求解矩形packing问题的贪心算法
Packing问题

Packing问题问题描述:如何把任意数量任意尺⼨矩形集⽆重复的放到⼀个⾯积最⼩的封闭矩形中。
算法思想:(为了便于描述,把要找的封闭矩形记为a,封闭矩形的集合记为as,把矩形集合记为rs,n为rs中矩形的个数,把可以插⼊矩形的位置记为corners)1.把所有矩形集中的矩形按⾼度从⼤到⼩排序,此时rs[0]⾼度最⼤2.把a初始化为:height = rs[0].height,width = rs[0].width + rs[1].width + ...... + rs[n - 1].width,corners初始化为:坐标顶点3.把rs[0]放⼊a中,并把由于rs[0]的插⼊产⽣的corner放⼊corners集合中,删除已经使⽤的corner,如下图所⽰:4.从rs[1]到rs[n - 1],每次插⼊时选择可以插⼊的X值最⼩的corner,插⼊成功之后,删除已经使⽤的corner,并且插⼊新产⽣的corner,由于a的初始化条件,可以保证rs中的所有矩形都可以插⼊a中5.所有矩形插⼊完成后,对a进⾏“瘦⾝”,让a正好包含所有的矩形,并记录此时a的宽度为width,a的⾯积为area(此时a是所有符合条件的封闭矩形中的‘最狭长’的,令as[0] = a)6.依次减少a.width,增加a.height,重复3-5,如果a的⾯积有所减少,则把a插⼊到as中,直到a.width = rs中最宽矩形的宽度7.as集合的最后⼀个元素就是可以包含rs中所有矩形的⾯积最⼩的封闭矩形,算法结束算法实现:在算法的实现中需要注意的地⽅:1.计算由于矩形的插⼊产⽣的新的corner(需要考虑很多特殊情况)2.判断矩形是否可以插⼊⼀个corner3.数据结构的设计以下程序是算法最核⼼的⽅法,插⼊矩形,并记录新产⽣的cornerprivate boolean putIn(PngRect png){if(pngs == null){pngs = new ArrayList<PngRect>();pngs.add(png);png.setStartP(new Point(0,0));if(png.getHeight() < mHeight){Rect owner = new Rect(0,png.getStopP().getY(),mWidth,mHeight);Corner corner = new Corner(new Point(0,png.getHeight()),owner);corners.add(corner);}if(png.getWidth() < mWidth){Rect owner = new Rect(png.getStopP().getX(),0,mWidth,mHeight);Corner corner = new Corner(new Point(png.getWidth(),0),owner);corners.add(corner);}return true;}else{Corner corner;int x;int y;Rect owner;for(int i = 0; i < corners.size(); ++i){corner = corners.get(i);x = corner.getPoint().getX();y = corner.getPoint().getY();owner = corner.getRect();Point startP = corner.getPoint();int endX = owner.right;int endY = owner.bottom;/** 可以放进该corner,此处存在覆盖问题,需要判断四条边是否有点在已经被使⽤,如果是,则返回,试下⼀个corner* v0-----v1* | |* | a |* | |* v2-----v3* 同时为了防⽌完全重叠,还需要判断额外⼀个点,例如图中的a点*/if(x + png.getWidth() <= mWidth && y + png.getHeight() <= mHeight){Point v0 = startP;Point v1 = new Point(startP.getX() + png.getWidth(),startP.getY());Point v2 = new Point(startP.getX(),startP.getY() + png.getHeight());Point v3 = new Point(startP.getX() + png.getWidth(),startP.getY() + png.getHeight());Point a = new Point(startP.getX() + png.getWidth()/2,startP.getY() + png.getHeight()/2);if(contains(v0,v1,true) || contains(v1,v3,false) || contains(v2,v3,true) || contains(v0,v2,false) || contains(a)){//有可能正好落在边上continue;}// if(contains(a) ||contains(v0) || contains(v1) || contains(v2) || contains(v3) ){//有可能正好落在边上// continue;// }if(x + png.getWidth() < endX){corners.add(i + 1,new Corner(new Point(x + png.getWidth(),y),new Rect(x + png.getWidth(),y,mWidth,mHeight)));//此处owner可能为空 }if(y + png.getHeight() < endY){corners.add(i + 1, new Corner(new Point(x,y + png.getHeight()),new Rect(x,y + png.getHeight(),mWidth,mHeight)));}corners.remove(i);sortCorners();png.setStartP(corner.getPoint());pngs.add(png);return true;}}}return false;}实体类:Cornerpublic class Corner {//Corner的起始点private Point point;//可以填充图⽚的区域private Rectangle rect;public Corner(Point point,Rectangle rect){this.point = point;this.rect = rect;}public Point getPoint(){return point;}public Rectangle getRect(){return rect;}}PngRectpublic class PngRect {private int mHeight;private int mWidth;private Point mStartP;//最左上⾓的点private Point mStopP;//最右下⾓的点private BufferedImage mBitmap;public PngRect(int width, int height, BufferedImage bitmap){this.mWidth = width;this.mHeight = height;this.mBitmap = bitmap;}public int getWidth(){return this.mWidth;}public int getHeight(){return this.mHeight;}public void setStartP(Point p){mStartP = p;mStopP = new Point(p.x + mWidth, p.y + mHeight);}public Point getStartP(){return mStartP;}public Point getStopP(){return mStopP;}public BufferedImage getBitmap(){return mBitmap;}//判断某个点是否包含在矩形内public boolean contains(Point p){if (mStartP == null){return false;}int x = p.x;int y = p.y;if (x > mStartP.getX() && x < mStopP.getX() && y > mStartP.getY() && y < mStopP.getY()) {return true;}return false;}//把pngs中的图⽚按⾼度从⼤到⼩排序public static void sortPngRects(ArrayList<PngRect> pngs){PngRect maxHeightrect;PngRect tempRect;PngRect currentRect;int k;for (int i = 0; i < pngs.size(); ++i){maxHeightrect = pngs.get(i);currentRect = pngs.get(i);k = i;for (int j = i + 1; j < pngs.size(); ++j){tempRect = pngs.get(j);if (tempRect.getHeight() > maxHeightrect.getHeight()){maxHeightrect = tempRect;k = j;}}if (k != i){pngs.set(i,maxHeightrect);pngs.set(k,currentRect);}}}}。
求解矩形Packing问题的基于遗传算法的启发式递归策略

