实验指针参考标准答案
材料力学实验报告参考答案(标准版)
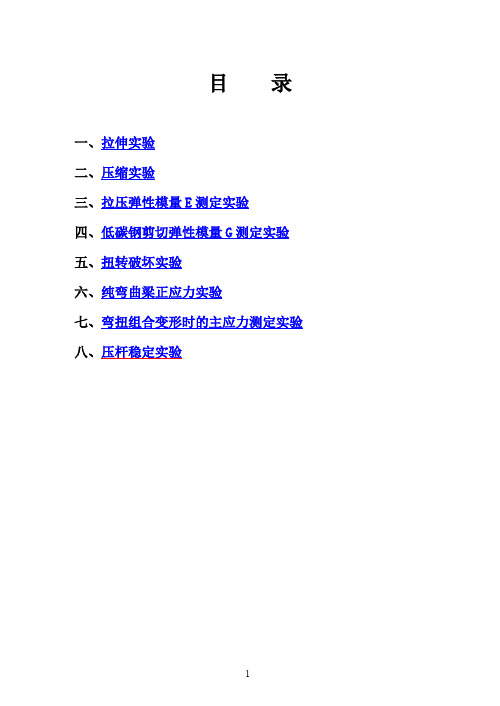
目录一、拉伸实验二、压缩实验三、拉压弹性模量E测定实验四、低碳钢剪切弹性模量G测定实验五、扭转破坏实验六、纯弯曲梁正应力实验七、弯扭组合变形时的主应力测定实验八、压杆稳定实验一、拉伸实验报告标准答案实验目的:见教材。
实验仪器见教材。
实验结果及数据处理:例:(一)低碳钢试件试验前试验后最小平均直径d=10.14mm 最小直径d= 5.70mm 截面面积A=80.71mm 2截面面积A 1=25.50mm 2计算长度L=100mm计算长度L 1=133.24mm试验前草图试验后草图强度指标:P s =__22.1___KN 屈服应力σs =P s /A __273.8___MP a P b =__33.2___KN 强度极限σb =P b /A __411.3___MP a塑性指标:1L -L100%Lδ=⨯=伸长率33.24%1100%A A Aψ-=⨯=面积收缩率68.40%低碳钢拉伸图:(二)铸铁试件试验前试验后最小平均直径d=10.16mm最小直径d=10.15mm截面面积A=81.03mm2截面面积A1=80.91mm2计算长度L=100mm计算长度L1≈100mm 试验前草图试验后草图强度指标:最大载荷Pb=__14.4___KN强度极限σb =Pb/A=_177.7__M Pa问题讨论:1、为何在拉伸试验中必须采用标准试件或比例试件,材料相同而长短不同的试件延伸率是否相同?答:拉伸实验中延伸率的大小与材料有关,同时与试件的标距长度有关.试件局部变形较大的断口部分,在不同长度的标距中所占比例也不同.因此拉伸试验中必须采用标准试件或比例试件,这样其有关性质才具可比性.材料相同而长短不同的试件通常情况下延伸率是不同的(横截面面积与长度存在某种特殊比例关系除外).2、分析比较两种材料在拉伸时的力学性能及断口特征.答:试件在拉伸时铸铁延伸率小表现为脆性,低碳钢延伸率大表现为塑性;低碳钢具有屈服现象,铸铁无.低碳钢断口为直径缩小的杯锥状,且有450的剪切唇,断口组织为暗灰色纤维状组织。
电工学实验教程答案
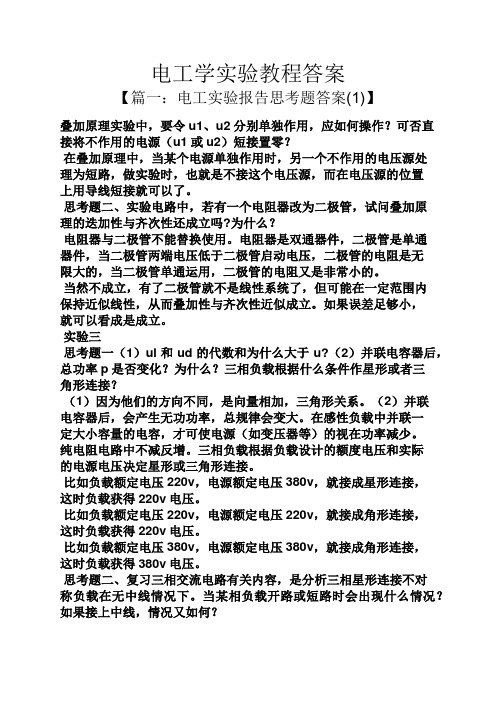
电工学实验教程答案【篇一:电工实验报告思考题答案(1)】叠加原理实验中,要令u1、u2分别单独作用,应如何操作?可否直接将不作用的电源(u1或u2)短接置零?在叠加原理中,当某个电源单独作用时,另一个不作用的电压源处理为短路,做实验时,也就是不接这个电压源,而在电压源的位置上用导线短接就可以了。
思考题二、实验电路中,若有一个电阻器改为二极管,试问叠加原理的迭加性与齐次性还成立吗?为什么?电阻器与二极管不能替换使用。
电阻器是双通器件,二极管是单通器件,当二极管两端电压低于二极管启动电压,二极管的电阻是无限大的,当二极管单通运用,二极管的电阻又是非常小的。
当然不成立,有了二极管就不是线性系统了,但可能在一定范围内保持近似线性,从而叠加性与齐次性近似成立。
如果误差足够小,就可以看成是成立。
实验三思考题一(1)ul和ud的代数和为什么大于u?(2)并联电容器后,总功率p是否变化?为什么?三相负载根据什么条件作星形或者三角形连接?(1)因为他们的方向不同,是向量相加,三角形关系。
(2)并联电容器后,会产生无功功率,总规律会变大。
在感性负载中并联一定大小容量的电容,才可使电源(如变压器等)的视在功率减少。
纯电阻电路中不减反增。
三相负载根据负载设计的额度电压和实际的电源电压决定星形或三角形连接。
比如负载额定电压220v,电源额定电压380v,就接成星形连接,这时负载获得220v电压。
比如负载额定电压220v,电源额定电压220v,就接成角形连接,这时负载获得220v电压。
比如负载额定电压380v,电源额定电压380v,就接成角形连接,这时负载获得380v电压。
思考题二、复习三相交流电路有关内容,是分析三相星形连接不对称负载在无中线情况下。
当某相负载开路或短路时会出现什么情况?如果接上中线,情况又如何?1、当某相负载开路时,就相当于另外两组串联在380v电压下使用,那么电阻大的那组,分得的电压高,如超过其额定电压就会烧毁。
试验检测题库(带答案)
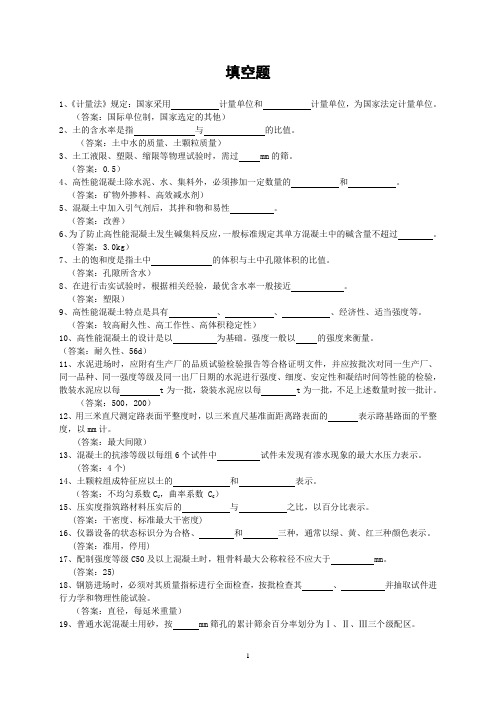
填空题1、《计量法》规定:国家采用计量单位和计量单位,为国家法定计量单位。
(答案:国际单位制,国家选定的其他)2、土的含水率是指与的比值。
(答案:土中水的质量、土颗粒质量)3、土工液限、塑限、缩限等物理试验时,需过 mm的筛。
(答案:0.5)4、高性能混凝土除水泥、水、集料外,必须掺加一定数量的和。
(答案:矿物外掺料、高效减水剂)5、混凝土中加入引气剂后,其拌和物和易性。
(答案:改善)6、为了防止高性能混凝土发生碱集料反应,一般标准规定其单方混凝土中的碱含量不超过。
(答案:3.0kg)7、土的饱和度是指土中的体积与土中孔隙体积的比值。
(答案:孔隙所含水)8、在进行击实试验时,根据相关经验,最优含水率一般接近。
(答案:塑限)9、高性能混凝土特点是具有、、、经济性、适当强度等。
(答案:较高耐久性、高工作性、高体积稳定性)10、高性能混凝土的设计是以为基础。
强度一般以的强度来衡量。
