Abstract Using Literate Programming to Teach
C#中abstract的用法详解

C#中abstract的⽤法详解abstract可以⽤来修饰类,⽅法,属性,索引器和时间,这⾥不包括字段. 使⽤abstrac修饰的类,该类只能作为其他类的基类,不能实例化,⽽且abstract修饰的成员在派⽣类中必须全部实现,不允许部分实现,否则编译异常. 如:using System;namespace ConsoleApplication8{class Program{static void Main(string[] args){BClass b = new BClass();b.m1();}}abstract class AClass{public abstract void m1();public abstract void m2();}class BClass : AClass{public override void m1(){throw new NotImplementedException();}//public override void m2()//{// throw new NotImplementedException();//}}}Abstract classes have the following features:抽象类拥有如下特征:1,抽象类不能被实例化, 但可以有实例构造函数, 类是否可以实例化取决于是否拥有实例化的权限 (对于抽象类的权限是abstract, 禁⽌实例化),即使不提供构造函数, 编译器也会提供默认构造函数;2,抽象类可以包含抽象⽅法和访问器;3,抽象类不能使⽤sealed修饰, sealed意为不能被继承;4,所有继承⾃抽象类的⾮抽象类必须实现所有的抽象成员,包括⽅法,属性,索引器,事件;abstract修饰的⽅法有如下特征:1,抽象⽅法即是虚拟⽅法(隐含);2,抽象⽅法只能在抽象类中声明;3,因为抽象⽅法只是声明, 不提供实现, 所以⽅法只以分号结束,没有⽅法体,即没有花括号部分;如public abstract void MyMethod();4,override修饰的覆盖⽅法提供实现,且只能作为⾮抽象类的成员;5,在抽象⽅法的声明上不能使⽤virtual或者是static修饰.即不能是静态的,⼜因为abstract已经是虚拟的,⽆需再⽤virtual强调.抽象属性尽管在⾏为上与抽象⽅法相似,但仍有有如下不同:1,不能在静态属性上应⽤abstract修饰符;2,抽象属性在⾮抽象的派⽣类中覆盖重写,使⽤override修饰符;抽象类与接⼝:1,抽象类必须提供所有接⼝成员的实现;2,继承接⼝的抽象类可以将接⼝的成员映射位抽象⽅法.如:interface I{void M();}abstract class C: I{public abstract void M();}抽象类实例:// abstract_keyword.cs// 抽象类using System;abstract class BaseClass // 抽象类{protected int _x = 100; //抽象类可以定义字段,但不可以是抽象字段,也没有这⼀说法.protected int _y = 150;public BaseClass(int i) //可以定义实例构造函数,仅供派⽣的⾮抽象类调⽤; 这⾥显式提供构造函数,编译器将不再提供默认构造函数. {fielda = i;}public BaseClass(){}private int fielda;public static int fieldsa = 0;public abstract void AbstractMethod(); // 抽象⽅法public abstract int X { get; } //抽象属性public abstract int Y { get; }public abstract string IdxString { get; set; } //抽象属性public abstract char this[int i] { get; } //抽象索引器}class DerivedClass : BaseClass{private string idxstring;private int fieldb;//如果基类中没有定义⽆参构造函数,但存在有参数的构造函数,//那么这⾥派⽣类得构造函数必须调⽤基类的有参数构造函数,否则编译出错public DerivedClass(int p): base(p) //这⾥的:base(p)可省略,因为基类定义了默认的⽆参构造函数{fieldb = p;}public override string IdxString //覆盖重新属性{get{return idxstring;}set{idxstring = value;}}public override char this[int i] //覆盖重写索引器{get { return IdxString[i]; }}public override void AbstractMethod(){_x++;_y++;}public override int X // 覆盖重写属性{get{return _x + 10;}}public override int Y // 覆盖重写属性{get{return _y + 10;}}static void Main(){DerivedClass o = new DerivedClass(1);o.AbstractMethod();Console.WriteLine("x = {0}, y = {1}", o.X, o.Y);}}以上所述是⼩编给⼤家介绍的C#中abstract的⽤法详解,希望对⼤家有所帮助,如果⼤家有任何疑问请给我留⾔,⼩编会及时回复⼤家的。
运用抽象写一段文字300字作文

运用抽象写一段文字300字作文英文回答:Abstract writing is a skill that allows us to express our thoughts and ideas in a concise and effective manner.It involves summarizing the main points of a text or atopic without going into too much detail. It requires us to focus on the key information and present it in a clear and organized way.One example of abstract writing is when we write a summary of a book or a movie. Instead of describing every single detail, we highlight the main plot, the key characters, and the main themes. This allows the reader to get a sense of what the book or movie is about without having to read or watch the entire thing.Another example of abstract writing is when we write a research paper. In the abstract section, we provide a brief overview of the study, including the research question, themethodology, and the main findings. This helps the readerto quickly understand the purpose and significance of the study without having to read the entire paper.Abstract writing is also useful in everyday life. For instance, when we give a presentation, we often start with an abstract or an introduction that gives a brief overviewof what we will be talking about. This helps the audienceto understand the main points and stay engaged throughout the presentation.中文回答:抽象写作是一种能让我们以简洁有效的方式表达思想和观点的技巧。
inherited abstract methods
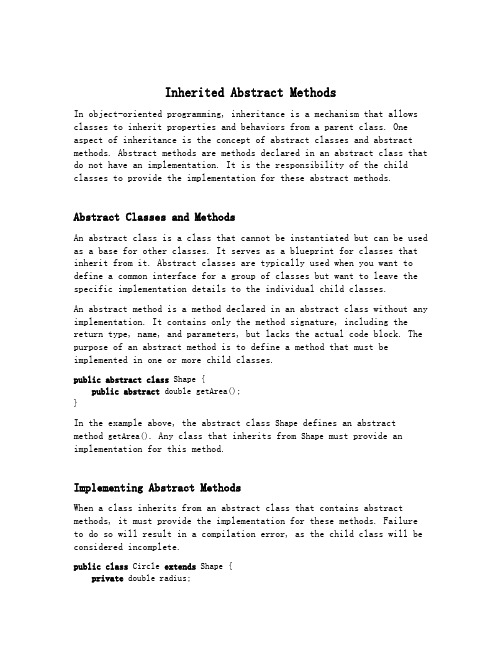
Inherited Abstract MethodsIn object-oriented programming, inheritance is a mechanism that allows classes to inherit properties and behaviors from a parent class. One aspect of inheritance is the concept of abstract classes and abstract methods. Abstract methods are methods declared in an abstract class that do not have an implementation. It is the responsibility of the child classes to provide the implementation for these abstract methods.Abstract Classes and MethodsAn abstract class is a class that cannot be instantiated but can be used as a base for other classes. It serves as a blueprint for classes that inherit from it. Abstract classes are typically used when you want to define a common interface for a group of classes but want to leave the specific implementation details to the individual child classes.An abstract method is a method declared in an abstract class without any implementation. It contains only the method signature, including the return type, name, and parameters, but lacks the actual code block. The purpose of an abstract method is to define a method that must be implemented in one or more child classes.public abstract class Shape {public abstract double getArea();}In the example above, the abstract class Shape defines an abstract method getArea(). Any class that inherits from Shape must provide an implementation for this method.Implementing Abstract MethodsWhen a class inherits from an abstract class that contains abstract methods, it must provide the implementation for these methods. Failure to do so will result in a compilation error, as the child class will be considered incomplete.public class Circle extends Shape {private double radius;public Circle(double radius) {this.radius = radius;}@Overridepublic double getArea() {return Math.PI * Math.pow(radius, 2);}}In the example above, the Circle class extends the abstract class Shape and provides an implementation of the getArea() method. The implementation calculates the area of a circle based on its radius.Multiple Levels of InheritanceAbstract classes and abstract methods can be inherited through multiple levels of inheritance. This means that a child class can inherit from an abstract class, which itself inherits from another abstract class.public abstract class Vehicle {public abstract void start();}public abstract class Car extends Vehicle {public abstract void accelerate();}public class ElectricCar extends Car {@Overridepublic void start() {// Start the electric car}@Overridepublic void accelerate() {// Accelerate the electric car}}In the example above, the class hierarchy represents a vehicle abstraction. The abstract class Vehicle declares the abstract methodstart(), which is inherited by the abstract class Car. The Car class, inturn, declares the abstract method accelerate(), which is finally implemented in the ElectricCar class.Benefits of Inherited Abstract MethodsInherited abstract methods provide several benefits in object-oriented programming:1.Code reusability: Abstract classes and abstract methods allowdevelopers to define common behavior and characteristics in a base class. Child classes can then inherit and extend this behaviorwithout duplicating code.2.Enforce method implementation: Abstract methods ensure that childclasses implement specific behavior. This enforces consistency and helps prevent incomplete implementations.3.Polymorphism: Abstract classes can be used as types to createcollections of objects that share a common interface. This allows for greater flexibility and reduces dependency on concreteimplementations.ConclusionInherited abstract methods play an essential role in object-oriented programming. They allow for code reusability, enforce method implementation, and enable polymorphism. Abstract classes and abstract methods provide a powerful mechanism to define common interfaces and leave the specifics to the inheriting classes. By understanding and utilizing inherited abstract methods effectively, developers can create more maintainable and extensible code.。
中考英语计算机编程单选题50题
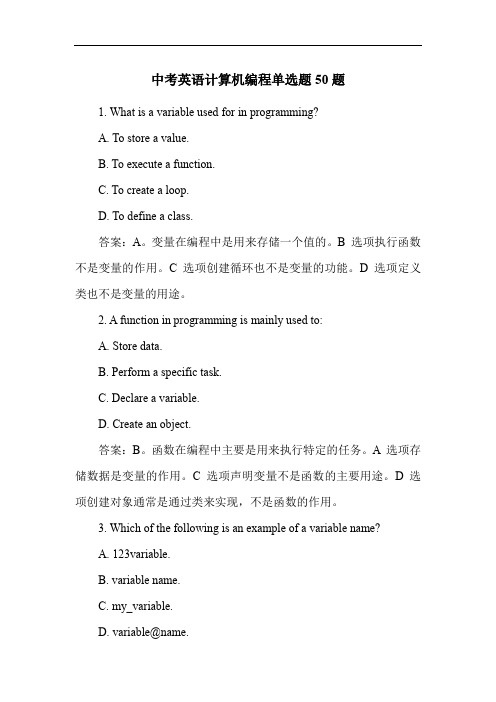
中考英语计算机编程单选题50题1. What is a variable used for in programming?A. To store a value.B. To execute a function.C. To create a loop.D. To define a class.答案:A。
变量在编程中是用来存储一个值的。
B 选项执行函数不是变量的作用。
C 选项创建循环也不是变量的功能。
D 选项定义类也不是变量的用途。
2. A function in programming is mainly used to:A. Store data.B. Perform a specific task.C. Declare a variable.D. Create an object.答案:B。
函数在编程中主要是用来执行特定的任务。
A 选项存储数据是变量的作用。
C 选项声明变量不是函数的主要用途。
D 选项创建对象通常是通过类来实现,不是函数的作用。
3. Which of the following is an example of a variable name?A. 123variable.B. variable name.C. my_variable.D. variable@name.答案:C。
变量名通常由字母、数字和下划线组成,且不能以数字开头,也不能包含特殊字符。
A 选项以数字开头不符合要求。
B 选项中有空格不符合规范。
D 选项中有特殊字符@不符合要求。
4. What does it mean when a function is called in programming?A. Running the code inside the function.B. Defining a new variable.C. Creating a loop.D. Declaring a class.答案:A。
Literate Programming

Literate ProgrammingDonald E.KnuthComputer Science Department,Stanford University,Stanford,CA94305,USAThe author and his associates have been experimenting for the past several years with a program-ming language and documentation system called WEB.This paper presents WEB by example,and discusses why the new system appears to be an improvement over previous ones.A.INTRODUCTIONThe past ten years have witnessed substantial improve-ments in programming methodology.This advance, carried out under the banner of“structured program-ming,”has led to programs that are more reliable and easier to comprehend;yet the results are not entirely satisfactory.My purpose in the present paper is to propose another motto that may be appropriate for the next decade,as we attempt to make further progress in the state of the art.I believe that the time is ripe for significantly better documentation of programs,and that we can best achieve this by considering programs to be works of literature.Hence,my title:“Literate Programming.”Let us change our traditional attitude to the con-struction of programs:Instead of imagining that our main task is to instruct a computer what to do,let us concentrate rather on explaining to human beings what we want a computer to do.The practitioner of literate programming can be re-garded as an essayist,whose main concern is with ex-position and excellence of style.Such an author,with thesaurus in hand,chooses the names of variables care-fully and explains what each variable means.He or she strives for a program that is comprehensible because its concepts have been introduced in an order that is best for human understanding,using a mixture of formal and informal methods that re¨ınforce each other.I dare to suggest that such advances in documenta-tion are possible because of the experiences I’ve had during the past several years while working intensively on software development.By making use of several ideas that have existed for a long time,and by applying them systematically in a slightly new way,I’ve stumbled across a method of composing programs that excites me very much.In fact,my enthusiasm is so great that I must warn the reader to discount much of what I shall say as the ravings of a fanatic who thinks he has just seen a great light.Programming is a very personal activity,so I can’t be certain that what has worked for me will work for everybody.Yet the impact of this new approach on my own style has been profound,and my excitement has continued unabated for more than two years.I enjoy the new methodology so much that it is hard for me to refrain from going back to every program that I’ve ever written and recasting it in“literate”form.Ifind myself unable to resist working on programming tasks that I would ordinarily have assigned to student research assistants;and why?Because it seems to me that at last I’m able to write programs as they should be written. My programs are not only explained better than ever before;they also are better programs,because the new methodology encourages me to do a better job.For these reasons I am compelled to write this paper,in hopes that my experiences will prove to be relevant to others.I must confess that there may also be a bit of mal-ice in my choice of a title.During the1970s I was coerced like everybody else into adopting the ideas of structured programming,because I couldn’t bear to be found guilty of writing unstructured programs.Now I have a chance to get even.By coining the phrase“liter-ate programming,”I am imposing a moral commitment on everyone who hears the term;surely nobody wants to admit writing an illiterate program.B.THE WEB SYSTEMI hope,however,to demonstrate in this paper that the title is not merely wordplay.The ideas of literate pro-gramming have been embodied in a language and a suite of computer programs that have been developed at Stanford University during the past few years as part of my research on algorithms and on digital typography. This language and its associated programs have come to be known as the WEB system.My goal in what fol-lows is to describe the philosophy that underlies WEB, to