顺序栈 链栈等C语言实现
数据结构——用C语言描述(第3版)教学课件第3章 栈和队列

if(S->top==-1) /*栈为空*/
return(FALSE);
else
{*x = S->elem[S->top];
return(TRUE);
}
返回主目录}[注意]:在实现GetTop操作时,也可将参数说明SeqStack *S 改为SeqStack S,也就是将传地址改为传值方式。传 值比传地址容易理解,但传地址比传值更节省时间、 空间。
返回主目录
算法:
void BracketMatch(char *str) {Stack S; int i; char ch; InitStack(&S); For(i=0; str[i]!='\0'; i++) {switch(str[i])
{case '(': case '[': case '{':
3.1.3 栈的应用举例
1. 括号匹配问题
思想:在检验算法中设置一个栈,若读入的是左括号, 则直接入栈,等待相匹配的同类右括号;若读入的是 右括号,且与当前栈顶的左括号同类型,则二者匹配, 将栈顶的左括号出栈,否则属于不合法的情况。另外, 如果输入序列已读尽,而栈中仍有等待匹配的左括号, 或者读入了一个右括号,而栈中已无等待匹配的左括 号,均属不合法的情况。当输入序列和栈同时变为空 时,说明所有括号完全匹配。
return(TRUE);
}
返回主目录
【思考题】
如果将可利用的空闲结点空间组织成链栈来管理,则申 请一个新结点(类似C语言中的malloc函数)相当于链 栈的什么操作?归还一个无用结点(类似C语言中的 free函数)相当于链栈的什么操作?试分别写出从链栈 中申请一个新结点和归还一个空闲结点的算法。
数据结构(C语言)第3章 栈和队列
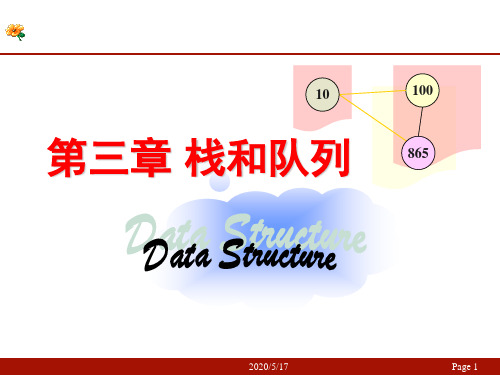
Data Structure
2013-8-6
Page 13
栈的顺序存储(顺序栈)
利用一组地址连续的存储单元依次存放自栈底到栈顶的数 据元素。 结构定义: #define STACK_INIT_SIZE 100; // 存储空间初始分配量 #define STACKINCREMENT 10; // 存储空间分配增量 typedef struct { SElemType *base; // 存储空间基址 SElemType *top; // 栈顶指针 int stacksize; // 当前已分配的存储空间,以元素位单位 } SqStack;
解决方案2:
顺序栈单向延伸——使用一个数组来存储两个栈
Data Structure 2013-8-6 Page 21
两栈共享空间 两栈共享空间:使用一个数组来存储两个栈,让一个 栈的栈底为该数组的始端,另一个栈的栈底为该数组 的末端,两个栈从各自的端点向中间延伸。
Data Structure
2013-8-6
链栈需要加头结点吗? 链栈不需要附设头结点。
Data Structure
2013-8-6
Page 27
栈的链接存储结构及实现
Data Structure
2013-8-6
Page 11
GetTop(S, &e) 初始条件:栈 S 已存在且非空。 操作结果:用 e 返回S的栈顶元素。 Push(&S, e) 初始条件:栈 S 已存在。 操作结果:插入元素 e 为新的栈顶元素。 Pop(&S, &e) 初始条件:栈 S 已存在且非空。 操作结果:删除 S 的栈顶元素,并用 e 返回其值。
Data Structure
数据结构c语言实现

数据结构c语言实现数据结构是计算机科学中重要的一个领域,它研究不同的数据组织方式,以及在这些数据上进行各种操作的算法。
常见的数据结构包括数组、栈、队列、链表、树、图等。
在C语言中,数据结构是通过使用结构体来实现的。
结构体是由一组数据成员组合而成的自定义数据类型,可以包含不同数据类型的数据成员。
以下是如何在C语言中实现不同的数据结构。
数组数组是数据结构中最基本的数据结构之一。
C语言中的数组定义方式如下:```int array[5];```这个代码定义了一个名为array的数组,其中有5个元素,每个元素的类型是整数。
要访问数组中的元素,可以通过下标访问:这个代码设置了数组中第一个元素的值为1。
栈栈是一种后进先出(LIFO)的数据结构。
使用C语言中的数组可以实现栈。
以下是一个简单的栈实现:```#define MAXSIZE 100int stack[MAXSIZE];int top = -1;void push(int data){if(top<MAXSIZE-1){ //判断栈是否满了stack[++top] = data; //插入数据}}int isEmpty(){return top==-1; //栈是否为空}队列链表链表是一个由节点组成的数据结构,每个节点包含一个数据成员和一个指向下一个节点的指针。
在C语言中,链表可以使用结构体和指针来实现。
以下是一个单向链表的实现:```struct node{int data;struct node *next;};struct node *head = NULL;void insert(int data){struct node *new_node = (struct node*) malloc(sizeof(struct node)); //分配内存new_node->data = data; //初始化数据new_node->next = head; //新节点指向当前头节点head = new_node; //更新头节点}void delete(int data){struct node *current_node = head; //从头节点开始查找struct node *previous_node = NULL;while(current_node!=NULL&¤t_node->data!=data){ //查找节点previous_node = current_node;current_node = current_node->next;}if(current_node!=NULL){ //找到了节点if(previous_node!=NULL){ //非头节点previous_node->next = current_node->next; }else{ //头节点head = current_node->next;}free(current_node); //释放内存}}树。
数据结构_c语言描述(第二版)答案_耿国华_西安电子科技大学

第1章绪论2.(1)×(2)×(3)√3.(1)A(2)C(3)C5.计算下列程序中x=x+1的语句频度for(i=1;i<=n;i++)for(j=1;j<=i;j++)for(k=1;k<=j;k++)x=x+1;【解答】x=x+1的语句频度为:T(n)=1+(1+2)+(1+2+3)+……+(1+2+……+n)=n(n+1)(n+2)/66.编写算法,求一元多项式p n(x)=a0+a1x+a2x2+…….+a n x n的值p n(x0),并确定算法中每一语句的执行次数和整个算法的时间复杂度,要求时间复杂度尽可能小,规定算法中不能使用求幂函数。
注意:本题中的输入为a i(i=0,1,…n)、x和n,输出为P n(x0)。
算法的输入和输出采用下列方法(1)通过参数表中的参数显式传递(2)通过全局变量隐式传递。
讨论两种方法的优缺点,并在算法中以你认为较好的一种实现输入输出。
【解答】(1)通过参数表中的参数显式传递优点:当没有调用函数时,不占用内存,调用结束后形参被释放,实参维持,函数通用性强,移置性强。
缺点:形参须与实参对应,且返回值数量有限。
(2)通过全局变量隐式传递优点:减少实参与形参的个数,从而减少内存空间以及传递数据时的时间消耗缺点:函数通用性降低,移植性差算法如下:通过全局变量隐式传递参数PolyValue(){ int i,n;float x,a[],p;printf(“\nn=”);scanf(“%f”,&n);printf(“\nx=”);scanf(“%f”,&x);for(i=0;i<n;i++)scanf(“%f ”,&a[i]); /*执行次数:n次*/p=a[0];for(i=1;i<=n;i++){ p=p+a[i]*x; /*执行次数:n次*/x=x*x;}printf(“%f”,p);}算法的时间复杂度:T(n)=O(n)通过参数表中的参数显式传递float PolyValue(float a[ ], float x, int n){float p,s;int i;p=x;s=a[0];for(i=1;i<=n;i++){s=s+a[i]*p; /*执行次数:n次*/p=p*x;}return(p);}算法的时间复杂度:T(n)=O(n)第2章线性表习题1.填空:(1)在顺序表中插入或删除一个元素,需要平均移动一半元素,具体移动的元素个数与插入或删除的位置有关。
《数据结构(C语言)》第3章 栈和队列

栈
❖ 栈的顺序存储与操作 ❖ 1.顺序栈的定义
(1) 栈的静态分配顺序存储结构描述 ② top为整数且指向栈顶元素 当top为整数且指向栈顶元素时,栈空、入栈、栈满 及出栈的情况如图3.2所示。初始化条件为 S.top=-1。
(a) 栈空S.top==-1 (b) 元素入栈S.stack[++S.top]=e (c) 栈满S.top>=StackSize-1 (d) 元素出栈e=S.stack[S.top--]
/*栈顶指针,可以指向栈顶
元素的下一个位置或者指向栈顶元素*/
int StackSize; /*当前分配的栈可使用的以 元素为单位的最大存储容量*/
}SqStack;
/*顺序栈*/
Data structures
栈
❖ 栈的顺序存储与操作 ❖ 1.顺序栈的定义
(2) 栈的动态分配顺序存储结构描述 ① top为指针且指向栈顶元素的下一个位置 当top为指针且指向栈顶元素的下一个位置时,栈空 、入栈、栈满及出栈的情况如图3.3所示。初始化条 件为S.top=S.base。
…,n-1,n≥0} 数据关系:R={< ai-1,ai>| ai-1,ai∈D,i=1,2
,…,n-1 } 约定an-1端为栈顶,a0端为栈底 基本操作:
(1) 初始化操作:InitStack(&S) 需要条件:栈S没有被创建过 操作结果:构建一个空的栈S (2) 销毁栈:DestroyStack(&S) 需要条件:栈S已经被创建 操作结果:清空栈S的所有值,释放栈S占用的内存空间
return 1;
}
Data structures
栈
c语言堆栈和队列函数大全
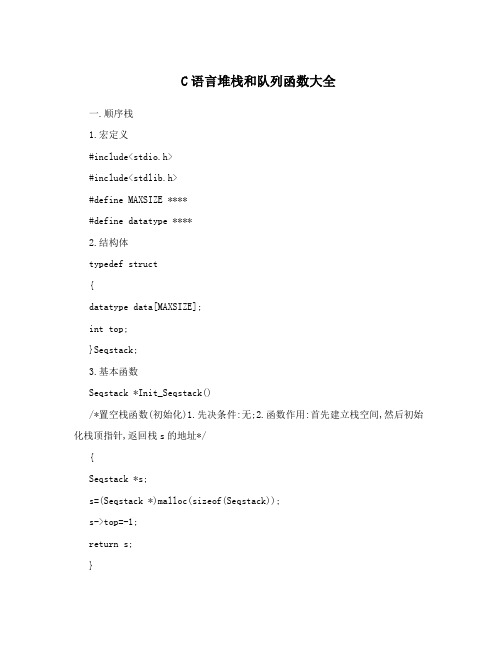
C语言堆栈和队列函数大全一.顺序栈1.宏定义#include<stdio.h>#include<stdlib.h>#define MAXSIZE ****#define datatype ****2.结构体typedef struct{datatype data[MAXSIZE];int top;}Seqstack;3.基本函数Seqstack *Init_Seqstack()/*置空栈函数(初始化)1.先决条件:无;2.函数作用:首先建立栈空间,然后初始化栈顶指针,返回栈s的地址*/{Seqstack *s;s=(Seqstack *)malloc(sizeof(Seqstack));s->top=-1;return s;}int Empty_Seqstack(Seqstack *s) /*判栈空函数1.先决条件:初始化顺序栈;2.函数作用:判断栈是否为空,空返回1,不空返回0*/ {if(s->top==-1) return 1;else return 0;}int Push_Seqstack(Seqstack *s,datatype x) /*入栈函数1.先决条件:初始化顺序栈2.函数作用:将数据x入栈,栈满则不能,成功返回1,因栈满失败返回0*/ {if(s->top==MAXSIZE-1)return 0;s->top=s->top+1;s->data[s->top]=x;return 1;}int Pop_Seqstack(Seqstack *s,datatype *x) acidity, mL.; M--calibration of the molar concentration of sodium hydroxide standard solution, moI/L; V--amount of the volume of sodium hydroxide standard solution, Ml; M--the weight of the sample, g. Such as poor meets the requirements, take the arithmetic mean of the second determination as a result. Results one decimal. 