二叉排序树的查找
二叉排序树
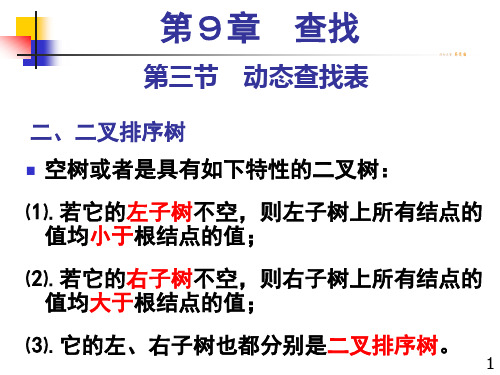
9
第9章
第三节
二、二叉排序树(插入)
查找
动态查找表
二叉排序树是一种动态查找表
当树中不存在查找的结点时,作插入操作
新插入的结点一定是叶子结点(只需改动一个 结点的指针) 该叶子结点是查找不成功时路径上访问的最后 一个结点的左孩子或右孩子(新结点值小于或 大于该结点值) 10
第9章
第三节
查找
19
在二叉排序树中查找关 键字值等于37,88,94
3
第9章
第三节
查找
动态查找表
二、二叉排序树(查找函数)中结点结构定义 二叉排序树通常采用二叉链表的形式进行存 储,其结点结构定义如下:
typedef struct BiNode { int data; BiNode *lChild, *rChild; }BiNode,*BitTree;
4
第9章
第三节
查找
动态查找表
2、二叉排序树的定义 定义二叉排序树所有用到的变量 BitTree root; int
//查找是否成功(1--成功,0--不成功) //查找位置(表示在BisCount层中的第几个位置
BisSuccess;
int
int
BisPos;
BisCount;
//查找次数(相当于树的层数)
7
第9章
第三节
查找
动态查找表
二、二叉排序树(查找函数)
else { BisSuccess = 0; root=GetNode(k);//查找不成功,插入新的结点}
} BiNode * GetNode(int k) { BiNode *s; s = new BiNode; s->data = k; s->lChild = NULL; s->rChild = NULL; return(s);}
数据结构-动态查找表
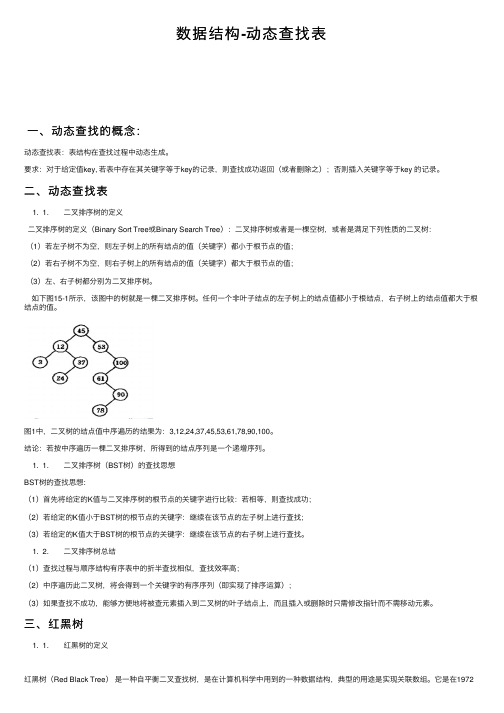
数据结构-动态查找表⼀、动态查找的概念:动态查找表:表结构在查找过程中动态⽣成。
要求:对于给定值key, 若表中存在其关键字等于key的记录,则查找成功返回(或者删除之);否则插⼊关键字等于key 的记录。
⼆、动态查找表1. 1. ⼆叉排序树的定义⼆叉排序树的定义(Binary Sort Tree或Binary Search Tree):⼆叉排序树或者是⼀棵空树,或者是满⾜下列性质的⼆叉树:(1)若左⼦树不为空,则左⼦树上的所有结点的值(关键字)都⼩于根节点的值;(2)若右⼦树不为空,则右⼦树上的所有结点的值(关键字)都⼤于根节点的值;(3)左、右⼦树都分别为⼆叉排序树。
如下图15-1所⽰,该图中的树就是⼀棵⼆叉排序树。
任何⼀个⾮叶⼦结点的左⼦树上的结点值都⼩于根结点,右⼦树上的结点值都⼤于根结点的值。
图1中,⼆叉树的结点值中序遍历的结果为:3,12,24,37,45,53,61,78,90,100。
结论:若按中序遍历⼀棵⼆叉排序树,所得到的结点序列是⼀个递增序列。
1. 1. ⼆叉排序树(BST树)的查找思想BST树的查找思想:(1)⾸先将给定的K值与⼆叉排序树的根节点的关键字进⾏⽐较:若相等,则查找成功;(2)若给定的K值⼩于BST树的根节点的关键字:继续在该节点的左⼦树上进⾏查找;(3)若给定的K值⼤于BST树的根节点的关键字:继续在该节点的右⼦树上进⾏查找。
1. 2. ⼆叉排序树总结(1)查找过程与顺序结构有序表中的折半查找相似,查找效率⾼;(2)中序遍历此⼆叉树,将会得到⼀个关键字的有序序列(即实现了排序运算);(3)如果查找不成功,能够⽅便地将被查元素插⼊到⼆叉树的叶⼦结点上,⽽且插⼊或删除时只需修改指针⽽不需移动元素。
三、红⿊树1. 1. 红⿊树的定义红⿊树(Red Black Tree)是⼀种⾃平衡⼆叉查找树,是在计算机科学中⽤到的⼀种数据结构,典型的⽤途是实现关联数组。
它是在1972年由Rudolf Bayer发明的,当时被称为平衡⼆叉B树(symmetric binary B-trees)。
二叉排序树
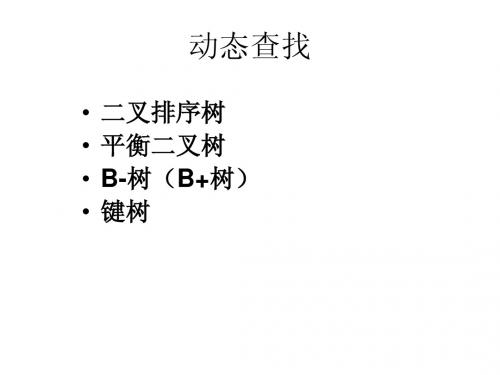
②若*p结点只有左子树,或只有右子树,则可将*p的左子 树或右子树直接改为其双亲结点*f的左子树,即: f->1child=p->1child(或f->1child=p->rchild); free(p); *f
F *p P P1
*f
F
*f
F *p P
*f
F
Pr
P1
Pr
③若*p既有左子树,又有右子树。则:
-1 0
47
-1
47
47
0
