眼球追踪
眼球追踪技术在游戏设计中的应用

眼球追踪技术在游戏设计中的应用在当今互动娱乐产业中,游戏设计者们竭力追求更加创新和引人入胜的方式来吸引玩家的注意力,并提供更加真实、沉浸式的游戏体验。
其中,眼球追踪技术成为了一种为游戏开发者们提供新思路和工具的创新技术。
本文将探讨眼球追踪技术在游戏设计中的应用,以及它给游戏体验带来的改变。
眼球追踪技术是一种通过红外或摄像头等设备对玩家的眼动进行监测的技术。
通过实时跟踪玩家的注视轨迹和焦点,游戏能够更准确地了解玩家的兴趣和注意力集中点,从而通过相关设计手段给予有效引导,以提高游戏的吸引力和用户体验。
首先,眼球追踪技术可以用于改进游戏中的观看方向控制。
传统的游戏控制方式仅局限于键盘、鼠标或手柄等固定设备,而眼球追踪技术则允许玩家通过眼神的方式来控制游戏中的观看方向。
这意味着玩家无需再通过手指操控设备,而可以直接通过视线来控制游戏中的角色或摄像机。
这种交互方式使得游戏操作更加自然、流畅,增强了沉浸感和操控精度。
其次,眼球追踪技术可以提高游戏中虚拟角色的表情交互的真实感。
传统的游戏中,角色的表情和交互通常是基于玩家的键盘或手柄输入,往往局限于预设的表情动作或选项。
而通过眼球追踪技术,游戏可以实时捕捉玩家的注视点和眼神表情,然后根据玩家的目光实时调整角色的表情和交互行为。
这样,游戏中的角色能够更加真实地反应玩家的情绪和需求,增加了游戏的互动性和代入感。
此外,眼球追踪技术还可以应用于游戏中的提示和引导。
通过分析玩家的注视点和眼动模式,游戏可以对玩家提供精准的提示和引导,使得玩家更容易找到游戏的关键任务、目标或隐藏的道具。
这种个性化的引导系统可以极大地提升游戏体验,减少傻瓜式的提示和指示,使得游戏更具挑战性和可玩性。
除了游戏设计方面的应用,眼球追踪技术还对游戏体验研究和用户行为分析产生了深远的影响。
通过分析玩家的注视热点、注视持续时间等数据,游戏设计者可以深入了解玩家的注意力分布和情绪变化,以便优化游戏的设计和体验。
识别 眼睛聚焦的方法
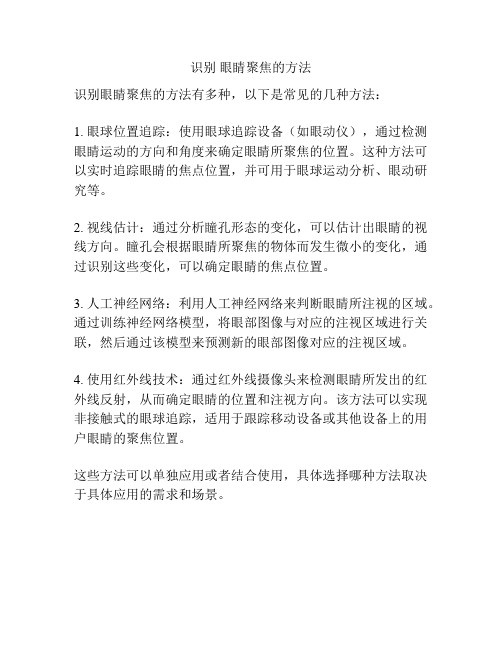
识别眼睛聚焦的方法
识别眼睛聚焦的方法有多种,以下是常见的几种方法:
1. 眼球位置追踪:使用眼球追踪设备(如眼动仪),通过检测眼睛运动的方向和角度来确定眼睛所聚焦的位置。
这种方法可以实时追踪眼睛的焦点位置,并可用于眼球运动分析、眼动研究等。
2. 视线估计:通过分析瞳孔形态的变化,可以估计出眼睛的视线方向。
瞳孔会根据眼睛所聚焦的物体而发生微小的变化,通过识别这些变化,可以确定眼睛的焦点位置。
3. 人工神经网络:利用人工神经网络来判断眼睛所注视的区域。
通过训练神经网络模型,将眼部图像与对应的注视区域进行关联,然后通过该模型来预测新的眼部图像对应的注视区域。
4. 使用红外线技术:通过红外线摄像头来检测眼睛所发出的红外线反射,从而确定眼睛的位置和注视方向。
该方法可以实现非接触式的眼球追踪,适用于跟踪移动设备或其他设备上的用户眼睛的聚焦位置。
这些方法可以单独应用或者结合使用,具体选择哪种方法取决于具体应用的需求和场景。
眼球追踪技术的原理
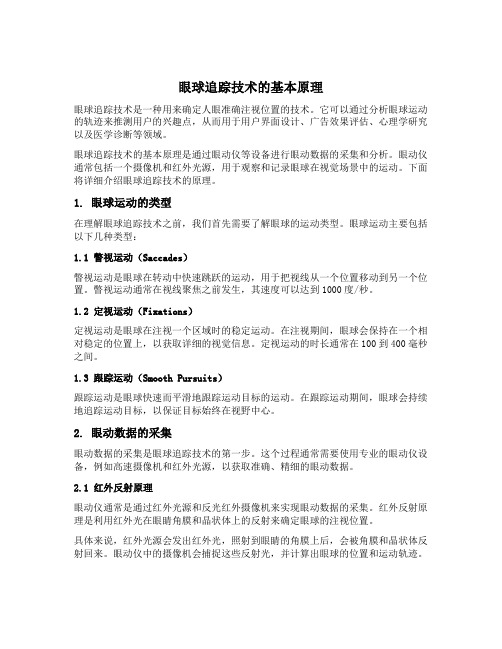
眼球追踪技术的基本原理眼球追踪技术是一种用来确定人眼准确注视位置的技术。
它可以通过分析眼球运动的轨迹来推测用户的兴趣点,从而用于用户界面设计、广告效果评估、心理学研究以及医学诊断等领域。
眼球追踪技术的基本原理是通过眼动仪等设备进行眼动数据的采集和分析。
眼动仪通常包括一个摄像机和红外光源,用于观察和记录眼球在视觉场景中的运动。
下面将详细介绍眼球追踪技术的原理。
1. 眼球运动的类型在理解眼球追踪技术之前,我们首先需要了解眼球的运动类型。
眼球运动主要包括以下几种类型:1.1 瞥视运动(Saccades)瞥视运动是眼球在转动中快速跳跃的运动,用于把视线从一个位置移动到另一个位置。
瞥视运动通常在视线聚焦之前发生,其速度可以达到1000度/秒。
1.2 定视运动(Fixations)定视运动是眼球在注视一个区域时的稳定运动。
在注视期间,眼球会保持在一个相对稳定的位置上,以获取详细的视觉信息。
定视运动的时长通常在100到400毫秒之间。
1.3 跟踪运动(Smooth Pursuits)跟踪运动是眼球快速而平滑地跟踪运动目标的运动。
在跟踪运动期间,眼球会持续地追踪运动目标,以保证目标始终在视野中心。
2. 眼动数据的采集眼动数据的采集是眼球追踪技术的第一步。
这个过程通常需要使用专业的眼动仪设备,例如高速摄像机和红外光源,以获取准确、精细的眼动数据。
2.1 红外反射原理眼动仪通常是通过红外光源和反光红外摄像机来实现眼动数据的采集。
红外反射原理是利用红外光在眼睛角膜和晶状体上的反射来确定眼球的注视位置。
具体来说,红外光源会发出红外光,照射到眼睛的角膜上后,会被角膜和晶状体反射回来。
眼动仪中的摄像机会捕捉这些反射光,并计算出眼球的位置和运动轨迹。
2.2 眼动数据的精确度眼动数据的精确度是影响眼球追踪技术的重要因素之一。
眼动仪的采样率和眼动仪的精度都会影响到眼动数据的准确性。
采样率是指眼动仪每秒对眼动数据的采样次数,通常以赫兹(Hz)为单位。
视觉训练的方法
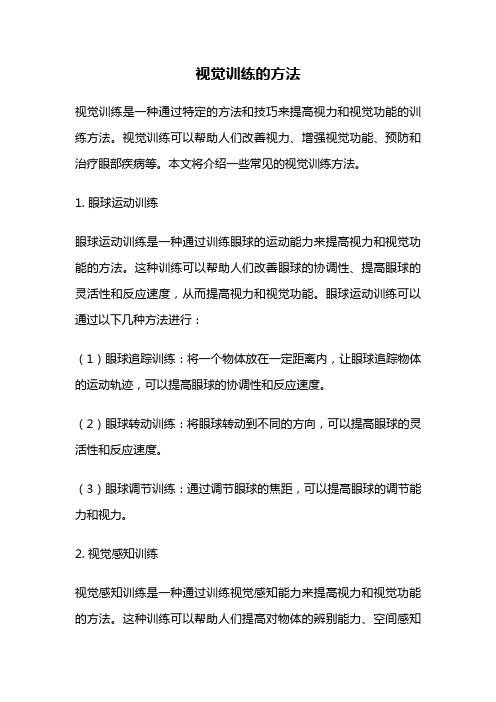
视觉训练的方法视觉训练是一种通过特定的方法和技巧来提高视力和视觉功能的训练方法。
视觉训练可以帮助人们改善视力、增强视觉功能、预防和治疗眼部疾病等。
本文将介绍一些常见的视觉训练方法。
1. 眼球运动训练眼球运动训练是一种通过训练眼球的运动能力来提高视力和视觉功能的方法。
