Chapter 11Working with Files
CFNetwork

CFNetwork Programming Guide Networking&Internet2011-06-06Apple Inc.©2011Apple Inc.All rights reserved.No part of this publication may be reproduced, stored in a retrieval system,or transmitted,in any form or by any means,mechanical, electronic,photocopying,recording,or otherwise,without prior written permission of Apple Inc.,with the following exceptions:Any person is hereby authorized to store documentation on a single computer for personal use only and to print copies of documentation for personal use provided that the documentation contains Apple’s copyright notice.The Apple logo is a trademark of Apple Inc. No licenses,express or implied,are granted with respect to any of the technology described in this document.Apple retains all intellectual property rights associated with the technology described in this document.This document is intended to assist application developers to develop applications only for Apple-labeled computers.Apple Inc.1Infinite LoopCupertino,CA95014408-996-1010Apple,the Apple logo,Bonjour,Cocoa,iChat, Keychain,Mac,Mac OS,Objective-C,and Safari are trademarks of Apple Inc.,registered in the United States and other countries.IOS is a trademark or registered trademark of Cisco in the U.S.and other countries and is used under license.UNIX is a registered trademark of The Open GroupEven though Apple has reviewed this document, APPLE MAKES NO WARRANTY OR REPRESENTATION, EITHER EXPRESS OR IMPLIED,WITH RESPECT TO THIS DOCUMENT,ITS QUALITY,ACCURACY, MERCHANTABILITY,OR FITNESS FOR A PARTICULAR PURPOSE.AS A RESULT,THIS DOCUMENT IS PROVIDED“AS IS,”AND YOU,THE READER,ARE ASSUMING THE ENTIRE RISK AS TO ITS QUALITY AND ACCURACY.IN NO EVENT WILL APPLE BE LIABLE FOR DIRECT, INDIRECT,SPECIAL,INCIDENTAL,OR CONSEQUENTIAL DAMAGES RESULTING FROM ANY DEFECT OR INACCURACY IN THIS DOCUMENT,even if advised of the possibility of such damages. THE WARRANTY AND REMEDIES SET FORTH ABOVE ARE EXCLUSIVE AND IN LIEU OF ALL OTHERS,ORAL OR WRITTEN,EXPRESS OR IMPLIED.No Apple dealer,agent,or employee is authorized to make any modification,extension,or addition to this warranty.Some states do not allow the exclusion or limitation of implied warranties or liability for incidental or consequential damages,so the above limitation or exclusion may not apply to you.This warranty gives you specific legal rights,and you may also have other rights which vary from state to state.ContentsIntroduction Introduction to CFNetwork Programming Guide7Organization of This Document7See Also8Chapter1CFNetwork Concepts9When to Use CFNetwork9CFNetwork Infrastructure10CFSocket API10CFStream API10CFNetwork API Concepts11CFFTP API11CFHTTP API12CFHTTPAuthentication API13CFHost API13CFNetServices API14CFNetDiagnostics API14Chapter2Working with Streams17Working with Read Streams17Creating a Read Stream17Reading from a Read Stream18Tearing Down a Read Stream18When all data has been read,you should call the CFReadStreamClose function to close the stream,thereby releasing system resources associated with it.Then release the stream reference bycalling the function CFRelease.You may also want to invalidate the reference by setting it toNULL.See Listing2-4for an example.18Working with Write Streams19Preventing Blocking When Working with Streams20Using a Run Loop to Prevent Blocking20Polling a Network Stream22Navigating Firewalls24Chapter3Communicating with HTTP Servers27Creating a CFHTTP Request27Creating a CFHTTP Response28Deserializing an Incoming HTTP Request28Deserializing an Incoming HTTP Response29Using a Read Stream to Serialize and Send HTTP Requests293CONTENTSSerializing and Sending an HTTP Request30Checking the Response30Handling Authentication Errors31Handling Redirection Errors31Canceling a Pending Request31Chapter4Communicating with Authenticating HTTP Servers33Handling Authentication33Keeping Credentials in Memory37Keeping Credentials in a Persistent Store38Authenticating Firewalls42Chapter5Working with FTP Servers43Downloading a File43Setting Up the FTP Streams43Implementing the Callback Function45Uploading a File46Creating a Remote Directory47Downloading a Directory Listing47Chapter6Using Network Diagnostics51Document Revision History534Figures and ListingsChapter1CFNetwork Concepts9Figure1-1CFNetwork and other software layers on Mac OS X9Figure1-2CFStream API structure11Figure1-3Network diagnostics assistant15Chapter2Working with Streams17Listing2-1Creating a read stream from a file17Listing2-2Opening a read stream17Listing2-3Reading from a read stream(blocking)18Listing2-4Releasing a read stream18Listing2-5Creating,opening,writing to,and releasing a write stream19Listing2-6Scheduling a stream on a run loop21Listing2-7Opening a nonblocking read stream21Listing2-8Network events callback function21Listing2-9Polling a read stream23Listing2-10Polling a write stream23Listing2-11Navigating a stream through a proxy server24Listing2-12Creating a handle to a dynamic store session24Listing2-13Adding a dynamic store reference to the run loop24Listing2-14Loading the proxy dictionary25Listing2-15Proxy callback function25Listing2-16Adding proxy information to a stream25Listing2-17Cleaning up proxy information25Chapter3Communicating with HTTP Servers27Listing3-1Creating an HTTP request27Listing3-2Releasing an HTTP request28Listing3-3Deserializing a message29Listing3-4Serializing an HTTP request with a read stream30Listing3-5Redirecting an HTTP stream31Chapter4Communicating with Authenticating HTTP Servers33Figure4-1Handling authentication34Figure4-2Finding an authentication object34Listing4-1Creating an authentication object35Listing4-2Finding a valid authentication object35Listing4-3Finding credentials(if necessary)and applying them365FIGURES AND LISTINGSListing4-4Applying the authentication object to a request37Listing4-5Looking for a matching authentication object38Listing4-6Searching the credentials store38Listing4-7Searching the keychain40Listing4-8Loading server credentials from the keychain40Listing4-9Modifying the keychain entry41Listing4-10Storing a new keychain entry41Chapter5Working with FTP Servers43Listing5-1A stream structure43Listing5-2Writing data to a write stream from the read stream45Listing5-3Writing data to the write stream46Listing5-4Loading data for a directory listing47Listing5-5Loading the directory listing and parsing it48Chapter6Using Network Diagnostics51Listing6-1Using the CFNetDiagnostics API when a stream error occurs51 6CFNetwork is a framework in the Core Services framework that provides a library of abstractions for network protocols.These abstractions make it easy to perform a variety of network tasks,such as:●Working with BSD sockets ●Creating encrypted connections using SSL or TLS ●Resolving DNS hosts ●Working with HTTP ,authenticating HTTP and HTTPS servers ●Working with FTP servers ●Publishing,resolving and browsing Bonjour services (described in NSNetServices and CFNetServicesProgramming Guide )This book is intended for developers who want to use network protocols in their applications.In order to fully understand this book,the reader should have a good understanding of network programming concepts such as BSD sockets,streams and HTTP protocols.Additionally,the reader should be familiar Mac OS X programming concepts including run loops.For more information about Mac OS X please read Mac OS X Technology Overview .Organization of This DocumentThis book contains the following chapters:●"CFNetwork Concepts" (page 9)describes each of the CFNetwork APIs and how they interact. ●"Working with Streams" (page 17)describes how to use the CFStream API to send and receive networkdata.●"Communicating with HTTP Servers" (page 27)describes how to send and receive HTTP messages. ●"Communicating with Authenticating HTTP Servers" (page 33)describes how to communicate with secure HTTP servers.●"Working with FTP Servers" (page 43)describes how to upload and download files from an FTP server,and how to download directory listings.●"Using Network Diagnostics" (page 51)describes how to add network diagnostics to your anization of This Document7Introduction to CFNetwork Programming GuideIntroduction to CFNetwork Programming GuideSee AlsoFor more information about the networking APIs in Mac OS X,read:●Getting Started With NetworkingRefer to the following reference documents for CFNetwork:●CFFTPStream Reference is the reference documentation for the CFFTPStream API.●CFHTTPMessage Reference is the reference documentation for the CFHTTPMessage API.●CFHTTPStream Reference is the reference documentation for the CFHTTPStream API.●CFHTTPAuthentication Reference is the reference documentation for the CFHTTPAuthentication API.●CFHost Reference is the reference documentation for the CFHost API.●CFNetServices Reference is the reference documentation for the CFNetServices API.●CFNetDiagnostics Reference is the reference documentation for the CFNetDiagnostics API.In addition to the documentation provided by Apple,the following is the reference book for socket-levelprogramming:●UNIX Network Programming,Volume1(Stevens,Fenner and Rudoff)8See AlsoCFNetwork ConceptsCFNetwork is a low-level,high-performance framework that gives you the ability to have detailed controlover the protocol stack.It is an extension to BSD sockets,the standard socket abstraction API that providesobjects to simplify tasks such as communicating with FTP and HTTP servers or resolving DNS hosts.CFNetworkis based,both physically and theoretically,on BSD sockets.Just as CFNetwork relies on BSD sockets,there are a number of Cocoa classes that rely on CFNetwork(NSURL,for example).In addition,the Web Kit is a set of Cocoa classes to display web content in windows.Both ofthese classes are very high level and implement most of the details of the networking protocols by themselves.Thus,the structure of the software layers looks like the image in Figure1-1.Figure1-1CFNetwork and other software layers on Mac OSXWhen to Use CFNetworkCFNetwork has a number of advantages over BSD sockets.It provides run-loop integration,so if yourapplication is run loop based you can use network protocols without implementing threads.CFNetwork alsocontains a number of objects to help you use network protocols without having to implement the detailsyourself.For example,you can use FTP protocols without having to implement all of the details with theCFFTP API.If you understand the networking protocols and need the low-level control they provide but don'twant to implement them yourself,then CFNetwork is probably the right choice.There are a number of advantages of using CFNetwork instead of Foundation-level networking APIs.CFNetworkis focused more on the network protocols,whereas the Foundation-level APIs are focused more on dataaccess,such as transferring data over HTTP or FTP.Although Foundation APIs do provide some configurability,CFNetwork provides a lot more.For more information on Foundation networking classes,read URL LoadingSystem Programming Guide.Now that you understand how CFNetwork interacts with the other Mac OS X networking APIs,you're readyto become familiar with the CFNetwork APIs along with two APIs that form the infrastructure for CFNetwork.When to Use CFNetwork9CFNetwork ConceptsCFNetwork InfrastructureBefore learning about the CFNetwork APIs,you must first understand the APIs which are the foundation forthe majority of CFNetwork.CFNetwork relies on two APIs that are part of the Core Foundation framework,CFSocket and CFStream.Understanding these APIs is essential to using CFNetwork.CFSocket APISockets are the most basic level of network communications.A socket acts in a similar manner to a telephonejack.It allows you to connect to another socket(either locally or over a network)and send data to that socket.The most common socket abstraction is BSD sockets.CFSocket is an abstraction for BSD sockets.With verylittle overhead,CFSocket provides almost all the functionality of BSD sockets,and it integrates the socketinto a run loop.CFSocket is not limited to stream-based sockets(for example,TCP),it can handle any typeof socket.You could create a CFSocket object from scratch using the CFSocketCreate function,or from a BSD socketusing the CFSocketCreateWithNative function.Then,you could create a run-loop source using thefunction CFSocketCreateRunLoopSource and add it to a run loop with the function CFRunLoopAddSource.This would allow your CFSocket callback function to be run whenever the CFSocket object receives a message.Read CFSocket Reference for more information about the CFSocket API.CFStream APIRead and write streams provide an easy way to exchange data to and from a variety of media in adevice-independent way.You can create streams for data located in memory,in a file,or on a network(usingsockets),and you can use streams without loading all of the data into memory at once.A stream is a sequence of bytes transmitted serially over a communications path.Streams are one-way paths,so to communicate bidirectionally an input(read)stream and output(write)stream are necessary.Exceptfor file-based streams,you cannot seek within a stream;once stream data has been provided or consumed,it cannot be retrieved again from the stream.CFStream is an API that provides an abstraction for these streams with two new CFType objects:CFReadStreamand CFWriteStream.Both types of stream follow all of the usual Core Foundation API conventions.For moreinformation about Core Foundation types,read Core Foundation Design Concepts.CFStream is built on top of CFSocket and is the foundation for CFHTTP and CFFTP.As you can see in Figure1-2,even though CFStream is not officially part of CFNetwork,it is the basis for almost all of CFNetwork. 10CFNetwork InfrastructureFigure1-2CFStream API structureFrameworkCFNetworkCore FoundationYou can use read and write streams in much the same way as you do UNIX file descriptors.First,you instantiatethe stream by specifying the stream type(memory,file,or socket)and set any options.Next,you open thestream and read or write any number of times.While the stream exists,you can get information about thestream by asking for its properties.A stream property is any information about the stream,such as its sourceor destination,that is not part of the actual data being read or written.When you no longer need the stream,close and dispose of it.CFStream functions that read or write a stream will suspend,or block,the current process until at least onebyte of the data can be read or written.To avoid trying to read from or write to a stream when the streamwould block,use the asynchronous version of the functions and schedule the stream on a run loop.Yourcallback function is called when it is possible to read and write without blocking.In addition,CFStream has built-in support for the Secure Sockets Layer(SSL)protocol.You can set up adictionary containing the stream's SSL information,such as the security level desired or self-signed certificates.Then pass it to your stream as the kCFStreamPropertySSLSettings property to make the stream an SSLstream.The chapter"Working with Streams" (page 17)describes how to use read and write streams. CFNetwork API ConceptsTo understand the CFNetwork framework,you need to be familiar with the building blocks that compose it.The CFNetwork framework is broken up into separate APIs,each covering a specific network protocol.TheseAPIs can be used in combination,or separately,depending on your application.Most of the programmingconventions are common among the APIs,so it's important to comprehend each of them.CFFTP APICommunicating with an FTP server is made easier with ing the CFFTP API,you can create FTP readstreams(for downloading)and FTP write streams(for uploading).Using FTP read and write streams you canperform functions such as:●Download a file from an FTP server●Upload a file to an FTP serverCFNetwork API Concepts112011-06-06 | © 2011 Apple Inc. All Rights Reserved.●Download a directory listing from an FTP server●Create directories on an FTP serverAn FTP stream works like all other CFNetwork streams.For example,you can create an FTP read stream bycalling the function CFReadStreamCreateWithFTPURL function.Then,you can call the functionCFReadStreamGetError at any time to check the status of the stream.By setting properties on FTP streams,you can adapt your stream for its particular application.For example,if the server that the stream is connecting to requires a user name and password,you need to set theappropriate properties so the stream can work properly.For more information about the different propertiesavailable to FTP streams read"Setting up the Streams" (page 43).A CFFTP stream can be used synchronously or asynchronously.To open the connection with the FTP serverthat was specified when the FTP read stream was created,call the function CFReadStreamOpen.To readfrom the stream,use the CFReadStreamRead function and provide the read stream reference,CFReadStreamRef,that was returned when the FTP read stream was created.The CFReadStreamReadfunction fills a buffer with the output from the FTP server.For more information on using CFFTP,see"Working with FTP Servers" (page 43).CFHTTP APITo send and receive HTTP messages,use the CFHTTP API.Just as CFFTP is an abstraction for FTP protocols,CFHTTP is an abstraction for HTTP protocols.Hypertext Transfer Protocol(HTTP)is a request/response protocol between a client and a server.The clientcreates a request message.This message is then serialized,a process that converts the message into a rawbyte stream.Messages cannot be transmitted until they are serialized first.Then the request message is sentto the server.The request typically asks for a file,such as a webpage.The server responds,sending back astring followed by a message.This process is repeated as many times as is necessary.To create an HTTP request message,you specify the following:●The request method,which can be one of the request methods defined by the Hypertext TransferProtocol,such as OPTIONS,GET,HEAD,POST,PUT,DELETE,TRACE,and CONNECT●The URL,such as ●The HTTP version,such as version1.0or1.1●The message’s headers,by specifying the header name,such as User-Agent,and its value,such asMyUserAgent●The message’s bodyAfter the message has been constructed,you serialize it.Following serialization,a request might look likethis:GET / HTTP/1.0\r\nUser-Agent: UserAgent\r\nContent-Length: 0\r\n\r\n12CFNetwork API Concepts2011-06-06 | © 2011 Apple Inc. All Rights Reserved.Deserialization is the opposite of serialization.With deserialization,a raw byte stream received from a client or server is restored to its native representation.CFNetwork provides all of the functions needed to get the message type(request or response),HTTP version,URL,headers,and body from an incoming,serialized message.More examples of using CFHTTP are available in"Communicating with HTTP Servers" (page 27). CFHTTPAuthentication APIIf you send an HTTP request to an authentication server without credentials(or with incorrect credentials), the server returns an authorization challenge(more commonly known as a401or407response).The CFHTTPAuthentication API applies authentication credentials to challenged HTTP messages. CFHTTPAuthentication supports the following authentication schemes:●Basic●Digest●NT LAN Manager(NTLM)●Simple and Protected GSS-API Negotiation Mechanism(SPNEGO)New in Mac OS X v10.4is the ability to carry persistency across requests.In Mac OS X v10.3each time a request was challenged,you had to start the authentication dialog from scratch.Now,you maintain a set of CFHTTPAuthentication objects for each server.When you receive a401or407response,you find the correct object and credentials for that server and apply them.CFNetwork uses the information stored in that object to process the request as efficiently as possible.By carrying persistency across request,this new version of CFHTTPAuthentication provides much better performance.More information about how to use CFHTTPAuthentication is available in"Communicating with Authenticating HTTP Servers" (page 33).CFHost APIYou use the CFHost API to acquire host information,including names,addresses,and reachability information. The process of acquiring information about a host is known as resolution.CFHost is used just like CFStream:●Create a CFHost object.●Start resolving the CFHost object.●Retrieve either the addresses,host names,or reachability information.●Destroy the CFHost object when you are done with it.Like all of CFNetwork,CFHost is IPv4and ing CFHost,you could write code that handles IPv4and IPv6completely transparently.CFNetwork API Concepts13 2011-06-06 | © 2011 Apple Inc. All Rights Reserved.CFHost is integrated closely with the rest of CFNetwork.For example,there is a CFStream function calledCFStreamCreatePairWithSocketToCFHost that will create a CFStream object directly from a CFHostobject.For more information about the CFHost object functions,see CFHost Reference.CFNetServices APIIf you want your application to use Bonjour to register a service or to discover services,use the CFNetServicesAPI.Bonjour is Apple's implementation of zero-configuration networking(ZEROCONF),which allows you topublish,discover,and resolve network services.To implement Bonjour the CFNetServices API defines three object types:CFNetService,CFNetServiceBrowser,and CFNetServiceMonitor.A CFNetService object represents a single network service,such as a printer or afile server.It contains all the information needed for another computer to resolve that server,such as name,type,domain and port number.A CFNetServiceBrowser is an object used to discover domains and networkservices within domains.And a CFNetServiceMonitor object is used to monitor a CFNetService object forchanges,such as a status message in iChat.For a full description of Bonjour,see Bonjour Overview.For more information about using CFNetServices andimplementing Bonjour,see NSNetServices and CFNetServices Programming Guide.CFNetDiagnostics APIApplications that connect to networks depend on a stable connection.If the network goes down,this causesproblems with the application.By adopting the CFNetDiagnostics API,the user can self-diagnose networkissues such as:●Physical connection failures(for example,a cable is unplugged)●Network failures(for example,DNS or DHCP server no longer responds)●Configuration failures(for example,the proxy configuration is incorrect)Once the network failure has been diagnosed,CFNetDiagnostics guides the user to fix the problem.You mayhave seen CFNetDiagnostics in action if Safari failed to connect to a website.The CFNetDiagnostics assistantcan be seen in Figure1-3.14CFNetwork API Concepts2011-06-06 | © 2011 Apple Inc. All Rights Reserved.Figure1-3Network diagnostics assistantBy providing CFNetDiagnostics with the context of the network failure,you can call the CFNetDiagnosticDiagnoseProblemInteractively function to lead the user through the prompts to find a solution.Additionally,you can use CFNetDiagnostics to query for connectivity status and provide uniform error messages to the user.To see how to integrate CFNetDiagnotics into your application read"Using Network Diagnostics" (page 51). CFNetDiagnostics is a new API in Mac OS X v10.4.CFNetwork API Concepts15 2011-06-06 | © 2011 Apple Inc. All Rights Reserved.16CFNetwork API Concepts2011-06-06 | © 2011 Apple Inc. All Rights Reserved.Working with StreamsThis chapter discusses how to create,open,and check for errors on read and write streams.It also describeshow to read from a read stream,how to write to a write stream,how to prevent blocking when reading fromor writing to a stream,and how to navigate a stream through a proxy server.Working with Read StreamsCore Foundation streams can be used for reading or writing files or working with network sockets.With theexception of the process of creating those streams,they behave similarly.Creating a Read StreamStart by creating a read stream.Listing2-1creates a read stream for a file.Listing2-1Creating a read stream from a fileCFReadStreamRef myReadStream = CFReadStreamCreateWithFile(kCFAllocatorDefault,fileURL);In this listing,the kCFAllocatorDefault parameter specifies that the current default system allocator beused to allocate memory for the stream and the fileURL parameter specifies the name of the file for whichthis read stream is being created,such as file:///Users/joeuser/Downloads/MyApp.sit.Similarly,you can create a pair of streams based on a network service by callingCFStreamCreatePairWithSocketToCFHost(described in“Using a Run Loop to Prevent Blocking” (page20))or CFStreamCreatePairWithSocketToNetService(described in NSNetServices and CFNetServicesProgramming Guide).Now that you have created the stream,you can open it.Opening a stream causes the stream to reserve anysystem resources that it requires,such as the file descriptor needed to open the file.Listing2-2is an exampleof opening the read stream.Listing2-2Opening a read streamif (!CFReadStreamOpen(myReadStream)) {CFStreamError myErr = CFReadStreamGetError(myReadStream);// An error has occurred.if (myErr.domain == kCFStreamErrorDomainPOSIX) {// Interpret myErr.error as a UNIX errno.} else if (myErr.domain == kCFStreamErrorDomainMacOSStatus) {// Interpret myErr.error as a MacOS error code.OSStatus macError = (OSStatus)myErr.error;// Check other error domains.}Working with Read Streams172011-06-06 | © 2011 Apple Inc. All Rights Reserved.}The CFReadStreamOpen function returns TRUE to indicate success and FALSE if the open fails for any reason.If CFReadStreamOpen returns FALSE,the example calls the CFReadStreamGetError function,whichreturns a structure of type CFStreamError consisting of two values:a domain code and an error code.Thedomain code indicates how the error code should be interpreted.For example,if the domain code iskCFStreamErrorDomainPOSIX,the error code is a UNIX errno value.The other error domains arekCFStreamErrorDomainMacOSStatus,which indicates that the error code is an OSStatus value definedin MacErrors.h,and kCFStreamErrorDomainHTTP,which indicates that the error code is the one of thevalues defined by the CFStreamErrorHTTP enumeration.Opening a stream can be a lengthy process,so the CFReadStreamOpen and CFWriteStreamOpen functionsavoid blocking by returning TRUE to indicate that the process of opening the stream has begun.To checkthe status of the open,call the functions CFReadStreamGetStatus and CFWriteStreamGetStatus,whichreturn kCFStreamStatusOpening if the open is still in progress,kCFStreamStatusOpen if the open iscomplete,or kCFStreamStatusErrorOccurred if the open has completed but failed.In most cases,itdoesn’t matter whether the open is complete because the CFStream functions that read and write will blockuntil the stream is open.Reading from a Read StreamTo read from a read stream,call the function CFReadStreamRead,which is similar to the UNIX read()system call.Both take buffer and buffer length parameters.Both return the number of bytes read,0if at theend of stream or file,or-1if an error occurred.Both block until at least one byte can be read,and bothcontinue reading as long as they can do so without blocking.Listing2-3is an example of reading from theread stream.Listing2-3Reading from a read stream(blocking)CFIndex numBytesRead;do {UInt8 buf[myReadBufferSize]; // define myReadBufferSize as desirednumBytesRead = CFReadStreamRead(myReadStream, buf, sizeof(buf));if( numBytesRead > 0 ) {handleBytes(buf, numBytesRead);} else if( numBytesRead < 0 ) {CFStreamError error = CFReadStreamGetError(myReadStream);reportError(error);}} while( numBytesRead > 0 );Tearing Down a Read StreamWhen all data has been read,you should call the CFReadStreamClose function to close the stream,therebyreleasing system resources associated with it.Then release the stream reference by calling the functionCFRelease.You may also want to invalidate the reference by setting it to NULL.See Listing2-4for anexample.Listing2-4Releasing a read streamCFReadStreamClose(myReadStream);CFRelease(myReadStream);18Working with Read Streams2011-06-06 | © 2011 Apple Inc. All Rights Reserved.。
胡壮麟《语言学教程》第十一章Linguistics_and_foreign_language_teaching
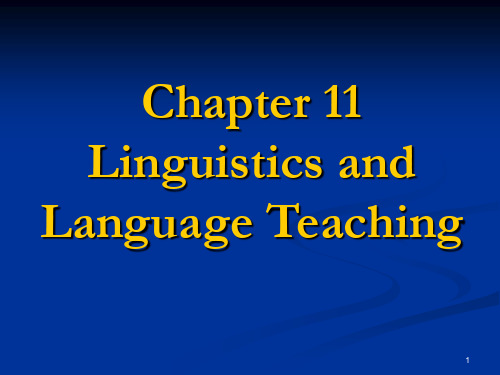
2
1.1 Grammar
Do we teach grammar? How do we teach grammar?
