层次遍历二叉树实验1
二叉树的建立与先序,中序,后序,层次遍历,图的深度优先搜索和广度优先搜索 实验报告

树和图的遍历实验报告2011-4-9实验题目:树和图的遍历实验目的:1.实现二叉树的建立与先序,中序,后序,层次遍历2.选择建立图的类型;根据所选择的类型用邻接矩阵的存储结构构建图;对图进行深度优先搜索和广度优先搜索;实验内容:一、算法描述:(1)二叉树的建立要建立一棵树就要有根节点和两棵子树。
两棵子树的建立同样需要这样的过程。
建立二叉树的过程也是遍历树的过程,实验要求以前序遍历的方式输入数据,因此我们也应按前序遍历的顺序,动态申请存储空间的方式建立这棵树并返回根节点的指针。
BiTNode *CreateBiTree(BiTNode *T){char ch;if((ch=getchar())==' ') T=NULL;else if(ch!='\n'){if(!(T=(BiTNode *)malloc(sizeof(BiTNode))))exit(1);T->data=ch;T->lchild=CreateBiTree(T->lchild);T->rchild=CreateBiTree(T->rchild);}return T;}(2)二叉树的遍历遍历作为二叉树所有操作的基础,因此我把它放在二叉树建立的前面。
前序遍历:即按照根节点,左子树,右子树的顺序访问。
具体操作:遇到节点,立即打印根节点的值,然后访问左子树,再访问右子树。
对左子树和右子树的访问也进行相同的操作。
void PreOrderTraverse(BiTree T){if(T){putchar(T->data);PreOrderTraverse(T->lchild);PreOrderTraverse(T->rchild);}}同理,易得中序遍历,后序遍历的操作。
//中序遍历二叉树void InOrderTraverse(BiTree T){if(T){InOrderTraverse(T->lchild);putchar(T->data);InOrderTraverse(T->rchild);}}//后序遍历二叉树void PostOrderTraverse(BiTree T){if(T){PostOrderTraverse(T->lchild);PostOrderTraverse(T->rchild);putchar(T->data);}}层次遍历:先访问的节点,其孩子节点也必然优先访问,这就用到了队列的思想。
二叉树的建立和遍历的实验报告doc

二叉树的建立和遍历的实验报告篇一:二叉树的建立及遍历实验报告实验三:二叉树的建立及遍历【实验目的】(1)掌握利用先序序列建立二叉树的二叉链表的过程。
(2)掌握二叉树的先序、中序和后序遍历算法。
【实验内容】1. 编写程序,实现二叉树的建立,并实现先序、中序和后序遍历。
如:输入先序序列abc###de###,则建立如下图所示的二叉树。
并显示其先序序列为:abcde中序序列为:cbaed后序序列为:cbeda【实验步骤】1.打开VC++。
2.建立工程:点File->New,选Project标签,在列表中选Win32 Console Application,再在右边的框里为工程起好名字,选好路径,点OK->finish。
至此工程建立完毕。
3.创建源文件或头文件:点File->New,选File标签,在列表里选C++ Source File。
给文件起好名字,选好路径,点OK。
至此一个源文件就被添加到了你刚创建的工程之中。
4.写好代码5.编译->链接->调试#include#include#define OK 1#define OVERFLOW -2typedef int Status;typedef char TElemType;typedef struct BiTNode{TElemType data;struct BiTNode *lchild, *rchild;}BiTNode,*BiTree;Status CreateBiTree(BiTree &T){TElemType ch;scanf("%c",&ch);if (ch=='#')T= NULL;else{if (!(T = (BiTNode *)malloc(sizeof(BiTNode))))return OVERFLOW;T->data = ch; CreateBiTree(T->lchild); CreateBiTree(T->rchild); }return OK;} // CreateBiTreevoid PreOrder(BiTree T) {if(T){printf("%c",T->data); PreOrder(T->lchild); PreOrder(T->rchild);}}void InOrder(BiTree T) {if(T){InOrder(T->lchild);printf("%c",T->data);InOrder(T->rchild);}}void PostOrder(BiTree T){if(T){PostOrder(T->lchild); PostOrder(T->rchild);printf("%c",T->data);}}void main(){BiTree T;CreateBiTree(T);printf("\n先序遍历序列:"); PreOrder(T);printf("\n中序遍历序列:"); InOrder(T);printf("\n后序遍历序列:"); PostOrder(T);}【实验心得】这次实验主要是通过先序序列建立二叉树,和二叉树的先序、中序、后续遍历算法。
二叉树的遍历(先序遍历、中序遍历、后序遍历全)实验报告

实验目的编写一个程序,实现二叉树的先序遍历,中序遍历,后序遍历。
实验内容编程序并上机调试运行。
编写一个程序,实现二叉树的先序遍历,中序遍历,后序遍历。
编写程序/***********二叉树的遍历**************/#include<stdio.h>#include<stdlib.h>typedef struct BiTNode{char data;struct BiTNode *lchild,*rchild;}BiTNode,*BiTree;/*************************************************///按先序次序构建的二叉树链表void CreatBiTree(BiTree *T){char ch;if((ch=getchar())==' ')*T=NULL;else{*T=(BiTNode*)malloc(sizeof(BiTNode));if(!