二值图像连通区域标记
二值图像连通区域标记

⼆值图像连通区域标记这⾥列举⼆值图像连通域标记算法包括直接扫描标记算法和⼆值图像连通域标记快速算法⼀、直接扫描标记算法把连续区域作同⼀个标记,常见的四邻域标记算法和⼋邻域标记算法。
1、四邻域标记算法:1)判断此点四邻域中的最左,最上有没有点,如果都没有点,则表⽰⼀个新的区域的开始。
2)如果此点四邻域中的最左有点,最上没有点,则标记此点为最左点的值;如果此点四邻域中的最左没有点,最上有点,则标记此点为最上点的值。
3)如果此点四邻域中的最左有点,最上都有点,则标记此点为这两个中的最⼩的标记点,并修改⼤标记为⼩标记。
2、⼋邻域标记算法:1)判断此点⼋邻域中的最左,左上,最上,上右点的情况。
如果都没有点,则表⽰⼀个新的区域的开始。
2)如果此点⼋邻域中的最左有点,上右都有点,则标记此点为这两个中的最⼩的标记点,并修改⼤标记为⼩标记。
3)如果此点⼋邻域中的左上有点,上右都有点,则标记此点为这两个中的最⼩的标记点,并修改⼤标记为⼩标记。
4)否则按照最左,左上,最上,上右的顺序,标记此点为四个中的⼀个。
代码实现:273. 功能说明:将所选出的等价关系,attach到list上⾥274. 参数说明:275. pEqualMark 等价关系276. num1 新的等价关系1277. num2 新的等价关系2278. nEqualNum 等价数组的个数279. plEqualMark 存放等价数组的list280. 返回值:⽆281. */282. template<typename elemType> void AttachEqualMark(EqualMark &pEqualMark,elemType num1, elemType num2, int & pEqualNum, std 283. {284. //num1⼩的情况285. if ( num1 < num2 )286. {287. if ( pEqualMark.MarkValue1 != num1288. || pEqualMark.MarkValue2 != num2 )289. {290. pEqualMark.MarkValue1=num1;291. pEqualMark.MarkValue2=num2;292. //插⼊到数组中293. pEqualNum++;294. plEqualMark.push_back(pEqualMark);295. }296. }297. //num2⼩的情况298. else299. {300. if ( pEqualMark.MarkValue2 != num1301. || pEqualMark.MarkValue1 != num2 )302. {303. pEqualMark.MarkValue1=num2;304. pEqualMark.MarkValue2=num1;305. //插⼊到数组中306. pEqualNum++;307. plEqualMark.push_back(pEqualMark);308. }309. }310.311. }312.313. /*314. 功能说明:快速⼆值图像连通域标记315. 参数说明:lpImgBits,表⽰图象数据区指针316. nMarkNumbers,表⽰标记数量317. iColorType,表⽰被标记颜⾊的值(,)318. nImageWidth,表⽰图象的宽319. nImageHeight,表⽰图象的⾼320. 返回值:BOOL类型,TRUE,表⽰成功;FLASE,表⽰失败321. */322. BOOL MarkImage(BYTE * lpImgBits,int & nMarkNumbers,int iColorType,long nImageWidth,long nImageHeigt,std::list< MarkRegion> &lis 323. {324. BYTE * lpImgBitsMove=NULL;//lpImgBitsMove,表⽰图象数据区偏移指针325. int * lpMark= NULL;//lpMark,表⽰标记数据指针326. int * lpMarkMove = NULL;//lpMarkMove,表⽰标记数据偏移指针327. //iColorType为⽬标的图像值328. long lSize = nImageWidth*nImageHeigt;329. lpMark= new int[lSize+1];330. lpMarkMove=lpMark;331. ::memset(lpMark,0,(lSize+1)*sizeof(int));332.333. int nMarkValue=1;334. /* 每次标识的值,nMarkValue会在后边递增,335. 来表⽰不同的区域,从开始标记。
基于FPGA的二值图像连通域快速标记

基于FPGA的二值图像连通域快速标记汪滴珠;安涛;何培龙【摘要】针对连通域标记算法运算量大、速度慢、硬件实现困难的缺点,提出一种适于现场可编程逻辑门阵列(FPGA)实现的二值图像连通域快速标记的算法,并用VHDL硬件开发语言在XILINX公司的FPGA上实现.实验结果表明了该算法能对二值图像复杂的连通关系正确标记,易于硬件实现,大大节约了硬件资源,电路结构简单,满足实时性要求.%In order to solve the prablems of low speed large computation and difficult hardware implementation of connected component labeling, a connected component fast labcling algorithm of binary image lageling applicable for field programmable gate array (FPGA) is proposed, which is implemented by VHDI. hardware description language based on FPGA platform of XILINX corporation. Experimental results show that the proposed algorithm can label binary image with complex connections correctly, implement hardware easily,save more hardware resource and meet real-time demands.【期刊名称】《现代电子技术》【年(卷),期】2011(034)008【总页数】3页(P115-117)【关键词】FPGA;二值图像;连通域;快速标记【作者】汪滴珠;安涛;何培龙【作者单位】中国科学院,光电技术研究所,四川,成都,610209;中国科学院研究生院,北京,100039;中国科学院,光电技术研究所,四川,成都,610209;中国科学院,光电技术研究所,四川,成都,610209【正文语种】中文【中图分类】TN919-34;TP391连通域标记算法是图像处理、计算机视觉和模式识别等领域的基本算法,它可以对图像中不同目标标上不同的标记,进而提取、分离目标,确定目标的特征和参数,从而对目标进行识别和跟踪。
