实验3. 贪心算法
实验三-贪心算法
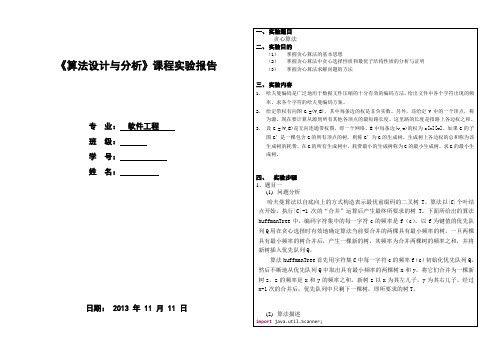
for(inti=0;i<s.length();i++){
buf.append(getEachCode(s.substring(i,i+1)));
}
returnbuf.toString();
}
publicString getEachCode(String name){
for(inti=0;i<buffer.length();i++){
if(name.equals(codes[i].name)){
returnhuffstring[i];
}
}
return"";
}
publicvoidgetCode(intn,String[] thecodes,String thebuffer){
importjava.util.Scanner;
classHuffmanCode{
Stringname;
doubleweight;
intlc,rc,pa;
publicHuffmanCode(){
name="";
weight=0;
lc=-1;rc=-1;pa=-1;
}
}
publicclassHuffman1 {
dist[j]=newdist;prev[j]=u;}}}}
(3)运行结果
3、题目三
(1)问题分析
设G=(V,E)是连通带权图,V={1,2,…,n}。构造G的最小生成树的Prim算法的基本思想是:首先置S{1},然后,只要S是V的真子集,就进行如下的贪心选择:选取满足条件i∈S,j∈V-S,且c[i][j]最小的边,将顶点j添加到S中。这个过程一直进行到S=V时为止。过程中所取到的边恰好构成G的一棵最小生成树。
实验3贪心算法(定稿)

实验3贪心算法(定稿)第一篇:实验3 贪心算法(定稿)《算法设计与分析》实验报告实验3贪心算法姓名学号班级实验日期实验地点一、实验目的1、掌握贪心算法的设计思想。
2、理解最小生成树的相关概念。
二、实验环境1、硬件环境 CPU:酷睿i5 内存:4GB 硬盘:1T2、软件环境操作系统:Windows10 编程环境:jdk 编程语言:Java三、实验内容:在Prim算法与Kruskal算法中任选一种求解最小生成树问题。
1、你选择的是:Prim算法2、数据结构(1)图的数据结构——图结构是研究数据元素之间的多对多的关系。
在这种结构中,任意两个元素之间可能存在关系,即结点之间的关系可以是任意的,图中任意元素之间都可能相关。
图形结构——多个对多个,如(2)树的数据结构——树结构是研究数据元素之间的一对多的关系。
在这种结构中,每个元素对下(层)可以有0个或多个元素相联系,对上(层)只有唯一的一个元素相关,数据元素之间有明显的层次关系。
树形结构——一个对多个,如3、算法伪代码 Prim(G,E,W)输入:连通图G 输出:G的最小生成树T 1.S←{1};T=∅ 2.While V-S ≠∅ do3.从V-S中选择j使得j到S中顶点的边e的权最小;T←T∪{e}4.S←S∪{j}3、算法分析时间复杂度:O(n)空间复杂度:O(n^2)4、关键代码(含注释)package Prim;import java.util.*;publicclass Main { staticintMAXCOST=Integer.MAX_VALUE;staticint Prim(intgraph[][], intn){ /* lowcost[i]记录以i为终点的边的最小权值,当lowcost[i]=0时表示终点i加入生成树 */ intlowcost[]=newint[n+1];/* mst[i]记录对应lowcost[i]的起点,当mst[i]=0时表示起点i加入生成树 */ intmst[]=newint[n+1];intmin, minid, sum = 0;/* 默认选择1号节点加入生成树,从2号节点开始初始化*/ for(inti = 2;i<= n;i++){/* 标记1号节点加入生成树 */ mst[1] = 0;/* n个节点至少需要n-1条边构成最小生成树 */ for(inti = 2;i<= n;i++){/* 找满足条件的最小权值边的节点minid */ for(intj = 2;j<= n;j++){/* 输出生成树边的信息:起点,终点,权值 */System.out.printf(“%c1, minid + 'A''A' + 1;intj = chy-'A' + 1;graph[i][j] = cost;graph[j][i] = cost;for(intj = 1;j<= n;j++){ } graph[i][j] = MAXCOST;} } System.out.println(”Total:"+cost);} }5、实验结果(1)输入(2)输出最小生成树的权值为:生成过程:(a)(b)(d)(e)(c)四、实验总结(心得体会、需要注意的问题等)这次实验,使我受益匪浅。
贪心算法 实验报告

贪心算法实验报告贪心算法实验报告引言:贪心算法是一种常用的算法设计策略,它通常用于求解最优化问题。
贪心算法的核心思想是在每一步选择中都选择当前最优的解,从而希望最终能够得到全局最优解。
本实验旨在通过实际案例的研究,探索贪心算法的应用和效果。
一、贪心算法的基本原理贪心算法的基本原理是每一步都选择当前最优解,而不考虑整体的最优解。
这种贪婪的选择策略通常是基于局部最优性的假设,即当前的选择对于后续步骤的选择没有影响。
贪心算法的优点是简单高效,但也存在一定的局限性。
二、实验案例:零钱兑换问题在本实验中,我们以零钱兑换问题为例,来说明贪心算法的应用。
问题描述:假设有不同面值的硬币,如1元、5元、10元、50元和100元,现在需要支付给客户x元,如何用最少的硬币数完成支付?解决思路:贪心算法可以通过每次选择当前面值最大的硬币来求解。
具体步骤如下:1. 初始化一个空的硬币集合,用于存放选出的硬币。
2. 从面值最大的硬币开始,如果当前硬币的面值小于等于待支付金额,则将该硬币放入集合中,并将待支付金额减去该硬币的面值。
3. 重复步骤2,直到待支付金额为0。
实验过程:以支付金额为36元为例,我们可以通过贪心算法求解最少硬币数。
首先,面值最大的硬币为100元,但36元不足以支付100元硬币,因此我们选择50元硬币。
此时,剩余待支付金额为36-50=-14元。
接下来,面值最大的硬币为50元,但待支付金额为负数,因此我们选择下一个面值最大的硬币,即10元硬币。
此时,剩余待支付金额为-14-10=-24元。
继续选择10元硬币,剩余待支付金额为-24-10=-34元。
再次选择10元硬币,剩余待支付金额为-34-10=-44元。
最后,选择5元硬币,剩余待支付金额为-44-5=-49元。
由于待支付金额已经为负数,我们无法继续选择硬币。
此时,集合中的硬币数为1个50元和3个10元,总共4个硬币。
实验结果:通过贪心算法,我们得到了36元支付所需的最少硬币数为4个。
实验3贪心算法和回溯法

实验3 贪心算法和回溯法一、实验目的1. 理解最小生成树算法——Prim算法和Kruskal算法的基本思想,学会编程实现这两种算法;2. 理解并查集的特点与适用环境,学会使用并查集解决常见的问题;3. 理解单源最短路径算法——Dijkstra算法的基本思想,学会编程实现Dijkstra算法;4. 理解回溯法的基本思想,学会使用回溯法解决常见的问题。
二、实验内容1. 编程实现Prim算法。
输入:顶点编号及边权重。
例:0 1 100 2 151 2 50输出:最小生成树。
例:0 1 100 2 152. 在某个城市里住着n个人,现在给定关于这n个人的m条信息(即某2个人认识)。
假设所有认识的人一定属于同一个单位,请计算该城市最多有多少单位?输入:第1行的第1个值表示总人数,第2个值表示总信息数;第2行开始为具体的认识关系信息。
