第5章 面向对象程序设计基础部分答案
python程序设计基础课后习题答案(第五章)

第五章答案5.2:实现i s o d d()函数,参数为整数,如果参数为奇数,返回t r u e,否则返回f a l s e。
def isodd(s):x=eval(s)if(x%2==0):return Falseelse:return Truex=input("请输入一个整数:")print(isodd(x))请输入一个整数:5True5.3:实现i s n u m()函数,参数为一个字符串,如果这个字符串属于整数、浮点数或复数的表示,则返回t r u e,否则返回f a l s e。
def isnum(s):try:x=eval(s)if((type(x)==int)|(type(x)==float)|(type(x)==complex)):return Trueelse:return Falseexcept NameError:return Falsex=input("请输入一个字符串:")print(isnum(x))请输入一个字符串:5True题5.4:实现m u l t i()函数,参数个数不限,返回所有参数的乘积。
def multi(x):xlist=x.split(",")xlist = [int(xlist[i]) for i in range(len(xlist))] #for循环,把每个字符转成int值num=1for i in xlist:num=num*iprint(num)s=input("请输入数字,并用,号隔开:")multi(s)请输入数字,并用,号隔开:5,420题5.5:实现i s p r i m e()函数,参数为整数,要有异常处理,如果整数是质数返回t u r e,否则返回f a l s e。
try:def isprime(s):i=2m=0for i in range(2,s-1):if(s%i==0):i+=1m+=1else:i+=1if(m>0):return Falseelse:return Trueexcept NameError:print("请输入一个整数!")s=eval(input("请输入任意一个整数:")) print(isprime(s))请输入任意一个整数:9False。
面向对象程序设计教程 答案

面向对象程序设计教程答案面向对象程序设计教程
第一章:介绍面向对象程序设计
⑴面向对象程序设计的概念
⑵面向对象程序设计的优势
⑶面向对象程序设计的基本原则
第二章:面向对象的基本概念
⑴类和对象的概念
⑵属性和方法的定义
⑶封装、继承和多态的概念
第三章:类的设计与实现
⑴类的设计原则
⑵类的成员变量和成员函数的设计
⑶类的构造函数和析构函数的设计
⑷类的访问控制和封装性的实现
第四章:继承与多态
⑴继承的概念和用法
⑵单继承和多继承的区别
⑶虚函数的定义和使用
⑷纯虚函数和抽象类的概念
第五章:面向对象的设计模式
⑴设计模式的概念和分类
⑵单例模式的实现
⑶工厂模式的实现
⑷观察者模式的实现
第六章:面向对象程序设计的实例
⑴车辆管理系统的设计与实现
⑵学生信息管理系统的设计与实现
⑶游戏角色的设计与实现
附件:
⒈示例代码
⒉ UML图
法律名词及注释:
⒈版权:根据国际公约,对作者创作的作品(包括文学、艺术、音乐、软件等)的独有权益进行法律保护。
⒉商标:商标是指为了区别商品或服务而在商品上、包装上、容器上或者商品的商业文件上使用的特定标识的任何符号。
⒊专利:专利是为了推动技术创新,提供创新者在一定时期内对其发明进行独占权利的一种法律制度。
⒋许可证:许可证是指一方在法律许可范围内允许他人使用自己的某种权利的文件。
面向对象程序设计教程 答案

面向对象程序设计教程答案面向对象程序设计教程第1章引言1.1 背景1.1.1 传统的结构化程序设计方法1.1.2 面向对象的程序设计方法1.2 目标1.3 本教程的组织结构第2章面向对象的基本概念2.1 类和对象2.1.1 类的定义2.1.2 对象的创建与使用2.2 封装和信息隐藏2.2.1 封装的概念2.2.2 信息隐藏的重要性2.3 继承和多态2.3.1 继承的概念与用法 2.3.2 多态的概念与用法 2.4 抽象和接口2.4.1 抽象的概念与用法 2.4.2 接口的概念与用法第3章面向对象的设计原则3.1 单一职责原则3.2 开放-封闭原则3.3 里氏替换原则3.4 依赖倒置原则3.5 接口隔离原则3.6 迪米特法则第4章面向对象的分析与设计 4.1 需求分析4.1.1 确定需求4.1.2 分析需求4.2 类的设计4.2.1 识别类的责任4.2.2 定义类的属性和行为 4.3 协作关系的设计4.3.1 关联关系4.3.2 依赖关系4.3.3 聚合关系4.3.4 组合关系4.3.5 继承关系第5章面向对象的编程语言5.1 Java5.2 C++5.3 Python5.4 Ruby第6章实例分析:学绩管理系统 6.1 需求分析6.2 类的设计6.3 协作关系的设计6.4 实现与测试第7章总结本文档涉及附件:无本文所涉及的法律名词及注释:1:封装:将数据和对数据的操作封装在一个类中,外部无法直接访问和修改内部数据,只能通过类提供的接口访问和修改。
2:信息隐藏:对于类的用户来说,只需要知道如何使用类的接口,而不需要知道类的实现细节。
3:继承:一个类可以从另一个类继承属性和方法,继承可以提高代码的复用性和可维护性。
4:多态:同一个方法可以根据不同的对象调用不同的实现,提高代码的灵活性和可扩展性。
5:抽象:将一组具有相似属性和行为的对象抽象成一个类,可以减少代码重复。
6:接口:描述类的行为,定义类应该实现的方法。
面向对象程序设计答案

m_fReal += c.m_fReal;
m_fImag += c.m_fImag;
return *this;
}
Complex& operator-= (const Complex &c)
{
m_fReal -= c.m_fReal;
m_fImag -= c.m_fImag;
return *this;
在日常生活或者说日常编程中,简单的问题用面向过程的思路解决,更加直接有效,但是当问题的规模稍大时,如要描述三万个人吃饭的问题,或者要构建一个航空母舰模型的时候,用面向过程的思想是远远不够的。而且面向过程程序的代码复用性、可扩展性、可移植性、灵活性、健壮性都会在处理大规模问题中出现问题。
二、综合回答(每小题15分,共30分)
一、简答题(每小题6分,共30分)
1、面向过程思想的优点是什么?
与人类思维习惯一致;稳定性好:以object模拟实体,需求变化不会引起结构的整体变化,因为实体相对稳定,故系统也相应稳定;可重用性好;可维护性好
2、比较Java和C++?
