第六章-树和森林习题
数据结构-习题-第六章-树
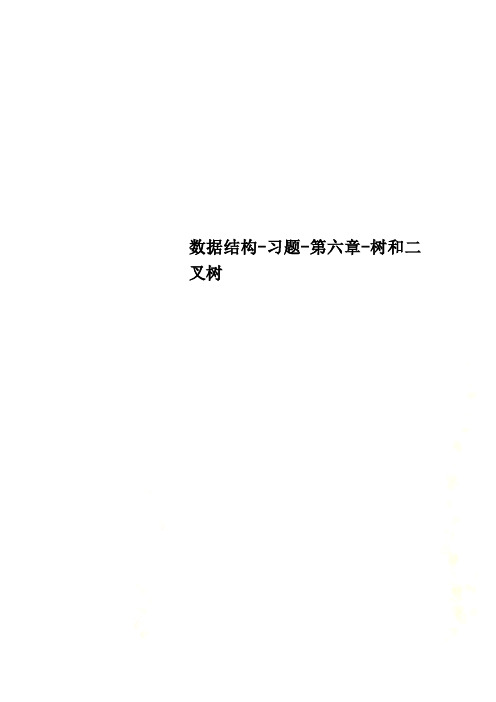
数据结构-习题-第六章-树和二叉树E F D G A B / + + * - C * 第六章 树和二叉树一、选择题1.已知一算术表达式的中缀形式为 A+B*C-D/E ,后缀形式为ABC*+DE/-,其前缀形式为( )A .-A+B*C/DE B. -A+B*CD/EC .-+*ABC/DE D. -+A*BC/DE【北京航空航天大学 1999 一、3 (2分)】2.算术表达式a+b*(c+d/e )转为后缀表达式后为( )【中山大学 1999 一、5】A .ab+cde/*B .abcde/+*+C .abcde/*++D .abcde*/++ 3. 设有一表示算术表达式的二叉树(见下图), 它所表示的算术表达式是( )【南京理工大学1999 一、20(2分)】A. A*B+C/(D*E)+(F-G)B.(A*B+C)/(D*E)+(F-G)C. (A*B+C)/(D*E+(F-G ))D.A*B+C/D*E+F-G4. 设树T 的度为4,其中度为1,2,3和4的结点个数分别为4,2,1,1 则T 中的叶子数为( )A .5B .6C .7D.8【南京理工大学 2000 一、8 (1.5分)】5. 在下述结论中,正确的是()【南京理工大学 1999 一、4 (1分)】①只有一个结点的二叉树的度为0; ②二叉树的度为2;③二叉树的左右子树可任意交换;④深度为K的完全二叉树的结点个数小于或等于深度相同的满二叉树。
A.①②③ B.②③④ C.②④ D.①④6. 设森林F对应的二叉树为B,它有m个结点,B的根为p,p的右子树结点个数为n,森林F中第一棵树的结点个数是()A.m-n B.m-n-1 C.n+1 D.条件不足,无法确定【南京理工大学2000 一、17(1.5分)】7. 树是结点的有限集合,它( (1))根结点,记为T。
其余结点分成为m(m>0)个((2))的集合T1,T2,…,Tm,每个集合又都是树,此时结点T称为Ti的父结点,Ti称为T的子结点(1≤i≤m)。
数据结构-第6章 树和二叉树---4. 树和森林(V1)
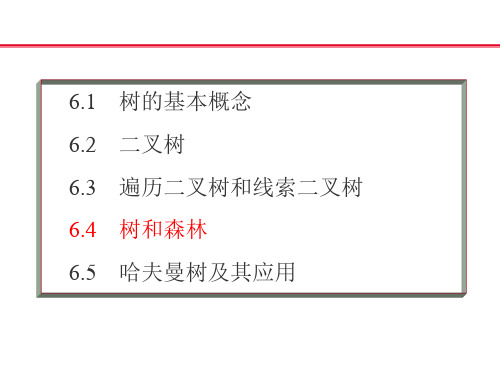
6.4.1 树的存储结构
R AB C D EG F
R⋀
A
⋀D
⋀B
⋀E ⋀
C⋀
⋀G
⋀F ⋀
6.4.2 树、森林和二叉树的转换
1. 树转换为二叉树 将树转换成二叉树在“孩子兄弟表示法”中已 给出,其详细步骤是: ⑴ 加线。在树的所有相邻兄弟结点之间加一 条连线。 ⑵ 去连线。除最左的第一个子结点外,父结点 与所有其它子结点的连线都去掉。 ⑶ 旋转。将树以根结点为轴心,顺时针旋转 450,使之层次分明。
B C
D
A E
L HK
M
技巧:无左孩子 者即为叶子结点
6.4.3 树和森林的遍历
1. 树的遍历 由树结构的定义可知,树的遍历有二种方法。 ⑴ 先序遍历:先访问根结点,然后依次先序 遍历完每棵子树等。价于对应二叉树的先序遍历
⑵ 后序遍历:先依次后序遍历完每棵子树,然 后访问根结点。等价于对应二叉树的中序遍历
0 R -1 1A 0 2B 0 3C 0
}Ptree ; R
4D 1 5E 1
AB C
6F 3
7G 6
DE
F
8H 6
9I 6
G H I 10~MAX_Size-1 ... ...
