数据结构(C语言版)例题(第三章:栈和队列)
数据结构第三章栈和队列3习题
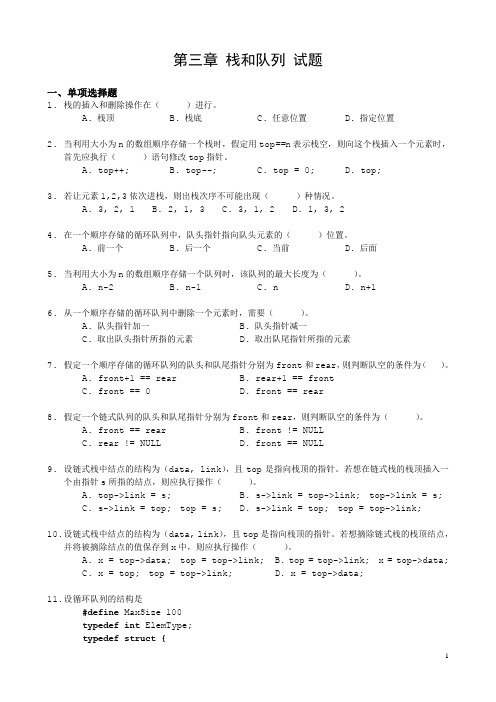
第三章栈和队列试题一、单项选择题1.栈的插入和删除操作在()进行。
A. 栈顶B. 栈底C. 任意位置D. 指定位置2.当利用大小为n的数组顺序存储一个栈时,假定用top==n表示栈空,则向这个栈插入一个元素时,首先应执行()语句修改top指针。
A. top++;B. top--;C. top = 0;D. top;3.若让元素1,2,3依次进栈,则出栈次序不可能出现()种情况。
A. 3, 2, 1B. 2, 1, 3C. 3, 1, 2D. 1, 3, 24.在一个顺序存储的循环队列中,队头指针指向队头元素的()位置。
A. 前一个B. 后一个C. 当前D. 后面5.当利用大小为n的数组顺序存储一个队列时,该队列的最大长度为()。
A. n-2B. n-1C. nD. n+16.从一个顺序存储的循环队列中删除一个元素时,需要()。
A. 队头指针加一B. 队头指针减一C. 取出队头指针所指的元素D. 取出队尾指针所指的元素7.假定一个顺序存储的循环队列的队头和队尾指针分别为front和rear,则判断队空的条件为()。
A. front+1 == rearB. rear+1 == frontC. front == 0D. front == rear8.假定一个链式队列的队头和队尾指针分别为front和rear,则判断队空的条件为()。
A. front == rearB. front != NULLC. rear != NULLD. front == NULL9.设链式栈中结点的结构为(data, link),且top是指向栈顶的指针。
若想在链式栈的栈顶插入一个由指针s所指的结点,则应执行操作()。
A. top->link = s;B.s->link = top->link; top->link = s;C. s->link = top; top = s;D. s->link = top; top = top->link;10.设链式栈中结点的结构为(data, link),且top是指向栈顶的指针。
数据结构(C语言版)第三四章习题答案(可编辑修改word版)
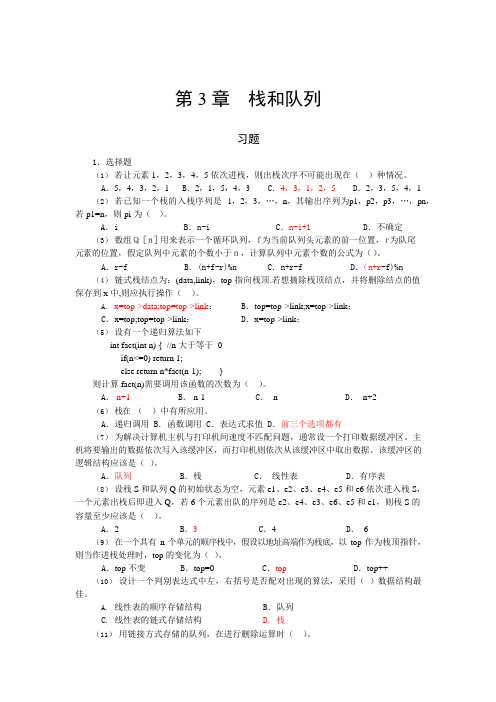
第3 章栈和队列习题1.选择题(1)若让元素1,2,3,4,5 依次进栈,则出栈次序不可能出现在()种情况。
A.5,4,3,2,1 B.2,1,5,4,3 C.4,3,1,2,5 D.2,3,5,4,1 (2)若已知一个栈的入栈序列是1,2,3,…,n,其输出序列为p1,p2,p3,…,pn,若p1=n,则pi 为()。
A.i B.n-i C.n-i+1 D.不确定(3)数组Q[n]用来表示一个循环队列,f为当前队列头元素的前一位置,r为队尾元素的位置,假定队列中元素的个数小于n,计算队列中元素个数的公式为()。
A.r-f B.(n+f-r)%n C.n+r-f D.(n+r-f)%n (4)链式栈结点为:(data,link),top 指向栈顶.若想摘除栈顶结点,并将删除结点的值保存到x 中,则应执行操作()。
A.x=top->data;top=top->link;B.top=top->link;x=top->link;C.x=top;top=top->link;D.x=top->link;(5)设有一个递归算法如下int fact(int n) { //n 大于等于0if(n<=0) return 1;else return n*fact(n-1); }则计算fact(n)需要调用该函数的次数为()。
A.n+1 B.n-1 C.n D.n+2 (6)栈在()中有所应用。
A.递归调用 B.函数调用 C.表达式求值 D.前三个选项都有(7)为解决计算机主机与打印机间速度不匹配问题,通常设一个打印数据缓冲区。
主机将要输出的数据依次写入该缓冲区,而打印机则依次从该缓冲区中取出数据。
该缓冲区的逻辑结构应该是()。
A.队列B.栈C.线性表D.有序表(8)设栈S 和队列Q 的初始状态为空,元素e1、e2、e3、e4、e5 和e6 依次进入栈S,一个元素出栈后即进入Q,若6 个元素出队的序列是e2、e4、e3、e6、e5 和e1,则栈S 的容量至少应该是()。
《数据结构》习题集:第3章栈和队列
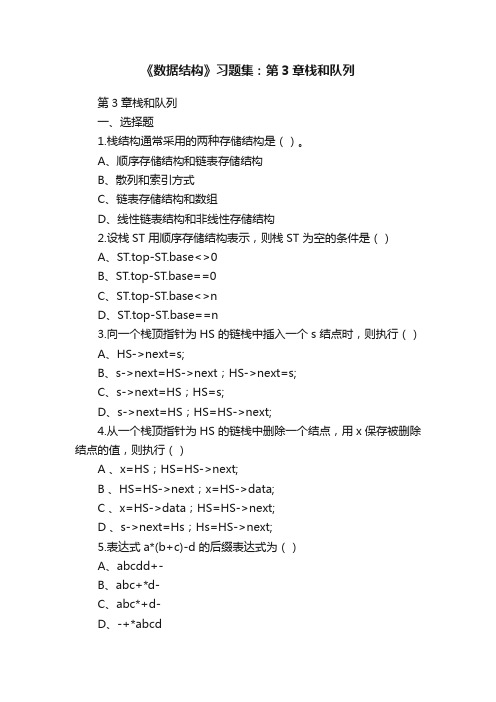
《数据结构》习题集:第3章栈和队列第3章栈和队列一、选择题1.栈结构通常采用的两种存储结构是()。
A、顺序存储结构和链表存储结构B、散列和索引方式C、链表存储结构和数组D、线性链表结构和非线性存储结构2.设栈ST 用顺序存储结构表示,则栈ST 为空的条件是()A、ST.top-ST.base<>0B、ST.top-ST.base==0C、ST.top-ST.base<>nD、ST.top-ST.base==n3.向一个栈顶指针为HS 的链栈中插入一个s 结点时,则执行()A、HS->next=s;B、s->next=HS->next;HS->next=s;C、s->next=HS;HS=s;D、s->next=HS;HS=HS->next;4.从一个栈顶指针为HS 的链栈中删除一个结点,用x 保存被删除结点的值,则执行()A 、x=HS;HS=HS->next;B 、HS=HS->next;x=HS->data;C 、x=HS->data;HS=HS->next;D 、s->next=Hs;Hs=HS->next;5.表达式a*(b+c)-d 的后缀表达式为()A、abcdd+-B、abc+*d-C、abc*+d-D、-+*abcd6.中缀表达式A-(B+C/D)*E 的后缀形式是()A、AB-C+D/E*B、ABC+D/E*C、ABCD/E*+-D、ABCD/+E*-7.一个队列的入列序列是1,2,3,4,则队列的输出序列是()A、4,3,2,1B、1,2,3,4C、1,4,3,2D、3,2,4,18.