JAVA和C++都是面向对象语言。也就是说,它们都能够实现面向对象思想(封装,继乘,多态)。而由于c++为了照顾大量的C语言使用者,从而兼容了C,使得自身仅仅成为了带类的C语言,多多少少影响了其面向对象的彻底性!JAVA则是完全的面向对象语言,它句法更清晰,规模更小,更易学。它是在对多种程序设计语言进行了深入细致研究的基础上,摒弃了其他语言的不足之处,从根本上解决了c++的固有缺陷。用C++可以使用纯过程化的编程,也可以是基于对象的编程,还可以是面向对象的编程,当然大部分是混合编程,c++可以跨平台
C++第5章习题参考答案
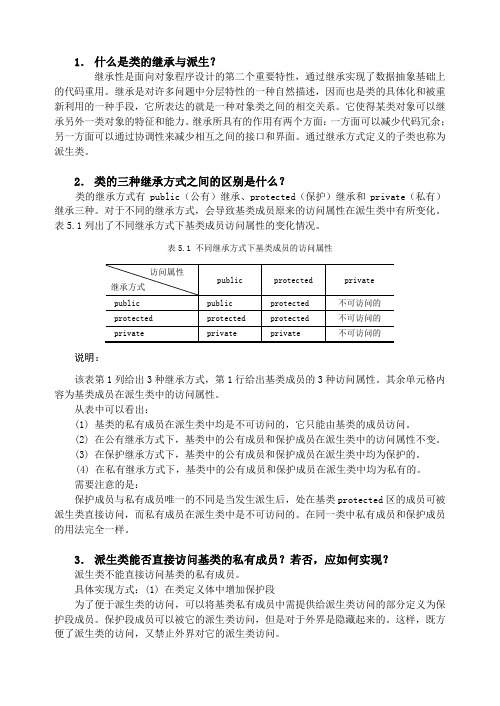
1.什么是类的继承与派生?继承性是面向对象程序设计的第二个重要特性,通过继承实现了数据抽象基础上的代码重用。
继承是对许多问题中分层特性的一种自然描述,因而也是类的具体化和被重新利用的一种手段,它所表达的就是一种对象类之间的相交关系。
它使得某类对象可以继承另外一类对象的特征和能力。
继承所具有的作用有两个方面:一方面可以减少代码冗余;另一方面可以通过协调性来减少相互之间的接口和界面。
通过继承方式定义的子类也称为派生类。
2.类的三种继承方式之间的区别是什么?类的继承方式有public(公有)继承、protected(保护)继承和private(私有)继承三种。
对于不同的继承方式,会导致基类成员原来的访问属性在派生类中有所变化。
表5.1列出了不同继承方式下基类成员访问属性的变化情况。
表5.1 不同继承方式下基类成员的访问属性说明:该表第1列给出3种继承方式,第1行给出基类成员的3种访问属性。
其余单元格内容为基类成员在派生类中的访问属性。
从表中可以看出:(1) 基类的私有成员在派生类中均是不可访问的,它只能由基类的成员访问。
(2) 在公有继承方式下,基类中的公有成员和保护成员在派生类中的访问属性不变。
(3) 在保护继承方式下,基类中的公有成员和保护成员在派生类中均为保护的。
(4) 在私有继承方式下,基类中的公有成员和保护成员在派生类中均为私有的。
需要注意的是:保护成员与私有成员唯一的不同是当发生派生后,处在基类protected区的成员可被派生类直接访问,而私有成员在派生类中是不可访问的。
在同一类中私有成员和保护成员的用法完全一样。
3.派生类能否直接访问基类的私有成员?若否,应如何实现?派生类不能直接访问基类的私有成员。
具体实现方式:(1) 在类定义体中增加保护段为了便于派生类的访问,可以将基类私有成员中需提供给派生类访问的部分定义为保护段成员。
保护段成员可以被它的派生类访问,但是对于外界是隐藏起来的。
这样,既方便了派生类的访问,又禁止外界对它的派生类访问。
《Java程序设计》教材第五章练习题答案
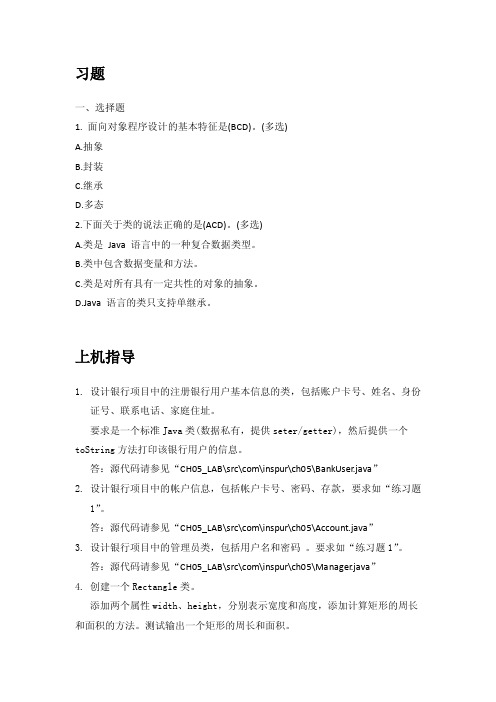
习题一、选择题1. 面向对象程序设计的基本特征是(BCD)。
(多选)A.抽象B.封装C.继承D.多态2.下面关于类的说法正确的是(ACD)。
(多选)A.类是Java 语言中的一种复合数据类型。
B.类中包含数据变量和方法。
C.类是对所有具有一定共性的对象的抽象。
D.Java 语言的类只支持单继承。
上机指导1.设计银行项目中的注册银行用户基本信息的类,包括账户卡号、姓名、身份证号、联系电话、家庭住址。
要求是一个标准Java类(数据私有,提供seter/getter),然后提供一个toString方法打印该银行用户的信息。
答:源代码请参见“CH05_LAB\src\com\inspur\ch05\BankUser.java”2.设计银行项目中的帐户信息,包括帐户卡号、密码、存款,要求如“练习题1”。
答:源代码请参见“CH05_LAB\src\com\inspur\ch05\Account.java”3.设计银行项目中的管理员类,包括用户名和密码。
要求如“练习题1”。
答:源代码请参见“CH05_LAB\src\com\inspur\ch05\Manager.java”4.创建一个Rectangle类。
添加两个属性width、height,分别表示宽度和高度,添加计算矩形的周长和面积的方法。
测试输出一个矩形的周长和面积。
答:源代码请参见“CH05_LAB\src\com\inspur\ch05\Rectangle.java”5.猜数字游戏:一个类A有一个成员变量v,有一个初值100。
定义一个类,对A类的成员变量v进行猜。
如果大了则提示大了,小了则提示小了。
等于则提示猜测成功。
答:源代码请参见“CH05_LAB\src\com\inspur\ch05\Guess.java”6.编写一个Java程序,模拟一个简单的计算器。
定义名为Computer的类,其中两个整型数据成员num1和num1,编写构造方法,赋予num1和num2初始值,再为该类定义加、减、乘、除等公有方法,分别对两个成员变量执行加减乘除的运算。
《C面向对象程序设计答案解析》-第五章谭浩强-清华大学出版社
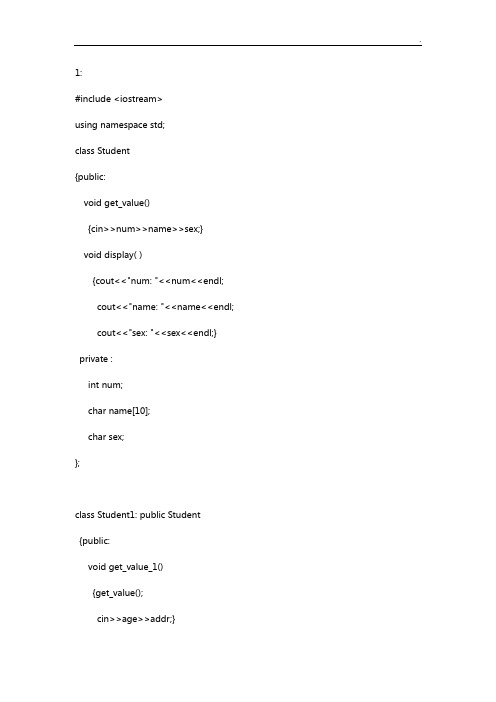
1:#include <iostream>using namespace std;class Student{public:void get_value(){cin>>num>>name>>sex;}void display( ){cout<<"num: "<<num<<endl;cout<<"name: "<<name<<endl;cout<<"sex: "<<sex<<endl;} private :int num;char name[10];char sex;};class Student1: public Student {public:void get_value_1(){get_value();cin>>age>>addr;}{ cout<<"age: "<<age<<endl; //引用派生类的私有成员,正确。
cout<<"address: "<<addr<<endl;} //引用派生类的私有成员,正确。
private:int age;char addr[30];};int main(){Student1 stud1;stud1.get_value_1();stud1.display();stud1.display_1();return 0;}2:#include <iostream>using namespace std;class Student{public:void get_value(){cin>>num>>name>>sex;}{cout<<"num: "<<num<<endl;cout<<"name: "<<name<<endl;cout<<"sex: "<<sex<<endl;}private :int num;char name[10];char sex;};class Student1: private Student{public:void get_value_1(){get_value();cin>>age>>addr;}void display_1(){display();cout<<"age: "<<age<<endl; //引用派生类的私有成员,正确。
C++面向对象程序设计教程课后习题答案
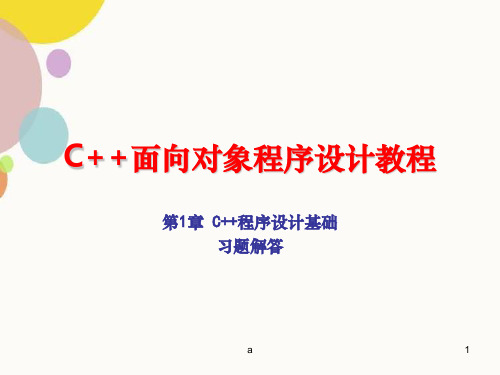
a
15
2.在面向对象方法中,实现信息隐蔽是依靠 。
A)对象的继承
B)对象的多态
C)对象的封装
D)对象的分类
解析:在面向对象方法中,封装性是指将数据和算法捆绑成一个 整体,这个整体就是对象,描述对象的数据被封装在内部,只可 以通过对象提供的算法来进行操作,从而实现信息隐蔽。 答案:C
a
16
3.下列关于类和对象的叙述中,错误的是 。 A)一个类只能有一个对象 B)对象是类的具体实例 C)类是某一类对象的抽象 D)类和对象的关系就像数据类型和变量的关系
}
}
……
a
11
*5.编一个程序,用同一个函数名对n个数据进行从小到大排序,数据类型可
以是整型、单精度实型、双精度实型,用重载函数实现。
参考程序:
……
int main()
// 主函数main()
{
int a[] = {1, 3, 2, 5, 6, 9, 0, 6};
// 定义a
float b[] = {1.6, 3.3, 2.8, 5.6, 6.8, 9.6, 0.6, 6.8}; // 定义b
所以先输出i的值1,再使i加1。
答案:A
a
4
