9.继承和多态 应用和示例
继承的经典案例

继承的经典案例继承是指在父类的基础上,派生出新的类,从而形成类之间的层次关系,新的派生类继承了父类的属性和方法。
继承是面向对象编程中的基本特性,也是软件开发中十分重要的概念。
下面,我们将介绍一些关于继承的经典案例。
1. 动物和猫动物是一个非常广泛的类别,它包含了很多不同的子类,如猫、狗、牛等。
如果我们想要设计一个程序来模拟这些不同的动物,就可以用继承的思想来解决这个问题。
我们可以定义一个基本的动物类 Animal,然后再定义一个猫类 Cat,让 Cat 继承 Animal。
Animal 类中可以定义一些基本的属性和方法,如颜色、体型等属性,以及吃、睡等方法。
Cat 类可以继承这些属性和方法,并增加一些猫特有的属性和方法,如抓老鼠等。
这样,在模拟不同的动物时,我们就可以共享基本属性和方法,同时又可以根据需要增加某些特定的属性和方法。
2. 图形和矩形另一个经典的继承案例是图形和矩形。
图形是一个抽象的概念,它可以包含很多不同种类的子类,如矩形、圆形、三角形等。
我们可以定义一个基本的图形类 Shape,然后再定义一个矩形类Rectangle,让 Rectangle 继承 Shape。
在 Shape 中,我们可以定义一些基本的属性和方法,如颜色、面积等属性,以及计算周长、面积等方法。
Rectangle 类可以继承这些属性和方法,并增加一些矩形特有的属性和方法,如宽、高等。
这样,在模拟不同的图形时,我们可以共享基本属性和方法,同时又可以根据需要增加某些特定的属性和方法。
3. 人和学生人和学生是另一组经典的继承案例。
人是一个非常广泛的类别,它可以包含很多不同种类的子类,如学生、教师、医生等。
我们可以定义一个基本的人类 Person,然后再定义一个学生类Student,让 Student 继承 Person。
在 Person 中,我们可以定义一些基本的属性和方法,如姓名、年龄等属性,以及吃、睡等方法。
Student 类可以继承这些属性和方法,并增加一些学生特有的属性和方法,如学号、课程等。
java_if复杂条件_转换_继承_多态实现_概述及解释说明
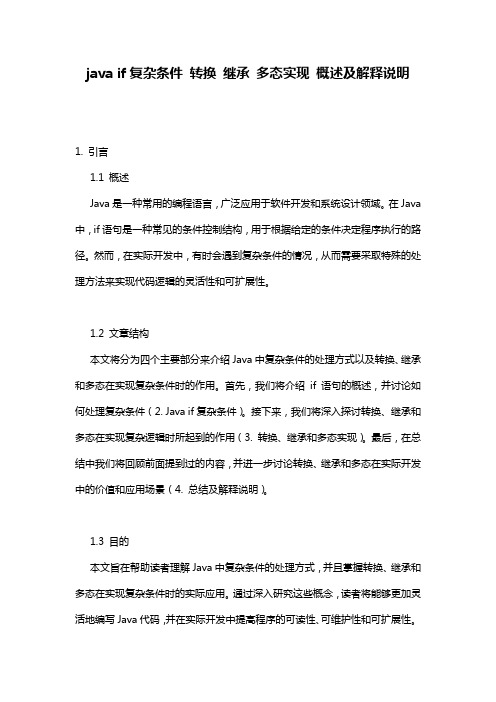
java if复杂条件转换继承多态实现概述及解释说明1. 引言1.1 概述Java是一种常用的编程语言,广泛应用于软件开发和系统设计领域。
在Java 中,if语句是一种常见的条件控制结构,用于根据给定的条件决定程序执行的路径。
然而,在实际开发中,有时会遇到复杂条件的情况,从而需要采取特殊的处理方法来实现代码逻辑的灵活性和可扩展性。
1.2 文章结构本文将分为四个主要部分来介绍Java中复杂条件的处理方式以及转换、继承和多态在实现复杂条件时的作用。
首先,我们将介绍if语句的概述,并讨论如何处理复杂条件(2. Java if复杂条件)。
接下来,我们将深入探讨转换、继承和多态在实现复杂逻辑时所起到的作用(3. 转换、继承和多态实现)。
最后,在总结中我们将回顾前面提到过的内容,并进一步讨论转换、继承和多态在实际开发中的价值和应用场景(4. 总结及解释说明)。
1.3 目的本文旨在帮助读者理解Java中复杂条件的处理方式,并且掌握转换、继承和多态在实现复杂条件时的实际应用。
通过深入研究这些概念,读者将能够更加灵活地编写Java代码,并在实际开发中提高程序的可读性、可维护性和可扩展性。
同时,本文还将对以后的研究方向进行一些展望,探讨转换、继承和多态在未来发展中的潜在作用和可能的创新点。
2. Java if复杂条件2.1 if语句概述在Java编程中,if语句用于根据给定的条件执行相应的代码块。
简单的if语句通常只涉及到一个条件判断,但是在实际开发中,我们常常会遇到更复杂的条件判断情况。
2.2 复杂条件的处理方式对于复杂条件的处理,可以利用逻辑运算符(如与&&、或||、非!)来组合多个简单条件,从而构成复杂条件。
通过将多个简单条件连接起来,我们可以得到更精确和具体的判断结果。
此外,在处理复杂条件时还可以使用括号来改变运算优先级,以确保条件表达式按照我们期望的顺序进行求值。
2.3 示例与说明下面是一个使用if语句处理复杂条件的示例:```javaint num = 10;if (num > 0 && num < 100) {System.out.println("num是一个介于0和100之间的数");} else if (num <= 0) {System.out.println("num是一个非正数");} else {System.out.println("num是一个大于等于100的数");}```在上述示例中,首先我们使用了两个简单条件`num > 0`和`num < 100`并通过逻辑运算符&&将它们连接起来形成一个复杂条件。
java模拟面试题目(3篇)
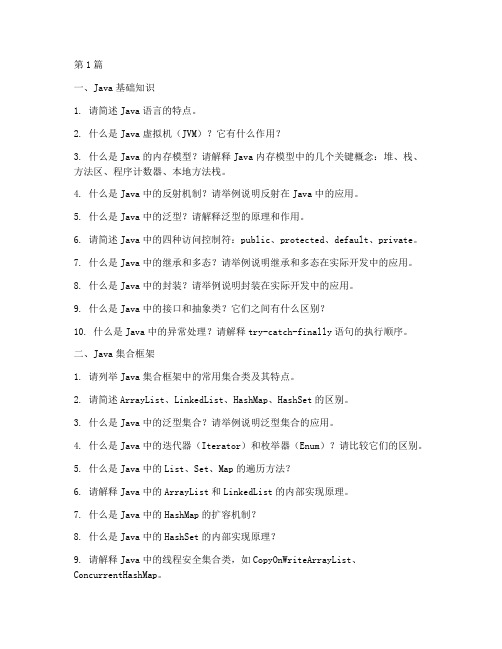
第1篇一、Java基础知识1. 请简述Java语言的特点。
2. 什么是Java虚拟机(JVM)?它有什么作用?3. 什么是Java的内存模型?请解释Java内存模型中的几个关键概念:堆、栈、方法区、程序计数器、本地方法栈。
4. 什么是Java中的反射机制?请举例说明反射在Java中的应用。
5. 什么是Java中的泛型?请解释泛型的原理和作用。
6. 请简述Java中的四种访问控制符:public、protected、default、private。
7. 什么是Java中的继承和多态?请举例说明继承和多态在实际开发中的应用。
8. 什么是Java中的封装?请举例说明封装在实际开发中的应用。
9. 什么是Java中的接口和抽象类?它们之间有什么区别?10. 什么是Java中的异常处理?请解释try-catch-finally语句的执行顺序。
二、Java集合框架1. 请列举Java集合框架中的常用集合类及其特点。
2. 请简述ArrayList、LinkedList、HashMap、HashSet的区别。
3. 什么是Java中的泛型集合?请举例说明泛型集合的应用。
4. 什么是Java中的迭代器(Iterator)和枚举器(Enum)?请比较它们的区别。
5. 什么是Java中的List、Set、Map的遍历方法?6. 请解释Java中的ArrayList和LinkedList的内部实现原理。
7. 什么是Java中的HashMap的扩容机制?8. 什么是Java中的HashSet的内部实现原理?9. 请解释Java中的线程安全集合类,如CopyOnWriteArrayList、ConcurrentHashMap。
三、Java多线程与并发1. 什么是Java中的线程?请解释线程的创建、调度和同步。
2. 请简述Java中的线程状态,如新建、就绪、运行、阻塞、等待、超时等待、终止。
3. 什么是Java中的同步机制?请解释synchronized关键字的作用。
实验三 继承和多态
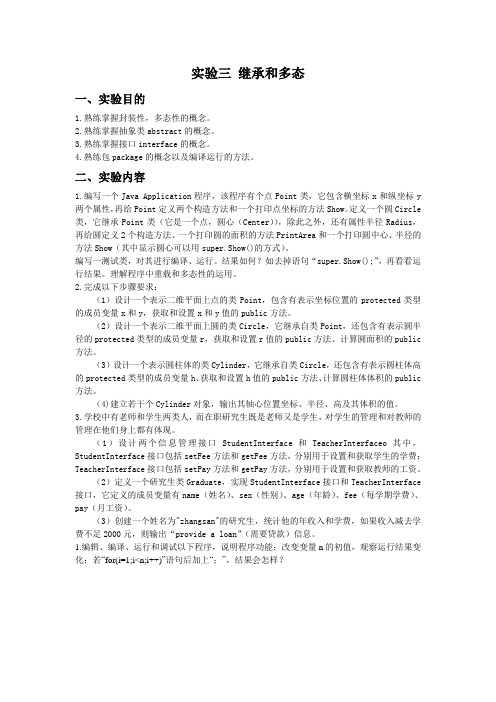
