用verilog语言编写交通灯程序
verilog课程设计交通灯
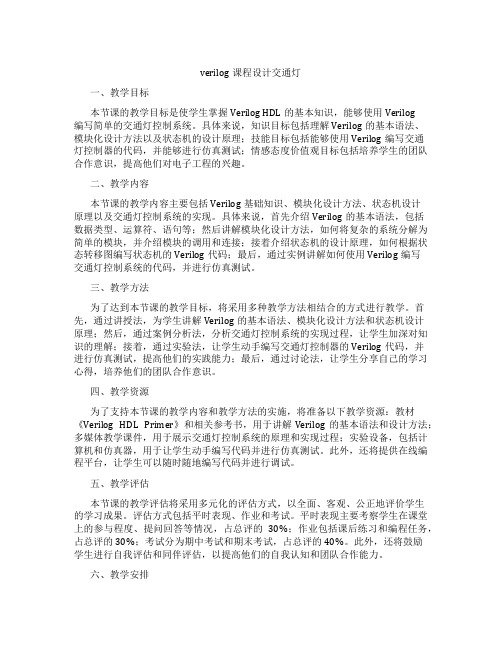
verilog课程设计交通灯一、教学目标本节课的教学目标是使学生掌握Verilog HDL的基本知识,能够使用Verilog编写简单的交通灯控制系统。
具体来说,知识目标包括理解Verilog的基本语法、模块化设计方法以及状态机的设计原理;技能目标包括能够使用Verilog编写交通灯控制器的代码,并能够进行仿真测试;情感态度价值观目标包括培养学生的团队合作意识,提高他们对电子工程的兴趣。
二、教学内容本节课的教学内容主要包括Verilog基础知识、模块化设计方法、状态机设计原理以及交通灯控制系统的实现。
具体来说,首先介绍Verilog的基本语法,包括数据类型、运算符、语句等;然后讲解模块化设计方法,如何将复杂的系统分解为简单的模块,并介绍模块的调用和连接;接着介绍状态机的设计原理,如何根据状态转移图编写状态机的Verilog代码;最后,通过实例讲解如何使用Verilog编写交通灯控制系统的代码,并进行仿真测试。
三、教学方法为了达到本节课的教学目标,将采用多种教学方法相结合的方式进行教学。
首先,通过讲授法,为学生讲解Verilog的基本语法、模块化设计方法和状态机设计原理;然后,通过案例分析法,分析交通灯控制系统的实现过程,让学生加深对知识的理解;接着,通过实验法,让学生动手编写交通灯控制器的Verilog代码,并进行仿真测试,提高他们的实践能力;最后,通过讨论法,让学生分享自己的学习心得,培养他们的团队合作意识。
四、教学资源为了支持本节课的教学内容和教学方法的实施,将准备以下教学资源:教材《Verilog HDL Primer》和相关参考书,用于讲解Verilog的基本语法和设计方法;多媒体教学课件,用于展示交通灯控制系统的原理和实现过程;实验设备,包括计算机和仿真器,用于让学生动手编写代码并进行仿真测试。
此外,还将提供在线编程平台,让学生可以随时随地编写代码并进行调试。
五、教学评估本节课的教学评估将采用多元化的评估方式,以全面、客观、公正地评价学生的学习成果。
《FPGA设计与应用》交通灯实验一
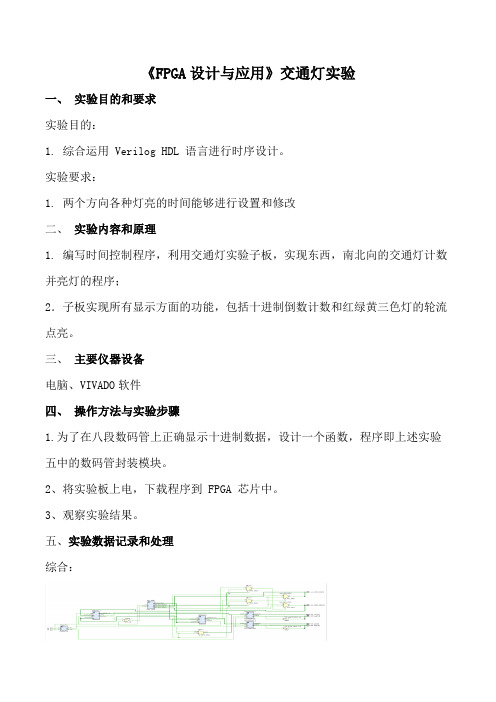
《FPGA设计与应用》交通灯实验
一、实验目的和要求
实验目的:
1. 综合运用 Verilog HDL 语言进行时序设计。
实验要求:
1. 两个方向各种灯亮的时间能够进行设置和修改
二、实验内容和原理
1. 编写时间控制程序,利用交通灯实验子板,实现东西,南北向的交通灯计数并亮灯的程序;
2.子板实现所有显示方面的功能,包括十进制倒数计数和红绿黄三色灯的轮流点亮。
三、主要仪器设备
电脑、VIVADO软件
四、操作方法与实验步骤
1.为了在八段数码管上正确显示十进制数据,设计一个函数,程序即上述实验五中的数码管封装模块。
2、将实验板上电,下载程序到 FPGA 芯片中。
3、观察实验结果。
五、实验数据记录和处理
综合:
程序:
六、实验结果与分析
实验仿真结果:
实物操作:
七、讨论和心得
通过这次实验,我加深了用Verilog语言来进行时序设计方法的理解,能够编写简单的时间控制程序,让我verilog语法的运用更加熟练,在实验中还用到了之前学到的模块调用,加深了我对之前知识的理解。
通过本次实验,我不仅学到了关于Verilog的知识,同时也让我感觉到了思考的重要性。
交通灯控制程序
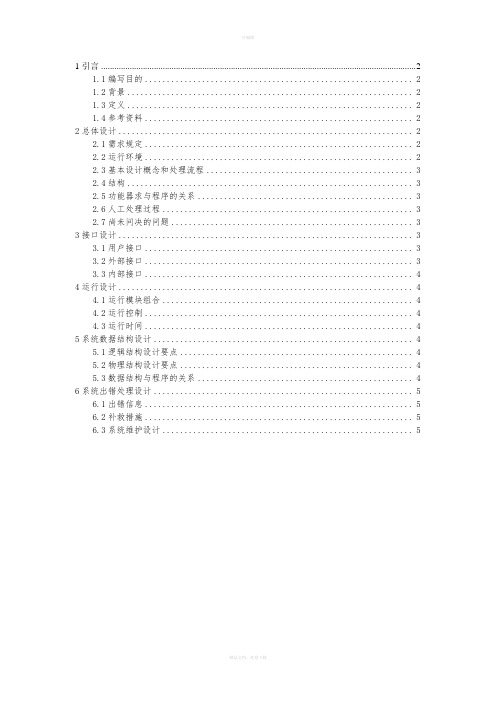
1引言 (2)1.1编写目的 (2)1.2背景 (2)1.3定义 (2)1.4参考资料 (2)2总体设计 (2)2.1需求规定 (2)2.2运行环境 (2)2.3基本设计概念和处理流程 (3)2.4结构 (3)2.5功能器求与程序的关系 (3)2.6人工处理过程 (3)2.7尚未问决的问题 (3)3接口设计 (3)3.1用户接口 (3)3.2外部接口 (3)3.3内部接口 (4)4运行设计 (4)4.1运行模块组合 (4)4.2运行控制 (4)4.3运行时间 (4)5系统数据结构设计 (4)5.1逻辑结构设计要点 (4)5.2物理结构设计要点 (4)5.3数据结构与程序的关系 (4)6系统出错处理设计 (5)6.1出错信息 (5)6.2补救措施 (5)6.3系统维护设计 (5)概要设计说明书1引言1.1编写目的创建一个可供日常交通灯使用的程序,目标读者为交通灯控制人员。
1.2背景说明:a.交通路口复杂交通信号灯的设计b.任务提出者,开发者:陈磊用户:交通指挥系统设计环境:使用Verilog HDL语言进行设计使用Quartus 2编程环境进行开发。
1.3定义ORDER 选择信号ROAD 亮灯控制信号EN5 5秒延时使能信号LIN5 5秒延时终止信号EN25 25秒延时使能信号LIN25 25秒延时终止信号RST 系统复位信号CLK 系统时钟信号1.4参考资料Verilog HDL数字系统设计与应用2总体设计2.1需求规定输入项目:CLK 系统时钟信号RST 系统复位信号输出项目:ROAD 亮灯控制信号,ROAD=00,红灯亮;ROAD=01,黄灯亮;ROAD=10,绿灯亮;ROAD=11,黄灯亮。
处理要求:根据ORDER的不同值,使相应的灯发亮。
2.2运行环境运行环境:。
Quartus II 9.02.3基本设计概念和处理流程2.4结构ORDER 选择某一灯的选择变量ROAD 控制某一灯亮的控制变量2.5功能器求与程序的关系2.6人工处理过程人工输入ORDER的值,根据ORDER的不同值,相应的灯变亮2.7尚未问决的问题创建一个稳定的时钟源3接口设计3.1用户接口light(red,amber,green,0,order)ORDER为控制变量,由它决定让哪一盏灯发亮。
四种亮灯方式自由切换的跑马灯(用veriloghdl语言编写的跑马灯程序)
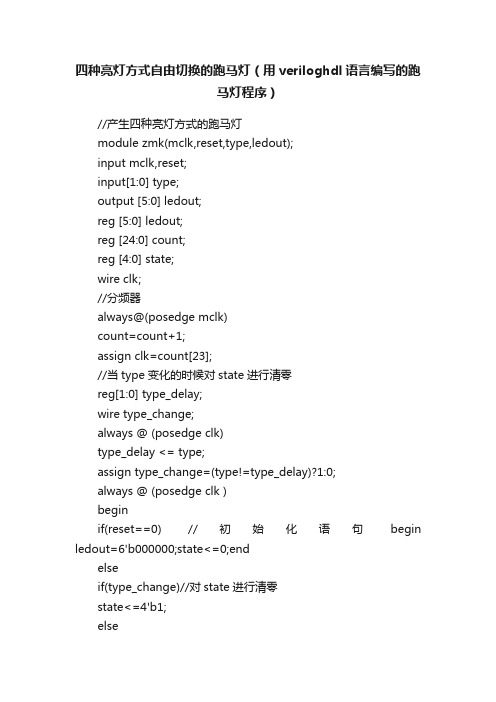
