兰州理工大学研究生课程VC++课件4
VC课件 (2)PPT教学课件

}
}
1
2020/12/09
3.1.2 在SDK上运行C#程序
3.1.2.1 步骤
① 单击“开始”菜单,选择“程序”菜单项,再选择“附件”,然后 单击“记事本”,打开记事本后,就将上述程序输入到记事本,见 图3.1。
② 输入完毕,请检查一下,保存文件,文件名后缀应该是“.cs”,保 存类型取“所有文件”,我们在E盘开设一个文件夹“C#练习”, 用来存放C#程序,见图3.2。
编译器选项 3.1.3 简单的输入输出和类型转换 3.1.3.2 System.Convert类
类型转换是编程时经常遇到,初学者特别困惑的问题之一,而 System.Convert类可以说是个万能的类型转换工具,用来将 一个基本数据类型转换为另一个基本数据类型。当然,转换 不可能是任意的,详细的语法规定请参见.NET的帮助文件或 以后章节。
③ 运行程序,单击“开始”菜单,选择“程序”,再选择“附件”, 然后单击“命令提示符”,打开了“命令提示符”窗体,见图3.3。
④ 在当前光标下键入“e:”,按回车键,转到E盘,然后键入命令“cd C#练习”,转到我们存放C#程序的文件夹,注:如果读者在其它区 域中开设存放C#程序的文件夹,则可自行改变文件夹名和路径。
的字符串可以嵌入在参数内;
反斜杠按其原义解释,除非双引号紧接在反斜杠之后;如果双引号紧接在反 斜杠之后,则它们的意义有如下规律: 前面有有一个反斜杠的双引号(\")被解释为原义,即字符双引号; 如果偶数个反斜杠后跟双引号,则每对反斜杠中的一个反斜杠放置在 argv数组中,并且双引号被解释为字符串分隔符。 如果奇数个反斜杠后跟双引号,则每对反斜杠中的一个反斜杠放置在 argv数组中,双引号由其余的反斜杠“转义”,使原义双引号被放 置在argv数组中。
visual C++课件第4章
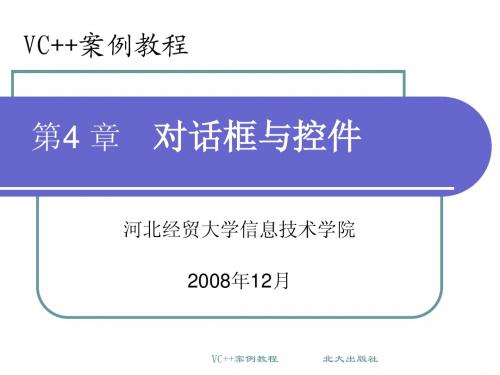
控件的创建与使用
在对话框中创建和使用控件的步骤如下:
用对话框编辑器将控件添加在对话框模板中; 利用类向导为对话框类增加与控件相关联的数据成 员; 利用类向导为对话框类增加与控件相关联的消息处 理函数; 在对话框类的OnInitDialog函数体中,为控件设置一 些初始条件; 在对话框类的控件消息处理函数中,添加控件处理 代码。
VC++案例教程 北大出版社
访问控件
一是用DDX(对话数据交换)技术 使用CWnd::UpdateData()函数可实现控件与其成员变量(值变量) 之间的数据的传输。其中: UpdateData(TRUE)——将控件中的数据传送给成员变量; UpdateData(FALSE)——将成员变量的数据传递给控件并显示。 例如,要在编辑框ID_NAME中显示“张”,则可以用下面两种方 法实现: 关联值变量,为编辑框ID_NAME关联CString变量m_Name,然后 执行下面的语句: m_Name=” 张”; UpdateData(false); 关联控件变量,为编辑框ID_NAME关联控件类CEdit变量 m_EditName,然后执行下面的语句: m_EditName.SetWindowText(“张”);
1. 对话框消息的发送 WM_INITDIALOG消息告诉你此时应初始化一个对话框。即所有 控件被创建并准备好。 WM_COMMAND消息是由控件发送的,用来通知对话框一些感兴 趣或有用的事件。当一个控件发送这个消息时,它在消息上签上 它的名字和一个通知码。 2. MFC对对话框的支持---CDialog 3. 公共对话框 CFileDialog CColorDialog CFontDialog CPrintDialog CFindReplaceDialog CPageSetupDialog