循环队列SQ 采用数组空间SQ.base[0,n-1]存放其元素值,已知其头尾指针分别是front 和rear,则判定此循环队列为空的条件是()A、Q.rear-Q.front==nB、Q.rear-Q.front-1==nC、Q.front==Q.rearD、Q.front==Q.rear+19.循环队列SQ 采用数组空间SQ.base[0,n-1]存放其元素值,已知其头尾指针分别是front 和rear,则判定此循环队列为满的条件是()A、Q.front==Q.rearB、Q.front!=Q.rearC、Q.front==(Q.rear+1)%nD、Q.front!=(Q.rear+1)%n10.若在一个大小为6 的数组上实现循环队列,且当前rear 和front 的值分别为0 和3,当从队列中删除一个元素,再加入两个元素后,rear 和front 的值分别为()A、1,5B、2, 4C、4,2D、5,111.用单链表表示的链式队列的队头在链表的()位置A、链头B、链尾C、链中12.判定一个链队列Q(最多元素为n 个)为空的条件是()A、Q.front==Q.rearB、Q.front!=Q.rearC、Q.front==(Q.rear+1)%nD、Q.front!=(Q.rear+1)%n13.在链队列Q 中,插入s 所指结点需顺序执行的指令是()A 、Q.front->next=s;f=s;B 、Q.rear->next=s;Q.rear=s;C 、s->next=Q.rear;Q.rear=s;D 、s->next=Q.front;Q.front=s;14.在一个链队列Q 中,删除一个结点需要执行的指令是()A、Q.rear=Q.front->next;B、Q.rear->next=Q.rear->next->next;C、Q.front->next=Q.front->next->next;D、Q.front=Q.rear->next;15.用不带头结点的单链表存储队列,其队头指针指向队头结点,队尾指针指向队尾结点,则在进行出队操作时()A、仅修改队头指针B、仅修改队尾指针C、队头尾指针都要修改D、队头尾指针都可能要修改。
C语言栈和队列课后题

• Status DeCyQueue(CyQueue &Q,int &x)// 带tag域的循环队列出队算法 { if(Q.front==Q.rear&&Q.tag==0) return INFEASIBLE; x=Q.base[Q.front]; Q.front=(Q.front+1)%MAXSIZE; if(Q.front==Q.rear) Q.tag=0; //队列空 return OK; }//DeCyQueue
datatype top (twostack *s,int i)
/* 两栈共享向量空间,i是0或1,表示两个栈,本算法是取栈顶元素操作 */
{ datatype x; if (s->top[0]==-1 && s->top[1]==m) return(0);/* 栈空 */ else {switch (i) {case 0: x=s->v[s->top[0]];break; case 1: x=s->v[s->top[1]];break; default: printf(“栈编号输入错误”);return(0); } return(x); /* 取栈顶元素成功 */ } } /* 算法结束 */
• Status EnCyQueue(CyQueue &Q,int x)//带 tag域的循环队列入队算法 { if(Q.front==Q.rear&&Q.tag==1) //tag域的值 为0表示"空",1表示"满" return OVERFLOW; Q.base[Q.rear]=x; Q.rear=(Q.rear+1)%MAXSIZE; if(Q.front==Q.rear) Q.tag=1; //队列满 }//EnCyQueue
c语言数据结构第3章栈和队列自测卷答案

head 第3章 栈和队列 自测卷答案 姓名 班级一、填空题〔每空1分,共15分〕1. 【李春葆】向量、栈和队列都是 线性 构造,可以在向量的 任何 位置插入和删除元素;对于栈只能在 栈顶 插入和删除元素;对于队列只能在 队尾 插入和 队首 删除元素。
2. 栈是一种特殊的线性表,允许插入和删除运算的一端称为 栈顶 。
不允许插入和删除运算的一端称为 栈底 。
3. 队列 是被限定为只能在表的一端进展插入运算,在表的另一端进展删除运算的线性表。
4. 在一个循环队列中,队首指针指向队首元素的 前一个 位置。
5. 在具有n 个单元的循环队列中,队满时共有 n-1 个元素。
6. 向栈中压入元素的操作是先 挪动栈顶指针 ,后 存入元素 。
7. 从循环队列中删除一个元素时,其操作是 先 挪动队首指针 ,后 取出元素 。
8. 〖00年统考题〗带表头结点的空循环双向链表的长度等于 0 。
解:二、判断正误〔判断以下概念的正确性,并作出简要的说明。
〕〔每题1分,共10分〕 〔 × 〕1. 线性表的每个结点只能是一个简单类型,而链表的每个结点可以是一个复杂类型。
错,线性表是逻辑构造概念,可以顺序存储或链式存储,与元素数据类型无关。
〔 × 〕2. 在表构造中最常用的是线性表,栈和队列不太常用。
错,不一定吧?调用子程序或函数常用,CPU 中也用队列。
〔 √ 〕3. 栈是一种对所有插入、删除操作限于在表的一端进展的线性表,是一种后进先出型构造。
〔 √ 〕4. 对于不同的使用者,一个表构造既可以是栈,也可以是队列,也可以是线性表。
正确,都是线性逻辑构造,栈和队列其实是特殊的线性表,对运算的定义略有不同而已。
〔 × 〕5. 栈和链表是两种不同的数据构造。
错,栈是逻辑构造的概念,是特殊殊线性表,而链表是存储构造概念,二者不是同类项。
〔 × 〕6. 栈和队列是一种非线性数据构造。
错,他们都是线性逻辑构造,栈和队列其实是特殊的线性表,对运算的定义略有不同而已。
栈和队列练习1

第三章栈和队列一、选择题部分1. 一个栈的入栈序列是a,b,c,d,e,则栈的不可能的输出序列是(C)。
(A) edcba(B)decba(C)dceab (D)abcde2.栈结构通常采用的两种存储结构是(A)。
(A)线性存储结构和链表存储结构(B)散列方式和索引方式(C)链表存储结构和数组(D)线性存储结构和非线性存储结构3.判定一个顺序栈ST(最多元素为m0)为空的条件是( B)。