二、编程题
1.编写一个C++程序,要求输出“欢迎学习C++语言!”。
参考程序: #include <iostream> using namespace std;
// 编译预处理命令 // 使用命名空间std
int main()
// 主函数main()
{
cout << "欢迎学习C++语言!"<< endl; // 用C++的方法输出一行
visualc程序设计应用教程习题答案郭力子第5章面向对象编程基础
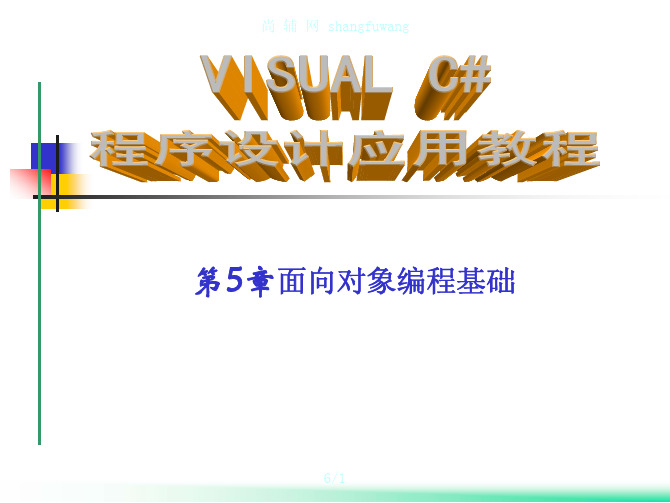
类的对象被撤销时,将自动调用析构函数。一些善后工作 可放在析构函数中完成。析构函数的名字为类名前面加一 个“~”符号,无返回类型,也无参数。
构造函数和析构函数的执行是无条件的,在声明一个对象 或销毁一个对象时,如果没有声明相应的构造函数和析构 函数,系统会自动为之产生,并在创建对象时调用构造函 数,而在销毁对象时调用析构函数
例:简单表达类A中的属性myProperties的声明:
class A {
private int i;
public int myProperties {
get {
//get访问器
return i;
}
set {
//set访问器
i=value;
}
}
}
6/1
《 Visual C#程序设计应用教程》
尚 辅 网 shangfuwang
果用上面的构造函数去构建了一个目标对象,则this便可以 用来代表所构建的对象。this用在构造函数中时,便于区别 同名的构造函数参数和类成员变量。
6/1
《 Visual C#程序设计应用教程》
尚 辅 网 shangfuwang
5.2.6 类的继承
继承允许在已有类的基础上创建新类,新类从已有类中继承类 成员,而且可以重新定义或增加新的成员,从而形成类的层 次或等级。
尚 辅 网 shangfuwang
set访问器:给属性赋值时使用,set访问器始终使用隐含的 参数value设置属性的值。获取属性值时使用get访问器。
get访问器:通过return返回属性的值。如果只有get访问 器,表示是只读属性;如果只有set访问器,表示只写属 性;如果既有get也有set访问器,表示读写属性。
C 面向对象程序设计习题解答(全)

4答案 n=2,sum=2 n=3,sum=5 n=5,sum=10
5答案 x=3 6答案 x=1,y=2 x=30,y=40 7答案 1 2 3 4 exit main 3 2 1 8答案 n=100 9答案 the student is:Li Hu the teacher is:Wang Ping 10答案 2 11答案 1035,789.504 12答案 13答案
一、选择题 1 2 3 4 D B B C
类和对象的进一步解析
5 D 6 D 7 D B 8 C B 9 10 11 12 13 14 15 16 B D B A A C B A
17 18 19 20 21 22 23 24 C C D B A D 二、填空题 1 this 2所有成员 3友元类、友元函数 4 friend 5 程序编译、程序结束 三、程序阅读题
第六章 派生与继承
一、选择题 1(1) 1(2) 2 A B 3 4 5 6 7 8 9 10 11 D C C C D D B C A D
二、填空题 1 继承 2 具体化、抽象 3 公有继承、保护继承、私有继承 4 子对象 5 public(共有的)、protected(保护的)、不可访问 6 protected(保护的)、protected(保护的)、不可访问的 7 private(私有的)、private(私有的)、不可访问的 8 二义性 三、判断下列描述的正确性 1 2 3 4 5 6 7 8 9 10 11 12 13 √ × × × × × √ √ × × √ √ ×
1、 选择题 1 2 3 4 5 6 7 C 8 9 10 D D D C A D C 2、 程序阅读题 1答案 a=639,b=78,c=12 2答案 a=5,b=8 a=8,b=5 3答案 10 4答案 x=20.6 y=5 z=A x=216.34 y=5 z=A x=216.34 y=2 z=A x=216.34 y=2 z=E 5答案 ic=11 fc=7.82 ic=5 fc=2.15 3、 判断下列描述的正确性 1 2 √ × D A
第五章 习题答案
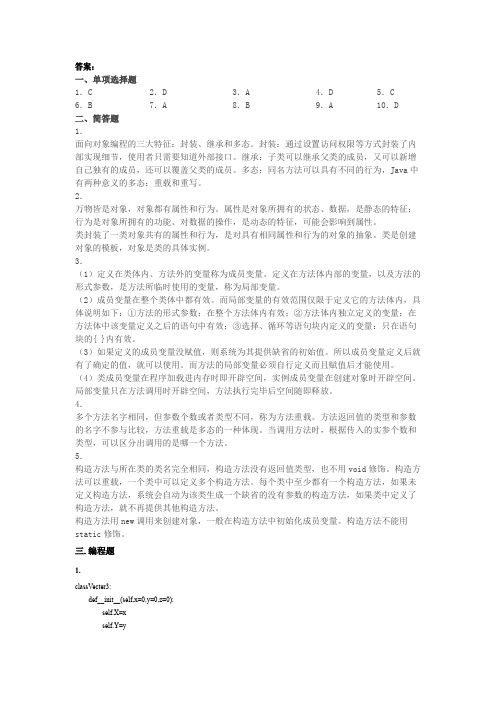
答案:一、单项选择题1.C 2.D 3.A 4.D 5.C6.B 7.A 8.B 9.A 10.D 二、简答题1.面向对象编程的三大特征:封装、继承和多态。
封装:通过设置访问权限等方式封装了内部实现细节,使用者只需要知道外部接口。
继承:子类可以继承父类的成员,又可以新增自己独有的成员,还可以覆盖父类的成员。
多态:同名方法可以具有不同的行为,Java中有两种意义的多态:重载和重写。
2.万物皆是对象,对象都有属性和行为。
属性是对象所拥有的状态、数据,是静态的特征;行为是对象所拥有的功能、对数据的操作,是动态的特征,可能会影响到属性。
类封装了一类对象共有的属性和行为,是对具有相同属性和行为的对象的抽象。
类是创建对象的模板,对象是类的具体实例。
3.(1)定义在类体内、方法外的变量称为成员变量。
定义在方法体内部的变量,以及方法的形式参数,是方法所临时使用的变量,称为局部变量。
(2)成员变量在整个类体中都有效。
而局部变量的有效范围仅限于定义它的方法体内,具体说明如下:①方法的形式参数:在整个方法体内有效;②方法体内独立定义的变量:在方法体中该变量定义之后的语句中有效;③选择、循环等语句块内定义的变量:只在语句块的{ }内有效。
(3)如果定义的成员变量没赋值,则系统为其提供缺省的初始值。
所以成员变量定义后就有了确定的值,就可以使用。
而方法的局部变量必须自行定义而且赋值后才能使用。
(4)类成员变量在程序加载进内存时即开辟空间,实例成员变量在创建对象时开辟空间。
局部变量只在方法调用时开辟空间,方法执行完毕后空间随即释放。
4.多个方法名字相同,但参数个数或者类型不同,称为方法重载。
方法返回值的类型和参数的名字不参与比较,方法重载是多态的一种体现。
当调用方法时,根据传入的实参个数和类型,可以区分出调用的是哪一个方法。
5.构造方法与所在类的类名完全相同,构造方法没有返回值类型,也不用void修饰。
构造方法可以重载,一个类中可以定义多个构造方法。
《C面向对象程序设计答案解析》-第五章谭浩强-清华大学出版社.docx
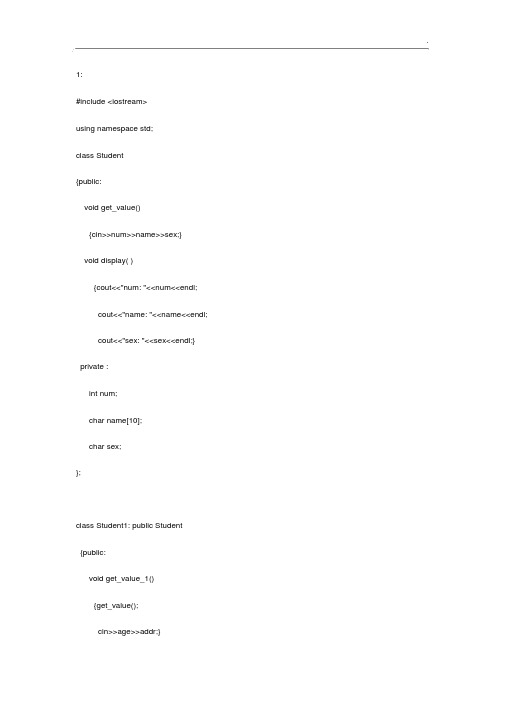
1:#include <iostream>using namespace std;class Student{public:void get_value(){cin>>num>>name>>sex;}void display( ){cout<<"num: "<<num<<endl;cout<<"name: "<<name<<endl;cout<<"sex: "<<sex<<endl;} private :int num;char name[10];char sex;};class Student1: public Student {public:void get_value_1(){get_value();cin>>age>>addr;}void display_1(){cout<<"age: "<<age<<endl;// 引用派生类的私有成员,正确。
cout<<"address: "<<addr<<endl;}// 引用派生类的私有成员,正确。
private:int age;char addr[30];};int main(){Student1 stud1;stud1.get_value_1();stud1.display();stud1.display_1();return 0;}2:#include <iostream>using namespace std;class Student{public:void get_value(){cin>>num>>name>>sex;}void display( ){cout<<"num: "<<num<<endl;cout<<"name: "<<name<<endl;cout<<"sex: "<<sex<<endl;}private :int num;char name[10];char sex;};class Student1: private Student{public:void get_value_1(){get_value();cin>>age>>addr;}void display_1(){display();cout<<"age: "<<age<<endl;// 引用派生类的私有成员,正确。