Vol.33,No.9ACTA AUTOMATICA SINICA September,2007 An Improved Heuristic Recursive Strategy Based on Genetic Algorithm for the Strip RectangularPacking ProblemZHANG De-Fu1CHEN Sheng-Da1LIU Yan-Juan1Abstract An improved heuristic recursive strategy combining with genetic algorithm is presented in this paper.Firstly,this method searches some rectangles,which have the same length or width,to form some layers without waste space,then it uses the heuristic recursive strategies to calculate the height of the remaining packing order and uses the evolutionary capability of genetic algorithm to reduce the height.The computational results on several classes of benchmark problems have shown that the presented algorithm can compete with known evolutionary heuristics.It performs better especially for large test problems.Key words Strip packing problems,heuristic,recursive,genetic algorithm1IntroductionMany industrial applications,which belong to cutting and packing problems,have been found.Each application incorporates different constraints and objectives.For ex-ample,in wood or glass industries,rectangular components have to be cut from large sheets of material.In warehousing contexts,goods have to be placed on shelves.In newspapers paging,articles and advertisements have to be arranged in pages.In the shipping industry,a batch of objects of var-ious sizes has to be shipped to the maximum extent in a larger container,and a bunch of opticalfibers has to be accommodated in a pipe with perimeter as small as possi-ble.In very-large scale integration(VLSI)floor planning, VLSI has to be laid out.These applications have a similar logical structure,which can be modeled by a set of pieces that must be arranged on a predefined stock sheet so that the pieces do not overlap with one another,so they can be formalized as the packing problem[1].For more extensive and detailed descriptions of packing problems,the reader can refer to[1∼3].A two-dimensional strip rectangular packing problem is considered in this paper.It has the following characteris-tics:a set of rectangular pieces and a larger rectangle with afixed width and infinite length,designated as the con-tainer.The objective is tofind a feasible layout of all the pieces in the container that minimizes the required con-tainer length and,where necessary,takes additional con-straints into account.This problem belongs to a subset of classical cutting and packing problems and has been shown to be non-deterministic polynomial(NP)hard[4,5].Opti-mal algorithms for orthogonal two-dimension cutting were proposed in[6,7].Gilmore and Gomory[8]solved prob-lem instances to optimality by linear programming tech-niques in1961.Christofides and Whitlock[9]solved the two-dimensional guillotine stock cutting problem to opti-mality by a tree-search method in1977.Cung et al.[10] developed a new version of the algorithm proposed in Hifiand Zissimopolous that used a best-first branch-and-bound approach to solve exactly some variants of two-dimensional stock-cutting problems in2000.However,these algorithms might not be practical for large problems.In order to solve large problems,some heuristic algorithms were developed. Received June20,2006;in revised form October24,2006 Supported by Academician Start-up Fund(X01109),985Informa-tion Technology Fund(0000-X07204)in Xiamen University1.Department of Computer Science,Xiamen University,Xiamen 361005,P.R.ChinaDOI:10.1360/aas-007-0911The most documented heuristics are the bottom-left(BL), bottom-left-fill(BLF)methods,and other heuristics[11∼13]. Although their computational speed is very fast,the so-lution quality is not desirable.Recently,genetic algo-rithms and its improved algorithms for the orthogonal pack-ing problem were proposed because of their powerful op-timization capability[14∼17].Kroger[14]used genetic algo-rithm for the guillotine variant of bin packing in1995. Jakobs[15]used a genetic algorithm for the packing of poly-gons using rectangular enclosures and a Bottom-left heuris-tic in1996.Liu et al.[16]further improved it.Hop-per and Turton[18]evaluated the use of the BLF heuris-tic with genetic algorithms on the nonguillotine rectangle-nesting problem in1999.In addition,an empirical in-vestigation of meta-heuristic and heuristic algorithms of the strip rectangular packing problems was given by[19]. Recently,some new models and algorithms were devel-oped by[20∼26].For example,quasi-human heuristic[20], constructive approach[21,22],a new placement heuristic[23], heuristic recursion(HR)algorithm[24],and hybrid heuristic algorithms[25,26]were developed.These heuristics are fast and effective,especially,the bestfit in[23]not only is very fast,but alsofinds better solutions than some well-known metaheuristics.In this paper,an improved heuristic recursive algorithm that combines with genetic algorithm is presented to solve the orthogonal strip rectangular packing problem.The computational results on a class of benchmark problems show that this algorithm can compete with known evolu-tionary heuristics,especially in large test problems.The rest of this paper is organized as follows.In Section 2,a clear mathematical formulation for the strip rectangu-lar packing problem is given.In Section3,the heuristic recursive algorithm is presented,and an improved heuris-tic recursive algorithm(IHR)is developed in detail.In Section4,the GA+IHR algorithm is puta-tional results are described in Section5.Conclusions are summarized in Section6.2Mathematical formulation of the problemGiven a rectangular board of given width and a set of rectangles with arbitrary sizes,the strip packing problem of rectangles is to pack each rectangle on the board so that no two rectangles overlap and the used board height is min-imized.This problem can also be stated as follows.912ACTA AUTOMATICA SINICA Vol.33Given a rectangular board with given width W,and n rectangles with length l i and width w i,1≤i≤n,let (x li,y li)denote the top-left corner coordinates of rectangle i,and(x ri,y ri)the bottom-right corner coordinates of rect-angle i.Other symbols are similar to[24].For all1≤i≤n, the coordinates of rectangles must satisfy the following con-ditions:1)(x ri−x li=l i and y li−y ri=w i)or(x ri−x li=w i and y li−y ri=l i);2)For all1≤j≤n,j=i,rectangle i and j can not overlap,namely,x ri≤x lj or x li≥x rj or y ri≥y lj or y li≤y rj;3)x L≤x li≤x R,x L≤x ri≤x R and y R≤y li≤h,y R≤y ri≤h.The problem is to pack all the rectangles on the board such that the used board height h is minimized.It is noted that for orthogonal rectangular packing prob-lems the packing process has to ensure the edges of each rectangle are parallel to the x-and y-axes,respectively, namely,all rectangles can not be packed aslant.In addi-tion,all rectangles except the rectangular board are allowed to rotate90degrees.3IHR algorithmThe HR algorithm for the strip rectangular packing prob-lem was presented in[24].It follows a divide-and-conquer approach:break the problem into several subproblems that are similar to the original problem but smaller in size,solve the subproblems recursively,and then combine these solu-tions to create a solution to the original problem.The HR algorithm is very simple and efficient,and can be stated as follows:1)Pack a rectangle into the space to be packed,and divide the unpacked space into two subspaces.2)Pack each subspace by packing it recursively.If the subspace size is small enough to only pack a rectangle,then just pack this rectangle into the subspace in a straightfor-ward manner.3)Combine the solutions to the subproblems for the so-lution of the rectangular packing problem.In order to enhance the performance of the HR algo-rithm,the author in[18]presented some heuristic strate-gies to select a rectangle to be packed,namely,the rect-angle with the maximum area is given priority to pack.In detail,unpacked rectangles should be sorted by nonincreas-ing ordering of area size.The rectangle with maximum area should be selected to packfirst if it can be packed into the unpacked subspace.In addition,the longer side of the rect-angle to be packed should be packed along the bottom side of the subspace.It is the disadvantage of the HR algorithm that may have waste space in each layer(See Fig.1).In order to overcome this disadvantage,some layers without waste space arefirst considered.Some definitions are given to clearly describe the idea of the improved algorithm.Definition1.The reference rectangle is the rectangle that is packedfirstly and can form one layer with other rectangles,and its short edge is the height of that layer.Definition2.The combination layer is the layer that has no waste space,and the rectangles packed into it have the same height as the height of the layer and are spliced together one by one along the direction of W.The sum of the edge length of the rectangles along the W direction is the combination width.From Fig.1to Fig.4,each rectangle at the top of the container is the referee rectangle.Thefirst layer in Fig.1 is not a combination layer because it has waste space.The first layer in Fig.2is a combination layer because it has no waste space and the spliced rectangles have the same width or length as the height of the layer.Although the second layer in Fig.2has no waste space,it is not a com-bination layer because the two middle rectangles are not spliced along the direction of W.By the definition of the combination layer,the combination width is W.If some combination layers are found before further computation, then they may decrease the cost of computation because the rectangles already packed into these combination lay-ers will not be considered in thefuture.From the above discussion,it is very important tofind the combination layer.Given a packing ordering,the pro-cedure offinding the combination layer is given as follows.Find thefirst unpacked and unreferenced rectangle as the reference rectangle,and put this rectangle into a two-dimensional array.Then seek downwards from the refer-ence rectangle orderly.If one canfind a rectangle whose length or width is equal to the width of the reference rect-angle,then put this rectangle into the two-dimensional ar-ray,repeat this until a combination layer or no combination layer is found.Repeat the above process until all rectan-gles are packed otherwise no rectangle can be the reference rectangle.In this process,the number of rectangles,which have been packed in the current layers,must be recorded. Finally,the number of all the combination layers must be recorded.The steps of the combination operator can be stated as follows:Combination()RepeatFind thefirst unpacked and unreferencedrectangle as the reference rectangle,and put thisrectangle into a two-dimensional array;For i=current position to nIf(the width or the length of rectangle i isequal to the width of the reference rectangle)If(combination width<W)Put the rectangle into the two-dimensionalarray;Else if(combination width=W)Pack all the rectangles of theNo.9ZHANG De-Fu et al.:An Improved Heuristic Recursive Strategy Based on Genetic Algorithm for (913)two-dimensional array on the container;Break;Record the number of all packed rectangles;Until all rectangles are packed or no rectangle is thereference rectangle;Record the number(Num)of all the combination layers;So,the IHR algorithm can be stated as follows:Step1.The combination layers are searched.Step2.Pack the remaining rectangles to the container by HR algorithm.By intuition,the more the number of the combination layers is,the faster the computational speed is.However, it is not always true that more combination layers can ob-tain a better solution.From Fig.1to Fig.4,we know that the best combination layer number is1.Fig.1has no com-bination layer,but layer1,layer3,and layer4waste a little space.Fig.2has one combination layer and is the optimal solution.Fig.3has two combination layers but layer3and layer4waste a little space.Fig.4has three combination layers,but layer4and layer5waste a little space.4GA+IHR4.1Genetic algorithm(GA)GA is a heuristic method used tofind approximate solu-tions to hard optimization problems through application of the principles of evolutionary biology to computer science. It is modeled loosely on the principles of the evolution via natural selection,which use a population of individuals that undergo selection in the presence of variation inducing op-erators such as recombination(crossover)and mutation.In order to run GA,we must be able to create an initial pop-ulation of feasible solutions.The initial population is very important for achieving a good solution.There are many ways to do this based on the form of the problems.The evolution starts from a population of completely random in-dividuals and happens in generations.In each generation, thefitness of the whole population is evaluated.Multiple individuals are stochastically selected from the current pop-ulation and are modified to form a new population,which becomes current population in the next iteration of the algorithm.Further detailed theoretical and practical de-scriptions of genetic algorithm,the interested reader can refer to[27].Combining GA with IHR,the GA+IHR algorithm to solve the strip rectangular packing problem can be stated as follows:GA+IHR()Sort all rectangles by non-increasing ordering of area size;Combination();For i=0to NumInitialization();For j=1to NumberFor k=1to N/2Select two individuals in the parentsrandomly,then crossover with probabilityP c or copy with probability(1−P c)tocreate two middle offspring;Mutate the middle offspring withprobability P m;Compare the parent and the middle offspring,if thefitness of the middle offspring is less thanthe parent s,we accept it as new offspring,otherwise we accept it with probability P b oraccept the parent with probability(1−P b);Select the best solution from the parents;Select the worse solution from the newgeneration;Replace the worse solution with the bestsolution;Save the best solution acquired from combinationlayer i to array A;Select the best solution from array A;where Num is the number of all the combination layers; Number is the iteration number of genetic algorithm;N is the number of population;P c=0.8,P m=0.2,but P m will increase as the parent chromosomes become more alike,P b=0.33.Thefitness value of genetic algorithm is calculated by HR algorithm.The required container length is the sum of combination height and currentfitness value.4.2InitializationThe GA is used to optimize the solution for unpacked rectangles.Thefitness value of GA is calculated by HR algorithm.In this paper,a string of integers,which forms an index into the set of rectangles,is used,and then the HR strategy is used to create the sequence of thefirst indi-viduals,and the sequence is divided into two equal parts, the two parts of thefirst individuals are then permuted to obtain N−1individuals(N is the size of population).The method of the permutation is to produce a point in each range randomly and exchange the position of the point for its neighbor.This initialization method can keep the diver-sification of each individual in the population.At the same time,it can keep the individual,which has betterfitness. From the experiment results,we know that the method has better effect.4.3CrossoverThe role of the crossover operator is to allow the advanta-geous traits to spread throughout the population such that the population as a whole may benefit from this chance dis-covery.The steps of the crossover operator are as follows:1)Choose two individuals Parent1and Parent2from the parents randomly.2)Get the items of individual Child1from Parent1and ly,if the sequence number of the Child1is odd,find an item orderly in Parent1until the item is different from all the items in Child1,otherwise find an item orderly in Parent2until the item is different from all the items in Child1.When the number of Child1 is n,a new individual is created successfully.3)Similarly,get the items of individual Child2from Parent2and ly,if the sequence number of the Child2is odd,find an item orderly in Par-ent2until the item is different from all the items in Child2, otherwisefind an item orderly in Parent1until the item is different from all the items in Child2.When the number of Child2is n,a new individual is created successfully.As an example of crossover,suppose two individuals are already selected:Parent1:53267841Parent2:86517324 According to the steps of the crossover operator,the sequences of the children can be obtained:Child1:58362174Child2:856312744.4MutationIn each individual A,two different points are chosen ran-domly,and the sequence within two points is inversed,then914ACTA AUTOMATICA SINICA Vol.33in the appointed iteration step,judge thefitness of the new individual B,if thefitness of B is less than that of A,B is accepted.As an example of mutation,suppose two mutation points (3and6)are already selected:A:24587136After mutation,we can get the new individualB:24178536Mutation is adaptive,that is,the mutation rate increases as the parent chromosomes become more alike.4.5ReplacementAfter the operations of crossover and mutation,a set of solutions are produced.For keeping the bestfitness and quickening the speed of convergence,a best solution is se-lected from the set of solutions,and it is saved to the next generation in each iteration step.5Computational resultsIn order to compare the relative performance of the pre-sented GA+IHR with other published heuristic and meta-heuristic algorithms,several test problems taken from the literature are used.Perhaps the most extensive instances given for this problem are found in[19],where21prob-lem instances are presented in seven different sized cate-gories ranging from16to197items.The optimal solu-tions of these21instances are all known.Table1(see next page)presents an overview of the test problem Class1from [19].As we wanted to extensively test our algorithm,other test problems were generated at random.Table2shows an overview of the test problem Class2generated randomly with known optimal solution.The problem Class2can be accessed in[23].In order to verify the performance of GA+IHR,two best meta-heuristic GA+BLF and SA+BLF[23],Bestfit[13],and HR[19]are selected.The computational results are listed in Tables3and4.20iterative times are chosen for GA and 80iterative times are chosen in the mutation operation.On this test problem Class1,as listed in Table3,Gap of GA+IHR ranges from0.83to4.44with the average Gap 2.06.The average Gaps of GA+BLF,SA+BLF,Bestfit, and HR are4.57,4,5.69,and3.97,respectively.The av-erage Gap of GA+IHR is lower than those of GA+BLF, SA+BLF,Bestfit,and HR.And as listed in Table4,the average running time of GA+IHR is also lower than those of GA+BLF and SA+BLF,but is larger than that of Best fit and HR.The packing results can be seen in Fig.5and Fig.6,where L denotes the optimal height.The heights of C11,C12,C13,and C72using GA+IHR are20,21,21,and 241,respectively.GA+IHR canfind the optimal heights for C11,C23,and C32.In order to extensively test the performance of our al-gorithm for randomly generated instances,especially for larger instances,12problem instances ranging from10to 500were generated at random.The computational results are listed in Table5.For such problem,100iterative times are chosen for the GA and10iterative times are chosen in the mutation operation.Although our algorithm can ob-tain a better solution,it needs much time,especially for large problems.So for N12,40iterative times are chosen for the GA and10iterative times are chosen in the muta-tion operation.From Table5,we observe that the running time is acceptable.What is more,the Gap is better thanothers.Fig.5Packed results of C1forGA+IHRFig.6Packed results of C72for GA+IHR6ConclusionsIn this paper,the GA+IHR algorithm for the orthogo-nal stock-cutting problem has been presented.IHR is very simple and intuitive,and can solve the orthogonal stock-cutting problem efficiently.GA is an adaptive heuristic search algorithm.It has the capability of global search within the solution space.The idea of combination layers to reduce the number of unpacked rectangles has been used. During the process of iteration search,HR is called repeat-edly to calculate the height of an individual.As we know,finding the optimal solution is more difficult for the packing problem as increasing the size of problem.But it can be overcome by using the characteristic of GA.The computa-tional results have shown that we can obtain the desirable solutions within acceptable computing time by combining GA with IHR.So GA+IHR can compete with other evolu-tion heuristic algorithms,especially for large test problems, it performs better.So GA+IHR may be of considerable practical value to the rational layout of the rectangular objects in the engineeringfields,such as the wood,glass, and paper industry,the ship building industry,and textile and leather industry.Future work is to further improve the performance of GA+IHR and minimize the influence of the parameters selection,and extend this algorithm for three-dimensional rectangular packing problems.No.9ZHANG De-Fu et al.:An Improved Heuristic Recursive Strategy Based on Genetic Algorithm for (915)Table1Test problem Class1Problem category Number of items:n Optimal height Object dimensionC1(C11,C12,C13)16(C11,C13),17(C12)2020×20C2(C21,C22,C23)25(C21,C22,C23)1515×40C3(C31,C32,C33)28(C31,C33),29(C32)3030×60C4(C41,C42,C43)49(C41,C42,C43)6060×60C5(C51,C52,C53)73(C51,C52,C53)9090×60C6(C61,C62,C63)97(C61,C62,C63)120120×80C7(C71,C72,C73)196(C71,C73),197(C72)240240×160Table2Test problem Class2Problem category Number of items:n Optimal height Object dimension N1104040×40N2205030×50N3305030×50N4408080×80N550100100×100N66010050×100N77010080×100N88080100×80N910015050×150N1020015070×150N1130015070×150N12500300100×300Table3Gaps of GA+BLF,SA+BLF,Bestfit,HR,and GA+IHR for the test problem Class1C1C2C3C4C5C6C7Average GA+BLF4753445 4.57 SA+BLF46533344Bestfit11.67 6.79.9 3.87 2.93 2.5 2.23 5.69HR8.33 4.45 6.67 2.22 1.85 2.5 1.8 3.97 GA+IHR 3.33 4.44 2.22 1.67 1.110.830.83 2.06Table4Average running time of GA+BLF,SA+BLF,and GA+HRC1C2C3C4C5C6C7Average GA+BLF 4.619.2213.8359.93165.96396.463581.97604.57 SA+BLF 3.22711.06418.4452.13530.151761.0219274.413107.2 Bestfit0.00.00.00.000.0030.0050.0070.005 HR000.030.140.69 2.2136.07 5.59 GA+IHR0.88 1.52 2.249.5630.0465.56426.0476.55Table5Gaps of GA+BLF,SA+BLF,Bestfit,HR,and GA+IHR for the test problem Class2n Optimal heightGA+BLF SA+BLF BF Heuristic GA+IHRh Time(s)h Time(s)h Time(s)h Time(s)N1104040 1.02400.2445<0.01450.68 N22050519.2528.1453<0.0154 3.32 N3305052 2.65239.552<0.0151 6.18 N440808312.6838483<0.018313.09 N55010010652.31062281050.0110333.01 N6601001032611033101030.0110250.12 N7701001066711065541070.0110457.04 N8808085114285810840.018231.36 N9100150155443115517151520.01152185.94 N102001501542×10415460661520.021511154.18 N113001501558×1041553×1041520.031513763.17 N125003003134×1053126×1043060.063045864.27 Average Gap(%)-- 3.72- 3.85- 4.35- 3.46-916ACTA AUTOMATICA SINICA Vol.33References1Lodi A,Martello S,Monaci M.Two-dimensional packing problems:a survey.European Journal of Operational Re-search,2002,141(2):241∼2522Dowsland K A,Dowsland W B.Packing problems.European Journal of Operational Research,1992,56(1):2∼143Pisinger D.Heuristics for the container loading problem.European Journal of Operational Research,2002,141(2): 382∼3924Hochbaum D S,Wolfgang M.Approximation schemes for covering and packing problems in image processing and VLSI.Journal of the Association for Computing Machinery, 1985,32(1):130∼1365Leung J Y,Tam T W,Wong C S,Young G H,Chin F Y L.Packing squares into a square.Journal of Parallel and Distributed Computing,1990,10(3):271∼2756Beasley J E.An exact two-dimensional non-guillotine cutting tree search procedure.Operations Research,1985,33(1): 49∼647Hadjiconstantinou E,Christofides N.An exact algorithm for the orthogonal2D cutting problems using guillotine cuts.European Journal of Operational Research,1995,83(1): 21∼388Gilmore P C,Gomory R E.A linear programming approach to the cutting stock problem(part I).Operational Research, 1961,9:849∼8599Christofides N,Whitlock C.An algorithm for two-dimensional cutting problems.Operational Research,1977, 25(1):30∼4410Cung V D,HifiM,Cun B C.Constrained two-dimensional cutting stock problems a best-first branch-and-bound algo-rithm.International Transactions in Operational Research, 2000,7(3):185∼21011Zhang De-Fu,Li Xin.A personified annealing algorithm for circles packing problem.Acta Automatica Sinica,2005, 31(4):590∼595(in Chinese)12Chazelle B.The bottom-left bin packing heuristic:an ef-ficient implementation.IEEE Transactions on Computers, 1983,32(8):697∼70713Berkey J,Wang P.Two-dimensionalfinite bin packing algo-rithms.Journal of the Operational Research Society,1987, 38:423∼42914Kroger B.Guillotineable bin packing:a genetic approach.European Journal of Operational Research,1995,84(3): 645∼66115Jakobs S.On genetic algorithms for the packing of polygons.European Journal of Operational Research,1996,88(1): 165∼18116Liu D,Teng H.An improved BL-algorithm for genetic al-gorithm of the orthogonal packing of rectangles.European Journal of Operational Research,1999,112(2):413∼419 17Dagli C,Poshyanonda P.New approaches to nesting rectan-gular patterns.Journal of Intelligent Manufacturing,1997, 8(3):177∼19018Hopper E,Turton B.A genetic algorithm for a2D indus-trial packing puters and Industrial Engineer-ing,1999,37(1):375∼37819Hopper E,Turton B.An empirical investigation of meta-heuristic and heuristic algorithms for a2D packing problem.European Journal of Operational Research,2001,128(1): 34∼5720Wu Y L,Huang W Q,Lau S C,Wong C K,Young G H.An effective quasi-human based heuristic for solving the rectan-gle packing problem.European Journal of Operational Re-search,2002,141(2):341∼35821HifiM,Hallah R.A best-local position procedure-based heuristic for the two-dimensional layout problem.Studia In-formatica Universalis,International Journal on Informatics–Special Issue on Cutting,Packing and Knapsacking Prob-lems,2002,2(1):33∼5622HifiM,Hallah R.A hybrid algorithm for the two-dimensional layout problem:the cases of regular and irregular shapes.International Transactions in Operational Research,2003, 10(3):195∼21623Burke E,Kendall G,Whitwell G.A new placement heuristic for the orthogonal stock-cutting problem.Operations Re-search,2004,52(4):655∼67124Zhang D F,Kang Y,Deng S.A new heuristic recursive algo-rithm for the strip rectangular packing puters &Operations Research,2006,33(8):2209∼221725Zhang D F,Deng A S,Kang Y.A hybrid heuristic algo-rithm for the rectangular packing problem.Lecture Notes in Computer Science,2005,3514:783∼79126Zhang D F,Liu Y J,Chen S D,Xie X G.A meta-heuristic algorithm for the strip rectangular packing problem.Lecture Notes in Computer Science,2005,3612:1235∼124127Davis L.Handbook of Genetic Algorithms.New York:Van Nostrand Reinhold,1991ZHANG De-Fu Associate professor atSchool of Information Science and Technol-ogy,Xiamen University.He received hisbachelor and master degrees in computa-tional mathematics from Xiangtan Univer-sity in1996and1999,respectively,and hisPh.D.degree in computer software and itstheory from Huazhong University of Sci-ence and Technology in2002.His researchinterest covers computational intelligence andfinancial data mining.Corresponding author of this paper. E-mail:dfzhangl@CHEN Sheng-Da Master student atXiamen University.He received his bach-elor degree from Jimei University in2004.His research interest is computational in-telligence.E-mail:cshengda@LIU Yan-Juan Master student in Xia-men University.She received her bache-lor degree from Shijiazhuang University ofEconomics in2004.Her research interest iscomputational intelligence.E-mail:jjj514@。
贪心算法之装箱问题