实验三继承和多态一、实验目的1.熟练掌握封装性,多态性的概念。
2.熟练掌握抽象类abstract的概念。
3.熟练掌握接口interface的概念。
4.熟练包package的概念以及编译运行的方法。
二、实验内容1.编写一个Java Application程序,该程序有个点Point类,它包含横坐标x和纵坐标y 两个属性,再给Point定义两个构造方法和一个打印点坐标的方法Show。
定义一个圆Circle 类,它继承Point类(它是一个点,圆心(Center)),除此之外,还有属性半径Radius,再给圆定义2个构造方法、一个打印圆的面积的方法PrintArea和一个打印圆中心、半径的方法Show(其中显示圆心可以用super.Show()的方式)。
编写一测试类,对其进行编译、运行。
结果如何?如去掉语句“super.Show();”,再看看运行结果。
理解程序中重载和多态性的运用。
2.完成以下步骤要求:(1)设计一个表示二维平面上点的类Point,包含有表示坐标位置的protected类型的成员变量x和y,获取和设置x和y值的public方法。
(2)设计一个表示二维平面上圆的类Circle,它继承自类Point,还包含有表示圆半径的protected类型的成员变量r,获取和设置r值的public方法、计算圆面积的public 方法。
(3)设计一个表示圆柱体的类Cylinder,它继承自类Circle,还包含有表示圆柱体高的protected类型的成员变量h、获取和设置h值的public方法、计算圆柱体体积的public 方法。
(4)建立若干个Cylinder对象,输出其轴心位置坐标、半径、高及其体积的值。
3.学校中有老师和学生两类人,而在职研究生既是老师又是学生,对学生的管理和对教师的管理在他们身上都有体现。
(1)设计两个信息管理接口StudentInterface和TeacherInterfaceo其中,StudentInterface接口包括setFee方法和getFee方法,分别用于设置和获取学生的学费;TeacherInterface接口包括setPay方法和getPay方法,分别用于设置和获取教师的工资。
C语言中的多态与继承
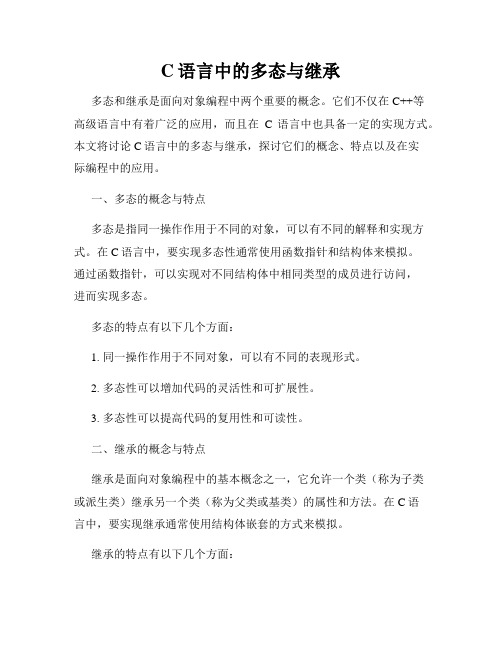
C语言中的多态与继承多态和继承是面向对象编程中两个重要的概念。
它们不仅在C++等高级语言中有着广泛的应用,而且在C语言中也具备一定的实现方式。
本文将讨论C语言中的多态与继承,探讨它们的概念、特点以及在实际编程中的应用。
一、多态的概念与特点多态是指同一操作作用于不同的对象,可以有不同的解释和实现方式。
在C语言中,要实现多态性通常使用函数指针和结构体来模拟。
通过函数指针,可以实现对不同结构体中相同类型的成员进行访问,进而实现多态。
多态的特点有以下几个方面:1. 同一操作作用于不同对象,可以有不同的表现形式。
2. 多态性可以增加代码的灵活性和可扩展性。
3. 多态性可以提高代码的复用性和可读性。
二、继承的概念与特点继承是面向对象编程中的基本概念之一,它允许一个类(称为子类或派生类)继承另一个类(称为父类或基类)的属性和方法。
在C语言中,要实现继承通常使用结构体嵌套的方式来模拟。
继承的特点有以下几个方面:1. 子类可以拥有父类的属性和方法。
2. 子类可以覆盖父类的方法,实现自己的特定功能。
3. 继承可以实现代码的重用和扩展,提高代码的效率和可维护性。
三、C语言中多态与继承的应用在C语言中,多态和继承可以通过结构体、函数指针以及函数调用的方式来实现。
首先,我们需要定义一个基类结构体,包含一些通用的属性和方法。
然后,针对不同的具体情况,可以定义多个不同的派生类结构体,继承基类的属性和方法,并在派生类中实现自己特定的操作。
接下来,我们需要定义一个函数指针成员,用于指向不同派生类中的方法。
通过函数指针的动态绑定,可以在运行时确定调用哪一个具体的方法,实现多态的效果。
最后,在调用函数的时候,可以使用基类的指针指向不同的派生类对象,通过函数指针调用对应的方法。
由于函数指针的动态绑定,程序会根据对象的实际类型来决定调用哪个方法,实现多态的效果。
通过上述方式,我们可以在C语言中模拟出多态和继承的特性,实现代码的复用、扩展和灵活调用。
java的封装,继承和多态(思维导图)
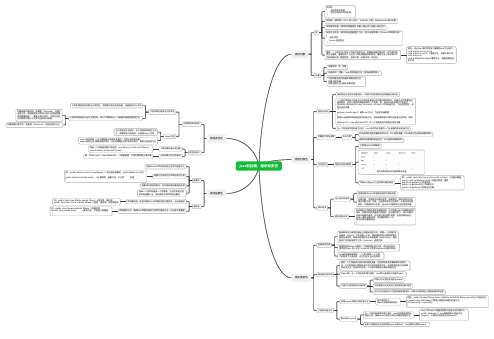
java的封装,继承和多态类和对象类类声明 { 成员变量的声明; 成员方法的声明及实现; }声明类:[修饰符] class 类<泛型> [extends 父类] [implements 接口列表]声明成员变量:[修饰符]数据类型 变量[=表达式]{,变量[=表达式]}成员方法声明:[修饰符]返回值类型 方法([形式参数列表])[throws 异常类列表]{语句序列;[return[返回值]]; }重载:一个类中可以有多个同名的成员方法,前提是参数列表不同,称为类的成员方法重载,重载的多个方法为一种功能提供多种实现。
重载方法之间必须以不同的参数列表(数据类型、参数个数、参数次序)来区别。
例如,MyDate 类可声明多个重载的set()方法如下: void set(int y,int m, int d)void set(int m, int d) //重载方法,参数个数不同void set(int d) void set(MyDate date)//重载方法,参数的数据类型不同对象对象声明:类 对象构造实例:对象 = new 类的构造方法([实际参数列表])引用对象的成员变量和调用成员方法:对象.成员变量 对象.成员方法([实际参数列表])类的封装性构造与析构类的构造方法用于创建类的一个实例并对实例的成员变量进行初始化一个类可声明多个构造方法对成员变量进行不同需求的初始化,构造方法不需要写返回值类型,因为它返回的就是该类的一个实例。
例:MyDate类声明以下构造方法:public MyDate(int year, int month, int day)// 声明构造方法,方法名同类名,初始化成员变量 {set(year, month day);// 调用 set()方法,为成员变量赋值}使用new运算符调用指定类的构造方法,实际参数列表必须符合构造方法声明。
例如:MyDate d1 = new MyDate(2017,10,1);//创建实例并初始化成员变量当一个类没有声明构造方法时,Java 自动为该类提供一个无参数的默认构造方法对象的引用与运算this引用访问本类的成员变量和成员方法:this.成员变量,this.成员方法([实际参数列表])调用本类重载的构造方法:this([实际参数列表])访问控制类的访问控制权限公有和(public)和缺省类中成员4级访问控制权限及范围声明set()和get()方法存取对象的属性例:public void set(int year, int month, int day) //设置日期值 public void set(MyDate date)//设置日期值,重载 public int getYear()//获得年份 public int getMonth()// 获得月份 public int getDay()//获得当月日期静态成员定义及访问格式使用关键字static声明的成员称为静态成员在类内部,可直接访问静态成员,省略类名。