(答案:耐久性、56d)11、水泥进场时,应附有生产厂的品质试验检验报告等合格证明文件,并应按批次对同一生产厂、同一品种、同一强度等级及同一出厂日期的水泥进行强度、细度、安定性和凝结时间等性能的检验,散装水泥应以每 t为一批,袋装水泥应以每 t为一批,不足上述数量时按一批计。
(答案:500,200)12、用三米直尺测定路表面平整度时,以三米直尺基准面距离路表面的表示路基路面的平整度,以mm计。
(答案:最大间隙)13、混凝土的抗渗等级以每组6个试件中试件未发现有渗水现象的最大水压力表示。
(答案:4个)14、土颗粒组成特征应以土的和表示。
(答案:不均匀系数C U,曲率系数 C C)15、压实度指筑路材料压实后的与之比,以百分比表示。
(答案:干密度、标准最大干密度)16、仪器设备的状态标识分为合格、和三种,通常以绿、黄、红三种颜色表示。
(答案:准用,停用)17、配制强度等级C50及以上混凝土时,粗骨料最大公称粒径不应大于 mm。
(答案:25)18、钢筋进场时,必须对其质量指标进行全面检查,按批检查其、并抽取试件进行力学和物理性能试验。
电气试验9(9)
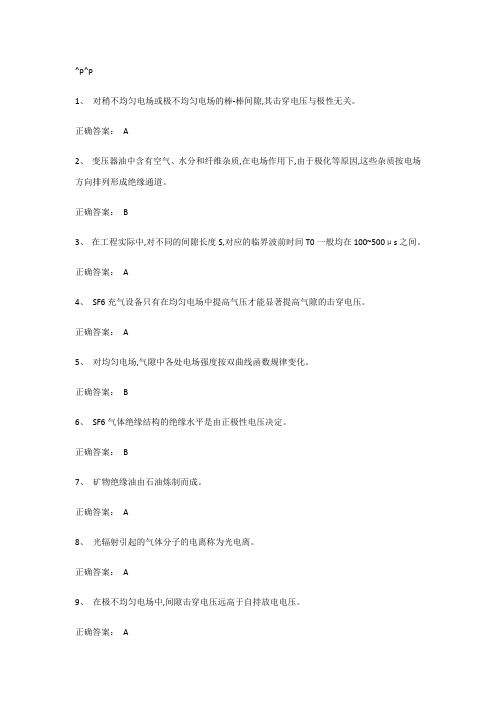
^p^p1、对稍不均匀电场或极不均匀电场的棒-棒间隙,其击穿电压与极性无关。
正确答案: A2、变压器油中含有空气、水分和纤维杂质,在电场作用下,由于极化等原因,这些杂质按电场方向排列形成绝缘通道。
正确答案: B3、在工程实际中,对不同的间隙长度S,对应的临界波前时间T0一般均在100~500μs之间。
正确答案: A4、SF6充气设备只有在均匀电场中提高气压才能显著提高气隙的击穿电压。
正确答案: A5、对均匀电场,气隙中各处电场强度按双曲线函数规律变化。
正确答案: B6、SF6气体绝缘结构的绝缘水平是由正极性电压决定。
正确答案: B7、矿物绝缘油由石油炼制而成。
正确答案: A8、光辐射引起的气体分子的电离称为光电离。
正确答案: A9、在极不均匀电场中,间隙击穿电压远高于自持放电电压。
正确答案: A10、固体电介质电击穿的击穿场强与电场均匀程度密切相关。
正确答案: A11、流注从空气间隙的阳极向阴极发展,称为阳极流注。
正确答案: A12、在直流电压作用下的棒-板间隙,板极附近的电场强度最大。
正确答案: B13、研究表明,长空气间隙的操作冲击击穿通常发生在波结束时刻。
正确答案: B14、直流击穿电压与工频交流击穿电压的幅值接近相等。
正确答案: A15、ZC-7型绝缘电阻表由手摇小发电机和比率型磁电系测量机构两部分组成。
正确答案: A16、通过手摇(或电动)直流发电机或交流发电机经倍压整流后输出直流电压作为电源的绝缘电阻表机型称为发电机型。
正确答案: A17、双臂电桥是在单臂电桥的基础上增加特殊机构,以消除测试时电池电压降低对测量结果的影响。
正确答案: B18、绝缘电阻表的负载特性曲线,当被测绝缘电阻阻值降低时,绝缘电阻的端电压快速升高。
正确答案: B19、测量吸收比,应分别读取绝缘电阻表15s和60s时的绝缘电阻值。
正确答案: A20、电气试验中,测量电气设备的直流电阻一般采用钳形电流表。
正确答案: B21、直流电阻速测仪测量结束后,可以马上拆除试验接线。
2023年电气试验备考押题2卷合1带答案34
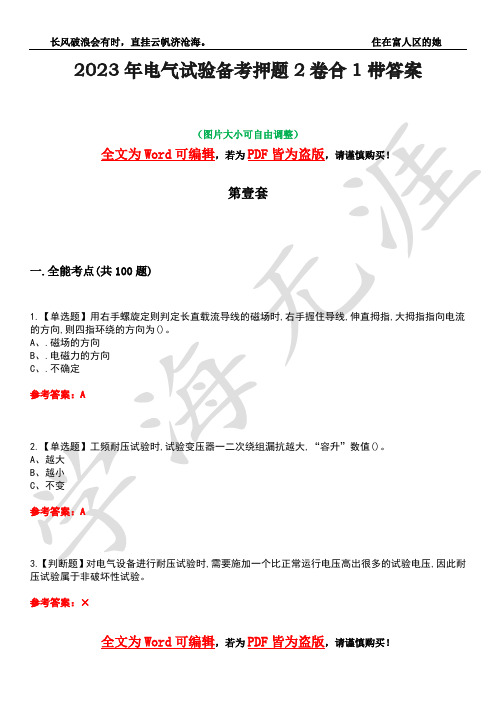
2023年电气试验备考押题2卷合1带答案(图片大小可自由调整)全文为Word可编辑,若为PDF皆为盗版,请谨慎购买!第壹套一.全能考点(共100题)1.【单选题】用右手螺旋定则判定长直载流导线的磁场时,右手握住导线,伸直拇指,大拇指指向电流的方向,则四指环绕的方向为()。
A、.磁场的方向B、.电磁力的方向C、.不确定参考答案:A2.【单选题】工频耐压试验时,试验变压器一二次绕组漏抗越大,“容升”数值()。
A、越大B、越小C、不变参考答案:A3.【判断题】对电气设备进行耐压试验时,需要施加一个比正常运行电压高出很多的试验电压,因此耐压试验属于非破坏性试验。
参考答案:×4.【判断题】在绝缘试验时,通过测量介质损耗角的正切值tanδ来检验介质损耗的大小。
参考答案:√5.【判断题】当绝缘内的缺陷不是整体分布性的,而是集中在某一小点,介质损耗正切值tanδ对这类局部缺陷的反应不够灵敏。
参考答案:√6.【判断题】电气设备在进行大修后,或者在进行局部改造后,要进行检修后试验,试验应执行电力行业标准《电气设备预防性试验规程》中规定的大修、小修试验项目和试验合格标准。
参考答案:√7.【单选题】在对称三相负载三角形连接中,线电流与相电流在相位上相差()。
A、.60°B、.30°C、.120°参考答案:B8.【单选题】规程规定,变压器的变压比误差是不允许超过()。
A、.+0.5%-+2%B、.+1%-+2%C、.+0.5%-+1%参考答案:C9.【判断题】直流电压作用下流过绝缘介质的电流,其电流可视为由电容充电电流吸收电流和泄漏电流三部分组成。
参考答案:√10.【单选题】利用直流升压装置产生一个可以调节的试验用直流高压,通过施加规程规定的直流试验电压和耐压时间来考核被试品的耐电强度的试验称为()。
A、.直流耐压试验B、.直流泄漏电流测量C、.交流耐压试验参考答案:A11.【单选题】对油浸式变压器及电抗器的绝缘试验应在充满合格油,并静止一定时间,待气泡消除后进行,一般来说设备额定电压为220kV及330kV时,静止时间要求()。