2024版C基础知识教学PPT课件

01 C语言概述ChapterC语言的历史与发展标准化起源1983年,美国国家标准协会(ANSI)制定了为ANSI C。
发展C语言的特点与优势简洁高效可移植性强强大的指针操作丰富的库函数01020304系统级编程游戏开发嵌入式开发算法与数据结构C 语言的应用领域02 C语言基础语法Chapter标识符、变量和常量标识符用来标识变量、函数、类型等程序实体的名称,由字母、数字和下划线组成,且第一个字符必须是字母或下划线。
变量用于存储数据的内存空间,其值可以改变。
在C语言中,必须先声明变量才能使用。
常量在程序运行过程中值不会改变的量。
C语言中可以使用`const`关键字来定义常量。
数据类型与运算符数据类型运算符选择结构根据条件判断结果,选择执行不同的代码块。
C 语言中提供了`if`、`switch`等选择结构语句。
顺序结构程序按照代码的顺序依次执行,没有分支和跳转。
循环结构根据循环条件重复执行某段代码块。
C 语言中提供了`for`、`while`、`do-while`等循环结构语句。
控制结构(顺序、选择、循环)函数与数组函数数组用于存储多个相同类型数据的集合。
数组中的元素按照顺序排列,可以通过下标访问。
C语言中提供了一维数组、二维数组等多维数组类型。
03指针与内存管理Chapter指针的概念与基本操作指针的定义与性质01指针的声明与初始化02指针的基本操作03内存分配与释放动态内存分配使用C标准库中的malloc、calloc等函数在堆区动态分配内存空间,以满足程序运行时的动态需求。
内存释放使用free函数释放之前分配的内存空间,防止内存泄漏和资源浪费。
内存分配失败处理检查动态内存分配函数的返回值,判断内存分配是否成功,并采取相应措施。
数组名作为指针指针访问数组元素指针与多维数组030201指针与数组的关系指针的高级应用指针与函数指针与结构体指向函数的指针指向指针的指针04文件操作与输入输出Chapter文件的基本概念与操作01020304文件的定义文件的分类文件指针文件的打开与关闭标准输入输出函数标准输入函数标准输出函数格式化输入输出文件读写操作文件的顺序读写文件的随机读写文件的二进制读写1 2 3文件定位文件错误处理文件结束判断文件定位与错误处理05数据结构与算法基础Chapter链表的创建与初始化链表的遍历与查找链表节点的插入与删除栈的基本概念与操作栈的定义与特点栈的创建与初始化入栈与出栈操作栈的应用举例队列的基本概念与操作01队列的定义与特点020304队列的创建与初始化入队与出队操作循环队列的实现与应用树的定义与基本术语树的表示方法与存储结构01 02 03树与二叉树123010203各种排序算法的时间复杂度分析与比较常见查找算法介绍与实现顺序查找、二分查找等查找算法的原理与实现哈希表查找算法的原理与实现各种查找算法的时间复杂度分析与比较06C语言程序设计与调试技巧Chapter01020304将程序划分为独立的功能模块,降低复杂度,提高可维护性。
C大学基础教程第一章精品PPT课件
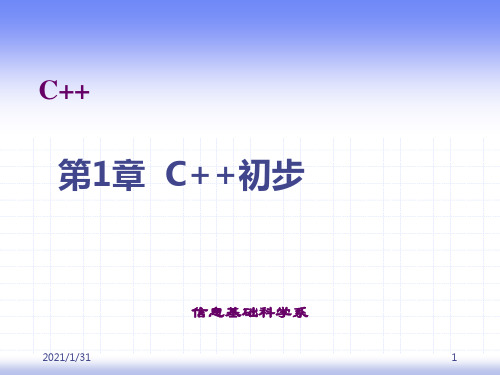
-18-
1.4 C++的诞生
C语言之父 dennis Ritchie
The C Programming Language
-19-
1.4 C++的诞生
-25-
1.6 简单的C++程序
-26-
1.6 简单的C++程序
/***************************************
程序文件:ch1_1.cpp
程序功能:在屏幕上输出hello world!