这种训练可以帮助人们改善眼球的协调性、提高眼球的灵活性和反应速度,从而提高视力和视觉功能。
眼球运动训练可以通过以下几种方法进行:(1)眼球追踪训练:将一个物体放在一定距离内,让眼球追踪物体的运动轨迹,可以提高眼球的协调性和反应速度。
(2)眼球转动训练:将眼球转动到不同的方向,可以提高眼球的灵活性和反应速度。
(3)眼球调节训练:通过调节眼球的焦距,可以提高眼球的调节能力和视力。
2. 视觉感知训练视觉感知训练是一种通过训练视觉感知能力来提高视力和视觉功能的方法。
这种训练可以帮助人们提高对物体的辨别能力、空间感知能力和视觉记忆能力,从而提高视力和视觉功能。
视觉感知训练可以通过以下几种方法进行:(1)视觉辨别训练:通过辨别不同的形状、颜色、大小等特征,可以提高对物体的辨别能力。
(2)空间感知训练:通过观察不同的空间结构和形状,可以提高空间感知能力。
(3)视觉记忆训练:通过观察一些图形或物体,然后关闭眼睛,尝试回忆这些图形或物体的形状、颜色、大小等特征,可以提高视觉记忆能力。
3. 视觉保健训练视觉保健训练是一种通过保护眼睛和预防眼部疾病来提高视力和视觉功能的方法。
这种训练可以帮助人们预防和治疗眼部疾病,保护眼睛健康,从而提高视力和视觉功能。
视觉保健训练可以通过以下几种方法进行:(1)眼保健操:通过一些简单的眼部运动,可以缓解眼部疲劳,预防眼部疾病。
(2)眼部按摩:通过按摩眼部周围的穴位,可以促进眼部血液循环,缓解眼部疲劳。
(3)眼部保湿:通过使用眼药水或眼部保湿剂,可以保持眼部湿润,预防眼部干燥和疾病。
视觉训练是一种非常重要的训练方法,可以帮助人们提高视力和视觉功能,预防和治疗眼部疾病。
眼球追踪具体的实施步骤是

眼球追踪具体的实施步骤是简介眼球追踪是一种通过追踪人眼运动来获取信息的技术。
它被广泛应用于用户界面设计、市场研究、眼科医学等领域。
本文将介绍眼球追踪具体的实施步骤,帮助读者了解如何进行眼动追踪研究。
步骤一:确定研究目标与问题在进行眼球追踪之前,首先需要明确研究目标与问题,确定需要收集什么样的眼动数据。
例如,你可能想要了解用户在浏览网页时的注意力焦点,或者评估不同图像对用户注意力的吸引程度。
步骤二:选择适当的设备与软件眼动追踪需要使用专用的设备和软件来实现。
根据研究的具体需求,选择合适的眼动仪和相应的软件。
现在市场上有很多种类和品牌的眼动仪可供选择,常见的眼动软件有Tobii Studio、SMI BeGaze等。
步骤三:招募参与者并取得知情同意眼动追踪需要招募参与者来进行实验。
根据研究的需求确定参与者的特征(例如年龄、性别、职业等),并通过适当的渠道招募他们。
在实验开始之前,必须取得参与者的知情同意,告知他们实验的目的和过程,并保证其个人信息的保密和权益。
步骤四:准备实验环境与材料在进行眼球追踪实验之前,需要准备合适的实验环境和材料。
实验室或研究场所应该保持安静、光线适中,以确保准确记录眼动数据。
根据研究目标,准备好实验所需的图像、视频、网页或其他媒体材料。
步骤五:校准眼动仪在实验开始之前,需要对眼动仪进行校准,以确保准确记录参与者的眼动数据。
校准过程通常包括让参与者注视特定位置或物体,并使用软件进行校准调整。
校准过程需要严格按照设备和软件的说明进行,确保数据的准确性和可靠性。
步骤六:实施实验并收集数据实验开始后,参与者需要按照实验设计和要求进行眼动追踪。
根据实验的不同,可以采用自由观看或任务驱动等不同的实验方式。
在实验过程中,使用眼动软件记录和保存眼动数据,同时记录参与者相关的行为数据。
步骤七:数据预处理与分析收集到眼动数据后,需要对数据进行预处理和分析,以得出有效的结果。
眼球追踪数据常常需要进行滤波、校正和注视区域划分等操作,以消除误差和噪音。
虚拟现实相关名词

虚拟现实相关名词虚拟现实(Virtual Reality,简称VR)是一种人工构造的、存在于计算机内部的环境。
在这个虚拟世界中,人们可以感受到视觉、听觉、触觉等方面的刺激,就像在现实世界中一样。
VR领域涉及许多专业名词,以下是一些常见的虚拟现实相关名词:1.头戴显示器(Head Mounted Display,简称HMD):这是虚拟现实领域中出现频率最高的词语,是一种戴在头上的设备,用于显示虚拟世界的图像。
2.头部追踪(Head tracking):利用传感器时刻追踪使用者头部的运动,然后根据头部的姿势移动所显示的内容。
3.眼球追踪(Eye tracking):类似于头部追踪,但图像的呈现取决于使用者眼睛所看的方向。
4.视野(Field of view):指眼睛可以看到影像的角度。
在VR 体验中,更广阔的视角能让使用者更有身临其境的感受。
5.延迟(Latency):当转动头部时,看到的图像并不会随着视线立即移动的现象。
这是虚拟现实技术中需要解决的一个重要问题。
6.模拟器眩晕症(Simulator sickness):由大脑和身体处理信息的不一致引起的VR设备带来的副作用之一,可能导致使用者在体验过程中出现头晕、恶心等症状。
7.增强现实(Augmented Reality,简称AR):这是一种将真实环境和虚拟信息或物体展现在同一个画面里的技术,可以将计算机生成的元素覆盖在真实世界上。
8.混合现实(Mixed Reality,简称MR):这是虚拟现实和增强现实的结合,提供一个新的可视化环境,其中物理实体和数字对象形成类似于全息影像的效果,可以进行一些交互行为。
这些名词都是虚拟现实领域中的重要概念和技术,对于理解和应用虚拟现实技术具有重要意义。
舞蹈眼球追踪教案

舞蹈眼球追踪教案一、教学目标。