贪⼼算法之装箱问题问题描述装箱问题可简述如下:设有编号为 0、1、…、n - 1 的 n 种物品,体积分别为v0、v1、…、vn-1。
将这 n 种物品装到容量都为 V 的若⼲箱⼦⾥。
约定这 n 种物品的体积均不超过 V,即对于 0≤ i<n,有 0<vi ≤ v。
不同的装箱⽅案所需要的箱⼦数⽬可能不同。
装箱问题要求使装尽这 n 种物品的箱⼦数要少。
贪⼼求解使⽤⼀种贪⼼策略:每次都想将当前体积最⼤的物品装⼊箱中,在这块类似于这个问题 ->>>其实在⽣活中这也是很常见的⼀种做法,在没有充⾜时间去考虑如何最优解决这类问题时直觉(第六感狗头保命)告诉我们可以这么去试试。
更直接的⼀个例⼦,⼒扣上有这么⼀道题:在柠檬⽔摊上,每⼀杯柠檬⽔的售价为 5 美元。
顾客排队购买你的产品,(按账单 bills ⽀付的顺序)⼀次购买⼀杯。
每位顾客只买⼀杯柠檬⽔,然后向你付 5 美元、10 美元或20美元。
你必须给每个顾客正确找零,也就是说净交易是每位顾客向你⽀付 5 美元。
注意,⼀开始你⼿头没有任何零钱。
注:上⾯⿊体部分内容引⾃很明显,当客户给我们$20进⾏找零时,⾃然⽽然地就给ta找了⼀张$10加上⼀张$5,为什么这么做?⾯对$20,我们有两种⽅案可以使⽤:找三张$5给顾客找⼀张$10 以及⼀张 $5 给顾客选择第⼆种⽅案的原因对于做⽣意的⽼板应该不陌⽣,营业过程中我们需要备上⼀部分零钱在交易时⽅便找零,不⾄于出现⽆法找零的尴尬局⾯,这是商⼈们所想的,在上题中也同样适⽤。
但贪⼼算法的弊端也很明显:不考虑之前解带来的影响,仅仅为了达到当前最优解,这样”⼀根筋“的策略也不能在任何情况下得到最优解。
如只有⾯值分别为 1、5 和 11 单位的硬币,⽽希望找回总额为 15 单位的硬币。
按贪婪算法,应找 1 个 11 单位⾯值的硬币和 4 个 1 单位⾯值的硬币,共找回 5 个硬币。
但最优的解应是 3 个 5 单位⾯值的硬币。
求解二维矩形装箱问题的算法研究

求解二维矩形装箱问题的算法研究下载提示:该文档是本店铺精心编制而成的,希望大家下载后,能够帮助大家解决实际问题。
文档下载后可定制修改,请根据实际需要进行调整和使用,谢谢!本店铺为大家提供各种类型的实用资料,如教育随笔、日记赏析、句子摘抄、古诗大全、经典美文、话题作文、工作总结、词语解析、文案摘录、其他资料等等,想了解不同资料格式和写法,敬请关注!Download tips: This document is carefully compiled by this editor. I hope that after you download it, it can help you solve practical problems. The document can be customized and modified after downloading, please adjust and use it according to actual needs, thank you! In addition, this shop provides you with various types of practical materials, such as educational essays, diary appreciation, sentence excerpts, ancient poems, classic articles, topic composition, work summary, word parsing, copy excerpts, other materials and so on, want to know different data formats and writing methods, please pay attention!一、引言随着物流行业的发展和电子商务的兴起,二维矩形装箱问题成为了一个备受关注的研究课题。
列举用贪心算法求解的经典问题

列举用贪心算法求解的经典问题贪心算法是一种简单而高效的问题求解方法,通常用于求解最优化问题。
它通过每一步选择当前状态下的最优解,最终得到全局最优解。
贪心算法的核心思想是:每一步都做出一个局部最优的选择,并认为这个选择一定可以达到全局最优。
以下是一些经典问题,可以用贪心算法求解:1. 零钱兑换问题(Coin Change Problem):给定一些不同面额的硬币和一个目标金额,找到最少的硬币数量,使得硬币总额等于目标金额。
贪心算法可以按照硬币的面额从大到小进行选择,每次选择尽量大面额的硬币。
2. 区间调度问题(Interval Scheduling Problem):给定一些区间,找到最多的不相交区间。
贪心算法可以按照区间的结束时间进行排序,每次选择结束时间最早的区间,确保选择的区间不重叠。
3. 分糖果问题(Candy Problem):给定一个数组表示每个孩子的评分,要求给这些孩子分糖果,满足以下要求:每个孩子至少分到一个糖果,评分高的孩子要比相邻孩子分到的糖果多。
贪心算法可以从左到右进行两次遍历,分别处理评分递增和评分递减的情况。
4. 跳跃游戏问题(Jump Game Problem):给定一个非负整数数组,表示每个位置的最大跳跃长度,判断是否能从第一个位置跳到最后一个位置。
贪心算法可以记录当前能够到达的最远位置,并且更新为更远的位置。
5. 任务调度器问题(Task Scheduler Problem):给定一串任务,每个任务需要一定的冷却时间,要求以最短的时间完成所有任务。
贪心算法可以按照出现次数进行排序,优先执行出现次数最多的任务,并在冷却时间内执行其他任务。
6. 区间覆盖问题(Interval Covering Problem):给定一些区间,找到最少的区间数,使得它们的并集覆盖了所有输入区间。
贪心算法可以根据区间的起始位置进行排序,每次选择最早结束的区间,并将它添加到最终结果中。
以上仅是一些经典问题的例子,实际上还有很多问题可以用贪心算法来求解。
matlab三维装箱问题的算法
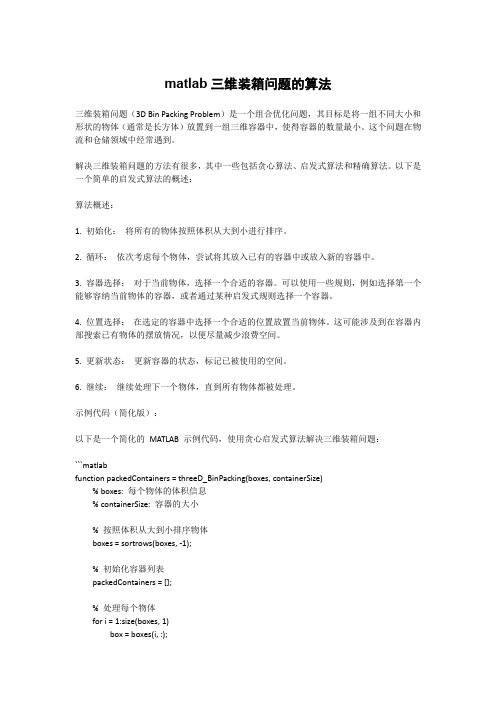
matlab三维装箱问题的算法三维装箱问题(3D Bin Packing Problem)是一个组合优化问题,其目标是将一组不同大小和形状的物体(通常是长方体)放置到一组三维容器中,使得容器的数量最小。
这个问题在物流和仓储领域中经常遇到。
解决三维装箱问题的方法有很多,其中一些包括贪心算法、启发式算法和精确算法。
以下是一个简单的启发式算法的概述:算法概述:1. 初始化:将所有的物体按照体积从大到小进行排序。
2. 循环:依次考虑每个物体,尝试将其放入已有的容器中或放入新的容器中。
3. 容器选择:对于当前物体,选择一个合适的容器。
可以使用一些规则,例如选择第一个能够容纳当前物体的容器,或者通过某种启发式规则选择一个容器。
4. 位置选择:在选定的容器中选择一个合适的位置放置当前物体。
这可能涉及到在容器内部搜索已有物体的摆放情况,以便尽量减少浪费空间。
5. 更新状态:更新容器的状态,标记已被使用的空间。
6. 继续:继续处理下一个物体,直到所有物体都被处理。
示例代码(简化版):以下是一个简化的MATLAB 示例代码,使用贪心启发式算法解决三维装箱问题:```matlabfunction packedContainers = threeD_BinPacking(boxes, containerSize)% boxes: 每个物体的体积信息% containerSize: 容器的大小% 按照体积从大到小排序物体boxes = sortrows(boxes, -1);% 初始化容器列表packedContainers = [];% 处理每个物体for i = 1:size(boxes, 1)box = boxes(i, :);% 尝试将物体放入已有容器placed = false;for j = 1:length(packedContainers)container = packedContainers{j};if fitsInContainer(box, containerSize, container)container = placeBox(box, containerSize, container);packedContainers{j} = container;placed = true;break;endend% 如果无法放入已有容器,创建新容器if ~placednewContainer = createContainer(containerSize, box);packedContainers = [packedContainers, newContainer];endendendfunction container = createContainer(containerSize, box)container.size = containerSize;container.remainingSpace = containerSize - box;endfunction fits = fitsInContainer(box, containerSize, container)fits = all(box <= container.remainingSpace);endfunction container = placeBox(box, containerSize, container)% 在容器中放置物体,更新容器状态container.remainingSpace = container.remainingSpace - box;end```请注意,这只是一个简化版本的启发式算法,实际情况中可能需要根据具体要求进行更复杂的算法设计。
最优包裹组合-贪心算法