(*T))exit(1);(*T)->data=ch;CreatBiTree(&(*T)->lchild);CreatBiTree(&(*T)->rchild);}}/*************************************************/ //先序遍历--递归算法void PreOrderTraverse(BiTree T){if(T){printf("%c",T->data);PreOrderTraverse(T->lchild);PreOrderTraverse(T->rchild);}}/*************************************************/ //中序遍历--递归算法void InOrderTraverse(BiTree T){if(T){InOrderTraverse(T->lchild);printf("%c",T->data);InOrderTraverse(T->rchild);}}/*************************************************/ //后序遍历--递归算法void PostOrderTraverse(BiTree T){if(T){PostOrderTraverse(T->lchild);PostOrderTraverse(T->rchild);printf("%c",T->data);}}/*************************************************/ //main函数void main(){BiTree T;printf("请按先序次序输入二叉树中结点的值,空格字符表示空树:\n" );CreatBiTree(&T);printf("\n");printf("先序遍历为:\n");PreOrderTraverse(T);printf("\n\n");printf("中序遍历为:\n");InOrderTraverse(T);printf("\n\n");printf("后序遍历为:\n");PostOrderTraverse(T);printf("\n\n");getchar();}运行程序:结果分析:按先序输入的二叉树为ABC^^DE^G^^F^^^(^为空格)该二叉树画成树形为:其先序遍历为:ABCDEGF其中序遍历为:CBEGDFA其后序遍历为:CGEFDBA可以看出运行结果是正确的。
二叉树的遍历算法实验报告

二叉树的遍历算法实验报告二叉树的遍历算法实验报告引言:二叉树是计算机科学中常用的数据结构之一,它是由节点组成的层次结构,每个节点最多有两个子节点。
在实际应用中,对二叉树进行遍历是一项重要的操作,可以帮助我们理解树的结构和节点之间的关系。
本文将介绍二叉树的三种遍历算法:前序遍历、中序遍历和后序遍历,并通过实验验证其正确性和效率。
一、前序遍历前序遍历是指先访问根节点,然后按照先左后右的顺序遍历左右子树。
具体的实现可以通过递归或者使用栈来实现。
我们以递归方式实现前序遍历算法,并进行实验验证。
实验步骤:1. 创建一个二叉树,并手动构造一些节点和它们之间的关系。
2. 实现前序遍历算法的递归函数,函数的输入为根节点。
3. 在递归函数中,首先访问当前节点,然后递归调用函数遍历左子树,最后递归调用函数遍历右子树。
4. 调用前序遍历函数,输出遍历结果。
实验结果:经过实验,我们得到了正确的前序遍历结果。
这证明了前序遍历算法的正确性。
二、中序遍历中序遍历是指按照先左后根再右的顺序遍历二叉树。
同样,我们可以使用递归或者栈来实现中序遍历算法。
在本实验中,我们选择使用递归方式来实现。
实验步骤:1. 继续使用前面创建的二叉树。
2. 实现中序遍历算法的递归函数,函数的输入为根节点。
3. 在递归函数中,首先递归调用函数遍历左子树,然后访问当前节点,最后递归调用函数遍历右子树。
4. 调用中序遍历函数,输出遍历结果。
实验结果:通过实验,我们得到了正确的中序遍历结果。
这证明了中序遍历算法的正确性。
三、后序遍历后序遍历是指按照先左后右再根的顺序遍历二叉树。
同样,我们可以使用递归或者栈来实现后序遍历算法。
在本实验中,我们选择使用递归方式来实现。
实验步骤:1. 继续使用前面创建的二叉树。
2. 实现后序遍历算法的递归函数,函数的输入为根节点。
3. 在递归函数中,首先递归调用函数遍历左子树,然后递归调用函数遍历右子树,最后访问当前节点。
4. 调用后序遍历函数,输出遍历结果。
二叉树的遍历实验报告

二叉树的遍历实验报告实验报告:二叉树的遍历(先序遍历、中序遍历、后序遍历)一、引言二叉树是一种非常常见的数据结构,在计算机领域有着广泛的应用。
对二叉树进行遍历操作是其中最基本的操作之一、本实验旨在通过对二叉树的先序遍历、中序遍历和后序遍历的实践,加深对二叉树遍历算法的理解和掌握。
二、目的1.掌握二叉树先序遍历的算法原理和实现方法;2.掌握二叉树中序遍历的算法原理和实现方法;3.掌握二叉树后序遍历的算法原理和实现方法;4.使用递归和非递归两种方式实现以上三种遍历算法;5.进行正确性验证和性能评估。
三、方法1.算法原理:1.1先序遍历:先访问根节点,然后递归遍历左子树,再递归遍历右子树;1.2中序遍历:先递归遍历左子树,然后访问根节点,最后递归遍历右子树;1.3后序遍历:先递归遍历左子树,再递归遍历右子树,最后访问根节点。
2.实现方法:2.1递归实现:采用函数递归调用的方式,实现对二叉树的遍历;2.2非递归实现:采用栈的数据结构,模拟递归的过程,实现对二叉树的遍历。
四、实验步骤1.数据结构设计:1.1定义二叉树的节点结构,包括节点值和两个指针(分别指向左子节点和右子节点);1.2定义一个栈结构,用于非递归实现时的辅助存储。
2.先序遍历:2.1递归实现:按照先序遍历的原理,通过递归调用遍历左子树和右子树,再输出根节点;2.2非递归实现:通过栈结构模拟递归的过程,先将根节点入栈,然后循环将栈顶节点弹出并输出,再将其右子节点入栈,最后将左子节点入栈,直到栈为空。
3.中序遍历:3.1递归实现:按照中序遍历的原理,通过递归调用先遍历左子树,再输出根节点,最后遍历右子树;3.2非递归实现:先将根节点入栈,然后循环将左子节点入栈,直到左子节点为空,然后弹出栈顶节点并输出,再将其右子节点入栈,重复以上过程直到栈为空。
4.后序遍历:4.1递归实现:按照后序遍历的原理,通过递归调用先遍历左子树,再遍历右子树,最后输出根节点;4.2非递归实现:通过栈结构模拟递归的过程,先将根节点入栈,然后重复以下步骤直到栈为空。
树和二叉树-层序遍历二叉树

通过上机实践,帮助学生进一步掌握指针变量和动态变量的含义,掌握二叉树的结构特性,以及各种存储结构的特点及适用范围,掌握用指针类型描述、访问和处理二叉树的运算。
【实验内容】实验题目一:层序遍历二叉树【实验步骤】1 已知二叉树以二叉链表作为存储结构,写一个算法按层序遍历它,通过程序在终端屏幕上打印出它的层序序列。
2 先建立二叉树的二叉链表存储结构,再遍历它。
3 利用队列完成算法。
编程实现以二叉链表存储的二叉树的中序遍历的非递归算法。
编程实现二叉树的中序线索链表的生成(线索化)及其遍历的算法。
实验题目二:交换二叉树的所有结点的左右子树【实验步骤】1 已知二叉树以二叉链表作为存储结构,写一个算法交换该二叉树的所有结点的左右子树。
2 先建立二叉树的二叉链表存储结构,再完成算法,注意结果的输出形式!3 利用栈。
可设二叉树的根指针为t,且以二叉链表表示,可利用一个指针栈来实现,且设栈单元包括数据域和指针域,当树非空时,将当前的树根结点进栈,同时将当前栈顶元素出栈当作根结点,然后依据当前的根结点是否具有孩子结点来判定是否将其左右指针交换;再将交换后的左指针或右指针入栈,如此反复,直到栈空。