As a compromise between the “purely formfocused approaches‖ and the ―purely meaningfocused‖ approaches, a recent movement called focus on form seems to take a more balanced view on the role of grammar in language learning.
15
Communicative competence
Dell Hymes
What a learners knows about how a language is used in particular situations for effective and appropriate communication. Includes knowledge of the grammar and vocabulary, knowledge of rules of speaking, knowledge of how to use and respond to different types of speech acts and social conventions, and knowledge of how to use language appropriately.
16
It is believed that language learning will successfully take place when language learners know how and when to use the language in various settings and when they have successfully cognized various forms of competence such as grammatical competence (lexis, morphology, syntax and phonology) and pragmatic competence (e.g., speech acts).
Stata 8 用户手册说明书

THE STATA NEWSSTATA Volume 18 Number 3 July/August/September 2003New features for Stata 8P . 1New books from Stata Press P . 2Latest NetCourse schedule P . 3Inside this issue:FDA file format now supported 1Time-series graphs in Stata 8 1Multiple-language dataset support 1New books from Stata Press 2 From the Stata Bookstore 3Latest NetCourse schedule 3FDA file format now supportedStata 8 can now read and write files in the format required for submissions to the U.S. Food and Drug Administration (FDA)–SAS XPORT format. Two new commands provide this ability: fdasave and fdause . The primary intent of these commands is to assist people in making submissions to the FDA, but the commands are general enough to use in transferring data between SAS and Stata.These new commands are included in the latest free update to Stata 8, which you obtain by launching Stata, typing update , and following the instructions. You can access online documentation after updating, by typing whelp fdasave .fdasave saves the data in the FDA’s official format for submittingand archiving new drug and device applications (NDAs). To format your Stata dataset for submission, type .fdasave filenameStata creates an FDA format file filename .xpt containing the data. If the data includes value labels, Stata automatically creates an additional FDA format file, formats.xpf , containing the value-label definitions. These files are in exactly the format expected by the FDA and can easily be read into Stata or into SAS Statistical Software (SAS Institute Inc., Cary, North Carolina).fdause reads FDA format files into Stata. You type.fdause filenameStata reads the FDA format file filename .xpt , and if the file formats.xpf also exists, Stata also reads the value-label definitions.For more information about FDA NDA submissions, see /cder/guidance/2867fnl.pdf .Time-series graphs in Stata 8Stata 8 has new features for the graphical display of time-series data. Stata’s graphics engine has an enhanced time axis and associated options that allow you to specify date strings instead of numeric values. The latest update also contains new time-series plottypes, tsline and tsrline , and a graph command for plotting panel data, xtline .When you tsset your data and identify the scale of the time variable (e.g., daily , weekly , monthly , …), Stata creates a time axis when plotting variables over time. You can now specify date strings when adding tick marks (both major and minor, labeled and unlabeled), vertical lines, and text boxes.tsline and tsrline automatically identify the time variable set by tsset . tsline generates line plots of one or more time series, and tsrline generates range plots with lines. These two plottypes can be combined; for example, you can plot a forecast with error bounds.. tsset time, daily. tsline ypred y || tsrline ll ul || in -60/l , ytitle(“”)tlabel(minmax 15apr2003) tline(15apr2003)���������������������������������������������������������������������������xtline automatically identifies the time and panel variables set by tsset . By default, xtline plots several variables versus time for each panel separately. Optionally, xtline can also produce a singlegraph with line plots overlaid by panel.You can obtain online documentation after updating by typing whelp tsline , whelp xtline , and whelp twoway_options .Multiple-language dataset supportJust added to Stata 8 is label language, which allows you to create datasets that contain data, variable, and value labels in different languages. A dataset might contain one set of labels in English, another in German, and a third in Spanish. Or, a dataset might contain labels all in the same language, one set of long labels and another set of shorter ones. A dataset may contain up to 100 sets of labels.This new capability is available for free to all Stata 8 users–just launch Stata, type update , and follow the instructions.Regression Models for Categorical DependentVariables Using Stata, Revised EditionWhile regression models ubiquitous, a discussion of how to interpret these models has been sorely lacking. Regression Models for Categorical Dependent Variables Using Stata, Revised Edition void. This book discusses how to fit and interpret regression models for categorical data with Stata and includes some commands written by the authors. Hypothesis testing and goodness-of-fit statistics are also discussed.Copyright: An Introduction Stata, Revised EditionAn Introduction to Survival Analysis Using Stata, Revised Edition is the ideal tutorial for professional data analysts who want to learn survival analysis for the first time or who are well versed in survival analysis but not as dexterous in using Stata to analyze survival data. This text also serves as a valuable reference to those who already have experience using Stata’s survival analysis routines.Survival analysis is a field of its own requiring specialized data man-Common Errors in StatisticsCommon Errors in Statistics contains common-sense, minimally technical advice on how to improve experimental design, analysis of data, and presentation of results. It provides a guide for experienced scientists as well as students learning to design and complete experiments and statistical analysis.The text begins with a section on foundations that covers sources of error, hypotheses, and data collection. The second section, on hypothesis testing and parameter estimation, takes a harder look at statistical evaluation of the data, including strengths and limitations of various statistical procedures, and guidelines for reporting results from what information to include to how to create an informative Essential Medical Statistics, 2d edEssential Medical Statistics gives an excellent overview of the basics of medical statistics and achieves a good balance contingency tables, and regression. As personal computers become more powerful, the use of complex models in leading medical journals is becoming more prevalent, and the second edition of this text follows this shift by focusing more on these models. A very nice section on basic meta-analysis is also included.Covered in the text are the basics of normal-based tests and inference, including linear regression, contingency tables and logistic regression, Copyright: Survival Analysis: Techniques for Censored and Truncated Data, 2d edSurvival Analysis: Techniques for Censored and Truncated Data John Klein and Melvin Moeschberger is an essential reference for any researcher using techniques of survival analysis. Ideal for self-study or for a two-term graduate sequence in survival analysis, this book maintains a technical level suited for both the medical researcher and the professional statistician. Most impressive are the number and scope of real-data examples that are presented in the first chapter and used subsequently throughout the text.Copyright:4HOW TO REACH USStata Corporation PHONE 979-696-46004905 Lakeway Drive FAX 979-696-4601College Station TX 77845 EMAIL stata@ USA WEB Please include your Stata serial number with all correspondence.THE STATA NEWS is published four times a year and is free to all registered users of Stata.I N T E R N A T I O N A L D I S T R I B U T O R SChips ElectronicsServing Brunei, Indonesia, Malaysia, Singapore tel: 62 - 21 - 452 17 61 email: puyuh23@.id Cosinus Computing BV Serving The Netherlands tel: +31 416-378 125 email: info@cosinus.nl Dittrich & Partner Consulting Serving Austria, Czech Republic, Germany, Hungary, Poland tel: +49 2 12 / 26 066 - 0 email: sales@dpc.de Ixon Technology Company Ltd Serving Taiwan tel: +886-(0)2-27045535 email: hank@ MercoStat Consultores Serving Argentina, Brazil, Paraguay, Uruguay tel: 598-2-613-7905email: mercost@.uy Metrika ConsultingServing the Baltic States, Denmark, Finland, Iceland, Norway, Sweden tel: +46-708-163128 email: sales@metrika.se MultiON Consulting S.A. de C.V. Serving Belize, Costa Rica, El Salvador, Guatemala, Honduras, Mexico, Nicaragua, Panama tel: 52 (55) 55 59 40 50 email: info@.mxRitme InformatiqueServing Belgium, France, Luxembourg tel: +33 (0)1 42 46 00 42 email: info@ Scientific Solutions S.A. Serving Switzerland tel: 41 (0)21 711 15 20email: info@scientific-solutions.ch Survey Design & Analysis Services Serving Australia, New Zealand tel: +61 (0)3 9878 7373 email: sales@.au Timberlake Consultants Serving Eire, U.K. tel: +44 (0)208 697 3377 email: info@ Timberlake Consulting S.L. Serving Spaintel: +34 (9) 5 560 14 30 email:timberlake@ Timberlake Consultores, Lda. Serving Portugal tel: +351 214 702 869 email:timberlake.co@mail.telepac.pt TStat S.r.l.Serving Italytel: +39 0864 210101email: tstat@tstat.it Vishvas Marketing-Mix Services Serving India tel: 91-22-25892639email: bandya@I N T E R N A T I O N A L R E S E L L E R SCreActive snc Serving Italy tel: +39 0575 333297 email: staff@ IEMServing Botswana, Lesotho,Mozambique, Namibia,South Africa, Swaziland, Zimbabwe tel: +27-11-8286169email: instruments@mweb.co.za Informatique Inc Serving Japan tel: +81-3-3505-1250email: sales@informatiq.co.jpSOFTWARE shop IncServing Bolivia, Chile, Colombia,Ecuador, Peru, Venezuela tel: 425-651-4090email: ventas@ Timberlake Consultores Brasil Serving Braziltel: +55-11-3263-1287 email: info@.br Timberlake Consultants Polska Serving Poland tel: +48 600 370 435 email: info@timberlake.plNC-101 is designed to take smart, knowledgeable people and turn theminto proficient interactive users of Stata. The course covers not just the obvious, such as getting data into Stata, but also covers detailed techniques and tricks to make you a powerful Stata user. From web update features and match-merging to using by groups and explicit subscripting, many of Stata’s key concepts are explored.NC-151 is intended for all Stata users. Through a combination of lectures, example applications, and carefully chosen exercises, the course addresses the full range of methods and techniques you will need to be most productive in the Stata environment. Beginning with effective ways to organize both simple and complicated analyses in Stata, NetCourse 151 moves into programming elements that can be used to work more efficiently. Key programming topics include macro processing, program flow of control, using do-files, programming ado-files, Monte Carlo simulations, and bootstrapped standard errors.From the Stata Bookstore Introduction to StataPrerequisites Stata 8Dates offered October 17 – November 28Course LeadersJames Hassell, Allen McDowell, and Derek WagnerEnrollment DeadlinesOctober 13Price $95From the Stata Bookstore Introduction to Stata programmingPrerequisitesStata 8; basic knowledge of using Stata interactivelyDates offered October 17 – November 28Course LeadersAllen McDowell and Kevin CrowEnrollment DeadlinesOctober 13Price $125。
C++ Primer英文版(第5版)

C++ Primer英文版(第5版)《C++ Primer英文版(第5版)》基本信息作者: (美)李普曼(Lippman,S.B.) (美)拉乔伊(Lajoie,J.) (美)默Moo,B.E.) 出版社:电子工业出版社ISBN:9787121200380上架时间:2013-4-23出版日期:2013 年5月开本:16开页码:964版次:5-1所属分类:计算机 > 软件与程序设计 > C++ > C++内容简介计算机书籍这本久负盛名的C++经典教程,时隔八年之久,终迎来史无前例的重大升级。
除令全球无数程序员从中受益,甚至为之迷醉的——C++大师Stanley B. Lippman的丰富实践经验,C++标准委员会原负责人Josée Lajoie对C++标准的深入理解,以及C++先驱Barbara E. Moo 在C++教学方面的真知灼见外,更是基于全新的C++11标准进行了全面而彻底的内容更新。
非常难能可贵的是,《C++ Primer英文版(第5版)》所有示例均全部采用C++11标准改写,这在经典升级版中极其罕见——充分体现了C++语言的重大进展极其全面实践。
书中丰富的教学辅助内容、醒目的知识点提示,以及精心组织的编程示范,让这本书在C++领域的权威地位更加不可动摇。
无论是初学者入门,或是中、高级程序员提升,本书均为不容置疑的首选。
目录《c++ primer英文版(第5版)》prefacechapter 1 getting started 11.1 writing a simple c++program 21.1.1 compiling and executing our program 31.2 afirstlookat input/output 51.3 awordaboutcomments 91.4 flowofcontrol 111.4.1 the whilestatement 111.4.2 the forstatement 131.4.3 readinganunknownnumberof inputs 141.4.4 the ifstatement 171.5 introducingclasses 191.5.1 the sales_itemclass 201.5.2 afirstlookatmemberfunctions 231.6 thebookstoreprogram. 24chaptersummary 26definedterms 26part i the basics 29chapter 2 variables and basic types 312.1 primitivebuilt-intypes 322.1.1 arithmetictypes 322.1.2 typeconversions 352.1.3 literals 382.2 variables 412.2.1 variabledefinitions 412.2.2 variabledeclarations anddefinitions 44 2.2.3 identifiers 462.2.4 scopeof aname 482.3 compoundtypes 502.3.1 references 502.3.2 pointers 522.3.3 understandingcompoundtypedeclarations 57 2.4 constqualifier 592.4.1 references to const 612.4.2 pointers and const 622.4.3 top-level const 632.4.4 constexprandconstantexpressions 652.5 dealingwithtypes 672.5.1 typealiases 672.5.2 the autotypespecifier 682.5.3 the decltypetypespecifier 702.6 definingourowndatastructures 722.6.1 defining the sales_datatype 722.6.2 using the sales_dataclass 742.6.3 writing our own header files 76 chaptersummary 78definedterms 78chapter 3 strings, vectors, and arrays 813.1 namespace usingdeclarations 823.2 library stringtype 843.2.1 defining and initializing strings 843.2.2 operations on strings 853.2.3 dealing with the characters in a string 90 3.3 library vectortype 963.3.1 defining and initializing vectors 973.3.2 adding elements to a vector 1003.3.3 other vectoroperations 1023.4 introducingiterators 1063.4.1 usingiterators 1063.4.2 iteratorarithmetic 1113.5 arrays 1133.5.1 definingandinitializingbuilt-inarrays 113 3.5.2 accessingtheelementsof anarray 1163.5.3 pointers andarrays 1173.5.4 c-stylecharacterstrings 1223.5.5 interfacingtooldercode 1243.6 multidimensionalarrays 125chaptersummary 131definedterms 131chapter 4 expressions 1334.1 fundamentals 1344.1.1 basicconcepts 1344.1.2 precedenceandassociativity 1364.1.3 orderofevaluation 1374.2 arithmeticoperators 1394.3 logical andrelationaloperators 1414.4 assignmentoperators 1444.5 increment anddecrementoperators 1474.6 thememberaccessoperators 1504.7 theconditionaloperator 1514.8 thebitwiseoperators 1524.9 the sizeofoperator 1564.10 commaoperator 1574.11 typeconversions 1594.11.1 thearithmeticconversions 1594.11.2 other implicitconversions 1614.11.3 explicitconversions 1624.12 operatorprecedencetable 166 chaptersummary 168definedterms 168chapter 5 statements 1715.1 simple statements 1725.2 statementscope 1745.3 conditional statements 1745.3.1 the ifstatement 1755.3.2 the switchstatement 1785.4 iterativestatements 1835.4.1 the whilestatement 1835.4.2 traditional forstatement 1855.4.3 range forstatement 1875.4.4 the do whilestatement 1895.5 jumpstatements 1905.5.1 the breakstatement 1905.5.2 the continuestatement 1915.5.3 the gotostatement 1925.6 tryblocks andexceptionhandling 1935.6.1 a throwexpression 1935.6.2 the tryblock 1945.6.3 standardexceptions 197 chaptersummary 199definedterms 199chapter 6 functions 2016.1 functionbasics 2026.1.1 localobjects 2046.1.2 functiondeclarations 2066.1.3 separatecompilation 2076.2 argumentpassing 2086.2.1 passingargumentsbyvalue 2096.2.2 passingargumentsbyreference 2106.2.3 constparametersandarguments 2126.2.4 arrayparameters 2146.2.5 main:handlingcommand-lineoptions 218 6.2.6 functionswithvaryingparameters 2206.3 return types and the returnstatement 222 6.3.1 functionswithnoreturnvalue 2236.3.2 functionsthatreturnavalue 2236.3.3 returningapointer toanarray 2286.4 overloadedfunctions 2306.4.1 overloadingandscope 2346.5 features forspecializeduses 2366.5.1 defaultarguments 2366.5.2 inline and constexprfunctions 2386.5.3 aids for debugging 2406.6 functionmatching 2426.6.1 argumenttypeconversions 2456.7 pointers tofunctions 247 chaptersummary 251definedterms 251chapter 7 classes 2537.1 definingabstractdatatypes 2547.1.1 designing the sales_dataclass 2547.1.2 defining the revised sales_dataclass 256 7.1.3 definingnonmemberclass-relatedfunctions 260 7.1.4 constructors 2627.1.5 copy,assignment, anddestruction 2677.2 accesscontrol andencapsulation 2687.2.1 friends 2697.3 additionalclassfeatures 2717.3.1 classmembersrevisited 2717.3.2 functions that return *this 2757.3.3 classtypes 2777.3.4 friendshiprevisited 2797.4 classscope 2827.4.1 namelookupandclassscope 2837.5 constructorsrevisited 2887.5.1 constructor initializerlist 2887.5.2 delegatingconstructors 2917.5.3 theroleof thedefaultconstructor 2937.5.4 implicitclass-typeconversions 2947.5.5 aggregateclasses 2987.5.6 literalclasses 2997.6 staticclassmembers 300chaptersummary 305definedterms 305contents xipart ii the c++ library 307chapter 8 the io library 3098.1 the ioclasses 3108.1.1 nocopyorassignfor ioobjects 3118.1.2 conditionstates 3128.1.3 managingtheoutputbuffer 3148.2 file input and output 3168.2.1 using file stream objects 3178.2.2 file modes 3198.3 stringstreams 3218.3.1 using an istringstream 3218.3.2 using ostringstreams 323chaptersummary 324definedterms 324chapter 9 sequential containers 3259.1 overviewof the sequentialcontainers 3269.2 containerlibraryoverview 3289.2.1 iterators 3319.2.2 containertypemembers 3329.2.3 begin and endmembers 3339.2.4 definingandinitializingacontainer 3349.2.5 assignment and swap 3379.2.6 containersizeoperations 3409.2.7 relationaloperators 3409.3 sequentialcontaineroperations 3419.3.1 addingelements toasequentialcontainer 3419.3.2 accessingelements 3469.3.3 erasingelements 3489.3.4 specialized forward_listoperations 3509.3.5 resizingacontainer 3529.3.6 containeroperationsmayinvalidateiterators 353 9.4 how a vectorgrows 3559.5 additional stringoperations 3609.5.1 other ways to construct strings 3609.5.2 other ways to change a string 3619.5.3 stringsearchoperations 3649.5.4 the comparefunctions 3669.5.5 numericconversions 3679.6 containeradaptors 368chaptersummary 372definedterms 372chapter 10 generic algorithms 37510.1 overview. 