作 者:XXX
创建时间:XX年XX月XX日
***************************************/
C++
第1章 C++初步
信息基础科学系
2021/1/31
1
C++语言是一门优秀的程序设 计语言,在计算机科学领域 中有着广泛的应用。
-2-
第一章 C++入门
1.1 程序设计语言的发展 1.2 面向过程的程序设计 1.3 面向对象的程序设计 1.4 C++的诞生 1.5 程序开发过程及开发环境 1.6 简单的C++程序
-11-
1.2 面向过程的程序设计
计算每门课的平均成绩
多少门课 学生人数
每门课总分
选课学生档案
每个学生该 门课的成绩
每门课总分 /学生人数
累加
选择一门课 选课的学生 读数据
vc-4绘图-vc入门PPT课件

❖ CClientDC类代表了客户区设备环境。当在客户区实 时绘图时,需要利用CClientDC类定义一个客户区设 备环境。
❖ CPaintDC类用于响应WM_PAINT消息以支持重画。客户 区的重绘由函数OnPaint()完成。客户区重绘也可使 用Invalidate()函数来完成。
void Invalidate(BOOL bErase=TRUE)
2021/7/23
3
Windows绘图过程和设备无关性的实现:
应用程序首先向设备环境提出输出的请求,设
备环境接收绘图器请求(表现为GDI函数调用),并 将它们传给相应的设备驱动程序,完成特定硬件的
输出。
2021/7/23
4
❖ 为了支持GDI绘图,MFC提供了两个类:
设备环境类(上下文类):用于设置绘图属性和 绘制图形。
深红 128,0,0
0,255,0
深绿 0,128,0
0,0,255
深蓝 0,0,128
255,255,0
深黄 128,128,0
0,255,255
深青 0,128,128
255,0,255
灰色 192,192,192
255,255,255
黑色 0,0,0
14
选择一个GDI对象: 要使用创建的对象,首先应将它选入到设备上下
GDI简介
❖ Windows提供了一个抽象的图形界面接口,称为 图形设备接口(Graphics Device Interface) , 或简称GDI。
❖ 它负责管理用户绘图操作时功能的转换。用户 通过调用GDI函数与设备打交道,GDI通过不同 设备提供的驱动程序将绘图语句转换为对应的 绘图指令,避免了直接对硬件进行操作,从而 实现设备无关性。
VC使用教程PPT课件
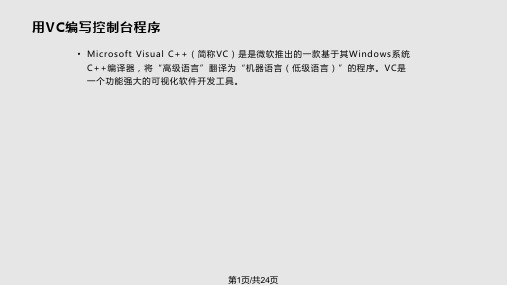
第2页/共24页
什么是控制台程序?
• 一个最简单的控制台程序如下:
/* helloworld.c */ #include<stdio.h>
/* 包含使用标准输入 输出的头文件 */
➢双击桌面上的vc++6.0图标 ➢鼠标左键单击Windows操作系统的“开始” →“程序” →“Microsoft Visual Studio 6.0” →“Microsoft Visual C++ 6.0”
第5页/共24页
新建
File → New (Ctrl+N)
第6页/共24页
创建一个新的项目文件
第21页/共24页
关闭文件和工作区
选择菜单“File\Close WorkSpace”,关闭当前 的工作区和所有打开的文件。
第22页/共24页
23
第23页/共24页
感谢您的观看!