1. 帮助学生了解眼球追踪的重要性和作用。
2. 教授学生舞蹈中眼球追踪的基本技巧和方法。
3. 培养学生的观察力和动作反应能力。
二、教学重点。
1. 眼球追踪的定义和作用。
2. 舞蹈中眼球追踪的基本技巧。
3. 练习眼球追踪的方法和注意事项。
三、教学难点。
1. 如何在舞蹈中保持良好的眼球追踪。
2. 如何将眼球追踪与舞蹈动作相结合。
四、教学过程。
1. 导入。
通过观看一段精彩的舞蹈视频,引导学生注意观察舞者的眼球追踪动作,激发学生的学习兴趣。
2. 讲解眼球追踪的定义和作用。
向学生介绍眼球追踪的概念,即眼睛跟随移动的目标物体或动作的能力。
解释眼球追踪在舞蹈中的重要性,它可以帮助舞者更准确地把握舞蹈动作的节奏和力度,提高舞蹈表现力。
3. 教授眼球追踪的基本技巧。
(1)眼球追踪的范围,教导学生如何在舞蹈中灵活地运用眼球追踪,包括头部、眼睛和颈部的协调运动。
(2)眼球追踪的速度,指导学生如何根据舞蹈节奏和动作的变化,调整眼球追踪的速度,保持与舞蹈动作的同步。
(3)眼球追踪的精准度,教授学生如何准确地将视线聚焦在目标物体或动作上,避免视线的漂移和模糊。
4. 练习眼球追踪的方法和注意事项。
(1)眼球追踪的基础练习,设计一些简单的眼球追踪练习,如追踪移动的手指、舞蹈动作等,帮助学生掌握基本的眼球追踪技巧。
(2)眼球追踪与舞蹈动作的结合练习,通过编排一些舞蹈动作,要求学生在执行舞蹈动作的同时,保持良好的眼球追踪,提高学生的观察力和动作反应能力。
五、教学总结。
通过本节课的教学,学生应该能够了解眼球追踪的重要性和作用,掌握舞蹈中眼球追踪的基本技巧和方法,并能够通过练习提高自己的眼球追踪能力。
同时,教师应该对学生的学习情况进行总结和评价,及时给予指导和帮助。
六、作业布置。
要求学生在家中练习眼球追踪的基本技巧,并结合舞蹈动作进行练习,以便下节课进行检查和指导。
七、教学反思。
通过本节课的教学,我发现学生对眼球追踪的理解和掌握程度还有待提高。
眼球追踪技术的原理

眼球追踪技术的原理眼球追踪技术是一种通过追踪人眼运动来获取信息的技术。
它的原理是通过摄像头或红外线传感器等设备来捕捉人眼的运动轨迹,然后将这些数据转化为数字信号,最终通过计算机算法来分析和解读这些信号,从而得出人眼的运动轨迹和注视点。
眼球追踪技术的原理可以分为两个方面:眼球运动和注视点。
眼球运动是指人眼在观察物体时的运动轨迹,包括眼球的转动、扫视和跳跃等。
这些运动轨迹可以通过摄像头或红外线传感器等设备来捕捉和记录。
在捕捉到这些数据后,计算机会将其转化为数字信号,并通过算法来分析和解读这些信号,从而得出人眼的运动轨迹。
注视点是指人眼在观察物体时停留的位置,也就是人眼的注意力所集中的点。
注视点可以通过眼球追踪技术来确定。
当人眼停留在某个位置时,摄像头或红外线传感器等设备会捕捉到这个位置的数据,并将其转化为数字信号。
计算机会通过算法来分析和解读这些信号,从而确定人眼的注视点。
眼球追踪技术的原理可以应用于很多领域,比如人机交互、广告营销、医学研究等。
在人机交互领域,眼球追踪技术可以用于改善用户体验,比如通过追踪用户的注视点来确定用户的兴趣点,从而提供更加个性化的服务。
在广告营销领域,眼球追踪技术可以用于评估广告的效果,比如通过追踪用户的注视点来确定用户对广告的关注度和反应。
在医学研究领域,眼球追踪技术可以用于诊断和治疗眼部疾病,比如通过追踪眼球的运动轨迹来评估眼部肌肉的功能和协调性。
总之,眼球追踪技术的原理是通过捕捉和记录人眼的运动轨迹和注视点,然后通过计算机算法来分析和解读这些数据,从而得出人眼的运动轨迹和注视点。
这项技术可以应用于很多领域,为人们的生活和工作带来更多的便利和效益。
眼动追踪优化方案

1.检查眼动追踪设备的所有硬件组件是否完整并正常工作。 2.确保硬件连接稳定,无松动或断开现象。 3.对眼动追踪设备进行电源测试,确保供电稳定。
设备软件安装与更新
1.安装最新的眼动追踪设备驱动程序和软件。 2.更新眼动追踪算法的库和模型,确保最新的优化和改进被包含在内。 3.验证所有软件组件之间的兼容性,确保系统稳定运行。
眼动追踪技术简介
▪ 眼动追踪技术的应用领域
1.眼动追踪技术可以应用于多个领域,如心理学、神经科学、 人机交互、市场营销等。 2.在心理学领域,眼动追踪技术可以用来研究个体的视觉注意 、知觉、记忆等认知过程。 3.在人机交互领域,眼动追踪技术可以用来优化界面设计,提 高用户体验。
▪ 眼动追踪技术的工作原理
▪ 眼动追踪实时性评估
1.眼动追踪实时性反映了系统对用户眼球运动的响应速度,是影响用户体验的关键 因素之一。 2.通过测量系统响应时间和帧率等指标,可以评估眼动追踪实时性能。 3.提高眼动追踪实时性可以减少用户在使用过程中感到的不适和延迟,提升用户体 验。 ---
眼动追踪性能评估
眼动追踪稳定性评估
总结与展望
技术发展趋势
1.眼动追踪技术将不断向高精度、高速度、高稳定性方向发展 。 2.随着人工智能技术的不断进步,眼动追踪技术将会更加智能 化和自主化。 3.未来眼动追踪技术将与虚拟现实、增强现实等技术相结合, 开拓更多的应用场景。
前沿技术应用
1.眼动追踪技术可用于研究人类认知和行为,为心理学、神经 科学等领域提供更多有价值的实验数据。 2.在医学领域,眼动追踪技术可用于诊断和治疗多种眼部疾病 ,如斜视、弱视等。 3.在交通领域,眼动追踪技术可用于监测驾驶员的疲劳和注意 力情况,提高交通安全性。
0019.VRAR:各类眼球追踪技术解读

VR/AR:各类眼球追踪技术解读眼睛可以为我们做很多事情,比如让我们看到旖旎的风景,漂亮的美女,传达我们的内心情绪,当然这些都是不需要依靠技术就能够做到的事情。