37610.2 afirstlookat thealgorithms 37810.2.1 read-onlyalgorithms 37910.2.2 algorithmsthatwritecontainerelements 380 10.2.3 algorithmsthatreordercontainerelements 383 10.3 customizingoperations 38510.3.1 passingafunctiontoanalgorithm 38610.3.2 lambdaexpressions 38710.3.3 lambdacapturesandreturns 39210.3.4 bindingarguments 39710.4 revisiting iterators 40110.4.1 insert iterators 40110.4.2 iostream iterators 40310.4.3 reverse iterators 40710.5 structureofgenericalgorithms 41010.5.1 thefive iteratorcategories 41010.5.2 algorithmparameterpatterns 41210.5.3 algorithmnamingconventions 41310.6 container-specificalgorithms 415 chaptersummary 417definedterms 417chapter 11 associative containers 41911.1 usinganassociativecontainer 42011.2 overviewof theassociativecontainers 423 11.2.1 defininganassociativecontainer 423 11.2.2 requirements onkeytype 42411.2.3 the pairtype 42611.3 operations onassociativecontainers 428 11.3.1 associativecontainer iterators 429 11.3.2 addingelements 43111.3.3 erasingelements 43411.3.4 subscripting a map 43511.3.5 accessingelements 43611.3.6 awordtransformationmap 44011.4 theunorderedcontainers 443 chaptersummary 447definedterms 447chapter 12 dynamicmemory 44912.1 dynamicmemoryandsmartpointers 45012.1.1 the shared_ptrclass 45012.1.2 managingmemorydirectly 45812.1.3 using shared_ptrs with new 46412.1.4 smartpointers andexceptions 46712.1.5 unique_ptr 47012.1.6 weak_ptr 47312.2 dynamicarrays 47612.2.1 newandarrays 47712.2.2 the allocatorclass 48112.3 usingthelibrary:atext-queryprogram 484 12.3.1 designof thequeryprogram 48512.3.2 definingthequeryprogramclasses 487 chaptersummary 491definedterms 491part iii tools for class authors 493chapter 13 copy control 49513.1 copy,assign, anddestroy 49613.1.1 thecopyconstructor 49613.1.2 thecopy-assignmentoperator 50013.1.3 thedestructor 50113.1.4 theruleofthree/five 50313.1.5 using = default 50613.1.6 preventingcopies 50713.2 copycontrol andresourcemanagement 51013.2.1 classesthatactlikevalues 51113.2.2 definingclassesthatactlikepointers 51313.3 swap 51613.4 acopy-controlexample 51913.5 classesthatmanagedynamicmemory 52413.6 movingobjects 53113.6.1 rvaluereferences 53213.6.2 moveconstructor andmoveassignment 53413.6.3 rvaluereferencesandmemberfunctions 544 chaptersummary 549definedterms 549chapter 14 overloaded operations and conversions 551 14.1 basicconcepts 55214.2 input andoutputoperators 55614.2.1 overloading the output operator [[55714.2.2 overloading the input operator ]]. 55814.3 arithmetic andrelationaloperators 56014.3.1 equalityoperators 56114.3.2 relationaloperators 56214.4 assignmentoperators 56314.5 subscriptoperator 56414.6 increment anddecrementoperators 56614.7 memberaccessoperators 56914.8 function-calloperator 57114.8.1 lambdasarefunctionobjects 57214.8.2 library-definedfunctionobjects 57414.8.3 callable objects and function 57614.9 overloading,conversions, andoperators 57914.9.1 conversionoperators 58014.9.2 avoidingambiguousconversions 58314.9.3 functionmatchingandoverloadedoperators 587 chaptersummary 590definedterms 590chapter 15 object-oriented programming 59115.1 oop:anoverview 59215.2 definingbaseandderivedclasses 59415.2.1 definingabaseclass 59415.2.2 definingaderivedclass 59615.2.3 conversions andinheritance 60115.3 virtualfunctions 60315.4 abstractbaseclasses 60815.5 accesscontrol andinheritance 61115.6 classscopeunder inheritance 61715.7 constructors andcopycontrol 62215.7.1 virtualdestructors 62215.7.2 synthesizedcopycontrol andinheritance 62315.7.3 derived-classcopy-controlmembers 62515.7.4 inheritedconstructors 62815.8 containers andinheritance 63015.8.1 writing a basketclass 63115.9 textqueriesrevisited 63415.9.1 anobject-orientedsolution 63615.9.2 the query_base and queryclasses 63915.9.3 thederivedclasses 64215.9.4 the evalfunctions 645chaptersummary 649definedterms 649chapter 16 templates and generic programming 65116.1 definingatemplate. 65216.1.1 functiontemplates 65216.1.2 classtemplates 65816.1.3 templateparameters 66816.1.4 membertemplates 67216.1.5 controlling instantiations 67516.1.6 efficiency and flexibility 67616.2 templateargumentdeduction 67816.2.1 conversions andtemplatetypeparameters 67916.2.2 function-templateexplicitarguments 68116.2.3 trailing return types and type transformation 683 16.2.4 functionpointers andargumentdeduction 68616.2.5 templateargumentdeductionandreferences 68716.2.6 understanding std::move 69016.2.7 forwarding 69216.3 overloadingandtemplates 69416.4 variadictemplates 69916.4.1 writingavariadicfunctiontemplate 70116.4.2 packexpansion 70216.4.3 forwardingparameterpacks 70416.5 template specializations 706chaptersummary 713definedterms 713part iv advanced topics 715chapter 17 specialized library facilities 71717.1 the tupletype 71817.1.1 defining and initializing tuples 71817.1.2 using a tuple toreturnmultiplevalues 72117.2 the bitsettype 72317.2.1 defining and initializing bitsets 723 17.2.2 operations on bitsets 72517.3 regularexpressions 72817.3.1 usingtheregularexpressionlibrary 729 17.3.2 thematchandregex iteratortypes 73417.3.3 usingsubexpressions 73817.3.4 using regex_replace 74117.4 randomnumbers 74517.4.1 random-numberengines anddistribution 745 17.4.2 otherkinds ofdistributions 74917.5 the iolibraryrevisited 75217.5.1 formattedinput andoutput 75317.5.2 unformattedinput/outputoperations 761 17.5.3 randomaccess toastream 763 chaptersummary 769definedterms 769chapter 18 tools for large programs 77118.1 exceptionhandling 77218.1.1 throwinganexception 77218.1.2 catchinganexception 77518.1.3 function tryblocks andconstructors 777 18.1.4 the noexceptexceptionspecification 779 18.1.5 exceptionclasshierarchies 78218.2 namespaces 78518.2.1 namespacedefinitions 78518.2.2 usingnamespacemembers 79218.2.3 classes,namespaces,andscope 79618.2.4 overloadingandnamespaces 80018.3 multiple andvirtual inheritance 80218.3.1 multiple inheritance 80318.3.2 conversions andmultiplebaseclasses 805 18.3.3 classscopeundermultiple inheritance 807 18.3.4 virtual inheritance 81018.3.5 constructors andvirtual inheritance 813 chaptersummary 816definedterms 816chapter 19 specialized tools and techniques 819 19.1 controlling memory allocation 82019.1.1 overloading new and delete 82019.1.2 placement newexpressions 82319.2 run-timetypeidentification 82519.2.1 the dynamic_castoperator 82519.2.2 the typeidoperator 82619.2.3 usingrtti 82819.2.4 the type_infoclass 83119.3 enumerations 83219.4 pointer toclassmember 83519.4.1 pointers todatamembers 83619.4.2 pointers tomemberfunctions 83819.4.3 usingmemberfunctions ascallableobjects 84119.5 nestedclasses 84319.6 union:aspace-savingclass 84719.7 localclasses 85219.8 inherentlynonportablefeatures 85419.8.1 bit-fields 85419.8.2 volatilequalifier 85619.8.3 linkage directives: extern "c" 857chaptersummary 862definedterms 862appendix a the library 865a.1 librarynames andheaders 866a.2 abrieftourof thealgorithms 870a.2.1 algorithms tofindanobject 871a.2.2 otherread-onlyalgorithms 872a.2.3 binarysearchalgorithms 873a.2.4 algorithmsthatwritecontainerelements 873a.2.5 partitioningandsortingalgorithms 875a.2.6 generalreorderingoperations 877a.2.7 permutationalgorithms 879a.2.8 setalgorithms forsortedsequences 880a.2.9 minimumandmaximumvalues 880a.2.10 numericalgorithms 881a.3 randomnumbers 882a.3.1 randomnumberdistributions 883a.3.2 randomnumberengines 884本图书信息来源:中国互动出版网。
les AS gR学生手册英文

Deploying with Oracle JDeveloper
To deploy an application with JDeveloper, perform the following steps: 1. Create the deployment profile. 2. Configure the deployment profile. 3. Create an application server connection to the target
第十六页,共33页,
Planning the Deployment Process
The deployment process includes:
1. Packaging code as J2EE applications or modules
2. Selecting a parent application
including those in a cluster
第十页,共33页,
Deploying with admin_client.jar
The admin_client.jar tool: • Is a command-line utility • Is executed by using the following basic command:
– defaultDataSource to select the data source used by the application for management of data by EJB entities
– dataSourcesPath to specify a application-specific data sources
SAS认证220道_练习题及详细答案(10-9)
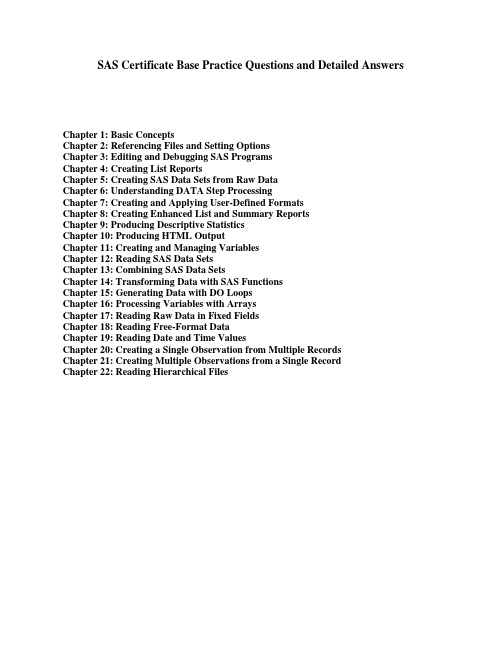
SAS Certificate Base Practice Questions and Detailed Answers Chapter 1: Basic ConceptsChapter 2: Referencing Files and Setting OptionsChapter 3: Editing and Debugging SAS ProgramsChapter 4: Creating List ReportsChapter 5: Creating SAS Data Sets from Raw DataChapter 6: Understanding DATA Step ProcessingChapter 7: Creating and Applying User-Defined FormatsChapter 8: Creating Enhanced List and Summary ReportsChapter 9: Producing Descriptive StatisticsChapter 10: Producing HTML OutputChapter 11: Creating and Managing VariablesChapter 12: Reading SAS Data SetsChapter 13: Combining SAS Data SetsChapter 14: Transforming Data with SAS FunctionsChapter 15: Generating Data with DO LoopsChapter 16: Processing Variables with ArraysChapter 17: Reading Raw Data in Fixed FieldsChapter 18: Reading Free-Format DataChapter 19: Reading Date and Time ValuesChapter 20: Creating a Single Observation from Multiple RecordsChapter 21: Creating Multiple Observations from a Single RecordChapter 22: Reading Hierarchical FilesChapter 1: Basic Concepts Answer Key1.How many observations and variables does the data set below contain?a. 3 observations, 4 variablesb. 3 observations, 3 variablesc. 4 observations, 3 variablesd.can't tell because some values are missingCorrect answer:cRows in the data set are called observations, and columns are called variables. Missing values don't affect the structure of the data set.2.How many program steps are executed when the program below is processed?data user.tables;infile jobs;input date name $ job $;run;proc sort data=user.tables;by name;run;proc print data=user.tables;run;a.threeb.fourc.fived.sixCorrect answer:aWhen it encounters a DATA, PROC, or RUN statement, SAS stops reading statements andexecutes the previous step in the program. The program above contains one DATA step and two PROC steps, for a total of three program steps.3.What type of variable is the variable AcctNum in the data set below?a.numericb.characterc.can be either character or numericd.can't tell from the data shownCorrect answer:bIt must be a character variable, because the values contain letters and underscores, which are not valid characters for numeric values.4.What type of variable is the variable Wear in the data set below?a.numericb.characterc.can be either character or numericd.can't tell from the data shownCorrect answer:aIt must be a numeric variable, because the missing value is indicated by a period rather than by a blank.5.Which of the following variable names is valid?a.4BirthDateb.$Costc._Items_d.Tax-RateCorrect answer:cVariable names follow the same rules as SAS data set names. They can be 1 to 32 characters long, must begin with a letter (A–Z, either uppercase or lowercase) or an underscore, and can continue with any combination of numbers, letters, or underscores.6.Which of the following files is a permanent SAS file?a.Sashelp.PrdSaleb.Sasuser.MySalesc.Profits.Quarter1d.all of the aboveCorrect answer:dTo store a file permanently in a SAS data library, you assign it a libref other than the default Work. For example, by assigning the libref Profits to a SAS data library, you specify that files within the library are to be stored until you delete them. Therefore, SAS files in the Sashelp and Sasuser libraries are permanent files.7.In a DATA step, how can you reference a temporary SAS data set named Forecast?a.Forecastb.Work.Forecastc.Sales.Forecast (after assigning the libref Sales)d.only a and b aboveCorrect answer:dTo reference a temporary SAS file in a DATA step or PROC step, you can specify the one-level name of the file (for example, Forecast) or the two-level name using the libref Work (for example, Work.Forecast).8.What is the default length for the numeric variable Balance?a. 5b. 6c.7d.8Correct answer:dThe numeric variable Balance has a default length of 8. Numeric values (no matter how many digits they contain) are stored in 8 bytes of storage unless you specify a different length.9.How many statements does the following SAS program contain?proc print data=new.prodsalelabel double;var state day price1 price2; where state='NC';label state='Name of State';run;a.threeb.fourc.fived.sixCorrect answer:cThe five statements are•PROC PRINT statement (two lines long)•VAR statement•WHERE statement (on the same line as the VAR statement)•LABEL statement•RUN statement (on the same line as the LABEL statement).10.What is a SAS data library?a. a collection of SAS files, such as SAS data sets and catalogsb.in some operating environments, a physical collection of SAS filesc.in some operating environments, a logically related collection of SAS filesd.all of the aboveCorrect answer:dEvery SAS file is stored in a SAS data library, which is a collection of SAS files, such as SAS data sets and catalogs. In some operating environments, a SAS data library is a physical collection of files. In others, the files are only logically related. In the Windows and UNIX environments, a SAS data library is typically a group of SAS files in the same folder or directory.Chapter 2: Referencing Files and Setting Options1.If you submit the following program, how does the output look?options pagesize=55 nonumber;proc tabulate data=clinic.admit;class actlevel;var age height weight;table actlevel,(age height weight)*mean;run;options linesize=80;proc means data=clinic.heart min max maxdec=1;var arterial heart cardiac urinary;class survive sex;run;a.The PROC MEANS output has a print line width of 80 characters, but the PROCTABULATE output has no print line width.b.The PROC TABULATE output has no page numbers, but the PROC MEANS outputhas page numbers.c.Each page of output from both PROC steps is 55 lines long and has no page numbers,and the PROC MEANS output has a print line width of 80 characters.d.The date does not appear on output from either PROC step.Correct: answer:cWhen you specify a system option, it remains in effect until you change the option or end your SAS session, so both PROC steps generate output that is printed 55 lines per page with no page numbers. If you don't specify a system option, SAS uses the default value for that system option.2.In order for the date values 05May1955 and 04Mar2046 to be read correctly, what value mustthe YEARCUTOFF= option have?a. a value between 1947 and 1954, inclusiveb.1955 or higherc.1946 or higherd.any valueCorrect answer:dAs long as you specify an informat with the correct field width for reading the entire date value, the YEARCUTOFF= option doesn't affect date values that have four-digit years.3.When you specify an engine for a library, you are always specifyinga.the file format for files that are stored in the library.b.the version of SAS that you are using.c.access to other software vendors' files.d.instructions for creating temporary SAS files.Correct answer:aA SAS engine is a set of internal instructions that SAS uses for writing to and reading from files in a SAS library. Each engine specifies the file format for files that are stored in the library, which in turn enables SAS to access files with a particular format. Some engines access SAS files, and other engines support access to other vendors' files.4.Which statement prints a summary of all the files stored in the library named Area51?a.proc contents data=area51._all_ nods;b.proc contents data=area51 _all_ nods;c.proc contents data=area51 _all_ noobs;d.proc contents data=area51 _all_.nods;Correct answer:aTo print a summary of library contents with the CONTENTS procedure, use a period to append the _ALL_ option to the libref. Adding the NODS option suppresses detailed information about the files.5.The following PROC PRINT output was created immediately after PROC TABULATEoutput. Which SAS system options were specified when the report was created?a.OBS=, DATE, and NONUMBERb.PAGENO=1, and DATEc.NUMBER and DATE onlyd.none of the aboveCorrect answer:bClearly, the DATE and PAGENO= options are specified. Because the page number on the output is 1, even though PROC TABULATE output was just produced. If you don't specify PAGENO=, all output in the Output window is numbered sequentially throughout your SAS session.6.Which of the following programs correctly references a SAS data set named SalesAnalysisthat is stored in a permanent SAS library?a.data saleslibrary.salesanalysis;set mydata.quarter1sales;if sales>100000;run;b.data mysales.totals;set sales_99.salesanalysis;if totalsales>50000;run;c.proc print data=salesanalysis.quarter1;var sales salesrep month;run;d.proc freq data=1999data.salesanalysis;tables quarter*sales; run;Correct answer:bLibrefs must be 1 to 8 characters long, must begin with a letter or underscore, and can contain only letters, numbers, or underscores. After you assign a libref, you specify it as the first element in the two-level name for a SAS file.7.Which time span is used to interpret two-digit year values if the YEARCUTOFF= option isset to 1950?a.1950-2049b.1950-2050c.1949-2050d.1950-2000Correct answer:aThe YEARCUTOFF= option specifies which 100-year span is used to interpret two-digit year values. The default value of YEARCUTOFF= is 1920. However, you can override the default and change the value of YEARCUTOFF= to the first year of another 100-year span. If you specify YEARCUTOFF=1950, then the 100-year span will be from 1950 to 2049.8.Asssuming you are using SAS code and not special SAS windows, which one of thefollowing statements is false?a.LIBNAME statements can be stored with a SAS program to reference the SAS libraryautomatically when you submit the program.b.When you delete a libref, SAS no longer has access to the files in the library.However, the contents of the library still exist on your operating system.c.Librefs can last from one SAS session to another.d.You can access files that were created with other vendors' software by submitting aLIBNAME statement.Correct answer:cThe LIBNAME statement is global, which means that librefs remain in effect until you modify them, cancel them, or end your SAS session. Therefore, the LIBNAME statement assigns the libref for the current SAS session only. You must assign a libref before accessingSAS files that are stored in a permanent SAS data library.9.What does the following statement do?libname osiris spss 'c:\myfiles\sasdata\data';a.defines a library called Spss using the OSIRIS engineb.defines a library called Osiris using the SPSS enginec.defines two libraries called Osiris and Spss using the default engined.defines the default library using the OSIRIS and SPSS enginesCorrect answer:bIn the LIBNAME statement, you specify the library name before the engine name. Both are followed by the path.10.What does the following OPTIONS statement do?options pagesize=15 nodate;a.suppresses the date and limits the page size of the logb.suppresses the date and limits the vertical page size for text outputc.suppresses the date and limits the vertical page size for text and HTML outputd.suppresses the date and limits the horizontal page size for text outputCorrect answer:bThese options affect the format of listing output only. NODATE suppresses the date and PAGESIZE= determines the number of rows to print on the page.Chapter 3: Editing and Debugging SAS Programs Answer Key1.As you write and edit SAS programs it's a good