6.4.1 树的存储结构
2. 孩子表示法
每个结点的孩子结点构成一个单链表,即有n 个结点就有n个孩子链表;
n个孩子的数据和n个孩子链表的头指针组成一 个顺序表; 结点结构定义: 顺序表定义:
typedef struct PTNode { ElemType data ;
数据结构练习第六章树
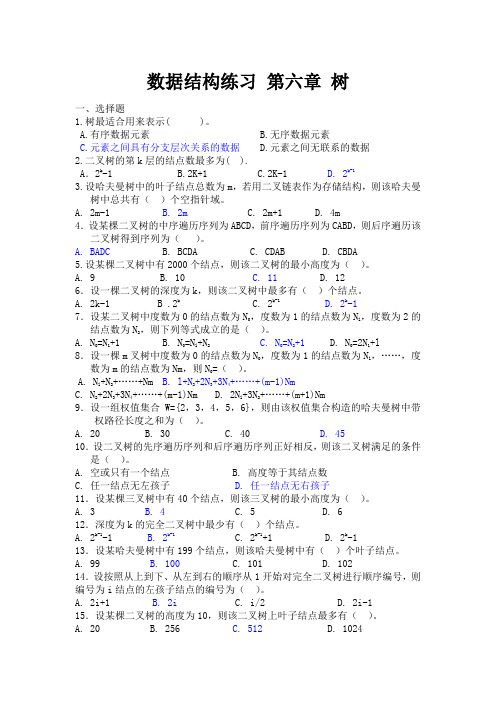
数据结构练习第六章树一、选择题1.树最适合用来表示( )。
A.有序数据元素B.无序数据元素C.元素之间具有分支层次关系的数据D.元素之间无联系的数据2.二叉树的第k层的结点数最多为( ).A.2k-1 B.2K+1 C.2K-1 D. 2k-13.设哈夫曼树中的叶子结点总数为m,若用二叉链表作为存储结构,则该哈夫曼树中总共有()个空指针域。
A. 2m-1B. 2mC. 2m+1D. 4m4.设某棵二叉树的中序遍历序列为ABCD,前序遍历序列为CABD,则后序遍历该二叉树得到序列为()。
A. BADCB. BCDAC. CDABD. CBDA5.设某棵二叉树中有2000个结点,则该二叉树的最小高度为()。
A. 9B. 10C. 11D. 126.设一棵二叉树的深度为k,则该二叉树中最多有()个结点。
A. 2k-1 B .2k C. 2k-1 D. 2k-17.设某二叉树中度数为0的结点数为N0,度数为1的结点数为Nl,度数为2的结点数为N2,则下列等式成立的是()。
A. N0=N1+1 B. N=Nl+N2C. N=N2+1 D. N=2N1+l8.设一棵m叉树中度数为0的结点数为N0,度数为1的结点数为Nl,……,度数为m的结点数为Nm,则N=()。
A. Nl +N2+……+Nm B. l+N2+2N3+3N4+……+(m-1)NmC. N2+2N3+3N4+……+(m-1)Nm D. 2Nl+3N2+……+(m+1)Nm9.设一组权值集合W={2,3,4,5,6},则由该权值集合构造的哈夫曼树中带权路径长度之和为()。
A. 20B. 30C. 40D. 4510.设二叉树的先序遍历序列和后序遍历序列正好相反,则该二叉树满足的条件是()。
A. 空或只有一个结点B. 高度等于其结点数C. 任一结点无左孩子D. 任一结点无右孩子11.设某棵三叉树中有40个结点,则该三叉树的最小高度为()。
A. 3B. 4C. 5D. 612.深度为k的完全二叉树中最少有()个结点。
第6章 树与森林测试题

第六章树与森林一、选择题1、设二叉树有n个结点且根结点处于第1层,则其高度为()。
A、n-1B、log2(n+1)-1C、log2n +1D、不确定2、设高度为h(空二叉树的高度为0,只有一个结点的二叉树的高度为1)的二叉树只有度为2和度为0的结点,则该二叉树中所含结点至少有()个。
A、2hB、2h -1C、2h +1D、h +13、设森林F中有4棵树,第1、2、3、4棵树的结点个数分别为n1、n2、n3、n4,当把森林F转换成一棵二叉树后,其根结点的右子树中有()个结点。
A、n1-1B、n1+n2+n3C、n2+n3+n4D、n14、将含有82个结点的完全二叉树从根结点开始顺序编号,根结点为第1号,其他结点自上向下,同一层自左向右连续编号。
则第40号结点的双亲结点的编号为()。
供选择的答案:A、20B、19C、81D、805、对二叉树从1开始编号,要求每个结点的编号大于其左右孩子的编号,同一个结点的左右孩子中,其左孩子的编号小于其右孩子的编号,则可采用()实现编号。
A、先序遍历B、中序遍历C、后序遍历D、从根开始进行层次遍历6、某二叉树的先序序列和后序序列正好相反,则该二叉树一定是()的二叉树。
A、空或只有一个结点B、高度等于其结点数C、任一结点无左孩子D、任一结点无右孩子7、在线索化二叉树中,t所指结点没有左子树的充要条件是()。
A、t-〉left==NULLB、t-〉ltag==1C、t-〉ltag==1且t-〉left==NULLD、.以上都不对8、二叉树按某种顺序线索化后,任一结点均有指向其前趋和后继的线索,这种说法()。
A、正确B、错误9、二叉树的前序遍历序列中,任意一个结点均处在其子女结点的前面,这种说法()。
A、正确B、错误10、设高度为h的二叉树上只有度为0和度为2的结点,则此类二叉树中所包含的结点数至少为()。
A、2hB、2h-1C、2h+1D、h+111、如果T2是由有序树T转换而来的二叉树,那么T中结点的前序就是T2中结点的()。
数据结构练习题--树(题)

第六章树一.名词解释:1 树 2。
结点的度 3。
叶子 4。
分支点 5。
树的度6.父结点、子结点 7兄弟 8结点的层数 9树的高度 10 二叉树11 空二叉树 12 左孩子、右孩子 13孩子数 14 满二叉树 15完全二叉树16 先根遍历 17 中根遍历 18后根遍历 19二叉树的遍历 20 判定树21 哈夫曼树二、填空题1、树(及一切树形结构)是一种“________”结构。
在树上,________结点没有直接前趋。
对树上任一结点X来说,X是它的任一子树的根结点惟一的________。
2、一棵树上的任何结点(不包括根本身)称为根的________。
若B是A的子孙,则称A是B的________3、一般的,二叉树有______二叉树、______的二叉树、只有______的二叉树、只有______ 的二叉树、同时有______的二叉树五种基本形态。
4、二叉树第i(i>=1)层上至多有______个结点。
5、深度为k(k>=1)的二叉树至多有______个结点。
6、对任何二叉树,若度为2的节点数为n2,则叶子数n0=______。
7、满二叉树上各层的节点数已达到了二叉树可以容纳的______。
满二叉树也是______二叉树,但反之不然。
8、具有n个结点的完全二叉树的深度为______。
9、如果将一棵有n个结点的完全二叉树按层编号,则对任一编号为i(1<=i<=n)的结点X有:(1)若i=1,则结点X是______;若i〉1,则X的双亲PARENT(X)的编号为______。
(2)若2i>n,则结点X无______且无______;否则,X的左孩子LCHILD(X)的编号为______。
(3)若2i+1>n,则结点X无______;否则,X的右孩子RCHILD(X)的编号为______。
10.二叉树通常有______存储结构和______存储结构两类存储结构。
11.每个二叉链表的访问只能从______结点的指针,该指针具有标识二叉链表的作用。
(无向)树没有回路的连通图.