生活实例解释面向对象的多态

生活实例解释面向对象的多态一、引言在现实生活中,多态是一个普遍存在的现象。
例如,动物世界中的狮子、老虎和豹,它们都属于猫科动物,但各自有着不同的特点。
同样,在人类社会中,人们从事着各种不同的职业,如医生、教师和工程师等。
这些生活实例都可以用来解释面向对象的多态。
二、多态的应用场景1.动物世界中的多态现象在动物世界中,多态现象无处不在。
以猫科动物为例,狮子、老虎和豹虽然都属于猫科,但它们在体型、毛色和习性等方面都有明显的差异。
这种多态性使得猫科动物能够在不同的生态环境中生存和繁衍。
2.人类职业的多态性在人类社会中,人们从事着各种不同的职业。
这些职业在职责、技能和待遇等方面都有所不同。
例如,医生、教师和工程师这三个职业,虽然都属于专业人士,但它们的职业特点和收入水平有很大差异。
这种多态性反映了社会分工的细化,使得人类社会能够更加繁荣发展。
三、面向对象编程中的多态1.基本概念与原理面向对象编程(OOP)是一种编程范式,它强调用对象来表示和处理问题。
多态是OOP的核心概念之一,它指的是同一操作在不同对象上具有不同的行为。
在面向对象编程中,多态主要有三种形式:继承、接口和抽象类。
2.多态的优势多态性使得程序具有更好的可扩展性和可维护性。
通过继承、接口和抽象类,我们可以轻松地为新对象添加新的行为,而无需修改原有代码。
这有助于降低代码的耦合度,提高代码的复用性。
四、多态的实现方法1.继承与多态继承是子类继承父类的属性和方法,从而实现多态。
例如,从动物类Animal继承出鸟类Bird和猫类Cat,使得Bird和Cat都具有Animal的属性和方法,但它们各自也有自己的特点。
2.接口与多态接口是一种抽象类型,它只定义了一些方法的签名,没有具体的实现。
实现类可以实现接口中的方法,从而实现多态。
例如,定义一个动物接口Animal,包含跑、跳和叫的方法。
猫类Cat和狗类Dog都可以实现这个接口,从而实现多态。
3.抽象类与多态抽象类是一种特殊的类,它不能被实例化,但可以被继承。
c类的继承和多态例子

c类的继承和多态例子继承是面向对象编程中的重要概念之一,它允许一个类“继承”另一个类的属性和方法。
在C++中,继承分为三种类型:公有继承、私有继承和保护继承。
其中,公有继承是最常用的一种方式,也是实现多态的基础。
本文将通过一个例子来介绍C++中的公有继承和多态特性。
假设我们要设计一个动物园的系统,其中包含不同类型的动物。
首先,我们定义一个基类Animal,代表所有动物的共有属性和方法。
然后,派生出几个具体的动物类,如Lion(狮子)、Elephant (大象)和Monkey(猴子),它们都是Animal类的派生类。
1. 基类Animal的定义:```c++class Animal {public:Animal() {} // 构造函数virtual ~Animal() {} // 虚析构函数virtual void move() const = 0; // 纯虚函数,用于表示不同动物的移动方式protected:int age; // 年龄double weight; // 体重};```2. 派生类Lion的定义:```c++class Lion : public Animal {public:Lion(int a, double w) : Animal(), color("yellow") { age = a;weight = w;}void move() const {std::cout << "Lion is running." << std::endl;}private:std::string color; // 颜色};```3. 派生类Elephant的定义:```c++class Elephant : public Animal {public:Elephant(int a, double w) : Animal(), height(3.5) { age = a;weight = w;}void move() const {std::cout << "Elephant is walking." << std::endl; }private:double height; // 身高};```4. 派生类Monkey的定义:```c++class Monkey : public Animal {public:Monkey(int a, double w) : Animal(), num_bananas(5) {age = a;weight = w;}void move() const {std::cout << "Monkey is jumping." << std::endl;}private:int num_bananas; // 香蕉数目};```以上就是实现动物园系统的基本类定义。
Java继承与多态实验报告.doc
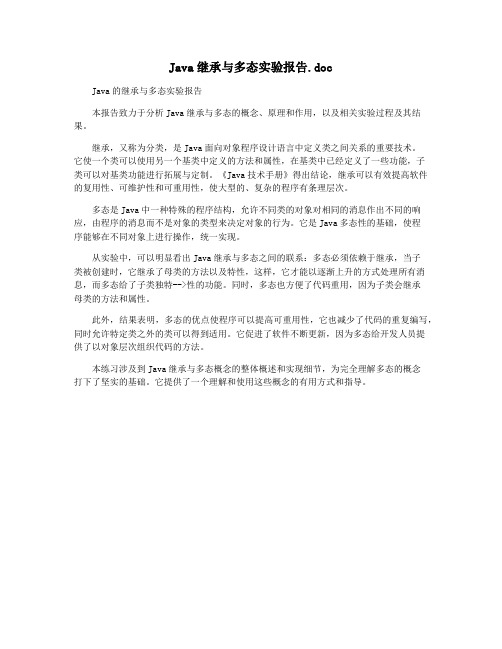
Java继承与多态实验报告.doc
Java的继承与多态实验报告
本报告致力于分析Java继承与多态的概念、原理和作用,以及相关实验过程及其结果。
继承,又称为分类,是Java面向对象程序设计语言中定义类之间关系的重要技术。
它使一个类可以使用另一个基类中定义的方法和属性,在基类中已经定义了一些功能,子
类可以对基类功能进行拓展与定制。
《Java技术手册》得出结论,继承可以有效提高软件的复用性、可维护性和可重用性,使大型的、复杂的程序有条理层次。
多态是Java中一种特殊的程序结构,允许不同类的对象对相同的消息作出不同的响应,由程序的消息而不是对象的类型来决定对象的行为。
它是Java多态性的基础,使程
序能够在不同对象上进行操作,统一实现。
从实验中,可以明显看出Java继承与多态之间的联系:多态必须依赖于继承,当子
类被创建时,它继承了母类的方法以及特性,这样,它才能以逐渐上升的方式处理所有消息,而多态给了子类独特-->性的功能。
同时,多态也方便了代码重用,因为子类会继承
母类的方法和属性。
此外,结果表明,多态的优点使程序可以提高可重用性,它也减少了代码的重复编写,同时允许特定类之外的类可以得到适用。
它促进了软件不断更新,因为多态给开发人员提
供了以对象层次组织代码的方法。
本练习涉及到Java继承与多态概念的整体概述和实现细节,为完全理解多态的概念
打下了坚实的基础。
它提供了一个理解和使用这些概念的有用方式和指导。
Java实验指导4继承与多态

《Java程序设计》实验指导实验四继承与多态一、实验目的:1.掌握类的继承方法。
2.掌握变量的继承和覆盖。
3.掌握方法的继承、重载和覆盖。
4.了解接口的实现方法。
二、实验原理新类可从现有的类中产生,并保留现有类的成员变量和方法,并可根据需要对它们加以修改。
