银行业务模拟(c源代码)
银行业务c语言

银行业务c语言银行业务涉及多种操作,如存款、取款、转账、查询余额等。
使用C 语言来模拟银行业务是一个很好的练习,可以帮助你了解基本的数据结构、循环、条件语句以及函数等编程概念。
以下是一个简单的C语言程序,用于模拟基本的银行业务:c复制代码#include <stdio.h>// 定义账户结构体typedef struct {int account_number;float balance;} BankAccount;// 存款函数void deposit(BankAccount *account, float amount) {account->balance += amount;printf("Deposit successful. Your new balance is %.2f\n", account->balance);}// 取款函数void withdraw(BankAccount *account, float amount) {if (account->balance >= amount) {account->balance -= amount;printf("Withdrawal successful. Your new balance is %.2f\n", account->balance);} else {printf("Insufficient balance. Withdrawal failed.\n");}}// 显示账户信息void displayAccountInfo(BankAccount *account) {printf("Account number: %d\n", account->account_number);printf("Balance: %.2f\n", account->balance);}int main() {BankAccount myAccount = {12345, 1000.00}; // 初始化账户// 显示初始账户信息printf("Initial account information:\n");displayAccountInfo(&myAccount);// 存款deposit(&myAccount, 500.00);// 取款withdraw(&myAccount, 200.00);// 再次显示账户信息printf("\nUpdated account information:\n");displayAccountInfo(&myAccount);return 0;}这个程序定义了一个名为BankAccount的结构体,该结构体包含账户号和余额两个字段。
银行业务模拟程序

银⾏业务模拟程序实验⼆银⾏业务模拟程序⼀、实验⽬的⽤Turbo C 语⾔设计实现⼀个⽤事件驱动的银⾏业务离散模型, 模拟每⼀个客户到达银⾏、排⼊⼈最少的业务窗⼝队列、排⾄窗⼝并处理完业务后离开的整个过程,统计客户在银⾏的平均逗留时间⼆、实验环境1、Windows7操作系统;2、VisualC++.三、实验内容假设某银⾏有4个窗⼝对外接待客户,从早上银⾏开门起不断有客户进⼊银⾏。
由于每个窗⼝在某时刻只能接待⼀个客户,因此在客户⼈数众多的时需在每个窗⼝前顺次排队,对于刚进⼊银⾏的客户,如果某个窗⼝的业务员正空闲,则可上前办理业务;反之,若4个窗⼝均有客户所占,他便会排在⼈数最少的队伍后⾯。
现在需要编制⼀个程序⼀模拟银⾏的这种业务活动并计算⼀天中客户在银⾏的平均逗留时间。
假设每个客户办理业务的时间不超过30分钟;两个相邻到达银⾏的客户的时间间隔不超过5分钟。
模拟程序从第⼀个客户到达时间为“0”开始起运⾏。
四、概要设计1、存储结构的类型定义:此模拟程序需要两种数据类型:有序链表和队列。
它们的数据元素类型定义分别如下:(1)typedef struct{int OccurTime; //事件发⽣时刻int NType; //事件类型,Qu表⽰到达事件,0-Qu-1表⽰Qu个窗⼝的离开事件}Event,ElemType;//事件类型,有序链表LinkList的数据元类型typedef LinkList EventList; // 事件链表类型,定义为有序链表(2)typedef struct{int ArrivalTime;//到达时刻int Duration;//办理业务所需时间}QElemType; //队列的数据元素类型2、单链表⽰意图:如课本P69图所⽰。
3、项⽬组成图:4、exp_yh.cpp的程序⽂件包含的函数原型及功能int cmp(Event a,Event b){ }:依事件a的发⽣时刻<、=或>事件b的发⽣时刻分别返回-1、0或1 ;Status InitList(LinkList &L):构造⼀个空的线性链表;Status InitQueue(LinkQueue &Q):构造⼀个空的队列;Status OrderInsert():已知L为有序线性链表,将元素e按⾮降序插⼊在L中;void OpenForDay():初始化操作;void Random():产⽣两个随机数,分别表⽰客户办理时间和两个相邻达到银⾏的客户的时间间隔;int QueueLength():求队列的长度;int Minimum() :返回最短队列的序号Status EnQueue():插⼊元素e为Q的新的队尾元素;Status DeQueue():若队列不空,删除Q的对头元素,⽤e返回其值,并返回OK,否则返回ERRORV oid CustomerArrived():处理客户到达事件;Status GetHead():若队列不空,⽤e返回Q的对头元素,并返回OK,否则返回ERROR;Position GetHead():返回线性链表L中头结点的位置;Status QueueEmpty():若Q为空队列,则返回TRUE,否则返回FALSE;void CustomerDeparture():处理客户离开事件Status ListEmpty():若线性链表L为空表,则返回TRUE,否则返回FALSE;Status DelFirst():h指向L的⼀个结点,把h当做头结点,删除链表中的第⼀个节点并以q返回,若链表为空,q=NULL,并返回FALSE;ElemType GetCurElem():已知p指向线性链表中的⼀个结点,返回p所指节点中数据元素的值;void Bank_Simulation():打印出窗⼝,平均逗留时间main():主函数5、项⽬的模块结构及函数调⽤关系:本程序包含5个模块:(1)主程序模块:void main(){ 接受命令;处理命令;}(2)事件链表表单元模块——实现链表的抽象数据类型;(3)队列单元模块——实现队列的抽象数据类型;(4)事件结点结构单元模块——定义链表的结点结构;(5)队列结点结构单元模块——定义队列的结点结构;各模块之间的调⽤关系如下:主程序模块—〉链表、队列表单元模块—>队列、链表结点结构单元模块。
C语言做的银行系统
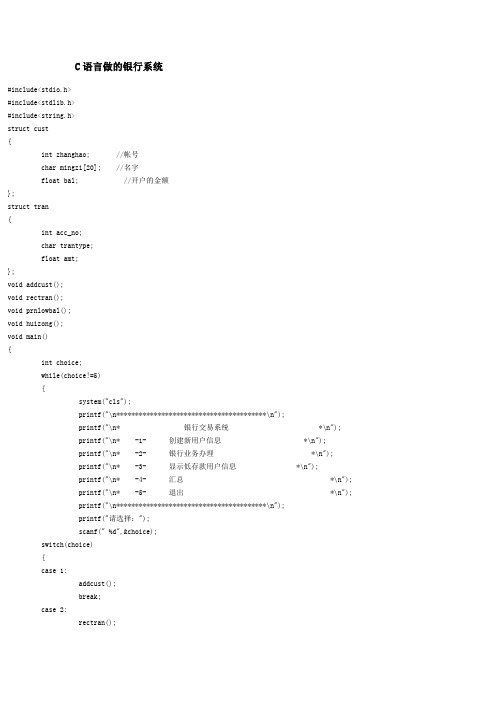
C语言做的银行系统#include<stdio.h>#include<stdlib.h>#include<string.h>struct cust{int zhanghao; //帐号char mingzi[20]; //名字float bal; //开户的金额};struct tran{int acc_no;char trantype;float amt;};void addcust();void rectran();void prnlowbal();void huizong();void main(){int choice;while(choice!=5){system("cls");printf("\n****************************************\n");printf("\n* 银行交易系统 *\n");printf("\n* -1- 创建新用户信息 *\n");printf("\n* -2- 银行业务办理 *\n"); printf("\n* -3- 显示低存款用户信息 *\n");printf("\n* -4- 汇总 *\n");printf("\n* -5- 退出 *\n");printf("\n****************************************\n");printf("请选择:");scanf(" %d",&choice);switch(choice){case 1:addcust();break;case 2:rectran();break;case 3:prnlowbal();break;case 4:huizong();break;default:exit(0);}}}void addcust(){FILE *fp;char flag='y';struct cust people;if((fp=fopen("sql.txt","w"))==NULL){printf("\n **** 操作错误,重新输入 ****\n"); getchar();return;}while(flag=='y'){system("cls");printf("\n 帐号:");scanf("%d",&people.zhanghao);printf("\n 姓名:");scanf("%s",&people.mingzi);printf("\n 金额:");scanf("%f",&people.bal);fwrite(&people,sizeof(struct cust),1,fp);getchar();printf("\n 是否继续(y/n)?:");scanf(" %c",&flag);}fclose(fp);}void rectran(){FILE *fp1,*fp2;char flag='y',found,val_flag;struct cust people;struct tran people2;int size=sizeof(struct cust);if((fp1=fopen("sql.txt","a+f"))==NULL){printf("\n **** 操作错误,重新输入! ****\n");getchar();exit(0);}if((fp2=fopen("trans.dat","a+f"))==NULL){printf("\n **** 操作错误,重新输入! ****\n");getchar();return;}while(flag=='y'){system("cls");printf("\n帐号:");scanf("%d",&people2.acc_no);found='n';val_flag='n';rewind(fp1);while((fread(&people,size,1,fp1))==1 && found=='n'){if(people.zhanghao==people2.acc_no){found='y';break;}}if(found=='y'){while(val_flag=='n'){printf("\n 选择交易方式存/取(D/W)?:");scanf(" %c",&people2.trantype);if(people2.trantype!='D'&&people2.trantype!='d'&&people2.trantype!='w'&&people2.trantype!='W') {printf("\n **** 交易错误,重新输入 ****\n");}else{val_flag='y';}}val_flag='n';while(val_flag=='n'){printf("\n 金额:");scanf("%f",&people2.amt);if(people2.trantype=='w'||people2.trantype=='W'){if(people2.amt>people.bal){printf("\n *** 余额:%.2f不足.重新输入。
c++银行业务模拟系统
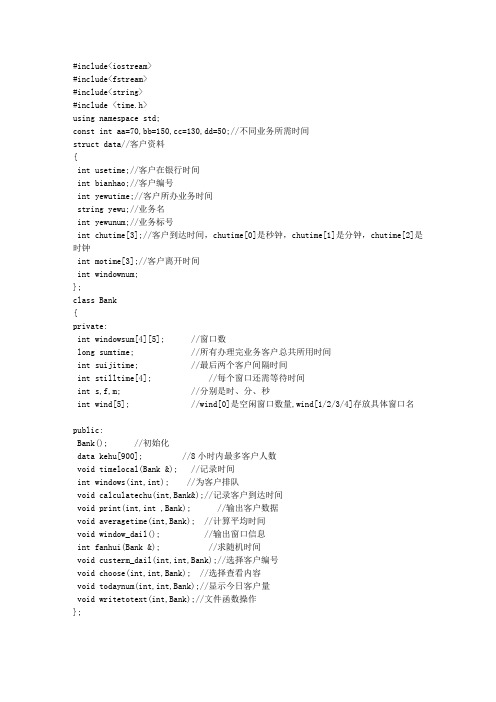
#include<iostream>#include<fstream>#include<string>#include <time.h>using namespace std;const int aa=70,bb=150,cc=130,dd=50;//不同业务所需时间struct data//客户资料{int usetime;//客户在银行时间int bianhao;//客户编号int yewutime;//客户所办业务时间string yewu;//业务名int yewunum;//业务标号int chutime[3];//客户到达时间,chutime[0]是秒钟,chutime[1]是分钟,chutime[2]是时钟int motime[3];//客户离开时间int windownum;};class Bank{private:int windowsum[4][5]; //窗口数long sumtime; //所有办理完业务客户总共所用时间int suijitime; //最后两个客户间隔时间int stilltime[4]; //每个窗口还需等待时间int s,f,m; //分别是时、分、秒int wind[5]; //wind[0]是空闲窗口数量,wind[1/2/3/4]存放具体窗口名public:Bank(); //初始化data kehu[900]; //8小时内最多客户人数void timelocal(Bank &); //记录时间int windows(int,int); //为客户排队void calculatechu(int,Bank&);//记录客户到达时间void print(int,int ,Bank); //输出客户数据void averagetime(int,Bank); //计算平均时间void window_dail(); //输出窗口信息int fanhui(Bank &); //求随机时间void custerm_dail(int,int,Bank);//选择客户编号void choose(int,int,Bank); //选择查看内容void todaynum(int,int,Bank);//显示今日客户量void writetotext(int,Bank);//文件函数操作};Bank::Bank()//构造{s=8;//时间f=0;m=0;suijitime=0; //随机间隔时间sumtime=0; //所有客户在银行所用时间之和for(int i=0;i<4;i++){stilltime[i]=0; //每个窗口处理剩下业务还需要的时间for(int j=0;j<5;j++)windowsum[i][j]=0; //每个窗口处理客户数}for(int a=0;a<5;a++)wind[a]=0; //wind[0]是空闲窗口数量,wind[1/2/3/4]存放具体窗口名}int Bank::fanhui(Bank &wait){suijitime=rand()%60; //客人随机到达m=m+suijitime; //m即是当地时间while(m/60>=1) //完成时间格式{m=m-60;f++;}while(f/60>=1){f=f-60;s++;}return s;}void Bank::timelocal(Bank &wait) //记录银行时间{cout<<"您到达的时间是: ";if(s<10)cout<<"0"<<s<<":";//特定格式输出else cout<<s<<":";if(f<10)cout<<"0"<<f<<":";else cout<<f<<":";if(m<10)cout<<"0"<<m<<endl;else cout<<m<<endl;}void Bank::averagetime(int x,Bank wait)//计算所有客户的平均等待时间{long aver=0;//平均时间int fen=0,miao=0;//平均时间以分、秒表示int j;sumtime=0;for(j=0;j<x;j++){sumtime=sumtime+wait.kehu[j].usetime;//总共时间}aver=sumtime/(x);//计算fen=aver/60;miao=aver;cout<<"所有客户平均等待了 "<<fen<<"分"<<miao<<"秒!"