四种亮灯方式自由切换的跑马灯(用veriloghdl语言编写的跑马灯程序)//产生四种亮灯方式的跑马灯module zmk(mclk,reset,type,ledout);input mclk,reset;input[1:0] type;output [5:0] ledout;reg [5:0] ledout;reg [24:0] count;reg [4:0] state;wire clk;//分频器always@(posedge mclk)count=count+1;assign clk=count[23];//当type变化的时候对state进行清零reg[1:0] type_delay;wire type_change;always @ (posedge clk)type_delay <= type;assign type_change=(type!=type_delay)?1:0;always @ (posedge clk )beginif(reset==0) //初始化语句begin ledout=6'b000000;state<=0;endelseif(type_change)//对state进行清零state<=4'b1;elsebegincase(state)4'b0001:ledout=6'b011111;//第一个灯亮4'b0010:ledout=6'b101111;//第二个灯亮4'b0011:ledout=6'b110111;//第三个灯亮4'b0100:ledout=6'b111011;//第四个灯亮4'b0101:ledout=6'b111101;//第五个灯亮4'b0110:ledout=6'b111110;//第六个灯亮4'b0111:ledout=6'b111101;//第五个灯亮4'b1000:ledout=6'b111011;//第四个灯亮4'b1001:ledout=6'b110111;//第三个灯亮4'b1010:ledout=6'b101111;//第二个灯亮default :ledout=6'b000000;endcasestate=state+1; //计数器产生state的各种状态if(state==4'b1011)state=5'b00001;endelseif(type==2'b01)begincase(state)4'b0001:ledout=6'b101010;4'b0010:ledout=6'b010101;default :ledout=6'b000000;endcasestate=state+1;if(state==4'b0011)state=5'b00001;endelsebegincase(state)4'b0001:ledout=6'b110011;4'b0010:ledout=6'b101101;4'b0011:ledout=6'b011110;4'b0100:ledout=6'b101101; default :ledout=6'b000000; endcasestate=state+1;if(state==4'b0101)state=5'b00001; endelseif(type==2'b11)begincase(state)5'b00001:ledout=6'b111110;5'b00010:ledout=6'b111101;5'b00011:ledout=6'b111011;5'b00100:ledout=6'b110111;5'b00101:ledout=6'b101111;5'b00110:ledout=6'b011111;5'b00111:ledout=6'b001111;5'b01000:ledout=6'b010111;5'b01001:ledout=6'b011011;5'b01010:ledout=6'b011101;5'b01011:ledout=6'b011110;5'b01100:ledout=6'b011100;5'b01101:ledout=6'b011010;5'b01110:ledout=6'b010110;5'b01111:ledout=6'b001110;5'b10000:ledout=6'b000110;5'b10001:ledout=6'b001010;5'b10010:ledout=6'b001100;5'b10011:ledout=6'b001000;5'b10100:ledout=6'b000100;5'b10101:ledout=6'b001000;5'b10110:ledout=6'b000000;5'b10111:ledout=6'b111111;5'b11000:ledout=6'b000000;5'b11001:ledout=6'b111111;5'b11010:ledout=6'b000000;default :ledout=6'b000000;endcasestate=state+1;if(state==5'b11011)state=5'b00001; end endendmodule。
实验五交通灯控制

操作规则实现电路
功能:根据交通红绿灯控制器的功能要求,确定不同工作状态下计时器的计数值。可用8位计数器来实现定时计数。
正常运行时,计数器按照规定的定时要求加1计数;若要人工放行某方向,只要使计时器运行到该放行状态的最后一刻时,计时器保持此时的计数值,使红绿灯信号生成器暂停状态的转移即可。
*
再按下键2,表示欲人工放行B方向,则相应LED有显示;同时两个方向的红绿灯按正常运行规律自动切换,当运行到放行B方向时,则保持放行该方向。
再按下键3,表示清除人工方向的控制,则交通灯开始自动转换红黄绿灯的状态。
3
2
1
4
*
1
2
3
4
5
6
预习时请画出其状态转移图。
*
设计方案的选择
01
图文混合设计方法:先将电路划分为几个子模块,每个子模块由Verilog HDL语言描述实现,然后生成逻辑符号,顶层文件采用图形文件来实现。
02
纯文本描述方法:每个子模块和顶层电路的连接关系都采用Verilog HDL语言描述实现,对子模块的调用采用模块元件例化的方法。
起始状态的选择
采用log2N个触发器来表示这N个状态 采用N个触发器来表示这N个状态——称为一位热码状态机编码(One-Hot State Machine Encoding)。
状态编码
采用Verilog HDL语言实现基于状态机的设计,就是在时钟信号的触发下,完成两项任务: 用case或if-else语句描述出状态的转移; 描述状态机的输出信号。
在线校验
下载后,仔细观察:红绿灯应按设定的时间规律自动切换,D1~D8八个LED分别对应的是:A方向的红黄绿,B方向的红黄绿,A方向的放行状态,B方向的放行状态。
verilog实验报告流水灯数码管秒表交通灯

流水灯实验目的:在basys2开发板上实现LED灯的花样流水的显示,如隔位显示,依次向左移位显示,依次向右移位显示,两边同时靠中间显示。
实验仪器:FPGA开发板一块,计算机一台。
实验原理:当一个正向的电流通过LED时,LED就会发光。
