Lecture02-LineDrawing 计算机图形学ppt课件
02-计算机图形学基础(第二版)PPT课件

透镜组
光 孔
触钮开关
导线
笔体 光导纤维
图2.3 光笔的结构
2021/7/22
7
图形输入设备
触摸屏(touch screen) 当用手指或者小杆触摸屏幕时,触点位置
便以光学的(红外线式触摸屏)、电子的(电 阻式触摸屏和电容式触摸屏)或声音的(声音 探测式)方式记录下来。
2021/7/22
8
图形输入设备
随机扫描(random-scan)的图形显示器中电 子束的定位和偏转具有随机性,即电子束的扫 描轨迹随显示内容而变化,只在需要的地方扫 描,而不必全屏扫描。
2021/7/22
31
随机扫描的图形显示器
2
Y
2
3
1
1
3
t
1
X
2
3
图2.16 随机扫描图形显示器的工作原理
2021/7/22
32
随机扫描的图形显示器
2021/7/22
52
2.4 显示子系统
光栅扫描图形显示子系统的结构 绘制流水线 相关概念
2021/7/22
53
光栅扫描图形显示子系统的结构
CPU
系统 主存
显示 控的光栅图形显示子系统
2021/7/22
54
光栅扫描图形显示子系统的结构
CPU
系统 主存
帧缓存
2021/7/22
41
液晶显示器——原理
液晶分子的排列在微弱的外部电场、磁场或者 应力、温度变化等作用下非常容易改变。当液 晶分子的某种排列状态在电场作用下变为另一 种状态时,液晶的光学性质随之改变,这种产 生光被电场调制的现象称为液晶的电光效应。
2021/7/22
计算机图形学ppt(共49张PPT)
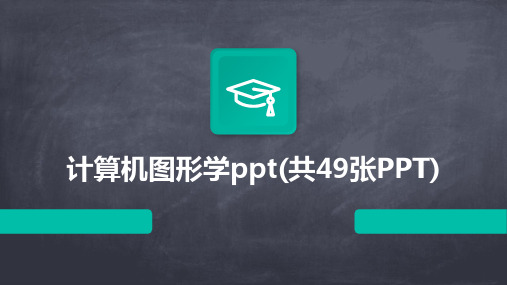
过程动画技术
过程动画的概念
通过定义物体的运动规律或过程,由计算机自动生成动画。
过程动画的实现方法
基于物理模拟、基于过程建模、基于行为建模等。
过程动画的应用场景
自然现象的模拟(如风、雨、雪)、物体的变形和破碎效果等。
基于物理的动画技术
基于物理的动画概念
利用物理引擎模拟现实世界中的物理现象,生成逼真的动画效果 。
表面模型(Surface Model)
用多边形面片逼近三维物体的表面。
实体模型(Solid Model)
定义三维物体的内部和外部,表示物体的实体。
光线追踪(Ray Tracing)
模拟光线在三维场景中的传播,生成真实感图形。
三维图形的变换与裁剪
几何变换(Geometric Trans…
包括平移、旋转、缩放等变换,用于改变三维物体的位置和形状。
如中点画圆算法,利用圆 的八对称性,通过计算决 策参数来生成圆。
多边形的生成算法
如扫描线填充算法,通过 扫描多边形并计算交点来 生成多边形。
二维图形的变换与裁剪
二维图形的变换
包括平移(Translation)、旋转(Rotation)、 缩放(Scaling)等变换,可以通过变换矩阵来实 现。
二维图形的裁剪
Screen-Space Methods
利用屏幕空间信息进行半透明 物体的渲染,如屏幕空间环境 光遮蔽(SSAO)和屏幕空间 反射(SSR)。
06
计算机动画技术
Chapter
计算机动画概述
计算机动画的定义
01
通过计算机生成连续的动态图像,实现虚拟场景和角色的动态
表现。
计算机动画的应用领域
02
影视特效、游戏设计、虚拟现实、工业设计等。
《计算机图形学》课件
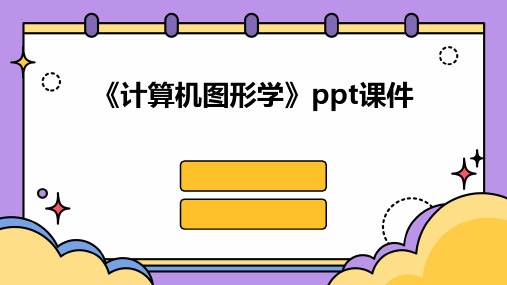