<<endl<<endl;}//求空窗口数int Bank::windows(int x,int i)//x是办理业务的客户数,i是最后一位顾客的编号,wait 是主函数里定义的对象{int a,b=1,t=0;for(a=0;a<5;a++)//初始化窗口空闲信息wind[a]=0;for(a=0;a<4;a++){stilltime[a]=stilltime[a]-suijitime;//特定窗口处理还需的时间等于上次处理所需的时间减去随机等待时间if(stilltime[a]<=0) //如果时间为负,重新赋值{stilltime[a]=0;wind[0]++; //空余窗口数wind[b++]=a+1; //指出特定的空闲窗口}}t=wind[0];b=1;while(x<=i && t>0) //窗口不空{stilltime[wind[b]-1]=stilltime[wind[b]-1]+kehu[x].yewutime;//特定窗口处理完业务所需时间等于处理完上次业务所需时间加上此次业务时间kehu[x].motime[0]=m;//客户离开时的秒钟kehu[x].motime[1]=f;//客户离开时的分钟kehu[x].motime[2]=s;//客户离开时的时钟kehu[x].usetime=kehu[x].motime[0]-kehu[x].chutime[0]+(kehu[x].motime[1]-kehu[x]. chutime[1])*60+(kehu[x].motime[2]-kehu[x].chutime[2])*3600+kehu[x].yewutime;//客户在银行等待时间windowsum[wind[b]-1][0]++;//特定窗口处理的客户数windowsum[wind[b]-1][kehu[x].yewunum]++; //特定窗口处理的业务数b++;x++;t--;}return x;}void Bank::calculatechu(int i,Bank &Custerm)//客户的到达时间{int j;for(j=0;j<3;j++)//初始化客户到达时间Custerm.kehu[i].chutime[j]=0;Custerm.kehu[i].chutime[0]=m;//为客户到达时间赋值Custerm.kehu[i].chutime[1]=f;Custerm.kehu[i].chutime[2]=s;}void Bank::print(int x,int i,Bank Custerm)//输出客户信息{int sec,min,hour;sec=Custerm.kehu[x].motime[0]+Custerm.kehu[x].yewutime;min=Custerm.kehu[x].motime[1];hour=Custerm.kehu[x].motime[2];while(sec>=60){sec=sec-60;min++;}while(min>=60){min=min-60;hour++;}cout<<"以下是"<<Custerm.kehu[x].bianhao<<"号客户的详细资料:"<<endl;cout<<"选择的业务:"<<Custerm.kehu[x].yewu<<endl;cout<<"选择的业务所需的时间:"<<Custerm.kehu[x].yewutime<<endl;cout<<"客户到达的时间:";for(int k=2;k>0;k--){if(Custerm.kehu[x].chutime[k]<10)cout<<"0"<<Custerm.kehu[x].chutime[k]<<":"; else cout<<Custerm.kehu[x].chutime[k]<<":";}if(Custerm.kehu[x].chutime[0]<10)cout<<"0"<<Custerm.kehu[x].chutime[0];else cout<<Custerm.kehu[x].chutime[0];cout<<endl;cout<<"客户离开的时间:";if(hour<10)cout<<"0"<<hour<<":";//特定格式输出else cout<<hour<<":";if(min<10)cout<<"0"<<min<<":";else cout<<min<<":";if(sec<10)cout<<"0"<<sec<<endl;else cout<<sec<<endl;cout<<endl;}void Bank::window_dail()//输出窗口办理业务的情况{int i;while(1){cout<<"请选择窗口(1--4),以0结束:"<<endl;cin>>i;if(i==0){system("cls");break;}if(i>=5){system("cls");cout<<"没有这个窗口,请重新选择"<<endl;}else{system("cls");cout<<" "<<i<<"窗口共处理了 "<<windowsum[i-1][0]<<"人"<<endl;cout<<"其中"<<endl;cout<<" 存款业务 "<<windowsum[i-1][1]<<"人"<<endl;cout<<" 取款业务 "<<windowsum[i-1][2]<<"人"<<endl;cout<<" 挂失业务 "<<windowsum[i-1][3]<<"人"<<endl;cout<<" 还贷业务 "<<windowsum[i-1][4]<<"人"<<endl;}}}void Bank::todaynum(int x,int i,Bank Custerm)//输出客户量{cout<<endl<<" 今日共来了"<<i<<"位客户!"<<endl;cout<<" 其中,处理了"<<x<<"位客户,还有"<<i-x<<"位客户未得到处理!"<<endl<<endl;}void Bank::custerm_dail(int i,int x,Bank Custerm)//选择输出的客户编号{int h;while(1){cout<<"请输入您想知道的客户编号(以 0 结束):";cin>>h;if(h==0){cout<<endl;break;}else if(h>i){system("cls");cout<<"没有这个编号,请重新选择!"<<endl<<endl;}else if(h>x&&h<i){system("cls");cout<<"银行停业前未处理该客户!"<<endl<<endl;}else {system("cls");print(h-1,i,Custerm);}}}void Bank::choose(int x,int i,Bank Custerm)//选择输出详细信息{int n;int flag=0;while(1){cout<<" ★★★★★★★★★★★★★★★"<<endl;cout<<endl;cout<<" ◎ 0 退出◎"<<endl<<endl;cout<<" ◎ 1 查看今日银行客户数量◎"<<endl<<endl;cout<<" ◎ 2 第n号窗口的详细信息◎"<<endl<<endl;cout<<" ◎ 3 客户平均等待时间◎"<<endl<<endl;cout<<endl;cout<<" ★★★★★★★★★★★★★★★"<<endl<<endl; cout<<" 请选择: ";cin>>n;switch(n){case 0:{flag=1;break;}case1:system("cls");todaynum(x,i,Custerm);system("pause");system("cls");break;case 2:system("cls");window_dail();break;// case 3:system("cls");custerm_dail(i,x,Custerm) ;break;case3:system("cls");averagetime(x,Custerm);system("pause");system("cls");break; default:system("cls");cout<<"没有这个选项,请重新选择!"<<endl;break;}if(flag==1)break;}}void Bank::writetotext(int x,Bank Custerm)//文件操作{int sec,min,hour;int j=0;char ch;sec=Custerm.kehu[j].motime[0]+Custerm.kehu[j].yewutime;min=Custerm.kehu[j].motime[1];hour=Custerm.kehu[j].motime[2];while(sec>=60){sec=sec-60;min++;}while(min>=60){min=min-60;hour++;}cout<<"是否生成文件?(y/n): ";cin>>ch;if(ch=='y'){if(x==0)cout<<"数据记录为空!"<<endl;else{ofstream outfile("f1.txt",ios::out);if(!outfile){cout<<"打开失败"<<endl;exit(1);}while(j<x){outfile<<" * * * * * * * * * * "<<endl;outfile<<" 客户编号: "<<kehu[j].bianhao<<endl;;outfile<<" 办理业务: "<<kehu[j].yewu<<endl;outfile<<" 业务时间: "<<kehu[j].yewutime<<endl;outfile<<" 到达时间: ";if(kehu[j].chutime[2]<10)outfile<<"0"<<kehu[j].chutime[2]<<":";else outfile<<kehu[j].chutime[2]<<":";if(kehu[j].chutime[1]<10)outfile<<"0"<<kehu[j].chutime[1]<<":";else outfile<<kehu[j].chutime[1]<<":";if(kehu[j].chutime[0]<10)outfile<<"0"<<kehu[j].chutime[0]<<":"<<endl;else outfile<<kehu[j].chutime[0]<<":"<<endl;outfile<<" 离开时间: ";sec=Custerm.kehu[j].motime[0]+Custerm.kehu[j].yewutime;min=Custerm.kehu[j].motime[1];hour=Custerm.kehu[j].motime[2];while(sec>=60){sec=sec-60;min++;}while(min>=60){min=min-60;hour++;}if(hour<10)outfile<<"0"<<hour<<":";else outfile<<hour<<":";if(min<10)outfile<<"0"<<min<<":";else outfile<<min<<":";if(sec<10)outfile<<"0"<<sec<<":"<<endl;else outfile<<sec<<":"<<endl;j++;}outfile.close();cout<<"成功生成文件!"<<endl;}}}int main (){int ff;time_t t = time(0); //时间函数char tmp[64];int x=0,i=0; //i是最后到的客户编号int flag1=1,flag=1,flag2=1; //x排在最前面的客户编号,,flag1是第一次选项的关键标志,flag标志int s=8; //16:00之前营业,s是时钟,m是最后两位客户间隔时间 int ch;Bank Custerm;string a="存款",b="取款",c="挂失",d="还贷";//业务名while(flag1){cout<<endl;cout<<" ★★★★★★★★★★★★★★★"<<endl;cout<<endl;cout<<" …………0:退出……………… "<<endl;cout<<" …………1:随机结果………… "<<endl;cout<<" …………2:输入业务………… "<<endl;cout<<" …………3:从文件读入…………"<<endl;cout<<" ★★★★★★★★★★★★★★★"<<endl;cout<<" 请选择: ";scanf("%d",&ch);switch(ch){case 0:exit(0);case 1:{flag=0;//选择是否使用系统暂停函数flag1=0;//停止本项选择break;}case 2:{flag1=0;break;}/*case 3:{flag1=0;break;}*/default:{cout<<" 没有这个选项,请重新选择!"<<endl;system("pause");break;}}system("cls");}while(s){cout<<endl;cout<<endl;cout<<"  ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄"<<endl;cout<<endl;cout<<" ★★★★★★★★★★★★★★★★★★★★★★★"<<endl;cout<<" ★★★★★★欢迎来到小辉辉银行★★★★★★"<<endl;cout<<" ★★★★★★"<<endl;cout<<" ★★"<<endl;cout<<" ★…………1:存款……………★"<<endl;cout<<" ★…………2:取款……………★"<<endl;cout<<" ★…………3:挂失……………★"<<endl;cout<<" ★…………4:还贷……………★"<<endl;cout<<" ★…………0:退出……………★"<<endl;cout<<endl;cout<<"  ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄"<<endl;if(flag==1){cin>>ff;}else{ff=(rand()%4+1);//随机选择4种业务}switch(ff){case 0:system("cls");break;{cout<<endl;cout<<"* * * * * * * * * * * * * * * "<<endl;strftime(tmp, sizeof(tmp), "%Y/%m/%d %A %z",localtime(&t) );//显示当地时间的函数puts(tmp);s=Custerm.fanhui(Custerm);if(s>=16){flag2=0;break;}Custerm.kehu[i].bianhao=i+1;//赋值编号Custerm.kehu[i].yewu=a;//赋值业务名Custerm.kehu[i].yewunum=1;//赋值业务编号Custerm.kehu[i].yewutime=aa;//赋值业务所用时间Custerm.timelocal(Custerm);//16:00之前营业,s是时钟Custerm.calculatechu(i,Custerm);//计算到达时间x=Custerm.windows(x,i);//x是排在前面的顾客编号i++;cout<<endl<<"您的号码是: "<<i<<" 办理的是: "<<a<<endl<<endl;cout<<"您前面还有"<<i-x<<"位客户"<<endl<<endl;break;}case 2:{cout<<endl;cout<<"* * * * * * * * * * * * * * * "<<endl;strftime( tmp, sizeof(tmp), "%Y/%m/%d %A %z",localtime(&t) );puts(tmp);s=Custerm.fanhui(Custerm);if(s>=16){flag2=0;break;}Custerm.kehu[i].bianhao=i+1;Custerm.kehu[i].yewu=b;Custerm.kehu[i].yewunum=2;Custerm.kehu[i].yewutime=bb;Custerm.timelocal(Custerm);Custerm.calculatechu(i,Custerm);x=Custerm.windows(x,i);cout<<endl<<"您的号码是: "<<i<<" 办理的是: "<<b<<endl<<endl; cout<<"您前面还有"<<i-x<<"位客户"<<endl<<endl;break;}case 3:{cout<<endl;cout<<"* * * * * * * * * * * * * * * "<<endl;strftime( tmp, sizeof(tmp), "%Y/%m/%d %A %z",localtime(&t) );puts(tmp);s=Custerm.fanhui(Custerm);if(s>=16){flag2=0;break;}Custerm.kehu[i].bianhao=i+1;Custerm.kehu[i].yewu=c;Custerm.kehu[i].yewunum=3;Custerm.kehu[i].yewutime=cc;Custerm.timelocal(Custerm);Custerm.calculatechu(i,Custerm);x=Custerm.windows(x,i);i++;cout<<endl<<"您的号码是: "<<i<<" 办理的是: "<<c<<endl<<endl; cout<<"您前面还有"<<i-x<<"位客户"<<endl<<endl;break;}case 4:{cout<<endl;cout<<"* * * * * * * * * * * * * * * "<<endl;strftime( tmp, sizeof(tmp), "%Y/%m/%d %A %z",localtime(&t) );puts(tmp);s=Custerm.fanhui(Custerm);if(s>=16){flag2=0;break;}Custerm.kehu[i].bianhao=i+1;Custerm.kehu[i].yewu=d;Custerm.kehu[i].yewunum=4;Custerm.kehu[i].yewutime=dd;Custerm.timelocal(Custerm);Custerm.calculatechu(i,Custerm);x=Custerm.windows(x,i);i++;cout<<endl<<"您的号码是: "<<i<<" 办理的是: "<<d<<endl<<endl; cout<<"您前面还有"<<i-x<<"位客户"<<endl<<endl;break;}default:cout<<"没有这个选项!"<<endl;break;}if(ff==0){break;}if(flag==1){system("pause");}system("cls");if(flag2==0){break;}}Custerm.choose(x,i,Custerm);Custerm.writetotext(x,Custerm);return 0;}。
