第十三章 输入输出流
教育心理学考试重点提示第十三章课堂管理
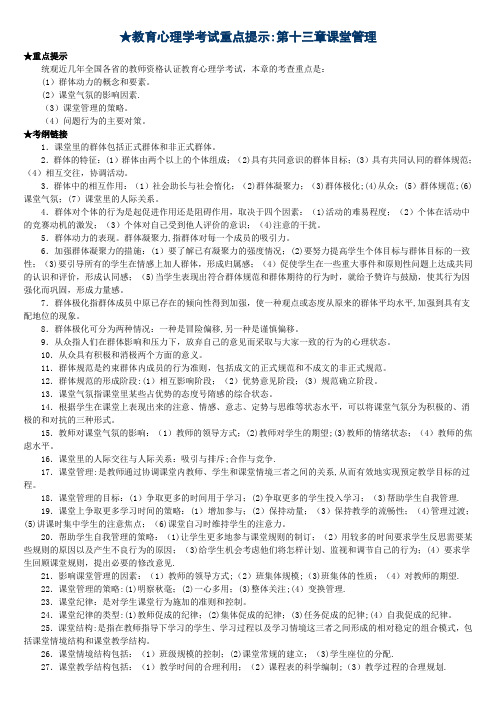
★教育心理学考试重点提示:第十三章课堂管理
★重点提示
统观近几年全国各省的教师资格认证教育心理学考试,本章的考查重点是:
(1)群体动力的概念和要素。
(2)课堂气氛的影响因素.
(3)课堂管理的策略。
(4)问题行为的主要对策。
★考纲链接
1.课堂里的群体包括正式群体和非正式群体。
2.群体的特征:(1)群体由两个以上的个体组成;(2)具有共同意识的群体目标;(3)具有共同认同的群体规范;(4)相互交往,协调活动。
3.群体中的相互作用:(1)社会助长与社会惰化;(2)群体凝聚力;(3)群体极化;(4)从众;(5)群体规范;(6)课堂气氛;(7)课堂里的人际关系。
4.群体对个体的行为是起促进作用还是阻碍作用,取决于四个因素:(1)活动的难易程度;(2)个体在活动中的竞赛动机的激发;(3)个体对自己受到他人评价的意识;(4)注意的干扰。
5.群体动力的表现。群体凝聚力,指群体对每一个成员的吸引力。
6.加强群体凝聚力的措施:(1)要了解已有凝聚力的强度情况;(2)要努力提高学生个体目标与群体目标的一致性;(3)要引导所有的学生在情感上加人群体,形成归属感;(4)促使学生在一些重大事件和原则性问题上达成共同的认识和评价,形成认同感;(5)当学生表现出符合群体规范和群体期待的行为时,就给予赞许与鼓励,使其行为因强化而巩固,形成力量感。
7.群体极化指群体成员中原已存在的倾向性得到加强,使一种观点或态度从原来的群体平均水平,加强到具有支配地位的现象。
8.群体极化可分为两种情况:一种是冒险偏移,另一种是谨慎偏移。
《输入输出流 》课件
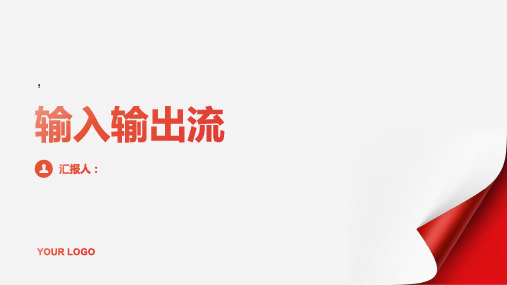
确保输入输出流的缓冲区大小合适,避免 数据传输过程中的延迟和阻塞
避免在输入输出流中直接进行字符串操作, 可能导致数据损坏或丢失
确保输入输出流的安全性,避免数据泄露 或被恶意攻击
确保输入输出流的性能优化,提高数据传 输效率和稳定性
异常类型:文件不存在、文件损坏、文件权限问题等 异常处理方法:使用try-catch语句捕获异常,并处理异常 异常处理策略:关闭文件、重新打开文件、重新读取文件等 异常处理示例:使用try-catch语句捕获文件不存在异常,并提示用户重新选择文件
更新数据:更新 数据库中的数据, 如修改记录中的 某个字段
删除数据:删除 数据库中的记录, 如删除某个表中 的所有记录
输入流和输出流的作用:实现数据在程序和外部数据源之间的传输和交换
输入流和输出流的分类:根据数据类型和传输方式,可以分为多种类型,如文件流、网 络流、内存流等
数据传输:实现程序与外部设备 之间的数据交换
错误处理:捕获和处理输入输出 过程中的错误
添加标题
添加标题
添源自文库标题
添加标题
程序控制:通过输入输出流控制 程序的执行流程
输出:计算机将处理后的数据通过显示器、打印机等设备输出