【程序说明】该程序集成了如下功能:(1)二叉树的建立(2)递归和非递归先序,中序和后序遍历二叉树(3)按层次遍历二叉树(4)交换二叉树的左右子树(5)输出叶子结点(6)递归和非递归计算叶子结点的数目#include <stdio.h>#include <malloc.h>#include <stdlib.h>#include <conio.h>#include <math.h>typedef struct Binnode{char data;struct Binnode *lchild;struct Binnode *rchild;}Binnode;/*按照前序遍历建立二叉树*/void Creat_Bintree(Binnode **t){char ch;scanf("\n%c",&ch);if(ch=='0') *t=NULL;else{*t=(Binnode*)malloc(sizeof(Binnode));if(!*t)exit(OVERFLOW);(*t)->data=ch;printf("%c: left",ch); Creat_Bintree(&(*t)->lchild);printf("%c: right",ch);Creat_Bintree(&(*t)->rchild);}}/*按照前序递归遍历二叉树*/void Preorder1(Binnode *t){if(t!=NULL){printf("%c",t->data);Preorder1(t->lchild);Preorder1(t->rchild);}}/*按照中序递归遍历二叉树*/void Inorder1(Binnode *t){ /* printf("\n输出中序递归遍历序列:");*/if(t!=NULL){Inorder1(t->lchild);printf("%c",t->data);Inorder1(t->rchild);}}/* 按照后序递归遍历二叉树*/void Posorder1(Binnode *t){ /* printf("\n输出后序递归遍历序列:");*/if(t!=NULL){Posorder1(t->lchild);Posorder1(t->rchild);printf("%c",t->data);}}/*按照前序非递归遍历二叉树*/void preorder2(Binnode *root)/*先序非递归遍历二叉树*/{ Binnode *p,*stack[100];int top ;p=root;if(root!=NULL){top=1;stack[top]=p;while(top>0){p=stack[top] ;/*将右小孩放入栈*/top--;printf("%c",p->data);if(p->rchild!=NULL){top++;stack[top]=p->rchild;}if(p->lchild!=NULL){top++;stack[top]=p->lchild;}}}}/*按照中序非递归遍历二叉树*/void Inorder2(Binnode *root)/*中序非递归遍历二叉树*/{ Binnode *p,*stack[100];int top=0;p=root;do{while(p!=NULL){top++;stack[top]=p;p=p->lchild;}if(top>0){p=stack[top];/*p所指的节点为无左子树或其左子树已经遍历过*/ top--;printf("%c",p->data);p=p->rchild;}}while(p!=NULL||top!=0);/*按照后序非递归遍历二叉树*/ void Posorder2(Binnode *t){Binnode *s[100];int top=-1;int flag[100];while(t!=NULL||top!=-1){while(t){top++;flag[top]=0;s[top]=t;t=t->lchild;}while(top>=0&&flag[top]==1){t=s[top];printf("%c",t->data);top--;}if(top>=0){t=s[top];flag[top]=1;t=t->rchild;}else{t=NULL;}}}/*按照层次遍历二叉树*/int front=0,rear=1;void Levelorder(Binnode *t){Binnode *q[100];q[0]=t;/* int front=0,rear=1;*/while(front<rear)if(q[front]){printf("%c",q[front]->data);q[rear++]=q[front]->lchild;q[rear++]=q[front]->rchild;front++;}else{front++;}}}/*递归法将二叉树的左右子树互换*/ void Exchange1(Binnode *t){Binnode *temp;if(t){Exchange1(t->lchild);Exchange1(t->rchild);temp=t->lchild;t->lchild=t->rchild;t->rchild=temp;}}/*非递归法将二叉树的左右子树互换*/ void Exchange2(Binnode *t){Binnode *temp;Binnode *s[100];int top=0;while(t||top){if(t){s[top++]=t;temp=t->lchild;t->lchild=t->rchild;t->rchild=temp;t=t->lchild;}else{t=s[--top]->rchild;}}}/*递归法求叶子结点个数*/int Leaves_Num1(Binnode *t){if(t){if(t->lchild==NULL&&t->rchild==NULL){return 1;}return (Leaves_Num1(t->lchild)+Leaves_Num1(t->rchild));}else return 0;}/*非递归法求叶子结点个数*/int Leaves_Num2(Binnode *t){Binnode *s[100];int count=0,top=0;while(t||top>0){if(t){s[top++]=t;if(t->lchild==NULL&&t->rchild==NULL){count++;printf("%c",t->data);/*输出叶子结点*/}t=t->lchild;}else{t=s[--top]->rchild;}}return count;}int main(){Binnode *proot=NULL;int p,q;printf("1.CreateBitree:\n");Creat_Bintree(&proot); /*建立二叉树时,无左右孩子的结点输入’0’值*/printf("\n2.Output Digui PreOrder:\n");Preorder1(proot);printf("\n3.Output Not Digui PreOrder:\n");preorder2(proot);printf("\n4.Output Digui InOrder:\n");Inorder1(proot);printf("\n5.Output Not Digui InOrder:\n");Inorder2(proot);printf("\n6.Output Digui PostOrder:\n");Posorder1(proot);printf("\n7.Output Not Digui PostOrder:\n");Posorder2(proot);printf("\n8.Output LevelOrde:\n");Levelorder(proot);Exchange1(proot);printf("\n9.Output Digui Exchange:\n");Preorder1(proot);Exchange2(proot);printf("\n10.Output Not Digui Exchange:\n");Preorder1(proot);printf("\n11.Leaves_Node:\n");q=Leaves_Num2(proot);printf("\n12.Output Not Digui Leaves_Num:%d\n",q);p=Leaves_Num1(proot);printf("13.Output Digui Leaves_Num:%d\n",p);printf("\nThis System Once Again\n");return 0;}【思考题】编程实现二叉树的中序线索链表的生成(线索化)及其遍历的算法。