6, allowing differential analyst simultaneously or in quick succession for the second determination, the absolute value of the difference of the results. This value should be no more than 1.0. 1, definitions and principles for determination of ash in starches, starch and ash: starch samples of ash the residue obtainedafter weight. Original sample residue weight of sample weight or weight expressed as a percentage of the dry weight of the sample. Samples ofash at 900 ? high temperature until ashing sample ... The Crucible: determination of Platinum or other conditions of the affected material, capacity of 50mL. Dryer: has effectively adequate drying agent and-perforated metal plate or porcelain. Ashing furnaces: device for controlling and regulating temperature, offers 900 incineration temperature of 25 c. Analytical balance. Electric hot plate or Bunsen. 3, crucible of analysis steps preparation: Crucible must first wash with boiling dilute hydrochloric acid, then wash with a lot of water and then rinse with distilled water. Wash the Crucible within ashing furnace, heated at 900 to 25 ? 30min, and in the desiccator to cool to room temperature and then weighing,/*出栈函数1.先决条件:初始化顺序栈2.函数作用:从栈中出一个数据,并将其存放到x中,成功返回1,因栈空失败返回0*/{if(s->top==-1)return 0;*x=s->data[s->top];s->top--;return 1;}int Top_Seqstack(Seqstack *s,datatype *x)/*取栈顶元素函数1.先决条件:初始化顺序栈2.函数作用:取栈顶元素,并把其存放到x中,成功返回1,因栈空失败返回0*/{if(s->top==-1)return 0;*x=s->data[s->top];return 1;}int Printf_Seqstack(Seqstack *s) /*遍历顺序栈函数1.先决条件:初始化顺序栈2.函数作用:遍历顺序栈,成功返回1*/ {int i,j=0;for(i=s->top;i>=0;i--){printf("%d ",s->data[i]);/*因datatype不同而不同*/j++;if(j%10==0)printf("\n");}printf("\n");return 1;}int Conversation_Seqstack(int N,int r) /*数制转换函数(顺序栈)1.先决条件:具有置空栈,入栈,出栈函数2.函数作用:将N转换为r进制的数*/{Seqstack *s;datatype x;printf("%d转为%d进制的数为:",N,r);/*以后可以删除去*/s=Init_Seqstack();do{Push_Seqstack(s,N%r);N=N/r;acidity, mL.; M--calibration of the molar concentration of sodium hydroxide standard solution, moI/L; V--amount of the volume of sodium hydroxide standard solution, Ml; M--the weight of the sample, g. Such as poor meets the requirements, take the arithmetic mean of the second determination as a result. Results one decimal. 6, allowing differential analyst simultaneously or in quick succession for the second determination, the absolute value of the difference of the results. This value should be no more than 1.0. 1, definitions and principles for determination of ash in starches, starch and ash: starch samples of ash the residue obtained after weight. Original sample residue weight of sample weight or weight expressed as a percentage of the dry weight of the sample. Samples of ash at 900 ? high temperature until ashing sample ... The Crucible: determination of Platinum or other conditions of the affected material, capacity of 50mL. Dryer: has effectivelyadequate drying agent and-perforated metal plate or porcelain. Ashing furnaces: device for controlling and regulating temperature, offers 900 incineration temperature of 25 c. Analytical balance. Electric hot plate or Bunsen. 3, crucible of analysis steps preparation: Crucible mustfirst wash with boiling dilute hydrochloric acid, then wash with a lot of water and then rinse with distilled water. Wash the Crucible within ashing furnace, heated at 900 to 25 ? 30min, and in the desiccator to cool to room temperature and then weighing,}while(N);while(Pop_Seqstack(s,&x)){if(x>=10)/*为了能转为十进制以上的*/printf("%c",x+55);elseprintf("%d",x);}free(s);/*释放顺序栈*/printf("\n");return 1;}4.主函数int main(){Seqstack *s;int choice;datatype x;do{printf("************************************************************ ****\n");printf("1.置空栈 2.判栈空 3.入栈 4.出栈 5.取栈顶元素 6.遍历 7.退出\n");printf("************************************************************ ****\n");printf("请输入选择(1~7):");scanf("%d",&choice);getchar();switch(choice){case 1:s=Init_Seqstack();if(s)printf("置空栈成功!\n");break;case 2:if(Empty_Seqstack(s))printf("此为空栈.\n");elseprintf("此不为空栈.\n");;break;case 3:printf("请输入一个整数:");scanf("%d",&x);if(Push_Seqstack(s,x))printf("入栈成功.\n");elseprintf("栈已满,无法入栈.\n");;break;case 4:if(Pop_Seqstack(s,&x)) acidity, mL.; M--calibration of the molar concentration of sodium hydroxide standard solution, moI/L; V--amount of the volume of sodium hydroxide standard solution, Ml; M--the weight of the sample, g. Such as poor meets the requirements, take the arithmetic mean of the second determination as a result. Results one decimal. 6, allowing differential analyst simultaneously or in quick succession for the second determination, the absolute value of the difference of the results. This value should be no more than 1.0. 1, definitions and principles for determination of ash in starches, starch and ash: starch samples of ash the residue obtained after weight. Original sample residue weight of sample weight or weight expressed as a percentage of the dry weight of the sample. Samples of ash at 900 ? high temperature until ashing sample ... The Crucible: determination of Platinum or other conditions of the affected material, capacity of 50mL. Dryer: has effectively adequate drying agent and-perforated metal plate or porcelain. Ashing furnaces: device for controlling and regulating temperature, offers 900 incineration temperature of 25 c. Analytical balance. Electric hot plate or Bunsen. 3, crucible of analysis steps preparation: Crucible must first wash with boiling dilute hydrochloric acid, then wash with a lot of water and then rinse with distilled water.Wash the Crucible within ashing furnace, heated at 900 to 25 ? 