第24页/共24页
c程序开发过程打开vc60双击桌面上的vc60图标鼠标左键单击windows操作系统的开始程序microsoftvisualstudio60microsoftvisual60新建filenewctrln创建一个新的项目文件新建工程命名修改工程存放路径创建一个空工程生成一个空工程vc60的编辑窗口自动生成的文件和文件夹工程管理结构workspaceprojectproject我们一般把自定义数据类型函数等的定义和声明放置在头文件中
.c
工程唯一的main函数
.c 把相应的实现细节放置在(*.c)文件中
Projec/共24页
兰州理工大学,研究生VC++课件6

• The general form of a declaration of a pointer to a function looks like this:
return_type (*pointer_name)(list_of_parameter_types);
• The declaration of a pointer to a function consists of three components: • The return type of the functions that can be pointed to • The pointer name preceded by an asterisk to indicate it is a pointer • The parameter types of the functions that can be pointed to
double (*pfun)(char*, int); // Pointer to function declaration
• This declares a pointer with the name pfun that can point to functions that accept two arguments, one of type pointer to char and another of type int, and return a value of type double.
• Clearly, this is going to restrict what you can store in a particular pointer to a function.
Declaring Pointers to Functions
VC 学习教程绪论.ppt

➢ ATL(ActiveX模板类库)
➢ 其他的SDK,如 OpenGL ,DirectX ,ActiveMoive ,DrawDib(WinG)
VC是什么?学VC是学什么?
VC是许许多多技术的综合,就好象少林72般绝技,一 般在应用中学会其中很少一部分就够了。我们的现在目的, 其实不过是学一套少林长拳。这套长拳应包括:
Why do we choose VC++?
VC++ vs Visual Basic
1. VB gained many third party supports and is widely used in somewhat quick programming.
2. VB is not real Object-Oriented Language, no data inheritance.
为什么用VC而不是其他编程语言?
大多数大型软件(包括Windows自己)都是用C、 C++编的,所以可以利用的源代码特别多。例如 Photoshop,就有VC的编程接口。其他的语言都不如VC 有历史优势。而且VC的开发工具特别多,从控件公司到硬 件开发商,其产品很少敢不提供VC接口的。
VC适合组织大工程(VB就不行)
1养成一种严谨的软件开发习惯熟悉软件工程的基本原则22c及c语言基础c及c语言基础类继承性封装性多态性的概念类继承性封装性多态性的概念3如何用向导建立一个程序框架4设计菜单工具条对话框熟悉最常用的对话框控件5知道怎样新建类成员函数成员变量消息处理函数6了解最常用的windows消息7了解最简单的gdi图形设备接口
如何学VC?
多交流
学习 VC尤其要和别人交流,你苦苦思考不明白的,也 许别人早就解决了。一定要善于学习:从书店找出有用的书; 向身边的高手学习;寻找可以利用的源代码、类库、组件、 控件、库;如果能上互连网,那就更加能解决问题了。
兰州理工大学研究生课程VC++课件5

Why Do You Need Байду номын сангаасunctions?
• One major advantage that a function offers is that it can be executed as many times as necessary from different points in a program. • Without the ability to package a block of code into a function, programs would end up being much larger, because you would typically need to replicate the same code at various points in them. • You also use functions to break up a program into easily manageable chunks for development and testing; a program of significant size and complexity that consists of several small blocks of code is much easier to understand and test than if it were written as one large chunk.