案例分析:用户界面显示是一个典型的输入输出流案例,用户可以通过键盘、鼠标等设备输入数 据,计算机将处理后的数据通过显示器、打印机等设备输出,实现了人机交互。
基础护理学第十三章 静脉输液与输血
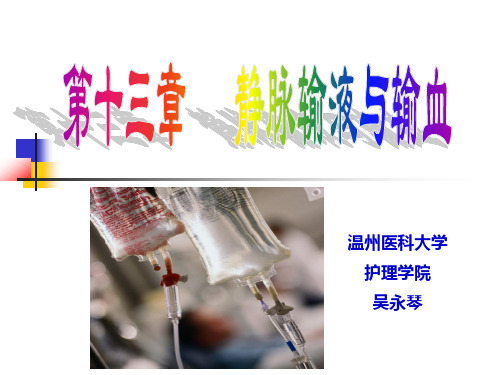
④静脉痉挛 用热水袋或热毛巾热敷注射部位 上端血管,可以缓解静脉痉挛。 ⑤压力过低及其他原因 当输液管折叠及病人姿势改变等 时也会出现输液溶液不滴。
输液反应及护理*
发热反应 fever reaction 循环负荷过重反应(急性肺水肿)
circulatory overload reaction
静脉炎 phlebitis 空气栓塞 air embolism
静脉高营养液
常用溶液有 供给患者热能, 复方氨基酸 (CoAA) 维持正氮平衡, 脂肪乳剂等 补充维生素和矿物质。
周围静脉输液法
颈外静脉穿刺置管输液法
锁骨下静脉穿刺置管输液法
PICC
周围静脉输液法
评估
实施
计划
评价
周围静脉输液法评估
病人状况 病人的用药史和目前用药情况 病人的心理状态及合作程度 穿刺部位皮肤、血管状况、肢体活动度
胶体溶液(colloidal solution)
分子大,存留时间长, 可维持血浆胶体渗透压, 增加血容量,改善微循环, 提高血压。
常用的胶体溶液
低分子右旋糖酐4万
改善微循环 和抗血栓形 提高血浆胶 成 中分子右旋糖酐7.5万 渗压、扩充 血容量 增加循环血量和 代血浆 心输出量在急性 提高血浆胶体渗透压 大出血时可与全 增加循环血容量 血共用。 补充蛋白质和抗体 血液制品
第13章 双口网络
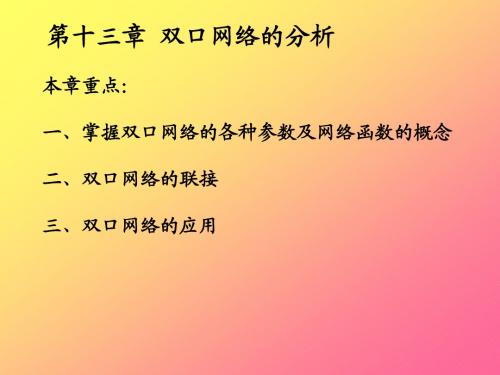
互易时:h21=-h12;对称时: h21=-h12 ,H=1
另一种混合参数G参数:
I1 g11 U1 g12 I 2 U 2 g 21 U1 g 22 I 2
注意:1、记住各方程的形式(先1后2,先U后I)
2、重点:Y、Z、T、 三、参数的物理意义和相互关系 1、物理意义: Y 参 数
1 r 则 Zi 2 G ZL ZL
2
+ u1 _
1
G
i2 ZL
+ u2 _
§13-6
互易定理
互易网络
网络内无独立源和受控源,只有 R 、 L 、 C 、 M 、 T 元件,称为互易网络 互易定理:单个激励的互易网络,激励电流(电 压)和响应电流(电压)可互易位置。 1 2 第一种: + I2 U S1 互易网络 (注意方向都是关 _ ’ 联的) ’ 2 1
I2 U2
U 1 0
短路输出导纳
Y参数称为短路导纳参数
Z 参 数
Z11
U1 Z11 I I Z12 I 2 U 2 Z 21 I1 Z 22 I 2
Z参数称为开路阻抗参数
U1 I1 U1 I2
I 1 0
开路输入阻抗
+ 1
U1
ຫໍສະໝຸດ Baidu
13.3C++标准输出流详解
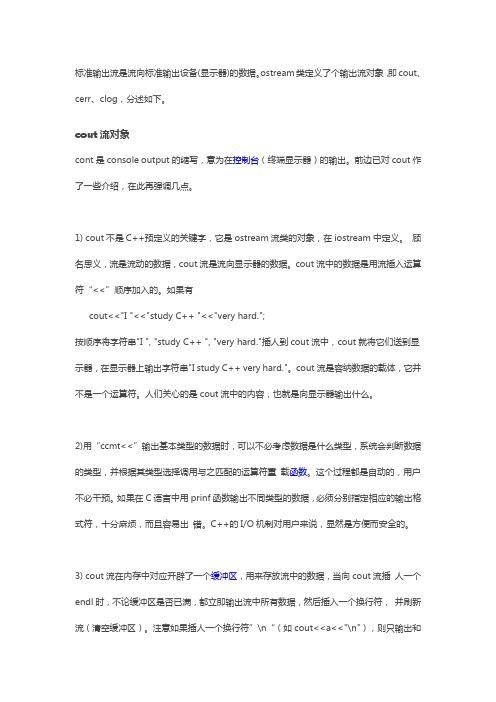
标准输出流是流向标准输出设备(显示器)的数据。ostream类定义了个输出流对象,即cout、cerr、clog,分述如下。