当阳极的电压高于阴极的电压时,LED就会有电流通过。
当在LED上增添一个典型值为1.5V—2.0V之间的电压时,LED就会有电流通过并发光。
实验内容:顶层模块:输入信号:clk_50MHz(主时钟信号),rst(重置信号),输出信号:[7:0] led(LED灯控制信号)。
module led_top(clkin,rst,led_out);input clkin, rst;output [7:0] led_out;wire clk_1hz;divider_1hz d0(clkin, rst, clk_1hz);led l0(clk_1hz, rst, led_out);endmodule分频模块:module divider_1hz(clkin,rst,clkout);input clkin,rst;output reg clkout;reg [24:0] cnt;always@(posedge clkin, posedge rst)beginif(rst) begincnt<=0;clkout<=0; endelse if(cnt==24999999) begincnt<=0;clkout=!clkout; endelse cnt<=cnt+1;endendmodule亮灯信号模块:module led(clkin,rst,led_out);input clkin,rst;output [7:0] led_out;reg [2:0] state;always@(posedge clkin, posedge rst)if(rst) state<=0;else state<=state+1;always@(state)case(state)3'b000:ledout<=8'b0000_0001;3'b001:ledout<=8'b0000_0010;3'b010:ledout<=8'b0000_0100;3'b011:ledout<=8'b0000_1000;3'b100:ledout<=8'b0001_0000;3'b101:ledout<=8'b0010_0000;3'b110:ledout<=8'b0100_0000;3'b111:ledout<=8'b1000_0000;endcaseendmodule实验中存在的问题:1 芯片选择问题automotive spartan3EXA3S100E XA3S250E CPG132spartan3EXC3S100E XC3S250E CP1322 时序逻辑部分,阻塞赋值和非阻塞赋值混用always@(posedge clk)begina=b+c;d<=e+f;end3 UCF文件格式错误NET “CLK” LOC = “B8”;NET “a” LOC = “N11”;NET “b” LOC = “G13”;NET “c[0]”LOC =“K11;数码管实验目的:设计一个数码管动态扫描程序,实现在四位数码管上动态循环显示“1”、“2”“3”“4”;实验仪器:FPGA开发板一块,计算机一台。
EDA实验报告:基于VHDL语言的交通灯控制系统设计与实现

图为k1=0时的输出状态s1,输出恒为011110。即亮灯为R1,y2。保持时间1S。
(k2=0)
上图为k2=0时的输出状态s2,输出恒为101011。即亮灯为G1,R2。保持时间2S。
(k3=0)
上图为k3=0时的输出状态s3,输出恒为110011。即亮灯为R1,G2。保持时间1S。
四、小结及心得体会
else
if counter<5 then
next_state<=s3;
else
next_state<=s0;
end if;
end if;
end if;
end if;
end if;
end case;
end process;
ouput:process(current_state)
begin ——显示程序
begin
u1: jiaotongdeng port map(
clk=>clki,
k0=>k0,
k1=>k1,
k2=>k2,
k3=>k3,
r1=>r1,r2=>r2,g1=>g1,g2=>g2,y1=>y1,y2=>y2
);
u2: div port map(clk=>clk1,clk_out=>clki);
end if;
end if;
end if;
when s3=>
if k0='0' then
next_state<=s0;
else
if k1='0' then
next_state<=s1;
交通灯控制器数电课程设计

交通灯控制器数电课程设计交通灯控制器是一个常见的数电课程设计项目,下面是一个简单的交通灯控制器的设计方案:1. 需求分析:- 交通灯要能够按照规定的时间间隔不断切换状态。
- 交通灯的状态包括红灯、黄灯和绿灯,分别对应停止、警告和通行状态。
- 红灯、黄灯和绿灯的时间间隔可以根据实际需要进行调整。
2. 设计方案:- 使用数字时钟芯片,如NE555,来生成固定频率的时钟信号。
- 使用多路选择器,如74LS151,来选择不同的灯的状态输出。
- 使用逻辑门电路,如与门和或门,来实现灯的状态切换。
3. 设计步骤:- 使用时钟芯片来产生一个频率为1Hz的时钟信号。
- 使用分频器电路,如74LS90,将时钟信号的频率分为三等份,分别用于控制红灯、黄灯和绿灯的持续时间。
- 使用多路选择器74LS151,根据时钟信号的状态与分频器的控制信号,选择对应的灯输出高电平或低电平。
- 使用逻辑门电路,通过组合逻辑将时钟信号和选择器输出的灯状态进行控制,实现交通灯的状态切换。
4. 硬件设计:- 使用电路实验板、面包板或PCB板等硬件平台进行电路连接。
- 导入时钟芯片、分频器、多路选择器和逻辑门等器件。
- 连接器件之间的引脚,构建交通灯控制器电路。
5. 软件设计:- 使用VHDL、Verilog或其他HDL语言进行交通灯控制器的逻辑设计和仿真。
- 根据交通灯的时序要求设置时钟频率、分频器的初始状态和选择器的状态等参数。
- 通过仿真软件进行功能验证和时序分析,优化电路设计。
6. 实现与调试:- 将硬件连接完成后,使用示波器、逻辑分析仪等仪器对电路进行调试。
- 观察交通灯的状态是否按照预期进行切换。
- 根据实际需要调整各个灯的持续时间和时钟频率等参数,进行效果调试。
7. 总结:- 对交通灯控制器的设计进行总结和评估,包括可靠性、灵活性和可扩展性等方面。
- 提出改进方案,进一步优化交通灯控制器的设计。
注意事项:- 在设计过程中,要遵守相关的电路布线规范和安全操作规程。
实训报告
