户端库建立HubConnection 的基本步骤:1. 引入SignalR Java 客户端库首先,需要引入SignalR Java 客户端库。
可以通过Maven 或其他构建工具将以下依赖项添加到项目中:<dependency><groupId>com.microsoft.signalr</groupId><artifactId>signalr</artifactId><version>1.2.2</version> <!--请使用最新版本--></dependency>2. 创建HubConnection在Java 中,可以使用HubConnectionBuilder 类来创建HubConnection 实例。
以下是一个简单的例子:import com.microsoft.signalr.HubConnection;import com.microsoft.signalr.HubConnectionBuilder;import com.microsoft.signalr.HubConnectionState;public class SignalRClient {public static void main(String[]args){String hubUrl ="https://your-signalr-hub-url";// 替换成实际的SignalR Hub URLHubConnection hubConnection =HubConnectionBuilder.crea te(hubUrl).build();hubConnection.start().thenRun(()->{if(hubConnection.getConnectionState()==HubCo nnectionState.CONNECTED){System.out.println("Hub connected successfull y!");}else{System.out.println("Hub connection failed!");}}).exceptionally(ex ->{System.err.println("Error during hub connection: " +ex.getMessage());return null;});// 在此处添加其他SignalR 相关的操作,如注册事件、发送消息等}}3. 注册事件和发送消息一旦建立了HubConnection,就可以注册事件以便接收来自服务器的消息,并发送消息到服务器。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
import .*;import java.io.*;import java.util.Calendar;import java.awt.*;import java.awt.event.*;class EchoClient extends Frame implements ActionListener,Runnable{ Thread t;static Calendar T;static int H;static int M;static int S;static String l;private Button b1 = new Button("发送");private Button b2 = new Button("关闭");private TextField t1 = new TextField(30);private TextArea t2 = new TextArea();private int m;private String n;Socket connection;DataInputStream in;DataOutputStream out;private class window extends WindowAdapter{public void windowClosing(WindowEvent e){System.exit(0);}}private void focusEvt(java.awt.event.WindowEvent evt) {t1.requestFocus();}public void clock(){T=Calendar.getInstance();H=T.get(Calendar.HOUR_OF_DAY);M=T.get(Calendar.MINUTE);S=T.get(Calendar.SECOND);l=String.valueOf(H)+":"+String.valueOf(M)+":"+String.valueOf(S); }public EchoClient(String i,int j){super("客户端");t = new Thread(this);m=j;n=i;t.start();}public void run(){Panel p1 = new Panel();p1.setLayout(new FlowLayout());p1.add(b2);p1.add(t1);p1.add(b1);setLayout(new BorderLayout());add("South",p1);add("Center",t2);b1.addActionListener(this);b2.addActionListener(this);t1.addActionListener(this);addWindowListener(new window());addWindowListener(new java.awt.event.WindowAdapter() {public void windowOpened(java.awt.event.WindowEvent evt) {focusEvt(evt);}});setSize(400,300);setVisible(true);try{connection = new Socket(n,m);in = new DataInputStream(connection.getInputStream());out = new DataOutputStream(connection.getOutputStream());String link = new String("");while(!link.toUpperCase().equals(".QUIT")){link = in.readUTF();clock();t2.append("服务端"+l+"\n"+link+"\n\n");} }catch(UnknownHostException uhe){System.err.println("Unknown Host:127.0.0.1");}catch(IOException ioe){System.err.println("IOException:"+ioe);}}public void actionPerformed(ActionEvent e){if(e.getSource()==b1||e.getSource()==t1){String line = t1.getText();clock();t2.append("客户端"+l+"\n"+line+"\n\n");t1.setText("");try{out.writeUTF(line);}catch(IOException ioe){System.err.println("IOException:"+ioe);}}else if(e.getSource()==b2){System.exit(0);}}public static String readString(){String string = new String();BufferedReader in = new BufferedReader(new InputStreamReader(System.in));try{string = in.readLine();}catch(IOException e){System.out.println("Console.readString:Unknown error...");System.exit(-1);}return string;}}class stat extends Frame implements ActionListener{private Label l1 = new