二叉树遍历实验报告

二叉树遍历实验报告二叉树遍历实验报告一、引言二叉树是计算机科学中常用的数据结构之一,它由节点组成,每个节点最多有两个子节点。
二叉树的遍历是指按照一定的规则访问二叉树中的所有节点。
本实验旨在通过实际操作,探索二叉树的三种遍历方式:前序遍历、中序遍历和后序遍历,并分析它们的应用场景和性能特点。
二、实验方法1. 实验环境本实验使用Python编程语言进行实现,并在Jupyter Notebook中运行代码。
2. 实验步骤(1)定义二叉树节点类首先,我们定义一个二叉树节点类,该类包含节点值、左子节点和右子节点三个属性。
(2)构建二叉树在主函数中,我们手动构建一个二叉树,包含多个节点,并将其保存为根节点。
(3)实现三种遍历方式通过递归的方式,实现二叉树的前序遍历、中序遍历和后序遍历。
具体实现过程如下:- 前序遍历:先访问根节点,然后递归遍历左子树,最后递归遍历右子树。
- 中序遍历:先递归遍历左子树,然后访问根节点,最后递归遍历右子树。
- 后序遍历:先递归遍历左子树,然后递归遍历右子树,最后访问根节点。
(4)测试遍历结果在主函数中,我们调用实现的三种遍历方式,对构建的二叉树进行遍历,并输出结果。
三、实验结果与分析经过实验,我们得到了二叉树的前序遍历、中序遍历和后序遍历的结果。
以下是我们的实验结果及分析:1. 前序遍历结果前序遍历结果为:A - B - D - E - C - F - G前序遍历的应用场景包括:复制整个二叉树、计算二叉树的深度和宽度等。
前序遍历的时间复杂度为O(n),其中n为二叉树的节点数。
2. 中序遍历结果中序遍历结果为:D - B - E - A - F - C - G中序遍历的应用场景包括:二叉搜索树的中序遍历可以得到有序的节点序列。
中序遍历的时间复杂度为O(n),其中n为二叉树的节点数。
3. 后序遍历结果后序遍历结果为:D - E - B - F - G - C - A后序遍历的应用场景包括:计算二叉树的高度、判断二叉树是否对称等。
数据结构实验之求二叉树后序遍历和层次遍历

}
}
}
void Preorder(struct node *root)
{
if(root!=NULL)
{
Preorder(root->left);d[j++]=root->a;Preorder(root->right);
}
else build(b,root);
}
Preorder(root);
for(i=0;i<j;i++)
{
printf("%d",d[i]);
if(u!=NULL)
{
u->a=0;
u->left=u->right=NULL;
}
return u;
}
void build(int n,struct node*root)
{
if(n<root->a)
{
if(root->left!=NULL)
Time Limit: 1000MS Memory limit: 65536K
题目描述
已知一棵二叉树的前序遍历和中序遍历,求二叉树的后序遍历。
输入
输入数据有多组,第一行是一个整数t (t<1000),代表有t组测试数据。每组包括两个长度小于50 的字符串,第一个字符串表示二叉树的先序遍历序列,第二个字符串表示二叉树的中序遍历序列。
{
root=(struct node*)malloc(sizeof(struct node));
root->a=b;
root->left=NULL;
二叉树的建立和遍历的实验报告
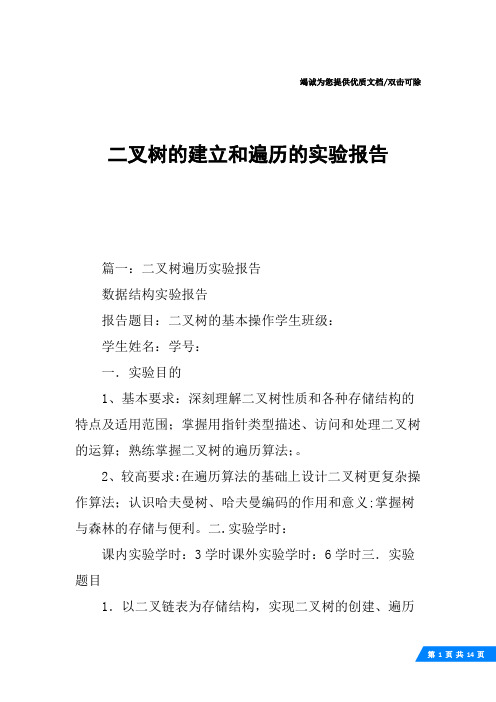
竭诚为您提供优质文档/双击可除二叉树的建立和遍历的实验报告篇一:二叉树遍历实验报告数据结构实验报告报告题目:二叉树的基本操作学生班级:学生姓名:学号:一.实验目的1、基本要求:深刻理解二叉树性质和各种存储结构的特点及适用范围;掌握用指针类型描述、访问和处理二叉树的运算;熟练掌握二叉树的遍历算法;。
2、较高要求:在遍历算法的基础上设计二叉树更复杂操作算法;认识哈夫曼树、哈夫曼编码的作用和意义;掌握树与森林的存储与便利。
二.实验学时:课内实验学时:3学时课外实验学时:6学时三.实验题目1.以二叉链表为存储结构,实现二叉树的创建、遍历(实验类型:验证型)1)问题描述:在主程序中设计一个简单的菜单,分别调用相应的函数功能:1…建立树2…前序遍历树3…中序遍历树4…后序遍历树5…求二叉树的高度6…求二叉树的叶子节点7…非递归中序遍历树0…结束2)实验要求:在程序中定义下述函数,并实现要求的函数功能:createbinTree(binTreestructnode*lchild,*rchild;}binTnode;元素类型:intcreatebinTree(binTreevoidpreorder(binTreevoidInorder(binTreevoidpostorder(binTreevoidInordern(binTreeintleaf(bi nTreeintpostTreeDepth(binTree2、编写算法实现二叉树的非递归中序遍历和求二叉树高度。
1)问题描述:实现二叉树的非递归中序遍历和求二叉树高度2)实验要求:以二叉链表作为存储结构3)实现过程:1、实现非递归中序遍历代码:voidcbiTree::Inordern(binTreeinttop=0;p=T;do{while(p!=nuLL){stack[top]=p;;top=top+1;p=p->lchild;};if(top>0){top=top-1;p=stack[top];printf("%3c",p->data);p=p->rchild;}}while(p!=nuLL||top!=0);}2、求二叉树高度:intcbiTree::postTreeDepth(binTreeif(T!=nuLL){l=postTreeDepth(T->lchild);r=postTreeDepth(T->rchil d);max=l>r?l:r;return(max+1);}elsereturn(0);}实验步骤:1)新建一个基于consoleApplication的工程,工程名称biTreeTest;2)新建一个类cbiTree二叉树类。
数据结构二叉树遍历实验报告简版
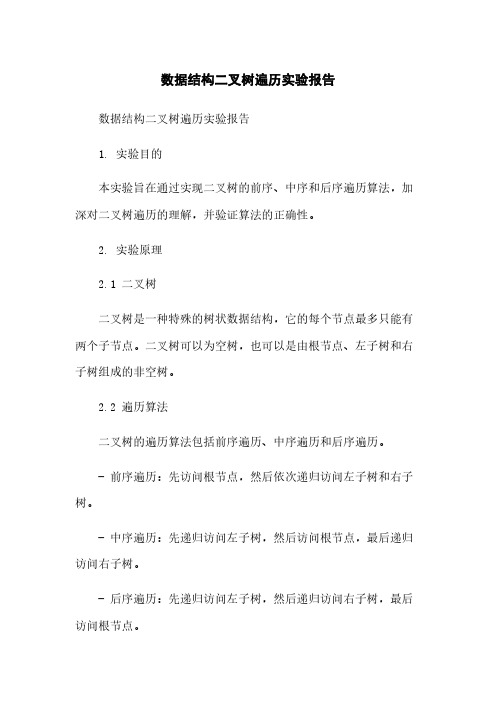
数据结构二叉树遍历实验报告数据结构二叉树遍历实验报告1. 实验目的本实验旨在通过实现二叉树的前序、中序和后序遍历算法,加深对二叉树遍历的理解,并验证算法的正确性。
2. 实验原理2.1 二叉树二叉树是一种特殊的树状数据结构,它的每个节点最多只能有两个子节点。
二叉树可以为空树,也可以是由根节点、左子树和右子树组成的非空树。
2.2 遍历算法二叉树的遍历算法包括前序遍历、中序遍历和后序遍历。
- 前序遍历:先访问根节点,然后依次递归访问左子树和右子树。
- 中序遍历:先递归访问左子树,然后访问根节点,最后递归访问右子树。
- 后序遍历:先递归访问左子树,然后递归访问右子树,最后访问根节点。
3. 实验过程3.1 数据结构设计首先,我们需要设计表示二叉树的数据结构。
在本次实验中,二叉树的每个节点包含三个成员变量:值、左子节点和右子节点。
我们可以使用面向对象编程语言提供的类来实现。
具体实现如下:```pythonclass TreeNode:def __init__(self, val=0, left=None, right=None): self.val = valself.left = leftself.right = right```3.2 前序遍历算法前序遍历算法的实现主要包括以下步骤:1. 若二叉树为空,则返回空列表。