31 69
69
25
0
47
0
25
0
47
-1 0
31
0
69
0
40
69
40
69
0
25 76
40
76
(a)
AL、BL、BR 都是空树
(b) AL、BL、BR 都是非空树
LR型调整操作示意图
2
A
-1
0
C
AR C BL CL CR AR
0 0
B BL CL S
B
A
CR
(a) 插入结点*s后失去平衡
31
0 0 -1
31
0 1
28
0
25
0 0
47
0
25
-1
47
0
25
0
31
0
16 0
28
16
28
0
16 30
30
47
(c) LR(R)型调整
RL型调整操作示意图
A B C A BR CR B BR
AL
C
AL
CL CR
数据结构 二叉排序树
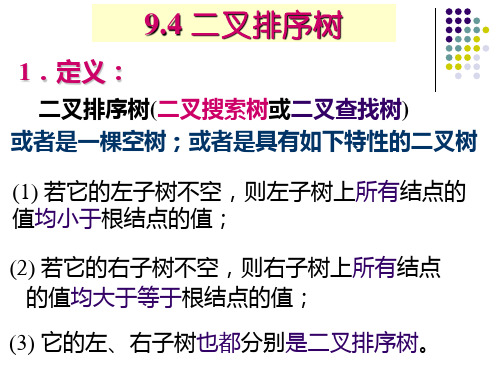
9.6.2 哈希函数的构造方法
构造哈希函数的目标:
哈希地址尽可能均匀分布在表空间上——均 匀性好; 哈希地址计算尽量简单。
考虑因素:
函数的复杂度; 关键字长度与表长的关系; 关键字分布情况; 元素的查找频率。
一、直接地址法 取关键字或关键字的某个线性函数值为哈希地址 即: H(key) = key 或: H(key) = a* key + b 其中,a, b为常数。 例:1949年后出生的人口调查表,关键字是年份 年份 1949 1950 1951 … 人数 … … … …
9.4 二叉排序树
1.定义:
二叉排序树(二叉搜索树或二叉查找树) 或者是一棵空树;或者是具有如下特性的二叉树
(1) 若它的左子树不空,则左子树上所有结点的 值均小于根结点的值;
(2) 若它的右子树不空,则右子树上所有结点 的值均大于等于根结点的值; (3) 它的左、右子树也都分别是二叉排序树。
例如:
H(key)
通常设定一个一维数组空间存储记录集合,则 H(key)指示数组中的下标。
称这个一维数组为哈希(Hash)表或散列表。 称映射函数 H 为哈希函数。 H(key)为哈希地址
例:假定一个线性表为: A = (18,75,60,43,54,90,46) 假定选取的哈希函数为
hash3(key) = key % 13
H(key) = key + (-1948) 此法仅适合于: 地址集合的大小 = = 关键字集合的大小
二、数字分析法
假设关键字集合中的每个关键字都是由 s 位数 字组成 (u1, u2, …, us),分析关键字集中的全体, 并从中提取分布均匀的若干位或它们的组合作为 地址。 例如:有若干记录,关键字为 8 位十进制数, 假设哈希表的表长为100, 对关键字进行分析, 取随机性较好的两位十进制数作为哈希地址。
二叉排序树

二叉排序树1.二叉排序树定义二叉排序树(Binary Sort Tree)或者是一棵空树;或者是具有下列性质的二叉树:(1)若左子树不空,则左子树上所有结点的值均小于根结点的值;若右子树不空,则右子树上所有结点的值均大于根结点的值。
(2)左右子树也都是二叉排序树,如图6-2所示。
2.二叉排序树的查找过程由其定义可见,二叉排序树的查找过程为:(1)若查找树为空,查找失败。
(2)查找树非空,将给定值key与查找树的根结点关键码比较。
(3)若相等,查找成功,结束查找过程,否则:①当给值key小于根结点关键码,查找将在以左孩子为根的子树上继续进行,转(1)。
②当给值key大于根结点关键码,查找将在以右孩子为根的子树上继续进行,转(1)。
3.二叉排序树插入操作和构造一棵二叉排序树向二叉排序树中插入一个结点的过程:设待插入结点的关键码为key,为将其插入,先要在二叉排序树中进行查找,若查找成功,按二叉排序树定义,该插入结点已存在,不用插入;查找不成功时,则插入之。
因此,新插入结点一定是作为叶子结点添加上去的。
构造一棵二叉排序树则是逐个插入结点的过程。
对于关键码序列为:{63,90,70,55,67,42,98,83,10,45,58},则构造一棵二叉排序树的过程如图6-3所示。
4.二叉排序树删除操作从二叉排序树中删除一个结点之后,要求其仍能保持二叉排序树的特性。
设待删结点为*p(p为指向待删结点的指针),其双亲结点为*f,删除可以分三种情况,如图6-4所示。
(1)*p结点为叶结点,由于删去叶结点后不影响整棵树的特性,所以,只需将被删结点的双亲结点相应指针域改为空指针,如图6-4(a)所示。
(2)*p结点只有右子树或只有左子树,此时,只需将或替换*f结点的*p子树即可,如图6-4(b)、(c)所示。