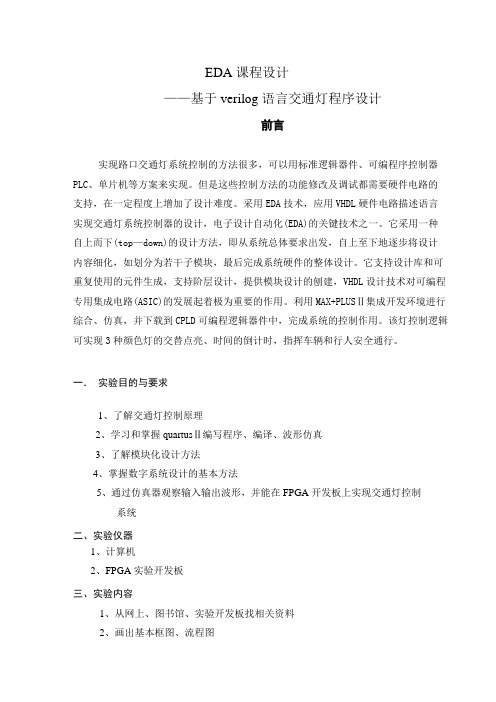
EDA课程设计——基于verilog语言交通灯程序设计前言实现路口交通灯系统控制的方法很多,可以用标准逻辑器件、可编程序控制器PLC、单片机等方案来实现。
但是这些控制方法的功能修改及调试都需要硬件电路的支持,在一定程度上增加了设计难度。
采用EDA技术,应用VHDL硬件电路描述语言实现交通灯系统控制器的设计,电子设计自动化(EDA)的关键技术之一。
它采用一种自上而下(top—down)的设计方法,即从系统总体要求出发,自上至下地逐步将设计内容细化,如划分为若干子模块,最后完成系统硬件的整体设计。
它支持设计库和可重复使用的元件生成,支持阶层设计,提供模块设计的刨建,VHDL设计技术对可编程专用集成电路(ASIC)的发展起着极为重要的作用。
利用MAX+PLUSⅡ集成开发环境进行综合、仿真,并下载到CPLD可编程逻辑器件中,完成系统的控制作用。
该灯控制逻辑可实现3种颜色灯的交替点亮、时间的倒计时,指挥车辆和行人安全通行。
一.实验目的与要求1、了解交通灯控制原理2、学习和掌握quartusⅡ编写程序、编译、波形仿真3、了解模块化设计方法4、掌握数字系统设计的基本方法5、通过仿真器观察输入输出波形,并能在FPGA开发板上实现交通灯控制系统二、实验仪器1、计算机2、FPGA实验开发板三、实验内容1、从网上、图书馆、实验开发板找相关资料2、画出基本框图、流程图3、完成控制器部分状态图的画法4、完成系统的模块划分5、使用V erilog HDL完成整个系统的代码编写6、用quartusⅡ编译并波形仿真7、在实验开发板上验证并调试四、实验步骤1、关于fpga和verilog的相关知识可编程器件的广泛应用,为数字系统的设计带来了极大的灵活性。
由于可编器件可以通过软件编程对硬件的结构和工作方式进行重构,使得硬件的设计可以如同软件设计那样快捷方便。
由于高速发展的FPGA/CPLD兼有串、并行工作方式和高速、高可靠性的特点[1],在电子系统设计中得到了广泛应用。
fpga课程设计交通灯
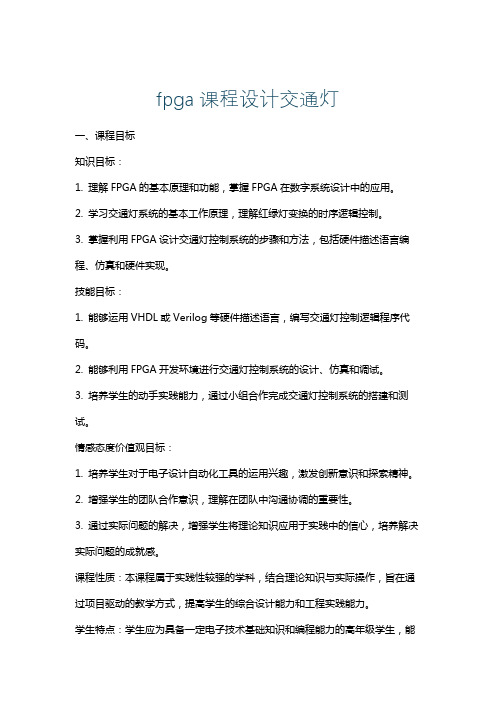
fpga课程设计交通灯一、课程目标知识目标:1. 理解FPGA的基本原理和功能,掌握FPGA在数字系统设计中的应用。
2. 学习交通灯系统的基本工作原理,理解红绿灯变换的时序逻辑控制。
3. 掌握利用FPGA设计交通灯控制系统的步骤和方法,包括硬件描述语言编程、仿真和硬件实现。
技能目标:1. 能够运用VHDL或Verilog等硬件描述语言,编写交通灯控制逻辑程序代码。
2. 能够利用FPGA开发环境进行交通灯控制系统的设计、仿真和调试。
3. 培养学生的动手实践能力,通过小组合作完成交通灯控制系统的搭建和测试。
情感态度价值观目标:1. 培养学生对于电子设计自动化工具的运用兴趣,激发创新意识和探索精神。
2. 增强学生的团队合作意识,理解在团队中沟通协调的重要性。
3. 通过实际问题的解决,增强学生将理论知识应用于实践中的信心,培养解决实际问题的成就感。
课程性质:本课程属于实践性较强的学科,结合理论知识与实际操作,旨在通过项目驱动的教学方式,提高学生的综合设计能力和工程实践能力。
学生特点:学生应为具备一定电子技术基础知识和编程能力的高年级学生,能够理解较为复杂的逻辑设计,并具有一定的自主学习能力。
教学要求:教学中应注重理论与实践相结合,通过案例教学和任务驱动,引导学生主动探索,注重过程评价与结果评价相结合,确保学生达到预定的学习目标。
二、教学内容1. FPGA基础理论:- 数字电路设计基本概念- FPGA芯片结构与工作原理- 硬件描述语言(VHDL/Verilog)基础2. 交通灯控制系统原理:- 交通灯系统的基本组成与功能- 交通灯控制逻辑及时序分析- 交通灯控制系统的安全性、可靠性要求3. FPGA设计流程:- 设计输入、综合与布局布线- 仿真与验证- 硬件实现与测试4. 交通灯控制系统设计:- 交通灯控制逻辑编程- FPGA硬件描述与接口设计- 系统集成与调试5. 教学大纲安排:- 第一周:FPGA基础理论,硬件描述语言入门- 第二周:交通灯系统原理学习,控制逻辑分析- 第三周:FPGA设计流程学习,交通灯控制逻辑编程- 第四周:交通灯控制系统仿真,硬件实现与测试教学内容关联教材章节:- 《FPGA原理与应用》第1章:FPGA基础理论- 《数字系统设计与实践》第3章:交通灯控制系统原理- 《硬件描述语言教程》第2章:硬件描述语言基础- 《FPGA设计实战教程》第4章:FPGA设计流程教学内容确保科学性和系统性,结合教材章节,按照教学大纲的安排和进度,使学生逐步掌握FPGA设计交通灯控制系统的相关知识和技能。
华中科技大学 电子技术课程设计报告(交通灯)

华中科技大学电子技术课程设计题目:交通灯控制器的设计院系:班级:姓名:联系方式:指导老师:目录一课题要求及功能分析 (1)1.1设计任务要求 (1)1.2课题分析 (1)1.3功能介绍及创新之处 (1)1.4 设计难点 (2)二系统框图及具体模块说明 (3)2.1系统框图 (3)2.2具体模块说明 (4)三模块程序清单及仿真波形 (8)3.1 模块程序及仿真波形 (8)3.2管脚分配清单 (12)四实验总结 (14)附录程序源代码 (16)第一章设计内容及要求1.1设计任务要求①设计一个十字路口交通信号灯的控制电路。
要求红、绿灯按一定的规律亮和灭,并在亮灯期间进行倒计时,并将运行时间用数码管显示出来。
②绿灯亮时,为该车道允许通行信号,红灯亮时,为该车道禁止通行信号。
要求主干道每次通行时间为Tx秒,支干道每次通行时间为Ty秒。
每次变换运行车道前绿灯闪烁,持续时间为5秒。
即车道要由主干道转换为支干道时,主干道在通行时间只剩下5秒钟时,绿灯闪烁显示,支干道仍为红灯,以便主干道上已过停车线的车继续通行,未过停车线的车停止通行。
同理,当车道由支干道转换为主干道时,支干道绿灯闪烁显示5秒钟,主干道仍为红灯。
1.2课题分析①本课题要求用Verilog语言描述一个十字路口交通信号灯的控制电路,设计测试方案并通过Muxplus II或者Quartus II软件对设计进行仿真验证,并下载到实验板上调试成功。
②设计参考交通灯十字交叉路口图如下:③本课题要求对红、绿灯的运行时间要能比较方便的进行重新设置。
1.3功能介绍及创新之处①本设计将十字路口分为主干道和支干道,每个干道拥有直行绿灯、左转绿灯及红灯,与现实中的实际情形一致。