例:10 42 34 54 85 8输出:单位个数。
例:73. 编程实现Kruskal算法。
输入:顶点编号及边权重。
例:0 1 100 2 151 2 50输出:最小生成树。
例:0 1 100 2 154. 编程实现Dijkstra算法。
输入:第1行第1个值表示顶点个数,第2个值表示边个数;第2行开始为边权重。
例:5 70 1 100 3 300 4 1001 2 502 4 103 2 203 4 60输出:顶点0到每一个顶点的最短路径长度。
例:0 10 50 30 605. 使用回溯法求解N皇后问题。
输入:皇后的个数。
例:4输出:每一个方案及总方案数。
例:0 1 0 00 0 0 23 0 0 00 0 4 0----------------0 0 1 02 0 0 00 0 0 30 4 0 0----------------总方案数为2。
6. 使用回溯法求解0-1背包问题。
输入:两个一维数组分别存储每一种物品的价值和重量,以及一个整数表示背包的总重量。
例:价值数组v[] = {6,3,6,5,4},重量数组w[] = {2,2,4,6,5},背包重量C=10。
贪心算法背包问题

题目:贪心算法
背包问题
专业:JAVA 技术 09——02 班 学号:540913100201 姓名:柏顺顺 指导老师:宋胜利
实验三:贪心算法
一、实验目的与要求
1、掌握背包问题的算法 2、初步掌握贪心算法
背包问题
二、实验题:
问题描述:与 0-1 背包问题相似,给定 n 种物品和一个背包。物品 i 的重量是 wi,其价 值为 vi,背包的容量为 c。与 0-1 背包问题不同的是,在选择物品 i 装入背包时,背包问题 的解决可以选择物品 i 的一部分,而不一定要全部装入背包,1< i < n。
JOptionPane.showMessageDialog(null, "重量不能为空!"); return; } else{ if (s1.equals("")){ JOptionPane.showMessageDialog(null, "效益值不能为空!"); return; }else { if (s3.equals("")) JOptionPane.showMessageDialog(null, "总重量不能为空!"); return; } } } catch (Exception e3) { // TODO: handle exception }
}
//可以执行贪心算法了 int [] wCopy = new int [w.length]; int [] pCopy = new int [w.length]; float temp2; int temp1; float totalx=0; float [] x = new float [w.length];//效益和重量的比值 float [] n =new float[w.length];//记录个数 float [] nCopy = new float[w.length]; for(int i=0;i<w.length;i++) { wCopy [i] = w[i]; pCopy [i] = p[i]; } for (int i=0;i<w.length;i++) x[i] = (float) ((p[i]*1.0)/w[i]); for(int i= 0;i<w.length;i++) for(int j=i+1;j<w.length;j++)
《算法导论》贪心算法实验指导书(二)

《算法导论》贪心算法实验指导书(二)
《算法导论》实验指导书
本书共分阶段4个实验,每个实验有基本题和提高题。
基本题必须完成,提高题根据自己实际情况进行取舍。
题目不限定如下题目,可根据自己兴趣爱好做一些与实验内容相关的其他题目,如动态规划法中的图象压缩,回溯法中的人机对弈等。
其具体要求和步骤如下:
实验三贪心算法(4学时)
一、实验要求与目的
1、熟悉贪心算法的基本原理与适用范围。
2、使用贪心算法编程,求解最小生成树问题。
二、实验内容
1、任选一种贪心算法(Prim或Kruskal),求解最小生成树。
对算法进行描述和复杂性分析。
编程实现,并给出测试实例
一、实验要求与目的
3、熟悉贪心算法的基本原理与适用范围。
4、使用贪心算法编程,求解霍夫曼编码问题。
二、实验内容
2、采用贪心算法,求解霍夫曼编码。
对算法进行描述和复杂性分析。
编程实现,并给出测试实例
一、实验目的与要求
1、掌握汽车加油问题的算法;
2、进一步掌握贪心算法;
二、实验题
一辆汽车加满油后可以行驶N千米。
旅途中有若干个加油站。
若要使沿途的加油次数最少,设计一个有效的算法,指出应在那些加油站停靠加油。
并证明你的算法能产生一个最优解。
三、实验提示
把两加油站的距离放在数组中,a[1..n]表示从起始位置开始跑,经过n个加油站,a[k]表示第k-1个加油站到第k个加油站的距离。
汽车在运行的过程中如果能跑到下一个站则不加油,否则要加油。
(算法略)。
算法实验报告贪心

一、实验背景贪心算法是一种在每一步选择中都采取当前状态下最好或最优的选择,从而希望导致结果是全局最好或最优的算法策略。
贪心算法并不保证能获得最优解,但往往能获得较好的近似解。
在许多实际应用中,贪心算法因其简单、高效的特点而被广泛应用。
本实验旨在通过编写贪心算法程序,解决经典的最小生成树问题,并分析贪心算法的优缺点。
二、实验目的1. 理解贪心算法的基本原理和应用场景;2. 掌握贪心算法的编程实现方法;3. 分析贪心算法的优缺点,并尝试改进;4. 比较贪心算法与其他算法在解决最小生成树问题上的性能。
三、实验内容1. 最小生成树问题最小生成树问题是指:给定一个加权无向图,找到一棵树,使得这棵树包含所有顶点,且树的总权值最小。
2. 贪心算法求解最小生成树贪心算法求解最小生成树的方法是:从任意一个顶点开始,每次选择与当前已选顶点距离最近的顶点,将其加入生成树中,直到所有顶点都被包含在生成树中。
3. 算法实现(1)数据结构- 图的表示:邻接矩阵- 顶点集合:V- 边集合:E- 已选顶点集合:selected- 最小生成树集合:mst(2)贪心算法实现```def greedy_mst(graph):V = set(graph.keys()) # 顶点集合selected = set() # 已选顶点集合mst = set() # 最小生成树集合for i in V:selected.add(i)mst.add((i, graph[i]))while len(selected) < len(V):min_edge = Nonefor edge in mst:u, v = edgeif v not in selected and (min_edge is None or graph[u][v] < graph[min_edge[0]][min_edge[1]]):min_edge = edgeselected.add(min_edge[1])mst.add(min_edge)return mst```4. 性能分析为了比较贪心算法与其他算法在解决最小生成树问题上的性能,我们可以采用以下两种算法:(1)Prim算法:从任意一个顶点开始,逐步添加边,直到所有顶点都被包含在生成树中。
算法分析与设计实验三贪心算法

实验三贪心算法实验目的1. 掌握贪心法的基本思想方法;2. 了解适用于用贪心法求解的问题类型,并能设计相应贪心法算法;3. 掌握贪心算法复杂性分析方法分析问题复杂性。
预习与实验要求1. 预习实验指导书及教材的有关内容,掌握贪心法的基本思想;2. 严格按照实验内容进行实验,培养良好的算法设计和编程的习惯;3. 认真听讲,服从安排,独立思考并完成实验。