(3)*p结点既有左子树又有右子树,可按中序遍历保持有序地进行调整,如图6-4(d)、(e)所示。
设删除*p结点前,中序遍历序列为:① P为F的左子女时有:…,Pi子树,P,Pj,S子树,Pk,Sk子树,…,P2,S2子树,P1,S1子树,F,…。
二叉排序树
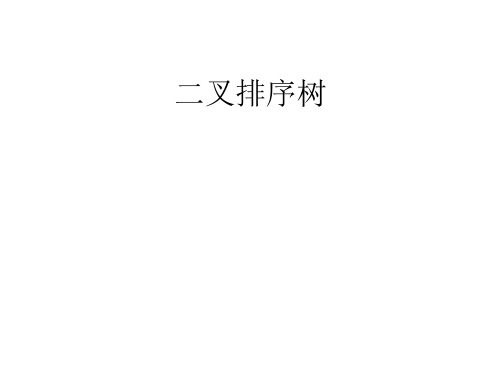
就维护表的有序性而言,二叉排序树无须移 动结点,只需修改指针即可完成插入和删 除操作,且其平均的执行时间均为O(lgn), 因此更有效。二分查找所涉及的有序表是 一个向量,若有插入和删除结点的操作, 则维护表的有序性所花的代价是O(n)。当 有序表是静态查找表时,宜用向量作为其 存储结构,而采用二分查找实现其查找操 作;若有序表里动态查找表,则应选择二 叉排序树作为其存储结构。
if(q->lchild) //*q的左子树非空,找*q的左子 树的最右节点r. {for(q=q->lchild;q->rchild;q=q->rchild); q->rchild=p->rchild; } if(parent->lchild==p)parent->lchild=p>lchild; else parent->rchild=p->lchild; free(p); /释放*p占用的空间 } //DelBSTNode
下图(a)所示的树,是按如下插入次序构成的: 45,24,55,12,37,53,60,28,40,70 下图(b)所示的树,是按如下插入次序构成的: 12,24,28,37,40,45,53,55,60,70
在二叉排序树上进行查找时的平均查找长度和二叉树的形态 有关: ①在最坏情况下,二叉排序树是通过把一个有序表的n 个结点依次插入而生成的,此时所得的二叉排序树蜕化为 棵深度为n的单支树,它的平均查找长度和单链表上的顺 序查找相同,亦是(n+1)/2。 ②在最好情况下,二叉排序树在生成的过程中,树的形 态比较匀称,最终得到的是一棵形态与二分查找的判定树 相似的二叉排序树,此时它的平均查找长度大约是lgn。 ③插入、删除和查找算法的时间复杂度均为O(lgn)。 (3)二叉排序树和二分查找的比较 就平均时间性能而言,二叉排序树上的查找和二分查找 差不多。
数据结构二叉排序树
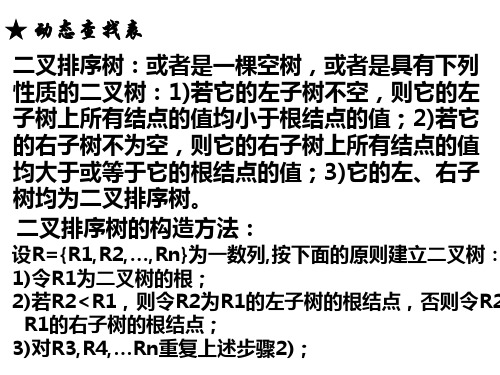
05
13
19
21
37
56
64
75
80
88
92
low mid high 因为r[mid].key<k,所以向右找,令low:=mid+1=4 (3) low=4;high=5;mid=(4+5) div 2=4
05
13
19
low
21
37
56
64
75
80
88
92
mid high
因为r[mid].key=k,查找成功,所查元素在表中的序号为mid 的值
平均查找长度:为确定某元素在表中某位置所进行的比 较次数的期望值。 在长度为n的表中找某一元素,查找成功的平均查找长度:
ASL=∑PiCi
Pi :为查找表中第i个元素的概率 Ci :为查到表中第i个元素时已经进行的比较次数
在顺序查找时, Ci取决于所查元素在表中的位置, Ci =i,设每个元素的查找概率相等,即Pi=1/n,则:
RL型的第一次旋转(顺时针) 以 53 为轴心,把 37 从 53 的左上转到 53 的左下,使得 53 的左 是 37 ;右是 90 ,原 53 的左变成了 37 的右。 RL型的第二次旋转(逆时针)
一般情况下,假设由于二叉排序树上插入结点而失去 平衡的最小子树的根结点指针为a(即a是离插入结点最 近,且平衡因子绝对值超过1的祖先结点),则失去平衡 后进行调整的规律可归纳为下列四种情况: ⒈RR型平衡旋转: a -2 b -1 h-1 a1
2.查找关键字k=85 的情况 (1) low=1;high=11;mid=(1+11) / 2=6
05
13
19
21
数据结构:第9章 查找2-二叉树和平衡二叉树

return(NULL); else
{if(t->data==x) return(t);
if(x<(t->data) return(search(t->lchild,x));
else return(search(t->lchild,x)); } }
——这种既查找又插入的过程称为动态查找。 二叉排序树既有类似于折半查找的特性,又采用了链表存储, 它是动态查找表的一种适宜表示。
注:若数据元素的输入顺序不同,则得到的二叉排序树形态 也不同!