光照模型与阴影生成算法的应用广泛,例如在游戏开发、虚拟现实和 电影制作等领域。
纹理映射算法
纹理映射算法用于将图像或纹理贴图映射到三维物体 的表面。
输标02入题
常用的纹理映射算法包括纹理坐标、纹理过滤和纹理 压缩等。
01
03
纹理映射算法的应用广泛,例如在游戏开发、虚拟现 实和数字艺术等领域。
04
工业设计
使用CAD等技术进行产品设计和原型制作 。
游戏开发
创建丰富的游戏场景和角色,提供沉浸式 的游戏体验。
科学可视化
将复杂数据以图形方式呈现,帮助人们理 解和分析数据。
虚拟现实与增强现实
构建虚拟环境,实现人机交互,增强现实 感知。
02
计算机图形学基础知识
图像与图形的关系
图像
由像素组成的二维或三维数据,通常 用于表示真实世界或模拟的视觉信息 。
全息投影技术
总结词
全息投影技术能够实现三维立体显示,为观众提供沉浸式的 观影体验。
详细描述
全息投影技术利用干涉和衍射原理,将三维物体以全息图像 的形式呈现出来,使观众能够从不同角度观察到物体的立体 形态。这种技术将为电影、游戏和其他娱乐领域带来革命性 的变化。
增强现实技术
总结词
增强现实技术能够将虚拟信息与现实世界相结合,提供更加丰富的交互体验。
HSL和HSV模型
基于色调、饱和度和亮度(或 明度)来描述颜色。
RGBA模型
在RGB基础上增加透明度通道 。
图像处理技术
滤波和锐化
通过改变图像的像素值 来减少噪声、突出边缘
或细节。
色彩调整
改变图像中颜色的分布 和强度,以达到特定的
视觉效果。
图像分割
计算机图形学_完整版 ppt课件

输入设备
键盘、鼠标 按钮盒、旋钮 跟踪球、空间球 操作杆 触觉反馈设备 数据手套、数据衣 数字化仪 扫描仪 触摸板 光笔 ……
硬拷贝设备
打印机 喷墨 激光 ……
绘图仪 台式 大型滚动传送式 ……
图形硬件系统组成模块示意图:
或称图形坐标系、用户坐标系、全局坐标系 如在世界坐标系中进行装配
观察坐标系(viewing coordinate)
对场景进行观察所对应的坐标系 对象经变换到该场景的一个二维投影——投影变换
规范化坐标系(normalized coordinate)
可使图形软件与特定输出设备的坐标范围无关 坐标范围:-1~1,或0 ~ 1 等等
在场景中对物体移动、旋转、缩 放、扭曲等,或转换模型坐标系
3D→2D,并对观察区域进行裁 剪和缩放
一种伪变换,对窗口上的最终输 出进行移动、缩放等
三维几何变换
可用4×4矩阵操作统一表示二维和三维几何变换
缩放、旋转、 对称、错切等
平移
投影
整体缩放
基本变换:平移、旋转、缩放
复合变换:可由平移、旋转、缩放和其他变换的矩阵乘积 (合并)形成。
图元的绘制、显示过程
顶点 法向量、颜色、纹理… 像素
图元操作、像素操作 光栅化(扫描转换)
像素信息 帧缓存 显示器
调用底层函数,如 setPixel (x,y);将当 前像素颜色设定值存 入帧缓存的整数坐标 位置(x,y)处。
图元描述与操作
几何图元由一组顶点(Vertex)描述 这一组顶点可以是一个或是多个。每个顶点信息二维或 三维,使用 2~4 个坐标。顶点信息由位置坐标、颜色 值、法向量、纹理坐标等组成。
计算机图形学课件
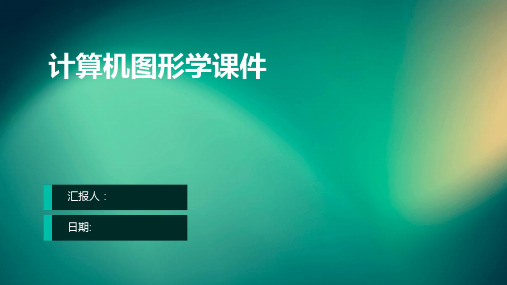
游戏开发中,计算机图形学还涉及实时渲染技术 ,包括OpenGL、DirectX等图形API的使用,以 及GPU加速等技术的应用。