present examples of programs in the WEB language, and to discuss what may be the future implications of this work.I chose the name WEB partly because it was one of the few three-letter words of English that hadn’t al-ready been applied to computers.But as time went on, I’ve become extremely pleased with the name,because I think that a complex piece of software is,indeed,best regarded as a web that has been delicately pieced to-gether from simple materials.We understand a compli-cated system by understanding its simple parts,and by understanding the simple relations between those parts and their immediate neighbors.If we express a pro-gram as a web of ideas,we can emphasize its structural properties in a natural and satisfying way.WEB itself is chiefly a combination of two other lan-guages:(1)a document formatting language and(2)a programming language.My prototype WEB system uses submitted to THE COMPUTER JOURNAL1D.E.KNUTHT E X as the document formatting language and PAS-CAL as the programming language,but the same prin-ciples would apply equally well if other languages were substituted.Instead of T E X,one could use a language like Scribe or Troff;instead of PASCAL ,one could use ADA ,ALGOL ,LISP ,COBOL ,FORTRAN ,APL ,C ,etc.,or even assembly language.The main point is that WEB is inherently bilingual,and that such a combination of languages proves to be much more powerful than either single language by itself.WEB does not make the other languages obsolete;on the contrary,it enhances them.I naturally chose T E X to be the document formatting language,in the first WEB system,because T E X is my own creation;1I wanted to acquire a lot of experience in harnessing T E X to a variety of different tasks.I chose PASCAL as the programming language because it has received such widespread support from educational in-stitutions all over the world;it is not my favorite lan-guage for system programming,but it has become a “second language”for so many programmers that it provides an exceptionally effective medium of commu-nication.Furthermore WEB itself has a macro-processing ability that makes PASCAL ’s limitations largely irrele-vant.Document formatting languages are newcomers to the computing scene,but their use is spreading rapidly.Therefore I’m confident that we will be able to expect each member of the next generation of programmers to be familiar with a document language as well as a pro-gramming language,as part of their basic education.Once a person knows both of the underlying languages,there’s no trick at all to learning WEB ,because the WEB user’s manual is fewer than ten pages long.A WEB user writes a program that serves as the source language for two different system routines.(See Fig-ure 1.)One line of processing is called weaving the web;it produces a document that describes the pro-gram clearly and that facilitates program maintenance.The other line of processing is called tangling the web;it produces a machine-executable program.The pro-gram and its documentation are both generated from the same source,so they are consistent with each other.T E X TEX−−−−→DVIWEAVEWEBTANGLEPAS−−−−→RELPASCALFigure 1.Dual usage of a WEB file.Let’s look at this process in slightly more detail.Sup-pose you have written a WEB program and put it intoa computer text file called COB.WEB (say).To gener-ate hardcopy documentation for your program,you can run the WEAVE processor;this is a system program that takes the file COB.WEB as input and produces another file COB.TEX as output.Then you run the T E X processor,which takes COB.TEX as input and produces COB.DVI as output.The latter file,COB.DVI ,is a “device-independent”binary description of how to typeset the documenta-tion,so you can get printed output by applying one more system routine to this file.You can also follow the other branch of Figure 1,by running the TANGLE processor;this is a system program that takes the file COB.WEB as input and produces a new file COB.PAS as output.Then you run the PASCAL com-piler,which converts COB.PAS to a binary file COB.REL (say).Finally,you can run your program by loading and executing COB.REL .The process of “compile,load,and go”has been slightly lengthened to “tangle,com-pile,load,and go.”C.A COMPLETE EXAMPLENow it’s time for me to stop presenting general plat-itudes and to move on to something tangible.Let us look at a real program that has been written in WEB .The numbered paragraphs that follow are the actual output of a WEB file that has been “woven”into a doc-ument;a computer has also generated the indexes that appear at the program’s end.If my claims for the ad-vantages of literate programming have any merit,you should be able to understand the following description more easily than you could have understood the same program when presented in a more conventional way.However,I am trying here to explain the format of WEB documentation at the same time as I am discussing the details of a nontrivial algorithm,so the description be-low is slightly longer than it would be if it were written for people who already have been introduced to WEB .Here,then,is the computer-generated output:Printing primes:An example of WEB ..............§1Plan of the program ..............................§3The output phase ................................§5Generating the primes ..........................§11The inner loop ..................................§22Index ...........................................§271.Printing primes:An example of WEB .The following program is essentially the same as Edsger Dijkstra’s “first example of step-wise program composi-tion,”found on pages 26–39of his Notes on Structured Programming ,2but it has been translated into the WEB language.[[Double brackets will be used in what follows to en-close comments relating to WEB itself,because the chief purpose of this program is to introduce the reader to the WEB style of documentation.WEB programs are al-ways broken into small sections,each of which has a serial number;the present section is number 1.]]Dijkstra’s program prints a table of the first thou-sand prime numbers.We shall begin as he did,by re-ducing the entire program to its top-level description.[[Every section in a WEB program begins with optional commentary about that section,and ends with optional program text for the section.For example,you are now reading part of the commentary in §1,and the program text for §1immediately follows the present paragraph.Program texts are specifications of PASCAL programs;they either use PASCAL language directly,or they use angle brackets to represent PASCAL code that appears2submitted to THE COMPUTER JOURNALLITERATE PROGRAMMINGin other sections.For example,the angle-bracket nota-tion‘ Program to print...numbers2 ’is WEB’s way of saying the following:“The PASCAL text to be inserted here is called‘Program to print...numbers’,and you canfind out all about it by looking at section2.”One of the main characteristics of WEB is that different parts of the program are usually abbreviated,by giving them such an informal top-level description.]]Program to print thefirst thousand primenumbers22.This program has no input,because we want to keep it rather simple.The result of the program will be to produce a list of thefirst thousand prime numbers, and this list will appear on the outputfile.Since there is no input,we declare the value m= 1000as a compile-time constant.The program itself is capable of generating thefirst m prime numbers for any positive m,as long as the computer’sfinite limitations are not exceeded.[[The program text below specifies the“expanded mean-ing”of‘ Program to print...numbers2 ’;notice that it involves the top-level descriptions of three other sec-tions.When those top-level descriptions are replaced by their expanded meanings,a syntactically correct PAS-CAL program will be obtained.]]Program to print thefirst thousand primenumbers2 ≡program print primes(output);const m=1000;Other constants of the program5var Variables of the program4begin Print thefirst m prime numbers3 ;end.This code is used in section1.3.Plan of the program.We shall proceed tofill out the rest of the program by making whatever deci-sions seem easiest at each step;the idea will be to strive for simplicityfirst and efficiency later,in order to see where this leads us.Thefinal program may not be op-timum,but we want it to be reliable,well motivated, and reasonably fast.Let us decide at this point to maintain a table that includes all of the prime numbers that will be gener-ated,and to separate the generation problem from the printing problem.[[The WEB description you are reading once again fol-lows a pattern that will soon be familiar:A typical section begins with comments and ends with program text.The comments motivate and explain noteworthy features of the program text.]]Print thefirst m prime numbers3 ≡Fill table p with thefirst m prime numbers11 ;Print table p8This code is used in section2.4.How should table p be represented?Two possi-bilities suggest themselves:We could construct a suffi-ciently large array of boolean values in which the k th entry is true if and only if the number k is prime;or we could build an array of integers in which the k th entry is the k th prime number.Let us choose the lat-ter alternative,by introducing an integer array called p[1..m].In the documentation below,the notation‘p[k]’will refer to the k th element of array p,while‘p k’will refer to the k th prime number.If the program is correct,p[k] will either be equal to p k or it will not yet have been assigned any value.[[Incidentally,our program will eventually make use of several more variables as we refine the data structures. All of the sections where variables are declared will be called‘ Variables of the program4 ’;the number ‘4’in this name refers to the present section,which is thefirst section to specify the expanded meaning of ‘ Variables of the program ’.The note‘See also...’refers to all of the other sections that have the same top-level description.The expanded meaning of‘ Variables of the program4 ’consists of all the program texts for this name,not just the text found in§4.]]Variables of the program4 ≡p:array[1..m]of integer;{thefirst m prime numbers,in increasing order}See also sections7,12,15,17,23,and24.This code is used in section2.5.The output phase.Let’s work on the second part of the programfirst.It’s not as interesting as the problem of computing prime numbers;but the job of printing must be done sooner or later,and we might as well do it sooner,since it will be good to have it done. [[And it is easier to learn WEB when reading a program that has comparatively few distracting complications.]] Since p is simply an array of integers,there is little difficulty in printing the output,except that we need to decide upon a suitable output format.Let us print the table on separate pages,with rr rows and cc columns per page,where every column is ww character positions wide.In this case we shall choose rr=50,cc=4,and ww=10,so that thefirst1000primes will appear on five pages.The program will not assume that m is an exact multiple of rr·cc.Other constants of the program5 ≡rr=50;{this many rows will be on each page in the output}cc=4;{this many columns will be on each page in the output}ww=10;{this many character positions will be used in each column}See also section19.This code is used in section2.6.In order to keep this program reasonably free of no-tations that are uniquely PASCAL esque,[[and in order to illustrate more of the facilities of WEB,]]a few macro definitions for low-level output instructions are intro-duced here.All of the output-oriented commands in the remainder of the program will be stated in terms of five simple primitives called print string,print integer, print entry,new line,and new page.[[Sections of a WEB program are allowed to contain macro definitions between the opening comments and the closing program text.The general format for each section is actually tripartite:commentary,then defini-tions,then program.Any of the three parts may be absent;for example,the present section contains no program text.]]submitted to THE COMPUTER JOURNAL3D.E.KNUTH[[Simple macros simply substitute a bit of PASCAL code for an identifier.Parametric macros are similar, but they also substitute an argument wherever‘#’oc-curs in the macro definition.Thefirst three macro def-initions here are parametric;the other two are simple.]] define print string(#)≡write(#){put a given string into the outputfile} define print integer(#)≡write(#:1){put a given integer into the outputfile,in decimal notation,using only as manydigit positions as necessary}define print entry(#)≡write(#:ww){likeprint integer,but ww character positionsarefilled,inserting blanks at the left} define new line≡write ln{advance to a new linein the outputfile}define new page≡page{advance to a new pagein the outputfile}7.Several variables are needed to govern the output process.When we begin to print a new page,the variable page number will be the ordinal number of that page,and page offset will be such that p[page offset]is thefirst prime to be printed.Similarly,p[row offset] will be thefirst prime in a given row.