随着技术的进步,我们现在已经能够利用人类眼睛进行虹膜识别,虹膜识别相较面部识别、指纹识别都更加有效和安全,三星已经在Note 7上面采用这项识别技术,不过这款手机已经停售。
不出意外的话,明年的Galaxy S8也将拥有虹膜识别功能。
除了虹膜识别之外,还有眼球追踪技术。
所谓眼球追踪,是指一项技术能够追踪眼球的运动,并利用这种眼球运动来增强某个产品或服务的体验。
眼球追踪曾被错用在手机上眼球追踪技术曾经在智能手机领域火了一阵,这可能要追溯到2013年Galaxy S4手机率先搭载了眼球追踪功能,这项功能主要应用在视频播放上面。
举个例子,如果你正在观看一个视频,然后你身后的同学拍了一下你肩膀,在你转过头的时候,由于你的眼睛已经不再看着屏幕,视频会自动暂停,而当你回过头来,视频会自动继续播放。
不需要你用手去点击暂停和播放;或者你在手机上看网页,当你眼睛看到屏幕底部的时候,网页会自动翻页。
同年,LG也推出了一款拥有眼球追踪功能的LG Optimus G Pro手机。
可惜,眼球追踪未能在手机领域掀起大风大浪,原因大概有两点。
首先用户没有需求,一款智能手机的平均尺寸大约只有5英寸,在这么一丁点儿大的地方,人们更喜欢直接用手指进行交互,何况手机绝大部分功能都是使用手指进行交互,所以也不多播放/暂停这个环节;第二个原因就是,当时技术不太成熟,分辨率低,识别不够精准,导致有用户觉得眼睛累。
VR/AR:眼球追踪快到我碗里来手机:1080p屏幕已经非常足够,2K就剩了VR/AR:1080p就是个渣渣,2K是基本的手机:处理器四核就好啦,上八核的神经病啊VR/AR:尽管上吧,处理器性能只有不够用,不会剩余手机:这么大点儿屏幕,10根手指日夜不停的摸,眼球追踪你走错地儿了吧?VR/AR:眼球追踪快到我碗里来和眼球追踪在手机上略显得有点鸡肋不同,这项技术对VR/AR来说是一个关键性技术。
眼球追踪仪

眼球追踪仪眼球追踪仪,也称为眼动仪,是一种通过红外线技术来追踪人眼运动的设备。
它可以准确地记录用户的注视点和注视时间,帮助研究人员深入了解人的视觉注意过程和认知行为。
本文将对眼球追踪仪的原理、应用领域和未来发展进行详细阐述。
眼球追踪仪的工作原理是通过红外光线来探测眼睛的位置,然后计算出眼球注视点的坐标。
一般来说,眼球追踪仪由红外摄像机、红外发射器和后处理软件组成。
红外发射器发射红外光线,摄像机捕捉红外反射光线,并将其转化为电信号。
后处理软件对这些信号进行分析处理,得出注视点的坐标。
眼球追踪仪在许多领域都有广泛的应用。
在心理学领域,它被用来研究人的视觉注意过程和认知行为。
通过追踪眼球运动,研究人员可以了解人在阅读、观看广告、认知任务等活动中的注意力分配和信息处理过程。
在人机交互领域,眼球追踪仪可以用于改进用户界面和设计更符合人的认知特点的交互方式。
在医学研究中,眼球追踪仪可以帮助研究人员诊断和治疗眼部疾病,例如斜视、弱视等。
随着技术的不断发展和创新,眼球追踪仪将迎来更广阔的应用前景。
近年来,虚拟现实和增强现实技术的兴起,为眼球追踪仪带来了新的应用可能。
在虚拟现实领域,眼球追踪仪可以实时追踪用户的注视点,根据用户的注视点来改变虚拟场景的呈现,提高用户的沉浸感和交互体验。
在增强现实领域,眼球追踪仪可以用来实现更自然、精准的手势识别和交互方式。
尽管眼球追踪仪在很多领域都有广泛的应用,但仍然存在一些挑战和限制。
首先,眼球追踪仪的价格较高,限制了它在一些场景下的推广应用。
其次,眼球追踪仪对环境光线和用户眼球的姿态有一定的要求,如果光线较暗或用户的眼球姿态较为复杂,会影响到眼球追踪的准确性。
此外,一些用户可能对将眼球暴露在红外光线中感到不舒服。
综上所述,眼球追踪仪作为一种能够追踪人眼运动的设备,在心理学、人机交互和医学研究中具有重要的应用价值。
随着技术的不断发展,眼球追踪仪的应用领域将进一步拓展,带来更多的创新和发展机遇。
眼球追踪技术的原理
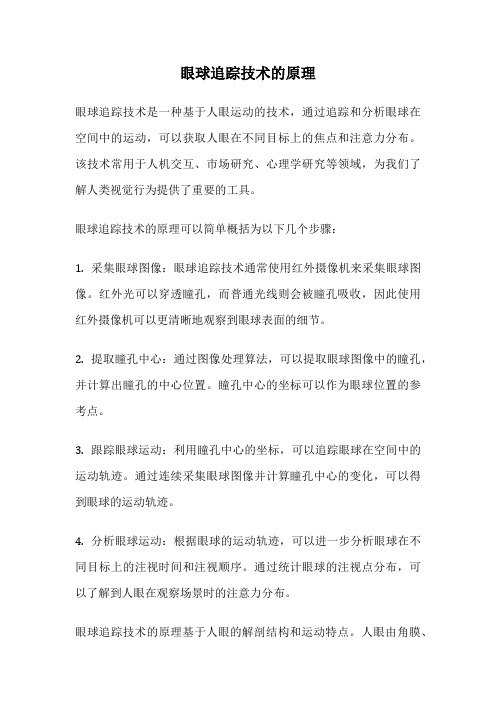
眼球追踪技术的原理眼球追踪技术是一种基于人眼运动的技术,通过追踪和分析眼球在空间中的运动,可以获取人眼在不同目标上的焦点和注意力分布。
该技术常用于人机交互、市场研究、心理学研究等领域,为我们了解人类视觉行为提供了重要的工具。
眼球追踪技术的原理可以简单概括为以下几个步骤:1. 采集眼球图像:眼球追踪技术通常使用红外摄像机来采集眼球图像。
红外光可以穿透瞳孔,而普通光线则会被瞳孔吸收,因此使用红外摄像机可以更清晰地观察到眼球表面的细节。
2. 提取瞳孔中心:通过图像处理算法,可以提取眼球图像中的瞳孔,并计算出瞳孔的中心位置。
瞳孔中心的坐标可以作为眼球位置的参考点。
3. 跟踪眼球运动:利用瞳孔中心的坐标,可以追踪眼球在空间中的运动轨迹。
通过连续采集眼球图像并计算瞳孔中心的变化,可以得到眼球的运动轨迹。
4. 分析眼球运动:根据眼球的运动轨迹,可以进一步分析眼球在不同目标上的注视时间和注视顺序。
通过统计眼球的注视点分布,可以了解到人眼在观察场景时的注意力分布。
眼球追踪技术的原理基于人眼的解剖结构和运动特点。
人眼由角膜、瞳孔、晶状体、视网膜等组成,角膜是眼球表面的透明薄膜,瞳孔是控制进入眼球的光线量的开闭孔,晶状体可以调节光线的折射度,视网膜是感受光线并将其转化为神经信号的重要组织。
当人眼注视一个目标时,光线通过角膜和晶状体的折射作用聚焦在视网膜上,形成一个清晰的图像。
同时,瞳孔的大小会根据光线的强度自动调节,以保证视网膜上的光线量适中。
当人眼转动时,眼球的肌肉会控制瞳孔的位置和大小,使光线能够准确地聚焦在视网膜上。