交互体验优化
通过计算机图形学技术,游戏开发者可以优化游 戏的交互体验,例如通过动画、音效等增强游戏 的沉浸感。
影视制作中的计算机图形学应用
01
特效制作
计算机图形学广泛应用于电影、电视剧等影视作品的特效制作中。
1990年代
个人电脑的出现使得计算机图形学进入普 及阶段,各种图形软件和游戏开始广泛使 用。
互联网的出现使得计算机图形学进入新的 发展阶段,网络图像传输和浏览技术得到 了广泛应用。
计算机图形学的应用领域
娱乐产业
电影、电视、游戏等娱乐产业是计算 机图形学的最大应用领域,需要大量 的特效和动画制作。
科学可视化
计算机图形学的发展也将进一步推动艺术 和科技的结合,为艺术家提供更多的创作 工具和展示方式,同时也为科技爱好者提 供更多的探索和创新的空间。
THANKS FOR WATCHING
感谢您的观看
计算机图形学用于生成和操作科学数 据,如气象预报、医学影像、物理模 拟等。
工业设计
计算机图形学可用于产品外观设计、 机械设计等领域,提高设计效率和准 确性。
虚拟现实
计算机图形学生成虚拟环境,提供沉 浸式的体验,广泛应用于教育、培训 、娱乐等领域。
02 计算机图形学基础知识
图像的数字化
图像采样
将连续的图像转换为离散的像素集合
网格建模
使用多边形网格来表示物体的形状,这些多边形可以是由三角形组 成的简单形状,也可以是更复杂的形状。
NURBS建模
使用非均匀有理B样条曲线来表示物体的形状,这些曲线可以创建更 复杂的形状,如自由曲线和曲面。
计算机图形学完整ppt课件

工业设计
利用计算机图形学进行产品设计、仿 真和可视化,提高设计效率和质量。
建筑设计
建筑师使用计算机图形学技术创建三 维模型,进行建筑设计和规划。
计算机图形学的相关学科
计算机科学
计算机图形学是计算机科学的一个重 要分支,涉及计算机算法、数据结构、 操作系统等方面的知识。
物理学
计算机图形学中的很多技术都借鉴了 物理学的原理,如光学、力学等,用 于实现逼真的渲染效果和物理模拟。
02
03
显示器
LCD、LED、OLED等,用 于呈现图形图像。
投影仪
将计算机生成的图像投影 到大屏幕上,用于会议、 教学等场合。
虚拟现实设备
如VR头盔,提供沉浸式的 3D图形体验。
图形输入设备
键盘和鼠标
最基本的图形输入设备,用于操 作图形界面和输入命令。
触摸屏
通过触摸操作输入图形指令,常 见于智能手机和平板电脑。
多边形裁剪算法
文字裁剪算法
判断一个多边形是否与另一个多边形相交, 如果相交则求出交集部分并保留。
针对文字的特殊性质,采用特殊的裁剪算法 进行处理,以保证文字的完整性和可读性。
05
光照模型与表面绘制
光照模型概述
光照模型是计算机图形学中用于模拟光线与物体表面交互的数学模型。
光照模型能够模拟光线在物体表面的反射、折射、阴影等效果,从而增强图形的真 实感。
二维纹理映射原理
根据物体表面的顶点坐标和纹理坐标,计算出每个像素点对应的纹 理坐标,从而确定像素点的颜色值。
二维纹理映射实现方法
使用OpenGL中的纹理映射函数,将纹理图像映射到物体表面。
三维纹理映射技术
三维纹理坐标
定义在三维空间中的坐标,表示纹理图像上的位置。
计算机图形学PPT课件

三维图形投影方法
正投影
平行光线垂直投射到投影面上 ,形成物体的正投影。
斜投影
平行光线与投影面成一定角度 投射,形成物体的斜投影。
透视投影
从视点出发,通过透视变换将 三维物体投影到二维平面上。