新类还可添加新的变量和方法。
这种现象就称为类的继承。
当建立一个新类时,不必写出全部成员变量和成员方法。
只要简单地声明这个类是从一个已定义的类继承下来的,就可以引用被继承类的全部成员。
被继承的类称为父类或超类(superclass),这个新类称为子类。
Java 提供了一个庞大的类库让开发人员继承和使用。
设计这些类是出于公用的目的,因此,很少有某个类恰恰满足你的需要。
你必须设计自己的能处理实际问题的类,如果你设计的这个类仅仅实现了继承,则和父类毫无两样。
所以,通常要对子类进行扩展,即添加新的属性和方法。
这使得子类要比父类大,但更具特殊性,代表着一组更具体的对象。
继承的意义就在于此。
语法格式:类访问限定符子类名extends 父类名{……}三、实验内容及要求:1. 定义一个接口(ShapeArea),其中包含返回面积的方法(getArea)。
定义一个矩形类(Rectangle),派生出一个正方形类(Square),再定义一个圆类(Circle),三者都要求实现接口ShapeArea,自行扩充成员变量和方法。
在主方法中建一数组,数组中放入一些上述类型的对象,并计算它们的面积之和。
2. 运行下面的程序,理解成员变量的继承与隐藏,方法的覆盖与重载。
class A{int sum,num1,num2;public A(){num1=10;num2=20;sum=0;}void sum1(){sum=num1+num2;System.out.println("sum="+num1+"+"+num2+"="+sum);}void sum2(int n){num1=n;sum=num1+num2;System.out.println("sum="+num1+"+"+num2+"="+sum);}}class B extends A{int num2;public B(){num2=200;}void sum2(){sum=num1+num2;System.out.println("sum="+num1+"+"+num2+"="+sum);}void sum2(int n){num1=n;sum=num1+num2;System.out.println("sum="+num1+"+"+num2+"="+sum);}void sum3(int n){super.sum2(n);System.out.println("sum="+num1+"+"+num2+"="+sum);}}public class test{public static void main (String arg[]){B m=new B();m.sum1();m.sum2();m.sum2(50);m.sum3(50);}}。
资料:《继承和多态》上机实践

《继承和多态》上机实践一、根据课上讲解内容,完成演示示例和课堂练习1、使用重写优化电子宠物系统需求说明:按照方法的重写或方法的覆盖的注意事项:(1)子类根据需求对从父类继承的方法进行重新编写;(2)重写时,可以用super.方法的方式来保留父类的方法;(3)构造方法不能被重写;回顾之前使用继承实现的电子宠物系统,请使用方法的重写或覆盖优化现有代码,重写宠物类的宠物自白方法,在不同类型的宠物进行自白时,介绍其不同的特性,如:狗狗的自白需要介绍其品种、企鹅的自白需要介绍其性别。
实现如图所示的输出效果。
2、方法重写规则需求说明:熟悉方法重写的以下规则:(1)方法名相同;(2)参数列表相同;(3)返回值类型相同或者是其子类;(4)访问权限不能严于父类;(5)父类的静态方法不能被子类覆盖为非静态方法,父类的非静态方法不能被子类覆盖为静态方法;(6)子类可以定义与父类同名的静态方法,以便在子类中隐藏父类的静态方法;(7)父类的私有方法不能被子类覆盖;(8)不能抛出比父类方法更多的异常正确使用方法的重写或覆盖;回忆老师课上演示的测试代码,请自行编写测试代码验证以上规则。
3、重写equlas()比较两个学员需求说明:重写equals()方法,如果学员学号(id)、姓名(name)都相同,证明是同一个学生,即两名学员(Student对象)为同一对象,输出如图所示的比较结果。
(注意:instanceof用于判断一个引用类型所引用的对象是否是一个类的实例)4、使用多态为宠物看病需求说明:(1)编写主人类(Master),添加不同的方法,根据输入的宠物类型不同,为不同的宠物看病。
编写测试类验证;(2)使用多态修改代码,为各宠物类添加看病的方法,主人类中保留一个为宠物看病方法即可,参数类型为宠物类类型。
编写测试类验证输出效果如图所示。
(注意:同一个引用类型,使用不同的实例而执行不同操作就是多态;方法重写是实现多态的基础)5、将Pet修改为抽象类需求说明:按照抽象方法的定义:(1)抽象方法没有方法体;(2)抽象方法必须在抽象类里;(3)抽象方法必须在子类中被实现,除非子类是抽象类;在作业4的基础上,将Pet修改为抽象类,实现如果所示的效果。
生活实例解释面向对象的多态

面向对象的多态什么是多态在面向对象编程中,多态(Polymorphism)是一个重要的概念。
它指的是同一个方法可以根据不同的对象产生不同的行为。
换句话说,多态允许我们使用统一的接口来处理不同类型的对象。
多态是面向对象编程的三大特性之一,其余两个特性是封装和继承。
封装是指将数据和方法包装在一个对象中,继承是指一个类可以继承另一个类的属性和方法。
多态使得程序更加灵活,可扩展性更强。
生活中的多态示例为了更好地理解多态,我们可以通过生活中的一些例子来解释它。
1. 动物发声想象一下,你在一座动物园里散步。
你经过了一些动物的笼子,每个动物都在发出自己独特的声音。
狗在汪汪叫,猫在喵喵叫,鸟在唧唧鸣。
尽管它们发出的声音不同,但它们都是动物,都具有发声的能力。
在这个例子中,动物园可以被看作是一个类,而每个动物则是该类的实例。
类定义了动物的共同属性和方法,而每个实例则可以根据自己的特性来表现不同的行为。
这就是多态的体现。
2. 图形的面积计算假设你正在画一些图形,包括圆形、矩形和三角形。
你需要计算每个图形的面积。
不同的图形有不同的计算公式,但它们都有一个共同的方法——计算面积。
在这个例子中,每个图形可以被看作是一个类,而计算面积的方法则是这个类的一个公共方法。
每个图形类可以根据自己的特性实现不同的计算面积的方式。
当你调用计算面积的方法时,程序会根据具体的图形类型来执行相应的计算。
多态的实现方式在面向对象编程中,实现多态有两种常见的方式:继承和接口。
1. 继承实现多态继承是面向对象编程中的一个重要概念,它允许一个类继承另一个类的属性和方法。
通过继承,子类可以重写父类的方法,从而实现多态。
以动物为例,我们可以定义一个基类 Animal,它有一个方法叫做 makeSound()。
然后我们定义几个子类,如 Dog、Cat 和 Bird,它们分别重写了 makeSound() 方法来发出不同的声音。