最优包裹组合-贪⼼算法周末时间基本都在带娃,断更了⼀段时间,难得有点时间还是得有毅⼒坚持写,坚持总结。
最近公司在拓展电商相关业务,其中⼀个环节是物流发货。
物流打包环节有⼀个需求是希望能给运营同事⼀个⼩⼯具能快速计算最优的包裹组合。
我们这⾥不关注过多业务细节,只是把这个问题抽象总结⼀下。
问题:假设我们有如下4种规格的包裹可供选择:name size weight pricePackage S205KG 4.99€Package M4010KG 6.99€Package L8020KG8.99€Package XL12030KG10.99€由于我们的货物是抛货, 所以只考虑给定任意总Size的的打包需求,能得到最优的包裹组合。
抛重:即是体积重,1、当实重⼤于体积重,就属于重货,按实重计费度;2、当体积重⼤于实重就叫抛货,按体积重计费;这⾥最优的标准⽐较简单:包裹个数最少,同时也是总运费最低。
例如,我需要发153个货,那么最优的就是Package XL * 1 + Package M * 1。
贪⼼算法很符合直觉的策略是先尽可能选⼤的包裹,先从⼩号开始匹配,⼩号的装不下就升级⼤⼀号的包裹,直到能装下或者没有更⼤的箱⼦了,依次类推。
例如总共要装箱150个,那么:先⽤Package S发现装不下,升级成Package M也装不下,继续升级成Package L也装不下,继续升级成Package XL也装不下,但是没有更⼤的选择了,所以(贪⼼)打包⼀次。
更新待装箱的商品数据量 = 150 - 120 = 30;这样每次⼀个周期结束,再次⽤剩余没装箱的数量继续这样做打包操作:先⽤Package S发现装不下,升级成Package M发现能装下了,所以打包⼀次。
更新待装箱的商品数据量 = 30 - 40 <= 0;总结上述过程,我们发现每次迭代打包本质都是把当前需要求解的问题分成了⼀个⼦问题,每次解决⼦问题的策略都是尽量优先⽤最⼤的箱⼦,且任意⼦问题是不依赖上次迭代的结果。
矩形装箱算法

矩形装箱算法简介矩形装箱算法(Rectangle Packing Algorithm)是一种用于解决装箱问题的算法。
装箱问题是指将一系列矩形物体放置到一个或多个矩形容器中,使得物体之间不重叠且尽可能紧密地填充容器的问题。
矩形装箱算法的目标是找到一种最优的方式来放置这些物体,以最大程度地减少容器的浪费。
矩形装箱算法在物流、运输、仓储等领域具有广泛的应用。
通过合理地安排物体的摆放,可以节省空间、减少运输次数,从而提高效率和降低成本。
常见的矩形装箱算法1. 最佳适应算法(Best Fit Algorithm)最佳适应算法是一种贪心算法,它在每次放置物体时选择一个最佳的位置。
具体步骤如下: 1. 遍历所有的物体,对于每个物体,找到一个已有容器中剩余空间最小且能够容纳该物体的容器。
2. 将物体放置到选定的容器中,更新容器的剩余空间。
3. 如果找不到合适的容器,则创建一个新的容器,并将物体放置其中。
最佳适应算法的优点是能够尽可能地紧密填充容器,但缺点是计算复杂度较高。
2. 最均匀装箱算法(Most Uniform Packing Algorithm)最均匀装箱算法是一种启发式算法,它通过将物体按照尺寸进行排序,并将尺寸相似的物体放置在相邻的位置,以实现均匀的装箱效果。
具体步骤如下: 1. 将所有物体按照尺寸进行排序。
2. 从第一个物体开始,将其放置在第一个容器中。
3. 对于每个后续物体,选择一个已有容器,使得容器中的物体尺寸与该物体尺寸最接近,并将物体放置在该容器中。
4. 如果找不到合适的容器,则创建一个新的容器,并将物体放置其中。
最均匀装箱算法的优点是能够实现均匀的装箱效果,但缺点是可能会导致容器利用率较低。
3. 旋转装箱算法(Rotation Packing Algorithm)旋转装箱算法是一种考虑物体旋转的装箱算法。
它通过将物体旋转90度,以获得更好的放置效果。
具体步骤如下: 1. 将所有物体按照尺寸进行排序。
Rectangle Packing问题的程序设计

Rectangle Packing问题贪心算法的Pascal程序设计报告Flowers bi**a*c**b【摘要】本文主要阐述了基于贪心算法的Rectangle Packing问题的Pascal程序实现方法,介绍了程序主要procedure的作用和部分功能模块的实现算法。
【关键词】贪心算法;Rectangle Packing;Pascal;程序;回溯。
一. 程序设计任务及要求设计一个程序利用贪心算法对Rectangle Packing问题进行求解。
输入容器和各个待填矩形块的长宽,输出尽可能高空间利用率的装填方法,填入矩形块数和空间未利用率。
二. Rectangle Packing问题的贪心算法简介基本原理在某一时刻,已经按放置规则向矩形容器中放置了若干矩形块,对将要放入的矩形块,最好是占据某个角,其次是贴边,最差的是悬空。
用贪心的思路,找到合适的矩形块并按合适的位置放入使其占角最多,贴边最多,剩余角点数最少,并用回溯对这种放置方法进行评估,以找到这一时刻最适合的放法。
基本流程1.对容器状态进行初始化。
2.按照规则选择一个最优的矩形块放入容器。
如果这个放置动作的穴度为1,则继续进行下一个放置动作,否则通过回溯找到当前最优的放置位置。
3.重复2步骤,直至所有矩形块都已经放入容器或者容器中已无法再放入任何矩形。
4.对容器中矩形放置状态进行分析,求出放入矩形块数和空间未利用率。
三. 需求分析根据算法的基本需求和流程,用程序实现时,总体应分为输入、初始化、放置矩形、结果分析、输出五个基本步骤来对问题进行求解,其流程方框图如下:四. 用Pascal实现Rectangle Packing问题的贪心算法1. 程序定义的主要的数组型变量:w,h:array [1..300] of integer; {width and height of each rectangles}used:array [1..300] of boolean; {Hash table for used rectangles}state:array [1..300,1..4] of integer; {location of put rectangles}legalpos:array [1..10000,1..7] of integer; {legal position of newly put rectangle} dmin:array [1..10000] of integer; {min distance of put rectangle and others} c:array [1..10000] of real; {caving degree of each rectangles}c1:array [1..1000] of integer; {rectangles of 1 caving degree} cornercheck:array [1..4,1..2] of boolean; {Hash table for occupied corners} corners:array [1..300] of integer; {occupied corners of each coa}2. 程序中主要的procedure的作用:procedure init “输入”——用于读入容器和各个矩形的长宽。
把不规则形状切割成n个相同矩形的算法
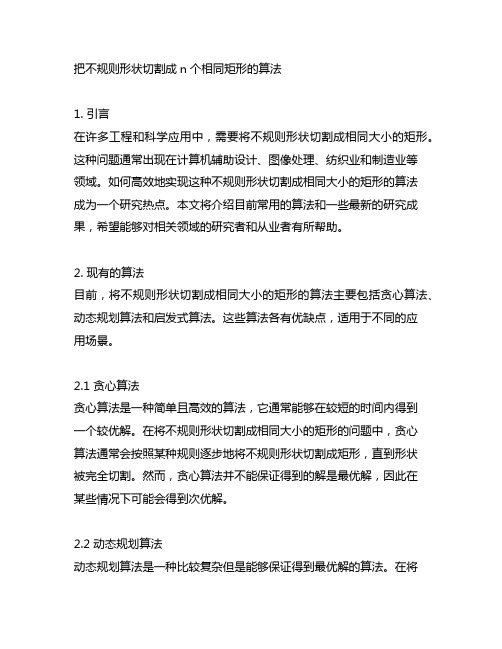
把不规则形状切割成n个相同矩形的算法1. 引言在许多工程和科学应用中,需要将不规则形状切割成相同大小的矩形。
这种问题通常出现在计算机辅助设计、图像处理、纺织业和制造业等领域。
如何高效地实现这种不规则形状切割成相同大小的矩形的算法成为一个研究热点。
本文将介绍目前常用的算法和一些最新的研究成果,希望能够对相关领域的研究者和从业者有所帮助。
2. 现有的算法目前,将不规则形状切割成相同大小的矩形的算法主要包括贪心算法、动态规划算法和启发式算法。
这些算法各有优缺点,适用于不同的应用场景。
2.1 贪心算法贪心算法是一种简单且高效的算法,它通常能够在较短的时间内得到一个较优解。
在将不规则形状切割成相同大小的矩形的问题中,贪心算法通常会按照某种规则逐步地将不规则形状切割成矩形,直到形状被完全切割。
然而,贪心算法并不能保证得到的解是最优解,因此在某些情况下可能会得到次优解。
2.2 动态规划算法动态规划算法是一种比较复杂但是能够保证得到最优解的算法。
在将不规则形状切割成相同大小的矩形的问题中,动态规划算法通常会通过建立状态转移方程来逐步计算最优解。
然而,动态规划算法的时间复杂度通常较高,因此在处理较大规模的问题时可能会无法满足实时性的要求。
2.3 启发式算法启发式算法是一种结合了贪心算法和随机搜索的算法,它通常能够在较短的时间内得到一个较优解。
在将不规则形状切割成相同大小的矩形的问题中,启发式算法通常会通过不断地调整切割方式来寻找最优解。
然而,启发式算法并不能保证得到最优解,因此在某些情况下可能会得到次优解。
3. 最新的研究成果近年来,研究人员提出了一些基于深度学习和强化学习的算法,这些算法在解决不规则形状切割成相同大小的矩形的问题上取得了一些突破性的进展。
这些算法通过利用大量的数据和强大的计算能力,能够在较短的时间内得到接近最优解的结果。
然而,这些算法通常需要大量的训练数据和计算资源,因此在实际应用中可能会存在一定的局限性。
矩形件排样算法探讨

矩形件排样算法探讨作者:苏厚仁钟相强来源:《科技视界》2015年第33期【摘要】针对二维矩形件优化排样问题,提出一种新型的算法——矩形动态匹配算法。
通过对零件的矩形化预处理,并自动正交排布使零件紧密靠接和定位,从而实现复杂不规则船体零件的矩形化排样,该算法亦可扩展用于三维空间零件的排样求解,实例证明其有效性。
【关键词】排样;矩形零件;优化;算法【Abstract】For optimal nesting of rectangular parts of a two dimensional problem, a new kind of algorithm is put forward. The rectangular pretreatment and automatically orthogonal configuration make the location of parts more close, the rectangular optimization nesting of complex irregular ship parts is realized, the algorithm can be extended to 3d space parts. Examples show its effectiveness.【Key words】Parking; Rectangular parts; Optimization; Algorithm0 引言排样优化技术是工业产品设计、制造中如何节约原材料、优化利用资源的重要手段。
现实零件形状复杂,多为不规则零件,且制造特征和方法各异,如何采用有效的算法实现最优布局、提高原材料的利用率尤为重要[1-3]。
文中基于对排样零件矩形化预处理提出了矩形动态匹配算法来实现零件的定位,具有较高的材料利用率。
1 算法简介1.1 实现算法的前提条件将一个矩形零件排放在矩形板材中,需要解决的问题有:(1)多个矩形零件排放时的排放次序。
二维矩形条带装箱问题的底部左齐择优匹配算法