阴影生成
根据光源位置和物体形状,计 算阴影的位置和形状。
05
真实感图形绘制技术
Chapter
消隐技术
消隐算法分类
计算机图形学PPT课件
目录
• 引言 • 图形系统基础 • 基本图形生成算法 • 三维图形变换与观察 • 真实感图形绘制技术 • 曲线与曲面绘制技术 • 计算机动画技术 • 计算机图形学前沿技术
01
引言
Chapter
计算机图形学概述
01
02
03
计算机图形学定义
研究计算机生成、处理和 显示图形的一门科学。
平移变换 旋转变换 缩放变换 镜像变换
将三维图形沿x、y、z方向移动一 定距离,不改变图形形状和大小 。
在x、y、z方向分别进行缩放,可 改变图形的大小和形状。
三维图形复合变换
变换顺序
先进行缩放、旋转,再进行平移,注意变换顺序对结果的影响。
变换矩阵
将各种基本变换表示为矩阵形式,便于进行复合变换的计算。
医学诊断
通过计算机图形学技术,医生可以更 直观地了解病人病情,进行更准确的 诊断和治疗。
军事模拟
计算机图形学在军事模拟和训练中发 挥重要作用,提高训练效果和作战能 力。
THANKS
感谢观看
通过模拟自然现象或物理过程,生成具有真实感的动画效 果。
过程动画制作流程
建立自然现象或物理过程的数学模型,利用计算机图形学 技术模拟模型的运动和变化过程,生成具有真实感的动画 效果。
2024版计算机图形学课件ppt课件

01计算机图形学概述Chapter计算机图形学的定义与发展定义发展历程虚拟现实和增强现实VR 图形学来生成和处理三维场景。
工业设计师使用计算机图形学技术来设计和模拟产品的外观和性能。
建筑设计建筑师使用计算机图形学技术来设计和可视化建筑模型。
游戏开发游戏中的场景、角色、特效等都需要计算机图形学的支持。
影视制作都需要用到计算机图形学技术。
计算机科学数学物理艺术02计算机图形学基础Chapter图形与图像的基本概念图形与图像的定义图形是指用矢量方法描述的图像,由几何图元(点、线、面等)组成;图像则是由像素点组成的位图。
图形与图像的区别图形具有矢量特性,可以无限放大而不失真;而图像放大后会失真,因为其由固定数量的像素点组成。
计算机图形学的研究内容研究如何在计算机中表示、生成、处理和显示图形的一门科学。
色彩模型与颜色空间色彩模型01颜色空间02常见的色彩模型与颜色空间031 2 3光栅图形矢量图形光栅图形与矢量图形的比较光栅图形与矢量图形图形显示设备与坐标系统图形显示设备01坐标系统02设备坐标系与逻辑坐标系0303图形生成技术Chapter直线生成算法DDA算法Bresenham算法中点画线法圆生成算法八分法画圆中点画圆法Bresenham画圆法扫描线填充算法边界填充算法洪水填充算法030201多边形填充算法01020304几何变换光照模型投影变换纹理映射三维图形生成技术04图形变换与裁剪技术Chapter01020304将图形在平面上沿某一方向移动一定的距离,不改变图形的大小和形状。
平移变换将图形绕某一点旋转一定的角度,不改变图形的大小和形状。
旋转变换将图形在某一方向上按比例放大或缩小,改变图形的大小但不改变形状。
缩放变换将图形关于某一直线或点进行对称,得到一个新的图形。
对称变换将三维物体在空间中沿某一方向移动一定的距离,不改变物体的大小和形状。
将三维物体绕某一轴旋转一定的角度,不改变物体的大小和形状。
计算机图形学基本知识PPT课件

通过仿射变换矩阵对图像进行变换,可以处理更复杂的几何变换。