面向对象软件工程 第五章 答案

Object-Oriented Software Engineering: Using UML, Patterns, and Java: Solutions to Exercises5. Analysis: Solutions5-1Consider a file system with a graphical user interface, such as Macintosh’s Finder, Microsoft’s Windows Explorer, or Linux’s KDE. The following objects were identified from a use case describing how to copy a file from a floppy disk to a hard disk: File, Icon, TrashCan, Folder, Disk, Pointer. Specify which are entity objects, which are boundary objects, and which are control objects.Entity objects: File, Folder, DiskBoundary objects: Icon, Pointer, TrashCanControl objects: none in this example.5–2Assuming the same file system as before, consider a scenario consisting of selecting a file on a floppy, dragging it to Folder and releasing the mouse. Identify and define at least one control object associated with this scenario.The purpose of a control object is to encapsulate the behavior associated with a user level transaction. In this example, we identify a CopyFile control object, which is responsible for remembering the path of the original file, the path of the destination folder, checking if the file can be copied (access control and disk space), and to initiate the file copying.5–3Arrange the objects listed in Exercises 5-1 and 5–2 horizontally on a sequence diagram, the boundary objects to the left, then the control object you identified, and finally, the entity objects. Draw the sequence of interactions resulting from dropping the file into a folder. For now, ignore the exceptional cases.Figure 5-1 depicts a possible solution to this exercise. The names and parameters of the operations may vary. The diagram, however, should at least contain the following elements:•Two boundary objects, one for the file being copied, and one of the destination folder.•At least one control object remembering the source and destination of the copy, and possibly checking for access rights.•Two entity objects, one for the file being copied, and one of the destination folder.In this specific solution, we did not focus on the Disk, Pointer, and TrashCan objects. The Disk object would be added to the sequence when checking if there is available space. The TrashCan object is needed for scenarios in which Files or Folders are deleted.Note that the interaction among boundary objects can be complex, depending on the user interface components that are used. This sequence diagram, however, only describes user level behavior and should not go into such details. As a result, the sequence diagram depicts a high level view of the interactions between these object, not the actual sequence of message sends that occurs in the delivered system.Object-Oriented Software Engineering: Using UML, Patterns, and Java: Solutions to ExercisesFigure 5-2Sample solution for Exercise 5–45–5Identify the attributes of each object that are relevant to this scenario (copying a file from a floppy disk to a hard disk). Also consider the exception cases “There is already a file with that name in the folder” and “There is no more space on disk.”Object-Oriented Software Engineering: Using UML, Patterns, and Java: Solutions to ExercisesThe scenario implies that icons have positions (since they can be moved) and that they can be selected. Each Item, Folder or File, has a size attribute that is checked against the available space in the Disk. The exception “There is already a file with that name in the folder” implies that Item names are unique within