2. 创建一个栈,用于存储遍历过程中的节点。
3. 将根节点入栈。
4. 循环执行以下步骤,直到栈为空:- 弹出栈顶节点,并将其值添加到结果列表中。
- 若当前节点存在右子节点,则将右子节点压入栈。
- 若当前节点存在左子节点,则将左子节点压入栈。
具体实现如下:```pythondef preorderTraversal(root):if not root:return []stack = []result = []stack.append(root)while stack:node = stack.pop()result.append(node.val)if node.right:stack.append(node.right)if node.left:stack.append(node.left)return result```3.3 中序遍历算法中序遍历算法的实现主要包括以下步骤:1. 若二叉树为空,则返回空列表。
二叉树的建立和遍历实验报告
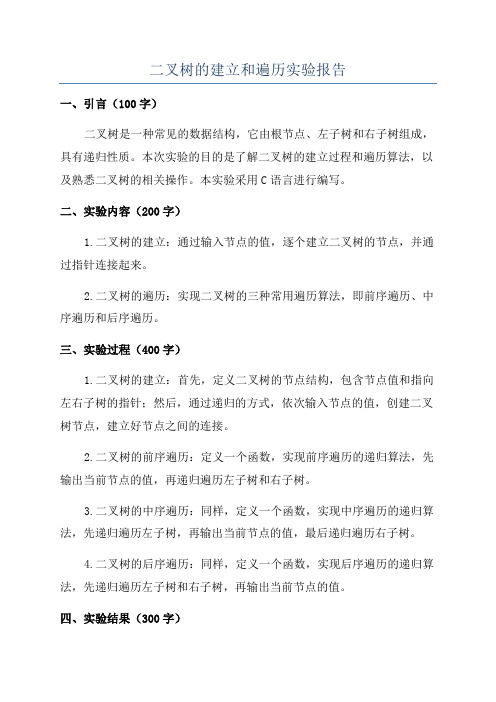
二叉树的建立和遍历实验报告一、引言(100字)二叉树是一种常见的数据结构,它由根节点、左子树和右子树组成,具有递归性质。
本次实验的目的是了解二叉树的建立过程和遍历算法,以及熟悉二叉树的相关操作。
本实验采用C语言进行编写。
二、实验内容(200字)1.二叉树的建立:通过输入节点的值,逐个建立二叉树的节点,并通过指针连接起来。
2.二叉树的遍历:实现二叉树的三种常用遍历算法,即前序遍历、中序遍历和后序遍历。
三、实验过程(400字)1.二叉树的建立:首先,定义二叉树的节点结构,包含节点值和指向左右子树的指针;然后,通过递归的方式,依次输入节点的值,创建二叉树节点,建立好节点之间的连接。
2.二叉树的前序遍历:定义一个函数,实现前序遍历的递归算法,先输出当前节点的值,再递归遍历左子树和右子树。
3.二叉树的中序遍历:同样,定义一个函数,实现中序遍历的递归算法,先递归遍历左子树,再输出当前节点的值,最后递归遍历右子树。
4.二叉树的后序遍历:同样,定义一个函数,实现后序遍历的递归算法,先递归遍历左子树和右子树,再输出当前节点的值。
四、实验结果(300字)通过实验,我成功建立了一个二叉树,并实现了三种遍历算法。
对于建立二叉树来说,只要按照递归的思路,先输入根节点的值,再分别输入左子树和右子树的值,即可依次建立好节点之间的连接。
建立好二叉树后,即可进行遍历操作。
在进行遍历算法的实现时,我首先定义了一个函数来进行递归遍历操作。
在每一次递归调用中,我首先判断当前节点是否为空,若为空则直接返回;若不为空,则按照特定的顺序进行遍历操作。
在前序遍历中,我先输出当前节点的值,再递归遍历左子树和右子树;在中序遍历中,我先递归遍历左子树,再输出当前节点的值,最后递归遍历右子树;在后序遍历中,我先递归遍历左子树和右子树,再输出当前节点的值。
通过运行程序,我成功进行了二叉树的建立和遍历,并得到了正确的结果。
可以看到,通过不同的遍历顺序,可以获得不同的遍历结果,这也是二叉树遍历算法的特性所在。
数据结构二叉树遍历实验报告
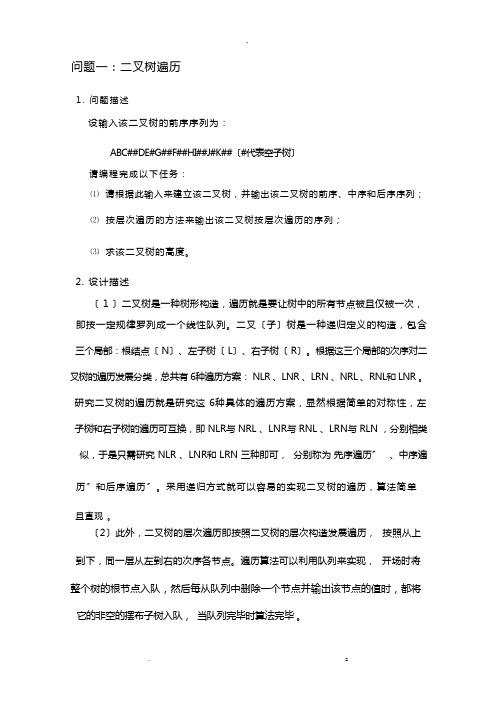
问题一:二叉树遍历1.问题描述设输入该二叉树的前序序列为:ABC##DE#G##F##HI##J#K##〔#代表空子树〕请编程完成以下任务:⑴请根据此输入来建立该二叉树,并输出该二叉树的前序、中序和后序序列;⑵按层次遍历的方法来输出该二叉树按层次遍历的序列;⑶求该二叉树的高度。
2. 设计描述〔 1 〕二叉树是一种树形构造,遍历就是要让树中的所有节点被且仅被一次,即按一定规律罗列成一个线性队列。
二叉〔子〕树是一种递归定义的构造,包含三个局部:根结点〔 N〕、左子树〔 L〕、右子树〔 R〕。
根据这三个局部的次序对二叉树的遍历发展分类,总共有 6种遍历方案: NLR 、LNR 、LRN 、NRL 、RNL和 LNR 。
研究二叉树的遍历就是研究这 6种具体的遍历方案,显然根据简单的对称性,左子树和右子树的遍历可互换,即 NLR与 NRL 、LNR与 RNL 、LRN与 RLN ,分别相类似,于是只需研究 NLR 、LNR和 LRN 三种即可,分别称为先序遍历〞、中序遍历〞和后序遍历〞。
采用递归方式就可以容易的实现二叉树的遍历,算法简单且直观。
〔2〕此外,二叉树的层次遍历即按照二叉树的层次构造发展遍历,按照从上到下,同一层从左到右的次序各节点。
遍历算法可以利用队列来实现,开场时将整个树的根节点入队,然后每从队列中删除一个节点并输出该节点的值时,都将它的非空的摆布子树入队,当队列完毕时算法完毕。
〔3〕计算二叉树高度也是利用递归来实现:假设一颗二叉树为空,则它的深度为 0 ,否则深度等于摆布子树的最大深度加一。
3 .源程序1 #include <stdio.h>2 #include <stdlib.h>3 #include <malloc.h>4 #define ElemType char5 struct BTreeNode {6 ElemType data;7 struct BTreeNode* left;8 struct BTreeNode* right;9 };10 void CreateBTree(struct BTreeNode** T)11 {12 char ch;1314 if (ch == '#') *T = NULL;15 else {16 (*T) = malloc(sizeof(struct BTreeNode));17 (*T)->data = ch;18 CreateBTree(&((*T)->left));19 CreateBTree(&((*T)->right));20 }21 }22 void Preorder(struct BTreeNode* T)23 {24 if (T != NULL) {2526 Preorder(T->left);27 Preorder(T->right);28 }29 }30 void Inorder(struct BTreeNode* T)31 {32 if (T != NULL) {33 Inorder(T->left);3435 Inorder(T->right);36 }37 }38 void Postorder(struct BTreeNode* T)39 {40 if (T != NULL) {41 Postorder(T->left);42 Postorder(T->right);4344 }45 }46 void Levelorder(struct BTreeNode* BT)47 {48 struct BTreeNode* p;49 struct BTreeNode* q[30];50 int front=0,rear=0;51 if(BT!=NULL) {52 rear=(rear+1)% 30;53 q[rear]=BT;54 }55 while(front!=rear) {56 front=(front+1)% 30;57 p=q[front];5859 if(p->left!=NULL) {60 rear=(rear+1)% 30;61 q[rear]=p->left;62 }63 if(p->right!=NULL) {64 rear=(rear+1)% 30;65 q[rear]=p->right;66 }67 }68 }69 int getHeight(struct BTreeNode* T)70 {71 int lh,rh;72 if (T == NULL) return 0;73 lh = getHeight(T->left);74 rh = getHeight(T->right);7576 }77 void main(void)78 {79 struct BTreeNode* T;80 CreateBTree(&T);81 前序序列:82 Preorder(T);8384 中序序列:85 Inorder(T);-4.运行结果问题二:哈夫曼编码、译码系统1. 问题描述 对一个ASCII 编码的文本文件中的字符发展哈夫曼编码,生成编码文件; 反过来,可将编码文件译码复原为一个文本文件〔选做〕 。