class Animal {public void makeSound() {System.out.println("Animal makes sound");}}class Dog extends Animal {@Overridepublic void makeSound() {System.out.println("Dog barks");}}class Cat extends Animal {@Overridepublic void makeSound() {System.out.println("Cat meows");}}class Bird extends Animal {@Overridepublic void makeSound() {System.out.println("Bird chirps");}}public class Main {public static void main(String[] args) {Animal dog = new Dog();Animal cat = new Cat();Animal bird = new Bird();dog.makeSound(); // 输出:Dog barkscat.makeSound(); // 输出:Cat meowsbird.makeSound(); // 输出:Bird chirps}}在上面的例子中,我们定义了一个 Animal 类作为基类,然后定义了三个子类 Dog、Cat 和 Bird,它们都重写了 makeSound() 方法。
04--继承与多态 Java编程实战宝典教学课件
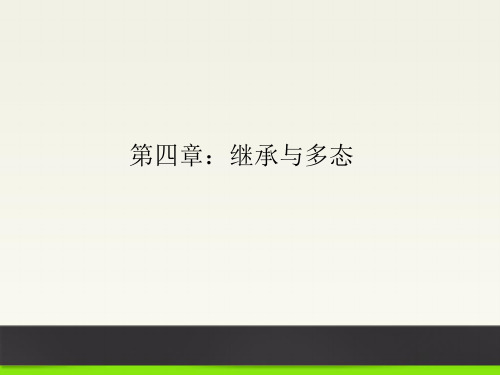
继承:一个类得到另一个类的全部或部分属性及方法的操作叫做继承
父类:具有该体系类通用的属性和方法
子类:从父类得到,同时也有自己的方法和属性
运输工具
汽车
轮船
飞机
空中加油机
客机
继承的特点
继承的特点
具有层次结构
子类继承了父类的 属性和方法
7
继承的优点
继承的优点
继承代避码免的了对可一重般用类和性特殊类之间共同特征进行的重可复描以述轻。松子同时地类,自通定过继义承
键字指明新定义类的父类,新定义的类称为指定父类的子类,这样就在两个类之间建
立了继承关系。这个新定义的子类可以从父类那里继承所有非private的属性和方法作 子类可以继承父类的所有非私有的数据成员。
为自己的成cla员ss。a1实{ 际上,在定义一个类而不给出extends关键字及父类名时,默认这 个类是系统ipn类rtivxoa=tb2ej5ei;nctt的z; 子//类不。能被子类继承的私有数据成员z
} class c5_6 extends a1 //a1是c5_6的父类,c5_6是a1的子类 {
public static void main(String[ ] argS) { c5_6 p=new c5_6( );
System.out.println("p.x="+p.x); //输出继承来的数据成员的值 //System.out.println(“p.z=”+p.z); //错,不能继承private修饰的z } }
在面向对象程序设计中运用继承原则,就是在每个由一般类和特殊类形成的一般—特殊结构中 ,把一般类的对象实例和所有特殊类的对象实例都共同具有的属性和操作一次性地在一般类中 进行显式的定义,在特殊类中不再重复地定义一般类中已经定义的东西,但是在语义上,特殊 类却自动地、隐含地拥有它的一般类(以及所有更上层的一般类)中定义的属性和操作。特殊类 的对象拥有其一般类的全部或部分属性与方法,称作特殊类对一般类的继承。
java 继承,多态 , 封装的灵活运用案例

java 继承,多态, 封装的灵活运用案例下面是一个简单的Java 程序,演示了继承、多态和封装的灵活运用案例:```java// 定义一个动物类public class Animal {private String name;public Animal(String name) { = name;}public void eat() {System.out.println(name + " is eating...");}}// 定义一个狗类,继承自Animal 类public class Dog extends Animal {public Dog(String name) {super(name);}public void bark() {System.out.println(name + " is barking...");}}// 定义一个猫类,继承自Animal 类public class Cat extends Animal {public Cat(String name) {super(name);}public void meow() {System.out.println(name + " is meowing...");}}// 定义一个主类,演示多态和封装的使用public class Main {public static void main(String[] args) {Animal animal = new Animal("Animal"); // 创建一个Animal 对象animal.eat(); // 调用Animal 类的eat() 方法Dog dog = new Dog("Dog"); // 创建一个Dog 对象,自动调用Animal 类的构造方法初始化name 属性dog.eat(); // 调用Dog 类的eat() 方法,实现多态性dog.bark(); // 调用Dog 类的bark() 方法,实现多态性Cat cat = new Cat("Cat"); // 创建一个Cat 对象,自动调用Animal 类的构造方法初始化name 属性cat.eat(); // 调用Cat 类的eat() 方法,实现多态性cat.meow(); // 调用Cat 类的meow() 方法,实现多态性}}```在上面的程序中,我们定义了一个Animal 类作为基类,然后分别定义了Dog 和Cat 类作为子类。
继承与多态实验报告

继承与多态实验报告继承与多态实验报告在面向对象编程中,继承和多态是两个重要的概念。
通过继承,我们可以创建新的类,并从现有的类中继承属性和方法。
而多态则允许我们使用父类的引用来指向子类的对象,实现同一操作具有不同的行为。
本实验旨在通过实际的编程实践,加深对继承和多态的理解。
实验一:继承在这个实验中,我们创建了一个动物类(Animal),并从它派生出了两个子类:狗类(Dog)和猫类(Cat)。
动物类具有一些共同的属性和方法,如名字(name)和发出声音(makeSound)。
子类继承了父类的属性和方法,并可以添加自己的特定属性和方法。
在编写代码时,我们首先定义了动物类,并在其中实现了共同的属性和方法。
然后,我们创建了狗类和猫类,并分别在这两个类中添加了自己的特定属性和方法。
通过继承,我们可以在子类中直接使用父类的方法,并且可以根据需要进行重写。
实验二:多态在这个实验中,我们使用多态的概念来实现一个动物园的场景。