一种二值图像连通区域标记的新算法

第2 7卷 第 1 期 1
20 0 7年 1 1月
文 章编 号 :0 1— 0 12 0 ) 1— 7 6— 2 10 9 8 ( 07 1 27 0
一
计 算机应 用
Co u e p i ain mp trAp lc t s o
Vo . 127 No. 1 】 No .2 7 v o0
Ke o d : on c drg n aen ;l elbl g e o rwn yw rs cn et i ;l l g i e n ;rg ngo g e eo b i n a i i i
0 引 言
灰度 图像进行 阈值分割得到二值 图像 ( 一般背 景像 素为
25 目标像 素为 0 , 了提取不 同区域的特 征 , 常先要 对 5, )为 常
GAO n ~ o W ANG e — i Ho g b , W ixng
( colfEet n n i e n,U &  ̄ yo l t nc c ne Tcnl yo C i , Sho o l r iE gn r g n e e r iSi c & eho g hn co c ei fE c o e o f a
C e g u S h n6 0 5 ,C i ) h n d i n 1 0 4 hn ca a
Ab ta t s r c :A e loih b s d o ie l b l g a d rg o — r w n s p o o e o i ay c n e td c mp n n n w ag r m a e n l — ei n e i n g o i g wa r p s d f r bn r o n ce o o e t t n a n
关 键词 : 连通 区域 ; 标记 ; 线标记 ; 区域增 长
二值化图像8联通域标记
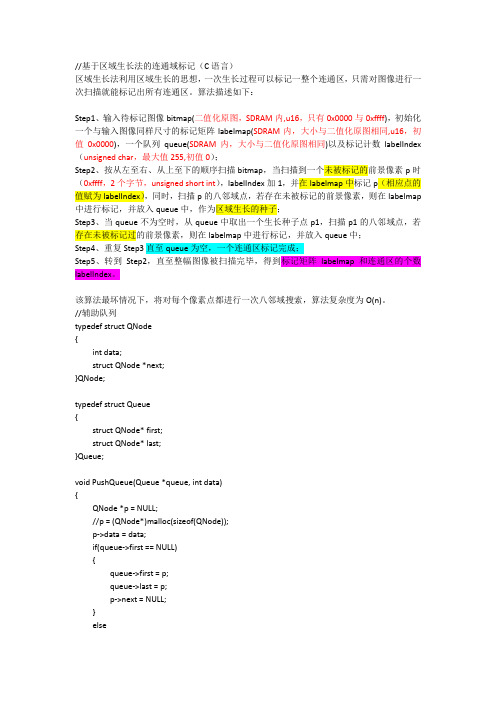
区域生长法利用区域生长的思想,一次生长过程可以标记一整个连通区,只需对图像进行一次扫描就能标记出所有连通区。算法描述如下:
Step1、输入待标记图像bitmap(二值化原图,SDRAM内,u16,只有0x0000与0xffff),初始化一个与输入图像同样尺寸的标记矩阵labelmap(SDRAM内,大小与二值化原图相同,u16,初值0x0000),一个队列queue(SDRAM内,大小与二值化原图相同)以及标记计数labelIndex(unsigned char,最大值255,初值0);
{
int searchIndex, i, length;
labelmap[pixelIndex] = labelIndex;
length = width * height;
for(i = 0;i < 8;i++)
{
searchIndex = pixelIndex + NeighborDirection[i][0] * width + NeighborDirection[i][1];//???
}
}
}
//头步骤1
int ConnectedComponentLabeling(unsigned char *bitmap, int width, int height, int *labelmap)
{
int cx, cy, index, popIndex, labelIndex = 0;
Queue *queue = NULL;
popIndex = PopQueue(queue);
}
}
}
}
//free(queue);
二值图像的贴标签算法

⼆值图像的贴标签算法 贴标签就是将⼆值图中属于同⼀个连通域的像素标记起来。
之前编的程序需要多次遍历图像,所以速度⽐较慢,最近有朋友告诉我⼀种更简单的⽅法。
这⾥对⼆值图中⽩⾊的连通域进⾏贴标签,具体步骤是: 1、按⾏遍历图像,当遇到⼀个⽩点时,说明遇到了⼀个标签区域; 2、将当前⽩点的坐标作为种⼦点⼊栈; 3、判断栈是否为空,若栈⾮空,则在栈顶元素所在位置贴上对应的标签号,同时将⼆值图上的该位置赋成别的颜⾊(表明当前元素已经贴过标签),弹出栈顶元素,并将其8邻域的⽩点⼊栈,重复3直到栈空,这时,当前连通域已经完成贴标签过程; 4、继续按⾏遍历图像,直到遇到下⼀个⽩点,然后重复步骤2-3,直到遍历完图像;1////////////////////////////////////////////////////2// 功能:⼆值图贴标签(针对感兴趣区域)3// 参数:4// pBin - ⼆值图像5// pLabel - 标签矩阵(在外申请,和图像同样⼤⼩,并全部置0)6// lineByte - 图像的每⾏字节数7// roi - 感兴趣区域(左闭右开、上闭下开区间)8// 返回值:9// nLabel - 标签个数10///////////////////////////////////////////////////1112int Labeling(unsigned char *pBin, int *pLabel, int lineByte, CRect roi)13 {14//感兴趣区域的4条边界15int xMin = roi.left;16int xMax = roi.right;17int yMin = roi.top;18int yMax = roi.bottom;1920 stack<CPoint> stk;21int i,j,x,y,k;22int nLabel = 0; //标签编号(从1号标签贴起,⾮标签区域贴0)23for (y=yMin; y<yMax; y++)24 {25for (x=xMin; x<xMax; x++)26 {27if (pBin[y*lineByte+x]==255) //以找到的第⼀个⽩点为种⼦点28 {29 nLabel++;30 stk.push(CPoint(x,y)); //将种⼦点⼊栈31 