眼球追踪技术利用了眼球的运动特点,通过追踪和分析眼球的运动轨迹,可以推断出人眼在观察场景时的注意力分布和视觉行为。
例如,在人机交互中,可以根据用户的眼球运动来预测其意图和需求,实现更智能、更自然的交互方式。
在市场研究中,可以通过眼球追踪技术来评估广告效果、产品包装设计等,为市场营销提供决策依据。
在心理学研究中,眼球追踪技术可以揭示人眼在观察情感表情、阅读文字、解决问题等任务时的认知过程和策略。
眼球追踪原理
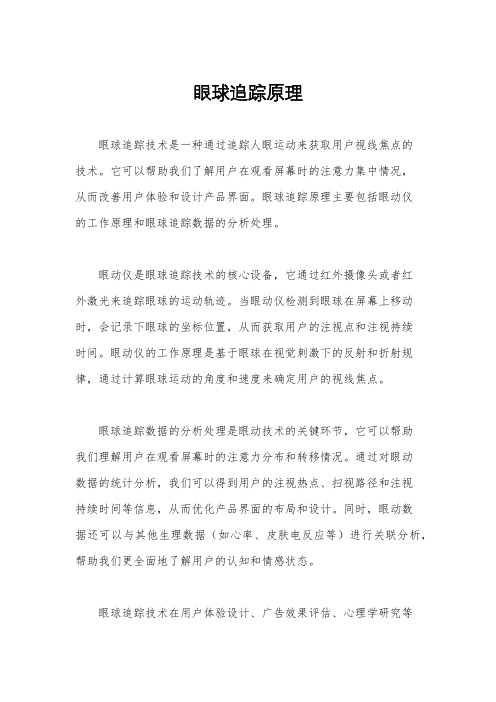
眼球追踪原理眼球追踪技术是一种通过追踪人眼运动来获取用户视线焦点的技术。
它可以帮助我们了解用户在观看屏幕时的注意力集中情况,从而改善用户体验和设计产品界面。
眼球追踪原理主要包括眼动仪的工作原理和眼球追踪数据的分析处理。
眼动仪是眼球追踪技术的核心设备,它通过红外摄像头或者红外激光来追踪眼球的运动轨迹。
当眼动仪检测到眼球在屏幕上移动时,会记录下眼球的坐标位置,从而获取用户的注视点和注视持续时间。
眼动仪的工作原理是基于眼球在视觉刺激下的反射和折射规律,通过计算眼球运动的角度和速度来确定用户的视线焦点。
眼球追踪数据的分析处理是眼动技术的关键环节,它可以帮助我们理解用户在观看屏幕时的注意力分布和转移情况。
通过对眼动数据的统计分析,我们可以得到用户的注视热点、扫视路径和注视持续时间等信息,从而优化产品界面的布局和设计。
同时,眼动数据还可以与其他生理数据(如心率、皮肤电反应等)进行关联分析,帮助我们更全面地了解用户的认知和情感状态。
眼球追踪技术在用户体验设计、广告效果评估、心理学研究等领域有着广泛的应用。
在用户体验设计中,我们可以利用眼动数据来评估用户对不同界面元素的关注度和易用性,从而指导界面设计和交互设计。
在广告效果评估中,我们可以通过眼动数据来分析用户对广告的注意力分配和情感反应,从而评估广告的吸引力和影响力。
在心理学研究中,我们可以利用眼动数据来探究人类视觉感知和认知加工的规律,从而揭示人类行为和心理活动的内在机制。
总之,眼球追踪原理是一项基于眼动仪的技术,通过追踪用户眼球运动来获取用户的视线焦点和注意力分布情况。
它可以帮助我们更好地理解用户的视觉行为和认知过程,从而指导产品设计和用户体验优化。
随着科技的不断进步,眼球追踪技术将在更多领域得到应用,并为人们的生活和工作带来更多便利和创新。
眼球追踪技术原理

眼球追踪技术原理
眼球追踪技术是一种使用专门的设备或传感器来跟踪和记录人眼运动的技术。
其原理基于眼球运动的生理特点和图像处理技术。
眼球追踪技术的原理如下:
1. 眼球运动的生理特点:人眼在视觉处理过程中会通过眼球运动来扫描周围环境。
这种眼球运动可以分为两种类型:注视和扫视。
注视是指眼球固定在某一点上,扫视则是眼球在视野中快速移动。
2. 离散或连续测量:眼球追踪技术可以通过离散或连续测量的方式记录眼球运动。
离散测量是在特定时间间隔内记录眼球位置,连续测量则是实时记录眼球位置。
3. 眼球追踪设备:眼球追踪设备通常包括红外摄像头、红外光源、红外反射物等。
红外摄像头用于捕捉眼球图像,红外光源用于提供适当的照明条件,红外反射物用于检测并定位眼球位置。
4. 眼球图像处理:通过对眼球图像进行处理,可以提取眼球位置和眼球运动特征。
常用的图像处理方法包括特征匹配、模式识别、学习算法等。
5. 数据分析和应用:应用领域种类繁多,可以用于用户界面交
互、用户体验设计、医学研究等。
通过分析眼球运动数据,可以了解用户的注意力分配、兴趣点、认知过程等。
如何提高反应速度和身体敏捷性的关键练习

如何提高反应速度和身体敏捷性的关键练习在现代社会快节奏的生活中,提高反应速度和身体敏捷性成为很多人的追求。
无论是在运动比赛中,还是在日常生活中,反应速度和身体的灵活性都起着至关重要的作用。
本文将介绍一些关键的练习方法,帮助您提高反应速度和身体敏捷性,让您在各个领域都能更加出色。
一、眼球追踪练习眼球追踪练习是提高反应速度和身体灵敏度的有效方法之一。
这种练习能够锻炼你的眼球灵活性,并加强你的注意力和反应能力。
以下是一些值得尝试的眼球追踪练习:1. 手指追踪:伸出一只手指,以均匀的速度在眼前左右移动,将注意力集中在手指上,并尽量追踪它的运动。
逐渐加快手指的速度,挑战你的反应能力。
2. 物体追踪:选择一个小而易于移动的物体,例如乒乓球或者小型玩具,将其投掷到空中,迅速移动你的眼球以追踪物体的运动轨迹。
尝试同时追踪两个或更多个物体,增加挑战的难度。
二、快速反应练习快速反应练习有助于提高你的神经反应速度,让你在关键时刻能够更加敏捷地做出正确的决策。
以下是一些快速反应练习的例子:1. 反应训练器:使用反应训练器可以锻炼你的反应速度和准确性。
这些设备会发出声音或光信号,你需要以最快的速度按下按钮或进行其他指定的动作。
根据你的进展逐渐增加难度和速度。
2. 头脑风暴游戏:玩一些头脑风暴游戏,例如快速解算数学题或者猜猜谜语,这可以帮助你迅速思考并做出反应。
与朋友进行比赛,增加乐趣和挑战。
三、爆发力和协调性练习爆发力和协调性是身体灵活性的重要组成部分。
通过下面这些练习,你可以提高你的爆发力和协调性:1. 跳绳:跳绳是一种全身性的运动,强化你的腿部力量和协调性。
尝试不同的跳绳技巧,例如单脚跳、交叉跳等,以提高你的爆发力和反应速度。
2. 捉迷藏:与朋友或家人一起玩捉迷藏游戏,这可以锻炼你的爆发力和身体协调性。
尝试寻找最佳的躲藏地点,并迅速移动以躲避追捕者的抓捕。
四、多样化的运动训练多样化的运动训练可以全面提高你的身体反应速度和灵活性,以下是几种推荐的训练方法:1. 间歇训练:进行高强度的间歇性运动训练,例如冲刺、跳跃和快速转身等,这可以培养你的身体反应速度和爆发力。