②交通灯的运行状态是:主干道绿灯同时支干道红灯,主干道左转绿灯同时支干道红灯,支干道绿灯同时主干道红灯,支干道左转绿灯同时主干道红灯,四种运行状态依次循环显示。
③支干道红灯时间=主干道绿灯时间+主干道左转时间主干道红灯时间=支干道绿灯时间+支干道左转时间④采用verilog编程,并可以通过DE2板上的按键,对每个干道上的每个灯时间进行自由设定。
基于FPGA的交通灯控制器设计_毕业设计论文

基于FPGA的交通灯控制器设计_毕业设计论文摘要:随着城市交通拥堵问题的日益严重,交通灯控制器作为城市交通管理的重要组成部分,起着至关重要的作用。
在传统的交通灯控制系统中,使用的是基于微控制器或PLC的硬件实现方式,无法满足日益复杂的交通需求。
本论文提出了一种基于FPGA的交通灯控制器设计方案,通过利用FPGA的高度可编程性和并行处理能力,实现了对交通灯状态的实时监控和控制。
设计方案通过数码管和按钮进行交互,利用图形化编程软件进行开发和调试。
实验结果表明,所设计的FPGA交通灯控制器具有优异的性能和稳定性,能够满足各种交通场景下的需求。
关键词:交通灯控制器;FPGA;并行处理;图形化编程一、引言随着城市交通流量的不断增加,传统的交通灯控制系统已经不能满足日益复杂的交通需求。
传统的交通灯控制器使用的是基于微控制器或PLC的硬件实现方式,无法提供足够的计算性能和并行处理能力。
因此,本论文提出了一种基于FPGA的交通灯控制器设计方案,通过利用FPGA的高度可编程性和并行处理能力,实现对交通灯状态的实时监控和控制。
二、设计方案本设计方案采用了FPGA作为控制器的核心,通过图形化编程软件进行开发和调试。
设计方案将交通灯控制分为四个主要模块:状态监控模块、状态控制模块、显示模块和按钮模块。
状态监控模块通过检测车辆和行人的状态,实时监控交通灯的状态。
状态控制模块根据交通流量和优先级进行状态切换和调度。
显示模块将交通灯状态显示在数码管上,方便行人和司机观察。
按钮模块通过按钮输入交通灯的初始状态,实现手动控制。
三、系统实现本系统采用Xilinx FPGA开发板进行实现,使用Verilog HDL进行程序编写。
在设计过程中,通过数码管和按钮进行交互,实现手动控制和状态显示。
图形化编程软件使得开发和调试更加便捷,节省了开发周期和人力资源。
四、实验结果通过对实验数据的分析和对比,我们发现所设计的FPGA交通灯控制器在交通流量大、复杂交叉路口和斑马线等特殊情况下,都能够稳定运行并保证交通流畅度。
Verilog代码(计数器、交通灯、串并转换)
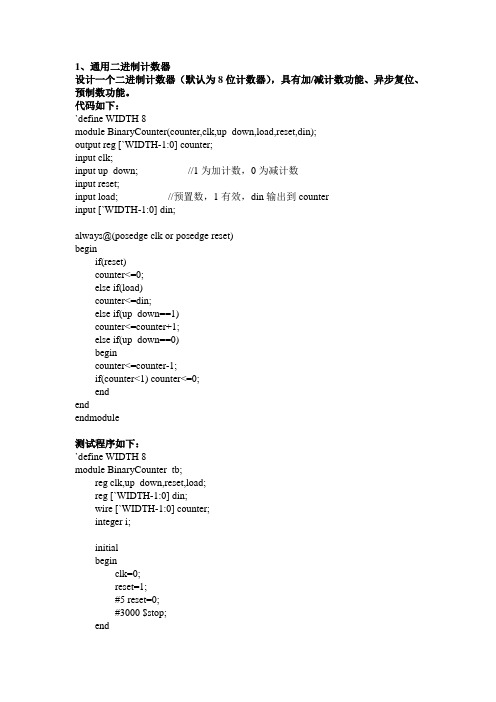
1、通用二进制计数器设计一个二进制计数器(默认为8位计数器),具有加/减计数功能、异步复位、预制数功能。
代码如下:`define WIDTH 8module BinaryCounter(counter,clk,up_down,load,reset,din);output reg [`WIDTH-1:0] counter;input clk;input up_down; //1为加计数,0为减计数input reset;input load; //预置数,1有效,din输出到counterinput [`WIDTH-1:0] din;always@(posedge clk or posedge reset)beginif(reset)counter<=0;else if(load)counter<=din;else if(up_down==1)counter<=counter+1;else if(up_down==0)begincounter<=counter-1;if(counter<1) counter<=0;endendendmodule测试程序如下:`define WIDTH 8module BinaryCounter_tb;reg clk,up_down,reset,load;reg [`WIDTH-1:0] din;wire [`WIDTH-1:0] counter;integer i;initialbeginclk=0;reset=1;#5 reset=0;#3000 $stop;endalways #5 clk=~clk;/*always@(posedge clk)beginup_down={$random}%2;load={$random}%2;din={$random}%256;end*/initialbegin#5;for(i=0;i<256;i=i+3)begindin=i;#10;endendinitialbeginup_down=1;load=1;#20 load=0;#30 load=1;#30 load=0;endalways #200 up_down=~up_down;BinaryCounter bcounter(.counter(counter),.clk(clk),.up_down(up_down),.load(load),.reset(reset),.din(din));endmodule波形图如下:图1 二进制计数器波形图图2 二进制计数器波形图从图像可看出,20s-50s为加计数,50s-80s时load=1,执行置数功能,80s-200s 时up_down=1,为加计数,200s-280s时up_down=0,为减计数。
基于verlilog hdl的交通信号灯电路设计

基于Verilog HDL的交通信号灯电路设计1. 引言交通信号灯是城市道路交通管理中不可或缺的一部分,它通过不同颜色的灯光来指示车辆和行人何时停止和通行。
本文将介绍基于Verilog HDL的交通信号灯电路设计。
2. 设计目标本次设计的目标是实现一个简单的交通信号灯电路,包括红、黄、绿三种状态。
具体要求如下:•红灯亮时,其他两个灯应熄灭。
•黄灯亮时,其他两个灯应熄灭。
•绿灯亮时,其他两个灯应熄灭。
•红、黄、绿三个状态按照固定时间间隔循环切换。
3. 电路设计3.1 总体结构本次设计采用有限状态机(FSM)来实现交通信号灯电路。
有限状态机是一种数学模型,可以描述系统在不同状态之间的转移和输出。
我们将使用Verilog HDL来描述并实现这个有限状态机。
3.2 状态定义根据设计目标,我们可以定义三种状态:红、黄、绿。
我们可以用二进制数来表示这三种状态,比如红色为00,黄色为01,绿色为10。
我们可以使用两个输出信号来表示当前状态:red和green。
3.3 状态转移根据设计目标,交通信号灯应该按照固定时间间隔循环切换状态。
我们可以使用一个计数器来实现这个功能。