30min, and in the desiccator to cool to room temperature and then weighing, printf("出栈成功,出栈元素为:%d\n",x);elseprintf("出栈失败,因栈为空.\n");break;case 5:if(Top_Seqstack(s,&x))printf("取栈顶元素成功,栈顶元素为:%d\n",x);elseprintf("取栈顶元素失败,因栈为空.\n");break;case 6:Printf_Seqstack(s);break;case 7:printf("谢谢使用!\n");break;default :printf("输入错误,请重新输入!\n");break;}}while(choice!=7);return 0;}二.链栈1.宏定义#include<stdio.h>#include<stdlib.h>#define datatype ****2.结构体typedef struct snode{datatype data;struct snode *next;}Stacknode,*Linkstack;3.基本函数Linkstack Init_Linkstack()/*初始化栈函数1.先决条件:无2.函数作用:初始化链栈,返回top地址*/ { Linkstack top;top=(Linkstack)malloc(sizeof(Stacknode));top->next=NULL;return top;}int Empty_Linkstack(Linkstack top) /*判栈空函数1.先决条件:初始化链栈2.函数作用:判断栈是否为空,空返回1,不空返回0*/{if(top->next==NULL)acidity, mL.; M--calibration of the molar concentration of sodium hydroxide standard solution, moI/L; V--amount of the volume of sodium hydroxide standard solution, Ml; M--the weight of the sample, g. Such as poor meets the requirements, take the arithmetic mean of the second determination as a result. Results one decimal. 6, allowing differential analyst simultaneously or in quick succession for the second determination, the absolute value of the difference of the results. This value should be no more than 1.0. 1, definitions and principles fordetermination of ash in starches, starch and ash: starch samples of ash the residue obtained after weight. Original sample residue weight of sample weight or weight expressed as a percentage of the dry weight of the sample. Samples of ash at 900 ? high temperature until ashing sample ... The Crucible: determination of Platinum or other conditions of the affected material, capacity of 50mL. Dryer: has effectively adequate drying agent and-perforated metal plate or porcelain. Ashing furnaces: device for controlling and regulating temperature, offers 900 incineration temperature of 25 c. Analytical balance. Electric hot plate or Bunsen. 3, crucible of analysis steps preparation: Crucible mustfirst wash with boiling dilute hydrochloric acid, then wash with a lot of water and then rinse with distilled water. Wash the Crucible within ashing furnace, heated at 900 to 25 ? 30min, and in the desiccator to cool to room temperature and then weighing,return 1;else return 0;}int Push_Linkstack(Linkstack top,datatype x) /*入栈函数1.先决条件:初始化链栈2.函数作用:将数据x入栈,成功返回1,失败返回0*/ { Stacknode *p;p=(Stacknode *)malloc(sizeof(Stacknode));p->data=x;p->next=top->next;top->next=p;return 1;}int Pop_Linkstack(Linkstack top,datatype *x) /*出栈函数1.先决条件:初始化链栈2.函数作用:若栈空退出,若没空则将数据出栈,并将其存放到x中,成功返回1,因栈空失败返回0*/{if(top->next==NULL)return 0;Stacknode *p=top->next;*x=p->data;top->next=p->next;free(p);return 1;}int Top_Linkstack(Linkstack top,datatype *x) /*取栈顶元素函数1.先决条件:初始化链栈2.函数作用:取栈顶元素并放到x中,成功返回1,因栈空失败返回0*/{if(top->next==NULL)return 0;*x=top->next->data;return 1;}int Printf_Linkstack(Linkstack top) /*遍历链栈函数1.先决条件:初始化链栈2.函数作用:遍历链栈,成功返回1*/ {Stacknode *p=top->next;int j=0;while(p){printf("%d ",p->data);/*因datatype不同而不同*/j++;if(j%10==0)acidity, mL.; M--calibration of the molar concentration of sodium hydroxide standard solution, moI/L; V--amount of the volume of sodium hydroxide standard solution, Ml; M--the weight of the sample, g. Such as poor meets the requirements, take the arithmetic mean of the second determination as a result. Results one decimal. 6, allowing differential analyst simultaneously or in quick succession for the second determination, the absolute value of the difference of the results. This value should be no more than 1.0. 1, definitions and principles for determination of ash in starches, starch and ash: starch samples of ash the residue obtained after weight. Original sample residue weight of sample weight or weight expressed as a percentage of the dry weight of the sample. Samples of ash at 900 ? high temperature until ashing sample ... The Crucible: determination of Platinum or other conditions of the affected material, capacity of 50mL. Dryer: has effectively adequate drying agent and-perforated metal plate or porcelain. Ashingfurnaces: device for controlling and regulating temperature, offers 900 incineration temperature of 25 c. Analytical balance. Electric hot plate or Bunsen. 3, crucible of analysis steps preparation: Crucible mustfirst wash with boiling dilute hydrochloric acid, then wash with a lot of water and then rinse with distilled water. Wash the Crucible within ashing furnace, heated at 900 to 25 ? 30min, and in the desiccator to cool to room temperature and then weighing,printf("\n");p=p->next;}printf("\n");return 1;}int Conversation_Linkstack(int N,int r)/*数制转换函数(链栈)1.先决条件:具有置空栈,入栈,出栈函数2.函数作用:将N转换为r进制的数*/{Linkstack top;datatype x;printf("%d转为%d进制的数为:",N,r);/*以后可以删除去*/top=Init_Linkstack();do{Push_Linkstack(top,N%r);N=N/r;}while(N);while(Pop_Linkstack(top,&x)){if(x>=10)/*为了能转为十进制以上的*/printf("%c",x+55);elseprintf("%d",x);}printf("\n");free(top);/*释放栈顶空间*/return 1;}4.主函数int main(){Linkstack top;int choice;datatype x;do{printf("************************************************************ ****\n");printf("1.置空栈 2.判栈空 3.入栈 4.出栈 5.取栈顶元素 6.遍历 7.退出\n");printf("************************************************************ ****\n");printf("请输入选择(1~7):");acidity, mL.; M--calibration of the molar concentration of sodium hydroxide standard solution, moI/L; V--amount of the volume of sodium hydroxide standard solution, Ml; M--the weight of the sample, g. Such as poor meets the requirements, take the arithmetic mean of the second determination as a result. Results one decimal. 