通过实例理解继承与多态原理与优点
对java多态和继承的总结

25. }
26.
27. public int getprice() {
28. return 20000
29. }
30. }
31.
32. // 汽车出售店
33. public class carshop {
抽象类可以继承(extends)普通类,可以继承(extends)抽象类,可以继承(implements)接口。
接口只能继承(extends)接口。
请注意上面三条规律中每种继承情况下使用的不同的关键字extends和implements,它们是不可以随意替换的。大家知道,一个普通类继承一个接 口后,必须这个接口中定义的所有方法,否则就只能被定义为抽象类。我在这里之所以没有对implements关键字使用“实现”这种说法是因为从概念上来 说它也是表示一种继承关系,而且对于抽象类implements接口的情况下,它并不是一定要实现这个接口定义的任何方法,因此使用继承的说法更为合理一 些。
java代码 复制代码
1. // 桑塔纳汽车
2. class santana implements car {
3. public string getname() {
4. return " santana"
5. }
6.
7. public int getprice() {
7. // other code
8. }
9. }
10.
11. class parent {
12. public void method() {
13. // do something here
继承跟多态的区别

继承跟多态的区别在计算机语言中有一种是JAVA的语言,里面有一些方法,继承,重载,重写。
下面是店铺为你整理的继承跟多态的区别,供大家阅览!重载,继承,重写和多态的区别:继承是子类获得父类的成员,重写是继承后重新实现父类的方法。
重载是在一个类里一系列参数不同名字相同的方法。
多态则是为了避免在父类里大量重载引起代码臃肿且难于维护。
网上看到一个有趣的说法是:继承是子类使用父类的方法,而多态则是父类使用子类的方法。
下面的例子包含了这四种实现:class Triangle extends Shape {public int getSides() { //重写return 3;}}class Rectangle extends Shape {public int getSides(int i) { //重载return i;}}public class Shape {public boolean isSharp(){return true;}public int getSides(){return 0 ;}public int getSides(Triangle tri){return 3 ;}public int getSides(Rectangle rec){return 4 ;}public static void main(String[] args) {Triangle tri = new Triangle(); //继承System.out.println("Triangle is a type of sharp? " + tri.isSharp());Shape shape = new Triangle(); //多态System.out.println("My shape has " + shape.getSides() + " sides.");}}注意Triangle类的方法是重写,而Rectangle类的方法是重载。
类的继承与多态性实验报告
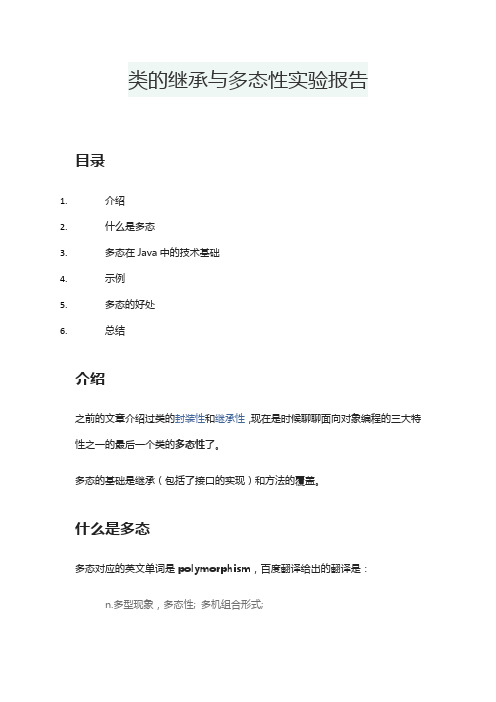
类的继承与多态性实验报告目录1.介绍2.什么是多态3.多态在Java中的技术基础4.示例5.多态的好处6.总结介绍之前的文章介绍过类的封装性和继承性,现在是时候聊聊面向对象编程的三大特性之一的最后一个类的多态性了。
多态的基础是继承(包括了接口的实现)和方法的覆盖。
什么是多态多态对应的英文单词是polymorphism,百度翻译给出的翻译是:n.多型现象,多态性; 多机组合形式;按字面意思就是多种状态、形态、姿态等等,潜台词就是某个东西具有多种状态、形态、姿态等等。
那是什么东西呢?在面向对象的编程语言里面(当然就包括Java了)就是某个方法或函数。
那方法的多种状态、形态、姿态有是个什么意思呢?这其实是指同一个方法具有多个方法体,就是方法的实现。
而方法的相同与否是由方法签名决定的。
所以,多态其实本质上是指同一个类的同一个方法却具有不同的行为特征。
状态、形态、姿态指的就是行为特征。
多态在Java中的技术基础然而,在Java中,同一个类里面是不可能存在两个签名相同而实现不同的方法的,否则的话会导致无法判断该执行哪个方法,因此在编译时就会报错。
所以,肯定是在两个类中才有可能存在两个签名相同而实现不同的方法,一个实现在这个类,另一个实现在另一个类。
而如果这两个类毫无瓜葛,那么肯定就与多态的本质(同一个类的同一个方法却具有不同的行为特征)自相矛盾了。
所以,这两个类肯定是有某种联系的。
我们再想想,什么概念是能够让两个不同的类却又能称为同一个类的?答案就是类的继承/扩展,就是现实中的“某东西是某类东西”的概念,就是“具体和抽象”的思想。
比如,男人是人,女人也是人,男人类和女人类就借助于人类建立了某种联系,而人类具有的某个行为在男人类和女人类中是有着不同体现的,比如人类的吃饭这个行为,男人类的体现是狼吞虎咽,女人类的体现是细嚼慢咽。
例子不是很恰当,但意思就是这么个意思。
所以说,Java里面多态的技术基础就是方法的覆盖,当然,在Java中覆盖不仅仅发生在类的继承/扩展上,还可能发生在接口的实现上。
继承与多态的概念及应用

继承与多态的概念及应用继承与多态是面向对象编程中两个重要的概念,它们在程序设计中起到了关键的作用。
本文将对继承与多态的概念进行详细解析,并介绍它们在实际应用中的各种用途和优势。