(A) ST-〉top!=0 (B)ST-〉top==0(C)ST-〉top!=m0 (D)ST-〉top=m04.判定一个栈ST(最多元素为m0)为栈满的条件是( C)。
(A)ST-〉top!=0 (B)ST-〉top==0(C)ST-〉top==m0(D)ST-〉top==m0-15.一个队列的入列序列是1,2,3,4,则队列的输出序列是( B)。
(A)4,3,2,1(B)1,2,3,4(C)1,4,3,2(D)3,2,4,16.循环队列用数组A[0,m-1]存放其元素值,已知其头尾指针分别是front和rear 则当前队列中的元素个数是(B )(A)(rear-front+m)%m (B) rear-front+1 (C)rear-front-1(D)rear-front7.栈和队列的共同点是(C )(A)都是先进后出(B)都是先进先出(C)只允许在端点处插入和删除元素(D)没有共同点8.表达式a*(b+c)-d的后缀表达式是(B)。
(A)abcd*+-(B)abc+*d- (C)abc*+d-(D)-+*abcd9.4个元素a1,a2,a3和a4依次通过一个栈,在a4进栈前,栈的状态,则不可能的出栈序是(C)(A)a4,a3,a2,a1 (B)a3,a2,a4,a1(C)a3,a1,a4,a2 (D)a3,a4,a2,a110.以数组Q[0..m-1]存放循环队列中的元素,变量rear和qulen分别指示循环队列中队尾元素的实际位置和当前队列中元素的个数,队列第一个元素的实际位置是( D )(A)rear-qulen (B)rear-qulen+m(C)m-qulen (D)(1+rear+m-qulen)% m二、填空题部分1.栈的特点是(先进后出),队列的特点是(先进先出)。
《数据结构》习题汇编03第三章栈和队列试题

第三章栈和队列试题一、单项选择题1.栈的插入和删除操作在()进行。
A. 栈顶B. 栈底C. 任意位置D. 指定位置2.当利用大小为n的数组顺序存储一个栈时,假定用top==n表示栈空,则向这个栈插入一个元素时,首先应执行()语句修改top指针。
A. top++;B. top--;C. top = 0;D. top;3.若让元素1,2,3依次进栈,则出栈次序不可能出现()种情况。
A. 3, 2, 1B. 2, 1, 3C. 3, 1, 2D. 1, 3, 24.在一个顺序存储的循环队列中,队头指针指向队头元素的()位置。
A. 前一个B. 后一个C. 当前D. 后面5.当利用大小为n的数组顺序存储一个队列时,该队列的最大长度为()。
A. n-2B. n-1C. nD. n+16.从一个顺序存储的循环队列中删除一个元素时,需要()。
A. 队头指针加一B. 队头指针减一C. 取出队头指针所指的元素D. 取出队尾指针所指的元素7.假定一个顺序存储的循环队列的队头和队尾指针分别为front和rear,则判断队空的条件为()。
A. front+1 == rearB. rear+1 == frontC. front == 0D. front == rear8.假定一个链式队列的队头和队尾指针分别为front和rear,则判断队空的条件为()。
A. front == rearB. front != NULLC. rear != NULLD. front == NULL9.设链式栈中结点的结构为(data, link),且top是指向栈顶的指针。
若想在链式栈的栈顶插入一个由指针s所指的结点,则应执行操作()。
A. top->link = s;B.s->link = top->link; top->link = s;C. s->link = top; top = s;D. s->link = top; top = top->link;10.设链式栈中结点的结构为(data, link),且top是指向栈顶的指针。
大学《数据结构》第三章:栈和队列-第一节-栈

第一节栈
一、栈的定义及其运算
1、栈的定义
栈(Stack):是限定在表的一端进行插入和删除运算的线性表,通常将插入、删除的一端称为栈项(top),另一端称为栈底(bottom)。
不含元素的空表称为空栈。
栈的修改是按后进先出的原则进行的,因此,栈又称为后进先出(Last In First Out)的线性表,简称为LIFO表。
真题选解
(例题·填空题)1、如图所示,设输入元素的顺序是(A,B,C,D),通过栈的变换,在输出端可得到各种排列。
若输出序列的第一个元素为D,则输出序列为。
隐藏答案
【答案】DCBA
【解析】根据堆栈"先进后出"的原则,若输出序列的第一个元素为D,则ABCD入栈,输出序列为DCBA
2、栈的基本运算
(1)置空栈InitStack(&S):构造一个空栈S。
第三章 栈与队列 习题及答案
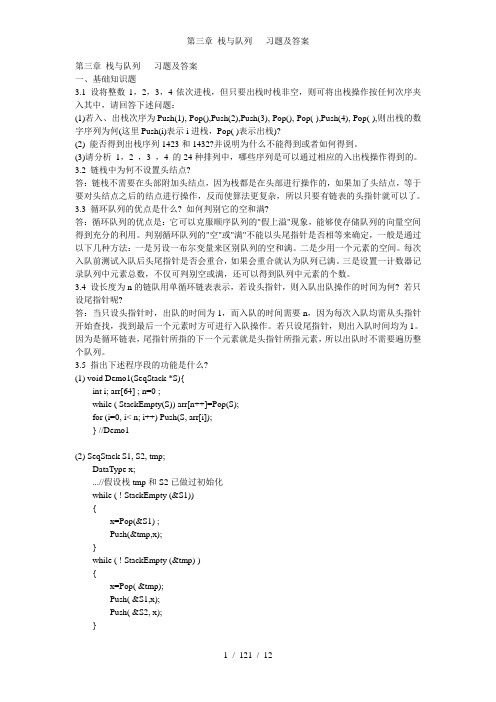
第三章栈与队列习题及答案一、基础知识题3.1 设将整数1,2,3,4依次进栈,但只要出栈时栈非空,则可将出栈操作按任何次序夹入其中,请回答下述问题:(1)若入、出栈次序为Push(1), Pop(),Push(2),Push(3), Pop(), Pop( ),Push(4), Pop( ),则出栈的数字序列为何(这里Push(i)表示i进栈,Pop( )表示出栈)?(2) 能否得到出栈序列1423和1432?并说明为什么不能得到或者如何得到。
(3)请分析1,2 ,3 ,4 的24种排列中,哪些序列是可以通过相应的入出栈操作得到的。
3.2 链栈中为何不设置头结点?答:链栈不需要在头部附加头结点,因为栈都是在头部进行操作的,如果加了头结点,等于要对头结点之后的结点进行操作,反而使算法更复杂,所以只要有链表的头指针就可以了。
3.3 循环队列的优点是什么? 如何判别它的空和满?答:循环队列的优点是:它可以克服顺序队列的"假上溢"现象,能够使存储队列的向量空间得到充分的利用。
判别循环队列的"空"或"满"不能以头尾指针是否相等来确定,一般是通过以下几种方法:一是另设一布尔变量来区别队列的空和满。
二是少用一个元素的空间。
每次入队前测试入队后头尾指针是否会重合,如果会重合就认为队列已满。