现在假定在图T的任何两个点顶点之间存在唯一简单 通路。则T是连通的,因为在它的任何两个顶点之间存在 通路。另外,T没有简单回路。为了看出这句话是真的, 假定T有包含顶点x和y的简单回路。则在x和y之间就有两 条简单通路,因为这条简单回路包含一条从x到y的简单通 路和一条从y到x的简单通路。因此,在任何两个顶点之间 存在唯一简单通路的图是树。
加一条边{vi, vj},因为G中原来存在vi到vj的通路,故此时 形成一条经过vi和vj的回路。
习题 证明:简单图是树,当且仅当它是连通的,但是删除它的 任何一条边就产生不连通的图。
6.2根树 定义 有向树:有向图,底图是有向树
根树:有一个顶点(称为根)的入度为0,其余顶点 的入度均为1
例
定理 根树中,从根到其余每个顶点有且仅有一条通路
证明:因为T是根树,则作为无向图而言,根结点到任何 结点均有通路,若无有向通路,则一定存在某个结点,其 入度为0,这与根树的定义矛盾。又若根节点到某结点有 两条有向通路,则作为无向图看待时,必存在回路,故T 不成树,矛盾。
解:设该树有n1片树叶,有m条边,n个顶点 根据树的性质 m n 1 (n1 2 3) 1 n1 4 由握手定理得
n1 2 4 3 3 2(n1 4)
数据结构树习题

16、 假定在一棵二叉树中, 度为2的结点数为15, 度为1的结点数为30, 则叶子结点数为 ( 个。 A. 15 B. 16 C. 17 D. 47
)
17、在下列情况中,可称为二叉树的是( B ) 。 A. 每个结点至多有两棵子树的树 B. 哈夫曼树 C. 每个结点至多有两棵子树的有序树 D. 每个结点只有一棵子树 18、用顺序存储的方法,将完全二叉树中所有结点按层逐个从左到右的顺序存放在一维数 组R[1..n]中,若结点R[i]有左孩子,则其左孩子是( ) 。 A. R[2i-1] B. R[2i+1] C. R[2i] D. R[2/i] 19、下面说法中正确的是( ) 。 A. 度为2的树是二叉树 B. 度为2的有序树是二叉树 C. 子树有严格左右之分的树是二叉树 D. 子树有严格左右之分,且度不超过2的树是二叉树 20、树的先根序列等同于与该树对应的二叉树的( ) 。 A. 先序序列 B. 中序序列 C. 后序序列 21、按照二叉树的定义,具有3个结点的二叉树有( )种。 A. 3 B. 4 C. 5 D. 6
D. 层序序列
22、 由权值为3, 6, 7, 2, 5的叶子结点生成一棵哈夫曼树, 它的带权路径长度为 ( A. 51 B. 23 C. 53 D. 74
) 。
二、判断题 ( )1、存在这样的二叉树,对它采用任何次序的遍历,结果相同。 ( )2、中序遍历一棵二叉排序树的结点,可得到排好序的结点序列。 ( )3、对于任意非空二叉树,要设计其后序遍历的非递归算法而不使用堆栈结构,最 适合的方法是对该二叉树采用三叉链表。 ( )4、在哈夫曼编码中,当两个字符出现的频率相同时,其编码也相同,对于这种情 况应做特殊处理。 ( )5、一个含有n个结点的完全二叉树,它的高度是log2n+1。 ( )6、完全二叉树的某结点若无左孩子,则它必是叶结点。 三、填空题 1、具有n个结点的完全二叉树的深度是 。 2、哈夫曼树是其树的带权路径长度 的二叉树。 3 、 在 一 棵 二 叉 树 中 , 度 为 0 的 结 点 的 个 数 是 n0 , 度 为 2 的 结 点 的 个 数 为 n2 , 则 有 n0= 。 4、树内各结点度的 称为树的度。 四、代码填空题 1、函数InOrderTraverse(Bitree bt)实现二叉树的中序遍历,请在空格处将算法补 充完整。 void InOrderTraverse(BiTree bt){ if( ){ InOrderTraverse(bt->lchild); printf(“%c”,bt->data); ; }
第六章树的习题

六、树和二叉树一、选择题:1、在具有n个结点的完全二叉树中,结点i(i>1)的父结点是(D )A.2i B.不存在C.2i+1 D.⌊ i/2⌋3、下列陈述中正确的(A )A.二叉树是度为2的有序树B.二叉树中结点只有一个孩子时无左右之分C.二叉树中必有度为2的结点D.二叉树中最多只有两棵子树,并且有左右之分4、以二叉链表作为二叉树的存储结构,在具有n个结点的二叉链表中(n>0),空链域的个数为( C )A.2n - 1 B.n - 1 C.n + 1 D.2n + 15、将一棵有100个结点的完全二叉树从上到下,从左到右依次对结点进行编号,根结点的编号为1,则编号为49的结点的左孩子编号为(B )A.99 B.98 C.50 D.486、在一棵具有五层的满二叉树中,结点总数为( A )A.31 B.32 C.33 D.167、在一棵二叉树中,第5层上的结点数最多为(C )A.8 B.15 C.16 D.328、由二叉树的(B)遍历,可以惟一确定一棵二叉树A.前序和后序B.前序和中序C.后序D.中序9、具有35个结点的完全二叉树的深度为( B )。
A.5B.6C.7D.810、已知一棵二叉树的先序遍历序列为EFHIGJK,中序遍历序列为HFIEJGK,则该二叉树根的右子树的根是( C )。
A.E B. F C. G D. J11、由4个结点构造出的不同的二叉树个数共有( C )。
A.8 B. 10 C.12 D.1412、在完全二叉树中,如果一个结点是叶子结点,则它没有(D )。
A.左孩子结点B. 右孩子结点C.左、右孩子结点D.左、右孩子结点和兄弟结点13、深度为6的二叉树最多有( B )个结点。
A.64 B.63 C.32 D.3114、二叉树使用二叉链表存储,若p指针指向二叉树的一个结点,当p->lchild=NULL时,则( A )。
A.p结点左儿子为空B.p结点有右儿子C.p结点右儿子为空D.p结点有左儿子15、在具有n个结点的完全二叉树中,若结点i有左孩子,则结点i的左孩子编号为( A )。