实验设备与器材硬件:PC机软件:C++或Java等编程环境实验原理有一类问题是要从所有的允许解中求出最优解,其策略之一是“贪心法”,即逐次实施“贪心选择”:在每个选择步骤上做出的选择都是当前状态下最优的。
贪心选择依赖于在此之前所做出的选择,但不依赖于后续步骤所需要的选择,即不依赖于后续待求解子问题。
显然,这种选择方法是局部最优的,但不是从问题求解的整体考虑进行选择,因此不能保证最后所得一定是最优解。
贪心法是求解问题的一种有效方法,所得到的结果如果不是最优的,通常也是近似最优的。
实验内容以下几个问题选做一项:1. 用贪心法实现带有期限作业排序的快速算法应用贪心设计策略来解决操作系统中单机、无资源约束且每个作业可在等量时间内完成的作业调度问题。
假定只能在一台机器上处理N个作业,每个作业均可在单位时间内完成;又假定每个作业i都有一个截止期限di>0(它是整数),当且仅当作业i在它的期限截止以前被完成时,则获得pi的效益。
这个问题的一个可行解是这N个作业的一个子集合J,J中的每个作业都能在各自的截止期限之前完成。
可行解的效益值是J中这些作业的效益之和,即Σp。
具有最大效益值的可行解就是最优解。
2. 实现K元归并树贪心算法两个分别包含n个和m个记录的已分类文件可以在O(n+m)时间内归并在一起而得到一个分类文件。
当要把两个以上的已分类文件归并在一起时,可以通过成对地重复归并已分类的文件来完成。
例如:假定X1,X2,X3,X4是要归并的文件,则可以首先把X1和X2归并成文件Y1,然后将Y1和X3归并成Y2,最后将Y2和X4归并,从而得到想要的分类文件;也可以先把X1和X2归并成Y1,然后将X3和X4归并成Y2,最后归并Y1和Y2而得到想要的分类文件。
贪心算法实验

实验三贪心算法的应用 (1)一、实验目的 (1)二、实验内容 (1)三、实验步骤 (2)实验三贪心算法的应用一、实验目的1.掌握贪心算法的基本概念和两个基本要素2.熟练掌握贪心算法解决问题的基本步骤。
3.学会利用贪心算法解决实际问题。
二、实验内容1.问题描述:题目一:找钱问题一个顾客买了价值x元的商品(不考虑角、分),并将y元的钱交给售货员。
售货员希望用张数最少的钱币找给顾客。
要求:键盘输入x与y输出找钱总数,各种钱币的张数,若张数为0不必输出。
输入输出实例:题目二:删数问题键盘输入一个高精度的正整数N,去掉其中任意S个数字后剩下的数字按原左右次序将组成一个新的正整数。
编程对给定的N和S,寻找一种方案使得剩下的数字组成的新数最小。
输出应包括所去掉的数字的位置和组成的新的正整数(N不超过100位)。
要求:键盘输入正整数N与删除位数s输出删除后的最小新数以及删除的位数输入输出实例:题目三:分数表示问题设计一个算法,把一个真分数表示为最少埃及分数之和的形式。
所谓埃及分数是指分子为1的分数。
如7/8=1/2+1/3+1/24。
三、实验步骤1.理解算法思想和问题要求;2.编程实现题目要求;3.上机输入和调试自己所编的程序;4.验证分析实验结果;5.整理出实验报告一.实验目的二.问题描述三.算法设计包含:数据结构与核心算法的设计描述、函数调用及主函数设计、主要算法流程图等对于高精度数的运算,应该讲输入的高精度数存储为字符串格式,根据输出要求设置数组,在删除数字是记录其位置。
在位数固定的前提下,让高位的数字尽量小,其值就较小,依据贪婪策略就可以解决这个问题。
另外,删除字符使用后面的字符覆盖已删除的字符的方法,但字符串长度会改变,可能会有比较多字符移动操作,算法效率不高。
定义一个del 函数负责删除字符,不断调用del 函数以删除高位较大的数。
是否四.程序调试及运行结果分析运行程序,输入一个正整数23146,输入要删除3个数字,然后成功运行程序后,输出删除后的最小数为14,删除的位数分别为2,1,5。
实验3 贪心算法
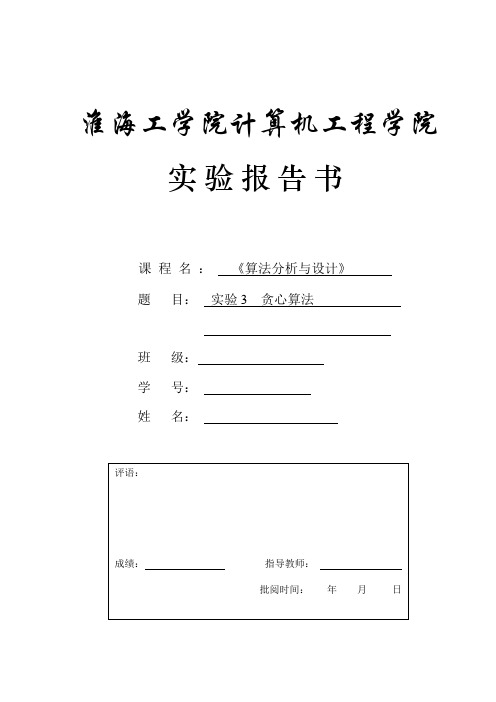
淮海工学院计算机工程学院实验报告书课程名:《算法分析与设计》题目:实验3 贪心算法班级:学号:姓名:实验3 贪心算法实验目的和要求(1)了解前缀编码的概念,理解数据压缩的基本方法;(2)掌握最优子结构性质的证明方法;(3)掌握贪心法的设计思想并能熟练运用(4)证明哈夫曼树满足最优子结构性质;(5)设计贪心算法求解哈夫曼编码方案;(6)设计测试数据,写出程序文档。
实验内容设需要编码的字符集为{d 1, d 2, …, dn },它们出现的频率为{w 1, w 2, …, wn },应用哈夫曼树构造最短的不等长编码方案。
实验环境Turbo C 或VC++实验学时2学时,必做实验数据结构与算法//构造哈夫曼结构体struct huffman{double weight; //用来存放各个结点的权值int lchild,rchild,parent; //指向双亲、孩子结点的指针 };核心源代码#include<iostream>#include <string>using namespace std;#include <stdio.h>//构造哈夫曼结构体struct huffman{double weight;∑=j i k k aint lchild,rchild,parent;};static int i1=0,i2=0;//选择权值较小的节点int Select(huffman huff[],int i){int min=11000;int min1;for(int k=0;k<i;k++){if(huff[k].weight<min && huff[k].parent==-1){min=huff[k].weight;min1=k;}}huff[min1].parent=1;return min1;}//定义哈夫曼树,并对各个节点进行赋权值void HuffmanTree(huffman huff[],int weight[],int n) {for(int i=0;i<2*n-1;i++){huff[i].lchild=-1;huff[i].parent=-1;huff[i].rchild=-1;}for(int l=0;l<n;l++){huff[l].weight=weight[l];}for(int k=n;k<2*n-1;k++){int i1=Select(huff,k);int i2=Select(huff,k);huff[i1].parent=k;huff[i2].parent=k;huff[k].weight= huff[i1].weight+huff[i2].weight;huff[k].lchild=i1;huff[k].rchild=i2;}}//哈夫曼编码,左0右1void huffmancode(huffman huff[],int n){string s;int j;for(int i=0;i<n;i++){s="";j=i;while(huff[j].parent!=-1){if(huff[huff[j].parent].lchild==j)s=s+"0";else s=s+"1";j=huff[j].parent;}cout<<"第"<<i+1<<"个节点的哈夫曼编码为:";for(int j=s.length();j>=0;j--){cout<<s[j];}cout<<endl;}}void main(){huffman huff[20];int n,w[20];printf("请输入节点的个数:");scanf("%d",&n);for(int i=0;i<n;i++){printf("请输入第%d个节点的权值:",i+1);scanf("%d",&w[i]);}printf("\n");HuffmanTree(huff,w,n);huffmancode(huff,n);}实验结果实验体会本次实验是用贪心法求解哈夫曼编码,其实贪心法和哈夫曼树的原理是一样的,每次将集合中两个权值最小的二叉树合并成一棵新二叉树,每次选择两个权值最小的二叉树时,规定了较小的为左子树。