三是设置一计数器记录队列中元素总数,不仅可判别空或满,还可以得到队列中元素的个数。
3.4 设长度为n的链队用单循环链表表示,若设头指针,则入队出队操作的时间为何? 若只设尾指针呢?答:当只设头指针时,出队的时间为1,而入队的时间需要n,因为每次入队均需从头指针开始查找,找到最后一个元素时方可进行入队操作。
若只设尾指针,则出入队时间均为1。
因为是循环链表,尾指针所指的下一个元素就是头指针所指元素,所以出队时不需要遍历整个队列。
3.5 指出下述程序段的功能是什么?(1) void Demo1(SeqStack *S){int i; arr[64] ; n=0 ;while ( StackEmpty(S)) arr[n++]=Pop(S);for (i=0, i< n; i++) Push(S, arr[i]);} //Demo1(2) SeqStack S1, S2, tmp;DataType x;...//假设栈tmp和S2已做过初始化while ( ! StackEmpty (&S1)){x=Pop(&S1) ;Push(&tmp,x);}while ( ! StackEmpty (&tmp) ){x=Pop( &tmp);Push( &S1,x);Push( &S2, x);}(3) void Demo2( SeqStack *S, int m){ // 设DataType 为int 型SeqStack T; int i;InitStack (&T);while (! StackEmpty( S))if(( i=Pop(S)) !=m) Push( &T,i);while (! StackEmpty( &T)){i=Pop(&T); Push(S,i);}}(4)void Demo3( CirQueue *Q){ // 设DataType 为int 型int x; SeqStack S;InitStack( &S);while (! QueueEmpty( Q )){x=DeQueue( Q); Push( &S,x);}while (! StackEmpty( &s)){ x=Pop(&S); EnQueue( Q,x );}}// Demo3(5) CirQueue Q1, Q2; // 设DataType 为int 型int x, i , m = 0;... // 设Q1已有内容,Q2已初始化过while ( ! QueueEmpty( &Q1) ){ x=DeQueue( &Q1 ) ; EnQueue(&Q2, x); m++;}for (i=0; i< n; i++){ x=DeQueue(&Q2) ;EnQueue( &Q1, x) ; EnQueue( &Q2, x);}二、算法设计题3.6 回文是指正读反读均相同的字符序列,如"abba"和"abdba"均是回文,但"good"不是回文。
数据结构第3章 栈与队列习题

第3章栈与队列一、单项选择题1.元素A、B、C、D依次进顺序栈后,栈顶元素是,栈底元素是。
A.A B.BC.C D.D2.经过以下栈运算后,x的值是。
InitStack(s);Push(s,a);Push(s,b);Pop(s,x);GetTop(s,x);A.a B.bC.1 D.03.已知一个栈的进栈序列是ABC,出栈序列为CBA,经过的栈操作是。
A.push,pop,push,pop,push,pop B.push,push,push,pop,pop,popC.push,push,pop,pop,push,pop D.push,pop,push,push,pop,pop 4.设一个栈的输入序列为A、B、C、D,则借助一个栈所得到的序列是。
A.A,B,C,D B.D,C,B,AC.A,C,D,B D.D,A,B,C5.一个栈的进栈序列是a,b,c,d,e,则栈的不可能的输出序列是。
A.edcba B.decbaC.dceab D.abcde6.已知一个栈的进栈序列是1,2,3,……,n,其输出序列的第一个元素是i,则第j个出栈元素是。
A.i B.n-iC.j-i+1 D.不确定7.已知一个栈的进栈序列是1,2,3,……,n,其输出序列是p1,p2,…,Pn,若p1=n,则pi的值。
A.i B.n-iC.n-i+1 D.不确定8.设n个元素进栈序列是1,2,3,……,n,其输出序列是p1,p2,…,p n,若p1=3,则p2的值。
A.一定是2 B.一定是1C.不可能是1 D.以上都不对9.设n个元素进栈序列是p1,p2,…,p n,其输出序列是1,2,3,……,n,若p3=1,则p1的值。
A.可能是2 B.一定是1C.不可能是2 D.不可能是310.设n个元素进栈序列是p1,p2,…,p n,其输出序列是1,2,3,……,n,若p3=3,则p1的值。
A.可能是2 B.一定是2C.不可能是1 D.一定是111.设n个元素进栈序列是p1,p2,…,p n,其输出序列是1,2,3,……,n,若p n=1,则p i(1≤i≤n-1)的值。
数据结构第3章 栈和队列习题课
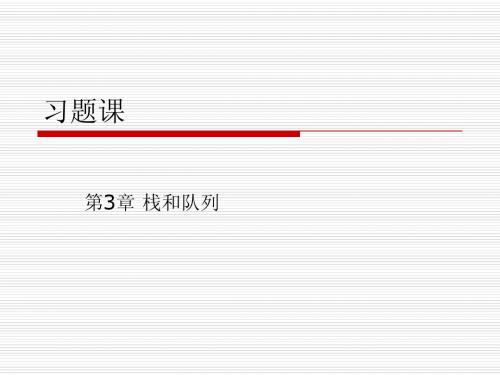
3.4简述以下算法的功能
(2) status algo2(Stack S , int e){ Stack T ; int d ; InitStack(T); while (! StackEmpty(S)){ Pop(S,d); if (d!=e) Push(T,d); } while(!Stackempty(T)){ Pop(T,d); Push(S,d); } }
习题课
第3章 栈和队列
3.1
(1)如果进站的车厢序列为123,则可能得到 的出站车厢序列是什么? (2)如果进站的车厢序列为123456,则能否 得到435612和135426出站序列,并请说 明为什么不能得到或如何得到。
(1) 123 , 132 , 213 , 231 , 321 (2) 435612不能得到(6出站时,12必须在站中,只能出站成21,无法 得到12) 135426可以 1S1X2S3S3X4S5S5X4X2X6S6X
3.28 带头指针的循环链表表示队列,并且 只设一个指针指向队尾结点,编写队列初始 化、入队列和出队列算法