6, allowing differential analyst simultaneously or in quick succession for the second determination, the absolute value of the difference of the results. This value should be no more than 1.0. 1, definitions and principles for determination of ash in starches, starch and ash: starch samples of ash the residue obtained after weight. Original sample residue weight of sample weight or weight expressed as a percentage of the dry weight of the sample. Samples of ash at 900 ? high temperature until ashing sample ... The Crucible: determination of Platinum or other conditions of the affected material, capacity of 50mL. Dryer: has effectively adequate drying agent and-perforated metal plate or porcelain. Ashing furnaces: device for controlling and regulating temperature, offers 900 incineration temperature of 25 c. Analytical balance. Electric hot plate or Bunsen. 3, crucible of analysis steps preparation: Crucible mustfirst wash with boiling dilute hydrochloric acid, then wash with a lotof water and then rinse with distilled water. Wash the Crucible within ashing furnace, heated at 900 to 25 ? 30min, and in the desiccator to cool to room temperature and then weighing,scanf("%d",&choice);getchar();switch(choice){case 1:top=Init_Linkstack();if(top)printf("置空栈成功!\n");break;case 2:if(Empty_Linkstack(top))printf("此为空栈.\n");elseprintf("此不为空栈.\n");;break;case 3:printf("请输入一个整数:");scanf("%d",&x);if(Push_Linkstack(top,x))printf("入栈成功.\n");elseprintf("栈已满,无法入栈.\n");;break;case 4:if(Pop_Linkstack(top,&x))printf("出栈成功,出栈元素为:%d\n",x);elseprintf("出栈失败,因栈为空.\n");break;case 5:if(Top_Linkstack(top,&x))printf("取栈顶元素成功,栈顶元素为:%d\n",x);elseprintf("取栈顶元素失败,因栈为空.\n");break;case 6:Printf_Linkstack(top);break;case 7:printf("谢谢使用!\n");break;default :printf("输入错误,请重新输入!\n");break;}}while(choice!=7);return 0;}二.队列1.宏定义2.结构体3.基本函数4.主函数acidity, mL.; M--calibration of the molar concentration of sodium hydroxide standard solution, moI/L; V--amount of the volume of sodium hydroxide standard solution, Ml; M--the weight of the sample, g. Such as poor meets the requirements, take the arithmetic mean of the second determination as a result. Results one decimal. 6, allowing differential analyst simultaneously or in quick succession for the second determination, the absolute value of the difference of the results. Thisvalue should be no more than 1.0. 1, definitions and principles for determination of ash in starches, starch and ash: starch samples of ash the residue obtained after weight. Original sample residue weight of sample weight or weight expressed as a percentage of the dry weight of the sample. Samples of ash at 900 ? high temperature until ashing sample ... The Crucible: determination of Platinum or other conditions of the affected material, capacity of 50mL. Dryer: has effectively adequate drying agent and-perforated metal plate or porcelain. Ashing furnaces: device for controlling and regulating temperature, offers 900 incineration temperature of 25 c. Analytical balance. Electric hot plate or Bunsen. 3, crucible of analysis steps preparation: Crucible mustfirst wash with boiling dilute hydrochloric acid, then wash with a lot of water and then rinse with distilled water. Wash the Crucible within ashing furnace, heated at 900 to 25 ? 30min, and in the desiccator to cool to room temperature and then weighing,。
数据结构 3.1栈和队列(顺序及链栈定义和应用)
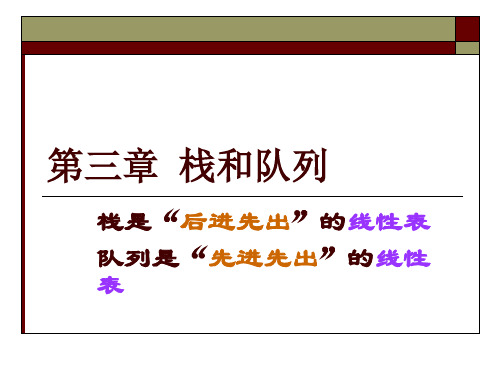
假设从终端接受了这样两行字符: whli##ilr#e(s#*s) outcha@putchar(*s=#++);
则实际有效的是下列两行: while (*s) putchar(*s++);
例4:迷宫求解
通常用 “回溯 试探方 法”求 解
##########
# Q # $ $ $ #
#
# #$ $ $ # #
3.1 栈的类型定义
实例引进 考虑问题:一个死胡同,宽度只能够一辆车进 出,现有三辆汽车依次进入胡同停车,后A车 要离开,如何处理? 用计算机模拟以上问题
小花车
小明家 小花家 能能家 点点家 强强家
小花车
点点车 强强车
基本概念
栈(STACK) ——一种限定性的 数据结构,限定只能在表的一端 进行插入和删除的线性表。
# $ $ # #
#
## ##
##
# #
##
# # #
#
## # ## # # #
#
Q #
##########
求迷宫路径算法的基本思想
若当前位置“可通”,则纳入路径,继续( 向东)前进; 若当前位置“不可通”,则后退,换方向 继续探索; 若四周“均无通路”,则将当前位置从路 径中删除出去。
一 顺序栈
顺序栈存储的特点 顺序栈各个基本操作顺序实现 完整的顺序栈c语言程序 模拟停车场
一 顺序栈
存储特点
利用一组地址连续的存储单元依次存放 自栈底到栈顶的数据元素
c语言中可用数组来实现顺序栈
设置栈顶指针Top
elem[arrmax]
a1 a2 a3 a4
Top
top的值
elem[arrmax]
c语言栈的名词解释

c语言栈的名词解释在计算机科学和编程中,栈(Stack)是一种重要的数据结构。
C语言作为一种广泛应用的编程语言,自然也涉及到栈的概念和使用。
在本文中,将对C语言栈进行详细的名词解释和功能介绍。
1. 栈的定义和特点栈是一种线性的数据结构,它的特点是后进先出(Last In First Out, LIFO)。
也就是说,最后一个进入栈的元素将是第一个被访问、被移除的。
栈采用两个基本操作,即压栈(Push)和弹栈(Pop),用于对数据的插入和删除。
2. 栈的结构和实现方式在C语言中,栈可以用数组或链表来实现。
使用数组实现的栈叫作顺序栈,使用链表实现的栈叫作链式栈。
顺序栈使用数组来存储数据,通过一个指针(栈顶指针)来指示栈顶元素的位置。
当有新元素要进栈时,栈顶指针先向上移动一位,然后将新元素存入该位置。
当要弹栈时,栈顶指针下移一位,表示将栈顶元素移除。
链式栈通过链表来存储数据,每个节点包含一个数据项和一个指向下一个节点的指针。
链式栈通过头指针指示栈顶节点的位置,新元素插入时构造一个新节点,并将其指针指向原栈顶节点,然后更新头指针。
弹栈时,只需将头指针指向下一个节点即可。
3. 栈的应用场景栈在计算机科学中有广泛的应用。
以下是一些常见的应用场景:a. 函数调用:在函数调用过程中,函数的参数、局部变量和返回地址等信息会以栈的形式压入内存中,而在函数返回时将逆序地从栈中弹出这些信息。
b. 表达式求值:中缀表达式在计算机中不方便直接求值,而将中缀表达式转换为后缀表达式后,利用栈可以方便地完成求值过程。
c. 内存分配:在程序运行时,栈用于管理变量和函数的内存分配。
当变量定义时,栈会为其分配内存空间,并在其作用域结束时将其回收。
d. 括号匹配:在处理一些语法相关的问题时,栈可以很好地用来检测括号的匹配情况,例如括号是否成对出现、嵌套层次是否正确等。
4. 栈的复杂度分析栈的操作主要包括入栈和出栈两种操作,它们的时间复杂度均为O(1)。
数据结构(C语言版CHAP3

S.top
n+1 n n-1 i-1 i-2
0
e an ai ai-1 a1
STA作图示
S.top
S.top n n-1 i-1 i-2 S.base 0
an ai ai-1
n+1 n n-1 i-1 i-2
e an
ai ai-1 a1
STACK_INIT_SIZE
3.1 栈
二 栈的基本操作 1) 初始化操作InitStack((&S) 功能:构造一个空栈S; 2) 销毁栈操作DestroyStack(&S) 功能:销毁一个已存在的栈; 3) 置空栈操作ClearStack(&S) 功能:将栈S置为空栈; 4) 取栈顶元素操作GetTop(S, &e) 功能:取栈顶元素,并用e 返回; 5)进栈操作Push(&S, e) 功能:元素e进栈; 6)退栈操作Pop(&S, &e) 功能:栈顶元素退栈,并用e返回; 7)判空操作StackEmpty(S) 功能:若栈S为空,则返回True,否则返回False; 第 9 页
S.top
n n-1
an
i-1 i-2
1 0
ai ai-1
a2 a1
STACK_INIT_SIZE
S.base
S.stacksize
第 13 页
3.1 栈
当栈用顺序结构存储时, 栈的基本操作如建空栈、 进栈、出栈等操作如何实现?