数据结构二叉树遍历实验报告[1]简版
![数据结构二叉树遍历实验报告[1]简版](https://img.taocdn.com/s3/m/c3328b25a9114431b90d6c85ec3a87c240288a2f.png)
数据结构二叉树遍历实验报告数据结构二叉树遍历实验报告实验目的本实验旨在通过二叉树的遍历方法,加深对二叉树结构的理解,并掌握其遍历的实现方法。
实验内容实验内容包括以下两个部分:1. 实现二叉树的先序遍历方法;2. 实现二叉树的中序遍历方法。
实验原理和实现方法1. 先序遍历先序遍历即从根节点开始,先输出当前节点的值,然后先序遍历左子树,最后先序遍历右子树。
先序遍历的实现方法有递归和迭代两种。
递归实现递归实现的核心是先输出当前节点的值,并递归调用函数对左子树和右子树进行先序遍历。
以下是递归实现的伪代码示例:```pythondef preOrderTraversal(node):if node is None:returnprint(node.value)preOrderTraversal(node.left)preOrderTraversal(node.right)```迭代实现迭代实现需要借助栈来保存节点的信息。
整体思路是先将根节点入栈,然后循环执行以下步骤:弹出栈顶节点并输出,将栈顶节点的右子节点和左子节点依次入栈。
当栈为空时,遍历结束。
以下是迭代实现的伪代码示例:```pythondef preOrderTraversal(node):if node is None:returnstack = [node]while stack:curr = stack.pop()print(curr.value)if curr.right:stack.append(curr.right)if curr.left:stack.append(curr.left)```2. 中序遍历中序遍历即从根节点开始,先中序遍历左子树,然后输出当前节点的值,最后中序遍历右子树。
中序遍历的实现方法同样有递归和迭代两种。
递归实现递归实现的核心是先中序遍历左子树,并输出当前节点的值,最后递归调用函数对右子树进行中序遍历。
以下是递归实现的伪代码示例:```pythondef inOrderTraversal(node):if node is None:returninOrderTraversal(node.left)print(node.value)inOrderTraversal(node.right)```迭代实现迭代实现同样需要借助栈来保存节点的信息。
二叉树的前序创建及层次遍历实验
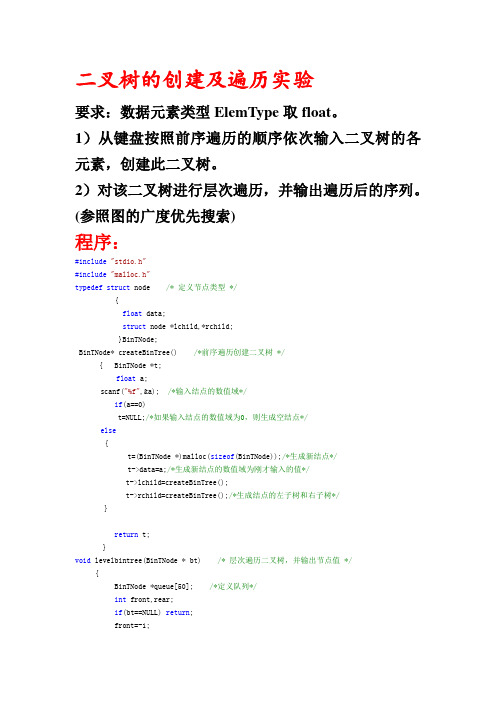
二叉树的创建及遍历实验要求:数据元素类型ElemType取float。
1)从键盘按照前序遍历的顺序依次输入二叉树的各元素,创建此二叉树。
2)对该二叉树进行层次遍历,并输出遍历后的序列。
(参照图的广度优先搜索)程序:#include"stdio.h"#include"malloc.h"typedef struct node /* 定义节点类型 */{float data;struct node *lchild,*rchild;}BinTNode;BinTNode* createBinTree() /*前序遍历创建二叉树 */{ BinTNode *t;float a;scanf("%f",&a); /*输入结点的数值域*/if(a==0)t=NULL;/*如果输入结点的数值域为0,则生成空结点*/else{t=(BinTNode *)malloc(sizeof(BinTNode));/*生成新结点*/t->data=a;/*生成新结点的数值域为刚才输入的值*/t->lchild=createBinTree();t->rchild=createBinTree();/*生成结点的左子树和右子树*/}return t;}void levelbintree(BinTNode * bt) /* 层次遍历二叉树,并输出节点值 */{BinTNode *queue[50]; /*定义队列*/int front,rear;if(bt==NULL) return;front=-1;rear=0;queue[rear]=bt;while(front!=rear){front++;printf("%f\n",queue[front]->data);/*输出对头元素的数值域*/ if(queue[front]->lchild!=NULL){rear++;queue[rear]=queue[front]->lchild;}/*如果左子树不为空,则入队*/if(queue[front]->rchild!=NULL){rear++;queue[rear]=queue[front]->rchild;}/*如果右子树不为空,则出队*/}}void Print_BinTree(BinTNode *T,int i ) // i表示结点所在层次,初次调用时i=0 { int j;if(T->rchild)Print_BinTree(T->rchild,i+1); //函数递归来建立层次。
层次遍历
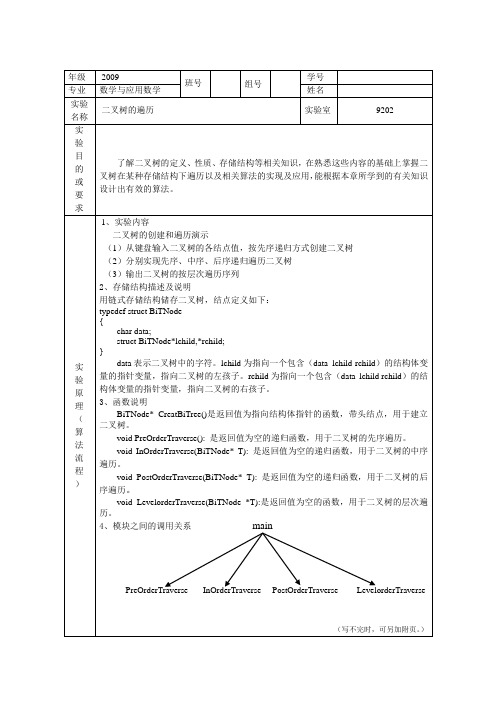
}
}
void LevelorderTraverse(BiTNode *T)
{
int front=0,rear=1; /*用队列进行存储并初始化*/
BiTNode *q[100];
q[0]=T; /*数组储存二叉树指针*/
while(front<rear) /*当队列为空时结束*/
}
}
}
main()
{
BiTNode*T;
printf("请按先序序列输入二叉树结点,以字符@作为空子树\n");
T=CreatBiTree();
printf("先序递归遍历二叉树结果如下:\n");
PreOrderTraverse(T);
printf("\n");
printf("中序递归遍历二叉树结果如下:\n");
{
if(q[front]) /*二叉树不为空*/
{
printf("%c",q[front]->data);
q[rear++]=q[front]->lchild; /*将左子树存入队列中*/
q[rear++]=q[front]->rchild; /*将右子书存入队列中*/
front++;
}
else
{
front++;
心得体会:
1.通过本次试验,让我更深刻的理解了二叉树的性质。
2.通过这次实验,我更深刻的理解了递归算法的内涵,并熟悉了队列的应用。
实现二叉树的各种遍历算法实验报告

实现二叉树的各种遍历算法实验报告一实验题目: 实现二叉树的各种遍历算法二实验要求:2.1:(1)输出二叉树 b(2)输出H节点的左右孩子节点值(3)输出二叉树b 的深度(4)输出二叉树 b的宽度(5)输出二叉树 b的节点个数(6)输出二叉树 b的叶子节点个数(7)释放二叉树 b2.2:(1)实现二叉树的先序遍历(2)实现二叉树的中序遍历(3)实现二叉树的后序遍历三实验内容:3.1 树的抽象数据类型:ADT Tree{数据对象D:D是具有相同特性的数据元素的集合。