讨论1:二叉排序树的插入和查找操作 例:输入待查找的关键字序列=(45,24,53,45,12,24,90)
二叉排序树的建立 对于已给定一待排序的数据序列,通常采用逐步插入结点的方 法来构造二叉排序树,即只要反复调用二叉排序树的插入算法 即可,算法描述为: BiTree *Creat (int n) //建立含有n个结点的二叉排序树 { BiTree *BST= NULL;
for ( int i=1; i<=n; i++) { scanf(“%d”,&x); //输入关键字序列
– 法2:令*s代替*p
将S的左子树成为S的双亲Q的右子树,用S取代p 。 若C无右子树,用C取代p。
例:请从下面的二叉排序树中删除结点P。
F P
法1:
F
P
C
PR
C
PR
CL Q
CL QL
Q SL
S PR
QL S
SL
法2:
F
PS
C
PR
CL Q
QL SL S SL
数据结构 -第12周查找第3讲-二叉排序树.pdf
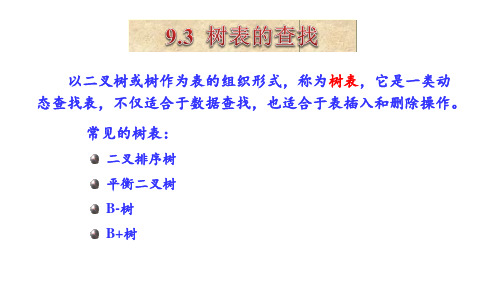
以二叉树或树作为表的组织形式,称为树表,它是一类动态查找表,不仅适合于数据查找,也适合于表插入和删除操作。
常见的树表:二叉排序树平衡二叉树B-树B+树9.3.1 二叉排序树二叉排序树(简称BST)又称二叉查找(搜索)树,其定义为:二叉排序树或者是空树,或者是满足如下性质(BST性质)的二叉树:❶若它的左子树非空,则左子树上所有节点值(指关键字值)均小于根节点值;❷若它的右子树非空,则右子树上所有节点值均大于根节点值;❸左、右子树本身又各是一棵二叉排序树。
注意:二叉排序树中没有相同关键字的节点。
二叉树结构满足BST性质:节点值约束二叉排序树503080209010854035252388例如:是二叉排序树。
66不试一试二叉排序树的中序遍历序列有什么特点?二叉排序树的节点类型如下:typedef struct node{KeyType key;//关键字项InfoType data;//其他数据域struct node*lchild,*rchild;//左右孩子指针}BSTNode;二叉排序树可看做是一个有序表,所以在二叉排序树上进行查找,和二分查找类似,也是一个逐步缩小查找范围的过程。
1、二叉排序树上的查找Nk< bt->keybtk> bt->key 每一层只和一个节点进行关键字比较!∧∧p查找到p所指节点若k<p->data,并且p->lchild=NULL,查找失败。
若k>p->data,并且p->rchild=NULL,查找失败。
查找失败的情况加上外部节点一个外部节点对应某内部节点的一个NULL指针递归查找算法SearchBST()如下(在二叉排序树bt上查找关键字为k的记录,成功时返回该节点指针,否则返回NULL):BSTNode*SearchBST(BSTNode*bt,KeyType k){if(bt==NULL||bt->key==k)//递归出口return bt;if(k<bt->key)return SearchBST(bt->lchild,k);//在左子树中递归查找elsereturn SearchBST(bt->rchild,k);//在右子树中递归查找}在二叉排序树中插入一个关键字为k的新节点,要保证插入后仍满足BST性质。
二叉树
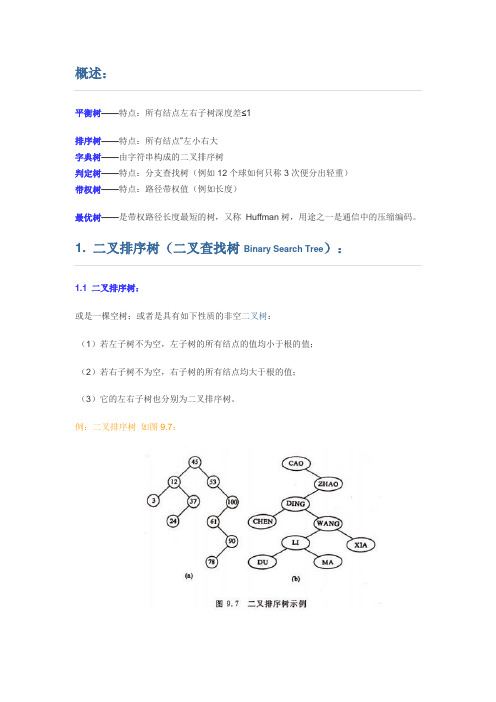
平衡树——特点:所有结点左右子树深度差≤1排序树——特点:所有结点―左小右大字典树——由字符串构成的二叉排序树判定树——特点:分支查找树(例如12个球如何只称3次便分出轻重)带权树——特点:路径带权值(例如长度)最优树——是带权路径长度最短的树,又称Huffman树,用途之一是通信中的压缩编码。
1.1 二叉排序树:或是一棵空树;或者是具有如下性质的非空二叉树:(1)若左子树不为空,左子树的所有结点的值均小于根的值;(2)若右子树不为空,右子树的所有结点均大于根的值;(3)它的左右子树也分别为二叉排序树。
例:二叉排序树如图9.7:二叉排序树的查找过程和次优二叉树类似,通常采取二叉链表作为二叉排序树的存储结构。
中序遍历二叉排序树可得到一个关键字的有序序列,一个无序序列可以通过构造一棵二叉排序树变成一个有序序列,构造树的过程即为对无序序列进行排序的过程。
每次插入的新的结点都是二叉排序树上新的叶子结点,在进行插入操作时,不必移动其它结点,只需改动某个结点的指针,由空变为非空即可。
搜索,插入,删除的复杂度等于树高,期望O(logn),最坏O(n)(数列有序,树退化成线性表).虽然二叉排序树的最坏效率是O(n),但它支持动态查询,且有很多改进版的二叉排序树可以使树高为O(logn),如SBT,AVL,红黑树等.故不失为一种好的动态排序方法.2.2 二叉排序树b中查找在二叉排序树b中查找x的过程为:1. 若b是空树,则搜索失败,否则:2. 若x等于b的根节点的数据域之值,则查找成功;否则:3. 若x小于b的根节点的数据域之值,则搜索左子树;否则:4. 查找右子树。