森林培育学习题及答案(最新整理)

绪论一、概念1、森林培育学;2、森林培育二、简述题1、森林培育学的内容2.、森林培育的对象3、森林的三大效益三、论述题1. 论述未来森林培育工作发展的趋势。
答案:一、1、森林培育是通过树木和森林利用太阳能和其它物质进行生物转化,生产人类所需的食物、工业原料、生物能源等的一种生产过程,同时创造并保护人类和生物生存所需环境的生产过程。
2、森林培育学(Silviculture)是研究营造和培育森林的理论和技术的科学。
二、1、良种生产,苗木培育,森林营造,森林抚育,森林主伐更新2、人工林和天然林3、经济效益:既森林生产木材和林副产品所产生的效益生态效益:主要是指森林在改善环境方面所带来的效益社会效益:森林对社会产生的效益。
包括提供就业机会,对人类精神产生的效益三、随着可持续发展战略在全球的普及,林业也必须走可持续发展的道路。
1)注重森林的可持续经营以木材生产和森林多功能发挥相协调的森林经营模式将普遍的被探讨,为此,森林培育将更注重森林的多目标(木材和非林产品的生产、生物多样性和野生动物保护、生态系统可持续能力、保持水土的能力、森林服务功能、更新的影响等)。
一些可持续经营模式将被提出,如森林的生态系统经营模式、新林业模式、接近自然的林业、生态林业等。
另外在具体经营技术上一些对环境产生负面影响的技术也将逐渐的被替代,如化肥、农药、除草剂等。
2)森林培育将更加集约化随着经济的发展木材等林产品的需求将不断增加,森林培育技术也将向集约化方向发展。
目前在发达的国家短轮伐期工业人工林培育技术已经基本建立,如桉树(轮伐期3-10年,年生长量可达60-90m3/hm2)等。
新的培育体系主要注重遗传控制(无性系造林)、立地控制、密度控制、地力维持等技术,在短期内可收获大量木材。
我国目前也在进行该方面的研究。
3)森林培育出现定向化趋势为了发挥森林的更大效益,在森林培育上将实行分类经营和定向培育。
对一个区域来讲,森林被划分成商品林和公益林。
人教版七年级生物上册第六章 爱护植被,绿化祖国 试题及答案.doc

第六章爱护植被,绿化祖国01知识管理1.我国主要的植被类型草原:组成草原的植物大多是适应________气候条件的________植物。
荒漠:荒漠的生态条件极为________,夏季________,土壤________。
荒漠的植被稀疏,植物种类________,这里生长的植物十分________。
热带雨林:热带雨林分布在全年________的地区,植物种类特别________,终年________,大部分植物都很高大。
常绿阔叶林:常绿阔叶林分布在气候比较________、________的地区,这里的植物以________树为主。
落叶阔叶林:落叶阔叶林分布区________,夏季________,冬季________,这里的植物主要是冬季________________树。
针叶林:针叶林分布在________、________的地区,这里的植物以________、________等________树为主。
2.我国植被面临的主要问题问题:(1)我国的植被中,森林占据了主体,但我国人均森林面积________,对森林资源的利用不合理,伐优留劣,甚至________,使森林生态系统呈现________的趋势。
(2)过度放牧使草原________、________。
3.从我做起,保护植被法律措施:我国于1984年和1985年分别颁布了《中华人民共和国森林法》《中华人民共和国草原法》,对森林和草原实行____________和____________管理,力求使我国对植物资源的利用和保护尽快走上________________的道路。
植树节:每年的____________是全国的“植树节”。
02例题解读【例1】下列关于我国植被特点的叙述,不正确的是( )A.草原沙漠化严重B.我国植被中,草原占据了主体C.人均森林面积少D.森林生态系统呈现衰退的趋势解析:我国主要的植被类型有草原、荒漠、热带雨林、常绿阔叶林、落叶阔叶林、针叶林。
图论 第6章 树和割集

1.3 极小连通图
定义2 若连通图G中去掉任一条边后得到一个不连通图,则称G 为极小连通图。 推论4 图G是树当且仅当G是极小连通图。
1.4 树的中心
定义3 设G=(V,E)是连通图,v∈V,数 e(v)=max{d(v,u)} 称为v在G中的偏心率。 数 r(G)=min{e(v)} 称为G的半径。 满足r(G)=e(v)的顶点v称为G的中心点。G的所有中心点组成 的集合称为G的中心,G的中心记为C(G)。 定理2 每棵树的中心或含有一个顶点,或含有两个邻接的顶点。
(2)∑deg v=2q
(3)根据具体的题设条件进行特殊的不等式的放缩[解题关键] 例3 设G是一棵树且Δ(G)≥k,证明:G中至少有k个1度顶点。
1.7 生成树(包含所有顶点的树)
定义1 设G=(V,E)是一个图,若G的一个生成子图 T=(V,F)是树,则称T是G的生成树。 定义2 设G=(V,E)是一个图,若G的一个生成子图 T=(V,F)是一个森林,则称T是G的生成森林。
1.8 生成树存在问题
定理1 图G有生成树的充分必要条件是G为一个连通图。
3极小连通图定义2若连通图g中去掉任一条边后得到一个不连通图则称g为极小连通图
第六章
树和割集
内容
树及其性质、生成树、割集
第一节
1.1 树和森林向树,简称树, 记为T。 定义2 无圈的无向图称为无向森林,简称森林。
1.2 树的特征性质
定理1 设G=(V,E)是一个(p,q)图,则下列命题等价: (1) G是树; (2) G的任两个不同顶点间有唯一的一条路联结; (3) G连通且 p=q+1; (4) G无圈且 p=q+1; (5) G无圈且任加一条边得到有唯一圈; (6) G连通且任去掉一条边得不连通图。