我们定义了一个动物园类(Zoo),并在其中创建了一个动物数组。
这个数组可以存储不同类型的动物对象,包括狗、猫等。
通过多态,我们可以使用动物类的引用来指向不同类型的动物对象。
例如,我们可以使用动物类的引用来指向狗对象,并调用狗类特有的方法。
这样,我们可以统一处理不同类型的动物对象,而不需要为每种类型编写特定的处理代码。
实验三:继承与多态的结合应用在这个实验中,我们进一步探索了继承和多态的结合应用。
我们定义了一个图形类(Shape),并从它派生出了三个子类:圆形类(Circle)、矩形类(Rectangle)和三角形类(Triangle)。
每个子类都实现了自己的特定属性和方法。
通过继承和多态,我们可以在图形类中定义一些通用的方法,如计算面积和周长。
然后,我们可以使用图形类的引用来指向不同类型的图形对象,并调用相应的方法。
这样,我们可以轻松地对不同类型的图形进行统一的处理。
结论:通过本次实验,我们进一步理解了继承和多态的概念,并学会了如何在编程中应用它们。
封装继承多肽的应用场景_概述及解释说明

封装继承多肽的应用场景概述及解释说明1. 引言1.1 概述在软件开发中,封装、继承和多态是面向对象编程(Object-Oriented Programming)的三个重要概念。
它们是用于实现代码的重用和灵活性增强的关键技术手段。
封装(Encapsulation)是将数据和方法组合成一个单一的实体,通过将数据隐藏在类中,只提供有限的访问方式来保护数据的完整性。
继承(Inheritance)允许我们定义新类,并从已存在的类中派生出特定属性和行为。
多态(Polymorphism)则允许不同类型之间互相交互,并以一种统一的方式处理它们。
本文将对封装、继承和多态这三个概念进行详细说明,并探讨它们在软件开发中的应用场景。
1.2 文章结构本文分为五个部分进行论述。
首先是引言部分,对文章主题进行概述;然后依次介绍封装、继承和多态的基本概念、优点以及实际应用场景;最后进行总结和结论。
1.3 目的本文旨在帮助读者更好地理解封装、继承和多态的概念,并认识到它们在软件开发中的重要性。
通过详细介绍它们在实际应用场景中的使用方式,读者将能够更加灵活地运用这些概念来设计高效、可扩展和易于维护的软件系统。
2. 封装的应用场景:2.1 封装的基本概念:封装是面向对象编程中的一个重要概念,指将数据和方法绑定在一起,以形成一个完整的单元。
通过封装,我们可以隐藏内部实现细节,只向外部提供必要的接口。
封装有助于保护数据的安全性和完整性。
2.2 封装的优点:封装具有以下优点:(a) 数据隐藏:封装可以将数据隐藏在类的内部,而只暴露必要的接口给外部使用。
这样可以保证数据不会被误操作或非法访问所破坏。
(b) 模块化编程:封装使得代码可以按模块进行组织和管理,提高代码可读性和可维护性。
(c) 降低耦合度:通过对外部隐藏实现细节,封装减少了代码间的依赖关系,降低了耦合度。
(d) 接口统一化:封装可以通过定义公共接口来统一访问方式,在修改实现时不影响外部调用者。
数据库设计中的多态关系模型与实例分析

数据库设计中的多态关系模型与实例分析多态关系模型是数据库设计中的重要概念之一。
它描述了一个实体类(父类)与其子类之间的关系,在不同的角色和属性之间建立联系。
在实际应用中,多态关系模型为数据库设计带来了很多便利和灵活性。
本文将介绍多态关系模型的基本概念和原理,并通过实例分析来说明其在数据库设计中的应用。
一、多态关系模型的基本概念多态关系模型是指在数据库设计中,一个父类与多个子类之间建立的关联关系。
父类包含了多个子类共有的属性和操作,而子类则拥有各自特有的属性和操作。
通过多态关系模型,可以将不同类型的实体对象存储在同一个关系表中,提高了数据库的灵活性和扩展性。
多态关系模型的主要特点包括:1. 继承关系:多态关系模型通过继承实现父类与子类之间的关联。
子类继承了父类的属性和操作,并可以扩展自己的特有属性和操作。
2. 接口描述:父类包含子类的共有属性和操作,通过定义接口描述这些共有的特征,子类可以根据需要选择性地进行实现。
3. 多态性:数据库查询操作可以根据实例的具体类型自动选择执行合适的操作。
这样,同一类型的多个实例可以使用统一的接口进行管理和操作,提高了代码的重用性和可维护性。
二、多态关系模型的实例分析为了更好地理解多态关系模型在数据库设计中的应用,我们将通过一个实例来进行分析。
假设我们要设计一个餐厅管理系统,其中包含了不同类型的员工和顾客。
我们可以使用多态关系模型来描述员工和顾客之间的关系。
1. 数据库表设计我们首先设计两个表,分别是员工表(Employee)和顾客表(Customer)。
员工表包含了所有员工的共有属性,如编号、姓名、年龄等;顾客表则包含了顾客的共有属性,如编号、姓名、电话等。
这两个表可以作为父类。
2. 员工子类设计在员工表的基础上,可以根据具体角色设计不同的子类,如厨师、服务员等。
这些子类可以继承员工表的属性,并扩展自己特有的属性,如技能、职称等。
3. 顾客子类设计在顾客表的基础上,可以设计不同类型的顾客子类,如会员、非会员等。
1. 应用继承和多态的思想,编写动物类,成员方法是动物叫声(cry())。写三个具体的类

1.应用继承和多态的思想,编写动物类,成员方法是动物叫声(cry())。
写三个具体的类1、应用继承和多态的思想,编写动物类,成员方法是动物叫声。
写三个具体的类(猫、狗、羊),它们都是动物类的子类,并重写父类的成员方法。
编写测试类,随机产生三种具体动物,调用叫声这个方法。
程序参考运行效果如图所示:任务分析:1、定义一个父类Animal类属性:kind(种类)方法:创建带参(kind为参数)构造方法创建cry():void方法2. 编写三个具体的子类Cat类、Dog类、Sheep类分别重写父类中的cry() 方法,输出信息分别为Cat类:小猫的叫声:喵喵喵~~~Dog类:小狗的叫声:汪汪汪~~~Sheep类:小羊的叫声:咩咩咩~~~3. 编写测试类,首先生成长度为5的父类对象数组,然后通过循环依次向数组中存入数据,现设定存储规则为:a) 每次随机产生一个0~2的正整数b) 若数值为0,则生成一个Cat 类的对象,存入数组c) 若数值为1,则生成一个Dog 类的对象,存入数组d) 若数值为2,则生成一个Sheep 类的对象,存入数组最后循环输出数组成员,并分别调用cry() 方法。