第 14 页
3.1 栈
二 顺序栈基本操作的算法 1)初始化操作InitStack_Sq((SqStack &S)
a1
STACK_INIT_SIZE
S.base S.stacksize
c语言数据结构及算法
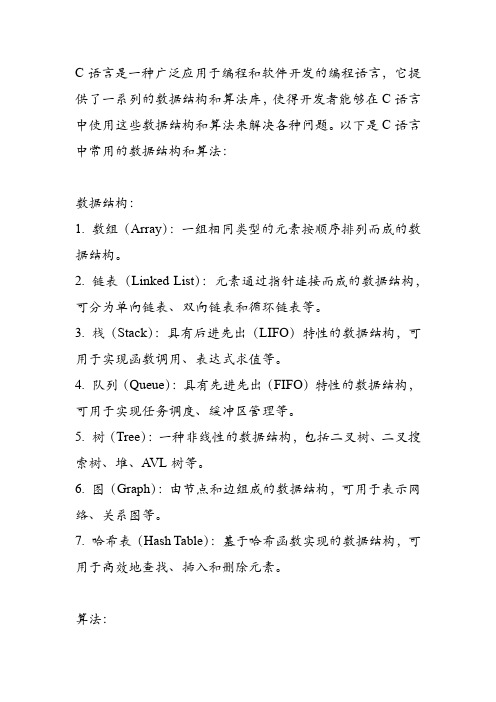
C语言是一种广泛应用于编程和软件开发的编程语言,它提供了一系列的数据结构和算法库,使得开发者能够在C语言中使用这些数据结构和算法来解决各种问题。
以下是C语言中常用的数据结构和算法:数据结构:1. 数组(Array):一组相同类型的元素按顺序排列而成的数据结构。
2. 链表(Linked List):元素通过指针连接而成的数据结构,可分为单向链表、双向链表和循环链表等。
3. 栈(Stack):具有后进先出(LIFO)特性的数据结构,可用于实现函数调用、表达式求值等。
4. 队列(Queue):具有先进先出(FIFO)特性的数据结构,可用于实现任务调度、缓冲区管理等。
5. 树(Tree):一种非线性的数据结构,包括二叉树、二叉搜索树、堆、A VL树等。
6. 图(Graph):由节点和边组成的数据结构,可用于表示网络、关系图等。
7. 哈希表(Hash Table):基于哈希函数实现的数据结构,可用于高效地查找、插入和删除元素。
算法:1. 排序算法:如冒泡排序、插入排序、选择排序、快速排序、归并排序等。
2. 查找算法:如线性查找、二分查找、哈希查找等。
3. 图算法:如深度优先搜索(DFS)、广度优先搜索(BFS)、最短路径算法(Dijkstra、Floyd-Warshall)、最小生成树算法(Prim、Kruskal)等。
4. 字符串匹配算法:如暴力匹配、KMP算法、Boyer-Moore 算法等。
5. 动态规划算法:如背包问题、最长公共子序列、最短编辑距离等。
6. 贪心算法:如最小生成树问题、背包问题等。
7. 回溯算法:如八皇后问题、0-1背包问题等。
这只是C语言中常用的一部分数据结构和算法,实际上还有更多的数据结构和算法可以在C语言中实现。
开发者可以根据具体需求选择适合的数据结构和算法来解决问题。
同时,C语言也支持自定义数据结构和算法的实现,开发者可以根据需要进行扩展和优化。
C语言数据结构_第04讲 栈
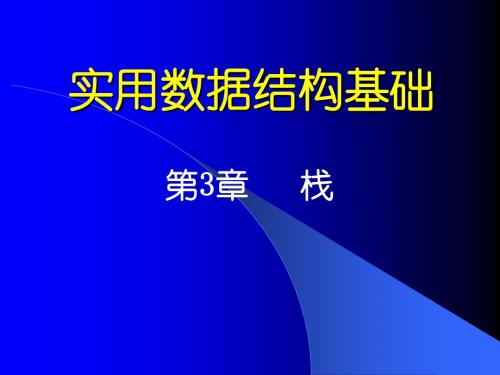
while(n); printf("转换后的二进制数值为:"); while(s.top) // 余数出栈处理 { printf("%d",s.top->data); // 输出栈顶的余数 stacknode* p=s.top; // 修改栈顶指针 s.top=s.top->next; delete p; // 回收一个结点,C语言中用free p } }
3-3-2 表达式求值
表达式是由运算对象、运算符、括号等组成的有意义的式子。 1.中缀表达式(Infix Notation) 一般我们所用表达式是将运算符号放在两运算对象的中 间,比如:a+b,c/d等等,我们把这样的式子称为中缀表达 式。 2.后缀表达式(Postfix Notation) 后缀表达式规定把运算符放在两个运算对象(操作数) 的后面。在后缀表达式中,不存在运算符的优先级问题,也 不存在任何括号,计算的顺序完全按照运算符出现的先后次 次序进行。 3.中缀表达式转换为后缀表达式 其转换方法采用运算符优先算法。转换过程需要两个栈: 一个运算符号栈和一个后缀表达式输出符号栈。
(4)读栈顶元素
datatype ReadTop(SeqStack *s) { if (SEmpty ( s ) ) return 0; // 若栈空,则返回0 else return (s->data[s->top] );
// 否则,读栈顶元素,但指针未移动
}
(5)判栈空
int SEmpty(SeqStack *s) { if (s->top= = –1) return 1; else return 0; }
2.顺序栈运算的基本算法 (1)置空栈 首先建立栈空间,然后初始化栈顶指针。 SeqStack *Snull() { SeqStack *s; s=new (SeqStack);
栈(C语言版)

本章主要介绍下列内容 栈的概念、存储结构及其基本操作 队列的概念、存储结构及其基本操作 栈与队列的应用举例
本章目录
1
2 3
3.1 栈
3.2 队列 3.3 栈和队列的应用举例 3.4 本章小结
4
结束
3.1 栈
3.1.1 栈的概念 3.1.2 栈的基本操作 3.1.3 顺序栈
3.1.4 链栈
返回到总目录
3.1.1 栈的概念
例如,在建筑工地上,使用的砖块从底往上一层一 层地码放,在使用时,将从最上面一层一层地拿取, 这种后进先出的线性结构称为栈(stack),栈又 称为后进先出(last in first out)的线性表,简 称LIFO表。 栈是一种特殊的线性表。其特殊性在于限定插入和 删除数据元素的操作只能在线性表的一端进行。插 入元素又称为进栈,删除元素又称为出栈。允许进 行插入和删除操作的一端称为栈顶(top),另一 端称为栈底(bottom)。处于栈顶位置的数据元 素称为栈顶元素,处于栈底位置的数据元素称为栈 底元素。不含任何数据元素的栈称为空栈。
3.1.3顺序栈
3. 顺序栈的基本操作实现
上述算法顺序栈的基本操作函数,可以对照执行主函数调用后的结果分析, 进一步了解顺序栈的各种操作的过程实现。 (7)设计主函数如下: main() { SeqStack S; DataType x; InitStack(&S); printf("依次进栈元素为:\n"); printf("r元素进栈\n"); Push(&S,'r'); printf("a元素进栈\n"); Push(&S,'a'); printf("h元素进栈\n"); Push(&S,'h'); 返回到本节目录
顺序栈的实现总结(推荐6篇)

顺序栈的实现总结第1篇栈是只允许在一端进行插入或删除的线性表。
注意栈是个操作受限的线性表栈顶:只允许插入或者删除栈底:固定的,不允许进行插入或删除的另一端空栈:不含任何数据元素的栈栈又被称为后进先出的线性表,简称为LIOF(last in first out)顺序栈的实现总结第2篇栈是只允许在一端进行插入或者删除操作的线性表。
如图所示栈顶(Top)。
线性表允许进行插入删除的那一端。
栈底(Bottom)。
固定的,不允许进行插入和删除的另一端。
空栈。
不含任何元素。
假设某个栈S=(a1,a2,a3,a4,a5),如上图所示,则a1为栈底元素,a5为栈顶元素。
由于栈只能在栈顶进行插入和删除操作,进栈次序依次是a1,a2,a3,a4,a5,而出栈次序为a5,a4,a3,a2 ,a1。
栈的操作特性为后进先出。
2.栈的基本操作InitStack(&S):初始化一个空栈S。
StackEmpty(S):判断一个栈是否为空,若为空则返回true,否则返回false。
Push(&S,x):进栈,若栈S未满,则将x加入使之成为新栈顶。
Pop(&S,x):出栈,若栈S非空,则弹出栈顶元素,并用x返回。
GetTop(S,&x):读取栈顶元素,若S非空,则用x返回栈顶元素。
DestroyStack(&S):销毁栈。
顺序栈的实现总结第3篇另外用stacksize表示栈可使用的最大容量简单、方便、但是容易溢出(数组大小固定)base == top是栈空的标志top - base == stacksize是栈满的标志。
具体栈空、栈满的示意图如下1.报错,返回系统2.分配更大的空间,作为栈的存储空间,将原栈的内容移入新栈上溢:栈已经满,又要压入元素下溢:栈已经空,还要弹出元素(注:上溢是一种错误,使问题的处理无法进行;而下溢一般认为是—种结束条件,即问题处理结束。
)bool InitStack(SqStack &S); //1.栈的初始化bool StackEmpty(SqStack S); //2.判断是否为空int StackLength(SqStack S); //3.求栈的长度void DestroyStack(SqStack &S); //4.销毁栈void ClearStack(SqStack &S); //5.清空顺序栈bool Push(SqStack &S, ElemType e); //6.入栈bool Pop(SqStack &S, ElemType &e); //7.出栈bool Gettop(SqStack &S, ElemType &e); //8.得到栈顶元素顺序栈的实现总结第4篇1.顺序栈的实现采用顺序存储的栈称为顺序栈,它利用一组连续的存储单元存放自栈底到栈顶的数据元素,同时附设一个指针top指示前栈顶元素的位置。