七年级-人教版-生物-上册-[综合训练]第六章-爱护植被,绿化祖国
![七年级-人教版-生物-上册-[综合训练]第六章-爱护植被,绿化祖国](https://img.taocdn.com/s3/m/b78e33aa0408763231126edb6f1aff00bed570cc.png)
第六章爱护植被,绿化祖国1.我国某地区终年高温多雨,叶片终年常绿,大部分植物都很高大,则该地区的植被类型为()A.常绿阔叶林B.落叶阔叶林C.针叶林D.热带雨林2.分析以下材料,并回答问题:材料一在联合国生物多样性峰会上,习近平主席强调,中国将秉持人类命运共同体理念,愿承担与中国发展水平相称的国际责任,提高国家自主贡献力度,采取更加有力的政策和措施,二氧化碳排放力争于2030年前达到峰值,努力争取2060年前实现碳中和,为实现应对气候变化《巴黎协定》确定的目标作出更大努力和贡献。
材料二我国保护生物多样性的法律法规体系越来越完善,如2021年4月施行的《生物安全法》。
材料三2021年3月,我国遭受了近十年来的最强沙尘暴,此次沙尘暴起源于蒙古国戈壁荒漠。
蒙古国因过度放牧而导致土壤荒漠化,再加上当年春气温较往年偏高5~8 ℃,导致干旱、大风,就此形成了超强沙尘暴。
(1)中国要在2060年前实现碳中和,一方面应保护现有森林,并大力植树造林,利用绿色植物进行_______________消耗二氧化碳;另一方面需从能源结构、工业制造和个人生活等方面减少二氧化碳的排放。
(2)《生物安全法》中规定加强对抗生素药物等抗微生物药物使用和残留的管理,因为这些药物残留可能会沿_______________逐渐积累危及人体健康,也可能污染环境。
(3)“呵护自然,人人有责。
”下列个人行为不利于保护大自然的是_______。
A.随手关灯B.光盘行动C.大量使用一次性餐具D.垃圾分类投放(4)我国可以采取哪些措施减少沙尘暴的发生?_____________________。
参考答案1.【答案】D【解析】常绿阔叶林分布在气候比较炎热、湿润的地区,这里的植物以常绿阔叶树为主;落叶阔叶林分布区四季分明,夏季炎热多雨,冬季寒冷,这里的植物主要是冬季完全落叶的阔叶树;针叶林分布在夏季温凉、冬季严寒的地区,这里的植物以松、杉等针叶树为主;热带雨林分布在全年高温多雨的地区,植物种类特别丰富,终年常绿,大部分植株都很高大,D项符合题意。
第6章 树和二叉树-习题-答案
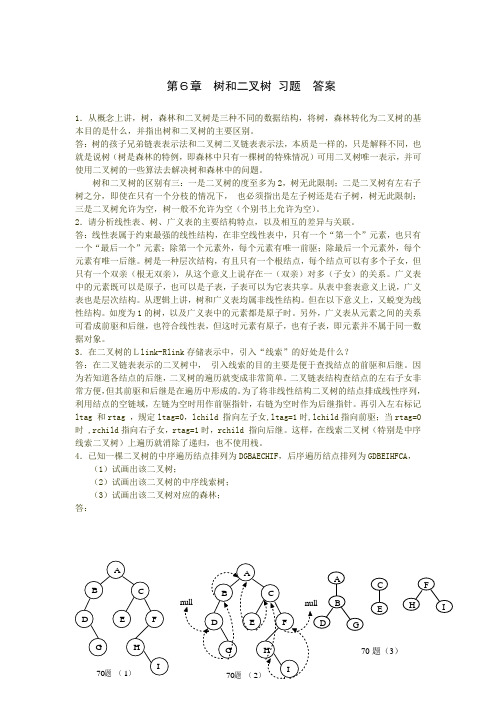
第6章树和二叉树习题答案1.从概念上讲,树,森林和二叉树是三种不同的数据结构,将树,森林转化为二叉树的基本目的是什么,并指出树和二叉树的主要区别。
答:树的孩子兄弟链表表示法和二叉树二叉链表表示法,本质是一样的,只是解释不同,也就是说树(树是森林的特例,即森林中只有一棵树的特殊情况)可用二叉树唯一表示,并可使用二叉树的一些算法去解决树和森林中的问题。
树和二叉树的区别有三:一是二叉树的度至多为2,树无此限制;二是二叉树有左右子树之分,即使在只有一个分枝的情况下,也必须指出是左子树还是右子树,树无此限制;三是二叉树允许为空,树一般不允许为空(个别书上允许为空)。
2.请分析线性表、树、广义表的主要结构特点,以及相互的差异与关联。
答:线性表属于约束最强的线性结构,在非空线性表中,只有一个“第一个”元素,也只有一个“最后一个”元素;除第一个元素外,每个元素有唯一前驱;除最后一个元素外,每个元素有唯一后继。
树是一种层次结构,有且只有一个根结点,每个结点可以有多个子女,但只有一个双亲(根无双亲),从这个意义上说存在一(双亲)对多(子女)的关系。
广义表中的元素既可以是原子,也可以是子表,子表可以为它表共享。
从表中套表意义上说,广义表也是层次结构。
从逻辑上讲,树和广义表均属非线性结构。
但在以下意义上,又蜕变为线性结构。
如度为1的树,以及广义表中的元素都是原子时。
另外,广义表从元素之间的关系可看成前驱和后继,也符合线性表,但这时元素有原子,也有子表,即元素并不属于同一数据对象。
3.在二叉树的Llink-Rlink存储表示中,引入“线索”的好处是什么?答:在二叉链表表示的二叉树中,引入线索的目的主要是便于查找结点的前驱和后继。
因为若知道各结点的后继,二叉树的遍历就变成非常简单。
二叉链表结构查结点的左右子女非常方便,但其前驱和后继是在遍历中形成的。
为了将非线性结构二叉树的结点排成线性序列,利用结点的空链域,左链为空时用作前驱指针,右链为空时作为后继指针。
兄弟树阅读答案

兄弟树阅读答案【篇一:第六章树习题答案】t>一、选择题1、已知一算术表达式的中缀形式为 a+b*c-d/e,后缀形式为abc*+de/-,其前缀形式为(d )a.-a+b*c/de b. -a+b*cd/ec.-+*abc/de d. -+a*bc/de2、算术表达式a+b*(c+d/e)转为后缀表达式后为( b )a.ab+cde/* b.abcde/+*+c.abcde/*++ d.abcde*/++3.设树t的度为4,其中度为1,2,3和4的结点个数分别为4,2,1,1 则t中的叶子数为( d)a.5b.6 c.7 d.84.在下述结论中,正确的是( d)①只有一个结点的二叉树的度为0; ②二叉树的度为2;③二叉树的左右子树可任意交换;④深度为k的完全二叉树的结点个数小于或等于深度相同的满二叉树。
a.①②③ b.②③④c.②④ d.①④5.设森林f对应的二叉树为b,它有m个结点,b的根为p,p的右子树结点个数为n,森林f中第一棵树的结点个数是( a )a.m-nb.m-n-1 c.n+1d.条件不足,无法确定6.若一棵二叉树具有10个度为2的结点,5个度为1的结点,则度为0的结点个数是( b )a.9b.11c.15 d.不确定7.在一棵三元树中度为3的结点数为2个,度为2的结点数为1个,度为1的结点数为2个,则度为0的结点数为( c )个a.4 b.5 c.6d.78.设森林f中有三棵树,第一,第二,第三棵树的结点个数分别为m1,m2和m3。