a Folder. We represent this by adding a qualifier on the relationship between Item and Folder. Finally, when copying a file, we need to copy its contents, hence we add a contents attribute on the File object.Figure 5-4 A naive model of the Gregorian calendar (UML class diagram).5–7Consider the object model of Figure 5-32 in the book. Using association multiplicity only, can you modify the model such that a developer unfamiliar with the Gregorian calendar could deduce the number of days in each month? Identify additional classes if necessary.The purpose of this exercise is to show the limitation of association multiplicities. There is no complete solution tothis problem. A partial solution indicating the number of days in each month is depicted in Figure 5-5. We createdfour abstract classes for each of the possible month lengths, 11 classes for each of the nonvariable months and twoclasses for the month of February. The part that cannot be resolved with association multiplicities is the definition ofa leap year and that the number of days in February depends on whether the year is leap or not. In practice, thisObject-Oriented Software Engineering: Using UML, Patterns, and Java: Solutions to Exercisesproblem can solved by using informal or OCL constraints, described in more detail in Chapter 9, Object Design: Specifying Interfaces.Figure 5-5Revised class diagram indicating how many days each month includes.5–8Consider a traffic light system at a four-way crossroads (e.g., two roads intersecting at right angles). Assume the simplest algorithm for cycling through the lights (e.g., all traffic on one road is allowed to go through the crossroad while the other traffic is stopped). Identify the states of this system and draw a statechart describing them. Remember that each individual traffic light has three states (i.e. green, yellow, and red).Object-Oriented Software Engineering: Using UML, Patterns, and Java: Solutions to ExercisesWe model this system as two groups of lights, one for each road. Opposing lights have always the same value. Lights from at least one group must always be red for safety reasons. We assume there are no separate lights for left and right turns and that cycles are fixed. Figure 5-6 depicts the startchart diagram for this solution.5–10Consider the addition of a nonfunctional requirement stipulating that the effort needed by Advertisers toobtain exclusive sponsorships should be minimized. Change the AnnounceTournament and the ManageAdvertisement use cases (solution of Exercise 4-12) so that the Advertiser can specify preferences in her pro file so that exclusive sponsorships can be decided automatically by the system.Figure 5-7Sample solution for Exercise 5–5Object-Oriented Software Engineering: Using UML, Patterns, and Java: Solutions to ExercisesA new use case, ManageSponsorships , should be written and included in the ManageAdvertisement use case and de fine the preferences that the Advertiser can specify for automatically accepting an exclusive sponsorship. Figure 5-8 depicts a possible solution for this use case.In the AnnounceTournament use case, steps 6 & 7 are the modi fied as follows:6.The system noti fies the selected sponsors about the upcoming tournament and the flat fee for exclusive sponsorships. For advertisers who selected an automatic sponsorship preferences, the system automatically generates a positive answer if the fee falls within the bounds speci fied by the Advertiser.7.The system communicates their answers to the LeagueOwner .5–11Identify and write de finitions for any additional entity, boundary, and control objects participating in theAnnounceTournament use case that were introduced by realizing the change speci fied in Exercise 5-10.As speci fied above, this exercise should result in the identi fication of two new entity objects:•SponsorshipPreferences stores the time frame during which a tournament can be considered for an automatic sponsor ship and the maximum fee the Advertiser is willing to pay without being noti fied. This object also has associations to the leagues that should be considered during the automatic sponsorship decision.