cout流对象
cont是console output的缩写,意为在控制台(终端显示器)的输出。前边已对cout作了一些介绍,在此再强调几点。
1) cout不是C++预定义的关键字,它是ostream流类的对象,在iostream中定义。顾名思义,流是流动的数据,cout流是流向显示器的数据。cout流中的数据是用流插入运算符“<<”顺序加入的。如果有
cout<<"I "<<"study C++ "<<"very hard.";
按顺序将字符串"I ", "study C++ ", "very hard."插人到cout流中,cout就将它们送到显示器,在显示器上输出字符串"I study C++ very hard."。cout流是容纳数据的载体,它并不是一个运算符。人们关心的是cout流中的内容,也就是向显示器输出什么。
2)用“ccmt<<”输出基本类型的数据时,可以不必考虑数据是什么类型,系统会判断数据的类型,并根据其类型选择调用与之匹配的运算符重载函数。这个过程都是自动的,用户不必干预。如果在C语言中用prinf函数输出不同类型的数据,必须分别指定相应的输出格式符,十分麻烦,而且容易出错。C++的I/O机制对用户来说,显然是方便而安全的。
3) cout流在内存中对应开辟了一个缓冲区,用来存放流中的数据,当向cout流插人一个endl时,不论缓冲区是否已满,都立即输出流中所有数据,然后插入一个换行符,并刷新流(清空缓冲区)。注意如果插人一个换行符”\n“(如cout<<a<<"\n"),则只输出和
Java程序员认证考试题
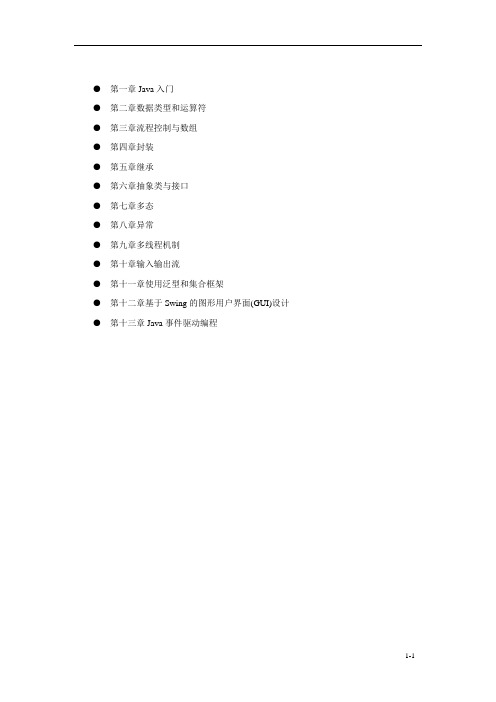
●第一章Java入门
●第二章数据类型和运算符
●第三章流程控制与数组
●第四章封装
●第五章继承
●第六章抽象类与接口
●第七章多态
●第八章异常
●第九章多线程机制
●第十章输入输出流
●第十一章使用泛型和集合框架
●第十二章基于Swing的图形用户界面(GUI)设计●第十三章Java事件驱动编程
第一章练习题(Java入门)
1.下列哪项不是JDK所包含的内容?(选一项)A.Java编程语言
B.工具及工具的API
C.Java EE扩展API
D.Java平台虚拟机
2.下列关于JDK、JRE和JVM的描述。哪项正确?A.JDK中包含了JRE,JVM中包含了JRE
B.JRE中包含了JDK,JDK中包含了JVM
C.JRE中包含了JDK,JVM中包含了JRE
D.JDK中包含了JRE,JRE中包含了JVM
3.下列哪个工具可以编译java源文件?
A.javac
B.jdb
C.javadoc
D.junit
4.JDK工具javadoc的作用是哪项?
A.生成Java文档
B.编译Java源文件
C.执行Java类文件
D.测试Java代码
5.以下哪些包是Java标准库中常用的包?(选三项)A.java.lang
B.javax.servlet .http
C.j ava. io
D.java.sql
6.使用JDK工具生成的Java文档的文件格式是?