[[Notice the notation‘+≡’below;this indicates that the present section has the same name as a previous section,so the program text will be appended to some text that was previously specified.]]Variables of the program4 +≡page number:integer;{one more than the number of pages printed so far}page offset:integer;{index into p for thefirst entry on the current page}row offset:integer;{index into p for thefirst entry in the current row}c:;{runs through the columns in a row}8.Now that appropriate auxiliary variables have been introduced,the process of outputting table p almost writes itself.Print table p8 ≡begin page number←1;page offset←1;while page offset≤m dobegin Output a page of answers9 ;page number←page number+1;page offset←page offset+rr∗cc;end;endThis code is used in section3.9.A simple heading is printed at the top of each page. Output a page of answers9 ≡begin print string(´The First ´);print integer(m);print string(´ Prime Numbers −−− Page ´);print integer(page number);new line;new line;{there’s a blank line after the heading}for row offset←page offset to page offset+rr−1 do Output a line of answers10 ;new page;endThis code is used in section8.10.Thefirst row will containp[1],p[1+rr],p[1+2∗rr],...;a similar pattern holds for each value of the row offset. Output a line of answers10 ≡begin for c←0to cc−1doif row offset+c∗rr≤m thenprint entry(p[row offset+c∗rr]);new line;endThis code is used in section9.11.Generating the primes.The remaining task is tofill table p with the correct numbers.Let us do this by generating its entries one at a time:Assuming that we have computed all primes that are j or less,we will advance j to the next suitable value,and continue doing this until the table is completely full.The program includes a provision to initialize the variables in certain data structures that will be intro-duced later.Fill table p with thefirst m prime numbers11 ≡Initialize the data structures16 ;while k<m dobegin Increase j until it is the next primenumber14 ;k←k+1;p[k]←j;endThis code is used in section3.12.We need to declare the two variables j and k that were just introduced.Variables of the program4 +≡j:integer;{all primes≤j are in table p}k:0..m;{this many primes are in table p}13.So far we haven’t needed to confront the issue of what a prime number is.But everything else has been taken care of,so we must delve into a bit of number theory now.By definition,a number is called prime if it is an integer greater than1that is not evenly divisible by any smaller prime number.Stating this another way, the integer j>1is not prime if and only if there exists a prime number p n<j such that j is a multiple of p n. Therefore the section of the program that is called ‘ Increase j until it is the next prime number ’could be coded very simply:‘repeat j←j+1; Give to j prime the meaning:j is a prime number ;until j prime’. And to compute the boolean value j prime,the follow-ing would suffice:‘j prime←true;for n←1to k do If p[n]divides j,set j prime←false ’.14.However,it is possible to obtain a much more ef-ficient algorithm by using more facts of number theory. In thefirst place,we can speed things up a bit by rec-ognizing that p1=2and that all subsequent primes are odd;therefore we can let j run through odd values only.Our program now takes the following form:Increase j until it is the next prime number14 ≡repeat j←j+2;Update variables that depend on j20 ;Give to j prime the meaning:j is a primenumber22 ;until j primeThis code is used in section11.4submitted to THE COMPUTER JOURNALLITERATE PROGRAMMING15.The repeat loop in the previous section intro-duces a boolean variable j prime ,so that it will not be necessary to resort to a goto statement.(We are following Dijkstra,2not Knuth.3) Variables of the program 4 +≡j prime :boolean ;{is j a prime number?}16.In order to make the odd-even trick work,we must of course initialize the variables j ,k ,and p [1]as follows.Initialize the data structures 16 ≡j ←1;k ←1;p [1]←2;See also section 18.This code is used in section 11.17.Now we can apply more number theory in order to obtain further economies.If j is not prime,its smallest prime factor p n will be √j or less.Thus if we know a number ord such thatp [ord ]2>j,and if j is odd,we need only test for divisors in the set {p [2],...,p [ord −1]}.This is much faster than testing divisibility by {p [2],...,p [k ]},since ord tends to be much smaller than k .(Indeed,when k is large,the celebrated “prime number theorem”implies that the value of ord will be approximately 2 k/ln k .)Let us therefore introduce ord into the data struc-ture.A moment’s thought makes it clear that ord changes in a simple way when j increases,and that an-other variable square facilitates the updating process. Variables of the program 4 +≡ord :2..ord max ;{the smallest index ≥2such that p 2ord >j }square :integer ;{square =p 2ord }18. Initialize the data structures 16 +≡ord ←2;square ←9;19.The value of ord will never get larger than a cer-tain value ord max ,which must be chosen sufficiently large.It turns out that ord never exceeds 30when m =1000.Other constants of the program 5 +≡ord max =30;{p 2ord max must exceed p m }20.When j has been increased by 2,we must increase ord by unity when j =p 2ord ,i.e.,when j =square . Update variables that depend on j 20 ≡if j =square thenbegin ord ←ord +1;Update variables that depend on ord 21 ;endThis code is used in section 14.21.At this point in the program,ord has just been increased by unity,and we want to set square :=p 2ord .A surprisingly subtle point arises here:How do we know that p ord has already been computed,i.e.,that ord ≤k ?If there were a gap in the sequence of prime numbers,such that p k +1>p 2k for some k ,then this part of the program would refer to the yet-uncomputed value p [k +1]unless some special test were made.Fortunately,there are no such gaps.But no sim-ple proof of this fact is known.For example,Euclid’sfamous demonstration that there are infinitely many prime numbers is strong enough to prove only that p k +1<=p 1...p k +1.Advanced books on number theory come to our rescue by showing that much more is true;for example,“Bertrand’s postulate”states that p k +1<2p k for all k .Update variables that depend on ord 21 ≡square ←p [ord ]∗p [ord ];{at this point ord ≤k }See also section 25.This code is used in section 20.22.The inner loop.Our remaining task is to de-termine whether or not a given integer j is prime.The general outline of this part of the program is quite sim-ple,using the value of ord as described above. Give to j prime the meaning:j is a primenumber 22 ≡n ←2;j prime ←true ;while (n <ord )∧j prime dobegin If p [n ]is a factor of j ,setj prime ←false 26 ;n ←n +1;endThis code is used in section 14.23. Variables of the program 4 +≡n :2..ord max ;{runs from 2to ord when testing divisibility }24.Let’s suppose that division is very slow or nonex-istent on our machine.We want to detect nonprime odd numbers,which are odd multiples of the set of primes {p 2,...,p ord }.Since ord max is small,it is reasonable to maintain an auxiliary table of the smallest odd multiples that haven’t already been used to show that some j is non-prime.In other words,our goal is to “knock out”all of the odd multiples of each p n in the set {p 2,...,p ord },and one way to do this is to introduce an auxiliary table that serves as a control structure for a set of knock-out procedures that are being simulated in parallel.(The so-called “sieve of Eratosthenes”generates primes by a similar method,but it knocks out the multiples of each prime serially.)The auxiliary table suggested by these considerations is a mult array that satisfies the following invariant condition:For 2≤n <ord ,mult [n ]is an odd multiple of p n such that mult [n ]<j +2p n . Variables of the program 4 +≡mult :array [2..ord max ]of integer ;{runs through multiples of primes }25.When ord has been increased,we need to ini-tialize a new element of the mult array.At this point j =p [ord −1]2,so there is no need for an elaborate computation.Update variables that depend on ord 21 +≡mult [ord −1]←j ;26.The remaining task is straightforward,given the data structures already prepared.Let us recapitulate the current situation:The goal is to test whether or not j is divisible by p n ,without actually performing a division.We know that j is odd,and that mult [n ]submitted to THE COMPUTER JOURNAL 5D.E.KNUTHis an odd multiple of p n such that mult[n]<j+2p n. If mult[n]<j,we can increase mult[n]by2p n and the same conditions will hold.On the other hand if mult[n]≥j,the conditions imply that j is divisible by p n if and only if j=mult[n].If p[n]is a factor of j,set j prime←false26 ≡while mult[n]<j domult[n]←mult[n]+p[n]+p[n];if mult[n]=j then j prime←falseThis code is used in section22.27.Index.Every identifier used in this program is shown here together with a list of the section numbers where that identifier appears.The section number is underlined if the identifier was defined in that section. However,one-letter identifiers are indexed only at their point of definition,since such identifiers tend to appear almost everywhere.[[An index like this is prepared au-tomatically by the WEB software,and it is appended to thefinal section of the program.However,underlining of section numbers is not automatic;the user is sup-posed to mark identifiers at their point of definition in the WEB sourcefile.]]This index also refers to some of the places where key elements of the program are treated.For example,the entries for‘Output format’and‘Page headings’indi-cate where details of the output format are discussed. Several other topics that appear in the documentation (e.g.,‘Bertrand’s postulate’)have also been indexed. [[Special instructions within a WEB sourcefile can be used to insert essentially anything into the index.]] Bertrand,Joseph,postulate:21.boolean:15.c:7.cc:5,7,8,10.Dijkstra,Edsger:1,15.Eratosthenes,sieve of:24.false:13,26.integer:4,7,12,17,24.j:12.j prime:13,14,15,22,26.k:12.Knuth,Donald E.:15.m: 2.mult:24,25,26.n:23.new line:6,9,10.new page:6,9.ord:17,18,19,20,21,22,23,24,25.ord max:17,19,23,24.output:2,6.output format:5,9.p: 4.page: 6.page headings:9.page number:7,8,9.page offset:7,8,9.prime number,definition of:13.print entry:6,10.print integer:6,9.print primes: 2.print string:6,9.row offset:7,9,10.rr:5,8,9,10.square:17,18,20,21.true:4,13,22.WEB: 1.write: 6.write ln: 6.ww:5,6.Fill table p with thefirst m prime numbers11 Used in3.Give to j prime the meaning:j is a prime number22 Used in14.If p[n]is a factor of j,set j prime←false26 Used in22.Increase j until it is the next prime number14 Used in11.Initialize the data structures16,18 Used in11.Other constants of the program5,19 Used in2.Output a line of answers10 Used in9.Output a page of answers9 Used in8.Print table p8 Used in3.Print thefirst m prime numbers3 Used in2.Program to print thefirst thousand prime numbers2 Used in1.Update variables that depend on j20 Used in14. Update variables that depend on ord21,25 Used in20.Variables of the program4,7,12,15,17,23,24 Used in2.D.HOW THE EXAMPLE W AS SPECIFIED Everything reproduced above,from the table of con-tents preceding the program to the indexes of identifiers and section names at the end,was generated by ap-plying the program WEAVE to a sourcefile PRIMES.WEB written in the WEB language.Let us now look at that file PRIMES.WEB,in order to get an idea of what a WEB user actually types.There’s no need to show very much of PRIMES.WEB, however,because thatfile is reflected quite faithfully by the formatted output.Figure2contains enough of the WEB source to indicate the generalflavor;a reader who is familiar with the rudiments of T E X will be able to reconstruct all of PRIMES.WEB by looking only at the formatted output and Figure2.Figure2a starts with T E X commands(not shown in full)that make it convenient to typeset double brack-ets[[...]]and to give special typographic treatment to names like‘WEB’and‘PASCAL’.A WEB user generally begins by declaring such special aspects of the docu-ment format;for example,if nonstandard fonts of type are needed,they are usually statedfirst.It may also be necessary to specify the correct hyphenation of non-English words that appear in the document.Then comes‘@*’,which starts the program proper. WEB uses the symbol‘@’as an escape character for spe-cial instructions to the WEAVE and TANGLE processors. Everything between such special commands is either expressed in T E X language or in PASCAL language,de-pending on the context.6submitted to THE COMPUTER JOURNAL。
英语抽象类作文模板范文

英语抽象类作文模板范文Abstract Class Essay Template。