5.Introducing Structure In Your Programs
201405
WHAT YOU WILL LEARN IN THIS CHAPTER:
• • • • • • How to declare and write your own C++ functions How function arguments are defined and used How to pass arrays to and from a function What pass-by-value means How to pass pointers to functions How to use references as function arguments, and what pass-by-reference means • How the const modifier affects function arguments • How to return values from a function • How to use recursion
《VC开发环境使用》PPT课件

2019/5/2
19
Visual C++的菜单栏------View菜单
(5) Class Info选项卡:提供有关类的信息。
2019/5/2
20
Visual C++的菜单栏------View菜单
2.Resource Symbols选项 该选项将打开资源符号浏览器,从中可以
浏览资源编辑符号
2019/5/2
Makefile
创建 Make 文件
MFC
ActiveX 创建 ActiveX 控件程
ControlWizard
序
MFC AppWizard(dll)
创 建 MFC 动 态 MFC
链接库
AppWizard(exe)
创建 MFC 可执行程 序
Win32 Application
创建 Win32 应用 Win32
选项用于复制选定的资源
2019/5/2
25
Visual C++的菜单栏----Insert菜单
5. New ATL Object选项 启动ATL Object Wizard,以便添加新 的ATL对象
2019/5/2
26
Visual C++的菜单栏------ Project菜单
用Project可创建、修改和存储正在编辑的工程文件 工程文件组合了一个应用程序的所有源文件的组成 部分,其扩展名为.MAK。并非所有的.MAK文件都是 Visual C++工程文件。使用AppWizard可创建与Visual C++兼容的工程文件,同时,也可创建只能由NMake使 用的工程文件。
Console 创 建 Win32 控 制 台
程序设计相关概念CCVC及VC使用PPT教案

程序编辑窗口
第25页/共39页
26
2. 输入和编辑源程序
(1)新建一个源程序 ① 在VC++主窗口的主菜单栏中选择“File” ,然后 选择 “New ”
第26页/共39页
27
单击屏幕上出现的“New”对话框上 方的“Files”, 在其下 拉菜单 中选择 “C+ + Source File”项;然后在对话框右半“Location”文本 框中输 入准备 编辑的 源程序 文件的 存储路 径(假 定为 D:\cc );在其上方的File(文件)文本框中 输入准 备编辑 的源程 序文件 的名字 (输入c 1-1.c ) 。
第14页/共39页
15
C++
C++:包括了整个C(C的全部特征、属性、优点)。
支持面向对象编程(OOP) 包括过程性语言部分和类部分
过程性语言部分:与C无本质上的区别。 类部分:面向对象程序设计的主体。
第15页/共39页
16
VC
Visual C++ 6.0是编辑/运行/调试C/C++程 序的集成环境.
第11页/共39页
12
C语言
C语言是国际上广泛流行的高级语言。
系统软件、应用软件
1973年贝尔实验室的D.M.Ritchie ,设计出了C语 言,并用之改写了原来用汇编编写的UNIX。
1978年影响深远的名著《The C Programming Language》由 Brian W.Kernighan和Dennis M.Ritchie 合著,被称为标准C。
✓ 语句 ✓ C程序由若干条语句组成。 ✓每条语句用“;”作为终止符。 非法
✓ 注释 例: /*This is the main /* of example1.1*/ */ ✓ /*……*/为注释,不能嵌套。 ✓不产生编译代码。
兰州理工大学研究生学位课程

教学用书:
朱吉庆, 冶金热力学,长沙:中南大学出版社,1995 华一新, 冶金过程动力学导论,北京:冶金工业出版社,2004
参考书:
马荣骏,湿法冶金原理,北京:冶金工业出版社,2007
梁连科 , 冶金热力学及动力学,沈阳:东北大学出版社,1990
莫鼎成, 冶金动力学,长沙:中南大学出版社,1987