A.XML格式
B.自定义格式
c.二进制格式
D.HTML格式
7.以下关于JVM的叙述,哪项正确?(选两项)
A.JVM运行于操作系统之上,它依赖于操作系统
B.JVM运行于操作系统之上,它与操作系统无关
戴红伟老师课件第13章 输入输出流 opload
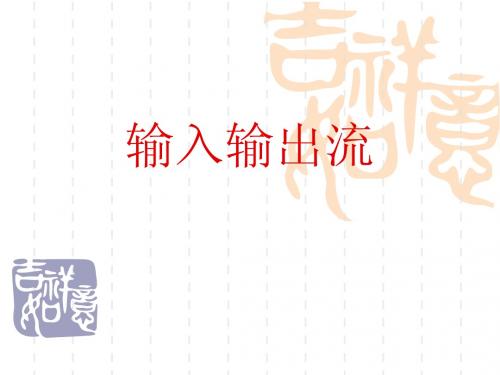
2. 关闭磁盘文件
在对已打开的磁盘文件的读写操作完成后,应关 闭该文件。关闭文件用成员函数close。如
outfile.close( );//将输出文件流所关联的磁盘文件关闭
关闭后,可以将文件流与其他磁盘文件建立关联, 通过文件流对新的文件进行输入或输出。如
outfile.open(″f2.dat″,ios::app|ios::nocreate);
如何定义流对象
可以用下面的方法建立一个输出文件流对 象: ofstream outfile; //定义输出流对象 但是有一个问题还未解决: 在定义cout时 已将它和标准输出设备建立关联,而现在 虽然建立了一个输出文件流对象,但是还 未指定它向哪一个磁盘文件输出,需要在 使用时加以指定。
13.4.3 文件的打开与关闭
13.4.2 文件流类与文件流对象
文件流是以外存文件为输入输出对象的数 据流。 输出文件流是从内存流向外存文件的数据, 输入文件流是从外存文件流向内存的数据。
稍显别扭。输出和输 入是针对内存而言。
要以磁盘文件为对象进行输入输出,必须 定义一个文件流类的对象,通过文件流对 象将数据从内存输出到磁盘文件,或者通 过文件流对象从磁盘文件将数据输入到内 存。 在用标准设备为对象的输入输出中,也是 要定义流对象的,如cin,cout就是流对象, C++是通过流对象进行输入输出的。
Sun_Java程序员认证考试题
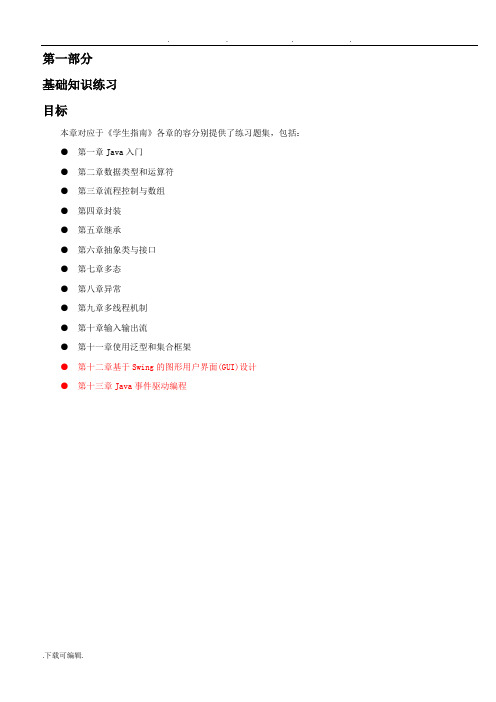
第一部分
基础知识练习
目标
本章对应于《学生指南》各章的容分别提供了练习题集,包括:
●第一章Java入门
●第二章数据类型和运算符
●第三章流程控制与数组
●第四章封装
●第五章继承
●第六章抽象类与接口
●第七章多态
●第八章异常
●第九章多线程机制
●第十章输入输出流
●第十一章使用泛型和集合框架
●第十二章基于Swing的图形用户界面(GUI)设计
●第十三章Java事件驱动编程
第一章练习题(Java入门)
1.下列哪项不是JDK所包含的容?(选一项)
A.Java编程语言
B.工具及工具的API
C.Java EE扩展API
D.Java平台虚拟机
2.下列关于JDK、JRE和JVM的描述。哪项正确?
A.JDK中包含了JRE,JVM中包含了JRE
B.JRE中包含了JDK,JDK中包含了JVM
C.JRE中包含了JDK,JVM中包含了JRE
D.JDK中包含了JRE,JRE中包含了JVM
3.下列哪个工具可以编译java源文件?
A.javac
B.jdb
C.javadoc
D.junit
4.JDK工具javadoc的作用是哪项?
A.生成Java文档
B.编译Java源文件
C.执行Java类文件
D.测试Java代码
5.以下哪些包是Java标准库中常用的包?(选三项) A.java.lang
B.javax.servlet .http
C.j ava. io
D.java.sql
6.使用JDK工具生成的Java文档的文件格式是?