电工作业-电气试验作业(有答案)

电工作业-电气试验作业一、判断题1、高压试验时,当间隙作为测量间隙使用时,保护电阻的阻值与试验电压的频率有关,工频试验电压时测量球隙的保护电阻按0.1~0.5Ω/V选取。
(X)2、局部放电试验的目的,主要是在规定的试验电压下检测局部放电量是否超过规程允许的数值。
(√)3、在交流耐压试验时利用被试变压器本身一、二次绕组之间的电磁感应原理产生高压对自身进行的耐压试验称为感应耐压试验。
(√)4、测量电气设备介质损耗角正切值tanδ时,消除电场干扰影响的方法中使用移相器改变试验电压的相位,在试验电流与干扰电流相同和相反两种情况下分别测量tanδ的方法称为移相法。
(√)5、规程规定,对于220kV及以上且容量为200MVA及以上的电力变压器均应测量极化指数。
(X)6、为了防止电气设备在投入运行时或运行中发生事故,必须对电气设备进行高压试验,以便及时发现设备中潜伏的缺陷。
(√)7、高压试验结東后,试验报告除交付被试设备所属单位收存外,还应上报调度。
(X)8、电气设备直流泄漏电流的测量,采用万用表测量其大小。
(X)9、电气试验中,防止误接线就是要做到试验接线正确。
(√)10、电气装置的金属外壳、配电装置的构架和线路杆塔等,由于绝缘损坏有可能带电,为防止其危及人身和设备的安全而设的接地称为工作接地。
(X)11、电气设备在运行时可能承受过电压,因此除了必须按规定采取过电压保护措施外,同时电气设备必须具备规定的机械强度。
(X)12、直流电压作用下流过绝缘介质的电流,吸收电流是衰减缓慢的有损耗极化电流。
(√)13、通常采用绝缘摇表来测量电气设备的绝缘电阻。
(√)14、当试品绝缘中存在气隙时,随着试验电压U的变化,tanδ值呈闭合的环状曲线。
(√)15、高压试验中,特性试验是指对电气设备绝缘状况的检查试验。
(X)16、采用西林电桥测量介质损耗角正切值时,通过调节可调电阻R和可变电容C使检流计指零,电桥平衡,这时可变电容C指示的电容量(取μF)值就是被试设备的tanδ值。
汽车试验技术课后习题参考答案
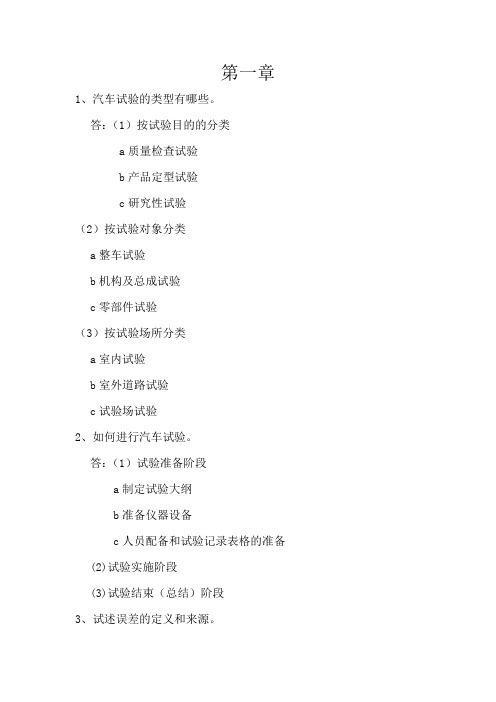
第一章1、汽车试验的类型有哪些。
答:(1)按试验目的的分类a质量检查试验b产品定型试验c研究性试验(2)按试验对象分类a整车试验b机构及总成试验c零部件试验(3)按试验场所分类a室内试验b室外道路试验c试验场试验2、如何进行汽车试验。
答:(1)试验准备阶段a制定试验大纲b准备仪器设备c人员配备和试验记录表格的准备(2)试验实施阶段(3)试验结束(总结)阶段3、试述误差的定义和来源。
答:误差是测量值与被测量之间的差。
主要有:a装置误差b方法误差c环境误差d人员误差4、系统误差的分类和消除方法有哪些。
答:(1)根据系统误差变化与否分类a恒值系统误差b变值系统误差(2)按误差产生的原因分类a工具误差b装置误差c 环境误差d方法误差e人员误差(3)根据误差的变化规律分类常值性的,累进性的、周期性的以及按复杂规律变化的系统误差系统误差的消除(1)固定不变的系统误差消除方法a代替法b交换法(2)线性系统误差消除方法对称测量法(3)周期性变换的系统误差消除方法每隔半个周期进行一次测量,取两次读数的平均值作为测量值,则可消除周期性误差。
5、试述随机误差的分布和消除方法。
答:a分布:呈正态分布b消除方法:消除趋势项c算术平均值,无限接近被测量的真值6、试述粗大误差的来源和消除方法。
答:①来源:a测量人员:由于测量者错误的读数和错误的记录造成的。
b客观外界条件:由于测量意外的改变(如外界振动)②引起仪器示值或被测对象位置的改变而产生粗大误差。
a消除方法:对于出大误差,除了设法从测量结果中发现和鉴别而加以剔除外;b此外,可采用不等精度测量和互相之间进行校核的方法3ð准则(莱以特准则)、罗曼诺夫斯基准则7、介绍回归分析处理数据的方法。
答:a一元线性回归b一元非线性回归c多元线性回归8、试述动态试验数据处理的方法。
1)数据分析2)数据准备3)数据性质检验4)数据分析类型9、试述试验数据修约的规则。
答:确定修约位数的表达方式a进舍规则b不许连续修约8、汽车道路试验的最大特点是什么?在汽车的道路试验中如何保证试验结果的真实性、重复性、可比性?试述试验前试验车辆应进行准备工作的内容答:⑪汽车道路试验的最大特点是接近实际使用情况。
密度试验专题(有答案)
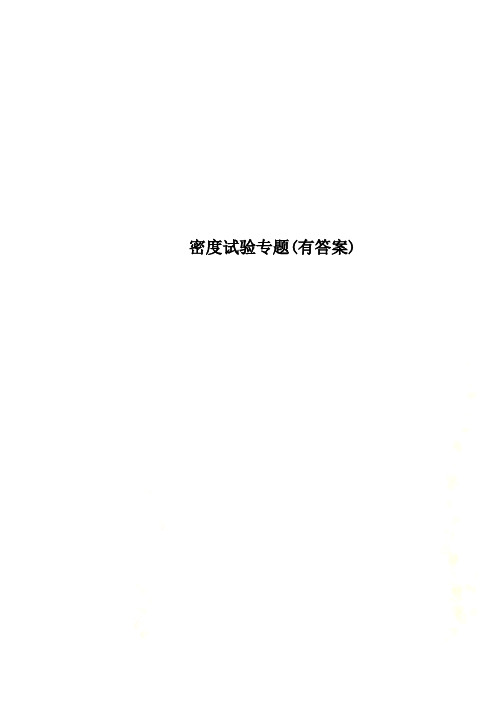
密度试验专题(有答案)密度实验1.在探究质量与体积的关系时,小明找来大小不同的塑料块和某种液体做实验.(1)如图甲是小明在水平桌面上使用托盘天平的情景,他的错误是.___________(2)改正错误后,小明正确操作,根据实验数据分别画出了塑料块和液体质量随体积变化的图像,如图乙所示.①分析图像可知,同种物质的不同物体,其质量与体积的比值___________(填“相同”或“不同”),物理学中将质量与体积的比值定义为密度,塑料的密度为___________kg/m3.②往烧杯内倒入10 cm3的液体,如图丙所示,用天平称得烧杯和液体的总质量为___________g,若烧杯内液体的体积为20 c m3,则烧杯和液体的总质量应为___________g.3.在测量小石块密度的实验中,(1)如图甲所示,小明在使用天平过程中的错误是:_____________.