数据关系R:若D为空集,则称为空树;若D仅含有一个数据元素,则R为空集,否则R={H},H是如下二元关系:(1) 在D中存在唯一的称为根的数据元素root,它在关系H下无前驱;(2) 若D-{root}≠NULL,则存在D-{root}的一个划分D1,D2,D3, …,Dm(m>0),对于任意j≠k(1≤j,k≤m)有Dj∩Dk=NULL,且对任意的i(1≤i≤m),唯一存在数据元素xi∈Di有<root,xi>∈H;(3) 对应于D-{root}的划分,H-{<root,xi>,…,<root,xm>}有唯一的一个划分H1,H2,…,Hm(m>0),对任意j≠k(1≤j,k≤m)有Hj∩Hk=NULL,且对任意i(1≤i≤m),Hi是Di上的二元关系,(Di,{Hi})是一棵符合本定义的树,称为根root的子树。
基本操作P:InitTree(&T);操作结果:构造空树T。
DestroyTree(&T);初始条件:树T存在。
操作结果:销毁树T。
CreateTree(&T,definition);初始条件:definition给出树T的定义。
操作结果:按definition构造树T。
ClearTree(&T);初始条件:树T存在。
操作结果:将树T清为空树。
TreeEmpty(T);初始条件:树T存在。
遍历二叉树
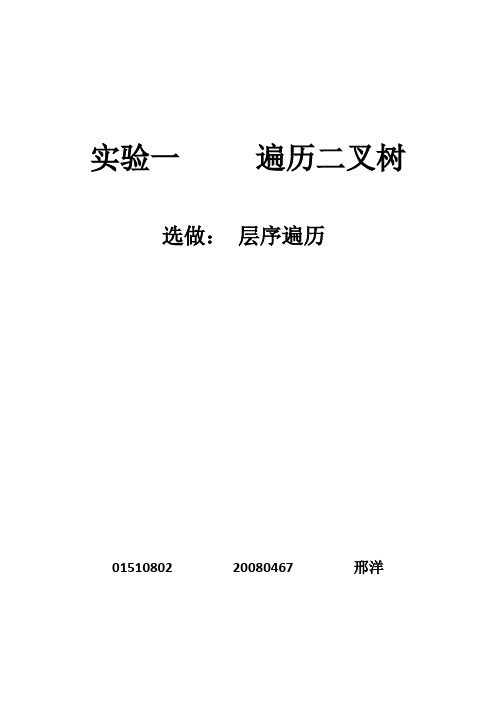
实验一遍历二叉树选做:层序遍历01510802 20080467 邢洋实验一:遍历二叉树一.实验目的:实现由扩展前序生成二叉树并实现该树的前序、中序和后序遍历。
二.实验内容:对由扩展的前序序列生成的二叉树分别进行前序、中序和后序遍历。
三、程序设计:程序设计思路:1.由二叉树前序遍历为跟左右的规律和扩展前序序列将节点下一位标为“*”的特点生成二叉树。
2.根据二叉树前序、中序、后序的各自特点对生成的二叉树进行遍历。
流程图:四、程序清单:编译与调试环境:Visual C++ 6.0#include<string.h>#include<ctype.h>#include<malloc.h>#include<limits.h>#include<stdio.h>#include<stdlib.h>#include<io.h>#include<math.h>#include<process.h>#define TRUE 1#define FALSE 0#define OK 1#define ERROR 0#define INFEASIBLE -1typedef char ElemType;typedef struct BiTNode {ElemType data;struct BiTNode *lchild,*rchild; }BiTNode,*BiTree;BiTree CreateBiTree(BiTree T){ ElemType ch;scanf("%c",&ch);if(ch=='*')T=NULL;else{ T=(BiTNode*)malloc(sizeof(BiTNode));T->data=ch;T->lchild=CreateBiTree(T->lchild);T->rchild=CreateBiTree(T->rchild); }return T;}Elemprintf(ElemType a ){ printf("%c",a);return OK;}PreOrderTraverse( BiTree T ){ if(T){if(Elemprintf(T->data))if(PreOrderTraverse(T->lchild))if(PreOrderTraverse(T->rchild))return OK;return ERROR;}else return OK;InOrderTraverse(BiTree T ){ if(T){if(InOrderTraverse(T->lchild))if(Elemprintf(T->data))if(InOrderTraverse(T->rchild))return OK;return ERROR;}else return OK;}PostOrderTraverse(BiTree T ){ if(T){if(PostOrderTraverse(T->lchild))if(PostOrderTraverse(T->rchild))if(Elemprintf(T->data))return OK;return ERROR;}else return OK;}main(){ BiTree T;T=CreateBiTree(T);printf("PreOrder:");PreOrderTraverse(T);printf("\n");printf("InOrder:");InOrderTraverse(T);printf("\n");printf("PostOrder:");PostOrderTraverse(T);printf("\n");}五.程序分析:1.初始化和定义首先定义一个结构来表示书的节点,其中包含三个变量:两个BiTNode型指针分别指向左孩子和右孩子,一个ElemType型变量data存放节点的数据。
二叉树的遍历实验报告
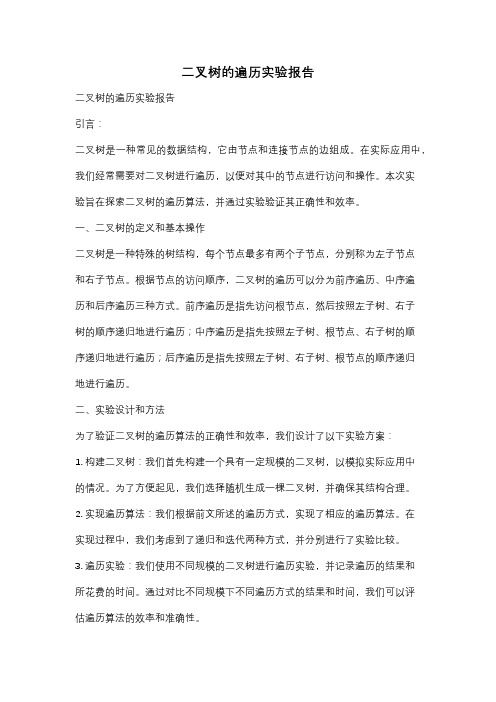
二叉树的遍历实验报告二叉树的遍历实验报告引言:二叉树是一种常见的数据结构,它由节点和连接节点的边组成。
在实际应用中,我们经常需要对二叉树进行遍历,以便对其中的节点进行访问和操作。
本次实验旨在探索二叉树的遍历算法,并通过实验验证其正确性和效率。
一、二叉树的定义和基本操作二叉树是一种特殊的树结构,每个节点最多有两个子节点,分别称为左子节点和右子节点。
根据节点的访问顺序,二叉树的遍历可以分为前序遍历、中序遍历和后序遍历三种方式。
前序遍历是指先访问根节点,然后按照左子树、右子树的顺序递归地进行遍历;中序遍历是指先按照左子树、根节点、右子树的顺序递归地进行遍历;后序遍历是指先按照左子树、右子树、根节点的顺序递归地进行遍历。
二、实验设计和方法为了验证二叉树的遍历算法的正确性和效率,我们设计了以下实验方案:1. 构建二叉树:我们首先构建一个具有一定规模的二叉树,以模拟实际应用中的情况。
为了方便起见,我们选择随机生成一棵二叉树,并确保其结构合理。
2. 实现遍历算法:我们根据前文所述的遍历方式,实现了相应的遍历算法。
在实现过程中,我们考虑到了递归和迭代两种方式,并分别进行了实验比较。
3. 遍历实验:我们使用不同规模的二叉树进行遍历实验,并记录遍历的结果和所花费的时间。
通过对比不同规模下不同遍历方式的结果和时间,我们可以评估遍历算法的效率和准确性。
三、实验结果和分析在实验中,我们构建了一棵具有1000个节点的二叉树,并分别使用前序、中序和后序遍历算法进行遍历。
通过实验结果的比较,我们得出以下结论:1. 遍历结果的正确性:无论是前序、中序还是后序遍历,我们都能够正确地访问到二叉树中的每个节点。
这表明我们所实现的遍历算法是正确的。
2. 遍历算法的效率:在1000个节点的二叉树中,我们发现中序遍历算法的执行时间最短,后序遍历算法的执行时间最长,前序遍历算法的执行时间居中。
这是因为中序遍历算法在访问节点时可以尽可能地减少递归次数,而后序遍历算法需要递归到最深层才能返回。
遍历二叉树实验报告
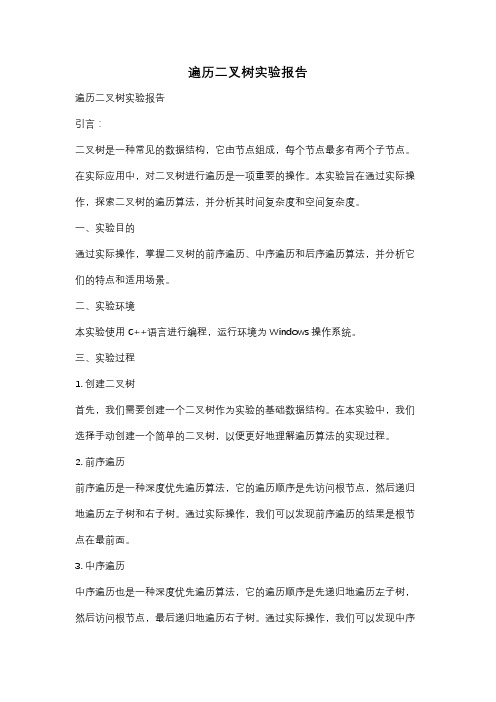