package com.dodoke.animal.fatherclass;/*** 定义一个父类Animal类* @author**/public class Animal {//属性:kind(种类)private String kind;//方法:创建带参(kind为参数)构造方法public Animal(String kind) {this.setKind(kind);}public Animal(){}//构建get/set方法public String getKind() {return kind;}public void setKind(String kind) {this.kind = kind;}// 创建cry():void方法public void cry(){System.out.println("动物叫声~~~");}}123457 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 2729303132package com.dodoke.animal.sonclass;import com.dodoke.animal.fatherclass.Animal;/*** 子类Cat类* @author**/public class Cat extends Animal {//重写父类中的cry() 方法public void cry(){System.out.println("小猫的叫声:喵喵喵~~~");}}12456789101112131415package com.dodoke.animal.sonclass;import com.dodoke.animal.fatherclass.Animal; /*** Dog类* @author**/public class Dog extends Animal {//重写父类中的cry() 方法public void cry(){System.out.println("小狗的叫声为:汪汪汪~~~");}}123456789101112131415package com.dodoke.animal.sonclass;import com.dodoke.animal.fatherclass.Animal;/*** Sheep类* @author**/public class Sheep extends Animal {//重写父类中的cry() 方法public void cry(){System.out.println("小羊的叫声:咩咩咩~~~");}}12345678101112131415package com.dodoke.animal.test;import com.dodoke.animal.fatherclass.Animal; import com.dodoke.animal.sonclass.Cat; import com.dodoke.animal.sonclass.Dog; import com.dodoke.animal.sonclass.Sheep;/*** 测试类* @author**/public class Test {public static void main(String[] args) {//首先生成长度为5的父类对象数组Animal ani[] = new Animal[5];//然后通过循环依次向数组中存入数据for(int i = 0; i < 5; i++) {int number = (int)(Math.random()*3);//每次随机产生一个0~2的正整数if(number == 0) {//若数值为0,则生成一个Cat 类的对象,存入数组ani[i] = new Cat();}else if(number == 1) {//若数值为1,则生成一个Dog 类的对象,存入数组ani[i] = new Dog();}else if(number == 2) {//若数值为2,则生成一个Sheep 类的对象,存入数组ani[i] = new Sheep();}}//最后循环输出数组成员,并分别调用cry() 方法for(int i = 0; i < 5; i++) {ani[i].cry();}}}。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
在银行业务中,有储蓄(Saving)账户和结算(Checking)账户之分,两个类的设计:
结算账户
继承的使用
//类的定义省略 #include<iostream.h> Saving::Saving(int accNo,float bal=0){ accNumber=accNo; balance=bal; } void Saving::Withdraw(float bal){ if(bal<balance && bal>0) balance-=bal; } void Saving::Deposit(float bal){ if(bal>0) balance+=bal; } void Saving::Display(){ cout<<“Saving Account[”<<accNo <<“]:”<<balance<<endl; } Checking::Checking(int accNo, float bal=0){ accNumber=accNo; balance=bal; }
base1::member=0; base2::member=0;
多重继承的内存布局
虚拟基类之前的内存布局
class base{ int b2 int b1 protected: int b; }; base1的内存布局 base2的内存布局 class base1: public base{ protected: int b1; int b 二义性 }; class base2: public base{ int b1 protected: int b2; int b }; class derived: public base1, public base2{ int b2 float d; float d public: int fun(); }; derived的内存布局 不能把derived对象当base对象使用 int b int b
继承的使用
从现实中捕获一般-特殊关系
class Automobile{ public: Automobile(); void start(); void stop(); void speedup(); void speeddown(); protected: char* brand; float price; float speed; }; class Car: public Automobile{ public: Car(); void absstop(); private: float gas_comsume; }; class Jeep: public Automobile{ public: Jeep(); void climb(); private: int drive; }; class Truck: public Automobile{ public: Truck(); void transport(); private: float load; }; Automobile
赋值相同规则
通过公有继承,派生类指针可以赋给基类指针