ISSN 1000-9825, CODEN RUXUEW E-mail: jos@Journal of Software, Vol.20, No.6, June 2009, pp.1528−1538 doi: 10.3724/SP.J.1001.2009.03395 Tel/Fax: +86-10-62562563© by Institute of Software, the Chinese Academy of Sciences. All rights reserved.∗二维矩形条带装箱问题的底部左齐择优匹配算法蒋兴波1,2, 吕肖庆1,3+, 刘成城11(北京大学计算机科学技术研究所,北京 100871)2(第二军医大学卫生勤务学系,上海 200433)3(北京大学电子出版新技术国家工程研究中心,北京 100871)Lowest-Level Left Align Best-Fit Algorithm for the 2D Rectangular Strip Packing ProblemJIANG Xing-Bo1,2, LÜ Xiao-Qing1,3+, LIU Cheng-Cheng11(Institute of Computer Science and Technology, Peking University, Beijing 100871, China)2(Faculty of Health Services, Second Military Medical University, Shanghai 200433, China)3(National Engineering Research Center of New Technology in Electronic Publishing, Peking University, Beijing 100871, China)+ Corresponding author: E-mail: lvxiaoqing@Jiang XB, Lü XQ, Liu CC. Lowest-Level left align best-fit algorithm for the 2D rectangular strip packingproblem. Journal of Software, 2009,20(6):1528−1538. /1000-9825/3395.htmAbstract: In this paper, a heuristic placement algorithm for the two-dimensional rectangular strip packingproblem, lowest-level left align best fit (LLABF) algorithm, is presented. The LLABF algorithm is based on thebest-fit priority principle with overall consideration of several heuristic rules, such as full-fit first rule, width-fit firstrule, height-fit first rule, joint-width-fit first rule and placeable first rule. Unlike the bottom-left (BL), theimproved-bottom-left (IBL) and the bottom-left-fill (BLF) heuristic placement algorithms, LLABF algorithmdynamically selects the best-fit rectangle for packing. The computation result shows that 2DR-SPP can be solvedmore effectively by combining the LLABF algorithm with the genetic algorithm (GA).Key words: lowest-level left align best fit (LLABF) algorithm; genetic algorithm; 2D rectangular strip packingproblem; heuristic placement algorithm摘要: 针对二维矩形条带装箱问题提出了一种启发式布局算法,即底部左齐择优匹配算法(lowest-level left alignbest fit,简称LLABF). LLABF算法遵循最佳匹配优先原则,该原则综合考虑完全匹配优先、宽度匹配优先、高度匹配优先、组合宽度匹配优先及可装入优先等启发式规则.与BL(bottom-left),IBL(improved-bottom-left)与BLF(bottom-left-fill)等启发算法不同的是,LLABF能够在矩形装入过程中自动选择与可装区域匹配的下一个待装矩形.计算结果表明,LLABF结合遗传算法(genetic algorithm,简称GA)解决二维条带装箱问题更加有效.关键词: 最低左对齐最佳匹配(LLABF)算法;遗传算法;二维矩形条带装箱问题;启发式布局算法中图法分类号: TP18文献标识码: A二维矩形条带装箱问题(2D rectangular strip packing problem,简称2DR-SPP)是一个典型的组合优化问题,∗ Received 2008-02-29; Revised 2008-04-15; Accepted 2008-06-03蒋兴波等:二维矩形条带装箱问题的底部左齐择优匹配算法1529其应用相当广泛,如工业领域中的新闻组版、布料切割、金属下料等.2DR-SPP通常是指将若干个不同规格的矩形{π1,π2,…,πn}装入宽度W固定、长度L不限的矩形容器C中,要求装完所有矩形后占用高度H packing最小,从而达到节省材料的目的.在矩形的装入过程中,要求满足:①πi,πj互不重叠,i≠j,i,j=1,2,…,n;②πi必须装入在矩形容器C内,即矩形在装入过程中不能超出容器C的宽度W;③πi的边必须与矩形容器C的边平行,πi可以90°旋转.2DR-SPP是一个NP完全问题,其计算复杂度随着问题规模的增大呈指数增长.针对该类问题,国内外相关学者作了大量的研究.Hifi[1]给出了一种基于分支定界方法(branch-and-bound procedure)的精确算法,适用于解决中小规模的2D装箱问题;Lesh等人[2]针对2D矩形完美装箱问题,提出了基于分支定界的穷举搜索算法,该算法已证明对于低于30个矩形的装箱是有效的.为了解决大规模的矩形装箱问题,一些启发式算法也相继提出来.Zhang等人[3]提出了一种启发式递归算法HR(heuristic recursive algorithm),该方法基于启发式策略和递归结构,实验结果表明,该算法能够在较短时间内获得较为理想的装箱高度,但其平均运行时间却达到了O(n3).陈端兵等人[4]根据先占角后占边的原则,提出了一种针对矩形装箱的贪心算法.Chen等人[5]给出了一种two-level搜索算法来求解2DR-SPP.Cui[6]给出了一种启发式递归分支定界算法HRBB(heuristic recursive branch-and-bound algorithm),该算法将递归结构和分支定界技术结合在一起来求解2DR-SPP,取得了较好的实验结果.Beltrán等人[7]按照随机搜索原则设计的GRASP(greedy randomized adaptive search procedure)算法与VNS(variable neighbourhood search)结合在一起来求解2DR-SPP.而Alvarez-Valdes等人[8]提出了Reactive GRASP算法,该算法分为构建和改进两个阶段,其测试结果优于文献[7].张德富等人[9]则提出了一种有效的砌墙式启发式算法PH,实验结果表明,该算法对规模较大的2DR-SPP能够获得较好的装箱效果.针对2DR-SPP,目前广泛采用的是遗传算法GA(genetic algorithm)、模拟退火算法SAA(simulated annealing algorithm)等搜索算法与BL(bottom-left)、IBL(improved BL)、BLF(bottom-left-fill)等启发式布局算法相结合的方式.Bortfeldt[10]采用了无任何编码的遗传算法来解决矩形装箱问题.Jackobs[11]提出了一种混合算法,通过GA 与BL启发式布局算法相结合,从而将矩形的装箱问题转换成相对简单的装入序列问题.由于BL算法容易出现即使穷举所有情况仍不能得到最优解的现象,因此,Liu[12]提出了一种IBL算法,并与GA相结合,取得了优于文献[11]的装箱效果.Yeung等人[13]针对布料切割问题提出了一种布局算法LFLA,该布局算法的计算复杂度为O(n),相对于BL算法(其复杂度为O(n2)),效率上有了较大的提高.Zhang等人[14]针对矩形装箱问题给出了meta-heuristic算法,该算法主要基于启发式递归策略和SAA.Dereli等人[15]则采用SAA与递归布局方法相结合来解决2DR-SPP.针对不同数量及不同类型的矩形排样,Hopper[16]分别使用了BL,BLF启发式布局算法和GA,NE(naïve evolution),SA,HC(hill-climbing),RS(random search)启发式搜索算法相结合的方式进行求解.迄今为止,虽然对2DR-SPP进行了大量的研究,但从相关文献给出的测试结果来看,该问题仍然有进一步研究的必要.例如,BL,IBL,BLF,LFLA以及贾志欣等人[17]提出的最低水平线LHL(lowest-horizontal-line)等常见的启发式布局算法,它们在对矩形装入的过程中严格按照矩形的某个排列序列进行,容易产生较大的空洞,浪费空间,因此装箱效果不够理想.相对于BL而言,BLF算法可以取得较好的装箱效果,但其算法的时间复杂度却达到了O(n3)[18],不适宜解决规模较大的2DR-SPP.本文针对上述问题设计出一种启发式布局算法,即底部左齐择优匹配算法(lowest-level left align best fit,简称LLABF).本文第1节重点介绍LLABF算法设计.第2节介绍GA+LLABF算法的实现.第3节主要对标准数据集进行模拟测试,并与相关文献结果进行对比分析.第4节给出结论.1 LLABF算法设计1.1 定义设容器宽度所在方向为横坐标X,长度方向为纵坐标Y,容器的左下角为坐标原点(0,0).容器的底部在y=0处,容器可以在Y轴正方向无限延伸.1530 Journal of Software软件学报 V ol.20, No.6, June 2009队列I={π1,π2,…,πn}表示n个矩形的某个装入序列,其中,πi是矩形编号,πi∈[1,n],对任意i,j∈[1,n],当i≠j时, πi≠πj.设I(i)表示队列I中第i个位置上对应的矩形,它由五元组构成,即I(i)={x,y,w,h,θ},其中,I(i).x,I(i).y分别表示该矩形装入后,其左下角的横、纵坐标;I(i).w,I(i).h,I(i).θ分别表示该矩形的宽度、高度和旋转角度.设队列E={e1,e2,…,e m}表示装入过程中产生的轮廓线集合,元素e k为水平线线段(与X坐标轴平行),它由三元组构成,即e k={x,y,w},其中,e k.x,e k.y表示第k个水平线线段的左端点坐标(起点坐标);e k.w表示第k个水平线线段的宽度;并且对任意0<k<m,有e k.x<e k+1.x,即按轮廓线起点的x坐标从小到大排列.队列E具有以下特征:其y坐标具备唯一性,如果相邻线段具有相同的高度y,则进行合并;所有线段在X坐标上的投影不重叠;所有线段的宽度之和刚好等于矩形容器宽度W.最低水平线定义为队列E中其y坐标最小的水平线.最优高度H opt表示穷举所有可能情况得到的最小装箱高度,也称为最优解.装箱高度H packing表示根据当前装入序列,按照布局算法将所有矩形装入矩形容器C内所得到的最小高度.空洞是指在装箱过程中,由矩形或者矩形与容器边界(如x=0,y=0,x=W或者y=H packing)围成的空白区.空洞越多,材料浪费的程度就越严重.1.2 LLABF启发规则设计1.2.1 基本思想一般情况下,对NP难问题很难直接构造出一个最优解或满意解,所以只能通过搜索的方法在整个解空间中寻找最优解或者满意解.当问题规模较大时,这种盲目搜索或者遍历就变得十分困难,甚至根本不可行.因此,相关学者常利用问题解本身的某些结构特征来构造出一些启发式规则,并按照该规则设计出启发式算法,由该算法求得问题的一个满意解.对于矩形条带装箱问题,它有以下几个可以利用的结构特征:(1) 面积较大的矩形装入后产生的空洞较大,面积较小的矩形装入后产生的空洞较小;(2) 面积较大的矩形装入后产生的空洞常常可以装入面积较小的矩形;(3) 装入过程中产生的轮廓线越规整,即组成轮廓线的水平线数量越少,就越有利于后期矩形的装入.本文中,LLABF启发式算法利用了矩形条带装箱问题的3个结构特征,采用了动态选择方法.该方法遵照最佳匹配优先原则来动态选择下一个待装矩形,使得在矩形装入的每一步都尽可能地获得一个较优的装箱结果.重复执行,直至最终获得整体较优解.最佳匹配优先原则(best-fit priority,简称BFP)即在矩形的装入过程中,优先考虑与当前可装入区域宽度或高度相匹配以及可装入的未排矩形,它包括完全匹配优先、宽度匹配优先、高度匹配优先、组合宽度匹配优先以及可装入优先这5种启发式规则.5种启发式规则中完全匹配优先、宽度匹配优先以及组合宽度匹配优先可以减少空洞的产生,特别是在装入初期,由于用来与可装区域进行比较的矩形较多,因此,其匹配的概率越高,就越不容易产生空洞;完全匹配优先以及高度匹配优先使得装箱轮廓相对规整;高度匹配优先以及可装入优先可以将较小矩形延后装入.5种启发式规则结合起来不但可以使矩形装入后产生的空洞数量较少,而且所有的空洞总面积相对也较小.1.2.2 启发规则设计完全匹配优先(full-fit first,简称FFF). 在可装入的轮廓线中选取最低的水平线e k,如果有多个线段,则优先选取最左边的一段.从待装矩形中按照装入序列依次将矩形与e k进行比较,如果存在宽度或者高度与该线段宽度e k.w相等且装入后刚好左填平或者右填平的矩形则优先装入.完全匹配优先能够减少装入后产生的轮廓线数量,使得装入轮廓朝着顶部平齐的方向发展.宽度匹配优先(width-fit first,简称WFF). 在装入过程中,优先装入宽度或者高度与最低水平线e k等宽的矩形,如果存在多个匹配矩形,则优先装入面积最大的.与完全匹配优先规则不同的是,宽度匹配优先并不要求装入后能够实现左填平或者右填平;同时,该规则使得较小矩形有推迟装入的趋势.另外,WFF不会增加装入轮廓线数量.蒋兴波 等:二维矩形条带装箱问题的底部左齐择优匹配算法1531高度匹配优先(height -fit first ,简称HFF ). 在待装矩形中,按照装入序列查询宽度或高度不大于最低水平线e k 宽度且装入后能够实现左填平的矩形,若存在则装入查询到的首个矩形.与FFF 和WFF 不同,HFF 可能会在最低水平线上产生新的、更小的可装入区域,但却增加了轮廓线e k −1的宽度.组合宽度匹配优先(joint -width -fit first ,简称JWFF ). 按装入序列对两个矩形进行组合,如果组合后的宽度与最低水平线宽度e k 相等,则优先装入组合序列中的首个矩形.例如,存在两种组合I (i 1).w +I (j 1).w =e k .w , I (i 2).w +I (j 2).w =e k .w ,如果I (i 1)的面积大于I (i 2),则首先装入I (i 1),否则装入I (i 2).JWFF,FFF 与WFF 规则避免了在最低水平线e k 上产生新的、更小的可装入区域,从而减少了整个装箱过程产生空洞的可能性.为了保证时效性,算法中设置了查询范围searchNum ,即从当前位置开始,最多可以进行searchNum ×searchNum 次连续矩形的两两组合查询.可装入优先(placeable first ,简称PF ). 在一定范围内,从待装矩形件中按照装入序列依次查找宽度或高度不大于最低水平线e k 宽度的矩形,若存在,则将其装入;若存在多个,则装入面积最大的矩形.PF 可能在最低水平线上产生新的、更小的可装入区域,同时使得较小矩形延迟装入.在矩形的装入过程中,满足FFF,WFF,HFF 以及JWFF 规则的矩形可能并不存在,此时就必须考虑PF.如果满足PF 规则的矩形仍不存在,那么必然会在最低水平线上产生空洞.与BL,IBL,LFLA 以及LHL 算法不同,该空洞是在一定范围内由于不存在可装入的矩形而产生的,因此其面积更小.与BLF 算法不同,LLABF 算法并不需要保存新产生的空洞.图1给出了按照最佳匹配优先原则的部分启发规则实现矩形装入的示意图,其中,矩形的装入序列是4 2 7 6 5 1 8 3.图1(a)表示已经装入了4个矩形,产生了由4个线段e 1,e 2,e 3,e 4组成的装入轮廓,其中,e 2为最低水平线,其对应的区域是接下来首先要考虑的区域.图1(b)采用完全匹配优先:与最低水平线e 2等宽的矩形有1号和3号,但由于只有3号矩形装入后能够实现左填平,因此首先选择该矩形装入.图1(c)采用宽度匹配优先:满足该条件的矩形有1号和3号,但由于1号矩形面积较大,所以优先装入.图1(d)采用高度优先:1,8,3这3个矩形都满足其宽度不大于e 2.w ,但只有8号和3号矩形装入后能够实现左填平,且8号矩形位置在3号之前,所以优先装入.(a) (b)(c) (d)Fig.1 Packing result according to BFP principle图1 按BFP 原则的装箱结果图1.3 LLABF 算法的实现LLABF 算法是按照BFP 原则进行装箱的启发式布局算法,其算法实现如下所示.1532 Journal of Software软件学报 V ol.20, No.6, June 2009LLABF (input: I; output: H packing)beginInitContours(E); InitCoordinate(I); H packing=0; // 初始化;H packing:装箱高度① for (ii=1; ii≤n; ii++)begin②if (I(ii).x>−1) goto ①; // 当前矩形件已被装入在E中取最低水平线e k;j=−1; //记录用于装入的矩形件所在序列号FullFitFirst(ii,I,e k,j); if (j>−1) goto ④;//完全匹配FFFWidthFitFirst(ii,I,e k,j); if (j>−1) goto ④; //宽度匹配WFFHeightFitFirst(ii,I,e k,j); if (j>−1) goto ④; //高度匹配HFFJointWidthFitFirst(ii,I,e k,7,j); if (j>−1) goto ④; // 组合宽度匹配JWFFPlaceableFirst(ii,I,e k,n/6,j); if (j>−1) goto ④; //可装入优先PF③合并E中的边,转②;④将I集合中第j位元素对应的矩形件I(j)装入在E中的最低水平线e k上;如果未旋转,则H packing=max(H packing,I(j).x+I(j).h),否则,H packing=max(H packing,I(j).x+I(j).w);更新集合I和E;如果j>ii,则转②;endend其中,InitCoordinate(I)是对所有矩形块的坐标x,y进行初始化.在初始阶段,由于所有的矩形都未进行装入, InitCoordinate(I)将其坐标x,y全部初始化为−1,即对任意i∈[1,n],I(i).x=I(i).y=−1.InitContours(E)对装箱轮廓线进行初始化.在初始阶段,由于容器C未被装入,因此,其轮廓线仅由一个水平线线段构成,即E={e1},其中,e1={0,0,W},W为容器C的宽度.FullFitFirst,WidthFitFirst,HeightFitFirst,JointWidthFitFirst,PlaceableFirst分别表示按照FFF,WFF,HFF, JWFF以及PF等启发式规则在队列I中第ii位开始向后依次查找与最低水平线e k相匹配矩形的查询过程,其返回值为j.其中,JointWidthFitFirst,PlaceableFirst中的查询范围分别设置为7,n/6,为多次实验后取得的经验值.1.4 LLABF算法举例图2(a)是5个矩形构成的一个完美装箱图,其装箱高度H packing等于最优高度H opt,空间的利用率为100%.对于BL,IBL,LFLA以及LHL算法,要实现图2(a)的完美装箱(不考虑旋转),其装入序列就必须为1 2 3 4 5.对于序列为1 3 4 5 2和1 3 2 4 5,其装箱结果分别如图2 (b)和图2(c)所示,这两种装入序列都会产生较大的空洞.(a)(b) (c)Fig.2 Packing result according to BL, IBL, LFLA or LHL algorithm图2 BL,IBL,LFLA或LHL算法的装箱结果图对于同样的装入序列,采用LLABF算法则能得到如图2(a)所示的完美装箱.图3显示了装入序列为1 3 4 5蒋兴波 等:二维矩形条带装箱问题的底部左齐择优匹配算法15332、采用LLABF 算法得到的装箱结果(其中,*表示该位置上的矩形已经装入容器中).初始化:E ={e 1},e 1={0,0,W };对任意i ∈[1,n ],I (i ).x =I (i ).y =−1; 开始阶段虽然所有矩形都不满足完全匹配FFF 、宽度匹配WFF 以及高度匹配,但I (1).w +I (5).w =e 1.w ,满足两两组合宽度匹配JWFF,所以将编号为1的矩形装入在矩形容器C 的左下角;装入结果如图3(c)所示,此时,E ={e 1,e 2};取最低水平线e 2,按照LLABF 启发式规则依次查询匹配矩形,其中I (5),即编号为2的矩形满足WFF,故将其装入在e 2上;装入结果如图3(d)所示,此时,E ={e 1,e 2};以此类推,其装入过程如图3(e)~图3(g)所示.根据LLABF 算法,如果装入序列符合1 2 3 * *或者1 3 * * * 模式(其中,*代表未列出的矩形编号),其结果都与1 2 3 4 5序列的装箱结果相同,即都能得到如图3(a)所示的完美装箱.在图3中,满足第1种模式的装入序列包括1 2 3 4 5,1 2 3 5 4两种,满足第2种模式的装入序列包括1 3 2 4 5,1 3 4 2 5,1 3 2 5 4,1 3 5 2 4,1 3 5 4 2,1 3 4 5 2这6种.针对图2中的5个矩形的装箱问题,LLABF 找到最优解的概率是BL,IBL,LFLA 以及LHL 的8倍之多.因此,相对于传统布局算法,LLABF 算法更容易找到最优解.Fig.3 Packing result according to LLABF algorithm图3 LLABF 算法的装箱结果图2 GA +LLABF 算法实现2.1 算法的总体流程 本文采用遗传算法GA 与LLABF 相结合的方式来解决二维矩形条带装箱问题.其中,GA 用来实现矩形装入序列的初步确定,LLABF 则根据自身的启发式规则将矩形装入到矩形容器中.GA+LLABF 的算法流程如下所示.(1) 个体染色体编码.(2) k =0;随机产生m 个个体组成初始群体pop (k )={I 1,I 2,…,I m }.(3) 对单个个体I i 执行LLABF(Ii ,H packing );记录个体适应度f (I i )(f (I i )=1/H packing ).(4) 判断是否满足停止条件.若是,则停止计算,输出最佳结果;否则,继续.(5) 利用排序选择操作选择m 个个体形成新的群体selpop (k +1).(6) 根据交叉概率P c 进行交叉操作,产生群体crosspop (k +1).(a) (b) 1 3 4 5 2(c) * 3 4 5 2 (d) * 3 4 5 * (e) * * 4 5 * (f) * * * 5 * (g) * * * * *1534 Journal of Software软件学报 V ol.20, No.6, June 2009(7) 根据变异概率P m进行变异操作,产生群体mutpop(k+1).(8) pop(k+1)=mutpop(k+1);k=k+1;转(3),循环.2.2 编码及适应度函数矩形装箱采用整数编码(encoding),即n个矩形分别用整数1,2,…,n编号,矩形的一个装箱方案对应于一个染色体编码,I j=(π1,π2,…,πn),其中,|πi|表示矩形编号(1≤|πi|≤n),当πi<0时,矩形旋转90°,否则不旋转;I j表示第j个个体.例如:个体I j的染色体编码为(−5 3 −7 6 1 10 8 −2 4 −9),表示装入序列为5 3 7 6 1 10 8 2 4 9.在装入过程中,编号为5,7,2,9的矩形进行90°旋转.适应度函数(fitness function)可以由装箱高度的倒数表示,即f(I j)=1/H packing(I j).2.3 选择为了避免丢失上一代产生的最佳个体,本文在采用排序选择方法(rank-based select model)的同时,还混合使用了最优保存策略(elitist model).排序选择方法主要依据个体适应度值之间的大小关系,对个体适应度是否取正值或负值以及个体适应度之间的数值差异程度并无特别要求.其步骤如下:(1) 对群体中所有个体按照适应度从大到小排序.(2) 根据下列公式计算每个个体的选择概率:()iP=α×(1−α/m)I,sP表示排列在第i个位置的个体的选择概率,m表示群体大小,α为调节参数.其中,()is(3) 根据步骤(2)计算得出的个体概率值作为其能够被遗传到下一代的概率,基于该值应用比例选择(赌盘选择)的方法产生下一代群体.2.4 交叉(crossover)交叉是产生新个体的主要方法.本文主要采用环形部分交叉(circular-based part crossover):①随机生成起始交叉点P cr∈[1,n];②随机生成交叉长度L cr∈[1,n];③将P cr后的L cr位基因进行交换,如果P cr+L cr>n,则将染色体前P cr+L cr−n位基因进行互换;④然后依次将未出现的基因填入空白基因座.2.5 变异(mutation)变异是产生新个体的辅助方法,它决定了遗传算法的局部搜索能力,保持群体的多样性.本文中的变异包括两部分:一是旋转变异,二是装入序列变异.(1) 旋转变异旋转变异采用了单点取反和环形部分取反两种方式.单点取反(single point reversion)变异:①随机生成变异点P mu∈[1,n];②取反.例如,对于个体I j=(π1,π2,…, πi,…,πn),P mu=i,则变异后产生的新个体为I′=(π1,π2,…,−πi,…,πn).j环形部分取反(circular-based part reversion)变异:①随机生成变异点P mu∈[1,n];②随机生成变异长度L mu∈[1,n];③将P mu后的L mu位取反,如果P mu+L mu>n,则将染色体首部的P mu+L mu−n位基因取反.(2) 装入序列变异装入序列变异采用了两点位置互换与环形部分逆转两种方式.两点位置互换(two point exchange)变异:①随机生成两个变异点i,j∈[1,n];②将两个基因互换.例如:I j=(π1, π2,…,πi1,…,πi2,…,πn),i1,i2∈[1,n],则变异后产生的新个体为I′=(π1,π2,…,πi2,…,πi1,…,πn).j环形部分逆转(circular-based part inversion)变异:①随机生成变异点P mu∈[1,n];②随机生成变异长度L mu∈[1,n];③将P mu后的L mu位逆转,即将(P mu+i) mod n(即P mu+i对n取模)与(P mu+L mu−i) mod n互换,i∈[0,L mu/2].蒋兴波等:二维矩形条带装箱问题的底部左齐择优匹配算法15353 实验结果实验平台:Pentium 4 3.0GHz,512M,Windows Server 2003.运行参数:交叉概率P c=0.95,变异概率P m=0.85.本文的实验针对两组标准测试问题来进行,第1组来自文献[11],第2组来自文献[16].3.1 实验1文献[11]给出了两组标准测试实例,均由40×15的大矩形切割而成.其中,第1组实例由25个矩形组成,第2组实例由50个矩形组成.本文实验中,GA的群体大小和运行代数参照文献[12],分别设为20和100.对每组实例皆重复运行100次.实验结果对比见表1.Table 1Computational results of four algorithms表14种算法的计算结果Jakobs S[11] GA+BL Liu DQ[12]GA+IBLBortfeldt A[10]SPGALGA+LLABFW×H nH min H avg H min H avg H min H avg H min H avg H max40×15 25 17 17.481616.9716 16.001515.001540×15 50 17 17.281617.0115 15.001515.0015 表1中,H min,H max,H avg分别指多次运行中得到的最小H packing、最大H packing及平均H packing.由表1可以看出,GA+LLABF算法在对2组标准实例进行的100次测试中均找到了最优解15,而其他3种算法中,除了SPGAL找到了第2组数据集的最优解之外,其余算法求得的H packing均大于H opt.因此,实验结果表明,本文的GA+LLABF算法更加有效.3.2 实验2在文献[16]中,Hopper等人给出了21组不同尺寸的测试实例,这些实例分成7个大类,每个大类由3组实例组成,其矩形个数从16个~197个不等.每个实例的最优高度H opt已经给出,其相关数据见文献[16].在本文的算法中,初始种群大小参照文献[16],设为50,终止条件为进化代数等于 2 500代,或者装箱高度H packin等于最优解H opt.对每组测试实例重复运行10次,记录每个大类30次(每个大类3个实例,每个实例进行10次测试)测试的平均装箱高度到最优解的相对距离RDBSOH,RDBSOH(%)=100×(30次平均H packing−H opt)/H opt.实验结果对比见表2.Table 2Computational results of 12 algorithms (relative distance of best solution to optimum height (%))表2 12种算法的计算结果(RDBSOH(%))Algorithm C1 C2 C3 C4 C5 C6 C7 AverageGA+BLF[16] 4 7 5 3 4 4 5 4.57 SA+BLF[16] 4 6 5 3 3 3 4 4.00 GRASP+VNS[7]14.40 17.33 12.93 6.80 4.51 3.55 2.89 8.92 HR[3]8.33 4.45 6.67 2.22 1.85 2.5 1.8 3.97 Hybrid SA[15] 1.66 4.88 4.00 3.94 3.18 3.30 3.38 3.48SA+HR[14] 5.00 4.47 2.23 2.22 1.86 2.5 3.24 3.07 PH[9] 5.00 4.44 4.44 3.33 1.11 1.11 1.25 2.95HRBB[6] 1.70 0.00 1.10 2.20 1.90 1.40 1.30 1.40GRASP[8]0.00 0.00 1.08 1.64 1.10 1.56 1.36 1.33SPGAL[10] 1.70 0.90 2.20 1.40 0.00 0.70 0.50 1.00 A1[5]0.00 0.00 1.11 1.67 1.11 0.83 0.42 0.73 GA+LLABF 0.00 0.00 0.00 0.00 0.00 0.00 0.40 0.06 从表2可以看出,在现有的算法中,按照Averag e即平均RDBSOH,位居前4位的较优算法依次为A1, SPGAL,GRASP与HRBB,它们对7大类实例计算得出的Average分别为0.73,1.0,1.33和1.4.而本文给出的GA+LLABF算法计算得出的Average为0.06,其中6大类实例的RDBSOH为0,即对应的所有实例其10次实验结果皆取得了最优解.因此,实验结果进一步表明,针对2DR-SPP,本文提出的GA+LLABF算法优于其他算法.1536Journal of Software 软件学报 V ol.20, No.6, June 2009表3给出了GRASP+VNS,GRASP,HRBB 以及本文的GA+LLABF 对21组实例求得的实际测试结果H packing .在GA+LLABF 算法对21组实例的测试中,10次实验皆能获得最优解H opt 的有18组(21组中,除C 71,C 72,C 73外),占全部实例的86%(18/21),而GRASP 为38%(8/21)、HRBB 为33%(7/21);10次实验中至少获得1次最优解的实例有19组(即最小高度H min =最优高度H opt ),占全部实例的91%(19/21),而GRASP 为38%(8/21)、HRBB 为43%(9/21);10次实验获得的最大高度H max 与最优解H opt 之间的距离最多为1个基本单位.因此,无论是最优解所占比例还是最小高度H min 、平均高度H avg 以及最大高度H max ,GA+LLABF 求得的结果均具有明显的优势.Table 3 Computational results (H packing ) of 21 instances表3 21组实例计算结果 Beltrán DJ GRASP+VNS [7] (20 runs) Alvarez-Valdes R GRASP [8] (10 runs) Cui YD HRBB [6] (6 runs) GA+LLABF(20 runs) CategoryH opt n H min H avg H max H min H avg H min H avg H max H min H avg H max C 1120 16 21 22.30 23 20 20.00 2020.0020 20 20.00 20 C 1220 17 21 23.20 25 20 20.00 2121.0021 20 20.00 20 C 1320 16 22 23.15 24 20 20.00 2020.0020 20 20.00 20 C 2115 25 16 17.20 19 15 15.00 1515.0015 15 15.00 15 C 2215 25 16 18.00 20 15 15.00 1515.0015 15 15.00 15 C 2315 25 16 17.60 20 15 15.00 1515.0015 15 15.00 15 C 3130 28 32 32.55 34 30 30.00 3030.0030 30 30.00 30 C 3230 29 32 34.25 37 31 31.00 3131.0031 30 30.00 30 C 3330 28 33 34.85 37 30 30.00 3030.0030 30 30.00 30 C 4160 49 63 63.55 66 61 61.00 6060.5061 60 60.00 60 C 4260 49 61 64.60 68 61 61.00 6161.3362 60 60.00 60 C 4360 49 62 64.10 66 61 61.00 6161.0061 60 60.00 60 C 5190 73 92 93.45 95 91 91.00 9090.6791 90 90.00 90 C 5290 73 93 94.75 97 91 91.00 9292.0092 90 90.00 90 C 5390 73 92 94.00 98 91 91.00 9191.1792 90 90.00 90 C 61120 97 123 124.40 130121121.90121121.00121120 120.00 120C 62120 97 123 124.65 128121121.90121121.83122120 120.00 120C 63120 97 122 123.75 126121121.90121121.33122120 120.00 120C 71240 196 244 246.45 248244244.00242242.17243241 241.00 241C 72240 197 244 247.05 255242242.90245245.00245241 241.00 241C 73 240 196 245 247.30 258243243.00241241.33242240 240.90 241图4(H packing =H opt =120)、图5(H packing =H opt =240)显示了由GA+LLABF 算法求得的部分实例装箱图,其装箱高度皆为最优高度.Fig.4 Packing result of C 63 Fig.5 Packing result of C 73图4 C 63的装箱结果图 图5 C 73的装箱结果图 LLABF 算法在对矩形的装入过程中,每一步均按照FFF,WFF,HFF,JWFF 以及PF 规则依次查询与最低水平蒋兴波等:二维矩形条带装箱问题的底部左齐择优匹配算法1537线相匹配的矩形.对于FFF,如果某个阶段存在i个待装矩形,那么最坏情况下将比较i次;其余4个启发式规则除了JWFF的比较次数为常数K以外,皆与完全匹配优先类似,因此,单步比较次数最坏情况下为4i+K.将所有n 个矩形全部装入容器中,总的比较次数最坏情况下为4×(n+n−1+n−2+…+2+1)+n×K,共2n×(n+1)+n×K,因此其复杂度为O(n2).对于矩形在容器中的装入,主要的比较次数在于查找最低水平线,而最低水平线的数量不会超过n,所以,其复杂度最坏情况下为O(n2).整体上,LLABF的时间复杂度为O(n2).同时,对于GA,由于群体规模和进化代数皆为常数,其本身的比较次数也为常数,所以GA+LLABF算法的复杂度主要由LLABF决定,即最坏情况下为O(n2).表4给出了表2中所有算法的计算时间.由于算法的实现方式、测试平台与环境的不同,要对算法的快慢进行精确比较是不科学也是不可行的.但是从表4中,我们能够大致看出各种算法的运行快慢程度.由于本文算法采用了装箱高度等于最优解作为终止运行条件之一,因此,虽然C5的规模大于C4,但其获得最优解的速度明显快于C4,所以运行时间相对较小.Table 4Run times of 12 algorithms (s)表412种算法的运行时间(s)Algorithm C1 C2 C3 C4 C5 C6 C7 Averagea[16]a SA+BLF[16]42.00 144.00 240.00 1 980.00 6 900.0022 920.00250 860.00 40 441.86b GRASP+VNS[7] 0.00 0.00 0.00 0.00 0.02 0.07 1.37 0.21c HR[3]0.000.000.03 0.14 0.69 2.21 36.07 5.59dHybrid SA[15] 3.64 6.36 18.78101.20287.27 757.20 1650.6 403.57e SA+HR[14] 23.20 42.80 59.20170.00343.60 444.90 1328.80 344.64f PH[9]0.00 0.00 0.00 1.51 5.69 23.74 707.12 105.45g HRBB[6] 0.27 0.33 0.88 2.19 2.83 2.24 4.26 1.86h GRASP[8]- - -- ---60.00i SPGAL[10]--- ----139.00j A1[5]0.370.611.71 0.15 0.40 3.98 45.02 7.46GA+LLABF0.000.032.233.6 1.3 33.2 328.3 52.67a—Pentium Pro 200MHz and 65MB memory c—Dell GX260 with a 2.4GHz CPUe—Dell GX270 with a 3.0GHz CPU g—Pentium 4 2.8GHZ, 512MB memory i—Pentium PC with 2GHz b—Pentium 166MHz and 96MB RAM d—Pentium Ⅲ 797MHZf—Pentium 4 1.6GHZ, 256MB memory h—Pentium 4 mobile 2.0GHZ, 512MB memory j—2.4GHz PC with 512MB memory4 结论与展望本文分析了传统布局算法的不足,提出了一种启发式布局算法LLABF,并将其与GA相结合.实验结果表明,GA+LLABF算法解决2DR-SPP问题更加有效.但是要评价一种算法的优劣,除了要考虑算法的求解结果以外,还应兼顾算法的运行效率.因此,改进算法以进一步提高运算速度,将是我们下一步的研究重点.References:[1] Hifi M. Exact algorithms for the guillotine strip cutting/packing problem. Computers & Operations Research, 1998,25(11):925−940.[2] Lesh N, Marks J, McMahon A, Mitzenmacher M. Exhaustive approaches to 2D rectanglar perfect packings. Information ProcessingLetters, 2004,90:7−14.[3] Zhang DF, Kang Y, Deng AS. A new heuristic recursive algorithm for the strip rectangular packing problem. Computers &Operations Research, 2006,33(8):2209−2217.[4] Chen DB, Huang WQ. Greedy algorithm for recatngle-packing problem. Computer Engineering, 2007,33(4):160−162 (in Chinesewith English abstract).[5] Chen M, Huang WQ. A two-level search algorithm for 2D rectangular packing problem. Computers & Industrial Engineering, 2007,53(1):123−136.。
使用JAVA实现算法——贪心算法解决背包问题