[cpp]view plaincopyprint?1.Status SearchBST(BiTree T, KeyType key, BiTree f, BiTree &p){2. //在根指针T所指二叉排序樹中递归地查找其关键字等于key的数据元素,若查找成功,3. //则指针p指向该数据元素节点,并返回TRUE,否则指针P指向查找路径上访问的4. //最好一个节点并返回FALSE,指针f指向T的双亲,其初始调用值为NULL5. if(!T){ p=f; return FALSE;} //查找不成功6. else if EQ(key, T->data.key) {P=T; return TRUE;} //查找成功7. else if LT(key,T->data.key)8. return SearchBST(T->lchild, key, T, p); //在左子树继续查找9. else return SearchBST(T->rchild, key, T, p); //在右子树继续查找10.}2.3 在二叉排序树插入结点的算法向一个二叉排序树b中插入一个结点s的算法,过程为:1. 若b是空树,则将s所指结点作为根结点插入,否则:2. 若s->data等于b的根结点的数据域之值,则返回,否则:3. 若s->data小于b的根结点的数据域之值,则把s所指结点插入到左子树中,否则:4. 把s所指结点插入到右子树中。
第四章二叉树_2
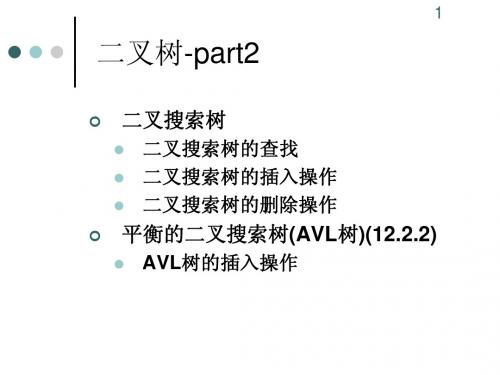
9
二叉搜索树插入操作
利用插入操作可以构造一棵二叉搜索树 首先给出结点序列: 首先给出结点序列 13、8、23、5、18、37 、 、 、 、 、
Φ13
8 5 5 18
83 18 5 2 37 23 37 37 18
10
template<class T> void BST<T>::insertNodeHelper(const T& el) { BSTNode<T> *p = root, *prev = 0; while (p != 0) { prev = p; if( p->data < el ) p = p->rchild; else p = p->lchild; } if( root == 0 ) root = new BSTNode<T>(el); else if( prev->data < el ) p = prev->rchild = new BSTNode<T>(el); else p = prev->lchild = new BSTNode<T>(el); } //find a place for inserting new node; //插入节点 插入节点
20
课堂练习
4.14 对于3个关键码值A、B、C,有多 少个不同的二叉搜索树?画出相应二叉 树。
21
平衡的二叉搜索树(AVL)
BST受输入顺序影响 受输入顺序影响
最好O(log n) 最好 最坏O(n) 最坏
怎样使得BST始终保持 始终保持O(log n)级的平衡状态? 级的平衡状态 怎样使得 始终保持 级的平衡状态? Adelson-Velskii和Landis发明了AVL树 发明了AVL Adelson-Velskii和Landis发明了AVL树
头歌二叉排序表的基本操作

头歌二叉排序表的基本操作一、概述二叉排序树,也称为二叉搜索树(Binary Search Tree, BST),是一种特殊的二叉树,其中每个节点都满足以下性质:对于任意节点,其左子树中所有节点的值都小于该节点的值,而其右子树中所有节点的值都大于该节点的值。
这种特性使得二叉排序树成为一种非常有效的数据结构,用于在各种算法中实现快速查找、插入和删除操作。
二、基本操作1. 插入操作插入操作是二叉排序树中最常用的操作之一。
其基本步骤如下:(1)创建一个新节点,并将要插入的值赋给该节点。
(2)如果二叉排序树为空,则将新节点作为根节点。
(3)否则,从根节点开始比较新节点的值与当前节点的值。
如果新节点的值小于当前节点的值,则将新节点插入到当前节点的左子树中;否则,将新节点插入到当前节点的右子树中。
(4)重复步骤3,直到找到一个空位置来插入新节点。
2. 删除操作删除操作是二叉排序树中比较复杂的操作之一。
其基本步骤如下:(1)找到要删除的节点。
如果找不到要删除的节点,则无法进行删除操作。
(2)如果找到要删除的节点,则将其从树中删除。
如果该节点只有一个子节点,则直接删除该节点;如果该节点有两个子节点,则可以选择将其中的一个子节点“提升”到该节点的位置,然后删除该子节点。
在提升子节点时,需要考虑子节点的值与要删除的节点的值之间的关系,以确保二叉排序树的性质不变。
(3)如果被提升的子节点仍然包含要删除的节点,则需要重复步骤2,直到找到要删除的节点并将其删除。
3. 查找操作查找操作用于在二叉排序树中查找指定的值。
其基本步骤如下:(1)从根节点开始,比较当前节点的值与要查找的值。
如果它们相等,则查找成功,返回当前节点的位置。
(2)如果当前节点的值大于要查找的值,则进入当前节点的左子树中进行查找;否则进入当前节点的右子树中进行查找。
(3)重复步骤2,直到找到要查找的值或者搜索路径上的所有节点都已访问过。
如果最终没有找到要查找的值,则返回空指针。
实验报告 实验三 二叉排序树的建立和查找
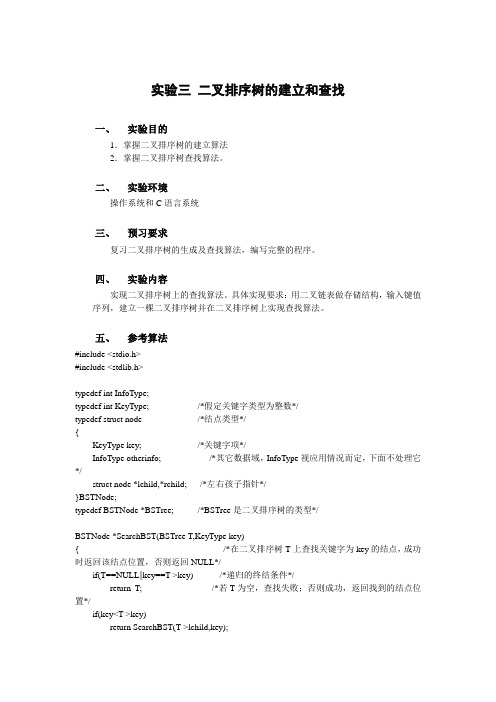
实验三二叉排序树的建立和查找一、实验目的1.掌握二叉排序树的建立算法2.掌握二叉排序树查找算法。
二、实验环境操作系统和C语言系统三、预习要求复习二叉排序树的生成及查找算法,编写完整的程序。
四、实验内容实现二叉排序树上的查找算法。
具体实现要求:用二叉链表做存储结构,输入键值序列,建立一棵二叉排序树并在二叉排序树上实现查找算法。