与森林f对应的二叉树根结点的右子树上的结点个数是( d )。
【北方交通大学 2001 一、16 (2分)】a.m1 b.m1+m2 c.m3 d.m2+m39.具有10个叶结点的二叉树中有( b)个度为2的结点,a.8 b.9 c.10 d.ll10.一棵完全二叉树上有1001个结点,其中叶子结点的个数是(e ) a. 250b. 500 c.254 d.505e.以上答案都不对11.设给定权值总数有n 个,其哈夫曼树的结点总数为( d)a.不确定 b.2nc.2n+1d.2n-112.有关二叉树下列说法正确的是( b)a.二叉树的度为2 b.一棵二叉树的度可以小于2c.二叉树中至少有一个结点的度为2d.二叉树中任何一个结点的度都为213.二叉树的第i层上最多含有结点数为( c )a.2i b. 2i-1-1 c. 2i-1d.2i -114.一个具有1025个结点的二叉树的高h为( c )a.11 b.10 c.11至1025之间d.10至1024之间15.一棵二叉树高度为h,所有结点的度或为0,或为2,则这棵二叉树最少有( b )结点a.2h b.2h -1 c.2h+1d.h+118.对于有n 个结点的二叉树, 其高度为(d )a.nlog2nb.log2n c.?log2n?|+1 d.不确定19. 一棵具有 n个结点的完全二叉树的树高度(深度)是( a)a.?logn?+1 b.logn+1 c.?logn?d.logn-120.深度为h的满m叉树的第k层有( a)个结点。
数据结构第6章 树习题+答案
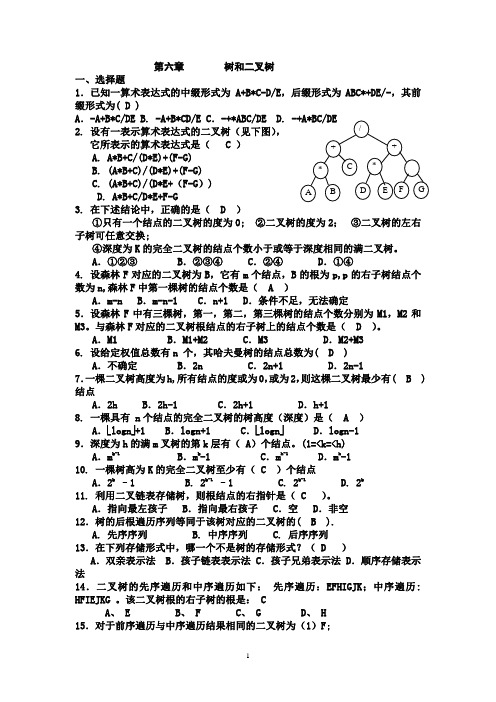
第六章 树和二叉树一、选择题1.已知一算术表达式的中缀形式为 A+B*C-D/E ,后缀形式为ABC*+DE/-,其前缀形式为( D )A .-A+B*C/DE B. -A+B*CD/E C .2. 设有一表示算术表达式的二叉树(见下图), 它所表示的算术表达式是( C ) A. A*B+C/(D*E)+(F-G) B. (A*B+C)/(D*E)+(F-G) C. (A*B+C)/(D*E+(F-G )) D. A*B+C/D*E+F-G 3. 在下述结论中,正确的是( D )①只有一个结点的二叉树的度为0; ②二叉树的度为2; ③二叉树的左右子树可任意交换;④深度为K 的完全二叉树的结点个数小于或等于深度相同的满二叉树。
A .①②③B .②③④C .②④D .①④4. 设森林F 对应的二叉树为B ,它有m 个结点,B 的根为p,p 的右子树结点个数为n,森林F 中第一棵树的结点个数是( A )A .m-nB .m-n-1C .n+1D .条件不足,无法确定5.设森林F 中有三棵树,第一,第二,第三棵树的结点个数分别为M1,M2和M3。
与森林F 对应的二叉树根结点的右子树上的结点个数是( D )。
A .M1B .M1+M2C .M3D .M2+M36. 设给定权值总数有n 个,其哈夫曼树的结点总数为( D )A .不确定B .2nC .2n+1D .2n-17.一棵二叉树高度为h,所有结点的度或为0,或为2,则这棵二叉树最少有( B )结点A .2hB .2h-1C .2h+1D .h+18. 一棵具有 n 个结点的完全二叉树的树高度(深度)是( A )A .⎣logn ⎦+1B .logn+1C .⎣logn ⎦D .logn-19.深度为h 的满m 叉树的第k 层有( A )个结点。
(1=<k=<h)A .m k-1B .m k -1C .m h-1D .m h -110. 一棵树高为K 的完全二叉树至少有( C )个结点A .2k –1 B. 2k-1 –1 C. 2k-1 D. 2k11. 利用二叉链表存储树,则根结点的右指针是( C )。
最新数据结构习题19325教学内容
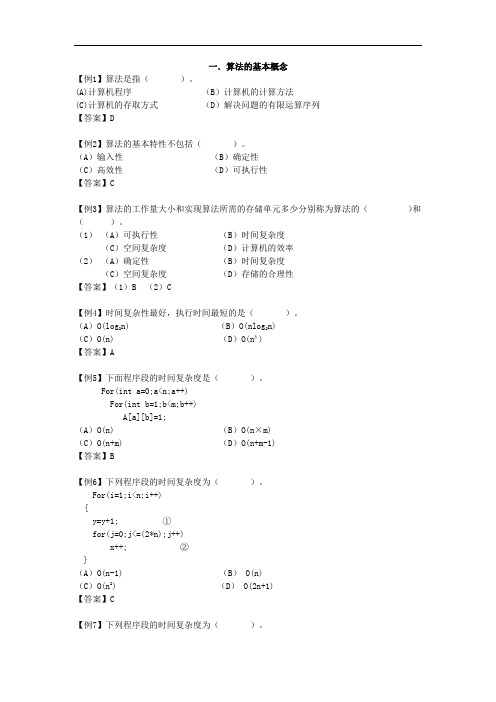
一.算法的基本概念【例1】算法是指()。
(A)计算机程序(B)计算机的计算方法(C)计算机的存取方式(D)解决问题的有限运算序列【答案】D【例2】算法的基本特性不包括()。
(A)输入性(B)确定性(C)高效性(D)可执行性【答案】C【例3】算法的工作量大小和实现算法所需的存储单元多少分别称为算法的()和()。
(1)(A)可执行性(B)时间复杂度(C)空间复杂度(D)计算机的效率(2)(A)确定性(B)时间复杂度(C)空间复杂度(D)存储的合理性【答案】(1)B (2)C【例4】时间复杂性最好,执行时间最短的是()。
(A)O(log2n) (B)O(nlog2n)(C)O(n) (D)O(n³)【答案】A【例5】下面程序段的时间复杂度是()。
For(int a=0;a<n;a++)For(int b=1;b<m;b++)A[a][b]=1;(A)O(n) (B)O(n×m)(C)O(n+m) (D)O(n+m-1)【答案】B【例6】下列程序段的时间复杂度为()。
For(i=1;i<n;i++){y=y+1; ①for(j=0;j<=(2*n);j++)x++; ②}(A)O(n-1) (B) O(n)(C)O(n2) (D) O(2n+1)【答案】C【例7】下列程序段的时间复杂度为()。
i=1;while(i<=n)i=i*2;(A)O(1) (B) O(n)(C)O(log2n) (D) O(2n)【答案】C【例8】算法的执行遵循“输入―计算―________”的模式,算法的________体现算法的功能。
【答案】输出输出【例9】式子a*(3a2+6a-3)的时间复杂度是________。
【答案】O(a3)【例10】当有非法数据输入时,算法能做出适当的处理,体现了算法的________。