使⽤JAVA实现算法——贪⼼算法解决背包问题解释等全部在代码中,⾃⼰跑了下,是没问题的package BasePart;import java.io.BufferedReader;import java.io.FileInputStream;import java.io.IOException;import java.io.InputStreamReader;/*** 使⽤贪⼼算法解决背包问题* 背包问题:* 旅⾏者有背包容量m公⽄* 现在有重量W1,W2,W3,W4....Wn* 对应的价值V1,V2,V3,V4....Vn* 运⾏重复携带,欲求得最⼤价值* 贪⼼算法:求得最好的选择,但是贪⼼算法不是对于所有的问题都得到整体最优解* 贪⼼算法基本思路:* 1.建⽴数学模型来描述问题* 2.把求解问题分成若⼲个⼦问题* 3.对于每⼀个⾃问题求得局部最优解* 4.把⼦问题的解局部最优解合成原来解问题的⼀个解* 贪⼼算法的实现过程:* 从⼀个初始解出发* while-do朝总⽬标前进* 求出可⾏解的⼀个解元素* 由所有解元素组成合成问题的⼀个可⾏解*/public class Greedy {/*解决背包问题*需要背包容量*背包价值*背包剩余容量*解向量集合*/private double total_weight;private double total_value;private double rest_weight;//储存排序数组private Good[] arrayValue;private Good[] arrayWeight;private Good[] arrayC_P;private int goodsNum;private Good[] goods;private double real_weight;public Greedy() {}public Greedy(int goodsNum,double total_weight) {this.goodsNum=goodsNum;this.total_weight=total_weight;}public void init(String filename) throws IOException {/** 1.初始化程序* 2.从TXT⽂件中得到商品重量和其价值数组* 3.初始化序列数组arrayValue/Weight/C_P*/goods=new Good[goodsNum];BufferedReader data=new BufferedReader(new InputStreamReader(new FileInputStream(filename)));String buff;String[] strs;//循环赋值for(int i=0;i<4;i++){buff=data.readLine();strs=buff.split(" ");//根据位次goods[i]=new Good();//对象数组不仅仅需要初始化数组,对于数组内的每⼀个对象也需要初始化goods[i].setName(strs[0]);goods[i].setValue(Double.parseDouble(strs[1]));goods[i].setWeight(Double.parseDouble(strs[2]));goods[i].figureC_P();}//关闭输⼊流//成员变量初始化arrayValue=new Good[goodsNum];arrayWeight=new Good[goodsNum];arrayC_P=new Good[goodsNum];//初始化数组/** 价值由⼤到⼩数组*/arrayValue=arrayCopy(goods, arrayValue);//按照价值对arrayValue数组进⾏重新排列,使⽤冒泡排序法for(int i=0;i<goodsNum-1;i++){//从⼤到⼩排列for(int j=i+1;j<goodsNum;j++){if(arrayValue[i].getValue()<arrayValue[j].getValue()){Good temp=arrayValue[i];arrayValue[i]=arrayValue[j];arrayValue[j]=temp;}}}/**质量由⼩到⼤数组*/arrayWeight=arrayCopy(goods, arrayWeight);//按照价值对arrayWeight数组进⾏重新排列,使⽤冒泡排序法for(int i=0;i<goodsNum-1;i++){//从⼩到⼤排列for(int j=i+1;j<goodsNum;j++){if(arrayWeight[i].getWeight()>arrayWeight[j].getWeight()){Good temp=arrayWeight[i];arrayWeight[i]=arrayWeight[j];arrayWeight[j]=temp;}}}/** 性价⽐由⼤到⼩排列*/arrayC_P=arrayCopy(goods, arrayC_P);//按照价值对arrayC_P数组进⾏重新排列,使⽤冒泡排序法for(int i=0;i<goodsNum-1;i++){//从⼤到⼩排列for(int j=i+1;j<goodsNum;j++){if(arrayC_P[i].getC_P()<arrayC_P[j].getC_P()){Good temp=arrayC_P[i];arrayC_P[i]=arrayC_P[j];arrayC_P[j]=temp;}}}}//⽤于数组拷贝public Good[] arrayCopy(Good[] goods,Good[] arr2){arr2=goods.clone();return arr2;}private void show(Good[] goodsarr) {for(Good good:goodsarr){System.out.println(good.getName()+"\t"+good.getValue()+"\t"+good.getWeight()+"\t"+good.getC_P()+"\t"+good.getNum()); }}/*三种策略:度量准则* 依次选取价值最⼤填充* 依次选取重量最轻填充* 依次选取⽐价最⼤填充** ⽅法设计:* 按照度量准则* 传递⼀个按照选择优先级排列的对象数组* 迭代计算剩余容量* 返回设计⽅案*/public void strategy(Good[] goodsArray){rest_weight=total_weight;for(Good good:goodsArray){int selectNum=(int)Math.floor(rest_weight/good.getWeight());rest_weight=rest_weight-selectNum*good.getWeight();good.setNum(selectNum);if(rest_weight<arrayWeight[0].getWeight()){continue;}}}public void calculate(Good[] goodsArray,String target){total_value=0;real_weight=0;//处理结果System.out.println("在以"+target+"为准则的情况下");for(Good good:goodsArray){System.out.println(good.getName()+"\t\t数量:"+good.getNum());total_value+=good.getValue()*good.getNum();real_weight+=good.getWeight()*good.getNum();}System.out.println("总价值是:\t"+total_value+"\t总重量是:\t"+real_weight); }public void solve() {/** 业务逻辑* 将优先级数组*/strategy(arrayValue);calculate(arrayValue,"价值");strategy(arrayWeight);calculate(arrayWeight,"重量");strategy(arrayC_P);calculate(arrayC_P,"⽐值");}public static void main(String[] args) throws IOException {Greedy greedy=new Greedy(4,50);greedy.init("goods.txt");greedy.solve();}}Txt⽂本为:ad钙奶 12 5ab胶带 6 1电脑 4000 30⾳响 500 15说明:第⼀列:名称第⼆列:价格第三列:重量程序运⾏结果为:待改善。
箱子的摆放问题