一、继承的定义与特点继承是面向对象编程中一种重要的概念,它是指一个类(称为子类或派生类)可以继承另一个类(称为父类或基类)的特征和行为。
子类可以继承父类的属性和方法,同时也可以在继承的基础上进行扩展和修改。
继承的特点主要有以下几点:1. 单继承:一个类只能继承一个父类,但是可以通过多层继承形成继承链。
2. 父类与子类之间的关系:子类继承了父类的特征和行为,可以直接使用父类的属性和方法。
3. 子类的扩展与修改:子类可以在继承父类的基础上进行扩展和修改,添加自己的属性和方法。
二、继承的实际应用继承在实际应用中有着广泛的用途,它可以提高代码的可重用性,减少重复编写相同的代码,并且使程序结构更加清晰。
以下是几个继承的实际应用场景:1. 标准类库中的继承标准类库中的很多类都使用了继承的概念,通过继承可以实现代码的复用,同时也可以更好地组织和管理代码。
以Java的标准类库为例,其中的各个类都是通过继承来实现的,如ArrayList继承自AbstractList,HashSet继承自AbstractSet等。
2. UI界面的继承在UI界面设计中,经常使用继承来实现不同UI组件之间的共性和差异。
例如,一个基础的Button类可以作为其他更具体的按钮类的父类,这些子类可以继承父类的属性和方法,同时也可以根据自身的需求进行扩展和修改。
3. 游戏开发中的继承在游戏开发中,继承也是一个常用的技巧。
通过继承,可以实现游戏中不同角色、怪物、道具等的共性和差异。
例如,一个基础的角色类可以定义所有角色都共有的属性和方法,然后不同的角色类可以继承这个基础类,并在此基础上添加自己独特的属性和方法。
三、多态的定义与特点多态是指同一个方法或操作具有多种不同的实现方式,通过多态可以提高代码的灵活性和扩展性。
python继承定义多态的实验体会

python继承定义多态的实验体会Python继承定义多态的实验体会一、Python继承的定义Python是一种面向对象编程语言,支持继承机制。
继承是指一个类可以从另一个类中继承属性和方法。
被继承的类称为父类或基类,继承的类称为子类或派生类。
子类可以使用父类中已有的属性和方法,也可以添加自己的属性和方法。
二、Python多态的定义多态是指不同对象对同一消息作出不同响应的能力。
在面向对象编程中,多态性是指一个接口(方法)具有多个实现方式(即多个子类对该接口进行了实现),调用该接口时会根据上下文自动选择合适的实现方式。
三、Python继承与多态的关系Python中继承与多态密切相关。
通过继承机制,可以将相同或相似功能封装到父类中,然后让不同的子类来重写这些方法以实现自己特定的功能。
这样就可以使用父类类型来引用不同子类对象,并且调用相同名称的方法时会根据上下文自动选择合适的实现方式,从而实现了多态性。
四、Python继承与多态实验体会1. 继承示例代码:class Animal:def __init__(self, name): = namedef speak(self):passclass Dog(Animal):def speak(self):return "woof"class Cat(Animal):def speak(self):return "meow"dog = Dog("Rufus")cat = Cat("Whiskers")print(dog.speak()) # 输出:woofprint(cat.speak()) # 输出:meow在上面的代码中,Animal类是父类,Dog和Cat是子类。
子类继承了父类中的name属性和speak方法,并且重写了speak方法以实现自己特定的功能。
在主程序中,使用父类类型来引用不同子类对象,并且调用相同名称的方法时会根据上下文自动选择合适的实现方式,从而实现了多态性。
面向对象设计中的继承与多态原则应用分析

面向对象设计中的继承与多态原则应用分析在面向对象的软件设计中,继承与多态是两个重要的原则,它们能够使软件系统更加灵活、可扩展和易维护。
本文将对继承与多态原则的应用进行分析,并探讨其在软件设计中的作用和优点。
一、继承原则的应用分析继承是一种面向对象的编程概念,通过继承可以让一个类从另一个类中继承属性和方法。
在继承关系中,有一个父类(也称为基类或超类)和一个或多个子类(也称为派生类)。
1. 实现代码重用继承允许子类继承父类的属性和方法,从而实现代码重用。
当多个类具有相似的属性和功能时,可以将这些共同的部分抽象为一个父类,子类可以继承父类并增加自己独有的功能。
这样可以减少代码的冗余,提高代码的可维护性和可扩展性。
2. 实现抽象和多层次结构通过继承可以实现抽象和多层次结构。
父类可以只定义一些共同的属性和方法,子类可以根据具体的需求实现自己的功能。
这样可以使程序更加灵活,根据不同的需求扩展功能。
3. 实现可替换性和多态继承还可以实现对象的可替换性和多态。
在面向对象的编程中,通过父类引用指向子类对象的实例,实现对不同子类的替换,从而提供了程序的可扩展性和灵活性。
这种能力被称为多态。
4. 要注意避免过度使用继承虽然继承有很多优点,但过度使用继承可能导致类之间的耦合度增加,使代码变得复杂和难以维护。
因此,在设计中应该遵循单一职责原则,尽量减少继承的层次,避免出现过于复杂的继承关系。
二、多态原则的应用分析多态是面向对象编程中的一个重要原则,它允许对象在不同的情况下表现出不同的行为,提高了代码的灵活性和可扩展性。
1. 实现接口的统一多态可以通过接口的方式实现,让不同的类实现相同的接口或继承相同的抽象类,从而实现接口的统一。
这样可以使代码具有更好的可读性和可维护性,能够更方便地替换不同的子类。
2. 提高程序的可扩展性多态提供了程序的可扩展性,当需要添加新的功能时,只需要添加新的子类并实现相应的接口或抽象类,而不需要修改已有的代码。
面向对象程序设计中的继承和多态

面向对象程序设计中的继承和多态面向对象程序设计是一种高级的编程思想,它将现实世界中的概念映射到程序设计中,使它们更易于理解和操作。
继承和多态是面向对象程序设计中的两个核心概念,它们可以使代码更加灵活、可重用性更强。
本文将详细解释继承和多态的概念、用途和示例。