A.XML格式
B.自定义格式
c.二进制格式
D.HTML格式
7.以下关于JVM的叙述,哪项正确?(选两项)
A.JVM运行于操作系统之上,它依赖于操作系统
电工学(电工技术)第七版 上册 第十三章 电子教案课件
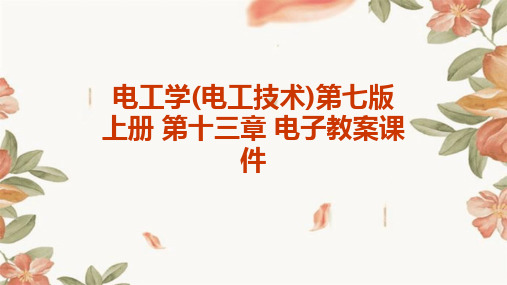
感谢您的观看
THANKS
。
答案3
共射极放大电路的电压放大倍数由三极管的 电流放大倍数、集电极电阻和基极电阻共同 决定,计算公式为$A_{v} = frac{V_{o}}{V_{i}} = frac{I_{c}R_{c}}{I_{b}R_{b}}$。
答案4
设计一个简单的音频放大器,可以通过选择 合适的晶体管、电阻和电容等元件来实现, 具体电路图和计算过程略。
放大电路的分析方法主要包括直流通路分析和交流通路分析,通过 这两种方法可以确定放大电路的性能参数。
集成运算放大器的应用
1 2 3
集成运算放大器的特点
集成运算放大器是一种高精度的放大器件,具有 低噪声、低失真、高带宽等优点,广泛应用于各 种模拟电路和数字电路中。
集成运算放大器的典型应用
集成运算放大器可以用于实现各种模拟运算功能 ,如加减法、乘法、除法等,还可以用于信号处 理、滤波器设计等领域。
培养学生对电子技术的兴 趣和探究精神。
章节教学内容
电子元件的种类和特性
包括电阻、电容、电感、二极管、晶体管等 。
电子电路的分析方法
包括欧姆定律、基尔霍夫定律、叠加定理等 。
电子电路的基本组成
包括电源、负载、导线和开关等。
电子电路的设计原则
包括优化电路性能、减小功耗、提高稳定性 等。
02
第十三章的基础知识
C语言的输入输出流
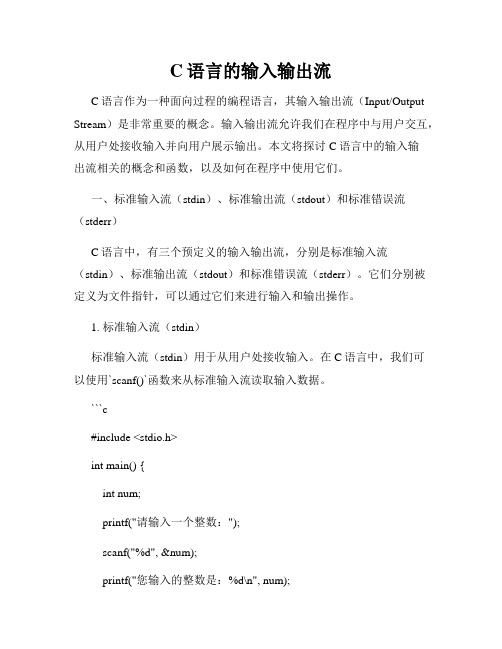
C语言的输入输出流
C语言作为一种面向过程的编程语言,其输入输出流(Input/Output Stream)是非常重要的概念。输入输出流允许我们在程序中与用户交互,从用户处接收输入并向用户展示输出。本文将探讨C语言中的输入输
出流相关的概念和函数,以及如何在程序中使用它们。
一、标准输入流(stdin)、标准输出流(stdout)和标准错误流(stderr)
C语言中,有三个预定义的输入输出流,分别是标准输入流(stdin)、标准输出流(stdout)和标准错误流(stderr)。它们分别被
定义为文件指针,可以通过它们来进行输入和输出操作。
1. 标准输入流(stdin)
标准输入流(stdin)用于从用户处接收输入。在C语言中,我们可
以使用`scanf()`函数来从标准输入流读取输入数据。
```c
#include
int main() {
int num;
printf("请输入一个整数:");
scanf("%d", &num);
printf("您输入的整数是:%d\n", num);
return 0;
}
```
在这个例子中,`scanf()`函数用于从标准输入流(stdin)中读取一个整数,并将其存储到变量`num`中。然后,我们使用`printf()`函数将输入的整数输出到标准输出流(stdout)上。
2. 标准输出流(stdout)
标准输出流(stdout)用于向用户展示输出。在C语言中,我们可以使用`printf()`函数向标准输出流打印输出。
```c
#include
int main() {
2020年智慧树知道网课《基于任务的Java程序设计》课后章节测试满分答案
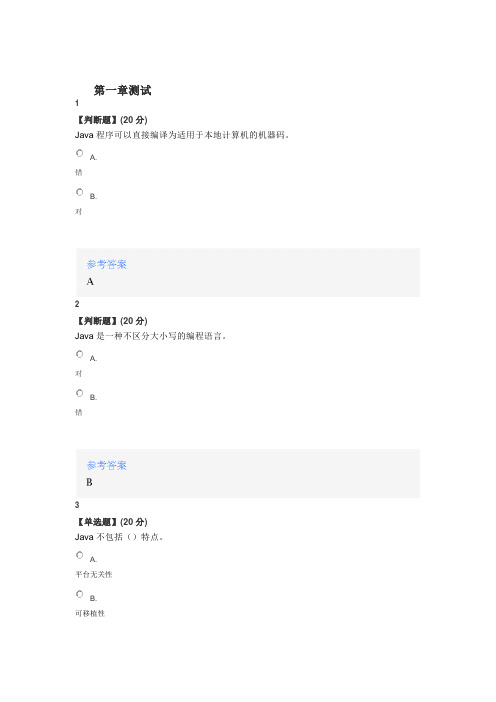
第一章测试
1
【判断题】(20分)
Java程序可以直接编译为适用于本地计算机的机器码。
A.
错
B.
对
2
【判断题】(20分)
Java是一种不区分大小写的编程语言。
A.
对
B.