箱子的摆放问题(总15页) -CAL-FENGHAI.-(YICAI)-Company One 1■CAL■本页仅作为文档封面,使用请直接删除箱子的摆放策摘要关键词:利用率最高循环嵌套式算法线性加权评价一、问题重述义车是指对成件托盘货物进行装卸、堆垛和短距离运输作业的各种轮式搬运车辆。
如何摆放箱子,使得义车能将最多的货物从生产车间运输至仓库是众多企业关心的问题。
现将箱子的底面统一简化为形状、尺寸相同的长方形,义车底板设定为一个边长为米的正方形。
要求建立一个通用的优化模型,在给定长方形箱子的长和宽之后,就能利用这个模型算岀使得箱子数量最多的摆放方法。
本题需要解决的问题有:问题一:在不允许箱子超出义车底板,也不允许箱子相互重叠的情况下,构建一个优化模型,并根据题H中提供的三种型号箱子的数据,确定可以摆放的个数及摆放示意图。
问题二:假设箱子密度均匀,允许箱子在正方形底板的上方,左边,右边部分超出底板,但不至于掉落出义车底板。
重建优化模型,考虑问题一中三种规格的箱子的摆放方式。
问题三:在不允许箱子相互重叠的条件下,另外设计出一种摆放方案,再将设计的方案与问题一中的摆放方案的进行优劣性对比。
二、模型假设 1.假设箱子的密度都是均匀的,若允许箱子在正方形底板的上方,左边,右边部分超岀底板(下方紧靠义车壁,不能超出),只要重心不超岀底板,就不至于掉落出义车底板。
2•假设箱子表面光滑,箱子间摆放无缝隙,即把箱子当做小矩形进行分析。
3•假设义车的承重能力无限大,能承载足够多的箱子。
三.符号说明符号解释说明a小矩形箱的长b小矩形箱的宽c长边向上叠加的矩形箱个数d宽边向上叠加的矩形箱个数m底边上矩形箱的长边个数n底边上矩形箱的宽边个数sum小矩形的总个数Wj摆放指标的权重系数Xj摆放指标无量纲化后的数值四、问题分析本文研究的是在一个边长为的正方形义车底板上堆放长方体箱子的问题。
不同规格的箱子最佳堆放方式是不同的,要尽量多的满足各种型号箱子摆放数量最多,就要设计一个通用的优化方案。
二维装箱问题的算法
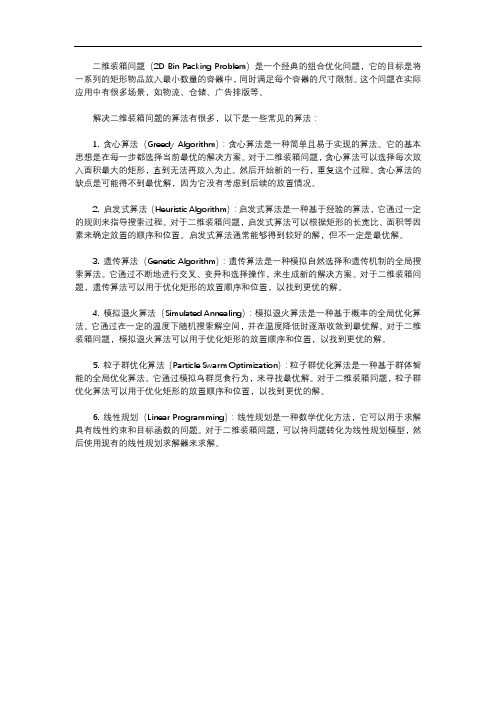
二维装箱问题(2D Bin Packing Problem)是一个经典的组合优化问题,它的目标是将一系列的矩形物品放入最小数量的容器中,同时满足每个容器的尺寸限制。
这个问题在实际应用中有很多场景,如物流、仓储、广告排版等。
解决二维装箱问题的算法有很多,以下是一些常见的算法:1. 贪心算法(Greedy Algorithm):贪心算法是一种简单且易于实现的算法。
它的基本思想是在每一步都选择当前最优的解决方案。
对于二维装箱问题,贪心算法可以选择每次放入面积最大的矩形,直到无法再放入为止。
然后开始新的一行,重复这个过程。
贪心算法的缺点是可能得不到最优解,因为它没有考虑到后续的放置情况。
2. 启发式算法(Heuristic Algorithm):启发式算法是一种基于经验的算法,它通过一定的规则来指导搜索过程。
对于二维装箱问题,启发式算法可以根据矩形的长宽比、面积等因素来确定放置的顺序和位置。
启发式算法通常能够得到较好的解,但不一定是最优解。
3. 遗传算法(Genetic Algorithm):遗传算法是一种模拟自然选择和遗传机制的全局搜索算法。
它通过不断地进行交叉、变异和选择操作,来生成新的解决方案。
对于二维装箱问题,遗传算法可以用于优化矩形的放置顺序和位置,以找到更优的解。
4. 模拟退火算法(Simulated Annealing):模拟退火算法是一种基于概率的全局优化算法。
它通过在一定的温度下随机搜索解空间,并在温度降低时逐渐收敛到最优解。
对于二维装箱问题,模拟退火算法可以用于优化矩形的放置顺序和位置,以找到更优的解。
5. 粒子群优化算法(Particle Swarm Optimization):粒子群优化算法是一种基于群体智能的全局优化算法。
它通过模拟鸟群觅食行为,来寻找最优解。
对于二维装箱问题,粒子群优化算法可以用于优化矩形的放置顺序和位置,以找到更优的解。
6. 线性规划(Linear Programming):线性规划是一种数学优化方法,它可以用于求解具有线性约束和目标函数的问题。
二维不等圆packing问题的现实求解途径