继承继承是面向对象程序设计中的一种特性,它允许开发人员通过重用已有的代码来创建新的程序。
当一个类继承自另一个类时,它将获得父类的属性和方法,同时可以添加和修改自己的功能。
例如,我们可以创建一个“动物”类,其中包含了一些所有动物都共有的属性和方法,如年龄、性别、音量和吃饭。
然后,我们可以创建更具体的类,如“狗”和“猫”,它们都继承自“动物”类,并具有自己的属性和方法。
例如,狗可以有品种、皮毛和学会新技能等属性和方法,而猫则可以有爪子长度、睡觉的时间和舔毛器等属性和方法。
继承可以使代码更简单、易于维护,因为我们不需要为每个新类编写相同的属性和方法,而是可以重用现有的代码。
这也有助于重用已有的测试用例,因为继承子类将自动通过从父类继承的测试用例。
多态多态是面向对象程序设计中的另一个核心概念。
它允许不同的对象对相同的消息做出不同的响应。
这是通过继承实现的,让子类对其继承的父类进行重新实现,从而创建一个新的对象类型。
多态也称为“动态绑定”或“运行时多态性”。
例如,如果我们有一个“动物”类,它有一个“发出声音”的方法,狗和猫类都继承自“动物”类,并重写了“发出声音”的方法,在这两个子类里,它们发出声音的方式是不同的。
现在,我们创建了一个“动物”列表,其中包含狗和猫的实例。
当我们通过“发出声音”的方法调用它们时,它们将响应自己的声音,而不管是哪个类的实例。
多态的好处是,它可以使代码更灵活和可扩展。
我们可以写一个方法来处理多个不同的对象,而不必知道每个对象的确切类型。
这样也可以简化代码,因为我们不需要为每个对象类型写出不同的处理逻辑。
继承和多态的示例继承和多态可以用几个实际的编程示例来解释。
多态的原理
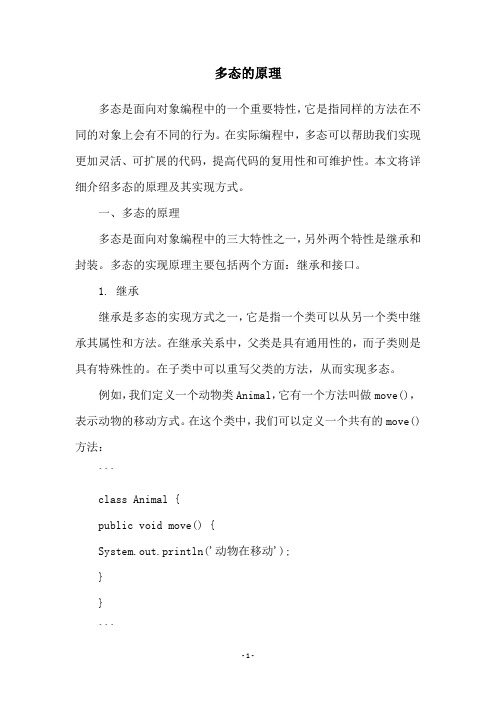
多态的原理多态是面向对象编程中的一个重要特性,它是指同样的方法在不同的对象上会有不同的行为。
在实际编程中,多态可以帮助我们实现更加灵活、可扩展的代码,提高代码的复用性和可维护性。
本文将详细介绍多态的原理及其实现方式。
一、多态的原理多态是面向对象编程中的三大特性之一,另外两个特性是继承和封装。
多态的实现原理主要包括两个方面:继承和接口。
1. 继承继承是多态的实现方式之一,它是指一个类可以从另一个类中继承其属性和方法。
在继承关系中,父类是具有通用性的,而子类则是具有特殊性的。
在子类中可以重写父类的方法,从而实现多态。
例如,我们定义一个动物类Animal,它有一个方法叫做move(),表示动物的移动方式。
在这个类中,我们可以定义一个共有的move()方法:```class Animal {public void move() {System.out.println('动物在移动');}}```现在我们再定义一个子类叫做Dog,它继承了Animal类,并且重写了move()方法,表示狗的移动方式:```class Dog extends Animal {@Overridepublic void move() {System.out.println('狗在跑');}}```在这个例子中,我们通过继承的方式实现了多态。
当我们调用move()方法时,如果是Animal类型的对象,它会调用Animal类中的move()方法;如果是Dog类型的对象,它会调用Dog类中重写的move()方法。
这就是多态的实现原理。
2. 接口除了继承之外,接口也是多态的实现方式之一。
接口是一种抽象的数据类型,它定义了一组方法的签名,但是没有实现这些方法的具体内容。
在接口中定义的方法可以被多个类实现,从而实现多态。
例如,我们定义一个接口叫做Shape,它有一个方法叫做draw(),表示绘制图形的方式。
在这个接口中,我们可以定义一个共有的draw()方法:```interface Shape {void draw();}```现在我们再定义两个类叫做Circle和Rectangle,它们都实现了Shape接口,并且实现了draw()方法,分别表示绘制圆形和矩形的方式:```class Circle implements Shape {@Overridepublic void draw() {System.out.println('绘制圆形');}}class Rectangle implements Shape {@Overridepublic void draw() {System.out.println('绘制矩形');}}```在这个例子中,我们通过接口的方式实现了多态。
继承和多态在面向对象中的作用和优势
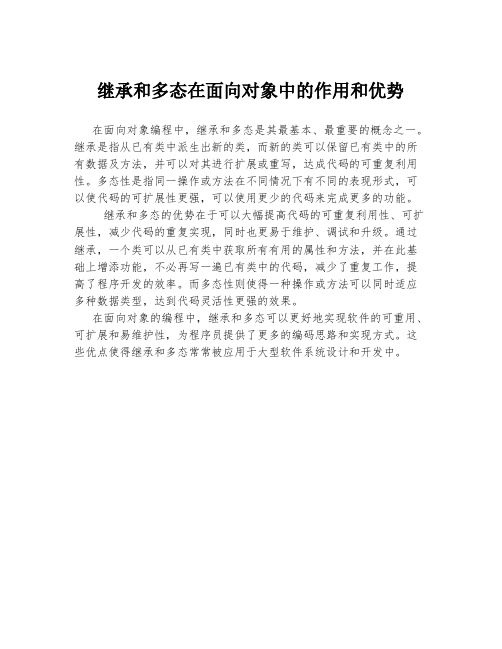
继承和多态在面向对象中的作用和优势
在面向对象编程中,继承和多态是其最基本、最重要的概念之一。
继承是指从已有类中派生出新的类,而新的类可以保留已有类中的所有数据及方法,并可以对其进行扩展或重写,达成代码的可重复利用性。
多态性是指同一操作或方法在不同情况下有不同的表现形式,可以使代码的可扩展性更强,可以使用更少的代码来完成更多的功能。
继承和多态的优势在于可以大幅提高代码的可重复利用性、可扩展性,减少代码的重复实现,同时也更易于维护、调试和升级。
通过继承,一个类可以从已有类中获取所有有用的属性和方法,并在此基础上增添功能,不必再写一遍已有类中的代码,减少了重复工作,提高了程序开发的效率。
而多态性则使得一种操作或方法可以同时适应多种数据类型,达到代码灵活性更强的效果。
在面向对象的编程中,继承和多态可以更好地实现软件的可重用、可扩展和易维护性,为程序员提供了更多的编码思路和实现方式。
这些优点使得继承和多态常常被应用于大型软件系统设计和开发中。
Python继承和多态的概念和实现

Python继承和多态的概念和实现继承和多态是面向对象编程中的两个重要概念,在Python中也得到了充分的支持和应用。
本文将介绍Python中继承和多态的概念,并通过相应的代码示例来展示其实现方法。
一、继承的概念和实现继承是指一个类(称为子类或派生类)从另一个类(称为父类或基类)中继承属性和方法的过程。