(2)小明纠正错误后继续实验,天平平衡时,右盘中所加砝码和游码的位置如图乙所示,再把小石块放入装有10mL水的量筒中,量筒内液面的位置如图丙所示,请你为小明设计实验表格,并填入数据,计算出小石块的密度_____________.4.学校研究性学习小组,测量一种矿石的密度.现有器材;小矿石块、天平(含砝码)、一个烧杯、足量的水、细线.小组同学们设计了一种测量矿石密度的方案如下:(1)调节天平平衡后,小明用天平测量矿石块的质量,如图,指出实验操作中的错误:______ .改正错误后,小明用正确的方法称矿石块的质量,平衡时放在盘中的砝码和游码在标尺上的位置如图,则矿石块的质量m为_________g;(2)在烧杯中倒满水,称出烧杯和水的总质量m1;(3)将矿石块轻轻放入装满水的烧杯中,矿石块沉入杯底,烧杯溢出水后,将烧杯壁外的水擦干净,放在天平左盘上,称出此时烧杯、杯内矿石和水的总质量m2,则溢出水的质量是__________(写表达式),V矿石=________(写表达式)。
工地试验室 试验考题及其答案(独家)

一判断题1.GB50164-2011《混凝土质量控制标准》适用于建设工程的混凝土质量控制。
()2.混凝土强度等级是按立方体抗压强度标准值(MPa)来划分的。
()3.在JGJ55-2011中规定“大体积、钢筋混凝土中胶凝材料使用普通硅酸盐水泥+复合掺合料、水胶比≤0.4时,复合掺合料的最大掺量为55%。
()4.混凝土拌合物中水溶性氯离子含量是指采用《水运工程混凝土试验规程》JTJ270中混凝土拌合物中氯离子含量快速测定值与水泥用量的质量百分比。
()5.基准水泥是指检验混凝土外加剂性能的专用水泥,是有硅酸盐水泥与二水石膏共同粉磨而成的42.5强度等级的P.I型硅酸盐水泥,其熟料中铝酸三钙C3A含量在6~8%、硅酸三钙C3S含量在55~60%、游离氧化钙fCaO含量不得超过1.2%,水泥中碱含量不得超过1.0%、比表面积在350±10m2/kg。
()6.高性能减水剂比高效减水剂具有更高的减水率、更好的塌落度保持性能、较小的干燥收缩,且具有一定的引气性能的减水剂。
()7.GB8076-2008《混凝土外加剂》中要求外加剂检验时混凝土试件的成型温度为20±5℃,预养温度为20±2℃。
()8.水泥凝结时间试验中,到达初凝时应立即重复一次,两次结论相同时才能确定到达初凝状态,到达终凝时,需要在试体另外两个不同点测试,结论相同时才能确定到达终凝状态。
()9.GB/T14685-2011《建设用卵石、碎石》标准适用于建设工程中水泥混凝土及其制品用卵石、碎石。
()10.混凝土拌合用水检验时,试验水样应与饮用进行胶砂强度比对试验,其配制的水泥胶砂3d和28d强度不应低于饮用水配制的水泥胶砂3d和28d强度的90%。
()11.用于水泥混凝土中的粉煤灰需水量比和矿渣粉流动度比试验用砂均为0.5mm~1.0mm 的中级砂。
()12.击实试验中,5个不同含水率的试样应有2个小于塑限、2个大于塑限和1个接近塑限。
2023年电气试验电工作业(复审)模拟考试题库试卷五(100题,含答案)

2023年电气试验电工作业(复审)模拟考试题库试卷五(IoO题,含答案)1、(判断题)采用ZC-8型接地电阻表测量接地电阻,一般情况下电压极、电流极和被测接地极三者之间成直线布置。
参考答案:正确2、(判断题)导体处于变化的磁场中时,导体内会产生感应电动势。
参考答案:正确3、(判断题)对电极形状不对称的棒-板间隙,当棒为负极时,间隙的直流击穿电压要远低于棒为正极时的直流击穿电压。
参考答案:错误4、(判断题)一般认为,电力变压器绕组tan8测试时的温度以10~40。
C为宜。
参考答案:正确5、(判断题)对于直流电流,在单位时间内通过导体横截面的电量是恒定不变的。
参考答案:正确6、(判断题)在外电场的作用下,原子中的电子运行轨道发生了变形位移,电子负电荷的作用中心与原子核的正电荷不再重合,形成电矩。
参考答案:正确7、(判断题)当电源内阻为零时,电源端电压等于电源电动势。
参考答案:正确8、(判断题)在电气施工中,必须遵守国家有关安全的规章制度,安装电气线路时应根据实际情况以方便使用者的原则来安装。
参考答案:错误9、(判断题)绝缘电阻表用来测量电气设备的绝缘中的功率损耗。
参考答案:错误10、(判断题)电阻分压式变比电桥测量变压比,测量中需调节可变电阻使检流计为零。
参考答案:正确11、(判断题)在电阻并联的电路中,电路总电阻的倒数等于各并联电阻的倒数之和。
参考答案:正确12、(判断题)采用QS1电桥测量tanδ的接线方式一般有差接线及和接线。
参考答案:错误13、(判断题)电气设备预防性试验报告等资料应技术交流,作为日后电气设备安排大、小修计划时的参考依据。
参考答案:错误14、(判断题)电流通过接地装置流入地中,接地电阻数值主要由接地体附近约20m半径范围内的电阻决定。
参考答案:正确15、(判断题)工频耐压试验时,接上试品后接通电源,开始进行升压试验时升压必须从零(或接近于零)开始。
参考答案:正确16、(判断题)QS1电桥内有一套低压电源和低压标准电容器,可测量电容量为300PF~100uF的试品。
2023年电气试验高频考点训练2卷合壹-8(带答案)

2023年电气试验高频考点训练2卷合壹(带答案)(图片大小可自由调整)全文为Word可编辑,若为PDF皆为盗版,请谨慎购买!第1套一.全能考点(共100题)1.【单选题】为了获得真实的试验数据,试验所用的仪器仪表必须有足够的精确度,为此应定期对这些仪器仪表经专业部门进行()。