银行业务模拟C++
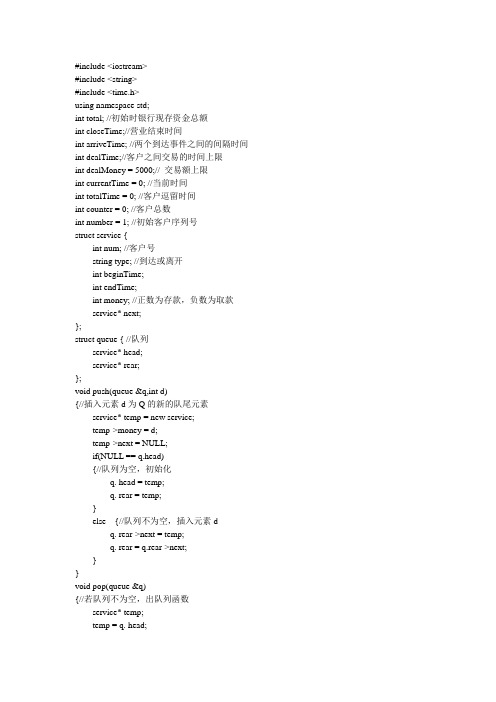
++counter;//更新客户总数
totalTime+= ( back(eq)->endTime- temped->beginTime);//更新逗留时间
delete temped; //删除节点
temped = NULL;
}
state = 0;
printf("1.开始模拟0.退出\n");
intn;
scanf("%d",&n);
while(n==1) {
srand(time(NULL)); //初始化随机函数
printf("输入银行的初始存款\n");
scanf("%d",&total);
printf("输入银行的营业时间\n");
scanf("%d",&closeTime);
while( NULL !=fq.head)
{
totalTime+= (closeTime-fq.head->beginTime);//更新结束时第一队列中为处理客户
cout<<fq.head->num <<" "<<endl;
++counter;
pop(fq);
}
cout<<"客户逗留平均时间为" <<totalTime/counter <<endl;
{//在队列中寻找可处理元素
service* sign = q. head; //标记头结点
模拟ATM银行自动取款系统.cpp 源代码和视图

模拟ATM银行自动取款系统.cpp 源代码和视图:#include<stdio.h>#include<conio.h>void part1();void part2();int c;void main(){part1();}void part1(){int a,b=622202;puts("********************************************************************** ***");puts(" 欢迎使用ATM银行自动取款系统!");printf("请插入您的卡!\n");scanf("%d",&a);if(a==b){ printf("请输入您的密码:");scanf("%d",&c);part2();//函数调用}else{printf("您的卡号不正确!");printf("谢谢惠顾!\n");getch();//从键盘接收一个字符,不回显;此处用作屏幕显示器缓冲关闭处理}}void part2(){int d=199092;if(c==d){printf("请输入取款金额:");scanf("%d",&c);printf("您的取款钱数为:%d RMB\n",c);printf("谢谢使用!\n");printf("************************************************************************* \n");getch();//从键盘接收一个字符,不回显;此处用作屏幕显示器缓冲关闭处理}elseprintf("您输入的密码错误!\n");getch();//从键盘接收一个字符,不回显;此处用作屏幕显示器缓冲关闭处理}。
银行业务系统源代码

#include<iostream>#include<windows.h>#include<string>#include<time.h>#include<stdlib.h>using namespace std;/******************窗口结构体********************/struct Windows{int deposit; /****************存款*****************/int withdrawals; /****************取款*****************/int reportloss; /****************挂失*****************/int borrowed; /****************还贷*****************/int anum[1000]; /**************保存客户***************/};/******************时间结构体********************/struct Time{int hour;int minute;int second;};/******************票号结构体********************/struct Ticket{Time arrivaltime; /****************到达时间*****************/ int number; /****************编号*****************/ int business; /****************办理业务*****************/ };const int QueueSize=1000;class BankBusinses{public:BankBusinses();void EnQueue();int GetQueue();void Personnel_Func(Ticket t2[],int n,int s); /***************手动业务生成函数***************/int Ticket_Produce_Function(Ticket t[],int n); /***************打号函数***************/int Personnel_Distribution_Func(int n,int flag); /***************业务办理函数***************/int Business_statistic_Func(Windows win[],int m); /***************业务统计函数***************/void Display_Business_Func(Windows win[],int m,int n,int coordinate); /***************业务显示函数***************/void Make_waittime_Func(int n); /***************等待时间函数***************/int Menu(); /***************菜单函数***************/Windows win[4]; /***************窗口***************/Ticket t[QueueSize]; /***************票***************/Ticket t2[QueueSize];private:string name; /***************业务名称***************/int businesstime; /***************办理业务所需时间***************/int alltime; /***************办理所有业务的总时间***************/int waittime; /***************等待时间***************/double averagetime; /***************平均等待时间***************/long *a,*b,*v;int data[QueueSize];Ticket data2[QueueSize];int front,rear;};#include "BankBusinses.h"BankBusinses::BankBusinses(){front=rear=QueueSize-1;}/*******************打号函数********************/int BankBusinses::Ticket_Produce_Function(Ticket t[],int n){int temp,sign,i,j=0;a=new long[n];b=new long[n];v=new long[n];/*******************对时间排序********************/for(i = 0;i < n;i++)for(j = i+1;j < n;j++){if(t[i].arrivaltime.hour>t[j].arrivaltime.hour){temp=t[i].arrivaltime.hour;t[i].arrivaltime.hour=t[j].arrivaltime.hour;t[j].arrivaltime.hour=temp;temp=t[i].arrivaltime.minute;t[i].arrivaltime.minute=t[j].arrivaltime.minute;t[j].arrivaltime.minute=temp;temp=t[i].arrivaltime.second;t[i].arrivaltime.second=t[j].arrivaltime.second;t[j].arrivaltime.second=temp;}}for(i = 0;i < n;i++)for(j = i+1;j < n;j++){if(t[i].arrivaltime.hour==t[j].arrivaltime.hour && t[i].arrivaltime.minute>t[j].arrivaltime.minute){temp=t[i].arrivaltime.minute;t[i].arrivaltime.minute=t[j].arrivaltime.minute;t[j].arrivaltime.minute=temp;temp=t[i].arrivaltime.second;t[i].arrivaltime.second=t[j].arrivaltime.second;t[j].arrivaltime.second=temp;}else continue;}for(i = 0;i < n;i++)for(j = i+1;j < n;j++){if(t[i].arrivaltime.hour==t[j].arrivaltime.hour && t[i].arrivaltime.minute==t[j].arrivaltime.minute && t[i].arrivaltime.second>t[j].arrivaltime.second){temp=t[i].arrivaltime.second;t[i].arrivaltime.second=t[j].arrivaltime.second;t[j].arrivaltime.second=temp;}else continue;}for(i = 0;i < n;i++){a[i]=v[i]=t[i].arrivaltime.hour*3600+t[i].arrivaltime.minute*60+t[i].arrivaltime.second;}srand(time(0));for(i = 0;i < n;i++){t[i].number = i; /************编号产生***********/rear=(rear+1)%QueueSize;data[rear]=t[i].number;sign = (rand()%(5-1))+1;t[i].business = sign; /************业务产生***********/switch(t[i].business){case 1:name = "取款";businesstime = 1;break;case 2:name = "存款";businesstime = 2;break;case 3:name = "挂失";businesstime = 3;break;case 4:name = "还贷";businesstime = 4;break;}b[i]=t[i].business*60;}return n;}/*******************手动业务生成客户编号函数********************/void BankBusinses::EnQueue(){int n,m;srand(time(0));while(front!=rear){n=(rand()%(200-1)+1);rear=(rear+1)%QueueSize;data2[rear].number=n;m=(rand()%(5-1))+1;data2[rear].business = m; /************业务产生***********/ }}int BankBusinses::GetQueue(){int i=0;while(front!=rear){front=(front+1)%QueueSize;switch(t[front].business){case 1:name = "取款";businesstime = 1;break;case 2:name = "存款";businesstime = 2;break;case 3:name = "挂失";businesstime = 3;break;case 4:name = "还贷";businesstime = 4;break;}}return data[front];}/*******************处理客户业务函数********************/int BankBusinses::Personnel_Distribution_Func(int n,int flag){int i,k=-1,j=0,m=0,g=0;int transh,transm,transs,transb;long first=0,end=0,next=0,nend=0;long c[4]={-4,-3,-2,-1},temp; //c[4]作用是对应储存四个窗口业务办理结束的时间,在循环过程中后一个业务办理结束时间将修改当前结束时间Ticket_Produce_Function(t,n); //调用时间生成函数for(i=0;i<4;i++){win[i].withdrawals=0;win[i].deposit=0;win[i].reportloss=0;win[i].borrowed=0;}c[0]=a[0]+b[0]; //第一个窗口结束时间初值为队列中第一个客户办理业务的结束时间waittime=b[0]; //第一个客户的逗留时间transh=a[0]/3600;transm=(a[0]%3600)/60;transs=(a[0]%3600)%60;transb=b[0]/60;switch(transb) //窗口各种业务累计{case 1:win[0].withdrawals++;businesstime = 1;break;case 2:win[0].deposit++;businesstime = 2;break;case 3:win[0].reportloss++;businesstime = 3;break;case 4:win[0].borrowed++;businesstime = 4;break;}v[0]=0;for(i=0;i<n;i++){int *d;d=new int[4]; //动态申请长度为四的一维数组,用于存放被修改结束时间的窗口的序号flag=i;first=a[i]; //开始时间end=a[i]+b[i]; //结束时间nend=a[i+1]+b[i+1]; //下一个结束时间next=a[i+1]; //下一个开始时间for(j=0;j<4;j++) //判断四个窗口中的结束时间是否大于下一个客户办理业务的开始时间{if(c[j]<=next){d[j]=j; //如果小于则数组的d[j]中保存该窗口的序号}else{d[j]=-1; //否则该地址存为-1}}for(j=0;j<4;j++) //判断窗口序号保存数组d[j]中是否有被保存的窗口序号{if(d[j] >= 