子类可以继承父类的属性和方法,并可以添加自己特有的属性和方法。
在Python中,继承可以通过在定义一个类时,在类名后使用圆括号指定父类的名称来实现。
具体的语法如下:```pythonclass 父类名:# 父类的属性和方法的定义class 子类名(父类名):# 子类的属性和方法的定义```在一个类继承自父类后,它就拥有了父类的所有属性和方法。
子类可以直接访问父类的属性和方法,并且可以在子类中调用父类的方法。
下面是一个简单的示例,演示了继承的概念和实现方法:```pythonclass Animal:def __init__(self, name): = namedef speak(self):print("动物发出声音")class Dog(Animal):def speak(self):print("汪汪汪")animal = Animal("动物")animal.speak() # 输出:动物发出声音dog = Dog("狗")dog.speak() # 输出:汪汪汪```在上面的示例中,Animal类是一个父类,它有一个speak方法,输出"动物发出声音"。
Dog类是Animal类的子类,它复写了speak方法,并输出"汪汪汪"。
最后通过实例化Dog类的对象dog,并调用speak方法,输出"汪汪汪"。
二、多态的概念和实现多态是指一个对象可以根据当前所处的环境采取不同的行为。
Python继承与多态
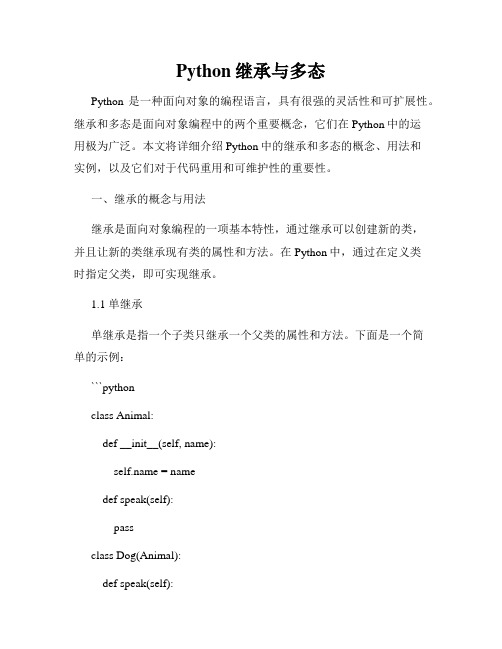
Python继承与多态Python是一种面向对象的编程语言,具有很强的灵活性和可扩展性。
继承和多态是面向对象编程中的两个重要概念,它们在Python中的运用极为广泛。
本文将详细介绍Python中的继承和多态的概念、用法和实例,以及它们对于代码重用和可维护性的重要性。
一、继承的概念与用法继承是面向对象编程的一项基本特性,通过继承可以创建新的类,并且让新的类继承现有类的属性和方法。
在Python中,通过在定义类时指定父类,即可实现继承。
1.1 单继承单继承是指一个子类只继承一个父类的属性和方法。
下面是一个简单的示例:```pythonclass Animal:def __init__(self, name): = namedef speak(self):passclass Dog(Animal):def speak(self):return "Woof!"dog = Dog("Bobby")print(dog.speak()) # 输出:Woof!```在上面的示例中,Animal是父类,Dog是子类,子类Dog通过继承父类Animal的属性和方法,并在自己的speak方法中进行了重写,实现了不同的功能。
1.2 多继承多继承是指一个子类可以同时继承多个父类的属性和方法。
下面是一个简单的示例:```pythonclass Flyable:def fly(self):passclass Swimable:def swim(self):passclass Bird(Flyable, Swimable):passbird = Bird()bird.fly() # 调用Flyable类的方法bird.swim() # 调用Swimable类的方法```在上述示例中,Bird类同时继承了Flyable和Swimable两个父类,因此可以调用这两个父类中的方法。
二、多态的概念与用法多态是面向对象编程中的另一个重要概念,它允许不同的对象对同一消息做出不同的响应。
面向对象程序设计中的继承与多态
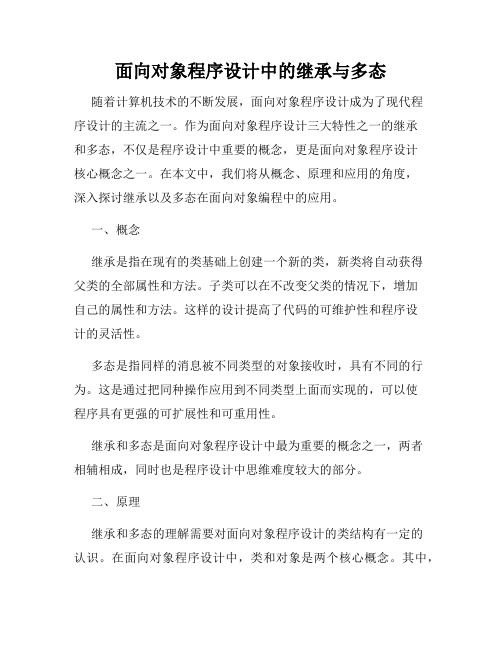
面向对象程序设计中的继承与多态随着计算机技术的不断发展,面向对象程序设计成为了现代程序设计的主流之一。
作为面向对象程序设计三大特性之一的继承和多态,不仅是程序设计中重要的概念,更是面向对象程序设计核心概念之一。
在本文中,我们将从概念、原理和应用的角度,深入探讨继承以及多态在面向对象编程中的应用。
一、概念继承是指在现有的类基础上创建一个新的类,新类将自动获得父类的全部属性和方法。
子类可以在不改变父类的情况下,增加自己的属性和方法。
这样的设计提高了代码的可维护性和程序设计的灵活性。
多态是指同样的消息被不同类型的对象接收时,具有不同的行为。
这是通过把同种操作应用到不同类型上面而实现的,可以使程序具有更强的可扩展性和可重用性。
继承和多态是面向对象程序设计中最为重要的概念之一,两者相辅相成,同时也是程序设计中思维难度较大的部分。
二、原理继承和多态的理解需要对面向对象程序设计的类结构有一定的认识。
在面向对象程序设计中,类和对象是两个核心概念。
其中,类是一个抽象的概念,具有相同特征和行为的对象的抽象模型。
而对象则是根据类所创建的实例。
在类的继承过程中,父类和子类之间不仅有继承关系还存在着多态性。
由于子类可以重写覆盖父类中的方法,当父类的引用指向子类时,同样的方法调用会产生不同的行为。
这种情况称之为多态性。
继承和多态的原理主要包括以下几个方面:1. 继承的封装性继承可以对已有的代码进行封装,从而避免重复代码,简化程序的设计过程。
继承让开发者可以将一些相同的属性和方法定义在一个基类中。
2. 继承的扩展性在父类中定义的方法可以被子类所继承并且可以被修改或者扩展。
利用继承机制可以帮助程序员更快速的编写出代码。
3. 多态性的覆盖性在子类中定义的方法会覆盖掉父类中相同签名的方法。
这样在父类引用指向子类时,同样的方法调用会产生不同的行为。
这种情况称之为多态性。
4. 多态性的重载性方法的重载是指在相同类中定义的方法,具有不同的参数列表,这样的方法称之为重载方法。
继承与多态实验报告心得

继承与多态实验报告心得
在进行继承与多态的实验过程中,我深刻理解了这两个面向对象编程的重要概念,并且在实践中体会到了它们的实际应用。
首先,继承是面向对象编程的一个基本特性,它允许创建新的类从已有的类中继承属性和方法。
在实验中,我创建了一个基类,然后通过继承来创建了多个子类。