idea toa.begin DATA and PROC steps in column one.b.indent statements within a step.c.begin RUN statements in column one.d.all of the aboveCorrect answer:dAlthough you can write SAS statements in almost any format, a consistent layout enhances readability and enables you to understand the program's purpose. It's a good idea to begin DATA and PROC steps in column one, to indent statements within a step, to begin RUN statements in column one, and to include a RUN statement after every DATA step or PROC step.2.What usually happens when an error is detected?a.SAS continues processing the step.b.SAS continues to process the step, and the log displays messages about the error.c.SAS stops processing the step in which the error occurred, and the log displaysmessages about the error.d.SAS stops processing the step in which the error occurred, and the program outputdisplays messages about the error.Correct answer:cSyntax errors generally cause SAS to stop processing the step in which the error occurred. When a program that contains an error is submitted, messages regarding the problem also appear in the SAS log. When a syntax error is detected, the SAS log displays the word ERROR, identifies the possible location of the error, and gives an explanation of the error.3. A syntax error occurs whena.some data values are not appropriate for the SAS statements that are specified in aprogram.b.the form of the elements in a SAS statement is correct, but the elements are not validfor that usage.c.program statements do not conform to the rules of the SAS language.d.none of the aboveCorrect canswer:Syntax errors are common types of errors. Some SAS system options, features of the Editorwindow, and the DATA step debugger can help you identify syntax errors. Other types oferrors include data errors, semantic errors, and execution-time errors.4.How can you tell whether you have specified an invalid option in a SAS program?a. A log message indicates an error in a statement that seems to be valid.b. A log message indicates that an option is not valid or not recognized.c.The message "PROC running" or "DATA step running" appears at the top of theactive window.d.You can't tell until you view the output from the program.Correct answer:bWhen you submit a SAS statement that contains an invalid option, a log message notifies you that the option is not valid or not recognized. You should recall the program, remove or replace the invalid option, check your statement syntax as needed, and resubmit the corrected program.5.Which of the following programs contains a syntax error?Correct answer:bThe DATA step contains a misspelled keyword (dat instead of data). However, this is such a common (and easily interpretable) error that SAS produces only a warning message, not an error.6.What does the following log indicate about your program?proc print data=sasuser.cargo99var origin dest cargorev;2276ERROR 22-322: Syntax error, expecting one of the following:;, (, DATA, DOUBLE, HEADING, LABEL, N, NOOBS, OBS, ROUND, ROWS, SPLIT, STYLE,UNIFORM, WIDTH.ERROR 76-322: Syntax error, statement will be ignored.11 run;a.SAS identifies a syntax error at the position of the VAR statement.b.SAS is reading VAR as an option in the PROC PRINT statement.c.SAS has stopped processing the program because of errors.d.all of the aboveCorrect answer:dBecause there is a missing semicolon at the end of the PROC PRINT statement, SAS interprets VAR as an option in PROC PRINT and finds a syntax error at that location. SAS stops processing programs when it encounters a syntax error.Chapter 4: Creating List Reports Answer Key 1.Which PROC PRINT step below creates the following output?Correct answer:cThe DATA= option specifies the data set that you are listing, and the ID statement replaces the Obs column with the specified variable. The VAR statement specifies variables and controls the order in which they appear, and the WHERE statement selects rows based on a condition. The LABEL option in the PROC PRINT statement causes the labels that are specified in the LABEL statement to be displayed.2.Which of the following PROC PRINT steps is correct if labels are not stored with thedata set?Correct aanswer:You use the DATA= option to specify the data set to be printed. The LABEL optionspecifies that variable labels appear in output instead of variable names.3.Which of the following statements selects from a data set only those observations forwhich the value of the variable Style is RANCH, SPLIT, or TWOSTORY?Correct answer:dIn the WHERE statement, the IN operator enables you to select observations based on several values. You specify values in parentheses and separate them by spaces or commas. Character values must be enclosed in quotation marks and must be in the same case as in the data set.4.If you want to sort your data and create a temporary data set named Calc to store thesorted data, which of the following steps should you submit?Correct answer:cIn a PROC SORT step, you specify the DATA= option to specify the data set to sort. The OUT= option specifies an output data set. The required BY statement specifies the variable(s) to use in sorting the data.5.Which options are used to create the following PROC PRINT output?13:27 Monday, March 22, 1999 Patient Arterial Heart Cardiac Urinary203 88 95 66 11054 83 183 95 0664 72 111 332 12210 74 97 369 0101 80 130 291 0a.the DATE system option and the LABEL option in PROC PRINTb.the DATE and NONUMBER system options and the DOUBLE and NOOBSoptions in PROC PRINTc.the DATE and NONUMBER system options and the DOUBLE option inPROC PRINTd.the DATE and NONUMBER system options and the NOOBS option in PROCPRINTCorrect answer:bThe DATE and NONUMBER system options cause the output to appear with the date but without page numbers. In the PROC PRINT step, the DOUBLE option specifies double spacing, and the NOOBS option removes the default Obs column.6.Which of the following statements can you use in a PROC PRINT step to create thisoutput?Correct answer:dYou do not need to name the variables in a VAR statement if you specify them in the SUM statement, but you can. If you choose not to name the variables in the VAR statement as well, then the SUM statement determines the order of the variables in the output.7.What happens if you submit the following program?proc sort data=clinic.diabetes;run;proc print data=clinic.diabetes;var age height weight pulse;where sex='F';run;a.The PROC PRINT step runs successfully, printing observations in their sortedorder.b.The PROC SORT step permanently sorts the input data set.c.The PROC SORT step generates errors and stops processing, but the PROCPRINT step runs successfully, printing observations in their original (unsorted)order.d.The PROC SORT step runs successfully, but the PROC PRINT step generateserrors and stops processing.Correct answer:cThe BY statement is required in PROC SORT. Without it, the PROC SORT step fails. However, the PROC PRINT step prints the original data set as requested.8.If you submit the following program, which output does it create?proc sort data=finance.loans out=work.loans;by months amount;run;proc print data=work.loans noobs; var months;sum amount payment;where months<360;run;a.b.c.d.Correct answer:aColumn totals appear at the end of the report in the same format as the values of the variables, so b is incorrect. Work.Loans is sorted by Month and Amount, so c isincorrect. The program sums both Amount and Payment, so d is incorrect.9.Choose the statement below that selects rows which•the amount is less than or equal to $5000•the account is 101-1092 or the rate equals 0.095.Correct answer:cTo ensure that the compound expression is evaluated correctly, you can use parentheses to groupaccount='101-1092' or rate eq 0.095OBS Account Amount Rate MonthsPayment1 101-1092 $22,000 10.00%60 $467.432 101-1731 $114,0009.50% 360 $958.573 101-1289 $10,000 10.50%36 $325.024 101-3144 $3,500 10.50%12 $308.525 103-1135 $8,700 10.50%24 $403.476 103-1994 $18,500 10.00%60 $393.077 103-2335 $5,000 10.50%48 $128.028 103-3864 $87,500 9.50% 360 $735.759 103-3891 $30,000 9.75% 360 $257.75For example, from the data set above, a and b above select observations 2 and 8 (those that have a rate of 0.095); c selects no observations; and d selects observations 4 and 7 (those that have an amount less than or equal to 5000).10.What does PROC PRINT display by default?a.PROC PRINT does not create a default report; you must specify the rows andcolumns to be displayed.b.PROC PRINT displays all observations and variables in the data set. If youwant an additional column for observation numbers, you can request it.c.PROC PRINT displays columns in the following order: a column forobservation numbers, all character variables, and all numeric variables.d.PROC PRINT displays all observations and variables in the data set, a columnfor observation numbers on the far left, and variables in the order in which they occur in the data set.Correct answer:dYou can remove the column for observation numbers. You can also specify the variables you want, and you can select observations according to conditions.Chapter 5: Creating SAS Data Sets from Raw Data Answer Key1.Which SAS statement associates the fileref Crime with the raw data fileC:\States\Data\Crime?a.filename crime 'c:\states\data\crime';b.filename crime c:\states\data\crime;c.fileref crime 'c:\states\data\crime';d.filename 'c:\states\data\crime' crime; Correct aanswer:Before you can read your raw data, you must reference the raw data file by creating afileref. You assign a fileref by using a FILENAME statement in the same way thatyou assign a libref by using a LIBNAME statement.2.Filerefs remain in effect untila.you change them.b.you cancel them.c.you end your SAS session.d.all of the aboveCorrect answer:dLike LIBNAME statements, FILENAME statements are global; they remain in effect until you change them, cancel them, or end your SAS session.3.Which statement identifies the name of a raw data file to be read with the filerefProducts and specifies that the DATA step read only records 1-15?a.infile products obs 15;b.infile products obs=15;c.input products obs=15;d.input products 1-15;Correct answer:bYou use an INFILE statement to specify the raw data file to be read. You can specify a fileref or an actual filename (in quotation marks). The OBS= option in the INFILE statement enables you to process only records 1 through n.4.Which of the following programs correctly writes the observations from the data setbelow to a raw data file?Correct answer:dThe keyword _NULL_ in the DATA statement enables you to use the power of the DATA step without actually creating a SAS data set. You use the FILE and PUT statements to write out the observations from a SAS data set to a raw data file. The FILE statement specifies the raw data file and the PUT statement describes the lines towrite to the raw data file. The filename and location that are specified in the FILE statement must be enclosed in quotation marks.5.Which raw data file can be read using column input?a.b.c.d.all of the aboveCorrect answer:bColumn input is appropriate only in some situations. When you use column input, your data must be standard character or numeric values, and they must be in fixed fields. That is, values for a particular variable must be in the same location in all records.6.Which program creates the output shown below?Correct answer:aThe INPUT statement creates a variable using the name that you assign to each field. Therefore, when you write an INPUT statement, you need to specify the variable names exactly as you want them to appear in the SAS data set.7.Which statement correctly reads the fields in the following order: StockNumber,Price, Item, Finish, Style?Field Name Start Column End Column Data TypeStockNumber 1 3 characterFinish 5 9 characterStyle 11 18 characterItem 20 24 characterPrice 27 32 numericCorrec t answer:bYou can use column input to read fields in any order. You must specify the variable name to be created, identify character values with a $, and name the correct starting column and ending column for each field.8.Which statement correctly re-defines the values of the variable Income as 100percent higher?a.income=income*1.00;b.income=income+(income*2.00);c.income=income*2;d.income=*2;Correct answer:cTo re-define the values of the variable Income in an Assignment statement, you specify the variable name on the left side of the equal sign and an appropriate expression including the variable name on the right side of the equal sign.9.Which program correctly reads instream data?a.data finance.newloan;input datalines;if country='JAPAN';MonthAvg=amount/12;1998 US CARS 194324.121998 US TRUCKS 142290.301998 CANADA CARS 10483.441998 CANADA TRUCKS 93543.641998 MEXICO CARS 22500.571998 MEXICO TRUCKS 10098.881998 JAPAN CARS 15066.431998 JAPAN TRUCKS 40700.34;b.data finance.newloan;input Year 1-4 Country $ 6-11Vehicle $ 13-18 Amount 20-28;if country='JAPAN';MonthAvg=amount/12;datalines;run;c.data finance.newloan;input Year 1-4 Country 6-11Vehicle 13-18 Amount 20-28;if country='JAPAN';MonthAvg=amount/12;datalines;1998 US CARS 194324.121998 US TRUCKS 142290.301998 CANADA CARS 10483.441998 CANADA TRUCKS 93543.641998 MEXICO CARS 22500.571998 MEXICO TRUCKS 10098.881998 JAPAN CARS 15066.431998 JAPAN TRUCKS 40700.34;d.data finance.newloan;input Year 1-4 Country $ 6-11Vehicle $ 13-18 Amount 20-28;if country='JAPAN';MonthAvg=amount/12;datalines;1998 US CARS 194324.121998 US TRUCKS 142290.301998 CANADA CARS 10483.441998 CANADA TRUCKS 93543.641998 MEXICO CARS 22500.571998 MEXICO TRUCKS 10098.881998 JAPAN CARS 15066.431998 JAPAN TRUCKS 40700.34;Correct answer:dTo read instream data, you specify a DATALINES statement and data lines, followed by a null statement (single semicolon) to indicate the end of the input data. Program a contains no DATALINES statement, and the INPUT statement doesn't specify the fields to read. Program b contains no data lines, and the INPUT statement in program c doesn't specify the necessary dollar signs for the character variables Country and Vehicle.10.Which SAS statement subsets the raw data shown below so that only the observationsin which Sex (in the second field) has a value of F are processed?a.if sex=f;b.if sex=F;c.if sex='F';d. a or bCorrect answer:cTo subset data, you can use a subsetting IF statement in any DATA step to process only those observations that meet a specified condition. Because Sex is a character variable, the value F must be enclosed in quotation marks and must be in the same case as in the data set.Chapter 6: Understanding DATA Step Processing Answer Key1.Which of the following is not created during the compilation phase?。
SAP ABAP 文件操作函数大全
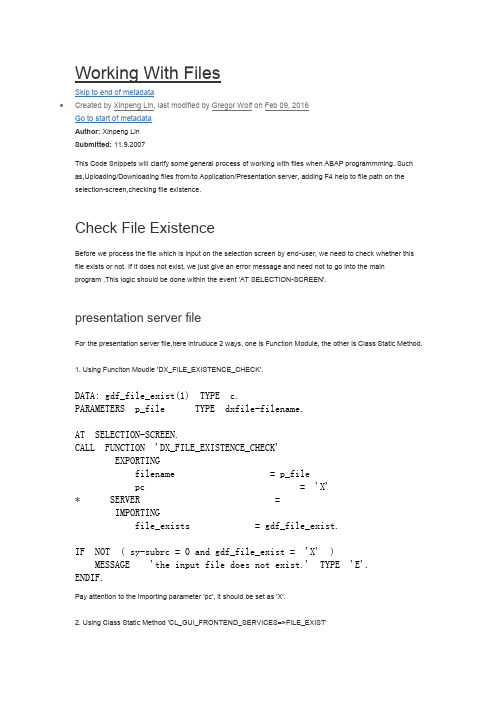
Working With FilesSkip to end of metadataCreated by Xinpeng Lin, last modified by Gregor Wolf on Feb 09, 2016Go to start of metadataAuthor: Xinpeng LinSubmitted: 11.9.2007This Code Snippets will clarify some general process of working with files when ABAP programmming. Such as,Uploading/Downloading files from/to Application/Presentation server, adding F4 help to file path on the selection-screen,checking file existence.Check File ExistenceBefore we process the file which is input on the selection screen by end-user, we need to check whether this file exists or not. If it does not exist, we just give an error message and need not to go into the mainprogram .This logic should be done within the event 'AT SELECTION-SCREEN'.presentation server fileFor the presentation server file,here intruduce 2 ways, one is Function Module, the other is Class Static Method.1. Using Funciton Moudle 'DX_FILE_EXISTENCE_CHECK'.DATA: gdf_file_exist(1) TYPE c.PARAMETERS p_file TYPE dxfile-filename.AT SELECTION-SCREEN.CALL FUNCTION 'DX_FILE_EXISTENCE_CHECK'EXPORTINGfilename = p_filepc = 'X'* SERVER =IMPORTINGfile_exists = gdf_file_exist.IF NOT ( sy-subrc = 0 and gdf_file_exist = 'X' )MESSAGE 'the input file does not exist.' TYPE 'E'.ENDIF.Pay attention to the importing parameter 'pc', it should be set as 'X'.2. Using Class Static Method 'CL_GUI_FRONTEND_SERVICES=>FILE_EXIST'DATA: gdf_file_exist(1) TYPE c.PARAMETERS p_file TYPE dxfile-filename.AT SELECTION-SCREEN.CALL METHOD cl_gui_frontend_services=>file_existEXPORTINGfile = p_fileRECEIVINGresult = gdf_file_exist.IF NOT ( sy-subrc = 0 and gdf_file_exist = 'X' )MESSAGE 'the input file does not exist.' TYPE 'E'.ENDIF.application server fileFor application server file, generally we open it first within the event 'AT SELECTION-SCREEN'.If it can be opened successfully, this file exists. After open,do not forget to close it.PARAMETERS p_file TYPE dxfile-filename.AT SELECTION-SCREEN.OPEN DATASET p_file FOR INPUT IN TEXT MODE ENCODING DEFAULT.IF sy-subrc = 0.CLOSE DATASET p_file.ELSE.MESSAGE 'the input file does not exist.' TYPE 'E'.ENDIF.There is also a Function Module can be used, named 'OCS_GET_FILE_INFO'.DATA: gdt_ocs_file TYPE TABLE OF ocs_file.PARAMETERS p_file TYPE dxfile-filename.AT SELECTION-SCREEN.CALL FUNCTION 'OCS_GET_FILE_INFO'EXPORTINGdir_name = p_filefile_name = '*'TABLESdir_list = gdt_ocs_file. Importing parameter 'file_name' is set as '*' means all the file in the specfied directory will be get and stored in the internal table 'gdt_ocs_file'. If the input file is included in the internal table, this file exists.Add F4 HelpAdding one F4 Help to the file path on the selection-screen will be very helpful to the end-user.The logic should be under the event 'ON VALUE-REQUEST'.presentation server fileFor the presentation server file,here intruduce 2 ways, one is Function Module, the other is Class Static Method.1. Using Funciton Module 'F4_FILENAME'.PARAMETERS p_file TYPE dxfile-filename.AT SELECTION-SCREEN ON VALUE-REQUEST FOR p_file.CALL FUNCTION 'F4_FILENAME'* EXPORTING* PROGRAM_NAME =* DYNPRO_NUMBER =* FIELD_NAME =IMPORTINGfile_name = p_file.2. Using Class Static Method 'CL_GUI_FRONTEND_SERVICES=>FILE_OPEN_DIALOG'.DATA: gdt_filetable TYPE filetable.DATA: gdf_rc TYPE I.PARAMETERS p_file TYPE dxfile-filename.AT SELECTION-SCREEN ON VALUE-REQUEST FOR p_file.CALL METHOD cl_gui_frontend_services=>file_open_dialogEXPORTINGWINDOW_TITLE = 'Choose a file'CHANGINGfile_table = gdt_filetablerc = gdf_rc.IF sy-subrc = 0.READ TABLE gdt_filetableINTO gds_filetable INDEX 1.p_file = gds_filetable-filename.ENDIF.gdf_rc is the number of selected file, if it is equal -1, error occured.application server fileGenerally it need not to provide F4 help for application server file. If we want it, there is also a Funciotn Module which can be used.PARAMETERS p_file TYPE dxfile-filename.AT SELECTION-SCREEN ON VALUE-REQUEST FOR p_file.CALL FUNCTION 'F4_DXFILENAME_4_DYNP'EXPORTINGdynpfield_filename = 'P_FILE'dyname = sy-repiddynumb = sy-dynnrfiletype = 'P'location = 'A'server = ' '. filetype: 'P' represents Physical file name; 'L' represents Logical file