0){k=j; //若有则结束,55且记住该窗口序号break;}else{k=-1;}}if(k>=0){c[k]=nend; //如果有窗口序号被修改,则继续修改当前结束时间为下一个客户的业务办理结束时间transh=next/3600;transm=(next%3600)/60;transs=(next%3600)%60;transb=b[i+1]/60;waittime +=b[i+1];v[i+1]=k;switch(transb) //窗口各种业务累计{case 1:win[k].withdrawals++;businesstime = 1;break;case 2:win[k].deposit++;businesstime = 2;break;case 3:win[k].reportloss++;businesstime = 3;break;case 4:win[k].borrowed++;businesstime = 4;break;}}else{ //如果当前窗口序号全未被修改,即当前所有窗口均处于忙碌状态,下一个客户需等待temp=c[0];k=0;for(j=1;j<4;j++) //找出当前所有正在办理业务的窗口中,结束时间最早的窗口{if(c[j]<temp){temp=c[j];k=j;}}if(temp<3600*17){c[k]=c[k]+b[i+1]; //修改该窗口的结束时间,即下一个在等待的客户到该窗口办理业务transh=next/3600;transm=(next%3600)/60;transs=(next%3600)%60;transb=b[i+1]/60;waittime +=temp-next+b[i+1];v[i+1]=k;switch(transb) //窗口各种业务累计{case 1:win[k].withdrawals++;businesstime = 1;break;case 2:win[k].deposit++;businesstime = 2;break;case 3:win[k].reportloss++;businesstime = 3;break;case 4:win[k].borrowed++;businesstime = 4;break;}}else break;}delete []d; //每次循环结束时撤销窗口序号保存数组d[j];}cout<<endl;return flag;}/*******************窗口业务统计函数********************/int BankBusinses::Business_statistic_Func(Windows win[],int m){int i,n=0,allnum=0;for(i=0;i<4;i++){allnum=win[i].withdrawals+win[i].deposit+win[i].reportloss+win[i].borrowed;cout <<"\t\t\t\t 窗口"<<i+1;cout <<endl;cout <<"取款人数"<<"\t存款人数"<<"\t挂失人数"<<"\t还贷人数"<<"\t办理业务总数";cout <<endl;cout<<win[i].withdrawals<<"\t\t"<<win[i].deposit<<"\t\t"<<win[i].reportloss<<"\t\t"<<win[i].borrowe d<<"\t\t"<<allnum;cout <<endl;}cout<<endl;return allnum;}/*******************窗口业务显示函数********************/void BankBusinses::Display_Business_Func(Windows win[],int m,int n,int coordinate){int j,flag=1;cout<<"\t\t\t\t窗口"<<coordinate+1<<"办理: ";cout<<endl;for(j=0;j<n;j++){if(v[j]==coordinate){cout<<j+1<<"号客户"<<"\t";flag=0;}}if(flag==1){cout<<"\t\t该窗口当天没有办理业务!\n";}cout<<endl;}/*******************等待时间函数********************/void BankBusinses::Make_waittime_Func(int n){int i,flag=0;long waittime2=0;double aver=0;flag=Personnel_Distribution_Func(n,flag);cout<<"\t\t总人数: "<<n;cout<<endl;cout<<"\t\t办理业务人数: "<<flag+1;cout<<endl;cout<<"\t\t未办理完业务人数: "<<n-flag-1;cout<<endl;if((n-flag-1)!=0){cout<<"\t\t未办理完业务的编号: "<<flag+2<<" →"<<n;}cout<<endl;aver=(waittime/(flag+1))/60.0;cout<<"\t\t已经办理业务客户平均逗留时间: "<<aver<<" 分钟";cout<<endl;for(i=flag+2;i<n;i++){waittime2 +=3600*17-a[i];}averagetime=((waittime+waittime2)/n)/60.0;cout<<"\t\t当日所有客户平均逗留时间: "<<averagetime<<" 分钟";cout<<endl;}/***************手动业务生成函数***************/void BankBusinses::Personnel_Func(Ticket t2[],int n,int s){int i=0,m=0;if(s){srand(time(0));t2[i].arrivaltime.hour = (rand()%(17-9)+9);t2[i].arrivaltime.minute = rand()%60;t2[i].arrivaltime.second = rand()%60;n=(rand()%(200-1)+1);t2[i].number=n;m=(rand()%(5-1))+1;t2[i].business = m; /************业务产生***********/switch(m){case 1:name = "取款";businesstime = 1;break;case 2:name = "存款";businesstime = 2;break;case 3:name = "挂失";businesstime = 3;break;case 4:name = "还贷";businesstime = 4;break;}system("cls"); //************清屏**********cout << "\t\t┌───────────────┐\n";cout << "\t\t│"<<"\t 中国银行"<<"\t\t│\n";cout << "\t\t││\n";cout << "\t\t│"<<"编号"<<"\t\t"<<t2[i].number<<"\t\t│\n";cout << "\t\t│"<<"业务"<<"\t\t"<<name<<"\t\t│\n";cout << "\t\t│"<<"到达时间: 2012/3/16"<<" "<<t2[i].arrivaltime.hour <<":" <<t2[i].arrivaltime.minute <<":" <<t2[i].arrivaltime.second<<"\t│\n";cout << "\t\t││\n";cout << "\t\t└───────────────┘\n";}}int BankBusinses:: Menu(){int m;while(m < 0 || m > 8){system("cls");//************清屏**********cout << "\t\t┌───────────────┐\n";cout << "\t\t│────银行业务系统─────│\n";cout << "\t\t└───────────────┘\n";cout << "\t\t┌───────────────┐\n";cout << "\t\t│1. 自动生成业务信息│\n";cout << "\t\t│2. 显示客户逗留时间│\n";cout << "\t\t│3. 显示各窗口业务统计信息│\n";cout << "\t\t│4. 显示窗口①业务办理信息│\n";cout << "\t\t│5. 显示窗口②业务办理信息│\n";cout << "\t\t│6. 显示窗口③业务办理信息│\n";cout << "\t\t│7. 显示窗口④业务办理信息│\n";cout << "\t\t│8. 手动生成业务│\n";cout << "\t\t│0. 退出程序│\n";cout << "\t\t└───────────────┘\n";cout << "\t\t请您选择(0-8): ";while(cin >>m,!cin.eof()) //流控制语句,判断变量输入是否是整型{if(!cin.good()) //如果不是整型则重新输入{cin.clear();cin.ignore();cout<<"\t\t输入错误!\n\t\t请重新输入!";Sleep(5000); //暂停1秒返回菜单重新输入return m;}else if(m<0 || m>8){cout<<"\t\t输入错误!\n\t\t请重新输入!";Sleep(5000); //暂停1秒返回菜单重新输入return m;}else if(cin.good() && (m>=0 && m<=8) ){break;}}}return m;}#include "BankBusinses.h"int main(){int i,n,m,flag=0;BankBusinses C;while(1){switch(C.Menu()){case 1:srand(time(0));n=(rand()%(500-1)+1);for(i = 0;i < n;i++){C.t[i].arrivaltime.hour = (rand()%(17-9)+9);C.t[i].arrivaltime.minute = rand()%60;C.t[i].arrivaltime.second = rand()%60;}C.Ticket_Produce_Function(C.t,n);C.EnQueue();C.GetQueue();C.Personnel_Distribution_Func(n,0);flag=1;cout<<"\t\t业务信息生成成功!\n";break;case 2:if(flag==0){cout<<"\t\t无可查询信息!\n";}else{C.Make_waittime_Func(n);}system("pause");break;case 3:if(flag==0){cout<<"\t\t无可查询信息!\n";}else{C.Business_statistic_Func(C.win,4);}system("pause");break;case 4:if(flag==0){cout<<"\t\t无可查询信息!\n";}else{C.Display_Business_Func(C.win,4,n,0);}system("pause");break;case 5:if(flag==0){cout<<"\t\t无可查询信息!\n";}else{C.Display_Business_Func(C.win,4,n,1);}break;case 6:if(flag==0){cout<<"\t\t无可查询信息!\n";}else{C.Display_Business_Func(C.win,4,n,2);}system("pause");break;case 7:if(flag==0){cout<<"\t\t无可查询信息!\n";}else{C.Display_Business_Func(C.win,4,n,3);}system("pause");break;case 8:system("cls"); //************清屏**********for(i=0;i<QueueSize;i++){cout << "\t\t┌────────────────┐\n";cout << "\t\t│────是否打号?(1/0)─────│\n";cout << "\t\t└────────────────┘\n";cout<<"\t\t是请输入1/否请输入0: ";int tag=0;while(cin >>m,!cin.eof()) //流控制语句,判断变量输入是否是整型{if(!cin.good()) //如果不是整型则重新输入{cin.clear();cin.ignore();cout<<"\t\t输入错误!\n\t\t请重新输入!";Sleep(5000); //暂停1秒返回菜单重新输入system("cls"); //************清屏**********cout << "\t\t┌────────────────┐\n";cout << "\t\t│────是否打号?(1/0)─────│\n";cout << "\t\t└────────────────┘\n";cout<<"\t\t是请输入1/否请输入0: ";}else if(m!=0 && m!=1){cout<<"\t\t输入错误!\n\t\t请重新输入!";Sleep(5000); //暂停1秒返回菜单重新输入system("cls"); //************清屏**********cout << "\t\t┌────────────────┐\n";cout << "\t\t│────是否打号?(1/0)─────│\n";cout << "\t\t└────────────────┘\n";cout<<"\t\t是请输入1/否请输入0: ";}else if(cin.good() && (m==0 || m==1) ){if(m==0){tag=1;}break;}}if(tag==1)break;C.Personnel_Func(C.t2,0,m);}system("pause");break;case 0: //如返回值为0则程序结束cout << "\t\tOVER !\n";exit(0);}}return 0;}。
银行管理系统c语言程序设计代码

银行管理系统C语言程序设计代码简介银行管理系统是一个用于模拟银行业务的计算机程序。
它可以实现用户账户的创建、存取款、转账等功能,同时还可以进行利息计算、账单管理等操作。
本文将详细介绍银行管理系统的设计和实现,包括系统的功能模块、数据结构和算法等内容。
功能模块银行管理系统主要包括以下功能模块:1.用户管理:包括用户账户的创建、修改、删除等操作。
2.账户管理:包括存款、取款、查询余额、转账等操作。
3.利息计算:根据存款金额和存款期限计算利息。
4.账单管理:记录用户的交易明细和账户余额变动。
数据结构银行管理系统使用了以下数据结构:1.用户账户结构体:包括账户ID、账户名称、账户类型等信息。
2.用户交易结构体:包括交易类型、交易金额、交易时间等信息。
3.用户账户链表:用于保存所有用户账户的信息。
4.用户交易链表:用于保存用户的交易明细。
算法设计银行管理系统使用了以下算法:1.用户账户创建算法:通过用户输入的信息创建新的账户,并将其添加到账户链表中。
2.存款算法:根据用户输入的存款金额,将其添加到账户余额中。
3.取款算法:根据用户输入的取款金额,从账户余额中扣除相应金额。
4.转账算法:根据用户输入的转账金额和目标账户ID,将相应金额从当前账户中转到目标账户中。
5.利息计算算法:根据存款金额和存款期限,计算相应的利息。
6.账单记录算法:将用户的交易明细和账户余额变动记录到交易链表中。