pLabel[y*lineByte+x] = nLabel;32 pBin[y*lineByte+x] = nLabel*40;3334//若栈⾮空,弹出⼀个元素,并将其8邻域内的⽩点⼊栈,栈空时当前连通域完成贴标签35while (!stk.empty())36 {37 CPoint pt = stk.top();38 stk.pop();39 i = pt.y;40 j = pt.x;41 k = i*lineByte+j;42if (i>yMin && j>xMin && pBin[k-lineByte-1]==255) //左上43 {44 stk.push(CPoint(j-1,i-1));45 pLabel[k-lineByte-1] = nLabel;46 pBin[k-lineByte-1] = nLabel*40;47 }48if (i>yMin && pBin[k-lineByte]==255) //上49 {50 stk.push(CPoint(j,i-1));51 pLabel[k-lineByte] = nLabel;52 pBin[k-lineByte] = nLabel*40;53 }54if (i>yMin && j<xMax-1 && pBin[k-lineByte+1]==255) //右上55 {56 stk.push(CPoint(j+1,i-1));57 pLabel[k-lineByte+1] = nLabel;58 pBin[k-lineByte+1] = nLabel*40;59 }60if (j>xMin && pBin[k-1]==255) //左61 {62 stk.push(CPoint(j-1,i));63 pLabel[k-1] = nLabel;64 pBin[k-1] = nLabel*40;65 }66if (j<xMax-1 && pBin[k+1]==255) //右67 {68 stk.push(CPoint(j+1,i));69 pLabel[k+1] = nLabel;70 pBin[k+1] = nLabel*40;71 }72if (i<yMax-1 && j>xMin && pBin[k+lineByte-1]==255) //左下73 {74 stk.push(CPoint(j-1,i+1));75 pLabel[k+lineByte-1] = nLabel;76 pBin[k+lineByte-1] = nLabel*40;77 }78if (i<yMax-1 && pBin[k+lineByte]==255) //下79 {80 stk.push(CPoint(j,i+1));81 pLabel[k+lineByte] = nLabel;82 pBin[k+lineByte] = nLabel*40;83 }84if (i<yMax-1 && j<xMax-1 && pBin[k+lineByte+1]==255) //右下85 {86 stk.push(CPoint(j+1,i+1));87 pLabel[k+lineByte+1] = nLabel;88 pBin[k+lineByte+1] = nLabel*40;89 }90 }91 }92 }93 }9495return nLabel;96 }。
matlab 二维坐标数组求解连通区域-概述说明以及解释

matlab 二维坐标数组求解连通区域-概述说明以及解释1.引言概述部分的内容可以如下编写:1.1 概述在数字图像处理和计算机视觉领域中,连通区域是常见的概念,它代表了具有相同像素值或特定属性的像素的集合。
本文将主要介绍使用MATLAB对二维坐标数组进行连通区域的求解方法。
二维坐标数组是一种常见的数据结构,用于存储和表示二维平面上的图像、地理信息等。
连通区域的求解在许多应用中都具有重要意义。
例如,在图像处理中,我们经常需要对目标进行分割和提取,而连通区域的求解可以帮助我们实现这一目标。
此外,在计算机视觉领域,连通区域的应用也非常广泛,如对象识别、目标跟踪等。
在正文部分,我们将首先介绍二维坐标数组的定义和特点,包括如何表示和访问数组中的元素。
然后,我们将详细解释连通区域的概念和应用,以及常见的连通区域求解算法和技术。
最后,在结论部分,我们将总结本文所介绍的二维坐标数组求解连通区域的方法,并给出相关实验结果和分析。
通过本文的阅读,读者将能够了解和掌握使用MATLAB对二维坐标数组进行连通区域求解的方法和技巧,从而在实际应用中能够灵活运用和扩展相关算法。
希望本文能够对读者在数字图像处理和计算机视觉领域的学习和研究工作有所帮助。
文章结构部分可以根据文章的主要内容和逻辑,介绍文章的主要章节和各个章节的内容概要。
下面是1.2 文章结构部分的内容示例:1.2 文章结构本文将按照以下结构进行叙述:第二部分:正文本部分主要介绍了二维坐标数组的定义和特点,并深入探讨了连通区域的概念和应用。
首先,我们将对二维坐标数组进行详细的定义,并解释其在实际问题中的应用。
其次,我们将介绍连通区域的概念和特点,并展示其在图像处理、地理信息系统等领域的广泛应用。
第三部分:结论本部分将重点讨论二维坐标数组求解连通区域的方法,并对实验结果进行分析。
我们将介绍一种有效的算法,基于二维坐标数组的特点,实现连通区域的快速求解。
同时,我们将通过实验结果验证该算法的准确性和效率,并分析不同参数对算法性能的影响。
基于递归的二值图像连通域像素标记算法
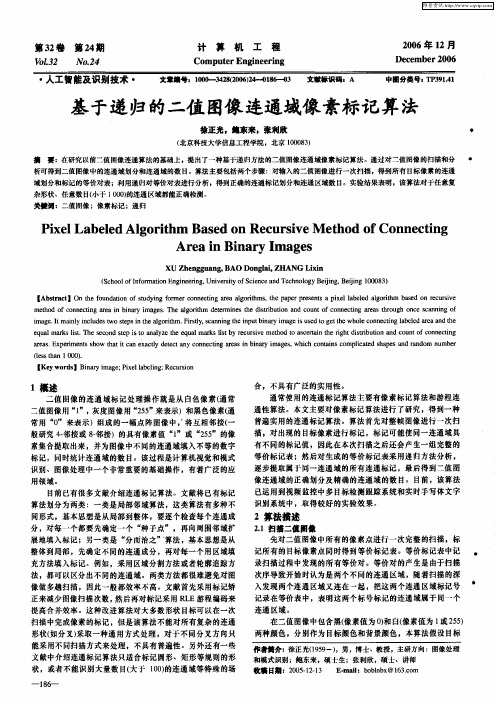
常 用 “ ” 来表示)组成 的一 幅点阵 图像 中 , 0 将互相邻接( 一 般研究 4邻接或 8邻 接)的具 有像素值 “ ”或 “ 5 ”的像 - 一 1 25
合 ,不具有广泛的实用性 。
通 常使 用的连通标记算法主 要有像 素标记算法和游程连 通 性算法。本文主要对像素标记 算法 进行 了研究 ,得到一种 普遍实用的连通标记算法 。算法 首先对整帧 图像进行一 次扫