A、.精确度检验B、.灵敏度检验C、.过电流检验参考答案:A2.【单选题】tanδ测试时,随着试验电压U的变化,tanδ值呈闭合的环状曲线,则试品属于()。
A、.绝缘中存在气隙B、.良好绝缘C、.绝缘受潮参考答案:A3.【判断题】根据变压器油辛烷值的不同,可分为10、25和45三个牌号。
参考答案:×4.【单选题】在标准大气压下,温度为200C时,均匀电场中空气间隙的击穿场强大约为()kV/cm(峰值)。
A、.10B、.30C、.20参考答案:B5.【单选题】在电路中,负载消耗功率等于电源产生的功率与内阻损耗的功率()。
A、.之和B、.之差C、.之积参考答案:B6.【判断题】直流电压作用下流过绝缘介质的电流,其电流可视为由电容充电电流吸收电流和泄漏电流三部分组成。
参考答案:√7.【判断题】当油间隙的距离一定时,如果在这个间隙中用绝缘隔板将这几个间隙分割成几个小间隙,则总的击穿电压将显著提高。
参考答案:√8.【单选题】在电路中,()称为负载,也叫负荷。
A、.使用电能的设备或元器件B、.使用光能的设备或元器件C、.使用热能的设备或元器件参考答案:A9.【单选题】电极间油纸绝缘的厚度和纸层的介电常数对油纸绝缘的击穿场强有影响,油纸绝缘愈厚,电场就愈不均匀,局部放电起始电压就()。
A、.基本不变B、.愈高C、.愈低参考答案:C10.【判断题】测量试品的绝缘电阻时,试品温度宜在10-400C之间。
参考答案:√11.【判断题】固体绝缘材料的击穿电压与电压作用时间有关,如电压作用时间很短就被击穿,则这种击穿很可能是电击穿。
参考答案:√12.【单选题】扑灭火灾时,灭火人员应站在()进行灭火。
深圳低压电工实操考试答案
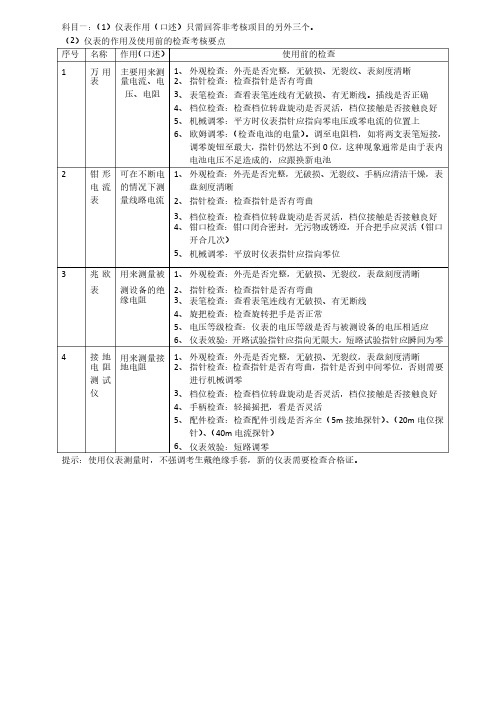
科目一:(1)仪表作用(口述)只需回答非考核项目的另外三个。
)仪表作用(口述)只需回答非考核项目的另外三个。
(2)仪表的作用及使用前的检查考核要点)仪表的作用及使用前的检查考核要点 序号序号 名称名称 作用(口述) 使用前的检查使用前的检查1万用表主要用来测量电流、电压、电阻压、电阻1、 外观检查:外壳是否完整,无破损、无裂纹、表刻度清晰外观检查:外壳是否完整,无破损、无裂纹、表刻度清晰2、 指针检查:检查指针是否有弯曲指针检查:检查指针是否有弯曲3、 表笔检查:查看表笔连线有无破损、有无断线。
插线是否正确表笔检查:查看表笔连线有无破损、有无断线。
插线是否正确4、 档位检查:检查档位转盘旋动是否灵活,档位接触是否接触良好档位检查:检查档位转盘旋动是否灵活,档位接触是否接触良好5、 机械调零:平方时仪表指针应指向零电压或零电流的位置上机械调零:平方时仪表指针应指向零电压或零电流的位置上6、 欧姆调零:(检查电池的电量)。
调至电阻档,如将两支表笔短接,调零旋钮至最大,指针仍然达不到0位,这种现象通常是由于表内电池电压不足造成的,应跟换新电池电池电压不足造成的,应跟换新电池2钳形电流表可在不断电的情况下测量线路电流量线路电流 1、 外观检查:外壳是否完整,无破损、无裂纹、手柄应清洁干燥,表盘刻度清晰盘刻度清晰 2、 指针检查:检查指针是否有弯曲指针检查:检查指针是否有弯曲3、 档位检查:检查档位转盘旋动是否灵活,档位接触是否接触良好档位检查:检查档位转盘旋动是否灵活,档位接触是否接触良好4、 钳口检查:钳口闭合密封,无污物或锈迹,开合把手应灵活(钳口开合几次)开合几次) 5、 机械调零:平放时仪表指针应指向零位机械调零:平放时仪表指针应指向零位3 兆欧表用来测量被测设备的绝缘电阻缘电阻1、 外观检查:外壳是否完整,无破损、无裂纹,表盘刻度清晰外观检查:外壳是否完整,无破损、无裂纹,表盘刻度清晰2、 指针检查:检查指针是否有弯曲指针检查:检查指针是否有弯曲3、 表笔检查:查看表笔连线有无破损、有无断线表笔检查:查看表笔连线有无破损、有无断线4、 旋把检查:检查旋转把手是否正常旋把检查:检查旋转把手是否正常5、 电压等级检查:仪表的电压等级是否与被测设备的电压相适应电压等级检查:仪表的电压等级是否与被测设备的电压相适应6、 仪表效验:开路试验指针应指向无限大,短路试验指针应瞬间为零短路试验指针应瞬间为零 4接地电阻测试仪用来测量接地电阻地电阻1、 外观检查:外壳是否完整,无破损、无裂纹,表盘刻度清晰外观检查:外壳是否完整,无破损、无裂纹,表盘刻度清晰2、 指针检查:检查指针是否有弯曲,检查指针是否有弯曲,指针是否到中间零位,指针是否到中间零位,指针是否到中间零位,否则需要否则需要进行机械调零进行机械调零 3、 档位检查:检查档位转盘旋动是否灵活,档位接触是否接触良好档位检查:检查档位转盘旋动是否灵活,档位接触是否接触良好 4、 手柄检查:轻摇摇把,看是否灵活手柄检查:轻摇摇把,看是否灵活 5、 配件检查:检查配件引线是否齐全(5m 接地探针)、(20m 电位探针)、(40m 电流探针)电流探针)6、 仪表效验:短路调零仪表效验:短路调零提示:使用仪表测量时,不强调考生戴绝缘手套,新的仪表需要检查合格证。
材料力学实验报告标准答案
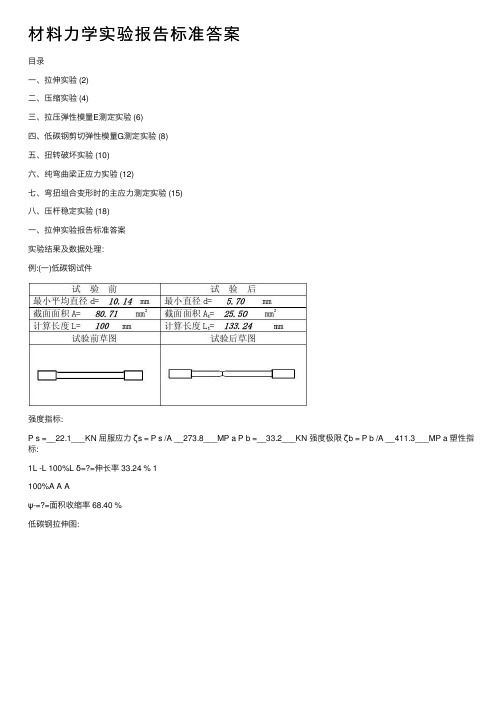