代码实现以下是银行管理系统的C语言代码示例:#include <stdio.h>// 用户账户结构体typedef struct {int accountId;char accountName[100];char accountType[100];float balance;} Account;// 用户交易结构体typedef struct {int accountId;char transactionType[100];float amount;char transactionTime[100];} Transaction;// 用户账户链表typedef struct {Account account;struct AccountNode* next;} AccountNode;// 用户交易链表typedef struct {Transaction transaction;struct TransactionNode* next;} TransactionNode;// 创建用户账户void createAccount(AccountNode** head, Account account) { // 创建新的账户节点AccountNode* newNode = (AccountNode*)malloc(sizeof(AccountNode)); newNode->account = account;newNode->next = NULL;// 将新的账户节点添加到链表中if (*head == NULL) {*head = newNode;} else {AccountNode* current = *head;while (current->next != NULL) {current = current->next;current->next = newNode;}}// 存款void deposit(AccountNode* head, int accountId, float amount) {AccountNode* current = head;while (current != NULL) {if (current->account.accountId == accountId) {current->account.balance += amount;break;}current = current->next;}}// 取款void withdraw(AccountNode* head, int accountId, float amount) {AccountNode* current = head;while (current != NULL) {if (current->account.accountId == accountId) {if (current->account.balance >= amount) {current->account.balance -= amount;} else {printf("Insufficient balance.\n");}break;}current = current->next;}}// 转账void transfer(AccountNode* head, int sourceAccountId, int targetAccountId, flo at amount) {AccountNode* current = head;while (current != NULL) {if (current->account.accountId == sourceAccountId) {if (current->account.balance >= amount) {current->account.balance -= amount;break;} else {printf("Insufficient balance.\n");}current = current->next;}current = head;while (current != NULL) {if (current->account.accountId == targetAccountId) {current->account.balance += amount;break;}current = current->next;}}// 利息计算float calculateInterest(float principal, int years) {float rate = 0.05; // 假设利率为5%return principal * rate * years;}// 账单记录void recordTransaction(TransactionNode** head, Transaction transaction) { // 创建新的交易节点TransactionNode* newNode = (TransactionNode*)malloc(sizeof(TransactionNod e));newNode->transaction = transaction;newNode->next = NULL;// 将新的交易节点添加到链表中if (*head == NULL) {*head = newNode;} else {TransactionNode* current = *head;while (current->next != NULL) {current = current->next;}current->next = newNode;}}int main() {AccountNode* accountList = NULL;TransactionNode* transactionList = NULL;// 创建账户Account account1 = {1, "John Doe", "Savings", 1000.0};createAccount(&accountList, account1);Account account2 = {2, "Jane Smith", "Checking", 2000.0};createAccount(&accountList, account2);// 存款deposit(accountList, 1, 500.0);// 取款withdraw(accountList, 1, 200.0);// 转账transfer(accountList, 1, 2, 300.0);// 利息计算float interest = calculateInterest(1000.0, 1);printf("Interest: %.2f\n", interest);// 账单记录Transaction transaction1 = {1, "Deposit", 500.0, "2022-01-01 10:00:00"};recordTransaction(&transactionList, transaction1);Transaction transaction2 = {1, "Withdraw", 200.0, "2022-01-02 11:00:00"};recordTransaction(&transactionList, transaction2);return 0;}总结银行管理系统是一个功能丰富的计算机程序,通过使用C语言进行设计和实现,可以实现用户账户的创建、存取款、转账等功能,同时还可以进行利息计算、账单管理等操作。
C++简单模拟银行系统
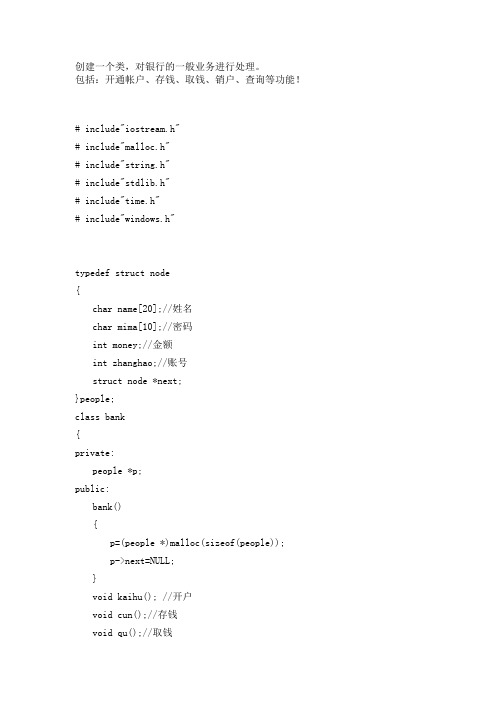
创建一个类,对银行的一般业务进行处理。
包括:开通帐户、存钱、取钱、销户、查询等功能!# include"iostream.h"# include"malloc.h"# include"string.h"# include"stdlib.h"# include"time.h"# include"windows.h"typedef struct node{char name[20];//姓名char mima[10];//密码int money;//金额int zhanghao;//账号struct node *next;}people;class bank{private:people *p;public:bank(){p=(people *)malloc(sizeof(people));p->next=NULL;}void kaihu(); //开户void cun();//存钱void qu();//取钱void xiaohu();//销户void chaxun();//查询void mulu();void xuanzhe();};void bank::kaihu(){system("cls");cout<<"\t\t\t开户"<<endl;cout<<"**************************************************"<<endl;people *m;char a[10],b[10];m=(people *)malloc(sizeof(people));srand(time(NULL));m->zhanghao=rand()%10000;memset(m->name,0,sizeof(m->name));memset(m->mima,0,sizeof(m->mima));cout<<"\n请输入姓名:";cin>>m->name;cout<<"\n请输入密码:";cin>>a;cout<<"\n请再次输入密码:";cin>>b;while(strcmp(a,b)!=0){cout<<"\n与第一次输入不符,请重新输入:";cout<<"\n请输入密码:";cin>>a;cout<<"\n请再次输入密码:";cin>>b;}strcpy(m->mima,a);m->money=100;m->next=p->next;cout<<"\n姓名:"<<m->name<<"\t账号:"<<m->zhanghao<<"\t金额:"<<m->money<<endl;p->next=m;xuanzhe();}void bank::cun(){system("cls");cout<<"\t\t\t存款"<<endl;cout<<"**************************************************"<<endl;int a,m;people *k=p;cout<<"\n请输入账号:";cin>>a;while(k!=NULL){if(k->zhanghao==a)break;else k=k->next;}if(k!=NULL){cout<<"\n姓名:"<<k->name<<"\t账号:"<<k->zhanghao<<endl;cout<<"\n请输入要存入的金额:";cin>>m;k->money=k->money+m;cout<<"\n已存入,卡中余额为:"<<k->money<<endl;}else cout<<"\n无此账户!"<<endl;xuanzhe();}void bank::qu(){system("cls");cout<<"\t\t\t取款"<<endl;cout<<"**************************************************"<<endl;char n[10];int b=2;int a,m;people *k=p;cout<<"\n请输入账号:";cin>>a;while(k!=NULL){if(k->zhanghao==a)break;else k=k->next;}if(k!=NULL){cout<<"\n请输入密码:";cin>>n;while(strcmp(k->mima,n)!=0&&b--){cout<<"\n密码错误!请重新输入,剩余次数"<<b+1<<endl;cout<<"\n请输入密码:";cin>>n;}if(b>=0){cout<<"\n姓名:"<<k->name<<"\t账号:"<<k->zhanghao<<"\t账户金额"<<k->money<<endl;cout<<"\n请输入取款金额:";cin>>m;while(m>k->money){cout<<"金额不足!";cout<<"\n请输入取款金额:";cin>>m;}k->money=k->money-m;cout<<"取款成功!账户余额:"<<k->money;}else cout<<"\n密码错误,退出!"<<endl;}else cout<<"\n无此账号!"<<endl;xuanzhe();}void bank::xiaohu(){system("cls");cout<<"\t\t\t销户"<<endl;cout<<"**************************************************"<<endl;int a,b=2,c;char n[10];memset(n,0,sizeof(n));people *k=p,*m;cout<<"\n请输入账号:"<<endl;cin>>a;while(k!=NULL){if(k->zhanghao==a)break;else{m=k;k=k->next;}}if(k!=NULL){cout<<"\n请输入密码:";cin>>n;while(strcmp(k->mima,n)!=0&&b--){cout<<"\n密码错误!请重新输入,剩余次数"<<b+1<<endl;cout<<"\n请输入密码:";cin>>n;}if(b>=0){cout<<"\n姓名:"<<k->name<<"\t账号:"<<k->zhanghao<<"\t账户金额"<<k->money<<endl;cout<<"\n确定销户请按1,返回请按2:";cin>>c;if(c==1){m->next=k->next;cout<<"\n已销户!"<<endl;xuanzhe();}if(c==2)xuanzhe();}}else xuanzhe();}void bank::chaxun(){int a,b=2,c;char n[10];memset(n,0,sizeof(n));people *k=p;cout<<"\n请输入账号:"<<endl;cin>>a;while(k!=NULL){if(k->zhanghao==a)break;else{k=k->next;}}if(k!=NULL){cout<<"\n请输入密码:";cin>>n;while(strcmp(k->mima,n)!=0&&b--){cout<<"\n密码错误!请重新输入,剩余次数"<<b+1<<endl;cout<<"\n请输入密码:";cin>>n;}if(b>=0){cout<<"\n姓名:"<<k->name<<"\t账号:"<<k->zhanghao<<"\t账户金额"<<k->money<<endl;}else cout<<"\n密码错误!退出!"<<endl;}else cout<<"\n无此账户!"<<endl;xuanzhe();}void bank::xuanzhe(){int a;cout<<"\n返回主菜单请按1,结束请按2:";cin>>a;if(a==1)mulu();if(a==2)exit(0);}void bank::mulu(){system("cls");int a;cout<<"\t\t银行系统"<<endl;cout<<"**************************************************"<<endl;cout<<"1.开户"<<endl;cout<<"2.存款"<<endl;cout<<"3.取款"<<endl;cout<<"4.销户"<<endl;cout<<"5.查询"<<endl;cout<<"**************************************************"<<endl;cout<<"\n请输入所需服务序号:";cin>>a;switch(a){case 1:kaihu();break;case 2:cun();break;case 3:qu();break;case 4:xiaohu();break;case 5:chaxun();break;}}void main(){bank b;b.mulu();}。
c语言课程设计银行模拟系统

c语言课程设计银行模拟系统一、教学目标本课程的教学目标是使学生掌握C语言的基本语法和编程技巧,能够运用C语言设计简单的银行模拟系统。
具体目标如下:1.知识目标:(1)理解C语言的基本数据类型、运算符、表达式和语句。
(2)掌握函数的定义、声明和调用。
(3)了解数组、字符串、指针的概念和应用。
(4)熟悉常用的库函数和编程规范。
2.技能目标:(1)能够使用C语言编写简单的程序,解决实际问题。
(2)能够运用C语言进行模块化编程,提高代码的可读性和可维护性。
(3)能够运用C语言设计银行模拟系统的核心功能模块,如账户管理、存款、取款、查询等。
3.情感态度价值观目标:(1)培养学生的编程兴趣,增强自信心。
(2)培养学生团队合作、自主学习的精神。
(3)使学生认识到C语言在实际应用中的重要性,激发学生的学习热情。
二、教学内容根据课程目标,教学内容主要包括以下几个部分:1.C语言基础知识:数据类型、运算符、表达式、语句等。
2.函数:函数的定义、声明和调用,函数的参数传递和返回值。
3.面向过程编程:数组、字符串、指针的概念和应用。
4.常用库函数和编程规范:标准输入输出、数学函数、字符处理等。
5.银行模拟系统设计:账户管理、存款、取款、查询等功能模块的实现。
三、教学方法本课程采用多种教学方法,以激发学生的学习兴趣和主动性:1.讲授法:讲解C语言的基本概念和语法,为学生提供清晰的知识结构。
2.案例分析法:分析实际案例,使学生更好地理解C语言的应用。
3.实验法:引导学生动手实践,培养学生的编程能力和解决问题的能力。
4.讨论法:学生进行小组讨论,促进学生之间的交流与合作。
四、教学资源为实现课程目标,我们将使用以下教学资源:1.教材:《C语言程序设计》等相关教材,为学生提供系统的学习资料。
2.参考书:提供一些经典的C语言编程书籍,供学生拓展阅读。
3.多媒体资料:制作精美的PPT,辅助讲解和展示实例。
4.实验设备:为学生提供计算机实验室,进行编程实践和实验。
c语言银行自动存取款机模拟
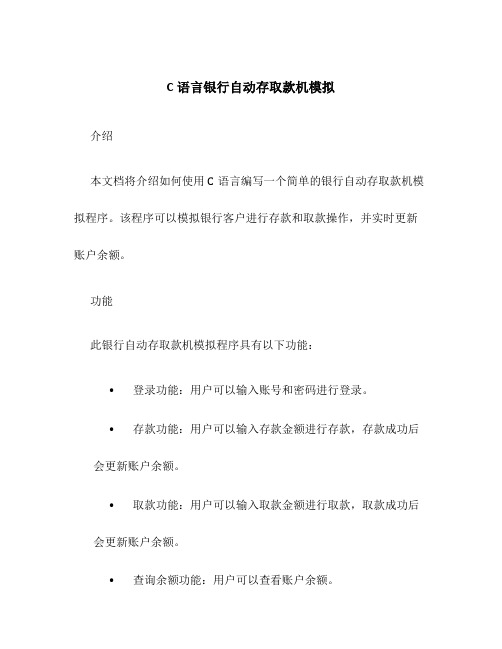
C语言银行自动存取款机模拟介绍本文档将介绍如何使用C语言编写一个简单的银行自动存取款机模拟程序。
该程序可以模拟银行客户进行存款和取款操作,并实时更新账户余额。