这样,我可以在基类中定义一些通用的属性和方法,然后在子类中进行扩展或覆盖,实现了代码的重用和灵活性的提高。
通过继承,我可以很方便地创建出一个对象的不同类型的实例,从而满足不同的需求。
其次,多态是继承的一个重要特性,它允许使用基类类型的引用来引用一个子类的对象。
在实验中,我通过多态实现了一个统一的接口,使得可以以一种统一的方式处理不同类型的对象。
这样,我可以将具有相同行为的对象进行统一管理,提高了代码的灵活性和可扩展性。
在实验中,我还学会了使用抽象类和接口来实现多态。
抽象类提供了一种定义通用行为的方式,而接口则定义了一组方法的规范。
通过使用抽象类和接口,我可以定义一些通用的方法和属性,然后在具体的子类中进行实现和具体化。
这种方式可以有效地提高代码的可读性和可维护性。
继承与多态的实验为我提供了一个很好的学习机会,让我更深入地理解了面向对象编程的原理和思想。
通过实际操作,我不仅加深了对这两个概念的理解,还提升了自己的编程能力。
我相信,在以后的学习和工作中,我会继续运用这些知识,开发出更加高效和灵活的程序。
简单易懂说明类继承多态

简单易懂说明类继承多态好嘞,咱们今天就聊聊类、继承和多态这三个有趣的概念,听起来可能有点高大上,其实没那么复杂,咱们来用简单的例子把它们说得明明白白。
想象一下,你在一个大大的动物园里,动物们各自有各自的特长。
比如说,狮子吼、猴子跳、鸟儿飞,每种动物都有自己的特点,对吧?这就像是类的概念。
每个动物都是一个类,狮子是狮子类,猴子是猴子类,鸟儿是鸟类。
它们都有自己的属性,比如狮子有一身漂亮的鬃毛,猴子爱吃香蕉,鸟儿能唱动听的歌。
听着是不是感觉挺有趣的?所以,类就像是一个蓝图,告诉我们怎么描述一个动物的特性。
咱们说说继承。
这个词一听就觉得跟家族有关系,没错,就是这样。
想象一下,狮子和老虎都是猫科动物,狮子有它自己的特点,老虎也有。
可是,它们有很多共同的特性,比如说,它们都能跑得飞快,都喜欢肉食。
这时候,咱们可以把猫科动物这个共同的部分提取出来,作为一个父类。
狮子和老虎就可以从这个父类里“继承”下来了。
这就像是老一辈传给小辈的基因,狮子和老虎虽然各有千秋,但它们身上总有一些共同的特征。
这种继承关系让程序设计变得更简单、更加高效,想想看,你写代码的时候,能省下不少时间,简直是太爽了!多态这个词听起来有点复杂,其实它的意思就像是一个人可以扮演不同的角色。
你可能是学生、儿子、朋友,面对不同的人你会表现出不同的一面。
就拿动物来说,狮子叫,猴子也叫,虽然它们都在“叫”,但是声音完全不一样。
这就是多态的魅力。
咱们可以通过同一个方法,不同的类来实现各自的功能。
比如,咱们要让动物们“叫”,就可以写一个叫的函数,然后让每个动物自己实现这个函数。
狮子吼的时候就发出“吼”的声音,猴子就“啼”,鸟儿则是“鸣”。
这些不同的声音就叫多态。
真的是“各有千秋”,虽然都是在叫,但各自风格迥异,让人耳目一新。
好啦,听我说了这么多,可能你会问,这些概念有什么用呢?这些东西在日常编程中大显身手。
想象一下,你在写一个游戏,里面有很多角色。
如果每个角色都是从同一个父类继承来的,你就能很方便地管理它们。
面向对象程序设计中的继承与多态性原理探究

面向对象程序设计中的继承与多态性原理探究面向对象程序设计(Object-Oriented Programming,OOP)是一种常见的编程思想,它将计算机程序看作由对象的集合组成,每个对象都有自己的属性和行为,并且可以与其他对象进行交互。
在 OOP 中,继承和多态性是两个重要的概念,下面我们将对它们进行探究。
一、继承的原理继承是指一个类(称为子类)继承另一个类(称为父类)的属性和方法。
在继承的关系中,子类可以使用父类中的方法和变量,并且可以根据需要增加自己的属性和方法。
继承的原理是通过类的层次结构来实现的。
一个类可以有多个子类,这些子类从父类中继承了属性和方法,并且可以根据需要进行修改和扩展。
继承的优点在于,可以避免在每个子类中重复定义相同的属性和方法,从而提高代码的重用性和可维护性。
在 Java 中,所有的类都是从 Object 类继承而来的。
因此,所有的类都可以使用 Object 类中定义的方法,例如 equals() 和toString() 等方法。
在创建一个类的时候,只需要通过 extends 关键字指定父类即可,例如:```javaclass MyClass extends MyParentClass {// 子类的实现代码}```当一个类继承另一个类时,它可以重写父类中的方法,也可以添加自己的方法。
如果子类定义了与父类相同的方法,那么子类的方法将覆盖父类的方法。
这种方法重写的机制可以实现多形式设计。
二、多态性的原理多态性是指同一个方法在不同的情况下产生不同的表现形式。
在 OOP 中,多态性是通过继承和接口实现的。
我们可以使用相同的方法名,但是在不同的对象和类中实现不同的功能。
多态性的实现方式有三种:方法重载、方法重写和接口实现。
方法重载是指在同一类中定义了两个或两个以上的方法,它们具有相同的方法名,但是参数列表不同。
例如:```javapublic void print(int a, int b) {System.out.println(a + ", " + b);}public void print(int a, int b, int c) {System.out.println(a + ", " + b + ", " + c);}```在上面的例子中,我们定义了两个 print() 方法,它们都具有相同的方法名,但是参数列表不同。
Python中的类继承和多态有什么特点
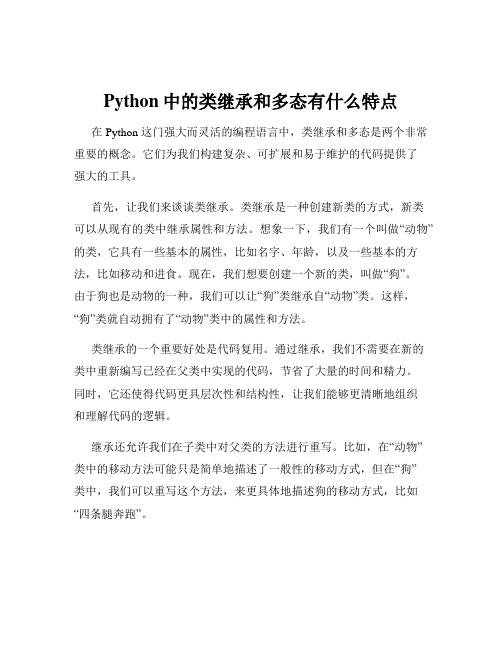
Python中的类继承和多态有什么特点在 Python 这门强大而灵活的编程语言中,类继承和多态是两个非常重要的概念。
它们为我们构建复杂、可扩展和易于维护的代码提供了强大的工具。
首先,让我们来谈谈类继承。
类继承是一种创建新类的方式,新类可以从现有的类中继承属性和方法。
想象一下,我们有一个叫做“动物”的类,它具有一些基本的属性,比如名字、年龄,以及一些基本的方法,比如移动和进食。