坚持五项训练,帮您的孩子告别斜弱视[1]
![坚持五项训练,帮您的孩子告别斜弱视[1]](https://img.taocdn.com/s3/m/200e5a765b8102d276a20029bd64783e09127dfc.png)
坚持五项训练,帮您的孩子告别斜弱视引言斜弱视(strabismic amblyopia)是一种常见的儿童眼病,指的是眼球运动不协调引起的视网膜形象落在不同地方,导致不同程度的视力下降。
斜弱视对孩子的生活和学习造成了很大的影响,但幸运的是,通过坚持五项训练,我们可以帮助孩子克服斜弱视,并恢复正常视力。
本文将介绍这五项训练,并详细解答相关问题。
一、眼球运动训练眼球运动训练是斜弱视治疗的重要一环,通过逐渐增加眼球运动的范围和灵活性,可以促进眼肌的协调运动,改善眼球的稳定性。
以下是几个常用的眼球运动训练方法:1.眼球追踪训练:让孩子用眼球追踪一个移动的物体,如一个笔尖或小球。
从左至右、从上至下进行移动,并控制速度和距离,逐渐增加难度。
2.眼球固定训练:让孩子用眼睛注视一个固定的物体,如一个字母或图片,保持几秒钟后再注视其他物体。
逐渐增加注视时间和困难度。
这些训练可以通过游戏的形式进行,让孩子感到愉快和有趣,提高他们的参与度和积极性。
二、视力训练视力训练是斜弱视治疗的核心内容之一,通过刺激和锻炼视觉系统,可以改善眼睛对图像的感知和处理能力,提高视力。
以下是几个常用的视力训练方法:1.视觉追踪训练:通过让孩子用眼睛追踪移动的物体,如一个飞镖或小球,可以提高眼睛对运动物体的感知和跟踪能力。
2.视觉空间定向训练:让孩子通过观察和指示,找到指定位置的物体或图案,提高眼睛对空间的感知和定向能力。
视力训练应该有计划地进行,每天坚持一定时间,逐渐增加训练的难度和强度。
三、眼睛协调训练眼睛协调训练是斜弱视治疗的重要组成部分,通过增强两只眼睛的协调性,可以有效改善斜弱视。
以下是几个常用的眼睛协调训练方法:1.双眼对焦训练:让孩子通过注视一个固定的物体,同时调整两只眼睛的焦距,使两只眼睛同时看清楚这个物体。
2.双眼交替训练:让孩子通过注视两个不同位置的物体,交替使用两只眼睛,提高两只眼睛的协调能力。
眼睛协调训练需要耐心和持之以恒,不能急于求成,要逐步提高练习的难度和时间。
眼球追踪法具体的实施步骤

眼球追踪法具体的实施步骤1. 眼球追踪法简介眼球追踪法是一种通过监测和记录眼球的运动来进行人机交互的技术。
它可以用于用户界面设计、用户行为研究、眼动数据分析等领域。
眼球追踪法的实施步骤主要包括设备准备、实验设计、实施实验、数据分析等过程。
2. 设备准备在进行眼球追踪实验之前,需要准备相应的设备。
常见的眼球追踪设备包括眼动仪、计算机、显示器等。
眼动仪用于跟踪眼球的运动,计算机用于收集和处理眼动数据,显示器用于展示实验内容。
3. 实验设计实验设计是眼球追踪法的重要组成部分。
在实验设计过程中,需要考虑以下几个方面:•实验目的:明确实验的目标和研究问题。
•受试者选择:选择符合实验需求的受试者,可以根据性别、年龄、职业等条件进行筛选。
•实验材料准备:根据实验目的和研究问题,准备相应的实验材料,例如图片、文字等。
•实验任务设计:设计不同类型的实验任务,例如浏览网页、搜索信息等。
4. 实施实验实施实验是眼球追踪法的核心环节。
在实施实验过程中,需要按照以下步骤进行操作:•让受试者进行适应训练:在实验开始前,让受试者进行适应训练,熟悉眼球追踪设备和实验操作流程。
•眼动仪校准:让受试者进行眼动仪的校准,确保准确获取眼动数据。
•实施实验任务:根据实验设计,让受试者完成实验任务,同时记录眼动数据。
•数据记录与保存:将受试者的眼动数据记录下来,并保存在计算机中,以备后续的数据分析。
5. 数据分析数据分析是眼球追踪法的关键步骤,通过对眼动数据的分析可以揭示受试者的注意力分布、信息处理过程等。
在数据分析过程中,可以采用以下方法:•热图分析:通过绘制受试者眼球停留点的热图,可以直观地展示注意力分布情况。
•注视点分析:分析受试者眼球停留点的持续时间和次数,可以了解受试者对特定信息的注意程度。
•扫视路径分析:分析受试者眼球在浏览网页等过程中的扫视路径,可以揭示受试者的浏览习惯和信息搜索策略。
6. 结果解释与应用根据数据分析的结果,可以进行结果的解释和应用。
眼球追踪技术的原理
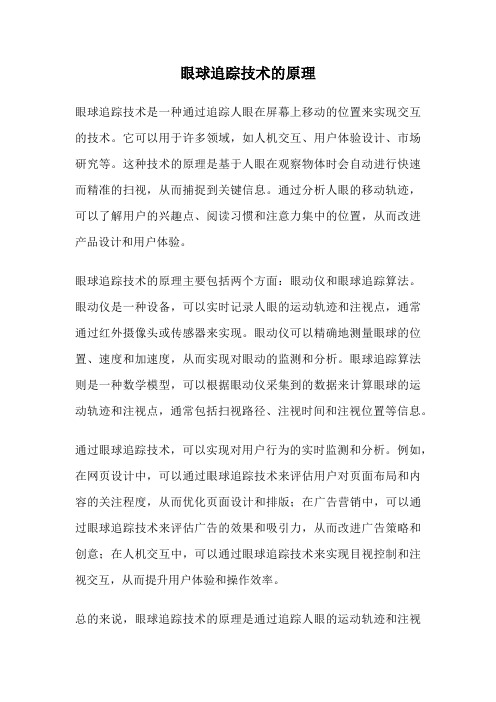
眼球追踪技术的原理
眼球追踪技术是一种通过追踪人眼在屏幕上移动的位置来实现交互的技术。
它可以用于许多领域,如人机交互、用户体验设计、市场研究等。
这种技术的原理是基于人眼在观察物体时会自动进行快速而精准的扫视,从而捕捉到关键信息。
通过分析人眼的移动轨迹,可以了解用户的兴趣点、阅读习惯和注意力集中的位置,从而改进产品设计和用户体验。
眼球追踪技术的原理主要包括两个方面:眼动仪和眼球追踪算法。
眼动仪是一种设备,可以实时记录人眼的运动轨迹和注视点,通常通过红外摄像头或传感器来实现。
眼动仪可以精确地测量眼球的位置、速度和加速度,从而实现对眼动的监测和分析。
眼球追踪算法则是一种数学模型,可以根据眼动仪采集到的数据来计算眼球的运动轨迹和注视点,通常包括扫视路径、注视时间和注视位置等信息。
通过眼球追踪技术,可以实现对用户行为的实时监测和分析。
例如,在网页设计中,可以通过眼球追踪技术来评估用户对页面布局和内容的关注程度,从而优化页面设计和排版;在广告营销中,可以通过眼球追踪技术来评估广告的效果和吸引力,从而改进广告策略和创意;在人机交互中,可以通过眼球追踪技术来实现目视控制和注视交互,从而提升用户体验和操作效率。