me o fc n e t e n bn r m a e ,Th lor m eemi st ed srb t n a d c u to o n c n e str ug n e s a n n f h t d o o n ci a ai iay i g s ng r e ag i h t d tr ne iti u o n o n fc n e t g a a o h o c c n i g o h i i r h i g I il n lde wo se nteag rtm . rt , c nn eipu iay i g su e og th oec n e tn a ee e n e ma e.t manyicu st tpsi lo h Fisl s a igt n t n r ma ei s dt e ewh l o n cig lb lda aa dt h i y n h b t r h e u l ak it Th e on tp i o a ay e tee a rksls y rc riem e o o a c r i e rg tds iu in a d c u t fc nn cig q a r sl . es c d se st lz qu lma itb e u sv t d t s et nt h it b to n o n o e t m s n h h a h i r o n
基于区域生长法提取二值图像中的连通区域

左下 、 8 相邻像素 , 左 个 如图 2 示。 所
p X
图1 P 的四邻域
像 中提取连通分量的过程 实际上也是标记连通 区域 的过程 。 二值 图像 的连通域标记处理就是 从 白色像 素( 通常二值 图
像用 “ ” 灰度 图像 用“ 5 ” l, 2 5 来表示)和黑色像 素( 通常用 … 来 0’
计算机 时代 2 1 年 第 6 02 期
・ 3・ 2
基于区域生长法提取二值图像 中的连通区域
聂欢 欢 ,伊 磊 。刘任 平 ( 方工业 大学 ,北 京 10 4 ) 北 0 1 4
摘 要 :标记 二值 图像连 通区域是 图像 处理过 程的基本 算法 , 器视 觉和模 式识 别 中常用此 方法提 取 目标和分析 目标 机 几何特征。文章 以人机交互方式获得初 始种 子点 , 增强种子点的可靠性 , 通过 区域 生长 法提取二值 图像 中的连通 区域。
t xrc bet ad aay e terg o tc fa rs h e se on s o tie ho g u ncmp tr it at n i re o o e t tojcs n n lz i eme i et e.T ed p iti band tru h h ma—o ue ne c o n odrt a h r u r i
1像 素 间的连 通性
逐个检查每个连通成分 , 对每一个都要 先确定一个“ 子 种 像 素间的连通性是这样 定义的 : s 令 代表 一幅 图像 中的像 整体 , , 另一类 是 “ 分而 治之 ” 算 素 子集 , 如果在 s 中全部像素之问存在一个通路 , 就称 2 个像 素 点 ” 再 向周 围邻域 扩展 地填入 标记 ; 法, 基本思想是从整体到局部 , 先确定不 同的连通成分 , 再对每 P和 Q在 s中是连 通的 。像 素问的连通 性是确定 区域 的一 个
一种二值图像连通区域标记的新方法
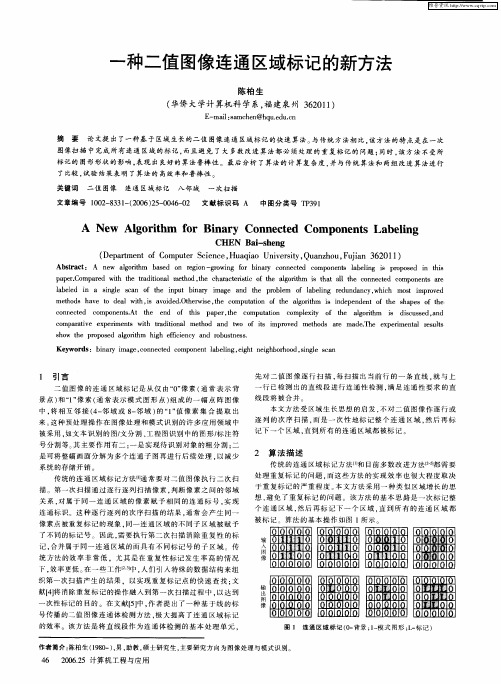
一
种二值 图像 连通 区域标 记的新方法
陈柏 生
( 华侨 大 学计算 机科 学 系, 建泉 州 32 1 ) 福 60 1
E mal s mc e @h ue u c — i: a h n q .d .n
摘
要 论 文 提 出 了一 种基 于 区域 生长 的二 值 图像 连 通 区域 标 记 的 快 速 算 法 。 与传 统 方 法相 比 该 方 法 的特 点 是 在 一 次 图像 扫 描 中 完 成 所 有 连 通 区域 的 标 记 , 而且 避 免 了大 多数 改 进 算 法 都 必 须 处理 的重 复标 记 的 问题 ; 时 . 方 法 不 受 所 同 该
c mp rtv e p r n s o a a i e x e me t i wi t d t n l t r i o a meh d n t o i i r v d h a i t o a d wo f t mp o e meh d ae s t o s r ma eT e x e me tl es l d . h e p r n a r ut i s s o h r p s d a g r h h g f ce c n o u t e s h w t e p o o e lo t m i h e in y a d r b sn s . i i Ke wo d : bn r ma e c n e t d c mp n n a e i g e g t n i h o h o sn l c n y rs i a i g , o n c e o o e t l b l , ih e g b r o d,i g e s a y n
CHEN Bai h ng —s e
( eateto o ue cec , uqa nvri , unhuFj n3 2 ) D pr n fC mptrSineH aioU iesy Q azo ,ui 6 0 m t a 1 1
二值图像连通域标记优化算法
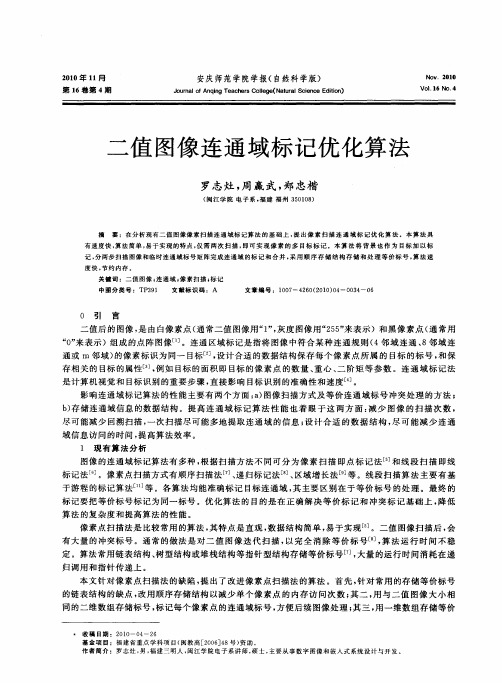
第 4 期
罗 志 灶 , 赢 武 , : 值 图 像 连 通 域 标 记 优化 算 法 周 等 二
。3 ‘ 5
标 号 的共 同连通域 标 号及 目标 属性 , 于解 决 冲突标 号 的处理 ; 用 最后 , 改进 等价 标号合 并 算法 , 重新 排序 标 号 , 得合 理 的 目标 标号 。 获
有 速 度 快 , 法 简单 , 于 实 现 的 特点 , 需 两 次 扫 描 , 可 实 现 像 素 的 多 目标 标 记 。本 算 法 将 背 景 也 作 为 目标 加 以 标 算 易 仅 即 记 , 两 步 扫 描 图像 和 临时 连 通 域 标 号矩 阵完 成 连 通 域 的标 记 和 合 并 , 用 顺 序 存 储 结 构 存 储 和处 理 等 价 标 号 , 法 速 分 采 算
存 相关 的 目标 的属性 [ , 如 目标 的面 积 即 目标 的像 素 点 的数 量 、 心 、 阶矩 等参 数 。连通 域 标 记法 3例 ] 重 二 是 计算机 视觉 和 目标 识别 的重要 步骤 , 直接影 响 目标 识别 的准 确性 和速度 [ 。 4 ]
影 响连通 域标 记算法 的性能 主要有 两个 方面 :) a 图像扫 描方 式及等 价连 通域标 号 冲突处理 的方法 ; b 存储 连通 域信息 的数据 结构 。提 高连 通 域标 记 算 法 性能 也 着 眼 于这 两 方 面 : ) 减少 图像 的扫描 次 数 , 尽 可能减 少 回溯扫 描 , 一次 扫描尽 可 能多地提 取连 通域 的信息 ; 设计 合 适 的数 据 结构 , 可 能减 少 连通 尽 域 信息访 问 的时间 , 提高算 法效率 。
Vo . 6 N 4 1 1 o.