现在,我们想要创建一个新的类,叫做“狗”。
由于狗也是动物的一种,我们可以让“狗”类继承自“动物”类。
这样,“狗”类就自动拥有了“动物”类中的属性和方法。
类继承的一个重要好处是代码复用。
通过继承,我们不需要在新的类中重新编写已经在父类中实现的代码,节省了大量的时间和精力。
同时,它还使得代码更具层次性和结构性,让我们能够更清晰地组织和理解代码的逻辑。
继承还允许我们在子类中对父类的方法进行重写。
比如,在“动物”类中的移动方法可能只是简单地描述了一般性的移动方式,但在“狗”类中,我们可以重写这个方法,来更具体地描述狗的移动方式,比如“四条腿奔跑”。
接下来,我们聊聊多态。
多态这个概念可能一开始会让人感到有些困惑,但其实它非常实用。
多态意味着不同的对象可以对相同的消息做出不同的响应。
比如说,我们有一个函数,它的参数是一个“动物”类型的对象。
当我们把“狗”对象传递给这个函数时,函数内部会调用“动物”类中的某个方法。
但由于我们传递的是“狗”对象,实际上会执行的是“狗”类中重写的那个方法。
这就是多态的体现。
多态的好处在于它增加了代码的灵活性和可扩展性。
我们不需要为每一种具体的动物类型都编写一个单独的函数,只需要一个通用的函数,就可以处理各种不同类型的动物对象。
再举个例子,假设我们有一个“展示动物信息”的函数。
它接收一个动物对象作为参数,并打印出动物的一些基本信息。
无论我们传递给它的是“狗”对象、“猫”对象还是其他动物对象,这个函数都能正常工作,并且会根据具体对象的类型,打印出相应的准确信息。
继承与多态实验报告

继承与多态实验报告继承与多态实验报告在面向对象编程中,继承和多态是两个重要的概念。
通过继承,我们可以创建新的类,并从现有的类中继承属性和方法。
而多态则允许我们使用父类的引用来指向子类的对象,实现同一操作具有不同的行为。
本实验旨在通过实际的编程实践,加深对继承和多态的理解。
实验一:继承在这个实验中,我们创建了一个动物类(Animal),并从它派生出了两个子类:狗类(Dog)和猫类(Cat)。
动物类具有一些共同的属性和方法,如名字(name)和发出声音(makeSound)。
子类继承了父类的属性和方法,并可以添加自己的特定属性和方法。
在编写代码时,我们首先定义了动物类,并在其中实现了共同的属性和方法。
然后,我们创建了狗类和猫类,并分别在这两个类中添加了自己的特定属性和方法。
通过继承,我们可以在子类中直接使用父类的方法,并且可以根据需要进行重写。
实验二:多态在这个实验中,我们使用多态的概念来实现一个动物园的场景。
我们定义了一个动物园类(Zoo),并在其中创建了一个动物数组。
这个数组可以存储不同类型的动物对象,包括狗、猫等。
通过多态,我们可以使用动物类的引用来指向不同类型的动物对象。
例如,我们可以使用动物类的引用来指向狗对象,并调用狗类特有的方法。
这样,我们可以统一处理不同类型的动物对象,而不需要为每种类型编写特定的处理代码。
实验三:继承与多态的结合应用在这个实验中,我们进一步探索了继承和多态的结合应用。
我们定义了一个图形类(Shape),并从它派生出了三个子类:圆形类(Circle)、矩形类(Rectangle)和三角形类(Triangle)。
每个子类都实现了自己的特定属性和方法。
通过继承和多态,我们可以在图形类中定义一些通用的方法,如计算面积和周长。
然后,我们可以使用图形类的引用来指向不同类型的图形对象,并调用相应的方法。
这样,我们可以轻松地对不同类型的图形进行统一的处理。
结论:通过本次实验,我们进一步理解了继承和多态的概念,并学会了如何在编程中应用它们。
Python中的继承和多态
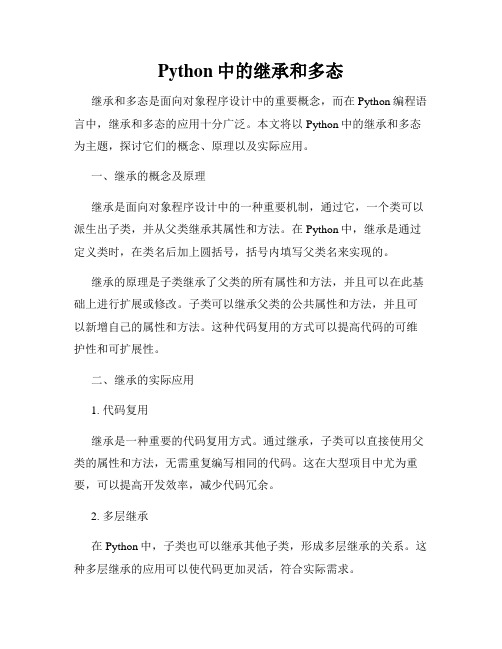
Python中的继承和多态继承和多态是面向对象程序设计中的重要概念,而在Python编程语言中,继承和多态的应用十分广泛。
本文将以Python中的继承和多态为主题,探讨它们的概念、原理以及实际应用。
一、继承的概念及原理继承是面向对象程序设计中的一种重要机制,通过它,一个类可以派生出子类,并从父类继承其属性和方法。
在Python中,继承是通过定义类时,在类名后加上圆括号,括号内填写父类名来实现的。
继承的原理是子类继承了父类的所有属性和方法,并且可以在此基础上进行扩展或修改。
子类可以继承父类的公共属性和方法,并且可以新增自己的属性和方法。
这种代码复用的方式可以提高代码的可维护性和可扩展性。
二、继承的实际应用1. 代码复用继承是一种重要的代码复用方式。
通过继承,子类可以直接使用父类的属性和方法,无需重复编写相同的代码。
这在大型项目中尤为重要,可以提高开发效率,减少代码冗余。
2. 多层继承在Python中,子类也可以继承其他子类,形成多层继承的关系。
这种多层继承的应用可以使代码更加灵活,符合实际需求。
三、多态的概念及原理多态是面向对象程序设计的另一个重要概念,它允许在不改变引用对象的情况下,调用不同类的同名方法。
在Python中,多态是通过将对象赋值给不同的类实例变量来实现的。
多态的原理是同一个方法名在不同的类中可以有不同的实现方式,通过将对象赋给父类或子类实例变量,可以调用到正确的方法实现。
这种机制使得代码具有更好的可扩展性和可维护性。
四、多态的实际应用1. 面向接口编程多态的应用使得对象的类型可以在运行时确定,而不是在编译时确定,这样可以更加灵活地进行面向接口编程。
通过定义接口,并在不同的类中实现该接口的方法,可以在不改变接口调用的情况下,调用不同类的同名方法。
2. 调用封装的方法在实际应用中,多态可以使代码更加简洁和易读。
通过将对象赋值给父类实例变量,可以统一调用封装的方法,无需考虑具体是哪个类的实例。
数据库设计中的继承和多态关系
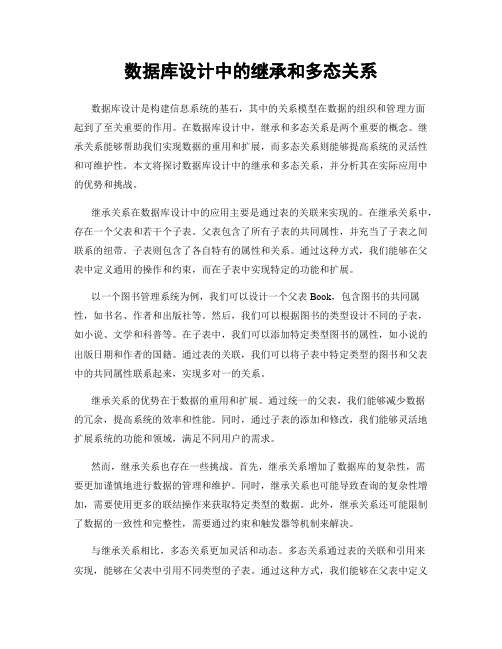
数据库设计中的继承和多态关系数据库设计是构建信息系统的基石,其中的关系模型在数据的组织和管理方面起到了至关重要的作用。
在数据库设计中,继承和多态关系是两个重要的概念。