二维不等圆packing问题的现实求解途径二维不等圆packing问题是一个经典的组合优化问题,涉及到在一个平面上放置一系列大小不等的圆,使得它们之间的重叠最小化。
该问题在许多实际应用中具有重要意义,例如物流布局、芯片设计、城市规划等。
为了解决这个问题,可以采用以下几种不同的现实求解途径。
1.贪心算法贪心算法是一种简单而直观的解决方法,它根据一定的规则选择最优解。
在二维不等圆packing问题中,可以按照圆的半径大小进行排序,然后依次尝试将每个圆放置在平面上,要求不与已放置的圆相交。
贪心算法的优势在于简单而高效,但是它无法保证得到全局最优解。
2.启发式算法启发式算法是一类基于经验规则或启发性规则的优化算法,它通过不断迭代尝试,逐步改善解的质量。
在二维不等圆packing问题中,可以采用模拟退火算法、遗传算法等启发式算法来求解。
这些算法通常结合了随机搜索和局部优化策略,能够得到较好的实际解。
3.精确算法精确算法是一种可以得到最优解的解决方法,它通过穷举搜索所有可能解空间来找到最优解。
在二维不等圆packing问题中,可以采用回溯算法、分支界限算法等精确算法来进行求解。
这些算法会遍历所有可能的圆的放置方式,从中选择最优解。
然而,由于该问题的复杂性,精确算法的计算复杂度往往很高。
4.近似算法近似算法是一种可以在较短时间内得到接近最优解的方法,它可以在牺牲一定解的质量的情况下,获得更高的效率。
在二维不等圆packing问题中,可以使用基于凸包的近似算法来求解,即将所有圆看作一个整体,并构造一个围绕它们的最小凸包。
该算法可以在较短时间内找到一个不错的解。
5.元启发式算法元启发式算法是一种将多个不同的启发式算法组合使用的方法,通过不同算法之间的合作与竞争,达到更好的效果。
在二维不等圆packing问题中,可以使用元启发式算法,将多个启发式算法的优势结合起来。
例如,可以先使用贪心算法进行初始化,然后再使用启发式或精确算法进行局部优化。
ACMHPU第五讲_贪心算法(1)实训

实训讲解二
有一些矩形(N个 ,长和宽分别为Li和 有一些矩形 个),长和宽分别为 和 Wi(0<i<=N) 所有的矩形都得放入到特别的容器里面一个容器 最多可以容纳一个矩形 但是, 但是,每个矩形里面可以放一个长和宽都不比自 己大的矩形 问最少需要多少个容器
问题转化
每个矩形可以用一个二元组来表示, 每个矩形可以用一个二元组来表示,那么就可以 表示为二维坐标系上的一个点 问题就变成了最少要找多少条只能向上和向右走 的线通过所有点。 的线通过所有点。
例: N=3 L1=3,L2=2,L3=2 L=4
则至少需要两块木板(如 则至少需要两块木板 如 右图) 右图
算法提出
每一个区域都需要有至少一块木板完全覆盖 首先考虑一块木板怎么放 覆盖最左边几个区域的木板, 覆盖最左边几个区域的木板,左边界对齐第一个 区域的左边界可以覆盖最多的区域 这块木板选择完了之后, 这块木板选择完了之后,已经被完全覆盖了的区 域可以不管了, 域可以不管了,剩下的依然如上选择下一块木板 的位置 这样是对的么? 这样是对的么?
矩形规划问题的求解方法及其应用

矩形规划问题的求解方法及其应用矩形规划问题是一类常见的优化问题,其涉及到如何在矩形之内,找到一组满足特定要求的最优解。
在工业、交通、电子等领域有着广泛的应用,例如机场跑道布局、自动化仓库设计、芯片布局等。
本篇文章将主要介绍矩形规划问题的求解方法及其应用。
一.矩形规划问题的基本形式矩形规划问题的基本形式是指,在一个矩形里,寻找一组满足特定要求的最优解。
其中,矩形是给定的,而特定要求则由具体问题所决定。
例如,在机场跑道布局中,矩形是指机场的总体布局图,而特定要求则是指跑道的长度、宽度、旋转角度等。
二.矩形规划问题的解法矩形规划问题的解法可以分为两类:贪心算法和动态规划算法。
1.贪心算法贪心算法是一种简单的近似算法,其思想是在每个决策点上做出最优的选择。
在矩形规划问题中,贪心算法的基本步骤如下:(1)按照一定的顺序排列矩形对象;(2)将矩形对象依次添加到目标矩形中,每次添加后计算目标矩形的剩余空间;(3)若剩余空间不足,则停止添加矩形对象。
贪心算法虽然简单,但也存在一定的局限性。
例如,当输入的矩形对象是乱序的,或者存在多个解时,贪心算法可能无法找到全局最优解。
2.动态规划算法动态规划算法是一种优化问题的常用求解方法,其基本思想是将一个大问题分解成若干个子问题,并以递归的形式求解每一个子问题,最终合并各个子问题的结果来得到大问题的最优解。
在矩形规划问题中,动态规划算法的基本步骤如下:(1)将问题拆分成一系列子问题,并计算每个子问题的最优解;(2)将每个子问题的最优解存储在表格中,以备后续的计算;(3)利用存储在表格中的子问题最优解,计算出整个问题的最优解。
动态规划算法能够找到全局最优解,并且能够对多个解进行排序。
但是,动态规划算法的时间复杂度较高,对于复杂的问题需要计算多次,可能导致求解时间过长。
三.矩形规划问题的应用矩形规划问题在多个领域都有着广泛的应用,主要涉及到以下三个方面:1.机场跑道布局机场跑道布局是矩形规划问题的经典应用之一。
C语言基于贪心算法解决装箱问题的方法

C语⾔基于贪⼼算法解决装箱问题的⽅法本⽂实例讲述了C语⾔基于贪⼼算法解决装箱问题的⽅法。
分享给⼤家供⼤家参考,具体如下:问题描述:有⼀些箱⼦,容量为V,同时有n个物品,每个物品有⼀个体积(⼩于等于箱⼦容量),要求将物品全部装⼊箱⼦中,使占⽤的箱⼦数尽量少。
贪⼼算法中要求每⼀步的解都是当前步骤中的最优解。
原问题的解可以通过⼀系列局部最优的选择来达到,这种选择并不依赖于⼦问题的解。
算法思想:1、数据结构要求求解箱⼦数⽬,也就是说不能确定会占⽤多少个箱⼦,因此采⽤链表的形式来存储箱⼦及其信息。
同时,每个箱⼦中物品的数⽬也⽆法确定,同理采⽤链表来存储每个箱⼦中的物品信息。
由此得出数据节点的定义:typedef struct{int gno;int gv;}Goods;typedef struct node{int gno;struct node *link;}GNode;typedef struct node1{int remainder;GNode * head;struct node1 * next;}GBox;2、求解思路使打开的箱⼦数尽量少,也就是说每个箱⼦容积被尽可能多地占⽤。
将物品按照体积降序排列后,再从第⼀个物品开始,挨个寻找能放下它的箱⼦,这样可以保证局部最优。
void GoodsSort(Goods goods[], int n){int i, j;Goods t;for (i = 0; i<n - 1; i++){for (j = i + 1; j<n; j++){if (goods[i].gv<goods[j].gv){t = goods[i];goods[i] = goods[j];goods[j] = t;}}}for (i = 0; i<n; i++)printf("%d %d\n", goods[i].gno, goods[i].gv);排序完成,就可以正式开始装箱⼦了。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
万方数据
万方数据
万方数据
求解矩形packing问题的贪心算法
作者:陈端兵, 黄文奇, CHEN Duanbing, HUANG Wenqi
作者单位:华中科技大学计算机科学与技术学院,武汉,430074
刊名:
计算机工程
英文刊名:COMPUTER ENGINEERING
年,卷(期):2007,33(4)
被引用次数:6次
1.Hopper E;Turton B An Empirical Investigation of Meta-heuristic and Heuristic Algorithm for a 2D Packing Problem[外文期刊] 2001(01)
2.Beasley J E A Population Heuristic for Constrained Two-dimensional Non-guillotine Cutting[外文期刊] 2004(03)
3.Wu Y L;Huang W Q;Lau S C An Effective Quasi-human Based Heuristic for Solving the Rectangle Packing Problem[外文期刊] 2002(02)
4.Zhang D F;Deng A S;Kang Y A Hybrid Heuristic Algorithm for the Rectangular Packing Problem[外文会议] 2005
5.Huang W Q;Li Y;Akeb H Greedy Algorithms for Packing Unequal Circles into a Rectangular Container [外文期刊] 2005(05)
1.孙煜.刘松风.马力.SUN Yu.LIU Song-Feng.MA Li贪心算法在系统故障诊断策略生成中的应用[期刊论文]-计算机系统应用2011,20(1)
2.陈端兵.黄文奇.CHEN Duan-Bing.HUANG Wen-Qi一种求解矩形块装填问题的拟人算法[期刊论文]-计算机科学2006,33(5)
3.张德富.韩水华.叶卫国.ZHANG De-Fu.HAN Shui-Hua.YE Wei-Guo求解矩形Packing问题的砌墙式启发式算法[期刊论文]-计算机学报2008,31(3)
4.谷斌.靳艳峰.王力娟.耿科明.庞贵法.GU Bin.JIN Yan-feng.WANG Li-juan.GENG Ke-ming.PANG Gui-fa基于贪心算法与多边形剖分的印鉴匹配算法[期刊论文]-计算机工程与设计2008,29(12)
5.陈端兵.黄文奇.CHEN Duan-bing.HUANG Wen-qi求解矩形和圆形装填问题的最大穴度算法[期刊论文]-计算机工程与应用2007,43(4)
1.陈仕军.曹炬一种"一刀切"式矩形件优化排样混合算法[期刊论文]-锻压技术 2009(4)
2.傅仰耿.郭昆.陈建华.王建南一种实用的铁制工艺品排料方案的设计与实现[期刊论文]-福建电脑 2008(10)
3.蒋兴波.吕肖庆.刘成城二维矩形条带装箱问题的底部左齐择优匹配算法[期刊论文]-软件学报 2009(6)
4.陈仕军.曹炬矩形件优化排样的一种启发式算法[期刊论文]-计算机工程与应用 2010(12)
5.梁策.潘万彬局部求精算法中的纹理动态拼装[期刊论文]-机电工程 2010(9)
6.蒋兴波.吕肖庆.刘成城二维矩形条带装箱问题的底部左齐择优匹配算法[期刊论文]-软件学报 2009(6)
本文链接:/Periodical_jsjgc200704055.aspx。