Label("请输入IP地址:");private Label l2 = new Label("端口号:");private TextField q1 = new TextField(10);private TextField q2 = new TextField(10);private Button r1 = new Button("确定");private Button r2 = new Button("关闭");private int j;private String i,k;private class window extends WindowAdapter{public void windowClosing(WindowEvent e){System.exit(0);}}private void focusEvt(java.awt.event.WindowEvent evt) {q1.requestFocus();}public stat(){Panel y = new Panel();y.setLayout(new GridLayout(4,1));y.add(l1);y.add(q1);y.add(l2);y.add(q2);Panel x = new Panel();x.setLayout(new FlowLayout());x.add(r2);x.add(r1);setLayout(new BorderLayout());add("South",x);add("Center",y);r1.addActionListener(this);r2.addActionListener(this);q1.addActionListener(this);q2.addActionListener(this);addWindowListener(new java.awt.event.WindowAdapter() {public void windowOpened(java.awt.event.WindowEvent evt) {focusEvt(evt);}});addWindowListener(new window());setSize(200,150);setVisible(true);}public void actionPerformed(ActionEvent e){if(e.getSource()==q1){q2.requestFocus();}else if(e.getSource()==r1||e.getSource()==q2){i=q1.getText();k=q2.getText();j=Integer.parseInt(k);new EchoClient(i,j);dispose();}else if(e.getSource()==r2){System.exit(0);}}public static void main(String args[]){stat a = new stat();}}import .*;import java.io.*;import java.util.Calendar;import java.awt.*;import java.awt.event.*;class EchoServer{private static boolean running = true;public static void main(String args[]){try{ServerSocket server = new ServerSocket(7777);while(running){Socket connection = server.accept();EchoServer1 handler = new EchoServer1(connection);}}catch(IOException ioe){System.err.println("IOException:"+ioe);}}}class EchoServer1 extends Frame implements ActionListener,Runnable{ Thread t;static Calendar T;static int H;static int M;static int S;static String l;private static boolean running = true;private Button b1 = new Button("发送");private Button b2 = new Button("关闭");private TextField t1 = new TextField(30);private TextArea t2 = new TextArea();DataInputStream in;DataOutputStream out;Socket connection;private class window extends WindowAdapter{public void windowClosing(WindowEvent e){System.exit(0);}}private void focusEvt(java.awt.event.WindowEvent evt) {t1.requestFocus();}public void clock(){T=Calendar.getInstance();H=T.get(Calendar.HOUR_OF_DAY);M=T.get(Calendar.MINUTE);S=T.get(Calendar.SECOND);l=String.valueOf(H)+":"+String.valueOf(M)+":"+String.valueOf(S);}public EchoServer1(Socket _connection){super("服务端");connection = _connection;Panel p1 = new Panel();p1.setLayout(new FlowLayout());p1.add(b2);p1.add(t1);p1.add(b1);setLayout(new BorderLayout());add("South",p1);add("Center",t2);b1.addActionListener(this);b2.addActionListener(this);t1.addActionListener(this);addWindowListener(new window());addWindowListener(new java.awt.event.WindowAdapter() {public void windowOpened(java.awt.event.WindowEvent evt) {focusEvt(evt);}});setSize(400,300);setVisible(true);t = new Thread(this);t.start();}public void run(){try{in = new DataInputStream(connection.getInputStream());out = new DataOutputStream(connection.getOutputStream());String line = new String("");while(!line.toUpperCase().equals(".QUIT")){line = in.readUTF();clock();t2.append("客户端"+l+"\n"+line+"\n\n");}}catch(IOException ioe){System.err.println("IOException:"+ioe);}}public void actionPerformed(ActionEvent e){if(e.getSource()==b1||e.getSource()==t1){String link = t1.getText();clock();t2.append("服务端"+l+"\n"+link+"\n\n");t1.setText("");try{out.writeUTF(link);}catch(IOException ioe){System.err.println("IOException:"+ioe);}}if(e.getSource()==b2)System.exit(0);}}。