typedef struct QNode{ QElemTypቤተ መጻሕፍቲ ባይዱ data; Struct Qnode *next; }QNode,*QueuePtr; typedef struct { QueuePtr rear; }LinkQueue;
3.4简述以下算法的功能
(1)status algol(Stack S){ int I,n,A[255]; n = 0; while (!Stackempty(S)) {n++;Pop(S,A[n]);} for (i=1 ; i<=n ; i++) Push(S,A[i]); }
《数据结构》习题集:第3章 栈和队列

《数据结构》习题集:第3章栈和队列数据结构课后练习题第3章栈和队列第3章栈和队列一、选择题1. 栈结构通常采用的两种存储结构是( A )。
A、顺序存储结构和链表存储结构B、散列和索引方式C、链表存储结构和数组D、线性链表结构和非线性存储结构 2. 设栈ST 用顺序存储结构表示,则栈ST 为空的条件是( B )A、ST.top-ST.base<>0B、ST.top-ST.base==0C、ST.top-ST.base<>nD、ST.top-ST.base==n 3. 向一个栈顶指针为HS 的链栈中插入一个s 结点时,则执行( B )A、HS->next=s;B、s->next=HS->next;HS->next=s;C、s->next=HS;HS=s; D、s->next=HS;HS=HS->next;4. 从一个栈顶指针为HS 的链栈中删除一个结点,用x 保存被删除结点的值,则执行(B )A 、x=HS;HS=HS->next;B 、HS=HS->next;x=HS->data;C 、x=HS->data;HS=HS->next;D 、s->next=Hs;Hs=HS->next; 5. 表达式a*(b+c)-d 的后缀表达式为( B )A、abcdd+-B、abc+*d-C、abc*+d-D、-+*abcd 6. 中缀表达式A-(B+C/D)*E 的后缀形式是( D )A、AB-C+D/E*B、ABC+D/E*C、ABCD/E*+-D、ABCD/+E*- 7. 一个队列的入列序列是1,2,3,4,则队列的输出序列是( B )A、4,3,2,1B、1,2,3,4C、1,4,3,2D、3,2,4,18. 循环队列SQ 采用数组空间SQ.base[0,n-1]存放其元素值,已知其头尾指针分别是front 和rear,则判定此循环队列为空的条件是( D ) A、Q.rear-Q.front==n B、Q.rear-Q.front-1==n C、Q.front==Q.rear D、Q.front==Q.rear+19. 循环队列SQ 采用数组空间SQ.base[0,n-1]存放其元素值,已知其头尾指针分别是front 和rear,则判定此循环队列为满的条件是( C ) A、Q.front==Q.rear B、Q.front!=Q.rear C、Q.front==(Q.rear+1)%n D、Q.front!=(Q.rear+1)%n10. 若在一个大小为6 的数组上实现循环队列,且当前rear 和front 的值分别为0 和3,当从队列中删除一个元素,再加入两个元素后,rear 和front 的值分别为( A ) A、1,5 B、2, 4 C、4,2 D、5,1 11. 用单链表表示的链式队列的队头在链表的( C )位置A、链头B、链尾C、链中12. 判定一个链队列Q(最多元素为n 个)为空的条件是( A )A、Q.front==Q.rearB、Q.front!=Q.rearC、Q.front==(Q.rear+1)%nD、Q.front!=(Q.rear+1)%n 13. 在链队列Q 中,插入s 所指结点需顺序执行的指令是( B )A 、Q.front->next=s;f=s;B 、Q.rear->next=s;Q.rear=s;C 、s->next=Q.rear;Q.rear=s; D 、s->next=Q.front;Q.front=s; 14. 在一个链队列Q 中,删除一个结点需要执行的指令是( C )1/7北京理工大学珠海学院计算机学院“数据结构”课程组编制 2021-3-1数据结构课后练习题第3章栈和队列A、Q.rear=Q.front->next;B、Q.rear->next=Q.rear->next->next;C、Q.front->next=Q.front->next->next; D、Q.front=Q.rear->next;15. 用不带头结点的单链表存储队列,其队头指针指向队头结点,队尾指针指向队尾结点,则在进行出队操作时(D )A、仅修改队头指针B、仅修改队尾指针C、队头尾指针都要修改D、队头尾指针都可能要修改。
数据结构第3章 栈与队列习题
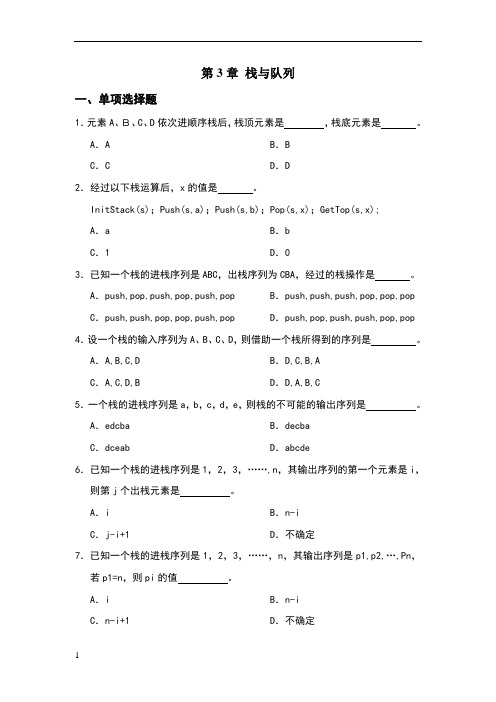
第3章栈与队列一、单项选择题1.元素A、B、C、D依次进顺序栈后,栈顶元素是,栈底元素是。
A.A B.BC.C D.D2.经过以下栈运算后,x的值是。
InitStack(s);Push(s,a);Push(s,b);Pop(s,x);GetTop(s,x);A.a B.bC.1 D.03.已知一个栈的进栈序列是ABC,出栈序列为CBA,经过的栈操作是。
A.push,pop,push,pop,push,pop B.push,push,push,pop,pop,pop C.push,push,pop,pop,push,pop D.push,pop,push,push,pop,pop 4.设一个栈的输入序列为A、B、C、D,则借助一个栈所得到的序列是。
A.A,B,C,D B.D,C,B,AC.A,C,D,B D.D,A,B,C5.一个栈的进栈序列是a,b,c,d,e,则栈的不可能的输出序列是。
A.edcba B.decbaC.dceab D.abcde6.已知一个栈的进栈序列是1,2,3,……,n,其输出序列的第一个元素是i,则第j个出栈元素是。
A.i B.n-iC.j-i+1 D.不确定7.已知一个栈的进栈序列是1,2,3,……,n,其输出序列是p1,p2,…,Pn,若p1=n,则pi的值。