总的来说,眼球追踪技术的原理是通过追踪人眼的运动轨迹和注视
点来实现对用户行为的监测和分析。
通过眼动仪和眼球追踪算法的配合,可以实现对用户兴趣点、阅读习惯和注意力集中的精准捕捉,从而改进产品设计和用户体验。
这种技术在人机交互、用户体验设计和市场研究等领域具有广泛的应用前景,将为我们的生活带来更多便利和乐趣。
20XX中考语文说明文阅读训练:眼球追踪

20XX中考语文说明文阅读训练:眼球追踪中考网整理“20XX中考语文说明文阅读训练集”,供考生备考练习!20XX 中考语文说明文阅读训练:眼球追踪①也许你已经看到身边的科技迷有了神奇的“特异功能”:动动眼珠,屏幕就会翻页;眨一下眼,手机就能拍照;开车时犯困眼皮耷拉,马上就会响起语音提示……这可不是因为他们的眼睛被改造了,而是因为他们使用的设备搭载了一项名为“眼球追踪”的技术。
②眼球追踪技术的原理并不复杂:当人的眼睛看向不同方向时,眼部会有细微的变化,这些变化会产生可以提取的特征,计算机可以通过图像捕捉或扫描提取这些特征,从而实时追踪眼睛的变化,预测用户的状态和需求,并进行响应,达到用眼睛控制设备的目的。
③现阶段所采用的主要设备包括红外设备和图像采集设备。
在精度方面,红外线投射方式有比较大的优势,大概能在30英寸的屏幕上精确到1厘米以内,辅以眨眼识别、注视识别等技术,已经可以在一定程度上替代鼠标、触摸板,进行一些有限的操作。
此外,其他图像采集设备,如电脑或手机上的摄像头,在软件的支持下也可以实现眼球跟踪,但是在准确性、速度和稳定性上各有差异。
④在日常生活中,眼球追踪技术最热门的载体是手机。
比如,三星上一代旗舰机GalaxyS3就可以通过检测用户眼睛状态来控制锁屏时间,只要检测到用户正盯着手机屏幕,即使用户没有进行任何操作,屏幕也不会关闭。
而GalaxyS4的发布,则将这一功能进一步延伸:可通过眼球来控制页面上下滚动。
⑤有没有什么办法让旧的设备也能实现眼球追踪呢?一家瑞典公司Tobii今年计划推出一款产品,让旧电脑也能接入这项新技术。
这款设备名叫Rex,是一个电脑外设设备,只要把它放置在屏幕顶部,再通过USB接口接入,用户就能利用视线来控制电脑完成部分操作,比如操控IE页面滚动、使用Windows8地图应用等。
⑥除了电脑和手机,汽车也很有可能是你最早接触到眼球追踪技术的地方。
通用和丰田都已经在这方面有了不小的投入。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
}
// add in every direction
cv::Point np(p.x + 1, p.y); // right
if (floodShouldPushPoint(np, mat)) toDo.push(np);
np.x = p.x - 1; np.y = p.y; // left
//compute the threshold
double gradientThresh = computeDynamicThreshold(mags, kGradientThreshold);
//double gradientThresh = kGradientThreshold;
const bool kPlotVectorField = false;
// Size constants
const int kEyePercentTop = 25;
const int kEyePercentSide = 13;
const int kEyePercentHeight = 30;
double computeDynamicThreshold(const cv::Mat &mat, double stdDevFactor) {
cv::Scalar stdMagnGrad, meanMagnGrad;//standard deviation and mean
cv::meanStdDev(mat, meanMagnGrad, stdMagnGrad);
if (floodShouldPushPoint(np, mat)) toDo.push(np);
// kill it
mat.at<float>(p) = 0.0f;
mask.at<uchar>(p) = 0;
}
return mask;
}
//return a threshold stdDevFactor * stdDev + meanMagnGrad[0] to remove all the gradients that are below this threshold
dotProduct = std::max(0.0,dotProduct);
// square and multiply by the weight
if (kEnableWeight) {
Or[cx] += dotProduct * dotProduct * (weight/kWeightDivisor);
} else {
Or[cx] += dotProduct * dotProduct;
}
}
}
}
cv::Point findEyeCenter(cv::Mat face, cv::Rect eye) {
cv::Mat eyeROIUnscaled = face(eye);
return inMat(np, mat.rows, mat.cols);
}
cv::Mat floodKillEdges(cv::Mat &mat) {