二值 图像 连通 域标记优 化算法
matlab bwlabel 原理

matlab bwlabel 原理Matlab的bwlabel函数是一种用于图像分割的工具,它能够将二值图像中的连通区域进行标记,从而提取出图像中的不同目标或物体。
在实际应用中,图像分割是一项非常重要的任务,它可以用于目标检测、图像识别、图像分析等领域。
而bwlabel函数作为Matlab中常用的图像分割函数之一,可以对二值图像进行连通区域标记,是图像分析与处理中必不可少的工具。
bwlabel函数的原理是基于连通区域的概念。
在二值图像中,连通区域指的是图像中具有相同像素值的像素点所构成的区域。
bwlabel 函数通过扫描图像中的像素,将具有相同像素值且相连的像素点分为一组,并为每个连通区域分配一个唯一的标签。
这个标签可以用于后续的图像分析和处理。
具体来说,bwlabel函数会从图像的左上角开始扫描,对于每个像素点,它会判断其是否为前景像素(像素值为1)。
如果是前景像素,则会检查其周围的像素点,如果周围的像素点中已经有标记过的像素点,则将当前像素点标记为相同的标签。
如果周围的像素点都没有标记过,则将当前像素点标记为一个新的标签。
通过不断扫描和标记,最终得到图像中所有连通区域的标签。
在bwlabel函数的输出中,每个像素点的标签值可以用于区分不同的连通区域。
同时,函数还会返回一个标签矩阵,该矩阵的大小与输入图像相同,每个像素点的值表示该像素点所属的连通区域的标签。
通过分析标签矩阵,我们可以得到图像中的不同连通区域的位置、大小等信息,从而实现对图像的分割和分析。
需要注意的是,bwlabel函数只能处理二值图像,即图像中的像素值只能为0或1。
如果需要处理灰度图像或彩色图像,可以先进行阈值分割,将图像转化为二值图像,然后再使用bwlabel函数进行连通区域标记。
总的来说,Matlab的bwlabel函数是一种用于图像分割的工具,通过对图像中的连通区域进行标记,可以提取出图像中的不同目标或物体。
它的原理是基于连通区域的概念,通过扫描图像中的像素,将具有相同像素值且相连的像素点分为一组,并为每个连通区域分配一个唯一的标签。
一种二值图像连通区域标记的新方法
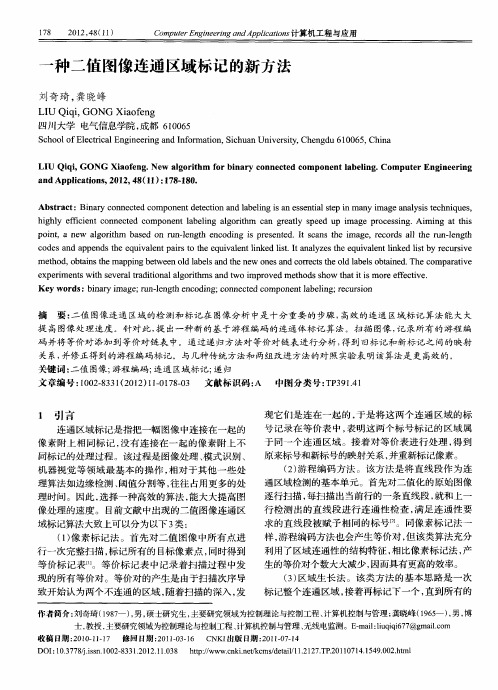
() 2 游程编码方法 。该方 法是将直线段作 为连 机器 视觉等领域最基本 的操作 , 相对 于其他 一些处 通 区域检测的基本单元。首先对二值化 的原始图像 理算法如边缘检测 、 阈值分割等 , 往往 占用更多的处 每扫描 出当前行的一条直线段 , 就和上一 理时 间。因此 , 选择一种高效的算法 , 大大提高图 逐行扫描 , 能 满足连 通性要 像处理的速度 。 目前文献中出现的二值 图像连通区 行检测 出的直线段进行连通性检查 , 域标记算法大致上可以分为以下 3 : 类 ( ) 素标记法 。首先 对二值图像 中所有 点进 1像 行一次完整扫描 , 标记所有的 目标像素点 , 同时得到 等价标 记表 u 。等价标记表 中记录着扫描过程 中发 现的所有等价对 。等价对 的产生是 由于扫描次序导 致开始认为两个不连通的区域 , 随着扫描的深入 , 发 求 的直线段被 赋予相 同的标号 。同像 素标 记法一 样, 游程编码方法也会产生等价对 , 但该类算法充分
关键 词 : 二值 图像 ; 游程 编码 ; 连通 区域标i ; 归 5递
文章 编 号 :0 283 (02 l一l80 文献 标识 码 : 10—3 12 1) l 7 —3 0 A 中图 分 类号 :P 9 .1 T 31 4
1 引言
连 通 区域 标 记 是 指把 一 幅 图像 中连 接 在 一 起 的
m eh d o t i s h p i gb t e l b l a d t en w n s n o r c s h l b l o t i e . h o a a i e t o , b an ema p n ewe n o d l es n e o e dc re t t eo d l e s b n d T e c mp r t t a h a a a v e p r n swi e e a a i o a l o i m sa d t o i r v dm eh d h w a r fe t e x ei me t t s v r l r d t n l g r h n h t i a t w mp o e t o ss o t t t s h i i mo e e f c i . v K e r s b n r a e r n ln t n o i g c n c e o o e t a e i g r c r in y wo d : i a i g ; u —e g h e c d n ; o ne td c mp n n b l ; e u so y m l n
二值图像连通域标记快速算法实现

二值图像连通域标记快速算法实现算法描述首先,在进行标记算法以前,利用硬件开辟独立的图像标记缓存和连通关系数组,接着在视频流的采集传输过程中,以流水线的方式按照视频传输顺序对图像进行逐行像素扫描,然后对每个像素的邻域分别按照逆时针方向和水平方向进行连通性检测和等价标记关系合并,检测出的结果对标记等价数组和标记缓存进行更新,在一帧图像采集传输结束后,得到图像的初步标记结果以及初步标记之间的连通关系,最后,根据标号对连通关系数组从小到大的传递过程进行标号的归并,利用归并后的连通关系数组对图像标记缓存中的标号进行替换,替换后的图像为最终标记结果,并且连通域按照扫描顺序被赋予唯一的连续自然数。
图 1 标记算法流程本文快速二值图像连通域标记算法分为三个环节:1.图像初步标记:为每个像素赋予临时标记,并且将临时标记的等价关系记录在等价表中2.整理等价表:这一环节分为两个步骤:(1)将具有等价关系的临时标记全部等价为其中的最小值;(2)对连通区域以自然数顺序重新编号,得到临时标记与最终标记之间的等价关系。
3.图像代换:对图像进行逐像素代换,将临时标记代换为最终标记.经过3个环节处理后,算法输出标记后的图像,图像中连通域按照由上到下,由左至右出现的顺序被标以连续的自然数。