继承关系能够帮助我们实现数据的重用和扩展,而多态关系则能够提高系统的灵活性和可维护性。
本文将探讨数据库设计中的继承和多态关系,并分析其在实际应用中的优势和挑战。
继承关系在数据库设计中的应用主要是通过表的关联来实现的。
在继承关系中,存在一个父表和若干个子表。
父表包含了所有子表的共同属性,并充当了子表之间联系的纽带。
子表则包含了各自特有的属性和关系。
通过这种方式,我们能够在父表中定义通用的操作和约束,而在子表中实现特定的功能和扩展。
以一个图书管理系统为例,我们可以设计一个父表Book,包含图书的共同属性,如书名、作者和出版社等。
然后,我们可以根据图书的类型设计不同的子表,如小说、文学和科普等。
在子表中,我们可以添加特定类型图书的属性,如小说的出版日期和作者的国籍。
通过表的关联,我们可以将子表中特定类型的图书和父表中的共同属性联系起来,实现多对一的关系。
继承关系的优势在于数据的重用和扩展。
通过统一的父表,我们能够减少数据的冗余,提高系统的效率和性能。
同时,通过子表的添加和修改,我们能够灵活地扩展系统的功能和领域,满足不同用户的需求。
然而,继承关系也存在一些挑战。
首先,继承关系增加了数据库的复杂性,需要更加谨慎地进行数据的管理和维护。
同时,继承关系也可能导致查询的复杂性增加,需要使用更多的联结操作来获取特定类型的数据。
此外,继承关系还可能限制了数据的一致性和完整性,需要通过约束和触发器等机制来解决。
与继承关系相比,多态关系更加灵活和动态。
多态关系通过表的关联和引用来实现,能够在父表中引用不同类型的子表。
通过这种方式,我们能够在父表中定义通用的操作和约束,而在子表中实现具体的功能。
这样,我们能够在系统运行时根据实际情况动态地选择具体的操作和处理逻辑。
编程语言面向对象编程中的继承与多态的应用

编程语言面向对象编程中的继承与多态的应用继承(inheritance)和多态(polymorphism)是面向对象编程(OOP)中两个重要的概念。
继承指的是一个类(子类)从另一个类(父类)获得属性和方法的能力,而多态指的是同一个方法可以根据调用对象的不同而表现出不同的行为。
在编程语言中,继承和多态为程序设计带来了灵活性和可扩展性。
本文将探讨继承和多态在编程语言中的应用和优势。
一、继承的概念与应用继承是OOP中的一个核心概念,通过继承,子类可以通过直接拥有父类的属性和方法,从而减少了代码的重复性,提高了代码的可重用性。
继承分为单继承和多继承两种形式。
1. 单继承在单继承中,一个子类只能继承一个父类的属性和方法。
通过继承,子类可以获得父类的非私有成员,并且可以对这些成员进行进一步的扩展和修改。
这种机制使得代码更加简洁和模块化,提高了代码的可读性和可维护性。
2. 多继承多继承允许一个子类同时继承多个父类的属性和方法。
这种机制使得代码之间的关联性更加灵活,可以根据实际需求选择不同的父类进行继承。
同时,多继承也带来了一些挑战,比如命名冲突和代码复杂度的增加,需要开发者具备更高的抽象和设计能力来合理应用多继承。
二、多态的概念与应用多态是OOP中的另一个重要概念,它允许不同的对象对于同一消息呈现出不同的行为。
通过多态,程序可以更加灵活地处理不同类型的对象,提高了代码的可扩展性和可维护性。
多态可以通过方法重写和接口实现来实现。
方法重写指的是子类重写父类的方法,从而改变了方法的行为。
接口实现指的是多个类实现了同一个接口,并且可以根据需要调用不同的实现。
三、继承与多态的优势继承和多态在面向对象编程中具有以下几个优势:1. 提高代码的重用性:通过继承可以将一些通用的属性和方法集中在父类中,子类可以直接继承使用,减少了代码的重复性。
2. 提高代码的可读性和可维护性:继承使代码更加模块化,减少了代码的复杂度,提高了代码的可读性和可维护性。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
string getName()
{
return _name;
}
public string statement()
{
double totalAmountБайду номын сангаас= 0; //总共的租金
int frequentRenterPoints = 0;//积分
string result = "\r-------------------------------------------\n\r " +
{
return 1;
}
public TYPE getPriceCode()
{
return (TYPE)_priceCode;
}
void setPriceCode(TYPE arg)
{
_priceCode = arg;
}
public string getTitle()
{
return _title;
}
}
if (daysRented > 3)
result += (daysRented - 3) * 1.5;
return result;
}
}
}
NewReleaseMovie子类的代码如下:重写租金计算方法(多态性)和积分计算方法(多态性)。
using System;
using System.Collections.Generic;
totalAmount += thisAmount;
}
result += "\n共消费" + totalAmount + "元" + "\n您增加了" + frequentRenterPoints + "个积分\n";
return result;
}
}
}
主程序代码如下:
using System;
using System.Collections.Generic;
}
public Movie(string title, TYPE priceCode)
{
_title = title;
_priceCode = priceCode;
}
public virtual double getCharge(int daysRented)
{
return 0;//收费
}