材料⼒学实验报告标准答案⽬录⼀、拉伸实验 (2)⼆、压缩实验 (4)三、拉压弹性模量E测定实验 (6)四、低碳钢剪切弹性模量G测定实验 (8)五、扭转破坏实验 (10)六、纯弯曲梁正应⼒实验 (12)七、弯扭组合变形时的主应⼒测定实验 (15)⼋、压杆稳定实验 (18)⼀、拉伸实验报告标准答案实验结果及数据处理:例:(⼀)低碳钢试件强度指标:P s =__22.1___KN 屈服应⼒ζs = P s /A __273.8___MP a P b =__33.2___KN 强度极限ζb = P b /A __411.3___MP a 塑性指标:1L -L 100%L δ=?=伸长率 33.24 % 1100%A A Aψ-=?=⾯积收缩率 68.40 %低碳钢拉伸图:(⼆)铸铁试件强度指标:最⼤载荷P b =__14.4___ KN强度极限ζb = P b / A = _177.7__ M P a问题讨论:1、为何在拉伸试验中必须采⽤标准试件或⽐例试件,材料相同⽽长短不同的试件延伸率是否相同?答:拉伸实验中延伸率的⼤⼩与材料有关,同时与试件的标距长度有关.试件局部变形较⼤的断⼝部分,在不同长度的标距中所占⽐例也不同.因此拉伸试验中必须采⽤标准试件或⽐例试件,这样其有关性质才具可⽐性.材料相同⽽长短不同的试件通常情况下延伸率是不同的(横截⾯⾯积与长度存在某种特殊⽐例关系除外).2、分析⽐较两种材料在拉伸时的⼒学性能及断⼝特征.答:试件在拉伸时铸铁延伸率⼩表现为脆性,低碳钢延伸率⼤表现为塑性;低碳钢具有屈服现象,铸铁⽆.低碳钢断⼝为直径缩⼩的杯锥状,且有450的剪切唇,断⼝组织为暗灰⾊纤维状组织。
铸铁断⼝为横断⾯,为闪光的结晶状组织。
.⼆、压缩实验报告标准答案实验数据记录及处理:例:(⼀)试验记录及计算结果问题讨论:1、分析铸铁试件压缩破坏的原因.答:铸铁试件压缩破坏,其断⼝与轴线成45°~50°夹⾓,在断⼝位置剪应⼒已达到其抵抗的最⼤极限值,抗剪先于抗压达到极限,因⽽发⽣斜⾯剪切破坏。
电能表修校专业技能复习题含参考答案

电能表修校专业技能复习题含参考答案一、单选题(共45题,每题1分,共45分)1.用直流法测量减极性电压互感器,正极接A端钮,负极接X端钮,检测表正极接a端钮,负极接x端钮,在合分开关瞬间检测表指针()方向摆动。
A、分别向正负B、分别向负正C、均向正D、均向负正确答案:A2.照明线路采用三相四线制供电线路,中线必须()。
A、取消或断开B、安装开关以控制其通断C、安装熔断器,防止中线断开D、安装牢靠,防止断开正确答案:D3.RLC串联电路中,如把L增大一倍,C减少到原有电容的1/4,则该电路的谐振频率变为原频率f的()。
A、1.414B、1/2C、2D、4正确答案:A4.智能表报警事件包括()。
A、逆相序电源异常负荷开关误动作等B、失压功率反向(双向表除外)电源异常等C、失压逆相序过载等D、失压逆相序掉电等正确答案:C5.用绝缘电阻表测量电流互感器一次绕组对二次绕组及对地间的绝缘电阻值,如(),不予检定。
A、>500MΩB、≤500MΩC、<500MΩD、≥500MΩ正确答案:C6.用直流法测量减极性电压互感器,正极接X端钮,负极接A端钮,检测表正极接a端钮,负极接x端钮,在合、分开关瞬间检测表指针()方向摆动。
A、分别向正、负B、分别向负、正C、均向正D、均向负正确答案:B7.若电流互感器二次线圈有多级抽头时,其中间抽头的首端极性标志为()。
A、K1B、K2C、K3D、K4正确答案:A8.关于功率因数角的计算,()是正确的A、功率因数角等于有功功率除以无功功率的反余切值B、功率因数角等于有功功率除以无功功率的反余弦值C、功率因数角等于有功功率除以无功功率的反正弦值D、功率因数角等于有功功率除以无功功率的反正切值正确答案:A9.S级电能表与普通电能表的主要区别在于()时的特性不同。
A、最大电流B、低负载C、标定电流D、宽负载正确答案:B10.35kV电能计量柜的电压互感器二次应采用()。
A、两台接成V、v形接线B、三台接成YN、yn形接线C、三台接成Y、y形接线D、三台接成△、Δ形接线正确答案:A11.智能电能表上电完成后()内可以使用RS-485接口进行通讯。
电气试验工考试:初级电气试验工找答案

电气试验工考试:初级电气试验工找答案1、判断题用万用表测量二极管的正向电阻时,应使用红表笔接二极管的正极,黑表笔接负极。
正确答案:错2、单选运行导体中切割磁力线而产生最大电动势时,导体与磁力线间的夹角应为()(江南博哥)。
A.0°B.45°C.60°D.90°正确答案:D3、单选三极管的输出特性曲线可分成三个区域即()。
A.放大区、饱和区、截止区B.放大区、饱和区、短路区C.放大区、导通区、截止区D.放大区、饱和区、断路区正确答案:A4、单选并联电路中,若两个相差很大的电阻并联,可以认为等效电阻近似等于()。
A.大电阻值B.小电阻值C.二电阻值之和D.近似于零欧姆正确答案:B5、单选当线圈中的磁通减小时,感应电流的磁通()。
A.与原磁通方向相同B.与原磁通方向相反C.与磁通方向无关D.无法判断正确答案:A6、单选绕组式转子的三相绕组的联接方式一般采用()联接。
A.星形B.三角形C.星形或三角形D.并联正确答案:A7、单选大型UPS电源的日常维护和检查中,观察和记录系统控制柜上观察UPS电源的三相输出电压值,属于()。