功能此银行自动存取款机模拟程序具有以下功能:•登录功能:用户可以输入账号和密码进行登录。
•存款功能:用户可以输入存款金额进行存款,存款成功后会更新账户余额。
•取款功能:用户可以输入取款金额进行取款,取款成功后会更新账户余额。
•查询余额功能:用户可以查看账户余额。
•退出功能:用户可以选择退出程序。
程序设计数据结构在程序中,需要定义一个结构体来表示银行客户的账户信息。
每个账户包含账号、密码和余额:struct Account {int accountNumber;char password[20];double balance;};登录功能首先,用户需要输入账号和密码进行登录。
可以定义一个函数来实现登录功能:int login(struct Account *accounts, int numAccounts, int accountNumber, char *password) {for (int i = 0; i < numAccounts; i++) {if (accounts[i].accountNumber == accountNumber && strcmp(accounts[i].password, password) == 0) {return i; // 返回账号在数组中的索引}}return -1; // 登录失败,返回-1}存款和取款功能存款和取款功能可以分别定义两个函数来实现。
存款函数会增加账户余额,取款函数会减少账户余额。
```c void deposit(struct Account *account, double amount) { account->balance += amount; }void withdraw(struct Account *account, double amount) { if (account->balance >= amount) { account->balance -= amount; printf(。
经典C源码 之银行事件驱动模拟程序
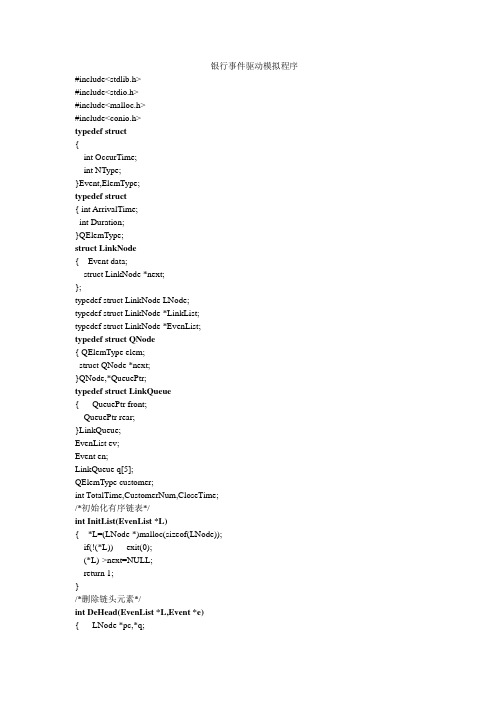
银行事件驱动模拟程序#include<stdlib.h>#include<stdio.h>#include<malloc.h>#include<conio.h>typedef struct{int OccurTime;int NType;}Event,ElemType;typedef struct{ int ArrivalTime;int Duration;}QElemType;struct LinkNode{ Event data;struct LinkNode *next;};typedef struct LinkNode LNode;typedef struct LinkNode *LinkList;typedef struct LinkNode *EvenList;typedef struct QNode{ QElemType elem;struct QNode *next;}QNode,*QueuePtr;typedef struct LinkQueue{ QueuePtr front;QueuePtr rear;}LinkQueue;EvenList ev;Event en;LinkQueue q[5];QElemType customer;int TotalTime,CustomerNum,CloseTime;/*初始化有序链表*/int InitList(EvenList *L){ *L=(LNode *)malloc(sizeof(LNode));if(!(*L)) exit(0);(*L)->next=NULL;return 1;}/*删除链头元素*/int DeHead(EvenList *L,Event *e){ LNode *pc,*q;pc=*L;q=pc->next;pc->next=q->next;*e=q->data;return 1;}/*判断有序链表是否为空*/int ListEmpty(LNode L){ LNode *p;int j=0;p=L.next;while(p){j++;break;}if(j==0) return 1;else return 0;}/*判断两个事件的发生时刻*/int CmpTime(Event a,Event b){ if(a.OccurTime>b.OccurTime) return 1;else if(a.OccurTime==b.OccurTime) return 0;else return -1;}int OrderInsert(EvenList *L,Event e,int (* cmp)(Event ,Event )) { LNode *p,*pc;/*把事件插入链表*/if((p=(LNode *)malloc(sizeof(LNode)))==NULL)/*分配空间*/ {printf("error\n");return(0);}if(ListEmpty(**L)){p->data=e;p->next=(*L)->next;(*L)->next=p;}else{switch(cmp(((*L)->next)->data,e)){case -1:pc=(*L)->next;while(pc->next!=NULL){if((pc->next)->data.OccurTime<=e.OccurTime)pc=pc->next;else break;}p->data=e;p->next=pc->next;/*把它接在比它大的前*/pc->next=p;break;case 0:pc=(*L)->next;/*相等时,接在相等的后面*/p->data=e;p->next=pc->next;pc->next=p;break;case 1:p->data=e;p->next=(*L)->next;(*L)->next=p;break;/*小于时,接在最前面*/}}return 1;}void DestroyList(EvenList *L)/*销毁表*/{ LNode *p;while(*L){p=(*L)->next;free(*L);*L=p;}}int InitQueue(LinkQueue *Q)/*初始化队列*/{ Q->front=Q->rear=(QueuePtr)malloc(sizeof(QNode)); if(!Q->front) exit(0);(Q->front)->next=NULL;return 1;}int DestroyQueue(LinkQueue *Q)/*销毁队列*/{ while(Q->front){Q->rear=Q->front->next;free(Q->front);Q->front=Q->rear;}return 1;}int EnQueue(LinkQueue *Q,QElemType e)/*插在队最后*/{ QueuePtr p;if((p=(QueuePtr)malloc(sizeof(QNode)))== NULL)exit(0);p->elem=e;p->next=NULL;(Q->rear)->next=p;Q->rear=p;/*重新设置队尾*/return 1;}int QueueEmpty(LinkQueue Q){if(Q.front==Q.rear) return 1;else return 0;}int DelQueue(LinkQueue *Q,QElemType *e)/*删除队的第一个元素*/ { QueuePtr p;if(QueueEmpty(*Q)) return 0;p=(Q->front)->next;*e=p->elem;(Q->front)->next=p->next;if(Q->rear==p) Q->rear=Q->front;free(p);return 1;}void GetHead(LinkQueue Q,QElemType *a){ QNode *p;if(Q.front==Q.rear) exit(0);p=(Q.front)->next;*a=p->elem;}int QueueLength(LinkQueue Q)/*队的长度*/{ int i=0;QNode *pc;if(Q.front==Q.rear) return 0;pc=Q.front;while(pc->next){i++;pc=pc->next;}return i;}int Mininum(LinkQueue *Q)/*求长度最短的队*/{ int a[4],e,j,i;for(i=1;i<=4;i++)a[i-1]=QueueLength(Q[i]);e=a[0];j=1;for(i=1;i<=3;i++)if(e>a[i]){e=a[i];j=i+1;}return j;}void OpenForDay()/*初始化操作*/{ int i;TotalTime=0;CustomerNum=0;/*初始化累计时间和客户数*/InitList(&ev);en.OccurTime=0;en.NType=0;/*设定第一个客户到达事件*/OrderInsert(&ev,en,CmpTime);/*把它插入事件表*/for(i=1;i<=4;i++)InitQueue(&q[i]);/*置空队列*/}void RandomTime(int *a,int *b)/*生成随机数,a为每个客户办理时间在30分钟内,b 为两相隔客户到达的间隔时间不超过5分钟*/{*a=0+rand()%30;*b=0+rand()%5;}void CustomerArrived()/*处理客户到达事件*/{ int durtime,intertime,t,i,b;++CustomerNum;/*记录客户数*/RandomTime(&durtime,&intertime);b=en.OccurTime;t=en.OccurTime+intertime;/*下一客户到达时刻*/if(t<CloseTime){ en.OccurTime=t;en.NType=0;OrderInsert(&ev,en,CmpTime);}i=Mininum(q);/*求队列最短*/customer.ArrivalTime=b;customer.Duration=durtime;/*为要插入队的客户设置到达时间和办理所需时间*/EnQueue(&q[i],customer);if(QueueLength(q[i])==1){en.OccurTime=b+durtime;en.NType=i;OrderInsert(&ev,en,CmpTime);/*设定第i 个离开事件并插入事件表*/ }}void CustomerDeparture()/*处理客户离开事件*/{ int i;i=en.NType;DelQueue(&q[i],&customer);/*删除第i队列的排头客户*/TotalTime+=en.OccurTime-customer.ArrivalTime;/*累计客户逗留时间*/if(!QueueEmpty(q[i]))/*设定第i队列的一个将要离开事件并插入事件表*/ {GetHead(q[i],&customer);/*得到它的资料*/en.OccurTime+=customer.Duration;en.NType=i;OrderInsert(&ev,en,CmpTime);}}void Bank_Simulation(){ OpenForDay();/*初始化*/while(!ListEmpty(*ev))/*非空时,删掉表里的第一个*/{DeHead(&ev,&en);if(en.NType==0)CustomerArrived();/*是客户还没办理的,就处理到达事件*/elseCustomerDeparture();/*否则处理离开事件*/}printf("The Average Time is %.3f\n\n",(float)TotalTime/CustomerNum);}void main(){ clrscr();puts("***************************************");puts("* This is a bank simulation program *");puts("***************************************");do{puts("please input the closetime of the bank:");scanf("%d",&CloseTime);/*输入关门时间*/Bank_Simulation();}while(CloseTime>=0);getch();}。
数据结构C语言实现之银行业务模拟
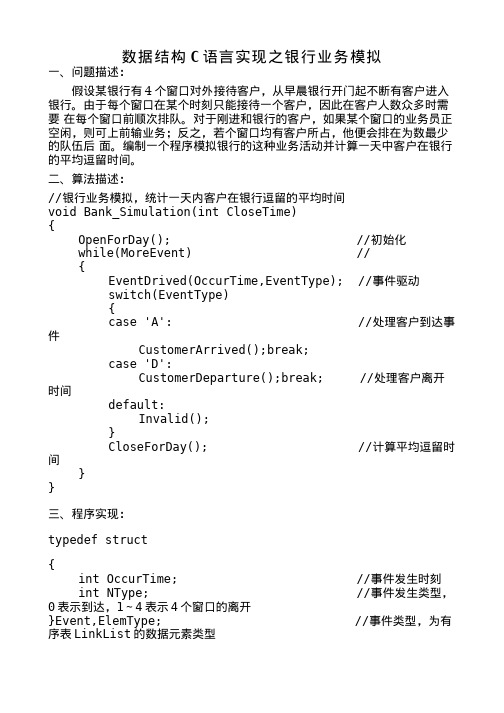
} return i;
}
void OpenForDay()
{
// 初始化操作
TotalTime = 0; CustomerNum = 0; // 初始化累计时间和客户数
}
数据结构 C 语言实现之银行业务模拟
一、问题描述:
假设某银行有 4 个窗口对外接待客户,从早晨银行开门起不断有客户进入 银行。由于每个窗口在某个时刻只能接待一个客户,因此在客户人数众多时需 要 在每个窗口前顺次排队。对于刚进和银行的客户,如果某个窗口的业务员正 空闲,则可上前输业务;反之,若个窗口均有客户所占,他便会排在为数最少 的队伍后 面。编制一个程序模拟银行的这种业务活动并计算一天中客户在银行 的平均逗留时间。
} // OpenForDay
void CustomerArrived()
{
// 处理客户到达事件,en.NType=0
int durtime, intertime, i, t;
++CustomerNum;
printf("Customer %d arrived at %d and ", CustomerNum,
typedef struct
{ int OccurTime; int NType;
0 表示到达,1~4 表示 4 个窗口的离开 }Event,ElemType; 序表 LinkList 的数据元素类型
//事件发生时刻 //事件发生类型, //事件类型,为有
typedef LinkList EventList;
C语言实现模拟银行系统
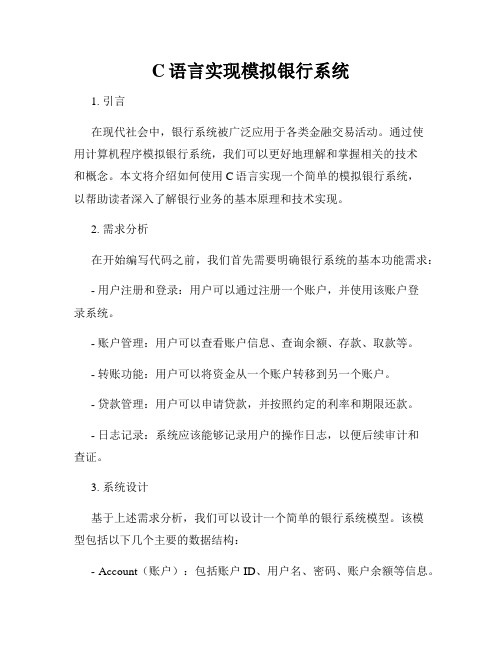
C语言实现模拟银行系统1. 引言在现代社会中,银行系统被广泛应用于各类金融交易活动。
通过使用计算机程序模拟银行系统,我们可以更好地理解和掌握相关的技术和概念。
本文将介绍如何使用C语言实现一个简单的模拟银行系统,以帮助读者深入了解银行业务的基本原理和技术实现。
2. 需求分析在开始编写代码之前,我们首先需要明确银行系统的基本功能需求:- 用户注册和登录:用户可以通过注册一个账户,并使用该账户登录系统。
- 账户管理:用户可以查看账户信息、查询余额、存款、取款等。
- 转账功能:用户可以将资金从一个账户转移到另一个账户。
- 贷款管理:用户可以申请贷款,并按照约定的利率和期限还款。
- 日志记录:系统应该能够记录用户的操作日志,以便后续审计和查证。
3. 系统设计基于上述需求分析,我们可以设计一个简单的银行系统模型。
该模型包括以下几个主要的数据结构:- Account(账户):包括账户ID、用户名、密码、账户余额等信息。
- Transaction(交易):包括交易ID、交易类型、交易金额、交易时间等信息。
除此之外,我们还需要定义一些功能函数,如账户管理、转账、贷款管理等。
这些功能函数将与主程序相互调用,实现整个模拟银行系统。
4. 系统实现在C语言中,我们可以使用结构体来定义账户和交易的数据结构,并使用数组来存储多个账户和交易记录。
以下为一个简单的示例:```c#include <stdio.h>#include <stdlib.h>#include <string.h>// 定义账户结构体struct Account {int accountId;char username[50];char password[50];float balance;};// 定义交易结构体struct Transaction {int transactionId;int accountId;char transactionType[50];float transactionAmount;char transactionTime[50];};// 声明全局变量和相关函数struct Account accountList[100]; // 最多100个账户struct Transaction transactionList[1000]; // 最多1000条交易记录int accountCount = 0; // 初始账户数量为0int transactionCount = 0; // 初始交易数量为0// 用户注册函数void userRegistration() {// TODO: 实现用户注册逻辑}// 用户登录函数void userLogin() {// TODO: 实现用户登录逻辑}// 账户管理函数void accountManagement() {// TODO: 实现账户管理逻辑}// 转账函数void transferMoney() {// TODO: 实现转账逻辑}// 贷款管理函数void loanManagement() {// TODO: 实现贷款管理逻辑}// 主函数int main() {int option;while (1) {// 打印菜单选项printf("\n==============================\n"); printf("欢迎使用模拟银行系统\n");printf("请选择以下操作:\n");printf("1. 用户注册\n");printf("2. 用户登录\n");printf("3. 账户管理\n");printf("4. 转账\n");printf("5. 贷款管理\n");printf("0. 退出系统\n");printf("==============================\n"); printf("请输入操作选项:");scanf("%d", &option);switch (option) {case 1:userRegistration();break;case 2:userLogin();break;case 3:accountManagement();break;case 4:transferMoney();break;case 5:loanManagement();break;case 0:exit(0);default:printf("无效的选项,请重新选择。