A.i B.n-iC.n-i+1 D.不确定8.设n个元素进栈序列是1,2,3,……,n,其输出序列是p1,p2,…,pn,若p1=3,则p2的值。
A.一定是2 B.一定是1 C.不可能是1 D.以上都不对9.设n个元素进栈序列是p1,p2,…,pn,其输出序列是1,2,3,……,n,若p3=1,则p1的值。
A.可能是2 B.一定是1 C.不可能是2 D.不可能是310.设n个元素进栈序列是p1,p2,…,pn,其输出序列是1,2,3,……,n,若p 3=3,则p1的值。
A.可能是2 B.一定是2 C.不可能是1 D.一定是111.设n个元素进栈序列是p1,p2,…,pn,其输出序列是1,2,3,……,n,若p n =1,则pi(1≤i≤n-1)的值。
第3章 栈与队列习题参考答案

习题三参考答案备注: 红色字体标明的是与书本内容有改动的内容。
一、选择题1.在栈中存取数据的原则是( B )。
A.先进先出 B. 先进后出C. 后进后出D. 没有限制2.若将整数1、2、3、4依次进栈,则不可能得到的出栈序列是( D )。
A.1234 B. 1324 C. 4321 D. 14233.在链栈中,进行出栈操作时(B )。
A.需要判断栈是否满 B. 需要判断栈是否为空C. 需要判断栈元素的类型D. 无需对栈作任何差别4.在顺序栈中,若栈顶指针top指向栈顶元素的下一个存储单元,且顺序栈的最大容量是maxSize,则顺序栈的判空条件是( A )。
A.top==0 B.top==-1 C. top==maxSize D.top==maxSize-15.在顺序栈中,若栈顶指针top指向栈顶元素的下一个存储单元,且顺序栈的最大容量是maxSize。
则顺序栈的判满的条件是( C )。
A.top==0 B.top==-1 C. top==maxSize D.top==maxSize-16.在队列中存取数据元素的原则是( A )。
A.先进先出 B. 先进后出C. 后进后出D. 没有限制7.在循环顺序队列中,假设以少用一个存储单元的方法来区分队列判满和判空的条件,front和rear分别为队首和队尾指针,它们分别指向队首元素和队尾元素的下一个存储单元,队列的最大存储容量为maxSize,则队列的判空条件是(A )。
A.front==rear B. front!=rearC. front==rear+1D. front==(rear+1)% maxSize8.在循环顺序队列中,假设以少用一个存储单元的方法来区分队列判满和判空的条件,front和rear分别为队首和队尾指针,它们分别指向队首元素和队尾元素的下一个存储单元,队列的最大存储容量为maxSize,则队列的判满条件是(D )。
A.front==rear B. front!=rearC. front==rear+1D. front==(rear+1)% maxSize9.在循环顺序队列中,假设以少用一个存储单元的方法来区分队列判满和判空的条件,front和rear分别为队首和队尾指针,它们分别指向队首元素和队尾元素的下一个存储单元,队列的最大存储容量为maxSize,则队列的长度是(C )。
数据结构-第3章--栈和队列练习题.doc
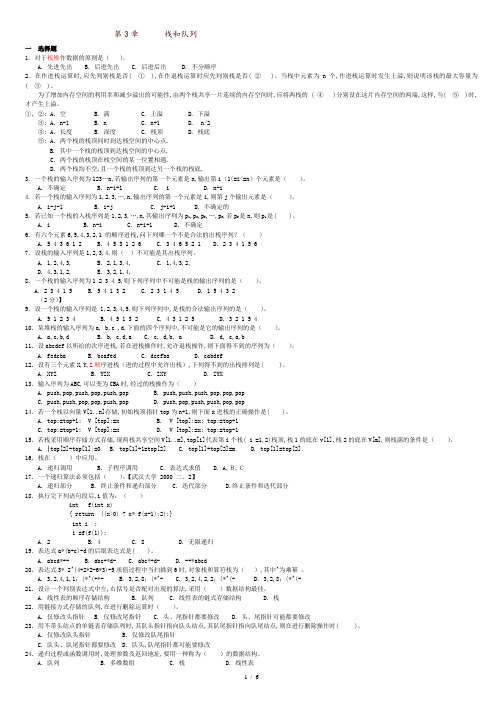
第3章栈和队列一选择题1. 对于栈操作数据的原则是()。
A. 先进先出B. 后进先出C. 后进后出D. 不分顺序2. 在作进栈运算时,应先判别栈是否( ① ),在作退栈运算时应先判别栈是否( ② )。
当栈中元素为n个,作进栈运算时发生上溢,则说明该栈的最大容量为( ③ )。
为了增加内存空间的利用率和减少溢出的可能性,由两个栈共享一片连续的内存空间时,应将两栈的 ( ④ )分别设在这片内存空间的两端,这样,当( ⑤ )时,才产生上溢。
①, ②: A. 空 B. 满 C. 上溢 D. 下溢③: A. n-1 B. n C. n+1 D. n/2④: A. 长度 B. 深度 C. 栈顶 D. 栈底⑤: A. 两个栈的栈顶同时到达栈空间的中心点.B. 其中一个栈的栈顶到达栈空间的中心点.C. 两个栈的栈顶在栈空间的某一位置相遇.D. 两个栈均不空,且一个栈的栈顶到达另一个栈的栈底.3. 一个栈的输入序列为123…n,若输出序列的第一个元素是n,输出第i(1<=i<=n)个元素是()。
A. 不确定B. n-i+1C. iD. n-i4. 若一个栈的输入序列为1,2,3,…,n,输出序列的第一个元素是i,则第j个输出元素是()。
A. i-j-1B. i-jC. j-i+1D. 不确定的5. 若已知一个栈的入栈序列是1,2,3,…,n,其输出序列为p1,p2,p3,…,p N,若p N是n,则p i是( )。
A. iB. n-iC. n-i+1D. 不确定6. 有六个元素6,5,4,3,2,1 的顺序进栈,问下列哪一个不是合法的出栈序列?()A. 5 4 3 6 1 2B. 4 5 3 1 2 6C. 3 4 6 5 2 1D. 2 3 4 1 5 67. 设栈的输入序列是1,2,3,4,则()不可能是其出栈序列。
A. 1,2,4,3,B. 2,1,3,4,C. 1,4,3,2,D. 4,3,1,2,E. 3,2,1,4,8. 一个栈的输入序列为1 2 3 4 5,则下列序列中不可能是栈的输出序列的是()。
《数据结构与算法(C++语言版)》第3章 栈与队列

例3-4
• 程序结构分为三层:上层为解释命令,中层包括执行上述 命令的操作模块,下层为几个服务模块,包括完成插入或 移出运算的子程序及一个送回当前队列长度的子程序。一 般发送和接收的信息多为字符,用不定长字符A和B分别表 示发送和接收的信息。因此,前面定义的队列qu中数组q的 基类型应为char *型。 • 发送模块算法如下。 • ① 计算当前队列长度l。 • ② 若队列有空(l<m−1)则:(a)要求用户输入A;(b )执行inq(qu, A)。(A插入队列中),否则算法结束。 • ③ 算法结束。
栈
• 在栈顶插入元素的操作通常称为入栈,删除栈顶元素的操 作称为出栈。除此之外,栈的基本操作还包括栈的初始化、 判空及取栈顶元素等。栈的抽象数据类型定义见以下ADT 。