An abstract class is a concept in object-oriented programming that serves as a blueprint for other classes. It cannot be instantiated on its own and is meant to be extended by other classes. In this essay, we will discuss the characteristics of an abstract class, its purpose, and its role in the development of software applications.An abstract class is a class that cannot be instantiated, meaning you cannot create an object of the abstract class itself. Instead, it serves as a base for other classes to inherit from. It can contain both abstract and non-abstract methods. An abstract method is a method that is declared but not implemented in the abstract class. It is meant to be implemented by the subclasses that inherit from the abstract class. On the other hand, a non-abstract method is a method that has an implementation in the abstract class itself.The purpose of an abstract class is to provide a common interface for its subclasses. It allows you to define a set of methods that must be implemented by all the subclasses, ensuring that they have a consistent behavior. This is useful when you have a group of classes that share some common characteristics but also have their own unique behavior. By using an abstract class, you can define the common methods in the abstract class and leave the specific implementation to the subclasses.Another important role of an abstract class is to provide a level of abstraction in the design of a software application. Abstraction is a fundamental concept in object-oriented programming that allows you to focus on the essential features of an object while hiding the unnecessary details. By using an abstract class, you can define the essential methods and properties that are common to a group of related classes, while hiding the implementation details from the outside world. This makes the design of the software application more modular and easier to maintain.In addition, an abstract class can also be used to enforce a specific contract on its subclasses. By defining abstract methods in the abstract class, you can require that all thesubclasses provide an implementation for those methods. This ensures that the subclasses adhere to a certain set of rules and guarantees a consistent behavior across the different subclasses.To create an abstract class in a programming language such as Java or C#, you use the "abstract" keyword in the class definition. For example, in Java, you would declare an abstract class like this:```java。
介绍一技之长的英文作文

Essay 1: Mastering the Art of CookingIn the bustling world we live in, having a skill that can bring people together and create moments of joy is invaluable. Cooking is such a skill. From the humble beginnings of learning to chop vegetables to mastering complex recipes, the journey of becoming a proficient cook is both fulfilling and delicious. Not only does it allow one to nourish their body with wholesome meals, but it also provides an opportunity to express creativity and share love through food. Cooking is not just about sustenance; it's an art form that combines science and passion, making every dish a masterpiece.Essay 2: The Power of Public SpeakingPublic speaking is a skill that can open doors to opportunities and influence. It is the ability to communicate ideas effectively to a large audience, whether at a conference, a school presentation, or a community gathering. A good speaker can captivate, inspire, and persuade. This skill requires practice, understanding of rhetoric, and the ability to connect with listeners on an emotional level. Mastering public speaking can boost confidence, improve leadership qualities, and make one a more persuasive communicator in all aspects of life.Essay 3: The Craft of WritingWriting is a timeless skill that tra nscends time and place. Whether it’s crafting a novel, composing a poem, or penning an article, writing allows individuals to express themselves and connect with others. It demands discipline, imagination, and a deep understanding of language. Good writers can evoke emotions, challenge perspectives, and entertain. Moreover, writing can be a therapeutic process, helping authors explore their own thoughts and feelings while sharing them with the world.Essay 4: Proficiency in a Foreign LanguageBeing fluent in another language is like gaining access to a different universe. It broadens horizons, enhances cultural understanding, and opens up new career paths. Learning a foreign language involves not only memorizing vocabulary and grammar rules but also immersing oneself in the culture and history of the language. It can enrich personal relationships and provide a deeper appreciation for diversity.Essay 5: The Gift of MusicMusic has the power to touch souls and bridge gaps between people. Playing a musical instrument or singing can be a deeply rewarding skill. It requires dedication, patience, and a keen ear. Musicians learn to read music, understand rhythm and melody, and often develop a strong sense of discipline and teamwork when performing in groups. Music is a universal language that can heal, uplift, and unite.Essay 6: The Skill of CodingIn today’s digital age, knowing how to code is akin to being literate in the language of technology. Coders create applications, websites, and software that shape our daily lives. Learning programming languages such as Python, Java, or JavaScript can lead to innovative solutions and career opportunities. Coding teaches problem-solving, logical thinking, and attention to detail—skills that are valuable in any field.Each of these skills represents a unique way to enrich one’s life and contribute to society. They require commitment and effort, but the rewards are immeasurable.。
体育专业课程思政建设应解决的问题及实施路径
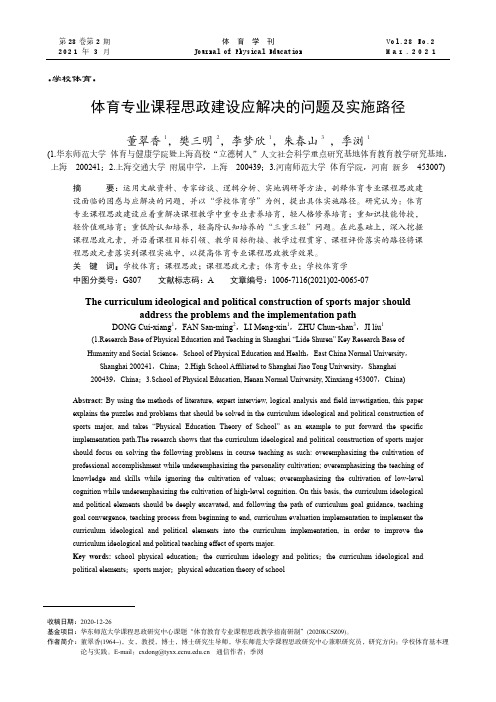
第28卷第2期 2021年3月体 育 学 刊Journal of Physical EducationVol.28 No.2M a r.2021·学校体育·体育专业课程思政建设应解决的问题及实施路径董翠香1,樊三明2,李梦欣1,朱春山3,季浏1(1.华东师范大学体育与健康学院暨上海高校“立德树人”人文社会科学重点研究基地体育教育教学研究基地,上海200241;2.上海交通大学附属中学,上海200439;3.河南师范大学体育学院,河南新乡453007)摘要:运用文献资料、专家访谈、逻辑分析、实地调研等方法,剖释体育专业课程思政建设面临的困惑与应解决的问题,并以“学校体育学”为例,提出具体实施路径。
研究认为:体育专业课程思政建设应着重解决课程教学中重专业素养培育,轻人格修养培育;重知识技能传授,轻价值观培育;重低阶认知培养,轻高阶认知培养的“三重三轻”问题。
在此基础上,深入挖掘课程思政元素,并沿着课程目标引领、教学目标衔接、教学过程贯穿、课程评价落实的路径将课程思政元素落实到课程实施中,以提高体育专业课程思政教学效果。
关键词:学校体育;课程思政;课程思政元素;体育专业;学校体育学中图分类号:G807 文献标志码:A 文章编号:1006-7116(2021)02-0065-07The curriculum ideological and political construction of sports major shouldaddress the problems and the implementation pathDONG Cui-xiang1,FAN San-ming2,LI Meng-xin1,ZHU Chun-shan3,JI liu1(1.Research Base of Physical Education and Teaching in Shanghai “Lide Shuren” Key Research Base ofHumanity and Social Science,School of Physical Education and Health,East China Normal University,Shanghai 200241,China;2.High School Affiliated to Shanghai Jiao Tong University,Shanghai 200439,China;3.School of Physical Education, Henan Normal University, Xinxiang 453007,China)Abstract: By using the methods of literature, expert interview, logical analysis and field investigation, this paperexplains the puzzles and problems that should be solved in the curriculum ideological and political construction ofsports major, and takes “Physical Education Theory of School” as an example to put forward the specificimplementation path.The research shows that the curriculum ideological and political construction of sports majorshould focus on solving the following problems in course teaching as such: overemphasizing the cultivation ofprofessional accomplishment while underemphasizing the personality cultivation; overemphasizing the teaching ofknowledge and skills while ignoring the cultivation of values; overemphasizing the cultivation of low-levelcognition while underemphasizing the cultivation of high-level cognition. On this basis, the curriculum ideologicaland political elements should be deeply excavated, and following the path of curriculum goal guidance, teachinggoal convergence, teaching process from beginning to end, curriculum evaluation implementation to implement thecurriculum ideological and political elements into the curriculum implementation, in order to improve thecurriculum ideological and political teaching effect of sports major.Key words:school physical education;the curriculum ideology and politics;the curriculum ideological andpolitical elements;sports major;physical education theory of school收稿日期:2020-12-26基金项目:华东师范大学课程思政研究中心课题“体育教育专业课程思政教学指南研制”(2020KCSZ09)。
中考英语计算机编程单选题50题

中考英语计算机编程单选题50题1.There are many programming languages. Java is a kind of _____.A.programming languageputer gameC.math subjectD.history book答案:A。
本题考查对编程相关术语的理解。
选项A“programming language”意为“编程语言”,Java 确实是一种编程语言;选项B“computer game”是“电脑游戏”;选项C“math subject”是“数学科目”;选项D“history book”是“历史书”。
2.In programming, a bug means _____.A.an insectB.a mistakeC.a flowerD.a book答案:B。
“bug”在编程中是“错误”的意思。
选项A“an insect”是“一只昆虫”;选项C“a flower”是“一朵花”;选项D“a book”是“一本书”。
3.A variable in programming can hold _____.A.numbers onlyB.letters onlyC.numbers and lettersD.just symbols答案:C。
变量在编程中可以存储数字和字母。
选项A 只说数字;选项B 只说字母;选项D 说只存储符号不准确。
4.When we write code, we use a(n) _____ to save and run it.A.textbookB.editorC.pencilD.eraser答案:B。
编写代码时,我们使用编辑器来保存和运行代码。
选项A“textbook”是“教科书”;选项C“pencil”是“铅笔”;选项D“eraser”是“橡皮”。
5.In programming, an algorithm is a set of _____.A.stepsB.picturesC.soundsD.colors答案:A。
高级语言编程一般流程

高级语言编程一般流程英文回答:High-level programming languages provide a more advanced level of abstraction compared to low-level languages. This abstraction allows programmers to writecode that is more concise, readable, and portable across different platforms. The general workflow for high-level language programming typically involves the following steps:1. Problem Definition and Analysis: The first step isto clearly define the problem that needs to be solved. This involves gathering requirements, understanding the problem domain, and analyzing the data that will be used.2. Algorithm Design: Once the problem is defined, an algorithm is designed to outline the steps that will be taken to solve the problem. The algorithm should be efficient, scalable, and robust.3. Code Implementation: The next step is to translate the algorithm into a high-level programming language. This involves writing the code, organizing it into modules and functions, and ensuring that it is readable and maintainable.4. Compilation or Interpretation: Depending on the language used, the code may need to be compiled or interpreted. Compilation converts the high-level code into machine code that can be directly executed by the computer. Interpretation, on the other hand, executes the code line by line without creating an intermediate machine code representation.5. Testing and Debugging: Once the code is compiled or interpreted, it is important to test it thoroughly to ensure that it is working as expected. This may involve writing unit tests, integration tests, and system tests to verify the functionality and reliability of the code.6. Deployment and Maintenance: The final step is to deploy the code to the target environment and maintain itover time. This may involve setting up the necessary infrastructure, monitoring the code's performance, and making updates as needed.中文回答:高级语言编程的一般流程。
抽象类英语作文
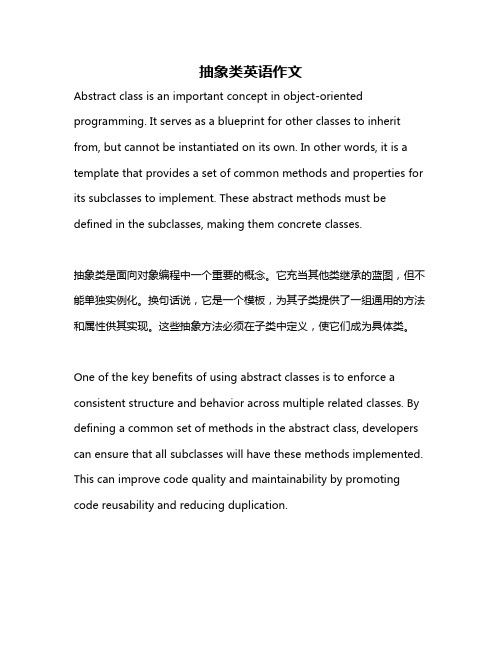
抽象类英语作文Abstract class is an important concept in object-oriented programming. It serves as a blueprint for other classes to inherit from, but cannot be instantiated on its own. In other words, it is a template that provides a set of common methods and properties for its subclasses to implement. These abstract methods must be defined in the subclasses, making them concrete classes.抽象类是面向对象编程中一个重要的概念。
它充当其他类继承的蓝图,但不能单独实例化。
换句话说,它是一个模板,为其子类提供了一组通用的方法和属性供其实现。
这些抽象方法必须在子类中定义,使它们成为具体类。
One of the key benefits of using abstract classes is to enforce a consistent structure and behavior across multiple related classes. By defining a common set of methods in the abstract class, developers can ensure that all subclasses will have these methods implemented. This can improve code quality and maintainability by promoting code reusability and reducing duplication.使用抽象类的主要好处之一是在多个相关类之间强制执行一致的结构和行为。
电脑知识很重要吗英语作文