银行业务模拟数据结构课设C语言版

题目:银行业务模拟(难度系数:1.3)[运行环境]VS[问题描述]客户业务分为两种。
第一种是申请从银行得到一笔资金,即取款或借款。
第二种是向银行投入一笔资金,即存款或还款。
银行有两个服务窗口,相应地有两个队列。
客户到达银行后先排第一个队。
处理每个客户业务时,如果属于第一种,且申请额超出银行现存资金总额而得不到满足,则立刻排入第二个队等候,直至满足时才离开银行;否则业务处理完后立刻离开银行。
每接待完一个第二种业务的客户,则顺序检查和处理(如果可能)第二个队列中的客户,对能满足的申请者予以满足,不能满足者重新排到第二个队列的队尾。
注意,在此检查过程中,一旦银行资金总额少于或等于刚才第一个队列中最后一个客户(第二种业务)被接待之前的数额,或者本次已将第二个队列检查或处理了一遍,就停止检查(因为此时已不可能还有能满足者)转而继续接待第一个队列的客户。
任何时刻都只开一个窗口。
假设检查不需要时间。
营业时间结束时所有客户立即离开银行。
写一个上述银行业务的事件驱动模拟系统,通过模拟方法求出客户在银行内逗留的平均时间。
[基本要求]利用动态存储结构实现模拟,即利用C语言的动态分配函数malloc和free。
[选做内容]自己实现动态数据类型。
(已作)1. 需求分析计算来银行办理业务的用户平均逗留时间:⑴ 在运行终端控制台进行信息输入:输入信息包括(信息都为整数):1.银行拥有的款额取值范围:[0,正无穷]2.银行营业时间取值范围:[0, 1440]3.用户最大交易金额取值范围:(0,银行拥有款额]4.俩个用户到达时间的最大值取值范围:(0,银行营业时间]5.用户最大交易时间取值范围:(0,银行营业时间]⑵ 在运行终端控制台进行信息输出:1.每个到达用户的到达时间,编号,要处理的金额,处理时间2.事件表3.总用户数4.总逗留时间5.平均逗留时间⑶ 程序所能达到的功能;1.输出每个用户的到达时间,编号,要处理的金额,处理时间2.银行运营过程的全部事件(包括到达事件和离开事件)3.计算银行运营过程的用户数,总逗留时间,平均逗留时间 ⑷ 三组测试数据:1 .正常情况:1.银行拥有的款额:10000 2 .银行营业时间: 600 3 .用户最大交易金额:20004 .俩个用户到达时间的最大值:205 .用户最大交易时间:15应输出:25.486 .极端的情况:两个到达事件之间的间隔时间很短,而客户的交易时间很长 1.银行拥有的款额:100007 .银行营业时间: 6008 .用户最大交易金额:20009 .俩个用户到达时间的最大值:5 10 用户最大交易时间:100应输出:20.011 极端的情况:两个到达事件之间的间隔时间很长,而客户的交易时间很短 1.银行拥有的款额:10000 2.银行营业时间: 600 3.用户最大交易金额:2000 12 俩个用户到达时间的最大值:100 13 用户最大交易时间:5应输出:88.38源代码如下:#include <stdio.h> #include <stdlib.h> #include <time.h>#include<Windows.h> typedef int Status; #define OK 1#define ERROR -1//定义银行运作时间(单位:分钟假设一分 //定义最大时间间隔 //定义最大操作时间 */typedef struct kehu_event{// 定义客户信息int handel_time; //事件处理时间 int money; //需要处理的钱数目(负数表示取钱,正数表示存钱) int No; //客户的序号}ElemType;typedef struct event{/*#define MAXTIME 600 钟等于一秒)#define MAX_TIMEJIANGE 5 #define MAX_TIMEJIAOYI/*定义事件类型*/int index; /*标志变量,1代表到达事件,其余代表离开事件*/int time; /*事件发生时间*/int No; /*触发该事件的客户序号*/}event;typedef struct { /*定义链表节点*/event data;struct LNode *next;}LNode, *Link;typedef struct { /*定义链表*/ Link head, tail;int len;}*LinkList;LinkList InitLink() { /*初始化链表操作*/LinkList L = (LinkList*)malloc(sizeof(LNode));if (L == NULL) return NULL;L->head = NULL;L->tail = NULL;L->len = 0;printf("链表初始化成功!!”); return L;};Status ClearLink(LinkList L) { /*链表的清空操作*/ if (L == NULL) return ERROR;Link p = L->head;Link k = L->head->next;while (p != L->tail) {free(p);p = k;if(k!=L->tail) k = k->next;}free(p);L->head = NULL;L->tail = NULL;L->len = 0;printf("链表已经清空!!"); return OK;}Status DestoryLink(LinkList L) { /*链表的销毁操作*/ if (L == NULL) return ERROR;ClearLink(L);free(L);printf("链表销毁完毕!!");return OK;Status InsertLink(LinkList L, event e) { /*链表的尾部插入操作*/if (L == NULL) return ERROR;Link p = (Link)malloc(sizeof(LNode));if (p == NULL) return ERROR;p->data = e;p->next = NULL;if (L->head == NULL) {L->head = p;L->tail = p;L->len = 1;}else{L->tail->next = p;L->tail = p; L->len++;}〃printf("节点插入成功!!"); return OK;}Status LinkTraverse(LinkList L) { /*链表的遍历操作*/ if (L == NULL) return ERROR;Link p = L->head;printf("\n 遍历结果:\n");printf(" 客户序号:事件触发时间:事件类型:");printf("\n ---------------------------------------------------------- ");while (p != NULL) {printf("\n %d %d", p->data.No, p->data. time);if (p->data.index == 1) {printf(" 到达");printf("\n ------------------------------------------- --------------- ");} else {printf(" 离开");printf("\n ------------------------------------------- --------------- ");}p = p->next;}return OK;typedef struct LQNode{ ElemType data;struct LQNode*next;}LQNode, *QueuePtr;/*定义队列的节点*/typedef struct {QueuePtr front;QueuePtr rear;int len;}LQueue;/*定义队列*/LQueue* InitQueue() { /*构造一个空队列*/ LQueue *Q = (LQueue*)malloc(sizeof(LQueue)); if (Q == NULL)return ERROR;Q->front = NULL;Q->rear = NULL;Q->len = 0;return Q;Status EnQueue(LQueue *Q, ElemType e) { 入eLQNode *p;p =(LQNode*)malloc(sizeof(LQNode));if (p == NULL) return ERROR;p->data = e;p->next = NULL;if ( NULL == Q->front) {Q->front = p;Q->rear = p;Q->len = 1;}else{Q->rear->next = p;Q->rear = p;Q->len++;}return OK;} //在队列的队尾插Status DeQueue(LQueue *Q, ElemType *e) { 〃是队列Q的队头出队并且返回到eLQNode *p;if (NULL == Q->front) returnERROR;p = Q->front;*e = p->data;Q->front = p->next;if (Q->rear == p) Q->rear = NULL;free(p);Q->len--;return OK;}Status DestoryQueue(LQueue *Q) { /*队列的销毁操作*/if (Q == NULL) return ERROR;QueuePtr p=NULL, q=NULL;p = Q->front;while (p != Q->rear) { q = p->next; myfree(p);p = q;}free(Q->rear);return OK;}Status InsertQueue(LQueue *Q, LQueue *E) {/*将E队列插入到Q队列的前面,并将Q队列作为新的队列释放E*/ if ( E == NULL || E->front == NULL) return ERROR;if (Q == NULL || Q->front == NULL || Q->rear == NULL) { Q->front = E->front;Q->rear = E->rear;}else{E->rear->next = Q->front;Q->front = E->front;}return OK;/* ----------------------------------- 定义需要使用的全局变量 -------------- ---------------- */int total ;银行拥有的款额为10000(元),并且默认取钱只能*/ //int total_time = 600;(分钟)*/int max_money;LQueue *handel_queue = NULL;LQueue *wait_queue = NULL;等候的客户*/LinkList event_link = NULL;/*一天营业开始时/*营业时间为600/*定义第一个队列用于处理业务*//* 定义第二个队列用于存储ElemType* mymalloc( int max_timejiaoyi) { /*动态申请客户节点*/ ElemType *e;e = (ElemType*)malloc(sizeof(ElemType)); if (e == NULL) return ;e->handel_time = (rand() % max_timejiaoyi) + 1;e->money = (rand() % (2 * max_money)) - max_money; e->No = kehu_NO; kehu_NO++; return e; }Status myfree(ElemType *e) {/*动态释放客户节点*/if (e == NULL) return ERROR; free(e); return OK;} /*Status suiji_kehu(ElemType *e,int max_timejiaoyi) { /*随机生成客户信息mymalloc(e, max_timejiaoyi); return OK; } */ElemType* Arrial_event(int i, int max_timejiange, int max_timejiaoyi) { /*用来处理新客户到达事件*/ if (nextTime == i) {printf("当前时间是:%d\n", i);nextTime = nextTime + (rand() % max_timejiange) + 1;//随机生成下一名客户到达时间,这里可以设置客户到达时间间隔的大小ElemType *p=NULL;mymalloc(max_timejiaoyi);//到达前面设置的到达时间时,随机生成刚到达用户的信息,包括客户编号,业务处理时间,业务操作钱的数目int nextTime = 0;为下一个客户的随机到达时间*/int nexthandelTime = 0;为正在排队的下一个客户处理时间*/int kehu_NO = 1; /ElemType *leave_kehu = NULL; 变量,用于记录*/ElemType *search_kehu = NULL; 户变量*//* ------------------------- -------------------- *//*定义一个变量作 /*定义一个变量作 /*客户的服务序号*/*定义一个办理完业务要离开的客户 /*定义一个用来存储待处理业务的客event *e = (event*)malloc(sizeof(event)); //生成该到达事件的信息节点e->index = 1;e->No = p->No;e->time = i;InsertLink(event_link, *e);//将该到达事件的信息节点加入事件链表if (nexthandelTime < i) {//如果当前时间已经超过了下一个用户操作时间,则将当前时间和该到达用户的时间相加作为下一个用户操作时间if (-(p->money) <= total) {nexthandelTime = p->handel_time + i;total = total + p->money;EnQueue(handel_queue, *p);}else {EnQueue(wait_queue, *p);}}else {if (nexthandelTime == 0) {//第一个进入操作的用户情况if (-(p->money) <= total) {nexthandelTime = p->handel_time + i;total = total + p->money;EnQueue(handel_queue, *p);}else { EnQueue(wait_queue, *p);}}else EnQueue(handel_qu eue, *p);}if (nexthandelTime == 0 ) {//第一个进入操作的用户情况if (-(p->money) <= total) {nexthandelTime = p->handel_time + i;total = total + p->money;EnQueue(handel_queue, *p);}else { EnQueue(wait_queue, *p);}}printf("\n客户编号:%d客户操作需要时间:%d客户需要处理的钱:%d 银行余额:%d\n\n\n”, p->No, p->handel_time, p->money, total);return p;}}Status handel_event(int i) {/*处理队列事件*/if (nexthandelTime == i) { /*到达下一个客户操作时间时*/DeQueue(handel_queue, leave_kehu); // 将处理完的客户出队,并将客户信息存储在leave_kehu变量中int last_money = total - leave_kehu->money; //获取刚离开的客户操作前银行的钱数目Leave_event(leave_kehu, i);//将离开事件的用户信息存入事件列表中if (((leave_kehu->money) > 0) && wait_queue->front != NULL) {/*如果刚刚离开的用户是存钱或者还钱,并且等待队列不为空*/int index = 0;//设置标记变量,用以标记等待队列已经查看的客户数目LQueue *e = InitQueue();//创造出一个可以被满足需求的等待用户组成的队列int linshi_total = total;//设置一个临时金额存储空间while (linshi_total > last_money &&wait_queue->front != NULL) { //如果当前银行拥有的款额比之前多,进入循环DeQueue(wait_queue, search_kehu); // 将等待队列的队头用户出队if (-(search_kehu->money) <= linshi_total) { /*如果等待用户的需求可以得到满足*/EnQueue(e, *search_kehu);//将可满足用户加入等待用户队列linshi_total = linshi_total + search_keh u->money;}else { EnQueue(wait_queue, *search_kehu);}index++;if (index >= wait_queue->len) break;}InsertQueue(handel_queue, e); // 将可以满足要求的等待队列并入前面//nexthandelTime = nexthandelTime +handel_queue->front->data.handel_time; //下一个处理用户时间更新if (handel_queue->front != NULL) { /*如果操作队列不为空*/while(handel_queue->front != NULL && -(handel_ queue->front->data.money) > total)/*如果无法满足要求*/{DeQueue(handel_queue, leave_kehu);EnQueue(wait_queue, *leave_kehu);}if (handel_queue->front != NULL) {nexthandelTime =handel_queue->front->data.handel_time + nexthandelTime; //下一位操作客户时间更新total = total +handel_queue->front->data.money; //银行金钱总额更新}}}else {/*刚刚离开的用户是取钱或者借钱,或者等待队列为空*/if (handel_queue->front != NULL) {while (handel_queue->front != NULL && -(handel _queue->front->data.money) > total ){/*无法满足的用户进入等待队列*/DeQueue(handel_queue, leave_kehu);EnQueue(wait_queue, *leave_kehu);}if (handel_queue->front != NULL) {/*处理队列不为空*/nexthandelTime = handel_queue->front->da ta.handel_time + nexthandelTime;total = total + handel_queue->front->dat a.money;}}}}}Status Leave_event(ElemType *e ,int i) {/*用来处理客户离开事件*/event *p = (event*)malloc(sizeof(event));p->index = 0;p->No = e->No;p->time = i;InsertLink(event_link, *p); return OK;}double average_StayTime(LinkList e,double final) { /*通过事件列表来计算用户平均逗留时间*/ double sum=0, a, b;int shumu=0;LNode *p = e->head;for (int i = 1; i <= e->len; i++) {while(p!=NULL) {if (p->data.No == i) {if (p->data.index == 1) {a = p->data.time;shumu = shumu + 1;}if (p->data.index == 0) b = p->data.time;} p = p->next;}p = e->head;if (b == -1.0) b = final;sum = sum + b - a;b = -1.0;}printf("全部用户数:%d \n总共消耗时间:%f \n”, shumu, sum);printf ("客户平均逗留时间:%f分",sum / shumu);return sum / shumu;}int main(void) {leave_kehu = (ElemType*)malloc(sizeof(ElemType));search_kehu = (ElemType*)malloc(sizeof(ElemType));handel_queue = InitQueue();wait_queue = InitQueue();event_link = InitLink();srand((unsigned int)time(NULL));int MAXTIME, MAX_TIMEJIANGE, MAX_TIMEJIAOYI;printf("请输入银行的运营时间(单位:分钟):");scanf_s("%d", &MAXTIME);printf("请输入银行的运营金额(单位:元):");scanf_s("%d", &total);printf("请输入用户最大交易金额(单位:元):");scanf_s("%d", &max_money);printf("请输入俩个到达事件的最大时间间隔:(单位:分钟):");scanf_s("%d", &MAX_TIMEJIANGE);printf("请输入用户最大交易时间:(单位:分钟):");scanf_s("%d", &MAX_TIMEJIAOYI);//ElemType *newKehu = (ElemType*)malloc(sizeof(ElemType));for (int i = 0; i < MAXTIME; i++) {Arrial_event(i, MAX_TIMEJIANGE, MAX_TIMEJIAOYI);handel_event(i);Sleep(50);}LinkTraverse(event_link);printf("\n");average_StayTime(event_link, MAXTIME-1);DestoryQueue(handel_queue);DestoryQueue(wait_queue);system("pause");return 1;}。
数据结构C语言版_银行业务模拟
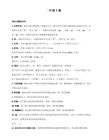
Event et; // 临时变量
LinkQueue q[Qu]; // Qu个客户队列
QElemType customer; // 客户记录
int CloseTime; // 银行营业时间(单位是分)
int TotalTime = 0; // 累计客户逗留时间初始为0
{
QueuePtr p;
p = Q.front->next;
while( p )
{
vi(p->data);
p = p->next;
}
printf("\n");
return 1;
}
// 依事件a的发生时刻<、=或>事件b的发生时刻分别返回-1、0或1。
int DestroyQueue(LinkQueue *Q)
{
while((*Q).front)
{
(*Q).rear = (*Q).front->next;
free((*Q).front);
(*Q).front = (*Q).rear;
}
return 1;
}
int CustomerNum = 0; // 客户数(初值为0)
// 释放p所指结点
void FreeNode(Link *p)
{
free(*p);
*p = NULL;
}
// 构造一个空的线性链表。
int InitList(LinkList *L)
{
Link p;
int i = 0;
QueuePtr p;
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
Event *p;
Event *q0;
Event *q;
p=head;p=p->next->next;q=head;q0=head;q=q->next;
while(p!=null)
{
if(q->occuitime>=p->occuitime)
{
int Lenth;
Event *p;
p=head;
p=p->next;
Lenth=GetEventLenth(head);
if(Lenth>0)
{
while(p!=null)
{
printf("事件发生事件:%d,事件类型:",p->occuitime);
if(Lenth==0)
head->next=q;
else
{
q->next=head->next;
head->next=q;
sortEnvetList(head);
}
return(head);
}
void DelEventHead(Event *head)
Creat4Window(Q);
float averagetime,a,b;
Bank_Simulation(head,Q);
// printf("平均逗留时间是:%f",averagetime);
printf("\n\n*******************银行业务模拟系统***********************\n\n\n");
int occuitime;
int eventtype;
top=(Event *)malloc(sizeof(Event));
OpenForDay(head);
while(GetEventLenth(head)!=0)
{
top=EventHead(head);kkk=(top->eventtype)%10;
{
int Lenth;
Event *p;
p=head;
p=p->next;
Lenth=GetEventLenth(head);
if(Lenth>0)
head->next=(head->next)->next,free(p);
}
void PrintEventList(Event *head)
void Creat4Window(Queue *Q[5]); //4个窗口创建
int GetShortQueue(Queue *Q[5]); //得到最短的 队列
void Bank_Simulation(Event *head,Queue *Q[5]); //模拟银行业务函数
{
int time=0,arrivetime;
int a,b,t;
arrivetime=GetTOpQueue(0,*(Q+num));
t=occuitime-arrivetime;
DeQueue(*(Q+num));
TotalTime=TotalTime+t;
if(GetQueueLenth(*(Q+num))!=0)
typedef struct Event{ //事件表 类型
int occuitime;
int eventtype; //0代表到达,1代表离开
struct Event *next;
}Event;
typedef struct QueueElem{ //队列 元素 类型
printf(" CustomerNum=%d\n",CustomerNum);
printf(" TotalTime=%d\n",TotalTime);
averagetime=(float)TotalTime/CustomerNum;
printf(" 平均逗留时间为:%0.2f\n",averagetime);
num=(top->eventtype)/10;
occuitime=top->occuitime;
DelEventHead(head);
if(kkk==0)
CustomerArrived(occuitime,Q,head);
else
CustomerDeparture(occuitime,num,head,Q);
if(p->eventtype==0)
printf("客户到达\n");
else if(p->eventtype==1)
printf("客户离开\n");
p=p->next;
}
}
else
printf("事件是空的!\n");
}
void sortEnvetList(Event *head)
}Queue; // 银行 窗口 队列
int CustomerNum=0; //总时间
int TotalTime=0; //总人数
Event *createventlist(Event *head); //创建 事件列表
//次代码是,数据结构(严蔚敏 吴伟民)第3章关于银行业务模拟算法的实现,可直接拷贝到VC6.0中运行
# include <stdio.h>
# include <malloc.h>
# include <stdlib.h>
# define null 0
# define closetime 100 //营业时间,可以自定义
Event *EventHead(Event *head)
{
Event *p=null;
int Lenth;
Lenth=GetEventLenth(head);
if(Lenth>0)
p=head->next;
return(p);
}
Event *addEvent(Event *head,int occuitime,int eventtype)
{
int Lenth;
Event *p;
Event *q;
q=(Event *)malloc(sizeof(Event));
q->occuitime=occuitime;
q->eventtype=eventtype;
q->next=null;
Lenth=GetEventLenth(head);
void CustomerDeparture(int occuitime,int num,Event *head,Queue *Q[5]);
void OpenForDay(Event *head);
Queue *CreatQueue(); //队列有关操作
void EnQueue(int arrivetime,int durationtime,Queue *head);
int arrivetime;
int durationtime; // 业务 所需办理 时间
struct QueueElem *next;
}QueueElem;
typedef struct{
QueueElem *front;
QueueElem *rear;
void OpenForDay(Event *head,Queue *Q[5]); //初始化
void main()
{
Event *head;
head=createventlist(head); //将 事件 加入到 事件列表
Queue *Q[5];
{
a=GetTOpQueue(1,*(Q+num));
b=a+occuitime;
addEvent(head,b,(num*10+1));
}
}
//******************事件表的操作,即链表的操作**********************************************************
void DeQueue(Queue *head);
void PrintQueue(Queue *head);
int GetQueueLenth(Queue *head);
int GetTOpQueue(int flag,Queue *head); //falg为0代表返回arrivetime,1代表返回durationtime;
{
int i=0; // i 为事件表 的 长度
Event *p;
p=head;
p=p->next;
while(p!=null)
{
p=p->next;i++;
}
// printf("iiii=====%d\n",i);
return(i);
}
Event *createventlist(Event *head)
{
head=(Event *)malloc(sizeof(Event));
if(head)
head->next=null;
return(head);