陈新民,陈启元,冶金热力学导论,北京:冶金工业出版社, 1986 傅杰,钢冶金过程动力学,北京:冶金工业出版社,2001
•
1.2 ΔGθ的计算
在等温方程式中,JP是由反应进行的实际条件来决定 的。要计算ΔG,首先必须计算ΔGθ。 由ΔGθ还可根据ΔGθ =-RTlnK求出反应的平衡常数。 ΔGθ的计算是冶金热力学计算的一个重要内容。
•
ΔGθ计算方法分类:
• 积分法 • 线性组合法(这是冶金中最常用的一种计
算ΔGθ的方法) • 自由能函数计算法 • 用相对焓和相对自由能数据的计算方法
•
线性组合法是冶金中最常用的一种计算ΔGθ的方法。
在物理化学中已经介绍过,一个反应的ΔHθ(298)等于该反 应所有产物的生成热之和减去所有各反应物的生成热之和。
H (298)
vi
f
H
i
(298)
这个公式不仅适用于反应热的计算,对反应的其它热力学
函数也适用。
G
vi
中,如果ΔGθ的绝对值很大(一般认为当G 41.8KJ / mol时) ΔGθ的符号(是正或是负)基本决定了ΔG的符号,很难通 过改变Jp的数值来改变ΔG的符号。 这个界限值(41.8kJ/mol)对常温下的许多反应大体上是可 用的,但对高温下的冶金反应就不适用了。
c课程设计南理工

c 课程设计南理工一、教学目标本课程的教学目标是使学生掌握南理工课程的核心知识,培养学生运用所学知识解决实际问题的能力,并培育学生对学科的兴趣和热情。
具体目标如下:1.知识目标:学生能够准确理解并掌握南理工课程中的基本概念、原理和知识点,构建系统化的知识结构。
2.技能目标:学生能够运用所学知识分析和解决实际问题,提高批判性思维和创新能力。
3.情感态度价值观目标:学生对南理工课程产生浓厚的兴趣,形成积极的学习态度,培养团队合作精神和责任感。
二、教学内容根据教学目标,本课程的教学内容选取南理工课程中的重要章节和知识点,确保内容的科学性和系统性。
具体内容包括:1.南理工课程的基本概念和原理,通过讲解和案例分析使学生深入理解并掌握。
2.南理工课程的重点和难点,通过讲解、讨论和实验等方法,帮助学生克服困难,提高学习效果。
3.南理工课程在实际应用中的案例分析,培养学生运用所学知识解决实际问题的能力。
三、教学方法为了激发学生的学习兴趣和主动性,本课程将采用多种教学方法,如讲授法、讨论法、案例分析法和实验法等。
具体方法如下:1.讲授法:通过讲解南理工课程的基本概念和原理,使学生掌握知识点。
2.讨论法:学生进行分组讨论,培养学生独立思考和团队协作的能力。
3.案例分析法:分析南理工课程在实际应用中的案例,培养学生解决实际问题的能力。
4.实验法:进行实验操作,使学生更好地理解南理工课程的知识点。
四、教学资源为了支持教学内容和教学方法的实施,丰富学生的学习体验,我们将选择和准备以下教学资源:1.教材:南理工课程教材,为学生提供系统化的学习资料。
2.参考书:推荐学生阅读相关参考书,拓展知识面。
3.多媒体资料:制作课件、视频等多媒体资料,提高学生的学习兴趣。
4.实验设备:准备实验所需的设备,为学生提供实践操作的机会。
五、教学评估本课程的教学评估将采用多元化的方式,以全面、客观、公正地评价学生的学习成果。
具体评估方式如下:1.平时表现:通过课堂参与、提问、讨论等环节,评估学生的学习态度和积极性。
兰州理工大学研究生重点学位课程

资助项目中期进展报告
(年项目)
课程名称:
课程负责人:
职称:
所在单位:
联系电话:
报告日期:
兰州理工大学研究生院制
一.课程建设完成情况
1.本课程的建设目标与任务完成情况:
1)主要建设与验收目标
2)任务完成情况:
教材建设(已出版或已签订协议待出版的教材)
教学方法与手段(教案、多媒体课件、相关动画、实验与课堂教学设计等)
签章:
年月日
教学研究成果(含已发表的教学研究论文、完成的研究报告等)
教学团队建设(含教学团队成员建设周期内上课安排、青年教师培养情施、计划
3.经费使用情况
二.考核结果
学院(部)意见:
学院院长 签字:
学院(部门)签章
年 月 日
专家组评审意见:
组长签字:
年月日
研究生院意见:
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
Arrays
• The basis for the solution to all of these problems is provided by the array. • An array is simply a number of memory locations called array elements or simply elements, each of which can store an item of data of the same given data type, and which are all referenced through the same variable name.