A、每日例行检查B、定期例行维护检查C、临时检查D、随机检查正确答案:A8、问答题常用滤波器有几种?正确答案:常用滤波器有:电容滤波器、电感滤波器、T型滤波器、П型滤波器和阻容滤波器五种.9、判断题磁力线是一组闭合曲线,它既不中断,也不会相交。
正确答案:对10、单选R、L、C串联电路处于谐振状态时,电容C两端的电压等于()。
A、电源电压与电路品质因数Q的乘积;B、电容器额定电压的Q倍;C、无穷大;D、电源电压。
正确答案:A11、问答题多油和少油断路器的主要区别是什么?正确答案:多油断路器的油箱是接地铁箱。
箱内的油既用于灭弧,还作为高压带电体对油箱和同相开断动静触头之间的绝缘(在三相式断路器中还用作不同相高压带电体之间的绝缘),所以其用油量很大,体积也较大。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
C语言程序设计实验教学(8)【实验目的】指针是C语言中非常重要的一章内容。
通过实验让学生掌握各类指针的定义与引用、指针作为函数参数的应用、字符指针的应用、函数指针的应用。
【实验要求】使用指针来完成变量的引用,编写程序将指针作为参数进行传递,理解指针的含义。
【实验课时】10.0【实验内容】1. 上机编程实现用函数来将从键盘上输入的三个数按由小到大的顺序输出。
要求编写自定义函数swap()用于交换两个变量的值;且函数原型为:void swap(int *p1,int *p2);;并在main函数中用指针变量作为实际参数调用函数swap();最后输出排序后的结果。
#include <stdio.h>main(){void swap(int *p1,int *p2);int n1,n2,n3; int *p1,*p2,*p3;printf("Input three integers n1,n2,n3: ");scanf("%d,%d,%d",&n1,&n2,&n3);p1=&n1; p2=&n2; p3=&n3;if(n1>n2) swap(p1,p2);if(n1>n3) swap(p1,p3);if(n2>n3) swap(p2,p3);printf("Now, the order is: %d,%d,%d\n",n1,n2,n3);}void swap(int *p1,int *p2){int p;p=*p1;*p1=*p2;*p2=p;}运行结果如下:Input three integers n1,n2,n3: 34,21,25↙Now, the order is: 21,25,342. 上机编程实现用函数来将从键盘上输入的三个字符串按由小到大的顺序输出。
要求编写自定义函数swap()用于交换两个变量的值;且函数原型为:void swap(char *p1,char *p2);;并在main函数中用字符数组名作为实际参数调用函数swap();最后输出排序后的结果。
#include <stdio.h>#include <string.h>main(){void swap (char *p1,char*p2);char str1[20],str2[20],str3[20];printf("Input three strings:\n");gets(str1); gets(str2); gets(str3);if(strcmp(str1,str2)>0) swap(str1,str2);if(strcmp(str1,str3)>0) swap(str1,str3);if(strcmp(str2,str3)>0) swap(str2,str3);printf("Now, the order is:\n");printf("%s\n%s\n%s\n",str1,str2,str3);}void swap (char *p1,char*p2) /*交换两个字符串*/{ char p[20];strcpy(p,p1); strcpy(p1,p2); strcpy(p2,p);}运行结果如下:Input three lines:I study very hard.↙C language is very interesting.↙He is a professor.↙Now, the order is:C language is very interesting.He is a professor.I study very hard.3. 上机编程实现用函数来返回一维数组a中的最大值。
要求设计一个自定义函数max()函数原型为:int max(int *p,int n);;在main函数中用数组名a和数组的长度n作为实际参数调用函数max();最后输出结果。
int max(int *p,int n){int i,max; max=p[0];for(i=1;i<n;i++)if(max<p[i]) max=p[i]; /*或者用if(max<*(p+i)) max=*(p+i); */return max;}main(){int i,a[6];for(i=0;i<6;i++)scanf("%d",&a[i]);printf("max=%d \n",max(a,6));} /*或者用max(&a[0],6) */4. 上机编程实现从键盘上输入10个整数,将其中最小的数与第一个数对换,把最大的数与最后一个数对换。
请编写3个函数:(1)输入10个数;input()函数原型为:void input(int *);;(2)进行处理;max_min_value()函数原型为:void max_min_value(int *);(3)输出10个数。