栈
栈
• 栈的抽象类 • 程序给出了栈的抽象类定义,从面向对象的观点定义了栈 的属性、方法,后面将介绍栈的顺序存储和链式存储所对 应的类均是该抽象类的派生类。从基本操作上看,栈是线 性表的子集,故应当是线性表的父类。这样,线性表类也 可共享栈的方法。
数据结构与算法 语言版) (C++语言版) 语言版
第3章 栈与队列 章
栈
• 栈的定义 • 栈(stack)是限制在表的一端进行插入和删除的线性表, 又称为后进先出(last in first out)的线性表(简称LIFO结 构)。进行插入和删除的一端是浮动端,称为栈顶(top), 并用一个“栈顶指针”指示;另一端是固定端,称为栈底 (bottom)。如图所示,栈S=(k1, k2, …, kn),其中k1为栈底元素,kn 为栈顶元素。栈中元素按k1, k2, …, kn的次序进栈,退栈的第一个元素 为栈顶元素。
栈
栈
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
数据结构(C语言版)例题(第三章:栈和队列)(2008-05-09 12:33:13)转载▼◆3.15③假设以顺序存储结构实现一个双向栈,即在一维数组的存储空间中存在着两个栈,它们的栈底分别设在数组的的两个端点。
试编写实现这个双向栈tws的三个操作:初始化inistack(tws)、入栈push(tws,i,x)和出栈pop(tws,i,x)的算法, 其中i为0或1,用以分别指示设在数组两端的两个栈,并讨论按过程(正/误状态变量可设为变参)或函数设计这些操作算法各有什么优缺点。
实现下列3个函数:Status InitStack(TwoWayStack &tws, int size);Status Push(TwoWayStack &tws, int i, SElemType x);Status Pop(TwoWayStack &tws, int i, SElemType &x);双向栈类型定义如下:typedef struct {SElemType *elem;int top[2];int size; // 分配给elem的总容量}TwoWayStack; // 双端栈Status InitStack(TwoWayStack &tws, int size){tws.elem=(SElemType*)malloc(sizeof(SElemType)*size);tws.size=size;tws.top[0]=0; //haotws.top[1]=size-1; //以数组下标作为指针;return OK;}Status Push(TwoWayStack &tws, int i, SElemType x){int w=tws.top[0];if(w==tws.top[1]) return ERROR;else if(i==0){tws.elem[tws.top[0]]=x;tws.top[0]=tws.top[0]+1;}else if(i==1){tws.elem[tws.top[1]]=x;tws.top[1]=tws.top[1]-1;}return OK;}Status Pop(TwoWayStack &tws, int i, SElemType &x){ if(tws.top[1]==tws.size-1&&i==1) return ERROR;else if(tws.top[0]==0&&i==0) return ERROR;else if(i==0){tws.top[0]-=1;x=tws.elem[tws.top[0]];}else if(i==1){tws.top[1]+=1;x=tws.elem[tws.top[1]];}return x;}◆3.16②假设如题3.1所述火车调度站的入口处有n节硬席或软席车厢(分别以H和S表示)等待调度,试编写算法,输出对这n节车厢进行调度的操作(即入栈或出栈操作)序列,以使所有的软席车厢都被调整到硬席车厢之前。
实现下列函数:void SwitchYard(SqList train, char *s);顺序表类型定义如下:typedef struct {ElemType *elem;int length;int listsize;} SqList; // 顺序表void SwitchYard(SqList train, char *s){ int i,j=0,L;char *p;L=train.length;p=s;for(i=0;i<=L-1;i++){if(train.elem[i]=='H'){*(p++)='U';j++;}else{*p='U'; p++;*p='O'; p++;}}for(;j>0;j--){ *p='O';p++; }}◆3.19④假设一个算术表达式中可以包含三种括号:圆括号"(" 和")",方括号"["和"]"和花括号"{"和"}",且这三种括号可按任意的次序嵌套使用(如:…*…,…-…*…+…+…*…+…(…)…)。
编写判别给定表达式中所含括号是否正确配对出现的算法(已知表达式已存入数据元素为字符的顺序表中)。
实现下列函数:Status MatchCheck(SqList exp);顺序表类型定义如下:typedef struct {ElemType *elem;int length;int listsize;} SqList; // 顺序表Stack是一个已实现的栈。
可使用的相关类型和函数:typedef char SElemType; // 栈Stack的元素类型Status InitStack(Stack &s);Status Push(Stack &s, SElemType e);Status Pop(Stack &s, SElemType &e);Status StackEmpty(Stack s);Status GetTop(Stack s, SElemType &e);Status MatchCheck(SqList exp){ Stack s;char *p;SElemType c;InitStack(s);for(p=exp.elem;*p;p++){if(*p=='('||*p=='['||*p=='{') Push(s,*p);else if(*p==')'||*p==']'||*p=='}'){if(StackEmpty(s)) return FALSE;Pop(s,c);if(*p==')'&&c!='(') return FALSE;if(*p==']'&&c!='[') return FALSE;if(*p=='}'&&c!='{') return FALSE;}}if(!StackEmpty(s)) return FALSE;return TRUE;}◆3.20③假设以二维数组g(1..m,1..n)表示一个图像区域,g[i,j]表示该区域中点(i,j)所具颜色,其值为从0到k的整数。