– }
• // Output results from 2nd entry to last entry
– for (int i = 1; i < count; i++) – {
• cout << endl
– – – – << setw(2) << i << "." // Output sequence number << "Gas purchased = " << gas[i] << " gallons" // Output gas << " resulted in " // Output miles per gallon << (miles[i] - miles[i - 1]) / gas[i] << " miles per gallon.";
• Suppose that you needed to write a payroll program. • Using a separately named variable for each individual’s pay, their tax liability, and so on, would be an uphill task to say the least.
long height[6];
• Because each long value occupies 4 bytes in memory, the whole array requires 24 bytes.
• You can declare arrays to be of any type.
• For example, to declare arrays intended to store the capacity and power output of a series of engines, you could write the following:
• • • • • • • • • •
// EX04-01.cpp // Calculating gas mileage #include <iostream> #include <iomanip> using std::cin; using std::cout; using std::endl; using std::setw; int main() {
Declaring Arrays
• You declare an array in essentially the same way as you declared the variables that you have seen up to now, the only difference being that the number of elements in the array is specified between square brackets immediately following the array name. • For example, you could declare the integer array height, shown in the previous figure, with the following declaration statement:
– – – – – – – const int MAX(20); // Maximum number of values double gas[MAX]; // Gas quantity in gallons long miles[MAX]; // Odometer readings int count(0); // Loop counter char indicator('y'); // Input indicator while (('y' == indicator || 'Y' == indicator) && count < MAX) {
HANDLING MULTIPLE DATA VALUES OF THE SAME TYPE
• You already know how to declare and initialize variables of various types that each holds a single item of information. • The most obvious extension to the idea of a variable is to be able to reference several data elements of a particular type with a single variable name. • This would enable you to handle applications of a much broader scope.
double engine_size[10]; // Engine size in cubic inches double horsepower[10]; // Engine power output
Using Arrays
• As a basis for an exercise in using arrays, imagine that you have kept a record of both the amount of gasoline you have bought for the car and the odometer reading on each occasion. • You can write a program to analyze this data to see how the gas consumption looks on each occasion that you bought gas:
• • • • • • • cout << endl << "Enter gas quantity: "; cin >> gas[count]; // Read gas quantity cout << "Enter odometer reading: "; cin >> miles[count]; // Read odometer value ++count; cout << "Do you want to enter another(y or n)? "; cin >> indicator;
•= 1) // count = 1 after 1 entry completed – { // ... we need at least 2
• cout << endl << "Sorry - at least two readings are necessary."; • return 0;
• A much more convenient way to handle such a problem would be to reference an employee by some kind of generic name — employeeName to take an imaginative example — and to have other generic names for the kinds of data related to each employee, such as pay, tax, and so on.
• To refer to a particular element, you write the array name, followed by the index value of the particular element between square brackets.
• The amount of memory required to store each element is determined by its type, and all the elements of an array are stored in a contiguous block of memory.
4. Arrays,Strings,and Pointers
201405
WHAT YOU WILL LEARN IN THIS CHAPTER:
• • • • • • • • How to use arrays How to declare and initialize arrays of different types How to use the range-based for loop with an array How to declare and use multidimensional arrays How to use pointers How to declare and initialize pointers of different types The relationship between arrays and pointers How to declare references and some initial ideas on their uses