电脑知识很重要吗英语作文Computer Knowledge: A Crucial Skill in the Modern WorldIn the era of digital transformation, the importance of computer knowledge cannot be overstated. It is a fundamental skill that has become essential for personal, educational, and professional development. This essay will explore the various reasons why computer literacy is vital in today's society.Firstly, computers have become an integral part of our daily lives. From managing personal finances to staying connected with friends and family through social media, our reliance on technology is evident. Understanding how to use computers effectively can enhance our ability to communicate, learn, and work more efficiently.Secondly, in the professional realm, computer knowledge is a prerequisite for many jobs. Whether it's using word processing software for documentation, spreadsheets for data analysis, or presentation tools for business meetings, computer skills are often listed as a basic requirement in job postings. Moreover, specialized computer skills such as programming or network administration can open up opportunities in the thriving tech industry.Thirdly, the ability to navigate the digital world iscritical for staying informed and educated. With the internetbeing a vast repository of information, those who arecomputer literate can access a wealth of knowledge on various subjects, from academic research to practical life skills.This can lead to lifelong learning and personal growth.Furthermore, computer knowledge empowers individuals to be creators, not just consumers, of digital content. From coding websites to designing graphics, having a grasp of computer applications can allow for creative expression and innovation. It can also provide a platform for entrepreneurship, as many businesses now operate primarily online.Lastly, as we become more interconnected, computer securityis a growing concern. Understanding basic computer security measures can protect individuals from cyber threats such as viruses, phishing, and identity theft. This knowledge is not only beneficial for personal safety but also for the security of the digital systems we rely on.In conclusion, computer knowledge is indeed a crucial skillin the modern world. It impacts our personal lives, opens doors in the professional sphere, enables continuous learning, fosters creativity, and ensures digital safety. As technology continues to evolve, the importance of computer literacy will only grow, making it a skill that everyone should strive to acquire.。
英语抽象类作文模板
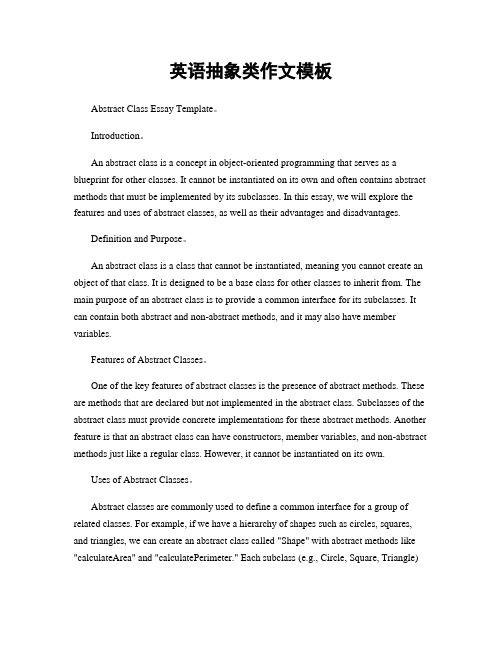
英语抽象类作文模板Abstract Class Essay Template。
Introduction。
An abstract class is a concept in object-oriented programming that serves as a blueprint for other classes. It cannot be instantiated on its own and often contains abstract methods that must be implemented by its subclasses. In this essay, we will explore the features and uses of abstract classes, as well as their advantages and disadvantages.Definition and Purpose。
An abstract class is a class that cannot be instantiated, meaning you cannot create an object of that class. It is designed to be a base class for other classes to inherit from. The main purpose of an abstract class is to provide a common interface for its subclasses. It can contain both abstract and non-abstract methods, and it may also have member variables.Features of Abstract Classes。
抽象类作文英语模版
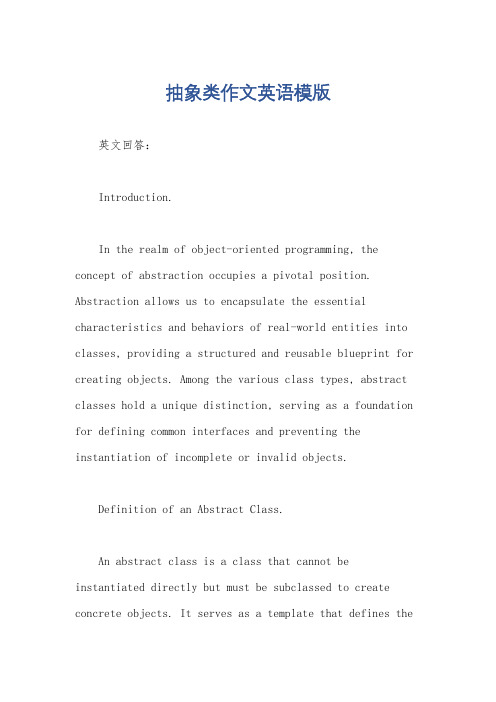
抽象类作文英语模版英文回答:Introduction.In the realm of object-oriented programming, the concept of abstraction occupies a pivotal position. Abstraction allows us to encapsulate the essential characteristics and behaviors of real-world entities into classes, providing a structured and reusable blueprint for creating objects. Among the various class types, abstract classes hold a unique distinction, serving as a foundation for defining common interfaces and preventing the instantiation of incomplete or invalid objects.Definition of an Abstract Class.An abstract class is a class that cannot be instantiated directly but must be subclassed to create concrete objects. It serves as a template that defines theinterface and shared functionality of a group of related classes. By declaring at least one of its methods as abstract, an abstract class ensures that subclasses must implement the method with specific behavior before they can be used.Characteristics of Abstract Classes.1. Cannot be instantiated: Abstract classes cannot be used to create objects directly. They exist solely to provide a blueprint for subclasses to inherit and expand upon.2. Contain abstract methods: Abstract classes declare one or more abstract methods, which are method signatures without implementations. Subclasses must override these abstract methods with concrete implementations to provide specific functionality.3. May contain concrete methods: In addition to abstract methods, abstract classes can also include concrete methods, which provide default implementationsthat subclasses can inherit or override.4. Act as a contract: Abstract classes define a contract that subclasses must adhere to. By implementing the abstract methods, subclasses guarantee that they possess the required functionality and follow the established interface.Uses of Abstract Classes.Abstract classes offer various advantages, including:1. Enforce method signatures: They ensure that subclasses implement the required methods with the correct signatures, preventing inconsistencies and enforcing a common interface.2. Promote code reusability: By providing a shared interface, abstract classes facilitate code reusability across subclasses, reducing duplication and promoting maintainability.3. Prevent incomplete objects: By prohibiting direct instantiation, abstract classes prevent the creation of invalid or incomplete objects, ensuring that only objects with complete and consistent functionality are created.4. Promote polymorphism: Abstract classes support polymorphism, allowing objects of different subclasses to be treated as objects of the abstract class, fostering flexibility and extensibility in code.Example of an Abstract Class.Consider the following abstract class representing the concept of a shape:java.public abstract class Shape {。
latex论文插图技巧总结
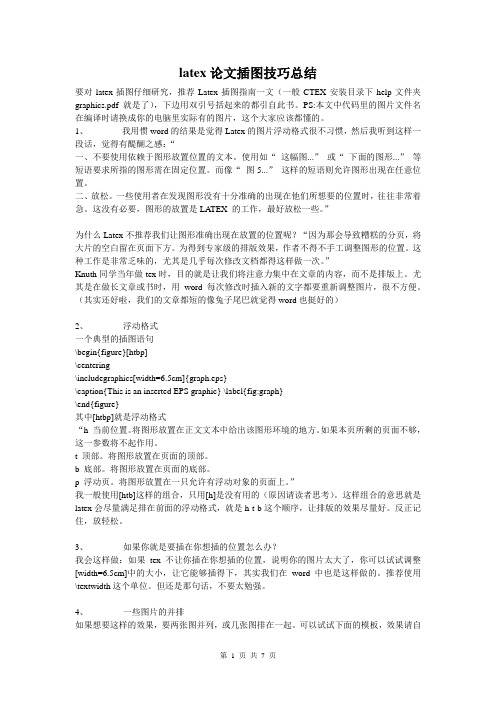
latex论文插图技巧总结要对latex插图仔细研究,推荐Latex插图指南一文(一般CTEX安装目录下help文件夹graphics.pdf就是了),下边用双引号括起来的都引自此书。
PS:本文中代码里的图片文件名在编译时请换成你的电脑里实际有的图片,这个大家应该都懂的。
1、我用惯word的结果是觉得Latex的图片浮动格式很不习惯,然后我听到这样一段话,觉得有醍醐之感:“一、不要使用依赖于图形放置位置的文本。
使用如“这幅图...”或“下面的图形...”等短语要求所指的图形需在固定位置。
而像“图5...”这样的短语则允许图形出现在任意位置。
二、放松。
一些使用者在发现图形没有十分准确的出现在他们所想要的位置时,往往非常着急。
这没有必要,图形的放置是LATEX 的工作,最好放松一些。
”为什么Latex不推荐我们让图形准确出现在放置的位置呢?“因为那会导致糟糕的分页,将大片的空白留在页面下方。
为得到专家级的排版效果,作者不得不手工调整图形的位置。
这种工作是非常乏味的,尤其是几乎每次修改文档都得这样做一次。
”Knuth同学当年做tex时,目的就是让我们将注意力集中在文章的内容,而不是排版上。
尤其是在做长文章或书时,用word每次修改时插入新的文字都要重新调整图片,很不方便。
(其实还好啦,我们的文章都短的像兔子尾巴就觉得word也挺好的)2、浮动格式一个典型的插图语句\begin{figure}[htbp]\centering\includegraphics[width=6.5cm]{graph.eps}\caption{This is an inserted EPS graphic} \label{fig:graph}\end{figure}其中[htbp]就是浮动格式“h 当前位置。
将图形放置在正文文本中给出该图形环境的地方。
如果本页所剩的页面不够,这一参数将不起作用。
t 顶部。
将图形放置在页面的顶部。
Literate Programming 教程说明书

Literate Programming using nowebTerry TherneauOctober11,20191IntroductionLet us change or traditional attitude to the construction of pro-grams.Instead of imagining that our main task is to instruct acomputer what to do,let us concentrate rather on explaining to hu-mans what we want the computer to do.(Donald E.Knuth,1984).This is the purpose of literate programming(LP for short).It reverses the ususal notion of writing a computer program with a few included comments,to one of writing a document with some embedded code.The primary organization of the document can then revolve around explaining the algorithm and logic of the code.Many different tools have been created for literate programming,and most have roots in the WEB system created by D.Knuth[2].Some of these have been language specific,e.g.CWEB or PascalWeb;this article focuses on Norman Ramsey’s noweb,an simple LP tool that is language agnostic[3,1].Most R users will already be familiar with the basic structure of a noweb document,since the noweb system was the inspiration or Sweave.2Why use LP for SDocumentation of code is often an afterthought,particularly in a high level language like S.Indeed,I have one colleague who proclaims his work to be “self-documenting code”and eschews comment lines.The counter argument is proven any time we look at someone else’s code(what are they doing?),and in fact by looking at any of our own code after a lapse of time.When we write code the thought process is from an overall structure to algorithm to R function to code;the result is clear and simple as long as that overall structure remains in our thought,but reconstructing that milleau is not easy given the code alone. For a larger project like a package,documentation is even more relevant.When I make a change to the survival package I ususallyfind that the revision is2/3 increased commentary and only1/3modified code,and a major portion of the time was spent puzzling out details that once were obvious.My use of LP methods was motivated by the coxme package.This is the most mathematically challenging part of the surival suite,and due to the need1to use sparse matrix methods it is algorithmically complex as well.It was one of the better programming decisions I’ve made.The old adage“more haste,less speed”holds for R code in general,but for packages and complex algorithms in particular.Many of you will have had the experience of puzzling over a coding or mathematics issue,thenfinally going to a colleage for advice.Then,while explaining the problem to them a solution suddenly becomes clear.The act of explaining was the key.In the same way, writing down and organizing the program logic within a document will get one to the endpoint of a working and reliable program faster.The literate programming literature contains more and better stated argu-ments for the benefit of this approach.3CodingLike an Sweavefile,the nowebfile consists of interleaved text and code chunks, the format is nearly identical.Here is thefirst section of the coxme code(after the document’s header and and an introduction).\section{Main program}The[[coxme]]code starts with a fairly standard argument list.<<coxme>>=coxme<-function(formula,data,weights,subset,na.action,init,control,ties=c("efron","breslow"),varlist,vfixed,vinit,sparse=c(50,.02),x=FALSE,y=TRUE,refine.n=0,random,fixed,variance,...){time0<-proc.time()#debugging lineties<-match.arg(ties)Call<-match.call()<<process-standard-arguments>><<decompose-formula>><<build-control-structures>><<call-computation-routine>><<finish-up>>}The arguments to the function are described below,omitting those that are identical to the\Verb!coxph!function.\begin{description}\item...The typeset code looks like this:2coxme =coxme<-function(formula,data,weights,subset,na.action,init,control,ties=c("efron","breslow"),varlist,vfixed,vinit,sparse=c(50,.02),x=FALSE,y=TRUE,refine.n=0,random,fixed,variance,...){time0<-proc.time()#debugging lineties<-match.arg(ties)Call<-match.call()process-standard-argumentsdecompose-formulabuild-control-structurescall-computation-routinefinish-up}In thefinal pdf document each of the chunks is hyperlinked to any prior or later instances of that chunk name.The structure of a noweb document is very similar to Sweave.The basic rules are1.Code sections begin with the name of the chunk surrounded by anglebrackets:<<chunk-name>>=;text chunks begin with an ampersand@.The primary difference with Sweave is that the name is required—it is the key to organizing the code—whereas in Sweave it is optional and usually omitted.There are no options within the brackets.2.Code chunks can refer to other chunks by including their names in anglebrackets without the trailing=sign.These chunks can refer to others, which refer to others,etc.In the created code the indentation of a ref-erence is obeyed.For instance in the above example the reference to “<<finish-up>>”is indented four spaces;when the definition offinish-up is plugged in that portion as a whole will be moved over4spaces.When the<<finish-up>>chunk is defined later in the document it starts at the left margin.As an author what this means is that you don’t have to remember the indentation from several pages ago,and the standard emacs indentation rules for R code work in each chunk de-novo.3.Code chunks can be in any order.4.The construct[[x<-3]]will cause the text in the interior of the bracketsto be set in the same font as a code chunk.5.Include\usepackage{noweb}in the latex document.It in turn makes useof the fancyvrb and hyperref packages.36.One can use either.Rnw or.nw as the suffix on source code.If thefirst isused then emacs will automatically set the default code mode to S,but is not as willing to recognize C code chunks.If the.nw suffix is used and you have a proper noweb mode installed1,the emacs menu bar(noweb:modes) can be used to set the default mode for the entirefile or for a chunk.The ability to refer to a chunk of code but then to defer its definition until later is an important feature.As in writing a textbook,it allows the author to concentrate on presenting the material in idea order rather than code order.To create the tex document,use the R command noweave(file)where“file”is the name of the.Rnw source.It is necessary to have a copy of noweb.sty available before running the latex or pdflatex command,a copy can be found in the inst directory of the distribution.Optional arguments to noweave areout The name of the outputfile.The default is the name of the inputfile,with thefile extension replaced by“.tex”.indent The number of spaces to indent code from the left margin.The default value is1.To extract a code chunk use the notangle command in R.Arguments are file the name of the.Rnw sourcefile.target