五、参考算法#include <stdio.h>#include <stdlib.h>typedef int InfoType;typedef int KeyType; /*假定关键字类型为整数*/typedef struct node /*结点类型*/{KeyType key; /*关键字项*/InfoType otherinfo; /*其它数据域,InfoType视应用情况而定,下面不处理它*/struct node *lchild,*rchild; /*左右孩子指针*/}BSTNode;typedef BSTNode *BSTree; /*BSTree是二叉排序树的类型*/BSTNode *SearchBST(BSTree T,KeyType key){ /*在二叉排序树T上查找关键字为key的结点,成功时返回该结点位置,否则返回NULL*/if(T==NULL||key==T->key) /*递归的终结条件*/return T; /*若T为空,查找失败;否则成功,返回找到的结点位置*/if(key<T->key)return SearchBST(T->lchild,key);elsereturn SearchBST(T->rchild,key); /*继续在右子树中查找*/}void InsertBST(BSTree *T,int key){ /*插入一个值为key的节点到二叉排序树中*/BSTNode *p,*q;if((*T)==NULL){ /*树为空树*/(*T)=(BSTree)malloc(sizeof(BSTNode));(*T)->key=key;(*T)->lchild=(*T)->rchild=NULL;}else{p=(*T);while(p){q=p;if(p->key>key)p=q->lchild;else if(p->key<key)p=q->rchild;else{printf("\n 该二叉排序树中含有关键字为%d的节点!\n",key);return;}}p=(BSTree)malloc(sizeof(BSTNode));p->key=key;p->lchild=p->rchild=NULL;if(q->key>key)q->lchild=p;elseq->rchild=p;}}BSTree CreateBST(void){ /*输入一个结点序列,建立一棵二叉排序树,将根结点指针返回*/BSTree T=NULL; /*初始时T为空树*/KeyType key;scanf("%d",&key); /*读入一个关键字*/while(key){ /*假设key=0是输入结束标志*/ InsertBST(&T,key); /*将key插入二叉排序树T*/scanf("%d",&key); /*读入下一关键字*/}return T; /*返回建立的二叉排序树的根指针*/ }void ListBinTree(BSTree T) /*用广义表示二叉树*/{if(T!=NULL){printf("%d",T->key);if(T->lchild!=NULL||T->rchild!=NULL){printf("(");ListBinTree(T->lchild);if(T->rchild!=NULL)printf(",");ListBinTree(T->rchild);printf(")");}}}void main(){BSTNode *SearchBST(BSTree T,KeyType key);void InsertBST(BSTree *Tptr,KeyType key);BSTree CreateBST();void ListBinTree(BSTree T);BSTree T;BSTNode *p;int key;printf("请输入关键字(输入0为结束标志):\n");T=CreateBST();ListBinTree(T);printf("\n");printf("请输入欲查找关键字:");scanf("%d",&key);p=SearchBST(T,key);if(p==NULL)printf("没有找到%d!\n",key);elseprintf("找到%d!\n",key);ListBinTree(p);printf("\n");}实验中出现的问题及对问题的解决方案输入数据时,总是不能得到结果,原因是在建立二叉树函数定义中,是对指针的值进行了修改。
数据结构_第9章_查找2-二叉树和平衡二叉树

F
PS
C
PR
CL Q
QL SL S SL
10
3
18
2
6 12
6 删除10
3
18
2
4 12
4
15
15
三、二叉排序树的查找分析
1) 二叉排序树上查找某关键字等于给定值的结点过程,其实 就是走了一条从根到该结点的路径。 比较的关键字次数=此结点的层次数; 最多的比较次数=树的深度(或高度),即 log2 n+1
-0 1 24
0 37
0 37
-0 1
需要RL平衡旋转 (绕C先顺后逆)
24
0
-012
13
3573
0
01
37
90
0 53 0 53
0 90
作业
已知如下所示长度为12的表:
(Jan, Feb, Mar, Apr, May, June, July, Aug, Sep, Oct, Nov, Dec)
(1) 试按表中元素的顺序依次插入一棵初始为空的二叉 排序树,画出插入完成之后的二叉排序树,并求其在 等概率的情况下查找成功的平均查找长度。
2) 一棵二叉排序树的平均查找长度为:
n i1
ASL 1
ni Ci
m
其中:
ni 是每层结点个数; Ci 是结点所在层次数; m 为树深。
最坏情况:即插入的n个元素从一开始就有序, ——变成单支树的形态!