【答案】健壮性【考点解析】一个算法必须能够处理非法数据的输入,且不能产生不可预测的结果,这是算法的健壮性所必须的,处理非法数据的能力体现了算法的健壮性。
树与森林专题培训
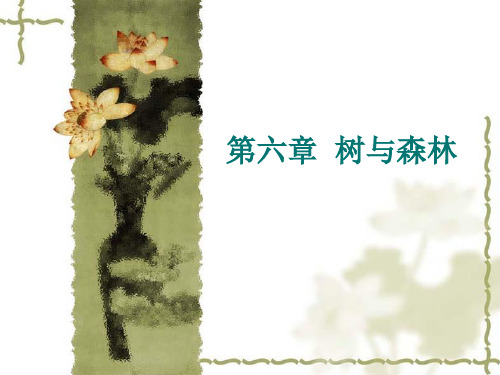
08
{
09
k = Qs[Qs_front] ;
/* 取队首元素赋值给k */
10
Qs_front++ ;
/* 队首元素出队 */
11
printf ("%c",tr[k].Data); /* 访问该结点 */
12
for (i=1; i<=m; i++)
/* 让该结点旳孩子结点进队列 */
13
if (tr[k].Child[i] != NULL)
5
E
E
—
F,G,H,I,J
6
F
F
K
G,H,I,J,K
7
G
G
—
H,I,J,K
8
H
H
—
I,J,K
9
I
I
—
J,K
10
J
J
—
K
11
K
K
—
空
18
2、层次遍历算法2
算法6-1 树旳层次遍历算法
已知一棵树T旳度为m,采用基于顺序表旳子结点表达法存储。对T进行层次 遍历以取得层次遍历序列。
00 Level_Tr(treenode tr[], int m,int root)源自Data LCHRS
数据域 第一个孩 下一个兄 子下标 弟下标
(a)
14
6.2.3 左子/右弟兄结点表达法存储2
能够分别按照顺序或链表方式进行进行存储。
下标 Data Lch
RS
0
A
1
-1
A
1
B
4
2
2
C
-1
3
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
习题6.6上图习题6.8:void Get_PreOrder(BiTree T,int k,TElemType &e){ //求先序序列中第k个位置上结点的值if(T){ c++; //每访问一个子树的根都会使前序序号计数器加1,c设为全局量if(c==k){ e=T->data; return;}else{ Get_PreOrder(T->lchild, k, e ); //在左子树中查找Get_PreOrder(T->rchild, k ,e); //在右子树中查找}}//if} //Get_PreOrder习题6.9:int LeafCount(BiTree T)//求二叉树中叶子结点的数目{if(!T) return 0; //空树没有叶子else if(!T->lchild&&!T->rchild)return 1; //叶子结点else return Leaf_Count(T->lchild)+Leaf_Count(T->rchild);//左子树的叶子数加上右子树的叶子数} //LeafCount习题6.10: //交换所有结点的左右子树void Change_BiTree (BiTree T){ BiTree tempif(T){ temp=T->lchild;T->lchild=T->rchild;T->rchild=temp} //交换左右子树if(T->lchild) Change_BiTree(T->lchild);if(T->rchild) Change_BiTree (T->rchild);//左右子树再分别交换各自的左右子树}//Change_BiTree习题6.11: //求二叉树中以值为x的结点为根的子树深度int Get_Sub_Depth(BiTree T,int x, int &depth){ if(T->data==x){ depth=Get_Depth(T); return;}//找到了值为x的结点,求其深度 else{ if(T->lchild) Get_Sub_Depth(T->lchild,x,depth);if(T->rchild) Get_Sub_Depth(T->rchild,x,depth);} //在左右子树中继续寻找} //Get_Sub_Depthint Get_Depth(BiTree T) //求子树深度的递归算法{ if(!T) return 0;else{ m=Get_Depth(T->lchild);n=Get_Depth(T->rchild);return (m>n?m:n)+1;}}//get depth习题6.12: // 删除所有以元素x为根的子树void Del_Sub_x(BiTree T,int x){ if(T->data==x) Del_Sub(T); //删除该子树else{ if(T->lchild) Del_Sub_x(T->lchild,x);if(T->rchild) Del_Sub_x(T->rchild,x);} //else 在左右子树中继续查找} //Del_Sub_xvoid Del_Sub(BiTree T) //删除子树T{ if(T->lchild) Del_Sub(T->lchild);if(T->rchild) Del_Sub(T->rchild);free(T);} //Del_Sub习题6.13: // 根据顺序存储结构建立二叉链表Status CreateBiTree_SqList(BiTree &T, SqList sa){ BiTree ptr[st+1]; //该数组储存与sa中各结点对应的树指针 if(!st){ T=NULL; return; } //空树ptr[1]=new BTNode;ptr[1]->data=sa.elem[1]; //建立树根T=ptr[1];T->lchild=NULL; T->rchild=NULL;for(i=2; i<=st; i++){ ptr[i]=new BTNode;ptr[i]->data=sa.elem[i];j=i/2; //找到结点i的双亲jif(i-j*2) ptr[j]->rchild=ptr[i]; //i是j的右孩子else