the name of the chunk to extract.By default,notangle will extract the chunk named“*”,which is usually a dummy at the beginning of thefile that names all the top level chunks.If this is not found thefirst named chunk is extracted.During extraction any included chunks are pulled in and properly indented.out The name of the outputfile.By default this will be the inputfilename with thefile extension replaced by“.R”.For Unix users the stand alone noweb package is an alternative route.I was not able tofind a simple installation process for MacIntosh,and no version of the package at all for Windows.For R users the package option is simpler, although the standalone package has a longer list of options.Many of these, however,are concerned with creating cross-references in the printed text,which is mostly obviated by the hyperlinks.The noweave program will create a texfile with the exact same number of lines as the input,which is a help when tracking back any latex error messages —almost.The R version fails at this if the@that ends a code chunk has other text after the ampersand on the same line.Most coders don’t do this so it is rarely an issue.1The phrase proper noweb mode requires some explanation.The classic nw mode for emacs has not been updated for years,and does not work properly in emacs22or higher.However, in versions2and earlier of ESS Rnw mode was built on top of nw mode,and ESS included a noweb.elfile that was updated to work with later emacs versions.If you are using ESS2.15, say,then noweb mode worksfine.The newer ESS version12created Rnw mode from scratch, does not include a nowebfile,and emacs reverts to the old,non-working nw code.44Incorporation into RIn my own packages,noweb sourcefiles are placed in a noweb directory.Myown style,purely personal,is to have source codefiles that are fairly small,2to10pages,because Ifind them easier to edit.I then include a Makefile:below isan example for a project with one C program and several R functions.makefile =PARTS=main.Rnw\basic.Rnw\build.Rnw\formula.Rnw\varfun.Rnw varfun2.Rnw\fit.Rnw\ranef.Rnw\lmekin.Rnw\bdsmatrix.Rnwall:fun docfun:../R/all.R../src/bds.cdoc:../inst/doc/sourcecode.pdfR=R--vanilla--slave../R/all.R:all.nwecho"require(noweb);notangle(’all.nw’)">$(R)echo"#Automatically created from all.nw by noweb">tempcat temp all.R>$@../src/bds.c:all.nwecho"/*Automatically created from all.nw by noweb*/">tempecho"require(noweb):notangle(’all.nw’,target=’bds’,out=’bds.c’)">$(R)$cat temp bds.c>$@../inst/doc/sourcecode.pdf:all.nwecho"require(noweb);noweave(’all.nw’)">$(R)texi2dvi--pdf all.texmv all.pdf$@all.nw:$(PARTS)cat($PARTS)>all.nwclean:-rm all.nw all.log all.aux all.toc all.tex all.pdf-rm temp all.R bds.cThefirstfile“main”contains the definition of<<*>>=early on<<*>>=5<<bdsmatrix>><<ghol>><<print.bdsmatrix>>...listing the top level chuncks defined in each of my sub-files,which in turn contain all the R function definitions.For a more sophisticated Makefile that creates each function as a separate.Rfile look at the source code for the coxme package on Rforge.One can add a configure script to the top level directory of the package to cause this Makefile to be run automatically when the package is built.See the source code for the coxme library for an example which had input from several CRAN gurus.I found out that it is very hard to write a Makefile that works across all the platforms that R runs on,and this one is not yet perfected for that task—though it does work on the Rforge servers.When submitting to CRAN my current strategy is to run make all locally to create the documentation and functions from the nowebfiles,and not include a configurefile.I then do a standard submission process:R CMD build to make the tar.gzfile for submission,R CMD check to check it thoroughly,and then submit the tarfile. 5DocumentationThis document is written in noweb,and found in the vignettes directory of my source ing a nowebfile as a vignette is very unusual—this may be the only case that ever arises—since the goal of noweb is to document source code and the goal of vignettes is to document usage of the function.We made use of the vignetteEngines facility available in R version3in order to use noweb instead of Sweave as the default engine for the document.The noweb function itself is written(not surprisingly)in noweb,and the pdf form of the code can be found in the inst/doc directory.References[1]Johnson,Andrew L.and Johnson,Brad C.(1997).Literate Programmingusing noweb,Linux Journal,42:64–69.[2]Donald Knuth(1984).Literate Programming.The Computer Journal(British Programming Society),27(2):97–111.[3]Norman Ramsay(1994).Literate programming simplified.IEEE Software,11(5):97–105.6。
学校的设施英语作文的
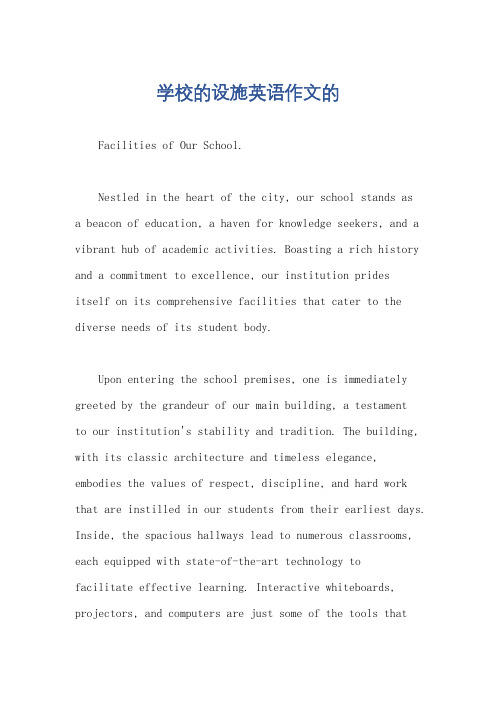
学校的设施英语作文的Facilities of Our School.Nestled in the heart of the city, our school stands asa beacon of education, a haven for knowledge seekers, and a vibrant hub of academic activities. Boasting a rich history and a commitment to excellence, our institution pridesitself on its comprehensive facilities that cater to the diverse needs of its student body.Upon entering the school premises, one is immediately greeted by the grandeur of our main building, a testamentto our institution's stability and tradition. The building, with its classic architecture and timeless elegance, embodies the values of respect, discipline, and hard work that are instilled in our students from their earliest days. Inside, the spacious hallways lead to numerous classrooms, each equipped with state-of-the-art technology tofacilitate effective learning. Interactive whiteboards, projectors, and computers are just some of the tools thatour teachers utilize to engage students and make learning fun and dynamic.Our school also boasts a fully equipped science laboratory, where students can conduct experiments and explore the wonders of the natural world. The laboratory is stocked with the latest scientific equipment, allowing students to gain practical experience and develop their skills in a safe and controlled environment. This hands-on approach to learning is crucial in fostering a generation of scientifically literate individuals who are well-prepared to contribute to society.In addition to its academic facilities, our school also places a strong emphasis on sports and physical activities. Our sports complex features multipurpose courts, a gymnasium, and an outdoor track, providing ample opportunities for students to stay fit and healthy while also developing teamwork and competitive spirits. Regular sports events and tournaments further enhance the school's vibrant sports culture, fostering a sense of camaraderie and unity among students.Moreover, our school recognizes the importance of artistic expression and creativity in the overall development of students. Our arts wing, therefore, boasts facilities such as music rooms, dance studios, and art galleries, where students can pursue their passions and express their creativity. Regular performances and exhibitions provide students with platforms to showcase their talents and receive feedback from peers and mentors.Our school also has a well-stocked library, a haven for booklovers and a repository of knowledge. The library is home to a diverse collection of books, journals, and reference materials, catering to the diverse interests and needs of our student body. Regular library hours and accessible online resources ensure that students have access to information at their fingertips, fostering a culture of curiosity and lifelong learning.Moreover, our school recognizes the importance of technology in modern education. Therefore, we have invested in creating a digital infrastructure that supportsinnovative teaching methods and enhances the learning experience. Our computer lab is equipped with the latest hardware and software, allowing students to gain skills in computer science, programming, and digital literacy. Our school also provides wireless internet access throughout the campus, facilitating easy access to educational resources and promoting collaboration among students and teachers.In addition to these facilities, our school also offers a range of extracurricular activities and clubs that cater to the interests and hobbies of students. These clubs range from academic clubs such as science clubs and debate clubs to cultural clubs such as music clubs and art clubs. These clubs provide students with opportunities to develop their leadership skills, enhance their social skills, and make new friends while pursuing their passions.Our school also places a strong emphasis on the well-being of its students. Therefore, we have a comprehensive health and counseling center that provides medical care and psychological support to students. The center is staffed byprofessional healthcare providers who are available to address any health concerns or emotional issues that students may face during their academic journey.In conclusion, our school's facilities are not just bricks and mortar; they are a testament to ourinstitution's commitment to providing a comprehensive and holistic education to our students. Our facilities cater to the diverse needs of our student body, fostering a culture of learning, discovery, and creativity. As we continue to evolve and grow, we remain committed to investing in our facilities and providing our students with the best possible learning environment.。
抽象类英语作文
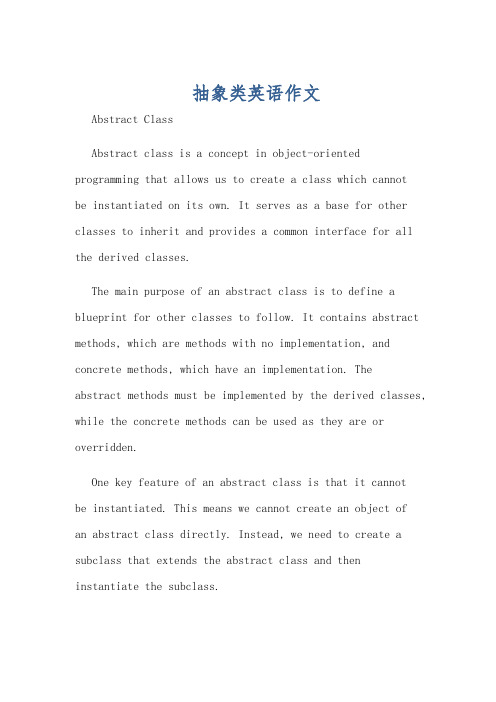
抽象类英语作文Abstract ClassAbstract class is a concept in object-oriented programming that allows us to create a class which cannotbe instantiated on its own. It serves as a base for other classes to inherit and provides a common interface for all the derived classes.The main purpose of an abstract class is to define a blueprint for other classes to follow. It contains abstract methods, which are methods with no implementation, and concrete methods, which have an implementation. Theabstract methods must be implemented by the derived classes, while the concrete methods can be used as they are or overridden.One key feature of an abstract class is that it cannotbe instantiated. This means we cannot create an object ofan abstract class directly. Instead, we need to create a subclass that extends the abstract class and theninstantiate the subclass.Another important aspect of abstract class is that itcan have member variables just like a regular class. These member variables can be inherited by the subclasses andused as per their requirements.In Java, we use the "abstract" keyword to declare an abstract class. Any class that contains at least oneabstract method must be declared as an abstract class. We also use the "extends" keyword to create a subclass that inherits from the abstract class.In conclusion, abstract class is a powerful tool inobject-oriented programming that allows us to define a common interface for a group of related classes. It helpsin achieving abstraction and reusability in our code, making it easier to maintain and extend our software system.抽象类抽象类是面向对象编程中的一个概念,允许我们创建一个不能单独实例化的类。
latex论文插图技巧总结
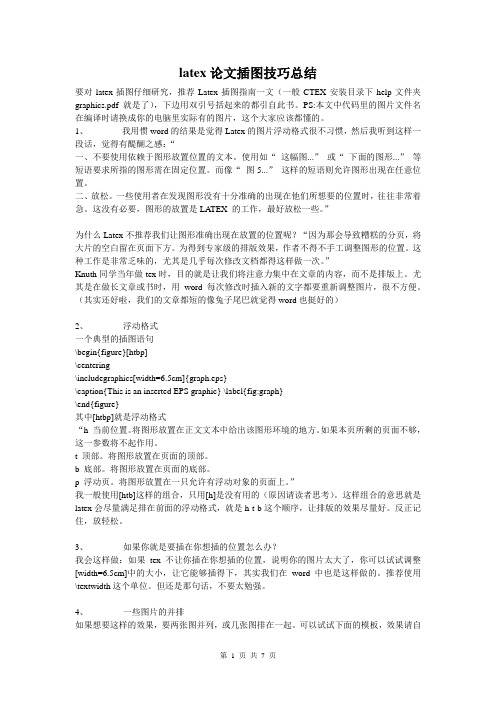
latex论文插图技巧总结要对latex插图仔细研究,推荐Latex插图指南一文(一般CTEX安装目录下help文件夹graphics.pdf就是了),下边用双引号括起来的都引自此书。
PS:本文中代码里的图片文件名在编译时请换成你的电脑里实际有的图片,这个大家应该都懂的。
1、我用惯word的结果是觉得Latex的图片浮动格式很不习惯,然后我听到这样一段话,觉得有醍醐之感:“一、不要使用依赖于图形放置位置的文本。
使用如“这幅图...”或“下面的图形...”等短语要求所指的图形需在固定位置。
而像“图5...”这样的短语则允许图形出现在任意位置。
二、放松。
一些使用者在发现图形没有十分准确的出现在他们所想要的位置时,往往非常着急。
这没有必要,图形的放置是LATEX 的工作,最好放松一些。
”为什么Latex不推荐我们让图形准确出现在放置的位置呢?“因为那会导致糟糕的分页,将大片的空白留在页面下方。
为得到专家级的排版效果,作者不得不手工调整图形的位置。
这种工作是非常乏味的,尤其是几乎每次修改文档都得这样做一次。
”Knuth同学当年做tex时,目的就是让我们将注意力集中在文章的内容,而不是排版上。
尤其是在做长文章或书时,用word每次修改时插入新的文字都要重新调整图片,很不方便。
(其实还好啦,我们的文章都短的像兔子尾巴就觉得word也挺好的)2、浮动格式一个典型的插图语句\begin{figure}[htbp]\centering\includegraphics[width=6.5cm]{graph.eps}\caption{This is an inserted EPS graphic} \label{fig:graph}\end{figure}其中[htbp]就是浮动格式“h 当前位置。
将图形放置在正文文本中给出该图形环境的地方。
如果本页所剩的页面不够,这一参数将不起作用。
t 顶部。
将图形放置在页面的顶部。
programming language笔记