name.location: 'A' represents Application Server; 'P' represents Presentation server.Download FilesSometimes we need to save the internal table data as a file for the further process.For download topresentation server and download to application server,they are 2 different kinds of process methods.to presentation serverWhen storing internal table data as a local file on the presentation server, there are 2 methods we can use.One is using the function modeule, the other is using the class static method.1. Using Function Module 'GUI_DOWNLOAD'.DATA: gdf_filepath type dxfile-filenameDATA: gdt_data type table of gts_data.START-OF-SELECTION.gdf_filepath = 'C:\mydata.txt'.CALL FUNCTION 'GUI_DOWNLOAD'EXPORTINGfilename = gdf_filepathfiletype = 'ASC'write_field_separator = 'X'TABLESdata_tab = gdt_data.filetype is set by 'X' mens separating columns by Tabs in case of ASCII download.If sy-subrc is equal 0, file downloading is successful.2. Using class static method 'CL_GUI_FRONTEND_SERVICES =>GUI_DOWNLOAD'DATA: gdf_filepath type dxfile-filenameDATA: gdt_data type table of gts_data.START-OF-SELECTION.gdf_filepath = 'C:\mydata.txt'.CALL METHOD cl_gui_frontend_services=>gui_downloadEXPORTINGfilename = gdf_filepathfiletype = 'ASC'write_field_separator = 'X'CHANGINGdata_tab = gdt_data.filetype is set by 'X' mens separating columns by Tabs in case of ASCII download.If sy-subrc is equal 0, file downloading is successful.to application serverIf we want to save the internal table data to the application server, there is no function module or class staticmethod which we can use, we must wirte the code by ourselves.DATA: gdf_filepath type dxfile-filenameDATA: gdt_data type table of gts_data.DATA: ldf_length type i.FIELD-SYMBOLS: <lfs_outfile> TYPE gts_dataSTART-OF-SELECTION.gdf_filepath = 'C:\mydata.txt'.OPEN DATASET gdf_filepath FOR OUTPUT IN TEXT MODE ENCODING DEFAULT.LOOP AT prt_data ASSIGNING <lfs_outfile>.DESCRIBE FIELD <lfs_outfile> LENGTH ldf_length IN BYTE MODE.TRANSFER <lfs_outfile> TO prf_file LENGTH ldf_length.ENDLOOP.CLOSE DATASET gdf_filepath.The prerequsite is the field of prt_data must be character type.Using this method, every field column will output as the length defined,without separator.If we want field columns are separated by tab, we can realize it as below.DATA: gdf_filepath type dxfile-filenameDATA: gdt_data type table of gts_data.DATA: ldf_length type i.FIELD-SYMBOLS: <lfs_outfile> TYPE gts_dataSTART-OF-SELECTION.gdf_filepath = 'C:\mydata.txt'.OPEN DATASET gdf_filepath FOR OUTPUT IN TEXT MODE ENCODING DEFAULT.LOOP AT prt_data ASSIGNING <lfs_outfile>.CONCATENATE <LFS_OUTFILE>-BUKRS<LFS_...INTO LDF_OUTDATASEPARATED BYCL_ABAP_CHAR_UTILITIES=>HORIZONTRANSFER LDF_OUTDATA TO gdf_filepath.ENDLOOP.CLOSE DATASET gdf_filepath.Upload FilesSometimes we need to upload the file data to the internal table first, and then process it.For upload frompresentation server and upload from application server,they are 2 different kinds of process methods.from presentation serverWhen we upload a file data from presentation server to the internal table, there are 2 method that we can choose.One is using the Function Moduel, the other is using the class static method.1. Using function module 'GUI_UPLOAD'.DATA: gdt_filedata TYPE TABLE OF gts_filedata.PARAMETERS p_file TYPE dxfile-filename.START-OF-SELECTION.CALL FUNCTION 'GUI_UPLOAD'EXPORTINGfilename = p_filehas_field_separator = 'X'TABLESdata_tab = gdt_filedata. parameter has_field_separator is set as 'X' means columns separated by tabs in case of ASCII upload.2. Using the class tatic method 'CL_GUI_FRONTEND_SERVICES =>GUI_UPLOAD'.DATA: gdt_filedata TYPE TABLE OF gts_filedata.PARAMETERS p_file TYPE dxfile-filename.START-OF-SELECTION.CALL METHOD cl_gui_frontend_services=>gui_uploadEXPORTINGfilename = p_filehas_field_separator = 'X'CHANGINGdata_tab = prt_table.parameter 'has_field_separator' is set as 'X' means columns separated by tabs in case of ASCII upload. from application serverIf we want to upload file data from the application server to the internal table, there is no function module or class static method which we can use, we must wirte the code by ourselves.1. For the file data which has no seperator between field columns.PARAMETERS p_file TYPE dxfile-filename.START-OF-SELECTION.OPEN DATASET p_file IN TEXT MODE ENCODING DEFAULT FOR INPUT.DO.READ DATASET p_file INTO gds_data.IF sy-subrc <> 0.EXIT.ENDIF.APPEND gds_data TO gdt_data.ENDDO.CLOSE DATASET p_file.2. For the file data which has tab separator between field columns.DATA: gds_field_split type gts_data.FIELD-SYMBOLS: <fs_field> TYPE gts_data.PARAMETERS p_file TYPE dxfile-filename.START-OF-SELECTION.OPEN DATASET prf_file IN TEXT MODE ENCODING DEFAULT FOR INPUT.DO.READ DATASET p_file INTO gds_field.SPLIT gds_field AT cl_abap_char_utilities=>horizontal_tabINTO TABLE gdt_field_split.LOOP AT gdt_field_split into gds_field_split.gdf_index = gdf_index + 1.ASSIGN COMPONENT gdf_index OF STRUCTUREgds_data to <fs_field>.IF sy-subrc = 0.<fs_field> = gds_field_split.ENDIF.ENDLOOP.APPEND gds_data TO gdt_data.ENDDO.CLOSE DATASET p_file.。
operating system《操作系统》ch11-file system implementation-50

I/O Using a Unified Buffer Cache
11.32
11.7 Recovery
Consistency checking – compares data in directory structure with data blocks on disk, and tries to fix inconsistencies
Brings all pointers together into the index block. Logical view.
index table
11.20
Example of Indexed Allocation
11.21
Indexed Allocation (Cont.)
Need index table Random access Dynamic access without external fragmentation, but
Efficiency dependent on:
disk allocation and directory algorithms types of data kept in file’s directory entry
Performance
disk cache – separate section of main memory for frequently used blocks
File-allocation table (FAT) – disk-space allocation used by MS-DOS and OS/2.
11.17
Linked Allocation
11.18
File-Allocation Table
Atom编辑器用户指南说明书

Table of ContentsAbout1 Chapter 1: Getting started with atom-editor2 Remarks2 Versions2 Examples6 What is Atom?6 Running a "Hello, World!" program in Python using Atom from scratch8 Step 1: Installing Python8 Step 2: Installing Atom8 Step 3: Configuring Atom8 Step 4: Programming and executing8 Chapter 2: Basic Editing With Atom10 Remarks10 Examples10 Opening Files and Directories10 Opening Files10 Opening Directories11 Interactive File Tree13 Find and Replace14 Chapter 3: Installation and Setup16 Remarks16 Examples16 Installing Atom on Windows16 Using the official installer16 Building from source16 Installing Atom on Mac16 Installing from a zip16 Building from Source17Installing from a package17 Debian, Ubuntu, etc.17 RedHat Enterprise, CentOS, Oracle Linux, Scientific Linux, etc.17 Fedora (DNF package manager)17 SUSE (Zypp package manager)17 Building from Source17 Chapter 4: Themes and Packages19 Introduction19 Examples19 Downloading and Installing Packages and Themes19 Packages19 Themes19 Use Atom Package Manager20 Credits21AboutYou can share this PDF with anyone you feel could benefit from it, downloaded the latest version from: atom-editorIt is an unofficial and free atom-editor ebook created for educational purposes. All the content is extracted from Stack Overflow Documentation, which is written by many hardworking individuals at Stack Overflow. It is neither affiliated with Stack Overflow nor official atom-editor.The content is released under Creative Commons BY-SA, and the list of contributors to each chapter are provided in the credits section at the end of this book. Images may be copyright of their respective owners unless otherwise specified. All trademarks and registered trademarks are the property of their respective company owners.Use the content presented in this book at your own risk; it is not guaranteed to be correct nor accurate, please send your feedback and corrections to ********************Chapter 1: Getting started with atom-editor RemarksThis section provides an overview of what atom-editor is, and why a developer might want to use it.It should also mention any large subjects within atom-editor, and link out to the related topics. Since the Documentation for atom-editor is new, you may need to create initial versions of those related topics.VersionsExamplesWhat is Atom?Atom is a hackable text editor created by GitHub and developed on top of the Electron desktop application platform.This means it can be used as a text editor for basic programming up to a full-sized IDE. It is also extremely customisable, it provides thousands of community-made packages (syntax highlighting, improved UX, etc.) and themes to suit everyone's needs. It is also available on Windows, MacOS, and Linux.Here is an example:Atom provides other helpful features including: Opening directories•Multiple editing tabs••Side-by-side editing panes•Line switching•File and directory tree managementRunning a "Hello, World!" program in Python using Atom from scratchAtom is versatile and flexible text editor and has hundreds of community-made, open-source packages that can compile and run source files, for many languages. This guide will show how easy it is to code Python with the Atom editor.This guide assumes you do not have Python nor Atom installed in your system.Step 1: Installing PythonPython can be installed from the either the official website, or if you're using Linux, through package managers (however Python usually comes pre-installed anyways).If you're a Windows user, do not forget to set python.exe to your %PATH%.Step 2: Installing AtomYou can install the Atom editor from the official website or through package managers.Step 3: Configuring AtomFor more information about installing packages, and themes, read this dedicated topic.In order to compile and run programs, the Atom community provides packages to fill that need. For this example, we will be using script to run our program.Go to File > Settings > Install.Type script in the search bar and install it. When it is installed, it should be listed in "Packages" in the Settings bar. It should be noted that script is not capable of user input.If you're using MacOS or Linux, you can use the apm package manager to install packages.Step 4: Programming and executingPick a directory where you would like to store your PY source file.Make sure you can see the Tree View pane; if you cannot see this pane, you can toggle it by1.going to View > Toggle Tree View.2.Go to File > Add Project Folder and select a directory which will be set as your root directory for a project.3.Right-click the folder and click New File, then enter in hello-world.py and type in thefollowing code:print("Hello, World!")Press CTRL+SHIFT+B to run the script. Alternatively, you can go to View > Toggle Command4.Palette and enter Script: Run.The script should return:Hello, World![Finished in 0.125s]Read Getting started with atom-editor online: https:///atom-editor/topic/8684/getting-started-with-atom-editorChapter 2: Basic Editing With AtomRemarksNote, the icons used at the end of the Opening Files and Directories example are not part of Atom's standard styling, but are the result of the file-icons styling package.ExamplesOpening Files and DirectoriesAlong with other more advanced text editors, Atom allows developers to open a single file or a directory.Opening FilesTo open files with Atom, either use File > Open File... in the menu as show below:or use the faster keyboard shortcut: Ctrl+O (For Mac OS: +O). This will open a file explorer (Finder for Mac OS) from which you can select a file to open, or to select multiple files, use the Ctrl (For Mac ) key while clicking on other files or hold the Shift key while selecting other files to select a range. When you have selected the files you wish to open, press the Open button on the file explorer. Atom, as a text editor, only elects to handle files under 2 megabytes.Opening DirectoriesEspecially for projects, Atom's directory opening feature can be quite useful. To do so, you may either use the option in Atom's file menu:or use the keyboard shortcut Ctrl+Shift+O (For Mac OS: +Shift+O). Opening directories will allow you to access other directories and files below the root directory:Interactive File TreeIn order to keep track of your projects' file structure, Atom, like many text editors and IDEs, uses a file tree model. These trees show the locations and names of your files and directory. To toggle the tree between visible and hidden, the keys Ctrl+\ may be used ( +\ for Mac OS). This tree also includes many operations for both files and directories as shown below:Hidden files will (unless set otherwise in Atom's settings) show up with shaded filenames. A common example is GitHub's repository configuration data in the .git directory.Find and ReplaceThe find and replace feature in Atom works in two ways, one operates locally only on the file you are in, and the other on a set of files or directories.To open the find and replace tool for a single file, press Ctrl+F (For Mac OS use +F). Enter in thefirst blank the string you are searching for. Press the Enter key to find all instances of the string. To the right of the Find button are the regex, case sensitive, in selection, and whole word option buttons. The Use Regex button allows you to search for regex characters such as \n, \t, \r and regex statements /^[a-z0-9_-]{3,16}$/. The Case Sensitive button - when active - will only find strings with the same case (capitalizations). The Only in Selection option will only find instances of the string in highlighted sections of the file. The Whole Word option will only find delimited instances, not when the string is part of a larger portion. Clicking the Replace button will take the first instance found with the Find method and replace them with the contents of the replace field (even if it is empty). Clicking the Replace All button will replace all instances found with the Find method and replace them all at once with the contents of the replace field.Read Basic Editing With Atom online: https:///atom-editor/topic/8717/basic-editing-with-atomChapter 3: Installation and SetupRemarksTo troubleshoot errors that occur with building from source, please view the build documents. ExamplesInstalling Atom on WindowsUsing the official installerDownload the installer from the official website. It will automatically add atom and apm (Atom Package Manager) to your %PATH% variable.Building from sourceRequirements:Node.js 4.4.x or later••Python 2.7.x•7zipVisual Studio (One of the versions below)•Visual C++ Build Tools 2015○Visual Studio 2013 Update 5 (Express Edition or better)○Visual Studio 2015 (Community Edition or better)○•GitRun the following commmands into Command Prompt:cd C:/git clone https:///atom/atom.gitcd atomscript/buildInstalling Atom on MacInstalling from a zip1.Download the atom-mac.zip zip file from the Atom GitHub repository here2.Unzip the file by double clicking on it in Finder3.Drag the Atom application into your "Applications" folderRun the Atom application.4.Building from SourceRequirements:macOS 10.8 or higher••Node.js 4.4x or laternpm 3.10.x or later••XcodeInstallation:git clone https:///atom/atom.gitcd atomscript/buildAfter building, install with script/build --installInstalling Atom on LinuxInstalling from a packageDebian, Ubuntu, etc.$ sudo dpkg -i atom-amd64.deb$ sudo apt-get -f installRedHat Enterprise, CentOS, Oracle Linux, Scientific Linux, etc.$ sudo yum install -y atom.x86_64.rpmFedora (DNF package manager)$ sudo dnf install -y atom.x86_64.rpmSUSE (Zypp package manager)$ sudo zypper in -y atom.x86_64.rpmBuilding from SourceRequirements:•OS with 64 or 32 bit architecture•C++ 11 toolchain•Git•Node.js 4.4x or later•npm 3.10.x or laterGNOME Keyring Development headers•Run the following commands:git clone https:///atom/atom.gitcd atomscript/buildFor specific instructions related to a single Linux distro, read these instructions.Read Installation and Setup online: https:///atom-editor/topic/8686/installation-and-setupChapter 4: Themes and PackagesIntroductionAtom's packages allow you to customize the editor to your needs. This topic will explains how packages and themes are created, published, and installed.ExamplesDownloading and Installing Packages and ThemesTo view your installed packages or themes, open settings with Ctrl+, and select either the "Packages" or "Themes" tab in the left-hand navigation bar. Note, the packages or themes you installed from other publishers will show up under the "Community Themes" section and themes pre-installed with Atom will show up under the "Core Themes" section.Packages1.Press Ctrl+, to open the settings tab2.Select the "Install" item on the left navigation pane3.Ensure the "Packages" button is selected in the top right4.Use the search bar at the top to find a package (ex. file icons)Click the Install button to download and install the package5.To view information on packages and their settings, click the package name.Browse Atom packages online here.ThemesDownloading and installing themes follows a similar process to that of packages.1.Press Ctrl+, to open the settings tab2.Select the "Install" item on the left navigation pane3.Ensure the "Themes" option is selected by the search bar.4.Search for a theme (ex. atom-sublime-monokai-syntax)5.Click the install button to download and installTo view information on themes and their settings, click the theme name.Browse Atom themes online here.Use Atom Package Managerapm is Atom's native package manager. It allows the user to manage packages and themes without having to initialise Atom itself. apm comes with the official installation and is automatically added to %PATH% if you're on Windows.To use apm, go to Command Prompt and type$ apm <command>Here is the list of what you can do with this package manager.clean, config, dedupe, deinstall, delete, dev, develop, disable, docs,enable, erase, featured, home, i, init, install, link, linked, links, list,ln, lns, login, ls, open, outdated, publish, rebuild, rebuild-module-cache,remove, rm, search, show, star, starred, stars, test, uninstall, unlink,unpublish, unstar, update, upgrade, view.For example, if you want to do upgrade all packages from atom:apm upgrade --confirm falseOr if you want to install a specific package:apm install <package_name>Read Themes and Packages online: https:///atom-editor/topic/8687/themes-and-packagesCredits。
Adobe Acrobat SDK 开发者指南说明书
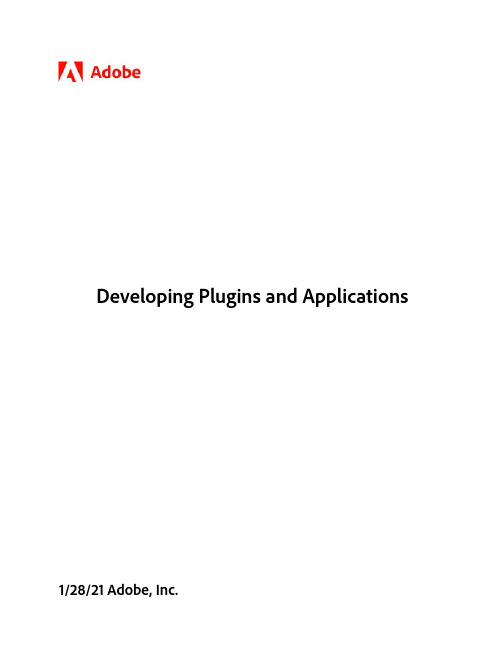
This guide is governed by the Adobe Acrobat SDK License Agreement and may be used or copied only in accordance with the terms of this agreement. Except as permitted by any such agreement, no part of this guide may be reproduced, stored in a retrieval system, or transmitted, in any form or by any means, electronic, mechanical, recording, or otherwise, without the prior written permission of Adobe. Please note that the content in this guide is protected under copyright law.