此时树的深度为n ; ASL= (n+1)/2 此时查找效率与顺序查找情况相同。
最好情况:即:与折半查找中的判ห้องสมุดไป่ตู้树相同(形态比较均衡) 树的深度为:log 2n +1 ; ASL=log 2(n+1) –1 ;与折半查找相同。
常见基本数据结构——树,二叉树,二叉查找树,AVL树

常见基本数据结构——树,⼆叉树,⼆叉查找树,AVL树常见数据结构——树处理⼤量的数据时,链表的线性时间太慢了,不宜使⽤。
在树的数据结构中,其⼤部分的运⾏时间平均为O(logN)。
并且通过对树结构的修改,我们能够保证它的最坏情形下上述的时间界。
树的定义有很多种⽅式。
定义树的⾃然的⽅式是递归的⽅式。
⼀棵树是⼀些节点的集合,这个集合可以是空集,若⾮空集,则⼀棵树是由根节点r以及0个或多个⾮空⼦树T1,T2,T3,......,Tk组成,这些⼦树中每⼀棵的根都有来⾃根r的⼀条有向的边所连接。
从递归的定义中,我们发现⼀棵树是N个节点和N-1条边组成的,每⼀个节点都有⼀条边连接⽗节点,但是根节点除外。
具有相同⽗亲的节点为兄弟,类似的⽅法可以定义祖⽗和孙⼦的关系。
从节点n1到nk的路径定义为节点n1,n2,...,nk的⼀个序列,并且ni是ni+1的⽗亲。
这个路径的长是路径上的边数,即k-1。
每个节点到⾃⼰有⼀条长为0的路径。
⼀棵树从根到叶⼦节点恰好存在⼀条路径。
对于任意的节点ni,ni的深度为从根到ni的唯⼀路径长。
ni的⾼是从ni到⼀⽚叶⼦的最长路径的长。
因此,所有的树叶的⾼度都是0,⼀棵树的⾼等于它的根节点的⾼。
⼀棵树的深度总是等于它最深叶⼦的深度;该深度等于这棵树的⾼度。
树的实现实现树的⼀种⽅法可以是在每⼀个节点除数据外还要有⼀些指针,使得该节点的每⼀个⼉⼦都有⼀个指针指向它。
但是由于每个节点的⼉⼦树可以变化很⼤⽽且事先不知道,故在各个节点建⽴⼦节点的链接是不可⾏的,这样将会浪费⼤量的空间。
实际的做法很简单:将每个节点的所有⼉⼦都放在树节点的链表中。
下⾯是典型的声明:typedef struct TreeNode *PtrToNodestruct TreeNode{ ElementType Element; PtrToNode FirstChild; PtrToNode NextSibling}下⾯是⼉⼦兄弟表⽰法的图⽰:树的遍历及应⽤⼀个常见的使⽤是操作系统中的⽬录结构。
树、二叉树、查找算法总结

树、⼆叉树、查找算法总结树的定义形式化定义树:T={D,R }。
D是包含n个结点的有限集合(n≥0)。
当n=0时为空树,否则关系R满⾜以下条件:l 有且仅有⼀个结点d0∈D,它对于关系R来说没有前驱结点,结点d0称作树的根结点。
l 除根结点外,每个结点有且仅有⼀个前驱结点。
l D中每个结点可以有零个或多个后继结点。
递归定义树是由n(n≥0)个结点组成的有限集合(记为T)。
其中:l 如果n=0,它是⼀棵空树,这是树的特例;l 如果n>0,这n个结点中存在⼀个唯⼀结点作为树的根结点(root),其余结点可分为m (m≥0)个互不相交的有限⼦集T1、T2、…、Tm,⽽每个⼦集本⾝⼜是⼀棵树,称为根结点root的⼦树。
ð 树中所有结点构成⼀种层次关系!树的基本术语度结点的度:⼀个结点的⼦树的个数树的度:各节点的度的最⼤值。
通常将度为m的树成为m次树或m叉树结点分⽀结点:度不为0的结点(也称⾮终端结点)度为1的结点成为单分⽀结点,度为2的结点称为双分⽀结点叶结点:度为0的结点路径与路径长度路径:两个结点di和dj的结点序列(di,di1,di2,…,dj)。
其中<dx,dy>是分⽀。
路径长度:等于路径所通过的结点数⽬减1(即路径上的分⽀数⽬)结点的层次和树⾼度层次:根结点层次为1,它的孩⼦结点层次为2。
以此类推。
树的⾼度(深度):结点中的最⼤层次;有序树和⽆序树有序树:若树中各结点的⼦树是按照⼀定的次序从左向右安排的,且相对次序是不能随意变换的⽆序树:和上⾯相反森林只要把树的根结点删去就成了森林。
反之,只要给n棵独⽴的树加上⼀个结点,并把这n棵树作为该结点的⼦树,则森林就变成了⼀颗树。
树的性质性质1:树中的结点数等于所有结点的度数之和加1。
证明:树的每个分⽀记为⼀个度,度数和=分⽀和,⽽再给根节点加个分⽀性质2:度为m的树中第i层上⾄多有mi-1个结点(i≥1)。
性质3 ⾼度为h的m次树⾄多有个结点。
数据结构第20讲--二叉排序树--2018

二叉排序树
• 任一结点 > 其左子树的所有结点,并且
< 其右子树的所有结点;
结点的左、右子树,也是二叉排序树;
≠
5
5
左孩子 < 父亲 <右孩子 另外,与堆进行区分!
4 9 49
3 12 7 12
×√
二叉排序树
• 任一结点 > 其左子树,并且
parentp->llink = Null;
else
//若*p是父亲的右孩子
parentp->rlink = Null;
free(p); return 1; //释放空间,返回 }
//2.1 若*p只有右孩子,则让右孩子取代*p
if(p->llink==Null && p->rlink!=Null)
18
< 其右子树; • 重要性质:
中序遍历二叉排序树
10 5
73 68 99
递增序列
4 9 27
• 如何判断1棵二叉树
是否是二叉排序树?