04 计算机图形学高级技术
光照模型与材质贴图
光照模型
描述物体表面如何反射光线的数 学模型,包括漫反射、镜面反射 和环境光等。
材质贴图
通过贴图技术将纹理映射到物体 表面,增强物体的真实感和细节 表现。
纹理映射
纹理映射技术
将图像或纹理图案映射到三维物体表 面,增强物体的表面细节和质感。
总结
计算机图形学在游戏设计、电影与动 画制作、虚拟现实与仿真等领域有着 广泛的应用。
计算机图形学的发展历程
起步阶段
20世纪50年代,计算机图形 学开始起步,主要应用于几 何形状的生成和简单图形的 处理。
发展阶段
20世纪80年代,随着计算机 性能的提高,计算机图形学 开始广泛应用于电影、游戏 等领域。
总结
计算机图形学利用计算机 技术生成、处理和显示图 形,实现真实世界的模拟 和再现。
计算机图形学的应用领域
游戏设计
游戏中的角色、场景和特效都需要用 到计算机图形学技术。
电影与动画制作
电影特效、角色建模和动画制作都离 不开计算机图形学。
虚拟现实与仿真
虚拟现实技术、军事仿真、工业设计 等领域都广泛应用计算机图形学。
向量图
向量图是矢量图的一种,通常用于描 述二维图形,如几何图形和图表。
图像的分辨率与质量
分辨率
分辨率是指图像中像素的数量, 通常以像素每英寸(PPI)或像素
每厘米(PPC)为单位。
质量
图像质量取决于分辨率、颜色深度 和压缩等因素。
压缩
图像压缩是一种减少图像文件大小 的方法,常见的图像压缩格式有 JPEG和PNG等。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
dx = (x1 > x0) ? 1 : -1;
// Set x increment.
while (x0 != x1) {
// Loop:
x0 += dx;
// Increment x.
y0 = (int)floor(m * x0 + b + 0.5); // Compute y and round.
CS 455 – Computer Graphics
Line Drawing
Line Drawing
• Scan-conversion or rasterization: Determining which pixels to illuminate - due to the scanning nature of raster displays.
(x(2),y(2)) = (x(2),y(1)+m)
(5,0) y
DDA Code
void lineDDA(int x0, int y0, int x1, int y1, Color c) { int dx = x1 – x0, dy = y1 – y0;
drawPoint(x0, y0, c); if (abs(dx) > abs(dy)) {
• In General:
▪ At x(k+1): y(k+1) = m(x0+k+1) + b = mx0 + km + m + b = y0 + km = yk + m
▪ Also: - xk = x(k) - yk = round(y(k))
Incremental/DDA Algorithm
while (/* Not done */) { // 3. Inner loop.
} }
// Loop.
• Can we move some computation outside the loop?