贪心算法实验报告

一、实验目的通过本次实验,使学生对贪心算法的概念、基本要素、设计步骤和策略有更深入的理解,掌握贪心算法的原理和应用,并能够运用贪心算法解决实际问题。
二、实验内容本次实验主要涉及以下两个问题:1. 使用贪心算法解决单起点最短路径问题;2. 使用贪心算法解决小船过河问题。
三、实验原理1. 贪心算法贪心算法(又称贪婪算法)是一种在每一步选择中都采取当前最优的选择,从而希望导致结果是全局最优的算法。
贪心算法在每一步只考虑当前的最优解,不保证最终结果是最优的,但很多情况下可以得到最优解。
2. 单起点最短路径问题单起点最短路径问题是指在一个有向无环图中,从某个顶点出发,找到到达其他所有顶点的最短路径。
3. 小船过河问题小船过河问题是指一群人需要划船过河,船只能容纳两个人,过河后需要一人将船开回,问最少需要多久让所有人过河。
四、实验步骤及说明1. 创建图结构,包括顶点数组和边信息。
2. 使用Dijkstra算法求解单起点最短路径问题,得到最短路径和前驱顶点。
3. 使用贪心算法找到两点之间的最短距离,并更新距离和前驱顶点信息。
4. 遍历所有顶点,找到未纳入已找到点集合的距离最小的顶点,并更新其距离和前驱顶点。
5. 最终输出从源顶点到达其余所有点的最短路径。
6. 使用贪心算法解决小船过河问题,按照以下步骤进行:(1)计算所有人过河所需的总时间;(2)计算每次划船往返所需时间;(3)计算剩余人数;(4)重复(2)和(3)步骤,直到所有人过河。
五、实验结果与分析1. 单起点最短路径问题实验中,我们选取了有向无环图G,其中包含6个顶点和8条边。
使用贪心算法和Dijkstra算法求解单起点最短路径问题,得到的实验结果如下:- 贪心算法求解单起点最短路径问题的时间复杂度为O(V^2),其中V为顶点数;- Dijkstra算法求解单起点最短路径问题的时间复杂度为O(V^2),其中V为顶点数。
2. 小船过河问题实验中,我们选取了一群人数为10的人过河,船每次只能容纳2人。
实验三 贪心算法

实验三贪心算法一、实验目的与要求1、熟悉多机调度问题的算法;2、初步掌握贪心算法;二、实验题要求给出一种作业调度方案,使所给的n个作业在尽可能短的时间内由m台机器加工处理完成。
约定,每个作业均可在任何一台机器上加工处理,但未完工前不允许中断处理。
作业不能拆分成更小的子作业。
三、实验提示1、把作业按加工所用的时间从大到小排序2、如果作业数目比机器的数目少或相等,则直接把作业分配下去3、如果作业数目比机器的数目多,则每台机器上先分配一个作业,如下的作业分配时,是选那个表头上s最小的链表加入新作业。
# include <iostream># include <iomanip>using namespace std;typedef struct Job //作业{int ID;int time;}Job;typedef struct JobNode //作业链表的节点{int ID;int time;JobNode *next;}JobNode,*pJobNode;typedef struct Header //链表的表头{int s; //处理机上的时间;JobNode *next;}Header,pHeader;int main(){void QuickSort(Job *job,int left,int right); //将job时间排序void outSort(Job *job,int n); //输出排序void display(Header *M,int m); //输出每个每台机器处理的工作序号数int SelectMin(Header *M,int m); //分配作业时选取机器函数;void solve(Header *head,Job*job,int n,int m); //作业分配函数;int m,n;cout<<"\t\t《多机调度问题》\n";cout<<"请输入机器台数m:";cin>>m;Header *head=new Header [m]; //动态构建数组结构体,用于记录机器的作业时间;cout<<"请输入作业个数n:";cin>>n;Job *job=new Job [n]; //动态构建作业的数组结构体;cout<<"\n请按序号输入每个作业调度所需时间time:";for(int i=0;i<n;i++){cin>>job[i].time;job[i].ID=i;}QuickSort(job,0,n-1); //作业排序outSort(job,n); //输出排序solve(head,job,n,m); //作业分配display(head,m); //输出分配cout<<endl<<endl;return 0;}int SelectMin(Header* M,int m) //选择s最小的机器序号k;{int k=0;for(int i=1;i<m;i++){if(M[i].s<M[k].s)k=i; //k记录S最小的序号;}return k;}void QuickSort(Job *job,int left,int right) //小到大,排序{int middle=0,i=left,j=right;Job itemp;middle=job[(left+right)/2].time;do{while((job[i].time>middle)&&(i<right))i++;while((job[j].time<middle)&&(j>left))j--;if(i<=j){itemp=job[j];job[j]=job[i];job[i]=itemp;i++;j--;}}while(i<=j);if(left<j)QuickSort(job,left,j);if(right>i)QuickSort(job,i,right);}void display(Header *M,int m) //作业分配输出函数;{JobNode *p;for(int i=0;i<m;i++){cout<<"\n第"<<i<<"台机器上处理的工作序号:";if(M[i].next==0)continue;p=M[i].next;do{cout<<p->ID<<' ';p=p->next;}while(p!=0);}}void outSort(Job *job,int n) //作业时间由大到小排序后输出函数;{cout<<"\n按工作时间由大到小为:\n时间:\t";for(int i=0;i<n;i++)cout<<setw(4)<<job[i].time;cout<<"\n序号:\t";for( i=0;i<n;i++)cout<<setw(4)<<job[i].ID;}void solve(Header *head,Job*job,int n,int m) //作业分配函数;{int k;for(int i=0;i<m&&i<n;i++){JobNode *jobnode=new JobNode;jobnode->time=job[i].time;jobnode->ID=job[i].ID;jobnode->next=0;head[i].s=jobnode->time;head[i].next=jobnode;}if(i<=m) //n<m的情况续处理;{for(i;i<m;i++){head[i].s=0;head[i].next=0;}}if(n>m){for(i;i<n;i++){JobNode *p;JobNode *jobnode=new JobNode;jobnode->time=job[i].time;jobnode->ID=job[i].ID;jobnode->next=0;k=SelectMin(head,m);p=head[k].next;head[k].s+=jobnode->time;while(p->next!=0)p=p->next;p->next=jobnode;}}}运行结果:提高题一:用贪心算法求解最小生成树一、实验要求与目的1、熟悉贪心算法的基本原理与适用范围。
贪心算法及其应用doc资料