programming language笔记Programming languages are formal languages designed to instruct computers and create software programs. They are used by developers to write, edit, and execute code for a variety of applications and tasks.There are numerous programming languages available, each with its own syntax, rules, and purposes. Some popular programming languages include:1. C: Developed in the early 1970s, C is a versatile and widely-used language known for its efficiency and low-level access to memory. It is mainly used for system programming and developing applications like operating systems and embedded systems.2. C++: C++ is an extension of the C programming language and is known for its object-oriented programming capabilities. It is commonly used for game development, desktop applications, and performance-critical programs.3. Java: Java is a high-level language that is popular for its "write once, run anywhere" approach, as it can run on any platform with Java Virtual Machine (JVM) support. It is commonly used for building enterprise applications, Android apps, and large-scale web applications.4. Python: Python is a high-level, interpreted language that emphasizes code readability and simplicity. It is widely used for a variety of applications, including web development, data analysis, artificial intelligence, and scientific computing.5. JavaScript: JavaScript is primarily used for web development to add interactivity and functionality to websites. It is a client-side scripting language that runs in the web browser.6. Ruby: Ruby is a dynamic, object-oriented language known for its simplicity and readability. It is often used for web development, specifically with the Ruby on Rails framework.7. Swift: Swift is a relatively new programming language developed by Apple for iOS, macOS, watchOS, and tvOS app development. It is designed to be fast, safe, and expressive.Each language has its own strengths and weaknesses, and the choice of programming language often depends on the specific requirements and goals of a project. Programmers often learn multiple programming languages to be versatile and adaptable to different development tasks.。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
Using Literate Programming to TeachGood Programming PracticesStephen ShumComputer Science DepartmentAugustana College Sioux Falls, SD 57197shum@ Curtis CookComputer Science Department Oregon State University Corvallis, Oregon 97331-3202cook@A b s t r a c tThe ability to comprehend a program written by other individuals is becoming increasingly important in software development and maintenance. In an attempt to encourage undergraduate Computer Science students to write informative and usable documentation, the literate programming paradigm was incorporated into the teaching of one undergraduate Computer Science course at Augustana College. This paper describes the concept of literate programming,the experience of using literate programming to teach good programming practices, and the results from the experiment that showed that literate programming encourages more documentation.IntroductionThe ability to comprehend a program written by other individuals is becoming increasingly important in software development and maintenance. Studies have shown that 30-90%of software expenditure is spent on maintainingexisting software [15, 12]. Studies have also shown that maintenance programmers spend about half of their time studying the code and related documentation. This has led Standish[12]to conclude that the cost of comprehending a program is the dominant cost of a program over its entire life cycle.A survey by Chapin[4] of maintainers showed that they perceived poor documentation as the biggest problem in software maintenance work.Poor documentation has one or more of the following characteristics [9, 4, 8] :a. Nonexistent and incomplete.b. Inconsistency between code and documentation.c. Difficulty in finding information.d. Not appropriate for all levels of programmer experience.There are a number of CASE tools available that claim to satisfy the documentation needs of software maintenance. These tools generate automatic documentation in the form of reports by static analysis of source code. Examples of documentation produced are: control flow chart,data flow chart, cross-reference listings, metric reports, call graphs, module hierarchy chart, etc. All of this information is helpful to maintenance programmers to become familiar with the structure of a program and to navigate around the program during maintenance investigation. What this documentation fails to provide is insight into why a particular program structure is used, or how the program functions. It also fails to provide information on other relationships between program components other than these syntactical relationships [4].As computer science teachers we have the responsibility to teach students about the importance of informative and usable documentation as well as readable code. However, students usually see documentation and sometimes the design process as non-essential and extra compared to coding. They do not see the need of documenting a program and writing readable code. One method we are using to encourage more readable programs is to assign a relatively high percentage of the programming assignment grade to documentation and readability. In fact, the grading scheme used by the author at Augustana College in all of his computer science courses: 25% each for correctness, design, readability, and documentation. Such a grading scheme has been found to be quite effective in forcing students into the habit of using meaningful names and effective program formatting, including internal comments, and providing external program design documentation in the form of a pseudocode. However, the quality of the documentation is far from being informative since most of the comments are usually commenting the obvious. In addition, most students admit that they generate the pseudocode document from the program code after the program has been tested.Literate programming [7] seemed an ideal solution to the problem of teaching good documentation practices and helping students write informative and usable documentation. Literate programming emphasizes writing programs that are intended to be read by humans rather than a computer. A literate program is a single documentation (file) containing both documentation and program. It presents the code and documentation in an interleaved fashion that matches the programmer's mental representation.Program design, design and code decisions, and other useful information for the reader are included in a literate program. One key feature of literate programming is that the source code and documentation are created simultaneously. Hence students writing literate programs see the importance of documentation and clearly see the relation between the design and code.To test these ideas and the claim that literate programming encourages more and better documentation we introduced literate programming into a junior level computer science programming in Spring semester 1992. The AOPS (Abstraction Oriented Programming System) literate programming system [11] was used. For an experiment in the class students did two program assignments in AOPS and two in Turbo C. This paper describes the experiences teaching literate programming and results from the experiment.Literate ProgrammingKnuth [7] coined the term "literate programming" to emphasize writingcode that is intended to be read by humans. He believes that the format and structure of a program should be designed to communicate primarily with the humans who read the program rather than the computers that execute the program. The presentation of the code should proceed according to the mental patterns of the author/programmer rather than the patterns demanded by the language and compiler. He claims that programming in this way produces better programs with better documentation.The literate programmer writes a source file with the program code and documentation interleaved. The literate paradigm recognizes that two different audiences, human readers and compilers, will receive the program. For the human readers, there is a filter program that transforms the source file program code and documentation into a form that can be processed by a document formatter. For the computer, there is a filter program that produces a source code program suitable for input to a compiler from the source file.It is important to note that in literate programming program code and documentation are created simultaneously. Literate programming systemscontain mechanisms for associating documentation with sections of code and give the programmer freedom to write the program sections in an order that maximizes program comprehension. Hence instead of being appended as extra information to the program, documentation is viewed as an integral part of the program. Its omission, rather than its inclusion, is what is most noticeable.WEB To promote the literate programming concept, Knuth [7] developed the WEB system as an example literate programming system. In WEB a programmer writes a source file with the program code and documentation interleaved. WEB has two filter programs: Weave and Tangle. Weave transforms the source file into a form that can be processed by T E X. Tangle extracts the program code from the source file and puts it into a form that can be input to a Pascal compiler. This process is shown in Figure 1.Weave TEX---------> file.human -------------> typeset| listing|file.web ---||| compiled---------> file.machine -----------> programTangle PascalcompilerFigure 1. The Processing Paths in Web.AOPS (Abstraction Oriented Programming System)Several Web-like literate programming systems have been developed since 1984 [1, 5, 6, 10, 13, 14, 15]. However, all of these systems including WEB are programming language and typesetting language dependent, and they do not allow flexible listing and viewing of program code and documentation. Although AOPS [11] is WEB-like, it is programming language and typesetting language independent. Among the other features of AOPS are a flexible lister which allows users to print selected portions of the program code and/or documentation and a hypertext browser which allows users to display relevant information on the screen while suppressing irrelevant details.AOPS ProgramAn AOPS program is written in levels of abstractions. It consists of AOPS rules defining the highest level abstraction and all the abstractions used directly and indirectly by the highest level abstraction. A rule consists of an abstraction name, equal sign, type and a body.The abstraction name (hereafter referred to as AO-name) is a string of characters of any length delimited by a character not used in the AOPS source file for any other purpose. (We will use the at sign, @, in our examples.) There are three basic types of AOPS rules.1. The Code RuleThe program code portion of an AOPS literate program is constructed from code rules of the form:AO-name=code AO-bodywhere AO-body consists of legal statements of the underlying programming language with embedded AO-names. (Think of an AO-name embedded in an AO-body of a code rule as a macro call.) The code rule in essence embodies the goal-plan structure of computer programs. The AO-name specifies the goal and the AO-body specifies the plan used to achieve the goal. AOPS provides the ability to explicitly describe this goal-plan structure in a very natural way that is not restricted by the syntax of the programming language. For example, usingPascal as the programming language, the code rule for @8-queens program@ is defined as: @8-queens program@=codeprogram eightqueens;var @variables of 8-queens@@procedures and functions of 8-queens@begin@8-queens solution@endThere are code rules that define each of the three abstractions, @variables of 8-queens@, @procedures and functions of 8-queens@, and @8-queens solution@. These may occur before or after the @8-queens program@ rule.2. The Textdoc RuleTo help readers understand the code definition of an abstraction, AOPS allows a programmer to associate textual or graphical documentation with the abstraction name. Textual documentation such as design decisions, alternate solutions, or anything that will help readers comprehend the code refinement is defined by a textdoc rule of the form:AO-name=textdoc AO-bodywhere AO-body is a string of any characters. The textdoc rule (and the graphicdoc rule described below) is completely invisible to the compiler of the programming language. Hence an AOPS user can use his or her favorite word processor to typeset the documentation. For example, the textdoc rule for @8queensprogram@ is: @8-queens program@=textdoc Description: Given are an 8X8 chessboard and 8 queens which are hostile to each other. Find a position for each queen such that no queen may be taken by any other queen, i.e., every row, column, and diagonal contains at most one queen.Input: noneOutput: The positions of the 8 hostile queens3. The Graphicdoc RuleOne major criticism of Knuth's several literate programs is the lack of diagrams. This is not because one cannot incorporate figures and diagrams in a WEB program, but more likely due to the fact that WEB does not encourage programmers to include pictorial documentation. AOPS, on the other hand, encourages users to include pictures and diagrams by providing a special rule for graphical documentation and allowing users to use their favorite word processors to compose the pictures. The graphicdoc rule has the form:AO-name=graphicdoc AO-bodywhere AO-body is a pictorial illustration. Figure 2 gives an example of a graphicdoc rule.AOPS imposes no restriction on the ordering of the rules so that an AOPS program can be designed and developed in an order or style preferred by the programmer