错
3
【单选题】(20分)
Java不包括()特点。
A.
平台无关性
B.
可移植性
C.
分布性
D.
面向过程
4
【单选题】(20分)
Java源程序的扩展名为()
A.
.class
B.
.js
C.
.java
D.
.jav
5
【单选题】(20分)
Java编译成功后生成文件的扩展名为()
A.
.java
B.
.js
C.
.class
D.
.jav
第二章测试
1
【单选题】(20分)
下列标识符名称不合法是()
A.
true
B.
$main
C.
square
D.
_123
2
【单选题】(20分)
下列选择中,不属于Java语言的简单数据类型的是()
A.
数组
B.
浮点型
C.
字符型
D.
整数型
3
【单选题】(20分)
下列名称不是Java语言中的关键字的是()
A.
private
B.
sizeof
C.
if
4
【判断题】(20分)
在Java程序中要使用一个变量,必须先对其进行声明()
A.
错
B.
对
5
【判断题】(20分)
以0x或0X开头的整数(如0x45)代表八进制整型常量()
A.
错
B.
对
第三章测试
1
【判断题】(20分)
简单if结构是顺序程序结构
A.
对
B.
错
2
【判断题】(20分)
多重if-else分支结构中的大括号不能省略
A.
对
B.
错
3
【判断题】(20分)
switchcase结构中的default为必选参数,必须得写上,否则程序会出错
A.
对
B.
错
4
【单选题】(20分)
在流程图中,下面说法正确的是()
13 第十三章 基于DSPBIOS测试FIR数字滤波器程序(20张)
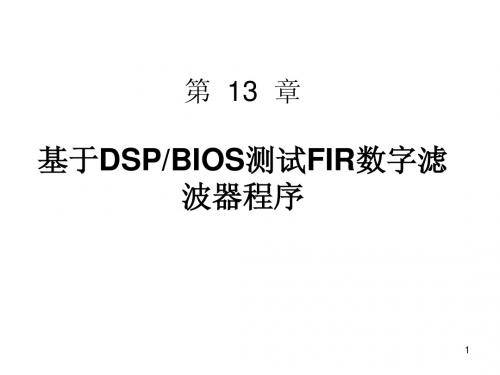
统计模块STS的传输机制
12
用DSP/BIOS模块测试FIR数字滤波 器程序
• • • • • 创建关于FIR数字滤波器程序的DSP/BIOS工程文件 为FIR数字滤波器程序添加DSP/BIOS模块函数 为FIR数字滤波器程序添加DSP/BIOS模块对象 用统计观察窗观察FIR数字滤波器程序的统计数据 用Message Log显示FIR数字滤波器程序的统计信息
14
变化后整个程序流程图
15
创建关于FIR数字滤波器程序的DSP/BIOS工程文件
• 为程序添加 为程序添加STS模块函数 模块函数 • 为程序添加 为程序添加LOG模块函数 模块函数 • 为程序添加头文件及声明函数
16
为FIR数字滤波器程序添加DSP/BIOS模块对象
• 在对FIR数字滤波器程序做了上小节中的改变后,如果在这时编译, 是会出错的。因为还没有在DSP/BIOS的配置文件中定义程序中所用 到的模块对象。 • 打开项目工程中的“firbios.cdb”文件,为程序添加如下模块对象: (1)添加LOG模块对象 (2)添加STS模块对象 (3)添加CLK模块对象 (4) 添加SWI模块对象
源自文库10
DSP/BIOS测试模块——LOG
• 在目标DSP内,LOG对象将要监控的信息实时的捕捉,并存放在DSP 的特定缓冲LOG BUFFER中,在DSP进入空闲的时刻,通过JTAG接 口传回主机,主机端的CCS集成环境将接收数据并显示在打开的LOG 观察窗口(DSP/BIOS菜单下的Message Log)中,从而在不打断正 常的程序运行情况下,获取必要的调试信息 。
c++面向对象程序设计第三版谭浩强教学大纲(完整版)
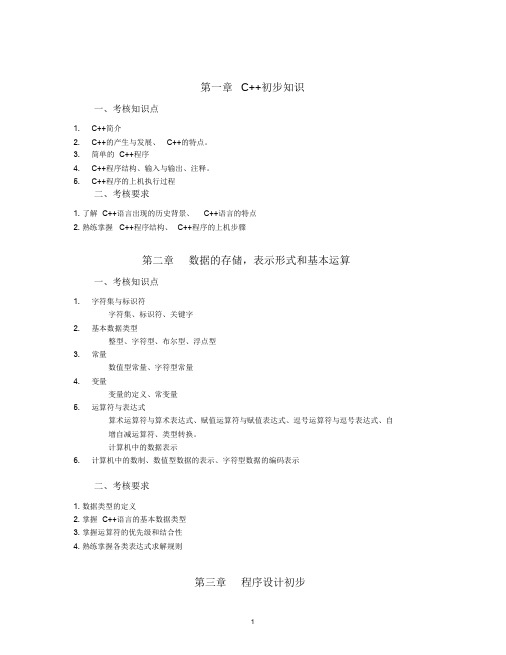
第一章C++初步知识
一、考核知识点
1. C++简介
2. C++的产生与发展、C++的特点。
3. 简单的C++程序
4. C++程序结构、输入与输出、注释。
5. C++程序的上机执行过程
二、考核要求
1. 了解C++语言出现的历史背景、C++语言的特点
2. 熟练掌握C++程序结构、C++程序的上机步骤
第二章数据的存储,表示形式和基本运算
一、考核知识点
1. 字符集与标识符
字符集、标识符、关键字
2. 基本数据类型
整型、字符型、布尔型、浮点型
3. 常量
数值型常量、字符型常量
4. 变量
变量的定义、常变量
5. 运算符与表达式
算术运算符与算术表达式、赋值运算符与赋值表达式、逗号运算符与逗号表达式、自