• ExclusiveSponsorship represents an exclusive sponsorship that was awarded to an Advertiser . It is associatedwith the tournament and Advertiser objects involved in the sponsorship. It stores a boolean indicating whether or not the sponsorship was decided automatically.However, the instructor may choose to extend the exercise so that the students also consider the objects participating in the ManageSponsorships use case. In this case, the student should identify a control object for the new use case and two boundary objects for displaying the exclusive sponsorships and the preferences, respectively.5–12Update the class diagrams of Figure 5-29 and Figure 5-31 to include the new objects you identi fied in Exercise5-11.Use case nameManageSponsorships Participating actorsInitiated by Advertiser Flow of events 1.The Advertiser requests the sponsorships area..2. ARENA displays the list of tournaments for which the Advertiserhas exclusive sponsorships and indicates for each tournamentwhether the exclusive sponsorship was decided by the system orthe Advertiser .3.The Advertiser may change the conditions under which atournament becomes a candidate for her exclusive sponsorship,including specifying a set of leagues, a time frame, or a maximumfee that she is willing to pay for an exclusive sponsorship. TheAdvertiser may also select not to use the automatic sponsorshipfeature and be noti fied about such opportunities directly.4.ARENA records the changes of preferences for the automatic sponsorships. Entry condition•The Advertiseris logged into ARENA .Exit conditions •ARENA checks the advertisers automatic sponsorship pro file for any new tournaments created after thecompletion of this use case.Figure 5-8ManageSponsorships use case.Figure 5-9 depicts the objects discussed in Exercise 5-11 and their associations.5–13The Figure 5-1 A UML startchart diagram for AnnounceTournamentControl .Create TournamentWait For Sponsor RepliesDecide on SponsorshipWait for Player Applicationsnotify sponsorship deadline expired notify players Decide on Interest Groupssponsors notify sponsors。
Java面向对象程序设计案例教程(王贺) 第五章习题答案[4页]
![Java面向对象程序设计案例教程(王贺) 第五章习题答案[4页]](https://img.taocdn.com/s3/m/a3b02cdc760bf78a6529647d27284b73f24236ea.png)
5.7 习题1. 选择题1)下列不属于面向对象编程的3个特征的是(B)A.封装B. 指针操作C. 多态D. 继承2)下列关于继承性的描述中,错误的是(C)A.一个类可以同时生成多个子类B.子类继承父类除了private修饰之外的所有成员C.Java语言支持单重继承和多重继承D.Java语言通过接口实现多重继承3)下列对于多态的描述中,错误的是(A)A.Java语言允许运算符重载B.Java语言允许方法符重载C.Java语言允许成员变量覆盖D.多态性提高了程序的抽象性和简洁性4)关键字super的作用是(D)A.用来访问父类被隐藏的成员变量B.用来调用父类中被重载的方法C.用来调用父类的构造方法D.以上都是5)下面程序定义了一个类,关于该类,说法正确的是(D)abstract class Aa{…}A.该类能调用Aa()构造方法实例化一个对象B.该类不能被继承C.该类的方法都不能被重载D.以上说法都不对6)下列关于接口的描述中,错误的是(D)A.接口实际上是由常量和抽象方法构成的特殊类B.一个类只允许继承一个接口C.定义接口使用的关键字是interfaceD.在继承接口的类中通常要给出接口中定义的抽象方法的具体实现7)下面关于包的描述中,错误的是(A)A.包是若干对象的集合B. 使用package语句创建包C. 使用import语句引入包D. 包分为有名包和无名包两种8)如果java.abc.def中包含xyz类,则该类可记作(C)A. java.xyzB. java.abc.xyzC. java.abc.def.xyzD. java.xyz.abc9)下列方法中,与方法public void add(int a){}为不合理的重载方法的是( B)A. public void add(char a)B. public int add(int a)C. public void add(int a, int b)D. public void add(float a)10)设有如下类的定义:public class parent{int change( ) {}}Class Child extends Parent{ }则下面的方法可加入Child类中的是(A)A. public int change( ){ }B. final int change(int i){ }C. private int change( ){ }D. abstract int change( ){ }2.填空题(1) 在Java程序中,把关键字(super)加到方法名称的前面,可实现子类调用父类的方法。