1 图像初始标记标记算法符号约定:算法在逆时钟方向检测连通域时用w1,w2表示连续两行的图像数据,在紧接着的顺时钟方向连通域检测时用k0,k表示连续两行经过逆时钟方向标记后的图像数据。
其在工作窗口的位置在图2、3中分别说明;对初始逆时针方向临时标记用Z表示。
Z初始标记值为1。
二值图像连通域标记算法采用8连通判断准则,通过缩小标记范围剔除了图像的边界效应。
为了简化标记处理过程,使标记处理在硬件对一帧图像传输操作时间内结束,标记处理利用中间数据缓存分为连续的两种类型,其中类型1用于直接图像序列传输,硬件发起图像序列传输时,类型1采用逆时钟顺序连通域检测,对2×3工作窗口中的二值像素进行初始标记。
图像分析:二值图像连通域标记
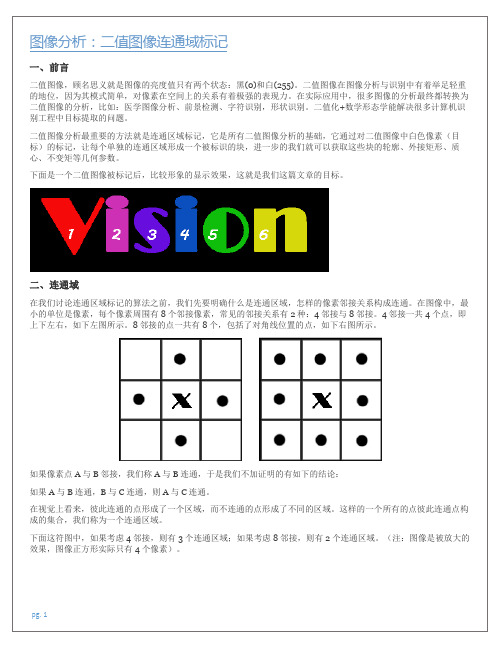
二、连通域
如果像素点A与B邻接,我们称A与B连通,于是我们不加证明的有如下的结论:
三、连通区域的标记
第一行,我们得到两个团:[2,6]和[10,13],同时给它们标记1和2。
下面是这个过程的C++实现,每个等价表用一个vector<int>来保存,等价对列表保存在map<pair<int,int>>里。
整个算法步骤,我们只扫描了一次图像,同时我们对图像中的像素进行标记,要么赋予一个新的标号,要么用它同行P点。
最后不要忘了把C的值加1。
这个过程如下面图像S1中所示。
情况3
1)如果P是外轮廓的起点,也就是说我们是从P点开始跟踪的,那么我们从7号(右上角)位置P1P1开始,看7号在L上标记为一个负值。
如下图所示,其中右图像标记的结果。
2)那么如果P是不是外轮廓的起点,即P是外轮廓路径上的一个点,那么它肯定是由一个点进入的,我们设置为P−
在OpenCV中查找轮廓的函数已经存在了,而且可以得到轮廓之间的层次关系。
这个函数按上面的算法实现起来并不。
基于CUDA的二值图像连通域快速标记算法改进研究
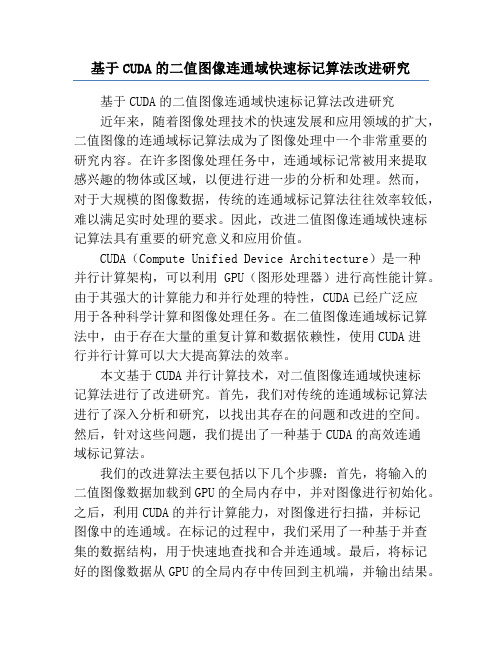
基于CUDA的二值图像连通域快速标记算法改进研究基于CUDA的二值图像连通域快速标记算法改进研究近年来,随着图像处理技术的快速发展和应用领域的扩大,二值图像的连通域标记算法成为了图像处理中一个非常重要的研究内容。
在许多图像处理任务中,连通域标记常被用来提取感兴趣的物体或区域,以便进行进一步的分析和处理。
然而,对于大规模的图像数据,传统的连通域标记算法往往效率较低,难以满足实时处理的要求。
因此,改进二值图像连通域快速标记算法具有重要的研究意义和应用价值。
CUDA(Compute Unified Device Architecture)是一种并行计算架构,可以利用GPU(图形处理器)进行高性能计算。
由于其强大的计算能力和并行处理的特性,CUDA已经广泛应用于各种科学计算和图像处理任务。
在二值图像连通域标记算法中,由于存在大量的重复计算和数据依赖性,使用CUDA进行并行计算可以大大提高算法的效率。
本文基于CUDA并行计算技术,对二值图像连通域快速标记算法进行了改进研究。
首先,我们对传统的连通域标记算法进行了深入分析和研究,以找出其存在的问题和改进的空间。
然后,针对这些问题,我们提出了一种基于CUDA的高效连通域标记算法。
我们的改进算法主要包括以下几个步骤:首先,将输入的二值图像数据加载到GPU的全局内存中,并对图像进行初始化。
之后,利用CUDA的并行计算能力,对图像进行扫描,并标记图像中的连通域。
在标记的过程中,我们采用了一种基于并查集的数据结构,用于快速地查找和合并连通域。
最后,将标记好的图像数据从GPU的全局内存中传回到主机端,并输出结果。
为了验证我们改进算法的效果,我们进行了大量的实验,并与传统的连通域标记算法进行了对比。
实验结果表明,我们的算法在速度和性能上都有显著的提升。
相比传统算法,我们的算法在处理大规模图像数据时,速度可以提升数十倍,并且具有更好的并行性能和扩展性。
除了提高算法的速度和性能,我们的改进算法还具有一定的优化空间。
matlab 连通域标记

matlab 连通域标记在图像处理中,连通域标记是指将图像中的相邻像素点组成的区域进行分组和标记的过程。
连通域标记可以用于图像分割、物体识别、图像分析等领域。
Matlab是一种功能强大的数值计算和科学编程软件,提供了丰富的图像处理工具箱,可以方便地实现连通域标记算法。
在Matlab中,可以使用bwlabel函数来实现连通域标记。
该函数可以将二值图像中的连通域进行标记,并返回每个像素所属的连通域编号。
具体步骤如下:1. 将图像二值化,即将图像转换成黑白两色(前景和背景)。
2. 使用bwlabel函数对二值图像进行连通域标记。
该函数的语法为:[L, num] = bwlabel(BW),其中BW为二值图像,L为标记后的图像,num为连通域的数量。
3. 可以使用regionprops函数对每个连通域进行属性分析。
该函数可以计算每个连通域的面积、重心、边界框等属性,并返回一个结构体数组。
以下是一个示例代码,演示了如何使用Matlab进行连通域标记和属性分析:```matlab% 读取图像image = imread('example.png');% 将图像转换为二值图像bw = imbinarize(image);% 进行连通域标记[L, num] = bwlabel(bw);% 对每个连通域进行属性分析props = regionprops(L, 'Area', 'Centroid','BoundingBox');% 输出每个连通域的属性信息for i = 1:numfprintf('连通域 %d:', i);fprintf('面积: %d', props(i).Area);fprintf('重心: (%d, %d)', props(i).Centroid(1), props(i).Centroid(2));fprintf('边界框: (%d, %d, %d, %d)', props(i).BoundingBox(1), props(i).BoundingBox(2), props(i).BoundingBox(3), props(i).BoundingBox(4));end```以上代码会输出每个连通域的面积、重心和边界框信息。
用VC++实现图像连通区域标记
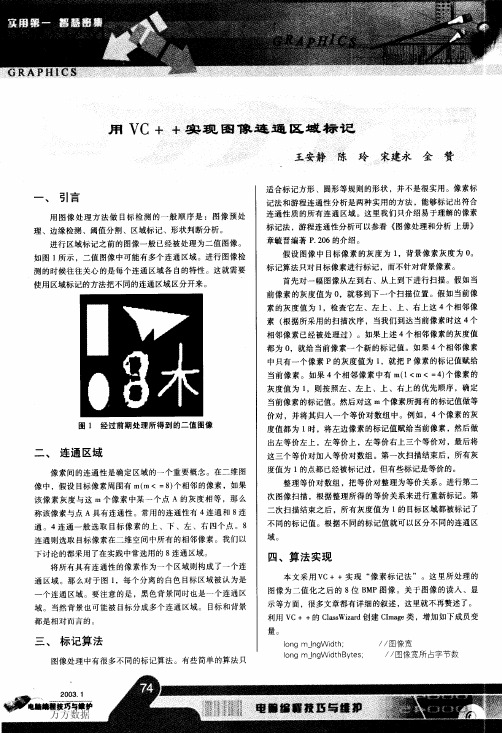