rectangle(mat,cv::Rect(0,0,mat.cols,mat.rows),255);
cv::Mat mask(mat.rows, mat.cols, CV_8U, 255);
std::queue<cv::Point> toDo;
toDo.push(cv::Point(0,0));
while (!toDo.empty()) {
cv::Point p = toDo.front();
toDo.pop();
if (mat.at<float>(p) == 0.0f) {
cv::resize(src, dst, cv::Size(kFastEyeWidth,(((float)kFastEyeWidth)/src.cols) * src.rows));
}
cv::Mat computeMatXGradient(const cv::Mat &mat) {
cv::Mat out(mat.rows,mat.cols,CV_64F);
if (floodShouldPushPoint(np, mat)) toDo.push(np);
np.x = p.x; np.y = p.y + 1; // down
if (floodShouldPushPoint(np, mat)) toDo.push(np);
np.x = p.x; np.y = p.y - 1; // up
const int kEyePercentWidth = 35;
bool inMat(cv::Point p,int rows,int cols) {
return p.x >= 0 && p.x < cols && p.y >= 0 && p.y < rows;
}
bool floodShouldPushPoint(const cv::Point &np, const cv::Mat &mat) {
#include "opencv2/objdetect/objdetect.hpp"
#include "opencv2/highgui/highgui.hpp"
#include "opencv2/imgproc/imgproc.hpp"
#include <iostream>
#include <queue>
continue;
}
// create a vector from the possible center to the gradient origin
double dx = x - cx;
double dy = y - cy;
//double gradientThresh = 0;
//normalize
for (int y = 0; y < eyeROI.rows; ++y) {
double *Xr = gradientX.ptr<double>(y), *Yr = gradientY.ptr<double>(y);
const double *Xr = matX.ptr<double>(y), *Yr = matY.ptr<double>(y);
double *Mr = mags.ptr<double>(y);
for (int x = 0; x < matX.cols; ++x) {
const double kGradientThreshold = 50.0;
const int kWeightBlurSize = 5;
const bool kEnablePostProcess = true;
const float kPostProcessThreshold = 0.97;
for (int cy = 0; cy < out.rows; ++cy) {
double *Or = out.ptr<double>(cy);
for (int cx = 0; cx < out.cols; ++cx) {
if (x == cx && y == cy) {
double gX = Xr[x], gY = Yr[x];
double magnitude = sqrt((gX * gX) + (gY * gY));
Mr[x] = magnitude;
}
}
return mags;
}
cv::Point unscalePoint(cv::Point p, cv::Rect origSize) {
// normalize d
double magnitude = sqrt((dx * dx) + (dy * dy));
dx = dx / magnitude;
dy = dy / magnitude;
double dotProduct = dx*gx + dy*gy;
double stdDev = stdMagnGrad[0] / sqrt((double)(mat.rows*mat.cols));
return stdDevFactor * stdDev + meanMagnGrad[0];
}
//compute the magnitude of gradient
for (int y = 0; y < mat.rows; ++y) {
const uchar *Mr = mat.ptr<uchar>(y);
double *Or = out.ptr<double>(y);
Or[0] = Mr[1] - Mr[0];
float ratio = (((float)kFastEyeWidth)/origSize.width);
int x = cvRound(p.x / ratio);
int y = cvRound(p.y / ratio);
return cv::Point(x,y);