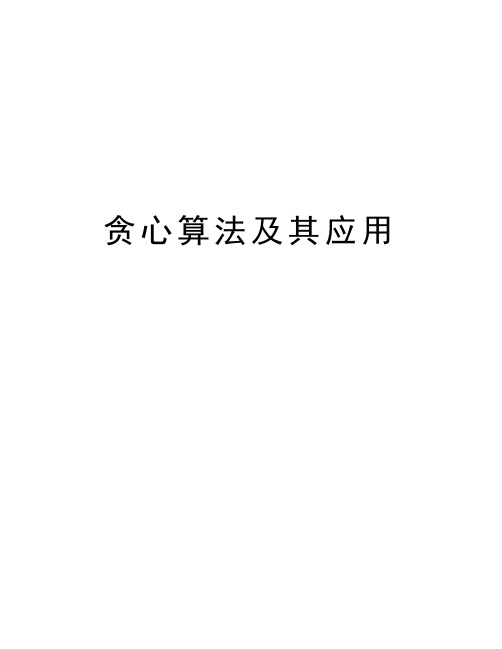
贪心算法及其应用湖州师范学院实验报告课程名称:算法实验三:贪心算法一、实验目的1、理解贪心算法的概念,掌握贪心算法的基本要素。
2、掌握设计贪心算法的一般步骤,针对具体问题,能应用贪心算法求解。
二、实验内容1、问题描述活动安排问题设有n个活动的集合E={1,2,…,n},其中每个活动都要求使用同一资源,如演讲会场等,而在同一时间内只有一个活动能使用这一资源。
每个活动i都有一个要求使用该资源的起始时间si和一个结束时间fi,且si <fi 。
如果选择了活动i,则它在半开时间区间[si, fi)内占用资源。
若区间[si, fi)与区间[sj, fj)不相交,则称活动i与活动j是相容的。
也就是说,当si≥fj或sj≥fi时,活动i与活动j相容。
2、数据输入:文件输入或键盘输入。
3、要求:1)完成上述两个问题中1个或全部,时间为 1 次课。
2)独立完成实验及实验报告。
三、实验步骤1、理解方法思想和问题要求。
2、采用编程语言实现题目要求。
3、上机输入和调试自己所写的程序。
4、附程序主要代码:2、活动规划问题#include<stdio.h>#include<iostream>#include<algorithm>using namespace std;struct node{int start;int end;} a[11111];bool cmp(node x,node y){if(x.end<y.end) return true;else if(x.end==y.end && x.start>y.start) return true; return false;}int main(){int n,i,j,ans,end;cin>>n;for(i=0;i<n;i++) cin>>a[i].start>>a[i].end;sort(a,a+n,cmp);ans=0;end=-1e9-100;for(i=0;i<n;i++) {if(a[i].start>=end) {ans++;end=a[i].end;}}cout<<ans<<endl;return 0;}5、实验结果:四、实验分析活动安排问题:结束时间越早的活动优先。
实验三贪心算法
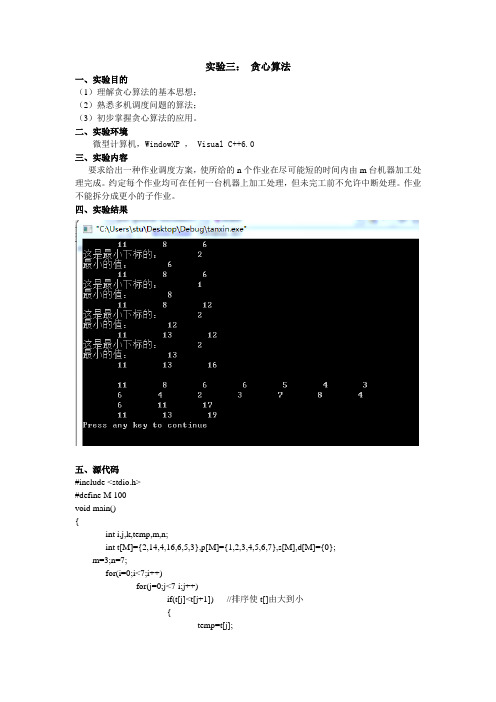
实验三:贪心算法一、实验目的(1)理解贪心算法的基本思想;(2)熟悉多机调度问题的算法;(3)初步掌握贪心算法的应用。
二、实验环境微型计算机,WindowXP , Visual C++6.0三、实验内容要求给出一种作业调度方案,使所给的n个作业在尽可能短的时间内由m台机器加工处理完成。
约定每个作业均可在任何一台机器上加工处理,但未完工前不允许中断处理。
作业不能拆分成更小的子作业。
四、实验结果五、源代码#include <stdio.h>#define M 100void main(){int i,j,k,temp,m,n;int t[M]={2,14,4,16,6,5,3},p[M]={1,2,3,4,5,6,7},s[M],d[M]={0};m=3;n=7;for(i=0;i<7;i++)for(j=0;j<7-i;j++)if(t[j]<t[j+1]) //排序使t[]由大到小{temp=t[j];t[j]=t[j+1];t[j+1]=temp;temp=p[j]; //p[]始终和t[]一一对应p[j]=p[j+1];p[j+1]=temp;}for(i=0;i<m;i++) //求时间。
{s[i]=p[i];d[i]=t[i];}for(k=0;k<m;k++)printf(" %d",d[k]);printf("\n");for(i=m;i<n;i++){for(k=0;k<m-1;k++) //求最小。
{temp=d[k];if(temp>d[k+1]){temp=d[k+1];j=k+1;}}printf("这是最小下标的:%d\n",j);printf("最小的值:%d\n",temp);for(k=0;k<m;k++)printf(" %d",d[k]);printf("\n");//j=temp;s[j]=s[j]+p[i];d[j]=d[j]+t[i];}printf("\n");for(k=0;k<7;k++)printf(" %d",t[k]);printf("\n");for(k=0;k<7;k++)printf(" %d",p[k]);printf("\n");for(k=0;k<m;k++)printf(" %d",s[k]);printf("\n");for(k=0;k<m;k++)printf(" %d",d[k]);printf("\n");}。
贪心算法实验
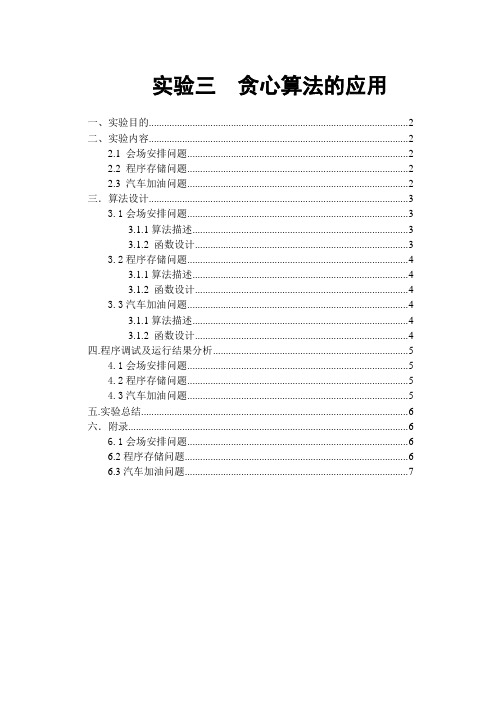
实验三贪心算法的应用一、实验目的 (2)二、实验内容 (2)2.1 会场安排问题 (2)2.2 程序存储问题 (2)2.3 汽车加油问题 (2)三.算法设计 (3)3.1会场安排问题 (3)3.1.1算法描述 (3)3.1.2 函数设计 (3)3.2程序存储问题 (4)3.1.1算法描述 (4)3.1.2 函数设计 (4)3.3汽车加油问题 (4)3.1.1算法描述 (4)3.1.2 函数设计 (4)四.程序调试及运行结果分析 (5)4.1会场安排问题 (5)4.2程序存储问题 (5)4.3汽车加油问题 (5)五.实验总结 (6)六.附录 (6)6.1会场安排问题 (6)6.2程序存储问题 (6)6.3汽车加油问题 (7)一、实验目的1.掌握贪心算法的基本概念和两个基本要素2.熟练掌握贪心算法解决问题的基本步骤。
3.学会利用贪心算法解决实际问题。
二、实验内容2.1 会场安排问题假设要在足够多的会场里安排一批活动,并希望使用尽可能少的会场。
设计一个有效的贪心算法来进行安排。
试编程实现对于给定的k个待安排活动,计算使用的最少会场。
输入数据中,第一行是k的值,接下来的k行中,每行有2个正整数,分别表示k个待安排活动的开始时间和结束时间,时间以0点开始的分钟计。
输出为最少的会场数。
2.2 程序存储问题设有n个程序{1,2,3,…,n}要存放在长度为L的磁带上。
程序i存放在磁带上的长度是li,1≤i≤n。
要求确定这n个程序在磁带上的一个存储方案,使得能够在磁带上存储尽可能多的程序。
输入数据中,第一行是2个正整数,分别表示程序文件个数和磁带长度L。
接下来的1行中,有n个正整数,表示程序存放在磁带上的长度。
输出为最多可以存储的程序个数。
2.3 汽车加油问题一辆汽车加满油后,可行使n千米。
旅途中有若干个加油站。
若要使沿途加油次数最少,设计一个有效算法,对于给定的n和k个加油站位置,指出应在哪些加油站停靠加油才能使加油次数最少。
贪心算法的实验原理
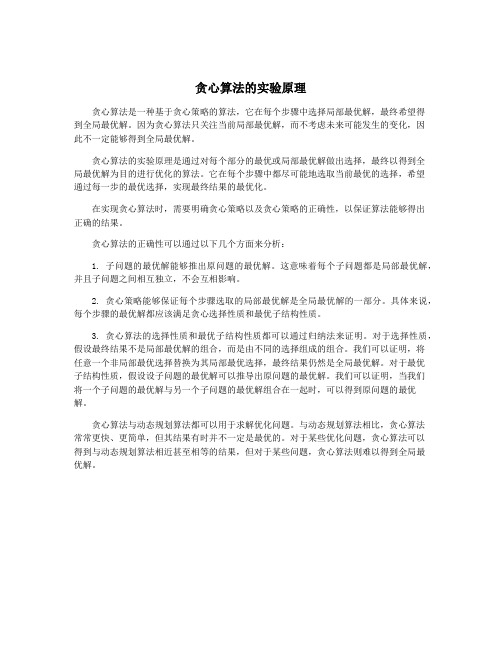
贪心算法的实验原理贪心算法是一种基于贪心策略的算法,它在每个步骤中选择局部最优解,最终希望得到全局最优解。
因为贪心算法只关注当前局部最优解,而不考虑未来可能发生的变化,因此不一定能够得到全局最优解。
贪心算法的实验原理是通过对每个部分的最优或局部最优解做出选择,最终以得到全局最优解为目的进行优化的算法。
它在每个步骤中都尽可能地选取当前最优的选择,希望通过每一步的最优选择,实现最终结果的最优化。