Int nMa×Ma rkValue=O:
//记录最大的标识的值
int i,j:
//循环控制变量
/+定义存放等价对的链表,其元素是EquaIMa rk类型,定义
Iist是为了节约存储空问要使用C|ist,应该#lncIude<Afx-
temOl h> }/
CList<EquaIMark,EqualMa rk>『EqualMark: //初始化图像移动指针
//pInne rListAdd,每次向exList中添加的新元素
CPtrList。pInnerListAdd=new CPtrList:
ASSERT(pInne rLjstAdd!=NULL):
/+添加第一个等价对到e×Llst的第一个元素所指向的h
ne r L』jst中
¥/
p JnnerLjstAdd一)AddTa JI(f vojd 半 )JEquaIMark GetHead f)
的判断。 Note2:可以先对等价对进行排序,每次都保证MarkVal—
uel<MarkValue2,这样易于管理等价对。
Note3:在实际工作中,连续寻找出的等价对很容易重
复,将本次找出的等价对和链表中保存的最后一个等价对相比 较,如果不相等的话再存入等价对链表,这样可以大大降低链
表中等价对的重复。 Note4:第一次扫描之后,nMarkValue一1即为nMa)【Mark—
用阳卡争|饕现唐≯’雾蓬通区匆《稼萄参I
|i |i。;::
玉案静。。豫玲。褰建永金赞
一、引言
用图像处理方法做目标检测的一般顺序是:图像预处 理、边缘检测、阈值分割、区域标记、形状判断分析。
进行区域标记之前的图像一般已经被处理为二值图像。 如图1所示,二值图像中可能有多个连通区域。进行图像检 测的时候往往关心的是每个连通区域各自的特性。这就需要 使用区域标记的方法把不同的连通区域区分开来。
GPU加速的二值图连通域标记并行算法

新 的二 值 图像 连 通 域 标 记 并 行 算 法 , 高速 有 效 地 标 识 出 了二 值 图 的 连 通 域 位 置 及 大 小 , 幅 缩 减 了标 记 时 间耗 费。 大
该算法通过搜 索邻域 内最小标 号值 的像 素点 对连通域进 行标记 , 各像 素点处理顺序 不分先后 并且 不相 互依赖 , 因此 可以并行执行。算法效率不受连通域形状及数量的影响 , 具有很好 的鲁棒性。实验 结果表 明, 该并行算法充分发挥 了 G U并行处理能力 , P 在处理 高分辨率与多连通域 图像 时效率为一般 C U标 记算 法的 30倍 , O eC P 0 比 p n V的优化 函数
Ab ta t I o bn t no V D A S rp is rc si n ( P )p rl l rh etr a dh rw r a rs n e s c : nc m ia o f I I ’ G a hc Po es gU i G U a l c i c e n ad aef t e d r r i N n t aea t u eu u C m ue U i e e i rht tr C D o p t nf d D v e A c i cue( U A) ac i cue a n w p r l a e n l r h fc n etd d m i w s i c e rht tr, e a l ll l g ag i m o o n ce o a a e ae b i ot n
第3 第 l 0卷 0期
21 0 0年 1 0月
计算机应 用
J un lo mp trAp l ain o ra fCo ue pi t s c o
Vo . 130 No. 0 1 0c .2O1 t 0
一种新的二值图像连通区域准确标记算法
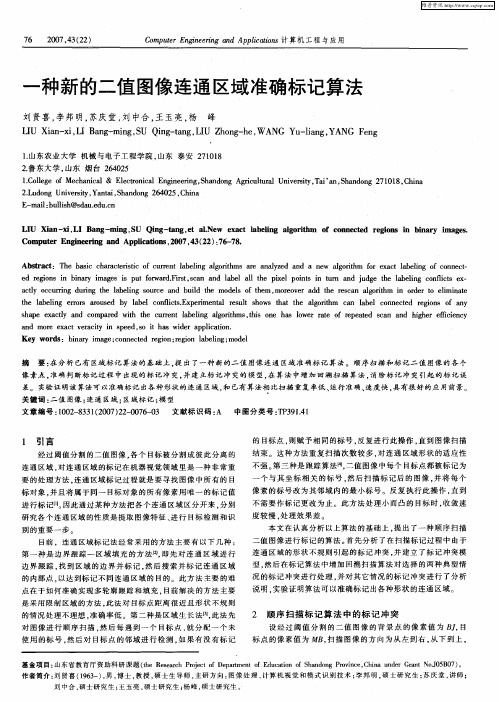
n r e a tv rct i p e S t a d ra pi t . a d mo x c ea i n s e d,O i h swie p l ain e y c o
Ke r s b n r ma e; o n ce e in; go a e i g mo e y wo d : i a i g c n e t d r go r in l b l ; d l y e n
2鲁 东 大 学 , . 山东 烟 台 2 4 2 605
1C l g f Me h n c l& Elc r n c g n e i g S a d n rc l r ie st T i a , h n o g 2 1 1 C i a . o l e o c a i a e e to ia En i e rn , h n o g Ag i u t a Un v r i l ul y, a ’ S a d n 7 0 8, h n n
摘
要 : 分析 已有 区域 标 记 算 法 的基 础 上 , 出 了一 种 新 的 二值 图像 连 通 区域 准确 标 记 算 法 。 顺 序 扫 描 和 标 记 二 值 图像 的各 个 在 提
像 素 点 , 确判 断标 记 过 程 中 出现 的标 记 冲 突 , 建 立 标 记 冲 突的 模 型 , 算 法 中增 加 回溯 扫 描 算 法 , 除标 记 冲 突 引起 的标 记 误 准 并 在 消 差 。 实验证 明该 算 法可 以 准确 标 记 出各 种 形状 的 连 通 区域 , 已有 算 法相 比 扫描 重 复 率低 、 和 运行 准 确 、 度 快 , 有 很 好 的应 用 前景 。 速 具
2 L d n ie i Ya t i S a d n 6 0 5, h n . u o g Un v r t s y, n a , h n o g 2 4 2 C i a E— i: u l h d u e u c mal b l s @s a .d .n i
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
{ // B, C, D not object - new object
// label and put into table
ONETWO(labels, r, c, nc) = tlabel;
}
}