class Point2d{ Point3d obj3d; Point2d obj2d; public: void show(); p3d p2d protected: float x float x p2d float x,y; float y float y }; class Point3d: public Point2d{ float z public: void show(); private: float z; //指针赋值 }; Point2d *p2d=&obj2d; void main(){ Point3d *p3d=&obj3d; Point2d obj2d; p2d=p3d; Point3d obj3d; p2d->show(); 调用Point2d::show() }
Point3d 子对象 Vertex子对象
ቤተ መጻሕፍቲ ባይዱ
Vertex3d obj3; //指针赋值 Point3d *p3d=&obj3; Vertex *pv=&obj3; //涉及指针偏移
赋值相容规则:
//对象赋值 obj1=obj3; obj2=obj3;
多重继承的内存布局
多重继承中数据成员的二义性
class base1{ protected: int member; public: base1(){ //… } }; class base2{ protected: int member; public: base2(){ //… } }; class derived: public base1, public base2{ private: int total; public: derived(){ //… } void func(){ member=0; //二义性 } }; int member 来自base1 int member 来自base2 int total derived对象布局
多重继承的内存布局
虚拟基类的内存布局
通过指针指向共享的虚拟基类 int b1 int b2 class base{ base* p base* p protected: int b; }; int b int b class base1: virtual public base{ base1的内存布局 base2的内存布局 protected: int b1; }; int b1 class base2: virtual public base{ protected: int b2; base* p }; int b2 class derived: public base1, public base2{ float d; base* p public: int fun(); derived的内存布局 float d }; 可以把derived对象当base对象使用 int b
赋值相同规则
通过公有继承,派生类的对象可以当作基类使用
class Point2d{ public: void show(); Point3d obj3d; Point2d obj2d; protected: float x,y; float x float x }; float y float y class Point3d: public Point2d{ public: void show(); float z private: float z; }; void main(){ 派生类对象赋值给基类对象 Point2d obj2d; Point3d obj3d; 向上映射、对象切片 obj2d=obj3d; //对象赋值 obj2d.show(); //调用Point2d::show() }
Car
Jeep
Truck
继承的使用
从相似的类中提取共同的特征,形成类层次
Saving accNumber balance Saving() Withdraw() Deposit() Display() 储蓄账户 Checking accNumber balance remittance Checking() Withdraw() Deposit() Display() SetRemit() Consume()
多重继承的内存布局
class Point2d{ public: //… Point2d Vertex protected: float x,y; }; class Point3d: public Point2d{ Point3d public: //… protected: float z; }; Vertex3d class Vertex{ public: //… protected: Vertex* next; }; class Vertex3d: public Point3d, public Vertex{ public: //… protected: float mumble; };
继承的使用
Checking类继承Saving类
Saving accNumber balance Saving() Withdraw() Deposit() Display() 储蓄账户 Checking remittance Checking() Withdraw() SetRemit() Consume() 结算账户
通过多重继承,派生类中包含各基类成员
多重继承的内存布局
Point2d 子对象 float x float y float z Point3d obj1; Vertex* next Vertex obj2; Point2d 子对象 float x float y float z
Vertex* next float mumble
继承的使用
class Saving{ protected: int accNumber; float balance; public: Saving(int accNo,float bal=0); void Withdraw(float bal); void Deposit(float bal); void Display(); }; class Checking: public Saving{ private: int remittance; public: Checking(int accNo,float bal=0); void Withdraw(float bal); void setRemit(int rem); void Consume(float price, float add); }; #include<iostream.h> //Saving的实现… Checking::Checking(int accNo, float bal=0): Saving(accNo,bal){} void Checking::Withdraw(float bal){ float tmp;//根据remit计算 if(tmp<balance&&tmp>0) balance-=tmp; } void Checking::setRemit(int rem){ remittance=remit; } Void Checking::Consume(float price, float add=0){ float tmp=price+add; if(tmp<balance && tmp>0) balance-=tmp; }