增自减运算符、类型转换。
计算机中的数据表示
6. 计算机中的数制、数值型数据的表示、字符型数据的编码表示
二、考核要求
1. 数据类型的定义
2. 掌握C++语言的基本数据类型
3. 掌握运算符的优先级和结合性
4. 熟练掌握各类表达式求解规则
第三章程序设计初步
6. 功能语句与顺序结构程序设计
赋值语句、空语句、复合语句、顺序结构程序设计
7. 分支语句与分支结构程序设计
关系表达式和逻辑表达式、if 语句、if ⋯else 语句、条件运算符与条件表达式、switch 语句
8. 循环语句与循环结构程序设计
for 循环语句、do⋯while 循环语句、while 循环语句、循环语句的嵌套
9. 转移语句
break 语句、continue 语句、goto 语句
10. 程序举例
。
算法与程序设计、算法设计与分析、程序设计风格
二、考核要求
输入输出数据流
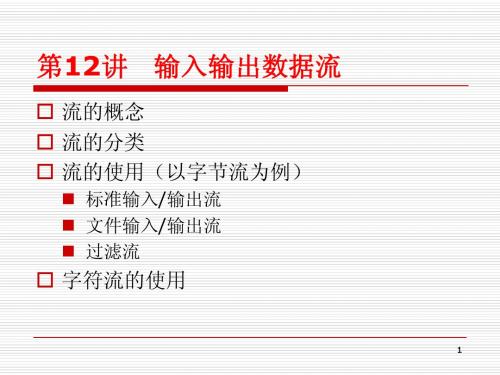
3Байду номын сангаас
流的概念
源
输入流的源可以是文件、标准输入(键盘)、 其他外部输入设备或者其他输入流。
目的地
输出流的目的地可以是文件、标准输出(显示 器)、其他外部输出设备或者其他输出流。
Java中输入输出是通过流来实现的。相关的 类都在java.io包中。
4
流的分类
输入流/输出流
按流与程序的关系分。如前所述。
14
本讲学习任务之二:
学会如何对文件进行读写操作 任务:编写一个程序,统计一个磁盘文本文 件中的单词数。
15
从文件读数据:FileInputStream
InputStream是抽象类,不能直接用new InputStream()构 造实例。 FileInputStream是InputStream的子类,可以生 成实例。 FileInputStream有三个构造方法,最常用的构造方 法如下: Public FileInputStream(String fileName) throws FileNotFoundException
2
流(Stream)的概念 )
流是从源到目的地的有序字节序列,具有先进先出的特征。 是从源 目的地的有序字节序列,具有先进先出的特征。 先进先出的特征 输入流和 两类。 根据流与程序的关系将流分为输入流 输出流两类 根据流与程序的关系将流分为输入流和输出流两类。 程序从输入流读取数据;向输出流写出数据。 读取数据 写出数据 程序从输入流读取数据;向输出流写出数据。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
第十三章 输入输出流
1. 输入三角形的三边a,b,c,计算三角形的面积公式是
构成三角形的条件是:a+b>c,b+c>a,c+a>b
编写程序,输入a,b,c是否满足以上条件,如不满足,由cerr输出有关出错信息。
#include <iostream>
#include <cmath>
using namespace std;
int main()
{
double a,b,c,s,area;
cout<<"Please input the length of 3 sides:"<<endl;
cout<<"a = ";
cin>>a;
cout<<"b = ";
cin>>b;
cout<<"c = ";
cin>>c;
if(a+b>c && b+c>a && a+c>b)
s = (a+b+c)/2.0;
else
{
cerr<<"The 3 sides can not form a triangle!"<<endl;
exit(1);
}
area = sqrt(s*(s-a)*(s-b)*(s-c));
cout<<"The area of the triangle is: "<<area<<endl;
return 0;
}
/* 运行结果
* chinsung@gentoo ~ $ g++ test.cpp
* chinsung@gentoo ~ $ ./a.out
* Please input the length of 3 sides:
* a = 3
* b = 1
* c = 1
* The 3 sides can not form a triangle!