当计数器的值达到一定阈值时,状态应该切换到下一个状态。
具体的状态转移如下:•当前状态为红色时(00),计数器的值递增。
–当计数器的值达到阈值时,切换到黄色状态(01),并重置计数器。
•当前状态为黄色时(01),计数器的值递增。
–当计数器的值达到阈值时,切换到绿色状态(10),并重置计数器。
•当前状态为绿色时(10),计数器的值递增。
–当计数器的值达到阈值时,切换到红色状态(00),并重置计数器。
3.4 Verilog HDL代码实现以下是基于Verilog HDL实现交通信号灯电路的代码:module traffic_light(input wire clk,output reg red,output reg yellow,output reg green);reg [1:0] state;reg [3:0] counter;parameter THRESHOLD = 4'b1010; // 阈值always @(posedge clk) begincase(state)2'b00: begin// 红灯状态if(counter == THRESHOLD) beginstate <= 2'b01; // 切换到黄灯状态counter <= 0;end else begincounter <= counter + 1;endend2'b01: begin// 黄灯状态if(counter == THRESHOLD) beginstate <= 2'b10; // 切换到绿灯状态counter <= 0;end else begincounter <= counter + 1;endend2'b10: begin// 绿灯状态if(counter == THRESHOLD) beginstate <= 2'b00; // 切换到红灯状态counter <= 0;end else begincounter <= counter + 1;endendendcaseendalways @(state) begincase(state)2'b00: begin// 红灯亮,其他两个灯熄灭red <= 1;yellow <= 0;green <= 0;end2'b01: begin// 黄灯亮,其他两个灯熄灭red <= 0;yellow <= 1;green <= 0;end2'b10: begin// 绿灯亮,其他两个灯熄灭red <= 0;yellow <= 0;green <= 1;endendcaseendendmodule4. 总结本文介绍了基于Verilog HDL的交通信号灯电路设计。
交通信号灯控制器代码及说明
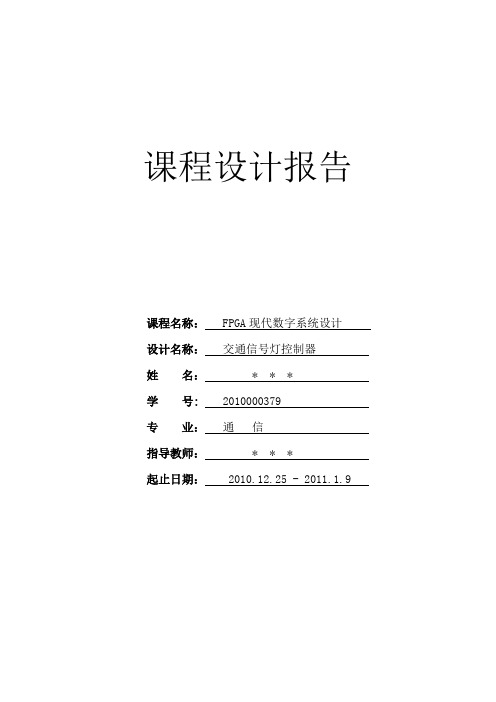
课程设计报告课程名称: FPGA现代数字系统设计设计名称:交通信号灯控制器姓名: * * *学号: ********** 专业:通信指导教师:* * *起止日期: 2010.12.25 - 2011.1.9课程设计任务书设计名称:一、设计目的和意义通过应用Verilog语言在QuartusⅡ软件平台上设计交通信号灯控制器,并借助硬件来测试仿真效果。
通过课程设计,熟悉硬件编程语言的应用,特别是有限状态机的灵活使用,为以后进一步的学习实践打下良好的基础。
二、设计原理(1) 主、支干道用传感器检测车辆到来情况,实验电路用逻辑开关代替。
(2) 选择1HZ时钟脉冲作为系统时钟。
(3) 45s、25s、5s定时信号可用顺计时,也可用倒计时,计时起始信号由主控电路给出,每当计满所需时间,启、闭三色信号灯,并启动另一计时电路。
(4) 交通灯状态变化如表1及图1所示:表1:交通灯状态图图1:交通灯状态图(5) 交通灯设计输入信号4个:CLK(时钟),EN(使能),EMERGENCY(紧急),BCHECK(检测) ;输出信号4个:LAMPA(主干道信号灯),LAMPB(支干道信号灯),ACOUNT(主干道计数器),BCOUNT(支干道计数器)。
交通灯控制原理如图2所示。
图2:交通灯原理图三、详细设计步骤(1) 确定4个输入信号与4个输出信号,具体见图2;(2) 将50MHZ时钟分频为1MHZ;(3) 设计红黄绿3中信号灯切换的时间及顺序;(4) 设计支路检测状态下的信号灯切换;(5) 设计紧急(EMERGENCY)状态下信号灯的切换;(6) 程序使用3always块[1],详细代码如下:module traffic_control(CLK,EN,EMERGENCY,BCHECK,LAMPA,LAMPB,ACOUNT,BCOUNT);output[2:0] ACOUNT,BCOUNT;output[2:0] LAMPA,LAMPB;reg clk1;input CLK,EN,EMERGENCY,BCHECK;reg[2:0] numa,numb;reg tempa,tempb;reg[25:0]count;reg[2:0] counta,countb;reg[2:0] ared,ayellow,agreen,aleft,bred,byellow,bgreen,bleft;reg[2:0] LAMPA,LAMPB;/* 信号定义与说明:CLK:为同步时钟;EN:使能信号,为1 的话,则控制器开始工作;LAMPA:控制A 方向四盏灯的亮灭;其中,LAMPA0~LAMPA2,分别控制A 方向的绿灯、黄灯和红灯;LAMPB:控制B 方向四盏灯的亮灭;其中,LAMPB0 ~ LAMPB2,分别控制B 方向的绿灯、黄灯和红灯;ACOUNT:用于A 方向灯的时间显示,8 位BCOUNT:用于B 方向灯的时间显示,8 位*/always @(posedge CLK ) //将50MHZ时钟分频为1MHZbeginif(count==49999999)begincount<=0;clk1<=~clk1;endelsecount<=count+1;endassign ACOUNT=numa;assign BCOUNT=numb;always @(EN )if(!EN)beginared <=6;ayellow <=2;agreen <=4;bred <=6;byellow <=2;bgreen <=4;endalways @(posedge clk1)//该进程控制A 方向beginif(EMERGENCY) //EMERGENCY高电平有效,手动控制beginnuma<=0;LAMPA<=4;counta<=0;tempa<=0;endelse if(EN)beginif(!tempa)//亮灯begintempa<=1;case(counta)//控制亮灯的顺序0: begin numa<=agreen; LAMPA<=1; counta<=1; end1: begin numa<=ayellow; LAMPA<=2; counta<=2; end2: begin numa<=ared; LAMPA<=4; counta<=0; enddefault: LAMPA<=4;endcaseendelse //倒计时beginif(numa>2) numa<=numa-1;if(numa==2) begin numa<=1;tempa<=0;if ((BCHECK) &&(counta==2)) counta<=0;end/*if(numa>1)if(numa[3:0]==0)beginnuma[3:0]<=4'b1001;numa[7:4]<=numa[7:4]-1;endelse numa[3:0]<=numa[3:0]-1;if (numa==2)begin tempa=0;if ((!BCHECK )&& (counta==1)) counta<=0;end*/endendelsebeginLAMPA<=3'b100;counta<=0; tempa<=0;endendalways @(posedge clk1)//该进程控制B 方向的四种灯beginif(EMERGENCY) //EMERGENCY高电平有效,手动控制beginnumb<=0;LAMPB<=4;countb<=0;tempb<=0;endelse if (EN)beginif(!tempb)begintempb<=1;case (countb)0: begin numb<=bred; LAMPB<=4; countb<=1; end1: begin numb<=bgreen; LAMPB<=1; countb<=2; end2: begin numb<=byellow; LAMPB<=2; countb<=0; enddefault: LAMPB<=4;endcaseendelsebeginif(numb>2) numb<=numb-1;if(numb==2) begin numb<=1;tempb<=0;if ((BCHECK )&& (countb==1)) countb<=0;end/*if(numb>1)if(!numb[3:0])beginnumb[3:0]<=9;numb[7:4]<=numb[7:4]-1;endelse numb[3:0]<=numb[3:0]-1;if(numb==2)begin tempb=0;if ((!BCHECK) && (countb==1)) countb<=0;end*/endendelsebeginLAMPB<=3'b100;tempb<=0; countb<=0;endendendmodulemodule四、结果分析程序仿真效果如图3和图4所示:为观察方便,将红黄绿灯显示时间分别缩短,图中[4]表示红灯亮,[2]表示黄灯亮,[1]表示绿灯亮,BCHECK信号代表支干道检测状况,低电平表示检测到有车辆正常通过,EMERGENCY代表紧急状态信号,低电平表示紧急信号无效。
LED模拟交通灯实验实训报告

实训报告FPGA系统LED模拟交通灯实验姓名:学号:班级:专业:指导教师:年月日一、大规模多FPGA系统概述大规模多FPGA系统是针对大学、研究机构和集成电路设计公司的教学及科研推出的多FPGA开发平台。
该平台采用了Altera公司的FPGA芯片,核心芯片选择了Cyclone II系列的EP2C20,该平台可以支持四块FPGA芯片的单独下载及调试,也支持级联下载以应对更为复杂的设计。
FPGA采用了逻辑单元阵列LCA(Logic Cell Array)这样一个概念,内部包括可配置逻辑模块CLB(Configurable Logic Block)、输入输出模块IOB(Input Output Block)和内部连线(Interconnect)三个部分。
现场可编程门阵列(FPGA)是可编程器件,与传统逻辑电路和门阵列(如PAL,GAL及CPLD器件)相比,FPGA具有不同的结构。
FPGA利用小型查找表(16×1RAM)来实现组合逻辑,每个查找表连接到一个D触发器的输入端,触发器再来驱动其他逻辑电路或驱动I/O,由此构成了既可实现组合逻辑功能又可实现时序逻辑功能的基本逻辑单元模块,这些模块间利用金属连线互相连接或连接到I/O模块。
FPGA的逻辑是通过向内部静态存储单元加载编程数据来实现的,存储在存储器单元中的值决定了逻辑单元的逻辑功能以及各模块之间或模块与I/O间的联接方式,并最终决定了FPGA所能实现的功能,FPGA允许无限次的编程。
二、FPGA的应用及本次试验主要器件FPGA的应用可分为三个层面:电路设计,产品设计,系统设计。
电路设计:连接逻辑,控制逻辑是FPGA早期发挥作用比较大的领域也是FPGA应用的基石。
事实上在电路设计中应用FPGA要求开发者要具备相应的硬件知识(电路知识)和软件应用能力(开发工具)。
产品设计:把相对成熟的技术应用到某些特定领域开发出满足行业需要并能被行业客户接受的产品。
基于Verilog HDL语言的带左转复杂交通灯设计
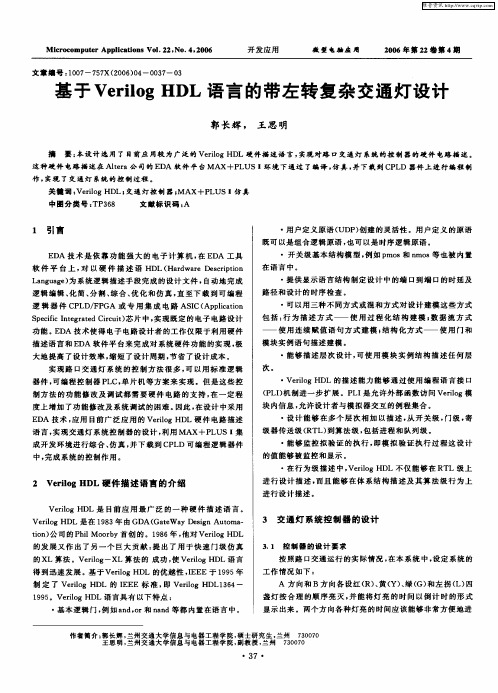
Mirc mp trA piain o. 2 N . ,06 coo ue p l t sV 12 , o 4 2 0 c o
文 章 编 号 :0 7 5 X(0 6 0 -O 3 一 O 1 0 —7 7 2 0 )4 O 7 3
开 发 应 用
在语言 中。
・
路径和设计 的时序检查 。
・
可 以 用 三 种 不 同方 式 或 混 和 方 式 对 设 计 建 模 这 些 方 式
包 括 : 为 描 述 方 式 —— 使 用 过 程 化 结 构 建 模 ; 据 流 方 式 行 数
— —
使 用 连 续 赋 值 语 句 方 式 建 模 ; 构 化 方 式 —— 使 用 门和 结 能 够 描 述 层 次 设 计 , 使 用 模 块 实 例 结 构 描 述 任 何 层 可
・
设计 能够在 多个层 次相加 以描述 , 开关级 , 级 , 从 门 寄 能 够 监 控 拟 验 证 的执 行 , 模 拟 验 证 执 行 过 程 这 设 计 即
级 器传 送 级 ( TL 到算 法 级 , 括 进 程 和 队 列 级 。 R ) 包
・
成 开 发 环 境 进 行 综 合 、 真 , 下 载 到 C L 可 编 程 逻 辑 器 件 仿 并 PD