(完整版)栈的操作(实验报告)

实验三栈和队列3.1实验目的:(1)熟悉栈的特点(先进后出)及栈的基本操作,如入栈、出栈等,掌握栈的基本操作在栈的顺序存储结构和链式存储结构上的实现;(2)熟悉队列的特点(先进先出)及队列的基本操作,如入队、出队等,掌握队列的基本操作在队列的顺序存储结构和链式存储结构上的实现。
3.2实验要求:(1)复习课本中有关栈和队列的知识;(2)用C语言完成算法和程序设计并上机调试通过;(3)撰写实验报告,给出算法思路或流程图和具体实现(源程序)、算法分析结果(包括时间复杂度、空间复杂度以及算法优化设想)、输入数据及程序运行结果(必要时给出多种可能的输入数据和运行结果)。
3.3基础实验[实验1] 栈的顺序表示和实现实验内容与要求:编写一个程序实现顺序栈的各种基本运算,并在此基础上设计一个主程序,完成如下功能:(1)初始化顺序栈(2)插入元素(3)删除栈顶元素(4)取栈顶元素(5)遍历顺序栈(6)置空顺序栈分析:栈的顺序存储结构简称为顺序栈,它是运算受限的顺序表。
对于顺序栈,入栈时,首先判断栈是否为满,栈满的条件为:p->top= =MAXNUM-1,栈满时,不能入栈; 否则出现空间溢出,引起错误,这种现象称为上溢。
出栈和读栈顶元素操作,先判栈是否为空,为空时不能操作,否则产生错误。
通常栈空作为一种控制转移的条件。
注意:(1)顺序栈中元素用向量存放(2)栈底位置是固定不变的,可设置在向量两端的任意一个端点(3)栈顶位置是随着进栈和退栈操作而变化的,用一个整型量top(通常称top为栈顶指针)来指示当前栈顶位置参考程序:#include<stdio.h>#include<stdlib.h>#define MAXNUM 20#define ElemType int/*定义顺序栈的存储结构*/typedef struct{ ElemType stack[MAXNUM];int top;}SqStack;/*初始化顺序栈*/void InitStack(SqStack *p){ if(!p)printf("Eorror");p->top=-1;}/*入栈*/void Push(SqStack *p,ElemType x){ if(p->top<MAXNUM-1){ p->top=p->top+1;p->stack[p->top]=x;}elseprintf("Overflow!\n");}/*出栈*/ElemType Pop(SqStack *p){ ElemType x;if(p->top!=0){ x=p->stack[p->top];printf("以前的栈顶数据元素%d已经被删除!\n",p->stack[p->top]);p->top=p->top-1;return(x);}else{ printf("Underflow!\n");return(0);}}/*获取栈顶元素*/ElemType GetTop(SqStack *p){ ElemType x;if(p->top!=0){ x=p->stack[p->top];return(x);}else{ printf("Underflow!\n");return(0);}}/*遍历顺序栈*/void OutStack(SqStack *p){ int i;printf("\n");if(p->top<0)printf("这是一个空栈!");printf("\n");for(i=p->top;i>=0;i--)printf("第%d个数据元素是:%6d\n",i,p->stack[i]); }/*置空顺序栈*/void setEmpty(SqStack *p){p->top= -1;}/*主函数*/main(){ SqStack *q;int y,cord;ElemType a;do{printf("\n");printf("第一次使用必须初始化!\n");printf("\n");printf("\n 主菜单\n");printf("\n 1 初始化顺序栈\n");printf("\n 2 插入一个元素\n");printf("\n 3 删除栈顶元素\n");printf("\n 4 取栈顶元素\n");printf("\n 5 置空顺序栈\n");printf("\n 6 结束程序运行\n");printf("\n\n");printf("请输入您的选择( 1, 2, 3, 4, 5,6)");scanf("%d",&cord);printf("\n");switch(cord){ q=(SqStack*)malloc(sizeof(SqStack));InitStack(q);OutStack(q);}break;case 2:{ printf("请输入要插入的数据元素:a=");scanf("%d",&a);Push(q,a);OutStack(q);}break;case 3:{ Pop(q);OutStack(q);}break;case 4:{ y=GetTop(q);printf("\n栈顶元素为:%d\n",y);OutStack(q);}break;case 5:{ setEmpty(q);printf("\n顺序栈被置空!\n");OutStack(q);}break;exit(0);}}while (cord<=6);}[实验2] 栈的链式表示和实现实验内容与要求:编写一个程序实现链栈的各种基本运算,并在此基础上设计一个主程序,完成如下功能:(1)初始化链栈(2)链栈置空(3)入栈(4)出栈(5)取栈顶元素(6)遍历链栈分析:链栈是没有附加头结点的运算受限的单链表。
c语言实现栈详细代码
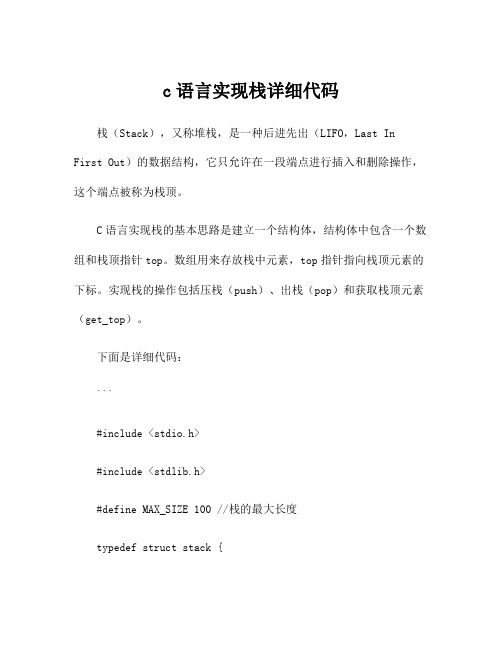
c语言实现栈详细代码栈(Stack),又称堆栈,是一种后进先出(LIFO,Last In First Out)的数据结构,它只允许在一段端点进行插入和删除操作,这个端点被称为栈顶。
C语言实现栈的基本思路是建立一个结构体,结构体中包含一个数组和栈顶指针top。
数组用来存放栈中元素,top指针指向栈顶元素的下标。
实现栈的操作包括压栈(push)、出栈(pop)和获取栈顶元素(get_top)。
下面是详细代码:```#include <stdio.h>#include <stdlib.h>#define MAX_SIZE 100 //栈的最大长度typedef struct stack {int data[MAX_SIZE]; //栈中元素int top; //栈顶指针} Stack;//初始化栈void init(Stack *s) {s->top = -1; //栈顶指针初始化为-1,表示栈为空}//判断栈是否为空int is_empty(Stack *s) {return s->top == -1;}//判断栈是否已满int is_full(Stack *s) {return s->top == MAX_SIZE-1;}//压栈void push(Stack *s, int value) {if (is_full(s)) {printf("Stack is full, cannot push!\n");return;}s->data[++(s->top)] = value; //栈顶指针先加1,再将元素入栈}//出栈void pop(Stack *s) {if (is_empty(s)) {printf("Stack is empty, cannot pop!\n");}s->top--; //栈顶指针减1,表示栈顶元素已删除}//获取栈顶元素int get_top(Stack *s) {if (is_empty(s)) {printf("Stack is empty, cannot get top element!\n"); return -1;}return s->data[s->top]; //返回栈顶元素}int main() {Stack s;init(&s); //初始化栈for (i = 0; i < 5; i++) {push(&s, i); //压入5个元素}printf("Top element: %d\n", get_top(&s)); //获取栈顶元素while (!is_empty(&s)) {printf("%d ", get_top(&s)); //依次输出栈中元素pop(&s); //弹出栈顶元素}return 0;}```代码中定义了一个结构体,包含一个整型数组data和一个整型变量top,数组用来存放栈中元素,top表示栈顶指针。