else if ( B ) // B is object but C is not
ntable++;
ONETWO(labels, r, c, nc) = lset[ ntable ] = ntable;
}
}
else if ( n == 8 )
#include "cv.h"
#include "highgui.h"
#include <string>
using namespace std;
#define NO_OBJECT 0
//#define MIN(x, y) (((x) < (y)) ? (x) : (y))
else
{ // B, C, D not object - new object
// label and put into table
ONETWO(labels, r, c, nc) = B;
else if ( C ) // C is object but B is not
ONETWO(labels, r, c, nc) = C;
if ( B == C )
ONETWO(labels, r, c, nc) = B;
else {
lset[C] = B;
#define ELEM(img, r, c) (CV_IMAGE_ELEM(img, unsigned char, r, c))
#define ONETWO(L, r, c, col) (L[(r) * (col) + c])
int find( int set[], int x )
int tlabel = MIN(B,C);
lset[B] = tlabel;
lset[C] = tlabel;
|B|A| |
+-+-+-+
| | | |
+-+-+-+
A is the center pixel of a neighborhood. In the 3 versions of
connectedness:
4: A connects to B and C
// n -- n connectedness
// labels -- label of each pixel, labels[row * col]
//output: number of connected regions
//
//
if ( n == 4 )
{
// apply 4 connectedness
if ( B && C )
{ // B and C are labeled
n = 4;
int nr = img->height;
int nc = img->width;
int total = nr * nc;
// results
memset(labels, 0, total * sizeof(int));
ONETWO(labels, r, c, nc) = D;
else if ( B && C )
{ // B and C are labeled
6: A connects to B, C, and D
8: A connects to B, C, D, and E
*/
/////////////////////////////
//by yysdsyl
//
//input:img -- gray image
if ( r == 0 || c == nc - 1 )
E = 0;
else
E = find( lset, ONETWO(labels, r - 1, c + 1, nc) );
{
if ( ELEM(img, r, c) ) // if A is an object
{
// get the neighboring pixels B, C, D, and E
//
int bwlabel(string filenane, int n, int* labels)
{
IplImage* img = (IplImage* )cvLoadImage(filenane.data(), 0);
if(n != 4 && n != 8)
{
int r = x;
while ( set[r] != r )
r = set[r];
return r;
}
/*
labeling scheme
+-+-+-+
|D|C|E|
+-+-+-+
int B, C, D, E;
if ( c == 0 )
B = 0;
Байду номын сангаасelse
B = find( lset, ONETWO(labels, r, c - 1, nc) );
ntable++;
ONETWO(labels, r, c, nc) = lset[ ntable ] = ntable;
}
}
int nobj = 0; // number of objects found in image
// other variables
int* lset = new int[total]; // label table
ONETWO(labels, r, c, nc) = B;
}
}
else if ( B ) // B is object but C is not
else if ( n == 6 )
{
// apply 6 connected ness
if ( D ) // D object, copy label and move on
memset(lset, 0, total * sizeof(int));
int ntable = 0;
for( int r = 0; r < nr; r++ )
{
for( int c = 0; c < nc; c++ )
ONETWO(labels, r, c, nc) = B;
else if ( C ) // C is object but B is not
ONETWO(labels, r, c, nc) = C;
if ( r == 0 || c == 0 )
D = 0;
else
D = find( lset, ONETWO(labels, r - 1, c - 1, nc) );
if ( r == 0 )
C = 0;
else
C = find( lset, ONETWO(labels, r - 1, c, nc) );
if ( B == C )
ONETWO(labels, r, c, nc) = B;
else
{