* chinsung@gentoo ~ $ ./a.out
* Please input the length of 3 sides:
* a = 3
* b = 4
* c = 5
* The area of the triangle is: 6
*/
143
area= s s?a s?b s?c
s=abc
2
第十三章 输入输出流
2. 从键盘输入一批数值,要求保留3位小数,在输出时上下行小数点对齐。
#include <iostream>
#include <iomanip>
using namespace std;
struct Double_number
{
double d;
Double_number* next;
};
void input_node(Double_number* p)
{
cout<<"Please input a number(0 for end): ";
cin>>p->d;
}
int main()
{
Double_number *head,*p1,*p2;
head=p1=p2=new Double_number;
input_node(head);
while(p2->d!=0)
{
p1=new Double_number;
input_node(p1);
p2->next=p1;
p2=p1;
}
p2->next=NULL;
p1=head;
while(p1->d!=0)
{
cout<<setw(10)<<setiosflags(ios::fixed)<<setprecision(3)<<p1->d<<endl;
p1=p1->next;
}
return 0;
}
/* 本题用链表完成,以下为运行结果:
* Please input a number(0 for end): 12.5
* Please input a number(0 for end): 126.3
* Please input a number(0 for end): 1.023
* Please input a number(0 for end): 0
* 12.500
* 126.300
* 1.023
*/
3. 编程序,在显示屏上显示一个由字母B组成的三角形。
B
BBB
BBBBB
BBBBBBB
BBBBBBBBB
BBBBBBBBBBB
BBBBBBBBBBBBB
BBBBBBBBBBBBBBB
#include <iostream>
144
第十三章 输入输出流
using namespace std;
int main()
{
int i,j,k;//will be used all over the function, so declared here
for(i=0;i<8;i++)//number of rows
{
for(j=0;j<7-i;j++)//number of whitespaces
cout<<' ';
for(k=0;k<2*(8-j)-1;k++)//number of Bs
cout<<'B';
cout<<endl;
}
return 0;
}
4. 建立两个磁盘文件f1.dat和f2.dat,编程序实现以下工作:
1. 从键盘输入20个整
数,分别存放在两个磁盘文件中(每个文件中放10个整数);
2. 从f1.dat读入10个数,然后存放到f2.dat文件原有数据的后面;
3. 从f2.dat中读入20个整数,对它们按从小到大的顺序存放到f2.dat中,不保留原来的数据。
//Question No.1
#include <iostream>
#include <fstream>
using namespace std;
int main
()
{
const int Len=20;
int a[Len],i,j,temp;//to store Len integers
fstream file1("f1.dat",ios::out),file2("f2.dat",ios::out);
if(file1==0 || file2==0)//if fail to open, the object==0
{
cout<<"Error while opening f1.dat or f2.dat!";
exit(1);
}
cout<<"Please input Len integers: "<<endl;
for(i=0;i<Len;i++)
{
cout<<"No."<<i+1<<": ";
cin>>a[i];
if(i<10)
file1<<a[i]<<' ';
else
file2<<a[i]<<' ';
}
file1.close();
file2.close();
//Question No.2
file1.open("f1.dat",ios::in);
file2.open("f2.dat",ios::out|ios::app);
if(file1==0 || file2==0)//if fail to open, the object==0
{
cout<<"Error while opening f1.dat or f2.dat!";
exit(1);
}
for(i=0;i<10;i++)
{
file1>>a[i];
file2<<a[i]<<' ';
}
145
第十三章 输入输出流
file1.close();
file2.close();
//Question No.3
file2.open("f2.dat",ios::in);
if(file2==0)
{
cout<<"Error while opening f2.dat!";
exit(1);
}
for(i=0;i<Len;i++)
file2>>a[i];
file2.close();
//sort numbers
for(i=0;i<Len-1;i++)
for(j=0;j<Len-i-1;j++)
if(a[j]>a[j+1])
{
temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
}
file2.open("f2.dat",ios::out);
for(i=0;i<Len;i++)
file2<<a[i]<<' ';
return 0;
}
/* 运行结果
* chinsung@gentoo ~ $ g++ test.cpp
* chinsung@gentoo ~ $ ./a.out
* Please input Len integers:
* No.1: 9
* No.2: 8
* No.3: 7
* No.4: 6
* No.5: 5
* No.6: 4
* No.7: 3
* No.8: 2
* No.9: 1
* No.10: 10
* No.11: 13
* No.12: 15
* No.13: 16
* No.14: 17
* No.15: 19
* No.16: 20
* No.17: 29
* No.18: 27
* No.19: 26
146
第十三章 输入输出流
* No.20: 11
* chinsung@gentoo ~ $ more f1.dat
* 9 8 7 6 5 4 3 2 1 10
* chinsung@gentoo ~ $ more f2.dat
* 1 2 3 4 5 6 7 8 9 10 11 13 15 16 17 19 20 26 27 29
*/
5. 编程实现以下功能:
1. 按职工号由小到大的顺序将5个员工的数据(包括号码、姓名、年龄、工资)输出到磁盘文件中保存
2. 从键盘输入两个员工的数据(职工号大于已有的职工号),增加到文件的末尾
3. 输出文件中全部职工的数据
4. 从键盘输入一个号码,从文件中查找有无此职工号,如有则显示从职工是第几个职工,以及此职工的全部数据。如
没有,就输出“无此人”。可以反复多次查询,如果查找的职工号为0,就结束
查询。
头痛,此题尚未完成...
147
第十四章 C++工具
第十四章 C++工具
待续...
148