在实现贪心算法时,需要明确贪心策略以及贪心策略的正确性,以保证算法能够得出正确的结果。
贪心算法的正确性可以通过以下几个方面来分析:1. 子问题的最优解能够推出原问题的最优解。
这意味着每个子问题都是局部最优解,并且子问题之间相互独立,不会互相影响。
2. 贪心策略能够保证每个步骤选取的局部最优解是全局最优解的一部分。
具体来说,每个步骤的最优解都应该满足贪心选择性质和最优子结构性质。
3. 贪心算法的选择性质和最优子结构性质都可以通过归纳法来证明。
对于选择性质,假设最终结果不是局部最优解的组合,而是由不同的选择组成的组合。
我们可以证明,将任意一个非局部最优选择替换为其局部最优选择,最终结果仍然是全局最优解。
对于最优子结构性质,假设设子问题的最优解可以推导出原问题的最优解。
我们可以证明,当我们将一个子问题的最优解与另一个子问题的最优解组合在一起时,可以得到原问题的最优解。
贪心算法与动态规划算法都可以用于求解优化问题。
与动态规划算法相比,贪心算法常常更快、更简单,但其结果有时并不一定是最优的。
对于某些优化问题,贪心算法可以得到与动态规划算法相近甚至相等的结果,但对于某些问题,贪心算法则难以得到全局最优解。
贪心算法实验报告

贪心算法实验报告贪心算法实验报告引言:贪心算法是一种常用的算法设计思想,它在求解最优化问题中具有重要的应用价值。
本实验报告旨在介绍贪心算法的基本原理、应用场景以及实验结果,并通过实例加以说明。
一、贪心算法的基本原理贪心算法是一种以局部最优解为基础,逐步构建全局最优解的算法。
其基本原理是在每一步选择中都采取当前状态下最优的选择,而不考虑之后的结果。
贪心算法通常具备以下特点:1. 贪心选择性质:当前状态下的最优选择一定是全局最优解的一部分。
2. 最优子结构性质:问题的最优解可以通过子问题的最优解来构造。
3. 无后效性:当前的选择不会影响以后的选择。
二、贪心算法的应用场景贪心算法适用于一些具有最优子结构性质的问题,例如:1. 路径选择问题:如Dijkstra算法中的最短路径问题,每次选择当前距离最短的节点进行扩展。
2. 区间调度问题:如活动选择问题,每次选择结束时间最早的活动进行安排。
3. 零钱找零问题:给定一些面额不同的硬币,如何用最少的硬币凑出指定的金额。
三、实验设计与实现本次实验选择了一个经典的贪心算法问题——零钱找零问题,旨在验证贪心算法的有效性。
具体实现步骤如下:1. 输入硬币面额和需要凑出的金额。
2. 对硬币面额进行排序,从大到小。
3. 从面额最大的硬币开始,尽可能多地选择该面额的硬币,直到不能再选择为止。
4. 重复步骤3,直到凑出的金额等于需要凑出的金额。
四、实验结果与分析我们通过对不同金额的零钱找零问题进行实验,得到了如下结果:1. 当需要凑出的金额为25元时,贪心算法的结果为1个25元硬币。
2. 当需要凑出的金额为42元时,贪心算法的结果为1个25元硬币、1个10元硬币、1个5元硬币、2个1元硬币。
3. 当需要凑出的金额为63元时,贪心算法的结果为2个25元硬币、1个10元硬币、1个1元硬币。
通过实验结果可以看出,贪心算法在零钱找零问题中取得了较好的效果。
然而,贪心算法并不是适用于所有问题的万能算法,它的有效性取决于问题的特性。
贪心算法解活动安排实验报告

实验3 贪心算法解活动安排问题一、实验要求1.要求按贪心法求解问题;2.要求读文本文件输入活动安排时间区间数据;3.要求显示结果。
二、实验仪器和软件平台仪器:带usb接口微机软件平台:WIN-XP + VC++6.0三、源程序#include "stdafx.h"#include<stdio.h>#include<stdlib.h>#include<algorithm>#define N 50#define TURE 1#define FALSE 0int s[N];/*开始时间*/int f[N];/*结束时间*/int A[N];/*用A存储所有的*/int Partition(int *b,int *a,int p,int r);void QuickSort(int *b,int *a,int p,int r);void GreedySelector(int n,int *s,int *f,int *A);int main(){int n=0,i;while(n<=0||n>50){printf("\n");printf("请输入活动的个数,n=");scanf("%d",&n);if(n<=0) printf("请输入大于零的数!");else if(n>50) printf("请输入小于50的数!");}printf("\n请分别输入开始时间s[i]和结束时间f[i]:\n\n");for(i=1;i<=n;i++){printf("s[%d]=",i,i);scanf("%d",&s[i]);printf("f[%d]=",i,i);scanf("%d",&f[i]);printf("\n");}QuickSort(s,f,1,n); //按结束时间非减序排列printf("按结束时间非减序排列如下:\n"); /*输出排序结果*/ printf("\n 序号\t开始时间结束时间\n");printf("-------------------------\n");for(i=1;i<=n;i++)printf(" %d\t %d\t %d\n",i,s[i],f[i]);printf("-------------------------\n");GreedySelector(n,s,f,A);//贪心算法实现活动安排printf("安排的活动序号依次为:");for(i=1;i<=n;i++){if(A[i])printf("\n%d %d-->%d",i,s[i],f[i]);}printf("\n");system("pause");return 0;}//快速排序void QuickSort(int *b,int *a,int p,int r){int q;if(p<r)q=Partition(b,a,p,r);QuickSort(b,a,p,q-1);/*对左半段排序*/ QuickSort(b,a,q+1,r);/*对右半段排序*/ }}//产生中间数int Partition(int *b,int *a,int p,int r){int k,m,y,i=p,j=r+1;int x=a[p];y=b[p];while(1){while(a[++i]<x);while(a[--j]>x);if(i>=j)break;else{k=a[i];a[i]=a[j];a[j]=k;m=b[i];b[i]=b[j];b[j]=m;}a[p]=a[j];b[p]=b[j];a[j]=x;b[j]=y;return j;}//贪心算法实现活动安排void GreedySelector(int n,int *s,int *f,int *A){//用集合A来存储所选择的活动A[1]=TURE; //默认从第一次活动开始执行int j=1; //j记录最近一次加入到A中的活动for(int i=2;i<=n;i++){//f[j]为当前集合A中所有活动的最大结束时间//活动i的开始时间不早于最近加入到集合A中的j的时间f[j]if(s[i]>=f[j]){A[i]=TURE; //当A[i]=TURE时,活动i在集合A中j=i;}else A[i]=FALSE; }}四、运行结果五、实验小结贪心算法总是做出在当前看来最好的选择,也就是说贪心算法并不从整体最优考虑,它所作出的选择只是在某种意义上的局部最优选择。
贪心算法 找零钱问题
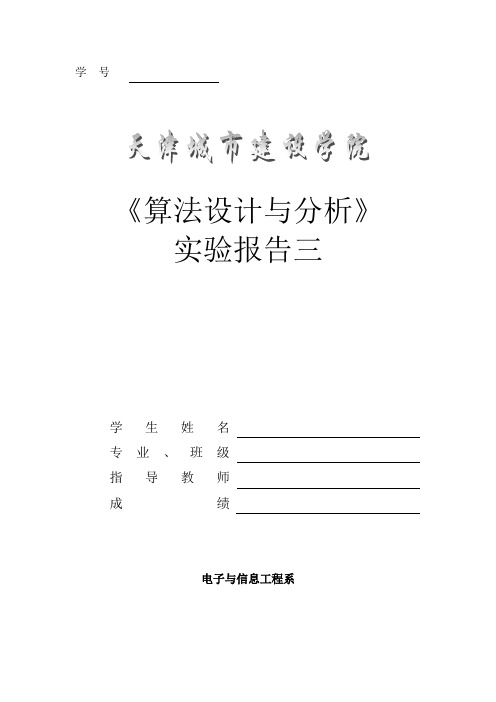
学号《算法设计与分析》实验报告三学生姓名专业、班级指导教师成绩电子与信息工程系实验三:贪心算法运用练习一、实验目的本次实验是针对贪心算法运用的算法设计及应用练习,旨在加深学生对该部分知识点的理解,提高学生运用该部分知识解决问题的能力。