【英文原版】操作系统_精髓与设计原理_第6版 - Chapter11-new
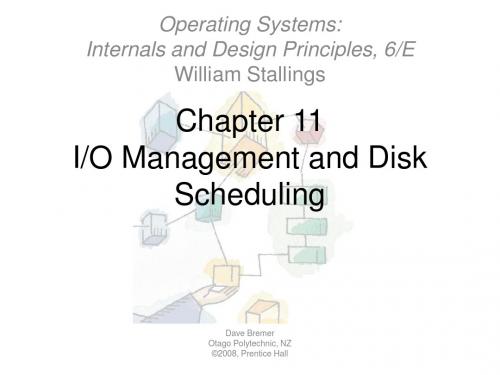
Human readable
• Devices used to communicate with the user • Printers and terminals
– Video display – Keyboard – Mouse etc
Direct Memory Address
• Processor delegates I/O operation to the DMA module • DMA module transfers data directly to or form memory • When complete DMA module sends an interrupt signal to the processor
DMA Configurations: Single Bus
• DMA can be configured in several ways • Shown here, all modules share the same system bus
DMA Configurations: Integrated DMA & I/O
Hierarchical design
• A hierarchical philosophy leads to organizing an OS into layers • Each layer relies on the next lower layer to perform more primitive functions • It provides services to the next higher layer. • Changes in one layer should not require changes in other layers
RTI Connext DDS User's Manual Version 2.3.3说明书
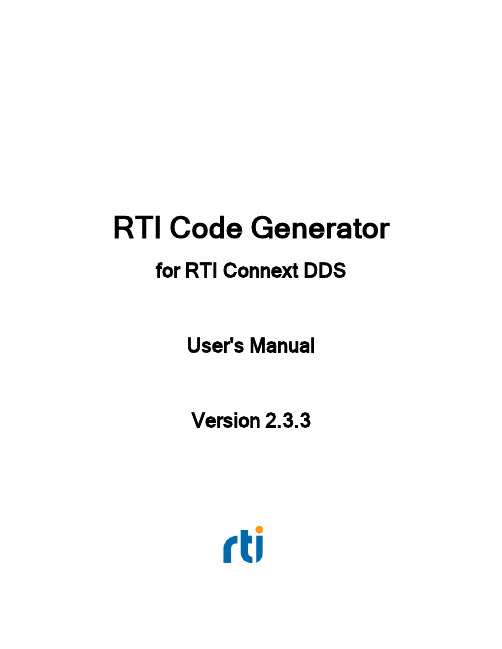
RTI Code Generator for RTI Connext DDSUser's ManualVersion2.3.3Copyrights©2016Real-Time Innovations,Inc.All rights reserved.Printed in U.S.A.First printing.April2016. TrademarksReal-Time Innovations,RTI,NDDS,RTI Data Distribution Service,DataBus,Connext,Micro DDS,the RTI logo,1RTI and the phrase,“Your Systems.Working as one,”are registered trademarks,trademarks or service marks of Real-Time Innovations,Inc.All other trademarks belong to their respective owners. Copy and Use RestrictionsNo part of this publication may be reproduced,stored in a retrieval system,or transmitted in any form (including electronic,mechanical,photocopy,and facsimile)without the prior written permission of Real-Time Innovations,Inc.The software described in this document is furnished under and subject to the RTI software license agreement.The software may be used or copied only under the terms of the license agreement.Technical SupportReal-Time Innovations,Inc.232E.Java DriveSunnyvale,CA94089Phone:(408)990-7444Email:***************Website:https:///Chapter1Introduction1 Chapter2Paths Mentioned in Documentation2 Chapter3Command-Line Arguments for rtiddsgen5 Chapter4Generated Files13 Chapter5Customizing the Generated Code17 Chapter6Boosting Performance with Server Mode20Chapter1IntroductionRTI Code Generator creates the code needed to define and register a user data type with Connext ing Code Generator is optional if:l You are using dynamic types(see Managing Memory for Built-in Types(Section3.2.7)in the RTI Connext DDS Core Libraries User’s Manual1).l You are using one of the built-in types(see Built-in Data Types(Section3.2)in the RTIConnext DDS Core Libraries User’s Manual).To use Code Generator,you will need to provide a description of your data type(s)in an IDL or XML file.You can define multiple data types in the same type-definition file.For details on these files,see the RTI Connext DDS Core Libraries User’s Manual(Sections3.3and3.4).1This document is provided with Connext DDS.You can also access it from the RTI Community’sDocumentation page.Chapter2Paths Mentioned inDocumentationThe documentation refers to:l<NDDSHOME>This refers to the installation directory for Connext DDS.The default installation paths are:l Mac OS X systems:/Applications/rti_connext_dds-versionl UNIX-based systems,non-root user:/home/your user name/rti_connext_dds-versionl UNIX-based systems,root user:/opt/rti_connext_dds-versionl Windows systems,user without Administrator privileges:<your home directory>\rti_connext_dds-versionl Windows systems,user with Administrator privileges:C:\Program Files\rti_connext_dds-version(for64-bits machines)orC:\Program Files(x86)\rti_connext_dds-version(for32-bit machines)You may also see$NDDSHOME or%NDDSHOME%,which refers to an environment variable set to the installation path.Wherever you see<NDDSHOME>used in a path,replace it with your installation path.Note for Windows Users:When using a command prompt to enter a command that includes the path C:\Program Files(or any directory name that has a space),enclose the path inquotation marks.For example:“C:\Program Files\rti_connext_dds-version\bin\rtiddsgen”or if you have defined the NDDSHOME environment variable:“%NDDSHOME%\bin\rtiddsgen”l RTI Workspace directory,rti_workspaceThe RTI Workspace is where all configuration files for the applications and example files are located.All configuration files and examples are copied here the first time you run RTI Launcher or any script in<NDDSHOME>/bin.The default path to the RTI Workspace directory is: l Mac OS X systems:/Users/your user name/rti_workspacel UNIX-based systems:/home/your user name/rti_workspacel Windows systems:your Windows documents folder\rti_workspaceNote:'your Windows documents folder'depends on your version of Windows.For example,on Windows7,the folder is C:\Users\your user name\Documents;onWindows Server2003,the folder is C:\Documents and Settings\your username\Documents.You can specify a different location for the rti_workspace directory.See the RTI Connext DDS Core Libraries Getting Started Guide for instructions.l<path to examples>Examples are copied into your home directory the first time you run RTI Launcher or any script in <NDDSHOME>/bin.This document refers to the location of these examples as<path to examples>.Wherever you see<path to examples>,replace it with the appropriate path.By default,the examples are copied to rti_workspace/version/examplesSo the paths are:l Mac OS X systems:/Users/your user name/rti_workspace/version/examplesl UNIX-based systems:/home/your user name/rti_workspace/version/examplesl Windows systems:your Windows documents folder\rti_workspace\version\examplesNote:'your Windows documents folder'is described above.You can specify that you do not want the examples copied to the workspace.See the RTI Connext DDS Core Libraries Getting Started Guide for instructions.Chapter3Command-Line Arguments forrtiddsgenOn Windows systems:Before running rtiddsgen,run VCVARS32.BAT in the same command prompt that you will use to run rtiddsgen.The VCVARS32.BAT file is usually located in<Visual Studio Installation Directory>/VC/bin.Alternatively,run rtiddsgen from the Visual Studio Command Prompt under the Visual Studio Tools folder.If you are generating code for Connext DDS,the options are:rtiddsgen[-help][-autoGenFiles<architecture>][-create<typefiles|examplefiles|makefiles>][-convertToIdl|-convertToXML|-convertToXsd][-D<name>[=<value>]][-d<outdir>][-disableXSDValidation][-dllExportMacroSuffix<suffix>][-enableEscapeChar][-example<architecture>][-express][-I<directory>][[-inputIdl]<IDLInputFile.idl>|[-inputXml]<XMLInputFile.xml>|[-inputXsd<IDLInputFile.idl>]][-language<Ada|C|C++|C++03|C++11|C++/CLI|C#|Java>][-namespace][-noCopyable][-notypecode][-obfuscate][-package<packagePrefix>][-platform<architecture>][-ppDisable][-ppPath<path to preprocessor>][-ppOption<option>][-reader][-replace][-sequenceSize<unbounded sequences size>][-sharedLib][-stringSize<unbounded strings size>][-U<name>][-unboundedSupport][-update<typefiles|examplefiles|makefiles>][-use42eAlignment][-use52CKeyhash][-use52JavaKeyhash][-V<name<[=<value>]][-verbosity[1-3]][-version][-writer]If you have RTI CORBA Compatibility Kit,you can use the above options,plus these: [-corba[CORBA Client header file]][-dataReaderSuffix<suffix>][-dataWriterSuffix<suffix>][-orb<CORBA ORB>][-typeSequenceSuffix<suffix>]If you are generating code for RTI Connext DDS Micro,the options are:rtiddsgen[-help][-create<typefiles|examplefiles|makefiles>][-convertToIdl|-convertToXML][-D<name>[=<value>]][-d<outdir>][-enableEscapeChar][-I<directory>][[-inputIdl]<IDLInputFile.idl>|[-inputXml]<XMLInputFile.xml>][-language<C|C++>][-micro][-namespace][-ppDisable][-ppPath<path to preprocessor>][-ppOption<option>][-reader][-replace][-sequenceSize<unbounded sequences size>][-stringSize<unbounded strings size>][-U<name>][-update<typefiles|examplefiles|makefiles>][-V<name<[=<value>]][-verbosity[1-3]][-version][-writer]Table3.1Options for rtiddsgen describes the options.Table3.1Options for rtiddsgenTable3.1Options for rtiddsgen8Table3.1Options for rtiddsgen9Table3.1Options for rtiddsgen10Table3.1Options for rtiddsgen11Table3.1Options for rtiddsgenNote:Before using a makefile created by Code Generatorto compile an application,make sure the ${NDDSHOME}environment variable is set as described in the RTI Connext DDS Core Libraries Getting Started Guide.12Chapter4Generated FilesThe following tables show the files that Code Generator creates for an example IDL file called Hello.idl.l Table4.1C,C++,C++/CLI,C#Files Created for Example“Hello.idl”l Table4.2Java Files Created for Example“Hello.idl”l Table4.3Ada Files Created for Example“Hello.idl”Table4.1C,C++,C++/CLI,C#Files Created for Example“Hello.idl”13Table4.1C,C++,C++/CLI,C#Files Created for Example“Hello.idl”Table4.2Java Files Created for Example“Hello.idl”14Table4.2Java Files Created for Example“Hello.idl”Table4.3Ada Files Created for Example“Hello.idl”15Table4.3Ada Files Created for Example“Hello.idl”16Chapter5Customizing the GeneratedCodeCode Generator allows you to customize the generated code for different languages by changing the provided templates.This version does not allow you to create new output files.You can load new templates using the following command in an existing template,where<pathToTemplate>is relative to the<NDDSHOME>/resource/app/app_support/rtiddsgen/templates folder:#parse(“<pathToTemplate>/template.vm”)If that template.vm file contains macros,you can use it within the original template.If template.vm contains just plain text without macros,that text will be included directly in the original file.You can customize the behavior of a template by using the predefined set of variables provided with Code Generator.For more information,see the tables in RTI_rtiddsgen_template_ variables.xlsx.This file contains two different sheets:Language-Templates and Template variables.The Language-Template sheet shows the correspondence between the Velocity Templates used and the generated files for each language.If,for example,we want to add a method in C in the Hello.c file, we would need to modify the template typeBody.vm under the templates/c directory.The scope of a template can be:l type:If we generate a file with that template for each type in the IDL file.For example inJava,where we generate a TypeSupport file for each type in the IDL.l file:If we generate a file with that template for each IDL file.For example in C,we generatea single plugin file containing all the types Plugin information.17l lastTopLevelType:If we generate a file with that template for the last top-level type in the IDL file.This is commonly used for the publisher/subscriber examples.l module:If we generate a file with that template for each module in the IDL file.This is used inAda,where there are files that contain all the types of a module.l topLevelType:if we generate a file with that template for each type in the idl file.This is used in ADA where the publisher/subscriber files are only generated for top level typesThe table also shows the top_level variables that can be used for that templates.These variables are explained in the sheet Template variables.For example in Java,the main unit of variables are the constructMap which is a hashMap of variables that represent a type.In C,we will have as the main unit the constructMapList,which is a List of constructMap.In the Template variables sheet,we can see which variables are contained in each constructMap,the constructKind or type that it is applicable to and the value that it contains depending on the language we use.One important variable that contains the constructMap for a type is the memberFieldMapList.This list represent the members contained within the type.Each member is also represented as a hashMap whose variables are also described in the Template variables sheet.Apart from that there are environmental or general variables that are not related with the types that are defined within a hashMap called envMap.Let’s see how to use these variables with an example.Suppose we want to generate a method in C that prints the members for a structure and,if it is an array or sequence,its corresponding size.For this IDL: module Mymodule{struct MyStruct{long longMember;long arrayMember[2][100];sequence<char,2>sequenceMember;sequence<long,5>arrayOfSequenceMember[28];};};We want to generate this:void MyModule_MyStruct_specialPrint(){printf("longMember\n");printf("arrayMember is an array[2,100]\n");printf("sequenceMember is a sequence<2>\n");printf("arrayOfSequenceMember is an array[28]is a sequence<5>");}The code in the template would look like this:##We go through all the list of types#foreach($node in$constructMapList)##We only want the method for structs#*--*##if($node.constructKind.equals(“struct”))void${node.nativeFQName}_specialPrint(){18##We go through all the members and call to the macros that check if they are array or sequences#*----*##foreach($member in$node.memberFieldMapList)print("$#isAnArray($member)#isASeq($member)\n");#*----*##end}#*--*##end#endThe isAnArray macro checks if the member is an array(i.e,has the variable dimensionList)and in that case,prints it:#macro(isAnArray$member)#if($member.dimensionList)is an array$member.dimensionList#end#endThe isASeq macro checks if the member is an sequence(i.e,has the variable seqSize)and in that case, prints it:#macro(isASeq$member)#if($member.seqSize)is a sequence<$member.seqSize>#end#endYou can add new variables to the templates using the-V<name<[=<value>]command-line option when starting Code Generator.This variable will be added to the userVarList hashMap.You can refer to it in the template as$ or$.equals(value).For more information on velocity templates,see /engine/releases/velocity-1.5/user-guide.html.19Chapter6Boosting Performance withServer ModeIf you need to invoke Code Generator multiple times with different parameters and/or type files, there will be a performance penalty derived from loading the JVM and compiling the velocity templates.To help with the above scenario,you can run Code Generator in server mode.Server mode runs a native executable that opens a TCP connection to a server instance of the code generator that is spawned the first time the executable is run,as depicted below:When Code Generator is used in server mode,JVM is loaded a single time when the server is started;the velocity templates are also compiled a single time.To invoke Code Generator in server mode,use the script rtiddsgen_server(.bat),which is in the scripts directory.The Code Generator server will automatically stop if it is not used for a certain amount of time. The default value is20seconds;you can change this by editing the rtiddsgen_server script and adjusting the value of the parameter,-n_servertimeout.20。
versapro编程软件用户手册
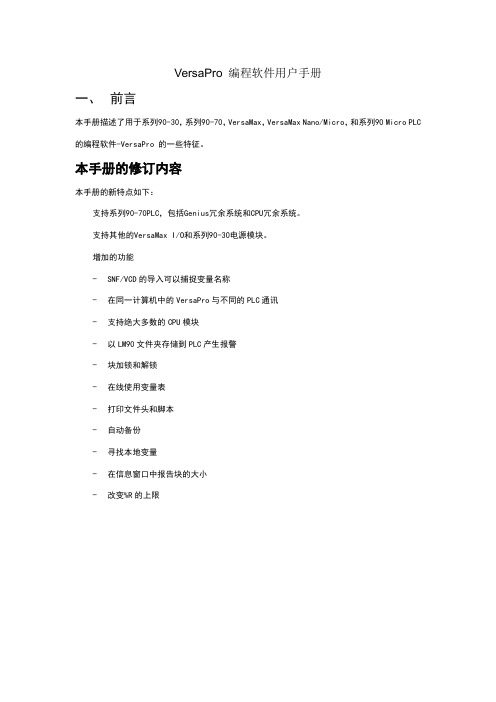
VersaPro 编程软件用户手册一、前言本手册描述了用于系列90-30,系列90-70,VersaMax,VersaMax Nano/Micro,和系列90 Micro PLC 的编程软件-VersaPro 的一些特征。
本手册的修订内容本手册的新特点如下:支持系列90-70PLC,包括Genius冗余系统和CPU冗余系统。
支持其他的VersaMax I/O和系列90-30电源模块。
增加的功能-SNF/VCD的导入可以捕捉变量名称-在同一计算机中的VersaPro与不同的PLC通讯-支持绝大多数的CPU模块-以LM90文件夹存储到PLC产生报警-块加锁和解锁-在线使用变量表-打印文件头和脚本-自动备份-寻找本地变量-在信息窗口中报告块的大小-改变%R的上限内容第一章介绍VersaPro简介1-1安装VersaPro软件1-2启动VersaPro软件Multiple Instances of VersaPro........................................................ 1-3 VersaPro的多请求Using VersaPro –Overview............................................................ 1-3使用VersaPro-概述The Workbench Window............................................................... .. 1-5桌面窗口Setting Workbench Options............................................................................ ..... 1-5设定桌面选项Setting Display Options............................................................................ ........... 1-7设定显示选项Setting Communications Options......................................................................... 1-8设定通讯选项Setting Ladder Options............................................................................ .......... 1-11设定梯图编辑选项Synchronizing VersaPro with HWC.............................................. 1-14VersaPro与HWC同步Chapter 2 Working WithFolders ............................................................ ...... 2-1第二章使用文件夹Creating and Opening VersaProFolders ..................................... 2-2创建和打开VersaPro文件夹Creating a New Folder............................................................... ...... 2-2创建一个新的文件夹To create a new, emptyfolder:............................................................................. 2-2创建一个新的空文件夹To create a new folder based on an existing VersaProfolder: ............................... 2-4创建一个新的基于现存的VersaPro文件夹To create a new folder, importing a Logicmaster 90Folder: ................................. 2-6创建一个新的,导入Logicmaster 90文件夹Importing Logicmaster Folders Earlier thanRelease ....................................... 2-7导入早于版本以前的Logicmaster文件夹When a folder contains a locked block................................................................. 2-8当文件夹包含一个加锁的块To create a new folder, importing logic from a Controlfolder: ............................. 2-9创建一个新的文件夹,从一Control文件夹中导入逻辑Rules for Folder Names and Nickname.............................................................. 2-11文件夹名称和绰号的命名规则Directory structure and files for a newfolder ..................................................... 2-12新文件夹的目录结构和文件Opening an ExistingFolder ...........................................................2-12打开一现存的文件夹Using the Folder Browser............................................................ 2-13使用文件夹浏览器Information Window............................................................... ...... 2-14信息窗口Context-sensitive Menu................................................................. 2-15上下文菜单Working with Windows in the VersaPro Workspace ..................... 2-16使用VersaPro窗口FolderOperations ......................................................... .............. 2-17文件夹操作Closing a VersaPro Folder............................................................. 2-17关闭VersaPro文件夹Saving aFolder .............................................................. ............... 2-17保存文件夹Folder............................................................... ............ 2-17删除文件夹Backing Up and Restoring............................................................. 2-18备份/恢复Backing Up a Folder ............................................................................ ............. 2-18备份文件夹Restoring a Folder fromBackup ........................................................................ 2-19恢复文件夹AutoBackup ............................................................................ ......................... 2-19自动备份Restricting FolderAccess .............................................................. 2-19限制文件夹的存取Locking aFolder ............................................................................ ................... 2-19锁定文件夹Folder............................................................................. ............... 2-21解锁文件夹Changing FolderProperties ........................................................... 2-22改变文件夹的属性Printing Your Program............................................................. .. 2-23打印程序Printing aReport .............................................................. ............. 2-23打印报告Printing Logic, Variable Declaration Table, or Information Window Contents............................................................. ........................... 2-25打印逻辑,变量声明表,信息窗口内容Custom Header/Footer Formatting................................................. 2-25设定文件头和脚本格式Chapter 3 Working With Blocks.............................................................. ...... 3-1第三章使用块Creating, Inserting and OpeningBlocks .......................................... 3-2创建、插入、打开块Creating a Subroutine......................................................................... ................. 3-2创建子程序Creating an External CBlock .............................................................................. 3-3创建外部块90-30 Timed Interrupt Block NameRequirements ............................................... 3-490-30定时中断块命名要求Name FieldRestrictions ...................................................................... ................ 3-4命名区的限制Description Field Restrictions....................................................................... ....... 3-4描述区的限制Inserting a Block from Another Folder................................................................. 3-5从另一个文件夹插入一个块Opening a Block.................................................................................................. 3-6打开一个块Maximum Block Size............................................................................... ........... 3-7最大的块容量Inserting and EditingObjects .......................................................... 3-7插入、编辑目标Working with Blocks and Objects in the Folder Browser ........... 3-8在文件浏览器中使用块和目标ArrangingBlocks ............................................................................ .................... 3-8安排块Viewing and Modifying BlockProperties ............................................................ 3-8查看、改变块属性Moving FolderComponents .......................................................... .. 3-9移动文件夹的内容Cutting Folder Components......................................................................... ...... 3-10剪切文件夹内容Copying FolderComponents ........................................................................ ..... 3-10拷贝文件夹内容Pasting FolderComponents ........................................................................ ....... 3-10粘贴文件夹内容Dragging and Dropping in the FolderBrowser ................................................... 3-11拖拉文件夹浏览器Deleting a Block................................................................ ............ 3-12删除块To delete a block or ViewTable: ....................................................................... 3-12删除块或查看表To delete the contents of the _MAIN block:....................................................... 3-12删除主程序块的内容Saving aBlock ............................................................... ............... 3-13保存块To save ablock ............................................................................. .................... 3-13保存块ClosingBlocks .............................................................. ................ 3-13关闭块Using Editing Operations on Folder Components .......................... 3-14使用编辑操作Using Undo andRedo .............................................................................. ......... 3-14使用Undo和RedoWorking with Variables while EditingBlocks ............................... 3-14编辑块的同时使用变量Searching and Replacing in VersaPro........................................ 3-16在VersaPro中进行寻找和替代操作Navigating inVersaPro ............................................................ ..... 3-16在VersaPro中浏览Locating and going to a Rung, Row or Variable................................................. 3-16定位到梯段,行,或变量Locating Names, Instructions, Addresses or Call Statements in Blocks............... 3-17定位到名字,指令,地址或调用指令Using Search andReplace ........................................................................... ...... 3-18使用搜寻和替代Performing SyntaxChecks ......................................................... 3-20执行语法检查Converting Block Logic............................................................... 3-21转换块逻辑Assigning Conversion Variables........................................................................ 3-21分配转换变量Converting BlockLogic ............................................................................. ....... 3-22转换块逻辑Rules for Successful Conversion........................................................................ 3-22转换成功的规则Converting Non-Nested Instructions.............................................. 3-23转换嵌套指令Chapter 4 Working in the LD Editor............................................................. 4-1第四章使用LD编辑器Overview of the LD Editor............................................................4-2LD编辑器概述Customizing the LD Editor Window............................................ 4-3定制LD编辑器窗口Customizing Ladder DisplayOptions .............................................. 