次。
・
用 户 定 义 原语 ( P) 建 的 灵 活 性 。用 户 定 义 的 原 语 UD 创
既 可 以是 组 合 逻 辑 原 语 , 可 以 是 时 序 逻 辑 原 语 。 也
・
开 关 级 基 本 结 构 模 型 , 如 p s n s 也 被 内置 例 mo 和 mo 等
提供 显示 语 言 结 构 制 定 设 计 中 的 端 口到 端 口的 时 延 及
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
交通灯
一、实验目的
写一个交通灯,要求:
①有东西南北四个方向,两组交通灯轮流交替变换,其中,红灯时间为30
个时间单位,绿灯时间为25个时间单位,黄灯时间为5个时间单位。
最后用modelsim软件进行仿真。
②要求设计是一个可综合设计。
二、实验原理
根据实验要求的逻辑功能描述,可以分析得出原理图如下:
控制器即可以设计为一个有限状态机的形式:
E-W方向S-N方向
状态R Y G R Y G
1 0 0 1 0 0 IDLE
1 0 0 0 0 1 S1
1 0 0 0 1 0 S2
0 0 1 1 0 0 S3
0 1 0 1 0 0 S4
根据实验要求画出控制器的状态转移图如下:
三、代码
1、源代码
(1)控制器模块
module traffic_lights(clk,rst,count,ew,sn);
input clk,rst;
input[5:0] count;
output[2:0] ew,sn;
reg[2:0] ew,sn;
reg[3:0] state;
parameter Idle=3'b000,s1=3'b001,s2=3'b010,s3=3'b011,s4=3'b100; always @(posedge clk)
if(!rst)
begin
state<=Idle;
end
else
casex(state)
Idle: if(rst)
begin
state<=s1;
end
s1: if(count=='d25)
begin
state<=s2;
end
s2: if(count=='d30)
begin
state<=s3;
end
s3: if(count=='d55)
begin
state<=s4;
end
s4: if(count=='d60)
begin
state<=s1;
end
endcase
always @(posedge clk)
begin
if(!rst)
begin
ew<=3'b100;
sn<=3'b100;
end
else
casex(state)
Idle: if(rst)
begin
ew<=3'b100;
sn<=3'b001;
end
s1: if(count=='d25)
begin
ew<=3'b100;
sn<=3'b010;
end
s2: if(count=='d30)
begin
ew<=3'b001;
sn<=3'b100;
end
s3: if(count=='d55)
begin
ew<=3'b010;
sn<=3'b100;
end
s4: if(count=='d60)
begin
ew<=3'b100;
sn<=3'b001;
end
default: state<=Idle;
endcase
end
endmodule
(2)计数器模块
module counter(en,clk,rst,out);
output[5:0]out;
input en,clk,rst;
reg[5:0] out;
always@(posedge clk or negedge rst) begin
if(!rst)
out<='d0;
else if(!en&&out<'d60)
out<=out+1;
else
out<='d1;
end
endmodule
(3)将控制器与计数器进行连接
module traffic_lights_top(out,clk,rst,en,ew,sn); input clk,rst,en;
output[2:0] ew,sn;
output[5:0]out;
wire[5:0] out;
traffic_lights u1(
.clk(clk),
.rst(rst),
.count(out),
.ew(ew),
.sn(sn)
);
counter u2(
.en(en),
.clk(clk),
.rst(rst),
.out(out)
);
endmodule
2、激励
`timescale 1ns/100ps
module traffic_lights_tb;
reg clk,rst,en;
wire[2:0] ew,sn;
wire[5:0]out;
traffic_lights_top m(
.clk(clk),
.rst(rst),
.en(en),
.ew(ew),
.sn(sn),
.out(out)
);
always
#5 clk=~clk;
initial
en<=1;
initial
begin
clk<=1;
en<=0;
rst<=0;
#5 rst<=1;
end
endmodule
四、仿真波形
(图一)
(图二)
五、波形说明
波形图中,从上至下依次为:时钟信号clk、复位信号rst、计数器使能端en、东西方向上灯的状态ew、南北方向上灯的状态sn、计数器的输出out。
该程序实现的功能是在一个十字路口的交通灯的轮流交替变换:
状态方向
灯的状态
0——25 25——30 30——55 55——60
东西方向ew 红红绿黄
南北方向sn 绿黄红红图一可以完整的看到60个时间单位内两个方向上灯交替的状况
图二可以清楚的看到在时间从0——30过程中灯的跳变时间和结果。
五、实验过程中遇到的问题及解决方法
1、在实验过程中得到的波形图跟我设计的时间间隔不一致,仔细检查过后
发现是因为控制器和计数器没有很好的连接在一起,导致灯的跳变跟计数器的控制脱离了,修改之后得到时间间隔比例跟设计一致的波形。
2、在检查波形的过程中发现计数器实现的不是模60,而是模64,将计数器程序中的选择条件从if(!en)改为if(!en&&out<’d60)之后得到了自己想要的计数器。
六、实验心得
刚开始,程序调试过程中始终都有些莫名其妙的错误,自己只能凭着自己的理解和单纯靠一些感觉去修改。
有时候越改错误越多,到后来程序显示没有错误了,但是仿真却无法执行,一长串的警告看的我有点崩溃。
静下心来后从头开始分析每一句的程序,最后发现其实只是一个很小的失误。