二、实验步骤与要求1.实验前复习课程所学知识以及阅读和理解指定的课外阅读材料;2.学生独自完成实验指定内容;3.实验结束后,用统一的实验报告模板编写实验报告。
4.提交说明:(1)电子版提交说明:a 需要提交Winrar压缩包,文件名为“《算法设计与分析》实验二_学号_姓名”,如“《算法设计与分析》实验二_09290101_张三”。
b 压缩包内为一个“《算法设计与分析》实验二_学号_姓名”命名的顶层文件夹,其下为两个文件夹,一个文件夹命名为“源程序”,另一个文件夹命名为“实验报告电子版”。
其下分别放置对应实验成果物。
(2)打印版提交说明:a 不可随意更改模板样式。
b 字体:中文为宋体,大小为10号字,英文为Time New Roman,大小为10号字。
c 行间距:单倍行距。
(3)提交截止时间:2012年12月7日16:00。
三、实验项目1.传统的找零钱问题的算法及程序实现。
2.特殊的0-1背包问题的求解:本次求解的0-1背包问题的特点为每种物品各有M件,已知每个物品的单位价值,求使得所获价值最大的装包方案。
四、实验过程找零钱问题:#include<iostream>using namespace std;void Zl(double num){int leave=0;int a[8];leave = (int)(num*10)%10;a[1] = leave/5;a[0] = (leave%5)/1;a[7] = num/50;a[6] = ((int)num%50)/20;a[5] = (((int)num%50)%20)/10;a[4] = ((((int)num%50)%20)%10)/5;a[3] = (((((int)num%50)%20)%10)%5)/2;a[2] = ((((((int)num%50)%20)%10)%5)%2)/1;if(a[0]!=0)cout<<"需要找的0.1元个数为:"<<a[0]<<endl;if(a[1]!=0)cout<<"需要找的0.5元个数为:"<<a[1]<<endl;if(a[2]!=0)cout<<"需要找的1元个数为:"<<a[2]<<endl;if(a[3]!=0)cout<<"需要找的2元个数为:"<<a[3]<<endl;if(a[4]!=0)cout<<"需要找的5元个数为:"<<a[4]<<endl;if(a[5]!=0)cout<<"需要找的10元个数为:"<<a[5]<<endl;if(a[6]!=0)cout<<"需要找的20元个数为:"<<a[6]<<endl;if(a[7]!=0)cout<<"需要找的50元个数为:"<<a[7]<<endl;}void main (){double num;// intcout<<"请输入你需要找的零钱数:"<<endl;cin>>num;Zl(num);cout<<endl;return;}五、实验总结。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
实验3.贪心算法一、实验目的1.理解贪心算法的基本思想。
2.运用贪心算法解决实际问题。
二、实验环境与地点1.实验环境:Windows7,Eclipse2.实验地点:网络工程实验室三、实验内容与步骤编写程序完成下列题目,上机调试并运行成功。
1.活动安排问题。
问题:有n个活动的集合A={1,2,…,n},其中每个活动都要求使用同一资源,如演讲会场等,而在同一时间内只有一个活动能使用这一资源。
求解:安排尽量多项活动在该场地进行,即求A的最大相容子集。
设待安排的11个活动的开始时间和结束时间按结束时间的升序排列如下:将此表数据作为实现该算法的测试数据。
(1)给出算法基本思想;(2)给出用java语言实现程序的代码;算法:public static int greedySelector(int[] s, int[] f, boolean a[]) {int n = s.length - 1;a[1] = true;int j = 1;int count = 1;for (int i = 2; i <= n; i++) {if (s[i] >= f[j]) {a[i] = true;j = i;count++;} elsea[i] = false;}return count;}2.哈夫曼编码是广泛地用于数据文件压缩的十分有效的编码方法。
统计字符串中各个字符出现的频率,求各个字符的哈夫曼编码方案。
输入:good good study,day day up输出:各字符的哈夫曼编码。
算法:算法中用到的类Huffman定义为:private static class Huffman implements Comparable {Bintree tree;float weight;// 权值private Huffman(Bintree tt, float ww) {tree = tt;weight = ww;}public int compareTo(Object x) {float xw = ((Huffman) x).weight;if (weight < xw)return -1;if (weight == xw)return 0;return 1;}}算法huffmanTree描述如下:public static Bintree huffmanTree(float[] f) {// 生成单结点树int n = f.length;Huffman[] w = new Huffman[n + 1];Bintree zero = new Bintree();for (int i = 0; i < n; i++) {Bintree x = new Bintree();x.makeTree(new MyInteger(i), zero, zero);w[i + 1] = new Huffman(x, f[i]);}// 建优先队列MinHeap H = new MinHeap();H.initialize(w, n);// 反复合并最小频率树for (int i = 1; i < n; i++) {Huffman x = (Huffman) H.removeMin();Huffman y = (Huffman) H.removeMin();Bintree z = new Bintree();z.makeTree(null, x.tree, y.tree);Huffman t = new Huffman(z, x.weight + y.weight);H.put(t);}return ((Huffman) H.removeMin()).tree;}提示:统计一个字符串中每个字符出现的次数思路:1.创建一个map key:出现的字符value:出现的次数2.获取字符串中的每一个字符3.