output()函数原型为:void output(int *);解:输入输出函数的N-S图见图10.1。
交换函数的N-S图见图10.2。
#include <stdio.h>main(){void input(int *); void max_min_value(int *); void output(int *);int number[10];input(number); max_min_value(number); output(number);}void input(int array[10]){int i;printf("input 10 numbers: ");for(i=0;i<10;i++)scanf("%d",&array[i]);}void max_min_value(int array[10]){int *max,*min,*p,*array_end;array_end=array+10;max=min=array;for(p=array+1;p<array_end;p++)if(*p>*max) max=p;else if(*p<*min) min=p;*p=array[0]; array[0]=*min; *min=*p;*p=array[9];array[9]=*max;*max=*p;}void output(int array[10]){int *p;printf("Now, they are: ");for(p=array;p<=array+9;p++)printf("%d ",*p);}运行结果如下:input 10 numbers: 32 24 56 78 198 36 44 29 6↙Now, they are: 1 24 56 78 326 36 44 29 985.编写一函数,函数原型为:void invert(char *p,int m);,实现将n个数按输入顺序的逆序存放,并输出。
#include <stdio.h>main(){void invert(int *p,int m);int i,n; int *p,num[20];printf("\ninput n: ");scanf("%d",&n);printf("please input these numbers:\n");for(i=0;i<n;i++)scanf("%d",&num[i]);p=&num[0];invert(p,n);printf("Now, the sequence is:\n");for(i=0;i<n;i++)printf("%5d",num[i]);}void invert(int *p,int m){int i; int temp,*p1,*p2;for(i=0;i<m/2;i++){p1=p+i; p2=p+(m-1-i);temp=*p1; *p1=*p2; *p2=temp;}}运行结果:input n: 10↙please input these numbers:10 9 8 7 6 5 4 3 2 1 ↙Now,the sequence is:1 2 3 4 5 6 7 8 9 106.在主函数中输入n个整数,编写一函数move(),是n个整数循环向后移一个位置,函数原型为:void move(int *,int,int);再通过函数的递归调用实现将前面各数顺序向后移m个位置,使得最后m个数变成最前面m个数,(效果见图10.3)。
并在主函数中输出调整后的n个数。
#include <stdio.h>main(){int number[20],n,m,i;void move(int array[20],int n,int m);printf("\nHow many numbers? "); /* 共有多少个数*/scanf("%d",&n);printf("Input %d numbers: \n",n); /* 输入n个数*/for(i=0;i<n;i++)scanf("%d",&number[i]);printf("How many place you want to move? "); /* 后移多少个位置*/ scanf("%d",&m);move(number,n,m); /* 调用move 函数*/printf("Now, they are: \n");for(i=0;i<n;i++)printf("%5d",number[i]);}void move(int array[20],int n,int m) /* 循环后移-次的函数*/{int *p,array_end;array_end=*(array+n-1);for(p=array+n-1;p>array;p--)*p=*(p-1);*array=array_end;m--;if(m>0) move(array,n,m); /*递归调用,当循环次数m减至0时,停止调用*/ }运行结果:How many numbers? 8↙Input 8 numbers:12 43 65 67 8 2 7 11↙How many place you want to move? 4↙Now, they are:8 2 7 1112 43 65 677.在主函数中输入二维数组a[3][3],编写一函数move(),将一个3×3的矩阵转置。