public virtual int getFrequentRenterPoints(int daysRented)//积分
"租碟记录--- " + getName() + "\n";
foreach (Rental iter in _rentals)
{
double thisAmount = 0;
Rental each = iter;
switch (each.getMovie().getTypeCode())
{
case TYPE.REGULAR:
using System.Linq;
using System.Text;
namespace _tbed
{
public class NewReleaseMovie:Movie
{
public NewReleaseMovie (string title)
{
_title = title;
}
public override double getCharge(int daysRented)
Rental r3 = new Rental(m3, 7);
Rental r4 = new Rental(m2, 5);
Rental r5 = new Rental(m3, 3);
Customer c1 = new Customer("孙红雷");
c1.addRental(r1);
c1.addRental(r4);
Movie m2 = new Movie("我是特种兵之利刃出鞘", TYPE.REGULAR);
Movie m3 = new Movie("熊出没之环球大冒险", TYPE.CHILDRENS);
Rental r1 = new Rental(m1, 4);
Rental r2 = new Rental(m1, 2);
1 using System;
2 using System.Collections.Generic;
3 using System.Linq;
4 using System.Text;
5
6 namespace _tbed
7 {
8 public enum TYPE
9 {
10 REGULAR,
11 NEW_RELEASE,
{
public class ChildrensMovie : Movie
{
public ChildrensMovie (string title)
{
_title = title;
}
public override double getCharge(int daysRented)
{
double result = 1.5;
break;
case TYPE.CHILDRENS:
thisAmount += 1.5;//3天之内1.5元
if (each.getDaysRented() > 3)
thisAmount += (each.getDaysRented() - 3) * 1.5;
break;
}
frequentRenterPoints++;//对于每一种类型的影片,一次租用积分加1
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace _tbed
{
class Rental
{
private Movie _movie;
int _daysRented;
Customer c4 = new Customer("孙俪");
c4.addRental(r2);
c4.addRental(r3);
c4.addRental(r5);
Console.Write(c1.statement());
Console.Write(c2.statement());
Console.Write(c3.statement());
using System.Linq;
using System.Text;
namespace _tbed
{
class Program
{
static voidMain(string[] args)
{
Console.Write("影碟店客户租碟明细");
Movie m1 = new Movie("致我们终将逝去的青春", TYPE.NEW_RELEASE);
public Rental(Movie movie, int daysRented)
{
_movie = movie;
_daysRented = daysRented;
}
public int getDaysRented()
{
return _daysRented;
}
public Movie getMovie()
35 }
36
37 void setTypeCode(TYPE arg)
38 {
39 _typeCode = arg;
40 }
41
42 public string getTitle()
43 {
44 return _title;
45 }
46 }
47 }
Rental类的代码如下,租用记录中包含了一个movie对象,以及一个租期成员(积分和租金与此有关):
12 CHILDRENS
13 }
14
15 class Movie
16 {
17 private string _title; //movie name
18 TYPE _typeCode; //price code
19
20 public Movie()
21 {
22 _title = "unname";
23 _typeCode = 0;
if ((each.getMovie().getTypeCode() == TYPE.NEW_RELEASE) &&
each.getDaysRented() > 1)
frequentRenterPoints++;
result += "\n\t" + each.getMovie().getTitle() + "\t" + thisAmount;
新的Movie类的代码如下:Movie类提供积分计算和租金计算的默认实现。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace _tbed
{
public enum TYPE