5、面向对象的程序设计基础

封面作者:PanHongliang仅供个人学习知识模块五面向对程序设计基础一、选择题1. ()不是构造函数的特征。
A、构造函数的函数名与类名相同;B、构造函数可以重载;C、构造函数可以设置缺省参数;D、构造函数必须指定类型说明。
2. ()是析构函数的特征。
A、析构函数可以有一个或多个参数;B、析构函数名与类名不同;C、析构函数的定义只能在类体内;D、一个类中只能定义一个析构函数; 3.要运行一个C语言编写的应用程序如下:main() {printf("hello world")。
}在VC6的开发环境中,应该建立哪种类型的工程?A, Win32 ApplicationB, Win32 Console ApplicationC, MFC AppWizard (exe)D, Utility Project4. 关于VC6建立的工程的说法,正确的是:A,工程只能包含.cpp和.h类型的文件B,工作区文件的后缀是.dspC, 用鼠标双击工作区文件可以打开整个工程D,在VC的FileView中可以看到工程中所有的文件,包括工作区文件,工程文件等5. 在VC6中提供了成员方法的自动列表和方法参数的自动提示功能,但有时不能正常显示,这是应该删除该工程目录下那种后缀类型的文件后,再次打开工程,就可以正常提示了。
A, clwB, ncbC, optD, aps6. 有关句柄(handle)的说法,下列不正确的是:A 句柄是一个4字节长的整数值B 句柄用来标识应用程序中不同的对象或同类对象中的不同实例C 消息是句柄的一种D 画笔的句柄类型是HPEN,画刷的句柄类型是HBRUSH7. C++对C语言作了很多改进,下列描述中()使得C语言发生了质变,从面向过程变成了面向对象。
A、增加了一些新的运算符;B、允许函数重载,并允许设置缺省参数;C、规定函数说明必须用原型;D、引进了类和对象的概念;8. 下列描述中,()是错误的。
Chp5 面向对象_参考答案
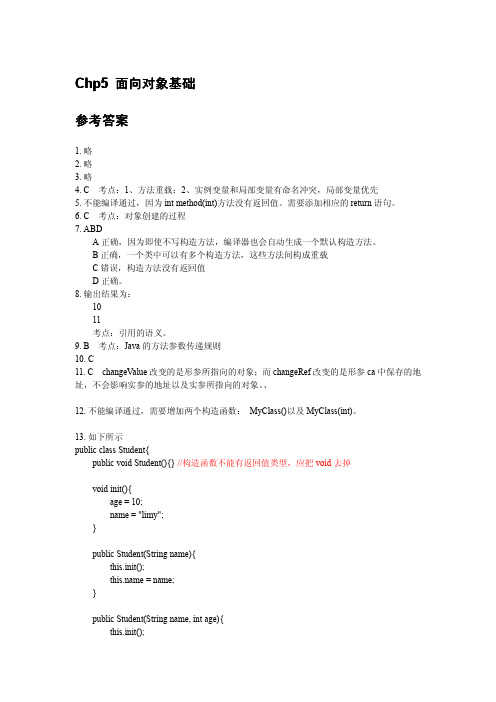
Chp5面向对象基础参考答案1.略2.略3.略4.C考点:1、方法重载;2、实例变量和局部变量有命名冲突,局部变量优先5.不能编译通过,因为int method(int)方法没有返回值。
需要添加相应的return语句。
6.C考点:对象创建的过程7.ABDA正确,因为即使不写构造方法,编译器也会自动生成一个默认构造方法。
B正确,一个类中可以有多个构造方法,这些方法间构成重载C错误,构造方法没有返回值D正确。
8.输出结果为:1011考点:引用的语义。
9.B考点:Java的方法参数传递规则10.C11.C changeValue改变的是形参所指向的对象;而changeRef改变的是形参ca中保存的地址,不会影响实参的地址以及实参所指向的对象。
、12.不能编译通过,需要增加两个构造函数:MyClass()以及MyClass(int)。
13.如下所示public class Student{public void Student(){}//构造函数不能有返回值类型,应把void去掉void init(){age=10;name="limy";}public Student(String name){this.init();=name;}public Student(String name,int age){this.init();this(name);//this()必须放在构造函数中的第一行this.age=age;}int age;String name;}14.见Ex14.java15.应当封装一个Point对象。
具体见Ex15.java16.为Point对象增加一个distance方法,用来计算两点间的距离。
具体见Ex16.java。
《C++面向对象程序设计答案》-第五章--谭浩强-清华大学出版社
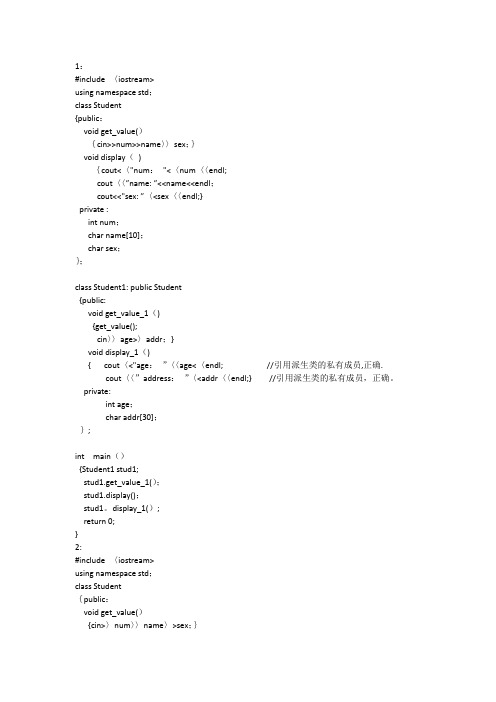
1:#include 〈iostream>using namespace std;class Student{public:void get_value(){cin>>num>>name〉〉sex;}void display(){cout<〈"num:"<〈num〈〈endl;cout〈〈”name: ”<<name<<endl;cout<<"sex: ”〈<sex〈〈endl;}private :int num;char name[10];char sex;};class Student1: public Student{public:void get_value_1(){get_value();cin〉〉age>〉addr;}void display_1(){ cout〈<"age:”〈〈age<〈endl; //引用派生类的私有成员,正确.cout〈〈”address:”〈<addr〈〈endl;} //引用派生类的私有成员,正确。
private:int age;char addr[30];};int main(){Student1 stud1;stud1.get_value_1();stud1.display();stud1。
display_1();return 0;}2:#include 〈iostream>using namespace std;class Student{public:void get_value(){cin>〉num〉〉name〉>sex;}void display(){cout〈〈”num: "〈<num<<endl;cout〈<"name:”〈〈name<〈endl;cout<<”sex: "<〈sex<<endl;}private :int num;char name[10];char sex;};class Student1: private Student{public:void get_value_1(){get_value();cin>〉age〉〉addr;}void display_1(){display();cout〈<"age:”〈〈age〈<endl;//引用派生类的私有成员,正确.cout<<"address:”<〈addr〈〈endl;}//引用派生类的私有成员,正确. private:int age;char addr[30];};int main(){Student1 stud1;stud1。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
namespace Inheritance {
class clsPoint {
private double dblX; private double dblY; public clsPoint(double _dblX, double _dblY) {
第 5 章 面向对象程序设计基础
5-9
//定义一个描述学生基本信息的类, //属性包括姓名、学号,C#、英语和数学成绩, //方法包括设置姓名和学号、设置三门课的成绩和输出相关学生的信息, //最后求出总成绩和平均成绩。
using System; using System.Collections.Generic; using System.Text;
strName = _strName; strPNo = _strPNo; strPSex = _strPSex; } public void Display() { Console.WriteLine("姓名为:" +
strName + ";编号为:" + strPNo + ";性别为:" + strPSex); } } public class clStudent : clsPerson {
5-11