数据结构c语言版实验报告

数据结构c语言版实验报告数据结构C语言版实验报告一、实验目的本次实验的目的是通过使用C语言编程,实现几种常见的数据结构,包括栈、队列和链表,并进行相关操作的实现和测试。
二、实验内容1. 栈的实现与应用栈是一种先进后出的数据结构,常用于实现递归、表达式求值和内存管理等场景。
在本次实验中,我们使用C语言实现了一个基于数组的顺序栈,并进行了以下操作的实现和测试:- 入栈(push):将元素插入到栈顶。
- 出栈(pop):将栈顶元素删除并返回。
- 获取栈顶元素(top):返回栈顶元素的值。
- 判断栈是否为空(isEmpty):判断栈是否为空栈。
2. 队列的实现与应用队列是一种先进先出的数据结构,常用于实现任务调度、消息传递和缓冲区等场景。
在本次实验中,我们使用C语言实现了一个基于数组的顺序队列,并进行了以下操作的实现和测试:- 入队(enqueue):将元素插入到队尾。
- 出队(dequeue):将队头元素删除并返回。
- 获取队头元素(front):返回队头元素的值。
- 判断队列是否为空(isEmpty):判断队列是否为空队列。
3. 链表的实现与应用链表是一种动态数据结构,常用于实现链式存储和数据的插入、删除等操作。
在本次实验中,我们使用C语言实现了一个单链表,并进行了以下操作的实现和测试:- 头插法(insertAtHead):在链表头部插入元素。
- 尾插法(insertAtTail):在链表尾部插入元素。
- 删除节点(deleteNode):删除指定节点。
- 查找节点(searchNode):查找指定节点的位置。
三、实验结果通过对栈、队列和链表的实现和测试,我们得到了以下实验结果:1. 栈的实现和操作的正确性得到了验证,栈的入栈、出栈、获取栈顶元素和判断是否为空的功能均正常运行。
2. 队列的实现和操作的正确性得到了验证,队列的入队、出队、获取队头元素和判断是否为空的功能均正常运行。
3. 链表的实现和操作的正确性得到了验证,链表的头插法、尾插法、删除节点和查找节点的功能均正常运行。
c语言 所有出栈顺序

在C语言中,可以使用一个二维数组来表示一个栈的所有出栈顺序。
假设栈的最大容量为n,那么二维数组的行数就是n+1行,每一行表示一个入栈顺序,列数也是n+1,表示每个入栈顺序下的出栈顺序。
下面是一个示例代码,可以输出一个栈的所有出栈顺序:```c#include <stdio.h>#define MAX_SIZE 10void print_stack_order(int stack[], int n) {int i, j;for (i = 0; i <= n; i++) {printf("入栈顺序%d:", i);for (j = 0; j <= n; j++) {if (j == n - i) {printf("(%d, %d)", stack[j], n - i);} else {printf("%d ", stack[j]);}}printf("\n");}}int main() {int stack[MAX_SIZE];int n = 5; // 栈的最大容量为5int i;for (i = 0; i <= n; i++) {stack[i] = i; // 初始化栈,每个元素为下标i}print_stack_order(stack, n);return 0;}```在上面的代码中,我们定义了一个函数print_stack_order,用于输出一个栈的所有出栈顺序。
该函数接受两个参数:一个整型数组stack,表示当前栈中的元素;一个整型变量n,表示栈的最大容量。
在函数中,我们使用两个循环来遍历所有入栈顺序和出栈顺序,并输出每个入栈顺序下的出栈顺序。
需要注意的是,在输出出栈顺序时,我们使用了一个判断语句来判断当前元素是否是最后一个出栈的元素,如果是,就输出它和当前的入栈顺序,否则只输出它本身。
数据结构c语言版第三版习题解答

数据结构c语言版第三版习题解答数据结构是计算机科学中非常重要的一门学科,它研究如何在计算机中存储和组织数据,以便有效地进行检索和操作。
数据结构的知识对于编写高效的程序和解决复杂的问题至关重要。
在学习和理解数据结构的过程中,解决习题是一种非常有效的方法。
本文将为读者提供《数据结构C语言版(第三版)》习题的解答。
1. 第一章:绪论第一章主要介绍了数据结构的基本概念和内容,包括算法和数据结构的概念、抽象数据类型(ADT)以及算法的评价等。
习题解答中,我们可以通过分析和讨论的方式对这些概念进行加深理解。
2. 第二章:算法分析第二章主要介绍了算法的基本概念和分析方法,包括时间复杂度和空间复杂度的计算方法。
习题解答中,我们可以通过具体的算法实例来计算其时间和空间复杂度,加深对算法分析的理解。
3. 第三章:线性表第三章主要介绍了线性表的概念和实现,包括顺序表和链表两种实现方式。
习题解答中,我们可以通过编写代码实现线性表的基本操作,并分析其时间和空间复杂度。
4. 第四章:栈和队列第四章主要介绍了栈和队列的概念和实现,包括顺序栈、链栈、顺序队列和链队列四种实现方式。
习题解答中,我们可以通过编写代码实现栈和队列的基本操作,并分析其时间和空间复杂度。
5. 第五章:串第五章主要介绍了串的概念和实现,包括顺序串和链串两种实现方式。
习题解答中,我们可以通过编写代码实现串的基本操作,并分析其时间和空间复杂度。
6. 第六章:树第六章主要介绍了树的概念和实现,包括二叉树、哈夫曼树和赫夫曼编码等内容。
习题解答中,我们可以通过编写代码实现树的基本操作,并分析其时间和空间复杂度。
7. 第七章:图第七章主要介绍了图的基本概念和实现,包括图的表示方法和图的遍历算法等。
习题解答中,我们可以通过编写代码实现图的基本操作,并分析其时间和空间复杂度。
8. 第八章:查找第八章主要介绍了查找算法的基本概念和实现,包括顺序查找、二分查找、哈希查找等内容。
顺序栈的实现代码
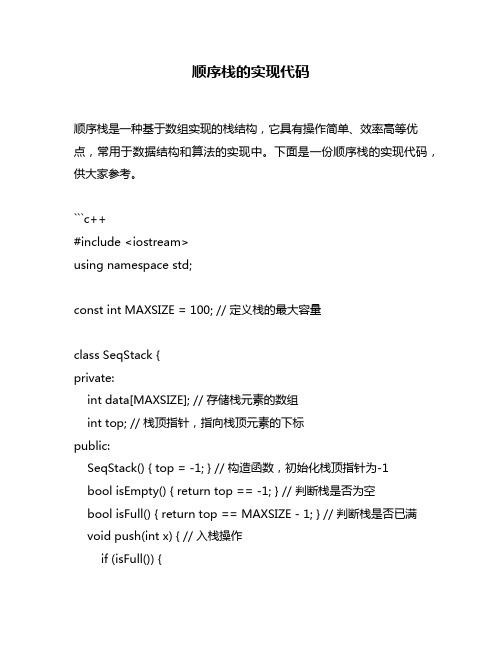
顺序栈的实现代码顺序栈是一种基于数组实现的栈结构,它具有操作简单、效率高等优点,常用于数据结构和算法的实现中。
下面是一份顺序栈的实现代码,供大家参考。
```c++#include <iostream>using namespace std;const int MAXSIZE = 100; // 定义栈的最大容量class SeqStack {private:int data[MAXSIZE]; // 存储栈元素的数组int top; // 栈顶指针,指向栈顶元素的下标public:SeqStack() { top = -1; } // 构造函数,初始化栈顶指针为-1bool isEmpty() { return top == -1; } // 判断栈是否为空bool isFull() { return top == MAXSIZE - 1; } // 判断栈是否已满void push(int x) { // 入栈操作if (isFull()) {cout << "Stack is full!" << endl;return;}data[++top] = x;}int pop() { // 出栈操作if (isEmpty()) {cout << "Stack is empty!" << endl; return -1;}return data[top--];}int getTop() { // 获取栈顶元素if (isEmpty()) {cout << "Stack is empty!" << endl; return -1;}return data[top];}};int main() {SeqStack s;s.push(1);s.push(2);s.push(3);cout << s.pop() << endl; // 输出3cout << s.getTop() << endl; // 输出2s.push(4);cout << s.pop() << endl; // 输出4cout << s.pop() << endl; // 输出2cout << s.pop() << endl; // 输出1cout << s.pop() << endl; // 输出"Stack is empty!"return 0;}```以上是一份简单的顺序栈实现代码,其中包含了栈的基本操作,如入栈、出栈、获取栈顶元素等。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