4-3定制梯图显示选项Adjusting the ZoomRatio ............................................................... 4-4调节大小比例Instructions and InstructionParameters ..................................... 4-5指令和指令参数EnteringInstructions ....................................................... ............. 4-6输入指令Entering Instructions using the Function Toolbar............................. 4-6用功能工具条输入指令Selecting Functions Using the Function Toolbar –Compact................................. 4-6使用功能工具条-紧凑型中选择功能Selecting Functions Using the Function Toolbar –Expanded ............................... 4-7使用功能工具条-扩展型中选择功能Entering Instructions using the LadderToolbar ............................... 4-8使用梯图工具条输入指令Entering Instructions using KeyboardEntry .................................... 4-9使用键盘输入指令Completing Address and LengthInformation .................................. 4-9完成地址和长度信息DATA_INITFunctions ........................................................... ...... 4-10DATA_INIT功能Completing Function BlockParameters .................................... 4-11完成功能块参数Assigning Variables to Function Block Parameters........................ 4-11分配变量给功能块参数Assigning Constants to Function Block Parameters .......................4-13分配常数给功能块参数Editing LadderLogic .............................................................. .... 4-15编辑梯图逻辑SelectingLogic ............................................................... .............. 4-15选择逻辑Using Cut, Copy, Paste and Delete in the LD Editor...................... 4-16在LD编辑器中使用剪切,拷贝,粘贴和删除Inserting an empty row in the LDEditor ....................................... 4-17在LD编辑器中插入一空行To go to the next coil:................................................................ .... 4-17转到下一个线圈Converting Logic Between PLC Families................................... 4-18在PLC不同产品中转换逻辑Converting Logic from 90-30/VersaMax to 90-70......................... 4-18把90-30/VersaMax的逻辑转换为90-70Tips for Converting 90-30 Logic to 90-7090-30转换为90-70的窍门Issues in Logic Converted from 90-30 to90-70.................................................. 4-2090-30转换为90-70的结果Converting Logic from 90-70 to 90-30/VersaMax ......................... 4-2190-70转换为90-30/VersaMax逻辑Tips for Converting 90-70 Logic to 90-30Logic ................................................ 4-2190-70转换到90-30逻辑的窍门Issues in Logic Converted from 90-70 to90-30.................................................. 4-2390-70转换为90-30的结果Differences in Language Capability in VersaPro: 90-70 vs. 90-30/VersaMax.......................................................... ....................... 4-2490-70与90-30/VersaMax在VersaPro中在梯图语言的区别Instruction SetDifferences ....................................................................... ......... 4-24指令设置区别Other LanguageDifferences ....................................................................... ....... 4-25其他区别Chapter 5 Working in the Instruction List第五章使用语句表编辑器(IL)Overview of the Instruction ListEditor ........................................... 5-2语句表编辑器概述Customizing the IL Editor Display....................................................................... 5-3定制IL显示Entering Instructions and Instruction Parameters...................... 5-4输入指令和指令参数Selecting theInstruction ....................................................................... ............... 5-4选择指令Completing Address and Length Information....................................................... 5-5完成地址和长度信息Completing InstructionParameters ......................................................................5-5完成指令参数Assigning Variables to Instruction Operands........................................................ 5-5分配指令以变量Assigning Constants to InstructionOperands ....................................................... 5-7分配指令以常数Using Cut, Copy, Paste and Delete in the ILEditor .............................................. 5-8在IL编辑器中使用剪切,拷贝,粘贴和删除Instruction List Operation............................................................ 5-9指令列表Constants ........................................................... ........................... 5-11常数Functions............................................................ ........................... 5-12功能FunctionBlocks .............................................................. .............. 5-12功能块PLC Built-in Functions and Function Blocks................................. 5-13PLC内置功能和功能块Nesting of BooleanExpressions .................................................... 5-17布尔操作的嵌套Using ENO.................................................................. .................. 5-18使用ENODefining TemporaryVariables ...................................................... 5-19定义临时变量Error Checking............................................................. ................. 5-19错误检查Chapter 6 Working with the Variable Declaration Table............................. 6-1第六章使用变量声明表Overview of the Variable Declaration Table................................ 6-2变量声明表的概述Variable Table FieldDescriptions ................................................... 6-3变量表区域描述ReservedWords ............................................................... ............... 6-4保留字Creating and ModifyingVariables ............................................... 6-5创建,改变变量To create a new variable or modify an existing variable in the VDT: .................... 6-5在VDT表中创建新的变量或改变存在的变量Navigating in theVDT .................................................................. 6-6在VDT中搜寻Navigating Using the Mouse orKeyboard ........................................................... 6-6使用鼠标或键盘搜寻Locating a Variable using the Go To Variable DialogBox ................................... 6-7使用Go To变量对话框定位变量Using Cut, Copy, Paste and Drag and Drop in the VDT................... 6-8在VDT中使用剪切,拷贝和拖曳功能To cut, copy and paste in the Variable DeclarationTable: .................................... 6-8在VDT中剪切,拷贝,粘贴Details on Cutting and Copying...........................................................................6-9在剪切和拷贝中的细节Details on Pasting............................................................................ .................... 6-9在粘贴中的细节To drag and drop-cut in the VDT:...................................................................... 6-10在VDT中拖曳剪切To drag and drop-copy in theVDT: ................................................................... 6-10在VDT中拷贝Details on Dragging and Dropping in theVDT .................................................. 6-11在VDT中拖曳的细节To drag and drop from the VDT to the LD or IL Editor:..................................... 6-11从VDT拖曳到LD和IL编辑器中Details on Dropping a Variable into an Editor.................................................... 6-11拖曳变量到变量表的细节Variable Declaration TableUtilities ........................................... 6-12变量声明表的应用Sorting Variables.......................................................................... ..................... 6-12排序变量Deleting Variables and the Contents of a VDT................................................... 6-12删除变量及内容Saving Edits in the VDT................................................................................ .... 6-13在VDT中保存编辑Compacting theVDT ................................................................. ... 6-14压缩VDTTo compact the VDT:............................................................................... ......... 6-14压缩VDTViewing the Highest References Used........................................... 6-14查看使用的最大变量Finding UnusedVariables ........................................................... .. 6-14寻找未使用的变量Creating a Cross-Reference Listing ofVariables ........................... 6-14创建变量的交叉参数列表To view online usetables ............................................................................ ...... 6-14查看再线使用表Importing and ExportingVariables ........................................... 6-15引入和导出变量To import avariable: ........................................................... .......... 6-15引入变量Details on Importing an SNFFile .................................................. 6-15引入SNF文件的细节To export a variable:............................................................ .......... 6-16导出变量Details on Exporting an SNFFile .................................................. 6-17导出SNF的细节Variable Resolution.......................................................... ........... 6-18变量的分辨率Chapter 7 Configuring PLCHardware .........................................................7-1第七章配置PLC硬件Accessing Hardware Configuration ..................................... 7-2存取硬件配置Hardware Configuration Toolbar..................................................... 7-3硬件配置工具条Using the Parameter Editor..............................................................7-3使用参数编辑器EditingTips .............................................................................. .......................... 7-4编辑窍门Undoing Changes............................................................................ .................... 7-5撤消改变Error Notification....................................................................... ......................... 7-5错误报告Correcting Errors............................................................................. .................... 7-6修正错误Shortcut Keys forHWC .................................................................. 7-6HWC的快捷键Setting Options in Hardware Configuration..................................... 7-7在硬件配置中设定选项Configuring a Series 90-30 or 90-70 Rack.................................... 7-8配置系列90-30或90-70的机架Changing Rack Type................................................................. ...... 7-8改变机架类型Changing/Configuring Power Supply and CPU............................... 7-9改变/配置电源模块和CPUConfiguring a Power Supply............................................................................. ... 7-9配置电源模块Configuring a CPU................................................................................ .............. 7-9配置CPUAdding/Configuring RackModules ............................................... 7-10增加/配置机架类型Configuring ExpansionRacks ....................................................... 7-10配置扩展机架Deleting RackModules ............................................................. .... 7-11删除机架模块Clearing theRack................................................................. ......... 7-11清除机架Genius Bus Configuration (Series 90-70PLCs) ............................. 7-12Genius总线配置(系列90-70 PLC)Bus Operations........................................................... ................... 7-12总线操作Block Operations........................................................... ................ 7-13块操作CPU Redundancy........................................................... ............... 7-14CPU冗余Configuring CPU Redundancy Over Genius.................................. 7-14配置Genius网的CPU冗余CPU Redundancy Over Genius Types................................................................ 7-14Genius网的CPU冗余General Procedure for Configuring CPU Redundancy Over Genius.................... 7-15 Genius网的CPU冗余配置过程Use of Rack 7 in CPU Redundancy Over Genius Schemes.................................7-15CPU冗余中机架7的使用Storing the Configurations to the PLCs.............................................................. 7-16保存配置到PLC中Configuring the Genius Redundant System................................... 7-17配置Genius冗余系统Genius RedundancyTypes ............................................................................. ... 7-17Genius冗余系统General Procedure for Configuring the Genius Redundant System ..................... 7-17Genius冗余系统的配置过程Configuring the Second PLC.............................................................................7-18配置第二个PLCConfiguring a VersaMax Modular Rack.................................... 7-19配置VersaMax模块机架Changing/Configuring the CPU..................................................... 7-20改变/配置CPURack/Module Operations...........................................................机架/模块操作Changing/Configuring the Power Supply........................................................... 7-21改变/配置电源模块Adding Module Carriers........................................................................... ......... 7-21增加模块机架Adding Power Supply BoosterBases ................................................................. 7-21增加电源提升模块的机架Configuring a Power Supply for a Booster Base................................................. 7-22配置提升机架的电源模块Adding/Configuring Modules............................................................................ 7-22增加/配置模块Configuring ModuleParameters ........................................................................ 7-22配置模块参数Deleting RackModules ........................................................................... .......... 7-23删除机架模块Carriers........................................................................... .................... 7-23删除机架Clearing the Rack............................................................................... ............... 7-23清除机架Configuring VersaMax ExpansionNetworks ..................................................... 7-23配置VersaMax扩展网络Loading VersaMax Hardware Configurations................................ 7-27载入VersaMax硬件配置VersaMax Modules with Shared IDs.................................................................. 7-27带共享ID的VersaMax模块Configuring a VersaMax Remote I/O Rack............................... 7-28配置VersaMax远程I/O机架Configuring anNIU ................................................................. ..... 7-28配置NIUConfiguring the RIO Rack.............................................................7-29配置远程I/O(RIO)机架Load/Store/Verify RIOConfiguration ........................................... 7-29载入/保存/校验RIO配置Communications Setup.............................................................................. ........ 7-29通讯设置Storing RIOConfiguration ..................................................................... ........... 7-30保存RIO配置Loading RIO Configuration...................................................................... ......... 7-31载入RIO配置Verifying RIO Configuration......................................................... 7-31校验RIO配置Clearing RIOConfiguration ....................................................... ... 7-32清除RIO配置Ethernet Global Data Configuration.......................................... 7-33以太网全局数据(EGD)配置Configuring the Ethernet Interface Adapter Name (CPU364) ........ 7-34配置以太网接口适配器的名字(CPU364)Setting Aliases for Remote Ethernet Interfaces (CPU364 andCMM742) ............................................................. ........................ 7-34设定远程接口的别名(CPU364和CMM742)Name Resolution and Routing Table Configuration (CPU364 andCMM742) ............................................................. ........................ 7-34名字解析和路由表配置(CPU364和CMM742)Defining EGD Exchanges............................................................ .. 7-36定义EGD交换PLC TimingConsiderations ...................................................... .... 7-37PLC定时考虑Ethernet Global Data: Example1 .................................................. 7-38EGD范例1Ethernet Global Data: Example2 .................................................. 7-39EGD范例2Restoring EGD and Name Resolution............................................ 7-41保存EGD和名字解析Advanced User Parameters for EGD (CPUE05) ............................ 7-41EGD的高级用户参数(CPUE05)Configuring VersaMax Nano and Micro PLCs.......................... 7-43配置VersaMax Nano和Micro PLCRack/Module Operations........................................................... .... 7-43机架/模块操作Counter, Pulse Width Modulation, and Pulse Train Output............ 7-44计数器,PWM,和PTO输出PWM Frequency and Duty Cycle....................................................................... 7-44 PWM频率和周期Configuring Averaging Filter for Analog Input Potentiometers...... 7-44配置模拟输入电位器的滤波器Configuring Series 90 Micro PLCs............................................. 7-45配置系列90 Micro PLCRack/Module Operations........................................................... .... 7-45机架/模块操作Counter, Pulse Width Modulation, and Pulse Train Output............ 7-46计数器,PWM和PTO输出PWM Frequency and Duty Cycle....................................................................... 7-46PWM频率和周期Configuring Averaging Filter for Analog Input Potentiometers...... 7-46配置模拟输入电位器的滤波器Hardware Configuration Reference View.................................. 7-47硬件配置参考区(CRV)Hardware Configuration Log View............................................ 7-48硬件配置登录区Hardware Configuration Power Consumption View................. 7-49硬件配置电源损耗区Power Consumption LimitCalculations ........................................ 7-49电源消耗计算Components of the Power Consumption View............................... 7-51电源消耗区的组成Converting RackSystems ...........................................................7-52转换机架系统Printing Hardware Configuration.............................................. 7-52打印硬件配置Chapter 8 Using Reference and Variable ViewTables ................................. 8-1第八章使用参考和变量浏览表。
CarSim_Quick_Start

CarSim Quick Start GuideVersion 8 Mechanical SimulationTable of Contents1. Introduction (3)CarSim Uses (3)Computer Requirements (3)Notation Conventions in This Guide (4)2. Working with CarSim: A Tutorial (5)Before You Start (5)Start CarSim and Create a New Database (5)The CarSim Run Control Screen (Home) (9)Use the Animator (13)View Plots (16)Run with a New Procedure (18)Run a Vehicle with a New Load Condition (23)Run a Vehicle with a Modified Suspension (29)Make a New CarSim Desktop Shortcut (38)3. More CarSim Features (39)Restart CarSim (39)Get More Information (39)Make New Plots (46)Manage Multiple Databases (50)Browse the Database (56)About Procedures (59)4. What's Next (65)NOTICEThis manual describes software that is furnished under a license agreement and may be used or copied only in accordance with the terms of such agreement. BikeSim, CarSim, TruckSim, VehSim, and VehicleSim are registered trademarks of Mechanical Simulation Corporation.© 1996 – 2011, Mechanical Simulation Corporation.Last updated June 2011.1. IntroductionThis guide is intended to help introduce you to CarSim. You should have CarSim installed on your computer, but no experience with using the software is assumed. If you do have some experience with CarSim, it is still a good idea to go through this guide; most people learn something from this process, even if they have used CarSim before. It typically takes about an hour and a half to cover the basics in Chapter 2 and less than an hour to cover additional features in Chapter 3.CarSim UsesCarSim predicts the performance of vehicles in response to driver control inputs (steering, throttle, brakes, clutch, and shifting) in a given environment (road geometry, coefficients of friction, wind). By performance, we mean vehicle motions, forces, and moments involved in acceleration, handling, and braking. Just about any test of a vehicle that would be conducted on a test track or road can be simulated.You can study changes in vehicle behavior that result from modifying any of the hundreds of vehicle parameters, control inputs, or the driving environment. You can add vehicle elements and systems like controls (ABS, traction control, stability control) to the vehicle and use them to develop control algorithms.Limits CarSim does not include structural flexibility, acoustics, or highfrequency vibrations. It is therefore not used to study noise or structuredeformations. Nor is it used to simulate individual component loads fordurability analysis, because in most cases CarSim models have internalequations for system behavior (e.g., total suspension motion), rather thancomponent behavior (e.g., tie rod or control arm motions).You can visit Mechanical Simulation’s website at for more information on applications, or help in deciding if CarSim is the appropriate tool to study a particular problem. Computer RequirementsCarSim runs on Intel PC’s (or equivalent) equipped with Windows 2000, XP, and Vista. It is self-contained, requiring no additional programs or tools to run.Note CarSim math models can be extended using other simulation tools suchas MATLAB®Simulink®, LabVIEW™, custom C code, etc. Thisintroductory guide does not cover the topic of extending CarSim models.Chapter 1 Introduction CarSim runs on 32-bit and 64-bit versions of Windows. Both versions of the math models run atabout the same speed; the main reason for using the 64-bit option is for compatibility with other64-bit software (e.g., the 64-bit version of Simulink).Most modern PC’s are well equipped to run CarSim. Here are some general guidelines when considering computer hardware options:·Speed: Get a fast CPU (it should run at a minimum of 1 GHz; faster is recommended). Although vehicle models run faster than real time, you will save timewith a fast CPU. Level-2 cache is also important for speeding up runs.·Graphics: Get a graphics card that supports OpenGL 2.0 hardware acceleration, with at least 128 MB of video memory (NVIDIA, ATI, or similar).·Storage: CarSim requires a little under a gigabyte (GB) of disk storage. With extensive use, you will need several GB.Notation Conventions in This GuideThe bulk of this guide is presented using a Times font. Other fonts and styles are used to convey special meanings.·The Courier font is used for names of computer files and folders.·Bold identifies items on the screen that you might click on—buttons, menu items, names of linked datasets, etc. Titles of screens are also shown in bold.·Underline is used to indicate text that you might type. For example, “In the speed field, type 160.”