查看字符是否在Map中作为key存在否,若存在,说明已经统计过则value+1 不存在:value=1代码如下:public class CountString {public static void main(String[] args) {String str = "good good study,day day up";Map<Character, Integer> map = new HashMap<Character, Integer>();for (int i = 0; i < str.length(); i++) {char c = str.charAt(i);// 用toCharArray()也可以if (map.containsKey(c)) {// 若统计过map.put(c, map.get(c) + 1);} else {map.put(c, 1);}}System.out.println(map);}}四、实验总结与分析参考代码:1.ActivityArrangementpublic class AvtivityArrangement {public static int[] s= { 0,1, 3, 0, 5, 3, 5, 6, 8, 8, 2, 12};public static int[] f= { 0,4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14};public static boolean[] a=new boolean[s.length];public static int greedySelector(int[] s, int[] f, boolean[] a) {int n = s.length - 1;a[1] = true;int j = 1;int count = 1;for (int i = 2; i <= n; i++) {if (s[i] >= f[j]) {a[i] = true;j = i;count++;} elsea[i] = false;}return count;}public static void main(String[] args) {// TODO Auto-generated method stubSystem.out.print("相容活动共有:"+greedySelector(s, f, a)+"个:");for (int i = 1; i < a.length; i++) {if (a[i]) {System.out.print(i+" ");}}}}2.Huffmanpublic class BtNode {Object data;BtNode lChild;BtNode rChild;public BtNode() {// 构造一个空节点this(null);}public BtNode(Object data) {// 构造左右孩子域为空的节点this(data,null,null);}public BtNode(Object data, BtNode lchild, BtNode rchild) {// TODO Auto-generated constructor stubthis.data=data;this.lChild=lchild;this.rChild=rchild;}}public class Bintree {BtNode root;public Bintree() {root=null;}public Bintree(BtNode root) {this.root=root;}public Bintree(Object data, BtNode lchild, BtNode rchild) { BtNode node=new BtNode(data, lchild, rchild);this.root=node;}}import java.util.Arrays;import java.util.HashMap;import java.util.List;import java.util.Map;import java.util.PriorityQueue;import java.util.Scanner;public class Huffman implements Comparable {Bintree tree;float weight;// 权值public static Object[]c;private Huffman(Bintree tt, float ww) {tree = tt;weight = ww;}public int compareTo(Object x) {float xw = ((Huffman) x).weight;if (weight < xw)return -1;if (weight == xw)return 0;return 1;}public static Bintree huffmanTree(int[] f) {// 生成单结点树int n = f.length;Huffman[] w = new Huffman[n ];Bintree zero = new Bintree();for (int i = 0; i < n; i++) {Bintree x = new Bintree(new Integer(i),null, null );//null, nullw[i] = new Huffman(x, f[i]);}// 建优先队列List list=Arrays.asList(w);PriorityQueue H = new PriorityQueue(list);System.out.println(H);//* 反复合并最小频率树*/for (int i = 1; i < n; i++) {Huffman x = (Huffman) H.poll();Huffman y = (Huffman) H.poll();Bintree z = new Bintree(null, x.tree.root, y.tree.root);Huffman t = new Huffman(z, x.weight + y.weight);H.offer(t);}return ((Huffman) H.poll()).tree;}public static void CountString(String str ,Map<Character, Integer> map) { for (int i = 0; i < str.length(); i++) {char c = str.charAt(i);// 用toCharArray()也可以if (map.containsKey(c)) {// 若统计过map.put(c, map.get(c) + 1);} else {map.put(c, 1);}}System.out.println(map);}public static void main(String[] args) {Map<Character, Integer> map = new HashMap<Character, Integer>();Scanner scanner=new Scanner(System.in);String string=scanner.nextLine();//输入字符串CountString(string ,map);//统计输入字符串的字符频率//得到字符数组和对应的频率数组c=map.keySet().toArray();int []f=new int[c.length];for (int i = 0; i < c.length; i++) {f[i]=map.get(c[i]);System.out.println(c[i]+":"+f[i]);}//创建huffman树Bintree huf=huffmanTree(f);//输出huffman编码String str="";traverseHuf(huf.root,str);}private static void traverseHuf(BtNode huf,String string) {String str1=""+string;if (huf.lChild==null) {System.out.println(c[(int) huf.data]+":"+str1);}else {traverseHuf( huf.lChild,str1+"0");traverseHuf( huf.rChild,str1+"1");}}}运行结果:good{d=1, g=1, o=2}o:0d:10g:11。