ptr[j]->lchild=ptr[i]; //i是j的左孩子}return OK;} //CreateBitree_SqList习题6.19: //求一棵以孩子兄弟链表表示的树的度int GetDegree_CSTree(CSTree T)//求孩子兄弟链表表示的树T的度{ if(!T->firstchild) return 0; //空树else{ degree=0;for( p=T->firstchild; p; p=p->nextsibling)degree++; //本结点的度for( p=T->firstchild; p; p=p->nextsibling){ d=GetDegree_CSTree(p);if(d>degree) degree=d; //孩子结点的度的最大值}return degree;}//else} //GetDegree_CSTree习题6.20: //求孩子兄弟链表表示的树T的叶子数目int LeafCount_CSTree(CSTree T ){ if(!T) return 0;else if(!T->firstchild) return 1; //叶子结点else{ count=0;for(p=T->firstchild;p;p=child->nextsibling)count+=LeafCount_CSTree(p);return count; //各子树的叶子数之和}} //LeafCount_CSTree习题6.20: //求孩子兄弟链表表示的树T的叶子数目int LeafCount(CSTree T ){ CSTree p=T;int k=0;if(p!=NULL){ if(p->firstchild==NULL)k=1+LeafCount(p->firstchild)+LeafCount (p->nextsibling);elsek=0+LeafCount(p->firstchild)+LeafCount(p->nextsibling);}else k=0;return k;} //LeafCount习题6.23: //按树状打印输出二叉树的元素,i表示结点所在层次,初次调用时i=0 void Print_BiTree(BiTree T,int i){ if(T->rchild) Print_BiTree(T->rchild , i+1);for(j=1;j<=i; j++ )printf (“ ”); //打印i个空格以表示出层次cout<<T->data<<endl; //打印T元素,换行if(T->lchild) Print_BiTree(T->rchild, i+1 );} //Print_BiTree分析:该递归算法实际上是带层次信息的中序遍历,只不过按照题目要求,顺序为先右后左.补充习题:1.树最适合用来表示()。
A)有序数据元素B)无序数据元素C)元素之间具有分支层次关系的数据D)元素之间无联系的数据2.设深度为h的二叉树上只有度为0和度为2的结点,则此类二叉树中所包含的结点数至多为( )。
A)2h-1 B)2(h-1) C)2*h-1 D)2*h3.在一棵二叉树中,第5层上的结点数最多有( )。
A)10 B)15 C)16 D)324.下图所示的二叉树中,( )不是完全二叉树。
5.有100个结点的完全二叉树,叶子结点的个数为:( )。
A)49 B)50 C)51 D)526.已知某二叉树的叶子结点数为20 ,10个结点有一个左孩子,15个结点有一个右孩子,求该二叉树的总结点数?解:该二叉树叶子结点数:n0=20,度为1的结点数:n1=10+15,根据二叉树的性质3,n0=n2+1,n2=n0-1=19, 则:n=n0+n1+n2=64.7.具有100个结点的二叉树中,若用二叉链表存储,其指针域部分用来指向结点的左、右孩子,其中()个指针域为空。
A)50 B)99 C)100 D)1018.首先访问结点的左子树,然后访问该结点,最后访问结点的右子树,这种遍历称为()。
A)前序遍历B)后序遍历C)中序遍历D)层次遍历9.任何一棵二叉树的叶结点在先序、中序和后序遍历的序列中的相对次序()。
A)不发生变化B)发生变化C)不能确定D)以上都不对10.某非空二叉树的前序序列和后序序列正好相反,则二叉树一定是()的二叉树。
A)空或只有一个结点B)高度等于其结点数C)任一结点无左孩子D)任一结点无右孩子11.如果某二叉树的先序遍历序列是abdcef,中序遍历序列是dbaefc,则其后序遍历序列是()。
A)dbafec B)fecdba C)efcdba D)dbfeca12.按照二叉树的定义,具有3个结点的二叉树形态有( )种。
A)3 B)4 C)5 D)613.二叉树的后序遍历序列中,任意一个结点均处在其孩子结点的前面()。
A)正确B)错误14.n个结点深度为h的二叉树的线索化所需的时间复杂度是( )。
A)O(1) B)O(hn) C)O(n) D)O(nlog2h)15.设a,b为一棵二叉树上的两个结点,在中序遍历时,a在b前的条件是( )。
A)a是b祖先B)a是b子孙C)a在b左方D)a在b右方16.关于二叉树的三种遍历,下列说法正确的是( )。
A)任意两种遍历序列都不可以唯一决定该二叉树B)任意两种遍历序列都可以唯一决定该二叉树C)先序遍历序列和后序遍历序列可以唯一决定该二叉树D)先序遍历序列和中序遍历序列可以唯一决定该二叉树17.在某棵二叉树的一种序列中,如果发现其中每一结点的左孩子均是其前趋,则可判断定这种序列为中序序列( )。
A)正确B)不正确18.已知某二叉树的后序遍历序列是dabec,中序遍历序列是debac,它的前序遍历序列是()。
A)acbed B)decab C)deabc D)cedba19.前序遍历和中序遍历结果相同的二叉树为()。