8 25 41
-- 中序遍历,得到递增序列才是。 32 51
二叉排序树--存储结构
struct BinSearchNode; //结点类型声明 typedef struct BinSearchNode * PBinSearchNode; struct BinSearchNode //结点类型
q
p = *ptree; //p指向树根
p
q = p; //后续:q用于记录p的父亲
5
49
7 12
while( p != Null) //p指向当前(子)树的根
二叉排序树的递归查找算法
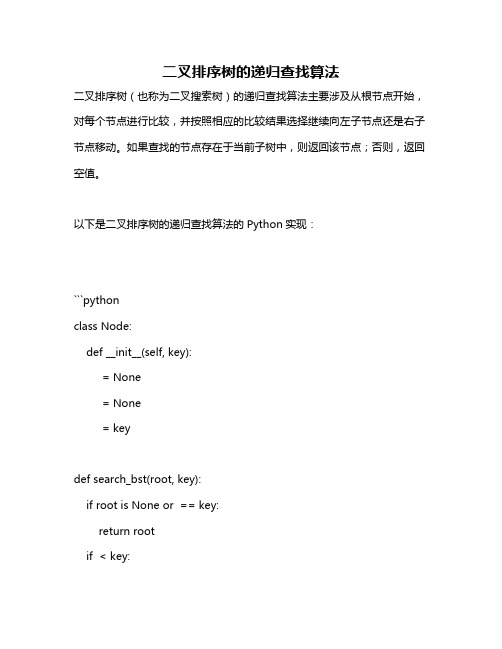
二叉排序树的递归查找算法
二叉排序树(也称为二叉搜索树)的递归查找算法主要涉及从根节点开始,对每个节点进行比较,并按照相应的比较结果选择继续向左子节点还是右子节点移动。
如果查找的节点存在于当前子树中,则返回该节点;否则,返回空值。
以下是二叉排序树的递归查找算法的Python实现:
```python
class Node:
def __init__(self, key):
= None
= None
= key
def search_bst(root, key):
if root is None or == key:
return root
if < key:
return search_bst(, key)
else:
return search_bst(, key)
```
在这个实现中,我们首先检查根节点是否为空或者是否等于要查找的键。
如果满足这些条件之一,我们返回根节点。
否则,我们比较根节点的值和要查找的键。
如果根节点的值小于要查找的键,我们递归地在右子树中查找。
否则,我们在左子树中查找。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
#include <stdlib.h>
#include <time.h>
#include <stdio.h>
#define INFMT "%d"
#define OUTFMT "%d "
/* #define NULL 0L */
#define BOOL int
#define TRUE 1
#define FALSE 0
#define LEN 10000
typedef int ElemType;
typedef struct BSTNode
{
ElemType data;
struct BSTNode *lchild, *rchild;
} BSTNode, *BSTree;
/* 插入新节点*/
void Insert(BSTree *tree, ElemType item)
{
BSTree node = (BSTree)malloc(sizeof(BSTNode)); node->data = item;
node->lchild = node->rchild = NULL;
if (!*tree)
*tree = node;
else
{
BSTree cursor = *tree;
while (1)
{
if (item < cursor->data)
{
if (NULL == cursor->lchild)
{
cursor->lchild = node;
}
cursor = cursor->lchild;
}
else
{
if (NULL == cursor->rchild)
{
cursor->rchild = node;
break;
}
cursor = cursor->rchild;
}
}
}
return;
}
/* 查找指定值*/
BSTree Search(BSTree tree, ElemType item) {
BSTree cursor = tree;
while (cursor)
{
if (item == cursor->data)
return cursor;
else if ( item < cursor->data)
cursor = cursor->lchild;
else
cursor = cursor->rchild;
}
return NULL;
}
void Inorder(BSTree tree)
{
BSTree cursor = tree;
if (cursor)
{
Inorder(cursor->lchild);
printf(OUTFMT, cursor->data); Inorder(cursor->rchild);
}
}
/* 回收资源*/
void Cleanup(BSTree tree)
{
BSTree cursor = tree, temp = NULL;
if (cursor)
{
Cleanup(cursor->lchild);
Cleanup(cursor->rchild);
free(cursor);
}
}
/* 产生一组随机数*/
void randnum(int *a, int s)
{
int i, j, mod = s * 10;
srand(time(NULL));
for (i = 0; i < s; ++i)
{
a[i] = rand() % mod + 1;
for (j = 0; j < i; ++j)
{
if (a[i] == a[j])
{
a[i] = rand() % mod + 1;
j = -1;
continue;
}
}
}
}
void main()
{
ElemType item;
char choice;
BSTree root = NULL, ret; /* 必须赋予NULL值,否则出错*/ BOOL finish = FALSE;
printf("***欢迎使用二叉排序树演示程序***\n\n");
printf("请选择创建树的方式:\n");
printf("1. 手动输入数据创建二叉排序树\n");
printf("2. 自动产生数据创建二叉排序树\n");
do
{
scanf("%c", &choice);
getchar();
if (choice == '1' || choice == '2')
finish = TRUE;
} while (FALSE == finish);
switch (choice)
{
case '1':
{
printf("请输入数据(-10000结束):\n");
while (1)
{
scanf(INFMT, &item);
if (-10000 != item)
Insert(&root, item);
else
break;
}
break;
}
case '2':
{
int ia[LEN], i = 0, loop = LEN; randnum(ia, LEN);
while (loop--)
{
Insert(&root, ia[i++]);
}
break;
}
}
printf("\n\n创建完成...\n"); Inorder(root);
printf("\n\n");
/* 二叉排序树的查找测试*/
do
{
printf("\n请输入查找数据:"); scanf("%d", &item);
getchar();
printf("Searching...\n");
ret = Search(root, item);
if (NULL == ret)
printf("查找失败!");
else
printf("查找成功!");
printf("\n继续测试按y,退出按其它键。
\n"); choice = getchar();
} while (choice=='y'||choice=='Y');
Cleanup(root);
}。