//设有一个描述坐标点的 clsPoint 类, //其私有变量 x 和 y 代表一个点的 x、y 坐标值。 //编写程序实现以下功能: //利用构造函数传递参数,并设其默认参数值为 60 和 75, //利用方法 display()输出这一默认的值; //利用公有方法 setPoint()将坐标值修改为(100,120), //并利用方法输出修改后的坐标值。
public double getDance, dblDistance1, dblDistance2; dblDistance1 = Math.Pow( point1.dblX - point2.dblX,2.0); dblDistance2 = Math.Pow( point1.dblY - point2.dblY,2.0); dblDistance = Math.Sqrt(dblDistance1 + dblDistance2); return dblDistance; } } public class clsRect : clsLine { clsLine line1, line2, line3, line4; public clsRect(clsLine _line1,clsLine _line2,clsLine _line3,clsLine _line4) { line1 = _line1; line2 = _line2; line3 = _line3; line4 = _line4; }
Console.WriteLine("该生的信息为:" ); Console.WriteLine("-------------------------------------"); Console.WriteLine("姓名为:" + strName); Console.WriteLine("学号为:" + strStuNo); Console.WriteLine("C#的成绩为:" + strCSharpScore); Console.WriteLine("英语的成绩为:" + strEnglishScore); Console.WriteLine("数学的成绩为:" + strMathsScore);
}
5-12
//把定义平面直角坐标系上的一个点的类 clsPoint 作为基类, //派生出描述一条直线的类 clsLine, //再派生出一个矩形类 clsRect。 //要求方法能求出两点间的距离、矩形的周长和面积等。 //设计一个测试程序,并构造出完整的程序。
//根号下(|X1-X2|的平方+|Y1-Y2|的平方)这个就是两点间距离公式
clsTeacher teacher = new clsTeacher(); teacher.SetPersonInfo("李老师", "20000304", "男"); teacher.SetSeniority(15); teacher.Display(); teacher.DisplaySeniority(); } } }
{ strName = _strName; strStuNo = _strStuNo; strCSharpScore = _strCShapeScore; strEnglishScore = _strEnglishScore; strMathsScore = _strMathsScore;
}
public void DisplayStudentInfo() {
clsLine line1 = new clsLine(point2, point1);
clsLine line2 = new clsLine(point3, point2); clsLine line3 = new clsLine(point4, point3); clsLine line4 = new clsLine(point1, point4);
int nScore; public void SetStudentScore(int _nScore) {
nScore = _nScore; }
public void DisplayScore() {
Console.WriteLine("成绩为:" + nScore); } } public class clsTeacher : clsPerson { int nSeniority; public void SetSeniority(int _nSeniority) {
//矩形的周长:(长+宽 )乘以 2 //矩形的面积:
using System; using System.Collections.Generic; using System.Text;
namespace Inheritance {
public class clsPoint {
public double dblX; public double dblY; public clsPoint() { } public clsPoint(double _dblX, double _dblY) {
double dblArea; dblArea = line1.getDistance() * line2.getDistance(); return dblArea; } } class Test { static void Main() { clsPoint point1=new clsPoint(0.0,10.0); clsPoint point2=new clsPoint(20.0,10.0); clsPoint point3 = new clsPoint(20.0, 0); clsPoint point4 = new clsPoint(0.0, 0.0);
} } class Test {
static void Main() {
clsStudent stu = new clsStudent("李三", "0900101", 90, 89, 99); stu.DisplayStudentInfo(); } } }
5-10
//定义一个人员类 clsPerson, //包括属性:姓名、编号、性别和用于输入输出的方法。 //在此基础上派生出学生类 clsStudent(增加成绩)和 //教师类 clsTeacher(增加教龄), //并实现对学生和教师信息的输入输出。
public double getPerimeter() {
double dblPerimeter; dblPerimeter = 2 * (line1.getDistance() + line2.getDistance()); return dblPerimeter; }
public double getArea() {
nSeniority = _nSeniority; }
public void DisplaySeniority() {
Console.WriteLine("教龄为:" + nSeniority); } } public class clsTest { static void Main() {
clStudent stu = new clStudent(); stu.SetPersonInfo("张三", "20090807", "男"); stu.SetStudentScore(900); stu.Display(); stu.DisplayScore();
dblX = _dblX; dblY = _dblY; } } public class clsLine : clsPoint { clsPoint point1, point2; public clsLine() { } public clsLine(clsPoint _clsPoint1, clsPoint _clsPoint2) { point1 = _clsPoint1; point2 = _clsPoint2; }
dblX = _dblX; dblY = _dblY; } public void display() { Console.WriteLine("坐标点的 x 坐标为:" + dblX + ";y 坐标为:" + dblY); } public void setPoint(double _dblX, double _dblY) { dblX = _dblX; dblY = _dblY; } } class Test { static void Main() { clsPoint point = new clsPoint(60.00, 75.00); point.display(); point.setPoint(100.00, 120.00); point.display(); Console.Read(); } }