▪ The remaining discussion will assume that m 1
Incremental/DDA Algorithm
x = x0 + 0.5; ... } }
// Start at (x0, y0). // Absolute slope is less than 1? // Compute the slope. // Initialize rounded y value. // Set x increment. // Set y increment. // Loop: // Increment x. // Compute y incrementally. // Draw point (truncating y).
• Algorithms are fundamental to both 2-D and 3-D computer graphics.
• Transforming the continuous into the discrete (sampling).
• Line drawing was easy for vector displays.
▪ Function call (floor) inside the loop
• Most line drawing code has the general form:
void line(int x0, int y0, int x1, int y1, Color c) { // 1. General setup. // 2. Slope case setup.
// Loop:
x0 += dx;
// Increment x.
y0 = (int)floor(m * x0 + b + 0.5); // Compute y and
// round.
drawPoint(x0, y0, c);
// Draw point.
}
}
}
• Does it work?
• How about speed?
• What about if x0 > x1?
▪ Reverse the roles of (x0, y0) and (x1, y1).
Case 3
Standard Case
DDA Example
Line: (1,3) (5,0)
dx = 4 dy = -3
xincr = 1 yincr = m = -¾
double m = (double)dy / (double)dx, // Compute the slope.
b = y0 – m * x0;
// Compute y-intercept.
dx = (x1 > x0) ? 1 : -1;
// Set x increment.
while (x0 != x1) {
Basic Algorithm (cont.)
Problem: Doesn’t work if slope > 1
Creates gaps since x gets incremented by 1 each iteration
(x1,y1)
(x0,y0)
“Ideal” line
Basic Algorithm (cont.)
// Start at (x0, y0). // Absolute slope is less than 1?
double m = (double)dy / (double)dx, // Compute the slope.
b = y0 – m * x0;
// Compute y-intercept.
We will use the following 2-D screen coordinate API:
▪ Pixel centers are at
integer coordinates.
y
▪ The y-axis points up,
x-axis points right
(3,2)
(0,1)
x (0,0) (1,0) (2,0)
(x0,y0)
“Ideal” line(x1,y1)源自Basic Algorithm
Use implicit (slope intercept) form of a line:
y = mx + b Given two endpoints (x0, y0) and (x1, y1)
m = Dy/Dx We will step through the line, incrementing x each iteration Then let xinc = 1 (step 1 in x each iteration, then compute y), With y = mx + b
• DDA (Digital Differential Algorithm) uses an incremental approach to compute y.
▪ Compute m ▪ Draw (x0, y0) ▪ For each iteration:
- Increment x - Add m to y - Draw (x, (round)y)
Goal
• Given integer descriptions of the endpoints of a line, produce a rasterized line
• The line may not fall on the discretized pixels
▪ Usually won’t ▪ So, we must choose the nearest pixels to illuminate
Solution: check slope
void lineBasic(int x0, int y0, int x1, int y1, Color c) { int dx = x1 – x0, dy = y1 – y0;
drawPoint(x0, y0, c); if (abs(dx) > abs(dy)) {
double m = (double)dy / (double)dx, y = y0 + 0.5;
dx = (x1 > x0) ? 1 : -1; m *= dx; while (x0 != x1) {
x0 += dx; y += m; drawPoint(x0, (int)y, c); } } else if (dy != 0) { double m = (double)dx / (double)dy,
Case 4
• What about 1 m ? (case 3)
▪ Want to increment y, then compute x. Reverse the roles of x and y.
Case 2
• What about - m -1? (case 4)
▪ Want to increment y, then compute x. M will be negative so it will be subtracted from the previous x
• What is y at x(0) = x0?
▪ At x(0): y(0) = mx0 + b
• At x(1) = x0+1?
▪ At x(1): y(1) = m(x0+1) + b = mx0 + m + b = y0 + m
• At x(2) = x0+2?
▪ At x(2): y(2) = m(x0+2) + b = mx0 + 2m + b = y0 + 2m = y1 + m