编写算法置换点(i0,j0)所在区域的颜色。
约定和(i0,j0)同色的上、下、左、右的邻接点为同色区域的点。
实现下列函数:void ChangeColor(GTYPE g, int m, int n, char c, int i0, int j0);表示图像区域的类型定义如下:typedef char GTYPE[m+1][n+1];Stack是一个已实现的栈。
可使用的相关类型和函数:typedef int SElemType; // 栈Stack的元素类型Status StackInit(Stack &s, int initsize);Status Push(Stack &s, SElemType e);Status Pop(Stack &s, SElemType &e);Status StackEmpty(Stack s);Status GetTop(Stack s, SElemType &e);void ChangeColor(GTYPE g, int m, int n,char c, int i0, int j0) { int x,y,old;Stack s;old=g[i0][j0];StackInit(s,m*n*2);Push(s,i0);Push(s,j0);while(!StackEmpty(s)){Pop(s,y);Pop(s,x);if(x>1)if(g[x-1][y]==old){g[x-1][y]=c;Push(s,x-1);Push(s,y);}if(y>1)if(g[x][y-1]==old){g[x][y-1]=c;Push(s,x);Push(s,y-1);}if(xif(g[x+1][y]==old){g[x+1][y]=c;Push(s,x+1);Push(s,y);}if(yif(g[x][y+1]==old){g[x][y+1]=c;Push(s,x);Push(s,y+1);}}}◆3.21③假设表达式由单字母变量和双目四则运算算符构成。
试写一个算法,将一个通常书写形式且书写正确的表达式转换为逆波兰式。
实现下列函数:char *RPexpression_r(char *e);Stack是一个已实现的栈。
可使用的相关类型和函数:typedef char SElemType; // 栈Stack的元素类型Status InitStack(Stack &s);Status Push(Stack &s, SElemType e);Status Pop(Stack &s, SElemType &e);Status StackEmpty(Stack s);SElemType Top(Stack s);char *RPexpression_r(char *e){ static char b[20];char *a=b;char w3='k';char w;char w1,w2;Stack S;InitStack(S);while(*e!='\0'){switch(*e){case '+':if(!StackEmpty(S)){Pop(S,w2);Push(S,w2);if(w2=='*'||w2=='/'||w2=='+'||w2=='-') {Pop(S,w);*a=w;a++;}w2=Top(S);if(w2=='+'||w2=='-'){Pop(S,w);*a=w;a++;}Push(S,*e);} //if(!StackEmpty(S))else Push(S,*e);e++;break;case '-':if(!StackEmpty(S)){Pop(S,w2);Push(S,w2);if(w2=='*'||w2=='/'||w2=='+'||w2=='-') {Pop(S,w);*a=w;a++;}w2=Top(S);if(w2=='+'||w2=='-'){Pop(S,w);*a=w;a++;}Push(S,*e);} //if(!StackEmpty(S)) else Push(S,*e);e++;break;case '*':if(!StackEmpty(S)){Pop(S,w2);Push(S,w2);if(w2=='*'||w2=='/') {Pop(S,w);*a=w;a++;}Push(S,*e);} //if(!StackEmpty(S)) else Push(S,*e);e++;break;case '/':if(!StackEmpty(S)){Pop(S,w2);Push(S,w2);if(w2=='*'||w2=='/') {Pop(S,w);*a=w;a++;}Push(S,*e);} //if(!StackEmpty(S)) else Push(S,*e);e++;break;case '(':Push(S,*e);break;case ')':while(w3!='('&&!StackEmpty(S)){Pop(S,w3);if(w3!='('){*a=w3;a++;}} //whilew3='k';e++;break;default:*a=*e;a++;e++;break;} //switch(*e)} //while(*e!='\0')while(!StackEmpty(S)){Pop(S,w);*a=w;a++;}*a='\0';return b;}◆3.24③试编写如下定义的递归函数的递归算法:g(m,n) = 0 当m=0,n>=0g(m,n) = g(m-1,2n)+n 当m>0,n>=0并根据算法画出求g(5,2)时栈的变化过程。