最近复习C和数据结构,顺序栈是最简单的栈实现。
它是用数组来存放数据,用一个int变量来记录当前栈顶元素的下标,用-1来表示空栈,结构简单。
写一个完整的测试程序,编译器虽然是C++的,但程序是按照C语言规范来写的。
程序实现:依次向栈中压入0~19二十个数字,然后依次出栈并输出。
头文件:SeqStack.h//SeqStack.h#define TRUE 1#define FALSE 0#define STACK_SIZE 20typedef int ElementType;typedef struct{ElementType data[STACK_SIZE];int top;}SeqStack;void InitStack(SeqStack *s) {s->top = -1;}int IsEmpty(SeqStack *s) {if(s->top == -1) return TRUE;return FALSE;}int IsFull(SeqStack *s) {/syntaxhighlighting/OutliningIndicators/InBlock.gif"/> if(s->top == STACK_SIZE-1) return TRUE;return FALSE;}int Push(SeqStack *s, ElementType element) {if(IsFull(s)) return FALSE;s->top ++;s->data[s->top] = element;return TRUE;int Pop(SeqStack *s, ElementType *element) {if(IsEmpty(s)) return FALSE;*element = s->data[s->top];s->top --;return TRUE;}int GetTop(SeqStack *s,ElementType *element) {if(IsEmpty(s)) return FALSE;*element = s->data[s->top];return TRUE;iningIndicators/ExpandedBlockEnd.gif" />}主文件:SeqStackTest.cpp//SeqStackTest.cpp#include <stdio.h>#include "SeqStack.h"void main() {//initializationSeqStack s;InitStack(&s);//inputfor(int i=0; i<20; i++) {Push(&s,i);}//outputwhile(!IsEmpty(&s)) {int rslt;Pop(&s,&rslt);printf("%d ",rslt);}g align="top" alt=""src="/syntaxhighlighting/OutliningIndicators/ExpandedBlockEnd.gif" />}链栈C语言实现顺序栈的实现依靠数组,而数组需要事先声明长度,一次性地静态地分配内存空间。
这样就给我们带来很多不便。
因为我们事先并不能精确地估计栈所需的大小,估计大了浪费空间,估计小了后果就严重了,导致程序无法正常运行。
所以我们通常使用链栈这种数据结构。
链栈用链表作为存储结构,栈初始化时仅需给栈顶指针分配内存空间,而后每当有数据入栈时再为该数据分配空间,这样实现了内存空间的动态分配。
理论上栈的大小可以是无限大的(小心撑爆你的内存o(∩_∩)o)。
不存在顺序栈的诸多问题。
程序实现:依次把0~99压栈,再依次出栈并打印。
程序清单:LinkStack.h LinkStackTest.cLinkStack.h/**//* LinkStack.h */#include <stdlib.h>#define TRUE 1#define FALSE 0#define NULL 0typedef int ElementType;typedef struct node ...{ElementType data;struct node *next;}StackNode, *LinkStack;void InitStack(LinkStack top) ...{top->next = NULL;}int IsEmpty(LinkStack top) ...{if(top->next == NULL) return TRUE;return FALSE;}int Push(LinkStack top, ElementType element) ...{ StackNode *temp;temp = (StackNode *)malloc(sizeof(StackNode));if(temp == NULL) return FALSE;temp->data = element;temp->next = top->next;top->next = temp;return TRUE;}int Pop(LinkStack top, ElementType *element) ...{ if(IsEmpty(top)) return FALSE;StackNode *temp = top->next;*element = temp->data;top->next = temp->next;free(temp);return TRUE;}void GetTop(LinkStack top, ElementType *element) ...{ *element = top->next->data;}LinkStackTest.c/**//* LinkStackTest.c */#include <stdio.h>#include "LinkStack.h"void main() ...{LinkStack s;s = (LinkStack)malloc(sizeof(StackNode));InitStack(s);for(int i=0; i<100; i++)Push(s,i);int rslt;while (!IsEmpty(s)) ...{Pop(s,&rslt);printf("%d ",rslt);}}两个链表的交叉合并#include<stdio.h>#include<stdlib.h>typedef struct Node{int data;Node *next;} node,*linklist;/*该函数用来创建链表*/linklist creat(linklist head){node *r,*s;int a;r = (linklist)malloc(sizeof(node));head = r;scanf("%d",&a);while(a != 0){s =(node*)malloc(sizeof(node));s->data=a;r->next=s;r=s;printf("please input a data:");scanf("%d",&a);}r->next=NULL;return head;}/*用于实现链表A,B的组合*/linklist mergel(linklist A,linklist B){node *p,*q,*s,*t;linklist C;p=A->next;q=B->next;C=A;while(p&&q){s=p->next;p->next=q;if(s){t=q->next;q->next=s;}p=s;q=t;}return C;}int main(){linklist p,A,B,C;printf("Creat linklist A:");A = creat(A);printf("Creat linklist B:");B = creat(B);C = mergel(A,B);p = C->next;while(p){printf("%d\n",p->data);p = p->next;}printf("Over\n");getchar();getchar();return 0;}。