free of the restrictions of the programming@8-queens program@=graphicdocOne acceptable solution to the 8-queens problem is:QQQQQQQQFigure 2: Example of graphicdoc rule.language syntax. Hence an AOPS programmer may break a task into subtasks, and tackle the subtasks in whatever order he or she prefers.Experience Teaching Literate ProgrammingIn Spring 1992, we used AOPS in one of our course offerings. The course syllabus was basically not affected by this incorporation except one class period was used to discuss AOPS. AOPS fit in nicely with teaching program design.AOPS implicitly suggests the design methodology Functional Design (FD) expressed using a pseudocode PDL (Program Design Language). Both FD and PDL are widely practiced and taught in undergraduate programming courses. AOPS treats the PDL design embedded as code rules and supports a smooth transition form design to code. Hence students no longer see PDL design as an extra and nonessential step but as a natural step in the software development process, even for small problems. The Experiment The experiment was designed to test one of the claims of the literate programming paradigm: that literate programming encourages more documentation.Hypothesis : Programmers are likely to includemore documentation, as measured by the ratio between the number of comment characters and the number of code characters, when using aliterate programming system than when using atraditional programming system.Independent and Dependent Variables :The independent variable is the programming system used: literate versus traditional. The dependent variable is the ratio (number of comment characters) /(number of codecharacters), although the ratios (number ofcomment words)/(number of code words) and (number of comment lines)/(number of code lines) were also computed and analyzed.The reason that these ratios were used instead of simply the comment counts is that the amount of comments should be in proportion to amount of code. That is, it seems reasonable for programs with more code to contain more ment counts alone ignore the amount of code. So even if literate programs are found to have more comments than traditional programs, it could be because they have more code thantraditional programs and not becauseprogrammers are likely to include morecomments using a literate system. The statistic(comment character)/(code character) gives the number of comment characters associated with each code character.Design : In order to control the wide individual variability, a within-subjects design was used.Each subject saw both experimental conditions which served as a control for his or her own performance.Subjects: Our subjects were 16 students enrolled in the class. Ten subjects were seniors, three juniors, and three post college. As a part of the class, they were required to learn and write programs using AOPS and to write programs using Turbo C.Procedure: The subjects were randomly divided into two groups, A and B, by matching the students according to their GPA. The two students in each of the matching pairs are randomly assigned, one to group A and one to group B. As part of the class all students were instructed in the use of AOPS. Two programming assignments were given. Group A was to do the first assignment in AOPS and the second in Turbo C while Group B was to do the first in Turbo C and the second in AOPS. Both groups were given the same problem specifications. Both assignments were to follow the same project guidelines handed out at the beginning of the semester. They had ten days to do each assignment. Graded assignments were not handed back until after both assignments were turned in.During that three-week period when the students are working on the programs, there was no discussion about the documentation for their program assignments. In addition, every example program discussed in class was presented both as a literate program and as a traditional program, with the same amount of documentation in both versions.Results: Two filter programs and the UNIX utility wc were used to obtain line, word, and character counts for the students programs. One filter program extracted the C source code without comments and the other filter program extracted the comments. Line, word, and character counts for the source code and comments for each program were obtained using wc. Tables 1 and 2 give the average line, word, and character counts for each group for program assignments 1 and 2 respectively. Recall that Group A did assignment 1 in AOPS and assignment 2 in Turbo C while Group B did assignment 1 in Turbo C and assignment 2 in AOPS. Notice that the source code and comment lines for each assignment are nearly identical, but the group using AOPS had substantially more comment words and comment characters.To compare the two methods (literate programming and traditional) we used the ratio of comment to source lines, words and characters (see Table 3). An ANOVA showed a significant difference (p = 0.01) between the two methods for both the ratio of comments to source code words and the ratio of comment to source code characters.Source Code CommentsLines Words Chars Lines Words Chars Group A2857294409279170610,673 Group B298785462027512778,686Table 1. Counts for Programming Assignment No. 1Source Code CommentsLines Words Chars Lines Words Chars Group A23661738562199846,424 Group B230619388522614189,223 Table 2. Counts for Programming Assignment No. 2Comment/Source CodeLines Words Characters Literate 0.98 2.32 2.52Traditional0.931.61 1.78Table 3. Ratio of Comment to Source Code Lines, Words and Characters.It was surprising that the literate programscontained significantly more comment words and characters, but not more comment lines than the traditional programs. Table 4 shows a detailed analysis of the non-blank comment lines for the AOPS and Turbo C programs. A Mann-Whitney U test showed a significant difference (p<0.001)between the two methods for the number of comments, lines per comment, words per comment, and characters per comment. A major reason for the differences is that the traditional programs contained many marker comments such as /*end of while*/ that occupied part of a line.Whereas the literate program comments were usually in paragraphs and occupied entire lines.To discover what the "more" documentation in literate programs is made up of, an analysis was done in which each comment in both groups was examined and a decision was made on whether the comment included a what element, a how element, or an example. A comment contains a what element if it describes the purpose of its associated block of code; it contains a how element if it describes the algorithm used by its associated block of code; and it contains an example if it gives an illustration of what its associated block of code does. The comment was also checked against its associated block of code to see if it was inconsistent. (An inconsistent comment is a comment that does not accurately describe its associated piece of code.)The data is summarized in Table 5.Non-Blank Line CommentsNumber Lines Words CharsLiterate 312531,5629,948Traditional672471,1307,554Table 4. Data for non-blank comment lines.Number of comments that include include include arewhathow exampleinconsistentLiterateall (512)2550Traditionalall (1072)00Table 5. Analysis of Information Contents of CommentsAll the comments examined in both groups were deemed to contain the what element. For literate programs, 25 comments (5%) were found to contain the how element and 5 comments (1%)were found to contain an example. No comment in the traditional group contained the howelement or an example. This finding was very surprising since both groups were using the same commenting guidelines and grading scheme for both assignments. That both the literate and traditional versions contained "what comments"follows from the grading scheme. What is surprising is that only the literate versions contained "how comments" and examples. This suggests that literate programming inspires more substantial comments than traditional programming.Although no inconsistencies were found between the internal comments and the code in both versions, we did find inconsistencies between the source code and external documentation in the traditional programs. For the traditional programs the students had to hand in a pseudocode design for their programs. The pseudocode design was embedded in the AOPS program. When we compared the pseudocode design and the source code for the Turbo C programs we found 10 instances of missing steps, extra steps, misspelled names, etc. We did not find any inconsistencies in the AOPS programs. For more information about this experiment, please refer to [11].Although this experiment showed that the literate programs contain more documentation and better documentation than the traditional programs, a survey of general opinions about literate programming drew mixed opinions. When asked whether they would use a literate programming system to develop their own software, 13 out of 16 students responded yes. When asked why they would use a literate programming system, the majority responded that it was because the pseudocode was embedded in the source code which made the design step a logical step to do. When asked if they would use AOPS again, only 5 out of the 16 said they would. When asked why they would not use AOPS, the majority said it was too confusing and difficult to use AOPS when debugging a program.ConclusionOur experiment shows the literate programming paradigm indeed encourages more and consistent documentation. The students seem to like the literate programming style, except they do not like the debugging process required by the literate programming paradigm. However, this may be a legacy of Knuth's belief that in literate programming the source program should not be changed without changing the associated documentation. He purposely wanted the source code to be difficult to read so that the source file would be changed. The net result was that it was more likely that the documentation would be updated when a change was made to the program source code.This seems to hint that the state of debugging in current literate programming systems is less than desirable. Although AOPS is language independent, it still could not escape the fact that debugging using a literate programming system is troublesome. Perhaps tools such as a debugger should be made as an integral part of a literate programming system.Finally, a version of AOPS program including the the processor AOP, the lister AOL, and the hypertext browser AOB have been implemented for the IBM-PC using Turbo C. We will gladly furnish a copy of the AOPS program to anyone who sends us a diskette.References1. Avenarius, A. and Oppermann, S., "FWEB:A Literate Programming System For FORTRAN8x", ACM SIGPLAN Notices, Vol.25, No.1, pp. 52-58, Jan 1990.2. Brown, M., and Cordes, D., "A Literate Programming Design Language", Proc. CompEuro 90, IEEE International Conf. Computer Systems and Software Engineering, IEEE CS Press, Los Alamitos, Calif., 1990, pp. 548-549.3. Chapin, N., "Software Maintenance: A Different View", AFIPS Conference Proceeding, 54th National Computer Conference, 1985, pp. 509-513.4. Fletton N., and Munro, M. "Redocumenting Software Systems Using Hypertext Technology", IEEE Conference on Software Maintenance, 1988, pp. 54-59.5. Gurari, E. and Wu, J., "A WYSIWYG Literate Programming System [Preliminary Report]",Proceedings CSC '91, 1991, pp. 94-104.6. Hyman, M., "Literate C++", Computer Language, Jul 1990, Vol. 7, No.7, pp. 67-79.7. Knuth, D. E., "Literate Programming", The Computer Journal, Vol. 27, No. 2, 1984, pp. 97-111.8. Martin, J. and McClure, C., Software Maintenance: The Problem and Its Solutions. Prentice-Hall, 1983.9. Y. Nakamoto, Y., Iwamoto, T., Hori, M., Hagihara, K., Tokura, N., "An Editor for Documentation in C-system to Support Software Development and Maintenance", IEEE 6th International Conference on Software Engineering, 1982, pp. 330-339.10. Reenskaug, T., Skaar A., "An Environment For Literate Smalltalk Programming", OOPSLA 1989 Proceedings, pp. 337-345.11. Shum, S., Cook, C., "AOPS: An Abstraction Oriented Programming System For Literate Programming", Software Engineering Journal , Vol. 8 No. 3 (May 1993), pp. 113-120.12. Standish, T., "An Essay on Software Reuse", IEEE Trans on Software Engineering, Vol.SE-10, No.5, pp. 494-497, Sep 1984.13. Thimbleby, H., "Experience of 'Literate Programming' Using CWEB [a Variant of Knuth's WEB]", The Computer Journal, Vol. 29, No. 3, 1986, pp. 201-211.14. Tung, S. H., "A Structured Method For Literate Programming", Structured Programming, 1989, Vol. 10, No. 2, pp.113-120.15. Wu,Y. and Baker,T., "A Source Code Documentation System For Ada", ACM Ada Letters, Vol.9, No.5, pp. 84-88, Jul/Aug 1989.16. Zvegintzov, N., "Nanotrends", Datamation, Aug 1983, pp. 106-116.。