遍历二叉树实验报告遍历二叉树实验报告引言:二叉树是一种常见的数据结构,它由节点组成,每个节点最多有两个子节点。
在实际应用中,对二叉树进行遍历是一项重要的操作。
本实验旨在通过实际操作,探索二叉树的遍历算法,并分析其时间复杂度和空间复杂度。
一、实验目的通过实际操作,掌握二叉树的前序遍历、中序遍历和后序遍历算法,并分析它们的特点和适用场景。
二、实验环境本实验使用C++语言进行编程,运行环境为Windows操作系统。
三、实验过程1. 创建二叉树首先,我们需要创建一个二叉树作为实验的基础数据结构。
在本实验中,我们选择手动创建一个简单的二叉树,以便更好地理解遍历算法的实现过程。
2. 前序遍历前序遍历是一种深度优先遍历算法,它的遍历顺序是先访问根节点,然后递归地遍历左子树和右子树。
通过实际操作,我们可以发现前序遍历的结果是根节点在最前面。
3. 中序遍历中序遍历也是一种深度优先遍历算法,它的遍历顺序是先递归地遍历左子树,然后访问根节点,最后递归地遍历右子树。
通过实际操作,我们可以发现中序遍历的结果是根节点在中间。
4. 后序遍历后序遍历同样是一种深度优先遍历算法,它的遍历顺序是先递归地遍历左子树和右子树,最后访问根节点。
通过实际操作,我们可以发现后序遍历的结果是根节点在最后面。
5. 分析与总结通过对前序遍历、中序遍历和后序遍历的实际操作,我们可以得出以下结论:- 前序遍历适合于需要先处理根节点的场景,例如树的构建和复制。
- 中序遍历适合于需要按照节点值的大小顺序进行处理的场景,例如搜索二叉树的构建和排序。
- 后序遍历适合于需要先处理叶子节点的场景,例如树的销毁和内存释放。
四、实验结果通过实际操作,我们成功实现了二叉树的前序遍历、中序遍历和后序遍历算法,并得到了相应的遍历结果。
这些结果验证了我们对遍历算法的分析和总结的正确性。
五、实验总结本实验通过实际操作,深入探索了二叉树的遍历算法,并分析了它们的特点和适用场景。
二叉树的遍历实验报告
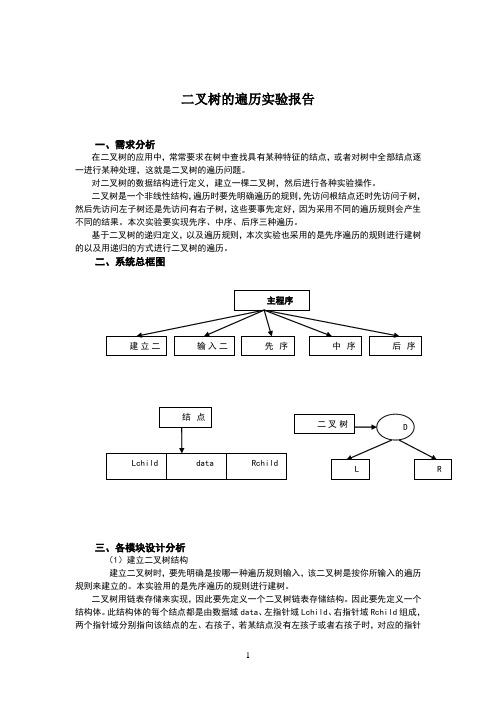
二叉树的遍历实验报告一、需求分析在二叉树的应用中,常常要求在树中查找具有某种特征的结点,或者对树中全部结点逐一进行某种处理,这就是二叉树的遍历问题。
对二叉树的数据结构进行定义,建立一棵二叉树,然后进行各种实验操作。
二叉树是一个非线性结构,遍历时要先明确遍历的规则,先访问根结点还时先访问子树,然后先访问左子树还是先访问有右子树,这些要事先定好,因为采用不同的遍历规则会产生不同的结果。
本次实验要实现先序、中序、后序三种遍历。
基于二叉树的递归定义,以及遍历规则,本次实验也采用的是先序遍历的规则进行建树的以及用递归的方式进行二叉树的遍历。
二、系统总框图三、各模块设计分析(1)建立二叉树结构建立二叉树时,要先明确是按哪一种遍历规则输入,该二叉树是按你所输入的遍历规则来建立的。
本实验用的是先序遍历的规则进行建树。
二叉树用链表存储来实现,因此要先定义一个二叉树链表存储结构。
因此要先定义一个结构体。
此结构体的每个结点都是由数据域data 、左指针域Lchild 、右指针域Rchild 组成,两个指针域分别指向该结点的左、右孩子,若某结点没有左孩子或者右孩子时,对应的指针域就为空。
最后,还需要一个链表的头指针指向根结点。
要注意的是,第一步的时候一定要先定义一个结束标志符号,例如空格键、#等。
当它遇到该标志时,就指向为空。
建立左右子树时,仍然是调用create()函数,依此递归进行下去,直到遇到结束标志时停止操作。
(2)输入二叉树元素输入二叉树时,是按上面所确定的遍历规则输入的。
最后,用一个返回值来表示所需要的结果。
(3)先序遍历二叉树当二叉树为非空时,执行以下三个操作:访问根结点、先序遍历左子树、先序遍历右子树。
(4)中序遍历二叉树当二叉树为非空时,程序执行以下三个操作:访问根结点、先序遍历左子树、先序遍历右子树。
(5)后序遍历二叉树当二叉树为非空时,程序执行以下三个操作:访问根结点、先序遍历左子树、先序遍历右子树。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
Q.rear=s;
}
int DeQueue(LinkQueue &Q,BiTree &p){//zhongyao
QueuePtr s;
if(Q.front==Q.rear){
printf("队列已空,不能进行出队操作\n");
return(ERROR);
}LinkQueue;
int CreateBiTree(BiTree &T){
char ch,c;
scanf("%c",&ch);
if(ch==' ')
T=NULL;
else{
if(!(T=(BiTree)malloc(sizeof(BiNode))))
return(ERROR);
CreateBiTree(T);
printf("按层次遍历二叉树各节点后的结果\n");
levelOrder(T);
printf("\n");
}
BiTree p;
LinkQueue Q;
InitQueue(Q);
p=T;
if(!T){
printf("树为空树\n");
return(ERROR);
}
EnQueue(Q,p);
while(!EmptyQueue(Q)){
DeQueue(Q,p);
}BiNode,*BiTree;
typedef struct QNode{
BiTree data;
struct QNode *next;
}QNode,*QueuePtr;
typedef struct {
QueuePtr front;
QueuePtr rear;
}
s=Q.front->next;
p=s->data;
printf("%c",p->data);
Q.front->next=s->next;
if(Q.rear==s)
Q.rear=Q.front;
free(s);
return(OK);
}
int levelOrder(BiTree &T){
return(OK);
else
return(ERROR);
}
void EnQueue(LinkQueue &Q,BiTree p){
QueuePtr s;
s=(QueuePtr)malloc(sizeof(QNode));
s->data=p;
s->next=NULL;
T->data=ch;
CreateBiTree(T->lchild);
CreateBiTree(T->rchild);
}
return(OK);
}
int InitQueue(LinkQueue &Q){
Q.front=Q.rear=(QueuePtr)malloc(sizeof(QNode));
if(p->lchild!=NULL){
EnQueue(Q,p->lchild);
}
if(p->rchild!=NULL){
EnQueue(
Q,p->rchild);
}
}
return(OK);
}
void main(){
BiTree T;
if(!Q.front){
printf("队列内存分配失败\n");
ቤተ መጻሕፍቲ ባይዱeturn(ERROR);
}
else
Q.front->next=NULL;
return(OK);
}
int EmptyQueue(LinkQueue &Q){
if(Q.front->next==NULL)
#include "stdio.h"
#include "malloc.h"
#define OK 1
#define ERROR 0
#define NULL 0
typedef struct BiNode{
char data;
struct BiNode *lchild,*rchild;