·The instruction to click a control means use the left mouse button; if the right-hand button is to be used, the instruction will be to right-click the control.2. Working with CarSim: A TutorialThis chapter has a hands-on tutorial for working with CarSim. You will view simulation results with the CarSim animator and plotter and make new runs with modified controls and vehicle properties. Most sections in this chapter have short, step-by-step instructions that you can follow along to quickly perform basic actions in CarSim. All sections conclude with a short Review summarizing the important concepts you have covered.Before You StartBefore starting, there are just a few requirements.1. CarSim must be installed on your computer. If you have not yet installed CarSim, loginto your computer as an administrator, insert the CD (or if it is downloaded, launch the program Setup_CarSim_8.0.exe), and follow the instructions.2. You must have a license key to run CarSim. You will have one of these three setups:a. a license file is matched with a hardware key plugged into a USB port of thecomputer (the key is called a dongle), orb. a license file is matched with hardware specific to your machine, orc.you are connected to a network with a license server.3. You must know where CarSim is located on your machine. The software is organized intwo parts.a. A folder with a name such as CarSim80_Prog is typically installed in yourProgram Files folder. You will need to locate this folder in one of the firststeps below.b.There is at least one database folder with a name such as CarSim_Data whoselocation is specified during installation. (The default location is in your folder MyDocuments). You must have full read and write access for this folder.Start CarSim and Create a New Database1.Start CarSim with one of these methods:e the start menu shortcut: Programs->CarSim 8.0->CarSim.b.Or, double-click on the CarSim icon on your desktop.c.Or, double-click the file carsim.exe in the CarSim80_Prog folder.12Figure 1: Select a database.Option In the unlikely event that there is no recently used database folder, click the button Browse for another database and jump to step 4.2.Click the button Continue with the selected database2Figure 2. Popup window with license settings.CarSim will start and show you a window whose title begins with the name CarSim Run Control (Figure 3).Figure 3. Make a new database from a consolidated parsfile.3.Select the File menu item New Database from a Consolidated Parsfile.4.The Windows file navigation window will appear (Figure 4). Navigate to theCarSim80_Prog folder installed on your machine (typically in C:\Program Files), and continue into Resources\Import_Examples to find the file Quick_Start.cpar. Select this file and click the Load button.Figure 4. Select the Quick_Start consolidated parsfile.5.CarSim will ask you to browse for a folder for the new database (Figure 5).234Figure 5. Create and select an empty folder for the new CarSim database.Shared Documents)and click thesuch as New Folderas CarSim_Data_QS OKA terminal window will pop up briefly listing files that are being copied. It will quickly disappear.Note If your installation is set to show license options, you will again view a pop-up window with these options (Figure 2). Whenever this happens,you can just click the Select button to continue. (If you are not using anetwork license server, you can first check the box to avoid the windowin future startups.)CarSim will take a minute or two to create the new database.Review You have just gone through the steps needed to create a clean database with only the data needed for the instructions that follow in this guide.Although these extra steps took a few minutes, they were done toensure that what you see on your screen matches the description in thisguide and to guard against modifying the examples installed in themain CarSim database while you are going through the initial learningprocess.The next time you startCarSim, you willcontinue workinginthis database. The steps you have just taken for creating a new database are used mainly when starting a major new project; they are not repeatedfor routine use.The CarSim Run Control Screen (Home)CarSim opens with a view of the Run Control screen (Figure 6).12458Figure 6. The CarSim Run Control screen.1. The CarSimscreen displays a dataset from a library . The window title bar identifies thelibrary name and the full name of the dataset. In Figure 6, the library name is CarSim RunControl * * Quick Start Guide Example , and the title of the datasetin that category is 2. Use the View menu to set the window size for your preference.3. Right-click and hold on the Sidebar45Figure 7. Right-click and hold for information about a button or other control. Note The right mousebutton is used to obtain information and tips.Right-click and hold on buttons, fields, and various control objects to obtainshort summaries of their functions.into view.4. Click the Linked Datatab (Figure 6), then the Notes tabto switch between the twosidebar functions. Leave the tab selected.5. Right-click and hold on the Lock buttonInitially, the dataset you view just aftercreating a new database is locked. Several visual cues indicate it is locked:a. The title of the window has a suffix [L][L] disappears.b. (light gray in the Windows XP theme that was in usewhen these figures were made); when unlocked they have yellow backgrounds.c.The Lock button show an image of a closed lockwhen unlocked the imageshows an open lock. d.Buttons might be dimmed, such as the Run Math Model e. 6.Click on the Lock button to unlock the dataset. Notice how the screen appearance changes to visually indicate that it is no longer locked. 7. Click the Help button This launches the Adobe Reader program, with a PDFHelp can be used to access this and many other documents that are installed withCarSim.Figure 8. On-line documentation about the current screen.Note The Help menu has the command Search Help, which brings up a window in Adobe Reader for searching the entire set of CarSimdocuments. This is the primary tool for finding information quickly fromover 1000 pages of documentation provided with the software.After you have gone through this tutorial, you can return to the documentation for the Run Control screen. For now, close the PDF window and continue with the next step.8. Right-click and hold on the Run Math Model button (Figure 6) for an on-linedescription of the button, then click the Run Math ModelCarSim. A status bar appears to show the progress of the run (Figure 9). The CarSim math model runs significantly faster than real time, so this will just take a few seconds.When the run is complete, the (Figure 10). Now that there are simulation results to view, theand buttons are active.Figure 9. Progress bar that is displayed during a simulation run.2Figure 10. The Animate and Plot buttons are active after a run is made. Review You have seen on-line help available by right-clicking, typing F1, using the Help button, and using the Help menu.You have just made your first simulation run in CarSim from the RunControl screen. This screen is the starting point to access the maintools and datasets in CarSim, as indicated graphically in Figure 11. Thenext two sections will cover the animator and plotter.Figure 11. The four main components of CarSim.Use the AnimatorThe animator combines the results of a simulated test run (made with a CarSim math model) with a simulated video camera.1. Right-click on the Animate button on the CarSim Run Controlfor an on-line description of the button.2. Click the Animate button. A new window will appear showing an animation of the run(Figure 12.). For all of the following instructions involving the animator, numbered items refer to this figure.Note If the animator fails to run or runs very slowly, you might need to adjust Windows settings for your computer. (This is more likely to be problemwith laptops that have lower-quality graphic accelerator hardware.) Ifthere is a serious problem with the animator display, please see theAnimator Performance tech memo (use the CarSim Help menu and goto the Technical Memos submenu).123456Figure 12. The animator screen.3.Click and hold a mouse button down in the animation display region and use simplesweeping motions to move the simulated camera:a.Click and hold anywhere in the viewing area with the left mouse button, then sweepleft and right to circle around the vehicle.b.Click and hold anywhere in the viewing area with the left mouse button, then sweepup and down to move the camera up and down.c.Click and hold anywhere in the viewing area with the right mouse button, then sweepup and down to move the camera closer or further away from the vehicle.d.Hold the shift key down and Click and hold in the viewing area with the left mousebutton. Sweep in any direction to “drag” the viewing area. This changes the aim ofthe camera, while leaving its position unaffected. A cross-hair will appear to showyou the new location.e.If you have a three-button mouse or clickable scroll wheel, press the middle buttonand sweep up and down to zoom in and out. (This does not move the camera, butchanges the camera zoom.)f.If you have a three-button mouse or clickable scroll wheel, hold the shift key downand at the same time press the middle button and sweep up and down. This does asimultaneous zoom and tracking (moving the camera), keeping the image of thevehicle about the same size, while changing the perspective effects related todistance. (Move down to simultaneously track back and zoom in; move up to track inand zoom out.)4.Notice the slider at the bottom of the screenvisible in your window, select the View menu item Toolbar to display them). As thesimulated run.a.Pause the animation by clicking the pause button or pressing the space key onyour keyboard.b.Play the animation forward by clicking the forward arrow (near or typingCtrl+®.c.¬.d.e.When the animator is stopped, use the ® or ¬ keys to jump the slider forward orbackward in the animation.f.Type Ctrl+S to play the animation from the start.5.a.While the animation is playing, move the slider bar down to see the effect of slowmotion. You can also move the bar with the ¯ and - keys. If you have a mouse with ascroll wheel, you can also use the scroll wheel to move the slider.b.Bring the animation speed to extreme slow motion, such that the spin of the wheels isbarely visible. Toggle between forward and reverse using Ctrl+® and Ctrl+¬.6.a.b.7.The animator can display shapes using solid surfaces or wireframes. Use the View menuitem Wireframe Rendering or type the keyboard command Ctrl+W to toggle between viewing modes.8. If the animator is still playing in slow motion, restore it to full speed. Then exit the animatorby clicking the X button in the upper-right corner of the window. You should once again be viewing the CarSim Run Control screen (it may have been hidden in the background).Review You have now gone through the main interactive controls for using theanimator. The following sections assume you are comfortablemanipulating the view and working with the animator. For morecomplete reference information, use the CarSim Help menu and accessthe Surface Animator reference manual (in the Reference Manualssubmenu).View PlotsAlthough the animator offers a quick way to see a simulated test such as the example double lane change, the plotter is the tool most frequently used to study the vehicle behavior. The plotter in CarSim is called WinEP (Windows-based Engineering Plotter).1.From the same Run Control screen, click the Plotseconds, the WinEP window will appear showing plots for of interest in the simulated double lane-change procedure (Figure 13).Figure 13. Viewing plots in WinEP.2.If this is the first time the plotter has been run on this computer, make some optionaladjustments for better viewing.a. Check to see if the toolbar is visible (Figure 14). If the toolbar is not visible, show itusing the View menu.Figure 14. Toolbar for WinEP.b. If the plot window is small, enlarge by double-clicking the title bar or clicking thezoom box in the upper-right corner of the window, then click a tile button , orselect a Tile option from the Window menu. After you exit the plotter, it will open inthe new layout the next time.3.Each individual sub-window can be moved or re-sized as desired. For example, try zoomingone of the plot windows by clicking the maximize box in its upper-right corner. Press the Page-Down key to cycle through the open plots. Other options for controlling and navigating among the windows are provided under the Window menu.4.To view a portion of any plot in more detail, click and hold the left mouse button in the plotarea and drag to create a rectangle. When you release the button, the plot will be re-drawn to show the region you selected.5.To restore the original scaling, type Ctrl+R (a shortcut for the Format menu item RedrawOriginal Scale). Alternatively, click the toolbar button .6.The plotter can show the numerical X and Y values for any point in any plot.a.Type Ctrl+D (a shortcut for the Data menu item Show Data Points), or, click thetoolbar button . A cross-hair cursor appears on the first point in the first dataset inthe active plot window.Note The cross-hair cursor is at the left edge of the plot area. Because it linesup with the vertical axis, it is hard to see until it has been moved.The values of the X and Y variables are shown in the status bar (the strip at thebottom of the window).The cursor position is controlled by key presses or by selecting an item from theData menu (under the sub-menu Cursor Position Info). Although the menu isfunctional, it is mainly used as a form of on-line documentation for reminding youwhich keys can be used to control the cursor.b.Move the cursor to the right using the ® key. Move it more quickly using the Shiftkey and ® key together (Shift+®). Move it even more quickly using Ctrl+Shift+®.To move to the left, use ¬, Shift+¬, and Ctrl+Shift+¬. There are also buttons inthe toolbar for moving the cursor in several increments:c.To move the cursor to a different dataset in the same plot (assuming the plot has anoverlay of two or more datasets), hit the Tab key or click the toolbar button .e the Home and End keys to jump to the beginning and end of the plot. Or, use thetoolbar buttons and .e the -and ¯keys to jump to the maximum and minimum values. Or, use thetoolbar buttons and .7.Exit WinEP to return to the CarSim Run Control screen.Review You have now gone through the main interactive controls for using theplotter. The following sections assume you are comfortable zoomingand viewing numerical values using the cross-hair cursor. For morecomplete reference information, use the CarSim Help menu and accessthe Plotter reference manual (in the Reference Manuals submenu).Run with a New ProcedureYou should be viewing the CarSim Run Control screen with the dataset named Baseline in the category Quick Start Guide Example, shown again in Figure 15.13Figure 15. The CarSim Run Control screen (repeated from earlier figure).In the previous sections, you ran the vehicle in a baseline condition, and viewed results using the animator and plotter. To do this, you used the three main buttons shown in Figure 15: Run Math Model, Animate, and Plotpart of the screen under the title Vehicle and Procedure(items and These link to datasets that define the properties of the simulated vehicle and procedure. In this section, you will run a new test with a new procedure involving a different test speed.1.Right-click the New Newfor the new test (Figure 16). Enter a new name in the title field: My New TestSet button to set the new name. The new screen display is nearly identical to the previous one, except that the Animate and Plot buttons are dimmed because the run has not yet been made (compare Figure 15 and Figure 17).12Figure 16. Setting the name of a new dataset.2. Notice the three controls that are identified by circled numbers in Figure 17. All threeinvolve another dataset that is linked to this one. Right-click on each for on-line descriptions:123Figure 17. The CarSim Run Control screen for a new dataset.a.The top control labeled Procedure is used to clear the link or switch to adifferent library of datasets.b.The blue button is a link to a dataset. Right-click to identify the library(Procedures), ({Handling Testing} DLC @ 120 km/h), and theunderlying file name (depends on the installation details).c.next to the blue button is used to select a dataset from thelinked library or create a new dataset and immediately link to it.Note These three controls all relate to the same linked dataset. Similar groups of three controls are used throughout CarSim. In future instructions, thegroup of three controls is sometimes identified as one item to shorten thedescriptions.3. Use the drop-down dataset selector (under Procedure)and choose the option to Copyand Link Dataset (Figure 18).123Figure 18. Use the Copy and Link Dataset command to copy the existing dataset.This action brings up a dialog box where you set the title and category for the dataset that is about to be created (Figure 19). The existing title indicates that this is a double lane change (DLC) and that the speed is 120 km/h.Figure 19. Specify title for new dataset.Change the speed in the title from 120 to 150, and then click the OK button. The CarSimRun Control screen now shows the modified linkidentical copy of the original linked dataset. Your next step is to edit the dataset to change the test speed.23Figure 20. Run screen with link to newly created procedure dataset.4. Click the blue button(Figure 20) to view the newly created dataset (Figure 21).This screen specifies details about a vehicle test procedure. It has links to datasets for driver controls, additional data that include a 3D road, starting and stopping conditions, and definitions of plots of interest. Although it has a new title, the rest of the information on the screen is an exact copy of the dataset that was used for the baseline run.5. is 120. Change it to 150 km/h.6. The Notes23Figure 21. Procedure screen for newly created dataset.7.Right-click on the Backbutton to confirm what it does, then click it to return to the previous library screen — the CarSim Run Control screen (Figure 20). 8.Click the Run Math Model button. As before, a status bar shows the progress of the run. 9. When the run is complete, the Animate and Plot buttons become active. Click the Animatebutton to run the animator and view the new simulated behavior. After viewing the results,close the animator.10.Overlay animations andplots with other runs(Figure 22), and then check the box. When checked, results of multiple runs can be overlaid; several potential links appear for selecting runs to overlay. 23Figure 22. Overlay a new run with the baseline run.11.to select an existing run to overlay. Choose Baseline12.Next to the Animate button, right-click the checkbox Set run color(Figure 23). Thepop-up indicates the vehicle color can be set here; check the to reveal a colorFigure 23. Option to set a run color.13.Press the color selector button to display a color palette (Figure 24). For example,choose yellow (the baseline vehicle was blue).12Figure 24. Color selector palette.14.Click the Animate button to view both runs in the animator (Figure 25). After viewing theresults, close the animator.Review You have navigated through the CarSim database, created a newprocedure dataset named My New Test, and used it to make a new run.You documented your new dataset using the Notes field. You haveoverlaid results from multiple runs in the animator and set the colorfrom the main screen to more easily identify vehicles from the differentruns.In the next section, you will create some new datasets from scratch todefine a vehicle under a new loading condition, run the test, and seehow the behavior is affected.Figure 25. Animation with overlay of two runs.Run a Vehicle with a New Load ConditionContinue with the CarSim Run Control screen, with the dataset created in the previous section: { * * Quick Start Guide Example } My New Test.1. Click the New(Figure 26) to create another new dataset, and give it the name MyNew Load Condition. Once again, the screen shows your new title. As before, the Animate and Plot buttons are dimmed because the run hasn’t been made yet.1345Figure 26: Selecting a different library of vehicle datasets.e the drop-down control configuration: Ind_Ind and select thelibraryThis switches to a library of datasets that include loading conditions (passengers,payloads, etc.). The link button is now gray and labeled {No dataset selected}, to3. and choose the option Link to New Dataset.This action brings up a dialog box where you specify the of the newly27). Enter the title Roof-top load condition and click thebutton The CarSim Run Control screen now shows a link new dataset (Figure 28).12Figure 27. Specify title and category for newly created loaded vehicle dataset.3Figure 28. Run screen with link to newly created vehicle dataset.Although the vehicle dataset has been created and linked, it does not yet have any values.CarSim cannot determine the vehicle layout. (Does it have independent or solid axle suspensions? Does it have a trailer?) Without the layout information, the Run Math Model button is dimmed to indicate a run cannot yet be made.In the next few steps, you will provide the necessary information.4. Click the blue button1Figure 29. Newly created vehicle dataset.5. Use the drop-down vehicle library controlto select the vehicle assemblylibrary (Figure 30).1Figure 30. Select the Vehicle: Assembly library.(Figure 31). Use thedrop-down dataset controllibrary, andchoose CS Hatchbackscreen changes (Figure 32);the and the image is updated to show34Figure 31. Select the vehicle assembly dataset for the C-class hatchback.If the 3x1 image stylebox is checked, uncheck it to adjust the display of the vehicle image. 6.Use the drop-down library control to select the Payload: Box Shape library action should immediately controls underneath to access a (Figure 34 shows the appearance of the screen after step 7.)。
Chapter 11 Welfare
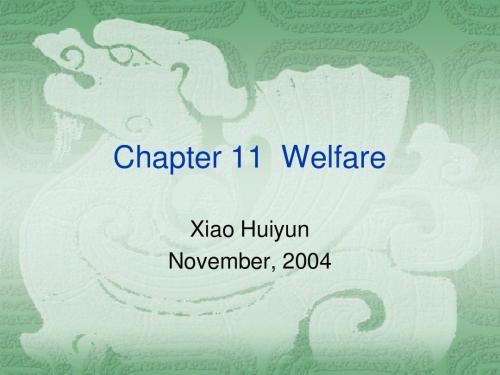
Workhouses
Workhouse, Winchester Workhouse, Andover
• Pictures of workhouse
The Poor Law in 1834
OLIVER TWIST
Charles Dickens
Dickens Centre, Rochester
Chapter 11 Welfare
Xiao Huilopment of “Welfare State”
1. Definition of Poverty 1.1 Absolute Poverty – families without minimum food, clothing and shelter needed for maintenance of merely physical health (concept at beginning of last century) 1.2 Relative Poverty – Despite adequate income for survival, people who do not have what is regarded as minimum necessary for decency and who cannot escape judgement that they are indecent can be labeled as poor.
The Reform by Thatcherism “I came to office with one deliberate intent: to change Britain from a dependent to a self-reliant society; from a give-it-tome to a do-it-yourself nation; a get-up-and-go instead of a sit-back-andwait Britain.” ( Margaret Thatcher, February1984)
会议室管理制度英文

6. Meeting Summarization
- At the end of the meeting, the chairperson or a designated note-taker shall summarize the key points, decisions, and action items.
3. Participation Principles
All participants shall adhere to the principles of punctuality, active engagement, respect, and constructive communication. Attendees are expected to prepare adequately for meetings and contribute positively to the discussion.
- Regular check-ins on time should be made to ensure the meeting stays on track and concludes within the allocated time frame.
5. Decision-Making
- Decisions should be made based on a thorough discussion of the topic, considering all viewpoints.
上级安排下级工作文件的模板

上级安排下级工作文件的模板英文回答:As a supervisor, it is important to provide clear instructions to subordinates on how to complete their work effectively. One way to do this is by using a work file template. This template should include all the necessary information and guidelines for the task at hand.For example, let's say I am assigning a project to my subordinate. In the work file template, I would include the project name, objectives, deadline, and any specific requirements or guidelines. This ensures that the subordinate has a clear understanding of what needs to be done.Furthermore, the template should also include a section for the subordinate to report their progress. This can bein the form of regular updates or checkpoints throughout the project. By including this in the template, I caneasily track the progress and address any issues or concerns that may arise.Additionally, it is important to provide any necessary resources or references in the work file template. This could include documents, links, or contacts that the subordinate may need to successfully complete the task. By providing these resources upfront, I am ensuring that the subordinate has all the necessary tools to complete the work effectively.In conclusion, using a work file template is an effective way to provide clear instructions and guidelines to subordinates. It helps ensure that everyone is on the same page and allows for easy tracking of progress. By including all the necessary information and resources, I am setting my subordinates up for success.中文回答:作为上级,向下属清晰地指示如何有效完成工作是很重要的。
Filename list.txt

CHAPTER
Create and edit file lists
Open a text editor such as Notepad or a more advanced editor like Visual Studio Code
Type or paste the names of the files you want to include in the list, each on a new line
Alternately, use software or scripting languages to automatically update the file list based on specific criteria or changes in the file system
03
CHAPTER
Audio files (. mp3,. wav)
These files contain sound recordings that can be played back using audio players They vary in quality and file size depending on the format
File management: In operating systems or file explorers, file lists are used to display the content of directors, allowing users to navigate and manage their files easilySoftware development: Programmers often use file lists to track and organize source code files, resource files, and other assets needed for software developmentData backup: File lists can be generated to record all files included in a backup, ensuring that all necessary data is accounted for and can be restored if neededNetwork file sharing: In network environments, file lists can be used to share specific groups of files with authorized users, facilitating collaboration and data exchange